-
Notifications
You must be signed in to change notification settings - Fork 350
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
## Summary <!-- Ideally, there is an attached Linear ticket that will describe the "why". If relevant, use this section to call out any additional information you'd like to _highlight_ to the reviewer. --> Currently, we can only do a direct match for key attributes (e.g. `workspace_id:1`). This PR improves the matching, namely: * Add wildcard support (`service:*foo*` will match `service:barfoobaz`) * Add multi-word support `service:'image processor'` will match `service:image processor`) Additionally this PR improves code quality: * Use a true query builder instead of rolling our code. I chose to use [squirrel](https://github.com/Masterminds/squirrel). This makes conditional queries a lot easier and removes usages of `fmt.Sprintf` which is just ripe for a sql injection. * Drop support for [testify suite](https://github.com/stretchr/testify#suite-package). This is currently unusable with vscode (golang/vscode-go#2414). ## How did you test this change? Verified space search works 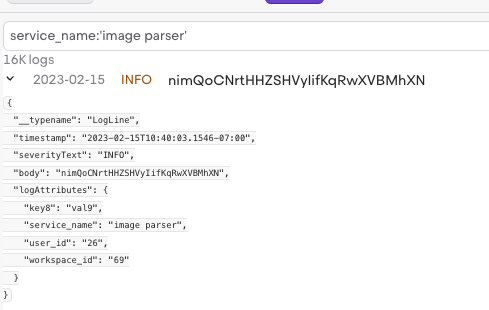 Verified wildcard search works 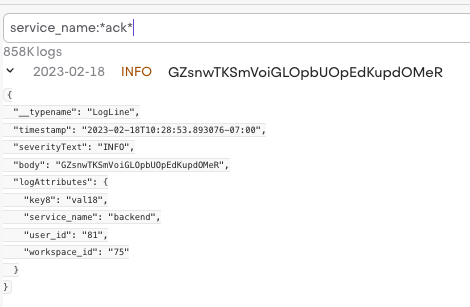 Verified we can search with body as well 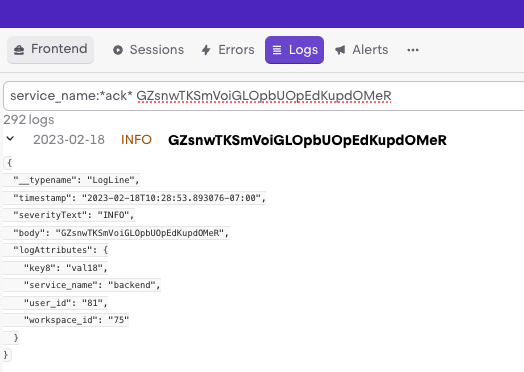 ## Are there any deployment considerations? <!-- Backend - Do we need to consider migrations or backfilling data? --> N/A
- Loading branch information
Showing
7 changed files
with
272 additions
and
224 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Oops, something went wrong.