+
Twilito
+
+
A tiny, zero dependency helper for sending text messages with Twilio. Just enough of a wrapper to abstract away Twilio's REST API for sending messages, without anything else.
+
+

+
+
Why
+
+
Twilio's full Ruby library does a lot, and has a large memory footprint to go with it—too large for just sending a message. It's also more difficult to mock and verify in tests than I'd like.
+
+
Using Twilio’s REST API directly is fine, but can be cumbersome.
+
+
You should consider using Twilito if the only thing you need to do is send text messages and you don't want to worry about making HTTP requests to Twilio yourself.
+
+
If you use more of Twilio, consider twilio-ruby or interact with the REST API in another way.
+
+
Usage
+
+
Twilito should work on Ruby 2.4 and up.
+
+
Install the gem
+
+
gem 'twilito'
+
+
+
Simplest case
+
+
result = Twilito.send_sms(
+ to: '+15555555555',
+ from: '+15554444444',
+ content: 'This is my content',
+ account_sid: '...', auth_token: '...'
+)
+
+
+
+result.success? result.errors result.sid result.response result.data
+
+
Use send_sms! to raise on error instead
+
+
begin
+ Twilito.send_sms!(
+ to: '+15555555555',
+ from: '+12333',
+ body: 'This is my content',
+ account_sid: '...',
+ auth_token: '...'
+ )
+rescue Twilito::SendError => e
+ e.message e.response end
+
+
+
Every argument can be defaulted
+
+
+Twilito.configure do |config|
+ config.account_sid = ENV['TWILIO_ACCOUNT_SID']
+ config.auth_token = ENV['TWILIO_AUTH_TOKEN']
+
+ config.from = '+16145555555'
+end
+
+
+
+Twilito.send_sms!(to: '+15555555555', body: 'Foo')
+
+
+
Everything can be defaulted, including the message body, so that a bare Twilio.send_sms!
can work in your code
+
+
Sending MMS
+
+
+result = Twilito.send_sms(
+ to: '+15555555555',
+ content: 'This is my content',
+ media_url: 'https://example.com/image.png',
+)
+
+
+
Testing your code
+
+
TODO: Add examples of mocking and/or test helpers for asserting your code sends an SMS
+
+
Contributing
+
+
Contribute Feedback, Ideas, and Bug Reports
+
+
+
Contribute Code
+
-
+
Find an open issue or create one
+ -
+
Fork this repo and open a PR
+ -
+
Write unit tests for your change
+
+
+
Contribute Docs
+
-
+
Open a PR to make the README better, or help with contribution guidelines, etc.
+ -
+
There is currently an open issue for adding RDoc/YARD documentation
+
+
+
Contribute Beer
+
+
Did Twilito save you some RAM?
+
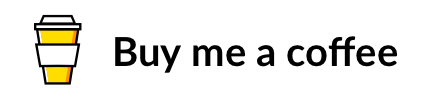