-
Notifications
You must be signed in to change notification settings - Fork 54
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
From integrating Typewriter at Segment, we've learned that throwing an error whenever Typewriter discovers a validation issues is a pretty poor DX because it forces you to align your instrumentation and spec from day 1. Instead, we want to offer developers the opportunity to iteratively adopt Typewriter. To do so, this PR exposes an `onError` handler that can be configured at run-time when constructing an instance of a Typewriter client. As an example, this is what happens now when you have a validation error: 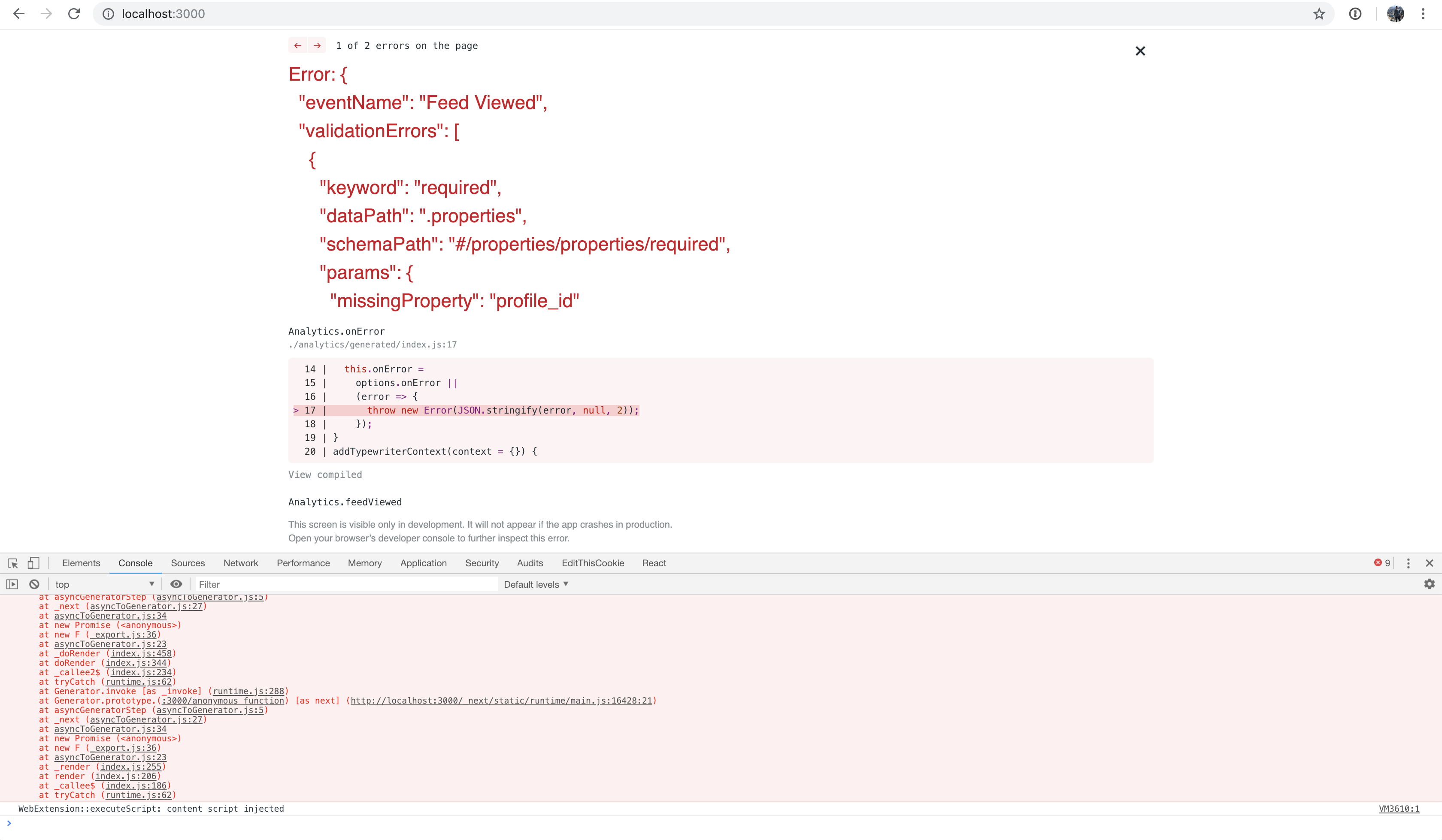 Your entire app breaks while developing locally -- and that interrupts your developer flow. Now, you can hook in to that error handling logic like so: ```js const analytics = new Analytics(window.analytics, { onError: (error) => { console.error(JSON.stringify(error, null, 2)) } }) ``` After doing this, the errors will get logged instead: 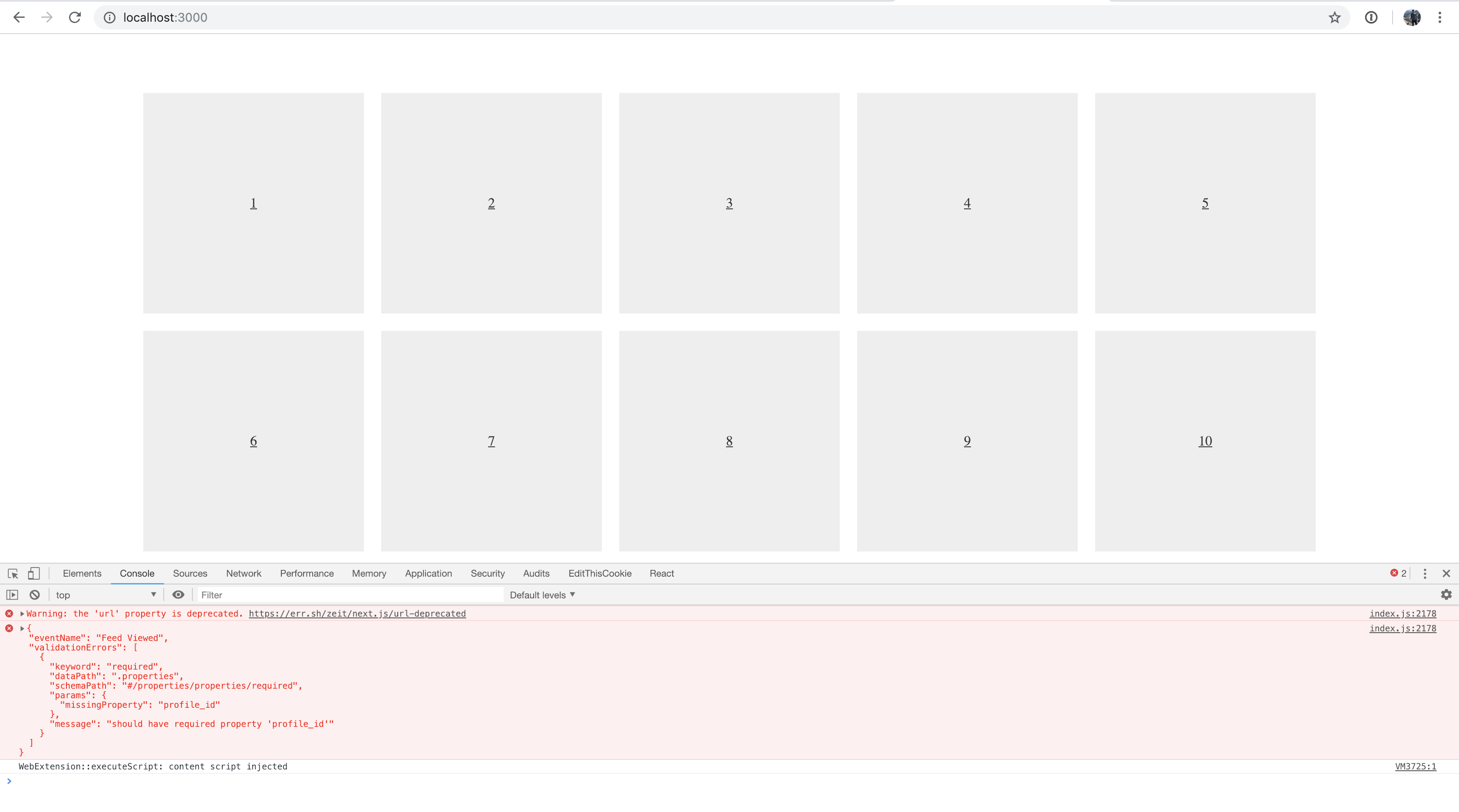 This allows you to handle errors however you want! For example, you could `alert` on errors: 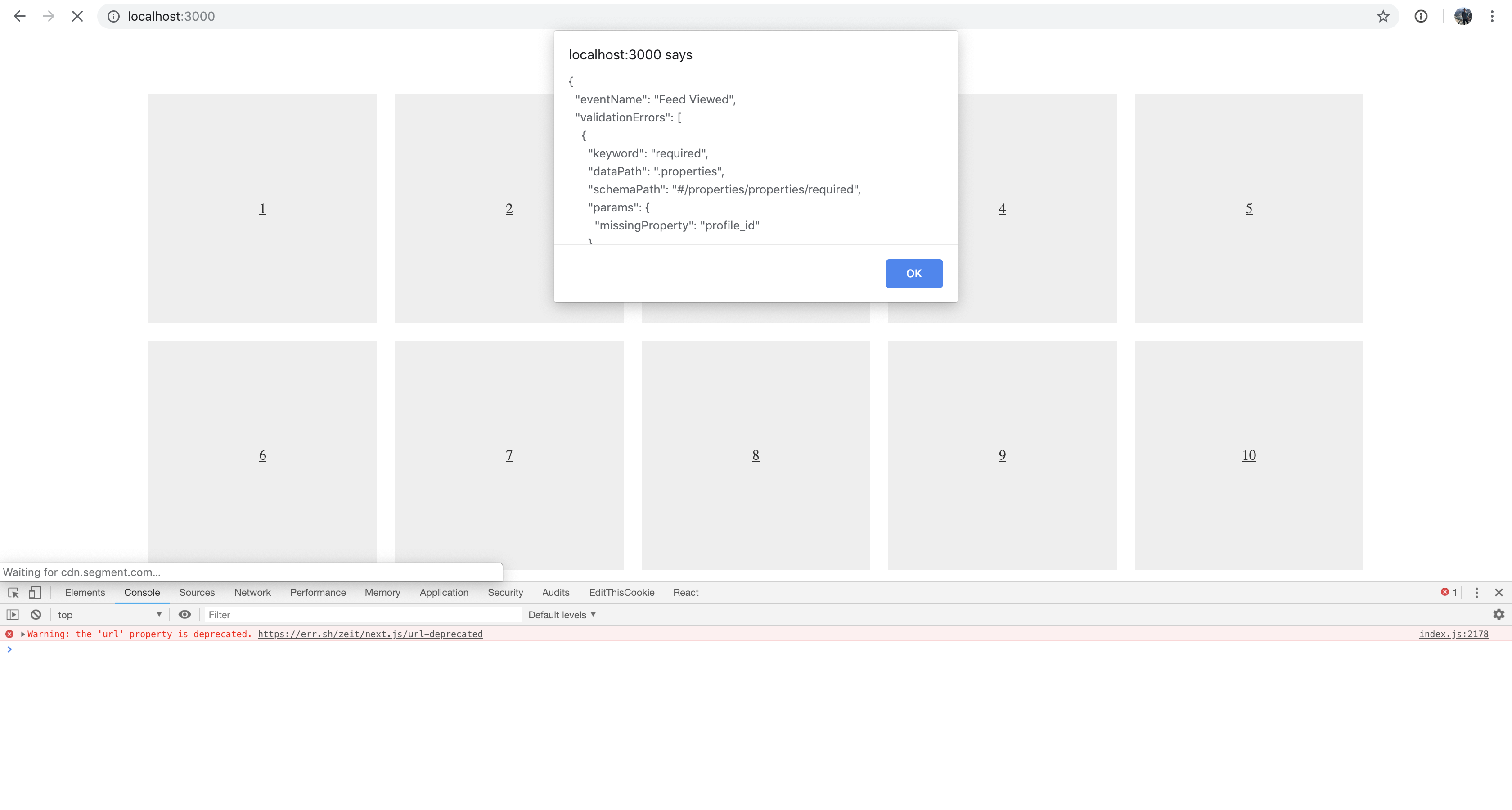 Or you could use this to ship validation to production without crashing your app and forward validation issues to Sentry/BugSnag/etc.
- Loading branch information
Showing
19 changed files
with
488 additions
and
75 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Oops, something went wrong.