You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
Hello, I am trying to generate Authorization token which I would like to re-use in order to grant tmp access to image files that I am storing on B2 bucket.
Here is my code:
type B2 struct {
ID string // unique identifier for B2 cloud storage
Key string // application key that controls access to B2 storage
BucketName string // name of the container that holds files
ObjectKey string // absolute path to specific file/directory inside given bucket
AuthToken string // authentication token which is used in order to grant access to bucket private files
Client *b2.Client // b2 client object
Context context.Context // environment context
Bucket *b2.Bucket // b2 bucket object
}
//
// Creates and initializes new B2 struct.
//
// Arguments:
// - id string: unique identifier for B2 cloud storage
// - key string: application key that controls access to B2 storage
// - bucket string: name of a bucket on b2 cloud storage
// - objectKey string: path to specific file or directory that is part of B2 bucket
//
// Returns:
// - Reference to new B2 instance
//
func NewB2Driver(id string, key string, bucketName string, objectKey string) (*B2, error) {
var b2Driver B2
b2Driver.Context = context.Background()
client, err := newB2Client(&b2Driver.Context, id, key)
if err != nil {
glog.Errorf("Failed to create new B2 client object, reason - %v", err)
return nil, err
}
b2Driver.Client = client
bucket, err := newB2Bucket(&b2Driver.Context, client, bucketName, objectKey)
if err != nil {
glog.Errorf("Failed to create new B2 bucket object, reason - %v", err)
return nil, err
}
b2Driver.Bucket = bucket
b2Driver.ID = id
b2Driver.BucketName = bucketName
b2Driver.Key = key
b2Driver.ObjectKey = objectKey
return &b2Driver, nil
}
func (b2Obj *B2) GenerateTmpAuthURL(prefix string, expireAfter time.Duration) (string, error) {
if b2Obj.AuthToken == "" {
authToken, err := b2Obj.Bucket.AuthToken(b2Obj.Context, prefix, expireAfter)
if err != nil {
glog.Errorf("Failed to generate temporary authorization token for B2 cloud storage, reason - %v", err)
return "", err
}
glog.V(2).Infof("[INFO]: Successfully generated tmp authorization token for B2 cloud storage. Token - %s", authToken)
b2Obj.AuthToken = authToken
}
objUrl := b2Obj.Bucket.Object(b2Obj.ObjectKey).URL()
objUrl = objUrl + "?Authorization=" + b2Obj.AuthToken
return objUrl, nil
}
And here is how resulting URL looks like once functions above are executed: https://f003.backblazeb2.com/file/cart-test-thumbnail-lambda-thumbnail-bucket/thumbnail_20181231235907_0_181094_0.jpeg?Authorization=3_20190917124617_ed5bc772862c3ab6ada9a331_0ed4df528f58a6e91bdee9dbb96a7425501100b6_003_20190917125117_0084_dnld
When I copy paste URL into the browser I get the following:
Is this suppose to happen? Shouldnt I have access to the file without having to SIGN IN? Also, whenever I enter my information I get back right to the same pop-up window, nothing happens. Its like I cannot sign in.
The text was updated successfully, but these errors were encountered:
Hello, I am trying to generate Authorization token which I would like to re-use in order to grant tmp access to image files that I am storing on B2 bucket.
Here is my code:
And here is how resulting URL looks like once functions above are executed:
https://f003.backblazeb2.com/file/cart-test-thumbnail-lambda-thumbnail-bucket/thumbnail_20181231235907_0_181094_0.jpeg?Authorization=3_20190917124617_ed5bc772862c3ab6ada9a331_0ed4df528f58a6e91bdee9dbb96a7425501100b6_003_20190917125117_0084_dnld
When I copy paste URL into the browser I get the following:
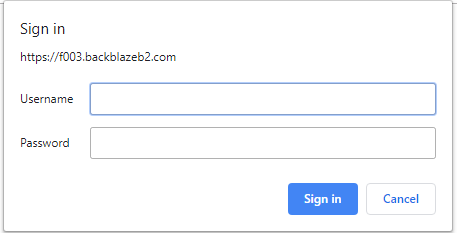
Is this suppose to happen? Shouldnt I have access to the file without having to SIGN IN? Also, whenever I enter my information I get back right to the same pop-up window, nothing happens. Its like I cannot sign in.
The text was updated successfully, but these errors were encountered: