We read every piece of feedback, and take your input very seriously.
To see all available qualifiers, see our documentation.
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
MongoDB 6.0.4 Community Edition
main.go
package main import ( "context" "fmt" "time" "github.com/qiniu/qmgo" "go.mongodb.org/mongo-driver/bson" "go.mongodb.org/mongo-driver/bson/primitive" "go.mongodb.org/mongo-driver/mongo" "go.mongodb.org/mongo-driver/mongo/options" ) type User struct { Id primitive.ObjectID `bson:"_id,omitempty"` Identity string `bson:"Identity,omitempty"` Name string `bson:"Name,omitempty"` Age int `bson:"Age,omitempty"` } type Log struct { Id primitive.ObjectID `bson:"_id,omitempty"` CreatedAt time.Time `bson:"CreatedAt,omitempty"` CreatedBy string `bson:"CreatedBy,omitempty"` ModelValue interface{} `bson:"ModelValue,omitempty"` } func main() { results := []*Log{} user := new(User) user.Id = primitive.NewObjectID() user.Identity = "E00000001" user.Name = "test user" log := new(Log) log.CreatedAt = time.Now() log.ModelValue = user log.CreatedBy = "E00000SYS" ctx := context.Background() client, err := qmgo.NewClient(ctx, &qmgo.Config{Uri: "mongodb://localhost:27018"}) if err != nil { fmt.Println("Create client failed: ", err) } collection := client.Database("mongo_test").Collection("Log") _, err = collection.InsertOne(ctx, log) if err != nil { fmt.Printf("Create document with error: %v\n", err) } collection.Find(context.Background(), bson.M{}).All(&results) for _, v := range results { fmt.Println(v.ModelValue) } }
Document in mongo db:
Query ModelValue field result:
Q: 怎样ModelValue可以返回bson.M (map[string]interface{})而不是bson.D ([]bson.E)?
谢谢!
The text was updated successfully, but these errors were encountered:
这个是mongodb官方行为,qmgo暂时不会改变这个行为
Sorry, something went wrong.
No branches or pull requests
MongoDB 6.0.4 Community Edition
main.go
Document in mongo db:
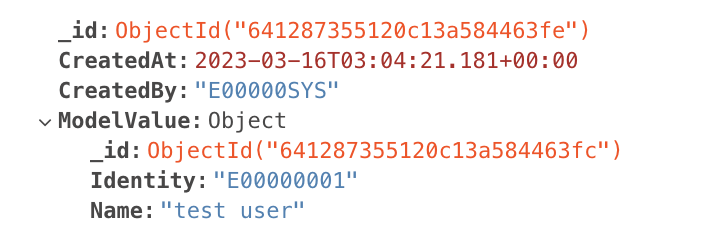
Query ModelValue field result:

Q:
怎样ModelValue可以返回bson.M (map[string]interface{})而不是bson.D ([]bson.E)?
谢谢!
The text was updated successfully, but these errors were encountered: