-
Notifications
You must be signed in to change notification settings - Fork 0
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Showing
2 changed files
with
176 additions
and
0 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,26 @@ | ||
--- | ||
title: nestjs中的一些基本概念 | ||
date: 2023-11-07 22:54:02 | ||
tags: nestjs | ||
categories: 后端 | ||
--- | ||
|
||
## AOP | ||
|
||
面向切片编程 | ||
|
||
[面向切面的程序设计](https://zh.wikipedia.org/wiki/面向切面的程序设计) | ||
|
||
## IOC | ||
|
||
[控制反转](https://zh.wikipedia.org/wiki/控制反转) | ||
|
||
控制反转是[面向对象编程](https://zh.wikipedia.org/wiki/%E9%9D%A2%E5%90%91%E5%AF%B9%E8%B1%A1%E7%BC%96%E7%A8%8B)中的一种设计原则,可以用来减低计算机代码之间的[耦合度](<https://zh.wikipedia.org/wiki/%E8%80%A6%E5%90%88%E5%BA%A6_(%E8%A8%88%E7%AE%97%E6%A9%9F%E7%A7%91%E5%AD%B8)>)。其中最常见的方式叫做**依赖注入**(Dependency Injection,简称**DI**) | ||
|
||
### DI | ||
|
||
[依赖注入](https://zh.wikipedia.org/wiki/依赖注入) | ||
|
||
## 生命周期 | ||
|
||
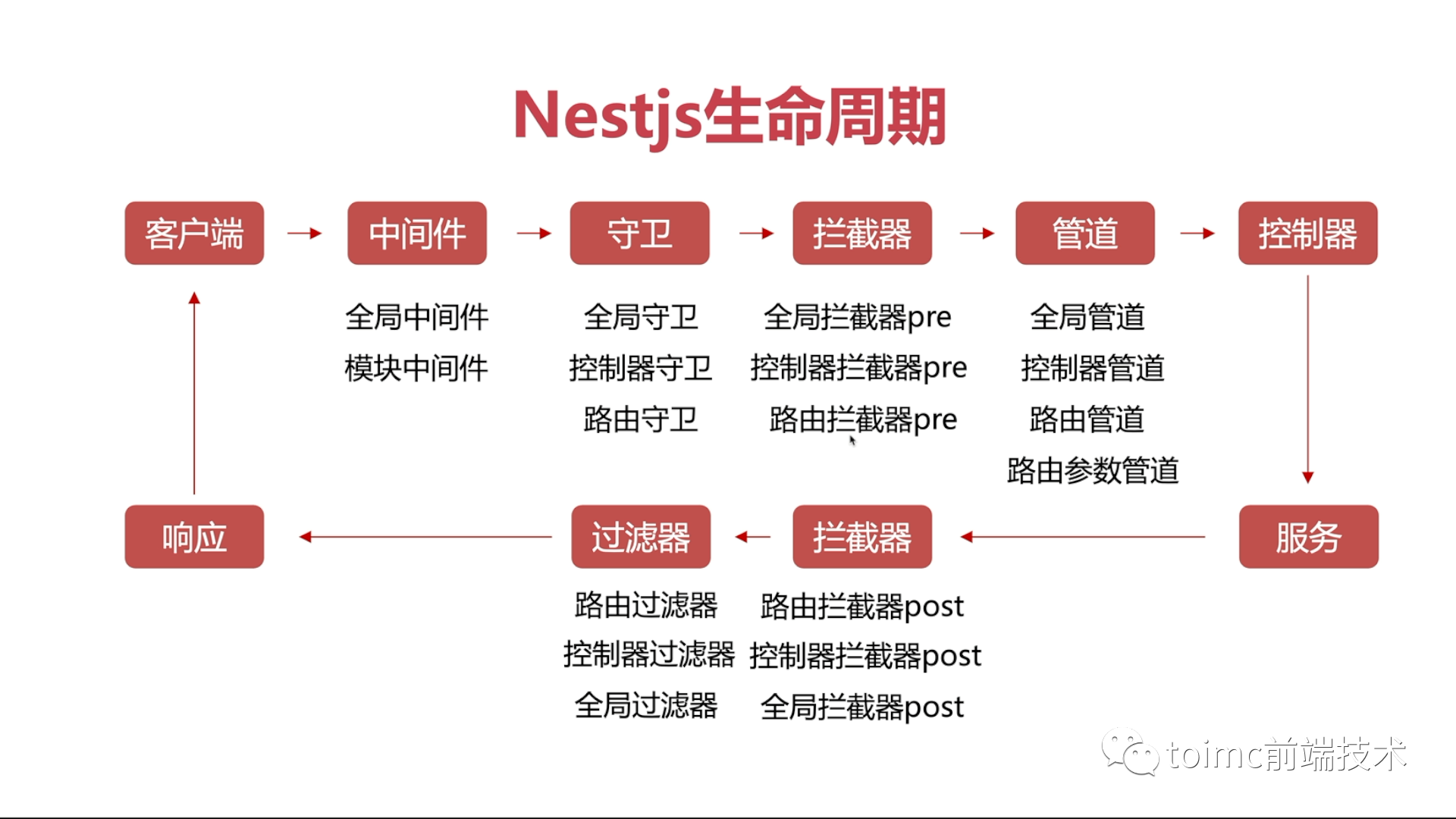 |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,150 @@ | ||
--- | ||
title: nestjs环境搭建 | ||
date: 2023-11-07 22:47:50 | ||
tags: nestjs | ||
categories: 后端 | ||
--- | ||
|
||
## 项目初始化 | ||
|
||
使用 nest cli 直接搭建即可 | ||
|
||
### nest cli 使用 | ||
|
||
先安装 | ||
|
||
```jsx | ||
npm install -g @nestjs/cli | ||
``` | ||
|
||
使用命令新建项目 | ||
|
||
```jsx | ||
nest new project-name | ||
``` | ||
|
||
常用命令 | ||
|
||
```jsx | ||
nest g <schematic> <name> [options] | ||
``` | ||
|
||
[Documentation | NestJS - A progressive Node.js framework](https://docs.nestjs.com/cli/usages#nest-generate) | ||
|
||
## 目录结构最佳实践 | ||
|
||
可以参考[nodepress](https://github.com/surmon-china/nodepress)这个项目的目录结构 | ||
|
||
## 环境变量 | ||
|
||
使用@nestjs/config 来进行环境变量的加载,@nestjs/config 内部使用了 dotenv | ||
|
||
先安装 cross-env | ||
|
||
```jsx | ||
pnpm install --save-dev cross-env | ||
``` | ||
|
||
修改一下 package.json 脚本 | ||
|
||
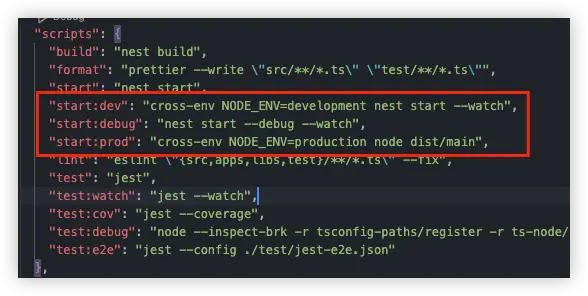 | ||
|
||
安装@nestjs/config | ||
|
||
```jsx | ||
pnpm i @nestjs/config | ||
``` | ||
|
||
在根目录创建 env 文件夹存放配置文件,一般都是一些 database 的定义 | ||
|
||
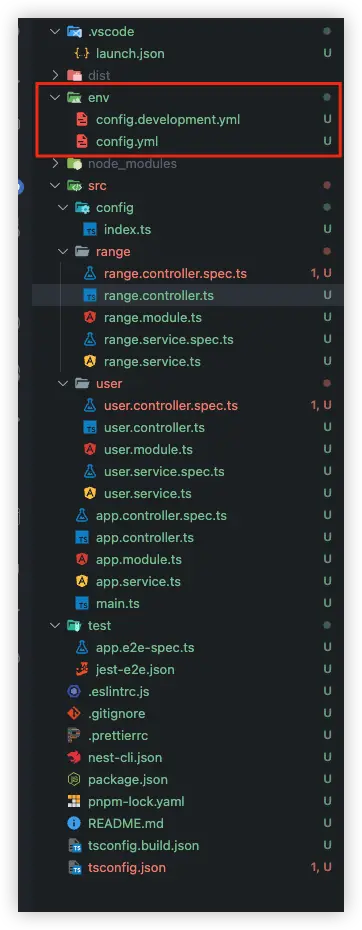 | ||
|
||
### 引入 | ||
|
||
```tsx | ||
import { readFileSync } from 'fs'; | ||
|
||
import * as yaml from 'js-yaml'; | ||
import { join } from 'path'; | ||
|
||
const env = process.env.NODE_ENV; | ||
|
||
console.log(env); | ||
|
||
const BASE_YAML_CONFIG_FILENAME = `env/config.yml`; | ||
const YAML_CONFIG_FILENAME = `env/config.${env}.yml`; | ||
const baseFilePath = join(__dirname, '../../', BASE_YAML_CONFIG_FILENAME); | ||
const filePath = join(__dirname, '../../', YAML_CONFIG_FILENAME); | ||
|
||
export const loadEnvConfig = () => { | ||
return [ | ||
() => yaml.load(readFileSync(baseFilePath, 'utf8')), | ||
() => yaml.load(readFileSync(filePath, 'utf8')), | ||
]; | ||
}; | ||
|
||
export const APP = { | ||
host: '127.0.0.1', | ||
port: '3000', | ||
prefix: 'api', | ||
version: 'v1', | ||
}; | ||
``` | ||
|
||
### 使用 | ||
|
||
在根 module 中引入 | ||
|
||
```tsx | ||
// app.module.ts | ||
import { Module } from '@nestjs/common'; | ||
import { AppController } from './app.controller'; | ||
import { AppService } from './app.service'; | ||
|
||
import { ConfigModule } from '@nestjs/config'; | ||
import { loadEnvConfig } from './config'; | ||
|
||
@Module({ | ||
imports: [ | ||
ConfigModule.forRoot({ | ||
isGlobal: true, | ||
load: [...loadEnvConfig()], | ||
}), | ||
], | ||
controllers: [AppController], | ||
providers: [AppService], | ||
}) | ||
export class AppModule {} | ||
``` | ||
|
||
之后在需要的 controller 中引入即可 | ||
|
||
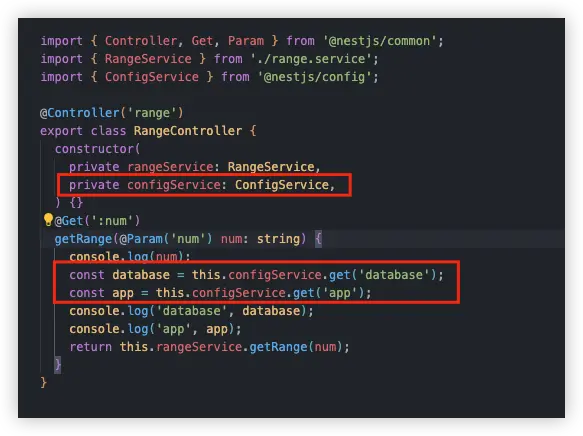 | ||
|
||
成功 | ||
|
||
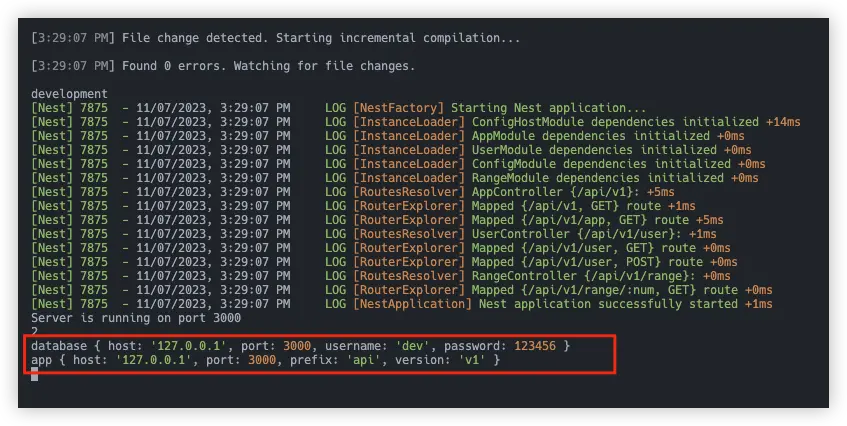 | ||
|
||
### 变量校验 | ||
|
||
使用 joi 进行校验 | ||
|
||
```tsx | ||
pnpm i joi | ||
``` | ||
|
||
## TypeORM | ||
|
||
### ORM | ||
|
||
对象关系映射,主要作用是把编程中的面向对象概念和数据库中的概念对应起来。 | ||
|
||
特点 | ||
|
||
- 方便维护 | ||
- 代码量少、对接多种库 | ||
- 工具多、自动化能力强 | ||
|
||
缺点 | ||
|
||
- 性能要比 sql 语句要慢,不好优化。 |