|
| 1 | +# Auto Size Text Field |
| 2 | + |
| 3 | +*Note*: This work is inspired by [Auto Size Text](https://github.com/leisim/auto_size_text). Please check it out if you want to auto size the display text. |
| 4 | + |
| 5 | +Flutter TextField widget that automatically resizes text field to fit perfectly within its bounds. |
| 6 | + |
| 7 | + |
| 8 | + |
| 9 | +**Show some ❤️ and star the repo to support the project** |
| 10 | + |
| 11 | +## Contents |
| 12 | + |
| 13 | +- [Usage](#usage) |
| 14 | + - [maxLines](#maxlines) |
| 15 | + - [minFontSize & maxFontSize](#minfontsize--maxfontsize) |
| 16 | + - [stepGranularity](#stepgranularity) |
| 17 | + - [presetFontSizes](#presetfontsizes) |
| 18 | + - [overflowReplacement](#overflowreplacement) |
| 19 | +- [Parameters](#parameters) |
| 20 | +- [Performance](#performance) |
| 21 | +- [Troubleshooting](#roubleshooting) |
| 22 | + - [Missing bounds](#missing-bounds) |
| 23 | + - [MinFontSize too large](#minfontsize-too-large) |
| 24 | + |
| 25 | + |
| 26 | +## Usage |
| 27 | + |
| 28 | +`AutoSizeTextField` behaves exactly like a `TextField`. The only difference is that it resizes text to fit within its bounds. |
| 29 | + |
| 30 | +```dart |
| 31 | +AutoSizeTextField( |
| 32 | + 'The text to display', |
| 33 | + style: TextStyle(fontSize: 20), |
| 34 | + maxLines: 2, |
| 35 | +) |
| 36 | +``` |
| 37 | +**Note:** `AutoSizeTextField` needs bounded constraints to resize the text. More info [here](#troubleshooting). |
| 38 | + |
| 39 | +### maxLines |
| 40 | + |
| 41 | +The `maxLines` parameter works like you are used to with the `Text` widget. If there is no `maxLines` parameter specified, the `AutoSizeTextField` only fits the text according to the available width and height. |
| 42 | + |
| 43 | +```dart |
| 44 | +AutoSizeTextField( |
| 45 | + controller: _textEditingController, |
| 46 | + style: TextStyle(fontSize: 30), |
| 47 | + maxLines: 2, |
| 48 | +) |
| 49 | +``` |
| 50 | + |
| 51 | +*Sample above* |
| 52 | + |
| 53 | + |
| 54 | +### minFontSize & maxFontSize |
| 55 | + |
| 56 | +The `AutoSizeTextField` starts with `TextStyle.fontSize`. It measures the resulting text and rescales it to fit within its bonds. You can however set the allowed range of the resulting font size. |
| 57 | + |
| 58 | +With `minFontSize` you can specify the smallest possible font size. If the text still doesn't fit, it will be handled according to `overflow`. The default `minFontSize` is `12`. |
| 59 | + |
| 60 | +`maxFontSize` sets the largest possible font size. This is useful if the `TextStyle` inherits the font size and you want to constrain it. |
| 61 | + |
| 62 | +```dart |
| 63 | +AutoSizeTextField( |
| 64 | + controller: _textEditingController, |
| 65 | + style: TextStyle(fontSize: 30), |
| 66 | + minFontSize: 18, |
| 67 | + maxLines: 4, |
| 68 | + overflow: TextOverflow.ellipsis, |
| 69 | +) |
| 70 | +``` |
| 71 | + |
| 72 | +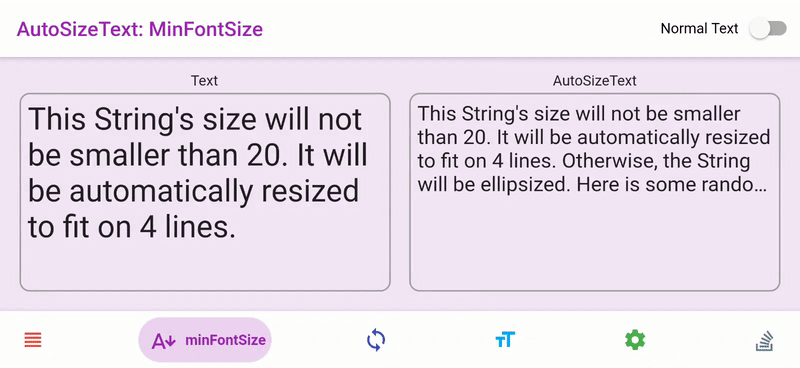 |
| 73 | + |
| 74 | +### stepGranularity |
| 75 | + |
| 76 | +The `AutoSizeTextField` will try each font size, starting with `TextStyle.fontSize` until the text fits within its bounds. |
| 77 | +`stepGranularity` specifies how much the font size is decreased each step. Usually, this value should not be below `1` for best performance. |
| 78 | + |
| 79 | +```dart |
| 80 | +AutoSizeTextField( |
| 81 | + controller: _textEditingController, |
| 82 | + style: TextStyle(fontSize: 40), |
| 83 | + minFontSize: 10, |
| 84 | + stepGranularity: 10, |
| 85 | + maxLines: 4, |
| 86 | + overflow: TextOverflow.ellipsis, |
| 87 | +) |
| 88 | +``` |
| 89 | + |
| 90 | +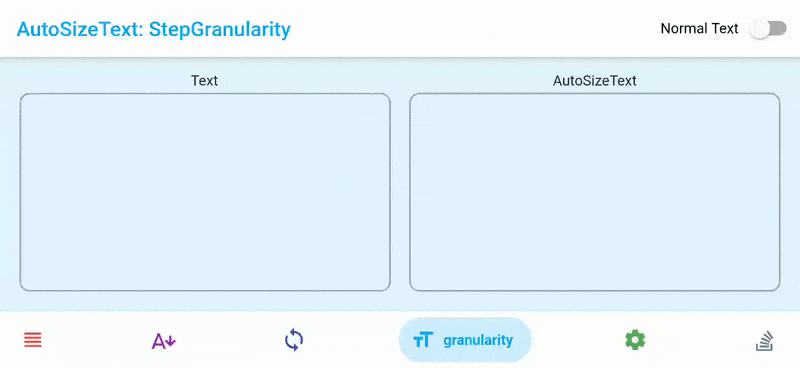 |
| 91 | + |
| 92 | + |
| 93 | +### presetFontSizes |
| 94 | + |
| 95 | +If you want to allow only specific font sizes, you can set them with `presetFontSizes`. |
| 96 | +If `presetFontSizes` is set, `minFontSize`, `maxFontSize` and `stepGranularity` will be ignored. |
| 97 | + |
| 98 | +```dart |
| 99 | +AutoSizeTextField( |
| 100 | + controller: _textEditingController, |
| 101 | + presetFontSizes: [40, 20, 14], |
| 102 | + maxLines: 4, |
| 103 | +) |
| 104 | +``` |
| 105 | + |
| 106 | +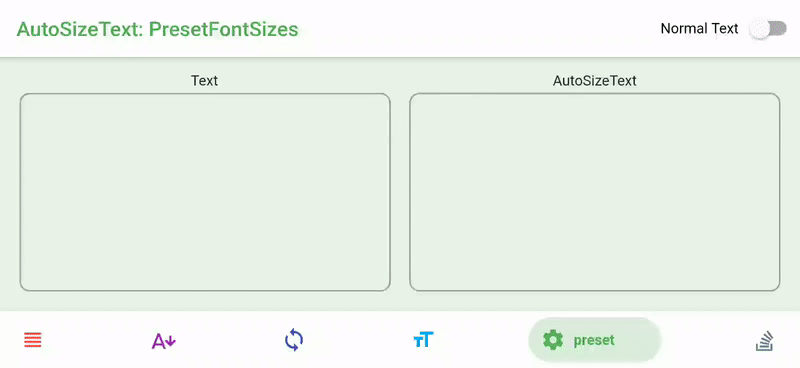 |
| 107 | + |
| 108 | + |
| 109 | +### overflowReplacement |
| 110 | + |
| 111 | +If the text is overflowing and does not fit its bounds, this widget is displayed instead. This can be useful to prevent text being too small to read. |
| 112 | + |
| 113 | +```dart |
| 114 | +AutoSizeTextField( |
| 115 | + maxLines: 1, |
| 116 | + controller: _textEditingController |
| 117 | +) |
| 118 | +``` |
| 119 | + |
| 120 | +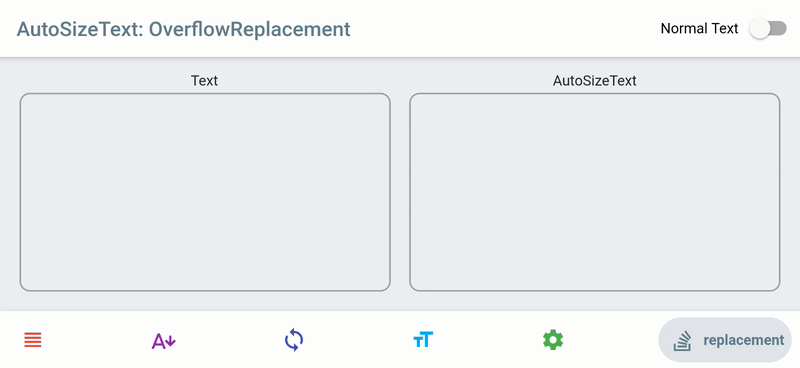 |
| 121 | + |
| 122 | +## Parameters |
| 123 | + |
| 124 | +| Parameter | Description | |
| 125 | +|---|---| |
| 126 | +| `key`* | Controls how one widget replaces another widget in the tree. | |
| 127 | +| `textKey` | Sets the key for the resulting `Text` widget | |
| 128 | +| `style`* | If non-null, the style to use for this text | |
| 129 | +| `minFontSize` | The **minimum** text size constraint to be used when auto-sizing text. <br>*Is being ignored if `presetFontSizes` is set.* | |
| 130 | +| `maxFontSize` | The **maximum** text size constraint to be used when auto-sizing text. <br>*Is being ignored if `presetFontSizes` is set.* | |
| 131 | +| `stepGranularity` | The step size in which the font size is being adapted to constraints. | |
| 132 | +| `presetFontSizes` | Predefines all the possible font sizes.<br> **Important:** `presetFontSizes` have to be in descending order. | |
| 133 | +| `textAlign`* | How the text should be aligned horizontally. | |
| 134 | +| `textDirection`* | The directionality of the text. This decides how `textAlign` values like `TextAlign.start` and `TextAlign.end` are interpreted. | |
| 135 | +| `locale`* | Used to select a font when the same Unicode character can be rendered differently, depending on the locale. | |
| 136 | +| `wrapWords` | Whether words which don't fit in one line should be wrapped. *Defaults to `true` to behave like `Text`.* | |
| 137 | +| `overflowReplacement` | If the text is overflowing and does not fit its bounds, this widget is displayed instead. | |
| 138 | +| `maxLines` | An optional maximum number of lines for the text to span. | |
| 139 | +| `semanticsLabel`* | An alternative semantics label for this text. | |
| 140 | + |
| 141 | +Parameters marked with \* behave exactly the same as in `Text` |
| 142 | + |
| 143 | + |
| 144 | +## Performance |
| 145 | + |
| 146 | +`AutoSizeTextField` is really fast. In fact, you can replace all your `Text` widgets with `AutoSizeTextField`.<br> |
| 147 | +Nevertheless you should not use an unreasonable high `fontSize` in your `TextStyle`. E.g. don't set the `fontSize` to `1000` if you know, that the text will never be larger than `30`. |
| 148 | + |
| 149 | +If your font size has a very large range, consider increasing `stepGranularity`. |
| 150 | + |
| 151 | + |
| 152 | +## Troubleshooting |
| 153 | + |
| 154 | +### Missing bounds |
| 155 | + |
| 156 | +If `AutoSizeTextField` overflows or does not resize the text, you should check if it has constrained width and height. |
| 157 | + |
| 158 | +**Wrong** code: |
| 159 | +```dart |
| 160 | +Row( |
| 161 | + children: <Widget>[ |
| 162 | + AutoSizeTextField( |
| 163 | + controller: _textEditingController, |
| 164 | + maxLines: 1, |
| 165 | + ), |
| 166 | + ], |
| 167 | +) |
| 168 | +``` |
| 169 | +Because `Row` and other widgets like `Container`, `Column` or `ListView` do not constrain their children, the text will overflow. |
| 170 | +You can fix this by constraining the `AutoSizeTextField`. Wrap it with `Expanded` in case of `Row` and `Column` or use a `SizedBox` or another widget with fixed width (and height). |
| 171 | + |
| 172 | +**Correct** code: |
| 173 | +```dart |
| 174 | +Row( |
| 175 | + children: <Widget>[ |
| 176 | + Expanded( // Constrains AutoSizeTextField to the width of the Row |
| 177 | + child: AutoSizeTextField( |
| 178 | + controller: _textEditingController, |
| 179 | + maxLines: 1, |
| 180 | + ) |
| 181 | + ), |
| 182 | + ], |
| 183 | +) |
| 184 | +} |
| 185 | +``` |
| 186 | + |
| 187 | +Further explanation can be found [here](https://stackoverflow.com/a/53908204). If you still have problems, please [open an issue](https://github.com/leisim/auto_size_text/issues/new). |
| 188 | + |
| 189 | + |
| 190 | +### MinFontSize too large |
| 191 | + |
| 192 | +`AutoSizeTextField` does not resize text below the `minFontSize` which defaults to 12.: |
| 193 | + |
| 194 | +## MIT License |
| 195 | +``` |
| 196 | +Copyright (c) 2020 Zhuoran LI |
| 197 | +
|
| 198 | +Permission is hereby granted, free of charge, to any person obtaining a copy |
| 199 | +of this software and associated documentation files (the 'Software'), to deal |
| 200 | +in the Software without restriction, including without limitation the rights |
| 201 | +to use, copy, modify, merge, publish, distribute, sublicense, and/or sell |
| 202 | +copies of the Software, and to permit persons to whom the Software is |
| 203 | +furnished to do so, subject to the following conditions: |
| 204 | +
|
| 205 | +The above copyright notice and this permission notice shall be included in all |
| 206 | +copies or substantial portions of the Software. |
| 207 | +
|
| 208 | +THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND, EXPRESS OR |
| 209 | +IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, |
| 210 | +FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE |
| 211 | +AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER |
| 212 | +LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, |
| 213 | +OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE |
| 214 | +SOFTWARE. |
| 215 | +``` |
0 commit comments