-
Notifications
You must be signed in to change notification settings - Fork 176
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Refactor content panels into an iframe #840
Conversation
src/content_script.js
Outdated
window.addEventListener("message", (e) => { | ||
if (e.data === "closeTheInjectedIframe") { | ||
closeIframe(); |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
One issue I had was figuring out how to enable cross-origin communication between the iframe
embedded site and the parent page. I thought this was impossible according to my research until I found the Window.postMessage() method! Super neat and useful to know for add on development.
window.addEventListener("message", (e) => { | ||
if ( | ||
e.data === "allowTriggered" | ||
&& iframeSrcVal.includes(e.origin) |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
Keeping the postMessage
method since specified the origin is the iframe's src value. I did more reading up on it on MDN and I saw this:
If you do expect to receive messages from other sites, always verify the sender's identity using the origin and possibly source properties.
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
Spot-checks look good. Most non-blocking comments but a couple things we should at least discuss & decide before merging, I think.
src/content_script.js
Outdated
button.appendChild(document.createTextNode( browser.i18n.getMessage(buttonString) )); | ||
htmlBadgeFragmentPromptButtonDiv.appendChild(button); | ||
// Mobile Orientation | ||
else { |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
suggestion (non-blocking): whenever possible I like to refactor if () { ... } else { ... }
code into if () { func(); return;} func2();
So here it would be something like:
const OFFSET_X = 20;
const OFFSET_Y = 55;
function desktopOrientation(iframeBox, iframeChevron, fencePos, fencePosition, iframePaddingAllowance) {
// Desktop Values
const xLeft = fencePosition.x - iframePaddingAllowance;
const xRight = fencePosition.x + OFFSET_X + fencePos.offsetWidth;
const yPos = fencePosition.y - OFFSET_Y;
// Position iframe relative to FBC Icon
iframeBox.style.marginLeft = `${xRight}px`;
iframeBox.style.marginTop = `${yPos}px`;
// Add Chevron (Default left arrow)
const xPosChevron = xRight - iframeChevron.offsetWidth;
const yPosChevron = yPos + OFFSET_Y;
iframeChevron.style.marginLeft = `${xPosChevron}px`;
iframeChevron.style.marginTop = `${yPosChevron}px`;
const calculateOffsetDiff = window.innerWidth - fencePosition.x;
iframeChevron.classList.remove("fbc-chevron-arrow-top");
// Flip the iframe to show on the left side when icon is too close to the edge
if (iframePaddingAllowance > calculateOffsetDiff) {
iframeBox.style.marginLeft = `${xLeft}px`;
iframeChevron.classList.add("fbc-chevron-arrow-right");
iframeChevron.style.marginLeft = `${xPosChevron - fencePos.offsetWidth - iframeChevron.offsetWidth - OFFSET_X}px`;
return;
}
iframeChevron.classList.remove("fbc-chevron-arrow-right");
}
function mobileOrientation(iframeElement, iframeChevron, iframeBox, fencePosition){
// Mobile Values
const xPosMobile = fencePosition.x;
const yPosMobile = fencePosition.y + OFFSET_Y;
for (const panels of iframeElement) {
panels.width = window.innerWidth;
if (window.innerWidth > 480) {
panels.width = 350;
}
}
iframeChevron.classList.add("fbc-chevron-arrow-top");
iframeBox.style.marginTop = `${yPosMobile}px`;
const xPosChevronMobile = xPosMobile;
const yPosChevronMobile = yPosMobile - iframeChevron.offsetWidth;
iframeChevron.style.marginLeft = `${xPosChevronMobile}px`;
iframeChevron.style.marginTop = `${yPosChevronMobile}px`;
}
function positionIframe(fencePos) {
const fencePosition = fencePos.getBoundingClientRect();
const iframeBox = document.querySelector(".fbc-content-box");
const iframeWrapper = document.querySelector(".fbc-wrapper");
const iframeElement = iframeWrapper.getElementsByTagName("iframe");
const iframeChevron = document.querySelector(".fbc-iframe-chevron");
const iframePaddingAllowance = iframeBox.offsetWidth + OFFSET_X;
const iconRightAllowance = window.innerWidth - fencePosition.x + fencePos.offsetWidth;
const iconLeftAllowance = window.innerWidth - iconRightAllowance;
if (iconRightAllowance > iframePaddingAllowance || iconLeftAllowance > iframePaddingAllowance) {
desktopOrientation(iframeBox, iframeChevron, fencePos, fencePosition, iframePaddingAllowance);
}
mobileOrientation(iframeElement, iframeChevron, iframeBox, fencePosition);
}
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
Refactored.
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
function positionIframe(fencePos) {
const fencePosition = fencePos.getBoundingClientRect();
const iframeBox = document.querySelector(".fbc-content-box");
const iframeWrapper = document.querySelector(".fbc-wrapper");
const iframeElement = iframeWrapper.getElementsByTagName("iframe");
const iframeChevron = document.querySelector(".fbc-iframe-chevron");
const offsetX = 20;
const offsetY = 55;
const iframePaddingAllowance = iframeBox.offsetWidth + offsetX;
const iconRightAllowance = window.innerWidth - fencePosition.x + fencePos.offsetWidth;
const iconLeftAllowance = window.innerWidth - iconRightAllowance;
if (iconRightAllowance > iframePaddingAllowance || iconLeftAllowance > iframePaddingAllowance) {
return desktopOrientation(iframeBox, iframeChevron, offsetY, fencePosition, iframePaddingAllowance, fencePos, offsetX);
}
return mobileOrientation(iframeElement, iframeChevron, iframeBox, fencePosition, offsetY);
}
function mobileOrientation(iframeElement, iframeChevron, iframeBox, fencePosition, offsetY){
// Mobile Values
const xPosMobile = fencePosition.x;
const yPosMobile = fencePosition.y + offsetY;
for (const panels of iframeElement) {
panels.width = window.innerWidth;
if (window.innerWidth > 480) {
panels.width = 350;
}
}
iframeChevron.classList.add("fbc-chevron-arrow-top");
iframeBox.style.marginTop = `${yPosMobile}px`;
const xPosChevronMobile = xPosMobile;
const yPosChevronMobile = yPosMobile - iframeChevron.offsetWidth;
iframeChevron.style.marginLeft = `${xPosChevronMobile}px`;
iframeChevron.style.marginTop = `${yPosChevronMobile}px`;
}
function desktopOrientation(iframeBox, iframeChevron, offsetY, fencePosition, iframePaddingAllowance, fencePos, offsetX) {
// Desktop Values
const xRight = fencePosition.x + offsetX + fencePos.offsetWidth;
const xLeft = fencePosition.x - iframePaddingAllowance;
const yPos = fencePosition.y - offsetY;
// Position iframe relative to FBC Icon
iframeBox.style.marginLeft = `${xRight}px`;
iframeBox.style.marginTop = `${yPos}px`;
// Add Chevron (Default left arrow)
const xPosChevron = xRight - iframeChevron.offsetWidth;
const yPosChevron = yPos + offsetY;
iframeChevron.style.marginLeft = `${xPosChevron}px`;
iframeChevron.style.marginTop = `${yPosChevron}px`;
const calculateOffsetDiff = window.innerWidth - fencePosition.x;
// Flip the iframe to show on the left side when icon is too close to the edge
if (iframePaddingAllowance > calculateOffsetDiff) {
iframeBox.style.marginLeft = `${xLeft}px`;
iframeChevron.classList.add("fbc-chevron-arrow-right");
iframeChevron.style.marginLeft = `${xPosChevron - fencePos.offsetWidth - iframeChevron.offsetWidth - offsetX}px`;
}
else {
iframeChevron.classList.remove("fbc-chevron-arrow-right");
}
iframeChevron.classList.remove("fbc-chevron-arrow-top");
}
I refactored it again. How is this?
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
Looks good. There's one more else {}
in desktopOrientation
there that could be turned into this?
// Flip the iframe to show on the left side when icon is too close to the edge
iframeChevron.classList.remove("fbc-chevron-arrow-top");
if (iframePaddingAllowance > calculateOffsetDiff) {
iframeBox.style.marginLeft = `${xLeft}px`;
iframeChevron.classList.add("fbc-chevron-arrow-right");
iframeChevron.style.marginLeft = `${xPosChevron - fencePos.offsetWidth - iframeChevron.offsetWidth - offsetX}px`;
return;
}
iframeChevron.classList.remove("fbc-chevron-arrow-right");
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
done!
Code LGTM. Lemme spot-check real quick though ... |
@groovecoder this change caused #863, can it be reverted until things are sorted out? |
@multun (and @zelch @alecvn who 👍'd it): it's been reverted, you can check for updates for the extension to fix the issue. |
This PR refactors the content panels into an iframe, instead of injecting it directly into the DOM.
Preview:
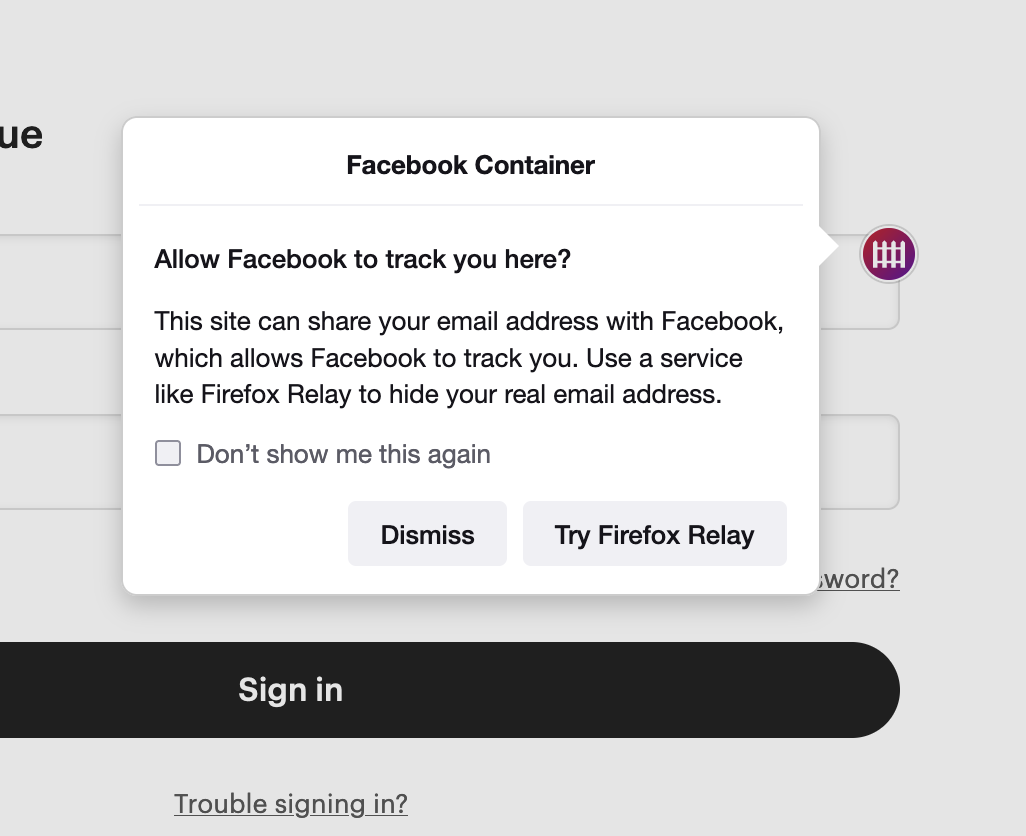
UI is updated to reflect new Firefox prompt designs.
Post-review To-Dos:
innerHTML
totextContent
Remaining To-Dos:
iframe
window according to FBC icon positioniframe
position responsive to screen resize/scrolliframe
window and when cancel + dismiss is selectediframe
windowallowClickSwitch
boolean tologin
switch caseTesting
Case 1 (Login popup)
iframe
windowCase 2 (Login + Email popup) Note: Popups should contain different content
iframe
windowiframe
window