diff --git a/README.md b/README.md
index 6d133c0..6ec629a 100644
--- a/README.md
+++ b/README.md
@@ -1,33 +1,187 @@
-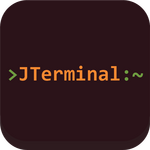
-#
-**JTerminal** is an easy to use library for printing custom outouts on terminal.
+
+
+
JTerminal
+
Is an easy to use library for printing custom outouts on terminal!
+ If you like this project, help me by giving me a star =))<3
+
+
+## What is in V1.0.0
+- Print and println with foreground and background color.
+- Support 8-bit 0-255 XTerm colors.
+- Get colors by name and theire XTerm code.
+- TextEntity class for assign different styles to a single string.
+- clear the terminal.
+- delete lines.
## Hello Terminal
To use JTerminal you just need to make a static call to your desired API.
For example:
-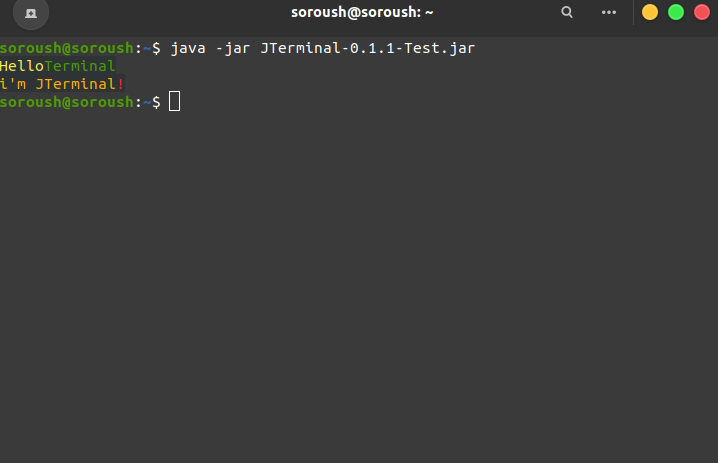
+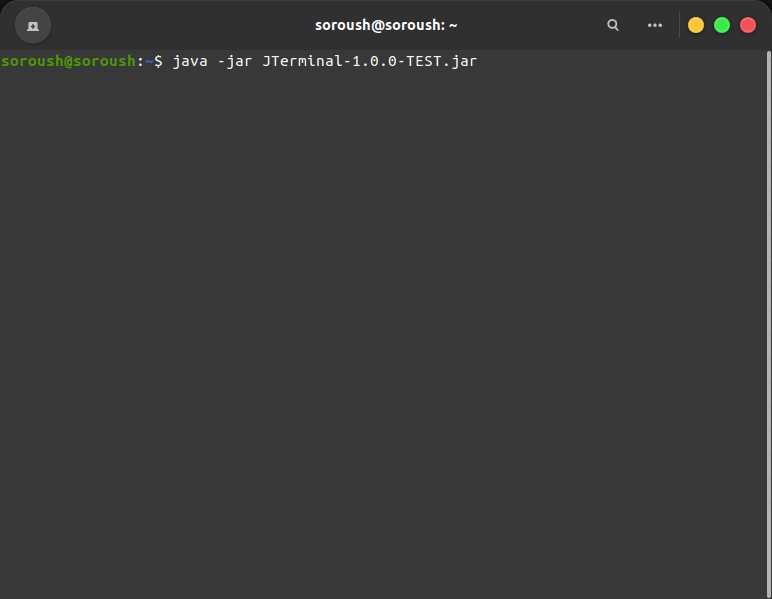
-The above image was the result of running this piece of code:
+The above gif was the result of running this piece of code:
```java
+import java.util.List;
+
import io.github.shuoros.jterminal.JTerminal;
import io.github.shuoros.jterminal.ansi.Color;
+import io.github.shuoros.jterminal.util.TextEntity;
public class Main {
public static void main(String[] args) {
- JTerminal.print("Hello", Color.YELLOW);
- JTerminal.println("Terminal", Color.GREEN);
- JTerminal.print("i'm JTerminal", Color.ORANGE);
- JTerminal.println("!", Color.RED);
+ // Clear screen with timer
+ JTerminal.println("Screen must clear after one second");
+ JTerminal.clear(1000);
+
+ // Delete line
+ JTerminal.println("The line below must be deleted after one second");
+ JTerminal.println("I should be deleted in a second");
+ try {
+ Thread.sleep(1000);
+ } catch (InterruptedException e) {
+ e.printStackTrace();
+ }
+ JTerminal.delete();
+ JTerminal.clear(1000);
+
+ // Print with default foreground and background colors
+ JTerminal.println("Hello I'm in your terminal's default foreground and background color");
+ JTerminal.println("\n");
+
+ // Print with foreground or background color
+ JTerminal.println("I'm an orange text with default background", Color.ORANGE);
+ JTerminal.println("I'm an orange text with white background", Color.ORANGE, Color.WHITE);
+ JTerminal.println("\n");
+
+ // Use custom XTerm code
+ JTerminal.println("I'm printed with 201 XTerm code", Color.xTerm(201));
+ JTerminal.println("\n");
+
+ // Print with TextEntity
+ JTerminal.println("My colors were set with TextEntity", List.of(//
+ new TextEntity(0, 24, Color.BLUE), //
+ new TextEntity(24, 33, Color.YELLOW, Color.RED)));
+ JTerminal.println("\n");
+
+ // All suppoerted XTerm colors (8-bit 0-255)
+ JTerminal.println("All suppoerted XTerm colors (8-bit 0-255) ->", List.of(//
+ new TextEntity(0, 27, Color.WHITE), //
+ new TextEntity(27, 42, Color.RED), //
+ new TextEntity(42, 44, Color.WHITE, Color.ORANGE)));
+ JTerminal.println("");
+ for (Color color : Color.values()) {
+ JTerminal.print(color.toString(), color);
+ JTerminal.print(" ");
+ }
+ JTerminal.println("\n");
+
+ // Credits
+ JTerminal.println(">JTerminal:~ 1.0.0", List.of(//
+ new TextEntity(0, 1, Color.DARK_SEA_GREEN_7), //
+ new TextEntity(1, 10, Color.ORANGE), //
+ new TextEntity(10, 12, Color.DARK_SEA_GREEN_7), //
+ new TextEntity(12, 18, Color.WHITE)));
+ JTerminal.println("By Soroush Shemshadi", Color.ORANGE);
}
}
```
-To see color cheat sheet please check [color docs](https://github.com/shuoros/JTerminal/blob/main/doc/colors)
+#### To see color cheat sheet please check [docs](https://github.com/shuoros/JTerminal/blob/main/doc).
+
+## Installation
+You can use **JTerminal** with any project management tool:
+
+### Maven
+
+```xml
+
+
+ io.github.shuoros
+ JTerminal
+ 1.0.0
+
+```
+
+### Gradle
+
+```gradle
+// https://mvnrepository.com/artifact/io.github.shuoros/JTerminal
+implementation group: 'io.github.shuoros', name: 'JTerminal', version: '1.0.0'
+```
+Or
+
+```gradle
+// https://mvnrepository.com/artifact/io.github.shuoros/JTerminal
+implementation 'io.github.shuoros:JTerminal:1.0.0'
+```
+
+And in **Kotlin**
+
+```gradle
+// https://mvnrepository.com/artifact/io.github.shuoros/JTerminal
+implementation("io.github.shuoros:JTerminal:1.0.0")
+```
+
+### SBT
+
+```sbt
+// https://mvnrepository.com/artifact/io.github.shuoros/JTerminal
+libraryDependencies += "io.github.shuoros" % "JTerminal" % "1.0.0"
+```
+
+### Ivy
+
+```xml
+
+
+```
+
+### Grape
+
+```java
+// https://mvnrepository.com/artifact/io.github.shuoros/JTerminal
+@Grapes(
+ @Grab(group='io.github.shuoros', module='JTerminal', version='1.0.0')
+)
+```
+
+### Leiningen
+
+```clj
+;; https://mvnrepository.com/artifact/io.github.shuoros/JTerminal
+[io.github.shuoros/JTerminal "1.0.0"]
+```
## Authors
JTerminal is developed by [Soroush Shemshadi](https://github.com/shuoros) and [contributors](https://github.com/shuoros/JTerminal/blob/main/CONTRIBUTORS.md).
@@ -43,3 +197,6 @@ If you encounter a bug or vulnerability, please read the [issue policy](https://
## Documentation
To learn how to work with JTerminal, please take a look at the [/doc](https://github.com/shuoros/JTerminal/tree/main/doc) folder.
+
+## Acknowledgement
+A greate thanks to [@sarahrajabi](https://github.com/sarahrajabi) for designing the logo.
diff --git a/RELEASENOTES.md b/RELEASENOTES.md
index 199e2a6..63e5d73 100644
--- a/RELEASENOTES.md
+++ b/RELEASENOTES.md
@@ -2,6 +2,15 @@
## next release
+## 1.0.0 (2021-11-08)
+### New Features
+- Implement clear feature to clean whole terminal.
+- Implement delete feature to delete lines in terminal.
+- Fix default foreground and background color for terminals which not have black background.
+- Add unit tests.
+- Add TextEntity support.
+- Add custom Xterm code support.
+
## 0.1.1 (2021-11-01)
### New Features
- Implement print and println features to print strings on terminal with customizable foreground and background color.
diff --git a/TODO.md b/TODO.md
new file mode 100644
index 0000000..8906f54
--- /dev/null
+++ b/TODO.md
@@ -0,0 +1,5 @@
+# TODO
+
+- Add font support.
+- Add RGB support.
+- Add windows command prompt support.
\ No newline at end of file
diff --git a/doc/APIs/01print.md b/doc/APIs/01print.md
index 09ec437..7525b1c 100644
--- a/doc/APIs/01print.md
+++ b/doc/APIs/01print.md
@@ -1,7 +1,9 @@
# Print And Println
+
To print or println your string on terminal, You can make a static call to JTerminal print and println API.
-## `print(String string)`
-It's print out your string on terminal with JTerminals default foreground and background color.
+
+## `print(String string)
+It's print out your string on terminal with JTerminals default foreground and background color.
```java
import io.github.shuoros.jterminal.JTerminal;
@@ -16,7 +18,6 @@ public class Main {
```
#### Implementation
-First of all, the ANSI code of the default background and foreground color are generate and print out using `AnsiUtils.generateCode`, Therefore, the next string that is sent to the terminal for printing has the desired colors. At the end terminal will be reset using ANSI reset escape code with `AnsiUtils.RESET`.
```java
/**
@@ -27,15 +28,13 @@ First of all, the ANSI code of the default background and foreground color are g
*/
public static void print(String string) {
System.out.print(//
- AnsiUtils.generateCode(//
- Map.of("foreground", defaultForeground, "background", defaultBackground)));
- System.out.print(string);
- System.out.print(AnsiUtils.RESET);
+ AnsiUtils.generateCode(//
+ string, List.of(new TextEntity())));
}
```
## `println(String string)`
-It's print out your string on terminal with end line and JTerminals default foreground and background color.
+It's print out your string on terminal with end line and JTerminals default foreground and background color.
```java
import io.github.shuoros.jterminal.JTerminal;
@@ -50,7 +49,6 @@ public class Main {
```
#### Implementation
-First of all, the ANSI code of the default background and foreground color are generate and print out using `AnsiUtils.generateCode`, Therefore, the next string that is sent to the terminal for printing has the desired colors. At the end terminal will be reset using ANSI reset escape code with `AnsiUtils.RESET` and end line.
```java
/**
@@ -60,16 +58,90 @@ First of all, the ANSI code of the default background and foreground color are g
* @param string : A string you want to print on terminal.
*/
public static void println(String string) {
+ System.out.println(//
+ AnsiUtils.generateCode(//
+ string, List.of(new TextEntity())));
+}
+```
+
+## `print(String string, , List textEnitities)`
+It's print out your string on terminal with your given list of entities.
+
+Read more about [TextEntity](https://github.com/shuoros/JTerminal/blob/main/doc/Utils/00textEntity.md)
+
+```java
+import io.github.shuoros.jterminal.JTerminal;
+
+public class Main {
+
+ public static void main(String[] args) {
+ JTerminal.print("Hello!", List.of(//
+ new TextEntity(Color.ORANGE));
+ }
+
+}
+```
+
+#### Implementation
+
+```java
+/**
+* Prints your given string on terminal with list of your
+* {@link io.github.shuoros.jterminal.util.TextEntity}s.
+*
+* @param string : A string you want to print on termina
+* @param textEnitities : A List of
+* {@link io.github.shuoros.jterminal.util.TextEntity}
+* which each of them can contain setting for a range of
+* your given string.
+*/
+public static void print(String string, List textEnitities) {
System.out.print(//
- AnsiUtils.generateCode(//
- Map.of("foreground", defaultForeground, "background", defaultBackground)));
- System.out.print(string);
- System.out.println(AnsiUtils.RESET);
+ AnsiUtils.generateCode(//
+ string, textEnitities));
+}
+```
+
+## `println(String string, , List textEnitities)`
+It's print out your string on terminal with end line and your given list of entities.
+
+Read more about [TextEntity](https://github.com/shuoros/JTerminal/blob/main/doc/Utils/00textEntity.md)
+
+```java
+import io.github.shuoros.jterminal.JTerminal;
+
+public class Main {
+
+ public static void main(String[] args) {
+ JTerminal.println("Hello!", List.of(//
+ new TextEntity(Color.ORANGE));
+ }
+
+}
+```
+
+#### Implementation
+
+```java
+/**
+* Prints your given string with end line on terminal list of your
+* {@link io.github.shuoros.jterminal.util.TextEntity}s.
+*
+* @param string : A string you want to print on termina
+* @param textEnitities : A List of
+* {@link io.github.shuoros.jterminal.util.TextEntity}
+* which each of them can contain setting for a range of
+* your given string.
+*/
+public static void println(String string, List textEnitities) {
+ System.out.println(//
+ AnsiUtils.generateCode(//
+ string, textEnitities));
}
```
## `print(String string, Color foreground)`
-It's print out your string on terminal with your given foreground color and JTerminals default background color.
+It's print out your string on terminal with your given foreground color and JTerminals default background color.
```java
import io.github.shuoros.jterminal.JTerminal;
@@ -85,7 +157,6 @@ public class Main {
```
#### Implementation
-First of all, the ANSI code of the default background and given foreground color are generate and print out using `AnsiUtils.generateCode`, Therefore, the next string that is sent to the terminal for printing has the desired colors. At the end terminal will be reset using ANSI reset escape code with `AnsiUtils.RESET`.
```java
/**
@@ -98,16 +169,14 @@ First of all, the ANSI code of the default background and given foreground color
* want print your string whit it on terminal.
*/
public static void print(String string, Color foreground) {
- System.out.print(//
- AnsiUtils.generateCode(//
- Map.of("foreground", foreground, "background", defaultBackground)));
- System.out.print(string);
- System.out.print(AnsiUtils.RESET);
+ System.out.print(//
+ AnsiUtils.generateCode(//
+ string, List.of(new TextEntity(foreground))));
}
```
## `println(String string, Color foreground)`
-It's print out your string on terminal with end line and your given foreground color and JTerminals default background color.
+It's print out your string on terminal with end line and your given foreground color and JTerminals default background color.
```java
import io.github.shuoros.jterminal.JTerminal;
@@ -123,7 +192,6 @@ public class Main {
```
#### Implementation
-First of all, the ANSI code of the default background and given foreground color are generate and print out using `AnsiUtils.generateCode`, Therefore, the next string that is sent to the terminal for printing has the desired colors. At the end terminal will be reset using ANSI reset escape code with `AnsiUtils.RESET` and end line.
```java
/**
@@ -136,16 +204,14 @@ First of all, the ANSI code of the default background and given foreground color
* want print your string whit it on terminal.
*/
public static void println(String string, Color foreground) {
- System.out.print(//
- AnsiUtils.generateCode(//
- Map.of("foreground", foreground, "background", defaultBackground)));
- System.out.print(string);
- System.out.println(AnsiUtils.RESET);
+ System.out.println(//
+ AnsiUtils.generateCode(//
+ string, List.of(new TextEntity(foreground))));
}
```
## `print(String string, Color foreground, Color background)`
-It's print out your string on terminal with your given foreground and background color.
+It's print out your string on terminal with your given foreground and background color.
```java
import io.github.shuoros.jterminal.JTerminal;
@@ -161,7 +227,6 @@ public class Main {
```
#### Implementation
-First of all, the ANSI code of given foreground and background color are generate and print out using `AnsiUtils.generateCode`, Therefore, the next string that is sent to the terminal for printing has the desired colors. At the end terminal will be reset using ANSI reset escape code with `AnsiUtils.RESET`.
```java
/**
@@ -179,15 +244,13 @@ First of all, the ANSI code of given foreground and background color are generat
*/
public static void print(String string, Color foreground, Color background) {
System.out.print(//
- AnsiUtils.generateCode(//
- Map.of("foreground", foreground, "background", background)));
- System.out.print(string);
- System.out.print(AnsiUtils.RESET);
+ AnsiUtils.generateCode(//
+ string, List.of(new TextEntity(foreground, background))));
}
```
## `println(String string, Color foreground, Color background)`
-It's print out your string on terminal with end line and your given foreground and background color.
+It's print out your string on terminal with end line and your given foreground and background color.
```java
import io.github.shuoros.jterminal.JTerminal;
@@ -203,7 +266,6 @@ public class Main {
```
#### Implementation
-First of all, the ANSI code of given foreground and background color are generate and print out using `AnsiUtils.generateCode`, Therefore, the next string that is sent to the terminal for printing has the desired colors. At the end terminal will be reset using ANSI reset escape code with `AnsiUtils.RESET` and end line.
```java
/**
@@ -220,10 +282,8 @@ First of all, the ANSI code of given foreground and background color are generat
* terminal.
*/
public static void println(String string, Color foreground, Color background) {
- System.out.print(//
- AnsiUtils.generateCode(//
- Map.of("foreground", foreground, "background", background)));
- System.out.print(string);
- System.out.println(AnsiUtils.RESET);
+ System.out.println(//
+ AnsiUtils.generateCode(//
+ string, List.of(new TextEntity(foreground, background))));
}
```
diff --git a/doc/APIs/02clear.md b/doc/APIs/02clear.md
new file mode 100644
index 0000000..ab75682
--- /dev/null
+++ b/doc/APIs/02clear.md
@@ -0,0 +1,64 @@
+# Clear
+
+To clear terminal, You can make a static call to JTerminal clear API.
+
+## `clear()`
+It's clears whole terminal.
+
+```java
+import io.github.shuoros.jterminal.JTerminal;
+
+public class Main {
+
+ public static void main(String[] args) {
+ JTerminal.println("Screen must clear");
+ JTerminal.clear();
+ }
+
+}
+```
+
+#### Implementation
+
+```java
+/**
+* Clear the terminal.
+*/
+public static void clear() {
+ System.out.print("\033\143");
+}
+```
+
+## `clear(long sleep)`
+It's clears whole terminal after sleep in `long sleep` seconds.
+
+```java
+import io.github.shuoros.jterminal.JTerminal;
+
+public class Main {
+
+ public static void main(String[] args) {
+ JTerminal.println("Screen must clear after one second");
+ JTerminal.clear(1000);
+ }
+
+}
+```
+
+#### Implementation
+
+```java
+/**
+* Call the {@code clear()} after sleep for {@code sleep} milliseconds.
+*
+* @param sleep : Sleep time in milliseconds.
+*/
+public static void clear(long sleep) {
+ try {
+ Thread.sleep(sleep);
+ } catch (InterruptedException e) {
+ e.printStackTrace();
+ }
+ clear();
+}
+```
\ No newline at end of file
diff --git a/doc/APIs/03delete.md b/doc/APIs/03delete.md
new file mode 100644
index 0000000..5e28823
--- /dev/null
+++ b/doc/APIs/03delete.md
@@ -0,0 +1,71 @@
+# Delete
+
+To delete line or lines in terminal, You can make a static call to JTerminal delete API.
+
+## `delete()`
+It deletes one line before cursor.
+
+```java
+import io.github.shuoros.jterminal.JTerminal;
+
+public class Main {
+
+ public static void main(String[] args) {
+ JTerminal.println("The line below must be deleted after one second");
+ JTerminal.println("I should be deleted in a second");
+ try {
+ Thread.sleep(1000);
+ } catch (InterruptedException e) {
+ e.printStackTrace();
+ }
+ JTerminal.delete();
+ }
+
+}
+```
+
+#### Implementation
+
+```java
+/**
+* Deletes the last line befour cursor.
+*/
+public static void delete() {
+ int line = 1;
+ System.out.print(String.format("\033[%dA", line)); // Move up
+ System.out.print("\033[2K"); // Erase line content
+}
+```
+
+## `delete(int line)`
+it deletes `int line` lines before cursor.
+
+public class Main {
+
+ public static void main(String[] args) {
+ JTerminal.println("The line below and I must be deleted after one second");
+ JTerminal.println("I should be deleted in a second");
+ try {
+ Thread.sleep(1000);
+ } catch (InterruptedException e) {
+ e.printStackTrace();
+ }
+ JTerminal.delete(2);
+ }
+
+}
+```
+
+#### Implementation
+
+```java
+/**
+* Delete last given {@code line}s befour cursor.
+*
+* @param line : Lines number you want to delete.
+*/
+public static void delete(int line) {
+ System.out.print(String.format("\033[%dA", line)); // Move up
+ System.out.print("\033[2K"); // Erase line content
+}
+```
\ No newline at end of file
diff --git a/doc/APIs/README.md b/doc/APIs/README.md
index a599841..b5376a7 100644
--- a/doc/APIs/README.md
+++ b/doc/APIs/README.md
@@ -2,3 +2,5 @@
- [defaults](https://github.com/shuoros/JTerminal/blob/main/doc/APIs/00defaults.md)
- [print and println](https://github.com/shuoros/JTerminal/blob/main/doc/APIs/01print.md)
+- [clear](https://github.com/shuoros/JTerminal/blob/main/doc/APIs/02clear.md)
+- [delete](https://github.com/shuoros/JTerminal/blob/main/doc/APIs/03delete.md)
diff --git a/doc/Colors/00cheatsheet.md b/doc/Colors/00cheatsheet.md
index df291a3..9e7c28e 100644
--- a/doc/Colors/00cheatsheet.md
+++ b/doc/Colors/00cheatsheet.md
@@ -264,3 +264,5 @@ For each color you can use its "Enum Name" like `Color.*`
255
Grey93
#eeeeee
rgb(238,238,238)
hsl(0,0%,93%)
+
+Table credit by @jonasjacek
\ No newline at end of file
diff --git a/doc/Colors/01xterm.md b/doc/Colors/01xterm.md
new file mode 100644
index 0000000..69f6847
--- /dev/null
+++ b/doc/Colors/01xterm.md
@@ -0,0 +1,18 @@
+# Xterm Colors
+
+To use colors by theire XTerm code you can call `xterm()` method in Color and give it XTerm code of color you want:
+
+```java
+import io.github.shuoros.jterminal.JTerminal;
+import io.github.shuoros.jterminal.ansi.Color;
+
+public class Main {
+
+ public static void main(String[] args) {
+ JTerminal.print("Hello!", Color.xTerm(214);
+ }
+
+}
+```
+
+**The printed "Hello!" will have orange foreground.**
\ No newline at end of file
diff --git a/doc/Colors/xterm.md b/doc/Colors/xterm.md
deleted file mode 100644
index b85fe3f..0000000
--- a/doc/Colors/xterm.md
+++ /dev/null
@@ -1 +0,0 @@
-# Xterm Colors
\ No newline at end of file
diff --git a/doc/README.md b/doc/README.md
index c4ef998..a199274 100644
--- a/doc/README.md
+++ b/doc/README.md
@@ -3,4 +3,5 @@
## Table of content
- [APIs](https://github.com/shuoros/JTerminal/tree/main/doc/APIs)
-- [Colors](https://github.com/shuoros/JTerminal/tree/main/doc/Colors)
\ No newline at end of file
+- [Colors](https://github.com/shuoros/JTerminal/tree/main/doc/Colors)
+- [Utils](https://github.com/shuoros/JTerminal/tree/main/doc/Utils)
\ No newline at end of file
diff --git a/doc/Utils/00textEntity.md b/doc/Utils/00textEntity.md
new file mode 100644
index 0000000..5dd03be
--- /dev/null
+++ b/doc/Utils/00textEntity.md
@@ -0,0 +1,29 @@
+# TextEntity
+
+TextEntity is a model to represent entity of a given text to generate ANSI code based on that entities.
+
+In TextEntity you can define the range of your entity on text and then set the foreground and background color for the selected range.
+
+Example:
+
+```java
+import java.util.List;
+
+import io.github.shuoros.jterminal.JTerminal;
+import io.github.shuoros.jterminal.ansi.Color;
+import io.github.shuoros.jterminal.util.TextEntity;
+
+public class Main {
+
+ public static void main(String[] args) {
+ JTerminal.println(">JTerminal:~ 1.0.0", List.of(//
+ new TextEntity(0, 1, Color.DARK_SEA_GREEN_7), //
+ new TextEntity(1, 10, Color.ORANGE), //
+ new TextEntity(10, 12, Color.DARK_SEA_GREEN_7), //
+ new TextEntity(12, 18, Color.WHITE)));
+ }
+
+}
+```
+
+**Please consider that ranges must not overlap and for now give your list of entities in order of ranges and you must cover all of your string with TextEnity/TextEnities(0 -> text.length()). this shortcomes (ranges order and full coverage) will be solve in next versions.**
\ No newline at end of file
diff --git a/doc/Utils/README.md b/doc/Utils/README.md
new file mode 100644
index 0000000..7cbc766
--- /dev/null
+++ b/doc/Utils/README.md
@@ -0,0 +1,3 @@
+# Documentation > Utils
+
+- [TextEntity](https://github.com/shuoros/JTerminal/blob/main/doc/Utils/00textEntity.md)
\ No newline at end of file
diff --git a/javadoc/allclasses-index.html b/javadoc/allclasses-index.html
index 3953aa0..cc8638c 100644
--- a/javadoc/allclasses-index.html
+++ b/javadoc/allclasses-index.html
@@ -2,10 +2,10 @@
-
+
All Classes (JTerminal 0.0.1-SNAPSHOT API)
-
+
@@ -27,8 +27,8 @@
catch(err) {
}
//-->
-var data = {"i0":2,"i1":4,"i2":4,"i3":2,"i4":2};
-var tabs = {65535:["t0","All Classes"],2:["t2","Class Summary"],4:["t3","Enum Summary"]};
+var data = {"i0":2,"i1":4,"i2":4,"i3":8,"i4":8,"i5":2,"i6":2,"i7":2};
+var tabs = {65535:["t0","All Classes"],2:["t2","Class Summary"],4:["t3","Enum Summary"],8:["t4","Exception Summary"]};
var altColor = "altColor";
var rowColor = "rowColor";
var tableTab = "tableTab";
@@ -106,7 +106,7 @@