|
1 | 1 | # Build Golang RESTful API with Gorm, Gin and Postgres
|
2 | 2 |
|
3 |
| -### 1. How to Setup Golang GORM RESTful API Project with Postgres |
| 3 | +## 1. How to Setup Golang GORM RESTful API Project with Postgres |
4 | 4 |
|
5 |
| -[How to Setup Golang GORM RESTful API Project with Postgres](https://codevoweb.com/setup-golang-gorm-restful-api-project-with-postgres/) |
| 5 | +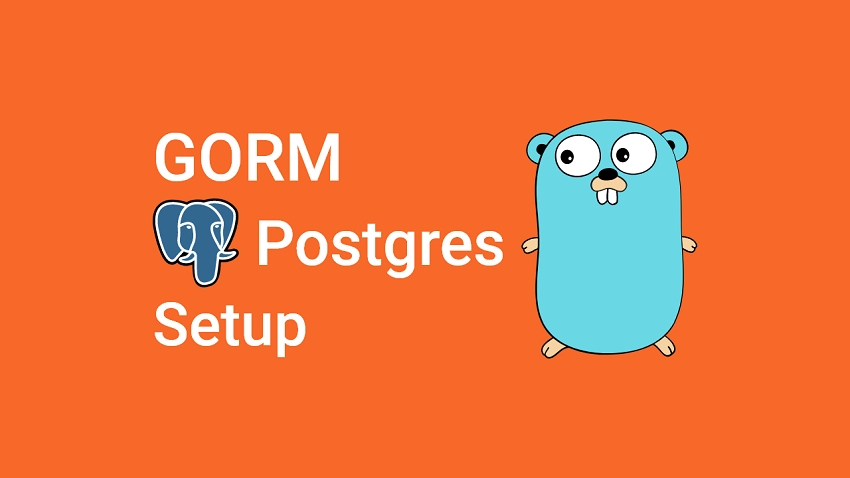 |
6 | 6 |
|
7 |
| -### 2. API with Golang + GORM + PostgreSQL: Access & Refresh Tokens |
| 7 | +This article will guide you on how you can set up a Golang project with the GORM library and PostgreSQL to build CRUD RESTful API to perform the basic Create/Get/Update/Delete operations |
8 | 8 |
|
9 |
| -[API with Golang + GORM + PostgreSQL: Access & Refresh Tokens](https://codevoweb.com/golang-gorm-postgresql-user-registration-with-refresh-tokens) |
| 9 | +### Topics Covered |
10 | 10 |
|
11 |
| -### 3. Golang and GORM - User Registration and Email Verification |
| 11 | +- What is an ORM? |
| 12 | +- Setup the Golang Project |
| 13 | + - Initialize the Golang Project |
| 14 | + - Create a PostgreSQL Docker Container |
| 15 | + - Load and Validate the Environment Variables |
| 16 | + - Create a Utility Function to Connect to PostgreSQL |
| 17 | +- Data Modeling and Migration with GORM |
| 18 | + - Creating the Database Model with GORM |
| 19 | + - Install the UUID OSSP Module for PostgreSQL |
| 20 | + - Migrating the Schema with GORM |
| 21 | +- Create the Golang Server with Gin Gonic |
| 22 | +- Testing the Golang API |
12 | 23 |
|
13 |
| -[Golang and GORM - User Registration and Email Verification](https://codevoweb.com/golang-and-gorm-user-registration-email-verification) |
| 24 | +Read the entire article here: [https://codevoweb.com/setup-golang-gorm-restful-api-project-with-postgres](https://codevoweb.com/setup-golang-gorm-restful-api-project-with-postgres) |
14 | 25 |
|
15 |
| -### 4. Forgot/Reset Passwords in Golang with SMTP HTML Email |
| 26 | +## 2. API with Golang + GORM + PostgreSQL: Access & Refresh Tokens |
16 | 27 |
|
17 |
| -[Forgot/Reset Passwords in Golang with SMTP HTML Email](https://codevoweb.com/forgot-reset-passwords-in-golang-with-html-email) |
| 28 | +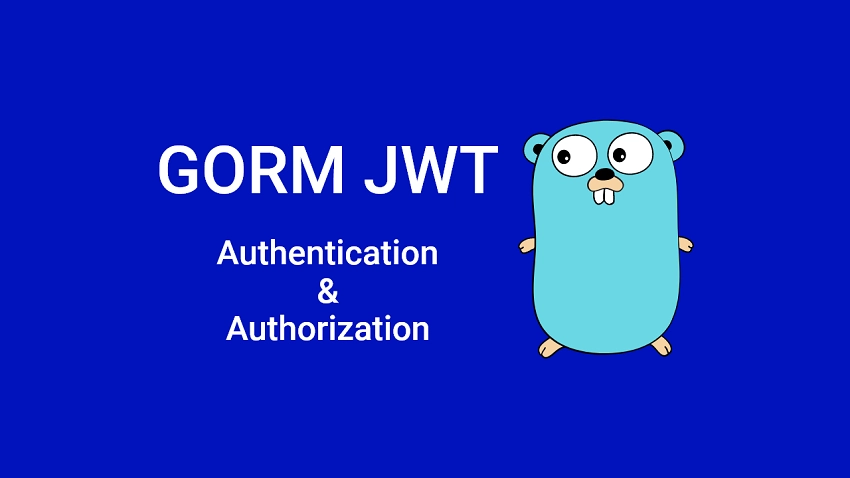 |
18 | 29 |
|
19 |
| -### 5. Build a RESTful CRUD API with Golang |
| 30 | +This article will teach you how to secure a Golang application with access and refresh tokens using GORM, Postgres, Docker, and Docker-compose. Also, you will learn how to use the RS256 algorithm with private and public keys to sign the tokens. |
20 | 31 |
|
21 |
| -[Build a RESTful CRUD API with Golang](https://codevoweb.com/build-restful-crud-api-with-golang) |
| 32 | +### Topics Covered |
| 33 | + |
| 34 | +- Golang, Gin & GORM JWT Authentication Overview |
| 35 | +- JWT Authentication Example with Golang and GORM |
| 36 | +- Generate the Private and Public Keys |
| 37 | +- Load and Validate the Environment Variables |
| 38 | +- Create the Database Models with GORM |
| 39 | +- Run the Database Migration with GORM |
| 40 | +- Hash and Verify the Passwords with Bcrypt |
| 41 | +- Sign and Verify the RS256 JSON Web Tokens |
| 42 | + - Function to Generate the Tokens |
| 43 | + - Function to Verify the Tokens |
| 44 | +- Create the Authentication Route Controllers |
| 45 | + - Register User Controller |
| 46 | + - Login User Controller |
| 47 | + - Refresh Access Token Controller |
| 48 | + - Logout Controller |
| 49 | +- Create a Middleware Guard |
| 50 | +- Create a User Controller |
| 51 | + - Authentication Routes |
| 52 | + - User Routes |
| 53 | +- Add the Routes to the Gin Middleware Stack |
| 54 | + |
| 55 | +Read the entire article here: [https://codevoweb.com/golang-gorm-postgresql-user-registration-with-refresh-tokens](https://codevoweb.com/golang-gorm-postgresql-user-registration-with-refresh-tokens) |
| 56 | + |
| 57 | + |
| 58 | +## 3. Golang and GORM - User Registration and Email Verification |
| 59 | + |
| 60 | +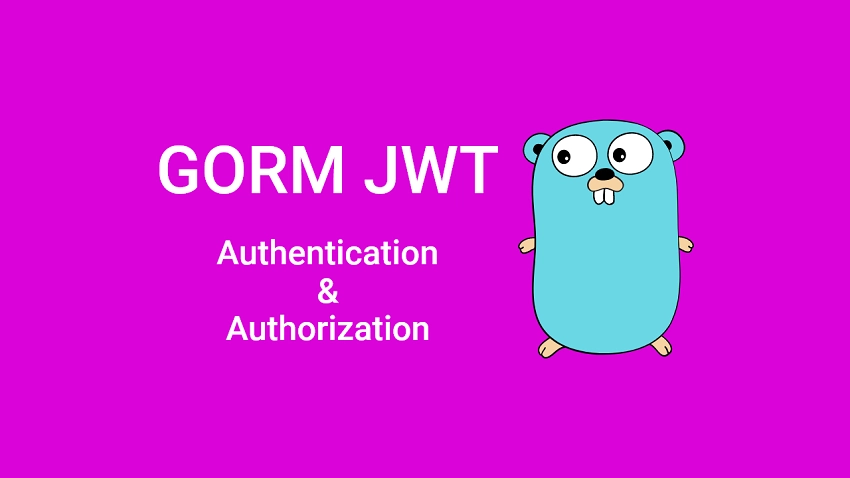 |
| 61 | + |
| 62 | +In this comprehensive guide, you will learn how to secure a Golang RESTful API with JSON Web Tokens and Email verification. We will start by registering the user, verifying the user's email address, logging in the registered user, and logging out the authenticated user. |
| 63 | + |
| 64 | +### Topics Covered |
| 65 | + |
| 66 | +- Golang and GORM JWT Authentication Overview |
| 67 | +- Create the Database Models with GORM |
| 68 | +- Database Migration with GORM |
| 69 | +- Generate and Verify the Password with Bcrypt |
| 70 | +- Sign and Verify the JWT (JSON Web Tokens) |
| 71 | + - Update the Environment Variables File |
| 72 | + - Validate the Variables with Viper |
| 73 | + - Generate the JSON Web Tokens |
| 74 | + - Verify the JSON Web Tokens |
| 75 | +- Create the SMTP Credentials |
| 76 | +- Setup the HTML Templates |
| 77 | + - Add the HTML Email Base Template |
| 78 | + - Add the HTML Email CSS Styles |
| 79 | + - Add the Email Verification Template |
| 80 | + - Create the Email Utility Function |
| 81 | +- Create the Controller Functions |
| 82 | + - Function to Generate the Verification Code |
| 83 | + - User Registration Controller |
| 84 | + - Verify Email Controller |
| 85 | + - Login User Controller |
| 86 | + - Logout User Controller |
| 87 | + - Get User Profile Controller |
| 88 | +- Create the Authentication Guard |
| 89 | +- Create Routes for the Controllers |
| 90 | + - Auth Routes |
| 91 | + - User Routes |
| 92 | +- Register the Routes and Start the Golang Server |
| 93 | + |
| 94 | +Read the entire article here: [https://codevoweb.com/golang-and-gorm-user-registration-email-verification](https://codevoweb.com/golang-and-gorm-user-registration-email-verification) |
| 95 | + |
| 96 | +## 4. Forgot/Reset Passwords in Golang with SMTP HTML Email |
| 97 | + |
| 98 | +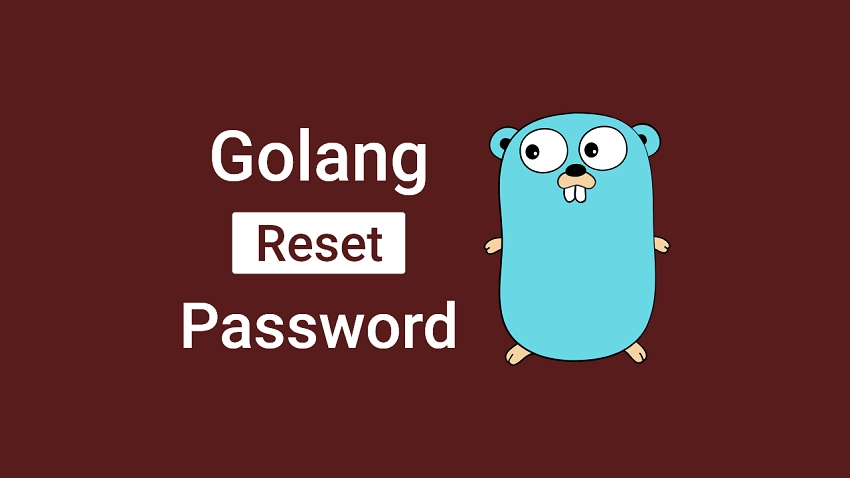 |
| 99 | + |
| 100 | +This article will teach you how to add a secure forgot/reset password feature to a Golang RESTful API application. We will generate the HTML Email templates with the standard Golang template package and send them via SMTP with the Gomail package. |
| 101 | + |
| 102 | +### Topics Covered |
| 103 | + |
| 104 | +- Forgot Password and Password Reset Flow |
| 105 | +- Create the Database Models with GORM |
| 106 | +- Create an SMTP Account |
| 107 | +- Setup the HTML Templates |
| 108 | + - Add the Email Template CSS |
| 109 | + - Add the Password Reset HTML Template |
| 110 | +- Encoding/Decoding the Password Reset Code |
| 111 | +- Create a Utility Function to Send the Emails |
| 112 | +- Add the Forgot Password Route Handler |
| 113 | +- Add the Reset Password Route Handler |
| 114 | +- Add the API Routes to the Gin Middleware Stack |
| 115 | + |
| 116 | +Read the entire article here: [https://codevoweb.com/forgot-reset-passwords-in-golang-with-html-email](https://codevoweb.com/forgot-reset-passwords-in-golang-with-html-email) |
| 117 | + |
| 118 | +## 5. Build a RESTful CRUD API with Golang |
| 119 | + |
| 120 | +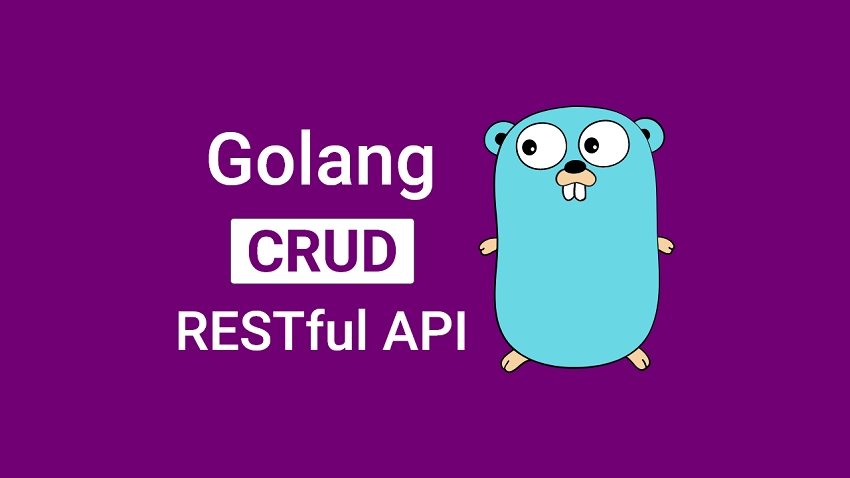 |
| 121 | + |
| 122 | +This article will teach you how to create a CRUD RESTful API in a Golang environment that runs on a Gin Gonic server and uses a PostgreSQL database. We’ll also discuss how you can build models, connect to the running SQL database server, and run database migrations with the GORM library. |
| 123 | + |
| 124 | +### Topics Covered |
| 125 | + |
| 126 | +- What is a REST API |
| 127 | +- What is a REST API |
| 128 | +- What is GORM |
| 129 | +- What is Gin Gonic |
| 130 | +- Create the Database Models with GORM |
| 131 | +- Generating the SQL Table with GORM |
| 132 | +- Creating CRUD Functions in a RESTful API |
| 133 | + - Create a Constructor for the CRUD Operations |
| 134 | + - Create Operation Route Handler |
| 135 | + - Update Operation Route Handler |
| 136 | + - Retrieve a Single Record Route Handler |
| 137 | + - Retrieve All Records Route Handler |
| 138 | + - Delete Operation Route Handler |
| 139 | +- Creating Routes for the CRUD Operations |
| 140 | +- Update/Configure the Golang API Server |
| 141 | +- Testing the Golang CRUD API with Postman |
| 142 | + - Log into the API |
| 143 | + - Creating a New Record |
| 144 | + - Updating the Record |
| 145 | + - Request a Single Record |
| 146 | + - Retrieve all Records with Paginated Results |
| 147 | + - Delete a Record |
| 148 | + |
| 149 | +Read the entire article here: [https://codevoweb.com/build-restful-crud-api-with-golang](https://codevoweb.com/build-restful-crud-api-with-golang) |
0 commit comments