|
| 1 | +3111\. Minimum Rectangles to Cover Points |
| 2 | + |
| 3 | +Medium |
| 4 | + |
| 5 | +You are given a 2D integer array `points`, where <code>points[i] = [x<sub>i</sub>, y<sub>i</sub>]</code>. You are also given an integer `w`. Your task is to **cover** **all** the given points with rectangles. |
| 6 | + |
| 7 | +Each rectangle has its lower end at some point <code>(x<sub>1</sub>, 0)</code> and its upper end at some point <code>(x<sub>2</sub>, y<sub>2</sub>)</code>, where <code>x<sub>1</sub> <= x<sub>2</sub></code>, <code>y<sub>2</sub> >= 0</code>, and the condition <code>x<sub>2</sub> - x<sub>1</sub> <= w</code> **must** be satisfied for each rectangle. |
| 8 | + |
| 9 | +A point is considered covered by a rectangle if it lies within or on the boundary of the rectangle. |
| 10 | + |
| 11 | +Return an integer denoting the **minimum** number of rectangles needed so that each point is covered by **at least one** rectangle_._ |
| 12 | + |
| 13 | +**Note:** A point may be covered by more than one rectangle. |
| 14 | + |
| 15 | +**Example 1:** |
| 16 | + |
| 17 | +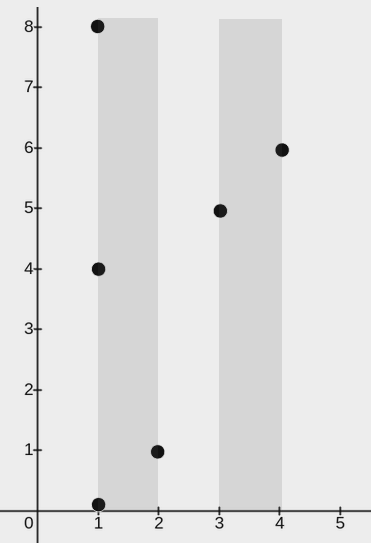 |
| 18 | + |
| 19 | +**Input:** points = [[2,1],[1,0],[1,4],[1,8],[3,5],[4,6]], w = 1 |
| 20 | + |
| 21 | +**Output:** 2 |
| 22 | + |
| 23 | +**Explanation:** |
| 24 | + |
| 25 | +The image above shows one possible placement of rectangles to cover the points: |
| 26 | + |
| 27 | +* A rectangle with a lower end at `(1, 0)` and its upper end at `(2, 8)` |
| 28 | +* A rectangle with a lower end at `(3, 0)` and its upper end at `(4, 8)` |
| 29 | + |
| 30 | +**Example 2:** |
| 31 | + |
| 32 | +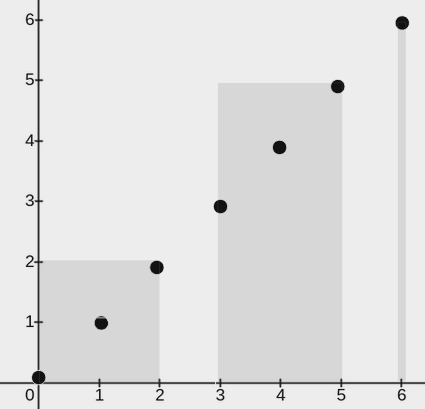 |
| 33 | + |
| 34 | +**Input:** points = [[0,0],[1,1],[2,2],[3,3],[4,4],[5,5],[6,6]], w = 2 |
| 35 | + |
| 36 | +**Output:** 3 |
| 37 | + |
| 38 | +**Explanation:** |
| 39 | + |
| 40 | +The image above shows one possible placement of rectangles to cover the points: |
| 41 | + |
| 42 | +* A rectangle with a lower end at `(0, 0)` and its upper end at `(2, 2)` |
| 43 | +* A rectangle with a lower end at `(3, 0)` and its upper end at `(5, 5)` |
| 44 | +* A rectangle with a lower end at `(6, 0)` and its upper end at `(6, 6)` |
| 45 | + |
| 46 | +**Example 3:** |
| 47 | + |
| 48 | +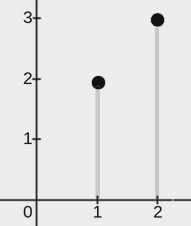 |
| 49 | + |
| 50 | +**Input:** points = [[2,3],[1,2]], w = 0 |
| 51 | + |
| 52 | +**Output:** 2 |
| 53 | + |
| 54 | +**Explanation:** |
| 55 | + |
| 56 | +The image above shows one possible placement of rectangles to cover the points: |
| 57 | + |
| 58 | +* A rectangle with a lower end at `(1, 0)` and its upper end at `(1, 2)` |
| 59 | +* A rectangle with a lower end at `(2, 0)` and its upper end at `(2, 3)` |
| 60 | + |
| 61 | +**Constraints:** |
| 62 | + |
| 63 | +* <code>1 <= points.length <= 10<sup>5</sup></code> |
| 64 | +* `points[i].length == 2` |
| 65 | +* <code>0 <= x<sub>i</sub> == points[i][0] <= 10<sup>9</sup></code> |
| 66 | +* <code>0 <= y<sub>i</sub> == points[i][1] <= 10<sup>9</sup></code> |
| 67 | +* <code>0 <= w <= 10<sup>9</sup></code> |
| 68 | +* All pairs <code>(x<sub>i</sub>, y<sub>i</sub>)</code> are distinct. |
0 commit comments