-
Notifications
You must be signed in to change notification settings - Fork 1
/
handlers.py
43 lines (36 loc) · 1.48 KB
/
handlers.py
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
#!/usr/bin/env python
# vim: set fileencoding=utf-8 :
# coding=utf-8
import json
import urllib
class SlashCommandHandler(object):
def handle(self, request):
raise NotImplementedError("Implement me!")
class EuroCommandHandler(SlashCommandHandler):
def format_latest_rate(self):
url = "http://stooq.pl/q/l/?s=eurpln&f=sd2t2ohlc&h&e=csv"
response = urllib.urlopen(url)
data = response.read()
rate = data.split("\r\n")[1].split(",")[-1]
date = data.split("\r\n")[1].split(",")[1]
return "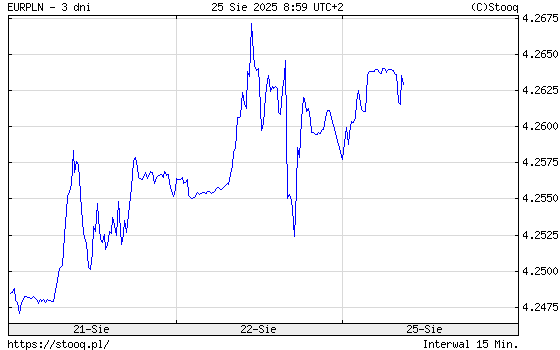\n\n" + self.format_rate_by_date(date, rate)
def handle(self, request):
if request.text:
date = request.text[0].encode("utf8")
rate = self.get_euro_rate_by_date(date)
stripped_date = date.replace("-", "")
formatted = self.format_rate_by_date(date, rate)
return ("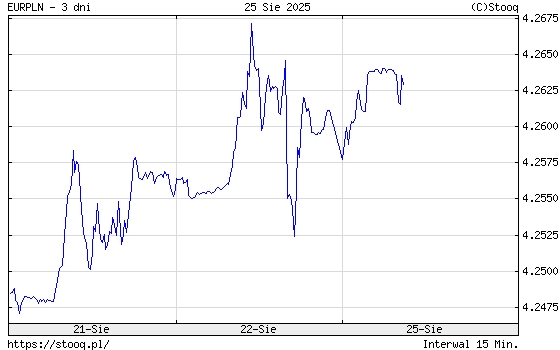\n\n" % stripped_date) + formatted
else:
return self.format_latest_rate()
def get_euro_rate_by_date(sefl, date):
url = "http://api.fixer.io/%s?symbols=PLN" % date
response = urllib.urlopen(url)
data = response.read()
rate = json.loads(data)["rates"]["PLN"]
return rate
def format_rate_by_date(self, date, rate):
formatted = """|Data | Kurs |
|:----------|----------:|
|%s | %s PLN|""" % (date, rate)
return formatted