|
| 1 | +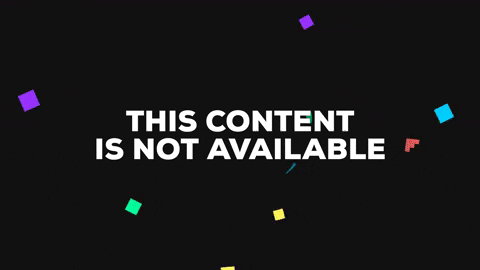 |
| 2 | + |
1 | 3 | # angular-vrviewer
|
| 4 | +Embed VR media in your Angular application. Used as a wrapper for [Google VR view for the Web](https://developers.google.com/vr/concepts/vrview). |
| 5 | +Currently supports only media type of 360 degree images. |
| 6 | + |
| 7 | +## Live Demo |
| 8 | +Visit https://theunreal.github.io/angular-vrview-example/ |
2 | 9 |
|
3 | 10 | ## Installation
|
4 | 11 |
|
5 |
| -To install this library, run: |
| 12 | +To install angular-vrviewer, simply run: |
6 | 13 |
|
7 | 14 | ```bash
|
8 | 15 | $ npm install angular-vrviewer --save
|
9 | 16 | ```
|
10 | 17 |
|
11 |
| -## Consuming your library |
12 |
| - |
13 |
| -Once you have published your library to npm, you can import your library in any Angular application by running: |
14 |
| - |
15 |
| -```bash |
16 |
| -$ npm install angular-vrviewer |
| 18 | +Include in your `index.html`: |
| 19 | +```xml |
| 20 | +<script src="//storage.googleapis.com/vrview/2.0/build/vrview.min.js"></script> |
17 | 21 | ```
|
18 | 22 |
|
19 |
| -and then from your Angular `AppModule`: |
| 23 | +## Usage |
| 24 | + |
| 25 | +Include the `VRViewModule` in your app: |
20 | 26 |
|
21 | 27 | ```typescript
|
22 | 28 | import { BrowserModule } from '@angular/platform-browser';
|
23 | 29 | import { NgModule } from '@angular/core';
|
24 | 30 |
|
25 | 31 | import { AppComponent } from './app.component';
|
26 | 32 |
|
27 |
| -// Import your library |
28 |
| -import { SampleModule } from 'angular-vrviewer'; |
| 33 | +// Add this line |
| 34 | +import { VRViewModule } from 'angular-vrviewer'; |
29 | 35 |
|
30 | 36 | @NgModule({
|
31 | 37 | declarations: [
|
32 | 38 | AppComponent
|
33 | 39 | ],
|
34 | 40 | imports: [
|
35 | 41 | BrowserModule,
|
36 |
| - |
37 |
| - // Specify your library as an import |
38 |
| - LibraryModule |
| 42 | + VRViewModule //<-- import this module |
39 | 43 | ],
|
40 | 44 | providers: [],
|
41 | 45 | bootstrap: [AppComponent]
|
42 | 46 | })
|
43 | 47 | export class AppModule { }
|
44 | 48 | ```
|
| 49 | +Now you can use the `<vrview>` selector to show 360 media in your angular application. |
45 | 50 |
|
46 |
| -Once your library is imported, you can use its components, directives and pipes in your Angular application: |
47 |
| - |
| 51 | +#### Example: |
48 | 52 | ```xml
|
49 |
| -<!-- You can now use your library component in app.component.html --> |
50 |
| -<h1> |
51 |
| - {{title}} |
52 |
| -</h1> |
53 |
| -<sampleComponent></sampleComponent> |
| 53 | +<vrview |
| 54 | +[scenes]="scenes" |
| 55 | +width="100%" |
| 56 | +[height]="400"> |
| 57 | +</vrview> |
54 | 58 | ```
|
55 | 59 |
|
56 |
| -## Development |
57 |
| - |
58 |
| -To generate all `*.js`, `*.d.ts` and `*.metadata.json` files: |
| 60 | +## Scenes and Hotspots |
| 61 | +### Scene Interface: |
| 62 | +```typescript |
| 63 | +export interface Scene { |
| 64 | + [key: string]: { |
| 65 | + image: string; |
| 66 | + hotspots: Hotspot |
| 67 | + } |
| 68 | +} |
| 69 | +``` |
59 | 70 |
|
60 |
| -```bash |
61 |
| -$ npm run build |
| 71 | +### Hotspot interface: |
| 72 | +```typescript |
| 73 | +export interface Hotspot { |
| 74 | + [key: string]: { |
| 75 | + pitch: number; |
| 76 | + yaw: number; |
| 77 | + radius: number, |
| 78 | + distance: number |
| 79 | + } |
| 80 | +} |
62 | 81 | ```
|
63 | 82 |
|
64 |
| -To lint all `*.ts` files: |
| 83 | +### Scenes with Hotspot example: |
65 | 84 |
|
66 |
| -```bash |
67 |
| -$ npm run lint |
| 85 | +```typescript |
| 86 | +scenes: Scene = { |
| 87 | + world: { |
| 88 | + image: 'assets/1.jpg', |
| 89 | + hotspots: { |
| 90 | + green_area: { |
| 91 | + pitch: 10, |
| 92 | + yaw: -15, |
| 93 | + radius: 0.05, |
| 94 | + distance: 1 |
| 95 | + }, |
| 96 | + } |
| 97 | + }, |
| 98 | + green_area: { |
| 99 | + image: 'assets/2.jpg', |
| 100 | + hotspots: { |
| 101 | + world: { |
| 102 | + pitch: 20, |
| 103 | + yaw: 0, |
| 104 | + radius: 0.05, |
| 105 | + distance: 1 |
| 106 | + }, |
| 107 | + } |
| 108 | + } |
| 109 | +}; |
68 | 110 | ```
|
69 | 111 |
|
| 112 | + |
70 | 113 | ## License
|
71 | 114 |
|
72 |
| -MIT © [Eliran Elnasi ](mailto:[email protected]) |
| 115 | +MIT © Eliran Elnasi and contributors |
0 commit comments