diff --git a/README.md b/README.md
index af7ff97..eeb9b91 100644
--- a/README.md
+++ b/README.md
@@ -1,6 +1,7 @@
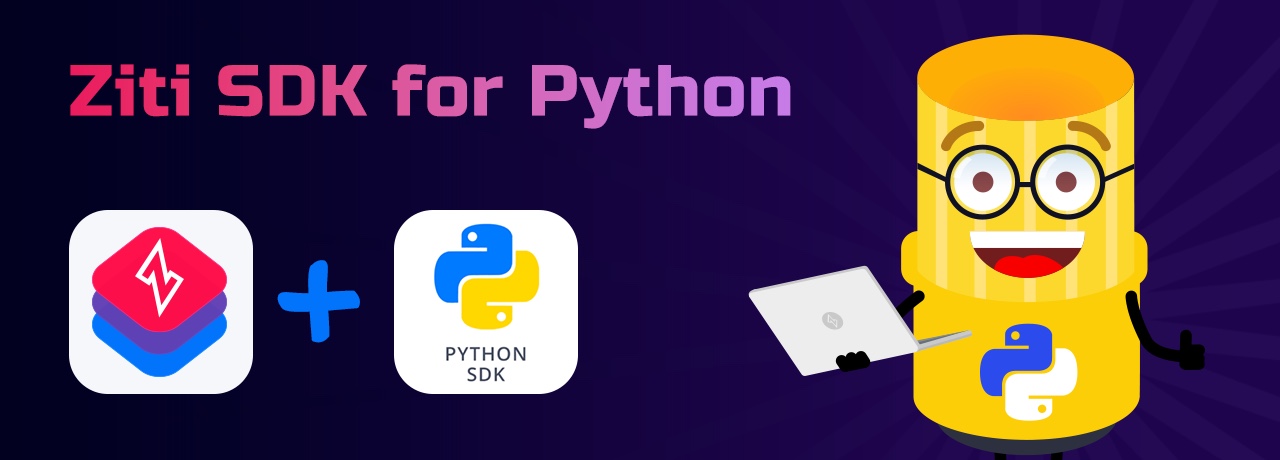
# Python SDK for OpenZiti
+
@@ -34,11 +35,13 @@ OpenZiti is an open-source project that provides secure, zero-trust networking f
More specifically, the SDK allows you to integrate zero trust at the application level. This means your data is never
exposed outside the application environment providing you with end-to-end encryption for ultimate security. See other
zero trust models [here](https://docs.openziti.io/docs/learn/core-concepts/zero-trust-models/overview).
+
## Getting Started
+
If you don't already have an OpenZiti network running, you can follow our [express install guides](https://docs.openziti.io/docs/learn/quickstarts/network/)
to set up the network that fits your needs. Or, you can try [CloudZiti](https://netfoundry.io/pricing/) for free, check out more [here](https://docs.openziti.io/).
@@ -52,6 +55,7 @@ pip install openziti
```
### Using Ziti Python SDK
+
With just two lines of code, you can turn your plain old web server into a secure, zero-trust embedded application.
Below is an example of just how simple it is to get started.
@@ -87,7 +91,9 @@ know what a monkey patch is! However, if you're interested in what a monkey patc
cfg = dict(ztx=openziti.load('/path/to/identity.json'), service="name-of-ziti-service")
openziti.monkeypatch(bindings={('127.0.0.1', 8000): cfg})
```
+
Or try our decorator pattern with a function annotation
+
```python
@openziti.zitify(bindings={('127.0.0.1', 18080): {'ztx': '/path/to/identity.json', 'service': 'name-of-ziti-service'}})
def yourFunction():
@@ -95,35 +101,46 @@ def yourFunction():
The `binding` dictionary configures what happens when the code tries to open a server socket. Standard network addresses
are mapped to ziti service configurations. For example, with his configuration
+
```python
bindings = {
('0.0.0.0', 8080): { 'ztx': 'my-identity.json', 'service':'my-service' }
}
```
+
when application opens a server socket and binds to address `0.0.0.0:8080` it will actually bind to the ziti service named `my-service`.
Binding addresses can be specified with tuples, strings, or ints(ports). `('0.0.0.0', 8080)`, `'0.0.0.0:8080'`, `':8080'`, `8080`
are all considered and treated the same.
## Examples
+
Try it out yourself with one of our [examples](sample%2FREADME.md)
+
* [Flazk](sample/flask-of-ziti)
* [Echo Server](sample/ziti-echo-server)
* [HTTP Server](sample/ziti-http-server)
* [Ziti Requests](sample/ziti-requests)
* [Ziti Socket](sample/ziti-socket)
* [Ziti urllib3](sample/ziti-urllib3)
+* [S3 Log Uploader (boto3)](sample/s3z)
## Support
+
### Looking for Help?
+
Please use these community resources for getting help. We use GitHub [issues](https://github.com/openziti/ziti-sdk-py/issues)
for tracking bugs and feature requests and have limited bandwidth to address them.
- Read the [offical docs](https://docs.openziti.io/docs/learn/introduction/)
- Join our [Developer Community](https://openziti.org)
- Participate in discussion on [Discourse](https://openziti.discourse.group/)
+
## Contributing
+
Do you want to get your hands dirty and help make OpenZiti better? Contribute to the OpenZiti open-source project
through bug reports, bug fixes, documentation, etc. Check out our guide on contributing to our projects [here](https://docs.openziti.io/policies/CONTRIBUTING.html).
+
## License
+
[Apache 2.0](./LICENSE)
diff --git a/sample/README.md b/sample/README.md
index 99f4576..3da2c48 100644
--- a/sample/README.md
+++ b/sample/README.md
@@ -2,12 +2,12 @@
## Setup
-In order to begin using the examples, you'll need an OpenZiti network. If you don't already have one running, you can follow our [express install guides](https://docs.openziti.io/docs/learn/quickstarts/network/)
+You will need an OpenZiti network to use the examples. If you don't already have one running, you can follow our [express install guides](https://docs.openziti.io/docs/learn/quickstarts/network/)
to set up the network that fits your needs. You could also use [ZEDS](https://zeds.openziti.org) (Ziti Edge Developer Sandbox) or, you can try cloud Ziti for free, check out more [here](https://docs.openziti.io/).
### Installing the SDK
-The Python SDK for OpenZiti is distributed via the Python Package Index (PyPI) and can be installed using
+The Python SDK for OpenZiti is distributed via the Python Package Index (PyPI) and can be installed using
[`pip`](https://pypi.org/project/openziti/) package manager.
```shell
@@ -15,25 +15,31 @@ pip install openziti
```
### Install Python Requirements
+
First, you'll need the dependent libraries used in the examples.
+
```bash
cd ./sample
pip install -r requirements
```
### Get and Enroll an Identity
+
You need an [identity](https://docs.openziti.io/docs/learn/core-concepts/identities/overview) to be used by the example
application. If using [ZEDS](https://zeds.openziti.org) you can grab one from there, otherwise you can find all the
information you need for creating and enrolling an identity in the [doc here](https://docs.openziti.io/docs/learn/core-concepts/identities/overview#creating-an-identity).
Alternatively, if you have an identity enrollment token (JWT file), you can perform the enrollment with the Python SDK.
+
```bash
python -m openziti enroll --jwt= --identity=
```
### Environment
+
The `ZITI_IDENTITIES` environment variable can be used to store the paths to any identity files you have. If you have
more than one identity file, you can use the `;` operator as a delimiter to provide additional identities.
+
```bash
export ZITI_IDENTITIES=
```
@@ -42,6 +48,7 @@ There is an optional environment variable `ZITI_LOG` which, by default is set to
output more or less log information. A `ZITI_LOG` level of `6` will output `TRACE` level logs.
### Network
+
Your network overlay needs to have a [Service](https://docs.openziti.io/docs/learn/core-concepts/services/overview),
and the proper [Service Configurations](https://docs.openziti.io/docs/learn/core-concepts/config-store/overview), the
documentation for which is linked.
@@ -50,28 +57,39 @@ If you happen to be using [ZEDS](https://zeds.openziti.org) you are in luck, the
that are already implemented in the developer sandbox.
## Examples
+
> **Note**
> All but the Flazk example scripts use predefined services in [ZEDS](https://zeds.openziti.org) by default.
### [Flazk](flask-of-ziti)
+
An example showing the simplicity in integrating zero trust into a web server or API using Flask. This example also
shows how to use the decorator to apply the monkeypatch.
`flask-of-ziti/helloFlazk.py`
### [Ziti Echo Server](ziti-echo-server)
+
An example showing how to open a socket to listen on the network overlay for a particular service and send all bytes
received back to the sender.
### [Ziti HTTP Server](ziti-http-server)
+
An example showing how to monkeypatch `http.server` to listen for HTTP requests on the network overlay. When a request
is captured, a response with a simple JSON document is sent to clients.
### [Ziti Requests](ziti-requests)
+
An example showing the use of Ziti monkey patching a standard socket, via the requests module, to intercept network
connections using Ziti overlay.
### [Ziti Socket](ziti-socket)
+
An example showing the use of a _raw_ Ziti socket.
### [Ziti urllib3](ziti-urllib3)
+
An example showing how to monkeypatch `urllib3` to fetch a Ziti service using HTTP.
+
+### [S3 Log Uploader](sample/s3z)
+
+Upload some log files to a private S3 bucket via the Ziti with the boto3 SDK.