|
| 1 | +# Prolem 3 - *(Human Compiler)(Hand-written) |
| 2 | + |
| 3 | +## Problem 3a: Swaps two arrays using pointers |
| 4 | + |
| 5 | +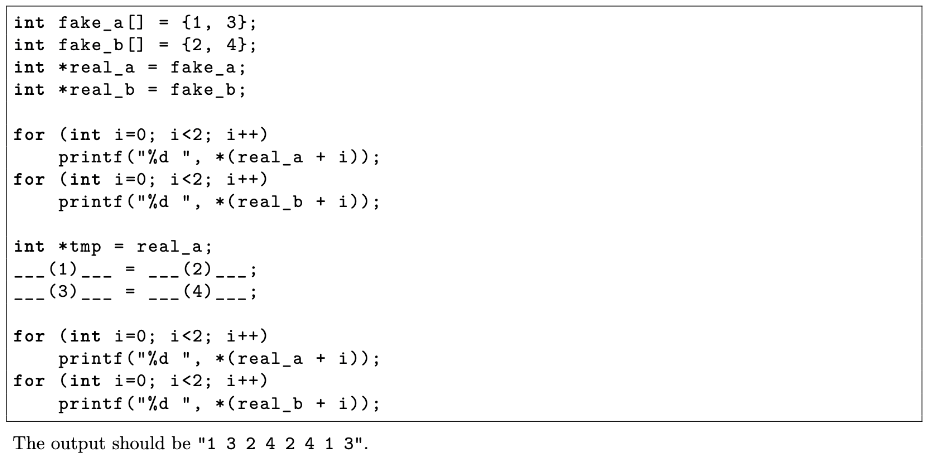 |
| 6 | + |
| 7 | + |
| 8 | +```c |
| 9 | +int fake_a[] = {1,3}; |
| 10 | +int fake_b[] = {2,4}; |
| 11 | +int *real_a = fake_a; |
| 12 | +int *real_b = fake_b; |
| 13 | + |
| 14 | +for(int i=0;i<2;i++) |
| 15 | + printf("%d", *(real_a + i)); |
| 16 | +for(int i=0;i<2;i++) |
| 17 | + printf("%d", *(real_b + i)); |
| 18 | + |
| 19 | +int *tmp = real_a; //tmp is a pointer to pointer |
| 20 | +real_b = fake_a; // fill the blanks |
| 21 | +real_a = fake_b; // fil the blanks |
| 22 | + |
| 23 | +for(int i=0;i<2;i++) |
| 24 | + printf("%d", *(real_a + i)); |
| 25 | +for(int i=0;i<2;i++) |
| 26 | + printf("%d", *(real_b + i)); |
| 27 | +``` |
| 28 | +
|
| 29 | +Test at: https://onlinegdb.com/SJ4JFi-7_ |
| 30 | +
|
| 31 | + |
| 32 | +
|
| 33 | +--- |
| 34 | +
|
| 35 | +## Problem 3b: An array supporting negative indices |
| 36 | +
|
| 37 | +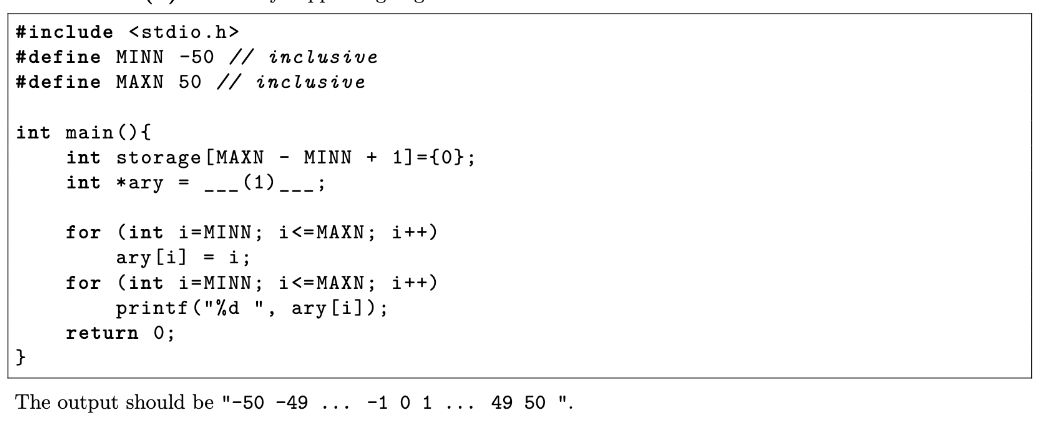 |
| 38 | +
|
| 39 | +```c |
| 40 | +#include <stdio.h> |
| 41 | +#define MINN -50 |
| 42 | +#define MAXN 50 |
| 43 | +
|
| 44 | +int main(){ |
| 45 | + int storage[MAXN - MINN + 1] = {0}; // 101 |
| 46 | + int *ary = storage - MINN ; //fill the blank |
| 47 | +
|
| 48 | + for(int i=MINN;i<=MAXN;i++) |
| 49 | + ary[i] = i; |
| 50 | + for(int i=MINN;i<=MAXN;i++) |
| 51 | + printf("%d", ary[i]); |
| 52 | + return 0; |
| 53 | +} |
| 54 | +``` |
| 55 | +Verified at: https://onlinegdb.com/rydhl3W7u |
| 56 | + |
| 57 | + |
| 58 | + |
| 59 | +--- |
| 60 | + |
| 61 | +## Problem 3C: Tranverses data nodes in a linked list. |
| 62 | + |
| 63 | +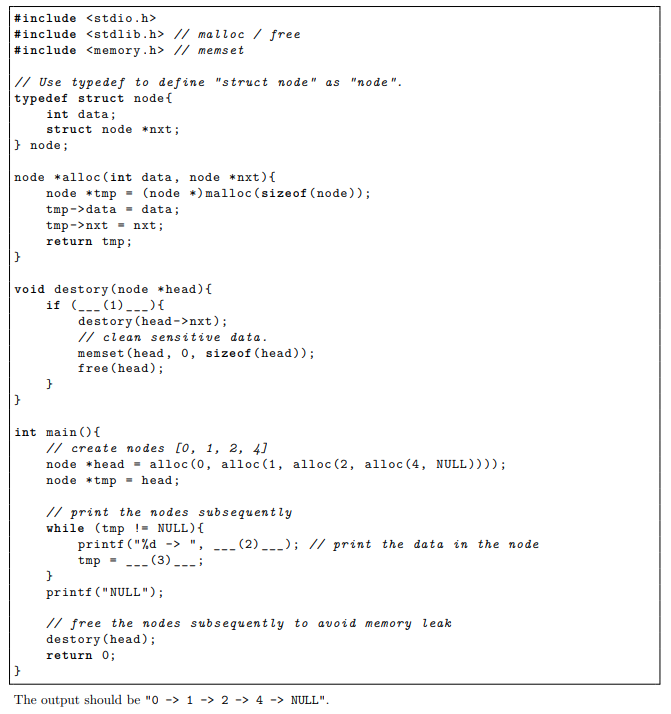 |
| 64 | + |
| 65 | +```c |
| 66 | +#include <stdio.h> |
| 67 | +#include <stdlib.h> //malloc / free |
| 68 | +#include <memory.h> //memset |
| 69 | + |
| 70 | +//use typedef to define "struct node" as "node" |
| 71 | +typedef struct node{ |
| 72 | + int data; |
| 73 | + struct node *nxt; |
| 74 | +} node; |
| 75 | + |
| 76 | +node* alloc(int data, node* nxt){ |
| 77 | + node *tmp = (node*)malloc(sizeof(node)); |
| 78 | + tmp->data=data; |
| 79 | + tmp->nxt = nxt; |
| 80 | + return tmp; |
| 81 | +} |
| 82 | + |
| 83 | +void destroy(node *head){ |
| 84 | + if(head != NULL){ //FIll the blank |
| 85 | + destroy(head->nxt); |
| 86 | + //clean sensitive data; |
| 87 | + memset(head,0,sizeof(head)); |
| 88 | + free(head); |
| 89 | + } |
| 90 | +} |
| 91 | + |
| 92 | +int main(){ |
| 93 | + // create nodes [0,1,2,4] |
| 94 | + node* head = alloc(0, alloc(1,alloc(2,alloc(4,NULL)))); |
| 95 | + node* tmp = head; |
| 96 | + //print the nodes subsequently |
| 97 | + while(tmp!=NULL){ |
| 98 | + printf("%d -> ", *tmp-> data ); //FIll the blank |
| 99 | + tmp = (*tmp)->nxt; //FIll the blank |
| 100 | + } |
| 101 | + printf("NULL"); |
| 102 | + |
| 103 | + //free the nodes subsequently to avoid memory leak |
| 104 | + destroy(head); |
| 105 | + return 0; |
| 106 | +} |
| 107 | +``` |
| 108 | +
|
| 109 | +### Curate |
| 110 | +
|
| 111 | +Use `temp->data` not `(*tmp)->data` |
| 112 | +
|
| 113 | +#### Copy a pointer to another pointer |
| 114 | +
|
| 115 | +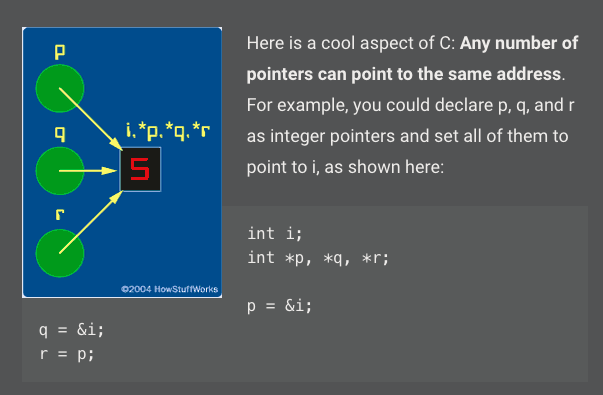 |
| 116 | +> From [Basics of C Programmin](https://computer.howstuffworks.com/c24.htm) |
| 117 | +
|
| 118 | +### Question |
| 119 | +
|
| 120 | +Wrong version: https://www.onlinegdb.com/ |
| 121 | +Corrected version: https://onlinegdb.com/BkReV0bXd |
| 122 | +
|
| 123 | +--- |
| 124 | +
|
| 125 | +## Problem 3D: Binary Tree |
| 126 | +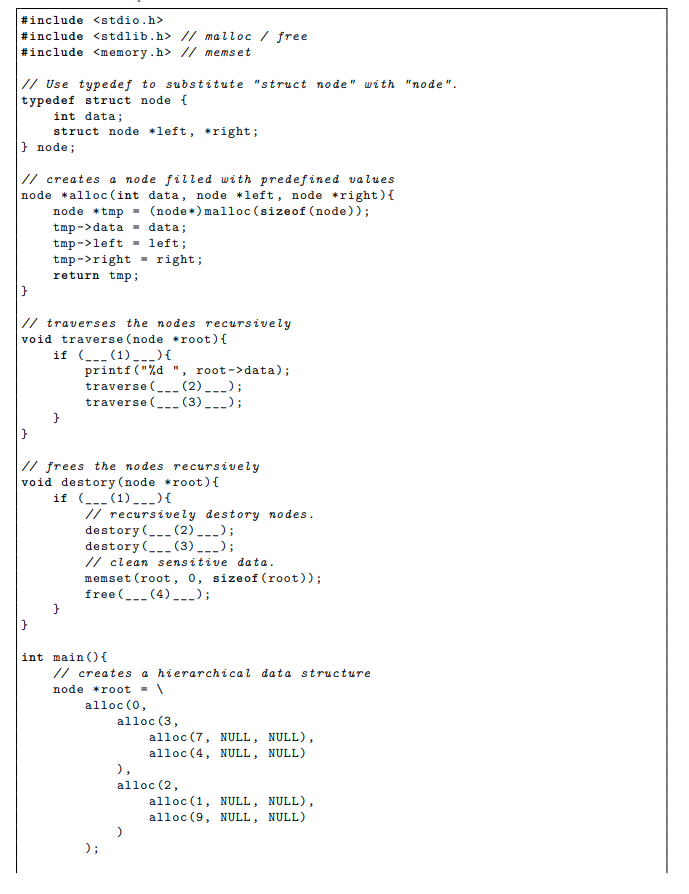 |
| 127 | +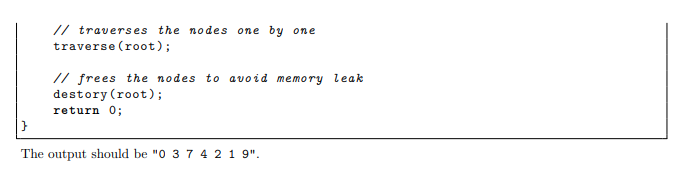 |
| 128 | +
|
| 129 | +**問題**: `printf` 應該在 `if` 前面 |
| 130 | +
|
| 131 | +```c |
| 132 | +#include <stdio.h> |
| 133 | +#include <stdlib.h> //malloc /free |
| 134 | +#include <memory.h> //memset |
| 135 | +
|
| 136 | +//use typedef to substitute "struct node with "node"" |
| 137 | +typedef struct node { |
| 138 | + int data; |
| 139 | + struct node *left, *right; |
| 140 | +} node; |
| 141 | +
|
| 142 | +//creates a node filledwith predefined values |
| 143 | +node* alloc(int data, node *left, node *right){ |
| 144 | + node *tmp = (node*)malloc(sizeof(node)); |
| 145 | + tmp->data = data, |
| 146 | + tmp->left = left; |
| 147 | + tmp->right = right; |
| 148 | + return tmp; |
| 149 | +} |
| 150 | +
|
| 151 | +//traverses (遍歷) the nodes recursively |
| 152 | +void traverse(node* root){ |
| 153 | + printf("%d", root->data); |
| 154 | + if ((root->left != NULL) & (root->right != NULL)){ |
| 155 | + traverse(root->left); |
| 156 | + traverse(root->right); |
| 157 | + } |
| 158 | +} |
| 159 | +
|
| 160 | +//frees the nodes recursively |
| 161 | +void destroy(node *root){ |
| 162 | + if ((root->left != NULL) & (root->right!=NULL)){ |
| 163 | + destroy(root->left); |
| 164 | + destroy(root->right); |
| 165 | + //clean sensitive data |
| 166 | + memset(root, 0, sizeof(root)); |
| 167 | + free(root); |
| 168 | + } |
| 169 | +} |
| 170 | +
|
| 171 | +int main(){ |
| 172 | + // creates a hierarchical data structure |
| 173 | + node *root = \ |
| 174 | + alloc(0, |
| 175 | + alloc(3, |
| 176 | + alloc(7, NULL, NULL), |
| 177 | + alloc(4, NULL, NULL) |
| 178 | + ), |
| 179 | + alloc(2, |
| 180 | + alloc(1, NULL, NULL), |
| 181 | + alloc(9, NULL, NULL) |
| 182 | + ) |
| 183 | + ); |
| 184 | +
|
| 185 | + traverse(root); |
| 186 | + destroy(root); |
| 187 | +} |
| 188 | +``` |
| 189 | +Verify at: https://onlinegdb.com/r1VxlA-7d |
| 190 | + |
| 191 | + |
| 192 | +--- |
| 193 | + |
| 194 | +## Notes |
| 195 | + |
| 196 | +### Null Pointer and `Segmentation fault` |
| 197 | + |
| 198 | +- Segmentation fault: |
| 199 | + > Segmentation fault is a specific kind of error caused by accessing memory that “does not belong to you |
| 200 | +- NULL Pointer |
| 201 | + > People assign NULL to pointer to indicate that it points to nothing. |
| 202 | +
|
| 203 | +### Read Only String and its Dynamical type |
| 204 | + |
| 205 | +- `char* str` Read only: able to share among functions |
| 206 | + ```c |
| 207 | + char *str = "gfg"; |
| 208 | + ``` |
| 209 | + Read only. `str[0]=a` leads to segmentation fault. Noted that read-only string can be passed among functions: |
| 210 | + ```c |
| 211 | + char *getString(){ |
| 212 | + char *str = "GfG"; /* Stored in read only part of shared segment */ |
| 213 | + |
| 214 | + /* No problem: remains at address str after getString() returns*/ |
| 215 | + return str; |
| 216 | + } |
| 217 | + |
| 218 | + int main(){ |
| 219 | + printf("%s", getString()); |
| 220 | + getchar(); |
| 221 | + return 0; |
| 222 | + } |
| 223 | + |
| 224 | + ``` |
| 225 | +- `char str[]` Mutable string: unable to share among functions. |
| 226 | + ```c |
| 227 | + int main(){ |
| 228 | + char str[]; |
| 229 | + str = "gfg"; |
| 230 | + } |
| 231 | + ``` |
| 232 | +- Store in heap: allow to share and modify |
| 233 | + ```c |
| 234 | + char *getString() |
| 235 | + { |
| 236 | + int size = 4; |
| 237 | + char *str = (char *)malloc(sizeof(char)*size); /*Stored in heap segment*/ |
| 238 | + *(str+0) = 'G'; |
| 239 | + *(str+1) = 'f'; |
| 240 | + *(str+2) = 'G'; |
| 241 | + *(str+3) = '\0'; |
| 242 | + |
| 243 | + /* No problem: string remains at str after getString() returns */ |
| 244 | + return str; |
| 245 | + } |
| 246 | + int main() |
| 247 | + { |
| 248 | + printf("%s", getString()); |
| 249 | + getchar(); |
| 250 | + return 0; |
| 251 | + } |
| 252 | + ``` |
| 253 | + |
| 254 | +--- |
| 255 | +## Reference |
| 256 | +1. Segmentation fault and pointer. [[stackoverflow](https://stackoverflow.com/questions/17873561/pointer-initialisation-gives-segmentation-fault)] |
| 257 | +2. String and storage. [[GreekforGeek](https://www.geeksforgeeks.org/storage-for-strings-in-c/)] |
| 258 | +3. Pointing to the same address. [[eBook-Basics of C Programming](https://computer.howstuffworks.com/c24.htm)] |
0 commit comments