diff --git "a/problems/0001.\344\270\244\346\225\260\344\271\213\345\222\214.md" "b/problems/0001.\344\270\244\346\225\260\344\271\213\345\222\214.md" index 712bc3f0df..4e44d0c3f6 100644 --- "a/problems/0001.\344\270\244\346\225\260\344\271\213\345\222\214.md" +++ "b/problems/0001.\344\270\244\346\225\260\344\271\213\345\222\214.md" @@ -37,20 +37,20 @@ [242. 有效的字母异位词](https://www.programmercarl.com/0242.有效的字母异位词.html) 这道题目是用数组作为哈希表来解决哈希问题,[349. 两个数组的交集](https://www.programmercarl.com/0349.两个数组的交集.html)这道题目是通过set作为哈希表来解决哈希问题。 -首先我在强调一下 **什么时候使用哈希法**,当我们需要查询一个元素是否出现过,或者一个元素是否在集合里的时候,就要第一时间想到哈希法。 +首先我再强调一下 **什么时候使用哈希法**,当我们需要查询一个元素是否出现过,或者一个元素是否在集合里的时候,就要第一时间想到哈希法。 本题呢,我就需要一个集合来存放我们遍历过的元素,然后在遍历数组的时候去询问这个集合,某元素是否遍历过,也就是 是否出现在这个集合。 那么我们就应该想到使用哈希法了。 -因为本地,我们不仅要知道元素有没有遍历过,还要知道这个元素对应的下标,**需要使用 key value结构来存放,key来存元素,value来存下标,那么使用map正合适**。 +因为本题,我们不仅要知道元素有没有遍历过,还要知道这个元素对应的下标,**需要使用 key value结构来存放,key来存元素,value来存下标,那么使用map正合适**。 再来看一下使用数组和set来做哈希法的局限。 * 数组的大小是受限制的,而且如果元素很少,而哈希值太大会造成内存空间的浪费。 * set是一个集合,里面放的元素只能是一个key,而两数之和这道题目,不仅要判断y是否存在而且还要记录y的下标位置,因为要返回x 和 y的下标。所以set 也不能用。 -此时就要选择另一种数据结构:map ,map是一种key value的存储结构,可以用key保存数值,用value在保存数值所在的下标。 +此时就要选择另一种数据结构:map ,map是一种key value的存储结构,可以用key保存数值,用value再保存数值所在的下标。 C++中map,有三种类型: diff --git "a/problems/0015.\344\270\211\346\225\260\344\271\213\345\222\214.md" "b/problems/0015.\344\270\211\346\225\260\344\271\213\345\222\214.md" index 4951c90cf1..91fc9d685a 100644 --- "a/problems/0015.\344\270\211\346\225\260\344\271\213\345\222\214.md" +++ "b/problems/0015.\344\270\211\346\225\260\344\271\213\345\222\214.md" @@ -171,7 +171,7 @@ public: #### a的去重 -说道去重,其实主要考虑三个数的去重。 a, b ,c, 对应的就是 nums[i],nums[left],nums[right] +说到去重,其实主要考虑三个数的去重。 a, b ,c, 对应的就是 nums[i],nums[left],nums[right] a 如果重复了怎么办,a是nums里遍历的元素,那么应该直接跳过去。 @@ -181,7 +181,7 @@ a 如果重复了怎么办,a是nums里遍历的元素,那么应该直接跳 其实不一样! -都是和 nums[i]进行比较,是比较它的前一个,还是比较他的后一个。 +都是和 nums[i]进行比较,是比较它的前一个,还是比较它的后一个。 如果我们的写法是 这样: @@ -191,7 +191,7 @@ if (nums[i] == nums[i + 1]) { // 去重操作 } ``` -那就我们就把 三元组中出现重复元素的情况直接pass掉了。 例如{-1, -1 ,2} 这组数据,当遍历到第一个-1 的时候,判断 下一个也是-1,那这组数据就pass了。 +那我们就把 三元组中出现重复元素的情况直接pass掉了。 例如{-1, -1 ,2} 这组数据,当遍历到第一个-1 的时候,判断 下一个也是-1,那这组数据就pass了。 **我们要做的是 不能有重复的三元组,但三元组内的元素是可以重复的!** diff --git "a/problems/0018.\345\233\233\346\225\260\344\271\213\345\222\214.md" "b/problems/0018.\345\233\233\346\225\260\344\271\213\345\222\214.md" index 28c20b7acd..17715b2e6f 100644 --- "a/problems/0018.\345\233\233\346\225\260\344\271\213\345\222\214.md" +++ "b/problems/0018.\345\233\233\346\225\260\344\271\213\345\222\214.md" @@ -649,6 +649,54 @@ object Solution { } } ``` +### Ruby: + +```ruby +def four_sum(nums, target) + #结果集 + result = [] + nums = nums.sort! + + for i in 0..nums.size - 1 + return result if i > 0 && nums[i] > target && nums[i] >= 0 + #对a进行去重 + next if i > 0 && nums[i] == nums[i - 1] + + for j in i + 1..nums.size - 1 + break if nums[i] + nums[j] > target && nums[i] + nums[j] >= 0 + #对b进行去重 + next if j > i + 1 && nums[j] == nums[j - 1] + left = j + 1 + right = nums.size - 1 + while left < right + sum = nums[i] + nums[j] + nums[left] + nums[right] + if sum > target + right -= 1 + elsif sum < target + left += 1 + else + result << [nums[i], nums[j], nums[left], nums[right]] + + #对c进行去重 + while left < right && nums[left] == nums[left + 1] + left += 1 + end + + #对d进行去重 + while left < right && nums[right] == nums[right - 1] + right -= 1 + end + + right -= 1 + left += 1 + end + end + end + end + + return result +end +```
diff --git "a/problems/0072.\347\274\226\350\276\221\350\267\235\347\246\273.md" "b/problems/0072.\347\274\226\350\276\221\350\267\235\347\246\273.md"
index 1ed9a86083..2b5a1925dc 100644
--- "a/problems/0072.\347\274\226\350\276\221\350\267\235\347\246\273.md"
+++ "b/problems/0072.\347\274\226\350\276\221\350\267\235\347\246\273.md"
@@ -403,6 +403,64 @@ int minDistance(char * word1, char * word2){
}
```
+Rust:
+
+```rust
+impl Solution {
+ pub fn min_distance(word1: String, word2: String) -> i32 {
+ let mut dp = vec![vec![0; word2.len() + 1]; word1.len() + 1];
+ for i in 1..=word2.len() {
+ dp[0][i] = i;
+ }
+
+ for (j, v) in dp.iter_mut().enumerate().skip(1) {
+ v[0] = j;
+ }
+ for (i, char1) in word1.chars().enumerate() {
+ for (j, char2) in word2.chars().enumerate() {
+ if char1 == char2 {
+ dp[i + 1][j + 1] = dp[i][j];
+ continue;
+ }
+ dp[i + 1][j + 1] = dp[i][j + 1].min(dp[i + 1][j]).min(dp[i][j]) + 1;
+ }
+ }
+
+ dp[word1.len()][word2.len()] as i32
+ }
+}
+```
+
+> 一维 dp
+
+```rust
+impl Solution {
+ pub fn min_distance(word1: String, word2: String) -> i32 {
+ let mut dp = vec![0; word1.len() + 1];
+ for (i, v) in dp.iter_mut().enumerate().skip(1) {
+ *v = i;
+ }
+
+ for char2 in word2.chars() {
+ // 相当于 dp[i][0] 的初始化
+ let mut pre = dp[0];
+ dp[0] += 1; // j = 0, 将前 i 个字符变成空串的个数
+ for (j, char1) in word1.chars().enumerate() {
+ let temp = dp[j + 1];
+ if char1 == char2 {
+ dp[j + 1] = pre;
+ } else {
+ dp[j + 1] = dp[j + 1].min(dp[j]).min(pre) + 1;
+ }
+ pre = temp;
+ }
+ }
+
+ dp[word1.len()] as i32
+ }
+}
+```
+
diff --git "a/problems/0130.\350\242\253\345\233\264\347\273\225\347\232\204\345\214\272\345\237\237.md" "b/problems/0130.\350\242\253\345\233\264\347\273\225\347\232\204\345\214\272\345\237\237.md"
index e244873ba3..e8a1f02f69 100644
--- "a/problems/0130.\350\242\253\345\233\264\347\273\225\347\232\204\345\214\272\345\237\237.md"
+++ "b/problems/0130.\350\242\253\345\233\264\347\273\225\347\232\204\345\214\272\345\237\237.md"
@@ -385,6 +385,55 @@ class Solution {
}
}
```
+### Python3
+
+```Python
+// 深度优先遍历
+class Solution:
+ dir_list = [(0, 1), (0, -1), (1, 0), (-1, 0)]
+ def solve(self, board: List[List[str]]) -> None:
+ """
+ Do not return anything, modify board in-place instead.
+ """
+ row_size = len(board)
+ column_size = len(board[0])
+ visited = [[False] * column_size for _ in range(row_size)]
+ # 从边缘开始,将边缘相连的O改成A。然后遍历所有,将A改成O,O改成X
+ # 第一行和最后一行
+ for i in range(column_size):
+ if board[0][i] == "O" and not visited[0][i]:
+ self.dfs(board, 0, i, visited)
+ if board[row_size-1][i] == "O" and not visited[row_size-1][i]:
+ self.dfs(board, row_size-1, i, visited)
+
+ # 第一列和最后一列
+ for i in range(1, row_size-1):
+ if board[i][0] == "O" and not visited[i][0]:
+ self.dfs(board, i, 0, visited)
+ if board[i][column_size-1] == "O" and not visited[i][column_size-1]:
+ self.dfs(board, i, column_size-1, visited)
+
+ for i in range(row_size):
+ for j in range(column_size):
+ if board[i][j] == "A":
+ board[i][j] = "O"
+ elif board[i][j] == "O":
+ board[i][j] = "X"
+
+
+ def dfs(self, board, x, y, visited):
+ if visited[x][y] or board[x][y] == "X":
+ return
+ visited[x][y] = True
+ board[x][y] = "A"
+ for i in range(4):
+ new_x = x + self.dir_list[i][0]
+ new_y = y + self.dir_list[i][1]
+ if new_x >= len(board) or new_y >= len(board[0]) or new_x < 0 or new_y < 0:
+ continue
+ self.dfs(board, new_x, new_y, visited)
+
+```
diff --git "a/problems/0200.\345\262\233\345\261\277\346\225\260\351\207\217.\345\271\277\346\220\234\347\211\210.md" "b/problems/0200.\345\262\233\345\261\277\346\225\260\351\207\217.\345\271\277\346\220\234\347\211\210.md"
index cd3ae70d71..5b9d90aa31 100644
--- "a/problems/0200.\345\262\233\345\261\277\346\225\260\351\207\217.\345\271\277\346\220\234\347\211\210.md"
+++ "b/problems/0200.\345\262\233\345\261\277\346\225\260\351\207\217.\345\271\277\346\220\234\347\211\210.md"
@@ -197,6 +197,7 @@ class Solution {
}
```
+
### Python
BFS solution
```python
@@ -236,6 +237,7 @@ class Solution:
continue
q.append((next_i, next_j))
visited[next_i][next_j] = True
+
```
diff --git "a/problems/0200.\345\262\233\345\261\277\346\225\260\351\207\217.\346\267\261\346\220\234\347\211\210.md" "b/problems/0200.\345\262\233\345\261\277\346\225\260\351\207\217.\346\267\261\346\220\234\347\211\210.md"
index c30ace1999..f610e32330 100644
--- "a/problems/0200.\345\262\233\345\261\277\346\225\260\351\207\217.\346\267\261\346\220\234\347\211\210.md"
+++ "b/problems/0200.\345\262\233\345\261\277\346\225\260\351\207\217.\346\267\261\346\220\234\347\211\210.md"
@@ -218,6 +218,67 @@ class Solution {
}
}
```
+
+Python:
+
+```python
+# 版本一
+class Solution:
+ def numIslands(self, grid: List[List[str]]) -> int:
+ m, n = len(grid), len(grid[0])
+ visited = [[False] * n for _ in range(m)]
+ dirs = [(-1, 0), (0, 1), (1, 0), (0, -1)] # 四个方向
+ result = 0
+
+ def dfs(x, y):
+ for d in dirs:
+ nextx = x + d[0]
+ nexty = y + d[1]
+ if nextx < 0 or nextx >= m or nexty < 0 or nexty >= n: # 越界了,直接跳过
+ continue
+ if not visited[nextx][nexty] and grid[nextx][nexty] == '1': # 没有访问过的同时是陆地的
+ visited[nextx][nexty] = True
+ dfs(nextx, nexty)
+
+ for i in range(m):
+ for j in range(n):
+ if not visited[i][j] and grid[i][j] == '1':
+ visited[i][j] = True
+ result += 1 # 遇到没访问过的陆地,+1
+ dfs(i, j) # 将与其链接的陆地都标记上 true
+
+ return result
+```
+
+```python
+# 版本二
+class Solution:
+ def numIslands(self, grid: List[List[str]]) -> int:
+ m, n = len(grid), len(grid[0])
+ visited = [[False] * n for _ in range(m)]
+ dirs = [(-1, 0), (0, 1), (1, 0), (0, -1)] # 四个方向
+ result = 0
+
+ def dfs(x, y):
+ if visited[x][y] or grid[x][y] == '0':
+ return # 终止条件:访问过的节点 或者 遇到海水
+ visited[x][y] = True
+ for d in dirs:
+ nextx = x + d[0]
+ nexty = y + d[1]
+ if nextx < 0 or nextx >= m or nexty < 0 or nexty >= n: # 越界了,直接跳过
+ continue
+ dfs(nextx, nexty)
+
+ for i in range(m):
+ for j in range(n):
+ if not visited[i][j] and grid[i][j] == '1':
+ result += 1 # 遇到没访问过的陆地,+1
+ dfs(i, j) # 将与其链接的陆地都标记上 true
+
+ return result
+```
+
diff --git "a/problems/0454.\345\233\233\346\225\260\347\233\270\345\212\240II.md" "b/problems/0454.\345\233\233\346\225\260\347\233\270\345\212\240II.md"
index 90c3733449..1c262c873d 100644
--- "a/problems/0454.\345\233\233\346\225\260\347\233\270\345\212\240II.md"
+++ "b/problems/0454.\345\233\233\346\225\260\347\233\270\345\212\240II.md"
@@ -41,7 +41,7 @@
## 思路
-本题咋眼一看好像和[0015.三数之和](https://programmercarl.com/0015.三数之和.html),[0018.四数之和](https://programmercarl.com/0018.四数之和.html)差不多,其实差很多。
+本题乍眼一看好像和[0015.三数之和](https://programmercarl.com/0015.三数之和.html),[0018.四数之和](https://programmercarl.com/0018.四数之和.html)差不多,其实差很多。
**本题是使用哈希法的经典题目,而[0015.三数之和](https://programmercarl.com/0015.三数之和.html),[0018.四数之和](https://programmercarl.com/0018.四数之和.html)并不合适使用哈希法**,因为三数之和和四数之和这两道题目使用哈希法在不超时的情况下做到对结果去重是很困难的,很有多细节需要处理。
diff --git "a/problems/0459.\351\207\215\345\244\215\347\232\204\345\255\220\345\255\227\347\254\246\344\270\262.md" "b/problems/0459.\351\207\215\345\244\215\347\232\204\345\255\220\345\255\227\347\254\246\344\270\262.md"
index f99102ab45..177c3878ba 100644
--- "a/problems/0459.\351\207\215\345\244\215\347\232\204\345\255\220\345\255\227\347\254\246\344\270\262.md"
+++ "b/problems/0459.\351\207\215\345\244\215\347\232\204\345\255\220\345\255\227\347\254\246\344\270\262.md"
@@ -52,7 +52,7 @@
也就是由前后相同的子串组成。
-那么既然前面有相同的子串,后面有相同的子串,用 s + s,这样组成的字符串中,后面的子串做前串,前后的子串做后串,就一定还能组成一个s,如图:
+那么既然前面有相同的子串,后面有相同的子串,用 s + s,这样组成的字符串中,后面的子串做前串,前面的子串做后串,就一定还能组成一个s,如图:
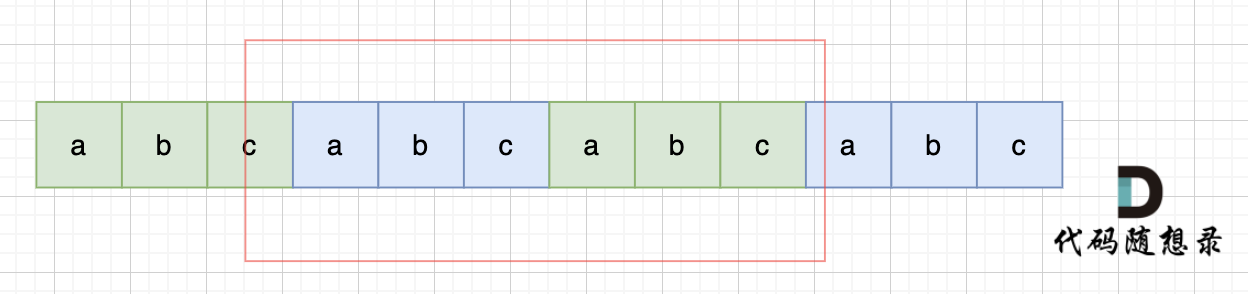
diff --git "a/problems/0463.\345\262\233\345\261\277\347\232\204\345\221\250\351\225\277.md" "b/problems/0463.\345\262\233\345\261\277\347\232\204\345\221\250\351\225\277.md"
index 14fa98dc10..48e64d0bd2 100644
--- "a/problems/0463.\345\262\233\345\261\277\347\232\204\345\221\250\351\225\277.md"
+++ "b/problems/0463.\345\262\233\345\261\277\347\232\204\345\221\250\351\225\277.md"
@@ -302,6 +302,104 @@ var islandPerimeter = function(grid) {
};
```
+TypeScript:
+
+```typescript
+/**
+ * 方法一:深度优先搜索(DFS)
+ * @param grid 二维网格地图,其中 grid[i][j] = 1 表示陆地, grid[i][j] = 0 表示水域
+ * @returns 岛屿的周长
+ */
+function islandPerimeter(grid: number[][]): number {
+ // 处理特殊情况:网格为空或行列数为 0,直接返回 0
+ if (!grid || grid.length === 0 || grid[0].length === 0) {
+ return 0;
+ }
+
+ // 获取网格的行数和列数
+ const rows = grid.length;
+ const cols = grid[0].length;
+ let perimeter = 0; // 岛屿的周长
+
+ /**
+ * 深度优先搜索函数
+ * @param i 当前格子的行索引
+ * @param j 当前格子的列索引
+ */
+ const dfs = (i: number, j: number) => {
+ // 如果当前位置超出网格范围,或者当前位置是水域(grid[i][j] === 0),则周长增加1
+ if (i < 0 || i >= rows || j < 0 || j >= cols || grid[i][j] === 0) {
+ perimeter++;
+ return;
+ }
+
+ // 如果当前位置已经访问过(grid[i][j] === -1),则直接返回
+ if (grid[i][j] === -1) {
+ return;
+ }
+
+ // 标记当前位置为已访问(-1),避免重复计算
+ grid[i][j] = -1;
+
+ // 继续搜索上、下、左、右四个方向
+ dfs(i + 1, j);
+ dfs(i - 1, j);
+ dfs(i, j + 1);
+ dfs(i, j - 1);
+ };
+
+ // 遍历整个网格,找到第一个陆地格子(grid[i][j] === 1),并以此为起点进行深度优先搜索
+ for (let i = 0; i < rows; i++) {
+ for (let j = 0; j < cols; j++) {
+ if (grid[i][j] === 1) {
+ dfs(i, j);
+ break;
+ }
+ }
+ }
+
+ return perimeter;
+}
+
+/**
+ * 方法二:遍历每个陆地格子,统计周长
+ * @param grid 二维网格地图,其中 grid[i][j] = 1 表示陆地, grid[i][j] = 0 表示水域
+ * @returns 岛屿的周长
+ */
+function islandPerimeter(grid: number[][]): number {
+ // 处理特殊情况:网格为空或行列数为 0,直接返回 0
+ if (!grid || grid.length === 0 || grid[0].length === 0) {
+ return 0;
+ }
+
+ // 获取网格的行数和列数
+ const rows = grid.length;
+ const cols = grid[0].length;
+ let perimeter = 0; // 岛屿的周长
+
+ // 遍历整个网格
+ for (let i = 0; i < rows; i++) {
+ for (let j = 0; j < cols; j++) {
+ // 如果当前格子是陆地(grid[i][j] === 1)
+ if (grid[i][j] === 1) {
+ perimeter += 4; // 周长先加上4个边
+
+ // 判断当前格子的上方是否也是陆地,如果是,则周长减去2个边
+ if (i > 0 && grid[i - 1][j] === 1) {
+ perimeter -= 2;
+ }
+
+ // 判断当前格子的左方是否也是陆地,如果是,则周长减去2个边
+ if (j > 0 && grid[i][j - 1] === 1) {
+ perimeter -= 2;
+ }
+ }
+ }
+ }
+
+ return perimeter;
+}
+```
diff --git "a/problems/0516.\346\234\200\351\225\277\345\233\236\346\226\207\345\255\220\345\272\217\345\210\227.md" "b/problems/0516.\346\234\200\351\225\277\345\233\236\346\226\207\345\255\220\345\272\217\345\210\227.md"
index 7df2aa3d15..44bdec1f19 100644
--- "a/problems/0516.\346\234\200\351\225\277\345\233\236\346\226\207\345\255\220\345\272\217\345\210\227.md"
+++ "b/problems/0516.\346\234\200\351\225\277\345\233\236\346\226\207\345\255\220\345\272\217\345\210\227.md"
@@ -276,7 +276,26 @@ function longestPalindromeSubseq(s: string): number {
};
```
-
+Rust:
+
+```rust
+impl Solution {
+ pub fn longest_palindrome_subseq(s: String) -> i32 {
+ let mut dp = vec![vec![0; s.len()]; s.len()];
+ for i in (0..s.len()).rev() {
+ dp[i][i] = 1;
+ for j in i + 1..s.len() {
+ if s[i..=i] == s[j..=j] {
+ dp[i][j] = dp[i + 1][j - 1] + 2;
+ continue;
+ }
+ dp[i][j] = dp[i + 1][j].max(dp[i][j - 1]);
+ }
+ }
+ dp[0][s.len() - 1]
+ }
+}
+```
diff --git "a/problems/0583.\344\270\244\344\270\252\345\255\227\347\254\246\344\270\262\347\232\204\345\210\240\351\231\244\346\223\215\344\275\234.md" "b/problems/0583.\344\270\244\344\270\252\345\255\227\347\254\246\344\270\262\347\232\204\345\210\240\351\231\244\346\223\215\344\275\234.md"
index 505a4e33ee..7dbb8ef542 100644
--- "a/problems/0583.\344\270\244\344\270\252\345\255\227\347\254\246\344\270\262\347\232\204\345\210\240\351\231\244\346\223\215\344\275\234.md"
+++ "b/problems/0583.\344\270\244\344\270\252\345\255\227\347\254\246\344\270\262\347\232\204\345\210\240\351\231\244\346\223\215\344\275\234.md"
@@ -370,7 +370,51 @@ function minDistance(word1: string, word2: string): number {
};
```
+Rust:
+
+```rust
+impl Solution {
+ pub fn min_distance(word1: String, word2: String) -> i32 {
+ let mut dp = vec![vec![0; word2.len() + 1]; word1.len() + 1];
+ for i in 0..word1.len() {
+ dp[i + 1][0] = i + 1;
+ }
+ for j in 0..word2.len() {
+ dp[0][j + 1] = j + 1;
+ }
+ for (i, char1) in word1.chars().enumerate() {
+ for (j, char2) in word2.chars().enumerate() {
+ if char1 == char2 {
+ dp[i + 1][j + 1] = dp[i][j];
+ continue;
+ }
+ dp[i + 1][j + 1] = dp[i][j + 1].min(dp[i + 1][j]) + 1;
+ }
+ }
+ dp[word1.len()][word2.len()] as i32
+ }
+}
+```
+> 版本 2
+
+```rust
+impl Solution {
+ pub fn min_distance(word1: String, word2: String) -> i32 {
+ let mut dp = vec![vec![0; word2.len() + 1]; word1.len() + 1];
+ for (i, char1) in word1.chars().enumerate() {
+ for (j, char2) in word2.chars().enumerate() {
+ if char1 == char2 {
+ dp[i + 1][j + 1] = dp[i][j] + 1;
+ continue;
+ }
+ dp[i + 1][j + 1] = dp[i][j + 1].max(dp[i + 1][j]);
+ }
+ }
+ (word1.len() + word2.len() - 2 * dp[word1.len()][word2.len()]) as i32
+ }
+}
+```
diff --git "a/problems/0647.\345\233\236\346\226\207\345\255\220\344\270\262.md" "b/problems/0647.\345\233\236\346\226\207\345\255\220\344\270\262.md"
index 0ed49bc1af..d94d18c5c0 100644
--- "a/problems/0647.\345\233\236\346\226\207\345\255\220\344\270\262.md"
+++ "b/problems/0647.\345\233\236\346\226\207\345\255\220\344\270\262.md"
@@ -525,8 +525,51 @@ function expandRange(s: string, left: number, right: number): number {
}
```
+Rust:
+```rust
+impl Solution {
+ pub fn count_substrings(s: String) -> i32 {
+ let mut dp = vec![vec![false; s.len()]; s.len()];
+ let mut res = 0;
+ for i in (0..s.len()).rev() {
+ for j in i..s.len() {
+ if s[i..=i] == s[j..=j] && (j - i <= 1 || dp[i + 1][j - 1]) {
+ dp[i][j] = true;
+ res += 1;
+ }
+ }
+ }
+ res
+ }
+}
+```
+
+> 双指针
+
+```rust
+impl Solution {
+ pub fn count_substrings(s: String) -> i32 {
+ let mut res = 0;
+ for i in 0..s.len() {
+ res += Self::extend(&s, i, i, s.len());
+ res += Self::extend(&s, i, i + 1, s.len());
+ }
+ res
+ }
+
+ fn extend(s: &str, mut i: usize, mut j: usize, len: usize) -> i32 {
+ let mut res = 0;
+ while i < len && j < len && s[i..=i] == s[j..=j] {
+ res += 1;
+ i = i.wrapping_sub(1);
+ j += 1;
+ }
+ res
+ }
+}
+```
diff --git "a/problems/0674.\346\234\200\351\225\277\350\277\236\347\273\255\351\200\222\345\242\236\345\272\217\345\210\227.md" "b/problems/0674.\346\234\200\351\225\277\350\277\236\347\273\255\351\200\222\345\242\236\345\272\217\345\210\227.md"
index 0c5e64b34e..485e321c99 100644
--- "a/problems/0674.\346\234\200\351\225\277\350\277\236\347\273\255\351\200\222\345\242\236\345\272\217\345\210\227.md"
+++ "b/problems/0674.\346\234\200\351\225\277\350\277\236\347\273\255\351\200\222\345\242\236\345\272\217\345\210\227.md"
@@ -302,8 +302,9 @@ func findLengthOfLCIS(nums []int) int {
}
```
-### Rust:
+### Rust:
+>动态规划
```rust
pub fn find_length_of_lcis(nums: Vec
diff --git "a/problems/0039.\347\273\204\345\220\210\346\200\273\345\222\214.md" "b/problems/0039.\347\273\204\345\220\210\346\200\273\345\222\214.md"
index 2a36ce5adb..37d5614e71 100644
--- "a/problems/0039.\347\273\204\345\220\210\346\200\273\345\222\214.md"
+++ "b/problems/0039.\347\273\204\345\220\210\346\200\273\345\222\214.md"
@@ -73,7 +73,7 @@ candidates 中的数字可以无限制重复被选取。
如果是多个集合取组合,各个集合之间相互不影响,那么就不用startIndex,例如:[17.电话号码的字母组合](https://programmercarl.com/0017.电话号码的字母组合.html)
-**注意以上我只是说求组合的情况,如果是排列问题,又是另一套分析的套路,后面我再讲解排列的时候就重点介绍**。
+**注意以上我只是说求组合的情况,如果是排列问题,又是另一套分析的套路,后面我在讲解排列的时候会重点介绍**。
代码如下:
@@ -311,7 +311,7 @@ class Solution:
for i in range(startIndex, len(candidates)):
if total + candidates[i] > target:
- break
+ continue
total += candidates[i]
path.append(candidates[i])
self.backtracking(candidates, target, total, i, path, result)
diff --git "a/problems/0054.\350\236\272\346\227\213\347\237\251\351\230\265.md" "b/problems/0054.\350\236\272\346\227\213\347\237\251\351\230\265.md"
index d855f1a166..c62eb2b12c 100644
--- "a/problems/0054.\350\236\272\346\227\213\347\237\251\351\230\265.md"
+++ "b/problems/0054.\350\236\272\346\227\213\347\237\251\351\230\265.md"
@@ -200,6 +200,58 @@ class Solution {
}
```
+### Javascript
+```
+/**
+ * @param {number[][]} matrix
+ * @return {number[]}
+ */
+var spiralOrder = function(matrix) {
+ let m = matrix.length
+ let n = matrix[0].length
+
+ let startX = startY = 0
+ let i = 0
+ let arr = new Array(m*n).fill(0)
+ let offset = 1
+ let loop = mid = Math.floor(Math.min(m,n) / 2)
+ while (loop--) {
+ let row = startX
+ let col = startY
+ // -->
+ for (; col < n + startY - offset; col++) {
+ arr[i++] = matrix[row][col]
+ }
+ // down
+ for (; row < m + startX - offset; row++) {
+ arr[i++] = matrix[row][col]
+ }
+ // <--
+ for (; col > startY; col--) {
+ arr[i++] = matrix[row][col]
+ }
+ for (; row > startX; row--) {
+ arr[i++] = matrix[row][col]
+ }
+ startX++
+ startY++
+ offset += 2
+ }
+ if (Math.min(m, n) % 2 === 1) {
+ if (n > m) {
+ for (let j = mid; j < mid + n - m + 1; j++) {
+ arr[i++] = matrix[mid][j]
+ }
+ } else {
+ for (let j = mid; j < mid + m - n + 1; j++) {
+ arr[i++] = matrix[j][mid]
+ }
+ }
+ }
+ return arr
+};
+```
+
diff --git "a/problems/0084.\346\237\261\347\212\266\345\233\276\344\270\255\346\234\200\345\244\247\347\232\204\347\237\251\345\275\242.md" "b/problems/0084.\346\237\261\347\212\266\345\233\276\344\270\255\346\234\200\345\244\247\347\232\204\347\237\251\345\275\242.md"
index 47ed0f3385..b54429ed22 100644
--- "a/problems/0084.\346\237\261\347\212\266\345\233\276\344\270\255\346\234\200\345\244\247\347\232\204\347\237\251\345\275\242.md"
+++ "b/problems/0084.\346\237\261\347\212\266\345\233\276\344\270\255\346\234\200\345\244\247\347\232\204\347\237\251\345\275\242.md"
@@ -729,62 +729,6 @@ impl Solution {
}
```
-Rust
-
-双指针预处理
-```rust
-
-impl Solution {
- pub fn largest_rectangle_area(v: Vec
diff --git "a/problems/0102.\344\272\214\345\217\211\346\240\221\347\232\204\345\261\202\345\272\217\351\201\215\345\216\206.md" "b/problems/0102.\344\272\214\345\217\211\346\240\221\347\232\204\345\261\202\345\272\217\351\201\215\345\216\206.md"
index ce9a247c1f..51d030e784 100644
--- "a/problems/0102.\344\272\214\345\217\211\346\240\221\347\232\204\345\261\202\345\272\217\351\201\215\345\216\206.md"
+++ "b/problems/0102.\344\272\214\345\217\211\346\240\221\347\232\204\345\261\202\345\272\217\351\201\215\345\216\206.md"
@@ -18,7 +18,7 @@
* [102.二叉树的层序遍历](https://leetcode.cn/problems/binary-tree-level-order-traversal/)
* [107.二叉树的层次遍历II](https://leetcode.cn/problems/binary-tree-level-order-traversal-ii/)
* [199.二叉树的右视图](https://leetcode.cn/problems/binary-tree-right-side-view/)
-* [637.二叉树的层平均值](https://leetcode.cn/problems/binary-tree-right-side-view/)
+* [637.二叉树的层平均值](https://leetcode.cn/problems/average-of-levels-in-binary-tree/)
* [429.N叉树的层序遍历](https://leetcode.cn/problems/n-ary-tree-level-order-traversal/)
* [515.在每个树行中找最大值](https://leetcode.cn/problems/find-largest-value-in-each-tree-row/)
* [116.填充每个节点的下一个右侧节点指针](https://leetcode.cn/problems/populating-next-right-pointers-in-each-node/)
diff --git "a/problems/0115.\344\270\215\345\220\214\347\232\204\345\255\220\345\272\217\345\210\227.md" "b/problems/0115.\344\270\215\345\220\214\347\232\204\345\255\220\345\272\217\345\210\227.md"
index b1de0cebbe..96ab2583f1 100644
--- "a/problems/0115.\344\270\215\345\220\214\347\232\204\345\255\220\345\272\217\345\210\227.md"
+++ "b/problems/0115.\344\270\215\345\220\214\347\232\204\345\255\220\345\272\217\345\210\227.md"
@@ -317,6 +317,63 @@ function numDistinct(s: string, t: string): number {
};
```
+### Rust:
+
+```rust
+impl Solution {
+ pub fn num_distinct(s: String, t: String) -> i32 {
+ if s.len() < t.len() {
+ return 0;
+ }
+ let mut dp = vec![vec![0; s.len() + 1]; t.len() + 1];
+ // i = 0, t 为空字符串,s 作为子序列的个数为 1(删除 s 所有元素)
+ dp[0] = vec![1; s.len() + 1];
+ for (i, char_t) in t.chars().enumerate() {
+ for (j, char_s) in s.chars().enumerate() {
+ if char_t == char_s {
+ // t 的前 i 个字符在 s 的前 j 个字符中作为子序列的个数
+ dp[i + 1][j + 1] = dp[i][j] + dp[i + 1][j];
+ continue;
+ }
+ dp[i + 1][j + 1] = dp[i + 1][j];
+ }
+ }
+ dp[t.len()][s.len()]
+ }
+}
+```
+
+> 滚动数组
+
+```rust
+impl Solution {
+ pub fn num_distinct(s: String, t: String) -> i32 {
+ if s.len() < t.len() {
+ return 0;
+ }
+ let (s, t) = (s.into_bytes(), t.into_bytes());
+ // 对于 t 为空字符串,s 作为子序列的个数为 1(删除 s 所有元素)
+ let mut dp = vec![1; s.len() + 1];
+ for char_t in t {
+ // dp[i - 1][j - 1],dp[j + 1] 更新之前的值
+ let mut pre = dp[0];
+ // 当开始遍历 t,s 的前 0 个字符无法包含任意子序列
+ dp[0] = 0;
+ for (j, &char_s) in s.iter().enumerate() {
+ let temp = dp[j + 1];
+ if char_t == char_s {
+ dp[j + 1] = pre + dp[j];
+ } else {
+ dp[j + 1] = dp[j];
+ }
+ pre = temp;
+ }
+ }
+ dp[s.len()]
+ }
+}
+```
+
diff --git "a/problems/0202.\345\277\253\344\271\220\346\225\260.md" "b/problems/0202.\345\277\253\344\271\220\346\225\260.md"
index 4a77e2b67c..719672a281 100644
--- "a/problems/0202.\345\277\253\344\271\220\346\225\260.md"
+++ "b/problems/0202.\345\277\253\344\271\220\346\225\260.md"
@@ -423,29 +423,61 @@ impl Solution {
### C:
```C
-typedef struct HashNodeTag {
- int key; /* num */
- struct HashNodeTag *next;
-}HashNode;
-
-/* Calcualte the hash key */
-static inline int hash(int key, int size) {
- int index = key % size;
- return (index > 0) ? (index) : (-index);
-}
-
-/* Calculate the sum of the squares of its digits*/
-static inline int calcSquareSum(int num) {
- unsigned int sum = 0;
- while(num > 0) {
- sum += (num % 10) * (num % 10);
- num = num/10;
+int get_sum(int n) {
+ int sum = 0;
+ div_t n_div = { .quot = n };
+ while (n_div.quot != 0) {
+ n_div = div(n_div.quot, 10);
+ sum += n_div.rem * n_div.rem;
}
return sum;
}
+// (版本1)使用数组
+bool isHappy(int n) {
+ // sum = a1^2 + a2^2 + ... ak^2
+ // first round:
+ // 1 <= k <= 10
+ // 1 <= sum <= 1 + 81 * 9 = 730
+ // second round:
+ // 1 <= k <= 3
+ // 1 <= sum <= 36 + 81 * 2 = 198
+ // third round:
+ // 1 <= sum <= 81 * 2 = 162
+ // fourth round:
+ // 1 <= sum <= 81 * 2 = 162
+
+ uint8_t visited[163] = { 0 };
+ int sum = get_sum(get_sum(n));
+ int next_n = sum;
+
+ while (next_n != 1) {
+ sum = get_sum(next_n);
+
+ if (visited[sum]) return false;
+
+ visited[sum] = 1;
+ next_n = sum;
+ };
+
+ return true;
+}
+
+// (版本2)使用快慢指针
+bool isHappy(int n) {
+ int slow = n;
+ int fast = n;
+
+ do {
+ slow = get_sum(slow);
+ fast = get_sum(get_sum(fast));
+ } while (slow != fast);
+
+ return (fast == 1);
+}
+```
-Scala:
+### Scala:
```scala
object Solution {
// 引入mutable
diff --git "a/problems/0236.\344\272\214\345\217\211\346\240\221\347\232\204\346\234\200\350\277\221\345\205\254\345\205\261\347\245\226\345\205\210.md" "b/problems/0236.\344\272\214\345\217\211\346\240\221\347\232\204\346\234\200\350\277\221\345\205\254\345\205\261\347\245\226\345\205\210.md"
index 9db7409e04..edb5ea3ea7 100644
--- "a/problems/0236.\344\272\214\345\217\211\346\240\221\347\232\204\346\234\200\350\277\221\345\205\254\345\205\261\347\245\226\345\205\210.md"
+++ "b/problems/0236.\344\272\214\345\217\211\346\240\221\347\232\204\346\234\200\350\277\221\345\205\254\345\205\261\347\245\226\345\205\210.md"
@@ -133,7 +133,7 @@ left与right的逻辑处理; // 中
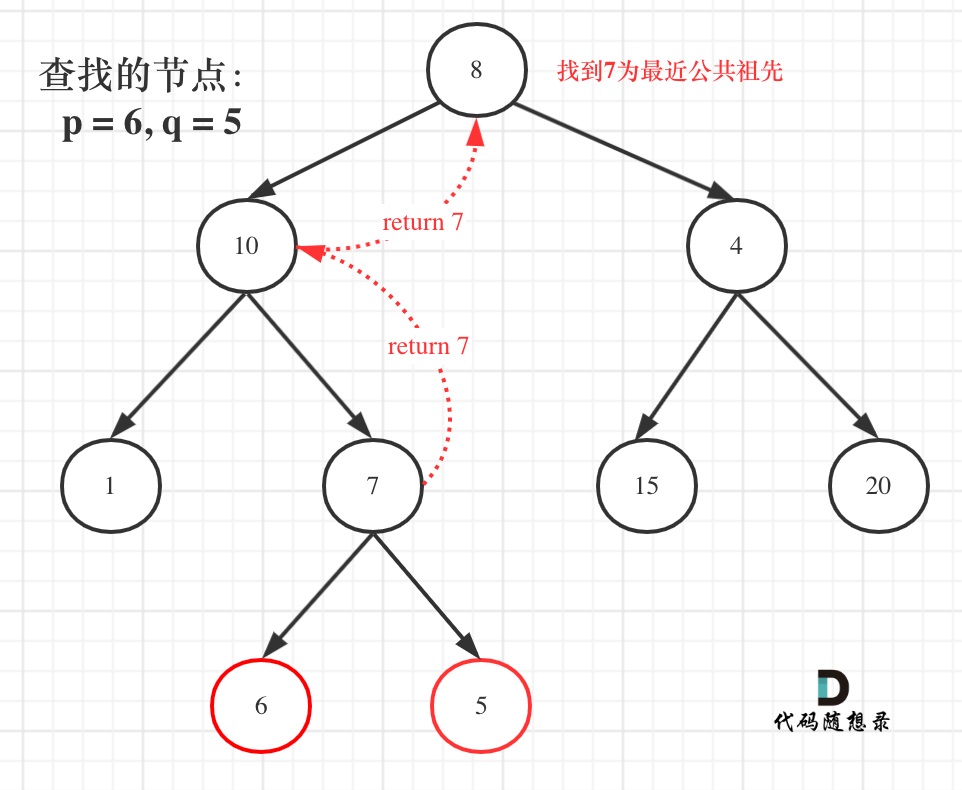
-就像图中一样直接返回7,多美滋滋。
+就像图中一样直接返回7。
但事实上还要遍历根节点右子树(即使此时已经找到了目标节点了),也就是图中的节点4、15、20。
diff --git "a/problems/0300.\346\234\200\351\225\277\344\270\212\345\215\207\345\255\220\345\272\217\345\210\227.md" "b/problems/0300.\346\234\200\351\225\277\344\270\212\345\215\207\345\255\220\345\272\217\345\210\227.md"
index 11cf13d9c5..677c72c6dd 100644
--- "a/problems/0300.\346\234\200\351\225\277\344\270\212\345\215\207\345\255\220\345\272\217\345\210\227.md"
+++ "b/problems/0300.\346\234\200\351\225\277\344\270\212\345\215\207\345\255\220\345\272\217\345\210\227.md"
@@ -130,18 +130,16 @@ public:
class Solution {
public int lengthOfLIS(int[] nums) {
int[] dp = new int[nums.length];
+ int res = 0;
Arrays.fill(dp, 1);
- for (int i = 0; i < dp.length; i++) {
+ for (int i = 1; i < dp.length; i++) {
for (int j = 0; j < i; j++) {
if (nums[i] > nums[j]) {
dp[i] = Math.max(dp[i], dp[j] + 1);
}
+ res = Math.max(res, dp[i]);
}
}
- int res = 0;
- for (int i = 0; i < dp.length; i++) {
- res = Math.max(res, dp[i]);
- }
return res;
}
}
@@ -294,7 +292,7 @@ function lengthOfLIS(nums: number[]): number {
```rust
pub fn length_of_lis(nums: Vec
diff --git "a/problems/0332.\351\207\215\346\226\260\345\256\211\346\216\222\350\241\214\347\250\213.md" "b/problems/0332.\351\207\215\346\226\260\345\256\211\346\216\222\350\241\214\347\250\213.md"
index 2795e31384..fcdeae7bde 100644
--- "a/problems/0332.\351\207\215\346\226\260\345\256\211\346\216\222\350\241\214\347\250\213.md"
+++ "b/problems/0332.\351\207\215\346\226\260\345\256\211\346\216\222\350\241\214\347\250\213.md"
@@ -237,13 +237,10 @@ public:
```cpp
for (pair
diff --git "a/problems/1035.\344\270\215\347\233\270\344\272\244\347\232\204\347\272\277.md" "b/problems/1035.\344\270\215\347\233\270\344\272\244\347\232\204\347\272\277.md"
index 74e94c842f..ff9e5dc4e3 100644
--- "a/problems/1035.\344\270\215\347\233\270\344\272\244\347\232\204\347\272\277.md"
+++ "b/problems/1035.\344\270\215\347\233\270\344\272\244\347\232\204\347\272\277.md"
@@ -155,21 +155,44 @@ func max(a, b int) int {
### Rust:
```rust
-pub fn max_uncrossed_lines(nums1: Vec