diff --git a/docs-util/packages/typedoc-config/extended-tsconfig/workflows.json b/docs-util/packages/typedoc-config/extended-tsconfig/workflows.json index 35d3b4171b784..d35b84c4f0e25 100644 --- a/docs-util/packages/typedoc-config/extended-tsconfig/workflows.json +++ b/docs-util/packages/typedoc-config/extended-tsconfig/workflows.json @@ -1,6 +1,6 @@ { "$schema": "http://json.schemastore.org/tsconfig", "extends": [ - "../../../../packages/workflows/tsconfig.json" + "../../../../packages/workflows-sdk/tsconfig.json" ] } \ No newline at end of file diff --git a/docs-util/packages/typedoc-config/workflows.js b/docs-util/packages/typedoc-config/workflows.js index 199ea0b5dbf7d..d3c436152f76f 100644 --- a/docs-util/packages/typedoc-config/workflows.js +++ b/docs-util/packages/typedoc-config/workflows.js @@ -7,12 +7,12 @@ const pathPrefix = path.join(__dirname, "..", "..", "..") module.exports = { ...globalTypedocOptions, entryPoints: [ - path.join(pathPrefix, "packages/workflows/src/utils/composer/index.ts"), + path.join(pathPrefix, "packages/workflows-sdk/src/utils/composer/index.ts"), ], out: [path.join(pathPrefix, "www/apps/docs/content/references/workflows")], tsconfig: path.join(__dirname, "extended-tsconfig", "workflows.json"), - name: "Workflows Reference", - indexTitle: "Workflows Reference", + name: "Workflows API Reference", + indexTitle: "Workflows API Reference", entryDocument: "index.mdx", hideInPageTOC: true, hideBreadcrumbs: true, @@ -43,6 +43,8 @@ module.exports = { }, }, "index\\.mdx": { + reflectionDescription: + "This section of the documentation provides a reference to the utility functions of the `@medusajs/workflows-sdk` package.", reflectionGroups: { Namespaces: false, Enumerations: false, @@ -55,6 +57,18 @@ module.exports = { }, functions: { maxLevel: 1, + reflectionDescription: + "This documentation provides a reference to the `{{alias}}` {{kind}}. It belongs to the `@medusajs/workflows-sdk` package.", + frontmatterData: { + displayed_sidebar: "workflowsSidebar", + slug: "/references/workflows/{{alias}}", + sidebar_label: "{{alias}}", + }, + reflectionTitle: { + kind: false, + typeParameters: false, + suffix: "- Workflows Reference", + }, }, "classes/StepResponse": { reflectionGroups: { diff --git a/www/apps/docs/content/development/workflows/index.mdx b/www/apps/docs/content/development/workflows/index.mdx new file mode 100644 index 0000000000000..8a8bac7761aee --- /dev/null +++ b/www/apps/docs/content/development/workflows/index.mdx @@ -0,0 +1,414 @@ +import Tabs from '@theme/Tabs'; +import TabItem from '@theme/TabItem'; +import DocCard from '@theme/DocCard'; +import Icons from '@theme/Icon'; + +# Workflows Introduction + +A workflow is a series of queries and actions that complete a task. Workflows are made up of a series of steps that interact with Medusa’s commerce modules, custom services, or external systems. + +You construct a Workflow similar to how you create a JavaScript function, but unlike regular functions, a Medusa Workflow creates an internal representation of your steps. This makes it possible to keep track of your Workflow’s progress, automatically retry failing steps, and, if necessary, roll back steps. + +Workflows can be used to define a flow with interactions across multiple systems, integrate third-party services into your commerce application, or automate actions within your application. Any flow with a series of steps can be implemented as a workflow. + +## Example: Your First Workflow + +The tools to build Workflows are installed by default in Medusa projects. For other Node.js projects, you can install the `@medusajs/workflows-sdk` package from npm. + +### Create a Step + +A workflow is made of a series of steps. A step is created using the `createStep` utility function. + +Create the file `src/workflows/hello-world.ts` with the following content: + +```ts title=src/workflows/hello-world.ts +import { + createStep, + StepResponse, +} from "@medusajs/workflows-sdk" + +const step1 = createStep("step-1", async () => { + return new StepResponse(`Hello from step one!`) +}) +``` + +This creates one step that returns a hello message. + +### Create a Workflow + +Next, you can create a workflow using the `createWorkflow` function: + +```ts title=src/workflows/hello-world.ts +import { + createStep, + StepResponse, + createWorkflow, +} from "@medusajs/workflows-sdk" + +type WorkflowOutput = { + message: string; +}; + +const step1 = createStep("step-1", async () => { + return new StepResponse(`Hello from step one!`) +}) + +const myWorkflow = createWorkflow<any, WorkflowOutput>( + "hello-world", + function () { + const str1 = step1({}) + + return { + message: str1, + } + } +) +``` + +This creates a `hello-world` workflow. When you create a workflow, it’s constructed but not executed yet. + +### Execute the Workflow + +You can execute a workflow from different places within Medusa. + +- Use API Routes if you want the workflow to execute in response to an API request or a webhook. +- Use Subscribers if you want to execute a workflow when an event is triggered. +- Use Scheduled Jobs if you want your workflow to execute on a regular schedule. + +To execute the workflow, invoke it passing the Medusa container as a parameter, then use its `run` method: + +<Tabs groupId="resource-type" isCodeTabs={true}> + <TabItem value="api-route" label="API Route" attributes={{ + title: "src/api/store/custom/route.ts" + }} default> + + ```ts + import type { + MedusaRequest, + MedusaResponse + } from "@medusajs/medusa"; + import myWorkflow from "../../../workflows/hello-world"; + + export async function GET( + req: MedusaRequest, + res: MedusaResponse + ) { + const { result } = await myWorkflow(req.scope) + .run() + + res.send(result) + } + ``` + + </TabItem> + <TabItem value="subscribers" label="Subscribers" attributes={{ + title: "src/subscribers/create-customer.ts" + }}> + + ```ts + import { + type SubscriberConfig, + type SubscriberArgs, + CustomerService, + Customer, + } from "@medusajs/medusa" + import myWorkflow from "../workflows/hello-world" + + export default async function handleCustomerCreate({ + data, eventName, container, pluginOptions + }: SubscriberArgs<Customer>) { + myWorkflow(container) + .run() + .then(({ result }) => { + console.log( + `New user: ${result.message}` + ) + }) + } + + export const config: SubscriberConfig = { + event: CustomerService.Events.CREATED, + context: { + subscriberId: "hello-customer" + } + } + ``` + + </TabItem> + <TabItem value="job" label="Scheduled Job" attributes={{ + title: "src/jobs/message-daily.ts" + }}> + + ```ts + import { + type ScheduledJobConfig, + type ScheduledJobArgs, + } from "@medusajs/medusa" + import myWorkflow from "../workflows/hello-world" + + export default async function handler({ + container, + data, + pluginOptions, + }: ScheduledJobArgs) { + myWorkflow(container) + .run() + .then(({ result }) => { + console.log( + result.message + ) + }) + } + + export const config: ScheduledJobConfig = { + name: "run-once-a-day", + schedule: "0 0 * * *", + data: {}, + } + ``` + + </TabItem> +</Tabs> + +If you run your backend and trigger the execution of the workflow (based on where you’re executing it), you should see the message `Hello from step one!`. + +### Pass Inputs to Steps + +Steps in a workflow can accept parameters. + +For example, create a new step that accepts an input and returns a message with that input: + +```ts title=src/workflows/hello-world.ts +type WorkflowInput = { + name: string; +}; + +const step2 = createStep( + "step-2", + async ({ name }: WorkflowInput) => { + return new StepResponse(`Hello ${name} from step two!`) + } +) +``` + +Then, update the workflow to accept input and pass it to the new step: + +```ts title=src/workflows/hello-world.ts +import { + // previous imports + transform, +} from "@medusajs/workflows-sdk" + +// ... + +const myWorkflow = createWorkflow< + WorkflowInput, WorkflowOutput +>( + "hello-world", + function (input) { + const str1 = step1({}) + const str2 = step2(input) + + const result = transform( + { + str1, + str2, + }, + (input) => ({ + message: `${input.str1}\n${input.str2}`, + }) + ) + + return result + } +) +``` + +Notice that to use the results of the steps, you must use the `transform` utility function. It gives you access to the real-time results of the steps once the workflow is executed. + +If you execute the workflow again, you’ll see: + +- A `Hello from step one!` message, indicating that step one ran first. +- A `Hello {name} from step two` message, indicating that step two ran after. + +### Add Error Handling + +Errors can occur in a workflow. To avoid data inconsistency, you can pass a compensation function as a third parameter to the `createStep` function. + +The compensation function only runs if an error occurs throughout the Workflow. It’s useful to undo or roll back actions you’ve performed in a step. + +For example, change step one to add a compensation function and step two to throw an error: + +```ts title=src/workflows/hello-world.ts +const step1 = createStep("step-1", async () => { + const message = `Hello from step one!` + + console.log(message) + + return new StepResponse(message) +}, async () => { + console.log("Oops! Rolling back my changes...") +}) + +const step2 = createStep( + "step-2", + async ({ name }: WorkflowInput) => { + throw new Error("Throwing an error...") + } +) +``` + +If you execute the Workflow, you should see: + +- `Hello from step one!` logged, indicating that the first step ran successfully. +- `Oops! Rolling back my changes...` logged, indicating that the second step failed and the compensation function of the first step ran consequently. + +:::note[Try it Out] + +You can try out this guide on [Stackblitz](https://stackblitz.com/edit/stackblitz-starters-etznpy?file=compensation-demo.ts&view=editor). + +::: + +--- + +## More Advanced Example + +Let’s cover a more realistic example. + +For example, you can build a workflow that updates a product’s CMS details both in Medusa and an external CMS service: + +```ts +import { createWorkflow } from "@medusajs/workflows-sdk" +import { Product } from "@medusajs/medusa" +import { updateProduct, sendProductDataToCms } from "./steps" + +type WorkflowInput = { + id: string + title: string, + description: string, + images: string[] +} + +const updateProductCmsWorkflow = createWorkflow< + WorkflowInput, + Product +>("update-product-cms", function (input) { + const product = updateProduct(input) + sendProductDataToCms(product) + + return product +}) +``` + +As these steps are making changes to data in the Medusa backend and a third-party service, it’s useful to provide a compensation function for each step that rolls back the changes. + +For example, you can pass a compensation function to the `updateProduct` step that reverts the product update in case an error occurs: + +```ts +const updateProduct = createStep( + "update-product", + async (input: WorkflowInput, context) => { + const productService: ProductService = + context.container.resolve("productService") + + const { id, ...updateData } = input + const previousProductData = await productService.retrieve( + id, { + select: ["title", "description", "images"], + } + ) + const product = productService.update(id, updateData) + + return new StepResponse(product, { + id, + previousProductData, + }) + }, async ({ id, previousProductData }, context) => { + const productService: ProductService = + context.container.resolve("productService") + + productService.update(id, previousProductData) + } +) +``` + +Your steps may interact with external systems. For example, the `sendProductDataToCms` step communicates with an external CMS service. With the error handling and roll-back features that workflows provide, developers can ensure data delivery between multiple systems in their stack. + +--- + +## Constraints on Workflow Constructor Function + +The Workflow Builder, `createWorkflow`, comes with a set of constraints: + +- The function passed to the `createWorkflow` can’t be an arrow function: + +```ts +// Don't +const myWorkflow = createWorkflow< + WorkflowInput, + WorkflowOutput +>("hello-world", (input) => { + // ... + } +) + +// Do +const myWorkflow = createWorkflow<WorkflowInput, WorkflowOutput> + ("hello-world", function (input) { + // ... + } +) +``` + +- The function passed to the `createWorkflow` can’t be an asynchronous function. +- Since the constructor function only defines how the workflow works, you can’t directly manipulate data within the function. To do that, you must use the `transform` function: + +```ts +1// Don't +const myWorkflow = createWorkflow< + WorkflowInput, + WorkflowOutput +>("hello-world", function (input) { + const str1 = step1(input) + const str2 = step2(input) + + return { + message: `${input.str1}${input.str2}`, + } + } +) + +// Do +const myWorkflow = createWorkflow< + WorkflowInput, + WorkflowOutput +>("hello-world", function (input) { + const str1 = step1(input) + const str2 = step2(input) + + const result = transform({ + str1, + str2, + }, (input) => ({ + message: `${input.str1}${input.str2}`, + })) + + return result + } +) +``` + +--- + +## Next Steps + +<DocCard item={{ + type: 'link', + href: '/references/workflows', + label: 'Workflows API Reference', + customProps: { + icon: Icons['academic-cap-solid'], + description: 'Learn about workflow\'s utility methods.' + } + }} +/> diff --git a/www/apps/docs/content/experimental/index.md b/www/apps/docs/content/experimental/index.md new file mode 100644 index 0000000000000..4ce7d23dd1380 --- /dev/null +++ b/www/apps/docs/content/experimental/index.md @@ -0,0 +1,23 @@ +# Experimental Features + +This section of the documentation includes features that are currently experimental. + +As Medusa moves towards modularization, commerce concepts such as Products or Pricing will be moved to isolated commerce modules shipped as NPM packages. This significantly changes Medusa's architecture, making it more flexible for custom digital commerce applications. + +## Enabling Experimental Features + +:::danger[Production Warning] + +All features guarded by the `medusa_v2` flag are not ready for production and will cause unexpected issues in your production server. + +::: + +Experimental Features are guarded in the Medusa backend by a feature flag. + +To use them, [enable the `medusa_v2` feature flag](../development/feature-flags/toggle.md) in your backend. + +Then, run migrations in your backend with the following command: + +```bash +npx medusa migrations run +``` diff --git a/www/apps/docs/content/experimental/pricing/concepts.md b/www/apps/docs/content/experimental/pricing/concepts.md new file mode 100644 index 0000000000000..d781f662a96fe --- /dev/null +++ b/www/apps/docs/content/experimental/pricing/concepts.md @@ -0,0 +1,83 @@ +# Pricing Concepts + +In this document, you’ll learn about the main concepts in the Pricing module, and how data is stored and related. + +## Money Amount + +A `MoneyAmount` represents a price. + +Money amounts can be conditioned by the `min_quantity` and `max_quantity` attributes, which are helpful when calculating the price for a specific quantity. + +If a money amount has its `min_quantity` or `max_quantity` attributes set, they’re only considered for the price calculation if they have a lower `min_quantity` or a higher `max_quantity` than the quantity specified for calculation. + +--- + +## Price Set + +A `PriceSet` represents a collection of money amounts that are linked to a resource (for example, a product or a shipping option). The Price Set and Money Amount relationship is represented by the `PriceSetMoneyAmount` entity. + +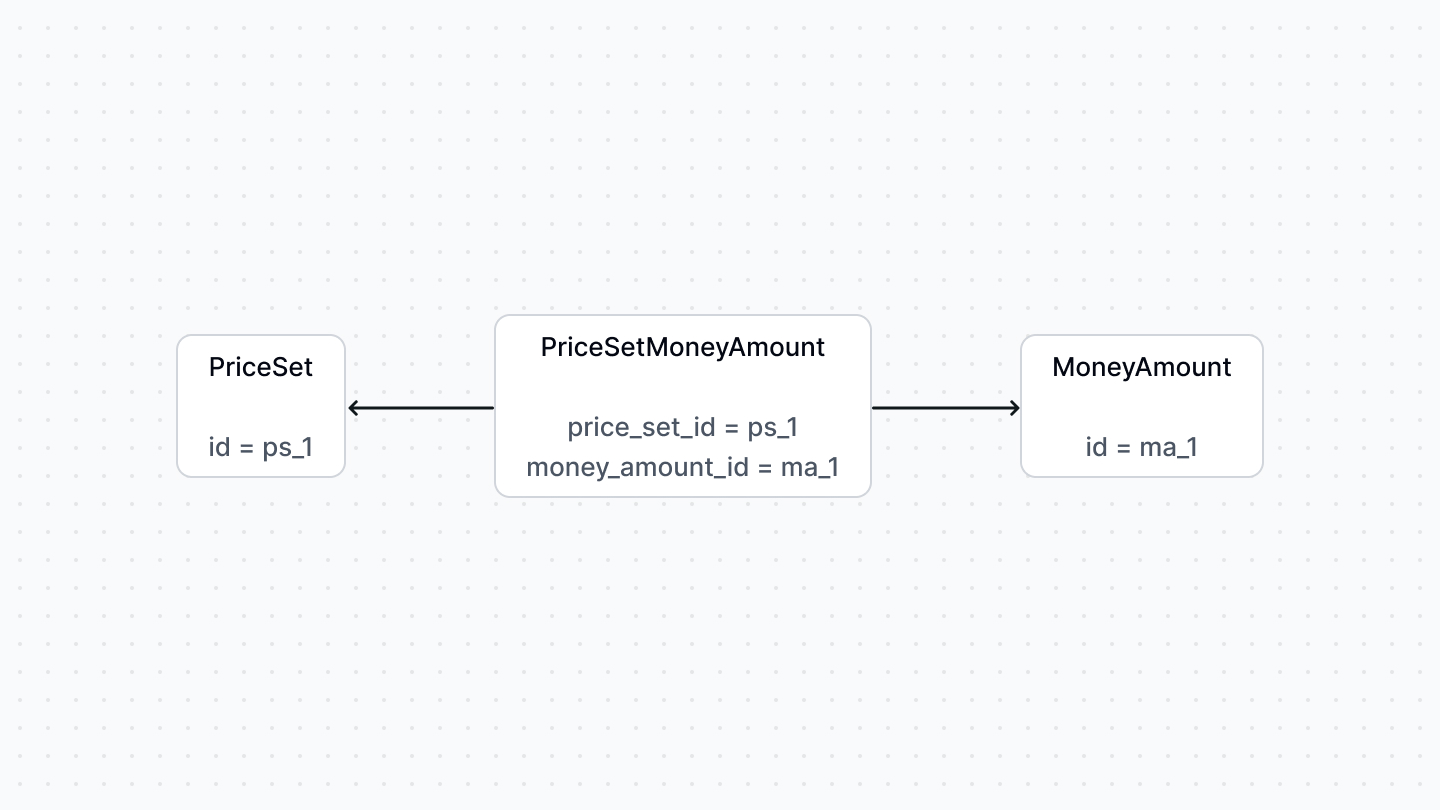 + +--- + +## Prices with Rules + +### Rule Type + +Each money amount within a price set can be a price that’s applied for different conditions. These conditions are represented as rule types. + +A `RuleType` defines custom conditions. Each rule type has a unique `rule_attribute`, referenced in rule values, such as when setting a rule of a money amount. + +### Price Rule + +Each rule of a money amount within a price set is represented by the `PriceRule` entity, which holds the value of a rule type. The `PriceSetMoneyAmount` has a `number_rules` attribute, which indicates how many rules, represented by `PriceRule`, are applied to the money amount. + +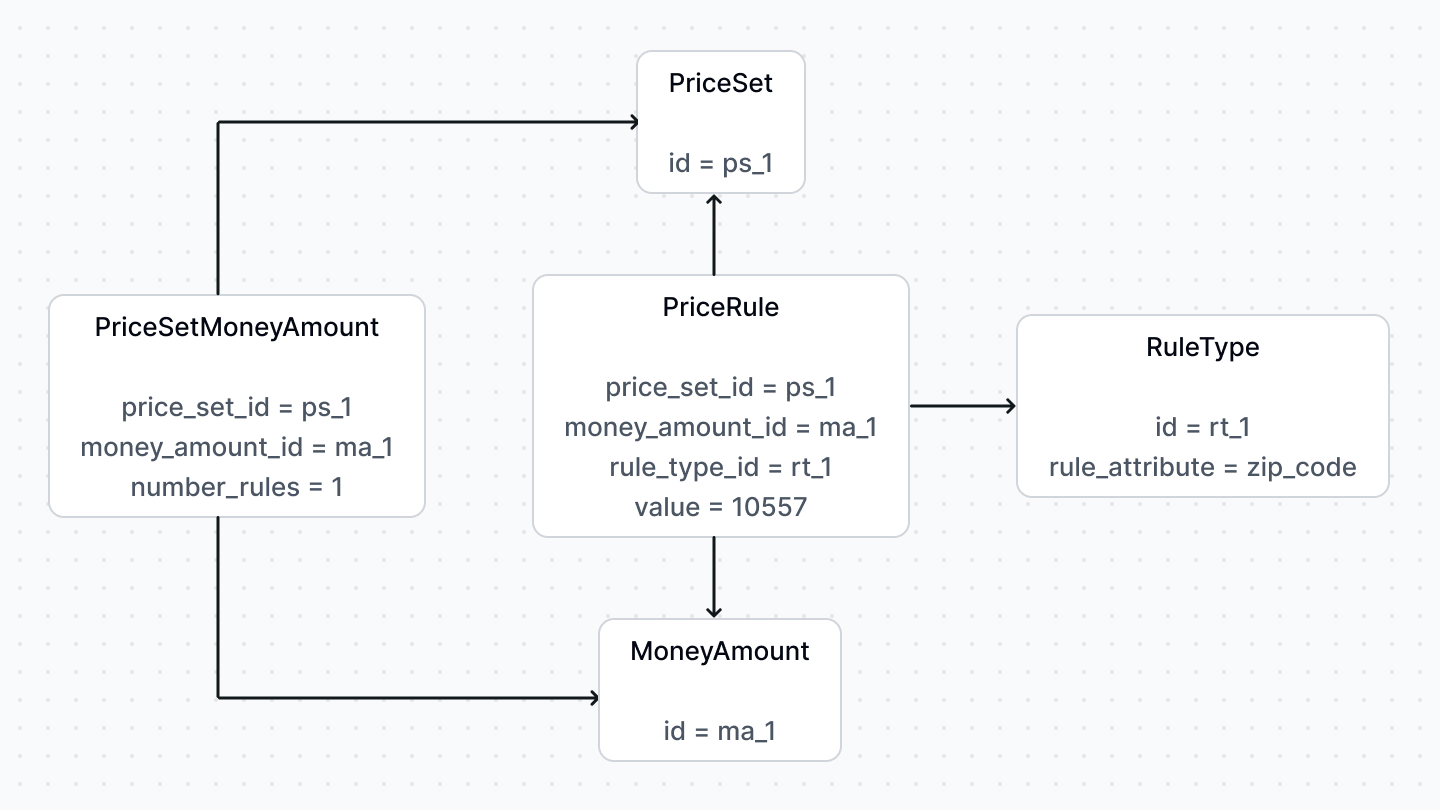 + +For example, you can create a `zip_code` rule type. Then, a money amount within the price set can have the rule value `zip_code: 10557`, indicating that the money amount can only be applied within the `10557` zip code. + +Each money amount within the price set can have different values for the same rule type. + +For example, this diagram showcases two money amounts having different values for the same rule type: + +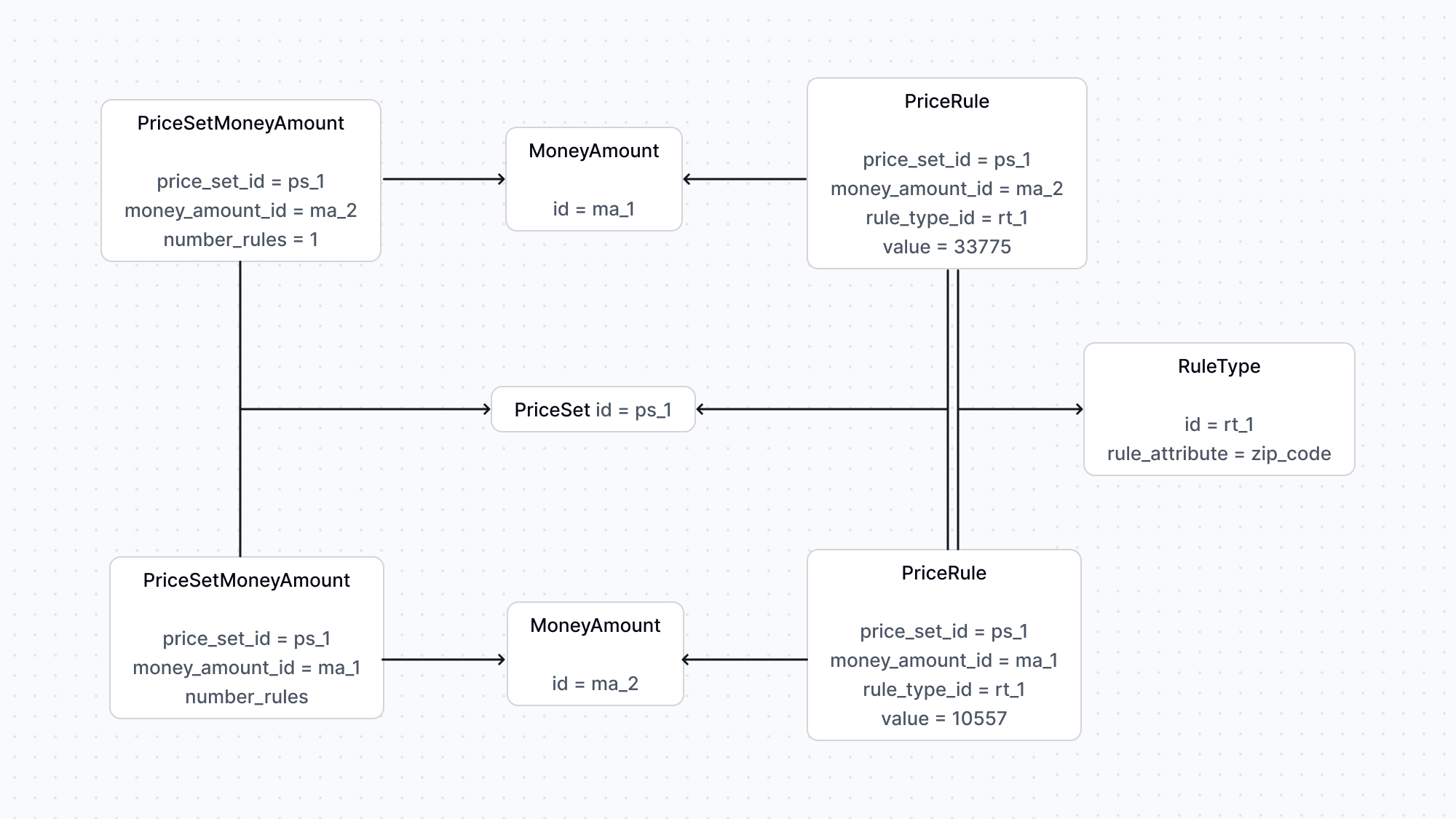 + +Each money amount can have multiple rules applied to it as well. + +For example, a money amount can have the rules `zip_code` and `region_id` applied to it. In this case, the value of each rule is represented by a `PriceRule`. + +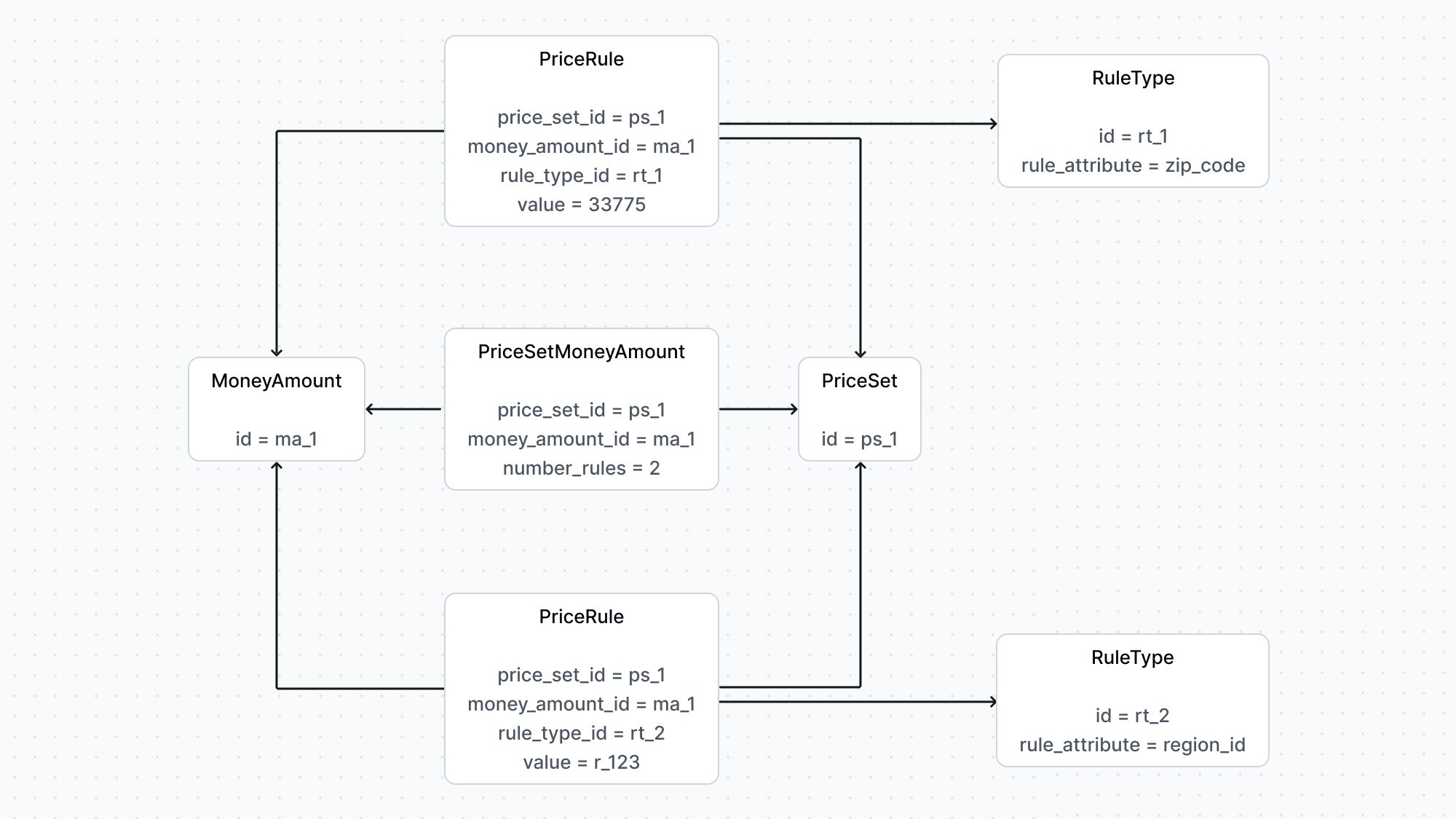 + +### PriceSetRuleType + +The `PriceSetRuleType` entity indicates what rules the money amounts can have within a price set. It creates a relation between the `PriceSet` and `RuleType` entities. + +For example, to use the `zip_code` rule type on a money amount in a price set, the rule type must first be enabled on the price set through the `PriceSetRuleType`. + +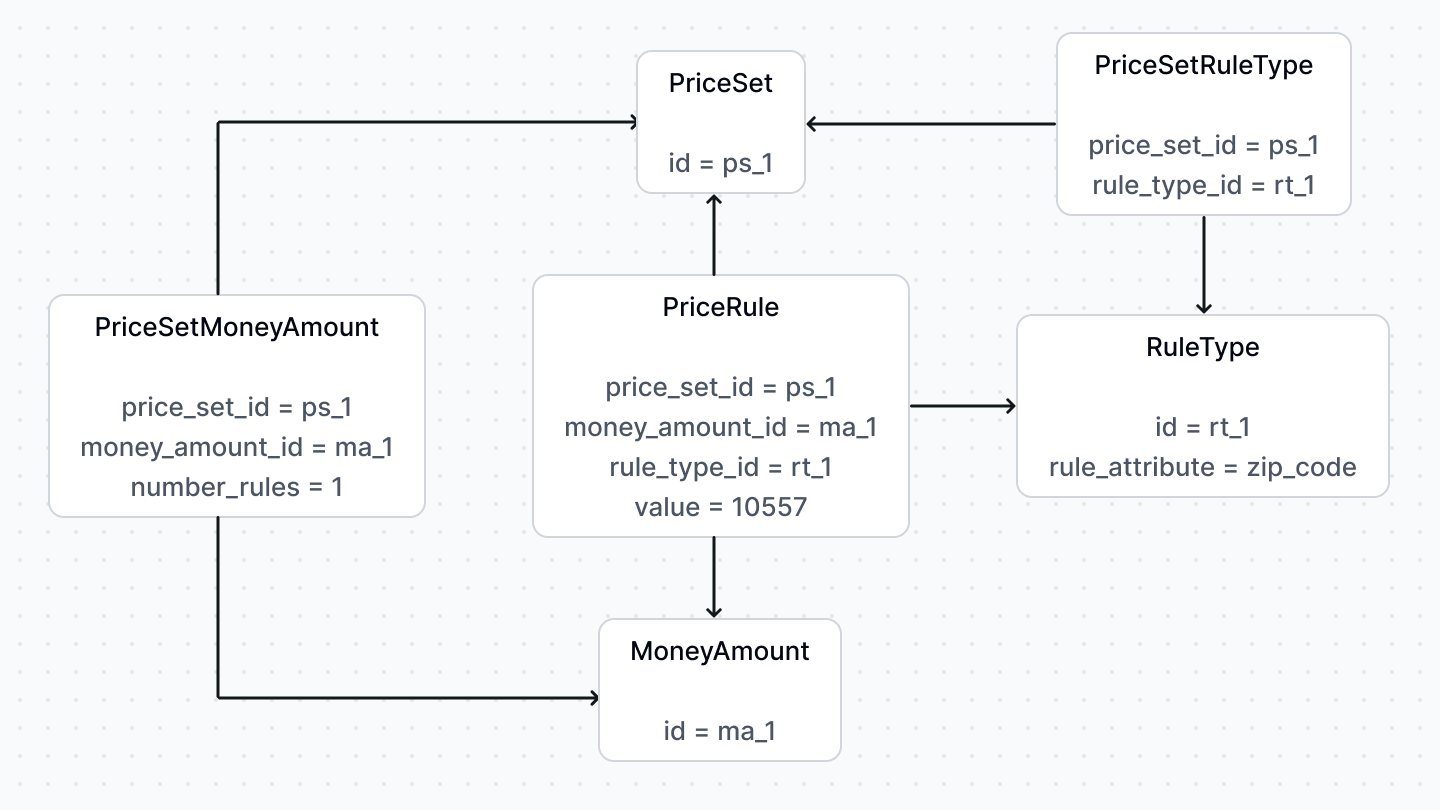 + +--- + +## Price List + +A `PriceList` is a group of prices only enabled if their rules are satisfied. A price list has optional `start_date` and `end_date` attributes, which indicate the date range in which a price list can be applied. + +Its associated prices are represented by the `PriceSetMoneyAmount` entity, which is used to store the money amounts of a price set. + +Each rule that can be applied to a price list is represented by the `PriceListRule` entity. The `number_rules` attribute of a `PriceList` indicates how many rules are applied to it. + +Each rule of a price list can have more than one value, representing its values by the `PriceListRuleValue` entity. + +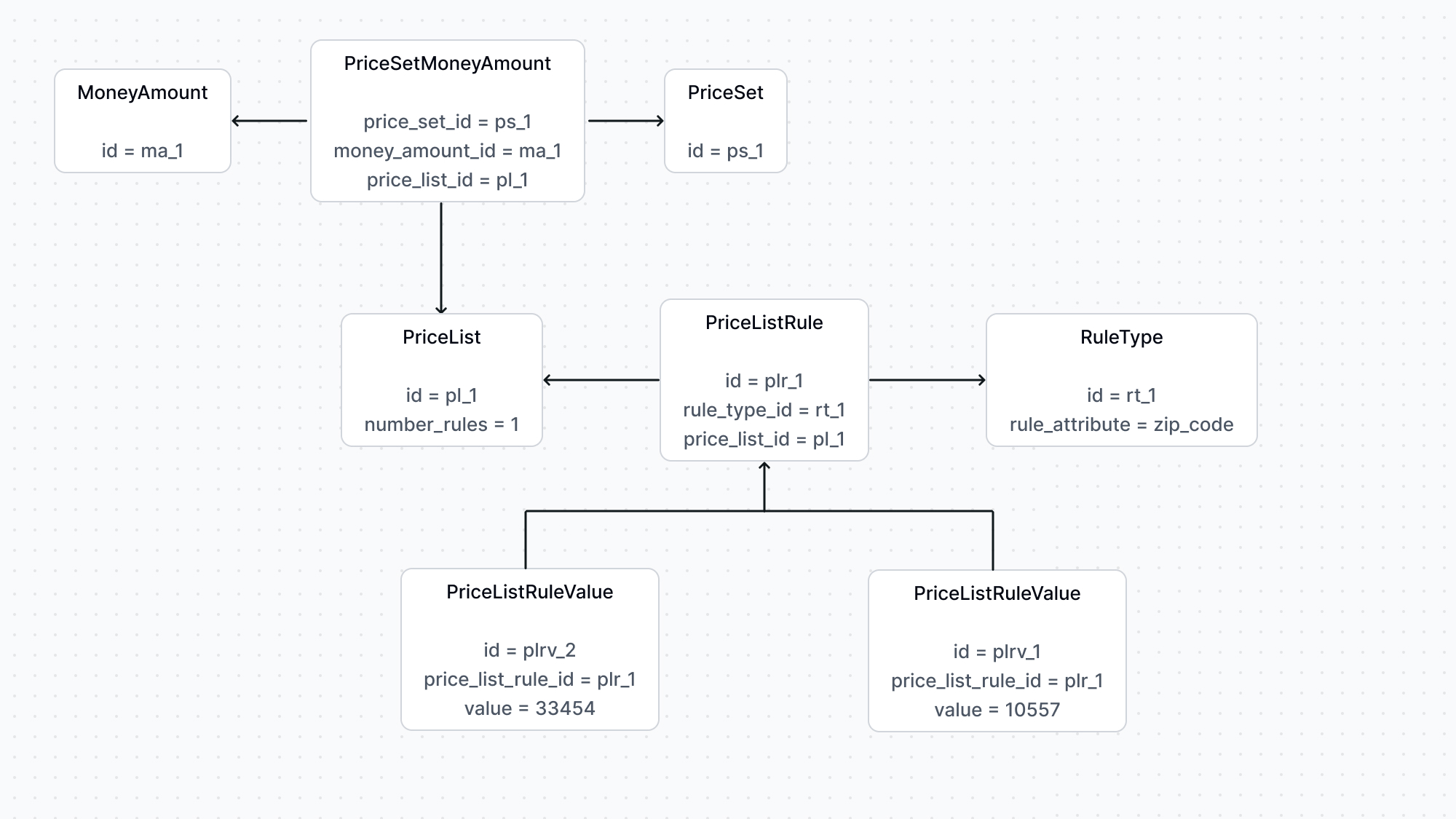 + +--- + +## Use Case Example: Pricing and Product Modules + +In a real use case, you would use the pricing module with your custom logic or other Medusa Commerce Modules, such as the Product Module. + +When used with the Product Module, a product variant’s prices are stored as money amounts belonging to a price set. A relation is formed between the `ProductVariant` and the `PriceSet` when the modules are linked. + +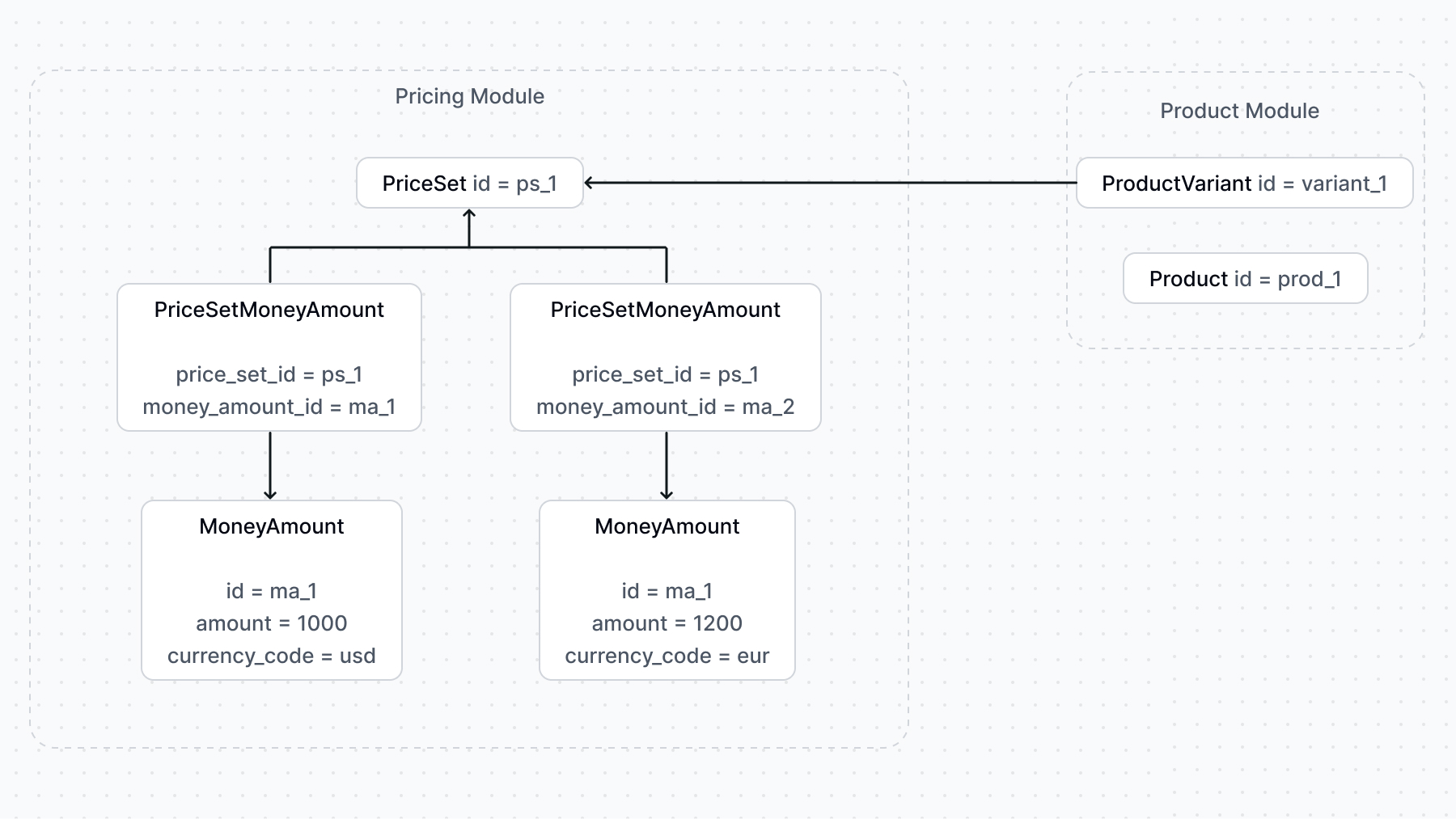 + +So, when you want to add prices for a product variant, you create a price set and add the prices as money amounts to it. You can then benefit from adding rules to prices or using the `calculatePrices` method to retrieve the price of a product variant within a specified context. diff --git a/www/apps/docs/content/experimental/pricing/examples.md b/www/apps/docs/content/experimental/pricing/examples.md new file mode 100644 index 0000000000000..4083b150f8a06 --- /dev/null +++ b/www/apps/docs/content/experimental/pricing/examples.md @@ -0,0 +1,251 @@ +# Examples of Pricing Module + +In this document, you’ll find common examples of how you can use the Pricing module in your application. + +:::note + +Examples in this section are in the context of a Next.js App Router. + +::: + +## Create a Price Set + +```ts +import { NextResponse } from "next/server" + +import { + initialize as initializePricingModule, +} from "@medusajs/pricing" + +export async function POST(request: Request) { + const pricingService = await initializePricingModule() + const body = await request.json() + + const priceSet = await pricingService.create([ + { + prices: [ + { + currency_code: body.currency_code, + amount: body.amount, + }, + ], + }, + ]) + + return NextResponse.json({ price_set: priceSet }) +} +``` + +## List Price Sets + +```ts +import { NextResponse } from "next/server" + +import { + initialize as initializePricingModule, +} from "@medusajs/pricing" + +export async function GET(request: Request) { + const pricingService = await initializePricingModule() + + const priceSets = await pricingService.list() + + return NextResponse.json({ price_sets: priceSets }) +} +``` + +## Retrieve a Price Set by its ID + +```ts +import { NextResponse } from "next/server" + +import { + initialize as initializePricingModule, +} from "@medusajs/pricing" + +type ContextType = { + params: { + id: string + } +} + +export async function GET( + request: Request, + { params }: ContextType +) { + const pricingService = await initializePricingModule() + + const priceSet = await pricingService.retrieve(params.id) + + return NextResponse.json({ price_set: priceSet }) +} +``` + +## Create a Rule Type + +```ts +import { NextResponse } from "next/server" + +import { + initialize as initializePricingModule, +} from "@medusajs/pricing" + +export async function POST(request: Request) { + const pricingService = await initializePricingModule() + const body = await request.json() + + const ruleTypes = await pricingService.createRuleTypes([ + { + name: body.name, + rule_attribute: body.rule_attribute, + }, + ]) + + return NextResponse.json({ rule_types: ruleTypes }) +} +``` + +## Add Prices with Rules + +```ts +import { NextResponse } from "next/server" + +import { + initialize as initializePricingModule, +} from "@medusajs/pricing" + +export async function POST(request: Request) { + const pricingService = await initializePricingModule() + const body = await request.json() + + const priceSet = await pricingService.addPrices({ + priceSetId: body.price_set_id, + prices: [ + { + amount: 500, + currency_code: "USD", + rules: { + region_id: body.region_id, + }, + }, + ], + }) + + return NextResponse.json({ price_set: priceSet }) +} +``` + +## Create a Currency + +```ts +import { NextResponse } from "next/server" + +import { + initialize as initializePricingModule, +} from "@medusajs/pricing" + +export async function POST(request: Request) { + const pricingService = await initializePricingModule() + const body = await request.json() + + const currencies = await pricingService.createCurrencies([{ + code: "EUR", + symbol: "€", + symbol_native: "€", + name: "Euro", + }]) + + return NextResponse.json({ currencies }) +} +``` + +## List Currencies + +```ts +import { NextResponse } from "next/server" + +import { + initialize as initializePricingModule, +} from "@medusajs/pricing" + +export async function GET(request: Request) { + const pricingService = await initializePricingModule() + + const currencies = await pricingService.listCurrencies() + + return NextResponse.json({ currencies }) +} +``` + +## Create Price List + +```ts +import { NextResponse } from "next/server" + +import { + initialize as initializePricingModule, +} from "@medusajs/pricing" + +export async function POST(request: Request) { + const pricingService = await initializePricingModule() + + const priceLists = await pricingService.createPriceLists({ + title: "My Sale", + type: "sale", + starts_at: Date.parse("01/10/2023"), + ends_at: Date.parse("31/10/2023"), + rules: { + region_id: ["DE", "DK"], + }, + prices: [ + { + amount: 400, + currency_code: "EUR", + price_set_id: priceSet.id, + }, + ], + }) + + return NextResponse.json({ price_lists: priceLists }) +} +``` + +## Calculate Prices For a Currency + +```ts +import { NextResponse } from "next/server" + +import { + initialize as initializePricingModule, +} from "@medusajs/pricing" + +type ContextType = { + params: { + id: string + currency_code: string + } +} + +export async function GET( + request: Request, + { params }: ContextType +) { + const pricingService = await initializePricingModule() + + const price = await pricingService.calculatePrices({ + id: [params.id], + }, { + context: { + currency_code: params.currency_code, + }, + }) + + return NextResponse.json({ price }) +} +``` + +--- + +## More Examples + +The [module interface reference](../../references/pricing/interfaces/IPricingModuleService.mdx) provides a reference to all the methods available for use with examples for each. \ No newline at end of file diff --git a/www/apps/docs/content/experimental/pricing/install-medusa.mdx b/www/apps/docs/content/experimental/pricing/install-medusa.mdx new file mode 100644 index 0000000000000..152286ed90b87 --- /dev/null +++ b/www/apps/docs/content/experimental/pricing/install-medusa.mdx @@ -0,0 +1,164 @@ +import DocCard from '@theme/DocCard' +import Icons from '@theme/Icon' +import Tabs from '@theme/Tabs'; +import TabItem from '@theme/TabItem'; + +# Install Pricing Module in Medusa + +In this document, you'll learn how to install the Pricing module using NPM in the Medusa backend. + +## Step 1: Install Module + +To install the Pricing module, run the following command in the root directory of the Medusa backend: + +```bash npm2yarn +npm install @medusajs/pricing +``` + +--- + +## Step 2: Add Module to Configurations + +In `medusa-config.js`, add the pricing module to the exported object under the `modules` property: + +```js title=medusa-config.js +module.exports = { + // ... + modules: { + // ... + pricingService: { + resolve: "@medusajs/pricing", + }, + }, +} +``` + +--- + +## Step 3: Run Migrations + +Run the following command to reflect schema changes into your database: + +```bash +npx medusa migrations run +``` + +--- + +## Use the Module + +You can now start using the module's `PricingModuleService` by resolving it through [dependency injection](../../development/fundamentals/dependency-injection.md). + +For example: + +<Tabs groupId="resource-type" isCodeTabs={true}> + <TabItem value="api-route" label="API Route" attributes={{ + title: "src/api/store/custom/route.ts" + }} default> + + ```ts + import type { + MedusaRequest, + MedusaResponse + } from "@medusajs/medusa"; + import { + PricingModuleService + } from "@medusajs/pricing" + + export async function GET( + req: MedusaRequest, + res: MedusaResponse + ) { + const pricingModuleService = req.scope.resolve( + "pricingModuleService" + ) + + return res.json({ + pricings: pricingModuleService.list() + }) + } + ``` + + </TabItem> + <TabItem value="subscribers" label="Subscribers" attributes={{ + title: "src/subscribers/create-customer.ts" + }}> + + ```ts + import { + type SubscriberConfig, + type SubscriberArgs, + PricingService, + } from "@medusajs/medusa" + import { + PricingModuleService + } from "@medusajs/pricing" + + export default async function handleListPriceSets({ + data, eventName, container, pluginOptions + }: SubscriberArgs<Customer>) { + const pricingModuleService = container.resolve( + "pricingModuleService" + ) + + console.log(await pricingModuleService.list()) + } + + export const config: SubscriberConfig = { + event: PricingService.Events.CREATED, + context: { + subscriberId: "list-pricings" + } + } + ``` + + </TabItem> + <TabItem value="service" label="Service" attributes={{ + title: "src/service/hello.ts" + }}> + + ```ts + import { TransactionBaseService } from "@medusajs/medusa" + import { + PricingModuleService + } from "@medusajs/pricing" + + class HelloService extends TransactionBaseService { + private pricingModuleService: PricingModuleService + + constructor(container) { + super(container) + this.pricingModuleService = container.pricingModuleService + } + + await listPriceSets() { + return await this.pricingModuleService.list() + } + } + + export default HelloService + ``` + + </TabItem> +</Tabs> + +:::tip + +In the Examples or API Reference guides, you may see an initialization of the pricing module. This is only necessary if you're using the module outside the Medusa backend. + +::: + +--- + +## Up Next + +<DocCard item={{ + type: 'link', + href: '/references/pricing', + label: 'Service Interface Reference', + customProps: { + icon: Icons['academic-cap-solid'], + description: 'Find a full reference of the module\' service interface\s methods.' + } + }} +/> \ No newline at end of file diff --git a/www/apps/docs/content/experimental/pricing/install-nodejs.md b/www/apps/docs/content/experimental/pricing/install-nodejs.md new file mode 100644 index 0000000000000..c3a519fe03cc3 --- /dev/null +++ b/www/apps/docs/content/experimental/pricing/install-nodejs.md @@ -0,0 +1,188 @@ +# Install in Node.js-Based Application + +In this document, you’ll learn how to setup and use the Pricing module in a Node.js based application. + +## Prerequisites + +Before installing the Pricing module in your application, make sure you have the following prerequisites: + +- Node.js v16 or greater +- PostgreSQL database. You can use an existing Medusa database, or set up a new PostgreSQL database. + +--- + +## Install Package + +In your Node.js-based applications, such as a Next.js application, you can install the Pricing module with the following command: + +```bash npm2yarn +npm install @medusajs/pricing +``` + +--- + +## Add Database Configuration + +Add the following environment variable to your application: + +```bash +POSTGRES_URL=<DATABASE_URL> +``` + +Where `<DATABASE_URL>` is your database connection URL of the format `postgres://[user][:password]@[host][:port]/[dbname]`. You can learn more about the connection URL format in [this guide](../../development/backend/configurations.md#database_url). + +You can also set the following optional environment variables: + +- `POSTGRES_SCHEMA`: a string indicating the PostgreSQL schema to use. By default, it's `public`. +- `POSTGRES_DRIVER_OPTIONS`: a stringified JSON object indicating the PostgreSQL options to use. The JSON object is then parsed to be used as a JavaScript object. By default, it's `{"connection":{"ssl":false}}` for local PostgreSQL databases, and `{"connection":{"ssl":{"rejectUnauthorized":false}}}` for remote databases. + +:::note + +If `POSTGRES_DRIVER_OPTIONS` is not specified, the PostgreSQL database is considered local if `POSTGRES_URL` includes `localhost`. Otherwise, it's considered remote. + +::: + +### Run Database Migrations + +:::note + +You can skip this step if you use an existing Medusa database. + +::: + +Migrations are used to create your database schema. Before you can run migrations, add in your `package.json` the following scripts: + +```json +"scripts": { + //...other scripts + "price:migrations:run": "medusa-pricing-migrations-up", + "price:seed": "medusa-pricing-seed ./pricing-seed-data.js" +}, + +``` + +The first command runs the migrations, and the second command allows you to seed your database with demo prices optionally. + +However, you’d need the following seed file added to the root of your project directory: + +<Details> + <Summary>Seed file</Summary> + + ```js + const currenciesData = [ + { + code: "USD", + symbol: "$", + symbol_native: "$", + name: "US Dollar", + }, + { + code: "CAD", + symbol: "CA$", + symbol_native: "$", + name: "Canadian Dollar", + }, + { + code: "EUR", + symbol: "€", + symbol_native: "€", + name: "Euro", + }, + ] + + const moneyAmountsData = [ + { + id: "money-amount-USD", + currency_code: "USD", + amount: 500, + min_quantity: 1, + max_quantity: 10, + }, + { + id: "money-amount-EUR", + currency_code: "EUR", + amount: 400, + min_quantity: 1, + max_quantity: 5, + }, + { + id: "money-amount-CAD", + currency_code: "CAD", + amount: 600, + min_quantity: 1, + max_quantity: 8, + }, + ] + + const priceSetsData = [ + { + id: "price-set-USD", + }, + { + id: "price-set-EUR", + }, + { + id: "price-set-CAD", + }, + ] + + const priceSetMoneyAmountsData = [ + { + title: "USD Price Set", + price_set: "price-set-USD", + money_amount: "money-amount-USD", + }, + { + title: "EUR Price Set", + price_set: "price-set-EUR", + money_amount: "money-amount-EUR", + }, + ] + + module.exports = { + currenciesData, + moneyAmountsData, + priceSetsData, + priceSetMoneyAmountsData, + } + ``` + +</Details> + +Then run the commands you added to migrate the database schema and optionally seed data: + +```bash npm2yarn +npm run price:migrations:run +# optionally +npm run price:seed +``` + +--- + +## Next.js Application: Adjust Configurations + +The Pricing module uses dependencies that aren’t Webpack optimized. Since Next.js uses Webpack for compilation, you need to add the Pricing module as an external dependency. + +To do that, add the `serverComponentsExternalPackages` option in `next.config.js`: + +```js title=next.config.js +/** @type {import('next').NextConfig} */ +const nextConfig = { + experimental: { + serverComponentsExternalPackages: [ + "@medusajs/pricing", + ], + }, +} + +module.exports = nextConfig + +``` + +--- + +## Start Development + +You can refer to the [Example Usages documentation page](./examples.md) for examples of using the Pricing module. + +You can also refer to the [Module Interface Reference](../../references/pricing/interfaces/IPricingModuleService.mdx) for a detailed reference on all available methods. \ No newline at end of file diff --git a/www/apps/docs/content/experimental/pricing/overview.mdx b/www/apps/docs/content/experimental/pricing/overview.mdx new file mode 100644 index 0000000000000..a8d87ac47e9b6 --- /dev/null +++ b/www/apps/docs/content/experimental/pricing/overview.mdx @@ -0,0 +1,122 @@ +import DocCard from '@theme/DocCard' +import Icons from '@theme/Icon' + +# Pricing Module Overview + +The Pricing module is the `@medusajs/pricing` NPM package that provides advanced pricing features in your Medusa and Node.js applications. It can be used to add prices to any resource, such as products or shipping options. + +## Features + +### Price Management + +With the Product module, you can store the prices of a resource and manage them through the main interface method. Prices are grouped in a price set, allowing you to add more than one price for a resource based on different conditions, such as currency code. + +```ts +const priceSet = await pricingService.create({ + rules: [], + prices: [ + { + amount: 500, + currency_code: "USD", + rules: {}, + }, + { + amount: 400, + currency_code: "EUR", + min_quantity: 0, + max_quantity: 4, + rules: {}, + }, + ], +}) +``` + +### Advanced Rule Engine + +You can create custom rules and apply them to prices. This gives you more flexibility in how you condition prices, filter them, and ensure the best prices are retrieved for custom contexts. + +```ts +const ruleTypes = await pricingService.createRuleTypes([ + { + name: "Region", + rule_attribute: "region_id", + }, +]) + +const priceSet = await pricingService.addPrices({ + priceSetId, + prices: [ + { + amount: 500, + currency_code: "EUR", + rules: { + region_id: "PL", + }, + }, + ], +}) +``` + +### Price Lists + +Price lists allow you to group prices and apply them only in specific conditions. You can also use them to override existing prices for the specified conditions. + +```ts +const priceList = await pricingService.createPriceLists({ + title: "My Sale", + type: "sale", + starts_at: Date.parse("01/10/2023"), + ends_at: Date.parse("31/10/2023"), + rules: { + region_id: ["DE", "DK"], + }, + prices: [ + { + amount: 400, + currency_code: "EUR", + price_set_id: priceSet.id, + }, + ], +}) +``` + +### Price Calculation Strategy + +The module’s main interface provides a `calculatePrices` method to retrieve the best price for a given context. You can benefit from your custom rules here to find the best price for the specified rule values. + +```ts +const price = await pricingService.calculatePrices( + { id: [priceSetId] }, + { + context: { + currency_code: "EUR", + region_id: "PL", + }, + } +) +``` + +--- + +## How to Use the Pricing Module + +The Pricing Module can be used in many use cases, including: + +- Medusa Backend: The Medusa backend uses the pricing module to implement some features. However, it's guarded by the [experimental feature flag](../index.md#enabling-experimental-features). If you want to use the pricing module in your backend's customizations, follow [this installation guide](./install-medusa.mdx). +- Serverless Application: Use the Pricing Module in a serverless application, such as a Next.js application, without having to manage a fully-fledged ecommerce system. You can use it by [installing it in your Node.js project as an NPM package](./install-nodejs.md). +- Node.js Application: Use the Pricing Module in any Node.js application. Follow [this guide](./install-nodejs.md) to learn how to install it. + +--- + +## Up Next + +<DocCard item={{ + type: 'link', + href: '/experimental/pricing/concepts', + label: 'Pricing Concepts', + customProps: { + icon: Icons['academic-cap-solid'], + description: 'Learn about the main concepts in the Pricing module.' + } + }} +/> diff --git a/www/apps/docs/content/experimental/pricing/prices-calculation.mdx b/www/apps/docs/content/experimental/pricing/prices-calculation.mdx new file mode 100644 index 0000000000000..4fd4af31a3d1d --- /dev/null +++ b/www/apps/docs/content/experimental/pricing/prices-calculation.mdx @@ -0,0 +1,470 @@ +import Tabs from '@theme/Tabs'; +import TabItem from '@theme/TabItem'; + +# Prices Calculation + +In this document, you'll learn how prices are calculated under the hood when you use the `calculatePrices` method of the Pricing module interface. + +## Overview + +The `calculatePrices` method accepts the ID of one or more price sets and a context. For each price set, it selects two types of prices that best match the context; one belongs to a price list, and one doesn't. + +Then, it returns an array of price objects, each price for every supplied price set ID. + +--- + +## Calculation Context + +The context is an object passed to the method. It must contain at least the `currency_code`. Only money amounts in the price sets with the same currency code are considered for the price selection. + +For example: + +```ts +import { + initialize as initializePricingModule, +} from "@medusajs/pricing" +async function calculatePrice( + priceSetId: string, + currencyCode: string +) { + const pricingService = await initializePricingModule() + + const price = await pricingService.calculatePrices( + { id: [priceSetId] }, + { + context: { + currency_code: currencyCode, + }, + } + ) +} +``` + +The context object can also contain any custom rules, with the key being the `rule_attribute` of a rule type and its value being the rule's value. + +For example: + +```ts +import { + initialize as initializePricingModule, +} from "@medusajs/pricing" +async function calculatePrice( + priceSetId: string, + currencyCode: string +) { + const pricingService = await initializePricingModule() + + const price = await pricingService.calculatePrices( + { id: [priceSetId] }, + { + context: { + currency_code: currencyCode, + region_id: "US", + }, + } + ) +} +``` + +--- + +## Prices Selection + +For each price set, the method selects two money amounts: + +- The calculated price: a money amount that belongs to a price list. If there are no money amounts associated with a price list, it’ll be the same as the original price. +- The original price: a money amount that doesn't belong to a price list. + +### Calculated Price Selection Process + +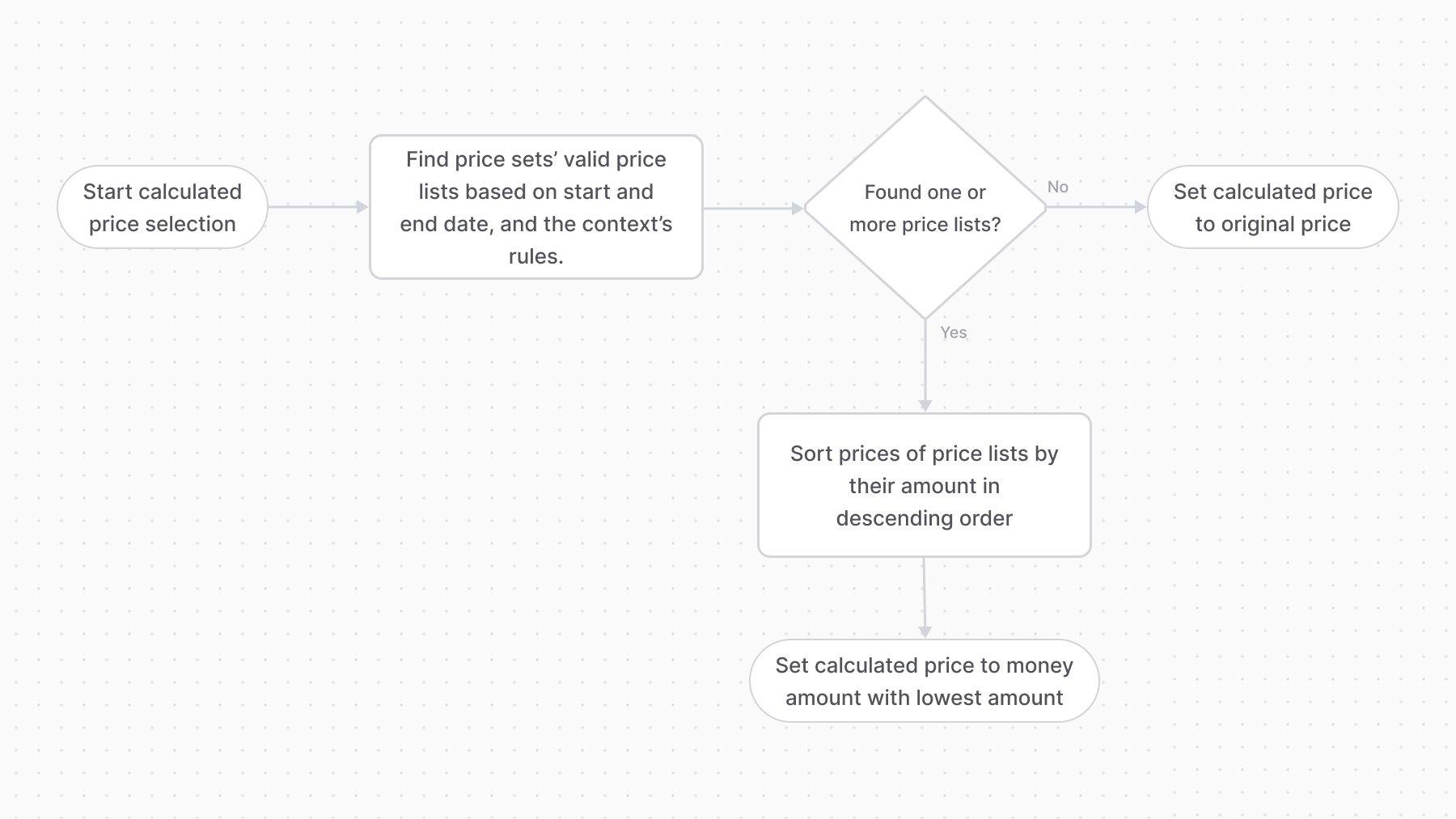 + +- Find the price set’s associated valid price lists. A price list is considered valid if: + - The current date is between its start and end dates. + - The price list's rules satisfy the context's rules. +- If valid price lists are found, the money amounts within them are sorted by their amount in ascending order. The one having the lowest amount is selected as the calculated price. +- If no valid price list is found, the selected calculated price will be the same as the original price. + +### Original Price Selection Process + +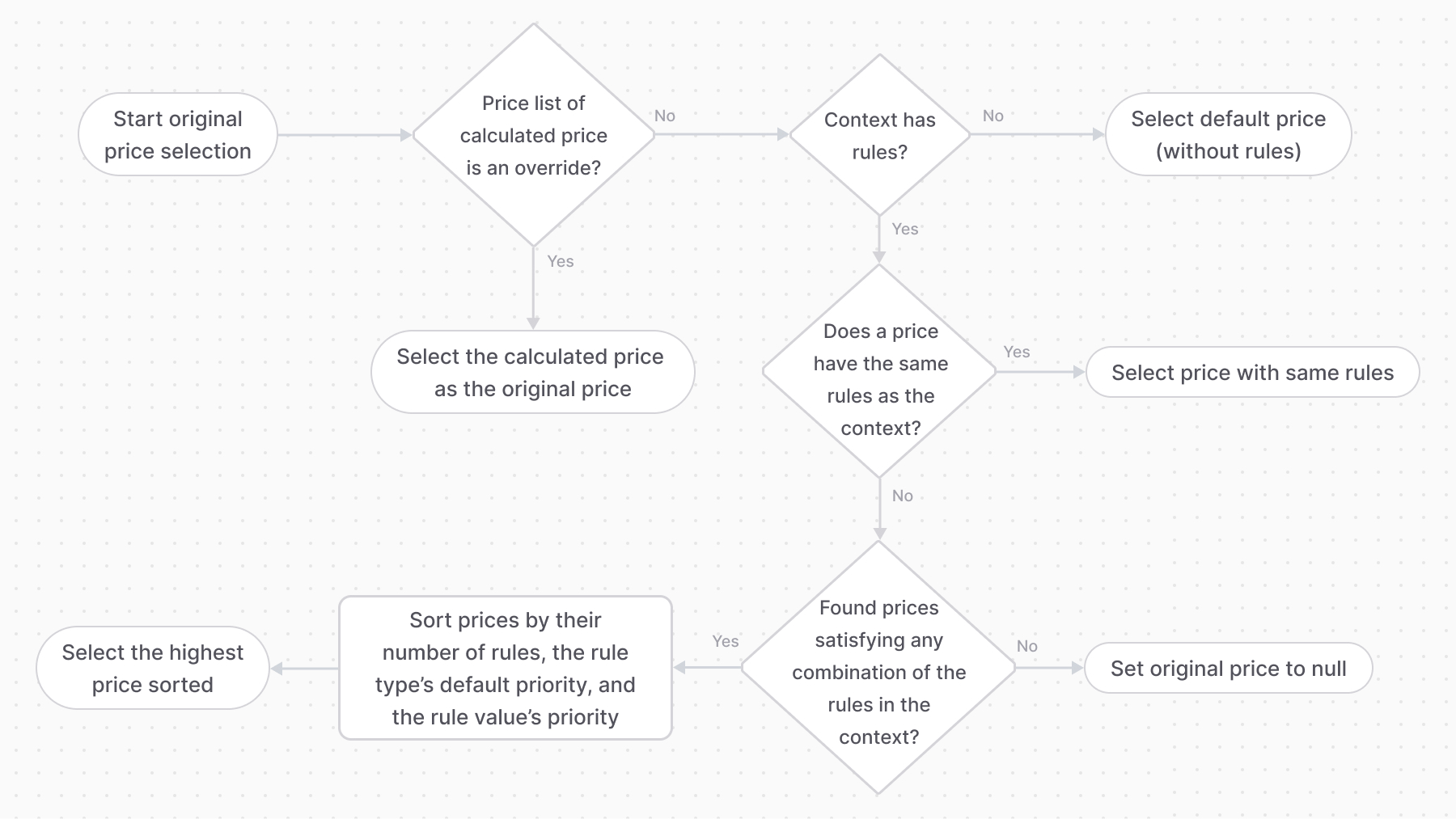 + +- If the price list associated with the calculated price is of type `override`, the selected original price is set to the calculated price. +- If no rules are provided in the context other than the `currency_code`, the default money amount is selected as the original price. The default money amount is a money amount having no rules applied to it. +- Otherwise, if a money amount exists in any price set with the same rules provided in the context, it's selected as the original price. +- If no money amount exists with the same rules as the context, all money amounts satisfying any combination of the provided rules are retrieved. + - The money amounts are sorted in descending order by the associated `PriceSetMoneyAmount`'s `number_rules`, the `default_priority` of the rule types, and the `priority` of the associated `PriceRule`. The `priority` attribute has a higher precedence than the `default_priority`. + - The highest money amount sorted is selected as the original price since it's considered the best price. + +--- + +## Returned Calculated Price + +After the original and calculated prices are selected, the method will use them to create the following price object for each pr + +```ts +const price = { + id: priceSetId, + is_calculated_price_price_list: + !!calculatedPrice?.price_list_id, + calculated_amount: parseInt(calculatedPrice?.amount || "") || + null, + + is_original_price_price_list: !!originalPrice?.price_list_id, + original_amount: parseInt(originalPrice?.amount || "") || + null, + + currency_code: calculatedPrice?.currency_code || + null, + + calculated_price: { + money_amount_id: calculatedPrice?.id || null, + price_list_id: calculatedPrice?.price_list_id || null, + price_list_type: calculatedPrice?.price_list_type || null, + min_quantity: parseInt( + calculatedPrice?.min_quantity || "" + ) || null, + max_quantity: parseInt( + calculatedPrice?.max_quantity || "" + ) || null, + }, + + original_price: { + money_amount_id: originalPrice?.id || null, + price_list_id: originalPrice?.price_list_id || null, + price_list_type: originalPrice?.price_list_type || null, + min_quantity: parseInt(originalPrice?.min_quantity || "") || + null, + max_quantity: parseInt(originalPrice?.max_quantity || "") || + null, + }, +} +``` + +Where: + +- `id`: The ID of the price set from which the money amount was selected. +- `is_calculated_price_price_list`: whether the calculated price belongs to a price list. As mentioned earlier, if no valid price list is found, the calculated price is set to the original price, which doesn't belong to a price list. +- `calculated_amount`: The amount of the calculated price, or `null` if there isn't a calculated price. +- `is_original_price_price_list`: whether the original price belongs to a price list. As mentioned earlier, if the price list of the calculated price is of type `override`, the original price will be the same as the calculated price. +- `original_amount`: The amount of the original price, or `null` if there isn't an original price. +- `currency_code`: The currency code of the calculated price, or `null` if there isn't a calculated price. +- `calculated_price`: An object containing the calculated price's money amount details and potentially its associated price list. +- `original_price`: An object containing the original price's money amount details and potentially its associated price list. + +The method returns an array of these price objects. + +--- + +## Example + +Consider the following rule types and price sets: + +```ts +const ruleTypes = await pricingService.createRuleTypes([ + { + name: "Region", + rule_attribute: "region_id", + }, + { + name: "City", + rule_attribute: "city", + }, +]) + +const priceSet = await service.create({ + rules: [ + { rule_attribute: "region_id" }, + { rule_attribute: "city" }, + ], + prices: [ + //default + { + amount: 500, + currency_code: "EUR", + rules: {}, + }, + // prices with rules + { + amount: 400, + currency_code: "EUR", + rules: { + region_id: "PL", + }, + }, + { + amount: 450, + currency_code: "EUR", + rules: { + city: "krakow", + }, + }, + { + amount: 500, + currency_code: "EUR", + rules: { + city: "warsaw", + region_id: "PL", + }, + }, + ], +}) +``` + +### Default Price Selection + +<Tabs groupId="pricing-calculation-example"> + <TabItem value="code" label="Code" default> + + ```ts + const price = await pricingService.calculatePrices( + { id: [priceSet.id] }, + { + context: { + currency_code: "EUR" + } + } + ) + ``` + + </TabItem> + <TabItem value="result" label="Result"> + + The returned price is: + + ```ts + const price = { + id: "<PRICE_SET_ID>", + is_calculated_price_price_list: false, + calculated_amount: 500, + + is_original_price_price_list: false, + original_amount: 500, + + currency_code: "EUR", + + calculated_price: { + money_amount_id: "<DEFAULT_MONEY_AMOUNT_ID>", + price_list_id: null, + price_list_type: null, + min_quantity: null, + max_quantity: null, + }, + + original_price: { + money_amount_id: "<DEFAULT_MONEY_AMOUNT_ID>", + price_list_id: null, + price_list_type: null, + min_quantity: null, + max_quantity: null, + }, + } + ``` + + - Original price selection: since there are no provided rules in the context, the original price is the default money amount. + - Calculated price selection: since there are no associated price lists, the calculated price is set to the original price. + + </TabItem> +</Tabs> + +### Exact Match Rule in Context + +<Tabs> + <TabItem value="code" label="Code" default> + + ```ts + const price = await pricingService.calculatePrices( + { id: [priceSet.id] }, + { + context: { + currency_code: "EUR", + region_id: "PL" + } + } + ) + ``` + + </TabItem> + <TabItem value="result" label="Result"> + + The returned price is: + + ```ts + const price = { + id: "<PRICE_SET_ID>", + is_calculated_price_price_list: false, + calculated_amount: 400, + + is_original_price_price_list: false, + original_amount: 400, + + currency_code: "EUR", + + calculated_price: { + money_amount_id: "<SECOND_MONEY_AMOUNT_ID>", + price_list_id: null, + price_list_type: null, + min_quantity: null, + max_quantity: null, + }, + + original_price: { + money_amount_id: "<SECOND_MONEY_AMOUNT_ID>", + price_list_id: null, + price_list_type: null, + min_quantity: null, + max_quantity: null, + }, + } + ``` + + - Original price selection: Since the second money amount of the price set has the same context as the provided one, it's selected as the original price. + - Calculated price selection: since there are no associated price lists, the calculated price is set to the original price. + + </TabItem> +</Tabs> + +### Context without Exact Matching Rules + +<Tabs> + <TabItem value="code" label="Code" default> + + ```ts + const price = await pricingService.calculatePrices( + { id: [priceSet.id] }, + { + context: { + currency_code: "EUR", + region_id: "PL", + city: "krakow" + } + } + ) + ``` + + </TabItem> + <TabItem value="result" label="Result"> + + The returned price is: + + ```ts + const price = { + id: "<PRICE_SET_ID>", + is_calculated_price_price_list: false, + calculated_amount: 500, + + is_original_price_price_list: false, + original_amount: 500, + + currency_code: "EUR", + + calculated_price: { + money_amount_id: "<FOURTH_MONEY_AMOUNT_ID>", + price_list_id: null, + price_list_type: null, + min_quantity: null, + max_quantity: null, + }, + + original_price: { + money_amount_id: "<FOURTH_MONEY_AMOUNT_ID>", + price_list_id: null, + price_list_type: null, + min_quantity: null, + max_quantity: null, + }, + } + ``` + + - Original price selection: The fourth money amount in the price list is selected based on the following process: + - There are no money amounts having the same rules as the context. + - Retrieve all money amounts matching some combination of the rules in the context. + - Sort the money amounts in descending order by their number of rules, each rule type's default priority, and the priority of the rule values. + - All rule types, in this case, don't have a priority. So, the sorting depends on the number of rules each money amount has. + - The fourth money amount has two rules, and the second and third have one rule. So, the fourth money amount is selected as the original price. + - Calculated price selection: since there are no associated price lists, the calculated price is set to the original price. + + </TabItem> +</Tabs> + +### Price Selection with Price List + +<Tabs> + <TabItem value="code" label="Code" default> + + ```ts + const priceList = pricingModuleService.createPriceList({ + name: "Test Price List", + starts_at: Date.parse("01/10/2023"), + ends_at: Date.parse("31/10/2023"), + rules: { + region_id: ['PL'] + }, + type: "sale" + prices: [ + { + amount: 400, + currency_code: "EUR", + price_set_id: priceSet.id, + }, + { + amount: 450, + currency_code: "EUR", + price_set_id: priceSet.id, + }, + ], + }); + + const price = await pricingService.calculatePrices( + { id: [priceSet.id] }, + { + context: { + currency_code: "EUR", + region_id: "PL", + city: "krakow" + } + } + ) + ``` + + </TabItem> + <TabItem value="result" label="Result"> + + The returned price is: + + ```ts + const price = { + id: "<PRICE_SET_ID>", + is_calculated_price_price_list: true, + calculated_amount: 400, + + is_original_price_price_list: false, + original_amount: 500, + + currency_code: "EUR", + + calculated_price: { + money_amount_id: "<FOURTH_MONEY_AMOUNT_ID>", + price_list_id: null, + price_list_type: null, + min_quantity: null, + max_quantity: null, + }, + + original_price: { + money_amount_id: "<PL_MONEY_AMOUNT_ID_1>", + price_list_id: "<PRICE_LIST_ID>", + price_list_type: "sale", + min_quantity: null, + max_quantity: null, + }, + } + ``` + + - Original price selection: The process is explained in the Context without Exact Matching Rules section. + - Calculated price selection: The first money amount of the price list is selected based on the following process: + - The price set has a price list associated with it. So, retrieve its money amounts and sort them in ascending order by their amount. + - Since the first money amount of the price list has the lowest amount, it's selected as the calculated price. + + </TabItem> +</Tabs> diff --git a/www/apps/docs/content/experimental/product/examples.md b/www/apps/docs/content/experimental/product/examples.md new file mode 100644 index 0000000000000..d62935c12d475 --- /dev/null +++ b/www/apps/docs/content/experimental/product/examples.md @@ -0,0 +1,160 @@ +# Examples of Product Module + +In this document, you’ll find common examples of how you can use the Product module in your application. + +:::note + +Examples in this section are in the context of a Next.js App Router. + +::: + +## Create Product + +```ts +import { NextResponse } from "next/server" + +import { + initialize as initializeProductModule, +} from "@medusajs/product" + +export async function POST(request: Request) { + const productService = await initializeProductModule() + + const products = await productService.create([ + { + title: "Medusa Shirt", + options: [ + { + title: "Color", + }, + ], + variants: [ + { + title: "Black Shirt", + options: [ + { + value: "Black", + }, + ], + }, + ], + }, + ]) + + return NextResponse.json({ products }) +} +``` + +## List Products + +```ts +import { NextResponse } from "next/server" + +import { + initialize as initializeProductModule, +} from "@medusajs/product" + +export async function GET(request: Request) { + const productService = await initializeProductModule() + + const data = await productService.list() + + return NextResponse.json({ products: data }) +} +``` + +## Retrieve a Product by its ID + +```ts +import { NextResponse } from "next/server" + +import { + initialize as initializeProductModule, +} from "@medusajs/product" + +export async function GET( + request: Request, + { params }: { params: Record<string, any> }) { + + const { id } = params + const productService = await initializeProductModule() + + const data = await productService.list({ + id, + }) + + return NextResponse.json({ product: data[0] }) +} +``` + +## Retrieve a Product by its Handle + +```ts +import { NextResponse } from "next/server" + +import { + initialize as initializeProductModule, +} from "@medusajs/product" + +export async function GET( + request: Request, + { params }: { params: Record<string, any> }) { + + const { handle } = params + const productService = await initializeProductModule() + + const data = await productService.list({ + handle, + }) + + return NextResponse.json({ product: data[0] }) +} +``` + +## Retrieve Categories + +```ts +import { NextResponse } from "next/server" + +import { + initialize as initializeProductModule, +} from "@medusajs/product" + +export async function GET(request: Request) { + const productService = await initializeProductModule() + + const data = await productService.listCategories() + + return NextResponse.json({ categories: data }) +} +``` + +## Retrieve Category by Handle + +```ts +import { NextResponse } from "next/server" + +import { + initialize as initializeProductModule, +} from "@medusajs/product" + +export async function GET( + request: Request, + { params }: { params: Record<string, any> }) { + + const { handle } = params + const productService = await initializeProductModule() + + const data = await productService.listCategories({ + handle, + }) + + return NextResponse.json({ category: data[0] }) +} +``` + +--- + +## More Examples + +The [module interface reference](../../references/product/interfaces/IProductModuleService.mdx) provides a reference to all the methods available for use with examples for each. diff --git a/www/apps/docs/content/experimental/product/install-medusa.mdx b/www/apps/docs/content/experimental/product/install-medusa.mdx new file mode 100644 index 0000000000000..aa8e1b068c000 --- /dev/null +++ b/www/apps/docs/content/experimental/product/install-medusa.mdx @@ -0,0 +1,164 @@ +import DocCard from '@theme/DocCard' +import Icons from '@theme/Icon' +import Tabs from '@theme/Tabs'; +import TabItem from '@theme/TabItem'; + +# Install Product Module in Medusa + +In this document, you'll learn how to install the Product module using NPM in the Medusa backend. + +## Step 1: Install Module + +To install the Product module, run the following command in the root directory of the Medusa backend: + +```bash npm2yarn +npm install @medusajs/product +``` + +--- + +## Step 2: Add Module to Configurations + +In `medusa-config.js`, add the product module to the exported object under the `modules` property: + +```js title=medusa-config.js +module.exports = { + // ... + modules: { + // ... + productService: { + resolve: "@medusajs/product", + }, + }, +} +``` + +--- + +## Step 3: Run Migrations + +Run the following command to reflect schema changes into your database: + +```bash +npx medusa migrations run +``` + +--- + +## Use the Module + +You can now start using the module's `ProductModuleService` by resolving it through [dependency injection](../../development/fundamentals/dependency-injection.md). + +For example: + +<Tabs groupId="resource-type" isCodeTabs={true}> + <TabItem value="api-route" label="API Route" attributes={{ + title: "src/api/store/custom/route.ts" + }} default> + + ```ts + import type { + MedusaRequest, + MedusaResponse + } from "@medusajs/medusa"; + import { + ProductModuleService + } from "@medusajs/product" + + export async function GET( + req: MedusaRequest, + res: MedusaResponse + ) { + const productModuleService = req.scope.resolve( + "productModuleService" + ) + + return res.json({ + products: productModuleService.list() + }) + } + ``` + + </TabItem> + <TabItem value="subscribers" label="Subscribers" attributes={{ + title: "src/subscribers/create-customer.ts" + }}> + + ```ts + import { + type SubscriberConfig, + type SubscriberArgs, + ProductService, + } from "@medusajs/medusa" + import { + ProductModuleService + } from "@medusajs/product" + + export default async function handleListProducts({ + data, eventName, container, pluginOptions + }: SubscriberArgs<Customer>) { + const productModuleService = container.resolve( + "productModuleService" + ) + + console.log(await productModuleService.list()) + } + + export const config: SubscriberConfig = { + event: ProductService.Events.CREATED, + context: { + subscriberId: "list-products" + } + } + ``` + + </TabItem> + <TabItem value="service" label="Service" attributes={{ + title: "src/service/hello.ts" + }}> + + ```ts + import { TransactionBaseService } from "@medusajs/medusa" + import { + ProductModuleService + } from "@medusajs/product" + + class HelloService extends TransactionBaseService { + private productModuleService: ProductModuleService + + constructor(container) { + super(container) + this.productModuleService = container.productModuleService + } + + await listProducts() { + return await this.productModuleService.list() + } + } + + export default HelloService + ``` + + </TabItem> +</Tabs> + +:::tip + +In the Examples or API Reference guides, you may see an initialization of the product module. This is only necessary if you're using the module outside the Medusa backend. + +::: + +--- + +## Up Next + +<DocCard item={{ + type: 'link', + href: '/references/product', + label: 'Service Interface Reference', + customProps: { + icon: Icons['academic-cap-solid'], + description: 'Find a full reference of the module\' service interface\s methods.' + } + }} +/> \ No newline at end of file diff --git a/www/apps/docs/content/experimental/product/install-nodejs.md b/www/apps/docs/content/experimental/product/install-nodejs.md new file mode 100644 index 0000000000000..d219d106eab4d --- /dev/null +++ b/www/apps/docs/content/experimental/product/install-nodejs.md @@ -0,0 +1,198 @@ +# Install in Node.js-Based Application + +In this document, you’ll learn how to setup and use the Product module in a Node.js based application. + +## Prerequisites + +Before installing the Product module in your application, make sure you have the following prerequisites: + +- Node.js v16 or greater +- PostgreSQL database. You can use an existing Medusa database, or set up a new PostgreSQL database. + +--- + +## Install Package + +In your Node.js-based applications, such as a Next.js application, you can install the Product module with the following command: + +```bash npm2yarn +npm install @medusajs/product +``` + +--- + +## Add Database Configuration + +Add the following environment variable to your application: + +```bash +POSTGRES_URL=<DATABASE_URL> +``` + +Where `<DATABASE_URL>` is your database connection URL of the format `postgres://[user][:password]@[host][:port]/[dbname]`. You can learn more about the connection URL format in [this guide](https://www.notion.so/development/backend/configurations.md#database_url). + +You can also set the following optional environment variables: + +- `POSTGRES_SCHEMA`: a string indicating the PostgreSQL schema to use. By default, it's `public`. +- `POSTGRES_DRIVER_OPTIONS`: a stringified JSON object indicating the PostgreSQL options to use. The JSON object is then parsed to be used as a JavaScript object. By default, it's `{"connection":{"ssl":false}}` for local PostgreSQL databases, and `{"connection":{"ssl":{"rejectUnauthorized":false}}}` for remote databases. + +:::note + +If `POSTGRES_DRIVER_OPTIONS` is not specified, the PostgreSQL database is considered local if `POSTGRES_URL` includes `localhost`. Otherwise, it's considered remote. + +::: + +### Run Database Migrations + +:::note + +You can skip this step if you use an existing Medusa database. + +::: + +Migrations are used to create your database schema. Before you can run migrations, add in your `package.json` the following scripts: + +```json +"scripts": { + //...other scripts + "product:migrations:run": "medusa-product-migrations-up", + "product:seed": "medusa-product-seed ./seed-data.js" +} +``` + +The first command runs the migrations, and the second command allows you to seed your database with demo products optionally. + +However, you’d need the following seed file added to the root of your project directory: + +<Details> + <Summary>Seed File</Summary> + + ```js + const productCategoriesData = [ + { + id: "category-0", + name: "category 0", + parent_category_id: null, + }, + { + id: "category-1", + name: "category 1", + parent_category_id: "category-0", + }, + { + id: "category-1-a", + name: "category 1 a", + parent_category_id: "category-1", + }, + { + id: "category-1-b", + name: "category 1 b", + parent_category_id: "category-1", + is_internal: true, + }, + { + id: "category-1-b-1", + name: "category 1 b 1", + parent_category_id: "category-1-b", + }, + ] + + const productsData = [ + { + id: "test-1", + title: "product 1", + status: "published", + descriptions: "Lorem ipsum dolor sit amet, consectetur.", + tags: [ + { + id: "tag-1", + value: "France", + }, + ], + categories: [ + { + id: "category-0", + }, + ], + }, + { + id: "test-2", + title: "product", + status: "published", + descriptions: "Lorem ipsum dolor sit amet, consectetur.", + tags: [ + { + id: "tag-2", + value: "Germany", + }, + ], + categories: [ + { + id: "category-1", + }, + ], + }, + ] + + const variantsData = [ + { + id: "test-1", + title: "variant title", + sku: "sku 1", + product: { id: productsData[0].id }, + inventory_quantity: 10, + }, + { + id: "test-2", + title: "variant title", + sku: "sku 2", + product: { id: productsData[1].id }, + inventory_quantity: 10, + }, + ] + + module.exports = { + productCategoriesData, + productsData, + variantsData, + } + ``` + +</Details> + +Then run the commands you added to migrate the database schema and optionally seed data: + +```bash npm2yarn +npm run product:migrations:run +# optionally +npm run product:seed +``` + +--- + +## Next.js Application: Adjust Configurations + +The Product module uses dependencies that aren’t Webpack optimized. Since Next.js uses Webpack for compilation, you need to add the Product module as an external dependency. + +To do that, add the `serverComponentsExternalPackages` option in `next.config.js`: + +```js title=next.config.js +/** @type {import('next').NextConfig} */ +const nextConfig = { + experimental: { + serverComponentsExternalPackages: [ + "@medusajs/product", + ], + }, +} + +module.exports = nextConfig +``` + +--- + +## Start Development + +You can refer to the [Example Usages documentation page](./examples.md) for examples of using the Product module. + +You can also refer to the [Module Interface Reference](../../references/product/interfaces/IProductModuleService.mdx) for a detailed reference on all available methods. diff --git a/www/apps/docs/content/experimental/product/overview.mdx b/www/apps/docs/content/experimental/product/overview.mdx new file mode 100644 index 0000000000000..491c6e473af96 --- /dev/null +++ b/www/apps/docs/content/experimental/product/overview.mdx @@ -0,0 +1,81 @@ +import DocCard from '@theme/DocCard' +import Icons from '@theme/Icon' + +# Product Module Overview + +The Product module is the `@medusajs/product` NPM package that provides product-related features in your Medusa and Node.js applications. It can be used to store products with variants, organize them into categories and collections, and more. + +## Features + +### Products Management + +With the Product module, you can store products and manage them through the main interface methods. Products can have custom options, such as color or size, and each variant in the product sets the value for these options. + +```ts +const products = await productService.create([ + { + title: "Medusa Shirt", + options: [ + { + title: "Color", + }, + ], + variants: [ + { + title: "Black Shirt", + options: [ + { + value: "Black", + }, + ], + }, + ], + }, +]) +``` + +### Product Organizations + +The Product module provides different entities that can be used to organize products, including categories, collections, tags, and more. + +```ts +const category = await productService.createCategory({ + name: "Shirts", +}) + +const products = await productService.update([ + { + id: product.id, + categories: [ + { + id: category.id, + }, + ], + }, +]) +``` + +--- + +## How to Use the Product Module + +The Product Module can be used in many use cases, including: + +- Medusa Backend: The Medusa backend uses the product module to implement some product features. However, it's guarded by the [experimental feature flag](../index.md#enabling-experimental-features). If you want to use the product module in your backend's customizations, follow [this installation guide](./install-medusa.mdx). +- Serverless Application: Use the Product Module in a serverless application, such as a Next.js application, without having to manage a fully-fledged ecommerce system. You can use it by [installing it in your Node.js project as an NPM package](./install-nodejs.md). +- Node.js Application: Use the Product Module in any Node.js application. Follow [this guide](./install-nodejs.md) to learn how to install it. + +--- + +## Up Next + +<DocCard item={{ + type: 'link', + href: '/experimental/product/examples', + label: 'Example Usages', + customProps: { + icon: Icons['academic-cap-solid'], + description: 'Find examples of common usages of the module\'s interface.' + } + }} +/> diff --git a/www/apps/docs/content/modules/products/serverless-module.mdx b/www/apps/docs/content/modules/products/serverless-module.mdx deleted file mode 100644 index 293df98ff206b..0000000000000 --- a/www/apps/docs/content/modules/products/serverless-module.mdx +++ /dev/null @@ -1,386 +0,0 @@ ---- -title: 'How to Use Serverless Product Module' -description: 'Learn how to use the product module in a serverless setup by installing it in a Next.js application.' -addHowToData: true -badge: - variant: orange - text: beta ---- - -In this document, you’ll learn how to use the Product module in a serverless setup. - -## Overview - -Medusa’s modules increase extensibility and customization capabilities. Instead of having to manage a fully-fledged ecommerce system, you can use these modules in serverless applications, such as a Next.js project. - -The module only needs to connect to a PostgreSQL database. You can also connect it to an existing Medusa database. Then, you can use the Product module to connect directly to the PostgreSQL database and retrieve or manipulate data. - -This guide explains how to use the Product Module in a Next.js application as an example. You can use it with other frameworks, such as Remix, as well. - -### Benefits of Serverless Modules - -- Keep packages small enough to be deployed to serverless Infrastructure easily -- Bring Medusa closer to Edge runtime compatibility. -- Make it easier to integrate modules into an ecosystem of existing commerce services. -- Make it easier to customize or extend core logic in Medusa. - -:::info - -The product module is currently in beta, and it provides only functionalities related to browsing products. In later versions, the product module would include more powerful ecommerce features. - -::: - ---- - -## Prerequisites - -- The Product Module requires a project using Node v16+. -- The Product Module must connect to a PostgreSQL database. You can refer to [this guide](../../development/backend/prepare-environment.mdx#postgresql) to learn how to install PostgreSQL locally. Alternatively, you can use free PostgreSQL database hosting, such as [Vercel Postgres](https://vercel.com/docs/storage/vercel-postgres). If you have an existing Medusa database, you can use it as well. - ---- - -## Installation in Next.js Project - -This section explains how to install the Product module in a Next.js project. - -If you don’t have a Next.js project, you can create one with the following command: - -```bash -npx create-next-app@latest -``` - -Refer to the [Next.js documentation](https://nextjs.org/docs/getting-started/installation) for other available installation options. - -### Step 1: Install Product Module - -In the root directory of your Next.js project, run the following command to install the product module: - -```bash npm2yarn -npm install @medusajs/product -``` - -### Step 2: Add Database Configurations - -Create a `.env` file and add the following environment variable: - -```bash -POSTGRES_URL=<DATABASE_URL> -``` - -Where `<DATABASE_URL>` is your database connection URL of the format `postgres://[user][:password]@[host][:port]/[dbname]`. You can learn more about the connection URL format in [this guide](../../development/backend/configurations.md#postgresql-configurations). - -You can also set the following optional environment variables: - -- `POSTGRES_SCHEMA`: a string indicating the PostgreSQL schema to use. By default, it's `public`. -- `POSTGRES_DRIVER_OPTIONS`: a stringified JSON object indicating the PostgreSQL options to use. The JSON object is then parsed to be used as a JavaScript object. By default, it's `{"connection":{"ssl":false}}` for local PostgreSQL databases, and `{"connection":{"ssl":{"rejectUnauthorized":false}}}` for remote databases. - -:::note - -If `POSTGRES_DRIVER_OPTIONS` is not specified, the PostgreSQL database is considered local if `POSTGRES_URL` includes `localhost`. Otherwise, it's considered remote. - -::: - -### Step 3: Run Database Migrations - -:::note - -If you are using an existing Medusa database, you can skip this step. - -::: - -Migrations are used to create your database schema. Before you can run migrations, add in your `package.json` the following scripts: - -```json title="package.json" -"scripts": { - //...other scripts - "product:migrations:run": "medusa-product-migrations-up", - "product:seed": "medusa-product-seed ./seed-data.js" -}, -``` - -The first command runs the migrations, and the second command allows you to optionally seed your database with demo products. However, you’d need the following seed file added in the root of your Next.js directory: - -<Details> - <Summary>Seed file</Summary> - - ```js title="seed-data.js" - const productCategoriesData = [ - { - id: "category-0", - name: "category 0", - parent_category_id: null, - }, - { - id: "category-1", - name: "category 1", - parent_category_id: "category-0", - }, - { - id: "category-1-a", - name: "category 1 a", - parent_category_id: "category-1", - }, - { - id: "category-1-b", - name: "category 1 b", - parent_category_id: "category-1", - is_internal: true, - }, - { - id: "category-1-b-1", - name: "category 1 b 1", - parent_category_id: "category-1-b", - }, - ] - - const productsData = [ - { - id: "test-1", - title: "product 1", - status: "published", - descriptions: "Lorem ipsum dolor sit amet, consectetur.", - tags: [ - { - id: "tag-1", - value: "France", - }, - ], - categories: [ - { - id: "category-0", - }, - ], - }, - { - id: "test-2", - title: "product", - status: "published", - descriptions: "Lorem ipsum dolor sit amet, consectetur.", - tags: [ - { - id: "tag-2", - value: "Germany", - }, - ], - categories: [ - { - id: "category-1", - }, - ], - }, - ] - - const variantsData = [ - { - id: "test-1", - title: "variant title", - sku: "sku 1", - product: { id: productsData[0].id }, - inventory_quantity: 10, - }, - { - id: "test-2", - title: "variant title", - sku: "sku 2", - product: { id: productsData[1].id }, - inventory_quantity: 10, - }, - ] - - module.exports = { - productCategoriesData, - productsData, - variantsData, - } - ``` - -</Details> - -Then run the first and optionally second commands to migrate the database schema: - -```bash npm2yarn -npm run product:migrations:run -# optionally -npm run product:seed -``` - -### Step 4: Adjust Next.js Configurations - -Next.js uses Webpack for compilation. Since quite a few of the dependencies used by the product module are not Webpack optimized, you have to add the product module as an external dependency. - -To do that, add the `serverComponentsExternalPackages` option in `next.config.js`: - -```js title="next.config.js" -/** @type {import('next').NextConfig} */ -const nextConfig = { - experimental: { - serverComponentsExternalPackages: [ - "@medusajs/product", - ], - }, -} - -module.exports = nextConfig -``` - -### Step 5: Create API Route - -The product module is ready for use now! You can now use it to create API routes within your Next.js application. - -:::note - -This guide uses Next.js's App Router. - -::: - -For example, create the file `app/api/products/route.ts` with the following content: - -```ts title="app/api/products/route.ts" -import { NextResponse } from "next/server" - -import { - initialize as initializeProductModule, -} from "@medusajs/product" - -export async function GET(request: Request) { - const productService = await initializeProductModule() - - const data = await productService.list() - - return NextResponse.json({ products: data }) -} -``` - -This creates a `GET` API route at the path `/api/products`. You import the `initialize` function, aliased as `initializeProductModule`, from `@medusajs/product`. Then, in the API route, you invoke the `initializeProductModule` function, which returns an instance of the `ProductModuleService`. Using the product module service’s `list` method, you retrieve all available products and return them in the response. - -### Step 6: Test Next.js Application - -To test the API route you added, start your Next.js application with the following command: - -```bash npm2yarn -npm run dev -``` - -Then, open in your browser the URL `http://localhost:3000/api/products`. If you seeded your database with demo products, or you’re using a Medusa database schema, you’ll receive the products in your database. Otherwise, the request will return an empty array. - ---- - -## Example Usages - -This section includes some examples of the different functionalities or ways you can use the product module. The code snippets are shown in the context of API routes. - -### List Products - -```ts title="app/api/products/route.ts" -import { NextResponse } from "next/server" - -import { - initialize as initializeProductModule, -} from "@medusajs/product" - -export async function GET(request: Request) { - const productService = await initializeProductModule() - - const data = await productService.list() - - return NextResponse.json({ products: data }) -} -``` - -### Retrieve Product by Id - -```ts title="app/api/product/[id]/route.ts" -import { NextResponse } from "next/server" - -import { - initialize as initializeProductModule, -} from "@medusajs/product" - -export async function GET( - request: Request, - { params }: { params: Record<string, any> }) { - - const { id } = params - const productService = await initializeProductModule() - - const data = await productService.list({ - id, - }) - - return NextResponse.json({ product: data[0] }) -} -``` - -### Retrieve Product by Handle - -```ts title="app/api/product/[handle]/route.ts" -import { NextResponse } from "next/server" - -import { - initialize as initializeProductModule, -} from "@medusajs/product" - -export async function GET( - request: Request, - { params }: { params: Record<string, any> }) { - - const { handle } = params - const productService = await initializeProductModule() - - const data = await productService.list({ - handle, - }) - - return NextResponse.json({ product: data[0] }) -} -``` - -### Retrieve Categories - -```ts title="app/api/categories/route.ts" -import { NextResponse } from "next/server" - -import { - initialize as initializeProductModule, -} from "@medusajs/product" - -export async function GET(request: Request) { - const productService = await initializeProductModule() - - const data = await productService.listCategories() - - return NextResponse.json({ categories: data }) -} -``` - -### Retrieve Category by Handle - -```ts title="app/api/category/[handle]/route.ts" -import { NextResponse } from "next/server" - -import { - initialize as initializeProductModule, -} from "@medusajs/product" - -export async function GET( - request: Request, - { params }: { params: Record<string, any> }) { - - const { handle } = params - const productService = await initializeProductModule() - - const data = await productService.listCategories({ - handle, - }) - - return NextResponse.json({ category: data[0] }) -} -``` - ---- - -## See Also - -- [Products Commerce Module Overview](./overview.mdx) -- [How to show products in a storefront](./storefront/show-products.mdx) -- [How to show categories in a storefront](./storefront/use-categories.mdx) diff --git a/www/apps/docs/content/modules/products/storefront/show-products.mdx b/www/apps/docs/content/modules/products/storefront/show-products.mdx index 585e2c244281c..f3c05530a67a4 100644 --- a/www/apps/docs/content/modules/products/storefront/show-products.mdx +++ b/www/apps/docs/content/modules/products/storefront/show-products.mdx @@ -50,7 +50,7 @@ If you follow the Medusa React code blocks, it's assumed you already have [Medus This guide also includes code snippets to utilize the `@medusajs/product` module in your storefront, among other methods. -If you follow the `@medusajs/product` code blocks, it's assumed you already have the [@medusajs/product](../serverless-module.mdx) installed. +If you follow the `@medusajs/product` code blocks, it's assumed you already have the [@medusajs/product](../../../experimental/product/install-nodejs.md) installed. --- diff --git a/www/apps/docs/content/modules/products/storefront/use-categories.mdx b/www/apps/docs/content/modules/products/storefront/use-categories.mdx index a866231bbafd5..e7d941258a380 100644 --- a/www/apps/docs/content/modules/products/storefront/use-categories.mdx +++ b/www/apps/docs/content/modules/products/storefront/use-categories.mdx @@ -48,7 +48,7 @@ If you follow the Medusa React code blocks, it's assumed you already have [Medus This guide also includes code snippets to utilize the `@medusajs/product` module in your storefront, among other methods. -If you follow the `@medusajs/product` code blocks, it's assumed you already have the [@medusajs/product](../serverless-module.mdx) installed. +If you follow the `@medusajs/product` code blocks, it's assumed you already have the [@medusajs/product](../../../experimental/product/install-nodejs.md) installed. --- diff --git a/www/apps/docs/content/references/workflows/.nojekyll b/www/apps/docs/content/references/workflows/.nojekyll new file mode 100644 index 0000000000000..e2ac6616addc2 --- /dev/null +++ b/www/apps/docs/content/references/workflows/.nojekyll @@ -0,0 +1 @@ +TypeDoc added this file to prevent GitHub Pages from using Jekyll. You can turn off this behavior by setting the `githubPages` option to false. \ No newline at end of file diff --git a/www/apps/docs/content/references/workflows/DistributedTransaction/methods/DistributedTransaction.addError.mdx b/www/apps/docs/content/references/workflows/DistributedTransaction/methods/DistributedTransaction.addError.mdx new file mode 100644 index 0000000000000..a4e5b7d9cf12c --- /dev/null +++ b/www/apps/docs/content/references/workflows/DistributedTransaction/methods/DistributedTransaction.addError.mdx @@ -0,0 +1,74 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# addError + +[DistributedTransaction](../../classes/DistributedTransaction.mdx).addError + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "action", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "handlerType", + "type": "[TransactionHandlerType](../../enums/TransactionHandlerType.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "COMPENSATE", + "type": "`\"compensate\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "INVOKE", + "type": "`\"invoke\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "error", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "void", + "type": "`void`", + "optional": true, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/DistributedTransaction/methods/DistributedTransaction.addResponse.mdx b/www/apps/docs/content/references/workflows/DistributedTransaction/methods/DistributedTransaction.addResponse.mdx new file mode 100644 index 0000000000000..2a9c14a430589 --- /dev/null +++ b/www/apps/docs/content/references/workflows/DistributedTransaction/methods/DistributedTransaction.addResponse.mdx @@ -0,0 +1,74 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# addResponse + +[DistributedTransaction](../../classes/DistributedTransaction.mdx).addResponse + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "action", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "handlerType", + "type": "[TransactionHandlerType](../../enums/TransactionHandlerType.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "COMPENSATE", + "type": "`\"compensate\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "INVOKE", + "type": "`\"invoke\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "response", + "type": "`unknown`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "void", + "type": "`void`", + "optional": true, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/DistributedTransaction/methods/DistributedTransaction.canInvoke.mdx b/www/apps/docs/content/references/workflows/DistributedTransaction/methods/DistributedTransaction.canInvoke.mdx new file mode 100644 index 0000000000000..deeec576dae45 --- /dev/null +++ b/www/apps/docs/content/references/workflows/DistributedTransaction/methods/DistributedTransaction.canInvoke.mdx @@ -0,0 +1,23 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# canInvoke + +[DistributedTransaction](../../classes/DistributedTransaction.mdx).canInvoke + +## Returns + +<ParameterTypes parameters={[ + { + "name": "boolean", + "type": "`boolean`", + "optional": true, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/DistributedTransaction/methods/DistributedTransaction.canRevert.mdx b/www/apps/docs/content/references/workflows/DistributedTransaction/methods/DistributedTransaction.canRevert.mdx new file mode 100644 index 0000000000000..412f94ef98d52 --- /dev/null +++ b/www/apps/docs/content/references/workflows/DistributedTransaction/methods/DistributedTransaction.canRevert.mdx @@ -0,0 +1,23 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# canRevert + +[DistributedTransaction](../../classes/DistributedTransaction.mdx).canRevert + +## Returns + +<ParameterTypes parameters={[ + { + "name": "boolean", + "type": "`boolean`", + "optional": true, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/DistributedTransaction/methods/DistributedTransaction.deleteCheckpoint.mdx b/www/apps/docs/content/references/workflows/DistributedTransaction/methods/DistributedTransaction.deleteCheckpoint.mdx new file mode 100644 index 0000000000000..42f6783b2be22 --- /dev/null +++ b/www/apps/docs/content/references/workflows/DistributedTransaction/methods/DistributedTransaction.deleteCheckpoint.mdx @@ -0,0 +1,23 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# deleteCheckpoint + +[DistributedTransaction](../../classes/DistributedTransaction.mdx).deleteCheckpoint + +## Returns + +<ParameterTypes parameters={[ + { + "name": "Promise", + "type": "Promise<void>", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/DistributedTransaction/methods/DistributedTransaction.getContext.mdx b/www/apps/docs/content/references/workflows/DistributedTransaction/methods/DistributedTransaction.getContext.mdx new file mode 100644 index 0000000000000..1ffa291d2b8b3 --- /dev/null +++ b/www/apps/docs/content/references/workflows/DistributedTransaction/methods/DistributedTransaction.getContext.mdx @@ -0,0 +1,23 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# getContext + +[DistributedTransaction](../../classes/DistributedTransaction.mdx).getContext + +## Returns + +<ParameterTypes parameters={[ + { + "name": "TransactionContext", + "type": "[TransactionContext](../../classes/TransactionContext.mdx)", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/DistributedTransaction/methods/DistributedTransaction.getErrors.mdx b/www/apps/docs/content/references/workflows/DistributedTransaction/methods/DistributedTransaction.getErrors.mdx new file mode 100644 index 0000000000000..f4db7f1ce7c98 --- /dev/null +++ b/www/apps/docs/content/references/workflows/DistributedTransaction/methods/DistributedTransaction.getErrors.mdx @@ -0,0 +1,66 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# getErrors + +[DistributedTransaction](../../classes/DistributedTransaction.mdx).getErrors + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "handlerType", + "type": "[TransactionHandlerType](../../enums/TransactionHandlerType.mdx)", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "COMPENSATE", + "type": "`\"compensate\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "INVOKE", + "type": "`\"invoke\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "TransactionStepError[]", + "type": "[TransactionStepError](../../classes/TransactionStepError.mdx)[]", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [ + { + "name": "TransactionStepError", + "type": "[TransactionStepError](../../classes/TransactionStepError.mdx)", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } + ] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/DistributedTransaction/methods/DistributedTransaction.getFlow.mdx b/www/apps/docs/content/references/workflows/DistributedTransaction/methods/DistributedTransaction.getFlow.mdx new file mode 100644 index 0000000000000..faa11612a73fd --- /dev/null +++ b/www/apps/docs/content/references/workflows/DistributedTransaction/methods/DistributedTransaction.getFlow.mdx @@ -0,0 +1,87 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# getFlow + +[DistributedTransaction](../../classes/DistributedTransaction.mdx).getFlow + +## Returns + +<ParameterTypes parameters={[ + { + "name": "TransactionFlow", + "type": "`object`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "definition", + "type": "[TransactionStepsDefinition](../../types/TransactionStepsDefinition.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "hasFailedSteps", + "type": "`boolean`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "hasSkippedSteps", + "type": "`boolean`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "modelId", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "state", + "type": "[TransactionState](../../enums/TransactionState.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "steps", + "type": "`object`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "transactionId", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/DistributedTransaction/methods/DistributedTransaction.getState.mdx b/www/apps/docs/content/references/workflows/DistributedTransaction/methods/DistributedTransaction.getState.mdx new file mode 100644 index 0000000000000..73b1cb6ea333e --- /dev/null +++ b/www/apps/docs/content/references/workflows/DistributedTransaction/methods/DistributedTransaction.getState.mdx @@ -0,0 +1,95 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# getState + +[DistributedTransaction](../../classes/DistributedTransaction.mdx).getState + +## Returns + +<ParameterTypes parameters={[ + { + "name": "COMPENSATING", + "type": "`\"compensating\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "DONE", + "type": "`\"done\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "DORMANT", + "type": "`\"dormant\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "FAILED", + "type": "`\"failed\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "INVOKING", + "type": "`\"invoking\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "NOT_STARTED", + "type": "`\"not_started\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "REVERTED", + "type": "`\"reverted\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "SKIPPED", + "type": "`\"skipped\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "WAITING_TO_COMPENSATE", + "type": "`\"waiting_to_compensate\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/DistributedTransaction/methods/DistributedTransaction.hasFinished.mdx b/www/apps/docs/content/references/workflows/DistributedTransaction/methods/DistributedTransaction.hasFinished.mdx new file mode 100644 index 0000000000000..6be1a5de9f4bd --- /dev/null +++ b/www/apps/docs/content/references/workflows/DistributedTransaction/methods/DistributedTransaction.hasFinished.mdx @@ -0,0 +1,23 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# hasFinished + +[DistributedTransaction](../../classes/DistributedTransaction.mdx).hasFinished + +## Returns + +<ParameterTypes parameters={[ + { + "name": "boolean", + "type": "`boolean`", + "optional": true, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/DistributedTransaction/methods/DistributedTransaction.loadTransaction.mdx b/www/apps/docs/content/references/workflows/DistributedTransaction/methods/DistributedTransaction.loadTransaction.mdx new file mode 100644 index 0000000000000..d5790ff2c2464 --- /dev/null +++ b/www/apps/docs/content/references/workflows/DistributedTransaction/methods/DistributedTransaction.loadTransaction.mdx @@ -0,0 +1,47 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# loadTransaction + +[DistributedTransaction](../../classes/DistributedTransaction.mdx).loadTransaction + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "transactionId", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "Promise", + "type": "Promise<null \\| [TransactionCheckpoint](../../classes/TransactionCheckpoint.mdx)>", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [ + { + "name": "null \\| TransactionCheckpoint", + "type": "`null` \\| [TransactionCheckpoint](../../classes/TransactionCheckpoint.mdx)", + "optional": true, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } + ] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/DistributedTransaction/methods/DistributedTransaction.saveCheckpoint.mdx b/www/apps/docs/content/references/workflows/DistributedTransaction/methods/DistributedTransaction.saveCheckpoint.mdx new file mode 100644 index 0000000000000..af4244df2e6a0 --- /dev/null +++ b/www/apps/docs/content/references/workflows/DistributedTransaction/methods/DistributedTransaction.saveCheckpoint.mdx @@ -0,0 +1,33 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# saveCheckpoint + +[DistributedTransaction](../../classes/DistributedTransaction.mdx).saveCheckpoint + +## Returns + +<ParameterTypes parameters={[ + { + "name": "Promise", + "type": "Promise<[TransactionCheckpoint](../../classes/TransactionCheckpoint.mdx)>", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [ + { + "name": "TransactionCheckpoint", + "type": "[TransactionCheckpoint](../../classes/TransactionCheckpoint.mdx)", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } + ] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/LocalWorkflow/methods/LocalWorkflow.addAction.mdx b/www/apps/docs/content/references/workflows/LocalWorkflow/methods/LocalWorkflow.addAction.mdx new file mode 100644 index 0000000000000..97b3a6f2c10f4 --- /dev/null +++ b/www/apps/docs/content/references/workflows/LocalWorkflow/methods/LocalWorkflow.addAction.mdx @@ -0,0 +1,74 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# addAction + +[LocalWorkflow](../../classes/LocalWorkflow.mdx).addAction + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "action", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "handler", + "type": "[StepHandler](../../types/StepHandler.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "compensate", + "type": "[WorkflowStepHandler](../../types/WorkflowStepHandler.mdx)", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "invoke", + "type": "[WorkflowStepHandler](../../types/WorkflowStepHandler.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "options", + "type": "Partial<[TransactionStepsDefinition](../../types/TransactionStepsDefinition.mdx)>", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "OrchestratorBuilder", + "type": "[OrchestratorBuilder](../../classes/OrchestratorBuilder.mdx)", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/LocalWorkflow/methods/LocalWorkflow.appendAction.mdx b/www/apps/docs/content/references/workflows/LocalWorkflow/methods/LocalWorkflow.appendAction.mdx new file mode 100644 index 0000000000000..83222b37c3a2a --- /dev/null +++ b/www/apps/docs/content/references/workflows/LocalWorkflow/methods/LocalWorkflow.appendAction.mdx @@ -0,0 +1,83 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# appendAction + +[LocalWorkflow](../../classes/LocalWorkflow.mdx).appendAction + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "action", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "to", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "handler", + "type": "[StepHandler](../../types/StepHandler.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "compensate", + "type": "[WorkflowStepHandler](../../types/WorkflowStepHandler.mdx)", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "invoke", + "type": "[WorkflowStepHandler](../../types/WorkflowStepHandler.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "options", + "type": "Partial<[TransactionStepsDefinition](../../types/TransactionStepsDefinition.mdx)>", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "OrchestratorBuilder", + "type": "[OrchestratorBuilder](../../classes/OrchestratorBuilder.mdx)", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/LocalWorkflow/methods/LocalWorkflow.assertHandler.mdx b/www/apps/docs/content/references/workflows/LocalWorkflow/methods/LocalWorkflow.assertHandler.mdx new file mode 100644 index 0000000000000..fd88aef04b559 --- /dev/null +++ b/www/apps/docs/content/references/workflows/LocalWorkflow/methods/LocalWorkflow.assertHandler.mdx @@ -0,0 +1,65 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# assertHandler + +[LocalWorkflow](../../classes/LocalWorkflow.mdx).assertHandler + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "handler", + "type": "[StepHandler](../../types/StepHandler.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "compensate", + "type": "[WorkflowStepHandler](../../types/WorkflowStepHandler.mdx)", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "invoke", + "type": "[WorkflowStepHandler](../../types/WorkflowStepHandler.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "action", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "void", + "type": "`void`", + "optional": true, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/LocalWorkflow/methods/LocalWorkflow.commit.mdx b/www/apps/docs/content/references/workflows/LocalWorkflow/methods/LocalWorkflow.commit.mdx new file mode 100644 index 0000000000000..3946c9dd5a4bc --- /dev/null +++ b/www/apps/docs/content/references/workflows/LocalWorkflow/methods/LocalWorkflow.commit.mdx @@ -0,0 +1,23 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# commit + +[LocalWorkflow](../../classes/LocalWorkflow.mdx).commit + +## Returns + +<ParameterTypes parameters={[ + { + "name": "void", + "type": "`void`", + "optional": true, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/LocalWorkflow/methods/LocalWorkflow.deleteAction.mdx b/www/apps/docs/content/references/workflows/LocalWorkflow/methods/LocalWorkflow.deleteAction.mdx new file mode 100644 index 0000000000000..c8de48985654d --- /dev/null +++ b/www/apps/docs/content/references/workflows/LocalWorkflow/methods/LocalWorkflow.deleteAction.mdx @@ -0,0 +1,46 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# deleteAction + +[LocalWorkflow](../../classes/LocalWorkflow.mdx).deleteAction + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "action", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "parentSteps", + "type": "`any`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "OrchestratorBuilder", + "type": "[OrchestratorBuilder](../../classes/OrchestratorBuilder.mdx)", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/LocalWorkflow/methods/LocalWorkflow.insertActionAfter.mdx b/www/apps/docs/content/references/workflows/LocalWorkflow/methods/LocalWorkflow.insertActionAfter.mdx new file mode 100644 index 0000000000000..21cefb5193414 --- /dev/null +++ b/www/apps/docs/content/references/workflows/LocalWorkflow/methods/LocalWorkflow.insertActionAfter.mdx @@ -0,0 +1,83 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# insertActionAfter + +[LocalWorkflow](../../classes/LocalWorkflow.mdx).insertActionAfter + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "existingAction", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "action", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "handler", + "type": "[StepHandler](../../types/StepHandler.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "compensate", + "type": "[WorkflowStepHandler](../../types/WorkflowStepHandler.mdx)", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "invoke", + "type": "[WorkflowStepHandler](../../types/WorkflowStepHandler.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "options", + "type": "Partial<[TransactionStepsDefinition](../../types/TransactionStepsDefinition.mdx)>", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "OrchestratorBuilder", + "type": "[OrchestratorBuilder](../../classes/OrchestratorBuilder.mdx)", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/LocalWorkflow/methods/LocalWorkflow.insertActionBefore.mdx b/www/apps/docs/content/references/workflows/LocalWorkflow/methods/LocalWorkflow.insertActionBefore.mdx new file mode 100644 index 0000000000000..3c2ef6aa21440 --- /dev/null +++ b/www/apps/docs/content/references/workflows/LocalWorkflow/methods/LocalWorkflow.insertActionBefore.mdx @@ -0,0 +1,83 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# insertActionBefore + +[LocalWorkflow](../../classes/LocalWorkflow.mdx).insertActionBefore + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "existingAction", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "action", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "handler", + "type": "[StepHandler](../../types/StepHandler.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "compensate", + "type": "[WorkflowStepHandler](../../types/WorkflowStepHandler.mdx)", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "invoke", + "type": "[WorkflowStepHandler](../../types/WorkflowStepHandler.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "options", + "type": "Partial<[TransactionStepsDefinition](../../types/TransactionStepsDefinition.mdx)>", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "OrchestratorBuilder", + "type": "[OrchestratorBuilder](../../classes/OrchestratorBuilder.mdx)", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/LocalWorkflow/methods/LocalWorkflow.mergeActions.mdx b/www/apps/docs/content/references/workflows/LocalWorkflow/methods/LocalWorkflow.mergeActions.mdx new file mode 100644 index 0000000000000..56e1d1016d64d --- /dev/null +++ b/www/apps/docs/content/references/workflows/LocalWorkflow/methods/LocalWorkflow.mergeActions.mdx @@ -0,0 +1,46 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# mergeActions + +[LocalWorkflow](../../classes/LocalWorkflow.mdx).mergeActions + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "where", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "actions", + "type": "`string`[]", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "OrchestratorBuilder", + "type": "[OrchestratorBuilder](../../classes/OrchestratorBuilder.mdx)", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/LocalWorkflow/methods/LocalWorkflow.moveAction.mdx b/www/apps/docs/content/references/workflows/LocalWorkflow/methods/LocalWorkflow.moveAction.mdx new file mode 100644 index 0000000000000..ccba66c37d984 --- /dev/null +++ b/www/apps/docs/content/references/workflows/LocalWorkflow/methods/LocalWorkflow.moveAction.mdx @@ -0,0 +1,46 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# moveAction + +[LocalWorkflow](../../classes/LocalWorkflow.mdx).moveAction + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "actionToMove", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "targetAction", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "OrchestratorBuilder", + "type": "[OrchestratorBuilder](../../classes/OrchestratorBuilder.mdx)", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/LocalWorkflow/methods/LocalWorkflow.moveAndMergeNextAction.mdx b/www/apps/docs/content/references/workflows/LocalWorkflow/methods/LocalWorkflow.moveAndMergeNextAction.mdx new file mode 100644 index 0000000000000..5af8c287d0b25 --- /dev/null +++ b/www/apps/docs/content/references/workflows/LocalWorkflow/methods/LocalWorkflow.moveAndMergeNextAction.mdx @@ -0,0 +1,46 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# moveAndMergeNextAction + +[LocalWorkflow](../../classes/LocalWorkflow.mdx).moveAndMergeNextAction + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "actionToMove", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "targetAction", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "OrchestratorBuilder", + "type": "[OrchestratorBuilder](../../classes/OrchestratorBuilder.mdx)", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/LocalWorkflow/methods/LocalWorkflow.pruneAction.mdx b/www/apps/docs/content/references/workflows/LocalWorkflow/methods/LocalWorkflow.pruneAction.mdx new file mode 100644 index 0000000000000..be8cbd2de2c65 --- /dev/null +++ b/www/apps/docs/content/references/workflows/LocalWorkflow/methods/LocalWorkflow.pruneAction.mdx @@ -0,0 +1,37 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# pruneAction + +[LocalWorkflow](../../classes/LocalWorkflow.mdx).pruneAction + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "action", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "OrchestratorBuilder", + "type": "[OrchestratorBuilder](../../classes/OrchestratorBuilder.mdx)", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/LocalWorkflow/methods/LocalWorkflow.registerStepFailure.mdx b/www/apps/docs/content/references/workflows/LocalWorkflow/methods/LocalWorkflow.registerStepFailure.mdx new file mode 100644 index 0000000000000..c6f6e4e5dd70d --- /dev/null +++ b/www/apps/docs/content/references/workflows/LocalWorkflow/methods/LocalWorkflow.registerStepFailure.mdx @@ -0,0 +1,111 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# registerStepFailure + +[LocalWorkflow](../../classes/LocalWorkflow.mdx).registerStepFailure + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "idempotencyKey", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "error", + "type": "`any`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "context", + "type": "[Context](../../interfaces/Context.mdx)", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "enableNestedTransactions", + "type": "`boolean`", + "description": "A boolean value indicating whether nested transactions are enabled.", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "isolationLevel", + "type": "`string`", + "description": "A string indicating the isolation level of the context. Possible values are `READ UNCOMMITTED`, `READ COMMITTED`, `REPEATABLE READ`, or `SERIALIZABLE`.", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "manager", + "type": "`TManager`", + "description": "An instance of a manager, typically an entity manager, of type `TManager`, which is a typed parameter passed to the context to specify the type of the `manager`.", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "transactionId", + "type": "`string`", + "description": "A string indicating the ID of the current transaction.", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "transactionManager", + "type": "`TManager`", + "description": "An instance of a transaction manager of type `TManager`, which is a typed parameter passed to the context to specify the type of the `transactionManager`.", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "Promise", + "type": "Promise<[DistributedTransaction](../../classes/DistributedTransaction.mdx)>", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [ + { + "name": "DistributedTransaction", + "type": "[DistributedTransaction](../../classes/DistributedTransaction.mdx)", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } + ] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/LocalWorkflow/methods/LocalWorkflow.registerStepSuccess.mdx b/www/apps/docs/content/references/workflows/LocalWorkflow/methods/LocalWorkflow.registerStepSuccess.mdx new file mode 100644 index 0000000000000..931dbf43d43dd --- /dev/null +++ b/www/apps/docs/content/references/workflows/LocalWorkflow/methods/LocalWorkflow.registerStepSuccess.mdx @@ -0,0 +1,111 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# registerStepSuccess + +[LocalWorkflow](../../classes/LocalWorkflow.mdx).registerStepSuccess + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "idempotencyKey", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "response", + "type": "`unknown`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "context", + "type": "[Context](../../interfaces/Context.mdx)", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "enableNestedTransactions", + "type": "`boolean`", + "description": "A boolean value indicating whether nested transactions are enabled.", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "isolationLevel", + "type": "`string`", + "description": "A string indicating the isolation level of the context. Possible values are `READ UNCOMMITTED`, `READ COMMITTED`, `REPEATABLE READ`, or `SERIALIZABLE`.", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "manager", + "type": "`TManager`", + "description": "An instance of a manager, typically an entity manager, of type `TManager`, which is a typed parameter passed to the context to specify the type of the `manager`.", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "transactionId", + "type": "`string`", + "description": "A string indicating the ID of the current transaction.", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "transactionManager", + "type": "`TManager`", + "description": "An instance of a transaction manager of type `TManager`, which is a typed parameter passed to the context to specify the type of the `transactionManager`.", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "Promise", + "type": "Promise<[DistributedTransaction](../../classes/DistributedTransaction.mdx)>", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [ + { + "name": "DistributedTransaction", + "type": "[DistributedTransaction](../../classes/DistributedTransaction.mdx)", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } + ] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/LocalWorkflow/methods/LocalWorkflow.replaceAction.mdx b/www/apps/docs/content/references/workflows/LocalWorkflow/methods/LocalWorkflow.replaceAction.mdx new file mode 100644 index 0000000000000..20710a60d8330 --- /dev/null +++ b/www/apps/docs/content/references/workflows/LocalWorkflow/methods/LocalWorkflow.replaceAction.mdx @@ -0,0 +1,83 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# replaceAction + +[LocalWorkflow](../../classes/LocalWorkflow.mdx).replaceAction + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "existingAction", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "action", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "handler", + "type": "[StepHandler](../../types/StepHandler.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "compensate", + "type": "[WorkflowStepHandler](../../types/WorkflowStepHandler.mdx)", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "invoke", + "type": "[WorkflowStepHandler](../../types/WorkflowStepHandler.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "options", + "type": "Partial<[TransactionStepsDefinition](../../types/TransactionStepsDefinition.mdx)>", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "OrchestratorBuilder", + "type": "[OrchestratorBuilder](../../classes/OrchestratorBuilder.mdx)", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/LocalWorkflow/methods/LocalWorkflow.run.mdx b/www/apps/docs/content/references/workflows/LocalWorkflow/methods/LocalWorkflow.run.mdx new file mode 100644 index 0000000000000..541ef256a08fd --- /dev/null +++ b/www/apps/docs/content/references/workflows/LocalWorkflow/methods/LocalWorkflow.run.mdx @@ -0,0 +1,111 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# run + +[LocalWorkflow](../../classes/LocalWorkflow.mdx).run + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "uniqueTransactionId", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "input", + "type": "`unknown`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "context", + "type": "[Context](../../interfaces/Context.mdx)", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "enableNestedTransactions", + "type": "`boolean`", + "description": "A boolean value indicating whether nested transactions are enabled.", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "isolationLevel", + "type": "`string`", + "description": "A string indicating the isolation level of the context. Possible values are `READ UNCOMMITTED`, `READ COMMITTED`, `REPEATABLE READ`, or `SERIALIZABLE`.", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "manager", + "type": "`TManager`", + "description": "An instance of a manager, typically an entity manager, of type `TManager`, which is a typed parameter passed to the context to specify the type of the `manager`.", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "transactionId", + "type": "`string`", + "description": "A string indicating the ID of the current transaction.", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "transactionManager", + "type": "`TManager`", + "description": "An instance of a transaction manager of type `TManager`, which is a typed parameter passed to the context to specify the type of the `transactionManager`.", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "Promise", + "type": "Promise<[DistributedTransaction](../../classes/DistributedTransaction.mdx)>", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [ + { + "name": "DistributedTransaction", + "type": "[DistributedTransaction](../../classes/DistributedTransaction.mdx)", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } + ] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.addAction.mdx b/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.addAction.mdx new file mode 100644 index 0000000000000..4c9c381d07e43 --- /dev/null +++ b/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.addAction.mdx @@ -0,0 +1,46 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# addAction + +[OrchestratorBuilder](../../classes/OrchestratorBuilder.mdx).addAction + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "action", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "options", + "type": "Partial<[TransactionStepsDefinition](../../types/TransactionStepsDefinition.mdx)>", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "OrchestratorBuilder", + "type": "[OrchestratorBuilder](../../classes/OrchestratorBuilder.mdx)", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.appendAction.mdx b/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.appendAction.mdx new file mode 100644 index 0000000000000..e09eff06f2181 --- /dev/null +++ b/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.appendAction.mdx @@ -0,0 +1,55 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# appendAction + +[OrchestratorBuilder](../../classes/OrchestratorBuilder.mdx).appendAction + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "action", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "to", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "options", + "type": "Partial<[TransactionStepsDefinition](../../types/TransactionStepsDefinition.mdx)>", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "OrchestratorBuilder", + "type": "[OrchestratorBuilder](../../classes/OrchestratorBuilder.mdx)", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.appendTo.mdx b/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.appendTo.mdx new file mode 100644 index 0000000000000..e9774b03c804f --- /dev/null +++ b/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.appendTo.mdx @@ -0,0 +1,155 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# appendTo + +[OrchestratorBuilder](../../classes/OrchestratorBuilder.mdx).appendTo + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "step", + "type": "`string` \\| [InternalStep](../../interfaces/InternalStep.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "newStep", + "type": "[InternalStep](../../interfaces/InternalStep.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "action", + "type": "`string`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "async", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "continueOnPermanentFailure", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "depth", + "type": "`number`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "maxRetries", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "next", + "type": "[InternalStep](../../interfaces/InternalStep.mdx) \\| [InternalStep](../../interfaces/InternalStep.mdx)[]", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "noCompensation", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "noWait", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "parent", + "type": "`null` \\| [InternalStep](../../interfaces/InternalStep.mdx)", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "retryInterval", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "saveResponse", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "timeout", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "OrchestratorBuilder", + "type": "[OrchestratorBuilder](../../classes/OrchestratorBuilder.mdx)", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.build.mdx b/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.build.mdx new file mode 100644 index 0000000000000..1d4ed7ec4a559 --- /dev/null +++ b/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.build.mdx @@ -0,0 +1,114 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# build + +[OrchestratorBuilder](../../classes/OrchestratorBuilder.mdx).build + +## Returns + +<ParameterTypes parameters={[ + { + "name": "TransactionStepsDefinition", + "type": "`object`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "action", + "type": "`string`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "async", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "continueOnPermanentFailure", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "maxRetries", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "next", + "type": "[TransactionStepsDefinition](../../types/TransactionStepsDefinition.mdx) \\| [TransactionStepsDefinition](../../types/TransactionStepsDefinition.mdx)[]", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "noCompensation", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "noWait", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "retryInterval", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "saveResponse", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "timeout", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.deleteAction.mdx b/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.deleteAction.mdx new file mode 100644 index 0000000000000..ec4c1e7f902ad --- /dev/null +++ b/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.deleteAction.mdx @@ -0,0 +1,155 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# deleteAction + +[OrchestratorBuilder](../../classes/OrchestratorBuilder.mdx).deleteAction + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "action", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "steps", + "type": "[InternalStep](../../interfaces/InternalStep.mdx)", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "action", + "type": "`string`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "async", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "continueOnPermanentFailure", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "depth", + "type": "`number`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "maxRetries", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "next", + "type": "[InternalStep](../../interfaces/InternalStep.mdx) \\| [InternalStep](../../interfaces/InternalStep.mdx)[]", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "noCompensation", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "noWait", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "parent", + "type": "`null` \\| [InternalStep](../../interfaces/InternalStep.mdx)", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "retryInterval", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "saveResponse", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "timeout", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "OrchestratorBuilder", + "type": "[OrchestratorBuilder](../../classes/OrchestratorBuilder.mdx)", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.findLastStep.mdx b/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.findLastStep.mdx new file mode 100644 index 0000000000000..748b78bb4de21 --- /dev/null +++ b/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.findLastStep.mdx @@ -0,0 +1,146 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# findLastStep + +[OrchestratorBuilder](../../classes/OrchestratorBuilder.mdx).findLastStep + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "steps", + "type": "[InternalStep](../../interfaces/InternalStep.mdx)", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "action", + "type": "`string`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "async", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "continueOnPermanentFailure", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "depth", + "type": "`number`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "maxRetries", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "next", + "type": "[InternalStep](../../interfaces/InternalStep.mdx) \\| [InternalStep](../../interfaces/InternalStep.mdx)[]", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "noCompensation", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "noWait", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "parent", + "type": "`null` \\| [InternalStep](../../interfaces/InternalStep.mdx)", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "retryInterval", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "saveResponse", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "timeout", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "InternalStep", + "type": "[InternalStep](../../interfaces/InternalStep.mdx)", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.findOrThrowStepByAction.mdx b/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.findOrThrowStepByAction.mdx new file mode 100644 index 0000000000000..bb27d9db4a424 --- /dev/null +++ b/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.findOrThrowStepByAction.mdx @@ -0,0 +1,155 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# findOrThrowStepByAction + +[OrchestratorBuilder](../../classes/OrchestratorBuilder.mdx).findOrThrowStepByAction + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "action", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "steps", + "type": "[InternalStep](../../interfaces/InternalStep.mdx)", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "action", + "type": "`string`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "async", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "continueOnPermanentFailure", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "depth", + "type": "`number`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "maxRetries", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "next", + "type": "[InternalStep](../../interfaces/InternalStep.mdx) \\| [InternalStep](../../interfaces/InternalStep.mdx)[]", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "noCompensation", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "noWait", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "parent", + "type": "`null` \\| [InternalStep](../../interfaces/InternalStep.mdx)", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "retryInterval", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "saveResponse", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "timeout", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "InternalStep", + "type": "[InternalStep](../../interfaces/InternalStep.mdx)", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.findParentStepByAction.mdx b/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.findParentStepByAction.mdx new file mode 100644 index 0000000000000..224df93b0a455 --- /dev/null +++ b/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.findParentStepByAction.mdx @@ -0,0 +1,155 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# findParentStepByAction + +[OrchestratorBuilder](../../classes/OrchestratorBuilder.mdx).findParentStepByAction + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "action", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "step", + "type": "[InternalStep](../../interfaces/InternalStep.mdx)", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "action", + "type": "`string`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "async", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "continueOnPermanentFailure", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "depth", + "type": "`number`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "maxRetries", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "next", + "type": "[InternalStep](../../interfaces/InternalStep.mdx) \\| [InternalStep](../../interfaces/InternalStep.mdx)[]", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "noCompensation", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "noWait", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "parent", + "type": "`null` \\| [InternalStep](../../interfaces/InternalStep.mdx)", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "retryInterval", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "saveResponse", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "timeout", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "undefined \\| InternalStep", + "type": "`undefined` \\| [InternalStep](../../interfaces/InternalStep.mdx)", + "optional": true, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.findStepByAction.mdx b/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.findStepByAction.mdx new file mode 100644 index 0000000000000..0c82d36b26433 --- /dev/null +++ b/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.findStepByAction.mdx @@ -0,0 +1,155 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# findStepByAction + +[OrchestratorBuilder](../../classes/OrchestratorBuilder.mdx).findStepByAction + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "action", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "step", + "type": "[InternalStep](../../interfaces/InternalStep.mdx)", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "action", + "type": "`string`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "async", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "continueOnPermanentFailure", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "depth", + "type": "`number`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "maxRetries", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "next", + "type": "[InternalStep](../../interfaces/InternalStep.mdx) \\| [InternalStep](../../interfaces/InternalStep.mdx)[]", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "noCompensation", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "noWait", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "parent", + "type": "`null` \\| [InternalStep](../../interfaces/InternalStep.mdx)", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "retryInterval", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "saveResponse", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "timeout", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "undefined \\| InternalStep", + "type": "`undefined` \\| [InternalStep](../../interfaces/InternalStep.mdx)", + "optional": true, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.insertActionAfter.mdx b/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.insertActionAfter.mdx new file mode 100644 index 0000000000000..209db531aa74e --- /dev/null +++ b/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.insertActionAfter.mdx @@ -0,0 +1,55 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# insertActionAfter + +[OrchestratorBuilder](../../classes/OrchestratorBuilder.mdx).insertActionAfter + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "existingAction", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "action", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "options", + "type": "Partial<[TransactionStepsDefinition](../../types/TransactionStepsDefinition.mdx)>", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "OrchestratorBuilder", + "type": "[OrchestratorBuilder](../../classes/OrchestratorBuilder.mdx)", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.insertActionBefore.mdx b/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.insertActionBefore.mdx new file mode 100644 index 0000000000000..88feeaabe368d --- /dev/null +++ b/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.insertActionBefore.mdx @@ -0,0 +1,55 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# insertActionBefore + +[OrchestratorBuilder](../../classes/OrchestratorBuilder.mdx).insertActionBefore + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "existingAction", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "action", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "options", + "type": "Partial<[TransactionStepsDefinition](../../types/TransactionStepsDefinition.mdx)>", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "OrchestratorBuilder", + "type": "[OrchestratorBuilder](../../classes/OrchestratorBuilder.mdx)", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.load.mdx b/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.load.mdx new file mode 100644 index 0000000000000..f2d70d2fc0d31 --- /dev/null +++ b/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.load.mdx @@ -0,0 +1,128 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# load + +[OrchestratorBuilder](../../classes/OrchestratorBuilder.mdx).load + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "steps", + "type": "[TransactionStepsDefinition](../../types/TransactionStepsDefinition.mdx)", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "action", + "type": "`string`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "async", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "continueOnPermanentFailure", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "maxRetries", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "next", + "type": "[TransactionStepsDefinition](../../types/TransactionStepsDefinition.mdx) \\| [TransactionStepsDefinition](../../types/TransactionStepsDefinition.mdx)[]", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "noCompensation", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "noWait", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "retryInterval", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "saveResponse", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "timeout", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "OrchestratorBuilder", + "type": "[OrchestratorBuilder](../../classes/OrchestratorBuilder.mdx)", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.mergeActions.mdx b/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.mergeActions.mdx new file mode 100644 index 0000000000000..abafbae8bedb0 --- /dev/null +++ b/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.mergeActions.mdx @@ -0,0 +1,46 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# mergeActions + +[OrchestratorBuilder](../../classes/OrchestratorBuilder.mdx).mergeActions + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "where", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "actions", + "type": "`string`[]", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "OrchestratorBuilder", + "type": "[OrchestratorBuilder](../../classes/OrchestratorBuilder.mdx)", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.move.mdx b/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.move.mdx new file mode 100644 index 0000000000000..46ca5e919aab8 --- /dev/null +++ b/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.move.mdx @@ -0,0 +1,92 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# move + +[OrchestratorBuilder](../../classes/OrchestratorBuilder.mdx).move + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "actionToMove", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "targetAction", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "__namedParameters", + "type": "`object`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "mergeNext", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "runInParallel", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "__namedParameters.mergeNext", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "__namedParameters.runInParallel", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "OrchestratorBuilder", + "type": "[OrchestratorBuilder](../../classes/OrchestratorBuilder.mdx)", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.moveAction.mdx b/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.moveAction.mdx new file mode 100644 index 0000000000000..ad5e95347ebdb --- /dev/null +++ b/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.moveAction.mdx @@ -0,0 +1,46 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# moveAction + +[OrchestratorBuilder](../../classes/OrchestratorBuilder.mdx).moveAction + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "actionToMove", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "targetAction", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "OrchestratorBuilder", + "type": "[OrchestratorBuilder](../../classes/OrchestratorBuilder.mdx)", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.moveAndMergeNextAction.mdx b/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.moveAndMergeNextAction.mdx new file mode 100644 index 0000000000000..5e5679fed8b72 --- /dev/null +++ b/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.moveAndMergeNextAction.mdx @@ -0,0 +1,46 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# moveAndMergeNextAction + +[OrchestratorBuilder](../../classes/OrchestratorBuilder.mdx).moveAndMergeNextAction + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "actionToMove", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "targetAction", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "OrchestratorBuilder", + "type": "[OrchestratorBuilder](../../classes/OrchestratorBuilder.mdx)", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.pruneAction.mdx b/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.pruneAction.mdx new file mode 100644 index 0000000000000..d6998c8bf0c9b --- /dev/null +++ b/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.pruneAction.mdx @@ -0,0 +1,37 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# pruneAction + +[OrchestratorBuilder](../../classes/OrchestratorBuilder.mdx).pruneAction + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "action", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "OrchestratorBuilder", + "type": "[OrchestratorBuilder](../../classes/OrchestratorBuilder.mdx)", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.replaceAction.mdx b/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.replaceAction.mdx new file mode 100644 index 0000000000000..bc4bcaf0e9452 --- /dev/null +++ b/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.replaceAction.mdx @@ -0,0 +1,55 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# replaceAction + +[OrchestratorBuilder](../../classes/OrchestratorBuilder.mdx).replaceAction + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "existingAction", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "action", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "options", + "type": "Partial<[TransactionStepsDefinition](../../types/TransactionStepsDefinition.mdx)>", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "OrchestratorBuilder", + "type": "[OrchestratorBuilder](../../classes/OrchestratorBuilder.mdx)", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.updateDepths.mdx b/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.updateDepths.mdx new file mode 100644 index 0000000000000..eb38c04fbe576 --- /dev/null +++ b/www/apps/docs/content/references/workflows/OrchestratorBuilder/methods/OrchestratorBuilder.updateDepths.mdx @@ -0,0 +1,173 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# updateDepths + +[OrchestratorBuilder](../../classes/OrchestratorBuilder.mdx).updateDepths + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "startingStep", + "type": "[InternalStep](../../interfaces/InternalStep.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "action", + "type": "`string`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "async", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "continueOnPermanentFailure", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "depth", + "type": "`number`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "maxRetries", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "next", + "type": "[InternalStep](../../interfaces/InternalStep.mdx) \\| [InternalStep](../../interfaces/InternalStep.mdx)[]", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "noCompensation", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "noWait", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "parent", + "type": "`null` \\| [InternalStep](../../interfaces/InternalStep.mdx)", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "retryInterval", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "saveResponse", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "timeout", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "parent", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "incr", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "beginFrom", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "void", + "type": "`void`", + "optional": true, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/TransactionOrchestrator/methods/TransactionOrchestrator.beginTransaction.mdx b/www/apps/docs/content/references/workflows/TransactionOrchestrator/methods/TransactionOrchestrator.beginTransaction.mdx new file mode 100644 index 0000000000000..686d3cfb8671b --- /dev/null +++ b/www/apps/docs/content/references/workflows/TransactionOrchestrator/methods/TransactionOrchestrator.beginTransaction.mdx @@ -0,0 +1,67 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# beginTransaction + +[TransactionOrchestrator](../../classes/TransactionOrchestrator.mdx).beginTransaction + +Create a new transaction + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "transactionId", + "type": "`string`", + "description": "unique identifier of the transaction", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "handler", + "type": "[TransactionStepHandler](../../types/TransactionStepHandler.mdx)", + "description": "function to handle action of the transaction", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "payload", + "type": "`unknown`", + "description": "payload to be passed to all the transaction steps", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "Promise", + "type": "Promise<[DistributedTransaction](../../classes/DistributedTransaction.mdx)>", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [ + { + "name": "DistributedTransaction", + "type": "[DistributedTransaction](../../classes/DistributedTransaction.mdx)", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } + ] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/TransactionOrchestrator/methods/TransactionOrchestrator.cancelTransaction.mdx b/www/apps/docs/content/references/workflows/TransactionOrchestrator/methods/TransactionOrchestrator.cancelTransaction.mdx new file mode 100644 index 0000000000000..9eb57ace9f159 --- /dev/null +++ b/www/apps/docs/content/references/workflows/TransactionOrchestrator/methods/TransactionOrchestrator.cancelTransaction.mdx @@ -0,0 +1,121 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# cancelTransaction + +[TransactionOrchestrator](../../classes/TransactionOrchestrator.mdx).cancelTransaction + +Cancel and revert a transaction compensating all its executed steps. It can be an ongoing transaction or a completed one + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "transaction", + "type": "[DistributedTransaction](../../classes/DistributedTransaction.mdx)", + "description": "The transaction to be reverted", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "context", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "errors", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "flow", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "handler", + "type": "[TransactionStepHandler](../../types/TransactionStepHandler.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "modelId", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "payload", + "type": "`any`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "transactionId", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "keyPrefix", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "keyValueStore", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "Promise", + "type": "Promise<void>", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/TransactionOrchestrator/methods/TransactionOrchestrator.getKeyName.mdx b/www/apps/docs/content/references/workflows/TransactionOrchestrator/methods/TransactionOrchestrator.getKeyName.mdx new file mode 100644 index 0000000000000..3125beb61af55 --- /dev/null +++ b/www/apps/docs/content/references/workflows/TransactionOrchestrator/methods/TransactionOrchestrator.getKeyName.mdx @@ -0,0 +1,37 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# getKeyName + +[TransactionOrchestrator](../../classes/TransactionOrchestrator.mdx).getKeyName + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "params", + "type": "`string`[]", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "string", + "type": "`string`", + "optional": true, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/TransactionOrchestrator/methods/TransactionOrchestrator.registerStepFailure.mdx b/www/apps/docs/content/references/workflows/TransactionOrchestrator/methods/TransactionOrchestrator.registerStepFailure.mdx new file mode 100644 index 0000000000000..f6cbc0b92051f --- /dev/null +++ b/www/apps/docs/content/references/workflows/TransactionOrchestrator/methods/TransactionOrchestrator.registerStepFailure.mdx @@ -0,0 +1,158 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# registerStepFailure + +[TransactionOrchestrator](../../classes/TransactionOrchestrator.mdx).registerStepFailure + +Register a step failure for a specific transaction and step + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "responseIdempotencyKey", + "type": "`string`", + "description": "The idempotency key for the step", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "error", + "type": "`any`", + "description": "The error that caused the failure", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "handler", + "type": "[TransactionStepHandler](../../types/TransactionStepHandler.mdx)", + "description": "The handler function to execute the step", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "transaction", + "type": "[DistributedTransaction](../../classes/DistributedTransaction.mdx)", + "description": "The current transaction", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "context", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "errors", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "flow", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "handler", + "type": "[TransactionStepHandler](../../types/TransactionStepHandler.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "modelId", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "payload", + "type": "`any`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "transactionId", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "keyPrefix", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "keyValueStore", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "Promise", + "type": "Promise<[DistributedTransaction](../../classes/DistributedTransaction.mdx)>", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [ + { + "name": "DistributedTransaction", + "type": "[DistributedTransaction](../../classes/DistributedTransaction.mdx)", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } + ] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/TransactionOrchestrator/methods/TransactionOrchestrator.registerStepSuccess.mdx b/www/apps/docs/content/references/workflows/TransactionOrchestrator/methods/TransactionOrchestrator.registerStepSuccess.mdx new file mode 100644 index 0000000000000..29bd3b00f1ccc --- /dev/null +++ b/www/apps/docs/content/references/workflows/TransactionOrchestrator/methods/TransactionOrchestrator.registerStepSuccess.mdx @@ -0,0 +1,158 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# registerStepSuccess + +[TransactionOrchestrator](../../classes/TransactionOrchestrator.mdx).registerStepSuccess + +Register a step success for a specific transaction and step + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "responseIdempotencyKey", + "type": "`string`", + "description": "The idempotency key for the step", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "handler", + "type": "[TransactionStepHandler](../../types/TransactionStepHandler.mdx)", + "description": "The handler function to execute the step", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "transaction", + "type": "[DistributedTransaction](../../classes/DistributedTransaction.mdx)", + "description": "The current transaction. If not provided it will be loaded based on the responseIdempotencyKey", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "context", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "errors", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "flow", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "handler", + "type": "[TransactionStepHandler](../../types/TransactionStepHandler.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "modelId", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "payload", + "type": "`any`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "transactionId", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "keyPrefix", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "keyValueStore", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "response", + "type": "`unknown`", + "description": "The response of the step", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "Promise", + "type": "Promise<[DistributedTransaction](../../classes/DistributedTransaction.mdx)>", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [ + { + "name": "DistributedTransaction", + "type": "[DistributedTransaction](../../classes/DistributedTransaction.mdx)", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } + ] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/TransactionOrchestrator/methods/TransactionOrchestrator.resume.mdx b/www/apps/docs/content/references/workflows/TransactionOrchestrator/methods/TransactionOrchestrator.resume.mdx new file mode 100644 index 0000000000000..10981b2e1152e --- /dev/null +++ b/www/apps/docs/content/references/workflows/TransactionOrchestrator/methods/TransactionOrchestrator.resume.mdx @@ -0,0 +1,121 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# resume + +[TransactionOrchestrator](../../classes/TransactionOrchestrator.mdx).resume + +Start a new transaction or resume a transaction that has been previously started + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "transaction", + "type": "[DistributedTransaction](../../classes/DistributedTransaction.mdx)", + "description": "The transaction to resume", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "context", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "errors", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "flow", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "handler", + "type": "[TransactionStepHandler](../../types/TransactionStepHandler.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "modelId", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "payload", + "type": "`any`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "transactionId", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "keyPrefix", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "keyValueStore", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "Promise", + "type": "Promise<void>", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/TransactionStep/methods/TransactionStep.beginCompensation.mdx b/www/apps/docs/content/references/workflows/TransactionStep/methods/TransactionStep.beginCompensation.mdx new file mode 100644 index 0000000000000..f7a02ced461c8 --- /dev/null +++ b/www/apps/docs/content/references/workflows/TransactionStep/methods/TransactionStep.beginCompensation.mdx @@ -0,0 +1,23 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# beginCompensation + +[TransactionStep](../../classes/TransactionStep.mdx).beginCompensation + +## Returns + +<ParameterTypes parameters={[ + { + "name": "void", + "type": "`void`", + "optional": true, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/TransactionStep/methods/TransactionStep.canCompensate.mdx b/www/apps/docs/content/references/workflows/TransactionStep/methods/TransactionStep.canCompensate.mdx new file mode 100644 index 0000000000000..7fa1d04848b6a --- /dev/null +++ b/www/apps/docs/content/references/workflows/TransactionStep/methods/TransactionStep.canCompensate.mdx @@ -0,0 +1,119 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# canCompensate + +[TransactionStep](../../classes/TransactionStep.mdx).canCompensate + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "flowState", + "type": "[TransactionState](../../enums/TransactionState.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "COMPENSATING", + "type": "`\"compensating\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "DONE", + "type": "`\"done\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "DORMANT", + "type": "`\"dormant\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "FAILED", + "type": "`\"failed\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "INVOKING", + "type": "`\"invoking\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "NOT_STARTED", + "type": "`\"not_started\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "REVERTED", + "type": "`\"reverted\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "SKIPPED", + "type": "`\"skipped\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "WAITING_TO_COMPENSATE", + "type": "`\"waiting_to_compensate\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "boolean", + "type": "`boolean`", + "optional": true, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/TransactionStep/methods/TransactionStep.canInvoke.mdx b/www/apps/docs/content/references/workflows/TransactionStep/methods/TransactionStep.canInvoke.mdx new file mode 100644 index 0000000000000..d40d8e6db297c --- /dev/null +++ b/www/apps/docs/content/references/workflows/TransactionStep/methods/TransactionStep.canInvoke.mdx @@ -0,0 +1,119 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# canInvoke + +[TransactionStep](../../classes/TransactionStep.mdx).canInvoke + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "flowState", + "type": "[TransactionState](../../enums/TransactionState.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "COMPENSATING", + "type": "`\"compensating\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "DONE", + "type": "`\"done\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "DORMANT", + "type": "`\"dormant\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "FAILED", + "type": "`\"failed\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "INVOKING", + "type": "`\"invoking\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "NOT_STARTED", + "type": "`\"not_started\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "REVERTED", + "type": "`\"reverted\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "SKIPPED", + "type": "`\"skipped\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "WAITING_TO_COMPENSATE", + "type": "`\"waiting_to_compensate\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "boolean", + "type": "`boolean`", + "optional": true, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/TransactionStep/methods/TransactionStep.canRetry.mdx b/www/apps/docs/content/references/workflows/TransactionStep/methods/TransactionStep.canRetry.mdx new file mode 100644 index 0000000000000..21418f4407dd5 --- /dev/null +++ b/www/apps/docs/content/references/workflows/TransactionStep/methods/TransactionStep.canRetry.mdx @@ -0,0 +1,23 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# canRetry + +[TransactionStep](../../classes/TransactionStep.mdx).canRetry + +## Returns + +<ParameterTypes parameters={[ + { + "name": "boolean", + "type": "`boolean`", + "optional": true, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/TransactionStep/methods/TransactionStep.changeState.mdx b/www/apps/docs/content/references/workflows/TransactionStep/methods/TransactionStep.changeState.mdx new file mode 100644 index 0000000000000..e6fcb45cbf72c --- /dev/null +++ b/www/apps/docs/content/references/workflows/TransactionStep/methods/TransactionStep.changeState.mdx @@ -0,0 +1,119 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# changeState + +[TransactionStep](../../classes/TransactionStep.mdx).changeState + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "toState", + "type": "[TransactionState](../../enums/TransactionState.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "COMPENSATING", + "type": "`\"compensating\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "DONE", + "type": "`\"done\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "DORMANT", + "type": "`\"dormant\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "FAILED", + "type": "`\"failed\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "INVOKING", + "type": "`\"invoking\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "NOT_STARTED", + "type": "`\"not_started\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "REVERTED", + "type": "`\"reverted\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "SKIPPED", + "type": "`\"skipped\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "WAITING_TO_COMPENSATE", + "type": "`\"waiting_to_compensate\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "void", + "type": "`void`", + "optional": true, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/TransactionStep/methods/TransactionStep.changeStatus.mdx b/www/apps/docs/content/references/workflows/TransactionStep/methods/TransactionStep.changeStatus.mdx new file mode 100644 index 0000000000000..909c946d8d53f --- /dev/null +++ b/www/apps/docs/content/references/workflows/TransactionStep/methods/TransactionStep.changeStatus.mdx @@ -0,0 +1,83 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# changeStatus + +[TransactionStep](../../classes/TransactionStep.mdx).changeStatus + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "toStatus", + "type": "[TransactionStepStatus](../../enums/TransactionStepStatus.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "IDLE", + "type": "`\"idle\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "OK", + "type": "`\"ok\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "PERMANENT_FAILURE", + "type": "`\"permanent_failure\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "TEMPORARY_FAILURE", + "type": "`\"temp_failure\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "WAITING", + "type": "`\"waiting_response\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "void", + "type": "`void`", + "optional": true, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/TransactionStep/methods/TransactionStep.getStates.mdx b/www/apps/docs/content/references/workflows/TransactionStep/methods/TransactionStep.getStates.mdx new file mode 100644 index 0000000000000..4a75429986dea --- /dev/null +++ b/www/apps/docs/content/references/workflows/TransactionStep/methods/TransactionStep.getStates.mdx @@ -0,0 +1,172 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# getStates + +[TransactionStep](../../classes/TransactionStep.mdx).getStates + +## Returns + +<ParameterTypes parameters={[ + { + "name": "object", + "type": "`object`", + "optional": true, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> + +<ParameterTypes parameters={[ + { + "name": "state", + "type": "[TransactionState](../../enums/TransactionState.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "COMPENSATING", + "type": "`\"compensating\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "DONE", + "type": "`\"done\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "DORMANT", + "type": "`\"dormant\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "FAILED", + "type": "`\"failed\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "INVOKING", + "type": "`\"invoking\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "NOT_STARTED", + "type": "`\"not_started\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "REVERTED", + "type": "`\"reverted\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "SKIPPED", + "type": "`\"skipped\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "WAITING_TO_COMPENSATE", + "type": "`\"waiting_to_compensate\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "status", + "type": "[TransactionStepStatus](../../enums/TransactionStepStatus.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "IDLE", + "type": "`\"idle\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "OK", + "type": "`\"ok\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "PERMANENT_FAILURE", + "type": "`\"permanent_failure\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "TEMPORARY_FAILURE", + "type": "`\"temp_failure\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "WAITING", + "type": "`\"waiting_response\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/TransactionStep/methods/TransactionStep.isCompensating.mdx b/www/apps/docs/content/references/workflows/TransactionStep/methods/TransactionStep.isCompensating.mdx new file mode 100644 index 0000000000000..7b5dc94f79bf6 --- /dev/null +++ b/www/apps/docs/content/references/workflows/TransactionStep/methods/TransactionStep.isCompensating.mdx @@ -0,0 +1,23 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# isCompensating + +[TransactionStep](../../classes/TransactionStep.mdx).isCompensating + +## Returns + +<ParameterTypes parameters={[ + { + "name": "boolean", + "type": "`boolean`", + "optional": true, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/classes/DistributedTransaction.mdx b/www/apps/docs/content/references/workflows/classes/DistributedTransaction.mdx new file mode 100644 index 0000000000000..26e9939496b7f --- /dev/null +++ b/www/apps/docs/content/references/workflows/classes/DistributedTransaction.mdx @@ -0,0 +1,284 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# DistributedTransaction + +DistributedTransaction represents a distributed transaction, which is a transaction that is composed of multiple steps that are executed in a specific order. + +## Methods + +- [addError](../DistributedTransaction/methods/DistributedTransaction.addError.mdx) +- [addResponse](../DistributedTransaction/methods/DistributedTransaction.addResponse.mdx) +- [canInvoke](../DistributedTransaction/methods/DistributedTransaction.canInvoke.mdx) +- [canRevert](../DistributedTransaction/methods/DistributedTransaction.canRevert.mdx) +- [deleteCheckpoint](../DistributedTransaction/methods/DistributedTransaction.deleteCheckpoint.mdx) +- [getContext](../DistributedTransaction/methods/DistributedTransaction.getContext.mdx) +- [getErrors](../DistributedTransaction/methods/DistributedTransaction.getErrors.mdx) +- [getFlow](../DistributedTransaction/methods/DistributedTransaction.getFlow.mdx) +- [getState](../DistributedTransaction/methods/DistributedTransaction.getState.mdx) +- [hasFinished](../DistributedTransaction/methods/DistributedTransaction.hasFinished.mdx) +- [saveCheckpoint](../DistributedTransaction/methods/DistributedTransaction.saveCheckpoint.mdx) +- [loadTransaction](../DistributedTransaction/methods/DistributedTransaction.loadTransaction.mdx) + +## constructor + +### Parameters + +<ParameterTypes parameters={[ + { + "name": "flow", + "type": "[TransactionFlow](../types/TransactionFlow.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "definition", + "type": "[TransactionStepsDefinition](../types/TransactionStepsDefinition.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "hasFailedSteps", + "type": "`boolean`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "hasSkippedSteps", + "type": "`boolean`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "modelId", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "state", + "type": "[TransactionState](../enums/TransactionState.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "steps", + "type": "`object`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "transactionId", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "handler", + "type": "[TransactionStepHandler](../types/TransactionStepHandler.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "payload", + "type": "`any`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "errors", + "type": "[TransactionStepError](TransactionStepError.mdx)[]", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "action", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "error", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "handlerType", + "type": "[TransactionHandlerType](../enums/TransactionHandlerType.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "context", + "type": "[TransactionContext](TransactionContext.mdx)", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "compensate", + "type": "`Record<string, unknown>`", + "description": "Object containing responses of Compensate handlers on steps flagged with saveResponse.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "invoke", + "type": "`Record<string, unknown>`", + "description": "Object containing responses of Invoke handlers on steps flagged with saveResponse.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "payload", + "type": "`unknown`", + "description": "Object containing the initial payload.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + } +]} /> + +<ParameterTypes parameters={[ + { + "name": "context", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "errors", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "flow", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "handler", + "type": "[TransactionStepHandler](../types/TransactionStepHandler.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "modelId", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "payload", + "type": "`any`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "transactionId", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "keyPrefix", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "keyValueStore", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +## isPartiallyCompleted + + **isPartiallyCompleted**: [object Object] diff --git a/www/apps/docs/content/references/workflows/classes/LocalWorkflow.mdx b/www/apps/docs/content/references/workflows/classes/LocalWorkflow.mdx new file mode 100644 index 0000000000000..1d8daad7bf750 --- /dev/null +++ b/www/apps/docs/content/references/workflows/classes/LocalWorkflow.mdx @@ -0,0 +1,200 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# LocalWorkflow + +## Methods + +- [addAction](../LocalWorkflow/methods/LocalWorkflow.addAction.mdx) +- [appendAction](../LocalWorkflow/methods/LocalWorkflow.appendAction.mdx) +- [assertHandler](../LocalWorkflow/methods/LocalWorkflow.assertHandler.mdx) +- [commit](../LocalWorkflow/methods/LocalWorkflow.commit.mdx) +- [deleteAction](../LocalWorkflow/methods/LocalWorkflow.deleteAction.mdx) +- [insertActionAfter](../LocalWorkflow/methods/LocalWorkflow.insertActionAfter.mdx) +- [insertActionBefore](../LocalWorkflow/methods/LocalWorkflow.insertActionBefore.mdx) +- [mergeActions](../LocalWorkflow/methods/LocalWorkflow.mergeActions.mdx) +- [moveAction](../LocalWorkflow/methods/LocalWorkflow.moveAction.mdx) +- [moveAndMergeNextAction](../LocalWorkflow/methods/LocalWorkflow.moveAndMergeNextAction.mdx) +- [pruneAction](../LocalWorkflow/methods/LocalWorkflow.pruneAction.mdx) +- [registerStepFailure](../LocalWorkflow/methods/LocalWorkflow.registerStepFailure.mdx) +- [registerStepSuccess](../LocalWorkflow/methods/LocalWorkflow.registerStepSuccess.mdx) +- [replaceAction](../LocalWorkflow/methods/LocalWorkflow.replaceAction.mdx) +- [run](../LocalWorkflow/methods/LocalWorkflow.run.mdx) + +## constructor + +### Parameters + +<ParameterTypes parameters={[ + { + "name": "workflowId", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "modulesLoaded", + "type": "[MedusaContainer](../types/MedusaContainer.mdx) \\| ``{ __definition: [ModuleDefinition](../types/ModuleDefinition.mdx) ; __joinerConfig: [ModuleJoinerConfig](../types/ModuleJoinerConfig.mdx) }``[]", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +<ParameterTypes parameters={[ + { + "name": "container", + "type": "[MedusaContainer](../types/MedusaContainer.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "createScope", + "type": "() => [MedusaContainer](../types/MedusaContainer.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "registerAdd", + "type": "`<T>`(`name`: `string`, `registration`: `T`) => [MedusaContainer](../types/MedusaContainer.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "flow", + "type": "[OrchestratorBuilder](OrchestratorBuilder.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "hasChanges_", + "type": "`boolean`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "steps", + "type": "[InternalStep](../interfaces/InternalStep.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "handlers", + "type": "Map<string, [StepHandler](../types/StepHandler.mdx)>", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "workflow", + "type": "[WorkflowDefinition](../interfaces/WorkflowDefinition.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "flow_", + "type": "[TransactionStepsDefinition](../types/TransactionStepsDefinition.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "handler", + "type": "(`container`: [MedusaContainer](../types/MedusaContainer.mdx), `context?`: [Context](../interfaces/Context.mdx)) => [TransactionStepHandler](../types/TransactionStepHandler.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "handlers_", + "type": "Map<string, { compensate?: [WorkflowStepHandler](../types/WorkflowStepHandler.mdx) ; invoke: [WorkflowStepHandler](../types/WorkflowStepHandler.mdx) }>", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "id", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "optionalModules", + "type": "Set<string>", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "orchestrator", + "type": "[TransactionOrchestrator](TransactionOrchestrator.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "requiredModules", + "type": "Set<string>", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "workflowId", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/classes/OrchestratorBuilder.mdx b/www/apps/docs/content/references/workflows/classes/OrchestratorBuilder.mdx new file mode 100644 index 0000000000000..e1e566f971c6a --- /dev/null +++ b/www/apps/docs/content/references/workflows/classes/OrchestratorBuilder.mdx @@ -0,0 +1,270 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# OrchestratorBuilder + +## Methods + +- [addAction](../OrchestratorBuilder/methods/OrchestratorBuilder.addAction.mdx) +- [appendAction](../OrchestratorBuilder/methods/OrchestratorBuilder.appendAction.mdx) +- [appendTo](../OrchestratorBuilder/methods/OrchestratorBuilder.appendTo.mdx) +- [build](../OrchestratorBuilder/methods/OrchestratorBuilder.build.mdx) +- [deleteAction](../OrchestratorBuilder/methods/OrchestratorBuilder.deleteAction.mdx) +- [findLastStep](../OrchestratorBuilder/methods/OrchestratorBuilder.findLastStep.mdx) +- [findOrThrowStepByAction](../OrchestratorBuilder/methods/OrchestratorBuilder.findOrThrowStepByAction.mdx) +- [findParentStepByAction](../OrchestratorBuilder/methods/OrchestratorBuilder.findParentStepByAction.mdx) +- [findStepByAction](../OrchestratorBuilder/methods/OrchestratorBuilder.findStepByAction.mdx) +- [insertActionAfter](../OrchestratorBuilder/methods/OrchestratorBuilder.insertActionAfter.mdx) +- [insertActionBefore](../OrchestratorBuilder/methods/OrchestratorBuilder.insertActionBefore.mdx) +- [load](../OrchestratorBuilder/methods/OrchestratorBuilder.load.mdx) +- [mergeActions](../OrchestratorBuilder/methods/OrchestratorBuilder.mergeActions.mdx) +- [move](../OrchestratorBuilder/methods/OrchestratorBuilder.move.mdx) +- [moveAction](../OrchestratorBuilder/methods/OrchestratorBuilder.moveAction.mdx) +- [moveAndMergeNextAction](../OrchestratorBuilder/methods/OrchestratorBuilder.moveAndMergeNextAction.mdx) +- [pruneAction](../OrchestratorBuilder/methods/OrchestratorBuilder.pruneAction.mdx) +- [replaceAction](../OrchestratorBuilder/methods/OrchestratorBuilder.replaceAction.mdx) +- [updateDepths](../OrchestratorBuilder/methods/OrchestratorBuilder.updateDepths.mdx) + +## constructor + +### Parameters + +<ParameterTypes parameters={[ + { + "name": "steps", + "type": "[TransactionStepsDefinition](../types/TransactionStepsDefinition.mdx)", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "action", + "type": "`string`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "async", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "continueOnPermanentFailure", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "maxRetries", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "next", + "type": "[TransactionStepsDefinition](../types/TransactionStepsDefinition.mdx) \\| [TransactionStepsDefinition](../types/TransactionStepsDefinition.mdx)[]", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "noCompensation", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "noWait", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "retryInterval", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "saveResponse", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "timeout", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + } +]} /> + +<ParameterTypes parameters={[ + { + "name": "hasChanges_", + "type": "`boolean`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "steps", + "type": "[InternalStep](../interfaces/InternalStep.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "action", + "type": "`string`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "async", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "continueOnPermanentFailure", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "depth", + "type": "`number`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "maxRetries", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "next", + "type": "[InternalStep](../interfaces/InternalStep.mdx) \\| [InternalStep](../interfaces/InternalStep.mdx)[]", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "noCompensation", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "noWait", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "parent", + "type": "`null` \\| [InternalStep](../interfaces/InternalStep.mdx)", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "retryInterval", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "saveResponse", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "timeout", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + } +]} /> + +## hasChanges + + **hasChanges**: [object Object] diff --git a/www/apps/docs/content/references/workflows/classes/StepResponse.mdx b/www/apps/docs/content/references/workflows/classes/StepResponse.mdx new file mode 100644 index 0000000000000..080247739bfd2 --- /dev/null +++ b/www/apps/docs/content/references/workflows/classes/StepResponse.mdx @@ -0,0 +1,82 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# StepResponse + +This class is used to create the response returned by a step. A step return its data by returning an instance of `StepResponse`. + +## Type parameters + +<ParameterTypes parameters={[ + { + "name": "TOutput", + "type": "`object`", + "description": "The type of the output of the step.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "TCompensateInput", + "type": "`object`", + "description": "The type of the compensation input. If the step doesn't specify any compensation input, then the type of `TCompensateInput` is the same as that of `TOutput`.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +## constructor + +The constructor of the StepResponse + +### Type Parameters + +<ParameterTypes parameters={[ + { + "name": "TOutput", + "type": "`object`", + "description": "The type of the output of the step.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "TCompensateInput", + "type": "`object`", + "description": "The type of the compensation input. If the step doesn't specify any compensation input, then the type of `TCompensateInput` is the same as that of `TOutput`.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +### Parameters + +<ParameterTypes parameters={[ + { + "name": "output", + "type": "`TOutput`", + "description": "The output of the step.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "compensateInput", + "type": "`TCompensateInput`", + "description": "The input to be passed as a parameter to the step's compensation function. If not provided, the `output` will be provided instead.", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/classes/TransactionCheckpoint.mdx b/www/apps/docs/content/references/workflows/classes/TransactionCheckpoint.mdx new file mode 100644 index 0000000000000..0e4375c749db5 --- /dev/null +++ b/www/apps/docs/content/references/workflows/classes/TransactionCheckpoint.mdx @@ -0,0 +1,311 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# TransactionCheckpoint + +## constructor + +### Parameters + +<ParameterTypes parameters={[ + { + "name": "flow", + "type": "[TransactionFlow](../types/TransactionFlow.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "definition", + "type": "[TransactionStepsDefinition](../types/TransactionStepsDefinition.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "hasFailedSteps", + "type": "`boolean`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "hasSkippedSteps", + "type": "`boolean`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "modelId", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "state", + "type": "[TransactionState](../enums/TransactionState.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "steps", + "type": "`object`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "transactionId", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "context", + "type": "[TransactionContext](TransactionContext.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "compensate", + "type": "`Record<string, unknown>`", + "description": "Object containing responses of Compensate handlers on steps flagged with saveResponse.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "invoke", + "type": "`Record<string, unknown>`", + "description": "Object containing responses of Invoke handlers on steps flagged with saveResponse.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "payload", + "type": "`unknown`", + "description": "Object containing the initial payload.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "errors", + "type": "[TransactionStepError](TransactionStepError.mdx)[]", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "action", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "error", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "handlerType", + "type": "[TransactionHandlerType](../enums/TransactionHandlerType.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + } +]} /> + +<ParameterTypes parameters={[ + { + "name": "context", + "type": "[TransactionContext](TransactionContext.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "compensate", + "type": "`Record<string, unknown>`", + "description": "Object containing responses of Compensate handlers on steps flagged with saveResponse.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "invoke", + "type": "`Record<string, unknown>`", + "description": "Object containing responses of Invoke handlers on steps flagged with saveResponse.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "payload", + "type": "`unknown`", + "description": "Object containing the initial payload.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "errors", + "type": "[TransactionStepError](TransactionStepError.mdx)[]", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "action", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "error", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "handlerType", + "type": "[TransactionHandlerType](../enums/TransactionHandlerType.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "flow", + "type": "[TransactionFlow](../types/TransactionFlow.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "definition", + "type": "[TransactionStepsDefinition](../types/TransactionStepsDefinition.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "hasFailedSteps", + "type": "`boolean`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "hasSkippedSteps", + "type": "`boolean`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "modelId", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "state", + "type": "[TransactionState](../enums/TransactionState.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "steps", + "type": "`object`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "transactionId", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/classes/TransactionContext.mdx b/www/apps/docs/content/references/workflows/classes/TransactionContext.mdx new file mode 100644 index 0000000000000..abdc7cf3f4e7d --- /dev/null +++ b/www/apps/docs/content/references/workflows/classes/TransactionContext.mdx @@ -0,0 +1,71 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# TransactionContext + +## constructor + +### Parameters + +<ParameterTypes parameters={[ + { + "name": "payload", + "type": "`unknown`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "invoke", + "type": "`Record<string, unknown>`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "compensate", + "type": "`Record<string, unknown>`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +<ParameterTypes parameters={[ + { + "name": "compensate", + "type": "`Record<string, unknown>`", + "description": "Object containing responses of Compensate handlers on steps flagged with saveResponse.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "invoke", + "type": "`Record<string, unknown>`", + "description": "Object containing responses of Invoke handlers on steps flagged with saveResponse.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "payload", + "type": "`unknown`", + "description": "Object containing the initial payload.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/classes/TransactionOrchestrator.mdx b/www/apps/docs/content/references/workflows/classes/TransactionOrchestrator.mdx new file mode 100644 index 0000000000000..6d02e2115a5c6 --- /dev/null +++ b/www/apps/docs/content/references/workflows/classes/TransactionOrchestrator.mdx @@ -0,0 +1,342 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# TransactionOrchestrator + +## Methods + +- [beginTransaction](../TransactionOrchestrator/methods/TransactionOrchestrator.beginTransaction.mdx) +- [cancelTransaction](../TransactionOrchestrator/methods/TransactionOrchestrator.cancelTransaction.mdx) +- [registerStepFailure](../TransactionOrchestrator/methods/TransactionOrchestrator.registerStepFailure.mdx) +- [registerStepSuccess](../TransactionOrchestrator/methods/TransactionOrchestrator.registerStepSuccess.mdx) +- [resume](../TransactionOrchestrator/methods/TransactionOrchestrator.resume.mdx) +- [getKeyName](../TransactionOrchestrator/methods/TransactionOrchestrator.getKeyName.mdx) + +## constructor + +### Parameters + +<ParameterTypes parameters={[ + { + "name": "id", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "definition", + "type": "[TransactionStepsDefinition](../types/TransactionStepsDefinition.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "action", + "type": "`string`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "async", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "continueOnPermanentFailure", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "maxRetries", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "next", + "type": "[TransactionStepsDefinition](../types/TransactionStepsDefinition.mdx) \\| [TransactionStepsDefinition](../types/TransactionStepsDefinition.mdx)[]", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "noCompensation", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "noWait", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "retryInterval", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "saveResponse", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "timeout", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + } +]} /> + +<ParameterTypes parameters={[ + { + "name": "canContinue", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "canMoveBackward", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "canMoveForward", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "checkAllSteps", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "compensateSteps", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "createTransactionFlow", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "definition", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "executeNext", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "flagStepsToRevert", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "getCompensationSteps", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "getInvokeSteps", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "getPreviousStep", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "id", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "invokeSteps", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "DEFAULT_RETRIES", + "type": "`number`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "ROOT_STEP", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "SEPARATOR", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "buildSteps", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "getStepByAction", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "getTransactionAndStepFromIdempotencyKey", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "loadTransactionById", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "setStepFailure", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "setStepSuccess", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/classes/TransactionPayload.mdx b/www/apps/docs/content/references/workflows/classes/TransactionPayload.mdx new file mode 100644 index 0000000000000..28626c763fa6a --- /dev/null +++ b/www/apps/docs/content/references/workflows/classes/TransactionPayload.mdx @@ -0,0 +1,255 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# TransactionPayload + +## constructor + +### Parameters + +<ParameterTypes parameters={[ + { + "name": "metadata", + "type": "[TransactionMetadata](../types/TransactionMetadata.mdx)", + "description": "The metadata of the transaction.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "action", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "action_type", + "type": "[TransactionHandlerType](../enums/TransactionHandlerType.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "attempt", + "type": "`number`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "idempotency_key", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "model_id", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "reply_to_topic", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "timestamp", + "type": "`number`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "data", + "type": "`Record<string, unknown>`", + "description": "The initial payload data to begin a transation.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "context", + "type": "[TransactionContext](TransactionContext.mdx)", + "description": "Object gathering responses of all steps flagged with saveResponse.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "compensate", + "type": "`Record<string, unknown>`", + "description": "Object containing responses of Compensate handlers on steps flagged with saveResponse.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "invoke", + "type": "`Record<string, unknown>`", + "description": "Object containing responses of Invoke handlers on steps flagged with saveResponse.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "payload", + "type": "`unknown`", + "description": "Object containing the initial payload.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + } +]} /> + +<ParameterTypes parameters={[ + { + "name": "context", + "type": "[TransactionContext](TransactionContext.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "compensate", + "type": "`Record<string, unknown>`", + "description": "Object containing responses of Compensate handlers on steps flagged with saveResponse.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "invoke", + "type": "`Record<string, unknown>`", + "description": "Object containing responses of Invoke handlers on steps flagged with saveResponse.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "payload", + "type": "`unknown`", + "description": "Object containing the initial payload.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "data", + "type": "`Record<string, unknown>`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "metadata", + "type": "[TransactionMetadata](../types/TransactionMetadata.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "action", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "action_type", + "type": "[TransactionHandlerType](../enums/TransactionHandlerType.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "attempt", + "type": "`number`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "idempotency_key", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "model_id", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "reply_to_topic", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "timestamp", + "type": "`number`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/classes/TransactionStep.mdx b/www/apps/docs/content/references/workflows/classes/TransactionStep.mdx new file mode 100644 index 0000000000000..61812c208e527 --- /dev/null +++ b/www/apps/docs/content/references/workflows/classes/TransactionStep.mdx @@ -0,0 +1,547 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# TransactionStep + +## Classdesc + +A class representing a single step in a transaction flow + +## Methods + +- [beginCompensation](../TransactionStep/methods/TransactionStep.beginCompensation.mdx) +- [canCompensate](../TransactionStep/methods/TransactionStep.canCompensate.mdx) +- [canInvoke](../TransactionStep/methods/TransactionStep.canInvoke.mdx) +- [canRetry](../TransactionStep/methods/TransactionStep.canRetry.mdx) +- [changeState](../TransactionStep/methods/TransactionStep.changeState.mdx) +- [changeStatus](../TransactionStep/methods/TransactionStep.changeStatus.mdx) +- [getStates](../TransactionStep/methods/TransactionStep.getStates.mdx) +- [isCompensating](../TransactionStep/methods/TransactionStep.isCompensating.mdx) + +## constructor + +<ParameterTypes parameters={[ + { + "name": "attempts", + "type": "`number`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "compensate", + "type": "`object`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "state", + "type": "[TransactionState](../enums/TransactionState.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "status", + "type": "[TransactionStepStatus](../enums/TransactionStepStatus.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "compensate.state", + "type": "[TransactionState](../enums/TransactionState.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "COMPENSATING", + "type": "`\"compensating\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "DONE", + "type": "`\"done\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "DORMANT", + "type": "`\"dormant\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "FAILED", + "type": "`\"failed\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "INVOKING", + "type": "`\"invoking\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "NOT_STARTED", + "type": "`\"not_started\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "REVERTED", + "type": "`\"reverted\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "SKIPPED", + "type": "`\"skipped\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "WAITING_TO_COMPENSATE", + "type": "`\"waiting_to_compensate\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "compensate.status", + "type": "[TransactionStepStatus](../enums/TransactionStepStatus.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "IDLE", + "type": "`\"idle\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "OK", + "type": "`\"ok\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "PERMANENT_FAILURE", + "type": "`\"permanent_failure\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "TEMPORARY_FAILURE", + "type": "`\"temp_failure\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "WAITING", + "type": "`\"waiting_response\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "definition", + "type": "[TransactionStepsDefinition](../types/TransactionStepsDefinition.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "action", + "type": "`string`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "async", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "continueOnPermanentFailure", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "maxRetries", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "next", + "type": "[TransactionStepsDefinition](../types/TransactionStepsDefinition.mdx) \\| [TransactionStepsDefinition](../types/TransactionStepsDefinition.mdx)[]", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "noCompensation", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "noWait", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "retryInterval", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "saveResponse", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "timeout", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "depth", + "type": "`number`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "failures", + "type": "`number`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "id", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "invoke", + "type": "`object`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "state", + "type": "[TransactionState](../enums/TransactionState.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "status", + "type": "[TransactionStepStatus](../enums/TransactionStepStatus.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "invoke.state", + "type": "[TransactionState](../enums/TransactionState.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "COMPENSATING", + "type": "`\"compensating\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "DONE", + "type": "`\"done\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "DORMANT", + "type": "`\"dormant\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "FAILED", + "type": "`\"failed\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "INVOKING", + "type": "`\"invoking\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "NOT_STARTED", + "type": "`\"not_started\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "REVERTED", + "type": "`\"reverted\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "SKIPPED", + "type": "`\"skipped\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "WAITING_TO_COMPENSATE", + "type": "`\"waiting_to_compensate\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "invoke.status", + "type": "[TransactionStepStatus](../enums/TransactionStepStatus.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "IDLE", + "type": "`\"idle\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "OK", + "type": "`\"ok\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "PERMANENT_FAILURE", + "type": "`\"permanent_failure\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "TEMPORARY_FAILURE", + "type": "`\"temp_failure\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "WAITING", + "type": "`\"waiting_response\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "lastAttempt", + "type": "`null` \\| `number`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "next", + "type": "`string`[]", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "saveResponse", + "type": "`boolean`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "stepFailed", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/classes/TransactionStepError.mdx b/www/apps/docs/content/references/workflows/classes/TransactionStepError.mdx new file mode 100644 index 0000000000000..dc58f15c553cf --- /dev/null +++ b/www/apps/docs/content/references/workflows/classes/TransactionStepError.mdx @@ -0,0 +1,109 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# TransactionStepError + +## constructor + +### Parameters + +<ParameterTypes parameters={[ + { + "name": "action", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "handlerType", + "type": "[TransactionHandlerType](../enums/TransactionHandlerType.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "COMPENSATE", + "type": "`\"compensate\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "INVOKE", + "type": "`\"invoke\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "error", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +<ParameterTypes parameters={[ + { + "name": "action", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "error", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "handlerType", + "type": "[TransactionHandlerType](../enums/TransactionHandlerType.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "COMPENSATE", + "type": "`\"compensate\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "INVOKE", + "type": "`\"invoke\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/enums/MODULE_RESOURCE_TYPE.mdx b/www/apps/docs/content/references/workflows/enums/MODULE_RESOURCE_TYPE.mdx new file mode 100644 index 0000000000000..3493e66e8cd3f --- /dev/null +++ b/www/apps/docs/content/references/workflows/enums/MODULE_RESOURCE_TYPE.mdx @@ -0,0 +1,17 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# MODULE\_RESOURCE\_TYPE + +## ISOLATED + + **ISOLATED** = `"isolated"` + +___ + +## SHARED + + **SHARED** = `"shared"` diff --git a/www/apps/docs/content/references/workflows/enums/TransactionHandlerType.mdx b/www/apps/docs/content/references/workflows/enums/TransactionHandlerType.mdx new file mode 100644 index 0000000000000..13d3025c028cb --- /dev/null +++ b/www/apps/docs/content/references/workflows/enums/TransactionHandlerType.mdx @@ -0,0 +1,17 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# TransactionHandlerType + +## COMPENSATE + + **COMPENSATE** = `"compensate"` + +___ + +## INVOKE + + **INVOKE** = `"invoke"` diff --git a/www/apps/docs/content/references/workflows/enums/TransactionState.mdx b/www/apps/docs/content/references/workflows/enums/TransactionState.mdx new file mode 100644 index 0000000000000..04241e2fc8191 --- /dev/null +++ b/www/apps/docs/content/references/workflows/enums/TransactionState.mdx @@ -0,0 +1,59 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# TransactionState + +## COMPENSATING + + **COMPENSATING** = `"compensating"` + +___ + +## DONE + + **DONE** = `"done"` + +___ + +## DORMANT + + **DORMANT** = `"dormant"` + +___ + +## FAILED + + **FAILED** = `"failed"` + +___ + +## INVOKING + + **INVOKING** = `"invoking"` + +___ + +## NOT\_STARTED + + **NOT\_STARTED** = `"not_started"` + +___ + +## REVERTED + + **REVERTED** = `"reverted"` + +___ + +## SKIPPED + + **SKIPPED** = `"skipped"` + +___ + +## WAITING\_TO\_COMPENSATE + + **WAITING\_TO\_COMPENSATE** = `"waiting_to_compensate"` diff --git a/www/apps/docs/content/references/workflows/enums/TransactionStepStatus.mdx b/www/apps/docs/content/references/workflows/enums/TransactionStepStatus.mdx new file mode 100644 index 0000000000000..0b79d803095b1 --- /dev/null +++ b/www/apps/docs/content/references/workflows/enums/TransactionStepStatus.mdx @@ -0,0 +1,35 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# TransactionStepStatus + +## IDLE + + **IDLE** = `"idle"` + +___ + +## OK + + **OK** = `"ok"` + +___ + +## PERMANENT\_FAILURE + + **PERMANENT\_FAILURE** = `"permanent_failure"` + +___ + +## TEMPORARY\_FAILURE + + **TEMPORARY\_FAILURE** = `"temp_failure"` + +___ + +## WAITING + + **WAITING** = `"waiting_response"` diff --git a/www/apps/docs/content/references/workflows/functions/createStep.mdx b/www/apps/docs/content/references/workflows/functions/createStep.mdx new file mode 100644 index 0000000000000..a4e91329d9b93 --- /dev/null +++ b/www/apps/docs/content/references/workflows/functions/createStep.mdx @@ -0,0 +1,133 @@ +--- +displayed_sidebar: workflowsSidebar +slug: /references/workflows/createStep +sidebar_label: createStep +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# createStep - Workflows Reference + +This documentation provides a reference to the `createStep` . It belongs to the `@medusajs/workflows-sdk` package. + +This function creates a [StepFunction](../types/StepFunction.mdx) that can be used as a step in a workflow constructed by the [createWorkflow](createWorkflow.mdx) function. + +## Example + +```ts +import { + createStep, + StepResponse, + StepExecutionContext, + WorkflowData +} from "@medusajs/workflows-sdk" + +interface CreateProductInput { + title: string +} + +export const createProductStep = createStep( + "createProductStep", + async function ( + input: CreateProductInput, + context + ) { + const productService = context.container.resolve( + "productService" + ) + const product = await productService.create(input) + return new StepResponse({ + product + }, { + product_id: product.id + }) + }, + async function ( + input, + context + ) { + const productService = context.container.resolve( + "productService" + ) + await productService.delete(input.product_id) + } +) +``` + +## Type Parameters + +<ParameterTypes parameters={[ + { + "name": "TInvokeInput", + "type": "`object`", + "description": "The type of the expected input parameter to the invocation function.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "TInvokeResultOutput", + "type": "`object`", + "description": "The type of the expected output parameter of the invocation function.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "TInvokeResultCompensateInput", + "type": "`object`", + "description": "The type of the expected input parameter to the compensation function.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "name", + "type": "`string`", + "description": "The name of the step.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "invokeFn", + "type": "[InvokeFn](../types/InvokeFn.mdx)<TInvokeInput, TInvokeResultOutput, TInvokeResultCompensateInput>", + "description": "An invocation function that will be executed when the workflow is executed. The function must return an instance of [StepResponse](../classes/StepResponse.mdx). The constructor of [StepResponse](../classes/StepResponse.mdx) accepts the output of the step as a first argument, and optionally as a second argument the data to be passed to the compensation function as a parameter.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "compensateFn", + "type": "[CompensateFn](../types/CompensateFn.mdx)<TInvokeResultCompensateInput>", + "description": "A compensation function that's executed if an error occurs in the workflow. It's used to roll-back actions when errors occur. It accepts as a parameter the second argument passed to the constructor of the [StepResponse](../classes/StepResponse.mdx) instance returned by the invocation function. If the invocation function doesn't pass the second argument to `StepResponse` constructor, the compensation function receives the first argument passed to the `StepResponse` constructor instead.", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "StepFunction", + "type": "[StepFunction](../types/StepFunction.mdx)<TInvokeInput, TInvokeResultOutput>", + "optional": false, + "defaultValue": "", + "description": "A step function to be used in a workflow.", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/functions/createWorkflow.mdx b/www/apps/docs/content/references/workflows/functions/createWorkflow.mdx new file mode 100644 index 0000000000000..4064401175409 --- /dev/null +++ b/www/apps/docs/content/references/workflows/functions/createWorkflow.mdx @@ -0,0 +1,130 @@ +--- +displayed_sidebar: workflowsSidebar +slug: /references/workflows/createWorkflow +sidebar_label: createWorkflow +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# createWorkflow - Workflows Reference + +This documentation provides a reference to the `createWorkflow` . It belongs to the `@medusajs/workflows-sdk` package. + +This function creates a workflow with the provided name and a constructor function. +The constructor function builds the workflow from steps created by the [createStep](createStep.mdx) function. +The returned workflow is an exported workflow of type [ReturnWorkflow](../types/ReturnWorkflow.mdx), meaning it's not executed right away. To execute it, +invoke the exported workflow, then run its `run` method. + +## Example + +```ts +import { createWorkflow } from "@medusajs/workflows-sdk" +import { MedusaRequest, MedusaResponse, Product } from "@medusajs/medusa" +import { + createProductStep, + getProductStep, + createPricesStep +} from "./steps" + +interface WorkflowInput { + title: string +} + +const myWorkflow = createWorkflow< + WorkflowInput, + Product + >("my-workflow", (input) => { + // Everything here will be executed and resolved later + // during the execution. Including the data access. + + const product = createProductStep(input) + const prices = createPricesStep(product) + return getProductStep(product.id) + } +) + +export async function GET( + req: MedusaRequest, + res: MedusaResponse +) { + const { result: product } = await myWorkflow(req.scope) + .run({ + input: { + title: "Shirt" + } + }) + + res.json({ + product + }) +} +``` + +## Type Parameters + +<ParameterTypes parameters={[ + { + "name": "TData", + "type": "`object`", + "description": "The type of the input passed to the composer function.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "TResult", + "type": "`object`", + "description": "The type of the output returned by the composer function.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "THooks", + "type": "`Record<string, Function>`", + "description": "The type of hooks defined in the workflow.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "name", + "type": "`string`", + "description": "The name of the workflow.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "composer", + "type": "(`input`: [WorkflowData](../types/WorkflowData.mdx)<TData>) => `void` \\| [WorkflowData](../types/WorkflowData.mdx)<TResult> \\| { [K in string \\| number \\| symbol]: WorkflowDataProperties<TResult[K]> \\| WorkflowData<TResult[K]> }", + "description": "The constructor function that is executed when the `run` method in [ReturnWorkflow](../types/ReturnWorkflow.mdx) is used. The function can't be an arrow function or an asynchronus function. It also can't directly manipulate data. You'll have to use the [transform](transform.mdx) function if you need to directly manipulate data.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "ReturnWorkflow", + "type": "[ReturnWorkflow](../types/ReturnWorkflow.mdx)<TData, TResult, THooks>", + "optional": false, + "defaultValue": "", + "description": "The created workflow. You can later execute the workflow by invoking it, then using its `run` method.", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/functions/parallelize.mdx b/www/apps/docs/content/references/workflows/functions/parallelize.mdx new file mode 100644 index 0000000000000..2bcaf31b6729a --- /dev/null +++ b/www/apps/docs/content/references/workflows/functions/parallelize.mdx @@ -0,0 +1,89 @@ +--- +displayed_sidebar: workflowsSidebar +slug: /references/workflows/parallelize +sidebar_label: parallelize +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# parallelize - Workflows Reference + +This documentation provides a reference to the `parallelize` . It belongs to the `@medusajs/workflows-sdk` package. + +This function is used to run multiple steps in parallel. The result of each step will be returned as part of the result array. + +## Example + +```ts +import { + createWorkflow, + parallelize +} from "@medusajs/workflows-sdk" +import { + createProductStep, + getProductStep, + createPricesStep, + attachProductToSalesChannelStep +} from "./steps" + +interface WorkflowInput { + title: string +} + +const myWorkflow = createWorkflow< + WorkflowInput, + Product +>("my-workflow", (input) => { + const product = createProductStep(input) + + const [prices, productSalesChannel] = parallelize( + createPricesStep(product), + attachProductToSalesChannelStep(product) + ) + + const id = product.id + return getProductStep(product.id) + } +) + +## Type Parameters + +<ParameterTypes parameters={[ + { + "name": "TResult", + "type": "[WorkflowDataProperties](../types/WorkflowDataProperties.mdx)<unknown>[]", + "description": "The type of the expected result.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "steps", + "type": "`TResult`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "TResult", + "type": "`TResult`", + "optional": false, + "defaultValue": "", + "description": "The step results. The results are ordered in the array by the order they're passed in the function's parameter.", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/functions/transform.mdx b/www/apps/docs/content/references/workflows/functions/transform.mdx new file mode 100644 index 0000000000000..bd2a4809dc1ad --- /dev/null +++ b/www/apps/docs/content/references/workflows/functions/transform.mdx @@ -0,0 +1,114 @@ +--- +displayed_sidebar: workflowsSidebar +slug: /references/workflows/transform +sidebar_label: transform +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# transform - Workflows Reference + +This documentation provides a reference to the `transform` . It belongs to the `@medusajs/workflows-sdk` package. + +This function transforms the output of other utility functions. + +For example, if you're using the value(s) of some step(s) as an input to a later step. As you can't directly manipulate data in the workflow constructor function passed to [createWorkflow](createWorkflow.mdx), +the `transform` function provides access to the runtime value of the step(s) output so that you can manipulate them. + +Another example is if you're using the runtime value of some step(s) as the output of a workflow. + +If you're also retrieving the output of a hook and want to check if its value is set, you must use a workflow to get the runtime value of that hook. + +## Example + +```ts +import { + createWorkflow, + transform +} from "@medusajs/workflows-sdk" +import { step1, step2 } from "./steps" + +type WorkflowInput = { + name: string +} + +type WorkflowOutput = { + message: string +} + +const myWorkflow = createWorkflow< + WorkflowInput, + WorkflowOutput + > + ("hello-world", (input) => { + const str1 = step1(input) + const str2 = step2(input) + + return transform({ + str1, + str2 + }, (input) => ({ + message: `${input.str1}${input.str2}` + })) +}) +``` + +## Type Parameters + +<ParameterTypes parameters={[ + { + "name": "T", + "type": "`object` \\| [WorkflowDataProperties](../types/WorkflowDataProperties.mdx)<unknown>", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "RFinal", + "type": "`object`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +## Parameters + +<ParameterTypes parameters={[ + { + "name": "values", + "type": "`T`", + "description": "The output(s) of other step functions.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "func", + "type": "[[Func1](../types/Func1.mdx)<T, RFinal>]", + "description": "The transform function used to perform action on the runtime values of the provided `values`.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +## Returns + +<ParameterTypes parameters={[ + { + "name": "WorkflowData", + "type": "[WorkflowData](../types/WorkflowData.mdx)<RFinal>", + "optional": false, + "defaultValue": "", + "description": "There's no expected value to be returned by the `transform` function.", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/index.mdx b/www/apps/docs/content/references/workflows/index.mdx new file mode 100644 index 0000000000000..3dcfaf7301d8f --- /dev/null +++ b/www/apps/docs/content/references/workflows/index.mdx @@ -0,0 +1,16 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# Workflows API Reference + +This section of the documentation provides a reference to the utility functions of the `@medusajs/workflows-sdk` package. + +## Functions + +- [createStep](functions/createStep.mdx) +- [createWorkflow](functions/createWorkflow.mdx) +- [parallelize](functions/parallelize.mdx) +- [transform](functions/transform.mdx) diff --git a/www/apps/docs/content/references/workflows/interfaces/Context.mdx b/www/apps/docs/content/references/workflows/interfaces/Context.mdx new file mode 100644 index 0000000000000..c016890faa5d8 --- /dev/null +++ b/www/apps/docs/content/references/workflows/interfaces/Context.mdx @@ -0,0 +1,71 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# Context + +A shared context object that is used to share resources between the application and the module. + +## Type parameters + +<ParameterTypes parameters={[ + { + "name": "TManager", + "type": "`object`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +<ParameterTypes parameters={[ + { + "name": "enableNestedTransactions", + "type": "`boolean`", + "description": "A boolean value indicating whether nested transactions are enabled.", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "isolationLevel", + "type": "`string`", + "description": "A string indicating the isolation level of the context. Possible values are `READ UNCOMMITTED`, `READ COMMITTED`, `REPEATABLE READ`, or `SERIALIZABLE`.", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "manager", + "type": "`TManager`", + "description": "An instance of a manager, typically an entity manager, of type `TManager`, which is a typed parameter passed to the context to specify the type of the `manager`.", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "transactionId", + "type": "`string`", + "description": "A string indicating the ID of the current transaction.", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "transactionManager", + "type": "`TManager`", + "description": "An instance of a transaction manager of type `TManager`, which is a typed parameter passed to the context to specify the type of the `transactionManager`.", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/interfaces/InternalStep.mdx b/www/apps/docs/content/references/workflows/interfaces/InternalStep.mdx new file mode 100644 index 0000000000000..dcaebffe4027d --- /dev/null +++ b/www/apps/docs/content/references/workflows/interfaces/InternalStep.mdx @@ -0,0 +1,118 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# InternalStep + +<ParameterTypes parameters={[ + { + "name": "action", + "type": "`string`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "async", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "continueOnPermanentFailure", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "depth", + "type": "`number`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "maxRetries", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "next", + "type": "[InternalStep](InternalStep.mdx) \\| [InternalStep](InternalStep.mdx)[]", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "noCompensation", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "noWait", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "parent", + "type": "`null` \\| [InternalStep](InternalStep.mdx)", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "retryInterval", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "saveResponse", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "timeout", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/interfaces/JoinerServiceConfig.mdx b/www/apps/docs/content/references/workflows/interfaces/JoinerServiceConfig.mdx new file mode 100644 index 0000000000000..d4bda20b1dbc4 --- /dev/null +++ b/www/apps/docs/content/references/workflows/interfaces/JoinerServiceConfig.mdx @@ -0,0 +1,165 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# JoinerServiceConfig + +<ParameterTypes parameters={[ + { + "name": "alias", + "type": "[JoinerServiceConfigAlias](JoinerServiceConfigAlias.mdx) \\| [JoinerServiceConfigAlias](JoinerServiceConfigAlias.mdx)[]", + "description": "Property name to use as entrypoint to the service", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "args", + "type": "`Record<string, any>`", + "description": "Extra arguments to pass to the remoteFetchData callback", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "extends", + "type": "``{ relationship: [JoinerRelationship](../types/JoinerRelationship.mdx) ; serviceName: string }``[]", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "relationship", + "type": "[JoinerRelationship](../types/JoinerRelationship.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "serviceName", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "fieldAlias", + "type": "`Record<string, string \\| { forwardArgumentsOnPath: string[] ; path: string }>`", + "description": "alias for deeper nested relationships (e.g. { 'price': 'prices.calculated\\_price\\_set.amount' })", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "primaryKeys", + "type": "`string`[]", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "relationships", + "type": "[JoinerRelationship](../types/JoinerRelationship.mdx)[]", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "alias", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "args", + "type": "`Record<string, any>`", + "description": "Extra arguments to pass to the remoteFetchData callback", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "foreignKey", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "inverse", + "type": "`boolean`", + "description": "In an inverted relationship the foreign key is on the other service and the primary key is on the current service", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "isInternalService", + "type": "`boolean`", + "description": "If true, the relationship is an internal service from the medusa core TODO: Remove when there are no more \"internal\" services", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "isList", + "type": "`boolean`", + "description": "Force the relationship to return a list", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "primaryKey", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "serviceName", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "serviceName", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/interfaces/JoinerServiceConfigAlias.mdx b/www/apps/docs/content/references/workflows/interfaces/JoinerServiceConfigAlias.mdx new file mode 100644 index 0000000000000..bfe78313e81d0 --- /dev/null +++ b/www/apps/docs/content/references/workflows/interfaces/JoinerServiceConfigAlias.mdx @@ -0,0 +1,28 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# JoinerServiceConfigAlias + +<ParameterTypes parameters={[ + { + "name": "args", + "type": "`Record<string, any>`", + "description": "Extra arguments to pass to the remoteFetchData callback", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "name", + "type": "`string` \\| `string`[]", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/interfaces/StepExecutionContext.mdx b/www/apps/docs/content/references/workflows/interfaces/StepExecutionContext.mdx new file mode 100644 index 0000000000000..dd8e3d52bbc06 --- /dev/null +++ b/www/apps/docs/content/references/workflows/interfaces/StepExecutionContext.mdx @@ -0,0 +1,168 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# StepExecutionContext + +The step's context. + +<ParameterTypes parameters={[ + { + "name": "container", + "type": "[MedusaContainer](../types/MedusaContainer.mdx)", + "description": "The container used to access resources, such as services, in the step.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "createScope", + "type": "() => [MedusaContainer](../types/MedusaContainer.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "registerAdd", + "type": "`<T>`(`name`: `string`, `registration`: `T`) => [MedusaContainer](../types/MedusaContainer.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "context", + "type": "[Context](Context.mdx)", + "description": "A shared context object that is used to share resources between the application and the module.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "enableNestedTransactions", + "type": "`boolean`", + "description": "A boolean value indicating whether nested transactions are enabled.", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "isolationLevel", + "type": "`string`", + "description": "A string indicating the isolation level of the context. Possible values are `READ UNCOMMITTED`, `READ COMMITTED`, `REPEATABLE READ`, or `SERIALIZABLE`.", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "manager", + "type": "`TManager`", + "description": "An instance of a manager, typically an entity manager, of type `TManager`, which is a typed parameter passed to the context to specify the type of the `manager`.", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "transactionId", + "type": "`string`", + "description": "A string indicating the ID of the current transaction.", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "transactionManager", + "type": "`TManager`", + "description": "An instance of a transaction manager of type `TManager`, which is a typed parameter passed to the context to specify the type of the `transactionManager`.", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "metadata", + "type": "[TransactionMetadata](../types/TransactionMetadata.mdx)", + "description": "Metadata passed in the input.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "action", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "action_type", + "type": "[TransactionHandlerType](../enums/TransactionHandlerType.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "attempt", + "type": "`number`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "idempotency_key", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "model_id", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "reply_to_topic", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "timestamp", + "type": "`number`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/interfaces/WorkflowDefinition.mdx b/www/apps/docs/content/references/workflows/interfaces/WorkflowDefinition.mdx new file mode 100644 index 0000000000000..a26395cbab905 --- /dev/null +++ b/www/apps/docs/content/references/workflows/interfaces/WorkflowDefinition.mdx @@ -0,0 +1,372 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# WorkflowDefinition + +<ParameterTypes parameters={[ + { + "name": "flow_", + "type": "[TransactionStepsDefinition](../types/TransactionStepsDefinition.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "action", + "type": "`string`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "async", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "continueOnPermanentFailure", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "maxRetries", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "next", + "type": "[TransactionStepsDefinition](../types/TransactionStepsDefinition.mdx) \\| [TransactionStepsDefinition](../types/TransactionStepsDefinition.mdx)[]", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "noCompensation", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "noWait", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "retryInterval", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "saveResponse", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "timeout", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "handler", + "type": "(`container`: [MedusaContainer](../types/MedusaContainer.mdx), `context?`: [Context](Context.mdx)) => [TransactionStepHandler](../types/TransactionStepHandler.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "handlers_", + "type": "Map<string, { compensate?: [WorkflowStepHandler](../types/WorkflowStepHandler.mdx) ; invoke: [WorkflowStepHandler](../types/WorkflowStepHandler.mdx) }>", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "id", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "optionalModules", + "type": "Set<string>", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "orchestrator", + "type": "[TransactionOrchestrator](../classes/TransactionOrchestrator.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "canContinue", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "canMoveBackward", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "canMoveForward", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "checkAllSteps", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "compensateSteps", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "createTransactionFlow", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "definition", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "executeNext", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "flagStepsToRevert", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "getCompensationSteps", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "getInvokeSteps", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "getPreviousStep", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "id", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "invokeSteps", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "DEFAULT_RETRIES", + "type": "`number`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "ROOT_STEP", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "SEPARATOR", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "buildSteps", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "getStepByAction", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "getTransactionAndStepFromIdempotencyKey", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "loadTransactionById", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "setStepFailure", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "setStepSuccess", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "requiredModules", + "type": "Set<string>", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/types/CompensateFn.mdx b/www/apps/docs/content/references/workflows/types/CompensateFn.mdx new file mode 100644 index 0000000000000..2a3b2d404a9cd --- /dev/null +++ b/www/apps/docs/content/references/workflows/types/CompensateFn.mdx @@ -0,0 +1,92 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# CompensateFn + + **CompensateFn**: (`input`: `T` \| `undefined`, `context`: [StepExecutionContext](../interfaces/StepExecutionContext.mdx)) => `unknown` \| Promise<unknown> + +## Type Parameters + +<ParameterTypes parameters={[ + { + "name": "T", + "type": "`object`", + "description": "The type of the argument passed to the compensation function. If not specified, then it will be the same type as the invocation function's output.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +## Type declaration + +The type of compensation function passed to a step. + +### Parameters + +<ParameterTypes parameters={[ + { + "name": "input", + "type": "`T` \\| `undefined`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "context", + "type": "[StepExecutionContext](../interfaces/StepExecutionContext.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "container", + "type": "[MedusaContainer](MedusaContainer.mdx)", + "description": "The container used to access resources, such as services, in the step.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "context", + "type": "[Context](../interfaces/Context.mdx)", + "description": "A shared context object that is used to share resources between the application and the module.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "metadata", + "type": "[TransactionMetadata](TransactionMetadata.mdx)", + "description": "Metadata passed in the input.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + } +]} /> + +### Returns + +<ParameterTypes parameters={[ + { + "name": "unknown \\| Promise<unknown>", + "type": "`unknown` \\| Promise<unknown>", + "optional": true, + "defaultValue": "", + "description": "There's no expected type to be returned by the compensation function.", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/types/CreateWorkflowComposerContext.mdx b/www/apps/docs/content/references/workflows/types/CreateWorkflowComposerContext.mdx new file mode 100644 index 0000000000000..78a9813fb7ce6 --- /dev/null +++ b/www/apps/docs/content/references/workflows/types/CreateWorkflowComposerContext.mdx @@ -0,0 +1,105 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# CreateWorkflowComposerContext + + **CreateWorkflowComposerContext**: `Object` + +## Type declaration + +<ParameterTypes parameters={[ + { + "name": "flow", + "type": "[OrchestratorBuilder](../classes/OrchestratorBuilder.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "hasChanges_", + "type": "`boolean`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "steps", + "type": "[InternalStep](../interfaces/InternalStep.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "handlers", + "type": "[WorkflowHandler](WorkflowHandler.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "hookBinder", + "type": "`<TOutput>`(`name`: `string`, `fn`: `Function`) => [WorkflowData](WorkflowData.mdx)<TOutput>", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "hooksCallback_", + "type": "`Record<string, Function[]>`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "hooks_", + "type": "`string`[]", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "parallelizeBinder", + "type": "`<TOutput>`(`fn`: (`this`: [CreateWorkflowComposerContext](CreateWorkflowComposerContext.mdx)) => `TOutput`) => `TOutput`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "stepBinder", + "type": "`<TOutput>`(`fn`: [StepFunctionResult](StepFunctionResult.mdx)) => [WorkflowData](WorkflowData.mdx)<TOutput>", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "workflowId", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/types/ExternalModuleDeclaration.mdx b/www/apps/docs/content/references/workflows/types/ExternalModuleDeclaration.mdx new file mode 100644 index 0000000000000..ef276d624e2aa --- /dev/null +++ b/www/apps/docs/content/references/workflows/types/ExternalModuleDeclaration.mdx @@ -0,0 +1,214 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# ExternalModuleDeclaration + + **ExternalModuleDeclaration**: `Object` + +## Type declaration + +<ParameterTypes parameters={[ + { + "name": "alias", + "type": "`string`", + "description": "If multiple modules are registered with the same key, the alias can be used to differentiate them", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "definition", + "type": "[ModuleDefinition](ModuleDefinition.mdx)", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "canOverride", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "defaultModuleDeclaration", + "type": "[InternalModuleDeclaration](InternalModuleDeclaration.mdx) \\| [ExternalModuleDeclaration](ExternalModuleDeclaration.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "defaultPackage", + "type": "`string` \\| `false`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "dependencies", + "type": "`string`[]", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "isLegacy", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "isQueryable", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "isRequired", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "key", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "label", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "registrationName", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "main", + "type": "`boolean`", + "description": "If the module is the main module for the key when multiple ones are registered", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "options", + "type": "`Record<string, unknown>`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "scope", + "type": "[EXTERNAL](../index.mdx#external)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "server", + "type": "`object`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "keepAlive", + "type": "`boolean`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "type", + "type": "`\"http\"`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "url", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "server.keepAlive", + "type": "`boolean`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "server.type", + "type": "`\"http\"`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "server.url", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/types/FlowRunOptions.mdx b/www/apps/docs/content/references/workflows/types/FlowRunOptions.mdx new file mode 100644 index 0000000000000..143a67fc89c27 --- /dev/null +++ b/www/apps/docs/content/references/workflows/types/FlowRunOptions.mdx @@ -0,0 +1,110 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# FlowRunOptions + + **FlowRunOptions**: `Object` + +## Type Parameters + +<ParameterTypes parameters={[ + { + "name": "TData", + "type": "`object`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +## Type declaration + +<ParameterTypes parameters={[ + { + "name": "context", + "type": "[Context](../interfaces/Context.mdx)", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "enableNestedTransactions", + "type": "`boolean`", + "description": "A boolean value indicating whether nested transactions are enabled.", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "isolationLevel", + "type": "`string`", + "description": "A string indicating the isolation level of the context. Possible values are `READ UNCOMMITTED`, `READ COMMITTED`, `REPEATABLE READ`, or `SERIALIZABLE`.", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "manager", + "type": "`TManager`", + "description": "An instance of a manager, typically an entity manager, of type `TManager`, which is a typed parameter passed to the context to specify the type of the `manager`.", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "transactionId", + "type": "`string`", + "description": "A string indicating the ID of the current transaction.", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "transactionManager", + "type": "`TManager`", + "description": "An instance of a transaction manager of type `TManager`, which is a typed parameter passed to the context to specify the type of the `transactionManager`.", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "input", + "type": "`TData`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "resultFrom", + "type": "`string` \\| `string`[]", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "throwOnError", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/types/Func.mdx b/www/apps/docs/content/references/workflows/types/Func.mdx new file mode 100644 index 0000000000000..e77757bae4695 --- /dev/null +++ b/www/apps/docs/content/references/workflows/types/Func.mdx @@ -0,0 +1,99 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# Func + + **Func**: (`input`: `T`, `context`: [StepExecutionContext](../interfaces/StepExecutionContext.mdx)) => `U` \| Promise<U> + +## Type Parameters + +<ParameterTypes parameters={[ + { + "name": "T", + "type": "`object`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "U", + "type": "`object`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +## Type declaration + +### Parameters + +<ParameterTypes parameters={[ + { + "name": "input", + "type": "`T`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "context", + "type": "[StepExecutionContext](../interfaces/StepExecutionContext.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "container", + "type": "[MedusaContainer](MedusaContainer.mdx)", + "description": "The container used to access resources, such as services, in the step.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "context", + "type": "[Context](../interfaces/Context.mdx)", + "description": "A shared context object that is used to share resources between the application and the module.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "metadata", + "type": "[TransactionMetadata](TransactionMetadata.mdx)", + "description": "Metadata passed in the input.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + } +]} /> + +### Returns + +<ParameterTypes parameters={[ + { + "name": "U \\| Promise<U>", + "type": "`U` \\| Promise<U>", + "optional": true, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/types/Func1.mdx b/www/apps/docs/content/references/workflows/types/Func1.mdx new file mode 100644 index 0000000000000..7c91f3daa74dc --- /dev/null +++ b/www/apps/docs/content/references/workflows/types/Func1.mdx @@ -0,0 +1,99 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# Func1 + + **Func1**: (`input`: `T` extends [WorkflowData](WorkflowData.mdx)<infer U> ? `U` : `T` extends `object` ? { [K in keyof T]: T[K] extends WorkflowData<infer U> ? U : T[K] } : ``{}``, `context`: [StepExecutionContext](../interfaces/StepExecutionContext.mdx)) => `U` \| Promise<U> + +## Type Parameters + +<ParameterTypes parameters={[ + { + "name": "T", + "type": "`object` \\| [WorkflowData](WorkflowData.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "U", + "type": "`object`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +## Type declaration + +### Parameters + +<ParameterTypes parameters={[ + { + "name": "input", + "type": "`T` extends [WorkflowData](WorkflowData.mdx)<infer U> ? `U` : `T` extends `object` ? { [K in keyof T]: T[K] extends WorkflowData<infer U> ? U : T[K] } : ``{}``", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "context", + "type": "[StepExecutionContext](../interfaces/StepExecutionContext.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "container", + "type": "[MedusaContainer](MedusaContainer.mdx)", + "description": "The container used to access resources, such as services, in the step.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "context", + "type": "[Context](../interfaces/Context.mdx)", + "description": "A shared context object that is used to share resources between the application and the module.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "metadata", + "type": "[TransactionMetadata](TransactionMetadata.mdx)", + "description": "Metadata passed in the input.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + } +]} /> + +### Returns + +<ParameterTypes parameters={[ + { + "name": "U \\| Promise<U>", + "type": "`U` \\| Promise<U>", + "optional": true, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/types/InternalModuleDeclaration.mdx b/www/apps/docs/content/references/workflows/types/InternalModuleDeclaration.mdx new file mode 100644 index 0000000000000..f25ce98c48455 --- /dev/null +++ b/www/apps/docs/content/references/workflows/types/InternalModuleDeclaration.mdx @@ -0,0 +1,196 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# InternalModuleDeclaration + + **InternalModuleDeclaration**: `Object` + +## Type declaration + +<ParameterTypes parameters={[ + { + "name": "alias", + "type": "`string`", + "description": "If multiple modules are registered with the same key, the alias can be used to differentiate them", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "definition", + "type": "[ModuleDefinition](ModuleDefinition.mdx)", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "canOverride", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "defaultModuleDeclaration", + "type": "[InternalModuleDeclaration](InternalModuleDeclaration.mdx) \\| [ExternalModuleDeclaration](ExternalModuleDeclaration.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "defaultPackage", + "type": "`string` \\| `false`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "dependencies", + "type": "`string`[]", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "isLegacy", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "isQueryable", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "isRequired", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "key", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "label", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "registrationName", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "dependencies", + "type": "`string`[]", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "main", + "type": "`boolean`", + "description": "If the module is the main module for the key when multiple ones are registered", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "options", + "type": "`Record<string, unknown>`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "resolve", + "type": "`string`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "resources", + "type": "[MODULE_RESOURCE_TYPE](../enums/MODULE_RESOURCE_TYPE.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "ISOLATED", + "type": "`\"isolated\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "SHARED", + "type": "`\"shared\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "scope", + "type": "[INTERNAL](../index.mdx#internal)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/types/InvokeFn.mdx b/www/apps/docs/content/references/workflows/types/InvokeFn.mdx new file mode 100644 index 0000000000000..1097c23e4df20 --- /dev/null +++ b/www/apps/docs/content/references/workflows/types/InvokeFn.mdx @@ -0,0 +1,110 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# InvokeFn + + **InvokeFn**: (`input`: { [Key in keyof TInput]: TInput[Key] }, `context`: [StepExecutionContext](../interfaces/StepExecutionContext.mdx)) => `void` \| [StepResponse](../classes/StepResponse.mdx)<TOutput, TCompensateInput extends undefined ? TOutput : TCompensateInput> \| Promise<void \| [StepResponse](../classes/StepResponse.mdx)<TOutput, TCompensateInput extends undefined ? TOutput : TCompensateInput>> + +## Type Parameters + +<ParameterTypes parameters={[ + { + "name": "TInput", + "type": "`object`", + "description": "The type of the input that the function expects.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "TOutput", + "type": "`object`", + "description": "The type of the output that the function returns.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "TCompensateInput", + "type": "`object`", + "description": "The type of the input that the compensation function expects.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +## Type declaration + +The type of invocation function passed to a step. + +### Parameters + +<ParameterTypes parameters={[ + { + "name": "input", + "type": "{ [Key in keyof TInput]: TInput[Key] }", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "context", + "type": "[StepExecutionContext](../interfaces/StepExecutionContext.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "container", + "type": "[MedusaContainer](MedusaContainer.mdx)", + "description": "The container used to access resources, such as services, in the step.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "context", + "type": "[Context](../interfaces/Context.mdx)", + "description": "A shared context object that is used to share resources between the application and the module.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "metadata", + "type": "[TransactionMetadata](TransactionMetadata.mdx)", + "description": "Metadata passed in the input.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + } +]} /> + +### Returns + +<ParameterTypes parameters={[ + { + "name": "void \\| StepResponse<TOutput, TCompensateInput extends undefined ? TOutput : TCompensateInput> \\| Promise<void \\| StepResponse<TOutput, TCompensateInput extends undefined ? TOutput : TCompensateInput>>", + "type": "`void` \\| [StepResponse](../classes/StepResponse.mdx)<TOutput, TCompensateInput extends undefined ? TOutput : TCompensateInput> \\| Promise<void \\| [StepResponse](../classes/StepResponse.mdx)<TOutput, TCompensateInput extends undefined ? TOutput : TCompensateInput>>", + "optional": true, + "defaultValue": "", + "description": "The expected output based on the type parameter `TOutput`.", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/types/JoinerRelationship.mdx b/www/apps/docs/content/references/workflows/types/JoinerRelationship.mdx new file mode 100644 index 0000000000000..42c22dbc76435 --- /dev/null +++ b/www/apps/docs/content/references/workflows/types/JoinerRelationship.mdx @@ -0,0 +1,86 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# JoinerRelationship + + **JoinerRelationship**: `Object` + +## Type declaration + +<ParameterTypes parameters={[ + { + "name": "alias", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "args", + "type": "`Record<string, any>`", + "description": "Extra arguments to pass to the remoteFetchData callback", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "foreignKey", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "inverse", + "type": "`boolean`", + "description": "In an inverted relationship the foreign key is on the other service and the primary key is on the current service", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "isInternalService", + "type": "`boolean`", + "description": "If true, the relationship is an internal service from the medusa core TODO: Remove when there are no more \"internal\" services", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "isList", + "type": "`boolean`", + "description": "Force the relationship to return a list", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "primaryKey", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "serviceName", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/types/MedusaContainer.mdx b/www/apps/docs/content/references/workflows/types/MedusaContainer.mdx new file mode 100644 index 0000000000000..0594167a11adc --- /dev/null +++ b/www/apps/docs/content/references/workflows/types/MedusaContainer.mdx @@ -0,0 +1,9 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# MedusaContainer + + **MedusaContainer**: `AwilixContainer` & ``{ createScope: () => [MedusaContainer](MedusaContainer.mdx) ; registerAdd: <T>(name: string, registration: T) => [MedusaContainer](MedusaContainer.mdx) }`` diff --git a/www/apps/docs/content/references/workflows/types/ModuleDefinition.mdx b/www/apps/docs/content/references/workflows/types/ModuleDefinition.mdx new file mode 100644 index 0000000000000..f194076bb96eb --- /dev/null +++ b/www/apps/docs/content/references/workflows/types/ModuleDefinition.mdx @@ -0,0 +1,104 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# ModuleDefinition + + **ModuleDefinition**: `Object` + +## Type declaration + +<ParameterTypes parameters={[ + { + "name": "canOverride", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "defaultModuleDeclaration", + "type": "[InternalModuleDeclaration](InternalModuleDeclaration.mdx) \\| [ExternalModuleDeclaration](ExternalModuleDeclaration.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "defaultPackage", + "type": "`string` \\| `false`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "dependencies", + "type": "`string`[]", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "isLegacy", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "isQueryable", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "isRequired", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "key", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "label", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "registrationName", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/types/ModuleJoinerConfig.mdx b/www/apps/docs/content/references/workflows/types/ModuleJoinerConfig.mdx new file mode 100644 index 0000000000000..a9100eb0426d9 --- /dev/null +++ b/www/apps/docs/content/references/workflows/types/ModuleJoinerConfig.mdx @@ -0,0 +1,9 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# ModuleJoinerConfig + + **ModuleJoinerConfig**: Omit<[JoinerServiceConfig](../interfaces/JoinerServiceConfig.mdx), "serviceName" \| "primaryKeys" \| "relationships" \| "extends"> & ``{ databaseConfig?: { extraFields?: Record<string, { defaultValue?: string ; nullable?: boolean ; options?: Record<string, unknown> ; type: "date" \| "time" \| "datetime" \| bigint \| "blob" \| "uint8array" \| "array" \| "enumArray" \| "enum" \| "json" \| "integer" \| "smallint" \| "tinyint" \| "mediumint" \| "float" \| "double" \| "boolean" \| "decimal" \| "string" \| "uuid" \| "text" }> ; idPrefix?: string ; tableName?: string } ; extends?: { fieldAlias?: Record<string, string \| { forwardArgumentsOnPath: string[] ; path: string }> ; relationship: [ModuleJoinerRelationship](ModuleJoinerRelationship.mdx) ; serviceName: string }[] ; isLink?: boolean ; isReadOnlyLink?: boolean ; linkableKeys?: Record<string, string> ; primaryKeys?: string[] ; relationships?: [ModuleJoinerRelationship](ModuleJoinerRelationship.mdx)[] ; schema?: string ; serviceName?: string }`` diff --git a/www/apps/docs/content/references/workflows/types/ModuleJoinerRelationship.mdx b/www/apps/docs/content/references/workflows/types/ModuleJoinerRelationship.mdx new file mode 100644 index 0000000000000..d58e852eececf --- /dev/null +++ b/www/apps/docs/content/references/workflows/types/ModuleJoinerRelationship.mdx @@ -0,0 +1,9 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# ModuleJoinerRelationship + + **ModuleJoinerRelationship**: [JoinerRelationship](JoinerRelationship.mdx) & ``{ deleteCascade?: boolean ; isInternalService?: boolean }`` diff --git a/www/apps/docs/content/references/workflows/types/ReturnWorkflow.mdx b/www/apps/docs/content/references/workflows/types/ReturnWorkflow.mdx new file mode 100644 index 0000000000000..ca2cd3e111882 --- /dev/null +++ b/www/apps/docs/content/references/workflows/types/ReturnWorkflow.mdx @@ -0,0 +1,88 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# ReturnWorkflow + + **ReturnWorkflow**: `<TDataOverride, TResultOverride>`(`container?`: [MedusaContainer](MedusaContainer.mdx) \| ``{ __definition: [ModuleDefinition](ModuleDefinition.mdx) ; __joinerConfig: [ModuleJoinerConfig](ModuleJoinerConfig.mdx) }``[]) => Omit<[LocalWorkflow](../classes/LocalWorkflow.mdx), "run"> & ``{ run: (args?: [FlowRunOptions](FlowRunOptions.mdx)<TDataOverride extends undefined ? TData : TDataOverride>) => Promise<[WorkflowResult](WorkflowResult.mdx)<TResultOverride extends undefined ? TResult : TResultOverride>> }`` & `THooks` + +An exported workflow, which is the type of a workflow constructed by the [createWorkflow](../functions/createWorkflow.mdx) function. The exported workflow can be invoked to create +an executable workflow, optionally within a specified container. So, to execute the workflow, you must invoke the exported workflow, then run the +`run` method of the exported workflow. + +## Example + +To execute a workflow: + +```ts +myWorkflow() + .run({ + input: { + name: "John", + }, + }) + .then(({ result }) => { + console.log(result) + }) +``` + +To specify the container of the workflow, you can pass it as an argument to the call of the exported workflow. This is necessary when executing the workflow +within a Medusa resource such as an API Route or a Subscriber. + +For example: + +```ts +import type { + MedusaRequest, + MedusaResponse +} from "@medusajs/medusa"; +import myWorkflow from "../../../workflows/hello-world"; + +export async function GET( + req: MedusaRequest, + res: MedusaResponse +) { + const { result } = await myWorkflow(req.scope) + .run({ + input: { + name: req.query.name as string + } + }) + + res.send(result) +} +``` + +## Type Parameters + +<ParameterTypes parameters={[ + { + "name": "TData", + "type": "`object`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "TResult", + "type": "`object`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "THooks", + "type": "`Record<string, Function>`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/types/StepFunction.mdx b/www/apps/docs/content/references/workflows/types/StepFunction.mdx new file mode 100644 index 0000000000000..a18cb900314b3 --- /dev/null +++ b/www/apps/docs/content/references/workflows/types/StepFunction.mdx @@ -0,0 +1,34 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# StepFunction + + **StepFunction**: (`input`: { [K in string \| number \| symbol]: WorkflowData<TInput[K]> }) => [WorkflowData](WorkflowData.mdx)<{ [K in string \| number \| symbol]: TOutput[K] }> & [WorkflowDataProperties](WorkflowDataProperties.mdx)<{ [K in keyof TOutput]: TOutput[K] }> + +A step function to be used in a workflow. + +## Type Parameters + +<ParameterTypes parameters={[ + { + "name": "TInput", + "type": "`object`", + "description": "The type of the input of the step.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "TOutput", + "type": "`object`", + "description": "The type of the output of the step.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/types/StepFunctionResult.mdx b/www/apps/docs/content/references/workflows/types/StepFunctionResult.mdx new file mode 100644 index 0000000000000..5176fa6239044 --- /dev/null +++ b/www/apps/docs/content/references/workflows/types/StepFunctionResult.mdx @@ -0,0 +1,126 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# StepFunctionResult + + **StepFunctionResult**: (`this`: [CreateWorkflowComposerContext](CreateWorkflowComposerContext.mdx)) => `TOutput` extends [] ? [...WorkflowData<{ [K in keyof TOutput]: TOutput[number][K] }>[]] : [WorkflowData](WorkflowData.mdx)<{ [K in keyof TOutput]: TOutput[K] }> + +## Type Parameters + +<ParameterTypes parameters={[ + { + "name": "TOutput", + "type": "`unknown` \\| `unknown`[]", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +## Type declaration + +### Parameters + +<ParameterTypes parameters={[ + { + "name": "this", + "type": "[CreateWorkflowComposerContext](CreateWorkflowComposerContext.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "flow", + "type": "[OrchestratorBuilder](../classes/OrchestratorBuilder.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "handlers", + "type": "[WorkflowHandler](WorkflowHandler.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "hookBinder", + "type": "`<TOutput>`(`name`: `string`, `fn`: `Function`) => [WorkflowData](WorkflowData.mdx)<TOutput>", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "hooksCallback_", + "type": "`Record<string, Function[]>`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "hooks_", + "type": "`string`[]", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "parallelizeBinder", + "type": "`<TOutput>`(`fn`: (`this`: [CreateWorkflowComposerContext](CreateWorkflowComposerContext.mdx)) => `TOutput`) => `TOutput`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "stepBinder", + "type": "`<TOutput>`(`fn`: [StepFunctionResult](StepFunctionResult.mdx)) => [WorkflowData](WorkflowData.mdx)<TOutput>", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "workflowId", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + } +]} /> + +### Returns + +<ParameterTypes parameters={[ + { + "name": "TOutput extends [] ? [...WorkflowData<{ [K in keyof TOutput]: TOutput[number][K] }>[]] : WorkflowData<{ [K in keyof TOutput]: TOutput[K] }>", + "type": "`TOutput` extends [] ? [...WorkflowData<{ [K in keyof TOutput]: TOutput[number][K] }>[]] : [WorkflowData](WorkflowData.mdx)<{ [K in keyof TOutput]: TOutput[K] }>", + "optional": true, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/types/StepHandler.mdx b/www/apps/docs/content/references/workflows/types/StepHandler.mdx new file mode 100644 index 0000000000000..2e44b49b0cec3 --- /dev/null +++ b/www/apps/docs/content/references/workflows/types/StepHandler.mdx @@ -0,0 +1,32 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# StepHandler + + **StepHandler**: `Object` + +## Type declaration + +<ParameterTypes parameters={[ + { + "name": "compensate", + "type": "[WorkflowStepHandler](WorkflowStepHandler.mdx)", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "invoke", + "type": "[WorkflowStepHandler](WorkflowStepHandler.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/types/TransactionFlow.mdx b/www/apps/docs/content/references/workflows/types/TransactionFlow.mdx new file mode 100644 index 0000000000000..50c5fba25fbe3 --- /dev/null +++ b/www/apps/docs/content/references/workflows/types/TransactionFlow.mdx @@ -0,0 +1,250 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# TransactionFlow + + **TransactionFlow**: `Object` + +## Type declaration + +<ParameterTypes parameters={[ + { + "name": "definition", + "type": "[TransactionStepsDefinition](TransactionStepsDefinition.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "action", + "type": "`string`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "async", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "continueOnPermanentFailure", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "maxRetries", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "next", + "type": "[TransactionStepsDefinition](TransactionStepsDefinition.mdx) \\| [TransactionStepsDefinition](TransactionStepsDefinition.mdx)[]", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "noCompensation", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "noWait", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "retryInterval", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "saveResponse", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "timeout", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "hasFailedSteps", + "type": "`boolean`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "hasSkippedSteps", + "type": "`boolean`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "modelId", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "state", + "type": "[TransactionState](../enums/TransactionState.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "COMPENSATING", + "type": "`\"compensating\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "DONE", + "type": "`\"done\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "DORMANT", + "type": "`\"dormant\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "FAILED", + "type": "`\"failed\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "INVOKING", + "type": "`\"invoking\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "NOT_STARTED", + "type": "`\"not_started\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "REVERTED", + "type": "`\"reverted\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "SKIPPED", + "type": "`\"skipped\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "WAITING_TO_COMPENSATE", + "type": "`\"waiting_to_compensate\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "steps", + "type": "`object`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "transactionId", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/types/TransactionMetadata.mdx b/www/apps/docs/content/references/workflows/types/TransactionMetadata.mdx new file mode 100644 index 0000000000000..b3482a3294123 --- /dev/null +++ b/www/apps/docs/content/references/workflows/types/TransactionMetadata.mdx @@ -0,0 +1,96 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# TransactionMetadata + + **TransactionMetadata**: `Object` + +## Type declaration + +<ParameterTypes parameters={[ + { + "name": "action", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "action_type", + "type": "[TransactionHandlerType](../enums/TransactionHandlerType.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "COMPENSATE", + "type": "`\"compensate\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "INVOKE", + "type": "`\"invoke\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "attempt", + "type": "`number`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "idempotency_key", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "model_id", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "reply_to_topic", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "timestamp", + "type": "`number`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/types/TransactionStepHandler.mdx b/www/apps/docs/content/references/workflows/types/TransactionStepHandler.mdx new file mode 100644 index 0000000000000..1864276ee2f73 --- /dev/null +++ b/www/apps/docs/content/references/workflows/types/TransactionStepHandler.mdx @@ -0,0 +1,205 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# TransactionStepHandler + + **TransactionStepHandler**: (`actionId`: `string`, `handlerType`: [TransactionHandlerType](../enums/TransactionHandlerType.mdx), `payload`: [TransactionPayload](../classes/TransactionPayload.mdx), `transaction?`: [DistributedTransaction](../classes/DistributedTransaction.mdx)) => Promise<unknown> + +## Type declaration + +### Parameters + +<ParameterTypes parameters={[ + { + "name": "actionId", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "handlerType", + "type": "[TransactionHandlerType](../enums/TransactionHandlerType.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "COMPENSATE", + "type": "`\"compensate\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "INVOKE", + "type": "`\"invoke\"`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "payload", + "type": "[TransactionPayload](../classes/TransactionPayload.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "context", + "type": "[TransactionContext](../classes/TransactionContext.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "data", + "type": "`Record<string, unknown>`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "metadata", + "type": "[TransactionMetadata](TransactionMetadata.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "transaction", + "type": "[DistributedTransaction](../classes/DistributedTransaction.mdx)", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "context", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "errors", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "flow", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "handler", + "type": "[TransactionStepHandler](TransactionStepHandler.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "modelId", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "payload", + "type": "`any`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "transactionId", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "keyPrefix", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "keyValueStore", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + } +]} /> + +### Returns + +<ParameterTypes parameters={[ + { + "name": "Promise", + "type": "Promise<unknown>", + "optional": false, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [ + { + "name": "unknown", + "type": "`unknown`", + "optional": true, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } + ] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/types/TransactionStepsDefinition.mdx b/www/apps/docs/content/references/workflows/types/TransactionStepsDefinition.mdx new file mode 100644 index 0000000000000..665f1a97eec54 --- /dev/null +++ b/www/apps/docs/content/references/workflows/types/TransactionStepsDefinition.mdx @@ -0,0 +1,104 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# TransactionStepsDefinition + + **TransactionStepsDefinition**: `Object` + +## Type declaration + +<ParameterTypes parameters={[ + { + "name": "action", + "type": "`string`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "async", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "continueOnPermanentFailure", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "maxRetries", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "next", + "type": "[TransactionStepsDefinition](TransactionStepsDefinition.mdx) \\| [TransactionStepsDefinition](TransactionStepsDefinition.mdx)[]", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "noCompensation", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "noWait", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "retryInterval", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "saveResponse", + "type": "`boolean`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "timeout", + "type": "`number`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/types/WorkflowData.mdx b/www/apps/docs/content/references/workflows/types/WorkflowData.mdx new file mode 100644 index 0000000000000..0756a1d397469 --- /dev/null +++ b/www/apps/docs/content/references/workflows/types/WorkflowData.mdx @@ -0,0 +1,25 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# WorkflowData + + **WorkflowData**: `T` extends `object` ? { [Key in keyof T]: WorkflowData<T[Key]> } : [WorkflowDataProperties](WorkflowDataProperties.mdx)<T> & [WorkflowDataProperties](WorkflowDataProperties.mdx)<T> + +This type is used to encapsulate the input or output type of all utils. + +## Type Parameters + +<ParameterTypes parameters={[ + { + "name": "T", + "type": "`object`", + "description": "The type of a step's input or result.", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/types/WorkflowDataProperties.mdx b/www/apps/docs/content/references/workflows/types/WorkflowDataProperties.mdx new file mode 100644 index 0000000000000..117f427ec5432 --- /dev/null +++ b/www/apps/docs/content/references/workflows/types/WorkflowDataProperties.mdx @@ -0,0 +1,46 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# WorkflowDataProperties + + **WorkflowDataProperties**: `Object` + +## Type Parameters + +<ParameterTypes parameters={[ + { + "name": "T", + "type": "`object`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +## Type declaration + +<ParameterTypes parameters={[ + { + "name": "__step__", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "__type", + "type": "`Symbol`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/types/WorkflowHandler.mdx b/www/apps/docs/content/references/workflows/types/WorkflowHandler.mdx new file mode 100644 index 0000000000000..468a30783024b --- /dev/null +++ b/www/apps/docs/content/references/workflows/types/WorkflowHandler.mdx @@ -0,0 +1,9 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# WorkflowHandler + + **WorkflowHandler**: Map<string, { compensate?: [WorkflowStepHandler](WorkflowStepHandler.mdx) ; invoke: [WorkflowStepHandler](WorkflowStepHandler.mdx) }> diff --git a/www/apps/docs/content/references/workflows/types/WorkflowResult.mdx b/www/apps/docs/content/references/workflows/types/WorkflowResult.mdx new file mode 100644 index 0000000000000..9c8067f74e2fe --- /dev/null +++ b/www/apps/docs/content/references/workflows/types/WorkflowResult.mdx @@ -0,0 +1,165 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# WorkflowResult + + **WorkflowResult**: `Object` + +## Type Parameters + +<ParameterTypes parameters={[ + { + "name": "TResult", + "type": "`object`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } +]} /> + +## Type declaration + +<ParameterTypes parameters={[ + { + "name": "errors", + "type": "[TransactionStepError](../classes/TransactionStepError.mdx)[]", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "action", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "error", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "handlerType", + "type": "[TransactionHandlerType](../enums/TransactionHandlerType.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "result", + "type": "`TResult`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "transaction", + "type": "[DistributedTransaction](../classes/DistributedTransaction.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "context", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "errors", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "flow", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "handler", + "type": "[TransactionStepHandler](TransactionStepHandler.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "modelId", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "payload", + "type": "`any`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "transactionId", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "keyPrefix", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "keyValueStore", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/types/WorkflowStepHandler.mdx b/www/apps/docs/content/references/workflows/types/WorkflowStepHandler.mdx new file mode 100644 index 0000000000000..6a8ec10559959 --- /dev/null +++ b/www/apps/docs/content/references/workflows/types/WorkflowStepHandler.mdx @@ -0,0 +1,294 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# WorkflowStepHandler + + **WorkflowStepHandler**: (`args`: ``{ compensate: { [actions: string]: unknown; } ; container: [MedusaContainer](MedusaContainer.mdx) ; context?: [Context](../interfaces/Context.mdx) ; invoke: { [actions: string]: unknown; } ; metadata: [TransactionMetadata](TransactionMetadata.mdx) ; payload: unknown ; transaction: [DistributedTransaction](../classes/DistributedTransaction.mdx) }``) => `unknown` + +## Type declaration + +### Parameters + +<ParameterTypes parameters={[ + { + "name": "args", + "type": "`object`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "compensate", + "type": "`object`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "container", + "type": "[MedusaContainer](MedusaContainer.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "context", + "type": "[Context](../interfaces/Context.mdx)", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "invoke", + "type": "`object`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "metadata", + "type": "[TransactionMetadata](TransactionMetadata.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "payload", + "type": "`unknown`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "transaction", + "type": "[DistributedTransaction](../classes/DistributedTransaction.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "args.compensate", + "type": "`object`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "args.container", + "type": "[MedusaContainer](MedusaContainer.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "args.context", + "type": "[Context](../interfaces/Context.mdx)", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "enableNestedTransactions", + "type": "`boolean`", + "description": "A boolean value indicating whether nested transactions are enabled.", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "isolationLevel", + "type": "`string`", + "description": "A string indicating the isolation level of the context. Possible values are `READ UNCOMMITTED`, `READ COMMITTED`, `REPEATABLE READ`, or `SERIALIZABLE`.", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "manager", + "type": "`TManager`", + "description": "An instance of a manager, typically an entity manager, of type `TManager`, which is a typed parameter passed to the context to specify the type of the `manager`.", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "transactionId", + "type": "`string`", + "description": "A string indicating the ID of the current transaction.", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "transactionManager", + "type": "`TManager`", + "description": "An instance of a transaction manager of type `TManager`, which is a typed parameter passed to the context to specify the type of the `transactionManager`.", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + }, + { + "name": "args.invoke", + "type": "`object`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "args.metadata", + "type": "[TransactionMetadata](TransactionMetadata.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "args.payload", + "type": "`unknown`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "args.transaction", + "type": "[DistributedTransaction](../classes/DistributedTransaction.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [ + { + "name": "context", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "errors", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "flow", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "handler", + "type": "[TransactionStepHandler](TransactionStepHandler.mdx)", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "modelId", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "payload", + "type": "`any`", + "description": "", + "optional": true, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "transactionId", + "type": "`string`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "keyPrefix", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + }, + { + "name": "keyValueStore", + "type": "`any`", + "description": "", + "optional": false, + "defaultValue": "", + "expandable": false, + "children": [] + } + ] + } +]} /> + +### Returns + +<ParameterTypes parameters={[ + { + "name": "unknown", + "type": "`unknown`", + "optional": true, + "defaultValue": "", + "description": "", + "expandable": false, + "children": [] + } +]} /> diff --git a/www/apps/docs/content/references/workflows/types/WorkflowTransactionContext.mdx b/www/apps/docs/content/references/workflows/types/WorkflowTransactionContext.mdx new file mode 100644 index 0000000000000..2bd5f692fb93c --- /dev/null +++ b/www/apps/docs/content/references/workflows/types/WorkflowTransactionContext.mdx @@ -0,0 +1,9 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# WorkflowTransactionContext + + **WorkflowTransactionContext**: [StepExecutionContext](../interfaces/StepExecutionContext.mdx) & [TransactionContext](../classes/TransactionContext.mdx) & ``{ invoke: { [key: string]: { output: any }; } }`` diff --git a/www/apps/docs/content/references/workflows/variables/SymbolInputReference.mdx b/www/apps/docs/content/references/workflows/variables/SymbolInputReference.mdx new file mode 100644 index 0000000000000..ba3a55f6c17b8 --- /dev/null +++ b/www/apps/docs/content/references/workflows/variables/SymbolInputReference.mdx @@ -0,0 +1,9 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# SymbolInputReference + + `Const` **SymbolInputReference**: typeof [SymbolInputReference](SymbolInputReference.mdx) diff --git a/www/apps/docs/content/references/workflows/variables/SymbolMedusaWorkflowComposerContext.mdx b/www/apps/docs/content/references/workflows/variables/SymbolMedusaWorkflowComposerContext.mdx new file mode 100644 index 0000000000000..5fd608de80cab --- /dev/null +++ b/www/apps/docs/content/references/workflows/variables/SymbolMedusaWorkflowComposerContext.mdx @@ -0,0 +1,9 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# SymbolMedusaWorkflowComposerContext + + `Const` **SymbolMedusaWorkflowComposerContext**: typeof [SymbolMedusaWorkflowComposerContext](SymbolMedusaWorkflowComposerContext.mdx) diff --git a/www/apps/docs/content/references/workflows/variables/SymbolWorkflowHook.mdx b/www/apps/docs/content/references/workflows/variables/SymbolWorkflowHook.mdx new file mode 100644 index 0000000000000..4cb5171e41ec6 --- /dev/null +++ b/www/apps/docs/content/references/workflows/variables/SymbolWorkflowHook.mdx @@ -0,0 +1,9 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# SymbolWorkflowHook + + `Const` **SymbolWorkflowHook**: typeof [SymbolWorkflowHook](SymbolWorkflowHook.mdx) diff --git a/www/apps/docs/content/references/workflows/variables/SymbolWorkflowStep.mdx b/www/apps/docs/content/references/workflows/variables/SymbolWorkflowStep.mdx new file mode 100644 index 0000000000000..a93e1e47042d4 --- /dev/null +++ b/www/apps/docs/content/references/workflows/variables/SymbolWorkflowStep.mdx @@ -0,0 +1,9 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# SymbolWorkflowStep + + `Const` **SymbolWorkflowStep**: typeof [SymbolWorkflowStep](SymbolWorkflowStep.mdx) diff --git a/www/apps/docs/content/references/workflows/variables/SymbolWorkflowStepBind.mdx b/www/apps/docs/content/references/workflows/variables/SymbolWorkflowStepBind.mdx new file mode 100644 index 0000000000000..49b42ce9be6f5 --- /dev/null +++ b/www/apps/docs/content/references/workflows/variables/SymbolWorkflowStepBind.mdx @@ -0,0 +1,9 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# SymbolWorkflowStepBind + + `Const` **SymbolWorkflowStepBind**: typeof [SymbolWorkflowStepBind](SymbolWorkflowStepBind.mdx) diff --git a/www/apps/docs/content/references/workflows/variables/SymbolWorkflowStepResponse.mdx b/www/apps/docs/content/references/workflows/variables/SymbolWorkflowStepResponse.mdx new file mode 100644 index 0000000000000..af3000fd7ab42 --- /dev/null +++ b/www/apps/docs/content/references/workflows/variables/SymbolWorkflowStepResponse.mdx @@ -0,0 +1,9 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# SymbolWorkflowStepResponse + + `Const` **SymbolWorkflowStepResponse**: typeof [SymbolWorkflowStepResponse](SymbolWorkflowStepResponse.mdx) diff --git a/www/apps/docs/content/references/workflows/variables/SymbolWorkflowStepTransformer.mdx b/www/apps/docs/content/references/workflows/variables/SymbolWorkflowStepTransformer.mdx new file mode 100644 index 0000000000000..6b87538ed038f --- /dev/null +++ b/www/apps/docs/content/references/workflows/variables/SymbolWorkflowStepTransformer.mdx @@ -0,0 +1,9 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# SymbolWorkflowStepTransformer + + `Const` **SymbolWorkflowStepTransformer**: typeof [SymbolWorkflowStepTransformer](SymbolWorkflowStepTransformer.mdx) diff --git a/www/apps/docs/content/references/workflows/variables/SymbolWorkflowWorkflowData.mdx b/www/apps/docs/content/references/workflows/variables/SymbolWorkflowWorkflowData.mdx new file mode 100644 index 0000000000000..2edf90ab8e10d --- /dev/null +++ b/www/apps/docs/content/references/workflows/variables/SymbolWorkflowWorkflowData.mdx @@ -0,0 +1,9 @@ +--- +displayed_sidebar: workflowsSidebar +--- + +import ParameterTypes from "@site/src/components/ParameterTypes" + +# SymbolWorkflowWorkflowData + + `Const` **SymbolWorkflowWorkflowData**: typeof [SymbolWorkflowWorkflowData](SymbolWorkflowWorkflowData.mdx) diff --git a/www/apps/docs/content/starters/nextjs-medusa-starter.mdx b/www/apps/docs/content/starters/nextjs-medusa-starter.mdx index 1b0fbd3f61209..68d09d8985ec6 100644 --- a/www/apps/docs/content/starters/nextjs-medusa-starter.mdx +++ b/www/apps/docs/content/starters/nextjs-medusa-starter.mdx @@ -157,7 +157,7 @@ NEXT_PUBLIC_MEDUSA_BACKEND_URL=http://localhost:9001 :::note -The Product Module is currently in beta. You can learn more about it [here](../modules/products/serverless-module.mdx). In addition, the product module in the Next.js storefront can't be used without the Medusa backend at the moment. +The Product Module is currently in beta. You can learn more about it [here](../experimental/product/install-nodejs.md). In addition, the product module in the Next.js storefront can't be used without the Medusa backend at the moment. ::: diff --git a/www/apps/docs/sidebars.js b/www/apps/docs/sidebars.js index f9403ff28b79f..40247aed5215d 100644 --- a/www/apps/docs/sidebars.js +++ b/www/apps/docs/sidebars.js @@ -639,18 +639,6 @@ module.exports = { id: "modules/products/categories", label: "Categories", }, - { - type: "html", - value: "References", - customProps: { - sidebar_is_group_divider: true, - }, - }, - { - type: "ref", - id: "references/product/interfaces/IProductModuleService", - label: "Product Module Interface Reference", - }, { type: "html", value: "How-to", @@ -673,11 +661,6 @@ module.exports = { id: "modules/products/admin/import-products", label: "Admin: Import Products", }, - { - type: "doc", - id: "modules/products/serverless-module", - label: "Storefront: Serverless Module", - }, { type: "doc", id: "modules/products/storefront/show-products", @@ -1161,18 +1144,6 @@ module.exports = { id: "modules/price-lists/price-selection-strategy", label: "Price Selection Strategy", }, - { - type: "html", - value: "References", - customProps: { - sidebar_is_group_divider: true, - }, - }, - { - type: "ref", - id: "references/pricing/interfaces/IPricingModuleService", - label: "Pricing Module Interface Reference", - }, { type: "html", value: "How-to", @@ -1378,6 +1349,29 @@ module.exports = { }, collapsible: false, items: [ + { + type: "category", + label: "Workflows", + items: [ + { + type: "doc", + id: "development/workflows/index", + label: "Introduction", + }, + { + type: "html", + value: "References", + customProps: { + sidebar_is_group_divider: true, + }, + }, + { + type: "ref", + id: "references/workflows/index", + label: "API Reference", + }, + ], + }, { type: "category", label: "Entity", @@ -1969,6 +1963,14 @@ module.exports = { }, ], }, + { + type: "ref", + label: "Experimental Features", + id: "experimental/index", + customProps: { + sidebar_is_group_headline: true, + }, + }, ], upgradeGuides: [ { @@ -2646,6 +2648,124 @@ module.exports = { dirName: "user-guide", }, ], + experimentalSidebar: [ + { + type: "ref", + id: "development/overview", + label: "Back to Medusa Development", + customProps: { + sidebar_is_back_link: true, + sidebar_icon: "back-arrow", + }, + }, + { + type: "doc", + id: "experimental/index", + label: "Experimental", + customProps: { + sidebar_is_title: true, + sidebar_icon: "beaker", + }, + }, + { + type: "category", + label: "Pricing Module", + customProps: { + sidebar_is_group_headline: true, + }, + collapsible: true, + collapsed: false, + items: [ + { + type: "doc", + label: "Overview", + id: "experimental/pricing/overview", + }, + { + type: "doc", + label: "Install in Medusa", + id: "experimental/pricing/install-medusa", + }, + { + type: "doc", + label: "Install in Node.js", + id: "experimental/pricing/install-nodejs", + }, + { + type: "doc", + label: "Examples", + id: "experimental/pricing/examples", + }, + { + type: "html", + value: "Architecture", + customProps: { + sidebar_is_group_divider: true, + }, + }, + { + type: "doc", + label: "Pricing Concepts", + id: "experimental/pricing/concepts", + }, + { + type: "doc", + label: "Prices Calculation", + id: "experimental/pricing/prices-calculation", + }, + { + type: "html", + value: "References", + customProps: { + sidebar_is_group_divider: true, + }, + }, + { + type: "ref", + id: "references/pricing/interfaces/IPricingModuleService", + label: "Interface Reference", + }, + ], + }, + { + type: "category", + label: "Product Module", + customProps: { + sidebar_is_group_headline: true, + }, + collapsible: true, + collapsed: false, + items: [ + { + type: "doc", + label: "Overview", + id: "experimental/product/overview", + }, + { + type: "doc", + label: "Install in Medusa", + id: "experimental/product/install-medusa", + }, + { + type: "doc", + label: "Install in Node.js", + id: "experimental/product/install-nodejs", + }, + { + type: "html", + value: "References", + customProps: { + sidebar_is_group_divider: true, + }, + }, + { + type: "ref", + id: "references/product/interfaces/IProductModuleService", + label: "Interface Reference", + }, + ], + }, + ], servicesSidebar: [ { type: "ref", @@ -3192,4 +3312,38 @@ module.exports = { ], }, ], + workflowsSidebar: [ + { + type: "ref", + id: "modules/overview", + label: "Back to Commerce Modules", + customProps: { + sidebar_is_back_link: true, + sidebar_icon: "back-arrow", + }, + }, + { + type: "doc", + id: "references/workflows/index", + label: "Workflows API Reference", + customProps: { + sidebar_is_title: true, + sidebar_icon: "folder-open", + }, + }, + { + type: "category", + label: "Functions", + collapsible: false, + customProps: { + sidebar_is_group_headline: true, + }, + items: [ + { + type: "autogenerated", + dirName: "references/workflows/functions", + }, + ], + }, + ], } diff --git a/www/apps/docs/src/css/_docusaurus.css b/www/apps/docs/src/css/_docusaurus.css index f93e68f5e829a..20166d298ad42 100644 --- a/www/apps/docs/src/css/_docusaurus.css +++ b/www/apps/docs/src/css/_docusaurus.css @@ -282,6 +282,6 @@ h1, h2, h3, h4, h5, h6 { @apply bg-medusa-bg-highlight; } -.prism-code *::selection, .code-header::selection { +.prism-code *::selection, .code-header *::selection { @apply bg-medusa-code-text-highlight; } \ No newline at end of file diff --git a/www/apps/docs/src/css/components/sidebar.css b/www/apps/docs/src/css/components/sidebar.css index b65693224bf16..2d86f29d4b852 100644 --- a/www/apps/docs/src/css/components/sidebar.css +++ b/www/apps/docs/src/css/components/sidebar.css @@ -153,15 +153,4 @@ .theme-doc-sidebar-item-link { @apply flex items-center; -} - -/** Redocly sidebar **/ - -.redocusaurus .menu-content > div > ul > li > ul label:not([type=tag]):not(.active) { - @apply text-medusa-fg-subtle; - @apply hover:text-medusa-fg-base; -} - -.redocusaurus .menu-content > div > ul > li > ul label:not([type=tag]).active { - @apply text-medusa-fg-base; } \ No newline at end of file diff --git a/www/apps/docs/src/css/custom.css b/www/apps/docs/src/css/custom.css index a1044a8b6ecaa..b24507f2fc922 100644 --- a/www/apps/docs/src/css/custom.css +++ b/www/apps/docs/src/css/custom.css @@ -38,6 +38,10 @@ .sidebar-group-headline:not(.theme-doc-sidebar-item-category-level-1) { @apply mb-[6px]; } + + .sidebar-group-headline > .menu__link { + @apply text-medusa-fg-base; + } .sidebar-group-headline > .menu__list-item-collapsible > .menu__link { @apply text-medusa-fg-base !p-0; diff --git a/www/apps/docs/src/theme/DocSidebarItem/Link/index.tsx b/www/apps/docs/src/theme/DocSidebarItem/Link/index.tsx index 8d8e2392128d4..f33533740c9ce 100644 --- a/www/apps/docs/src/theme/DocSidebarItem/Link/index.tsx +++ b/www/apps/docs/src/theme/DocSidebarItem/Link/index.tsx @@ -24,6 +24,7 @@ export default function DocSidebarItemLink({ const { href, label, className, autoAddBaseUrl, customProps } = item const isActive = isActiveSidebarItem(item, activePath) const isInternalLink = isInternalUrl(href) + return ( <li className={clsx( diff --git a/www/apps/docs/src/theme/Icon/Beaker/index.tsx b/www/apps/docs/src/theme/Icon/Beaker/index.tsx new file mode 100644 index 0000000000000..432c801677a7b --- /dev/null +++ b/www/apps/docs/src/theme/Icon/Beaker/index.tsx @@ -0,0 +1,27 @@ +import { IconProps } from "@medusajs/icons/dist/types" +import clsx from "clsx" +import React from "react" + +const IconBeaker = (props: IconProps) => { + return ( + <svg + width={props.width || 20} + height={props.height || 20} + viewBox="0 0 20 20" + fill="none" + xmlns="http://www.w3.org/2000/svg" + {...props} + className={clsx("text-ui-fg-subtle", props.className)} + > + <path + d="M8.12499 2.5865V7.34817C8.12499 7.5944 8.0765 7.83822 7.98227 8.0657C7.88805 8.29319 7.74993 8.49989 7.57583 8.674L4.16666 12.0832M8.12499 2.5865C7.91583 2.60567 7.70749 2.62817 7.49999 2.65483M8.12499 2.5865C9.3723 2.47052 10.6277 2.47052 11.875 2.5865M4.16666 12.0832L4.80833 11.9223C6.55249 11.4913 8.39317 11.6961 9.99999 12.4998C11.6068 13.3036 13.4475 13.5083 15.1917 13.0773L16.5 12.7498M4.16666 12.0832L2.33166 13.919C1.30416 14.9448 1.78916 16.6832 3.22083 16.9273C5.42416 17.304 7.68916 17.4998 9.99999 17.4998C12.2718 17.5006 14.5396 17.3091 16.7792 16.9273C18.21 16.6832 18.695 14.9448 17.6683 13.9182L16.5 12.7498M11.875 2.5865V7.34817C11.875 7.84567 12.0725 8.32317 12.4242 8.674L16.5 12.7498M11.875 2.5865C12.0842 2.60567 12.2925 2.62817 12.5 2.65483" + stroke="currentColor" + strokeWidth="1.25" + strokeLinecap="round" + strokeLinejoin="round" + /> + </svg> + ) +} + +export default IconBeaker diff --git a/www/apps/docs/src/theme/Icon/index.tsx b/www/apps/docs/src/theme/Icon/index.tsx index c02911ee0481f..191304aa15085 100644 --- a/www/apps/docs/src/theme/Icon/index.tsx +++ b/www/apps/docs/src/theme/Icon/index.tsx @@ -102,6 +102,7 @@ import { import IconPuzzleSolid from "./PuzzleSolid" import IconNextjs from "./Nextjs" import IconFlagMini from "./FlagMini" +import IconBeaker from "./Beaker" export default { "academic-cap-solid": AcademicCapSolid, @@ -111,6 +112,7 @@ export default { "arrow-down-tray": ArrowDownTray, "back-arrow": ArrowUturnLeft, "bars-three": BarsThree, + beaker: IconBeaker, bell: BellAlert, "bell-alert-solid": BellAlertSolid, bolt: Bolt, diff --git a/www/apps/docs/src/theme/Tabs/index.tsx b/www/apps/docs/src/theme/Tabs/index.tsx index b6dfed9178ad8..c216b71ae5f13 100644 --- a/www/apps/docs/src/theme/Tabs/index.tsx +++ b/www/apps/docs/src/theme/Tabs/index.tsx @@ -3,6 +3,7 @@ import React, { useRef, type ReactElement, useEffect, + useState, } from "react" import clsx from "clsx" import { @@ -41,6 +42,7 @@ function TabList({ useScrollPositionBlocker() const codeTabSelectorRef = useRef(null) const codeTabsWrapperRef = useRef(null) + const [tabsTitle, setTabsTitle] = useState(codeTitle) const handleTabChange = ( event: @@ -108,6 +110,15 @@ function TabList({ } }, [codeTabSelectorRef, tabRefs]) + useEffect(() => { + const selectedTab = tabValues.find((tab) => tab.value === selectedValue) + if (selectedTab?.attributes?.title) { + setTabsTitle(selectedTab.attributes.title as string) + } else { + setTabsTitle(codeTitle) + } + }, [selectedValue]) + return ( <div className={clsx(isCodeTabs && "code-header", !isCodeTabs && "[&+*]:pt-1")} @@ -183,7 +194,7 @@ function TabList({ "text-compact-small-plus text-medusa-code-text-subtle hidden xs:block" )} > - {codeTitle} + {tabsTitle} </span> )} </div> diff --git a/www/apps/docs/vercel.json b/www/apps/docs/vercel.json index 1be82ee6e28d3..43b8d42056211 100644 --- a/www/apps/docs/vercel.json +++ b/www/apps/docs/vercel.json @@ -488,6 +488,10 @@ { "source": "/development/endpoints/:path", "destination": "/development/api-routes/:path" + }, + { + "source": "/modules/products/serverless-module", + "destination": "/experimental/product/overview" } ], "framework": "docusaurus-2",