-
Notifications
You must be signed in to change notification settings - Fork 28
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Showing
5 changed files
with
157 additions
and
47 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,89 @@ | ||
--- | ||
title: Request/Response Hooks | ||
--- | ||
|
||
Hooks allow running code as part of the Request/Response pipeline. Hooks are | ||
typically added using a plugin as shown in this document, but they can also be | ||
added globally using [Runtime Extensions](./runtime-extensions.md). | ||
|
||
:::tip | ||
|
||
All hooks can be either synchronous or asynchronous. To make your hook | ||
asynchronous simply add the `async` keyword on the function. | ||
|
||
::: | ||
|
||
### Hook: OnResponseSending | ||
|
||
The `OnResponseSending` hook on `ZuploContext` fires just before the response is | ||
sent to the client. The `Response` can be modified by returning a new `Response` | ||
from this hook. This hook is useful for creating an inbound policy that also | ||
needs to run some logic after the response is returned from the handler. | ||
|
||
The example below shows a simple tracing policy that adds a trace header to the | ||
request and ensures the same header is returned with the response. | ||
|
||
```ts | ||
import { ZuploContext, ZuploRequest } from "@zuplo/runtime"; | ||
|
||
export async function tracingPlugin( | ||
request: ZuploRequest, | ||
context: ZuploContext, | ||
policyName: string, | ||
) { | ||
// Get the trace header | ||
let traceparent = request.headers.get("traceparent"); | ||
|
||
// If not set, add the header to the request | ||
if (!traceparent) { | ||
traceparent = crypto.randomUUID(); | ||
const headers = new Headers(request.headers); | ||
headers.set("traceparent", traceparent); | ||
return new Request(request, { headers }); | ||
} | ||
|
||
context.addResponseSendingHook((response, latestRequest, context) => { | ||
// If the response doesn't have the trace header that matches, set it | ||
if (response.headers.get("traceparent") !== traceparent) { | ||
const headers = new Headers(response.headers); | ||
headers.set("traceparent", traceparent); | ||
return new Response(response.body, { | ||
headers, | ||
}); | ||
} | ||
return response; | ||
}); | ||
|
||
return request; | ||
} | ||
``` | ||
|
||
### Hook: OnResponseSendingFinal | ||
|
||
The `OnResponseSendingFinal` hook on `ZuploContext` fires immediately after the | ||
response is sent to the client. The `Response` in this hook is immutable and the | ||
body has been used. This hook is useful for custom performing various tasks like | ||
logging or analytics. | ||
|
||
```ts | ||
import { ZuploContext, ZuploRequest } from "@zuplo/runtime"; | ||
|
||
export async function pluginWithHook( | ||
request: ZuploRequest, | ||
context: ZuploContext, | ||
policyName: string, | ||
) { | ||
const cloned = request.clone(); | ||
context.addResponseSendingFinalHook( | ||
async (response, latestRequest, context) => { | ||
const body = await cloned.text(); | ||
await fetch("https://example.com", { | ||
method: "GET", | ||
body, | ||
}); | ||
}, | ||
); | ||
|
||
return request; | ||
} | ||
``` |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
|
@@ -28,9 +28,10 @@ JSON. Note, | |
The API key auth policy should usually be one of the first policies in your | ||
request pipeline, drag it to the top if you have multiple policies. | ||
|
||
<Screenshot src="https://cdn.zuplo.com/assets/8f698429-f265-40d9-99d7-156b28b7ef1b.gif" size="sm" /> | ||
::: | ||
|
||
<Screenshot src="https://cdn.zuplo.com/assets/8f698429-f265-40d9-99d7-156b28b7ef1b.gif" size="sm" /> | ||
|
||
If you test your route, you should get a 401 Unauthorized response | ||
|
||
``` | ||
|
@@ -68,25 +69,25 @@ Since we need to send the key in a header, it's hard to use the browser for this | |
test. We'll use our built in test client in Zuplo but you could also use Postman | ||
for this part. | ||
|
||
Go to the API Test Console and create a new **Manual Test**. Set the **path** to | ||
`/todos` and hit **Test**. | ||
Next to the path of your route in Route Designer click the **Test** button. Set | ||
the **path** to `/todos` and hit **Test**. | ||
|
||
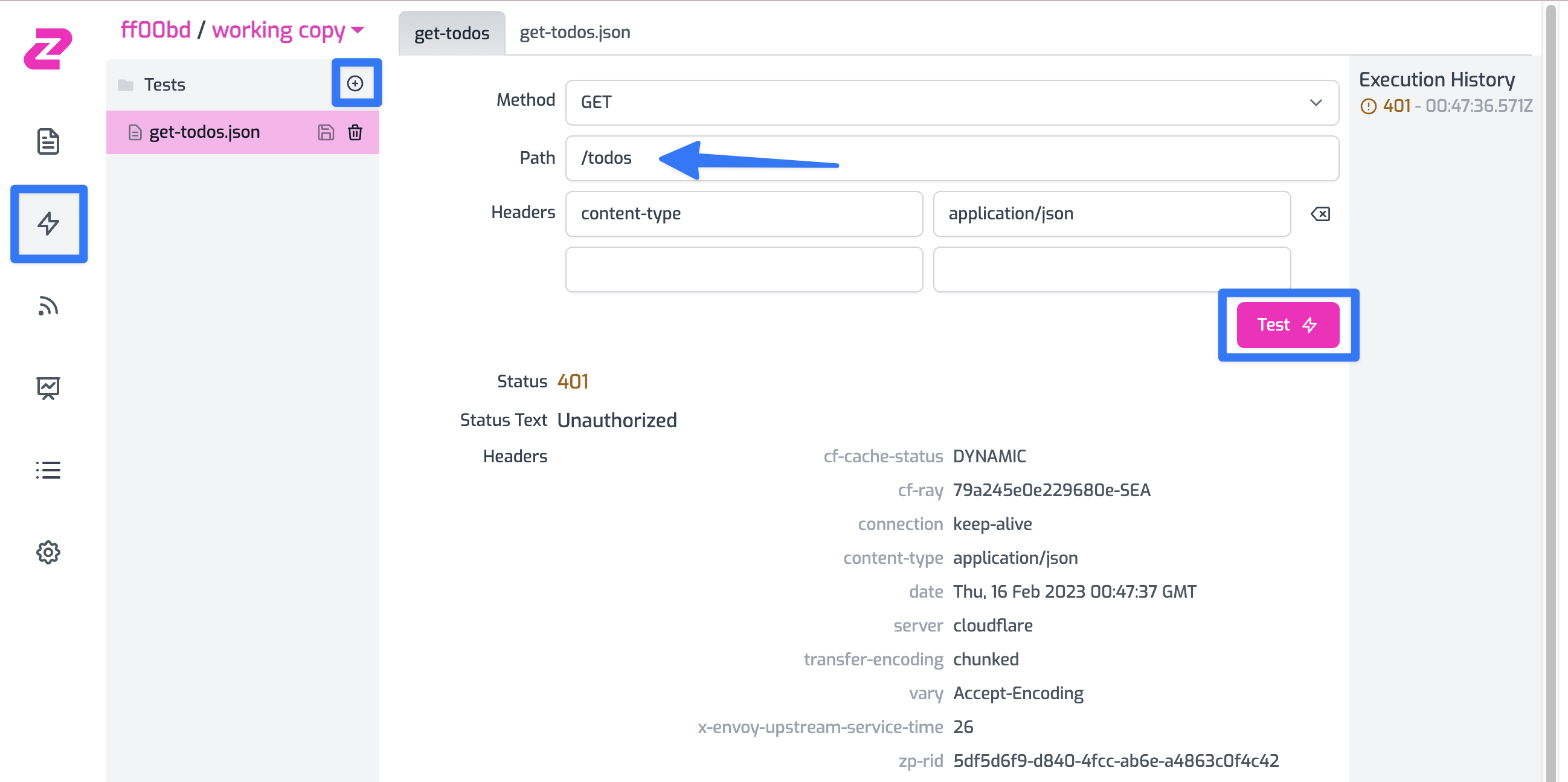 | ||
<Screenshot src="https://cdn.zuplo.com/assets/c2db1247-eb72-474d-bfed-8b14b3b62b5e.png" /> | ||
|
||
You should get a 401 Unauthorized response. Add an new `authorization` header | ||
with the value `Bearer YOUR_API_KEY` and insert the API Key you got from the | ||
developer portal. | ||
|
||
You should now get a 200 OK. | ||
|
||
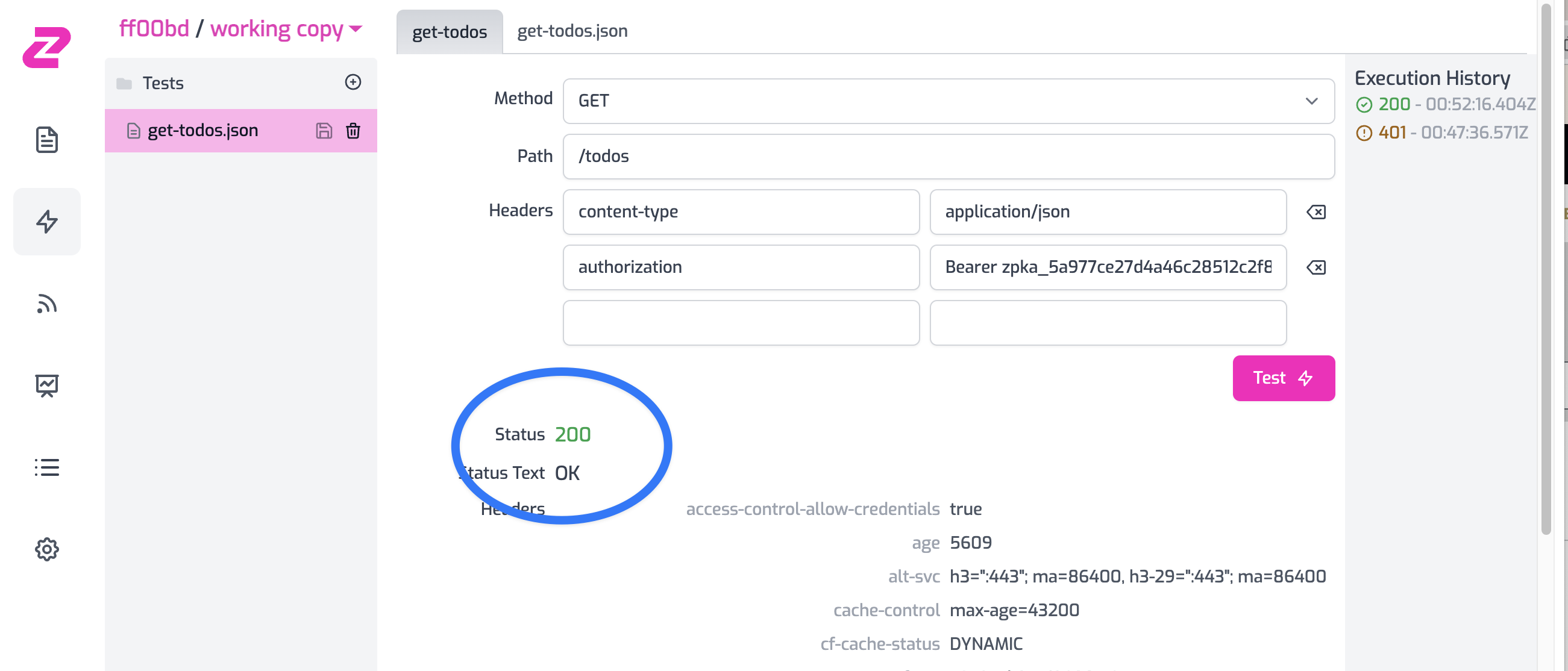 | ||
<Screenshot src="https://cdn.zuplo.com/assets/87c03fc4-4525-43dd-8eb7-15808b545fef.png" /> | ||
|
||
:::note | ||
|
||
We also offer an API for our API key service that allows you to programmatically | ||
create consumers and even create your own developer portal or integrate key | ||
management into your existing dashboard. Contact us at `[email protected]` for | ||
access. | ||
management into your existing dashboard. See | ||
[this document for details](./api-key-api.md). | ||
|
||
::: | ||
|
||
|
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
bb22e7f
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
Successfully deployed to the following URLs:
docs – ./
docs-git-main.zuplopreview.net
docs.zuplopreview.net
docs.zuplo.site