-
Notifications
You must be signed in to change notification settings - Fork 0
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
1 parent
bf559c7
commit 922c826
Showing
3 changed files
with
76 additions
and
49 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,4 +1,5 @@ | ||
from setuptools import setup, find_packages | ||
import os | ||
|
||
# Version of the package | ||
VERSION = "0.1.0.post1" | ||
|
@@ -11,6 +12,21 @@ | |
) | ||
|
||
|
||
def get_long_description(): | ||
with open("README.md", "r", encoding="utf-8") as f: | ||
content = f.read() | ||
|
||
# Adjust the content for PyPI: Remove the last 4 lines and replace with markdown link | ||
content_lines = content.splitlines() | ||
if len(content_lines) >= 4: | ||
content_lines = content_lines[:-4] # Remove the last 4 lines | ||
content_lines.append( | ||
'[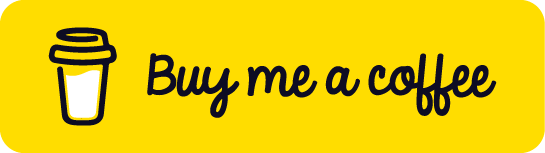]' | ||
'(https://www.buymeacoffee.com/smartnodes)' | ||
) | ||
return "\n".join(content_lines) | ||
|
||
|
||
# Parse requirements from requirements.txt | ||
def parse_requirements(filename): | ||
"""Load requirements from a pip requirements file.""" | ||
|
@@ -26,7 +42,7 @@ def parse_requirements(filename): | |
author="Smartnodes Lab", | ||
author_email="[email protected]", | ||
description=DESCRIPTION, | ||
long_description=open("README.md").read(), | ||
long_description=get_long_description(), | ||
long_description_content_type="text/markdown", | ||
packages=find_packages(), # Automatically find packages in the current directory | ||
include_package_data=True, | ||
|
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters