Commit c738d70
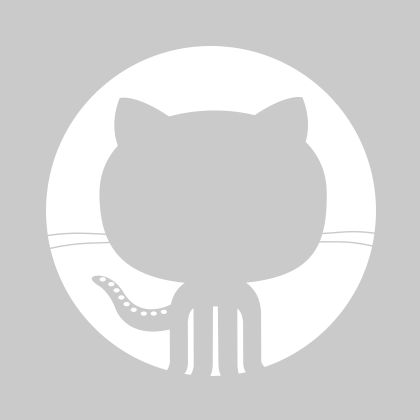
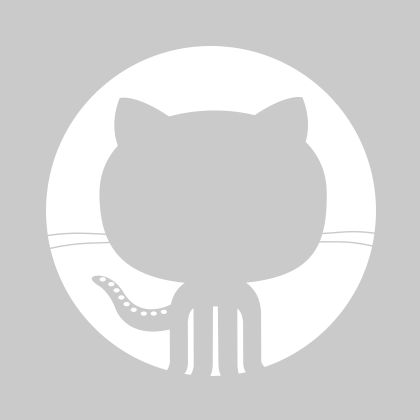
Alec Cunningham
Alec Cunningham
1 parent b5a5350 commit c738d70
File tree
9 files changed
+68
-28
lines changed- cmd/server
- docs
- pkg
- api
- auth
- worker
- tests/fixtures/api/workflow-[id]-stats
9 files changed
+68
-28
lines changedLines changed: 1 addition & 1 deletion
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
41 | 41 |
| |
42 | 42 |
| |
43 | 43 |
| |
44 |
| - | |
| 44 | + | |
45 | 45 |
| |
46 | 46 |
| |
47 | 47 |
| |
|
Lines changed: 5 additions & 5 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
73 | 73 |
| |
74 | 74 |
| |
75 | 75 |
| |
76 |
| - | |
77 |
| - | |
78 |
| - | |
79 |
| - | |
80 |
| - | |
| 76 | + | |
| 77 | + | |
| 78 | + | |
| 79 | + | |
| 80 | + | |
81 | 81 |
| |
82 | 82 |
| |
83 | 83 |
| |
|
Lines changed: 29 additions & 13 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 |
| - | |
2 |
| - | |
3 | 1 |
| |
4 | 2 |
| |
5 | 3 |
| |
| |||
80 | 78 |
| |
81 | 79 |
| |
82 | 80 |
| |
83 |
| - | |
| 81 | + | |
84 | 82 |
| |
85 | 83 |
| |
86 | 84 |
| |
| 85 | + | |
| 86 | + | |
| 87 | + | |
87 | 88 |
| |
88 | 89 |
| |
89 | 90 |
| |
90 | 91 |
| |
91 | 92 |
| |
92 | 93 |
| |
93 | 94 |
| |
| 95 | + | |
| 96 | + | |
| 97 | + | |
| 98 | + | |
| 99 | + | |
| 100 | + | |
94 | 101 |
| |
95 | 102 |
| |
96 | 103 |
| |
97 | 104 |
| |
98 |
| - | |
| 105 | + | |
99 | 106 |
| |
100 | 107 |
| |
101 | 108 |
| |
| 109 | + | |
| 110 | + | |
| 111 | + | |
102 | 112 |
| |
103 | 113 |
| |
104 | 114 |
| |
105 | 115 |
| |
106 |
| - | |
107 |
| - | |
108 |
| - | |
109 |
| - | |
110 |
| - | |
111 |
| - | |
112 |
| - | |
113 |
| - | |
114 |
| - | |
| 116 | + | |
| 117 | + | |
| 118 | + | |
| 119 | + | |
| 120 | + | |
| 121 | + | |
| 122 | + | |
| 123 | + | |
| 124 | + | |
| 125 | + | |
| 126 | + | |
| 127 | + | |
| 128 | + | |
| 129 | + | |
| 130 | + | |
115 | 131 |
| |
116 | 132 |
|
Lines changed: 11 additions & 1 deletion
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
2 | 2 |
| |
3 |
| - | |
| 3 | + | |
| 4 | + | |
| 5 | + | |
| 6 | + | |
| 7 | + | |
| 8 | + | |
| 9 | + | |
| 10 | + | |
| 11 | + | |
| 12 | + | |
| 13 | + |
Lines changed: 3 additions & 0 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
12 | 12 |
| |
13 | 13 |
| |
14 | 14 |
| |
| 15 | + | |
| 16 | + | |
| 17 | + | |
15 | 18 |
| |
16 | 19 |
| |
17 | 20 |
| |
|
Lines changed: 0 additions & 7 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
9 | 9 |
| |
10 | 10 |
| |
11 | 11 |
| |
12 |
| - | |
13 | 12 |
| |
14 | 13 |
| |
15 | 14 |
| |
| |||
54 | 53 |
| |
55 | 54 |
| |
56 | 55 |
| |
57 |
| - | |
58 |
| - | |
59 |
| - | |
60 |
| - | |
61 |
| - | |
62 |
| - | |
63 | 56 |
| |
64 | 57 |
| |
65 | 58 |
| |
|
Lines changed: 1 addition & 1 deletion
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
69 | 69 |
| |
70 | 70 |
| |
71 | 71 |
| |
72 |
| - | |
| 72 | + | |
73 | 73 |
| |
74 | 74 |
| |
75 | 75 |
| |
|
Lines changed: 1 addition & 0 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
| 1 | + |
Lines changed: 17 additions & 0 deletions
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
| 1 | + | |
| 2 | + | |
| 3 | + | |
| 4 | + | |
| 5 | + | |
| 6 | + | |
| 7 | + | |
| 8 | + | |
| 9 | + | |
| 10 | + | |
| 11 | + | |
| 12 | + | |
| 13 | + | |
| 14 | + | |
| 15 | + | |
| 16 | + | |
| 17 | + |
0 commit comments