0) {
+ if(p < this.DB && (d = this[i]>>p) > 0) { m = true; r = int2char(d); }
+ while(i >= 0) {
+ if(p < k) {
+ d = (this[i]&((1<>(p+=this.DB-k);
+ }
+ else {
+ d = (this[i]>>(p-=k))&km;
+ if(p <= 0) { p += this.DB; --i; }
+ }
+ if(d > 0) m = true;
+ if(m) r += int2char(d);
+ }
+ }
+ return m?r:"0";
+ }
+
+ // (public) -this
+ function bnNegate() { var r = nbi(); BigInteger.ZERO.subTo(this,r); return r; }
+
+ // (public) |this|
+ function bnAbs() { return (this.s<0)?this.negate():this; }
+
+ // (public) return + if this > a, - if this < a, 0 if equal
+ function bnCompareTo(a) {
+ var r = this.s-a.s;
+ if(r != 0) return r;
+ var i = this.t;
+ r = i-a.t;
+ if(r != 0) return (this.s<0)?-r:r;
+ while(--i >= 0) if((r=this[i]-a[i]) != 0) return r;
+ return 0;
+ }
+
+ // returns bit length of the integer x
+ function nbits(x) {
+ var r = 1, t;
+ if((t=x>>>16) != 0) { x = t; r += 16; }
+ if((t=x>>8) != 0) { x = t; r += 8; }
+ if((t=x>>4) != 0) { x = t; r += 4; }
+ if((t=x>>2) != 0) { x = t; r += 2; }
+ if((t=x>>1) != 0) { x = t; r += 1; }
+ return r;
+ }
+
+ // (public) return the number of bits in "this"
+ function bnBitLength() {
+ if(this.t <= 0) return 0;
+ return this.DB*(this.t-1)+nbits(this[this.t-1]^(this.s&this.DM));
+ }
+
+ // (protected) r = this << n*DB
+ function bnpDLShiftTo(n,r) {
+ var i;
+ for(i = this.t-1; i >= 0; --i) r[i+n] = this[i];
+ for(i = n-1; i >= 0; --i) r[i] = 0;
+ r.t = this.t+n;
+ r.s = this.s;
+ }
+
+ // (protected) r = this >> n*DB
+ function bnpDRShiftTo(n,r) {
+ for(var i = n; i < this.t; ++i) r[i-n] = this[i];
+ r.t = Math.max(this.t-n,0);
+ r.s = this.s;
+ }
+
+ // (protected) r = this << n
+ function bnpLShiftTo(n,r) {
+ var bs = n%this.DB;
+ var cbs = this.DB-bs;
+ var bm = (1<= 0; --i) {
+ r[i+ds+1] = (this[i]>>cbs)|c;
+ c = (this[i]&bm)<= 0; --i) r[i] = 0;
+ r[ds] = c;
+ r.t = this.t+ds+1;
+ r.s = this.s;
+ r.clamp();
+ }
+
+ // (protected) r = this >> n
+ function bnpRShiftTo(n,r) {
+ r.s = this.s;
+ var ds = Math.floor(n/this.DB);
+ if(ds >= this.t) { r.t = 0; return; }
+ var bs = n%this.DB;
+ var cbs = this.DB-bs;
+ var bm = (1<>bs;
+ for(var i = ds+1; i < this.t; ++i) {
+ r[i-ds-1] |= (this[i]&bm)<>bs;
+ }
+ if(bs > 0) r[this.t-ds-1] |= (this.s&bm)<>= this.DB;
+ }
+ if(a.t < this.t) {
+ c -= a.s;
+ while(i < this.t) {
+ c += this[i];
+ r[i++] = c&this.DM;
+ c >>= this.DB;
+ }
+ c += this.s;
+ }
+ else {
+ c += this.s;
+ while(i < a.t) {
+ c -= a[i];
+ r[i++] = c&this.DM;
+ c >>= this.DB;
+ }
+ c -= a.s;
+ }
+ r.s = (c<0)?-1:0;
+ if(c < -1) r[i++] = this.DV+c;
+ else if(c > 0) r[i++] = c;
+ r.t = i;
+ r.clamp();
+ }
+
+ // (protected) r = this * a, r != this,a (HAC 14.12)
+ // "this" should be the larger one if appropriate.
+ function bnpMultiplyTo(a,r) {
+ var x = this.abs(), y = a.abs();
+ var i = x.t;
+ r.t = i+y.t;
+ while(--i >= 0) r[i] = 0;
+ for(i = 0; i < y.t; ++i) r[i+x.t] = x.am(0,y[i],r,i,0,x.t);
+ r.s = 0;
+ r.clamp();
+ if(this.s != a.s) BigInteger.ZERO.subTo(r,r);
+ }
+
+ // (protected) r = this^2, r != this (HAC 14.16)
+ function bnpSquareTo(r) {
+ var x = this.abs();
+ var i = r.t = 2*x.t;
+ while(--i >= 0) r[i] = 0;
+ for(i = 0; i < x.t-1; ++i) {
+ var c = x.am(i,x[i],r,2*i,0,1);
+ if((r[i+x.t]+=x.am(i+1,2*x[i],r,2*i+1,c,x.t-i-1)) >= x.DV) {
+ r[i+x.t] -= x.DV;
+ r[i+x.t+1] = 1;
+ }
+ }
+ if(r.t > 0) r[r.t-1] += x.am(i,x[i],r,2*i,0,1);
+ r.s = 0;
+ r.clamp();
+ }
+
+ // (protected) divide this by m, quotient and remainder to q, r (HAC 14.20)
+ // r != q, this != m. q or r may be null.
+ function bnpDivRemTo(m,q,r) {
+ var pm = m.abs();
+ if(pm.t <= 0) return;
+ var pt = this.abs();
+ if(pt.t < pm.t) {
+ if(q != null) q.fromInt(0);
+ if(r != null) this.copyTo(r);
+ return;
+ }
+ if(r == null) r = nbi();
+ var y = nbi(), ts = this.s, ms = m.s;
+ var nsh = this.DB-nbits(pm[pm.t-1]); // normalize modulus
+ if(nsh > 0) { pm.lShiftTo(nsh,y); pt.lShiftTo(nsh,r); }
+ else { pm.copyTo(y); pt.copyTo(r); }
+ var ys = y.t;
+ var y0 = y[ys-1];
+ if(y0 == 0) return;
+ var yt = y0*(1<1)?y[ys-2]>>this.F2:0);
+ var d1 = this.FV/yt, d2 = (1<= 0) {
+ r[r.t++] = 1;
+ r.subTo(t,r);
+ }
+ BigInteger.ONE.dlShiftTo(ys,t);
+ t.subTo(y,y); // "negative" y so we can replace sub with am later
+ while(y.t < ys) y[y.t++] = 0;
+ while(--j >= 0) {
+ // Estimate quotient digit
+ var qd = (r[--i]==y0)?this.DM:Math.floor(r[i]*d1+(r[i-1]+e)*d2);
+ if((r[i]+=y.am(0,qd,r,j,0,ys)) < qd) { // Try it out
+ y.dlShiftTo(j,t);
+ r.subTo(t,r);
+ while(r[i] < --qd) r.subTo(t,r);
+ }
+ }
+ if(q != null) {
+ r.drShiftTo(ys,q);
+ if(ts != ms) BigInteger.ZERO.subTo(q,q);
+ }
+ r.t = ys;
+ r.clamp();
+ if(nsh > 0) r.rShiftTo(nsh,r); // Denormalize remainder
+ if(ts < 0) BigInteger.ZERO.subTo(r,r);
+ }
+
+ // (public) this mod a
+ function bnMod(a) {
+ var r = nbi();
+ this.abs().divRemTo(a,null,r);
+ if(this.s < 0 && r.compareTo(BigInteger.ZERO) > 0) a.subTo(r,r);
+ return r;
+ }
+
+ // Modular reduction using "classic" algorithm
+ function Classic(m) { this.m = m; }
+ function cConvert(x) {
+ if(x.s < 0 || x.compareTo(this.m) >= 0) return x.mod(this.m);
+ else return x;
+ }
+ function cRevert(x) { return x; }
+ function cReduce(x) { x.divRemTo(this.m,null,x); }
+ function cMulTo(x,y,r) { x.multiplyTo(y,r); this.reduce(r); }
+ function cSqrTo(x,r) { x.squareTo(r); this.reduce(r); }
+
+ Classic.prototype.convert = cConvert;
+ Classic.prototype.revert = cRevert;
+ Classic.prototype.reduce = cReduce;
+ Classic.prototype.mulTo = cMulTo;
+ Classic.prototype.sqrTo = cSqrTo;
+
+ // (protected) return "-1/this % 2^DB"; useful for Mont. reduction
+ // justification:
+ // xy == 1 (mod m)
+ // xy = 1+km
+ // xy(2-xy) = (1+km)(1-km)
+ // x[y(2-xy)] = 1-k^2m^2
+ // x[y(2-xy)] == 1 (mod m^2)
+ // if y is 1/x mod m, then y(2-xy) is 1/x mod m^2
+ // should reduce x and y(2-xy) by m^2 at each step to keep size bounded.
+ // JS multiply "overflows" differently from C/C++, so care is needed here.
+ function bnpInvDigit() {
+ if(this.t < 1) return 0;
+ var x = this[0];
+ if((x&1) == 0) return 0;
+ var y = x&3; // y == 1/x mod 2^2
+ y = (y*(2-(x&0xf)*y))&0xf; // y == 1/x mod 2^4
+ y = (y*(2-(x&0xff)*y))&0xff; // y == 1/x mod 2^8
+ y = (y*(2-(((x&0xffff)*y)&0xffff)))&0xffff; // y == 1/x mod 2^16
+ // last step - calculate inverse mod DV directly;
+ // assumes 16 < DB <= 32 and assumes ability to handle 48-bit ints
+ y = (y*(2-x*y%this.DV))%this.DV; // y == 1/x mod 2^dbits
+ // we really want the negative inverse, and -DV < y < DV
+ return (y>0)?this.DV-y:-y;
+ }
+
+ // Montgomery reduction
+ function Montgomery(m) {
+ this.m = m;
+ this.mp = m.invDigit();
+ this.mpl = this.mp&0x7fff;
+ this.mph = this.mp>>15;
+ this.um = (1<<(m.DB-15))-1;
+ this.mt2 = 2*m.t;
+ }
+
+ // xR mod m
+ function montConvert(x) {
+ var r = nbi();
+ x.abs().dlShiftTo(this.m.t,r);
+ r.divRemTo(this.m,null,r);
+ if(x.s < 0 && r.compareTo(BigInteger.ZERO) > 0) this.m.subTo(r,r);
+ return r;
+ }
+
+ // x/R mod m
+ function montRevert(x) {
+ var r = nbi();
+ x.copyTo(r);
+ this.reduce(r);
+ return r;
+ }
+
+ // x = x/R mod m (HAC 14.32)
+ function montReduce(x) {
+ while(x.t <= this.mt2) // pad x so am has enough room later
+ x[x.t++] = 0;
+ for(var i = 0; i < this.m.t; ++i) {
+ // faster way of calculating u0 = x[i]*mp mod DV
+ var j = x[i]&0x7fff;
+ var u0 = (j*this.mpl+(((j*this.mph+(x[i]>>15)*this.mpl)&this.um)<<15))&x.DM;
+ // use am to combine the multiply-shift-add into one call
+ j = i+this.m.t;
+ x[j] += this.m.am(0,u0,x,i,0,this.m.t);
+ // propagate carry
+ while(x[j] >= x.DV) { x[j] -= x.DV; x[++j]++; }
+ }
+ x.clamp();
+ x.drShiftTo(this.m.t,x);
+ if(x.compareTo(this.m) >= 0) x.subTo(this.m,x);
+ }
+
+ // r = "x^2/R mod m"; x != r
+ function montSqrTo(x,r) { x.squareTo(r); this.reduce(r); }
+
+ // r = "xy/R mod m"; x,y != r
+ function montMulTo(x,y,r) { x.multiplyTo(y,r); this.reduce(r); }
+
+ Montgomery.prototype.convert = montConvert;
+ Montgomery.prototype.revert = montRevert;
+ Montgomery.prototype.reduce = montReduce;
+ Montgomery.prototype.mulTo = montMulTo;
+ Montgomery.prototype.sqrTo = montSqrTo;
+
+ // (protected) true iff this is even
+ function bnpIsEven() { return ((this.t>0)?(this[0]&1):this.s) == 0; }
+
+ // (protected) this^e, e < 2^32, doing sqr and mul with "r" (HAC 14.79)
+ function bnpExp(e,z) {
+ if(e > 0xffffffff || e < 1) return BigInteger.ONE;
+ var r = nbi(), r2 = nbi(), g = z.convert(this), i = nbits(e)-1;
+ g.copyTo(r);
+ while(--i >= 0) {
+ z.sqrTo(r,r2);
+ if((e&(1< 0) z.mulTo(r2,g,r);
+ else { var t = r; r = r2; r2 = t; }
+ }
+ return z.revert(r);
+ }
+
+ // (public) this^e % m, 0 <= e < 2^32
+ function bnModPowInt(e,m) {
+ var z;
+ if(e < 256 || m.isEven()) z = new Classic(m); else z = new Montgomery(m);
+ return this.exp(e,z);
+ }
+
+ // protected
+ BigInteger.prototype.copyTo = bnpCopyTo;
+ BigInteger.prototype.fromInt = bnpFromInt;
+ BigInteger.prototype.fromString = bnpFromString;
+ BigInteger.prototype.clamp = bnpClamp;
+ BigInteger.prototype.dlShiftTo = bnpDLShiftTo;
+ BigInteger.prototype.drShiftTo = bnpDRShiftTo;
+ BigInteger.prototype.lShiftTo = bnpLShiftTo;
+ BigInteger.prototype.rShiftTo = bnpRShiftTo;
+ BigInteger.prototype.subTo = bnpSubTo;
+ BigInteger.prototype.multiplyTo = bnpMultiplyTo;
+ BigInteger.prototype.squareTo = bnpSquareTo;
+ BigInteger.prototype.divRemTo = bnpDivRemTo;
+ BigInteger.prototype.invDigit = bnpInvDigit;
+ BigInteger.prototype.isEven = bnpIsEven;
+ BigInteger.prototype.exp = bnpExp;
+
+ // public
+ BigInteger.prototype.toString = bnToString;
+ BigInteger.prototype.negate = bnNegate;
+ BigInteger.prototype.abs = bnAbs;
+ BigInteger.prototype.compareTo = bnCompareTo;
+ BigInteger.prototype.bitLength = bnBitLength;
+ BigInteger.prototype.mod = bnMod;
+ BigInteger.prototype.modPowInt = bnModPowInt;
+
+ // "constants"
+ BigInteger.ZERO = nbv(0);
+ BigInteger.ONE = nbv(1);
+
+ // Copyright (c) 2005-2009 Tom Wu
+ // All Rights Reserved.
+ // See "LICENSE" for details.
+
+ // Extended JavaScript BN functions, required for RSA private ops.
+
+ // Version 1.1: new BigInteger("0", 10) returns "proper" zero
+ // Version 1.2: square() API, isProbablePrime fix
+
+ // (public)
+ function bnClone() { var r = nbi(); this.copyTo(r); return r; }
+
+ // (public) return value as integer
+ function bnIntValue() {
+ if(this.s < 0) {
+ if(this.t == 1) return this[0]-this.DV;
+ else if(this.t == 0) return -1;
+ }
+ else if(this.t == 1) return this[0];
+ else if(this.t == 0) return 0;
+ // assumes 16 < DB < 32
+ return ((this[1]&((1<<(32-this.DB))-1))<>24; }
+
+ // (public) return value as short (assumes DB>=16)
+ function bnShortValue() { return (this.t==0)?this.s:(this[0]<<16)>>16; }
+
+ // (protected) return x s.t. r^x < DV
+ function bnpChunkSize(r) { return Math.floor(Math.LN2*this.DB/Math.log(r)); }
+
+ // (public) 0 if this == 0, 1 if this > 0
+ function bnSigNum() {
+ if(this.s < 0) return -1;
+ else if(this.t <= 0 || (this.t == 1 && this[0] <= 0)) return 0;
+ else return 1;
+ }
+
+ // (protected) convert to radix string
+ function bnpToRadix(b) {
+ if(b == null) b = 10;
+ if(this.signum() == 0 || b < 2 || b > 36) return "0";
+ var cs = this.chunkSize(b);
+ var a = Math.pow(b,cs);
+ var d = nbv(a), y = nbi(), z = nbi(), r = "";
+ this.divRemTo(d,y,z);
+ while(y.signum() > 0) {
+ r = (a+z.intValue()).toString(b).substr(1) + r;
+ y.divRemTo(d,y,z);
+ }
+ return z.intValue().toString(b) + r;
+ }
+
+ // (protected) convert from radix string
+ function bnpFromRadix(s,b) {
+ this.fromInt(0);
+ if(b == null) b = 10;
+ var cs = this.chunkSize(b);
+ var d = Math.pow(b,cs), mi = false, j = 0, w = 0;
+ for(var i = 0; i < s.length; ++i) {
+ var x = intAt(s,i);
+ if(x < 0) {
+ if(s.charAt(i) == "-" && this.signum() == 0) mi = true;
+ continue;
+ }
+ w = b*w+x;
+ if(++j >= cs) {
+ this.dMultiply(d);
+ this.dAddOffset(w,0);
+ j = 0;
+ w = 0;
+ }
+ }
+ if(j > 0) {
+ this.dMultiply(Math.pow(b,j));
+ this.dAddOffset(w,0);
+ }
+ if(mi) BigInteger.ZERO.subTo(this,this);
+ }
+
+ // (protected) alternate constructor
+ function bnpFromNumber(a,b,c) {
+ if("number" == typeof b) {
+ // new BigInteger(int,int,RNG)
+ if(a < 2) this.fromInt(1);
+ else {
+ this.fromNumber(a,c);
+ if(!this.testBit(a-1)) // force MSB set
+ this.bitwiseTo(BigInteger.ONE.shiftLeft(a-1),op_or,this);
+ if(this.isEven()) this.dAddOffset(1,0); // force odd
+ while(!this.isProbablePrime(b)) {
+ this.dAddOffset(2,0);
+ if(this.bitLength() > a) this.subTo(BigInteger.ONE.shiftLeft(a-1),this);
+ }
+ }
+ }
+ else {
+ // new BigInteger(int,RNG)
+ var x = new Array(), t = a&7;
+ x.length = (a>>3)+1;
+ b.nextBytes(x);
+ if(t > 0) x[0] &= ((1< 0) {
+ if(p < this.DB && (d = this[i]>>p) != (this.s&this.DM)>>p)
+ r[k++] = d|(this.s<<(this.DB-p));
+ while(i >= 0) {
+ if(p < 8) {
+ d = (this[i]&((1<>(p+=this.DB-8);
+ }
+ else {
+ d = (this[i]>>(p-=8))&0xff;
+ if(p <= 0) { p += this.DB; --i; }
+ }
+ if((d&0x80) != 0) d |= -256;
+ if(k == 0 && (this.s&0x80) != (d&0x80)) ++k;
+ if(k > 0 || d != this.s) r[k++] = d;
+ }
+ }
+ return r;
+ }
+
+ function bnEquals(a) { return(this.compareTo(a)==0); }
+ function bnMin(a) { return(this.compareTo(a)<0)?this:a; }
+ function bnMax(a) { return(this.compareTo(a)>0)?this:a; }
+
+ // (protected) r = this op a (bitwise)
+ function bnpBitwiseTo(a,op,r) {
+ var i, f, m = Math.min(a.t,this.t);
+ for(i = 0; i < m; ++i) r[i] = op(this[i],a[i]);
+ if(a.t < this.t) {
+ f = a.s&this.DM;
+ for(i = m; i < this.t; ++i) r[i] = op(this[i],f);
+ r.t = this.t;
+ }
+ else {
+ f = this.s&this.DM;
+ for(i = m; i < a.t; ++i) r[i] = op(f,a[i]);
+ r.t = a.t;
+ }
+ r.s = op(this.s,a.s);
+ r.clamp();
+ }
+
+ // (public) this & a
+ function op_and(x,y) { return x&y; }
+ function bnAnd(a) { var r = nbi(); this.bitwiseTo(a,op_and,r); return r; }
+
+ // (public) this | a
+ function op_or(x,y) { return x|y; }
+ function bnOr(a) { var r = nbi(); this.bitwiseTo(a,op_or,r); return r; }
+
+ // (public) this ^ a
+ function op_xor(x,y) { return x^y; }
+ function bnXor(a) { var r = nbi(); this.bitwiseTo(a,op_xor,r); return r; }
+
+ // (public) this & ~a
+ function op_andnot(x,y) { return x&~y; }
+ function bnAndNot(a) { var r = nbi(); this.bitwiseTo(a,op_andnot,r); return r; }
+
+ // (public) ~this
+ function bnNot() {
+ var r = nbi();
+ for(var i = 0; i < this.t; ++i) r[i] = this.DM&~this[i];
+ r.t = this.t;
+ r.s = ~this.s;
+ return r;
+ }
+
+ // (public) this << n
+ function bnShiftLeft(n) {
+ var r = nbi();
+ if(n < 0) this.rShiftTo(-n,r); else this.lShiftTo(n,r);
+ return r;
+ }
+
+ // (public) this >> n
+ function bnShiftRight(n) {
+ var r = nbi();
+ if(n < 0) this.lShiftTo(-n,r); else this.rShiftTo(n,r);
+ return r;
+ }
+
+ // return index of lowest 1-bit in x, x < 2^31
+ function lbit(x) {
+ if(x == 0) return -1;
+ var r = 0;
+ if((x&0xffff) == 0) { x >>= 16; r += 16; }
+ if((x&0xff) == 0) { x >>= 8; r += 8; }
+ if((x&0xf) == 0) { x >>= 4; r += 4; }
+ if((x&3) == 0) { x >>= 2; r += 2; }
+ if((x&1) == 0) ++r;
+ return r;
+ }
+
+ // (public) returns index of lowest 1-bit (or -1 if none)
+ function bnGetLowestSetBit() {
+ for(var i = 0; i < this.t; ++i)
+ if(this[i] != 0) return i*this.DB+lbit(this[i]);
+ if(this.s < 0) return this.t*this.DB;
+ return -1;
+ }
+
+ // return number of 1 bits in x
+ function cbit(x) {
+ var r = 0;
+ while(x != 0) { x &= x-1; ++r; }
+ return r;
+ }
+
+ // (public) return number of set bits
+ function bnBitCount() {
+ var r = 0, x = this.s&this.DM;
+ for(var i = 0; i < this.t; ++i) r += cbit(this[i]^x);
+ return r;
+ }
+
+ // (public) true iff nth bit is set
+ function bnTestBit(n) {
+ var j = Math.floor(n/this.DB);
+ if(j >= this.t) return(this.s!=0);
+ return((this[j]&(1<<(n%this.DB)))!=0);
+ }
+
+ // (protected) this op (1<>= this.DB;
+ }
+ if(a.t < this.t) {
+ c += a.s;
+ while(i < this.t) {
+ c += this[i];
+ r[i++] = c&this.DM;
+ c >>= this.DB;
+ }
+ c += this.s;
+ }
+ else {
+ c += this.s;
+ while(i < a.t) {
+ c += a[i];
+ r[i++] = c&this.DM;
+ c >>= this.DB;
+ }
+ c += a.s;
+ }
+ r.s = (c<0)?-1:0;
+ if(c > 0) r[i++] = c;
+ else if(c < -1) r[i++] = this.DV+c;
+ r.t = i;
+ r.clamp();
+ }
+
+ // (public) this + a
+ function bnAdd(a) { var r = nbi(); this.addTo(a,r); return r; }
+
+ // (public) this - a
+ function bnSubtract(a) { var r = nbi(); this.subTo(a,r); return r; }
+
+ // (public) this * a
+ function bnMultiply(a) { var r = nbi(); this.multiplyTo(a,r); return r; }
+
+ // (public) this^2
+ function bnSquare() { var r = nbi(); this.squareTo(r); return r; }
+
+ // (public) this / a
+ function bnDivide(a) { var r = nbi(); this.divRemTo(a,r,null); return r; }
+
+ // (public) this % a
+ function bnRemainder(a) { var r = nbi(); this.divRemTo(a,null,r); return r; }
+
+ // (public) [this/a,this%a]
+ function bnDivideAndRemainder(a) {
+ var q = nbi(), r = nbi();
+ this.divRemTo(a,q,r);
+ return new Array(q,r);
+ }
+
+ // (protected) this *= n, this >= 0, 1 < n < DV
+ function bnpDMultiply(n) {
+ this[this.t] = this.am(0,n-1,this,0,0,this.t);
+ ++this.t;
+ this.clamp();
+ }
+
+ // (protected) this += n << w words, this >= 0
+ function bnpDAddOffset(n,w) {
+ if(n == 0) return;
+ while(this.t <= w) this[this.t++] = 0;
+ this[w] += n;
+ while(this[w] >= this.DV) {
+ this[w] -= this.DV;
+ if(++w >= this.t) this[this.t++] = 0;
+ ++this[w];
+ }
+ }
+
+ // A "null" reducer
+ function NullExp() {}
+ function nNop(x) { return x; }
+ function nMulTo(x,y,r) { x.multiplyTo(y,r); }
+ function nSqrTo(x,r) { x.squareTo(r); }
+
+ NullExp.prototype.convert = nNop;
+ NullExp.prototype.revert = nNop;
+ NullExp.prototype.mulTo = nMulTo;
+ NullExp.prototype.sqrTo = nSqrTo;
+
+ // (public) this^e
+ function bnPow(e) { return this.exp(e,new NullExp()); }
+
+ // (protected) r = lower n words of "this * a", a.t <= n
+ // "this" should be the larger one if appropriate.
+ function bnpMultiplyLowerTo(a,n,r) {
+ var i = Math.min(this.t+a.t,n);
+ r.s = 0; // assumes a,this >= 0
+ r.t = i;
+ while(i > 0) r[--i] = 0;
+ var j;
+ for(j = r.t-this.t; i < j; ++i) r[i+this.t] = this.am(0,a[i],r,i,0,this.t);
+ for(j = Math.min(a.t,n); i < j; ++i) this.am(0,a[i],r,i,0,n-i);
+ r.clamp();
+ }
+
+ // (protected) r = "this * a" without lower n words, n > 0
+ // "this" should be the larger one if appropriate.
+ function bnpMultiplyUpperTo(a,n,r) {
+ --n;
+ var i = r.t = this.t+a.t-n;
+ r.s = 0; // assumes a,this >= 0
+ while(--i >= 0) r[i] = 0;
+ for(i = Math.max(n-this.t,0); i < a.t; ++i)
+ r[this.t+i-n] = this.am(n-i,a[i],r,0,0,this.t+i-n);
+ r.clamp();
+ r.drShiftTo(1,r);
+ }
+
+ // Barrett modular reduction
+ function Barrett(m) {
+ // setup Barrett
+ this.r2 = nbi();
+ this.q3 = nbi();
+ BigInteger.ONE.dlShiftTo(2*m.t,this.r2);
+ this.mu = this.r2.divide(m);
+ this.m = m;
+ }
+
+ function barrettConvert(x) {
+ if(x.s < 0 || x.t > 2*this.m.t) return x.mod(this.m);
+ else if(x.compareTo(this.m) < 0) return x;
+ else { var r = nbi(); x.copyTo(r); this.reduce(r); return r; }
+ }
+
+ function barrettRevert(x) { return x; }
+
+ // x = x mod m (HAC 14.42)
+ function barrettReduce(x) {
+ x.drShiftTo(this.m.t-1,this.r2);
+ if(x.t > this.m.t+1) { x.t = this.m.t+1; x.clamp(); }
+ this.mu.multiplyUpperTo(this.r2,this.m.t+1,this.q3);
+ this.m.multiplyLowerTo(this.q3,this.m.t+1,this.r2);
+ while(x.compareTo(this.r2) < 0) x.dAddOffset(1,this.m.t+1);
+ x.subTo(this.r2,x);
+ while(x.compareTo(this.m) >= 0) x.subTo(this.m,x);
+ }
+
+ // r = x^2 mod m; x != r
+ function barrettSqrTo(x,r) { x.squareTo(r); this.reduce(r); }
+
+ // r = x*y mod m; x,y != r
+ function barrettMulTo(x,y,r) { x.multiplyTo(y,r); this.reduce(r); }
+
+ Barrett.prototype.convert = barrettConvert;
+ Barrett.prototype.revert = barrettRevert;
+ Barrett.prototype.reduce = barrettReduce;
+ Barrett.prototype.mulTo = barrettMulTo;
+ Barrett.prototype.sqrTo = barrettSqrTo;
+
+ // (public) this^e % m (HAC 14.85)
+ function bnModPow(e,m) {
+ var i = e.bitLength(), k, r = nbv(1), z;
+ if(i <= 0) return r;
+ else if(i < 18) k = 1;
+ else if(i < 48) k = 3;
+ else if(i < 144) k = 4;
+ else if(i < 768) k = 5;
+ else k = 6;
+ if(i < 8)
+ z = new Classic(m);
+ else if(m.isEven())
+ z = new Barrett(m);
+ else
+ z = new Montgomery(m);
+
+ // precomputation
+ var g = new Array(), n = 3, k1 = k-1, km = (1< 1) {
+ var g2 = nbi();
+ z.sqrTo(g[1],g2);
+ while(n <= km) {
+ g[n] = nbi();
+ z.mulTo(g2,g[n-2],g[n]);
+ n += 2;
+ }
+ }
+
+ var j = e.t-1, w, is1 = true, r2 = nbi(), t;
+ i = nbits(e[j])-1;
+ while(j >= 0) {
+ if(i >= k1) w = (e[j]>>(i-k1))&km;
+ else {
+ w = (e[j]&((1<<(i+1))-1))<<(k1-i);
+ if(j > 0) w |= e[j-1]>>(this.DB+i-k1);
+ }
+
+ n = k;
+ while((w&1) == 0) { w >>= 1; --n; }
+ if((i -= n) < 0) { i += this.DB; --j; }
+ if(is1) { // ret == 1, don't bother squaring or multiplying it
+ g[w].copyTo(r);
+ is1 = false;
+ }
+ else {
+ while(n > 1) { z.sqrTo(r,r2); z.sqrTo(r2,r); n -= 2; }
+ if(n > 0) z.sqrTo(r,r2); else { t = r; r = r2; r2 = t; }
+ z.mulTo(r2,g[w],r);
+ }
+
+ while(j >= 0 && (e[j]&(1< 0) {
+ x.rShiftTo(g,x);
+ y.rShiftTo(g,y);
+ }
+ while(x.signum() > 0) {
+ if((i = x.getLowestSetBit()) > 0) x.rShiftTo(i,x);
+ if((i = y.getLowestSetBit()) > 0) y.rShiftTo(i,y);
+ if(x.compareTo(y) >= 0) {
+ x.subTo(y,x);
+ x.rShiftTo(1,x);
+ }
+ else {
+ y.subTo(x,y);
+ y.rShiftTo(1,y);
+ }
+ }
+ if(g > 0) y.lShiftTo(g,y);
+ return y;
+ }
+
+ // (protected) this % n, n < 2^26
+ function bnpModInt(n) {
+ if(n <= 0) return 0;
+ var d = this.DV%n, r = (this.s<0)?n-1:0;
+ if(this.t > 0)
+ if(d == 0) r = this[0]%n;
+ else for(var i = this.t-1; i >= 0; --i) r = (d*r+this[i])%n;
+ return r;
+ }
+
+ // (public) 1/this % m (HAC 14.61)
+ function bnModInverse(m) {
+ var ac = m.isEven();
+ if((this.isEven() && ac) || m.signum() == 0) return BigInteger.ZERO;
+ var u = m.clone(), v = this.clone();
+ var a = nbv(1), b = nbv(0), c = nbv(0), d = nbv(1);
+ while(u.signum() != 0) {
+ while(u.isEven()) {
+ u.rShiftTo(1,u);
+ if(ac) {
+ if(!a.isEven() || !b.isEven()) { a.addTo(this,a); b.subTo(m,b); }
+ a.rShiftTo(1,a);
+ }
+ else if(!b.isEven()) b.subTo(m,b);
+ b.rShiftTo(1,b);
+ }
+ while(v.isEven()) {
+ v.rShiftTo(1,v);
+ if(ac) {
+ if(!c.isEven() || !d.isEven()) { c.addTo(this,c); d.subTo(m,d); }
+ c.rShiftTo(1,c);
+ }
+ else if(!d.isEven()) d.subTo(m,d);
+ d.rShiftTo(1,d);
+ }
+ if(u.compareTo(v) >= 0) {
+ u.subTo(v,u);
+ if(ac) a.subTo(c,a);
+ b.subTo(d,b);
+ }
+ else {
+ v.subTo(u,v);
+ if(ac) c.subTo(a,c);
+ d.subTo(b,d);
+ }
+ }
+ if(v.compareTo(BigInteger.ONE) != 0) return BigInteger.ZERO;
+ if(d.compareTo(m) >= 0) return d.subtract(m);
+ if(d.signum() < 0) d.addTo(m,d); else return d;
+ if(d.signum() < 0) return d.add(m); else return d;
+ }
+
+ var lowprimes = [2,3,5,7,11,13,17,19,23,29,31,37,41,43,47,53,59,61,67,71,73,79,83,89,97,101,103,107,109,113,127,131,137,139,149,151,157,163,167,173,179,181,191,193,197,199,211,223,227,229,233,239,241,251,257,263,269,271,277,281,283,293,307,311,313,317,331,337,347,349,353,359,367,373,379,383,389,397,401,409,419,421,431,433,439,443,449,457,461,463,467,479,487,491,499,503,509,521,523,541,547,557,563,569,571,577,587,593,599,601,607,613,617,619,631,641,643,647,653,659,661,673,677,683,691,701,709,719,727,733,739,743,751,757,761,769,773,787,797,809,811,821,823,827,829,839,853,857,859,863,877,881,883,887,907,911,919,929,937,941,947,953,967,971,977,983,991,997];
+ var lplim = (1<<26)/lowprimes[lowprimes.length-1];
+
+ // (public) test primality with certainty >= 1-.5^t
+ function bnIsProbablePrime(t) {
+ var i, x = this.abs();
+ if(x.t == 1 && x[0] <= lowprimes[lowprimes.length-1]) {
+ for(i = 0; i < lowprimes.length; ++i)
+ if(x[0] == lowprimes[i]) return true;
+ return false;
+ }
+ if(x.isEven()) return false;
+ i = 1;
+ while(i < lowprimes.length) {
+ var m = lowprimes[i], j = i+1;
+ while(j < lowprimes.length && m < lplim) m *= lowprimes[j++];
+ m = x.modInt(m);
+ while(i < j) if(m%lowprimes[i++] == 0) return false;
+ }
+ return x.millerRabin(t);
+ }
+
+ // (protected) true if probably prime (HAC 4.24, Miller-Rabin)
+ function bnpMillerRabin(t) {
+ var n1 = this.subtract(BigInteger.ONE);
+ var k = n1.getLowestSetBit();
+ if(k <= 0) return false;
+ var r = n1.shiftRight(k);
+ t = (t+1)>>1;
+ if(t > lowprimes.length) t = lowprimes.length;
+ var a = nbi();
+ for(var i = 0; i < t; ++i) {
+ //Pick bases at random, instead of starting at 2
+ a.fromInt(lowprimes[Math.floor(Math.random()*lowprimes.length)]);
+ var y = a.modPow(r,this);
+ if(y.compareTo(BigInteger.ONE) != 0 && y.compareTo(n1) != 0) {
+ var j = 1;
+ while(j++ < k && y.compareTo(n1) != 0) {
+ y = y.modPowInt(2,this);
+ if(y.compareTo(BigInteger.ONE) == 0) return false;
+ }
+ if(y.compareTo(n1) != 0) return false;
+ }
+ }
+ return true;
+ }
+
+ // protected
+ BigInteger.prototype.chunkSize = bnpChunkSize;
+ BigInteger.prototype.toRadix = bnpToRadix;
+ BigInteger.prototype.fromRadix = bnpFromRadix;
+ BigInteger.prototype.fromNumber = bnpFromNumber;
+ BigInteger.prototype.bitwiseTo = bnpBitwiseTo;
+ BigInteger.prototype.changeBit = bnpChangeBit;
+ BigInteger.prototype.addTo = bnpAddTo;
+ BigInteger.prototype.dMultiply = bnpDMultiply;
+ BigInteger.prototype.dAddOffset = bnpDAddOffset;
+ BigInteger.prototype.multiplyLowerTo = bnpMultiplyLowerTo;
+ BigInteger.prototype.multiplyUpperTo = bnpMultiplyUpperTo;
+ BigInteger.prototype.modInt = bnpModInt;
+ BigInteger.prototype.millerRabin = bnpMillerRabin;
+
+ // public
+ BigInteger.prototype.clone = bnClone;
+ BigInteger.prototype.intValue = bnIntValue;
+ BigInteger.prototype.byteValue = bnByteValue;
+ BigInteger.prototype.shortValue = bnShortValue;
+ BigInteger.prototype.signum = bnSigNum;
+ BigInteger.prototype.toByteArray = bnToByteArray;
+ BigInteger.prototype.equals = bnEquals;
+ BigInteger.prototype.min = bnMin;
+ BigInteger.prototype.max = bnMax;
+ BigInteger.prototype.and = bnAnd;
+ BigInteger.prototype.or = bnOr;
+ BigInteger.prototype.xor = bnXor;
+ BigInteger.prototype.andNot = bnAndNot;
+ BigInteger.prototype.not = bnNot;
+ BigInteger.prototype.shiftLeft = bnShiftLeft;
+ BigInteger.prototype.shiftRight = bnShiftRight;
+ BigInteger.prototype.getLowestSetBit = bnGetLowestSetBit;
+ BigInteger.prototype.bitCount = bnBitCount;
+ BigInteger.prototype.testBit = bnTestBit;
+ BigInteger.prototype.setBit = bnSetBit;
+ BigInteger.prototype.clearBit = bnClearBit;
+ BigInteger.prototype.flipBit = bnFlipBit;
+ BigInteger.prototype.add = bnAdd;
+ BigInteger.prototype.subtract = bnSubtract;
+ BigInteger.prototype.multiply = bnMultiply;
+ BigInteger.prototype.divide = bnDivide;
+ BigInteger.prototype.remainder = bnRemainder;
+ BigInteger.prototype.divideAndRemainder = bnDivideAndRemainder;
+ BigInteger.prototype.modPow = bnModPow;
+ BigInteger.prototype.modInverse = bnModInverse;
+ BigInteger.prototype.pow = bnPow;
+ BigInteger.prototype.gcd = bnGCD;
+ BigInteger.prototype.isProbablePrime = bnIsProbablePrime;
+
+ // JSBN-specific extension
+ BigInteger.prototype.square = bnSquare;
+
+ // Expose the Barrett function
+ BigInteger.prototype.Barrett = Barrett
+
+ // BigInteger interfaces not implemented in jsbn:
+
+ // BigInteger(int signum, byte[] magnitude)
+ // double doubleValue()
+ // float floatValue()
+ // int hashCode()
+ // long longValue()
+ // static BigInteger valueOf(long val)
+
+ // Random number generator - requires a PRNG backend, e.g. prng4.js
+
+ // For best results, put code like
+ //
+ // in your main HTML document.
+
+ var rng_state;
+ var rng_pool;
+ var rng_pptr;
+
+ // Mix in a 32-bit integer into the pool
+ function rng_seed_int(x) {
+ rng_pool[rng_pptr++] ^= x & 255;
+ rng_pool[rng_pptr++] ^= (x >> 8) & 255;
+ rng_pool[rng_pptr++] ^= (x >> 16) & 255;
+ rng_pool[rng_pptr++] ^= (x >> 24) & 255;
+ if(rng_pptr >= rng_psize) rng_pptr -= rng_psize;
+ }
+
+ // Mix in the current time (w/milliseconds) into the pool
+ function rng_seed_time() {
+ rng_seed_int(new Date().getTime());
+ }
+
+ // Initialize the pool with junk if needed.
+ if(rng_pool == null) {
+ rng_pool = new Array();
+ rng_pptr = 0;
+ var t;
+ if(typeof window !== "undefined" && window.crypto) {
+ if (window.crypto.getRandomValues) {
+ // Use webcrypto if available
+ var ua = new Uint8Array(32);
+ window.crypto.getRandomValues(ua);
+ for(t = 0; t < 32; ++t)
+ rng_pool[rng_pptr++] = ua[t];
+ }
+ else if(navigator.appName == "Netscape" && navigator.appVersion < "5") {
+ // Extract entropy (256 bits) from NS4 RNG if available
+ var z = window.crypto.random(32);
+ for(t = 0; t < z.length; ++t)
+ rng_pool[rng_pptr++] = z.charCodeAt(t) & 255;
+ }
+ }
+ while(rng_pptr < rng_psize) { // extract some randomness from Math.random()
+ t = Math.floor(65536 * Math.random());
+ rng_pool[rng_pptr++] = t >>> 8;
+ rng_pool[rng_pptr++] = t & 255;
+ }
+ rng_pptr = 0;
+ rng_seed_time();
+ //rng_seed_int(window.screenX);
+ //rng_seed_int(window.screenY);
+ }
+
+ function rng_get_byte() {
+ if(rng_state == null) {
+ rng_seed_time();
+ rng_state = prng_newstate();
+ rng_state.init(rng_pool);
+ for(rng_pptr = 0; rng_pptr < rng_pool.length; ++rng_pptr)
+ rng_pool[rng_pptr] = 0;
+ rng_pptr = 0;
+ //rng_pool = null;
+ }
+ // TODO: allow reseeding after first request
+ return rng_state.next();
+ }
+
+ function rng_get_bytes(ba) {
+ var i;
+ for(i = 0; i < ba.length; ++i) ba[i] = rng_get_byte();
+ }
+
+ function SecureRandom() {}
+
+ SecureRandom.prototype.nextBytes = rng_get_bytes;
+
+ // prng4.js - uses Arcfour as a PRNG
+
+ function Arcfour() {
+ this.i = 0;
+ this.j = 0;
+ this.S = new Array();
+ }
+
+ // Initialize arcfour context from key, an array of ints, each from [0..255]
+ function ARC4init(key) {
+ var i, j, t;
+ for(i = 0; i < 256; ++i)
+ this.S[i] = i;
+ j = 0;
+ for(i = 0; i < 256; ++i) {
+ j = (j + this.S[i] + key[i % key.length]) & 255;
+ t = this.S[i];
+ this.S[i] = this.S[j];
+ this.S[j] = t;
+ }
+ this.i = 0;
+ this.j = 0;
+ }
+
+ function ARC4next() {
+ var t;
+ this.i = (this.i + 1) & 255;
+ this.j = (this.j + this.S[this.i]) & 255;
+ t = this.S[this.i];
+ this.S[this.i] = this.S[this.j];
+ this.S[this.j] = t;
+ return this.S[(t + this.S[this.i]) & 255];
+ }
+
+ Arcfour.prototype.init = ARC4init;
+ Arcfour.prototype.next = ARC4next;
+
+ // Plug in your RNG constructor here
+ function prng_newstate() {
+ return new Arcfour();
+ }
+
+ // Pool size must be a multiple of 4 and greater than 32.
+ // An array of bytes the size of the pool will be passed to init()
+ var rng_psize = 256;
+
+ BigInteger.SecureRandom = SecureRandom;
+ BigInteger.BigInteger = BigInteger;
+ if (typeof exports !== 'undefined') {
+ exports = module.exports = BigInteger;
+ } else {
+ this.BigInteger = BigInteger;
+ this.SecureRandom = SecureRandom;
+ }
+
+}).call(this);
diff --git a/node_modules/jsbn/package.json b/node_modules/jsbn/package.json
new file mode 100644
index 0000000..792477c
--- /dev/null
+++ b/node_modules/jsbn/package.json
@@ -0,0 +1,90 @@
+{
+ "_args": [
+ [
+ {
+ "raw": "jsbn@~0.1.0",
+ "scope": null,
+ "escapedName": "jsbn",
+ "name": "jsbn",
+ "rawSpec": "~0.1.0",
+ "spec": ">=0.1.0 <0.2.0",
+ "type": "range"
+ },
+ "/Users/ramswaroop/MyProjects/RamAndAntara/node_modules/sshpk"
+ ]
+ ],
+ "_from": "jsbn@>=0.1.0 <0.2.0",
+ "_id": "jsbn@0.1.1",
+ "_inCache": true,
+ "_location": "/jsbn",
+ "_nodeVersion": "6.3.1",
+ "_npmOperationalInternal": {
+ "host": "packages-18-east.internal.npmjs.com",
+ "tmp": "tmp/jsbn-0.1.1.tgz_1486886593983_0.3002306066919118"
+ },
+ "_npmUser": {
+ "name": "andyperlitch",
+ "email": "andyperlitch@gmail.com"
+ },
+ "_npmVersion": "3.10.3",
+ "_phantomChildren": {},
+ "_requested": {
+ "raw": "jsbn@~0.1.0",
+ "scope": null,
+ "escapedName": "jsbn",
+ "name": "jsbn",
+ "rawSpec": "~0.1.0",
+ "spec": ">=0.1.0 <0.2.0",
+ "type": "range"
+ },
+ "_requiredBy": [
+ "/ecc-jsbn",
+ "/sshpk"
+ ],
+ "_resolved": "https://registry.npmjs.org/jsbn/-/jsbn-0.1.1.tgz",
+ "_shasum": "a5e654c2e5a2deb5f201d96cefbca80c0ef2f513",
+ "_shrinkwrap": null,
+ "_spec": "jsbn@~0.1.0",
+ "_where": "/Users/ramswaroop/MyProjects/RamAndAntara/node_modules/sshpk",
+ "author": {
+ "name": "Tom Wu"
+ },
+ "bugs": {
+ "url": "https://github.com/andyperlitch/jsbn/issues"
+ },
+ "dependencies": {},
+ "description": "The jsbn library is a fast, portable implementation of large-number math in pure JavaScript, enabling public-key crypto and other applications on desktop and mobile browsers.",
+ "devDependencies": {},
+ "directories": {},
+ "dist": {
+ "shasum": "a5e654c2e5a2deb5f201d96cefbca80c0ef2f513",
+ "tarball": "https://registry.npmjs.org/jsbn/-/jsbn-0.1.1.tgz"
+ },
+ "gitHead": "ed7e7ab56bd2b8a4447bc0c1ef08548b6dad89a2",
+ "homepage": "https://github.com/andyperlitch/jsbn#readme",
+ "keywords": [
+ "biginteger",
+ "bignumber",
+ "big",
+ "integer"
+ ],
+ "license": "MIT",
+ "main": "index.js",
+ "maintainers": [
+ {
+ "name": "andyperlitch",
+ "email": "andyperlitch@gmail.com"
+ }
+ ],
+ "name": "jsbn",
+ "optionalDependencies": {},
+ "readme": "ERROR: No README data found!",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/andyperlitch/jsbn.git"
+ },
+ "scripts": {
+ "test": "mocha test.js"
+ },
+ "version": "0.1.1"
+}
diff --git a/node_modules/json-schema-traverse/.eslintrc.yml b/node_modules/json-schema-traverse/.eslintrc.yml
new file mode 100644
index 0000000..ab1762d
--- /dev/null
+++ b/node_modules/json-schema-traverse/.eslintrc.yml
@@ -0,0 +1,27 @@
+extends: eslint:recommended
+env:
+ node: true
+ browser: true
+rules:
+ block-scoped-var: 2
+ complexity: [2, 13]
+ curly: [2, multi-or-nest, consistent]
+ dot-location: [2, property]
+ dot-notation: 2
+ indent: [2, 2, SwitchCase: 1]
+ linebreak-style: [2, unix]
+ new-cap: 2
+ no-console: [2, allow: [warn, error]]
+ no-else-return: 2
+ no-eq-null: 2
+ no-fallthrough: 2
+ no-invalid-this: 2
+ no-return-assign: 2
+ no-shadow: 1
+ no-trailing-spaces: 2
+ no-use-before-define: [2, nofunc]
+ quotes: [2, single, avoid-escape]
+ semi: [2, always]
+ strict: [2, global]
+ valid-jsdoc: [2, requireReturn: false]
+ no-control-regex: 0
diff --git a/node_modules/json-schema-traverse/.travis.yml b/node_modules/json-schema-traverse/.travis.yml
new file mode 100644
index 0000000..7ddce74
--- /dev/null
+++ b/node_modules/json-schema-traverse/.travis.yml
@@ -0,0 +1,8 @@
+language: node_js
+node_js:
+ - "4"
+ - "6"
+ - "7"
+ - "8"
+after_script:
+ - coveralls < coverage/lcov.info
diff --git a/node_modules/json-schema-traverse/LICENSE b/node_modules/json-schema-traverse/LICENSE
new file mode 100644
index 0000000..7f15435
--- /dev/null
+++ b/node_modules/json-schema-traverse/LICENSE
@@ -0,0 +1,21 @@
+MIT License
+
+Copyright (c) 2017 Evgeny Poberezkin
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in all
+copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
+SOFTWARE.
diff --git a/node_modules/json-schema-traverse/README.md b/node_modules/json-schema-traverse/README.md
new file mode 100644
index 0000000..d5ccaf4
--- /dev/null
+++ b/node_modules/json-schema-traverse/README.md
@@ -0,0 +1,83 @@
+# json-schema-traverse
+Traverse JSON Schema passing each schema object to callback
+
+[](https://travis-ci.org/epoberezkin/json-schema-traverse)
+[](https://www.npmjs.com/package/json-schema-traverse)
+[](https://coveralls.io/github/epoberezkin/json-schema-traverse?branch=master)
+
+
+## Install
+
+```
+npm install json-schema-traverse
+```
+
+
+## Usage
+
+```javascript
+const traverse = require('json-schema-traverse');
+const schema = {
+ properties: {
+ foo: {type: 'string'},
+ bar: {type: 'integer'}
+ }
+};
+
+traverse(schema, {cb});
+// cb is called 3 times with:
+// 1. root schema
+// 2. {type: 'string'}
+// 3. {type: 'integer'}
+
+// Or:
+
+traverse(schema, {cb: {pre, post}});
+// pre is called 3 times with:
+// 1. root schema
+// 2. {type: 'string'}
+// 3. {type: 'integer'}
+//
+// post is called 3 times with:
+// 1. {type: 'string'}
+// 2. {type: 'integer'}
+// 3. root schema
+
+```
+
+Callback function `cb` is called for each schema object (not including draft-06 boolean schemas), including the root schema, in pre-order traversal. Schema references ($ref) are not resolved, they are passed as is. Alternatively, you can pass a `{pre, post}` object as `cb`, and then `pre` will be called before traversing child elements, and `post` will be called after all child elements have been traversed.
+
+Callback is passed these parameters:
+
+- _schema_: the current schema object
+- _JSON pointer_: from the root schema to the current schema object
+- _root schema_: the schema passed to `traverse` object
+- _parent JSON pointer_: from the root schema to the parent schema object (see below)
+- _parent keyword_: the keyword inside which this schema appears (e.g. `properties`, `anyOf`, etc.)
+- _parent schema_: not necessarily parent object/array; in the example above the parent schema for `{type: 'string'}` is the root schema
+- _index/property_: index or property name in the array/object containing multiple schemas; in the example above for `{type: 'string'}` the property name is `'foo'`
+
+
+## Traverse objects in all unknown keywords
+
+```javascript
+const traverse = require('json-schema-traverse');
+const schema = {
+ mySchema: {
+ minimum: 1,
+ maximum: 2
+ }
+};
+
+traverse(schema, {allKeys: true, cb});
+// cb is called 2 times with:
+// 1. root schema
+// 2. mySchema
+```
+
+Without option `allKeys: true` callback will be called only with root schema.
+
+
+## License
+
+[MIT](https://github.com/epoberezkin/json-schema-traverse/blob/master/LICENSE)
diff --git a/node_modules/json-schema-traverse/index.js b/node_modules/json-schema-traverse/index.js
new file mode 100644
index 0000000..d4a18df
--- /dev/null
+++ b/node_modules/json-schema-traverse/index.js
@@ -0,0 +1,89 @@
+'use strict';
+
+var traverse = module.exports = function (schema, opts, cb) {
+ // Legacy support for v0.3.1 and earlier.
+ if (typeof opts == 'function') {
+ cb = opts;
+ opts = {};
+ }
+
+ cb = opts.cb || cb;
+ var pre = (typeof cb == 'function') ? cb : cb.pre || function() {};
+ var post = cb.post || function() {};
+
+ _traverse(opts, pre, post, schema, '', schema);
+};
+
+
+traverse.keywords = {
+ additionalItems: true,
+ items: true,
+ contains: true,
+ additionalProperties: true,
+ propertyNames: true,
+ not: true
+};
+
+traverse.arrayKeywords = {
+ items: true,
+ allOf: true,
+ anyOf: true,
+ oneOf: true
+};
+
+traverse.propsKeywords = {
+ definitions: true,
+ properties: true,
+ patternProperties: true,
+ dependencies: true
+};
+
+traverse.skipKeywords = {
+ default: true,
+ enum: true,
+ const: true,
+ required: true,
+ maximum: true,
+ minimum: true,
+ exclusiveMaximum: true,
+ exclusiveMinimum: true,
+ multipleOf: true,
+ maxLength: true,
+ minLength: true,
+ pattern: true,
+ format: true,
+ maxItems: true,
+ minItems: true,
+ uniqueItems: true,
+ maxProperties: true,
+ minProperties: true
+};
+
+
+function _traverse(opts, pre, post, schema, jsonPtr, rootSchema, parentJsonPtr, parentKeyword, parentSchema, keyIndex) {
+ if (schema && typeof schema == 'object' && !Array.isArray(schema)) {
+ pre(schema, jsonPtr, rootSchema, parentJsonPtr, parentKeyword, parentSchema, keyIndex);
+ for (var key in schema) {
+ var sch = schema[key];
+ if (Array.isArray(sch)) {
+ if (key in traverse.arrayKeywords) {
+ for (var i=0; i
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+]>
+
+
+
+
+
+
+
+
+ A JSON Media Type for Describing the Structure and Meaning of JSON Documents
+
+
+ SitePen (USA)
+
+
+ 530 Lytton Avenue
+ Palo Alto, CA 94301
+ USA
+
+ +1 650 968 8787
+ kris@sitepen.com
+
+
+
+
+
+
+
+ Calgary, AB
+ Canada
+
+ gary.court@gmail.com
+
+
+
+
+ Internet Engineering Task Force
+ JSON
+ Schema
+ JavaScript
+ Object
+ Notation
+ Hyper Schema
+ Hypermedia
+
+
+
+ JSON (JavaScript Object Notation) Schema defines the media type "application/schema+json",
+ a JSON based format for defining
+ the structure of JSON data. JSON Schema provides a contract for what JSON
+ data is required for a given application and how to interact with it. JSON
+ Schema is intended to define validation, documentation, hyperlink
+ navigation, and interaction control of JSON data.
+
+
+
+
+
+
+
+ JSON (JavaScript Object Notation) Schema is a JSON media type for defining
+ the structure of JSON data. JSON Schema provides a contract for what JSON
+ data is required for a given application and how to interact with it. JSON
+ Schema is intended to define validation, documentation, hyperlink
+ navigation, and interaction control of JSON data.
+
+
+
+
+
+
+
+ The key words "MUST", "MUST NOT", "REQUIRED", "SHALL", "SHALL NOT", "SHOULD",
+ "SHOULD NOT", "RECOMMENDED", "MAY", and "OPTIONAL" in this document are to be
+ interpreted as described in RFC 2119 .
+
+
+
+
+
+
+
+ JSON Schema defines the media type "application/schema+json" for
+ describing the structure of other
+ JSON documents. JSON Schema is JSON-based and includes facilities
+ for describing the structure of JSON documents in terms of
+ allowable values, descriptions, and interpreting relations with other resources.
+
+
+ JSON Schema format is organized into several separate definitions. The first
+ definition is the core schema specification. This definition is primary
+ concerned with describing a JSON structure and specifying valid elements
+ in the structure. The second definition is the Hyper Schema specification
+ which is intended define elements in a structure that can be interpreted as
+ hyperlinks.
+ Hyper Schema builds on JSON Schema to describe the hyperlink structure of
+ other JSON documents and elements of interaction. This allows user agents to be able to successfully navigate
+ JSON documents based on their schemas.
+
+
+ Cumulatively JSON Schema acts as a meta-document that can be used to define the required type and constraints on
+ property values, as well as define the meaning of the property values
+ for the purpose of describing a resource and determining hyperlinks
+ within the representation.
+
+
+ An example JSON Schema that describes products might look like:
+
+
+
+
+ This schema defines the properties of the instance JSON documents,
+ the required properties (id, name, and price), as well as an optional
+ property (tags). This also defines the link relations of the instance
+ JSON documents.
+
+
+
+
+
+ For this specification, schema will be used to denote a JSON Schema
+ definition, and an instance refers to a JSON value that the schema
+ will be describing and validating.
+
+
+
+
+
+ The JSON Schema media type does not attempt to dictate the structure of JSON
+ representations that contain data, but rather provides a separate format
+ for flexibly communicating how a JSON representation should be
+ interpreted and validated, such that user agents can properly understand
+ acceptable structures and extrapolate hyperlink information
+ with the JSON document. It is acknowledged that JSON documents come
+ in a variety of structures, and JSON is unique in that the structure
+ of stored data structures often prescribes a non-ambiguous definite
+ JSON representation. Attempting to force a specific structure is generally
+ not viable, and therefore JSON Schema allows for a great flexibility
+ in the structure of the JSON data that it describes.
+
+
+ This specification is protocol agnostic.
+ The underlying protocol (such as HTTP) should sufficiently define the
+ semantics of the client-server interface, the retrieval of resource
+ representations linked to by JSON representations, and modification of
+ those resources. The goal of this
+ format is to sufficiently describe JSON structures such that one can
+ utilize existing information available in existing JSON
+ representations from a large variety of services that leverage a representational state transfer
+ architecture using existing protocols.
+
+
+
+
+
+
+ JSON Schema instances are correlated to their schema by the "describedby"
+ relation, where the schema is defined to be the target of the relation.
+ Instance representations may be of the "application/json" media type or
+ any other subtype. Consequently, dictating how an instance
+ representation should specify the relation to the schema is beyond the normative scope
+ of this document (since this document specifically defines the JSON
+ Schema media type, and no other), but it is recommended that instances
+ specify their schema so that user agents can interpret the instance
+ representation and messages may retain the self-descriptive
+ characteristic, avoiding the need for out-of-band information about
+ instance data. Two approaches are recommended for declaring the
+ relation to the schema that describes the meaning of a JSON instance's (or collection
+ of instances) structure. A MIME type parameter named
+ "profile" or a relation of "describedby" (which could be defined by a Link header) may be used:
+
+
+
+
+
+
+
+ or if the content is being transferred by a protocol (such as HTTP) that
+ provides headers, a Link header can be used:
+
+
+
+; rel="describedby"
+]]>
+
+
+
+ Instances MAY specify multiple schemas, to indicate all the schemas that
+ are applicable to the data, and the data SHOULD be valid by all the schemas.
+ The instance data MAY have multiple schemas
+ that it is defined by (the instance data SHOULD be valid for those schemas).
+ Or if the document is a collection of instances, the collection MAY contain
+ instances from different schemas. When collections contain heterogeneous
+ instances, the "pathStart" attribute MAY be specified in the
+ schema to disambiguate which schema should be applied for each item in the
+ collection. However, ultimately, the mechanism for referencing a schema is up to the
+ media type of the instance documents (if they choose to specify that schemas
+ can be referenced).
+
+
+
+
+ JSON Schemas can themselves be described using JSON Schemas.
+ A self-describing JSON Schema for the core JSON Schema can
+ be found at http://json-schema.org/schema for the latest version or
+ http://json-schema.org/draft-03/schema for the draft-03 version. The hyper schema
+ self-description can be found at http://json-schema.org/hyper-schema
+ or http://json-schema.org/draft-03/hyper-schema . All schemas
+ used within a protocol with media type definitions
+ SHOULD include a MIME parameter that refers to the self-descriptive
+ hyper schema or another schema that extends this hyper schema:
+
+
+
+
+
+
+
+
+
+
+
+
+ A JSON Schema is a JSON Object that defines various attributes
+ (including usage and valid values) of a JSON value. JSON
+ Schema has recursive capabilities; there are a number of elements
+ in the structure that allow for nested JSON Schemas.
+
+
+
+ An example JSON Schema definition could look like:
+
+
+
+
+
+
+ A JSON Schema object may have any of the following properties, called schema
+ attributes (all attributes are optional):
+
+
+
+
+ This attribute defines what the primitive type or the schema of the instance MUST be in order to validate.
+ This attribute can take one of two forms:
+
+
+
+ A string indicating a primitive or simple type. The following are acceptable string values:
+
+
+ Value MUST be a string.
+ Value MUST be a number, floating point numbers are allowed.
+ Value MUST be an integer, no floating point numbers are allowed. This is a subset of the number type.
+ Value MUST be a boolean.
+ Value MUST be an object.
+ Value MUST be an array.
+ Value MUST be null. Note this is mainly for purpose of being able use union types to define nullability. If this type is not included in a union, null values are not allowed (the primitives listed above do not allow nulls on their own).
+ Value MAY be of any type including null.
+
+
+ If the property is not defined or is not in this list, then any type of value is acceptable.
+ Other type values MAY be used for custom purposes, but minimal validators of the specification
+ implementation can allow any instance value on unknown type values.
+
+
+
+ An array of two or more simple type definitions. Each item in the array MUST be a simple type definition or a schema.
+ The instance value is valid if it is of the same type as one of the simple type definitions, or valid by one of the schemas, in the array.
+
+
+
+
+
+ For example, a schema that defines if an instance can be a string or a number would be:
+
+
+
+
+
+
+ This attribute is an object with property definitions that define the valid values of instance object property values. When the instance value is an object, the property values of the instance object MUST conform to the property definitions in this object. In this object, each property definition's value MUST be a schema, and the property's name MUST be the name of the instance property that it defines. The instance property value MUST be valid according to the schema from the property definition. Properties are considered unordered, the order of the instance properties MAY be in any order.
+
+
+
+ This attribute is an object that defines the schema for a set of property names of an object instance. The name of each property of this attribute's object is a regular expression pattern in the ECMA 262/Perl 5 format, while the value is a schema. If the pattern matches the name of a property on the instance object, the value of the instance's property MUST be valid against the pattern name's schema value.
+
+
+
+ This attribute defines a schema for all properties that are not explicitly defined in an object type definition. If specified, the value MUST be a schema or a boolean. If false is provided, no additional properties are allowed beyond the properties defined in the schema. The default value is an empty schema which allows any value for additional properties.
+
+
+
+ This attribute defines the allowed items in an instance array, and MUST be a schema or an array of schemas. The default value is an empty schema which allows any value for items in the instance array.
+ When this attribute value is a schema and the instance value is an array, then all the items in the array MUST be valid according to the schema.
+ When this attribute value is an array of schemas and the instance value is an array, each position in the instance array MUST conform to the schema in the corresponding position for this array. This called tuple typing. When tuple typing is used, additional items are allowed, disallowed, or constrained by the "additionalItems" attribute using the same rules as "additionalProperties" for objects.
+
+
+
+ This provides a definition for additional items in an array instance when tuple definitions of the items is provided. This can be false to indicate additional items in the array are not allowed, or it can be a schema that defines the schema of the additional items.
+
+
+
+ This attribute indicates if the instance must have a value, and not be undefined. This is false by default, making the instance optional.
+
+
+
+ This attribute is an object that defines the requirements of a property on an instance object. If an object instance has a property with the same name as a property in this attribute's object, then the instance must be valid against the attribute's property value (hereafter referred to as the "dependency value").
+
+ The dependency value can take one of two forms:
+
+
+
+ If the dependency value is a string, then the instance object MUST have a property with the same name as the dependency value.
+ If the dependency value is an array of strings, then the instance object MUST have a property with the same name as each string in the dependency value's array.
+
+
+ If the dependency value is a schema, then the instance object MUST be valid against the schema.
+
+
+
+
+
+
+ This attribute defines the minimum value of the instance property when the type of the instance value is a number.
+
+
+
+ This attribute defines the maximum value of the instance property when the type of the instance value is a number.
+
+
+
+ This attribute indicates if the value of the instance (if the instance is a number) can not equal the number defined by the "minimum" attribute. This is false by default, meaning the instance value can be greater then or equal to the minimum value.
+
+
+
+ This attribute indicates if the value of the instance (if the instance is a number) can not equal the number defined by the "maximum" attribute. This is false by default, meaning the instance value can be less then or equal to the maximum value.
+
+
+
+ This attribute defines the minimum number of values in an array when the array is the instance value.
+
+
+
+ This attribute defines the maximum number of values in an array when the array is the instance value.
+
+
+
+ This attribute indicates that all items in an array instance MUST be unique (contains no two identical values).
+
+ Two instance are consider equal if they are both of the same type and:
+
+
+ are null; or
+ are booleans/numbers/strings and have the same value; or
+ are arrays, contains the same number of items, and each item in the array is equal to the corresponding item in the other array; or
+ are objects, contains the same property names, and each property in the object is equal to the corresponding property in the other object.
+
+
+
+
+
+ When the instance value is a string, this provides a regular expression that a string instance MUST match in order to be valid. Regular expressions SHOULD follow the regular expression specification from ECMA 262/Perl 5
+
+
+
+ When the instance value is a string, this defines the minimum length of the string.
+
+
+
+ When the instance value is a string, this defines the maximum length of the string.
+
+
+
+ This provides an enumeration of all possible values that are valid for the instance property. This MUST be an array, and each item in the array represents a possible value for the instance value. If this attribute is defined, the instance value MUST be one of the values in the array in order for the schema to be valid. Comparison of enum values uses the same algorithm as defined in "uniqueItems" .
+
+
+
+ This attribute defines the default value of the instance when the instance is undefined.
+
+
+
+ This attribute is a string that provides a short description of the instance property.
+
+
+
+ This attribute is a string that provides a full description of the of purpose the instance property.
+
+
+
+ This property defines the type of data, content type, or microformat to be expected in the instance property values. A format attribute MAY be one of the values listed below, and if so, SHOULD adhere to the semantics describing for the format. A format SHOULD only be used to give meaning to primitive types (string, integer, number, or boolean). Validators MAY (but are not required to) validate that the instance values conform to a format.
+
+
+ The following formats are predefined:
+
+
+ This SHOULD be a date in ISO 8601 format of YYYY-MM-DDThh:mm:ssZ in UTC time. This is the recommended form of date/timestamp.
+ This SHOULD be a date in the format of YYYY-MM-DD. It is recommended that you use the "date-time" format instead of "date" unless you need to transfer only the date part.
+ This SHOULD be a time in the format of hh:mm:ss. It is recommended that you use the "date-time" format instead of "time" unless you need to transfer only the time part.
+ This SHOULD be the difference, measured in milliseconds, between the specified time and midnight, 00:00 of January 1, 1970 UTC. The value SHOULD be a number (integer or float).
+ A regular expression, following the regular expression specification from ECMA 262/Perl 5.
+ This is a CSS color (like "#FF0000" or "red"), based on CSS 2.1 .
+ This is a CSS style definition (like "color: red; background-color:#FFF"), based on CSS 2.1 .
+ This SHOULD be a phone number (format MAY follow E.123).
+ This value SHOULD be a URI.
+ This SHOULD be an email address.
+ This SHOULD be an ip version 4 address.
+ This SHOULD be an ip version 6 address.
+ This SHOULD be a host-name.
+
+
+
+ Additional custom formats MAY be created. These custom formats MAY be expressed as an URI, and this URI MAY reference a schema of that format.
+
+
+
+ This attribute defines what value the number instance must be divisible by with no remainder (the result of the division must be an integer.) The value of this attribute SHOULD NOT be 0.
+
+
+
+ This attribute takes the same values as the "type" attribute, however if the instance matches the type or if this value is an array and the instance matches any type or schema in the array, then this instance is not valid.
+
+
+
+ The value of this property MUST be another schema which will provide a base schema which the current schema will inherit from. The inheritance rules are such that any instance that is valid according to the current schema MUST be valid according to the referenced schema. This MAY also be an array, in which case, the instance MUST be valid for all the schemas in the array. A schema that extends another schema MAY define additional attributes, constrain existing attributes, or add other constraints.
+
+ Conceptually, the behavior of extends can be seen as validating an
+ instance against all constraints in the extending schema as well as
+ the extended schema(s). More optimized implementations that merge
+ schemas are possible, but are not required. Some examples of using "extends":
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ This attribute defines the current URI of this schema (this attribute is
+ effectively a "self" link). This URI MAY be relative or absolute. If
+ the URI is relative it is resolved against the current URI of the parent
+ schema it is contained in. If this schema is not contained in any
+ parent schema, the current URI of the parent schema is held to be the
+ URI under which this schema was addressed. If id is missing, the current URI of a schema is
+ defined to be that of the parent schema. The current URI of the schema
+ is also used to construct relative references such as for $ref.
+
+
+
+
+
+ This attribute defines a URI of a schema that contains the full representation of this schema.
+ When a validator encounters this attribute, it SHOULD replace the current schema with the schema referenced by the value's URI (if known and available) and re-validate the instance.
+ This URI MAY be relative or absolute, and relative URIs SHOULD be resolved against the URI of the current schema.
+
+
+
+
+
+ This attribute defines a URI of a JSON Schema that is the schema of the current schema.
+ When this attribute is defined, a validator SHOULD use the schema referenced by the value's URI (if known and available) when resolving Hyper Schema links .
+
+
+
+ A validator MAY use this attribute's value to determine which version of JSON Schema the current schema is written in, and provide the appropriate validation features and behavior.
+ Therefore, it is RECOMMENDED that all schema authors include this attribute in their schemas to prevent conflicts with future JSON Schema specification changes.
+
+
+
+
+
+
+ The following attributes are specified in addition to those
+ attributes that already provided by the core schema with the specific
+ purpose of informing user agents of relations between resources based
+ on JSON data. Just as with JSON
+ schema attributes, all the attributes in hyper schemas are optional.
+ Therefore, an empty object is a valid (non-informative) schema, and
+ essentially describes plain JSON (no constraints on the structures).
+ Addition of attributes provides additive information for user agents.
+
+
+
+
+ The value of the links property MUST be an array, where each item
+ in the array is a link description object which describes the link
+ relations of the instances.
+
+
+
+
+ A link description object is used to describe link relations. In
+ the context of a schema, it defines the link relations of the
+ instances of the schema, and can be parameterized by the instance
+ values. The link description format can be used on its own in
+ regular (non-schema documents), and use of this format can
+ be declared by referencing the normative link description
+ schema as the the schema for the data structure that uses the
+ links. The URI of the normative link description schema is:
+ http://json-schema.org/links (latest version) or
+ http://json-schema.org/draft-03/links (draft-03 version).
+
+
+
+
+ The value of the "href" link description property
+ indicates the target URI of the related resource. The value
+ of the instance property SHOULD be resolved as a URI-Reference per RFC 3986
+ and MAY be a relative URI. The base URI to be used for relative resolution
+ SHOULD be the URI used to retrieve the instance object (not the schema)
+ when used within a schema. Also, when links are used within a schema, the URI
+ SHOULD be parametrized by the property values of the instance
+ object, if property values exist for the corresponding variables
+ in the template (otherwise they MAY be provided from alternate sources, like user input).
+
+
+
+ Instance property values SHOULD be substituted into the URIs where
+ matching braces ('{', '}') are found surrounding zero or more characters,
+ creating an expanded URI. Instance property value substitutions are resolved
+ by using the text between the braces to denote the property name
+ from the instance to get the value to substitute.
+
+
+ For example, if an href value is defined:
+
+
+
+ Then it would be resolved by replace the value of the "id" property value from the instance object.
+
+
+
+ If the value of the "id" property was "45", the expanded URI would be:
+
+
+
+
+
+ If matching braces are found with the string "@" (no quotes) between the braces, then the
+ actual instance value SHOULD be used to replace the braces, rather than a property value.
+ This should only be used in situations where the instance is a scalar (string,
+ boolean, or number), and not for objects or arrays.
+
+
+
+
+
+ The value of the "rel" property indicates the name of the
+ relation to the target resource. The relation to the target SHOULD be interpreted as specifically from the instance object that the schema (or sub-schema) applies to, not just the top level resource that contains the object within its hierarchy. If a resource JSON representation contains a sub object with a property interpreted as a link, that sub-object holds the relation with the target. A relation to target from the top level resource MUST be indicated with the schema describing the top level JSON representation.
+
+
+
+ Relationship definitions SHOULD NOT be media type dependent, and users are encouraged to utilize existing accepted relation definitions, including those in existing relation registries (see RFC 4287 ). However, we define these relations here for clarity of normative interpretation within the context of JSON hyper schema defined relations:
+
+
+
+ If the relation value is "self", when this property is encountered in
+ the instance object, the object represents a resource and the instance object is
+ treated as a full representation of the target resource identified by
+ the specified URI.
+
+
+
+ This indicates that the target of the link is the full representation for the instance object. The object that contains this link possibly may not be the full representation.
+
+
+
+ This indicates the target of the link is the schema for the instance object. This MAY be used to specifically denote the schemas of objects within a JSON object hierarchy, facilitating polymorphic type data structures.
+
+
+
+ This relation indicates that the target of the link
+ SHOULD be treated as the root or the body of the representation for the
+ purposes of user agent interaction or fragment resolution. All other
+ properties of the instance objects can be regarded as meta-data
+ descriptions for the data.
+
+
+
+
+
+ The following relations are applicable for schemas (the schema as the "from" resource in the relation):
+
+
+ This indicates the target resource that represents collection of instances of a schema.
+ This indicates a target to use for creating new instances of a schema. This link definition SHOULD be a submission link with a non-safe method (like POST).
+
+
+
+
+
+ For example, if a schema is defined:
+
+
+
+
+
+
+ And if a collection of instance resource's JSON representation was retrieved:
+
+
+
+
+
+ This would indicate that for the first item in the collection, its own
+ (self) URI would resolve to "/Resource/thing" and the first item's "up"
+ relation SHOULD be resolved to the resource at "/Resource/parent".
+ The "children" collection would be located at "/Resource/?upId=thing".
+
+
+
+
+ This property value is a schema that defines the expected structure of the JSON representation of the target of the link.
+
+
+
+
+ The following properties also apply to link definition objects, and
+ provide functionality analogous to HTML forms, in providing a
+ means for submitting extra (often user supplied) information to send to a server.
+
+
+
+
+ This attribute defines which method can be used to access the target resource.
+ In an HTTP environment, this would be "GET" or "POST" (other HTTP methods
+ such as "PUT" and "DELETE" have semantics that are clearly implied by
+ accessed resources, and do not need to be defined here).
+ This defaults to "GET".
+
+
+
+
+
+ If present, this property indicates a query media type format that the server
+ supports for querying or posting to the collection of instances at the target
+ resource. The query can be
+ suffixed to the target URI to query the collection with
+ property-based constraints on the resources that SHOULD be returned from
+ the server or used to post data to the resource (depending on the method).
+
+
+ For example, with the following schema:
+
+
+
+ This indicates that the client can query the server for instances that have a specific name.
+
+
+
+ For example:
+
+
+
+
+
+ If no enctype or method is specified, only the single URI specified by
+ the href property is defined. If the method is POST, "application/json" is
+ the default media type.
+
+
+
+
+
+ This attribute contains a schema which defines the acceptable structure of the submitted
+ request (for a GET request, this schema would define the properties for the query string
+ and for a POST request, this would define the body).
+
+
+
+
+
+
+
+
+ This property indicates the fragment resolution protocol to use for
+ resolving fragment identifiers in URIs within the instance
+ representations. This applies to the instance object URIs and all
+ children of the instance object's URIs. The default fragment resolution
+ protocol is "slash-delimited", which is defined below. Other fragment
+ resolution protocols MAY be used, but are not defined in this document.
+
+
+
+ The fragment identifier is based on RFC 2396, Sec 5 , and defines the
+ mechanism for resolving references to entities within a document.
+
+
+
+
+ With the slash-delimited fragment resolution protocol, the fragment
+ identifier is interpreted as a series of property reference tokens that start with and
+ are delimited by the "/" character (\x2F). Each property reference token
+ is a series of unreserved or escaped URI characters. Each property
+ reference token SHOULD be interpreted, starting from the beginning of
+ the fragment identifier, as a path reference in the target JSON
+ structure. The final target value of the fragment can be determined by
+ starting with the root of the JSON structure from the representation of
+ the resource identified by the pre-fragment URI. If the target is a JSON
+ object, then the new target is the value of the property with the name
+ identified by the next property reference token in the fragment. If the
+ target is a JSON array, then the target is determined by finding the
+ item in array the array with the index defined by the next property
+ reference token (which MUST be a number). The target is successively
+ updated for each property reference token, until the entire fragment has
+ been traversed.
+
+
+
+ Property names SHOULD be URI-encoded. In particular, any "/" in a
+ property name MUST be encoded to avoid being interpreted as a property
+ delimiter.
+
+
+
+
+ For example, for the following JSON representation:
+
+
+
+
+
+
+ The following fragment identifiers would be resolved:
+
+
+
+
+
+
+
+
+
+ The dot-delimited fragment resolution protocol is the same as
+ slash-delimited fragment resolution protocol except that the "." character
+ (\x2E) is used as the delimiter between property names (instead of "/") and
+ the path does not need to start with a ".". For example, #.foo and #foo are a valid fragment
+ identifiers for referencing the value of the foo propery.
+
+
+
+
+
+ This attribute indicates that the instance property SHOULD NOT be changed. Attempts by a user agent to modify the value of this property are expected to be rejected by a server.
+
+
+
+ If the instance property value is a string, this attribute defines that the string SHOULD be interpreted as binary data and decoded using the encoding named by this schema property. RFC 2045, Sec 6.1 lists the possible values for this property.
+
+
+
+
+ This attribute is a URI that defines what the instance's URI MUST start with in order to validate.
+ The value of the "pathStart" attribute MUST be resolved as per RFC 3986, Sec 5 ,
+ and is relative to the instance's URI.
+
+
+
+ When multiple schemas have been referenced for an instance, the user agent
+ can determine if this schema is applicable for a particular instance by
+ determining if the URI of the instance begins with the the value of the "pathStart"
+ attribute. If the URI of the instance does not start with this URI,
+ or if another schema specifies a starting URI that is longer and also matches the
+ instance, this schema SHOULD NOT be applied to the instance. Any schema
+ that does not have a pathStart attribute SHOULD be considered applicable
+ to all the instances for which it is referenced.
+
+
+
+
+ This attribute defines the media type of the instance representations that this schema is defining.
+
+
+
+
+
+ This specification is a sub-type of the JSON format, and
+ consequently the security considerations are generally the same as RFC 4627 .
+ However, an additional issue is that when link relation of "self"
+ is used to denote a full representation of an object, the user agent
+ SHOULD NOT consider the representation to be the authoritative representation
+ of the resource denoted by the target URI if the target URI is not
+ equivalent to or a sub-path of the the URI used to request the resource
+ representation which contains the target URI with the "self" link.
+
+
+ For example, if a hyper schema was defined:
+
+
+
+
+
+
+ And a resource was requested from somesite.com:
+
+
+
+
+
+
+ With a response of:
+
+
+
+
+
+
+
+
+ The proposed MIME media type for JSON Schema is "application/schema+json".
+ Type name: application
+ Subtype name: schema+json
+ Required parameters: profile
+
+ The value of the profile parameter SHOULD be a URI (relative or absolute) that
+ refers to the schema used to define the structure of this structure (the
+ meta-schema). Normally the value would be http://json-schema.org/draft-03/hyper-schema,
+ but it is allowable to use other schemas that extend the hyper schema's meta-
+ schema.
+
+ Optional parameters: pretty
+ The value of the pretty parameter MAY be true or false to indicate if additional whitespace has been included to make the JSON representation easier to read.
+
+
+
+ This registry is maintained by IANA per RFC 4287 and this specification adds
+ four values: "full", "create", "instances", "root". New
+ assignments are subject to IESG Approval, as outlined in RFC 5226 .
+ Requests should be made by email to IANA, which will then forward the
+ request to the IESG, requesting approval.
+
+
+
+
+
+
+
+
+ &rfc2045;
+ &rfc2119;
+ &rfc2396;
+ &rfc3339;
+ &rfc3986;
+ &rfc4287;
+
+
+ &rfc2616;
+ &rfc4627;
+ &rfc5226;
+ &iddiscovery;
+ &uritemplate;
+ &linkheader;
+ &html401;
+ &css21;
+
+
+
+
+
+
+
+ Added example and verbiage to "extends" attribute.
+ Defined slash-delimited to use a leading slash.
+ Made "root" a relation instead of an attribute.
+ Removed address values, and MIME media type from format to reduce confusion (mediaType already exists, so it can be used for MIME types).
+ Added more explanation of nullability.
+ Removed "alternate" attribute.
+ Upper cased many normative usages of must, may, and should.
+ Replaced the link submission "properties" attribute to "schema" attribute.
+ Replaced "optional" attribute with "required" attribute.
+ Replaced "maximumCanEqual" attribute with "exclusiveMaximum" attribute.
+ Replaced "minimumCanEqual" attribute with "exclusiveMinimum" attribute.
+ Replaced "requires" attribute with "dependencies" attribute.
+ Moved "contentEncoding" attribute to hyper schema.
+ Added "additionalItems" attribute.
+ Added "id" attribute.
+ Switched self-referencing variable substitution from "-this" to "@" to align with reserved characters in URI template.
+ Added "patternProperties" attribute.
+ Schema URIs are now namespace versioned.
+ Added "$ref" and "$schema" attributes.
+
+
+
+
+
+ Replaced "maxDecimal" attribute with "divisibleBy" attribute.
+ Added slash-delimited fragment resolution protocol and made it the default.
+ Added language about using links outside of schemas by referencing its normative URI.
+ Added "uniqueItems" attribute.
+ Added "targetSchema" attribute to link description object.
+
+
+
+
+
+ Fixed category and updates from template.
+
+
+
+
+
+ Initial draft.
+
+
+
+
+
+
+
+
+
+ Should we give a preference to MIME headers over Link headers (or only use one)?
+ Should "root" be a MIME parameter?
+ Should "format" be renamed to "mediaType" or "contentType" to reflect the usage MIME media types that are allowed?
+ How should dates be handled?
+
+
+
+
+
diff --git a/node_modules/json-schema/draft-zyp-json-schema-04.xml b/node_modules/json-schema/draft-zyp-json-schema-04.xml
new file mode 100644
index 0000000..8ede6bf
--- /dev/null
+++ b/node_modules/json-schema/draft-zyp-json-schema-04.xml
@@ -0,0 +1,1072 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+]>
+
+
+
+
+
+
+
+
+ A JSON Media Type for Describing the Structure and Meaning of JSON Documents
+
+
+ SitePen (USA)
+
+
+ 530 Lytton Avenue
+ Palo Alto, CA 94301
+ USA
+
+ +1 650 968 8787
+ kris@sitepen.com
+
+
+
+
+
+
+
+ Calgary, AB
+ Canada
+
+ gary.court@gmail.com
+
+
+
+
+ Internet Engineering Task Force
+ JSON
+ Schema
+ JavaScript
+ Object
+ Notation
+ Hyper Schema
+ Hypermedia
+
+
+
+ JSON (JavaScript Object Notation) Schema defines the media type "application/schema+json",
+ a JSON based format for defining the structure of JSON data. JSON Schema provides a contract for what JSON
+ data is required for a given application and how to interact with it. JSON
+ Schema is intended to define validation, documentation, hyperlink
+ navigation, and interaction control of JSON data.
+
+
+
+
+
+
+
+ JSON (JavaScript Object Notation) Schema is a JSON media type for defining
+ the structure of JSON data. JSON Schema provides a contract for what JSON
+ data is required for a given application and how to interact with it. JSON
+ Schema is intended to define validation, documentation, hyperlink
+ navigation, and interaction control of JSON data.
+
+
+
+
+
+
+
+ The key words "MUST", "MUST NOT", "REQUIRED", "SHALL", "SHALL NOT", "SHOULD",
+ "SHOULD NOT", "RECOMMENDED", "MAY", and "OPTIONAL" in this document are to be
+ interpreted as described in RFC 2119 .
+
+
+
+ The terms "JSON", "JSON text", "JSON value", "member", "element", "object",
+ "array", "number", "string", "boolean", "true", "false", and "null" in this
+ document are to be interpreted as defined in RFC 4627 .
+
+
+
+ This specification also uses the following defined terms:
+
+
+ A JSON Schema object.
+ Equivalent to "JSON value" as defined in RFC 4627 .
+ Equivalent to "member" as defined in RFC 4627 .
+ Equivalent to "element" as defined in RFC 4627 .
+ A property of a JSON Schema object.
+
+
+
+
+
+
+ JSON Schema defines the media type "application/schema+json" for
+ describing the structure of JSON text. JSON Schemas are also written in JSON and includes facilities
+ for describing the structure of JSON in terms of
+ allowable values, descriptions, and interpreting relations with other resources.
+
+
+ This document is organized into several separate definitions. The first
+ definition is the core schema specification. This definition is primary
+ concerned with describing a JSON structure and specifying valid elements
+ in the structure. The second definition is the Hyper Schema specification
+ which is intended to define elements in a structure that can be interpreted as
+ hyperlinks.
+ Hyper Schema builds on JSON Schema to describe the hyperlink structure of
+ JSON values. This allows user agents to be able to successfully navigate
+ documents containing JSON based on their schemas.
+
+
+ Cumulatively JSON Schema acts as meta-JSON that can be used to define the
+ required type and constraints on JSON values, as well as define the meaning
+ of the JSON values for the purpose of describing a resource and determining
+ hyperlinks within the representation.
+
+
+ An example JSON Schema that describes products might look like:
+
+
+
+
+ This schema defines the properties of the instance,
+ the required properties (id, name, and price), as well as an optional
+ property (tags). This also defines the link relations of the instance.
+
+
+
+
+
+ The JSON Schema media type does not attempt to dictate the structure of JSON
+ values that contain data, but rather provides a separate format
+ for flexibly communicating how a JSON value should be
+ interpreted and validated, such that user agents can properly understand
+ acceptable structures and extrapolate hyperlink information
+ from the JSON. It is acknowledged that JSON values come
+ in a variety of structures, and JSON is unique in that the structure
+ of stored data structures often prescribes a non-ambiguous definite
+ JSON representation. Attempting to force a specific structure is generally
+ not viable, and therefore JSON Schema allows for a great flexibility
+ in the structure of the JSON data that it describes.
+
+
+ This specification is protocol agnostic.
+ The underlying protocol (such as HTTP) should sufficiently define the
+ semantics of the client-server interface, the retrieval of resource
+ representations linked to by JSON representations, and modification of
+ those resources. The goal of this
+ format is to sufficiently describe JSON structures such that one can
+ utilize existing information available in existing JSON
+ representations from a large variety of services that leverage a representational state transfer
+ architecture using existing protocols.
+
+
+
+
+
+
+ JSON values are correlated to their schema by the "describedby"
+ relation, where the schema is the target of the relation.
+ JSON values MUST be of the "application/json" media type or
+ any other subtype. Consequently, dictating how a JSON value should
+ specify the relation to the schema is beyond the normative scope
+ of this document since this document specifically defines the JSON
+ Schema media type, and no other. It is RECOMMNENDED that JSON values
+ specify their schema so that user agents can interpret the instance
+ and retain the self-descriptive characteristics. This avoides the need for out-of-band information about
+ instance data. Two approaches are recommended for declaring the
+ relation to the schema that describes the meaning of a JSON instance's (or collection
+ of instances) structure. A MIME type parameter named
+ "profile" or a relation of "describedby" (which could be specified by a Link header) may be used:
+
+
+
+
+
+
+
+ or if the content is being transferred by a protocol (such as HTTP) that
+ provides headers, a Link header can be used:
+
+
+
+; rel="describedby"
+]]>
+
+
+
+ Instances MAY specify multiple schemas, to indicate all the schemas that
+ are applicable to the data, and the data SHOULD be valid by all the schemas.
+ The instance data MAY have multiple schemas
+ that it is described by (the instance data SHOULD be valid for those schemas).
+ Or if the document is a collection of instances, the collection MAY contain
+ instances from different schemas. The mechanism for referencing a schema is
+ determined by the media type of the instance (if it provides a method for
+ referencing schemas).
+
+
+
+
+ JSON Schemas can themselves be described using JSON Schemas.
+ A self-describing JSON Schema for the core JSON Schema can
+ be found at http://json-schema.org/schema for the latest version or
+ http://json-schema.org/draft-04/schema for the draft-04 version. The hyper schema
+ self-description can be found at http://json-schema.org/hyper-schema
+ or http://json-schema.org/draft-04/hyper-schema . All schemas
+ used within a protocol with a media type specified SHOULD include a MIME parameter that refers to the self-descriptive
+ hyper schema or another schema that extends this hyper schema:
+
+
+
+
+
+
+
+
+
+
+
+
+ A JSON Schema is a JSON object that defines various attributes
+ (including usage and valid values) of a JSON value. JSON
+ Schema has recursive capabilities; there are a number of elements
+ in the structure that allow for nested JSON Schemas.
+
+
+
+ An example JSON Schema could look like:
+
+
+
+
+
+
+ A JSON Schema object MAY have any of the following optional properties:
+
+
+
+
+
+
+
+ This attribute defines what the primitive type or the schema of the instance MUST be in order to validate.
+ This attribute can take one of two forms:
+
+
+
+ A string indicating a primitive or simple type. The string MUST be one of the following values:
+
+
+ Instance MUST be an object.
+ Instance MUST be an array.
+ Instance MUST be a string.
+ Instance MUST be a number, including floating point numbers.
+ Instance MUST be the JSON literal "true" or "false".
+ Instance MUST be the JSON literal "null". Note that without this type, null values are not allowed.
+ Instance MAY be of any type, including null.
+
+
+
+
+ An array of one or more simple or schema types.
+ The instance value is valid if it is of the same type as one of the simple types, or valid by one of the schemas, in the array.
+
+
+
+ If this attribute is not specified, then all value types are accepted.
+
+
+
+ For example, a schema that defines if an instance can be a string or a number would be:
+
+
+
+
+
+
+
+ This attribute is an object with properties that specify the schemas for the properties of the instance object.
+ In this attribute's object, each property value MUST be a schema.
+ When the instance value is an object, the value of the instance's properties MUST be valid according to the schemas with the same property names specified in this attribute.
+ Objects are unordered, so therefore the order of the instance properties or attribute properties MUST NOT determine validation success.
+
+
+
+
+
+ This attribute is an object that defines the schema for a set of property names of an object instance.
+ The name of each property of this attribute's object is a regular expression pattern in the ECMA 262/Perl 5 format, while the value is a schema.
+ If the pattern matches the name of a property on the instance object, the value of the instance's property MUST be valid against the pattern name's schema value.
+
+
+
+
+ This attribute specifies how any instance property that is not explicitly defined by either the "properties" or "patternProperties" attributes (hereafter referred to as "additional properties") is handled. If specified, the value MUST be a schema or a boolean.
+ If a schema is provided, then all additional properties MUST be valid according to the schema.
+ If false is provided, then no additional properties are allowed.
+ The default value is an empty schema, which allows any value for additional properties.
+
+
+
+ This attribute provides the allowed items in an array instance. If specified, this attribute MUST be a schema or an array of schemas.
+ When this attribute value is a schema and the instance value is an array, then all the items in the array MUST be valid according to the schema.
+ When this attribute value is an array of schemas and the instance value is an array, each position in the instance array MUST be valid according to the schema in the corresponding position for this array. This called tuple typing. When tuple typing is used, additional items are allowed, disallowed, or constrained by the "additionalItems" attribute the same way as "additionalProperties" for objects is.
+
+
+
+ This attribute specifies how any item in the array instance that is not explicitly defined by "items" (hereafter referred to as "additional items") is handled. If specified, the value MUST be a schema or a boolean.
+ If a schema is provided:
+
+ If the "items" attribute is unspecified, then all items in the array instance must be valid against this schema.
+ If the "items" attribute is a schema, then this attribute is ignored.
+ If the "items" attribute is an array (during tuple typing), then any additional items MUST be valid against this schema.
+
+
+ If false is provided, then any additional items in the array are not allowed.
+ The default value is an empty schema, which allows any value for additional items.
+
+
+
+ This attribute is an array of strings that defines all the property names that must exist on the object instance.
+
+
+
+ This attribute is an object that specifies the requirements of a property on an object instance. If an object instance has a property with the same name as a property in this attribute's object, then the instance must be valid against the attribute's property value (hereafter referred to as the "dependency value").
+
+ The dependency value can take one of two forms:
+
+
+
+ If the dependency value is a string, then the instance object MUST have a property with the same name as the dependency value.
+ If the dependency value is an array of strings, then the instance object MUST have a property with the same name as each string in the dependency value's array.
+
+
+ If the dependency value is a schema, then the instance object MUST be valid against the schema.
+
+
+
+
+
+
+ This attribute defines the minimum value of the instance property when the type of the instance value is a number.
+
+
+
+ This attribute defines the maximum value of the instance property when the type of the instance value is a number.
+
+
+
+ This attribute indicates if the value of the instance (if the instance is a number) can not equal the number defined by the "minimum" attribute. This is false by default, meaning the instance value can be greater then or equal to the minimum value.
+
+
+
+ This attribute indicates if the value of the instance (if the instance is a number) can not equal the number defined by the "maximum" attribute. This is false by default, meaning the instance value can be less then or equal to the maximum value.
+
+
+
+ This attribute defines the minimum number of values in an array when the array is the instance value.
+
+
+
+ This attribute defines the maximum number of values in an array when the array is the instance value.
+
+
+
+ This attribute defines the minimum number of properties required on an object instance.
+
+
+
+ This attribute defines the maximum number of properties the object instance can have.
+
+
+
+ This attribute indicates that all items in an array instance MUST be unique (contains no two identical values).
+
+ Two instance are consider equal if they are both of the same type and:
+
+
+ are null; or
+ are booleans/numbers/strings and have the same value; or
+ are arrays, contains the same number of items, and each item in the array is equal to the item at the corresponding index in the other array; or
+ are objects, contains the same property names, and each property in the object is equal to the corresponding property in the other object.
+
+
+
+
+
+ When the instance value is a string, this provides a regular expression that a string instance MUST match in order to be valid. Regular expressions SHOULD follow the regular expression specification from ECMA 262/Perl 5
+
+
+
+ When the instance value is a string, this defines the minimum length of the string.
+
+
+
+ When the instance value is a string, this defines the maximum length of the string.
+
+
+
+ This provides an enumeration of all possible values that are valid for the instance property. This MUST be an array, and each item in the array represents a possible value for the instance value. If this attribute is defined, the instance value MUST be one of the values in the array in order for the schema to be valid. Comparison of enum values uses the same algorithm as defined in "uniqueItems" .
+
+
+
+ This attribute defines the default value of the instance when the instance is undefined.
+
+
+
+ This attribute is a string that provides a short description of the instance property.
+
+
+
+ This attribute is a string that provides a full description of the of purpose the instance property.
+
+
+
+ This attribute defines what value the number instance must be divisible by with no remainder (the result of the division must be an integer.) The value of this attribute SHOULD NOT be 0.
+
+
+
+ This attribute takes the same values as the "type" attribute, however if the instance matches the type or if this value is an array and the instance matches any type or schema in the array, then this instance is not valid.
+
+
+
+ The value of this property MUST be another schema which will provide a base schema which the current schema will inherit from. The inheritance rules are such that any instance that is valid according to the current schema MUST be valid according to the referenced schema. This MAY also be an array, in which case, the instance MUST be valid for all the schemas in the array. A schema that extends another schema MAY define additional attributes, constrain existing attributes, or add other constraints.
+
+ Conceptually, the behavior of extends can be seen as validating an
+ instance against all constraints in the extending schema as well as
+ the extended schema(s). More optimized implementations that merge
+ schemas are possible, but are not required. Some examples of using "extends":
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ This attribute defines the current URI of this schema (this attribute is
+ effectively a "self" link). This URI MAY be relative or absolute. If
+ the URI is relative it is resolved against the current URI of the parent
+ schema it is contained in. If this schema is not contained in any
+ parent schema, the current URI of the parent schema is held to be the
+ URI under which this schema was addressed. If id is missing, the current URI of a schema is
+ defined to be that of the parent schema. The current URI of the schema
+ is also used to construct relative references such as for $ref.
+
+
+
+
+
+ This attribute defines a URI of a schema that contains the full representation of this schema.
+ When a validator encounters this attribute, it SHOULD replace the current schema with the schema referenced by the value's URI (if known and available) and re-validate the instance.
+ This URI MAY be relative or absolute, and relative URIs SHOULD be resolved against the URI of the current schema.
+
+
+
+
+
+ This attribute defines a URI of a JSON Schema that is the schema of the current schema.
+ When this attribute is defined, a validator SHOULD use the schema referenced by the value's URI (if known and available) when resolving Hyper Schema links .
+
+
+
+ A validator MAY use this attribute's value to determine which version of JSON Schema the current schema is written in, and provide the appropriate validation features and behavior.
+ Therefore, it is RECOMMENDED that all schema authors include this attribute in their schemas to prevent conflicts with future JSON Schema specification changes.
+
+
+
+
+
+
+ The following attributes are specified in addition to those
+ attributes that already provided by the core schema with the specific
+ purpose of informing user agents of relations between resources based
+ on JSON data. Just as with JSON
+ schema attributes, all the attributes in hyper schemas are optional.
+ Therefore, an empty object is a valid (non-informative) schema, and
+ essentially describes plain JSON (no constraints on the structures).
+ Addition of attributes provides additive information for user agents.
+
+
+
+
+ The value of the links property MUST be an array, where each item
+ in the array is a link description object which describes the link
+ relations of the instances.
+
+
+
+
+
+
+ A link description object is used to describe link relations. In
+ the context of a schema, it defines the link relations of the
+ instances of the schema, and can be parameterized by the instance
+ values. The link description format can be used without JSON Schema,
+ and use of this format can
+ be declared by referencing the normative link description
+ schema as the the schema for the data structure that uses the
+ links. The URI of the normative link description schema is:
+ http://json-schema.org/links (latest version) or
+ http://json-schema.org/draft-04/links (draft-04 version).
+
+
+
+
+ The value of the "href" link description property
+ indicates the target URI of the related resource. The value
+ of the instance property SHOULD be resolved as a URI-Reference per RFC 3986
+ and MAY be a relative URI. The base URI to be used for relative resolution
+ SHOULD be the URI used to retrieve the instance object (not the schema)
+ when used within a schema. Also, when links are used within a schema, the URI
+ SHOULD be parametrized by the property values of the instance
+ object, if property values exist for the corresponding variables
+ in the template (otherwise they MAY be provided from alternate sources, like user input).
+
+
+
+ Instance property values SHOULD be substituted into the URIs where
+ matching braces ('{', '}') are found surrounding zero or more characters,
+ creating an expanded URI. Instance property value substitutions are resolved
+ by using the text between the braces to denote the property name
+ from the instance to get the value to substitute.
+
+
+ For example, if an href value is defined:
+
+
+
+ Then it would be resolved by replace the value of the "id" property value from the instance object.
+
+
+
+ If the value of the "id" property was "45", the expanded URI would be:
+
+
+
+
+
+ If matching braces are found with the string "@" (no quotes) between the braces, then the
+ actual instance value SHOULD be used to replace the braces, rather than a property value.
+ This should only be used in situations where the instance is a scalar (string,
+ boolean, or number), and not for objects or arrays.
+
+
+
+
+
+ The value of the "rel" property indicates the name of the
+ relation to the target resource. The relation to the target SHOULD be interpreted as specifically from the instance object that the schema (or sub-schema) applies to, not just the top level resource that contains the object within its hierarchy. If a resource JSON representation contains a sub object with a property interpreted as a link, that sub-object holds the relation with the target. A relation to target from the top level resource MUST be indicated with the schema describing the top level JSON representation.
+
+
+
+ Relationship definitions SHOULD NOT be media type dependent, and users are encouraged to utilize existing accepted relation definitions, including those in existing relation registries (see RFC 4287 ). However, we define these relations here for clarity of normative interpretation within the context of JSON hyper schema defined relations:
+
+
+
+ If the relation value is "self", when this property is encountered in
+ the instance object, the object represents a resource and the instance object is
+ treated as a full representation of the target resource identified by
+ the specified URI.
+
+
+
+ This indicates that the target of the link is the full representation for the instance object. The object that contains this link possibly may not be the full representation.
+
+
+
+ This indicates the target of the link is the schema for the instance object. This MAY be used to specifically denote the schemas of objects within a JSON object hierarchy, facilitating polymorphic type data structures.
+
+
+
+ This relation indicates that the target of the link
+ SHOULD be treated as the root or the body of the representation for the
+ purposes of user agent interaction or fragment resolution. All other
+ properties of the instance objects can be regarded as meta-data
+ descriptions for the data.
+
+
+
+
+
+ The following relations are applicable for schemas (the schema as the "from" resource in the relation):
+
+
+ This indicates the target resource that represents collection of instances of a schema.
+ This indicates a target to use for creating new instances of a schema. This link definition SHOULD be a submission link with a non-safe method (like POST).
+
+
+
+
+
+ For example, if a schema is defined:
+
+
+
+
+
+
+ And if a collection of instance resource's JSON representation was retrieved:
+
+
+
+
+
+ This would indicate that for the first item in the collection, its own
+ (self) URI would resolve to "/Resource/thing" and the first item's "up"
+ relation SHOULD be resolved to the resource at "/Resource/parent".
+ The "children" collection would be located at "/Resource/?upId=thing".
+
+
+
+
+ This property value is a string that defines the templating language used in the "href" attribute. If no templating language is defined, then the default Link Description Object templating langauge is used.
+
+
+
+ This property value is a schema that defines the expected structure of the JSON representation of the target of the link.
+
+
+
+
+ The following properties also apply to link definition objects, and
+ provide functionality analogous to HTML forms, in providing a
+ means for submitting extra (often user supplied) information to send to a server.
+
+
+
+
+ This attribute defines which method can be used to access the target resource.
+ In an HTTP environment, this would be "GET" or "POST" (other HTTP methods
+ such as "PUT" and "DELETE" have semantics that are clearly implied by
+ accessed resources, and do not need to be defined here).
+ This defaults to "GET".
+
+
+
+
+
+ If present, this property indicates a query media type format that the server
+ supports for querying or posting to the collection of instances at the target
+ resource. The query can be
+ suffixed to the target URI to query the collection with
+ property-based constraints on the resources that SHOULD be returned from
+ the server or used to post data to the resource (depending on the method).
+
+
+ For example, with the following schema:
+
+
+
+ This indicates that the client can query the server for instances that have a specific name.
+
+
+
+ For example:
+
+
+
+
+
+ If no enctype or method is specified, only the single URI specified by
+ the href property is defined. If the method is POST, "application/json" is
+ the default media type.
+
+
+
+
+
+ This attribute contains a schema which defines the acceptable structure of the submitted
+ request (for a GET request, this schema would define the properties for the query string
+ and for a POST request, this would define the body).
+
+
+
+
+
+
+
+
+ This property indicates the fragment resolution protocol to use for
+ resolving fragment identifiers in URIs within the instance
+ representations. This applies to the instance object URIs and all
+ children of the instance object's URIs. The default fragment resolution
+ protocol is "json-pointer", which is defined below. Other fragment
+ resolution protocols MAY be used, but are not defined in this document.
+
+
+
+ The fragment identifier is based on RFC 3986, Sec 5 , and defines the
+ mechanism for resolving references to entities within a document.
+
+
+
+ The "json-pointer" fragment resolution protocol uses a JSON Pointer to resolve fragment identifiers in URIs within instance representations.
+
+
+
+
+
+
+ This attribute indicates that the instance value SHOULD NOT be changed. Attempts by a user agent to modify the value of this property are expected to be rejected by a server.
+
+
+
+ If the instance property value is a string, this attribute defines that the string SHOULD be interpreted as binary data and decoded using the encoding named by this schema property. RFC 2045, Sec 6.1 lists the possible values for this property.
+
+
+
+
+ This attribute is a URI that defines what the instance's URI MUST start with in order to validate.
+ The value of the "pathStart" attribute MUST be resolved as per RFC 3986, Sec 5 ,
+ and is relative to the instance's URI.
+
+
+
+ When multiple schemas have been referenced for an instance, the user agent
+ can determine if this schema is applicable for a particular instance by
+ determining if the URI of the instance begins with the the value of the "pathStart"
+ attribute. If the URI of the instance does not start with this URI,
+ or if another schema specifies a starting URI that is longer and also matches the
+ instance, this schema SHOULD NOT be applied to the instance. Any schema
+ that does not have a pathStart attribute SHOULD be considered applicable
+ to all the instances for which it is referenced.
+
+
+
+
+ This attribute defines the media type of the instance representations that this schema is defining.
+
+
+
+
+
+ This specification is a sub-type of the JSON format, and
+ consequently the security considerations are generally the same as RFC 4627 .
+ However, an additional issue is that when link relation of "self"
+ is used to denote a full representation of an object, the user agent
+ SHOULD NOT consider the representation to be the authoritative representation
+ of the resource denoted by the target URI if the target URI is not
+ equivalent to or a sub-path of the the URI used to request the resource
+ representation which contains the target URI with the "self" link.
+
+
+ For example, if a hyper schema was defined:
+
+
+
+
+
+
+ And a resource was requested from somesite.com:
+
+
+
+
+
+
+ With a response of:
+
+
+
+
+
+
+
+
+ The proposed MIME media type for JSON Schema is "application/schema+json".
+ Type name: application
+ Subtype name: schema+json
+ Required parameters: profile
+
+ The value of the profile parameter SHOULD be a URI (relative or absolute) that
+ refers to the schema used to define the structure of this structure (the
+ meta-schema). Normally the value would be http://json-schema.org/draft-04/hyper-schema,
+ but it is allowable to use other schemas that extend the hyper schema's meta-
+ schema.
+
+ Optional parameters: pretty
+ The value of the pretty parameter MAY be true or false to indicate if additional whitespace has been included to make the JSON representation easier to read.
+
+
+
+ This registry is maintained by IANA per RFC 4287 and this specification adds
+ four values: "full", "create", "instances", "root". New
+ assignments are subject to IESG Approval, as outlined in RFC 5226 .
+ Requests should be made by email to IANA, which will then forward the
+ request to the IESG, requesting approval.
+
+
+
+
+
+
+
+
+ &rfc2045;
+ &rfc2119;
+ &rfc3339;
+ &rfc3986;
+ &rfc4287;
+
+
+ JSON Pointer
+
+ ForgeRock US, Inc.
+
+
+ SitePen (USA)
+
+
+
+
+
+
+ &rfc2616;
+ &rfc4627;
+ &rfc5226;
+ &iddiscovery;
+ &uritemplate;
+ &linkheader;
+ &html401;
+ &css21;
+
+
+
+
+
+
+
+ Changed "required" attribute to an array of strings.
+ Removed "format" attribute.
+ Added "minProperties" and "maxProperties" attributes.
+ Replaced "slash-delimited" fragment resolution with "json-pointer".
+ Added "template" LDO attribute.
+ Removed irrelevant "Open Issues" section.
+ Merged Conventions and Terminology sections.
+ Defined terms used in specification.
+ Removed "integer" type in favor of {"type":"number", "divisibleBy":1}.
+ Restricted "type" to only the core JSON types.
+ Improved wording of many sections.
+
+
+
+
+
+ Added example and verbiage to "extends" attribute.
+ Defined slash-delimited to use a leading slash.
+ Made "root" a relation instead of an attribute.
+ Removed address values, and MIME media type from format to reduce confusion (mediaType already exists, so it can be used for MIME types).
+ Added more explanation of nullability.
+ Removed "alternate" attribute.
+ Upper cased many normative usages of must, may, and should.
+ Replaced the link submission "properties" attribute to "schema" attribute.
+ Replaced "optional" attribute with "required" attribute.
+ Replaced "maximumCanEqual" attribute with "exclusiveMaximum" attribute.
+ Replaced "minimumCanEqual" attribute with "exclusiveMinimum" attribute.
+ Replaced "requires" attribute with "dependencies" attribute.
+ Moved "contentEncoding" attribute to hyper schema.
+ Added "additionalItems" attribute.
+ Added "id" attribute.
+ Switched self-referencing variable substitution from "-this" to "@" to align with reserved characters in URI template.
+ Added "patternProperties" attribute.
+ Schema URIs are now namespace versioned.
+ Added "$ref" and "$schema" attributes.
+
+
+
+
+
+ Replaced "maxDecimal" attribute with "divisibleBy" attribute.
+ Added slash-delimited fragment resolution protocol and made it the default.
+ Added language about using links outside of schemas by referencing its normative URI.
+ Added "uniqueItems" attribute.
+ Added "targetSchema" attribute to link description object.
+
+
+
+
+
+ Fixed category and updates from template.
+
+
+
+
+
+ Initial draft.
+
+
+
+
+
+
+
diff --git a/node_modules/json-schema/lib/links.js b/node_modules/json-schema/lib/links.js
new file mode 100644
index 0000000..8a87f02
--- /dev/null
+++ b/node_modules/json-schema/lib/links.js
@@ -0,0 +1,66 @@
+/**
+ * JSON Schema link handler
+ * Copyright (c) 2007 Kris Zyp SitePen (www.sitepen.com)
+ * Licensed under the MIT (MIT-LICENSE.txt) license.
+ */
+(function (root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define([], function () {
+ return factory();
+ });
+ } else if (typeof module === 'object' && module.exports) {
+ // Node. Does not work with strict CommonJS, but
+ // only CommonJS-like environments that support module.exports,
+ // like Node.
+ module.exports = factory();
+ } else {
+ // Browser globals
+ root.jsonSchemaLinks = factory();
+ }
+}(this, function () {// setup primitive classes to be JSON Schema types
+var exports = {};
+exports.cacheLinks = true;
+exports.getLink = function(relation, instance, schema){
+ // gets the URI of the link for the given relation based on the instance and schema
+ // for example:
+ // getLink(
+ // "brother",
+ // {"brother_id":33},
+ // {links:[{rel:"brother", href:"Brother/{brother_id}"}]}) ->
+ // "Brother/33"
+ var links = schema.__linkTemplates;
+ if(!links){
+ links = {};
+ var schemaLinks = schema.links;
+ if(schemaLinks && schemaLinks instanceof Array){
+ schemaLinks.forEach(function(link){
+ /* // TODO: allow for multiple same-name relations
+ if(links[link.rel]){
+ if(!(links[link.rel] instanceof Array)){
+ links[link.rel] = [links[link.rel]];
+ }
+ }*/
+ links[link.rel] = link.href;
+ });
+ }
+ if(exports.cacheLinks){
+ schema.__linkTemplates = links;
+ }
+ }
+ var linkTemplate = links[relation];
+ return linkTemplate && exports.substitute(linkTemplate, instance);
+};
+
+exports.substitute = function(linkTemplate, instance){
+ return linkTemplate.replace(/\{([^\}]*)\}/g, function(t, property){
+ var value = instance[decodeURIComponent(property)];
+ if(value instanceof Array){
+ // the value is an array, it should produce a URI like /Table/(4,5,8) and store.get() should handle that as an array of values
+ return '(' + value.join(',') + ')';
+ }
+ return value;
+ });
+};
+return exports;
+}));
\ No newline at end of file
diff --git a/node_modules/json-schema/lib/validate.js b/node_modules/json-schema/lib/validate.js
new file mode 100644
index 0000000..e4dc151
--- /dev/null
+++ b/node_modules/json-schema/lib/validate.js
@@ -0,0 +1,273 @@
+/**
+ * JSONSchema Validator - Validates JavaScript objects using JSON Schemas
+ * (http://www.json.com/json-schema-proposal/)
+ *
+ * Copyright (c) 2007 Kris Zyp SitePen (www.sitepen.com)
+ * Licensed under the MIT (MIT-LICENSE.txt) license.
+To use the validator call the validate function with an instance object and an optional schema object.
+If a schema is provided, it will be used to validate. If the instance object refers to a schema (self-validating),
+that schema will be used to validate and the schema parameter is not necessary (if both exist,
+both validations will occur).
+The validate method will return an array of validation errors. If there are no errors, then an
+empty list will be returned. A validation error will have two properties:
+"property" which indicates which property had the error
+"message" which indicates what the error was
+ */
+(function (root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define([], function () {
+ return factory();
+ });
+ } else if (typeof module === 'object' && module.exports) {
+ // Node. Does not work with strict CommonJS, but
+ // only CommonJS-like environments that support module.exports,
+ // like Node.
+ module.exports = factory();
+ } else {
+ // Browser globals
+ root.jsonSchema = factory();
+ }
+}(this, function () {// setup primitive classes to be JSON Schema types
+var exports = validate
+exports.Integer = {type:"integer"};
+var primitiveConstructors = {
+ String: String,
+ Boolean: Boolean,
+ Number: Number,
+ Object: Object,
+ Array: Array,
+ Date: Date
+}
+exports.validate = validate;
+function validate(/*Any*/instance,/*Object*/schema) {
+ // Summary:
+ // To use the validator call JSONSchema.validate with an instance object and an optional schema object.
+ // If a schema is provided, it will be used to validate. If the instance object refers to a schema (self-validating),
+ // that schema will be used to validate and the schema parameter is not necessary (if both exist,
+ // both validations will occur).
+ // The validate method will return an object with two properties:
+ // valid: A boolean indicating if the instance is valid by the schema
+ // errors: An array of validation errors. If there are no errors, then an
+ // empty list will be returned. A validation error will have two properties:
+ // property: which indicates which property had the error
+ // message: which indicates what the error was
+ //
+ return validate(instance, schema, {changing: false});//, coerce: false, existingOnly: false});
+ };
+exports.checkPropertyChange = function(/*Any*/value,/*Object*/schema, /*String*/property) {
+ // Summary:
+ // The checkPropertyChange method will check to see if an value can legally be in property with the given schema
+ // This is slightly different than the validate method in that it will fail if the schema is readonly and it will
+ // not check for self-validation, it is assumed that the passed in value is already internally valid.
+ // The checkPropertyChange method will return the same object type as validate, see JSONSchema.validate for
+ // information.
+ //
+ return validate(value, schema, {changing: property || "property"});
+ };
+var validate = exports._validate = function(/*Any*/instance,/*Object*/schema,/*Object*/options) {
+
+ if (!options) options = {};
+ var _changing = options.changing;
+
+ function getType(schema){
+ return schema.type || (primitiveConstructors[schema.name] == schema && schema.name.toLowerCase());
+ }
+ var errors = [];
+ // validate a value against a property definition
+ function checkProp(value, schema, path,i){
+
+ var l;
+ path += path ? typeof i == 'number' ? '[' + i + ']' : typeof i == 'undefined' ? '' : '.' + i : i;
+ function addError(message){
+ errors.push({property:path,message:message});
+ }
+
+ if((typeof schema != 'object' || schema instanceof Array) && (path || typeof schema != 'function') && !(schema && getType(schema))){
+ if(typeof schema == 'function'){
+ if(!(value instanceof schema)){
+ addError("is not an instance of the class/constructor " + schema.name);
+ }
+ }else if(schema){
+ addError("Invalid schema/property definition " + schema);
+ }
+ return null;
+ }
+ if(_changing && schema.readonly){
+ addError("is a readonly field, it can not be changed");
+ }
+ if(schema['extends']){ // if it extends another schema, it must pass that schema as well
+ checkProp(value,schema['extends'],path,i);
+ }
+ // validate a value against a type definition
+ function checkType(type,value){
+ if(type){
+ if(typeof type == 'string' && type != 'any' &&
+ (type == 'null' ? value !== null : typeof value != type) &&
+ !(value instanceof Array && type == 'array') &&
+ !(value instanceof Date && type == 'date') &&
+ !(type == 'integer' && value%1===0)){
+ return [{property:path,message:(typeof value) + " value found, but a " + type + " is required"}];
+ }
+ if(type instanceof Array){
+ var unionErrors=[];
+ for(var j = 0; j < type.length; j++){ // a union type
+ if(!(unionErrors=checkType(type[j],value)).length){
+ break;
+ }
+ }
+ if(unionErrors.length){
+ return unionErrors;
+ }
+ }else if(typeof type == 'object'){
+ var priorErrors = errors;
+ errors = [];
+ checkProp(value,type,path);
+ var theseErrors = errors;
+ errors = priorErrors;
+ return theseErrors;
+ }
+ }
+ return [];
+ }
+ if(value === undefined){
+ if(schema.required){
+ addError("is missing and it is required");
+ }
+ }else{
+ errors = errors.concat(checkType(getType(schema),value));
+ if(schema.disallow && !checkType(schema.disallow,value).length){
+ addError(" disallowed value was matched");
+ }
+ if(value !== null){
+ if(value instanceof Array){
+ if(schema.items){
+ var itemsIsArray = schema.items instanceof Array;
+ var propDef = schema.items;
+ for (i = 0, l = value.length; i < l; i += 1) {
+ if (itemsIsArray)
+ propDef = schema.items[i];
+ if (options.coerce)
+ value[i] = options.coerce(value[i], propDef);
+ errors.concat(checkProp(value[i],propDef,path,i));
+ }
+ }
+ if(schema.minItems && value.length < schema.minItems){
+ addError("There must be a minimum of " + schema.minItems + " in the array");
+ }
+ if(schema.maxItems && value.length > schema.maxItems){
+ addError("There must be a maximum of " + schema.maxItems + " in the array");
+ }
+ }else if(schema.properties || schema.additionalProperties){
+ errors.concat(checkObj(value, schema.properties, path, schema.additionalProperties));
+ }
+ if(schema.pattern && typeof value == 'string' && !value.match(schema.pattern)){
+ addError("does not match the regex pattern " + schema.pattern);
+ }
+ if(schema.maxLength && typeof value == 'string' && value.length > schema.maxLength){
+ addError("may only be " + schema.maxLength + " characters long");
+ }
+ if(schema.minLength && typeof value == 'string' && value.length < schema.minLength){
+ addError("must be at least " + schema.minLength + " characters long");
+ }
+ if(typeof schema.minimum !== undefined && typeof value == typeof schema.minimum &&
+ schema.minimum > value){
+ addError("must have a minimum value of " + schema.minimum);
+ }
+ if(typeof schema.maximum !== undefined && typeof value == typeof schema.maximum &&
+ schema.maximum < value){
+ addError("must have a maximum value of " + schema.maximum);
+ }
+ if(schema['enum']){
+ var enumer = schema['enum'];
+ l = enumer.length;
+ var found;
+ for(var j = 0; j < l; j++){
+ if(enumer[j]===value){
+ found=1;
+ break;
+ }
+ }
+ if(!found){
+ addError("does not have a value in the enumeration " + enumer.join(", "));
+ }
+ }
+ if(typeof schema.maxDecimal == 'number' &&
+ (value.toString().match(new RegExp("\\.[0-9]{" + (schema.maxDecimal + 1) + ",}")))){
+ addError("may only have " + schema.maxDecimal + " digits of decimal places");
+ }
+ }
+ }
+ return null;
+ }
+ // validate an object against a schema
+ function checkObj(instance,objTypeDef,path,additionalProp){
+
+ if(typeof objTypeDef =='object'){
+ if(typeof instance != 'object' || instance instanceof Array){
+ errors.push({property:path,message:"an object is required"});
+ }
+
+ for(var i in objTypeDef){
+ if(objTypeDef.hasOwnProperty(i)){
+ var value = instance[i];
+ // skip _not_ specified properties
+ if (value === undefined && options.existingOnly) continue;
+ var propDef = objTypeDef[i];
+ // set default
+ if(value === undefined && propDef["default"]){
+ value = instance[i] = propDef["default"];
+ }
+ if(options.coerce && i in instance){
+ value = instance[i] = options.coerce(value, propDef);
+ }
+ checkProp(value,propDef,path,i);
+ }
+ }
+ }
+ for(i in instance){
+ if(instance.hasOwnProperty(i) && !(i.charAt(0) == '_' && i.charAt(1) == '_') && objTypeDef && !objTypeDef[i] && additionalProp===false){
+ if (options.filter) {
+ delete instance[i];
+ continue;
+ } else {
+ errors.push({property:path,message:(typeof value) + "The property " + i +
+ " is not defined in the schema and the schema does not allow additional properties"});
+ }
+ }
+ var requires = objTypeDef && objTypeDef[i] && objTypeDef[i].requires;
+ if(requires && !(requires in instance)){
+ errors.push({property:path,message:"the presence of the property " + i + " requires that " + requires + " also be present"});
+ }
+ value = instance[i];
+ if(additionalProp && (!(objTypeDef && typeof objTypeDef == 'object') || !(i in objTypeDef))){
+ if(options.coerce){
+ value = instance[i] = options.coerce(value, additionalProp);
+ }
+ checkProp(value,additionalProp,path,i);
+ }
+ if(!_changing && value && value.$schema){
+ errors = errors.concat(checkProp(value,value.$schema,path,i));
+ }
+ }
+ return errors;
+ }
+ if(schema){
+ checkProp(instance,schema,'',_changing || '');
+ }
+ if(!_changing && instance && instance.$schema){
+ checkProp(instance,instance.$schema,'','');
+ }
+ return {valid:!errors.length,errors:errors};
+};
+exports.mustBeValid = function(result){
+ // summary:
+ // This checks to ensure that the result is valid and will throw an appropriate error message if it is not
+ // result: the result returned from checkPropertyChange or validate
+ if(!result.valid){
+ throw new TypeError(result.errors.map(function(error){return "for property " + error.property + ': ' + error.message;}).join(", \n"));
+ }
+}
+
+return exports;
+}));
diff --git a/node_modules/json-schema/package.json b/node_modules/json-schema/package.json
new file mode 100644
index 0000000..cb2c912
--- /dev/null
+++ b/node_modules/json-schema/package.json
@@ -0,0 +1,100 @@
+{
+ "_args": [
+ [
+ {
+ "raw": "json-schema@0.2.3",
+ "scope": null,
+ "escapedName": "json-schema",
+ "name": "json-schema",
+ "rawSpec": "0.2.3",
+ "spec": "0.2.3",
+ "type": "version"
+ },
+ "/Users/ramswaroop/MyProjects/RamAndAntara/node_modules/jsprim"
+ ]
+ ],
+ "_from": "json-schema@0.2.3",
+ "_id": "json-schema@0.2.3",
+ "_inCache": true,
+ "_location": "/json-schema",
+ "_nodeVersion": "6.1.0",
+ "_npmOperationalInternal": {
+ "host": "packages-12-west.internal.npmjs.com",
+ "tmp": "tmp/json-schema-0.2.3.tgz_1473699189380_0.7420965158380568"
+ },
+ "_npmUser": {
+ "name": "kriszyp",
+ "email": "kriszyp@gmail.com"
+ },
+ "_npmVersion": "3.8.9",
+ "_phantomChildren": {},
+ "_requested": {
+ "raw": "json-schema@0.2.3",
+ "scope": null,
+ "escapedName": "json-schema",
+ "name": "json-schema",
+ "rawSpec": "0.2.3",
+ "spec": "0.2.3",
+ "type": "version"
+ },
+ "_requiredBy": [
+ "/jsprim"
+ ],
+ "_resolved": "https://registry.npmjs.org/json-schema/-/json-schema-0.2.3.tgz",
+ "_shasum": "b480c892e59a2f05954ce727bd3f2a4e882f9e13",
+ "_shrinkwrap": null,
+ "_spec": "json-schema@0.2.3",
+ "_where": "/Users/ramswaroop/MyProjects/RamAndAntara/node_modules/jsprim",
+ "author": {
+ "name": "Kris Zyp"
+ },
+ "bugs": {
+ "url": "https://github.com/kriszyp/json-schema/issues"
+ },
+ "dependencies": {},
+ "description": "JSON Schema validation and specifications",
+ "devDependencies": {
+ "vows": "*"
+ },
+ "directories": {
+ "lib": "./lib"
+ },
+ "dist": {
+ "shasum": "b480c892e59a2f05954ce727bd3f2a4e882f9e13",
+ "tarball": "https://registry.npmjs.org/json-schema/-/json-schema-0.2.3.tgz"
+ },
+ "gitHead": "07ae2c618b5f581dbc108e065f4f95dcf0a1d85f",
+ "homepage": "https://github.com/kriszyp/json-schema#readme",
+ "keywords": [
+ "json",
+ "schema"
+ ],
+ "licenses": [
+ {
+ "type": "AFLv2.1",
+ "url": "http://trac.dojotoolkit.org/browser/dojo/trunk/LICENSE#L43"
+ },
+ {
+ "type": "BSD",
+ "url": "http://trac.dojotoolkit.org/browser/dojo/trunk/LICENSE#L13"
+ }
+ ],
+ "main": "./lib/validate.js",
+ "maintainers": [
+ {
+ "name": "kriszyp",
+ "email": "kriszyp@gmail.com"
+ }
+ ],
+ "name": "json-schema",
+ "optionalDependencies": {},
+ "readme": "ERROR: No README data found!",
+ "repository": {
+ "type": "git",
+ "url": "git+ssh://git@github.com/kriszyp/json-schema.git"
+ },
+ "scripts": {
+ "test": "echo TESTS DISABLED vows --spec test/*.js"
+ },
+ "version": "0.2.3"
+}
diff --git a/node_modules/json-schema/test/tests.js b/node_modules/json-schema/test/tests.js
new file mode 100644
index 0000000..2938aea
--- /dev/null
+++ b/node_modules/json-schema/test/tests.js
@@ -0,0 +1,95 @@
+var assert = require('assert');
+var vows = require('vows');
+var path = require('path');
+var fs = require('fs');
+
+var validate = require('../lib/validate').validate;
+
+
+var revision = 'draft-03';
+var schemaRoot = path.join(__dirname, '..', revision);
+var schemaNames = ['schema', 'hyper-schema', 'links', 'json-ref' ];
+var schemas = {};
+
+schemaNames.forEach(function(name) {
+ var file = path.join(schemaRoot, name);
+ schemas[name] = loadSchema(file);
+});
+
+schemaNames.forEach(function(name) {
+ var s, n = name+'-nsd', f = path.join(schemaRoot, name);
+ schemas[n] = loadSchema(f);
+ s = schemas[n];
+ delete s['$schema'];
+});
+
+function loadSchema(path) {
+ var data = fs.readFileSync(path, 'utf-8');
+ var schema = JSON.parse(data);
+ return schema;
+}
+
+function resultIsValid() {
+ return function(result) {
+ assert.isObject(result);
+ //assert.isBoolean(result.valid);
+ assert.equal(typeof(result.valid), 'boolean');
+ assert.isArray(result.errors);
+ for (var i = 0; i < result.errors.length; i++) {
+ assert.notEqual(result.errors[i], null, 'errors['+i+'] is null');
+ }
+ }
+}
+
+function assertValidates(doc, schema) {
+ var context = {};
+
+ context[': validate('+doc+', '+schema+')'] = {
+ topic: validate(schemas[doc], schemas[schema]),
+ 'returns valid result': resultIsValid(),
+ 'with valid=true': function(result) { assert.equal(result.valid, true); },
+ 'and no errors': function(result) {
+ // XXX work-around for bug in vows: [null] chokes it
+ if (result.errors[0] == null) assert.fail('(errors contains null)');
+ assert.length(result.errors, 0);
+ }
+ };
+
+ return context;
+}
+
+function assertSelfValidates(doc) {
+ var context = {};
+
+ context[': validate('+doc+')'] = {
+ topic: validate(schemas[doc]),
+ 'returns valid result': resultIsValid(),
+ 'with valid=true': function(result) { assert.equal(result.valid, true); },
+ 'and no errors': function(result) { assert.length(result.errors, 0); }
+ };
+
+ return context;
+}
+
+var suite = vows.describe('JSON Schema').addBatch({
+ 'Core-NSD self-validates': assertSelfValidates('schema-nsd'),
+ 'Core-NSD/Core-NSD': assertValidates('schema-nsd', 'schema-nsd'),
+ 'Core-NSD/Core': assertValidates('schema-nsd', 'schema'),
+
+ 'Core self-validates': assertSelfValidates('schema'),
+ 'Core/Core': assertValidates('schema', 'schema'),
+
+ 'Hyper-NSD self-validates': assertSelfValidates('hyper-schema-nsd'),
+ 'Hyper self-validates': assertSelfValidates('hyper-schema'),
+ 'Hyper/Hyper': assertValidates('hyper-schema', 'hyper-schema'),
+ 'Hyper/Core': assertValidates('hyper-schema', 'schema'),
+
+ 'Links-NSD self-validates': assertSelfValidates('links-nsd'),
+ 'Links self-validates': assertSelfValidates('links'),
+ 'Links/Hyper': assertValidates('links', 'hyper-schema'),
+ 'Links/Core': assertValidates('links', 'schema'),
+
+ 'Json-Ref self-validates': assertSelfValidates('json-ref'),
+ 'Json-Ref/Hyper': assertValidates('json-ref', 'hyper-schema'),
+ 'Json-Ref/Core': assertValidates('json-ref', 'schema')
+}).export(module);
diff --git a/node_modules/json-stringify-safe/.npmignore b/node_modules/json-stringify-safe/.npmignore
new file mode 100644
index 0000000..17d6b36
--- /dev/null
+++ b/node_modules/json-stringify-safe/.npmignore
@@ -0,0 +1 @@
+/*.tgz
diff --git a/node_modules/json-stringify-safe/CHANGELOG.md b/node_modules/json-stringify-safe/CHANGELOG.md
new file mode 100644
index 0000000..42bcb60
--- /dev/null
+++ b/node_modules/json-stringify-safe/CHANGELOG.md
@@ -0,0 +1,14 @@
+## Unreleased
+- Fixes stringify to only take ancestors into account when checking
+ circularity.
+ It previously assumed every visited object was circular which led to [false
+ positives][issue9].
+ Uses the tiny serializer I wrote for [Must.js][must] a year and a half ago.
+- Fixes calling the `replacer` function in the proper context (`thisArg`).
+- Fixes calling the `cycleReplacer` function in the proper context (`thisArg`).
+- Speeds serializing by a factor of
+ Big-O(h-my-god-it-linearly-searched-every-object) it had ever seen. Searching
+ only the ancestors for a circular references speeds up things considerably.
+
+[must]: https://github.com/moll/js-must
+[issue9]: https://github.com/isaacs/json-stringify-safe/issues/9
diff --git a/node_modules/json-stringify-safe/LICENSE b/node_modules/json-stringify-safe/LICENSE
new file mode 100644
index 0000000..19129e3
--- /dev/null
+++ b/node_modules/json-stringify-safe/LICENSE
@@ -0,0 +1,15 @@
+The ISC License
+
+Copyright (c) Isaac Z. Schlueter and Contributors
+
+Permission to use, copy, modify, and/or distribute this software for any
+purpose with or without fee is hereby granted, provided that the above
+copyright notice and this permission notice appear in all copies.
+
+THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES
+WITH REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF
+MERCHANTABILITY AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR
+ANY SPECIAL, DIRECT, INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES
+WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN
+ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT OF OR
+IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE.
diff --git a/node_modules/json-stringify-safe/Makefile b/node_modules/json-stringify-safe/Makefile
new file mode 100644
index 0000000..36088c7
--- /dev/null
+++ b/node_modules/json-stringify-safe/Makefile
@@ -0,0 +1,35 @@
+NODE_OPTS =
+TEST_OPTS =
+
+love:
+ @echo "Feel like makin' love."
+
+test:
+ @node $(NODE_OPTS) ./node_modules/.bin/_mocha -R dot $(TEST_OPTS)
+
+spec:
+ @node $(NODE_OPTS) ./node_modules/.bin/_mocha -R spec $(TEST_OPTS)
+
+autotest:
+ @node $(NODE_OPTS) ./node_modules/.bin/_mocha -R dot --watch $(TEST_OPTS)
+
+autospec:
+ @node $(NODE_OPTS) ./node_modules/.bin/_mocha -R spec --watch $(TEST_OPTS)
+
+pack:
+ @file=$$(npm pack); echo "$$file"; tar tf "$$file"
+
+publish:
+ npm publish
+
+tag:
+ git tag "v$$(node -e 'console.log(require("./package").version)')"
+
+clean:
+ rm -f *.tgz
+ npm prune --production
+
+.PHONY: love
+.PHONY: test spec autotest autospec
+.PHONY: pack publish tag
+.PHONY: clean
diff --git a/node_modules/json-stringify-safe/README.md b/node_modules/json-stringify-safe/README.md
new file mode 100644
index 0000000..a11f302
--- /dev/null
+++ b/node_modules/json-stringify-safe/README.md
@@ -0,0 +1,52 @@
+# json-stringify-safe
+
+Like JSON.stringify, but doesn't throw on circular references.
+
+## Usage
+
+Takes the same arguments as `JSON.stringify`.
+
+```javascript
+var stringify = require('json-stringify-safe');
+var circularObj = {};
+circularObj.circularRef = circularObj;
+circularObj.list = [ circularObj, circularObj ];
+console.log(stringify(circularObj, null, 2));
+```
+
+Output:
+
+```json
+{
+ "circularRef": "[Circular]",
+ "list": [
+ "[Circular]",
+ "[Circular]"
+ ]
+}
+```
+
+## Details
+
+```
+stringify(obj, serializer, indent, decycler)
+```
+
+The first three arguments are the same as to JSON.stringify. The last
+is an argument that's only used when the object has been seen already.
+
+The default `decycler` function returns the string `'[Circular]'`.
+If, for example, you pass in `function(k,v){}` (return nothing) then it
+will prune cycles. If you pass in `function(k,v){ return {foo: 'bar'}}`,
+then cyclical objects will always be represented as `{"foo":"bar"}` in
+the result.
+
+```
+stringify.getSerialize(serializer, decycler)
+```
+
+Returns a serializer that can be used elsewhere. This is the actual
+function that's passed to JSON.stringify.
+
+**Note** that the function returned from `getSerialize` is stateful for now, so
+do **not** use it more than once.
diff --git a/node_modules/json-stringify-safe/package.json b/node_modules/json-stringify-safe/package.json
new file mode 100644
index 0000000..0561b3c
--- /dev/null
+++ b/node_modules/json-stringify-safe/package.json
@@ -0,0 +1,102 @@
+{
+ "_args": [
+ [
+ {
+ "raw": "json-stringify-safe@~5.0.1",
+ "scope": null,
+ "escapedName": "json-stringify-safe",
+ "name": "json-stringify-safe",
+ "rawSpec": "~5.0.1",
+ "spec": ">=5.0.1 <5.1.0",
+ "type": "range"
+ },
+ "/Users/ramswaroop/MyProjects/RamAndAntara/node_modules/request"
+ ]
+ ],
+ "_from": "json-stringify-safe@>=5.0.1 <5.1.0",
+ "_id": "json-stringify-safe@5.0.1",
+ "_inCache": true,
+ "_location": "/json-stringify-safe",
+ "_nodeVersion": "2.0.1",
+ "_npmUser": {
+ "name": "isaacs",
+ "email": "isaacs@npmjs.com"
+ },
+ "_npmVersion": "2.10.0",
+ "_phantomChildren": {},
+ "_requested": {
+ "raw": "json-stringify-safe@~5.0.1",
+ "scope": null,
+ "escapedName": "json-stringify-safe",
+ "name": "json-stringify-safe",
+ "rawSpec": "~5.0.1",
+ "spec": ">=5.0.1 <5.1.0",
+ "type": "range"
+ },
+ "_requiredBy": [
+ "/request"
+ ],
+ "_resolved": "https://registry.npmjs.org/json-stringify-safe/-/json-stringify-safe-5.0.1.tgz",
+ "_shasum": "1296a2d58fd45f19a0f6ce01d65701e2c735b6eb",
+ "_shrinkwrap": null,
+ "_spec": "json-stringify-safe@~5.0.1",
+ "_where": "/Users/ramswaroop/MyProjects/RamAndAntara/node_modules/request",
+ "author": {
+ "name": "Isaac Z. Schlueter",
+ "email": "i@izs.me",
+ "url": "http://blog.izs.me"
+ },
+ "bugs": {
+ "url": "https://github.com/isaacs/json-stringify-safe/issues"
+ },
+ "contributors": [
+ {
+ "name": "Andri Möll",
+ "email": "andri@dot.ee",
+ "url": "http://themoll.com"
+ }
+ ],
+ "dependencies": {},
+ "description": "Like JSON.stringify, but doesn't blow up on circular refs.",
+ "devDependencies": {
+ "mocha": ">= 2.1.0 < 3",
+ "must": ">= 0.12 < 0.13",
+ "sinon": ">= 1.12.2 < 2"
+ },
+ "directories": {},
+ "dist": {
+ "shasum": "1296a2d58fd45f19a0f6ce01d65701e2c735b6eb",
+ "tarball": "https://registry.npmjs.org/json-stringify-safe/-/json-stringify-safe-5.0.1.tgz"
+ },
+ "gitHead": "3890dceab3ad14f8701e38ca74f38276abc76de5",
+ "homepage": "https://github.com/isaacs/json-stringify-safe",
+ "keywords": [
+ "json",
+ "stringify",
+ "circular",
+ "safe"
+ ],
+ "license": "ISC",
+ "main": "stringify.js",
+ "maintainers": [
+ {
+ "name": "isaacs",
+ "email": "i@izs.me"
+ },
+ {
+ "name": "moll",
+ "email": "andri@dot.ee"
+ }
+ ],
+ "name": "json-stringify-safe",
+ "optionalDependencies": {},
+ "readme": "ERROR: No README data found!",
+ "repository": {
+ "type": "git",
+ "url": "git://github.com/isaacs/json-stringify-safe.git"
+ },
+ "scripts": {
+ "test": "node test.js"
+ },
+ "version": "5.0.1"
+}
diff --git a/node_modules/json-stringify-safe/stringify.js b/node_modules/json-stringify-safe/stringify.js
new file mode 100644
index 0000000..124a452
--- /dev/null
+++ b/node_modules/json-stringify-safe/stringify.js
@@ -0,0 +1,27 @@
+exports = module.exports = stringify
+exports.getSerialize = serializer
+
+function stringify(obj, replacer, spaces, cycleReplacer) {
+ return JSON.stringify(obj, serializer(replacer, cycleReplacer), spaces)
+}
+
+function serializer(replacer, cycleReplacer) {
+ var stack = [], keys = []
+
+ if (cycleReplacer == null) cycleReplacer = function(key, value) {
+ if (stack[0] === value) return "[Circular ~]"
+ return "[Circular ~." + keys.slice(0, stack.indexOf(value)).join(".") + "]"
+ }
+
+ return function(key, value) {
+ if (stack.length > 0) {
+ var thisPos = stack.indexOf(this)
+ ~thisPos ? stack.splice(thisPos + 1) : stack.push(this)
+ ~thisPos ? keys.splice(thisPos, Infinity, key) : keys.push(key)
+ if (~stack.indexOf(value)) value = cycleReplacer.call(this, key, value)
+ }
+ else stack.push(value)
+
+ return replacer == null ? value : replacer.call(this, key, value)
+ }
+}
diff --git a/node_modules/json-stringify-safe/test/mocha.opts b/node_modules/json-stringify-safe/test/mocha.opts
new file mode 100644
index 0000000..2544e58
--- /dev/null
+++ b/node_modules/json-stringify-safe/test/mocha.opts
@@ -0,0 +1,2 @@
+--recursive
+--require must
diff --git a/node_modules/json-stringify-safe/test/stringify_test.js b/node_modules/json-stringify-safe/test/stringify_test.js
new file mode 100644
index 0000000..5b32583
--- /dev/null
+++ b/node_modules/json-stringify-safe/test/stringify_test.js
@@ -0,0 +1,246 @@
+var Sinon = require("sinon")
+var stringify = require("..")
+function jsonify(obj) { return JSON.stringify(obj, null, 2) }
+
+describe("Stringify", function() {
+ it("must stringify circular objects", function() {
+ var obj = {name: "Alice"}
+ obj.self = obj
+ var json = stringify(obj, null, 2)
+ json.must.eql(jsonify({name: "Alice", self: "[Circular ~]"}))
+ })
+
+ it("must stringify circular objects with intermediaries", function() {
+ var obj = {name: "Alice"}
+ obj.identity = {self: obj}
+ var json = stringify(obj, null, 2)
+ json.must.eql(jsonify({name: "Alice", identity: {self: "[Circular ~]"}}))
+ })
+
+ it("must stringify circular objects deeper", function() {
+ var obj = {name: "Alice", child: {name: "Bob"}}
+ obj.child.self = obj.child
+
+ stringify(obj, null, 2).must.eql(jsonify({
+ name: "Alice",
+ child: {name: "Bob", self: "[Circular ~.child]"}
+ }))
+ })
+
+ it("must stringify circular objects deeper with intermediaries", function() {
+ var obj = {name: "Alice", child: {name: "Bob"}}
+ obj.child.identity = {self: obj.child}
+
+ stringify(obj, null, 2).must.eql(jsonify({
+ name: "Alice",
+ child: {name: "Bob", identity: {self: "[Circular ~.child]"}}
+ }))
+ })
+
+ it("must stringify circular objects in an array", function() {
+ var obj = {name: "Alice"}
+ obj.self = [obj, obj]
+
+ stringify(obj, null, 2).must.eql(jsonify({
+ name: "Alice", self: ["[Circular ~]", "[Circular ~]"]
+ }))
+ })
+
+ it("must stringify circular objects deeper in an array", function() {
+ var obj = {name: "Alice", children: [{name: "Bob"}, {name: "Eve"}]}
+ obj.children[0].self = obj.children[0]
+ obj.children[1].self = obj.children[1]
+
+ stringify(obj, null, 2).must.eql(jsonify({
+ name: "Alice",
+ children: [
+ {name: "Bob", self: "[Circular ~.children.0]"},
+ {name: "Eve", self: "[Circular ~.children.1]"}
+ ]
+ }))
+ })
+
+ it("must stringify circular arrays", function() {
+ var obj = []
+ obj.push(obj)
+ obj.push(obj)
+ var json = stringify(obj, null, 2)
+ json.must.eql(jsonify(["[Circular ~]", "[Circular ~]"]))
+ })
+
+ it("must stringify circular arrays with intermediaries", function() {
+ var obj = []
+ obj.push({name: "Alice", self: obj})
+ obj.push({name: "Bob", self: obj})
+
+ stringify(obj, null, 2).must.eql(jsonify([
+ {name: "Alice", self: "[Circular ~]"},
+ {name: "Bob", self: "[Circular ~]"}
+ ]))
+ })
+
+ it("must stringify repeated objects in objects", function() {
+ var obj = {}
+ var alice = {name: "Alice"}
+ obj.alice1 = alice
+ obj.alice2 = alice
+
+ stringify(obj, null, 2).must.eql(jsonify({
+ alice1: {name: "Alice"},
+ alice2: {name: "Alice"}
+ }))
+ })
+
+ it("must stringify repeated objects in arrays", function() {
+ var alice = {name: "Alice"}
+ var obj = [alice, alice]
+ var json = stringify(obj, null, 2)
+ json.must.eql(jsonify([{name: "Alice"}, {name: "Alice"}]))
+ })
+
+ it("must call given decycler and use its output", function() {
+ var obj = {}
+ obj.a = obj
+ obj.b = obj
+
+ var decycle = Sinon.spy(function() { return decycle.callCount })
+ var json = stringify(obj, null, 2, decycle)
+ json.must.eql(jsonify({a: 1, b: 2}, null, 2))
+
+ decycle.callCount.must.equal(2)
+ decycle.thisValues[0].must.equal(obj)
+ decycle.args[0][0].must.equal("a")
+ decycle.args[0][1].must.equal(obj)
+ decycle.thisValues[1].must.equal(obj)
+ decycle.args[1][0].must.equal("b")
+ decycle.args[1][1].must.equal(obj)
+ })
+
+ it("must call replacer and use its output", function() {
+ var obj = {name: "Alice", child: {name: "Bob"}}
+
+ var replacer = Sinon.spy(bangString)
+ var json = stringify(obj, replacer, 2)
+ json.must.eql(jsonify({name: "Alice!", child: {name: "Bob!"}}))
+
+ replacer.callCount.must.equal(4)
+ replacer.args[0][0].must.equal("")
+ replacer.args[0][1].must.equal(obj)
+ replacer.thisValues[1].must.equal(obj)
+ replacer.args[1][0].must.equal("name")
+ replacer.args[1][1].must.equal("Alice")
+ replacer.thisValues[2].must.equal(obj)
+ replacer.args[2][0].must.equal("child")
+ replacer.args[2][1].must.equal(obj.child)
+ replacer.thisValues[3].must.equal(obj.child)
+ replacer.args[3][0].must.equal("name")
+ replacer.args[3][1].must.equal("Bob")
+ })
+
+ it("must call replacer after describing circular references", function() {
+ var obj = {name: "Alice"}
+ obj.self = obj
+
+ var replacer = Sinon.spy(bangString)
+ var json = stringify(obj, replacer, 2)
+ json.must.eql(jsonify({name: "Alice!", self: "[Circular ~]!"}))
+
+ replacer.callCount.must.equal(3)
+ replacer.args[0][0].must.equal("")
+ replacer.args[0][1].must.equal(obj)
+ replacer.thisValues[1].must.equal(obj)
+ replacer.args[1][0].must.equal("name")
+ replacer.args[1][1].must.equal("Alice")
+ replacer.thisValues[2].must.equal(obj)
+ replacer.args[2][0].must.equal("self")
+ replacer.args[2][1].must.equal("[Circular ~]")
+ })
+
+ it("must call given decycler and use its output for nested objects",
+ function() {
+ var obj = {}
+ obj.a = obj
+ obj.b = {self: obj}
+
+ var decycle = Sinon.spy(function() { return decycle.callCount })
+ var json = stringify(obj, null, 2, decycle)
+ json.must.eql(jsonify({a: 1, b: {self: 2}}))
+
+ decycle.callCount.must.equal(2)
+ decycle.args[0][0].must.equal("a")
+ decycle.args[0][1].must.equal(obj)
+ decycle.args[1][0].must.equal("self")
+ decycle.args[1][1].must.equal(obj)
+ })
+
+ it("must use decycler's output when it returned null", function() {
+ var obj = {a: "b"}
+ obj.self = obj
+ obj.selves = [obj, obj]
+
+ function decycle() { return null }
+ stringify(obj, null, 2, decycle).must.eql(jsonify({
+ a: "b",
+ self: null,
+ selves: [null, null]
+ }))
+ })
+
+ it("must use decycler's output when it returned undefined", function() {
+ var obj = {a: "b"}
+ obj.self = obj
+ obj.selves = [obj, obj]
+
+ function decycle() {}
+ stringify(obj, null, 2, decycle).must.eql(jsonify({
+ a: "b",
+ selves: [null, null]
+ }))
+ })
+
+ it("must throw given a decycler that returns a cycle", function() {
+ var obj = {}
+ obj.self = obj
+ var err
+ function identity(key, value) { return value }
+ try { stringify(obj, null, 2, identity) } catch (ex) { err = ex }
+ err.must.be.an.instanceof(TypeError)
+ })
+
+ describe(".getSerialize", function() {
+ it("must stringify circular objects", function() {
+ var obj = {a: "b"}
+ obj.circularRef = obj
+ obj.list = [obj, obj]
+
+ var json = JSON.stringify(obj, stringify.getSerialize(), 2)
+ json.must.eql(jsonify({
+ "a": "b",
+ "circularRef": "[Circular ~]",
+ "list": ["[Circular ~]", "[Circular ~]"]
+ }))
+ })
+
+ // This is the behavior as of Mar 3, 2015.
+ // The serializer function keeps state inside the returned function and
+ // so far I'm not sure how to not do that. JSON.stringify's replacer is not
+ // called _after_ serialization.
+ xit("must return a function that could be called twice", function() {
+ var obj = {name: "Alice"}
+ obj.self = obj
+
+ var json
+ var serializer = stringify.getSerialize()
+
+ json = JSON.stringify(obj, serializer, 2)
+ json.must.eql(jsonify({name: "Alice", self: "[Circular ~]"}))
+
+ json = JSON.stringify(obj, serializer, 2)
+ json.must.eql(jsonify({name: "Alice", self: "[Circular ~]"}))
+ })
+ })
+})
+
+function bangString(key, value) {
+ return typeof value == "string" ? value + "!" : value
+}
diff --git a/node_modules/jsprim/CHANGES.md b/node_modules/jsprim/CHANGES.md
new file mode 100644
index 0000000..c52d39d
--- /dev/null
+++ b/node_modules/jsprim/CHANGES.md
@@ -0,0 +1,49 @@
+# Changelog
+
+## not yet released
+
+None yet.
+
+## v1.4.1 (2017-08-02)
+
+* #21 Update verror dep
+* #22 Update extsprintf dependency
+* #23 update contribution guidelines
+
+## v1.4.0 (2017-03-13)
+
+* #7 Add parseInteger() function for safer number parsing
+
+## v1.3.1 (2016-09-12)
+
+* #13 Incompatible with webpack
+
+## v1.3.0 (2016-06-22)
+
+* #14 add safer version of hasOwnProperty()
+* #15 forEachKey() should ignore inherited properties
+
+## v1.2.2 (2015-10-15)
+
+* #11 NPM package shouldn't include any code that does `require('JSV')`
+* #12 jsl.node.conf missing definition for "module"
+
+## v1.2.1 (2015-10-14)
+
+* #8 odd date parsing behaviour
+
+## v1.2.0 (2015-10-13)
+
+* #9 want function for returning RFC1123 dates
+
+## v1.1.0 (2015-09-02)
+
+* #6 a new suite of hrtime manipulation routines: `hrtimeAdd()`,
+ `hrtimeAccum()`, `hrtimeNanosec()`, `hrtimeMicrosec()` and
+ `hrtimeMillisec()`.
+
+## v1.0.0 (2015-09-01)
+
+First tracked release. Includes everything in previous releases, plus:
+
+* #4 want function for merging objects
diff --git a/node_modules/jsprim/CONTRIBUTING.md b/node_modules/jsprim/CONTRIBUTING.md
new file mode 100644
index 0000000..750cef8
--- /dev/null
+++ b/node_modules/jsprim/CONTRIBUTING.md
@@ -0,0 +1,19 @@
+# Contributing
+
+This repository uses [cr.joyent.us](https://cr.joyent.us) (Gerrit) for new
+changes. Anyone can submit changes. To get started, see the [cr.joyent.us user
+guide](https://github.com/joyent/joyent-gerrit/blob/master/docs/user/README.md).
+This repo does not use GitHub pull requests.
+
+See the [Joyent Engineering
+Guidelines](https://github.com/joyent/eng/blob/master/docs/index.md) for general
+best practices expected in this repository.
+
+Contributions should be "make prepush" clean. The "prepush" target runs the
+"check" target, which requires these separate tools:
+
+* https://github.com/davepacheco/jsstyle
+* https://github.com/davepacheco/javascriptlint
+
+If you're changing something non-trivial or user-facing, you may want to submit
+an issue first.
diff --git a/node_modules/jsprim/LICENSE b/node_modules/jsprim/LICENSE
new file mode 100644
index 0000000..cbc0bb3
--- /dev/null
+++ b/node_modules/jsprim/LICENSE
@@ -0,0 +1,19 @@
+Copyright (c) 2012, Joyent, Inc. All rights reserved.
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in
+all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
+THE SOFTWARE
diff --git a/node_modules/jsprim/README.md b/node_modules/jsprim/README.md
new file mode 100644
index 0000000..b3f28a4
--- /dev/null
+++ b/node_modules/jsprim/README.md
@@ -0,0 +1,287 @@
+# jsprim: utilities for primitive JavaScript types
+
+This module provides miscellaneous facilities for working with strings,
+numbers, dates, and objects and arrays of these basic types.
+
+
+### deepCopy(obj)
+
+Creates a deep copy of a primitive type, object, or array of primitive types.
+
+
+### deepEqual(obj1, obj2)
+
+Returns whether two objects are equal.
+
+
+### isEmpty(obj)
+
+Returns true if the given object has no properties and false otherwise. This
+is O(1) (unlike `Object.keys(obj).length === 0`, which is O(N)).
+
+### hasKey(obj, key)
+
+Returns true if the given object has an enumerable, non-inherited property
+called `key`. [For information on enumerability and ownership of properties, see
+the MDN
+documentation.](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Enumerability_and_ownership_of_properties)
+
+### forEachKey(obj, callback)
+
+Like Array.forEach, but iterates enumerable, owned properties of an object
+rather than elements of an array. Equivalent to:
+
+ for (var key in obj) {
+ if (Object.prototype.hasOwnProperty.call(obj, key)) {
+ callback(key, obj[key]);
+ }
+ }
+
+
+### flattenObject(obj, depth)
+
+Flattens an object up to a given level of nesting, returning an array of arrays
+of length "depth + 1", where the first "depth" elements correspond to flattened
+columns and the last element contains the remaining object . For example:
+
+ flattenObject({
+ 'I': {
+ 'A': {
+ 'i': {
+ 'datum1': [ 1, 2 ],
+ 'datum2': [ 3, 4 ]
+ },
+ 'ii': {
+ 'datum1': [ 3, 4 ]
+ }
+ },
+ 'B': {
+ 'i': {
+ 'datum1': [ 5, 6 ]
+ },
+ 'ii': {
+ 'datum1': [ 7, 8 ],
+ 'datum2': [ 3, 4 ],
+ },
+ 'iii': {
+ }
+ }
+ },
+ 'II': {
+ 'A': {
+ 'i': {
+ 'datum1': [ 1, 2 ],
+ 'datum2': [ 3, 4 ]
+ }
+ }
+ }
+ }, 3)
+
+becomes:
+
+ [
+ [ 'I', 'A', 'i', { 'datum1': [ 1, 2 ], 'datum2': [ 3, 4 ] } ],
+ [ 'I', 'A', 'ii', { 'datum1': [ 3, 4 ] } ],
+ [ 'I', 'B', 'i', { 'datum1': [ 5, 6 ] } ],
+ [ 'I', 'B', 'ii', { 'datum1': [ 7, 8 ], 'datum2': [ 3, 4 ] } ],
+ [ 'I', 'B', 'iii', {} ],
+ [ 'II', 'A', 'i', { 'datum1': [ 1, 2 ], 'datum2': [ 3, 4 ] } ]
+ ]
+
+This function is strict: "depth" must be a non-negative integer and "obj" must
+be a non-null object with at least "depth" levels of nesting under all keys.
+
+
+### flattenIter(obj, depth, func)
+
+This is similar to `flattenObject` except that instead of returning an array,
+this function invokes `func(entry)` for each `entry` in the array that
+`flattenObject` would return. `flattenIter(obj, depth, func)` is logically
+equivalent to `flattenObject(obj, depth).forEach(func)`. Importantly, this
+version never constructs the full array. Its memory usage is O(depth) rather
+than O(n) (where `n` is the number of flattened elements).
+
+There's another difference between `flattenObject` and `flattenIter` that's
+related to the special case where `depth === 0`. In this case, `flattenObject`
+omits the array wrapping `obj` (which is regrettable).
+
+
+### pluck(obj, key)
+
+Fetch nested property "key" from object "obj", traversing objects as needed.
+For example, `pluck(obj, "foo.bar.baz")` is roughly equivalent to
+`obj.foo.bar.baz`, except that:
+
+1. If traversal fails, the resulting value is undefined, and no error is
+ thrown. For example, `pluck({}, "foo.bar")` is just undefined.
+2. If "obj" has property "key" directly (without traversing), the
+ corresponding property is returned. For example,
+ `pluck({ 'foo.bar': 1 }, 'foo.bar')` is 1, not undefined. This is also
+ true recursively, so `pluck({ 'a': { 'foo.bar': 1 } }, 'a.foo.bar')` is
+ also 1, not undefined.
+
+
+### randElt(array)
+
+Returns an element from "array" selected uniformly at random. If "array" is
+empty, throws an Error.
+
+
+### startsWith(str, prefix)
+
+Returns true if the given string starts with the given prefix and false
+otherwise.
+
+
+### endsWith(str, suffix)
+
+Returns true if the given string ends with the given suffix and false
+otherwise.
+
+
+### parseInteger(str, options)
+
+Parses the contents of `str` (a string) as an integer. On success, the integer
+value is returned (as a number). On failure, an error is **returned** describing
+why parsing failed.
+
+By default, leading and trailing whitespace characters are not allowed, nor are
+trailing characters that are not part of the numeric representation. This
+behaviour can be toggled by using the options below. The empty string (`''`) is
+not considered valid input. If the return value cannot be precisely represented
+as a number (i.e., is smaller than `Number.MIN_SAFE_INTEGER` or larger than
+`Number.MAX_SAFE_INTEGER`), an error is returned. Additionally, the string
+`'-0'` will be parsed as the integer `0`, instead of as the IEEE floating point
+value `-0`.
+
+This function accepts both upper and lowercase characters for digits, similar to
+`parseInt()`, `Number()`, and [strtol(3C)](https://illumos.org/man/3C/strtol).
+
+The following may be specified in `options`:
+
+Option | Type | Default | Meaning
+------------------ | ------- | ------- | ---------------------------
+base | number | 10 | numeric base (radix) to use, in the range 2 to 36
+allowSign | boolean | true | whether to interpret any leading `+` (positive) and `-` (negative) characters
+allowImprecise | boolean | false | whether to accept values that may have lost precision (past `MAX_SAFE_INTEGER` or below `MIN_SAFE_INTEGER`)
+allowPrefix | boolean | false | whether to interpret the prefixes `0b` (base 2), `0o` (base 8), `0t` (base 10), or `0x` (base 16)
+allowTrailing | boolean | false | whether to ignore trailing characters
+trimWhitespace | boolean | false | whether to trim any leading or trailing whitespace/line terminators
+leadingZeroIsOctal | boolean | false | whether a leading zero indicates octal
+
+Note that if `base` is unspecified, and `allowPrefix` or `leadingZeroIsOctal`
+are, then the leading characters can change the default base from 10. If `base`
+is explicitly specified and `allowPrefix` is true, then the prefix will only be
+accepted if it matches the specified base. `base` and `leadingZeroIsOctal`
+cannot be used together.
+
+**Context:** It's tricky to parse integers with JavaScript's built-in facilities
+for several reasons:
+
+- `parseInt()` and `Number()` by default allow the base to be specified in the
+ input string by a prefix (e.g., `0x` for hex).
+- `parseInt()` allows trailing nonnumeric characters.
+- `Number(str)` returns 0 when `str` is the empty string (`''`).
+- Both functions return incorrect values when the input string represents a
+ valid integer outside the range of integers that can be represented precisely.
+ Specifically, `parseInt('9007199254740993')` returns 9007199254740992.
+- Both functions always accept `-` and `+` signs before the digit.
+- Some older JavaScript engines always interpret a leading 0 as indicating
+ octal, which can be surprising when parsing input from users who expect a
+ leading zero to be insignificant.
+
+While each of these may be desirable in some contexts, there are also times when
+none of them are wanted. `parseInteger()` grants greater control over what
+input's permissible.
+
+### iso8601(date)
+
+Converts a Date object to an ISO8601 date string of the form
+"YYYY-MM-DDTHH:MM:SS.sssZ". This format is not customizable.
+
+
+### parseDateTime(str)
+
+Parses a date expressed as a string, as either a number of milliseconds since
+the epoch or any string format that Date accepts, giving preference to the
+former where these two sets overlap (e.g., strings containing small numbers).
+
+
+### hrtimeDiff(timeA, timeB)
+
+Given two hrtime readings (as from Node's `process.hrtime()`), where timeA is
+later than timeB, compute the difference and return that as an hrtime. It is
+illegal to invoke this for a pair of times where timeB is newer than timeA.
+
+### hrtimeAdd(timeA, timeB)
+
+Add two hrtime intervals (as from Node's `process.hrtime()`), returning a new
+hrtime interval array. This function does not modify either input argument.
+
+
+### hrtimeAccum(timeA, timeB)
+
+Add two hrtime intervals (as from Node's `process.hrtime()`), storing the
+result in `timeA`. This function overwrites (and returns) the first argument
+passed in.
+
+
+### hrtimeNanosec(timeA), hrtimeMicrosec(timeA), hrtimeMillisec(timeA)
+
+This suite of functions converts a hrtime interval (as from Node's
+`process.hrtime()`) into a scalar number of nanoseconds, microseconds or
+milliseconds. Results are truncated, as with `Math.floor()`.
+
+
+### validateJsonObject(schema, object)
+
+Uses JSON validation (via JSV) to validate the given object against the given
+schema. On success, returns null. On failure, *returns* (does not throw) a
+useful Error object.
+
+
+### extraProperties(object, allowed)
+
+Check an object for unexpected properties. Accepts the object to check, and an
+array of allowed property name strings. If extra properties are detected, an
+array of extra property names is returned. If no properties other than those
+in the allowed list are present on the object, the returned array will be of
+zero length.
+
+### mergeObjects(provided, overrides, defaults)
+
+Merge properties from objects "provided", "overrides", and "defaults". The
+intended use case is for functions that accept named arguments in an "args"
+object, but want to provide some default values and override other values. In
+that case, "provided" is what the caller specified, "overrides" are what the
+function wants to override, and "defaults" contains default values.
+
+The function starts with the values in "defaults", overrides them with the
+values in "provided", and then overrides those with the values in "overrides".
+For convenience, any of these objects may be falsey, in which case they will be
+ignored. The input objects are never modified, but properties in the returned
+object are not deep-copied.
+
+For example:
+
+ mergeObjects(undefined, { 'objectMode': true }, { 'highWaterMark': 0 })
+
+returns:
+
+ { 'objectMode': true, 'highWaterMark': 0 }
+
+For another example:
+
+ mergeObjects(
+ { 'highWaterMark': 16, 'objectMode': 7 }, /* from caller */
+ { 'objectMode': true }, /* overrides */
+ { 'highWaterMark': 0 }); /* default */
+
+returns:
+
+ { 'objectMode': true, 'highWaterMark': 16 }
+
+
+# Contributing
+
+See separate [contribution guidelines](CONTRIBUTING.md).
diff --git a/node_modules/jsprim/lib/jsprim.js b/node_modules/jsprim/lib/jsprim.js
new file mode 100644
index 0000000..f7d0d81
--- /dev/null
+++ b/node_modules/jsprim/lib/jsprim.js
@@ -0,0 +1,735 @@
+/*
+ * lib/jsprim.js: utilities for primitive JavaScript types
+ */
+
+var mod_assert = require('assert-plus');
+var mod_util = require('util');
+
+var mod_extsprintf = require('extsprintf');
+var mod_verror = require('verror');
+var mod_jsonschema = require('json-schema');
+
+/*
+ * Public interface
+ */
+exports.deepCopy = deepCopy;
+exports.deepEqual = deepEqual;
+exports.isEmpty = isEmpty;
+exports.hasKey = hasKey;
+exports.forEachKey = forEachKey;
+exports.pluck = pluck;
+exports.flattenObject = flattenObject;
+exports.flattenIter = flattenIter;
+exports.validateJsonObject = validateJsonObjectJS;
+exports.validateJsonObjectJS = validateJsonObjectJS;
+exports.randElt = randElt;
+exports.extraProperties = extraProperties;
+exports.mergeObjects = mergeObjects;
+
+exports.startsWith = startsWith;
+exports.endsWith = endsWith;
+
+exports.parseInteger = parseInteger;
+
+exports.iso8601 = iso8601;
+exports.rfc1123 = rfc1123;
+exports.parseDateTime = parseDateTime;
+
+exports.hrtimediff = hrtimeDiff;
+exports.hrtimeDiff = hrtimeDiff;
+exports.hrtimeAccum = hrtimeAccum;
+exports.hrtimeAdd = hrtimeAdd;
+exports.hrtimeNanosec = hrtimeNanosec;
+exports.hrtimeMicrosec = hrtimeMicrosec;
+exports.hrtimeMillisec = hrtimeMillisec;
+
+
+/*
+ * Deep copy an acyclic *basic* Javascript object. This only handles basic
+ * scalars (strings, numbers, booleans) and arbitrarily deep arrays and objects
+ * containing these. This does *not* handle instances of other classes.
+ */
+function deepCopy(obj)
+{
+ var ret, key;
+ var marker = '__deepCopy';
+
+ if (obj && obj[marker])
+ throw (new Error('attempted deep copy of cyclic object'));
+
+ if (obj && obj.constructor == Object) {
+ ret = {};
+ obj[marker] = true;
+
+ for (key in obj) {
+ if (key == marker)
+ continue;
+
+ ret[key] = deepCopy(obj[key]);
+ }
+
+ delete (obj[marker]);
+ return (ret);
+ }
+
+ if (obj && obj.constructor == Array) {
+ ret = [];
+ obj[marker] = true;
+
+ for (key = 0; key < obj.length; key++)
+ ret.push(deepCopy(obj[key]));
+
+ delete (obj[marker]);
+ return (ret);
+ }
+
+ /*
+ * It must be a primitive type -- just return it.
+ */
+ return (obj);
+}
+
+function deepEqual(obj1, obj2)
+{
+ if (typeof (obj1) != typeof (obj2))
+ return (false);
+
+ if (obj1 === null || obj2 === null || typeof (obj1) != 'object')
+ return (obj1 === obj2);
+
+ if (obj1.constructor != obj2.constructor)
+ return (false);
+
+ var k;
+ for (k in obj1) {
+ if (!obj2.hasOwnProperty(k))
+ return (false);
+
+ if (!deepEqual(obj1[k], obj2[k]))
+ return (false);
+ }
+
+ for (k in obj2) {
+ if (!obj1.hasOwnProperty(k))
+ return (false);
+ }
+
+ return (true);
+}
+
+function isEmpty(obj)
+{
+ var key;
+ for (key in obj)
+ return (false);
+ return (true);
+}
+
+function hasKey(obj, key)
+{
+ mod_assert.equal(typeof (key), 'string');
+ return (Object.prototype.hasOwnProperty.call(obj, key));
+}
+
+function forEachKey(obj, callback)
+{
+ for (var key in obj) {
+ if (hasKey(obj, key)) {
+ callback(key, obj[key]);
+ }
+ }
+}
+
+function pluck(obj, key)
+{
+ mod_assert.equal(typeof (key), 'string');
+ return (pluckv(obj, key));
+}
+
+function pluckv(obj, key)
+{
+ if (obj === null || typeof (obj) !== 'object')
+ return (undefined);
+
+ if (obj.hasOwnProperty(key))
+ return (obj[key]);
+
+ var i = key.indexOf('.');
+ if (i == -1)
+ return (undefined);
+
+ var key1 = key.substr(0, i);
+ if (!obj.hasOwnProperty(key1))
+ return (undefined);
+
+ return (pluckv(obj[key1], key.substr(i + 1)));
+}
+
+/*
+ * Invoke callback(row) for each entry in the array that would be returned by
+ * flattenObject(data, depth). This is just like flattenObject(data,
+ * depth).forEach(callback), except that the intermediate array is never
+ * created.
+ */
+function flattenIter(data, depth, callback)
+{
+ doFlattenIter(data, depth, [], callback);
+}
+
+function doFlattenIter(data, depth, accum, callback)
+{
+ var each;
+ var key;
+
+ if (depth === 0) {
+ each = accum.slice(0);
+ each.push(data);
+ callback(each);
+ return;
+ }
+
+ mod_assert.ok(data !== null);
+ mod_assert.equal(typeof (data), 'object');
+ mod_assert.equal(typeof (depth), 'number');
+ mod_assert.ok(depth >= 0);
+
+ for (key in data) {
+ each = accum.slice(0);
+ each.push(key);
+ doFlattenIter(data[key], depth - 1, each, callback);
+ }
+}
+
+function flattenObject(data, depth)
+{
+ if (depth === 0)
+ return ([ data ]);
+
+ mod_assert.ok(data !== null);
+ mod_assert.equal(typeof (data), 'object');
+ mod_assert.equal(typeof (depth), 'number');
+ mod_assert.ok(depth >= 0);
+
+ var rv = [];
+ var key;
+
+ for (key in data) {
+ flattenObject(data[key], depth - 1).forEach(function (p) {
+ rv.push([ key ].concat(p));
+ });
+ }
+
+ return (rv);
+}
+
+function startsWith(str, prefix)
+{
+ return (str.substr(0, prefix.length) == prefix);
+}
+
+function endsWith(str, suffix)
+{
+ return (str.substr(
+ str.length - suffix.length, suffix.length) == suffix);
+}
+
+function iso8601(d)
+{
+ if (typeof (d) == 'number')
+ d = new Date(d);
+ mod_assert.ok(d.constructor === Date);
+ return (mod_extsprintf.sprintf('%4d-%02d-%02dT%02d:%02d:%02d.%03dZ',
+ d.getUTCFullYear(), d.getUTCMonth() + 1, d.getUTCDate(),
+ d.getUTCHours(), d.getUTCMinutes(), d.getUTCSeconds(),
+ d.getUTCMilliseconds()));
+}
+
+var RFC1123_MONTHS = [
+ 'Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun',
+ 'Jul', 'Aug', 'Sep', 'Oct', 'Nov', 'Dec'];
+var RFC1123_DAYS = [
+ 'Sun', 'Mon', 'Tue', 'Wed', 'Thu', 'Fri', 'Sat'];
+
+function rfc1123(date) {
+ return (mod_extsprintf.sprintf('%s, %02d %s %04d %02d:%02d:%02d GMT',
+ RFC1123_DAYS[date.getUTCDay()], date.getUTCDate(),
+ RFC1123_MONTHS[date.getUTCMonth()], date.getUTCFullYear(),
+ date.getUTCHours(), date.getUTCMinutes(),
+ date.getUTCSeconds()));
+}
+
+/*
+ * Parses a date expressed as a string, as either a number of milliseconds since
+ * the epoch or any string format that Date accepts, giving preference to the
+ * former where these two sets overlap (e.g., small numbers).
+ */
+function parseDateTime(str)
+{
+ /*
+ * This is irritatingly implicit, but significantly more concise than
+ * alternatives. The "+str" will convert a string containing only a
+ * number directly to a Number, or NaN for other strings. Thus, if the
+ * conversion succeeds, we use it (this is the milliseconds-since-epoch
+ * case). Otherwise, we pass the string directly to the Date
+ * constructor to parse.
+ */
+ var numeric = +str;
+ if (!isNaN(numeric)) {
+ return (new Date(numeric));
+ } else {
+ return (new Date(str));
+ }
+}
+
+
+/*
+ * Number.*_SAFE_INTEGER isn't present before node v0.12, so we hardcode
+ * the ES6 definitions here, while allowing for them to someday be higher.
+ */
+var MAX_SAFE_INTEGER = Number.MAX_SAFE_INTEGER || 9007199254740991;
+var MIN_SAFE_INTEGER = Number.MIN_SAFE_INTEGER || -9007199254740991;
+
+
+/*
+ * Default options for parseInteger().
+ */
+var PI_DEFAULTS = {
+ base: 10,
+ allowSign: true,
+ allowPrefix: false,
+ allowTrailing: false,
+ allowImprecise: false,
+ trimWhitespace: false,
+ leadingZeroIsOctal: false
+};
+
+var CP_0 = 0x30;
+var CP_9 = 0x39;
+
+var CP_A = 0x41;
+var CP_B = 0x42;
+var CP_O = 0x4f;
+var CP_T = 0x54;
+var CP_X = 0x58;
+var CP_Z = 0x5a;
+
+var CP_a = 0x61;
+var CP_b = 0x62;
+var CP_o = 0x6f;
+var CP_t = 0x74;
+var CP_x = 0x78;
+var CP_z = 0x7a;
+
+var PI_CONV_DEC = 0x30;
+var PI_CONV_UC = 0x37;
+var PI_CONV_LC = 0x57;
+
+
+/*
+ * A stricter version of parseInt() that provides options for changing what
+ * is an acceptable string (for example, disallowing trailing characters).
+ */
+function parseInteger(str, uopts)
+{
+ mod_assert.string(str, 'str');
+ mod_assert.optionalObject(uopts, 'options');
+
+ var baseOverride = false;
+ var options = PI_DEFAULTS;
+
+ if (uopts) {
+ baseOverride = hasKey(uopts, 'base');
+ options = mergeObjects(options, uopts);
+ mod_assert.number(options.base, 'options.base');
+ mod_assert.ok(options.base >= 2, 'options.base >= 2');
+ mod_assert.ok(options.base <= 36, 'options.base <= 36');
+ mod_assert.bool(options.allowSign, 'options.allowSign');
+ mod_assert.bool(options.allowPrefix, 'options.allowPrefix');
+ mod_assert.bool(options.allowTrailing,
+ 'options.allowTrailing');
+ mod_assert.bool(options.allowImprecise,
+ 'options.allowImprecise');
+ mod_assert.bool(options.trimWhitespace,
+ 'options.trimWhitespace');
+ mod_assert.bool(options.leadingZeroIsOctal,
+ 'options.leadingZeroIsOctal');
+
+ if (options.leadingZeroIsOctal) {
+ mod_assert.ok(!baseOverride,
+ '"base" and "leadingZeroIsOctal" are ' +
+ 'mutually exclusive');
+ }
+ }
+
+ var c;
+ var pbase = -1;
+ var base = options.base;
+ var start;
+ var mult = 1;
+ var value = 0;
+ var idx = 0;
+ var len = str.length;
+
+ /* Trim any whitespace on the left side. */
+ if (options.trimWhitespace) {
+ while (idx < len && isSpace(str.charCodeAt(idx))) {
+ ++idx;
+ }
+ }
+
+ /* Check the number for a leading sign. */
+ if (options.allowSign) {
+ if (str[idx] === '-') {
+ idx += 1;
+ mult = -1;
+ } else if (str[idx] === '+') {
+ idx += 1;
+ }
+ }
+
+ /* Parse the base-indicating prefix if there is one. */
+ if (str[idx] === '0') {
+ if (options.allowPrefix) {
+ pbase = prefixToBase(str.charCodeAt(idx + 1));
+ if (pbase !== -1 && (!baseOverride || pbase === base)) {
+ base = pbase;
+ idx += 2;
+ }
+ }
+
+ if (pbase === -1 && options.leadingZeroIsOctal) {
+ base = 8;
+ }
+ }
+
+ /* Parse the actual digits. */
+ for (start = idx; idx < len; ++idx) {
+ c = translateDigit(str.charCodeAt(idx));
+ if (c !== -1 && c < base) {
+ value *= base;
+ value += c;
+ } else {
+ break;
+ }
+ }
+
+ /* If we didn't parse any digits, we have an invalid number. */
+ if (start === idx) {
+ return (new Error('invalid number: ' + JSON.stringify(str)));
+ }
+
+ /* Trim any whitespace on the right side. */
+ if (options.trimWhitespace) {
+ while (idx < len && isSpace(str.charCodeAt(idx))) {
+ ++idx;
+ }
+ }
+
+ /* Check for trailing characters. */
+ if (idx < len && !options.allowTrailing) {
+ return (new Error('trailing characters after number: ' +
+ JSON.stringify(str.slice(idx))));
+ }
+
+ /* If our value is 0, we return now, to avoid returning -0. */
+ if (value === 0) {
+ return (0);
+ }
+
+ /* Calculate our final value. */
+ var result = value * mult;
+
+ /*
+ * If the string represents a value that cannot be precisely represented
+ * by JavaScript, then we want to check that:
+ *
+ * - We never increased the value past MAX_SAFE_INTEGER
+ * - We don't make the result negative and below MIN_SAFE_INTEGER
+ *
+ * Because we only ever increment the value during parsing, there's no
+ * chance of moving past MAX_SAFE_INTEGER and then dropping below it
+ * again, losing precision in the process. This means that we only need
+ * to do our checks here, at the end.
+ */
+ if (!options.allowImprecise &&
+ (value > MAX_SAFE_INTEGER || result < MIN_SAFE_INTEGER)) {
+ return (new Error('number is outside of the supported range: ' +
+ JSON.stringify(str.slice(start, idx))));
+ }
+
+ return (result);
+}
+
+
+/*
+ * Interpret a character code as a base-36 digit.
+ */
+function translateDigit(d)
+{
+ if (d >= CP_0 && d <= CP_9) {
+ /* '0' to '9' -> 0 to 9 */
+ return (d - PI_CONV_DEC);
+ } else if (d >= CP_A && d <= CP_Z) {
+ /* 'A' - 'Z' -> 10 to 35 */
+ return (d - PI_CONV_UC);
+ } else if (d >= CP_a && d <= CP_z) {
+ /* 'a' - 'z' -> 10 to 35 */
+ return (d - PI_CONV_LC);
+ } else {
+ /* Invalid character code */
+ return (-1);
+ }
+}
+
+
+/*
+ * Test if a value matches the ECMAScript definition of trimmable whitespace.
+ */
+function isSpace(c)
+{
+ return (c === 0x20) ||
+ (c >= 0x0009 && c <= 0x000d) ||
+ (c === 0x00a0) ||
+ (c === 0x1680) ||
+ (c === 0x180e) ||
+ (c >= 0x2000 && c <= 0x200a) ||
+ (c === 0x2028) ||
+ (c === 0x2029) ||
+ (c === 0x202f) ||
+ (c === 0x205f) ||
+ (c === 0x3000) ||
+ (c === 0xfeff);
+}
+
+
+/*
+ * Determine which base a character indicates (e.g., 'x' indicates hex).
+ */
+function prefixToBase(c)
+{
+ if (c === CP_b || c === CP_B) {
+ /* 0b/0B (binary) */
+ return (2);
+ } else if (c === CP_o || c === CP_O) {
+ /* 0o/0O (octal) */
+ return (8);
+ } else if (c === CP_t || c === CP_T) {
+ /* 0t/0T (decimal) */
+ return (10);
+ } else if (c === CP_x || c === CP_X) {
+ /* 0x/0X (hexadecimal) */
+ return (16);
+ } else {
+ /* Not a meaningful character */
+ return (-1);
+ }
+}
+
+
+function validateJsonObjectJS(schema, input)
+{
+ var report = mod_jsonschema.validate(input, schema);
+
+ if (report.errors.length === 0)
+ return (null);
+
+ /* Currently, we only do anything useful with the first error. */
+ var error = report.errors[0];
+
+ /* The failed property is given by a URI with an irrelevant prefix. */
+ var propname = error['property'];
+ var reason = error['message'].toLowerCase();
+ var i, j;
+
+ /*
+ * There's at least one case where the property error message is
+ * confusing at best. We work around this here.
+ */
+ if ((i = reason.indexOf('the property ')) != -1 &&
+ (j = reason.indexOf(' is not defined in the schema and the ' +
+ 'schema does not allow additional properties')) != -1) {
+ i += 'the property '.length;
+ if (propname === '')
+ propname = reason.substr(i, j - i);
+ else
+ propname = propname + '.' + reason.substr(i, j - i);
+
+ reason = 'unsupported property';
+ }
+
+ var rv = new mod_verror.VError('property "%s": %s', propname, reason);
+ rv.jsv_details = error;
+ return (rv);
+}
+
+function randElt(arr)
+{
+ mod_assert.ok(Array.isArray(arr) && arr.length > 0,
+ 'randElt argument must be a non-empty array');
+
+ return (arr[Math.floor(Math.random() * arr.length)]);
+}
+
+function assertHrtime(a)
+{
+ mod_assert.ok(a[0] >= 0 && a[1] >= 0,
+ 'negative numbers not allowed in hrtimes');
+ mod_assert.ok(a[1] < 1e9, 'nanoseconds column overflow');
+}
+
+/*
+ * Compute the time elapsed between hrtime readings A and B, where A is later
+ * than B. hrtime readings come from Node's process.hrtime(). There is no
+ * defined way to represent negative deltas, so it's illegal to diff B from A
+ * where the time denoted by B is later than the time denoted by A. If this
+ * becomes valuable, we can define a representation and extend the
+ * implementation to support it.
+ */
+function hrtimeDiff(a, b)
+{
+ assertHrtime(a);
+ assertHrtime(b);
+ mod_assert.ok(a[0] > b[0] || (a[0] == b[0] && a[1] >= b[1]),
+ 'negative differences not allowed');
+
+ var rv = [ a[0] - b[0], 0 ];
+
+ if (a[1] >= b[1]) {
+ rv[1] = a[1] - b[1];
+ } else {
+ rv[0]--;
+ rv[1] = 1e9 - (b[1] - a[1]);
+ }
+
+ return (rv);
+}
+
+/*
+ * Convert a hrtime reading from the array format returned by Node's
+ * process.hrtime() into a scalar number of nanoseconds.
+ */
+function hrtimeNanosec(a)
+{
+ assertHrtime(a);
+
+ return (Math.floor(a[0] * 1e9 + a[1]));
+}
+
+/*
+ * Convert a hrtime reading from the array format returned by Node's
+ * process.hrtime() into a scalar number of microseconds.
+ */
+function hrtimeMicrosec(a)
+{
+ assertHrtime(a);
+
+ return (Math.floor(a[0] * 1e6 + a[1] / 1e3));
+}
+
+/*
+ * Convert a hrtime reading from the array format returned by Node's
+ * process.hrtime() into a scalar number of milliseconds.
+ */
+function hrtimeMillisec(a)
+{
+ assertHrtime(a);
+
+ return (Math.floor(a[0] * 1e3 + a[1] / 1e6));
+}
+
+/*
+ * Add two hrtime readings A and B, overwriting A with the result of the
+ * addition. This function is useful for accumulating several hrtime intervals
+ * into a counter. Returns A.
+ */
+function hrtimeAccum(a, b)
+{
+ assertHrtime(a);
+ assertHrtime(b);
+
+ /*
+ * Accumulate the nanosecond component.
+ */
+ a[1] += b[1];
+ if (a[1] >= 1e9) {
+ /*
+ * The nanosecond component overflowed, so carry to the seconds
+ * field.
+ */
+ a[0]++;
+ a[1] -= 1e9;
+ }
+
+ /*
+ * Accumulate the seconds component.
+ */
+ a[0] += b[0];
+
+ return (a);
+}
+
+/*
+ * Add two hrtime readings A and B, returning the result as a new hrtime array.
+ * Does not modify either input argument.
+ */
+function hrtimeAdd(a, b)
+{
+ assertHrtime(a);
+
+ var rv = [ a[0], a[1] ];
+
+ return (hrtimeAccum(rv, b));
+}
+
+
+/*
+ * Check an object for unexpected properties. Accepts the object to check, and
+ * an array of allowed property names (strings). Returns an array of key names
+ * that were found on the object, but did not appear in the list of allowed
+ * properties. If no properties were found, the returned array will be of
+ * zero length.
+ */
+function extraProperties(obj, allowed)
+{
+ mod_assert.ok(typeof (obj) === 'object' && obj !== null,
+ 'obj argument must be a non-null object');
+ mod_assert.ok(Array.isArray(allowed),
+ 'allowed argument must be an array of strings');
+ for (var i = 0; i < allowed.length; i++) {
+ mod_assert.ok(typeof (allowed[i]) === 'string',
+ 'allowed argument must be an array of strings');
+ }
+
+ return (Object.keys(obj).filter(function (key) {
+ return (allowed.indexOf(key) === -1);
+ }));
+}
+
+/*
+ * Given three sets of properties "provided" (may be undefined), "overrides"
+ * (required), and "defaults" (may be undefined), construct an object containing
+ * the union of these sets with "overrides" overriding "provided", and
+ * "provided" overriding "defaults". None of the input objects are modified.
+ */
+function mergeObjects(provided, overrides, defaults)
+{
+ var rv, k;
+
+ rv = {};
+ if (defaults) {
+ for (k in defaults)
+ rv[k] = defaults[k];
+ }
+
+ if (provided) {
+ for (k in provided)
+ rv[k] = provided[k];
+ }
+
+ if (overrides) {
+ for (k in overrides)
+ rv[k] = overrides[k];
+ }
+
+ return (rv);
+}
diff --git a/node_modules/jsprim/package.json b/node_modules/jsprim/package.json
new file mode 100644
index 0000000..75d27da
--- /dev/null
+++ b/node_modules/jsprim/package.json
@@ -0,0 +1,86 @@
+{
+ "_args": [
+ [
+ {
+ "raw": "jsprim@^1.2.2",
+ "scope": null,
+ "escapedName": "jsprim",
+ "name": "jsprim",
+ "rawSpec": "^1.2.2",
+ "spec": ">=1.2.2 <2.0.0",
+ "type": "range"
+ },
+ "/Users/ramswaroop/MyProjects/RamAndAntara/node_modules/http-signature"
+ ]
+ ],
+ "_from": "jsprim@>=1.2.2 <2.0.0",
+ "_id": "jsprim@1.4.1",
+ "_inCache": true,
+ "_location": "/jsprim",
+ "_nodeVersion": "0.10.45",
+ "_npmOperationalInternal": {
+ "host": "s3://npm-registry-packages",
+ "tmp": "tmp/jsprim-1.4.1.tgz_1501691396911_0.08959000837057829"
+ },
+ "_npmUser": {
+ "name": "dap",
+ "email": "dap@cs.brown.edu"
+ },
+ "_npmVersion": "2.15.1",
+ "_phantomChildren": {},
+ "_requested": {
+ "raw": "jsprim@^1.2.2",
+ "scope": null,
+ "escapedName": "jsprim",
+ "name": "jsprim",
+ "rawSpec": "^1.2.2",
+ "spec": ">=1.2.2 <2.0.0",
+ "type": "range"
+ },
+ "_requiredBy": [
+ "/http-signature"
+ ],
+ "_resolved": "https://registry.npmjs.org/jsprim/-/jsprim-1.4.1.tgz",
+ "_shasum": "313e66bc1e5cc06e438bc1b7499c2e5c56acb6a2",
+ "_shrinkwrap": null,
+ "_spec": "jsprim@^1.2.2",
+ "_where": "/Users/ramswaroop/MyProjects/RamAndAntara/node_modules/http-signature",
+ "bugs": {
+ "url": "https://github.com/joyent/node-jsprim/issues"
+ },
+ "dependencies": {
+ "assert-plus": "1.0.0",
+ "extsprintf": "1.3.0",
+ "json-schema": "0.2.3",
+ "verror": "1.10.0"
+ },
+ "description": "utilities for primitive JavaScript types",
+ "devDependencies": {},
+ "directories": {},
+ "dist": {
+ "shasum": "313e66bc1e5cc06e438bc1b7499c2e5c56acb6a2",
+ "tarball": "https://registry.npmjs.org/jsprim/-/jsprim-1.4.1.tgz"
+ },
+ "engines": [
+ "node >=0.6.0"
+ ],
+ "gitHead": "f7d80a9e8e3f79c0b76448ad9ceab252fb309b32",
+ "homepage": "https://github.com/joyent/node-jsprim#readme",
+ "license": "MIT",
+ "main": "./lib/jsprim.js",
+ "maintainers": [
+ {
+ "name": "dap",
+ "email": "dap@cs.brown.edu"
+ }
+ ],
+ "name": "jsprim",
+ "optionalDependencies": {},
+ "readme": "ERROR: No README data found!",
+ "repository": {
+ "type": "git",
+ "url": "git://github.com/joyent/node-jsprim.git"
+ },
+ "scripts": {},
+ "version": "1.4.1"
+}
diff --git a/node_modules/kind-of/CHANGELOG.md b/node_modules/kind-of/CHANGELOG.md
new file mode 100644
index 0000000..fb30b06
--- /dev/null
+++ b/node_modules/kind-of/CHANGELOG.md
@@ -0,0 +1,157 @@
+# Release history
+
+All notable changes to this project will be documented in this file.
+
+The format is based on [Keep a Changelog](http://keepachangelog.com/en/1.0.0/)
+and this project adheres to [Semantic Versioning](http://semver.org/spec/v2.0.0.html).
+
+
+ Guiding Principles
+
+- Changelogs are for humans, not machines.
+- There should be an entry for every single version.
+- The same types of changes should be grouped.
+- Versions and sections should be linkable.
+- The latest version comes first.
+- The release date of each versions is displayed.
+- Mention whether you follow Semantic Versioning.
+
+
+
+
+ Types of changes
+
+Changelog entries are classified using the following labels _(from [keep-a-changelog](http://keepachangelog.com/)_):
+
+- `Added` for new features.
+- `Changed` for changes in existing functionality.
+- `Deprecated` for soon-to-be removed features.
+- `Removed` for now removed features.
+- `Fixed` for any bug fixes.
+- `Security` in case of vulnerabilities.
+
+
+
+## [6.0.0] - 2017-10-13
+
+- refactor code to be more performant
+- refactor benchmarks
+
+## [5.1.0] - 2017-10-13
+
+**Added**
+
+- Merge pull request #15 from aretecode/patch-1
+- adds support and tests for string & array iterators
+
+**Changed**
+
+- updates benchmarks
+
+## [5.0.2] - 2017-08-02
+
+- Merge pull request #14 from struct78/master
+- Added `undefined` check
+
+## [5.0.0] - 2017-06-21
+
+- Merge pull request #12 from aretecode/iterator
+- Set Iterator + Map Iterator
+- streamline `isbuffer`, minor edits
+
+## [4.0.0] - 2017-05-19
+
+- Merge pull request #8 from tunnckoCore/master
+- update deps
+
+## [3.2.2] - 2017-05-16
+
+- fix version
+
+## [3.2.1] - 2017-05-16
+
+- add browserify
+
+## [3.2.0] - 2017-04-25
+
+- Merge pull request #10 from ksheedlo/unrequire-buffer
+- add `promise` support and tests
+- Remove unnecessary `Buffer` check
+
+## [3.1.0] - 2016-12-07
+
+- Merge pull request #7 from laggingreflex/err
+- add support for `error` and tests
+- run update
+
+## [3.0.4] - 2016-07-29
+
+- move tests
+- run update
+
+## [3.0.3] - 2016-05-03
+
+- fix prepublish script
+- remove unused dep
+
+## [3.0.0] - 2015-11-17
+
+- add typed array support
+- Merge pull request #5 from miguelmota/typed-arrays
+- adds new tests
+
+## [2.0.1] - 2015-08-21
+
+- use `is-buffer` module
+
+## [2.0.0] - 2015-05-31
+
+- Create fallback for `Array.isArray` if used as a browser package
+- Merge pull request #2 from dtothefp/patch-1
+- Merge pull request #3 from pdehaan/patch-1
+- Merge branch 'master' of https://github.com/chorks/kind-of into chorks-master
+- optimizations, mostly date and regex
+
+## [1.1.0] - 2015-02-09
+
+- adds `buffer` support
+- adds tests for `buffer`
+
+## [1.0.0] - 2015-01-19
+
+- update benchmarks
+- optimizations based on benchmarks
+
+## [0.1.2] - 2014-10-26
+
+- return `typeof` value if it's not an object. very slight speed improvement
+- use `.slice`
+- adds benchmarks
+
+## [0.1.0] - 2014-9-26
+
+- first commit
+
+[6.0.0]: https://github.com/jonschlinkert/kind-of/compare/5.1.0...6.0.0
+[5.1.0]: https://github.com/jonschlinkert/kind-of/compare/5.0.2...5.1.0
+[5.0.2]: https://github.com/jonschlinkert/kind-of/compare/5.0.1...5.0.2
+[5.0.1]: https://github.com/jonschlinkert/kind-of/compare/5.0.0...5.0.1
+[5.0.0]: https://github.com/jonschlinkert/kind-of/compare/4.0.0...5.0.0
+[4.0.0]: https://github.com/jonschlinkert/kind-of/compare/3.2.2...4.0.0
+[3.2.2]: https://github.com/jonschlinkert/kind-of/compare/3.2.1...3.2.2
+[3.2.1]: https://github.com/jonschlinkert/kind-of/compare/3.2.0...3.2.1
+[3.2.0]: https://github.com/jonschlinkert/kind-of/compare/3.1.0...3.2.0
+[3.1.0]: https://github.com/jonschlinkert/kind-of/compare/3.0.4...3.1.0
+[3.0.4]: https://github.com/jonschlinkert/kind-of/compare/3.0.3...3.0.4
+[3.0.3]: https://github.com/jonschlinkert/kind-of/compare/3.0.0...3.0.3
+[3.0.0]: https://github.com/jonschlinkert/kind-of/compare/2.0.1...3.0.0
+[2.0.1]: https://github.com/jonschlinkert/kind-of/compare/2.0.0...2.0.1
+[2.0.0]: https://github.com/jonschlinkert/kind-of/compare/1.1.0...2.0.0
+[1.1.0]: https://github.com/jonschlinkert/kind-of/compare/1.0.0...1.1.0
+[1.0.0]: https://github.com/jonschlinkert/kind-of/compare/0.1.2...1.0.0
+[0.1.2]: https://github.com/jonschlinkert/kind-of/compare/0.1.0...0.1.2
+[0.1.0]: https://github.com/jonschlinkert/kind-of/commit/2fae09b0b19b1aadb558e9be39f0c3ef6034eb87
+
+[Unreleased]: https://github.com/jonschlinkert/kind-of/compare/0.1.2...HEAD
+[keep-a-changelog]: https://github.com/olivierlacan/keep-a-changelog
+
diff --git a/node_modules/kind-of/LICENSE b/node_modules/kind-of/LICENSE
new file mode 100644
index 0000000..3f2eca1
--- /dev/null
+++ b/node_modules/kind-of/LICENSE
@@ -0,0 +1,21 @@
+The MIT License (MIT)
+
+Copyright (c) 2014-2017, Jon Schlinkert.
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in
+all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
+THE SOFTWARE.
diff --git a/node_modules/kind-of/README.md b/node_modules/kind-of/README.md
new file mode 100644
index 0000000..4b0d4a8
--- /dev/null
+++ b/node_modules/kind-of/README.md
@@ -0,0 +1,365 @@
+# kind-of [](https://www.npmjs.com/package/kind-of) [](https://npmjs.org/package/kind-of) [](https://npmjs.org/package/kind-of) [](https://travis-ci.org/jonschlinkert/kind-of)
+
+> Get the native type of a value.
+
+Please consider following this project's author, [Jon Schlinkert](https://github.com/jonschlinkert), and consider starring the project to show your :heart: and support.
+
+## Install
+
+Install with [npm](https://www.npmjs.com/):
+
+```sh
+$ npm install --save kind-of
+```
+
+Install with [bower](https://bower.io/)
+
+```sh
+$ bower install kind-of --save
+```
+
+## Why use this?
+
+1. [it's fast](#benchmarks) | [optimizations](#optimizations)
+2. [better type checking](#better-type-checking)
+
+## Usage
+
+> es5, es6, and browser ready
+
+```js
+var kindOf = require('kind-of');
+
+kindOf(undefined);
+//=> 'undefined'
+
+kindOf(null);
+//=> 'null'
+
+kindOf(true);
+//=> 'boolean'
+
+kindOf(false);
+//=> 'boolean'
+
+kindOf(new Buffer(''));
+//=> 'buffer'
+
+kindOf(42);
+//=> 'number'
+
+kindOf('str');
+//=> 'string'
+
+kindOf(arguments);
+//=> 'arguments'
+
+kindOf({});
+//=> 'object'
+
+kindOf(Object.create(null));
+//=> 'object'
+
+kindOf(new Test());
+//=> 'object'
+
+kindOf(new Date());
+//=> 'date'
+
+kindOf([1, 2, 3]);
+//=> 'array'
+
+kindOf(/foo/);
+//=> 'regexp'
+
+kindOf(new RegExp('foo'));
+//=> 'regexp'
+
+kindOf(new Error('error'));
+//=> 'error'
+
+kindOf(function () {});
+//=> 'function'
+
+kindOf(function * () {});
+//=> 'generatorfunction'
+
+kindOf(Symbol('str'));
+//=> 'symbol'
+
+kindOf(new Map());
+//=> 'map'
+
+kindOf(new WeakMap());
+//=> 'weakmap'
+
+kindOf(new Set());
+//=> 'set'
+
+kindOf(new WeakSet());
+//=> 'weakset'
+
+kindOf(new Int8Array());
+//=> 'int8array'
+
+kindOf(new Uint8Array());
+//=> 'uint8array'
+
+kindOf(new Uint8ClampedArray());
+//=> 'uint8clampedarray'
+
+kindOf(new Int16Array());
+//=> 'int16array'
+
+kindOf(new Uint16Array());
+//=> 'uint16array'
+
+kindOf(new Int32Array());
+//=> 'int32array'
+
+kindOf(new Uint32Array());
+//=> 'uint32array'
+
+kindOf(new Float32Array());
+//=> 'float32array'
+
+kindOf(new Float64Array());
+//=> 'float64array'
+```
+
+## Benchmarks
+
+Benchmarked against [typeof](http://github.com/CodingFu/typeof) and [type-of](https://github.com/ForbesLindesay/type-of).
+
+```bash
+# arguments (32 bytes)
+ kind-of x 17,024,098 ops/sec ±1.90% (86 runs sampled)
+ lib-type-of x 11,926,235 ops/sec ±1.34% (83 runs sampled)
+ lib-typeof x 9,245,257 ops/sec ±1.22% (87 runs sampled)
+
+ fastest is kind-of (by 161% avg)
+
+# array (22 bytes)
+ kind-of x 17,196,492 ops/sec ±1.07% (88 runs sampled)
+ lib-type-of x 8,838,283 ops/sec ±1.02% (87 runs sampled)
+ lib-typeof x 8,677,848 ops/sec ±0.87% (87 runs sampled)
+
+ fastest is kind-of (by 196% avg)
+
+# boolean (24 bytes)
+ kind-of x 16,841,600 ops/sec ±1.10% (86 runs sampled)
+ lib-type-of x 8,096,787 ops/sec ±0.95% (87 runs sampled)
+ lib-typeof x 8,423,345 ops/sec ±1.15% (86 runs sampled)
+
+ fastest is kind-of (by 204% avg)
+
+# buffer (38 bytes)
+ kind-of x 14,848,060 ops/sec ±1.05% (86 runs sampled)
+ lib-type-of x 3,671,577 ops/sec ±1.49% (87 runs sampled)
+ lib-typeof x 8,360,236 ops/sec ±1.24% (86 runs sampled)
+
+ fastest is kind-of (by 247% avg)
+
+# date (30 bytes)
+ kind-of x 16,067,761 ops/sec ±1.58% (86 runs sampled)
+ lib-type-of x 8,954,436 ops/sec ±1.40% (87 runs sampled)
+ lib-typeof x 8,488,307 ops/sec ±1.51% (84 runs sampled)
+
+ fastest is kind-of (by 184% avg)
+
+# error (36 bytes)
+ kind-of x 9,634,090 ops/sec ±1.12% (89 runs sampled)
+ lib-type-of x 7,735,624 ops/sec ±1.32% (86 runs sampled)
+ lib-typeof x 7,442,160 ops/sec ±1.11% (90 runs sampled)
+
+ fastest is kind-of (by 127% avg)
+
+# function (34 bytes)
+ kind-of x 10,031,494 ops/sec ±1.27% (86 runs sampled)
+ lib-type-of x 9,502,757 ops/sec ±1.17% (89 runs sampled)
+ lib-typeof x 8,278,985 ops/sec ±1.08% (88 runs sampled)
+
+ fastest is kind-of (by 113% avg)
+
+# null (24 bytes)
+ kind-of x 18,159,808 ops/sec ±1.92% (86 runs sampled)
+ lib-type-of x 12,927,635 ops/sec ±1.01% (88 runs sampled)
+ lib-typeof x 7,958,234 ops/sec ±1.21% (89 runs sampled)
+
+ fastest is kind-of (by 174% avg)
+
+# number (22 bytes)
+ kind-of x 17,846,779 ops/sec ±0.91% (85 runs sampled)
+ lib-type-of x 3,316,636 ops/sec ±1.19% (86 runs sampled)
+ lib-typeof x 2,329,477 ops/sec ±2.21% (85 runs sampled)
+
+ fastest is kind-of (by 632% avg)
+
+# object-plain (47 bytes)
+ kind-of x 7,085,155 ops/sec ±1.05% (88 runs sampled)
+ lib-type-of x 8,870,930 ops/sec ±1.06% (83 runs sampled)
+ lib-typeof x 8,716,024 ops/sec ±1.05% (87 runs sampled)
+
+ fastest is lib-type-of (by 112% avg)
+
+# regex (25 bytes)
+ kind-of x 14,196,052 ops/sec ±1.65% (84 runs sampled)
+ lib-type-of x 9,554,164 ops/sec ±1.25% (88 runs sampled)
+ lib-typeof x 8,359,691 ops/sec ±1.07% (87 runs sampled)
+
+ fastest is kind-of (by 158% avg)
+
+# string (33 bytes)
+ kind-of x 16,131,428 ops/sec ±1.41% (85 runs sampled)
+ lib-type-of x 7,273,172 ops/sec ±1.05% (87 runs sampled)
+ lib-typeof x 7,382,635 ops/sec ±1.17% (85 runs sampled)
+
+ fastest is kind-of (by 220% avg)
+
+# symbol (34 bytes)
+ kind-of x 17,011,537 ops/sec ±1.24% (86 runs sampled)
+ lib-type-of x 3,492,454 ops/sec ±1.23% (89 runs sampled)
+ lib-typeof x 7,471,235 ops/sec ±2.48% (87 runs sampled)
+
+ fastest is kind-of (by 310% avg)
+
+# template-strings (36 bytes)
+ kind-of x 15,434,250 ops/sec ±1.46% (83 runs sampled)
+ lib-type-of x 7,157,907 ops/sec ±0.97% (87 runs sampled)
+ lib-typeof x 7,517,986 ops/sec ±0.92% (86 runs sampled)
+
+ fastest is kind-of (by 210% avg)
+
+# undefined (29 bytes)
+ kind-of x 19,167,115 ops/sec ±1.71% (87 runs sampled)
+ lib-type-of x 15,477,740 ops/sec ±1.63% (85 runs sampled)
+ lib-typeof x 19,075,495 ops/sec ±1.17% (83 runs sampled)
+
+ fastest is lib-typeof,kind-of
+
+```
+
+## Optimizations
+
+In 7 out of 8 cases, this library is 2x-10x faster than other top libraries included in the benchmarks. There are a few things that lead to this performance advantage, none of them hard and fast rules, but all of them simple and repeatable in almost any code library:
+
+1. Optimize around the fastest and most common use cases first. Of course, this will change from project-to-project, but I took some time to understand how and why `typeof` checks were being used in my own libraries and other libraries I use a lot.
+2. Optimize around bottlenecks - In other words, the order in which conditionals are implemented is significant, because each check is only as fast as the failing checks that came before it. Here, the biggest bottleneck by far is checking for plain objects (an object that was created by the `Object` constructor). I opted to make this check happen by process of elimination rather than brute force up front (e.g. by using something like `val.constructor.name`), so that every other type check would not be penalized it.
+3. Don't do uneccessary processing - why do `.slice(8, -1).toLowerCase();` just to get the word `regex`? It's much faster to do `if (type === '[object RegExp]') return 'regex'`
+4. There is no reason to make the code in a microlib as terse as possible, just to win points for making it shorter. It's always better to favor performant code over terse code. You will always only be using a single `require()` statement to use the library anyway, regardless of how the code is written.
+
+## Better type checking
+
+kind-of seems to be more consistently "correct" than other type checking libs I've looked at. For example, here are some differing results from other popular libs:
+
+### [typeof](https://github.com/CodingFu/typeof) lib
+
+Incorrectly identifies instances of custom constructors (pretty common):
+
+```js
+var typeOf = require('typeof');
+function Test() {}
+console.log(typeOf(new Test()));
+//=> 'test'
+```
+
+Returns `object` instead of `arguments`:
+
+```js
+function foo() {
+ console.log(typeOf(arguments)) //=> 'object'
+}
+foo();
+```
+
+### [type-of](https://github.com/ForbesLindesay/type-of) lib
+
+Incorrectly returns `object` for generator functions, buffers, `Map`, `Set`, `WeakMap` and `WeakSet`:
+
+```js
+function * foo() {}
+console.log(typeOf(foo));
+//=> 'object'
+console.log(typeOf(new Buffer('')));
+//=> 'object'
+console.log(typeOf(new Map()));
+//=> 'object'
+console.log(typeOf(new Set()));
+//=> 'object'
+console.log(typeOf(new WeakMap()));
+//=> 'object'
+console.log(typeOf(new WeakSet()));
+//=> 'object'
+```
+
+## About
+
+
+Contributing
+
+Pull requests and stars are always welcome. For bugs and feature requests, [please create an issue](../../issues/new).
+
+
+
+
+Running Tests
+
+Running and reviewing unit tests is a great way to get familiarized with a library and its API. You can install dependencies and run tests with the following command:
+
+```sh
+$ npm install && npm test
+```
+
+
+
+
+Building docs
+
+_(This project's readme.md is generated by [verb](https://github.com/verbose/verb-generate-readme), please don't edit the readme directly. Any changes to the readme must be made in the [.verb.md](.verb.md) readme template.)_
+
+To generate the readme, run the following command:
+
+```sh
+$ npm install -g verbose/verb#dev verb-generate-readme && verb
+```
+
+
+
+### Related projects
+
+You might also be interested in these projects:
+
+* [is-glob](https://www.npmjs.com/package/is-glob): Returns `true` if the given string looks like a glob pattern or an extglob pattern… [more](https://github.com/jonschlinkert/is-glob) | [homepage](https://github.com/jonschlinkert/is-glob "Returns `true` if the given string looks like a glob pattern or an extglob pattern. This makes it easy to create code that only uses external modules like node-glob when necessary, resulting in much faster code execution and initialization time, and a bet")
+* [is-number](https://www.npmjs.com/package/is-number): Returns true if the value is a number. comprehensive tests. | [homepage](https://github.com/jonschlinkert/is-number "Returns true if the value is a number. comprehensive tests.")
+* [is-primitive](https://www.npmjs.com/package/is-primitive): Returns `true` if the value is a primitive. | [homepage](https://github.com/jonschlinkert/is-primitive "Returns `true` if the value is a primitive. ")
+
+### Contributors
+
+| **Commits** | **Contributor** |
+| --- | --- |
+| 98 | [jonschlinkert](https://github.com/jonschlinkert) |
+| 3 | [aretecode](https://github.com/aretecode) |
+| 2 | [miguelmota](https://github.com/miguelmota) |
+| 1 | [dtothefp](https://github.com/dtothefp) |
+| 1 | [ianstormtaylor](https://github.com/ianstormtaylor) |
+| 1 | [ksheedlo](https://github.com/ksheedlo) |
+| 1 | [pdehaan](https://github.com/pdehaan) |
+| 1 | [laggingreflex](https://github.com/laggingreflex) |
+| 1 | [charlike-old](https://github.com/charlike-old) |
+
+### Author
+
+**Jon Schlinkert**
+
+* [linkedin/in/jonschlinkert](https://linkedin.com/in/jonschlinkert)
+* [github/jonschlinkert](https://github.com/jonschlinkert)
+* [twitter/jonschlinkert](https://twitter.com/jonschlinkert)
+
+### License
+
+Copyright © 2017, [Jon Schlinkert](https://github.com/jonschlinkert).
+Released under the [MIT License](LICENSE).
+
+***
+
+_This file was generated by [verb-generate-readme](https://github.com/verbose/verb-generate-readme), v0.6.0, on December 01, 2017._
\ No newline at end of file
diff --git a/node_modules/kind-of/index.js b/node_modules/kind-of/index.js
new file mode 100644
index 0000000..aa2bb39
--- /dev/null
+++ b/node_modules/kind-of/index.js
@@ -0,0 +1,129 @@
+var toString = Object.prototype.toString;
+
+module.exports = function kindOf(val) {
+ if (val === void 0) return 'undefined';
+ if (val === null) return 'null';
+
+ var type = typeof val;
+ if (type === 'boolean') return 'boolean';
+ if (type === 'string') return 'string';
+ if (type === 'number') return 'number';
+ if (type === 'symbol') return 'symbol';
+ if (type === 'function') {
+ return isGeneratorFn(val) ? 'generatorfunction' : 'function';
+ }
+
+ if (isArray(val)) return 'array';
+ if (isBuffer(val)) return 'buffer';
+ if (isArguments(val)) return 'arguments';
+ if (isDate(val)) return 'date';
+ if (isError(val)) return 'error';
+ if (isRegexp(val)) return 'regexp';
+
+ switch (ctorName(val)) {
+ case 'Symbol': return 'symbol';
+ case 'Promise': return 'promise';
+
+ // Set, Map, WeakSet, WeakMap
+ case 'WeakMap': return 'weakmap';
+ case 'WeakSet': return 'weakset';
+ case 'Map': return 'map';
+ case 'Set': return 'set';
+
+ // 8-bit typed arrays
+ case 'Int8Array': return 'int8array';
+ case 'Uint8Array': return 'uint8array';
+ case 'Uint8ClampedArray': return 'uint8clampedarray';
+
+ // 16-bit typed arrays
+ case 'Int16Array': return 'int16array';
+ case 'Uint16Array': return 'uint16array';
+
+ // 32-bit typed arrays
+ case 'Int32Array': return 'int32array';
+ case 'Uint32Array': return 'uint32array';
+ case 'Float32Array': return 'float32array';
+ case 'Float64Array': return 'float64array';
+ }
+
+ if (isGeneratorObj(val)) {
+ return 'generator';
+ }
+
+ // Non-plain objects
+ type = toString.call(val);
+ switch (type) {
+ case '[object Object]': return 'object';
+ // iterators
+ case '[object Map Iterator]': return 'mapiterator';
+ case '[object Set Iterator]': return 'setiterator';
+ case '[object String Iterator]': return 'stringiterator';
+ case '[object Array Iterator]': return 'arrayiterator';
+ }
+
+ // other
+ return type.slice(8, -1).toLowerCase().replace(/\s/g, '');
+};
+
+function ctorName(val) {
+ return val.constructor ? val.constructor.name : null;
+}
+
+function isArray(val) {
+ if (Array.isArray) return Array.isArray(val);
+ return val instanceof Array;
+}
+
+function isError(val) {
+ return val instanceof Error || (typeof val.message === 'string' && val.constructor && typeof val.constructor.stackTraceLimit === 'number');
+}
+
+function isDate(val) {
+ if (val instanceof Date) return true;
+ return typeof val.toDateString === 'function'
+ && typeof val.getDate === 'function'
+ && typeof val.setDate === 'function';
+}
+
+function isRegexp(val) {
+ if (val instanceof RegExp) return true;
+ return typeof val.flags === 'string'
+ && typeof val.ignoreCase === 'boolean'
+ && typeof val.multiline === 'boolean'
+ && typeof val.global === 'boolean';
+}
+
+function isGeneratorFn(name, val) {
+ return ctorName(name) === 'GeneratorFunction';
+}
+
+function isGeneratorObj(val) {
+ return typeof val.throw === 'function'
+ && typeof val.return === 'function'
+ && typeof val.next === 'function';
+}
+
+function isArguments(val) {
+ try {
+ if (typeof val.length === 'number' && typeof val.callee === 'function') {
+ return true;
+ }
+ } catch (err) {
+ if (err.message.indexOf('callee') !== -1) {
+ return true;
+ }
+ }
+ return false;
+}
+
+/**
+ * If you need to support Safari 5-7 (8-10 yr-old browser),
+ * take a look at https://github.com/feross/is-buffer
+ */
+
+function isBuffer(val) {
+ if (val.constructor && typeof val.constructor.isBuffer === 'function') {
+ return val.constructor.isBuffer(val);
+ }
+ return false;
+}
diff --git a/node_modules/kind-of/package.json b/node_modules/kind-of/package.json
new file mode 100644
index 0000000..a4baae6
--- /dev/null
+++ b/node_modules/kind-of/package.json
@@ -0,0 +1,157 @@
+{
+ "_from": "kind-of@^6.0.2",
+ "_id": "kind-of@6.0.2",
+ "_inBundle": false,
+ "_integrity": "sha512-s5kLOcnH0XqDO+FvuaLX8DDjZ18CGFk7VygH40QoKPUQhW4e2rvM0rwUq0t8IQDOwYSeLK01U90OjzBTme2QqA==",
+ "_location": "/kind-of",
+ "_phantomChildren": {},
+ "_requested": {
+ "type": "range",
+ "registry": true,
+ "raw": "kind-of@^6.0.2",
+ "name": "kind-of",
+ "escapedName": "kind-of",
+ "rawSpec": "^6.0.2",
+ "saveSpec": null,
+ "fetchSpec": "^6.0.2"
+ },
+ "_requiredBy": [
+ "/base/is-accessor-descriptor",
+ "/base/is-data-descriptor",
+ "/base/is-descriptor",
+ "/define-property/is-accessor-descriptor",
+ "/define-property/is-data-descriptor",
+ "/define-property/is-descriptor",
+ "/extglob/is-accessor-descriptor",
+ "/extglob/is-data-descriptor",
+ "/extglob/is-descriptor",
+ "/make-iterator",
+ "/micromatch",
+ "/nanomatch",
+ "/snapdragon-node/is-accessor-descriptor",
+ "/snapdragon-node/is-data-descriptor",
+ "/snapdragon-node/is-descriptor"
+ ],
+ "_resolved": "https://registry.npmjs.org/kind-of/-/kind-of-6.0.2.tgz",
+ "_shasum": "01146b36a6218e64e58f3a8d66de5d7fc6f6d051",
+ "_spec": "kind-of@^6.0.2",
+ "_where": "/Users/rpatra16/MyProjects/RamAndAntara/node_modules/micromatch",
+ "author": {
+ "name": "Jon Schlinkert",
+ "url": "https://github.com/jonschlinkert"
+ },
+ "bugs": {
+ "url": "https://github.com/jonschlinkert/kind-of/issues"
+ },
+ "bundleDependencies": false,
+ "contributors": [
+ {
+ "name": "David Fox-Powell",
+ "url": "https://dtothefp.github.io/me"
+ },
+ {
+ "name": "James",
+ "url": "https://twitter.com/aretecode"
+ },
+ {
+ "name": "Jon Schlinkert",
+ "url": "http://twitter.com/jonschlinkert"
+ },
+ {
+ "name": "Ken Sheedlo",
+ "url": "kensheedlo.com"
+ },
+ {
+ "name": "laggingreflex",
+ "url": "https://github.com/laggingreflex"
+ },
+ {
+ "name": "Miguel Mota",
+ "url": "https://miguelmota.com"
+ },
+ {
+ "name": "Peter deHaan",
+ "url": "http://about.me/peterdehaan"
+ },
+ {
+ "name": "tunnckoCore",
+ "url": "https://i.am.charlike.online"
+ }
+ ],
+ "deprecated": false,
+ "description": "Get the native type of a value.",
+ "devDependencies": {
+ "benchmarked": "^2.0.0",
+ "browserify": "^14.4.0",
+ "gulp-format-md": "^1.0.0",
+ "mocha": "^4.0.1",
+ "write": "^1.0.3"
+ },
+ "engines": {
+ "node": ">=0.10.0"
+ },
+ "files": [
+ "index.js"
+ ],
+ "homepage": "https://github.com/jonschlinkert/kind-of",
+ "keywords": [
+ "arguments",
+ "array",
+ "boolean",
+ "check",
+ "date",
+ "function",
+ "is",
+ "is-type",
+ "is-type-of",
+ "kind",
+ "kind-of",
+ "number",
+ "object",
+ "of",
+ "regexp",
+ "string",
+ "test",
+ "type",
+ "type-of",
+ "typeof",
+ "types"
+ ],
+ "license": "MIT",
+ "main": "index.js",
+ "name": "kind-of",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/jonschlinkert/kind-of.git"
+ },
+ "scripts": {
+ "prepublish": "browserify -o browser.js -e index.js -s index --bare",
+ "test": "mocha"
+ },
+ "verb": {
+ "toc": false,
+ "layout": "default",
+ "tasks": [
+ "readme"
+ ],
+ "plugins": [
+ "gulp-format-md"
+ ],
+ "lint": {
+ "reflinks": true
+ },
+ "related": {
+ "list": [
+ "is-glob",
+ "is-number",
+ "is-primitive"
+ ]
+ },
+ "reflinks": [
+ "type-of",
+ "typeof",
+ "verb"
+ ]
+ },
+ "version": "6.0.2"
+}
diff --git a/node_modules/lcid/index.js b/node_modules/lcid/index.js
new file mode 100644
index 0000000..69bd3d2
--- /dev/null
+++ b/node_modules/lcid/index.js
@@ -0,0 +1,22 @@
+'use strict';
+var invertKv = require('invert-kv');
+var all = require('./lcid.json');
+var inverted = invertKv(all);
+
+exports.from = function (lcidCode) {
+ if (typeof lcidCode !== 'number') {
+ throw new TypeError('Expected a number');
+ }
+
+ return inverted[lcidCode];
+};
+
+exports.to = function (localeId) {
+ if (typeof localeId !== 'string') {
+ throw new TypeError('Expected a string');
+ }
+
+ return all[localeId];
+};
+
+exports.all = all;
diff --git a/node_modules/lcid/lcid.json b/node_modules/lcid/lcid.json
new file mode 100644
index 0000000..9c89f6a
--- /dev/null
+++ b/node_modules/lcid/lcid.json
@@ -0,0 +1,203 @@
+{
+ "af_ZA": 1078,
+ "am_ET": 1118,
+ "ar_AE": 14337,
+ "ar_BH": 15361,
+ "ar_DZ": 5121,
+ "ar_EG": 3073,
+ "ar_IQ": 2049,
+ "ar_JO": 11265,
+ "ar_KW": 13313,
+ "ar_LB": 12289,
+ "ar_LY": 4097,
+ "ar_MA": 6145,
+ "ar_OM": 8193,
+ "ar_QA": 16385,
+ "ar_SA": 1025,
+ "ar_SY": 10241,
+ "ar_TN": 7169,
+ "ar_YE": 9217,
+ "arn_CL": 1146,
+ "as_IN": 1101,
+ "az_AZ": 2092,
+ "ba_RU": 1133,
+ "be_BY": 1059,
+ "bg_BG": 1026,
+ "bn_IN": 1093,
+ "bo_BT": 2129,
+ "bo_CN": 1105,
+ "br_FR": 1150,
+ "bs_BA": 8218,
+ "ca_ES": 1027,
+ "co_FR": 1155,
+ "cs_CZ": 1029,
+ "cy_GB": 1106,
+ "da_DK": 1030,
+ "de_AT": 3079,
+ "de_CH": 2055,
+ "de_DE": 1031,
+ "de_LI": 5127,
+ "de_LU": 4103,
+ "div_MV": 1125,
+ "dsb_DE": 2094,
+ "el_GR": 1032,
+ "en_AU": 3081,
+ "en_BZ": 10249,
+ "en_CA": 4105,
+ "en_CB": 9225,
+ "en_GB": 2057,
+ "en_IE": 6153,
+ "en_IN": 18441,
+ "en_JA": 8201,
+ "en_MY": 17417,
+ "en_NZ": 5129,
+ "en_PH": 13321,
+ "en_TT": 11273,
+ "en_US": 1033,
+ "en_ZA": 7177,
+ "en_ZW": 12297,
+ "es_AR": 11274,
+ "es_BO": 16394,
+ "es_CL": 13322,
+ "es_CO": 9226,
+ "es_CR": 5130,
+ "es_DO": 7178,
+ "es_EC": 12298,
+ "es_ES": 3082,
+ "es_GT": 4106,
+ "es_HN": 18442,
+ "es_MX": 2058,
+ "es_NI": 19466,
+ "es_PA": 6154,
+ "es_PE": 10250,
+ "es_PR": 20490,
+ "es_PY": 15370,
+ "es_SV": 17418,
+ "es_UR": 14346,
+ "es_US": 21514,
+ "es_VE": 8202,
+ "et_EE": 1061,
+ "eu_ES": 1069,
+ "fa_IR": 1065,
+ "fi_FI": 1035,
+ "fil_PH": 1124,
+ "fo_FO": 1080,
+ "fr_BE": 2060,
+ "fr_CA": 3084,
+ "fr_CH": 4108,
+ "fr_FR": 1036,
+ "fr_LU": 5132,
+ "fr_MC": 6156,
+ "fy_NL": 1122,
+ "ga_IE": 2108,
+ "gbz_AF": 1164,
+ "gl_ES": 1110,
+ "gsw_FR": 1156,
+ "gu_IN": 1095,
+ "ha_NG": 1128,
+ "he_IL": 1037,
+ "hi_IN": 1081,
+ "hr_BA": 4122,
+ "hr_HR": 1050,
+ "hu_HU": 1038,
+ "hy_AM": 1067,
+ "id_ID": 1057,
+ "ii_CN": 1144,
+ "is_IS": 1039,
+ "it_CH": 2064,
+ "it_IT": 1040,
+ "iu_CA": 2141,
+ "ja_JP": 1041,
+ "ka_GE": 1079,
+ "kh_KH": 1107,
+ "kk_KZ": 1087,
+ "kl_GL": 1135,
+ "kn_IN": 1099,
+ "ko_KR": 1042,
+ "kok_IN": 1111,
+ "ky_KG": 1088,
+ "lb_LU": 1134,
+ "lo_LA": 1108,
+ "lt_LT": 1063,
+ "lv_LV": 1062,
+ "mi_NZ": 1153,
+ "mk_MK": 1071,
+ "ml_IN": 1100,
+ "mn_CN": 2128,
+ "mn_MN": 1104,
+ "moh_CA": 1148,
+ "mr_IN": 1102,
+ "ms_BN": 2110,
+ "ms_MY": 1086,
+ "mt_MT": 1082,
+ "my_MM": 1109,
+ "nb_NO": 1044,
+ "ne_NP": 1121,
+ "nl_BE": 2067,
+ "nl_NL": 1043,
+ "nn_NO": 2068,
+ "ns_ZA": 1132,
+ "oc_FR": 1154,
+ "or_IN": 1096,
+ "pa_IN": 1094,
+ "pl_PL": 1045,
+ "ps_AF": 1123,
+ "pt_BR": 1046,
+ "pt_PT": 2070,
+ "qut_GT": 1158,
+ "quz_BO": 1131,
+ "quz_EC": 2155,
+ "quz_PE": 3179,
+ "rm_CH": 1047,
+ "ro_RO": 1048,
+ "ru_RU": 1049,
+ "rw_RW": 1159,
+ "sa_IN": 1103,
+ "sah_RU": 1157,
+ "se_FI": 3131,
+ "se_NO": 1083,
+ "se_SE": 2107,
+ "si_LK": 1115,
+ "sk_SK": 1051,
+ "sl_SI": 1060,
+ "sma_NO": 6203,
+ "sma_SE": 7227,
+ "smj_NO": 4155,
+ "smj_SE": 5179,
+ "smn_FI": 9275,
+ "sms_FI": 8251,
+ "sq_AL": 1052,
+ "sr_BA": 7194,
+ "sr_SP": 3098,
+ "sv_FI": 2077,
+ "sv_SE": 1053,
+ "sw_KE": 1089,
+ "syr_SY": 1114,
+ "ta_IN": 1097,
+ "te_IN": 1098,
+ "tg_TJ": 1064,
+ "th_TH": 1054,
+ "tk_TM": 1090,
+ "tmz_DZ": 2143,
+ "tn_ZA": 1074,
+ "tr_TR": 1055,
+ "tt_RU": 1092,
+ "ug_CN": 1152,
+ "uk_UA": 1058,
+ "ur_IN": 2080,
+ "ur_PK": 1056,
+ "uz_UZ": 2115,
+ "vi_VN": 1066,
+ "wen_DE": 1070,
+ "wo_SN": 1160,
+ "xh_ZA": 1076,
+ "yo_NG": 1130,
+ "zh_CHS": 4,
+ "zh_CHT": 31748,
+ "zh_CN": 2052,
+ "zh_HK": 3076,
+ "zh_MO": 5124,
+ "zh_SG": 4100,
+ "zh_TW": 1028,
+ "zu_ZA": 1077
+}
diff --git a/node_modules/lcid/license b/node_modules/lcid/license
new file mode 100644
index 0000000..654d0bf
--- /dev/null
+++ b/node_modules/lcid/license
@@ -0,0 +1,21 @@
+The MIT License (MIT)
+
+Copyright (c) Sindre Sorhus (sindresorhus.com)
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in
+all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
+THE SOFTWARE.
diff --git a/node_modules/lcid/package.json b/node_modules/lcid/package.json
new file mode 100644
index 0000000..7a13c1d
--- /dev/null
+++ b/node_modules/lcid/package.json
@@ -0,0 +1,109 @@
+{
+ "_args": [
+ [
+ {
+ "raw": "lcid@^1.0.0",
+ "scope": null,
+ "escapedName": "lcid",
+ "name": "lcid",
+ "rawSpec": "^1.0.0",
+ "spec": ">=1.0.0 <2.0.0",
+ "type": "range"
+ },
+ "/Users/ramswaroop/MyProjects/RamAndAntara/node_modules/os-locale"
+ ]
+ ],
+ "_from": "lcid@>=1.0.0 <2.0.0",
+ "_id": "lcid@1.0.0",
+ "_inCache": true,
+ "_location": "/lcid",
+ "_nodeVersion": "0.12.0",
+ "_npmUser": {
+ "name": "sindresorhus",
+ "email": "sindresorhus@gmail.com"
+ },
+ "_npmVersion": "2.5.1",
+ "_phantomChildren": {},
+ "_requested": {
+ "raw": "lcid@^1.0.0",
+ "scope": null,
+ "escapedName": "lcid",
+ "name": "lcid",
+ "rawSpec": "^1.0.0",
+ "spec": ">=1.0.0 <2.0.0",
+ "type": "range"
+ },
+ "_requiredBy": [
+ "/os-locale"
+ ],
+ "_resolved": "https://registry.npmjs.org/lcid/-/lcid-1.0.0.tgz",
+ "_shasum": "308accafa0bc483a3867b4b6f2b9506251d1b835",
+ "_shrinkwrap": null,
+ "_spec": "lcid@^1.0.0",
+ "_where": "/Users/ramswaroop/MyProjects/RamAndAntara/node_modules/os-locale",
+ "author": {
+ "name": "Sindre Sorhus",
+ "email": "sindresorhus@gmail.com",
+ "url": "sindresorhus.com"
+ },
+ "bugs": {
+ "url": "https://github.com/sindresorhus/lcid/issues"
+ },
+ "dependencies": {
+ "invert-kv": "^1.0.0"
+ },
+ "description": "Mapping between standard locale identifiers and Windows locale identifiers (LCID)",
+ "devDependencies": {
+ "ava": "0.0.4"
+ },
+ "directories": {},
+ "dist": {
+ "shasum": "308accafa0bc483a3867b4b6f2b9506251d1b835",
+ "tarball": "https://registry.npmjs.org/lcid/-/lcid-1.0.0.tgz"
+ },
+ "engines": {
+ "node": ">=0.10.0"
+ },
+ "files": [
+ "index.js",
+ "lcid.json"
+ ],
+ "gitHead": "96bb3e617f77f5f8ceb78653c77de5a85abb3b1e",
+ "homepage": "https://github.com/sindresorhus/lcid",
+ "keywords": [
+ "lcid",
+ "locale",
+ "string",
+ "str",
+ "id",
+ "identifier",
+ "windows",
+ "language",
+ "lang",
+ "map",
+ "mapping",
+ "convert",
+ "json",
+ "bcp47",
+ "ietf",
+ "tag"
+ ],
+ "license": "MIT",
+ "maintainers": [
+ {
+ "name": "sindresorhus",
+ "email": "sindresorhus@gmail.com"
+ }
+ ],
+ "name": "lcid",
+ "optionalDependencies": {},
+ "readme": "ERROR: No README data found!",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/sindresorhus/lcid.git"
+ },
+ "scripts": {
+ "test": "node test.js"
+ },
+ "version": "1.0.0"
+}
diff --git a/node_modules/lcid/readme.md b/node_modules/lcid/readme.md
new file mode 100644
index 0000000..bee4a70
--- /dev/null
+++ b/node_modules/lcid/readme.md
@@ -0,0 +1,35 @@
+# lcid [](https://travis-ci.org/sindresorhus/lcid)
+
+> Mapping between [standard locale identifiers](http://en.wikipedia.org/wiki/Locale) and [Windows locale identifiers (LCID)](http://en.wikipedia.org/wiki/Locale#Specifics_for_Microsoft_platforms)
+
+Based on the [mapping](https://github.com/python/cpython/blob/be2a1a76fa43bb1ea1b3577bb5bdd506a2e90e37/Lib/locale.py#L1395-L1604) used in the Python standard library.
+
+The mapping itself is just a [JSON file](lcid.json) and can be used wherever.
+
+
+## Install
+
+```
+$ npm install --save lcid
+```
+
+
+## Usage
+
+```js
+var lcid = require('lcid');
+
+lcid.from(1044);
+//=> 'nb_NO'
+
+lcid.to('nb_NO');
+//=> 1044
+
+lcid.all;
+//=> {'af_ZA': 1078, ...}
+```
+
+
+## License
+
+MIT © [Sindre Sorhus](http://sindresorhus.com)
diff --git a/node_modules/liftoff/CHANGELOG b/node_modules/liftoff/CHANGELOG
new file mode 100644
index 0000000..e612f4e
--- /dev/null
+++ b/node_modules/liftoff/CHANGELOG
@@ -0,0 +1,127 @@
+v2.2.2:
+ date: 2016-05-20
+ changes:
+ - Update dependencies.
+v2.2.1:
+ date: 2016-03-23
+ changes:
+ - Make sure that v8 flags are passed properly through the `respawn` event
+v2.1.0:
+ date: 2015-05-20
+ changes:
+ - Use rechoir to autoload modules.
+v2.0.3:
+ date: 2015-03-31
+ changes:
+ - Internal bugfix, don't wrap callback error in another error, idiot.
+v2.0.2:
+ date: 2015-02-24
+ changes:
+ - Support process.env.NODE_PATH when resolving module.
+v2.0.1:
+ date: 2015-02-01
+ changes:
+ - Find modulePath correctly when devving against yourself.
+v2.0.0:
+ date: 2015-01-15
+ changes:
+ - Rename `nodeFlags` to `v8Flags` and make it async.
+v1.0.4:
+ date: 2015-01-04
+ changes:
+ - Detect config extension using basename, not full path.
+v1.0.0:
+ date: 2014-12-16
+ changes:
+ - Update dependencies
+v0.13.6:
+ date: 2014-11-07
+ changes:
+ - Don't include artwork on npm.
+v0.13.5:
+ date: 2014-10-10
+ changes:
+ - Only attempt to resolve the real path of configFile if it is actually a symlink.
+v0.13.4:
+ date: 2014-10-07
+ changes:
+ - Set configBase to the directory of the symlink, not the directory of its real location.
+v0.13.3:
+ date: 2014-10-06
+ changes:
+ - Return the real location of symlinked config files.
+v0.13.2:
+ date: 2014-09-12
+ changes:
+ - Include flags in respawn event. I really miss `npm publish --force`.
+v0.13.1:
+ date: 2014-09-12
+ changes:
+ - Slight performance tweak.
+v0.13.0:
+ date: 2014-09-12
+ changes:
+ - Support passing flags to node with `nodeFlags` option.
+v0.12.1:
+ date: 2014-06-27
+ changes:
+ - Support preloading modules for compound extensions like `.coffee.md`.
+v0.12.0:
+ date: 2014-06-27
+ changes:
+ - Respect order of extensions when searching for config.
+ - Rename `configNameRegex` environment property to `configNameSearch`.
+v0.11.3:
+ date: 2014-06-09
+ changes:
+ - Make cwd match configBase if cwd isn't explicitly provided
+v0.11.2:
+ date: 2014-06-04
+ changes:
+ - Regression fix: coerce preloads into array before attempting to push more
+v0.11.1:
+ date: 2014-06-02
+ changes:
+ - Update dependencies.
+v0.11.0:
+ date: 2014-05-27
+ changes:
+ - Refactor and remove options parsing.
+v0.10.0:
+ date: 2014-05-06
+ changes:
+ - Remove `addExtension` in favor of `extension` option.
+ - Support preloading modules based on extension.
+v0.9.7:
+ date: 2014-04-28
+ changes:
+ - Locate local module in cwd even if config isn't present.
+v0.9.6:
+ date: 2014-04-02
+ changes:
+ - Fix regression where external modules are not properly required.
+ - Ignore configPathFlag / cwdFlag if the value isn't a string
+v0.9.3:
+ date: 2014-02-28
+ changes:
+ - Fix regression where developing against self doesn't correctly set cwd.
+v0.9.0:
+ date: 2014-02-28
+ changes:
+ - Use liftoff instance as context (`this`) for launch callback.
+ - Support split --cwd and --configfile locations.
+ - Rename `configLocationFlag` to `configPathFlag`
+ - Support node 0.8+
+v0.8.7:
+ date: 2014-02-24
+ changes:
+ - Pass environment as first argument to `launch`.
+v0.8.5:
+ date: 2014-02-19
+ changes:
+ - Implement `addExtensions` option.
+ - Default to `index.js` if `modulePackage` has no `main` property.
+v0.8.4:
+ date: 2014-02-05
+ changes:
+ - Initial public release.
diff --git a/node_modules/liftoff/LICENSE b/node_modules/liftoff/LICENSE
new file mode 100644
index 0000000..a55f5b7
--- /dev/null
+++ b/node_modules/liftoff/LICENSE
@@ -0,0 +1,22 @@
+Copyright (c) 2014 Tyler Kellen
+
+Permission is hereby granted, free of charge, to any person
+obtaining a copy of this software and associated documentation
+files (the "Software"), to deal in the Software without
+restriction, including without limitation the rights to use,
+copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the
+Software is furnished to do so, subject to the following
+conditions:
+
+The above copyright notice and this permission notice shall be
+included in all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
+EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES
+OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
+NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT
+HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY,
+WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
+FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR
+OTHER DEALINGS IN THE SOFTWARE.
diff --git a/node_modules/liftoff/README.md b/node_modules/liftoff/README.md
new file mode 100644
index 0000000..892f03d
--- /dev/null
+++ b/node_modules/liftoff/README.md
@@ -0,0 +1,451 @@
+
+
+
+
+
+
+# liftoff [](http://travis-ci.org/js-cli/js-liftoff) [](https://ci.appveyor.com/project/phated/js-liftoff)
+
+
+> Launch your command line tool with ease.
+
+[](https://nodei.co/npm/liftoff/)
+
+## What is it?
+[See this blog post](http://weblog.bocoup.com/building-command-line-tools-in-node-with-liftoff/), [check out this proof of concept](https://github.com/js-cli/js-hacker), or read on.
+
+Say you're writing a CLI tool. Let's call it [hacker](https://github.com/js-cli/js-hacker). You want to configure it using a `Hackerfile`. This is node, so you install `hacker` locally for each project you use it in. But, in order to get the `hacker` command in your PATH, you also install it globally.
+
+Now, when you run `hacker`, you want to configure what it does using the `Hackerfile` in your current directory, and you want it to execute using the local installation of your tool. Also, it'd be nice if the `hacker` command was smart enough to traverse up your folders until it finds a `Hackerfile`—for those times when you're not in the root directory of your project. Heck, you might even want to launch `hacker` from a folder outside of your project by manually specifying a working directory. Liftoff manages this for you.
+
+So, everything is working great. Now you can find your local `hacker` and `Hackerfile` with ease. Unfortunately, it turns out you've authored your `Hackerfile` in coffee-script, or some other JS variant. In order to support *that*, you have to load the compiler for it, and then register the extension for it with node. Good news, Liftoff can do that, and a whole lot more, too.
+
+## API
+
+### constructor(opts)
+
+Create an instance of Liftoff to invoke your application.
+
+An example utilizing all options:
+```js
+const Hacker = new Liftoff({
+ name: 'hacker',
+ processTitle: 'hacker',
+ moduleName: 'hacker',
+ configName: 'hackerfile',
+ extensions: {
+ '.js': null,
+ '.json': null,
+ '.coffee': 'coffee-script/register'
+ },
+ v8flags: ['--harmony'] // or v8flags: require('v8flags')
+});
+```
+
+#### opts.name
+
+Sugar for setting `processTitle`, `moduleName`, `configName` automatically.
+
+Type: `String`
+Default: `null`
+
+These are equivalent:
+```js
+const Hacker = Liftoff({
+ processTitle: 'hacker',
+ moduleName: 'hacker',
+ configName: 'hackerfile'
+});
+```
+```js
+const Hacker = Liftoff({name:'hacker'});
+```
+
+#### opts.moduleName
+
+Sets which module your application expects to find locally when being run.
+
+Type: `String`
+Default: `null`
+
+#### opts.configName
+
+Sets the name of the configuration file Liftoff will attempt to find. Case-insensitive.
+
+Type: `String`
+Default: `null`
+
+#### opts.extensions
+
+Set extensions to include when searching for a configuration file. If an external module is needed to load a given extension (e.g. `.coffee`), the module name should be specified as the value for the key.
+
+Type: `Object`
+Default: `{".js":null,".json":null}`
+
+**Examples:**
+
+In this example Liftoff will look for `myappfile{.js,.json,.coffee}`. If a config with the extension `.coffee` is found, Liftoff will try to require `coffee-script/require` from the current working directory.
+```js
+const MyApp = new Liftoff({
+ name: 'myapp',
+ extensions: {
+ '.js': null,
+ '.json': null,
+ '.coffee': 'coffee-script/register'
+ }
+});
+```
+
+In this example, Liftoff will look for `.myapp{rc}`.
+```js
+const MyApp = new Liftoff({
+ name: 'myapp',
+ configName: '.myapp',
+ extensions: {
+ 'rc': null
+ }
+});
+```
+
+In this example, Liftoff will automatically attempt to load the correct module for any javascript variant supported by [interpret](https://github.com/js-cli/js-interpret) (as long as it does not require a register method).
+
+```js
+const MyApp = new Liftoff({
+ name: 'myapp',
+ extensions: require('interpret').jsVariants
+});
+```
+#### opts.v8flags
+
+Any flag specified here will be applied to node, not your program. Useful for supporting invocations like `myapp --harmony command`, where `--harmony` should be passed to node, not your program. This functionality is implemented using [flagged-respawn](http://github.com/js-cli/js-flagged-respawn). To support all v8flags, see [v8flags](https://github.com/js-cli/js-v8flags).
+
+Type: `Array|Function`
+Default: `null`
+
+If this method is a function, it should take a node-style callback that yields an array of flags.
+
+#### opts.processTitle
+
+Sets what the [process title](http://nodejs.org/api/process.html#process_process_title) will be.
+
+Type: `String`
+Default: `null`
+
+#### opts.completions(type)
+
+A method to handle bash/zsh/whatever completions.
+
+Type: `Function`
+Default: `null`
+
+#### opts.configFiles
+
+An object of configuration files to find. Each property is keyed by the default basename of the file being found, and the value is an object of [path arguments](#path-arguments) keyed by unique names.
+
+__Note:__ This option is useful if, for example, you want to support an `.apprc` file in addition to an `appfile.js`. If you only need a single configuration file, you probably don't need this. In addition to letting you find multiple files, this option allows more fine-grained control over how configuration files are located.
+
+Type: `Object`
+Default: `null`
+
+#### Path arguments
+
+The [`fined`](https://github.com/js-cli/fined) module accepts a string representing the path to search or an object with the following keys:
+
+* `path` __(required)__
+
+ The path to search. Using only a string expands to this property.
+
+ Type: `String`
+ Default: `null`
+
+* `name`
+
+ The basename of the file to find. Extensions are appended during lookup.
+
+ Type: `String`
+ Default: Top-level key in `configFiles`
+
+* `extensions`
+
+ The extensions to append to `name` during lookup. See also: [`opts.extensions`](#optsextensions).
+
+ Type: `String|Array|Object`
+ Default: The value of [`opts.extensions`](#optsextensions)
+
+* `cwd`
+
+ The base directory of `path` (if relative).
+
+ Type: `String`
+ Default: The value of [`opts.cwd`](#optscwd)
+
+* `findUp`
+
+ Whether the `path` should be traversed up to find the file.
+
+ Type: `Boolean`
+ Default: `false`
+
+**Examples:**
+
+In this example Liftoff will look for the `.hacker.js` file relative to the `cwd` as declared in `configFiles`.
+```js
+const MyApp = new Liftoff({
+ name: 'hacker',
+ configFiles: {
+ '.hacker': {
+ cwd: '.'
+ }
+ }
+});
+```
+
+In this example, Liftoff will look for `.hackerrc` in the home directory.
+```js
+const MyApp = new Liftoff({
+ name: 'hacker',
+ configFiles: {
+ '.hacker': {
+ home: {
+ path: '~',
+ extensions: {
+ 'rc': null
+ }
+ }
+ }
+ }
+});
+```
+
+In this example, Liftoff will look in the `cwd` and then lookup the tree for the `.hacker.js` file.
+```js
+const MyApp = new Liftoff({
+ name: 'hacker',
+ configFiles: {
+ '.hacker': {
+ up: {
+ path: '.',
+ findUp: true
+ }
+ }
+ }
+});
+```
+
+In this example, the `name` is overridden and the key is ignored so Liftoff looks for `.override.js`.
+```js
+const MyApp = new Liftoff({
+ name: 'hacker',
+ configFiles: {
+ hacker: {
+ override: {
+ path: '.',
+ name: '.override'
+ }
+ }
+ }
+});
+```
+
+In this example, Liftoff will use the home directory as the `cwd` and looks for `~/.hacker.js`.
+```js
+const MyApp = new Liftoff({
+ name: 'hacker',
+ configFiles: {
+ '.hacker': {
+ home: {
+ path: '.',
+ cwd: '~'
+ }
+ }
+ }
+});
+```
+
+## launch(opts, callback(env))
+Launches your application with provided options, builds an environment, and invokes your callback, passing the calculated environment as the first argument.
+
+##### Example Configuration w/ Options Parsing:
+```js
+const Liftoff = require('liftoff');
+const MyApp = new Liftoff({name:'myapp'});
+const argv = require('minimist')(process.argv.slice(2));
+const invoke = function (env) {
+ console.log('my environment is:', env);
+ console.log('my cli options are:', argv);
+ console.log('my liftoff config is:', this);
+};
+MyApp.launch({
+ cwd: argv.cwd,
+ configPath: argv.myappfile,
+ require: argv.require,
+ completion: argv.completion
+}, invoke);
+```
+
+#### opts.cwd
+
+Change the current working directory for this launch. Relative paths are calculated against `process.cwd()`.
+
+Type: `String`
+Default: `process.cwd()`
+
+**Example Configuration:**
+```js
+const argv = require('minimist')(process.argv.slice(2));
+MyApp.launch({
+ cwd: argv.cwd
+}, invoke);
+```
+
+**Matching CLI Invocation:**
+```
+myapp --cwd ../
+```
+
+#### opts.configPath
+
+Don't search for a config, use the one provided. **Note:** Liftoff will assume the current working directory is the directory containing the config file unless an alternate location is explicitly specified using `cwd`.
+
+Type: `String`
+Default: `null`
+
+**Example Configuration:**
+```js
+var argv = require('minimist')(process.argv.slice(2));
+MyApp.launch({
+ configPath: argv.myappfile
+}, invoke);
+```
+
+**Matching CLI Invocation:**
+```
+myapp --myappfile /var/www/project/Myappfile.js
+```
+
+**Examples using `cwd` and `configPath` together:**
+
+These are functionally identical:
+```
+myapp --myappfile /var/www/project/Myappfile.js
+myapp --cwd /var/www/project
+```
+
+These can run myapp from a shared directory as though it were located in another project:
+```
+myapp --myappfile /Users/name/Myappfile.js --cwd /var/www/project1
+myapp --myappfile /Users/name/Myappfile.js --cwd /var/www/project2
+```
+
+#### opts.require
+
+A string or array of modules to attempt requiring from the local working directory before invoking the launch callback.
+
+Type: `String|Array`
+Default: `null`
+
+**Example Configuration:**
+```js
+var argv = require('minimist')(process.argv.slice(2));
+MyApp.launch({
+ require: argv.require
+}, invoke);
+```
+
+**Matching CLI Invocation:**
+```js
+myapp --require coffee-script/register
+```
+
+#### opts.forcedFlags
+
+Allows you to force node or V8 flags during the launch. This is useful if you need to make sure certain flags will always be enabled or if you need to enable flags that don't show up in `opts.v8flags` (as these flags aren't validated against `opts.v8flags`).
+
+If this is specified as a function, it will receive the built `env` as its only argument and must return a string or array of flags to force.
+
+Type: `String|Array|Function`
+Default: `null`
+
+**Example Configuration:**
+```js
+MyApp.launch({
+ forcedFlags: ['--trace-deprecation']
+}, invoke);
+```
+
+**Matching CLI Invocation:**
+```js
+myapp --trace-deprecation
+```
+
+#### callback(env)
+
+A function to start your application. When invoked, `this` will be your instance of Liftoff. The `env` param will contain the following keys:
+
+- `cwd`: the current working directory
+- `require`: an array of modules that liftoff tried to pre-load
+- `configNameSearch`: the config files searched for
+- `configPath`: the full path to your configuration file (if found)
+- `configBase`: the base directory of your configuration file (if found)
+- `modulePath`: the full path to the local module your project relies on (if found)
+- `modulePackage`: the contents of the local module's package.json (if found)
+- `configFiles`: an object of filepaths for each found config file (filepath values will be null if not found)
+
+### events
+
+#### require(name, module)
+
+Emitted when a module is pre-loaded.
+
+```js
+var Hacker = new Liftoff({name:'hacker'});
+Hacker.on('require', function (name, module) {
+ console.log('Requiring external module: '+name+'...');
+ // automatically register coffee-script extensions
+ if (name === 'coffee-script') {
+ module.register();
+ }
+});
+```
+
+#### requireFail(name, err)
+
+Emitted when a requested module cannot be preloaded.
+
+```js
+var Hacker = new Liftoff({name:'hacker'});
+Hacker.on('requireFail', function (name, err) {
+ console.log('Unable to load:', name, err);
+});
+```
+
+#### respawn(flags, child)
+
+Emitted when Liftoff re-spawns your process (when a [`v8flags`](#optsv8flags) is detected).
+
+```js
+var Hacker = new Liftoff({
+ name: 'hacker',
+ v8flags: ['--harmony']
+});
+Hacker.on('respawn', function (flags, child) {
+ console.log('Detected node flags:', flags);
+ console.log('Respawned to PID:', child.pid);
+});
+```
+
+Event will be triggered for this command:
+`hacker --harmony commmand`
+
+## Examples
+
+Check out how [gulp](https://github.com/gulpjs/gulp-cli/blob/master/index.js) uses Liftoff.
+
+For a bare-bones example, try [the hacker project](https://github.com/js-cli/js-hacker/blob/master/bin/hacker.js).
+
+To try the example, do the following:
+
+1. Install the sample project `hacker` with `npm install -g hacker`.
+2. Make a `Hackerfile.js` with some arbitrary javascript it.
+3. Install hacker next to it with `npm install hacker`.
+3. Run `hacker` while in the same parent folder.
diff --git a/node_modules/liftoff/index.js b/node_modules/liftoff/index.js
new file mode 100644
index 0000000..7415dcf
--- /dev/null
+++ b/node_modules/liftoff/index.js
@@ -0,0 +1,211 @@
+const fs = require('fs');
+const util = require('util');
+const path = require('path');
+const EE = require('events').EventEmitter;
+
+const extend = require('extend');
+const resolve = require('resolve');
+const flaggedRespawn = require('flagged-respawn');
+const isPlainObject = require('is-plain-object');
+const mapValues = require('object.map');
+const fined = require('fined');
+
+const findCwd = require('./lib/find_cwd');
+const findConfig = require('./lib/find_config');
+const fileSearch = require('./lib/file_search');
+const parseOptions = require('./lib/parse_options');
+const silentRequire = require('./lib/silent_require');
+const buildConfigName = require('./lib/build_config_name');
+const registerLoader = require('./lib/register_loader');
+const getNodeFlags = require('./lib/get_node_flags');
+
+
+function Liftoff (opts) {
+ EE.call(this);
+ extend(this, parseOptions(opts));
+}
+util.inherits(Liftoff, EE);
+
+Liftoff.prototype.requireLocal = function (module, basedir) {
+ try {
+ var result = require(resolve.sync(module, {basedir: basedir}));
+ this.emit('require', module, result);
+ return result;
+ } catch (e) {
+ this.emit('requireFail', module, e);
+ }
+};
+
+Liftoff.prototype.buildEnvironment = function (opts) {
+ opts = opts || {};
+
+ // get modules we want to preload
+ var preload = opts.require || [];
+
+ // ensure items to preload is an array
+ if (!Array.isArray(preload)) {
+ preload = [preload];
+ }
+
+ // make a copy of search paths that can be mutated for this run
+ var searchPaths = this.searchPaths.slice();
+
+ // calculate current cwd
+ var cwd = findCwd(opts);
+
+ // if cwd was provided explicitly, only use it for searching config
+ if (opts.cwd) {
+ searchPaths = [cwd];
+ } else {
+ // otherwise just search in cwd first
+ searchPaths.unshift(cwd);
+ }
+
+ // calculate the regex to use for finding the config file
+ var configNameSearch = buildConfigName({
+ configName: this.configName,
+ extensions: Object.keys(this.extensions)
+ });
+
+ // calculate configPath
+ var configPath = findConfig({
+ configNameSearch: configNameSearch,
+ searchPaths: searchPaths,
+ configPath: opts.configPath
+ });
+
+ // if we have a config path, save the directory it resides in.
+ var configBase;
+ if (configPath) {
+ configBase = path.dirname(configPath);
+ // if cwd wasn't provided explicitly, it should match configBase
+ if (!opts.cwd) {
+ cwd = configBase;
+ }
+ // resolve symlink if needed
+ if (fs.lstatSync(configPath).isSymbolicLink()) {
+ configPath = fs.realpathSync(configPath);
+ }
+ }
+
+ // TODO: break this out into lib/
+ // locate local module and package next to config or explicitly provided cwd
+ var modulePath, modulePackage;
+ try {
+ var delim = path.delimiter,
+ paths = (process.env.NODE_PATH ? process.env.NODE_PATH.split(delim) : []);
+ modulePath = resolve.sync(this.moduleName, {basedir: configBase || cwd, paths: paths});
+ modulePackage = silentRequire(fileSearch('package.json', [modulePath]));
+ } catch (e) {}
+
+ // if we have a configuration but we failed to find a local module, maybe
+ // we are developing against ourselves?
+ if (!modulePath && configPath) {
+ // check the package.json sibling to our config to see if its `name`
+ // matches the module we're looking for
+ var modulePackagePath = fileSearch('package.json', [configBase]);
+ modulePackage = silentRequire(modulePackagePath);
+ if (modulePackage && modulePackage.name === this.moduleName) {
+ // if it does, our module path is `main` inside package.json
+ modulePath = path.join(path.dirname(modulePackagePath), modulePackage.main || 'index.js');
+ cwd = configBase;
+ } else {
+ // clear if we just required a package for some other project
+ modulePackage = {};
+ }
+ }
+
+ // load any modules which were requested to be required
+ if (preload.length) {
+ // unique results first
+ preload.filter(function (value, index, self) {
+ return self.indexOf(value) === index;
+ }).forEach(function (dep) {
+ this.requireLocal(dep, findCwd(opts));
+ }, this);
+ }
+
+ var exts = this.extensions;
+ var eventEmitter = this;
+ registerLoader(eventEmitter, exts, configPath, cwd);
+
+ var configFiles = {};
+ if (isPlainObject(this.configFiles)) {
+ var notfound = { path: null };
+ configFiles = mapValues(this.configFiles, function(prop, name) {
+ var defaultObj = { name: name, cwd: cwd, extensions: exts };
+ return mapValues(prop, function(pathObj) {
+ var found = fined(pathObj, defaultObj) || notfound;
+ if (isPlainObject(found.extension)) {
+ registerLoader(eventEmitter, found.extension, found.path, cwd);
+ }
+ return found.path;
+ });
+ });
+ }
+
+ return {
+ cwd: cwd,
+ require: preload,
+ configNameSearch: configNameSearch,
+ configPath: configPath,
+ configBase: configBase,
+ modulePath: modulePath,
+ modulePackage: modulePackage || {},
+ configFiles: configFiles
+ };
+};
+
+Liftoff.prototype.handleFlags = function (cb) {
+ if (typeof this.v8flags === 'function') {
+ this.v8flags(function (err, flags) {
+ if (err) {
+ cb(err);
+ } else {
+ cb(null, flags);
+ }
+ });
+ } else {
+ process.nextTick(function () {
+ cb(null, this.v8flags);
+ }.bind(this));
+ }
+};
+
+Liftoff.prototype.launch = function (opts, fn) {
+ if (typeof fn !== 'function') {
+ throw new Error('You must provide a callback function.');
+ }
+ process.title = this.processTitle;
+
+ var completion = opts.completion;
+ if (completion && this.completions) {
+ return this.completions(completion);
+ }
+
+ this.handleFlags(function (err, flags) {
+ if (err) {
+ throw err;
+ }
+ flags = flags || [];
+
+ var env = this.buildEnvironment(opts);
+
+ var forcedFlags = getNodeFlags.arrayOrFunction(opts.forcedFlags, env);
+ flaggedRespawn(flags, process.argv, forcedFlags, execute.bind(this));
+
+ function execute(ready, child, argv) {
+ if (child !== process) {
+ var execArgv = getNodeFlags.fromReorderedArgv(argv);
+ this.emit('respawn', execArgv, child);
+ }
+ if (ready) {
+ fn.call(this, env, argv);
+ }
+ }
+ }.bind(this));
+};
+
+
+
+module.exports = Liftoff;
diff --git a/node_modules/liftoff/lib/build_config_name.js b/node_modules/liftoff/lib/build_config_name.js
new file mode 100644
index 0000000..b83e185
--- /dev/null
+++ b/node_modules/liftoff/lib/build_config_name.js
@@ -0,0 +1,17 @@
+module.exports = function (opts) {
+ opts = opts || {};
+ var configName = opts.configName;
+ var extensions = opts.extensions;
+ if (!configName) {
+ throw new Error('Please specify a configName.');
+ }
+ if (configName instanceof RegExp) {
+ return [configName];
+ }
+ if (!Array.isArray(extensions)) {
+ throw new Error('Please provide an array of valid extensions.');
+ }
+ return extensions.map(function (ext) {
+ return configName + ext;
+ });
+};
diff --git a/node_modules/liftoff/lib/file_search.js b/node_modules/liftoff/lib/file_search.js
new file mode 100644
index 0000000..76dadd6
--- /dev/null
+++ b/node_modules/liftoff/lib/file_search.js
@@ -0,0 +1,14 @@
+const findup = require('findup-sync');
+
+module.exports = function (search, paths) {
+ var path;
+ var len = paths.length;
+ for (var i = 0; i < len; i++) {
+ if (path) {
+ break;
+ } else {
+ path = findup(search, {cwd: paths[i], nocase: true});
+ }
+ }
+ return path;
+};
diff --git a/node_modules/liftoff/lib/find_config.js b/node_modules/liftoff/lib/find_config.js
new file mode 100644
index 0000000..71c3f07
--- /dev/null
+++ b/node_modules/liftoff/lib/find_config.js
@@ -0,0 +1,25 @@
+const fs = require('fs');
+const path = require('path');
+const fileSearch = require('./file_search');
+
+module.exports = function (opts) {
+ opts = opts || {};
+ var configNameSearch = opts.configNameSearch;
+ var configPath = opts.configPath;
+ var searchPaths = opts.searchPaths;
+ // only search for a config if a path to one wasn't explicitly provided
+ if (!configPath) {
+ if (!Array.isArray(searchPaths)) {
+ throw new Error('Please provide an array of paths to search for config in.');
+ }
+ if (!configNameSearch) {
+ throw new Error('Please provide a configNameSearch.');
+ }
+ configPath = fileSearch(configNameSearch, searchPaths);
+ }
+ // confirm the configPath exists and return an absolute path to it
+ if (fs.existsSync(configPath)) {
+ return path.resolve(configPath);
+ }
+ return null;
+};
diff --git a/node_modules/liftoff/lib/find_cwd.js b/node_modules/liftoff/lib/find_cwd.js
new file mode 100644
index 0000000..2a029b9
--- /dev/null
+++ b/node_modules/liftoff/lib/find_cwd.js
@@ -0,0 +1,18 @@
+const path = require('path');
+
+module.exports = function (opts) {
+ if (!opts) {
+ opts = {};
+ }
+ var cwd = opts.cwd;
+ var configPath = opts.configPath;
+ // if a path to the desired config was specified
+ // but no cwd was provided, use configPath dir
+ if (typeof configPath === 'string' && !cwd) {
+ cwd = path.dirname(path.resolve(configPath));
+ }
+ if (typeof cwd === 'string') {
+ return path.resolve(cwd);
+ }
+ return process.cwd();
+};
diff --git a/node_modules/liftoff/lib/get_node_flags.js b/node_modules/liftoff/lib/get_node_flags.js
new file mode 100644
index 0000000..c58165e
--- /dev/null
+++ b/node_modules/liftoff/lib/get_node_flags.js
@@ -0,0 +1,30 @@
+function arrayOrFunction(arrayOrFunc, env) {
+ if (typeof arrayOrFunc === 'function') {
+ return arrayOrFunc.call(this, env);
+ }
+ if (Array.isArray(arrayOrFunc)) {
+ return arrayOrFunc;
+ }
+ if (typeof arrayOrFunc === 'string') {
+ return [arrayOrFunc];
+ }
+ return [];
+}
+
+function fromReorderedArgv(reorderedArgv) {
+ var nodeFlags = [];
+ for (var i = 1, n = reorderedArgv.length; i < n; i++) {
+ var arg = reorderedArgv[i];
+ if (!/^-/.test(arg) || arg === '--') {
+ break;
+ }
+ nodeFlags.push(arg);
+ }
+ return nodeFlags;
+}
+
+module.exports = {
+ arrayOrFunction: arrayOrFunction,
+ fromReorderedArgv: fromReorderedArgv,
+};
+
diff --git a/node_modules/liftoff/lib/parse_options.js b/node_modules/liftoff/lib/parse_options.js
new file mode 100644
index 0000000..ab416b5
--- /dev/null
+++ b/node_modules/liftoff/lib/parse_options.js
@@ -0,0 +1,35 @@
+const extend = require('extend');
+
+module.exports = function (opts) {
+ var defaults = {
+ extensions: {
+ '.js': null,
+ '.json': null
+ },
+ searchPaths: []
+ };
+ if (!opts) {
+ opts = {};
+ }
+ if (opts.name) {
+ if (!opts.processTitle) {
+ opts.processTitle = opts.name;
+ }
+ if (!opts.configName) {
+ opts.configName = opts.name + 'file';
+ }
+ if (!opts.moduleName) {
+ opts.moduleName = opts.name;
+ }
+ }
+ if (!opts.processTitle) {
+ throw new Error('You must specify a processTitle.');
+ }
+ if (!opts.configName) {
+ throw new Error('You must specify a configName.');
+ }
+ if (!opts.moduleName) {
+ throw new Error('You must specify a moduleName.');
+ }
+ return extend(defaults, opts);
+};
diff --git a/node_modules/liftoff/lib/register_loader.js b/node_modules/liftoff/lib/register_loader.js
new file mode 100644
index 0000000..e29b08a
--- /dev/null
+++ b/node_modules/liftoff/lib/register_loader.js
@@ -0,0 +1,24 @@
+const rechoir = require('rechoir');
+
+module.exports = function(eventEmitter, extensions, configPath, cwd) {
+ extensions = extensions || {};
+
+ if (typeof configPath !== 'string') {
+ return;
+ }
+
+ var autoloads = rechoir.prepare(extensions, configPath, cwd, true);
+ if (autoloads instanceof Error) {
+ autoloads = autoloads.failures;
+ }
+
+ if (Array.isArray(autoloads)) {
+ autoloads.forEach(function (attempt) {
+ if (attempt.error) {
+ eventEmitter.emit('requireFail', attempt.moduleName, attempt.error);
+ } else {
+ eventEmitter.emit('require', attempt.moduleName, attempt.module);
+ }
+ });
+ }
+};
diff --git a/node_modules/liftoff/lib/silent_require.js b/node_modules/liftoff/lib/silent_require.js
new file mode 100644
index 0000000..7b4dfe4
--- /dev/null
+++ b/node_modules/liftoff/lib/silent_require.js
@@ -0,0 +1,5 @@
+module.exports = function (path) {
+ try {
+ return require(path);
+ } catch (e) {}
+};
diff --git a/node_modules/liftoff/package.json b/node_modules/liftoff/package.json
new file mode 100644
index 0000000..b62cf22
--- /dev/null
+++ b/node_modules/liftoff/package.json
@@ -0,0 +1,79 @@
+{
+ "_from": "liftoff@^2.1.0",
+ "_id": "liftoff@2.5.0",
+ "_inBundle": false,
+ "_integrity": "sha1-IAkpG7Mc6oYbvxCnwVooyvdcMew=",
+ "_location": "/liftoff",
+ "_phantomChildren": {},
+ "_requested": {
+ "type": "range",
+ "registry": true,
+ "raw": "liftoff@^2.1.0",
+ "name": "liftoff",
+ "escapedName": "liftoff",
+ "rawSpec": "^2.1.0",
+ "saveSpec": null,
+ "fetchSpec": "^2.1.0"
+ },
+ "_requiredBy": [
+ "/gulp"
+ ],
+ "_resolved": "https://registry.npmjs.org/liftoff/-/liftoff-2.5.0.tgz",
+ "_shasum": "2009291bb31cea861bbf10a7c15a28caf75c31ec",
+ "_spec": "liftoff@^2.1.0",
+ "_where": "/Users/rpatra16/MyProjects/RamAndAntara/node_modules/gulp",
+ "author": {
+ "name": "Tyler Kellen",
+ "url": "http://goingslowly.com/"
+ },
+ "bugs": {
+ "url": "https://github.com/js-cli/js-liftoff/issues"
+ },
+ "bundleDependencies": false,
+ "contributors": [],
+ "dependencies": {
+ "extend": "^3.0.0",
+ "findup-sync": "^2.0.0",
+ "fined": "^1.0.1",
+ "flagged-respawn": "^1.0.0",
+ "is-plain-object": "^2.0.4",
+ "object.map": "^1.0.0",
+ "rechoir": "^0.6.2",
+ "resolve": "^1.1.7"
+ },
+ "deprecated": false,
+ "description": "Launch your command line tool with ease.",
+ "devDependencies": {
+ "chai": "^3.5.0",
+ "coffeescript": "^1.10.0",
+ "jscs": "^3.0.7",
+ "jshint": "^2.9.2",
+ "mocha": "^3.5.3",
+ "nyc": "^11.2.1",
+ "sinon": "~1.17.4"
+ },
+ "engines": {
+ "node": ">= 0.8"
+ },
+ "files": [
+ "index.js",
+ "lib",
+ "LICENSE"
+ ],
+ "homepage": "https://github.com/js-cli/js-liftoff#readme",
+ "keywords": [
+ "command line"
+ ],
+ "license": "MIT",
+ "main": "index.js",
+ "name": "liftoff",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/js-cli/js-liftoff.git"
+ },
+ "scripts": {
+ "cover": "nyc --reporter=lcov --reporter=text-summary npm test",
+ "test": "jshint lib index.js && jscs lib index.js && mocha -t 5000 -b -R spec test/index"
+ },
+ "version": "2.5.0"
+}
diff --git a/node_modules/load-json-file/index.js b/node_modules/load-json-file/index.js
new file mode 100644
index 0000000..96d4d9f
--- /dev/null
+++ b/node_modules/load-json-file/index.js
@@ -0,0 +1,21 @@
+'use strict';
+var path = require('path');
+var fs = require('graceful-fs');
+var stripBom = require('strip-bom');
+var parseJson = require('parse-json');
+var Promise = require('pinkie-promise');
+var pify = require('pify');
+
+function parse(x, fp) {
+ return parseJson(stripBom(x), path.relative(process.cwd(), fp));
+}
+
+module.exports = function (fp) {
+ return pify(fs.readFile, Promise)(fp, 'utf8').then(function (data) {
+ return parse(data, fp);
+ });
+};
+
+module.exports.sync = function (fp) {
+ return parse(fs.readFileSync(fp, 'utf8'), fp);
+};
diff --git a/node_modules/load-json-file/license b/node_modules/load-json-file/license
new file mode 100644
index 0000000..654d0bf
--- /dev/null
+++ b/node_modules/load-json-file/license
@@ -0,0 +1,21 @@
+The MIT License (MIT)
+
+Copyright (c) Sindre Sorhus (sindresorhus.com)
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in
+all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
+THE SOFTWARE.
diff --git a/node_modules/load-json-file/node_modules/graceful-fs/LICENSE b/node_modules/load-json-file/node_modules/graceful-fs/LICENSE
new file mode 100644
index 0000000..9d2c803
--- /dev/null
+++ b/node_modules/load-json-file/node_modules/graceful-fs/LICENSE
@@ -0,0 +1,15 @@
+The ISC License
+
+Copyright (c) Isaac Z. Schlueter, Ben Noordhuis, and Contributors
+
+Permission to use, copy, modify, and/or distribute this software for any
+purpose with or without fee is hereby granted, provided that the above
+copyright notice and this permission notice appear in all copies.
+
+THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES
+WITH REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF
+MERCHANTABILITY AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR
+ANY SPECIAL, DIRECT, INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES
+WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN
+ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT OF OR
+IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE.
diff --git a/node_modules/load-json-file/node_modules/graceful-fs/README.md b/node_modules/load-json-file/node_modules/graceful-fs/README.md
new file mode 100644
index 0000000..5273a50
--- /dev/null
+++ b/node_modules/load-json-file/node_modules/graceful-fs/README.md
@@ -0,0 +1,133 @@
+# graceful-fs
+
+graceful-fs functions as a drop-in replacement for the fs module,
+making various improvements.
+
+The improvements are meant to normalize behavior across different
+platforms and environments, and to make filesystem access more
+resilient to errors.
+
+## Improvements over [fs module](https://nodejs.org/api/fs.html)
+
+* Queues up `open` and `readdir` calls, and retries them once
+ something closes if there is an EMFILE error from too many file
+ descriptors.
+* fixes `lchmod` for Node versions prior to 0.6.2.
+* implements `fs.lutimes` if possible. Otherwise it becomes a noop.
+* ignores `EINVAL` and `EPERM` errors in `chown`, `fchown` or
+ `lchown` if the user isn't root.
+* makes `lchmod` and `lchown` become noops, if not available.
+* retries reading a file if `read` results in EAGAIN error.
+
+On Windows, it retries renaming a file for up to one second if `EACCESS`
+or `EPERM` error occurs, likely because antivirus software has locked
+the directory.
+
+## USAGE
+
+```javascript
+// use just like fs
+var fs = require('graceful-fs')
+
+// now go and do stuff with it...
+fs.readFileSync('some-file-or-whatever')
+```
+
+## Global Patching
+
+If you want to patch the global fs module (or any other fs-like
+module) you can do this:
+
+```javascript
+// Make sure to read the caveat below.
+var realFs = require('fs')
+var gracefulFs = require('graceful-fs')
+gracefulFs.gracefulify(realFs)
+```
+
+This should only ever be done at the top-level application layer, in
+order to delay on EMFILE errors from any fs-using dependencies. You
+should **not** do this in a library, because it can cause unexpected
+delays in other parts of the program.
+
+## Changes
+
+This module is fairly stable at this point, and used by a lot of
+things. That being said, because it implements a subtle behavior
+change in a core part of the node API, even modest changes can be
+extremely breaking, and the versioning is thus biased towards
+bumping the major when in doubt.
+
+The main change between major versions has been switching between
+providing a fully-patched `fs` module vs monkey-patching the node core
+builtin, and the approach by which a non-monkey-patched `fs` was
+created.
+
+The goal is to trade `EMFILE` errors for slower fs operations. So, if
+you try to open a zillion files, rather than crashing, `open`
+operations will be queued up and wait for something else to `close`.
+
+There are advantages to each approach. Monkey-patching the fs means
+that no `EMFILE` errors can possibly occur anywhere in your
+application, because everything is using the same core `fs` module,
+which is patched. However, it can also obviously cause undesirable
+side-effects, especially if the module is loaded multiple times.
+
+Implementing a separate-but-identical patched `fs` module is more
+surgical (and doesn't run the risk of patching multiple times), but
+also imposes the challenge of keeping in sync with the core module.
+
+The current approach loads the `fs` module, and then creates a
+lookalike object that has all the same methods, except a few that are
+patched. It is safe to use in all versions of Node from 0.8 through
+7.0.
+
+### v4
+
+* Do not monkey-patch the fs module. This module may now be used as a
+ drop-in dep, and users can opt into monkey-patching the fs builtin
+ if their app requires it.
+
+### v3
+
+* Monkey-patch fs, because the eval approach no longer works on recent
+ node.
+* fixed possible type-error throw if rename fails on windows
+* verify that we *never* get EMFILE errors
+* Ignore ENOSYS from chmod/chown
+* clarify that graceful-fs must be used as a drop-in
+
+### v2.1.0
+
+* Use eval rather than monkey-patching fs.
+* readdir: Always sort the results
+* win32: requeue a file if error has an OK status
+
+### v2.0
+
+* A return to monkey patching
+* wrap process.cwd
+
+### v1.1
+
+* wrap readFile
+* Wrap fs.writeFile.
+* readdir protection
+* Don't clobber the fs builtin
+* Handle fs.read EAGAIN errors by trying again
+* Expose the curOpen counter
+* No-op lchown/lchmod if not implemented
+* fs.rename patch only for win32
+* Patch fs.rename to handle AV software on Windows
+* Close #4 Chown should not fail on einval or eperm if non-root
+* Fix isaacs/fstream#1 Only wrap fs one time
+* Fix #3 Start at 1024 max files, then back off on EMFILE
+* lutimes that doens't blow up on Linux
+* A full on-rewrite using a queue instead of just swallowing the EMFILE error
+* Wrap Read/Write streams as well
+
+### 1.0
+
+* Update engines for node 0.6
+* Be lstat-graceful on Windows
+* first
diff --git a/node_modules/load-json-file/node_modules/graceful-fs/clone.js b/node_modules/load-json-file/node_modules/graceful-fs/clone.js
new file mode 100644
index 0000000..028356c
--- /dev/null
+++ b/node_modules/load-json-file/node_modules/graceful-fs/clone.js
@@ -0,0 +1,19 @@
+'use strict'
+
+module.exports = clone
+
+function clone (obj) {
+ if (obj === null || typeof obj !== 'object')
+ return obj
+
+ if (obj instanceof Object)
+ var copy = { __proto__: obj.__proto__ }
+ else
+ var copy = Object.create(null)
+
+ Object.getOwnPropertyNames(obj).forEach(function (key) {
+ Object.defineProperty(copy, key, Object.getOwnPropertyDescriptor(obj, key))
+ })
+
+ return copy
+}
diff --git a/node_modules/load-json-file/node_modules/graceful-fs/graceful-fs.js b/node_modules/load-json-file/node_modules/graceful-fs/graceful-fs.js
new file mode 100644
index 0000000..ac20675
--- /dev/null
+++ b/node_modules/load-json-file/node_modules/graceful-fs/graceful-fs.js
@@ -0,0 +1,279 @@
+var fs = require('fs')
+var polyfills = require('./polyfills.js')
+var legacy = require('./legacy-streams.js')
+var clone = require('./clone.js')
+
+var queue = []
+
+var util = require('util')
+
+function noop () {}
+
+var debug = noop
+if (util.debuglog)
+ debug = util.debuglog('gfs4')
+else if (/\bgfs4\b/i.test(process.env.NODE_DEBUG || ''))
+ debug = function() {
+ var m = util.format.apply(util, arguments)
+ m = 'GFS4: ' + m.split(/\n/).join('\nGFS4: ')
+ console.error(m)
+ }
+
+if (/\bgfs4\b/i.test(process.env.NODE_DEBUG || '')) {
+ process.on('exit', function() {
+ debug(queue)
+ require('assert').equal(queue.length, 0)
+ })
+}
+
+module.exports = patch(clone(fs))
+if (process.env.TEST_GRACEFUL_FS_GLOBAL_PATCH && !fs.__patched) {
+ module.exports = patch(fs)
+ fs.__patched = true;
+}
+
+// Always patch fs.close/closeSync, because we want to
+// retry() whenever a close happens *anywhere* in the program.
+// This is essential when multiple graceful-fs instances are
+// in play at the same time.
+module.exports.close = (function (fs$close) { return function (fd, cb) {
+ return fs$close.call(fs, fd, function (err) {
+ if (!err)
+ retry()
+
+ if (typeof cb === 'function')
+ cb.apply(this, arguments)
+ })
+}})(fs.close)
+
+module.exports.closeSync = (function (fs$closeSync) { return function (fd) {
+ // Note that graceful-fs also retries when fs.closeSync() fails.
+ // Looks like a bug to me, although it's probably a harmless one.
+ var rval = fs$closeSync.apply(fs, arguments)
+ retry()
+ return rval
+}})(fs.closeSync)
+
+// Only patch fs once, otherwise we'll run into a memory leak if
+// graceful-fs is loaded multiple times, such as in test environments that
+// reset the loaded modules between tests.
+// We look for the string `graceful-fs` from the comment above. This
+// way we are not adding any extra properties and it will detect if older
+// versions of graceful-fs are installed.
+if (!/\bgraceful-fs\b/.test(fs.closeSync.toString())) {
+ fs.closeSync = module.exports.closeSync;
+ fs.close = module.exports.close;
+}
+
+function patch (fs) {
+ // Everything that references the open() function needs to be in here
+ polyfills(fs)
+ fs.gracefulify = patch
+ fs.FileReadStream = ReadStream; // Legacy name.
+ fs.FileWriteStream = WriteStream; // Legacy name.
+ fs.createReadStream = createReadStream
+ fs.createWriteStream = createWriteStream
+ var fs$readFile = fs.readFile
+ fs.readFile = readFile
+ function readFile (path, options, cb) {
+ if (typeof options === 'function')
+ cb = options, options = null
+
+ return go$readFile(path, options, cb)
+
+ function go$readFile (path, options, cb) {
+ return fs$readFile(path, options, function (err) {
+ if (err && (err.code === 'EMFILE' || err.code === 'ENFILE'))
+ enqueue([go$readFile, [path, options, cb]])
+ else {
+ if (typeof cb === 'function')
+ cb.apply(this, arguments)
+ retry()
+ }
+ })
+ }
+ }
+
+ var fs$writeFile = fs.writeFile
+ fs.writeFile = writeFile
+ function writeFile (path, data, options, cb) {
+ if (typeof options === 'function')
+ cb = options, options = null
+
+ return go$writeFile(path, data, options, cb)
+
+ function go$writeFile (path, data, options, cb) {
+ return fs$writeFile(path, data, options, function (err) {
+ if (err && (err.code === 'EMFILE' || err.code === 'ENFILE'))
+ enqueue([go$writeFile, [path, data, options, cb]])
+ else {
+ if (typeof cb === 'function')
+ cb.apply(this, arguments)
+ retry()
+ }
+ })
+ }
+ }
+
+ var fs$appendFile = fs.appendFile
+ if (fs$appendFile)
+ fs.appendFile = appendFile
+ function appendFile (path, data, options, cb) {
+ if (typeof options === 'function')
+ cb = options, options = null
+
+ return go$appendFile(path, data, options, cb)
+
+ function go$appendFile (path, data, options, cb) {
+ return fs$appendFile(path, data, options, function (err) {
+ if (err && (err.code === 'EMFILE' || err.code === 'ENFILE'))
+ enqueue([go$appendFile, [path, data, options, cb]])
+ else {
+ if (typeof cb === 'function')
+ cb.apply(this, arguments)
+ retry()
+ }
+ })
+ }
+ }
+
+ var fs$readdir = fs.readdir
+ fs.readdir = readdir
+ function readdir (path, options, cb) {
+ var args = [path]
+ if (typeof options !== 'function') {
+ args.push(options)
+ } else {
+ cb = options
+ }
+ args.push(go$readdir$cb)
+
+ return go$readdir(args)
+
+ function go$readdir$cb (err, files) {
+ if (files && files.sort)
+ files.sort()
+
+ if (err && (err.code === 'EMFILE' || err.code === 'ENFILE'))
+ enqueue([go$readdir, [args]])
+
+ else {
+ if (typeof cb === 'function')
+ cb.apply(this, arguments)
+ retry()
+ }
+ }
+ }
+
+ function go$readdir (args) {
+ return fs$readdir.apply(fs, args)
+ }
+
+ if (process.version.substr(0, 4) === 'v0.8') {
+ var legStreams = legacy(fs)
+ ReadStream = legStreams.ReadStream
+ WriteStream = legStreams.WriteStream
+ }
+
+ var fs$ReadStream = fs.ReadStream
+ if (fs$ReadStream) {
+ ReadStream.prototype = Object.create(fs$ReadStream.prototype)
+ ReadStream.prototype.open = ReadStream$open
+ }
+
+ var fs$WriteStream = fs.WriteStream
+ if (fs$WriteStream) {
+ WriteStream.prototype = Object.create(fs$WriteStream.prototype)
+ WriteStream.prototype.open = WriteStream$open
+ }
+
+ fs.ReadStream = ReadStream
+ fs.WriteStream = WriteStream
+
+ function ReadStream (path, options) {
+ if (this instanceof ReadStream)
+ return fs$ReadStream.apply(this, arguments), this
+ else
+ return ReadStream.apply(Object.create(ReadStream.prototype), arguments)
+ }
+
+ function ReadStream$open () {
+ var that = this
+ open(that.path, that.flags, that.mode, function (err, fd) {
+ if (err) {
+ if (that.autoClose)
+ that.destroy()
+
+ that.emit('error', err)
+ } else {
+ that.fd = fd
+ that.emit('open', fd)
+ that.read()
+ }
+ })
+ }
+
+ function WriteStream (path, options) {
+ if (this instanceof WriteStream)
+ return fs$WriteStream.apply(this, arguments), this
+ else
+ return WriteStream.apply(Object.create(WriteStream.prototype), arguments)
+ }
+
+ function WriteStream$open () {
+ var that = this
+ open(that.path, that.flags, that.mode, function (err, fd) {
+ if (err) {
+ that.destroy()
+ that.emit('error', err)
+ } else {
+ that.fd = fd
+ that.emit('open', fd)
+ }
+ })
+ }
+
+ function createReadStream (path, options) {
+ return new ReadStream(path, options)
+ }
+
+ function createWriteStream (path, options) {
+ return new WriteStream(path, options)
+ }
+
+ var fs$open = fs.open
+ fs.open = open
+ function open (path, flags, mode, cb) {
+ if (typeof mode === 'function')
+ cb = mode, mode = null
+
+ return go$open(path, flags, mode, cb)
+
+ function go$open (path, flags, mode, cb) {
+ return fs$open(path, flags, mode, function (err, fd) {
+ if (err && (err.code === 'EMFILE' || err.code === 'ENFILE'))
+ enqueue([go$open, [path, flags, mode, cb]])
+ else {
+ if (typeof cb === 'function')
+ cb.apply(this, arguments)
+ retry()
+ }
+ })
+ }
+ }
+
+ return fs
+}
+
+function enqueue (elem) {
+ debug('ENQUEUE', elem[0].name, elem[1])
+ queue.push(elem)
+}
+
+function retry () {
+ var elem = queue.shift()
+ if (elem) {
+ debug('RETRY', elem[0].name, elem[1])
+ elem[0].apply(null, elem[1])
+ }
+}
diff --git a/node_modules/load-json-file/node_modules/graceful-fs/legacy-streams.js b/node_modules/load-json-file/node_modules/graceful-fs/legacy-streams.js
new file mode 100644
index 0000000..d617b50
--- /dev/null
+++ b/node_modules/load-json-file/node_modules/graceful-fs/legacy-streams.js
@@ -0,0 +1,118 @@
+var Stream = require('stream').Stream
+
+module.exports = legacy
+
+function legacy (fs) {
+ return {
+ ReadStream: ReadStream,
+ WriteStream: WriteStream
+ }
+
+ function ReadStream (path, options) {
+ if (!(this instanceof ReadStream)) return new ReadStream(path, options);
+
+ Stream.call(this);
+
+ var self = this;
+
+ this.path = path;
+ this.fd = null;
+ this.readable = true;
+ this.paused = false;
+
+ this.flags = 'r';
+ this.mode = 438; /*=0666*/
+ this.bufferSize = 64 * 1024;
+
+ options = options || {};
+
+ // Mixin options into this
+ var keys = Object.keys(options);
+ for (var index = 0, length = keys.length; index < length; index++) {
+ var key = keys[index];
+ this[key] = options[key];
+ }
+
+ if (this.encoding) this.setEncoding(this.encoding);
+
+ if (this.start !== undefined) {
+ if ('number' !== typeof this.start) {
+ throw TypeError('start must be a Number');
+ }
+ if (this.end === undefined) {
+ this.end = Infinity;
+ } else if ('number' !== typeof this.end) {
+ throw TypeError('end must be a Number');
+ }
+
+ if (this.start > this.end) {
+ throw new Error('start must be <= end');
+ }
+
+ this.pos = this.start;
+ }
+
+ if (this.fd !== null) {
+ process.nextTick(function() {
+ self._read();
+ });
+ return;
+ }
+
+ fs.open(this.path, this.flags, this.mode, function (err, fd) {
+ if (err) {
+ self.emit('error', err);
+ self.readable = false;
+ return;
+ }
+
+ self.fd = fd;
+ self.emit('open', fd);
+ self._read();
+ })
+ }
+
+ function WriteStream (path, options) {
+ if (!(this instanceof WriteStream)) return new WriteStream(path, options);
+
+ Stream.call(this);
+
+ this.path = path;
+ this.fd = null;
+ this.writable = true;
+
+ this.flags = 'w';
+ this.encoding = 'binary';
+ this.mode = 438; /*=0666*/
+ this.bytesWritten = 0;
+
+ options = options || {};
+
+ // Mixin options into this
+ var keys = Object.keys(options);
+ for (var index = 0, length = keys.length; index < length; index++) {
+ var key = keys[index];
+ this[key] = options[key];
+ }
+
+ if (this.start !== undefined) {
+ if ('number' !== typeof this.start) {
+ throw TypeError('start must be a Number');
+ }
+ if (this.start < 0) {
+ throw new Error('start must be >= zero');
+ }
+
+ this.pos = this.start;
+ }
+
+ this.busy = false;
+ this._queue = [];
+
+ if (this.fd === null) {
+ this._open = fs.open;
+ this._queue.push([this._open, this.path, this.flags, this.mode, undefined]);
+ this.flush();
+ }
+ }
+}
diff --git a/node_modules/load-json-file/node_modules/graceful-fs/package.json b/node_modules/load-json-file/node_modules/graceful-fs/package.json
new file mode 100644
index 0000000..8a13bbc
--- /dev/null
+++ b/node_modules/load-json-file/node_modules/graceful-fs/package.json
@@ -0,0 +1,78 @@
+{
+ "_from": "graceful-fs@^4.1.2",
+ "_id": "graceful-fs@4.1.15",
+ "_inBundle": false,
+ "_integrity": "sha512-6uHUhOPEBgQ24HM+r6b/QwWfZq+yiFcipKFrOFiBEnWdy5sdzYoi+pJeQaPI5qOLRFqWmAXUPQNsielzdLoecA==",
+ "_location": "/load-json-file/graceful-fs",
+ "_phantomChildren": {},
+ "_requested": {
+ "type": "range",
+ "registry": true,
+ "raw": "graceful-fs@^4.1.2",
+ "name": "graceful-fs",
+ "escapedName": "graceful-fs",
+ "rawSpec": "^4.1.2",
+ "saveSpec": null,
+ "fetchSpec": "^4.1.2"
+ },
+ "_requiredBy": [
+ "/load-json-file"
+ ],
+ "_resolved": "https://registry.npmjs.org/graceful-fs/-/graceful-fs-4.1.15.tgz",
+ "_shasum": "ffb703e1066e8a0eeaa4c8b80ba9253eeefbfb00",
+ "_spec": "graceful-fs@^4.1.2",
+ "_where": "/Users/rpatra16/MyProjects/RamAndAntara/node_modules/load-json-file",
+ "bugs": {
+ "url": "https://github.com/isaacs/node-graceful-fs/issues"
+ },
+ "bundleDependencies": false,
+ "deprecated": false,
+ "description": "A drop-in replacement for fs, making various improvements.",
+ "devDependencies": {
+ "import-fresh": "^2.0.0",
+ "mkdirp": "^0.5.0",
+ "rimraf": "^2.2.8",
+ "tap": "^12.0.1"
+ },
+ "directories": {
+ "test": "test"
+ },
+ "files": [
+ "fs.js",
+ "graceful-fs.js",
+ "legacy-streams.js",
+ "polyfills.js",
+ "clone.js"
+ ],
+ "homepage": "https://github.com/isaacs/node-graceful-fs#readme",
+ "keywords": [
+ "fs",
+ "module",
+ "reading",
+ "retry",
+ "retries",
+ "queue",
+ "error",
+ "errors",
+ "handling",
+ "EMFILE",
+ "EAGAIN",
+ "EINVAL",
+ "EPERM",
+ "EACCESS"
+ ],
+ "license": "ISC",
+ "main": "graceful-fs.js",
+ "name": "graceful-fs",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/isaacs/node-graceful-fs.git"
+ },
+ "scripts": {
+ "postpublish": "git push origin --all; git push origin --tags",
+ "postversion": "npm publish",
+ "preversion": "npm test",
+ "test": "node test.js | tap -"
+ },
+ "version": "4.1.15"
+}
diff --git a/node_modules/load-json-file/node_modules/graceful-fs/polyfills.js b/node_modules/load-json-file/node_modules/graceful-fs/polyfills.js
new file mode 100644
index 0000000..b964ed0
--- /dev/null
+++ b/node_modules/load-json-file/node_modules/graceful-fs/polyfills.js
@@ -0,0 +1,329 @@
+var constants = require('constants')
+
+var origCwd = process.cwd
+var cwd = null
+
+var platform = process.env.GRACEFUL_FS_PLATFORM || process.platform
+
+process.cwd = function() {
+ if (!cwd)
+ cwd = origCwd.call(process)
+ return cwd
+}
+try {
+ process.cwd()
+} catch (er) {}
+
+var chdir = process.chdir
+process.chdir = function(d) {
+ cwd = null
+ chdir.call(process, d)
+}
+
+module.exports = patch
+
+function patch (fs) {
+ // (re-)implement some things that are known busted or missing.
+
+ // lchmod, broken prior to 0.6.2
+ // back-port the fix here.
+ if (constants.hasOwnProperty('O_SYMLINK') &&
+ process.version.match(/^v0\.6\.[0-2]|^v0\.5\./)) {
+ patchLchmod(fs)
+ }
+
+ // lutimes implementation, or no-op
+ if (!fs.lutimes) {
+ patchLutimes(fs)
+ }
+
+ // https://github.com/isaacs/node-graceful-fs/issues/4
+ // Chown should not fail on einval or eperm if non-root.
+ // It should not fail on enosys ever, as this just indicates
+ // that a fs doesn't support the intended operation.
+
+ fs.chown = chownFix(fs.chown)
+ fs.fchown = chownFix(fs.fchown)
+ fs.lchown = chownFix(fs.lchown)
+
+ fs.chmod = chmodFix(fs.chmod)
+ fs.fchmod = chmodFix(fs.fchmod)
+ fs.lchmod = chmodFix(fs.lchmod)
+
+ fs.chownSync = chownFixSync(fs.chownSync)
+ fs.fchownSync = chownFixSync(fs.fchownSync)
+ fs.lchownSync = chownFixSync(fs.lchownSync)
+
+ fs.chmodSync = chmodFixSync(fs.chmodSync)
+ fs.fchmodSync = chmodFixSync(fs.fchmodSync)
+ fs.lchmodSync = chmodFixSync(fs.lchmodSync)
+
+ fs.stat = statFix(fs.stat)
+ fs.fstat = statFix(fs.fstat)
+ fs.lstat = statFix(fs.lstat)
+
+ fs.statSync = statFixSync(fs.statSync)
+ fs.fstatSync = statFixSync(fs.fstatSync)
+ fs.lstatSync = statFixSync(fs.lstatSync)
+
+ // if lchmod/lchown do not exist, then make them no-ops
+ if (!fs.lchmod) {
+ fs.lchmod = function (path, mode, cb) {
+ if (cb) process.nextTick(cb)
+ }
+ fs.lchmodSync = function () {}
+ }
+ if (!fs.lchown) {
+ fs.lchown = function (path, uid, gid, cb) {
+ if (cb) process.nextTick(cb)
+ }
+ fs.lchownSync = function () {}
+ }
+
+ // on Windows, A/V software can lock the directory, causing this
+ // to fail with an EACCES or EPERM if the directory contains newly
+ // created files. Try again on failure, for up to 60 seconds.
+
+ // Set the timeout this long because some Windows Anti-Virus, such as Parity
+ // bit9, may lock files for up to a minute, causing npm package install
+ // failures. Also, take care to yield the scheduler. Windows scheduling gives
+ // CPU to a busy looping process, which can cause the program causing the lock
+ // contention to be starved of CPU by node, so the contention doesn't resolve.
+ if (platform === "win32") {
+ fs.rename = (function (fs$rename) { return function (from, to, cb) {
+ var start = Date.now()
+ var backoff = 0;
+ fs$rename(from, to, function CB (er) {
+ if (er
+ && (er.code === "EACCES" || er.code === "EPERM")
+ && Date.now() - start < 60000) {
+ setTimeout(function() {
+ fs.stat(to, function (stater, st) {
+ if (stater && stater.code === "ENOENT")
+ fs$rename(from, to, CB);
+ else
+ cb(er)
+ })
+ }, backoff)
+ if (backoff < 100)
+ backoff += 10;
+ return;
+ }
+ if (cb) cb(er)
+ })
+ }})(fs.rename)
+ }
+
+ // if read() returns EAGAIN, then just try it again.
+ fs.read = (function (fs$read) { return function (fd, buffer, offset, length, position, callback_) {
+ var callback
+ if (callback_ && typeof callback_ === 'function') {
+ var eagCounter = 0
+ callback = function (er, _, __) {
+ if (er && er.code === 'EAGAIN' && eagCounter < 10) {
+ eagCounter ++
+ return fs$read.call(fs, fd, buffer, offset, length, position, callback)
+ }
+ callback_.apply(this, arguments)
+ }
+ }
+ return fs$read.call(fs, fd, buffer, offset, length, position, callback)
+ }})(fs.read)
+
+ fs.readSync = (function (fs$readSync) { return function (fd, buffer, offset, length, position) {
+ var eagCounter = 0
+ while (true) {
+ try {
+ return fs$readSync.call(fs, fd, buffer, offset, length, position)
+ } catch (er) {
+ if (er.code === 'EAGAIN' && eagCounter < 10) {
+ eagCounter ++
+ continue
+ }
+ throw er
+ }
+ }
+ }})(fs.readSync)
+
+ function patchLchmod (fs) {
+ fs.lchmod = function (path, mode, callback) {
+ fs.open( path
+ , constants.O_WRONLY | constants.O_SYMLINK
+ , mode
+ , function (err, fd) {
+ if (err) {
+ if (callback) callback(err)
+ return
+ }
+ // prefer to return the chmod error, if one occurs,
+ // but still try to close, and report closing errors if they occur.
+ fs.fchmod(fd, mode, function (err) {
+ fs.close(fd, function(err2) {
+ if (callback) callback(err || err2)
+ })
+ })
+ })
+ }
+
+ fs.lchmodSync = function (path, mode) {
+ var fd = fs.openSync(path, constants.O_WRONLY | constants.O_SYMLINK, mode)
+
+ // prefer to return the chmod error, if one occurs,
+ // but still try to close, and report closing errors if they occur.
+ var threw = true
+ var ret
+ try {
+ ret = fs.fchmodSync(fd, mode)
+ threw = false
+ } finally {
+ if (threw) {
+ try {
+ fs.closeSync(fd)
+ } catch (er) {}
+ } else {
+ fs.closeSync(fd)
+ }
+ }
+ return ret
+ }
+ }
+
+ function patchLutimes (fs) {
+ if (constants.hasOwnProperty("O_SYMLINK")) {
+ fs.lutimes = function (path, at, mt, cb) {
+ fs.open(path, constants.O_SYMLINK, function (er, fd) {
+ if (er) {
+ if (cb) cb(er)
+ return
+ }
+ fs.futimes(fd, at, mt, function (er) {
+ fs.close(fd, function (er2) {
+ if (cb) cb(er || er2)
+ })
+ })
+ })
+ }
+
+ fs.lutimesSync = function (path, at, mt) {
+ var fd = fs.openSync(path, constants.O_SYMLINK)
+ var ret
+ var threw = true
+ try {
+ ret = fs.futimesSync(fd, at, mt)
+ threw = false
+ } finally {
+ if (threw) {
+ try {
+ fs.closeSync(fd)
+ } catch (er) {}
+ } else {
+ fs.closeSync(fd)
+ }
+ }
+ return ret
+ }
+
+ } else {
+ fs.lutimes = function (_a, _b, _c, cb) { if (cb) process.nextTick(cb) }
+ fs.lutimesSync = function () {}
+ }
+ }
+
+ function chmodFix (orig) {
+ if (!orig) return orig
+ return function (target, mode, cb) {
+ return orig.call(fs, target, mode, function (er) {
+ if (chownErOk(er)) er = null
+ if (cb) cb.apply(this, arguments)
+ })
+ }
+ }
+
+ function chmodFixSync (orig) {
+ if (!orig) return orig
+ return function (target, mode) {
+ try {
+ return orig.call(fs, target, mode)
+ } catch (er) {
+ if (!chownErOk(er)) throw er
+ }
+ }
+ }
+
+
+ function chownFix (orig) {
+ if (!orig) return orig
+ return function (target, uid, gid, cb) {
+ return orig.call(fs, target, uid, gid, function (er) {
+ if (chownErOk(er)) er = null
+ if (cb) cb.apply(this, arguments)
+ })
+ }
+ }
+
+ function chownFixSync (orig) {
+ if (!orig) return orig
+ return function (target, uid, gid) {
+ try {
+ return orig.call(fs, target, uid, gid)
+ } catch (er) {
+ if (!chownErOk(er)) throw er
+ }
+ }
+ }
+
+
+ function statFix (orig) {
+ if (!orig) return orig
+ // Older versions of Node erroneously returned signed integers for
+ // uid + gid.
+ return function (target, cb) {
+ return orig.call(fs, target, function (er, stats) {
+ if (!stats) return cb.apply(this, arguments)
+ if (stats.uid < 0) stats.uid += 0x100000000
+ if (stats.gid < 0) stats.gid += 0x100000000
+ if (cb) cb.apply(this, arguments)
+ })
+ }
+ }
+
+ function statFixSync (orig) {
+ if (!orig) return orig
+ // Older versions of Node erroneously returned signed integers for
+ // uid + gid.
+ return function (target) {
+ var stats = orig.call(fs, target)
+ if (stats.uid < 0) stats.uid += 0x100000000
+ if (stats.gid < 0) stats.gid += 0x100000000
+ return stats;
+ }
+ }
+
+ // ENOSYS means that the fs doesn't support the op. Just ignore
+ // that, because it doesn't matter.
+ //
+ // if there's no getuid, or if getuid() is something other
+ // than 0, and the error is EINVAL or EPERM, then just ignore
+ // it.
+ //
+ // This specific case is a silent failure in cp, install, tar,
+ // and most other unix tools that manage permissions.
+ //
+ // When running as root, or if other types of errors are
+ // encountered, then it's strict.
+ function chownErOk (er) {
+ if (!er)
+ return true
+
+ if (er.code === "ENOSYS")
+ return true
+
+ var nonroot = !process.getuid || process.getuid() !== 0
+ if (nonroot) {
+ if (er.code === "EINVAL" || er.code === "EPERM")
+ return true
+ }
+
+ return false
+ }
+}
diff --git a/node_modules/load-json-file/node_modules/strip-bom/index.js b/node_modules/load-json-file/node_modules/strip-bom/index.js
new file mode 100644
index 0000000..5695c5c
--- /dev/null
+++ b/node_modules/load-json-file/node_modules/strip-bom/index.js
@@ -0,0 +1,17 @@
+'use strict';
+var isUtf8 = require('is-utf8');
+
+module.exports = function (x) {
+ // Catches EFBBBF (UTF-8 BOM) because the buffer-to-string
+ // conversion translates it to FEFF (UTF-16 BOM)
+ if (typeof x === 'string' && x.charCodeAt(0) === 0xFEFF) {
+ return x.slice(1);
+ }
+
+ if (Buffer.isBuffer(x) && isUtf8(x) &&
+ x[0] === 0xEF && x[1] === 0xBB && x[2] === 0xBF) {
+ return x.slice(3);
+ }
+
+ return x;
+};
diff --git a/node_modules/load-json-file/node_modules/strip-bom/license b/node_modules/load-json-file/node_modules/strip-bom/license
new file mode 100644
index 0000000..654d0bf
--- /dev/null
+++ b/node_modules/load-json-file/node_modules/strip-bom/license
@@ -0,0 +1,21 @@
+The MIT License (MIT)
+
+Copyright (c) Sindre Sorhus (sindresorhus.com)
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in
+all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
+THE SOFTWARE.
diff --git a/node_modules/load-json-file/node_modules/strip-bom/package.json b/node_modules/load-json-file/node_modules/strip-bom/package.json
new file mode 100644
index 0000000..e24e013
--- /dev/null
+++ b/node_modules/load-json-file/node_modules/strip-bom/package.json
@@ -0,0 +1,105 @@
+{
+ "_args": [
+ [
+ {
+ "raw": "strip-bom@^2.0.0",
+ "scope": null,
+ "escapedName": "strip-bom",
+ "name": "strip-bom",
+ "rawSpec": "^2.0.0",
+ "spec": ">=2.0.0 <3.0.0",
+ "type": "range"
+ },
+ "/Users/ramswaroop/MyProjects/RamAndAntara/node_modules/load-json-file"
+ ]
+ ],
+ "_from": "strip-bom@>=2.0.0 <3.0.0",
+ "_id": "strip-bom@2.0.0",
+ "_inCache": true,
+ "_location": "/load-json-file/strip-bom",
+ "_nodeVersion": "0.12.5",
+ "_npmUser": {
+ "name": "sindresorhus",
+ "email": "sindresorhus@gmail.com"
+ },
+ "_npmVersion": "2.11.2",
+ "_phantomChildren": {},
+ "_requested": {
+ "raw": "strip-bom@^2.0.0",
+ "scope": null,
+ "escapedName": "strip-bom",
+ "name": "strip-bom",
+ "rawSpec": "^2.0.0",
+ "spec": ">=2.0.0 <3.0.0",
+ "type": "range"
+ },
+ "_requiredBy": [
+ "/load-json-file"
+ ],
+ "_resolved": "https://registry.npmjs.org/strip-bom/-/strip-bom-2.0.0.tgz",
+ "_shasum": "6219a85616520491f35788bdbf1447a99c7e6b0e",
+ "_shrinkwrap": null,
+ "_spec": "strip-bom@^2.0.0",
+ "_where": "/Users/ramswaroop/MyProjects/RamAndAntara/node_modules/load-json-file",
+ "author": {
+ "name": "Sindre Sorhus",
+ "email": "sindresorhus@gmail.com",
+ "url": "sindresorhus.com"
+ },
+ "bugs": {
+ "url": "https://github.com/sindresorhus/strip-bom/issues"
+ },
+ "dependencies": {
+ "is-utf8": "^0.2.0"
+ },
+ "description": "Strip UTF-8 byte order mark (BOM) from a string/buffer",
+ "devDependencies": {
+ "mocha": "*"
+ },
+ "directories": {},
+ "dist": {
+ "shasum": "6219a85616520491f35788bdbf1447a99c7e6b0e",
+ "tarball": "https://registry.npmjs.org/strip-bom/-/strip-bom-2.0.0.tgz"
+ },
+ "engines": {
+ "node": ">=0.10.0"
+ },
+ "files": [
+ "index.js"
+ ],
+ "gitHead": "851b9c126dba9561cc14ef3dc2634dcc11df4d11",
+ "homepage": "https://github.com/sindresorhus/strip-bom",
+ "keywords": [
+ "bom",
+ "strip",
+ "byte",
+ "mark",
+ "unicode",
+ "utf8",
+ "utf-8",
+ "remove",
+ "delete",
+ "trim",
+ "text",
+ "buffer",
+ "string"
+ ],
+ "license": "MIT",
+ "maintainers": [
+ {
+ "name": "sindresorhus",
+ "email": "sindresorhus@gmail.com"
+ }
+ ],
+ "name": "strip-bom",
+ "optionalDependencies": {},
+ "readme": "ERROR: No README data found!",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/sindresorhus/strip-bom.git"
+ },
+ "scripts": {
+ "test": "mocha"
+ },
+ "version": "2.0.0"
+}
diff --git a/node_modules/load-json-file/node_modules/strip-bom/readme.md b/node_modules/load-json-file/node_modules/strip-bom/readme.md
new file mode 100644
index 0000000..8ecf258
--- /dev/null
+++ b/node_modules/load-json-file/node_modules/strip-bom/readme.md
@@ -0,0 +1,39 @@
+# strip-bom [](https://travis-ci.org/sindresorhus/strip-bom)
+
+> Strip UTF-8 [byte order mark](http://en.wikipedia.org/wiki/Byte_order_mark#UTF-8) (BOM) from a string/buffer
+
+From Wikipedia:
+
+> The Unicode Standard permits the BOM in UTF-8, but does not require nor recommend its use. Byte order has no meaning in UTF-8.
+
+
+## Install
+
+```
+$ npm install --save strip-bom
+```
+
+
+## Usage
+
+```js
+var fs = require('fs');
+var stripBom = require('strip-bom');
+
+stripBom('\uFEFFunicorn');
+//=> 'unicorn'
+
+stripBom(fs.readFileSync('unicorn.txt'));
+//=> 'unicorn'
+```
+
+
+## Related
+
+- [strip-bom-cli](https://github.com/sindresorhus/strip-bom-cli) - CLI for this module
+- [strip-bom-stream](https://github.com/sindresorhus/strip-bom-stream) - Stream version of this module
+
+
+## License
+
+MIT © [Sindre Sorhus](http://sindresorhus.com)
diff --git a/node_modules/load-json-file/package.json b/node_modules/load-json-file/package.json
new file mode 100644
index 0000000..2f6705a
--- /dev/null
+++ b/node_modules/load-json-file/package.json
@@ -0,0 +1,111 @@
+{
+ "_args": [
+ [
+ {
+ "raw": "load-json-file@^1.0.0",
+ "scope": null,
+ "escapedName": "load-json-file",
+ "name": "load-json-file",
+ "rawSpec": "^1.0.0",
+ "spec": ">=1.0.0 <2.0.0",
+ "type": "range"
+ },
+ "/Users/ramswaroop/MyProjects/RamAndAntara/node_modules/read-pkg"
+ ]
+ ],
+ "_from": "load-json-file@>=1.0.0 <2.0.0",
+ "_id": "load-json-file@1.1.0",
+ "_inCache": true,
+ "_location": "/load-json-file",
+ "_nodeVersion": "4.2.1",
+ "_npmUser": {
+ "name": "sindresorhus",
+ "email": "sindresorhus@gmail.com"
+ },
+ "_npmVersion": "2.14.7",
+ "_phantomChildren": {
+ "is-utf8": "0.2.1"
+ },
+ "_requested": {
+ "raw": "load-json-file@^1.0.0",
+ "scope": null,
+ "escapedName": "load-json-file",
+ "name": "load-json-file",
+ "rawSpec": "^1.0.0",
+ "spec": ">=1.0.0 <2.0.0",
+ "type": "range"
+ },
+ "_requiredBy": [
+ "/read-pkg"
+ ],
+ "_resolved": "https://registry.npmjs.org/load-json-file/-/load-json-file-1.1.0.tgz",
+ "_shasum": "956905708d58b4bab4c2261b04f59f31c99374c0",
+ "_shrinkwrap": null,
+ "_spec": "load-json-file@^1.0.0",
+ "_where": "/Users/ramswaroop/MyProjects/RamAndAntara/node_modules/read-pkg",
+ "author": {
+ "name": "Sindre Sorhus",
+ "email": "sindresorhus@gmail.com",
+ "url": "sindresorhus.com"
+ },
+ "bugs": {
+ "url": "https://github.com/sindresorhus/load-json-file/issues"
+ },
+ "dependencies": {
+ "graceful-fs": "^4.1.2",
+ "parse-json": "^2.2.0",
+ "pify": "^2.0.0",
+ "pinkie-promise": "^2.0.0",
+ "strip-bom": "^2.0.0"
+ },
+ "description": "Read and parse a JSON file",
+ "devDependencies": {
+ "ava": "*",
+ "xo": "*"
+ },
+ "directories": {},
+ "dist": {
+ "shasum": "956905708d58b4bab4c2261b04f59f31c99374c0",
+ "tarball": "https://registry.npmjs.org/load-json-file/-/load-json-file-1.1.0.tgz"
+ },
+ "engines": {
+ "node": ">=0.10.0"
+ },
+ "files": [
+ "index.js"
+ ],
+ "gitHead": "115157a417380d3160da418d4ff25bb33b0051eb",
+ "homepage": "https://github.com/sindresorhus/load-json-file",
+ "keywords": [
+ "json",
+ "read",
+ "parse",
+ "file",
+ "fs",
+ "graceful",
+ "load"
+ ],
+ "license": "MIT",
+ "maintainers": [
+ {
+ "name": "sindresorhus",
+ "email": "sindresorhus@gmail.com"
+ }
+ ],
+ "name": "load-json-file",
+ "optionalDependencies": {},
+ "readme": "ERROR: No README data found!",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/sindresorhus/load-json-file.git"
+ },
+ "scripts": {
+ "test": "xo && ava"
+ },
+ "version": "1.1.0",
+ "xo": {
+ "ignores": [
+ "test.js"
+ ]
+ }
+}
diff --git a/node_modules/load-json-file/readme.md b/node_modules/load-json-file/readme.md
new file mode 100644
index 0000000..fa982b5
--- /dev/null
+++ b/node_modules/load-json-file/readme.md
@@ -0,0 +1,45 @@
+# load-json-file [](https://travis-ci.org/sindresorhus/load-json-file)
+
+> Read and parse a JSON file
+
+[Strips UTF-8 BOM](https://github.com/sindresorhus/strip-bom), uses [`graceful-fs`](https://github.com/isaacs/node-graceful-fs), and throws more [helpful JSON errors](https://github.com/sindresorhus/parse-json).
+
+
+## Install
+
+```
+$ npm install --save load-json-file
+```
+
+
+## Usage
+
+```js
+const loadJsonFile = require('load-json-file');
+
+loadJsonFile('foo.json').then(json => {
+ console.log(json);
+ //=> {foo: true}
+});
+```
+
+
+## API
+
+### loadJsonFile(filepath)
+
+Returns a promise that resolves to the parsed JSON.
+
+### loadJsonFile.sync(filepath)
+
+Returns the parsed JSON.
+
+
+## Related
+
+- [write-json-file](https://github.com/sindresorhus/write-json-file) - Stringify and write JSON to a file atomically
+
+
+## License
+
+MIT © [Sindre Sorhus](http://sindresorhus.com)
diff --git a/node_modules/lodash._basecopy/LICENSE.txt b/node_modules/lodash._basecopy/LICENSE.txt
new file mode 100644
index 0000000..9cd87e5
--- /dev/null
+++ b/node_modules/lodash._basecopy/LICENSE.txt
@@ -0,0 +1,22 @@
+Copyright 2012-2015 The Dojo Foundation
+Based on Underscore.js, copyright 2009-2015 Jeremy Ashkenas,
+DocumentCloud and Investigative Reporters & Editors
+
+Permission is hereby granted, free of charge, to any person obtaining
+a copy of this software and associated documentation files (the
+"Software"), to deal in the Software without restriction, including
+without limitation the rights to use, copy, modify, merge, publish,
+distribute, sublicense, and/or sell copies of the Software, and to
+permit persons to whom the Software is furnished to do so, subject to
+the following conditions:
+
+The above copyright notice and this permission notice shall be
+included in all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
+EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
+MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
+NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
+LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
+OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
+WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
diff --git a/node_modules/lodash._basecopy/README.md b/node_modules/lodash._basecopy/README.md
new file mode 100644
index 0000000..acdfa29
--- /dev/null
+++ b/node_modules/lodash._basecopy/README.md
@@ -0,0 +1,20 @@
+# lodash._basecopy v3.0.1
+
+The [modern build](https://github.com/lodash/lodash/wiki/Build-Differences) of [lodash’s](https://lodash.com/) internal `baseCopy` exported as a [Node.js](http://nodejs.org/)/[io.js](https://iojs.org/) module.
+
+## Installation
+
+Using npm:
+
+```bash
+$ {sudo -H} npm i -g npm
+$ npm i --save lodash._basecopy
+```
+
+In Node.js/io.js:
+
+```js
+var baseCopy = require('lodash._basecopy');
+```
+
+See the [package source](https://github.com/lodash/lodash/blob/3.0.1-npm-packages/lodash._basecopy) for more details.
diff --git a/node_modules/lodash._basecopy/index.js b/node_modules/lodash._basecopy/index.js
new file mode 100644
index 0000000..b586d31
--- /dev/null
+++ b/node_modules/lodash._basecopy/index.js
@@ -0,0 +1,32 @@
+/**
+ * lodash 3.0.1 (Custom Build)
+ * Build: `lodash modern modularize exports="npm" -o ./`
+ * Copyright 2012-2015 The Dojo Foundation
+ * Based on Underscore.js 1.8.3
+ * Copyright 2009-2015 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license
+ */
+
+/**
+ * Copies properties of `source` to `object`.
+ *
+ * @private
+ * @param {Object} source The object to copy properties from.
+ * @param {Array} props The property names to copy.
+ * @param {Object} [object={}] The object to copy properties to.
+ * @returns {Object} Returns `object`.
+ */
+function baseCopy(source, props, object) {
+ object || (object = {});
+
+ var index = -1,
+ length = props.length;
+
+ while (++index < length) {
+ var key = props[index];
+ object[key] = source[key];
+ }
+ return object;
+}
+
+module.exports = baseCopy;
diff --git a/node_modules/lodash._basecopy/package.json b/node_modules/lodash._basecopy/package.json
new file mode 100644
index 0000000..53d724d
--- /dev/null
+++ b/node_modules/lodash._basecopy/package.json
@@ -0,0 +1,75 @@
+{
+ "_from": "lodash._basecopy@^3.0.0",
+ "_id": "lodash._basecopy@3.0.1",
+ "_inBundle": false,
+ "_integrity": "sha1-jaDmqHbPNEwK2KVIghEd08XHyjY=",
+ "_location": "/lodash._basecopy",
+ "_phantomChildren": {},
+ "_requested": {
+ "type": "range",
+ "registry": true,
+ "raw": "lodash._basecopy@^3.0.0",
+ "name": "lodash._basecopy",
+ "escapedName": "lodash._basecopy",
+ "rawSpec": "^3.0.0",
+ "saveSpec": null,
+ "fetchSpec": "^3.0.0"
+ },
+ "_requiredBy": [
+ "/lodash.template"
+ ],
+ "_resolved": "https://registry.npmjs.org/lodash._basecopy/-/lodash._basecopy-3.0.1.tgz",
+ "_shasum": "8da0e6a876cf344c0ad8a54882111dd3c5c7ca36",
+ "_spec": "lodash._basecopy@^3.0.0",
+ "_where": "/Users/rpatra16/MyProjects/RamAndAntara/node_modules/lodash.template",
+ "author": {
+ "name": "John-David Dalton",
+ "email": "john.david.dalton@gmail.com",
+ "url": "http://allyoucanleet.com/"
+ },
+ "bugs": {
+ "url": "https://github.com/lodash/lodash/issues"
+ },
+ "bundleDependencies": false,
+ "contributors": [
+ {
+ "name": "John-David Dalton",
+ "email": "john.david.dalton@gmail.com",
+ "url": "http://allyoucanleet.com/"
+ },
+ {
+ "name": "Benjamin Tan",
+ "email": "demoneaux@gmail.com",
+ "url": "https://d10.github.io/"
+ },
+ {
+ "name": "Blaine Bublitz",
+ "email": "blaine@iceddev.com",
+ "url": "http://www.iceddev.com/"
+ },
+ {
+ "name": "Kit Cambridge",
+ "email": "github@kitcambridge.be",
+ "url": "http://kitcambridge.be/"
+ },
+ {
+ "name": "Mathias Bynens",
+ "email": "mathias@qiwi.be",
+ "url": "https://mathiasbynens.be/"
+ }
+ ],
+ "deprecated": false,
+ "description": "The modern build of lodash’s internal `baseCopy` as a module.",
+ "homepage": "https://lodash.com/",
+ "icon": "https://lodash.com/icon.svg",
+ "license": "MIT",
+ "name": "lodash._basecopy",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/lodash/lodash.git"
+ },
+ "scripts": {
+ "test": "echo \"See https://travis-ci.org/lodash/lodash-cli for testing details.\""
+ },
+ "version": "3.0.1"
+}
diff --git a/node_modules/lodash._basetostring/LICENSE b/node_modules/lodash._basetostring/LICENSE
new file mode 100644
index 0000000..9cd87e5
--- /dev/null
+++ b/node_modules/lodash._basetostring/LICENSE
@@ -0,0 +1,22 @@
+Copyright 2012-2015 The Dojo Foundation
+Based on Underscore.js, copyright 2009-2015 Jeremy Ashkenas,
+DocumentCloud and Investigative Reporters & Editors
+
+Permission is hereby granted, free of charge, to any person obtaining
+a copy of this software and associated documentation files (the
+"Software"), to deal in the Software without restriction, including
+without limitation the rights to use, copy, modify, merge, publish,
+distribute, sublicense, and/or sell copies of the Software, and to
+permit persons to whom the Software is furnished to do so, subject to
+the following conditions:
+
+The above copyright notice and this permission notice shall be
+included in all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
+EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
+MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
+NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
+LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
+OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
+WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
diff --git a/node_modules/lodash._basetostring/README.md b/node_modules/lodash._basetostring/README.md
new file mode 100644
index 0000000..f81145e
--- /dev/null
+++ b/node_modules/lodash._basetostring/README.md
@@ -0,0 +1,20 @@
+# lodash._basetostring v3.0.1
+
+The [modern build](https://github.com/lodash/lodash/wiki/Build-Differences) of [lodash’s](https://lodash.com/) internal `baseToString` exported as a [Node.js](http://nodejs.org/)/[io.js](https://iojs.org/) module.
+
+## Installation
+
+Using npm:
+
+```bash
+$ {sudo -H} npm i -g npm
+$ npm i --save lodash._basetostring
+```
+
+In Node.js/io.js:
+
+```js
+var baseToString = require('lodash._basetostring');
+```
+
+See the [package source](https://github.com/lodash/lodash/blob/3.0.1-npm-packages/lodash._basetostring) for more details.
diff --git a/node_modules/lodash._basetostring/index.js b/node_modules/lodash._basetostring/index.js
new file mode 100644
index 0000000..db8ecc9
--- /dev/null
+++ b/node_modules/lodash._basetostring/index.js
@@ -0,0 +1,22 @@
+/**
+ * lodash 3.0.1 (Custom Build)
+ * Build: `lodash modern modularize exports="npm" -o ./`
+ * Copyright 2012-2015 The Dojo Foundation
+ * Based on Underscore.js 1.8.3
+ * Copyright 2009-2015 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license
+ */
+
+/**
+ * Converts `value` to a string if it's not one. An empty string is returned
+ * for `null` or `undefined` values.
+ *
+ * @private
+ * @param {*} value The value to process.
+ * @returns {string} Returns the string.
+ */
+function baseToString(value) {
+ return value == null ? '' : (value + '');
+}
+
+module.exports = baseToString;
diff --git a/node_modules/lodash._basetostring/package.json b/node_modules/lodash._basetostring/package.json
new file mode 100644
index 0000000..565df66
--- /dev/null
+++ b/node_modules/lodash._basetostring/package.json
@@ -0,0 +1,75 @@
+{
+ "_from": "lodash._basetostring@^3.0.0",
+ "_id": "lodash._basetostring@3.0.1",
+ "_inBundle": false,
+ "_integrity": "sha1-0YYdh3+CSlL2aYMtyvPuFVZqB9U=",
+ "_location": "/lodash._basetostring",
+ "_phantomChildren": {},
+ "_requested": {
+ "type": "range",
+ "registry": true,
+ "raw": "lodash._basetostring@^3.0.0",
+ "name": "lodash._basetostring",
+ "escapedName": "lodash._basetostring",
+ "rawSpec": "^3.0.0",
+ "saveSpec": null,
+ "fetchSpec": "^3.0.0"
+ },
+ "_requiredBy": [
+ "/lodash.template"
+ ],
+ "_resolved": "https://registry.npmjs.org/lodash._basetostring/-/lodash._basetostring-3.0.1.tgz",
+ "_shasum": "d1861d877f824a52f669832dcaf3ee15566a07d5",
+ "_spec": "lodash._basetostring@^3.0.0",
+ "_where": "/Users/rpatra16/MyProjects/RamAndAntara/node_modules/lodash.template",
+ "author": {
+ "name": "John-David Dalton",
+ "email": "john.david.dalton@gmail.com",
+ "url": "http://allyoucanleet.com/"
+ },
+ "bugs": {
+ "url": "https://github.com/lodash/lodash/issues"
+ },
+ "bundleDependencies": false,
+ "contributors": [
+ {
+ "name": "John-David Dalton",
+ "email": "john.david.dalton@gmail.com",
+ "url": "http://allyoucanleet.com/"
+ },
+ {
+ "name": "Benjamin Tan",
+ "email": "demoneaux@gmail.com",
+ "url": "https://d10.github.io/"
+ },
+ {
+ "name": "Blaine Bublitz",
+ "email": "blaine@iceddev.com",
+ "url": "http://www.iceddev.com/"
+ },
+ {
+ "name": "Kit Cambridge",
+ "email": "github@kitcambridge.be",
+ "url": "http://kitcambridge.be/"
+ },
+ {
+ "name": "Mathias Bynens",
+ "email": "mathias@qiwi.be",
+ "url": "https://mathiasbynens.be/"
+ }
+ ],
+ "deprecated": false,
+ "description": "The modern build of lodash’s internal `baseToString` as a module.",
+ "homepage": "https://lodash.com/",
+ "icon": "https://lodash.com/icon.svg",
+ "license": "MIT",
+ "name": "lodash._basetostring",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/lodash/lodash.git"
+ },
+ "scripts": {
+ "test": "echo \"See https://travis-ci.org/lodash/lodash-cli for testing details.\""
+ },
+ "version": "3.0.1"
+}
diff --git a/node_modules/lodash._basevalues/LICENSE.txt b/node_modules/lodash._basevalues/LICENSE.txt
new file mode 100644
index 0000000..1776432
--- /dev/null
+++ b/node_modules/lodash._basevalues/LICENSE.txt
@@ -0,0 +1,22 @@
+Copyright 2012-2015 The Dojo Foundation
+Based on Underscore.js 1.7.0, copyright 2009-2015 Jeremy Ashkenas,
+DocumentCloud and Investigative Reporters & Editors
+
+Permission is hereby granted, free of charge, to any person obtaining
+a copy of this software and associated documentation files (the
+"Software"), to deal in the Software without restriction, including
+without limitation the rights to use, copy, modify, merge, publish,
+distribute, sublicense, and/or sell copies of the Software, and to
+permit persons to whom the Software is furnished to do so, subject to
+the following conditions:
+
+The above copyright notice and this permission notice shall be
+included in all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
+EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
+MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
+NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
+LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
+OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
+WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
diff --git a/node_modules/lodash._basevalues/README.md b/node_modules/lodash._basevalues/README.md
new file mode 100644
index 0000000..206ba71
--- /dev/null
+++ b/node_modules/lodash._basevalues/README.md
@@ -0,0 +1,20 @@
+# lodash._basevalues v3.0.0
+
+The [modern build](https://github.com/lodash/lodash/wiki/Build-Differences) of [lodash’s](https://lodash.com/) internal `baseValues` exported as a [Node.js](http://nodejs.org/)/[io.js](https://iojs.org/) module.
+
+## Installation
+
+Using npm:
+
+```bash
+$ {sudo -H} npm i -g npm
+$ npm i --save lodash._basevalues
+```
+
+In Node.js/io.js:
+
+```js
+var baseValues = require('lodash._basevalues');
+```
+
+See the [package source](https://github.com/lodash/lodash/blob/3.0.0-npm-packages/lodash._basevalues) for more details.
diff --git a/node_modules/lodash._basevalues/index.js b/node_modules/lodash._basevalues/index.js
new file mode 100644
index 0000000..28c8215
--- /dev/null
+++ b/node_modules/lodash._basevalues/index.js
@@ -0,0 +1,31 @@
+/**
+ * lodash 3.0.0 (Custom Build)
+ * Build: `lodash modern modularize exports="npm" -o ./`
+ * Copyright 2012-2015 The Dojo Foundation
+ * Based on Underscore.js 1.7.0
+ * Copyright 2009-2015 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license
+ */
+
+/**
+ * The base implementation of `_.values` and `_.valuesIn` which creates an
+ * array of `object` property values corresponding to the property names
+ * returned by `keysFunc`.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @param {Array} props The property names to get values for.
+ * @returns {Object} Returns the array of property values.
+ */
+function baseValues(object, props) {
+ var index = -1,
+ length = props.length,
+ result = Array(length);
+
+ while (++index < length) {
+ result[index] = object[props[index]];
+ }
+ return result;
+}
+
+module.exports = baseValues;
diff --git a/node_modules/lodash._basevalues/package.json b/node_modules/lodash._basevalues/package.json
new file mode 100644
index 0000000..88596fe
--- /dev/null
+++ b/node_modules/lodash._basevalues/package.json
@@ -0,0 +1,75 @@
+{
+ "_from": "lodash._basevalues@^3.0.0",
+ "_id": "lodash._basevalues@3.0.0",
+ "_inBundle": false,
+ "_integrity": "sha1-W3dXYoAr3j0yl1A+JjAIIP32Ybc=",
+ "_location": "/lodash._basevalues",
+ "_phantomChildren": {},
+ "_requested": {
+ "type": "range",
+ "registry": true,
+ "raw": "lodash._basevalues@^3.0.0",
+ "name": "lodash._basevalues",
+ "escapedName": "lodash._basevalues",
+ "rawSpec": "^3.0.0",
+ "saveSpec": null,
+ "fetchSpec": "^3.0.0"
+ },
+ "_requiredBy": [
+ "/lodash.template"
+ ],
+ "_resolved": "https://registry.npmjs.org/lodash._basevalues/-/lodash._basevalues-3.0.0.tgz",
+ "_shasum": "5b775762802bde3d3297503e26300820fdf661b7",
+ "_spec": "lodash._basevalues@^3.0.0",
+ "_where": "/Users/rpatra16/MyProjects/RamAndAntara/node_modules/lodash.template",
+ "author": {
+ "name": "John-David Dalton",
+ "email": "john.david.dalton@gmail.com",
+ "url": "http://allyoucanleet.com/"
+ },
+ "bugs": {
+ "url": "https://github.com/lodash/lodash/issues"
+ },
+ "bundleDependencies": false,
+ "contributors": [
+ {
+ "name": "John-David Dalton",
+ "email": "john.david.dalton@gmail.com",
+ "url": "http://allyoucanleet.com/"
+ },
+ {
+ "name": "Benjamin Tan",
+ "email": "demoneaux@gmail.com",
+ "url": "https://d10.github.io/"
+ },
+ {
+ "name": "Blaine Bublitz",
+ "email": "blaine@iceddev.com",
+ "url": "http://www.iceddev.com/"
+ },
+ {
+ "name": "Kit Cambridge",
+ "email": "github@kitcambridge.be",
+ "url": "http://kitcambridge.be/"
+ },
+ {
+ "name": "Mathias Bynens",
+ "email": "mathias@qiwi.be",
+ "url": "https://mathiasbynens.be/"
+ }
+ ],
+ "deprecated": false,
+ "description": "The modern build of lodash’s internal `baseValues` as a module.",
+ "homepage": "https://lodash.com/",
+ "icon": "https://lodash.com/icon.svg",
+ "license": "MIT",
+ "name": "lodash._basevalues",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/lodash/lodash.git"
+ },
+ "scripts": {
+ "test": "echo \"See https://travis-ci.org/lodash/lodash-cli for testing details.\""
+ },
+ "version": "3.0.0"
+}
diff --git a/node_modules/lodash._getnative/LICENSE b/node_modules/lodash._getnative/LICENSE
new file mode 100644
index 0000000..9cd87e5
--- /dev/null
+++ b/node_modules/lodash._getnative/LICENSE
@@ -0,0 +1,22 @@
+Copyright 2012-2015 The Dojo Foundation
+Based on Underscore.js, copyright 2009-2015 Jeremy Ashkenas,
+DocumentCloud and Investigative Reporters & Editors
+
+Permission is hereby granted, free of charge, to any person obtaining
+a copy of this software and associated documentation files (the
+"Software"), to deal in the Software without restriction, including
+without limitation the rights to use, copy, modify, merge, publish,
+distribute, sublicense, and/or sell copies of the Software, and to
+permit persons to whom the Software is furnished to do so, subject to
+the following conditions:
+
+The above copyright notice and this permission notice shall be
+included in all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
+EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
+MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
+NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
+LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
+OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
+WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
diff --git a/node_modules/lodash._getnative/README.md b/node_modules/lodash._getnative/README.md
new file mode 100644
index 0000000..7835cec
--- /dev/null
+++ b/node_modules/lodash._getnative/README.md
@@ -0,0 +1,20 @@
+# lodash._getnative v3.9.1
+
+The [modern build](https://github.com/lodash/lodash/wiki/Build-Differences) of [lodash’s](https://lodash.com/) internal `getNative` exported as a [Node.js](http://nodejs.org/)/[io.js](https://iojs.org/) module.
+
+## Installation
+
+Using npm:
+
+```bash
+$ {sudo -H} npm i -g npm
+$ npm i --save lodash._getnative
+```
+
+In Node.js/io.js:
+
+```js
+var getNative = require('lodash._getnative');
+```
+
+See the [package source](https://github.com/lodash/lodash/blob/3.9.1-npm-packages/lodash._getnative) for more details.
diff --git a/node_modules/lodash._getnative/index.js b/node_modules/lodash._getnative/index.js
new file mode 100644
index 0000000..a32063d
--- /dev/null
+++ b/node_modules/lodash._getnative/index.js
@@ -0,0 +1,137 @@
+/**
+ * lodash 3.9.1 (Custom Build)
+ * Build: `lodash modern modularize exports="npm" -o ./`
+ * Copyright 2012-2015 The Dojo Foundation
+ * Based on Underscore.js 1.8.3
+ * Copyright 2009-2015 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license
+ */
+
+/** `Object#toString` result references. */
+var funcTag = '[object Function]';
+
+/** Used to detect host constructors (Safari > 5). */
+var reIsHostCtor = /^\[object .+?Constructor\]$/;
+
+/**
+ * Checks if `value` is object-like.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is object-like, else `false`.
+ */
+function isObjectLike(value) {
+ return !!value && typeof value == 'object';
+}
+
+/** Used for native method references. */
+var objectProto = Object.prototype;
+
+/** Used to resolve the decompiled source of functions. */
+var fnToString = Function.prototype.toString;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Used to resolve the [`toStringTag`](http://ecma-international.org/ecma-262/6.0/#sec-object.prototype.tostring)
+ * of values.
+ */
+var objToString = objectProto.toString;
+
+/** Used to detect if a method is native. */
+var reIsNative = RegExp('^' +
+ fnToString.call(hasOwnProperty).replace(/[\\^$.*+?()[\]{}|]/g, '\\$&')
+ .replace(/hasOwnProperty|(function).*?(?=\\\()| for .+?(?=\\\])/g, '$1.*?') + '$'
+);
+
+/**
+ * Gets the native function at `key` of `object`.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @param {string} key The key of the method to get.
+ * @returns {*} Returns the function if it's native, else `undefined`.
+ */
+function getNative(object, key) {
+ var value = object == null ? undefined : object[key];
+ return isNative(value) ? value : undefined;
+}
+
+/**
+ * Checks if `value` is classified as a `Function` object.
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is correctly classified, else `false`.
+ * @example
+ *
+ * _.isFunction(_);
+ * // => true
+ *
+ * _.isFunction(/abc/);
+ * // => false
+ */
+function isFunction(value) {
+ // The use of `Object#toString` avoids issues with the `typeof` operator
+ // in older versions of Chrome and Safari which return 'function' for regexes
+ // and Safari 8 equivalents which return 'object' for typed array constructors.
+ return isObject(value) && objToString.call(value) == funcTag;
+}
+
+/**
+ * Checks if `value` is the [language type](https://es5.github.io/#x8) of `Object`.
+ * (e.g. arrays, functions, objects, regexes, `new Number(0)`, and `new String('')`)
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is an object, else `false`.
+ * @example
+ *
+ * _.isObject({});
+ * // => true
+ *
+ * _.isObject([1, 2, 3]);
+ * // => true
+ *
+ * _.isObject(1);
+ * // => false
+ */
+function isObject(value) {
+ // Avoid a V8 JIT bug in Chrome 19-20.
+ // See https://code.google.com/p/v8/issues/detail?id=2291 for more details.
+ var type = typeof value;
+ return !!value && (type == 'object' || type == 'function');
+}
+
+/**
+ * Checks if `value` is a native function.
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a native function, else `false`.
+ * @example
+ *
+ * _.isNative(Array.prototype.push);
+ * // => true
+ *
+ * _.isNative(_);
+ * // => false
+ */
+function isNative(value) {
+ if (value == null) {
+ return false;
+ }
+ if (isFunction(value)) {
+ return reIsNative.test(fnToString.call(value));
+ }
+ return isObjectLike(value) && reIsHostCtor.test(value);
+}
+
+module.exports = getNative;
diff --git a/node_modules/lodash._getnative/package.json b/node_modules/lodash._getnative/package.json
new file mode 100644
index 0000000..6e00fe4
--- /dev/null
+++ b/node_modules/lodash._getnative/package.json
@@ -0,0 +1,75 @@
+{
+ "_from": "lodash._getnative@^3.0.0",
+ "_id": "lodash._getnative@3.9.1",
+ "_inBundle": false,
+ "_integrity": "sha1-VwvH3t5G1hzc3mh9ZdPuy6o6r/U=",
+ "_location": "/lodash._getnative",
+ "_phantomChildren": {},
+ "_requested": {
+ "type": "range",
+ "registry": true,
+ "raw": "lodash._getnative@^3.0.0",
+ "name": "lodash._getnative",
+ "escapedName": "lodash._getnative",
+ "rawSpec": "^3.0.0",
+ "saveSpec": null,
+ "fetchSpec": "^3.0.0"
+ },
+ "_requiredBy": [
+ "/lodash.keys"
+ ],
+ "_resolved": "https://registry.npmjs.org/lodash._getnative/-/lodash._getnative-3.9.1.tgz",
+ "_shasum": "570bc7dede46d61cdcde687d65d3eecbaa3aaff5",
+ "_spec": "lodash._getnative@^3.0.0",
+ "_where": "/Users/rpatra16/MyProjects/RamAndAntara/node_modules/lodash.keys",
+ "author": {
+ "name": "John-David Dalton",
+ "email": "john.david.dalton@gmail.com",
+ "url": "http://allyoucanleet.com/"
+ },
+ "bugs": {
+ "url": "https://github.com/lodash/lodash/issues"
+ },
+ "bundleDependencies": false,
+ "contributors": [
+ {
+ "name": "John-David Dalton",
+ "email": "john.david.dalton@gmail.com",
+ "url": "http://allyoucanleet.com/"
+ },
+ {
+ "name": "Benjamin Tan",
+ "email": "demoneaux@gmail.com",
+ "url": "https://d10.github.io/"
+ },
+ {
+ "name": "Blaine Bublitz",
+ "email": "blaine@iceddev.com",
+ "url": "http://www.iceddev.com/"
+ },
+ {
+ "name": "Kit Cambridge",
+ "email": "github@kitcambridge.be",
+ "url": "http://kitcambridge.be/"
+ },
+ {
+ "name": "Mathias Bynens",
+ "email": "mathias@qiwi.be",
+ "url": "https://mathiasbynens.be/"
+ }
+ ],
+ "deprecated": false,
+ "description": "The modern build of lodash’s internal `getNative` as a module.",
+ "homepage": "https://lodash.com/",
+ "icon": "https://lodash.com/icon.svg",
+ "license": "MIT",
+ "name": "lodash._getnative",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/lodash/lodash.git"
+ },
+ "scripts": {
+ "test": "echo \"See https://travis-ci.org/lodash/lodash-cli for testing details.\""
+ },
+ "version": "3.9.1"
+}
diff --git a/node_modules/lodash._isiterateecall/LICENSE.txt b/node_modules/lodash._isiterateecall/LICENSE.txt
new file mode 100644
index 0000000..9cd87e5
--- /dev/null
+++ b/node_modules/lodash._isiterateecall/LICENSE.txt
@@ -0,0 +1,22 @@
+Copyright 2012-2015 The Dojo Foundation
+Based on Underscore.js, copyright 2009-2015 Jeremy Ashkenas,
+DocumentCloud and Investigative Reporters & Editors
+
+Permission is hereby granted, free of charge, to any person obtaining
+a copy of this software and associated documentation files (the
+"Software"), to deal in the Software without restriction, including
+without limitation the rights to use, copy, modify, merge, publish,
+distribute, sublicense, and/or sell copies of the Software, and to
+permit persons to whom the Software is furnished to do so, subject to
+the following conditions:
+
+The above copyright notice and this permission notice shall be
+included in all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
+EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
+MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
+NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
+LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
+OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
+WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
diff --git a/node_modules/lodash._isiterateecall/README.md b/node_modules/lodash._isiterateecall/README.md
new file mode 100644
index 0000000..0c5c701
--- /dev/null
+++ b/node_modules/lodash._isiterateecall/README.md
@@ -0,0 +1,20 @@
+# lodash._isiterateecall v3.0.9
+
+The [modern build](https://github.com/lodash/lodash/wiki/Build-Differences) of [lodash’s](https://lodash.com/) internal `isIterateeCall` exported as a [Node.js](http://nodejs.org/)/[io.js](https://iojs.org/) module.
+
+## Installation
+
+Using npm:
+
+```bash
+$ {sudo -H} npm i -g npm
+$ npm i --save lodash._isiterateecall
+```
+
+In Node.js/io.js:
+
+```js
+var isIterateeCall = require('lodash._isiterateecall');
+```
+
+See the [package source](https://github.com/lodash/lodash/blob/3.0.9-npm-packages/lodash._isiterateecall) for more details.
diff --git a/node_modules/lodash._isiterateecall/index.js b/node_modules/lodash._isiterateecall/index.js
new file mode 100644
index 0000000..ea3761b
--- /dev/null
+++ b/node_modules/lodash._isiterateecall/index.js
@@ -0,0 +1,132 @@
+/**
+ * lodash 3.0.9 (Custom Build)
+ * Build: `lodash modern modularize exports="npm" -o ./`
+ * Copyright 2012-2015 The Dojo Foundation
+ * Based on Underscore.js 1.8.3
+ * Copyright 2009-2015 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license
+ */
+
+/** Used to detect unsigned integer values. */
+var reIsUint = /^\d+$/;
+
+/**
+ * Used as the [maximum length](https://people.mozilla.org/~jorendorff/es6-draft.html#sec-number.max_safe_integer)
+ * of an array-like value.
+ */
+var MAX_SAFE_INTEGER = 9007199254740991;
+
+/**
+ * The base implementation of `_.property` without support for deep paths.
+ *
+ * @private
+ * @param {string} key The key of the property to get.
+ * @returns {Function} Returns the new function.
+ */
+function baseProperty(key) {
+ return function(object) {
+ return object == null ? undefined : object[key];
+ };
+}
+
+/**
+ * Gets the "length" property value of `object`.
+ *
+ * **Note:** This function is used to avoid a [JIT bug](https://bugs.webkit.org/show_bug.cgi?id=142792)
+ * that affects Safari on at least iOS 8.1-8.3 ARM64.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @returns {*} Returns the "length" value.
+ */
+var getLength = baseProperty('length');
+
+/**
+ * Checks if `value` is array-like.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is array-like, else `false`.
+ */
+function isArrayLike(value) {
+ return value != null && isLength(getLength(value));
+}
+
+/**
+ * Checks if `value` is a valid array-like index.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @param {number} [length=MAX_SAFE_INTEGER] The upper bounds of a valid index.
+ * @returns {boolean} Returns `true` if `value` is a valid index, else `false`.
+ */
+function isIndex(value, length) {
+ value = (typeof value == 'number' || reIsUint.test(value)) ? +value : -1;
+ length = length == null ? MAX_SAFE_INTEGER : length;
+ return value > -1 && value % 1 == 0 && value < length;
+}
+
+/**
+ * Checks if the provided arguments are from an iteratee call.
+ *
+ * @private
+ * @param {*} value The potential iteratee value argument.
+ * @param {*} index The potential iteratee index or key argument.
+ * @param {*} object The potential iteratee object argument.
+ * @returns {boolean} Returns `true` if the arguments are from an iteratee call, else `false`.
+ */
+function isIterateeCall(value, index, object) {
+ if (!isObject(object)) {
+ return false;
+ }
+ var type = typeof index;
+ if (type == 'number'
+ ? (isArrayLike(object) && isIndex(index, object.length))
+ : (type == 'string' && index in object)) {
+ var other = object[index];
+ return value === value ? (value === other) : (other !== other);
+ }
+ return false;
+}
+
+/**
+ * Checks if `value` is a valid array-like length.
+ *
+ * **Note:** This function is based on [`ToLength`](https://people.mozilla.org/~jorendorff/es6-draft.html#sec-tolength).
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a valid length, else `false`.
+ */
+function isLength(value) {
+ return typeof value == 'number' && value > -1 && value % 1 == 0 && value <= MAX_SAFE_INTEGER;
+}
+
+/**
+ * Checks if `value` is the [language type](https://es5.github.io/#x8) of `Object`.
+ * (e.g. arrays, functions, objects, regexes, `new Number(0)`, and `new String('')`)
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is an object, else `false`.
+ * @example
+ *
+ * _.isObject({});
+ * // => true
+ *
+ * _.isObject([1, 2, 3]);
+ * // => true
+ *
+ * _.isObject(1);
+ * // => false
+ */
+function isObject(value) {
+ // Avoid a V8 JIT bug in Chrome 19-20.
+ // See https://code.google.com/p/v8/issues/detail?id=2291 for more details.
+ var type = typeof value;
+ return !!value && (type == 'object' || type == 'function');
+}
+
+module.exports = isIterateeCall;
diff --git a/node_modules/lodash._isiterateecall/package.json b/node_modules/lodash._isiterateecall/package.json
new file mode 100644
index 0000000..33caad6
--- /dev/null
+++ b/node_modules/lodash._isiterateecall/package.json
@@ -0,0 +1,75 @@
+{
+ "_from": "lodash._isiterateecall@^3.0.0",
+ "_id": "lodash._isiterateecall@3.0.9",
+ "_inBundle": false,
+ "_integrity": "sha1-UgOte6Ql+uhCRg5pbbnPPmqsBXw=",
+ "_location": "/lodash._isiterateecall",
+ "_phantomChildren": {},
+ "_requested": {
+ "type": "range",
+ "registry": true,
+ "raw": "lodash._isiterateecall@^3.0.0",
+ "name": "lodash._isiterateecall",
+ "escapedName": "lodash._isiterateecall",
+ "rawSpec": "^3.0.0",
+ "saveSpec": null,
+ "fetchSpec": "^3.0.0"
+ },
+ "_requiredBy": [
+ "/lodash.template"
+ ],
+ "_resolved": "https://registry.npmjs.org/lodash._isiterateecall/-/lodash._isiterateecall-3.0.9.tgz",
+ "_shasum": "5203ad7ba425fae842460e696db9cf3e6aac057c",
+ "_spec": "lodash._isiterateecall@^3.0.0",
+ "_where": "/Users/rpatra16/MyProjects/RamAndAntara/node_modules/lodash.template",
+ "author": {
+ "name": "John-David Dalton",
+ "email": "john.david.dalton@gmail.com",
+ "url": "http://allyoucanleet.com/"
+ },
+ "bugs": {
+ "url": "https://github.com/lodash/lodash/issues"
+ },
+ "bundleDependencies": false,
+ "contributors": [
+ {
+ "name": "John-David Dalton",
+ "email": "john.david.dalton@gmail.com",
+ "url": "http://allyoucanleet.com/"
+ },
+ {
+ "name": "Benjamin Tan",
+ "email": "demoneaux@gmail.com",
+ "url": "https://d10.github.io/"
+ },
+ {
+ "name": "Blaine Bublitz",
+ "email": "blaine@iceddev.com",
+ "url": "http://www.iceddev.com/"
+ },
+ {
+ "name": "Kit Cambridge",
+ "email": "github@kitcambridge.be",
+ "url": "http://kitcambridge.be/"
+ },
+ {
+ "name": "Mathias Bynens",
+ "email": "mathias@qiwi.be",
+ "url": "https://mathiasbynens.be/"
+ }
+ ],
+ "deprecated": false,
+ "description": "The modern build of lodash’s internal `isIterateeCall` as a module.",
+ "homepage": "https://lodash.com/",
+ "icon": "https://lodash.com/icon.svg",
+ "license": "MIT",
+ "name": "lodash._isiterateecall",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/lodash/lodash.git"
+ },
+ "scripts": {
+ "test": "echo \"See https://travis-ci.org/lodash/lodash-cli for testing details.\""
+ },
+ "version": "3.0.9"
+}
diff --git a/node_modules/lodash._reescape/LICENSE.txt b/node_modules/lodash._reescape/LICENSE.txt
new file mode 100644
index 0000000..1776432
--- /dev/null
+++ b/node_modules/lodash._reescape/LICENSE.txt
@@ -0,0 +1,22 @@
+Copyright 2012-2015 The Dojo Foundation
+Based on Underscore.js 1.7.0, copyright 2009-2015 Jeremy Ashkenas,
+DocumentCloud and Investigative Reporters & Editors
+
+Permission is hereby granted, free of charge, to any person obtaining
+a copy of this software and associated documentation files (the
+"Software"), to deal in the Software without restriction, including
+without limitation the rights to use, copy, modify, merge, publish,
+distribute, sublicense, and/or sell copies of the Software, and to
+permit persons to whom the Software is furnished to do so, subject to
+the following conditions:
+
+The above copyright notice and this permission notice shall be
+included in all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
+EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
+MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
+NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
+LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
+OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
+WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
diff --git a/node_modules/lodash._reescape/README.md b/node_modules/lodash._reescape/README.md
new file mode 100644
index 0000000..c80ae07
--- /dev/null
+++ b/node_modules/lodash._reescape/README.md
@@ -0,0 +1,20 @@
+# lodash._reescape v3.0.0
+
+The [modern build](https://github.com/lodash/lodash/wiki/Build-Differences) of [lodash’s](https://lodash.com/) internal `reEscape` exported as a [Node.js](http://nodejs.org/)/[io.js](https://iojs.org/) module.
+
+## Installation
+
+Using npm:
+
+```bash
+$ {sudo -H} npm i -g npm
+$ npm i --save lodash._reescape
+```
+
+In Node.js/io.js:
+
+```js
+var reEscape = require('lodash._reescape');
+```
+
+See the [package source](https://github.com/lodash/lodash/blob/3.0.0-npm-packages/lodash._reescape) for more details.
diff --git a/node_modules/lodash._reescape/index.js b/node_modules/lodash._reescape/index.js
new file mode 100644
index 0000000..1a3b8cf
--- /dev/null
+++ b/node_modules/lodash._reescape/index.js
@@ -0,0 +1,13 @@
+/**
+ * lodash 3.0.0 (Custom Build)
+ * Build: `lodash modern modularize exports="npm" -o ./`
+ * Copyright 2012-2015 The Dojo Foundation
+ * Based on Underscore.js 1.7.0
+ * Copyright 2009-2015 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license
+ */
+
+/** Used to match template delimiters. */
+var reEscape = /<%-([\s\S]+?)%>/g;
+
+module.exports = reEscape;
diff --git a/node_modules/lodash._reescape/package.json b/node_modules/lodash._reescape/package.json
new file mode 100644
index 0000000..62a6ff9
--- /dev/null
+++ b/node_modules/lodash._reescape/package.json
@@ -0,0 +1,75 @@
+{
+ "_from": "lodash._reescape@^3.0.0",
+ "_id": "lodash._reescape@3.0.0",
+ "_inBundle": false,
+ "_integrity": "sha1-Kx1vXf4HyKNVdT5fJ/rH8c3hYWo=",
+ "_location": "/lodash._reescape",
+ "_phantomChildren": {},
+ "_requested": {
+ "type": "range",
+ "registry": true,
+ "raw": "lodash._reescape@^3.0.0",
+ "name": "lodash._reescape",
+ "escapedName": "lodash._reescape",
+ "rawSpec": "^3.0.0",
+ "saveSpec": null,
+ "fetchSpec": "^3.0.0"
+ },
+ "_requiredBy": [
+ "/gulp-util"
+ ],
+ "_resolved": "https://registry.npmjs.org/lodash._reescape/-/lodash._reescape-3.0.0.tgz",
+ "_shasum": "2b1d6f5dfe07c8a355753e5f27fac7f1cde1616a",
+ "_spec": "lodash._reescape@^3.0.0",
+ "_where": "/Users/rpatra16/MyProjects/RamAndAntara/node_modules/gulp-util",
+ "author": {
+ "name": "John-David Dalton",
+ "email": "john.david.dalton@gmail.com",
+ "url": "http://allyoucanleet.com/"
+ },
+ "bugs": {
+ "url": "https://github.com/lodash/lodash/issues"
+ },
+ "bundleDependencies": false,
+ "contributors": [
+ {
+ "name": "John-David Dalton",
+ "email": "john.david.dalton@gmail.com",
+ "url": "http://allyoucanleet.com/"
+ },
+ {
+ "name": "Benjamin Tan",
+ "email": "demoneaux@gmail.com",
+ "url": "https://d10.github.io/"
+ },
+ {
+ "name": "Blaine Bublitz",
+ "email": "blaine@iceddev.com",
+ "url": "http://www.iceddev.com/"
+ },
+ {
+ "name": "Kit Cambridge",
+ "email": "github@kitcambridge.be",
+ "url": "http://kitcambridge.be/"
+ },
+ {
+ "name": "Mathias Bynens",
+ "email": "mathias@qiwi.be",
+ "url": "https://mathiasbynens.be/"
+ }
+ ],
+ "deprecated": false,
+ "description": "The modern build of lodash’s internal `reEscape` as a module.",
+ "homepage": "https://lodash.com/",
+ "icon": "https://lodash.com/icon.svg",
+ "license": "MIT",
+ "name": "lodash._reescape",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/lodash/lodash.git"
+ },
+ "scripts": {
+ "test": "echo \"See https://travis-ci.org/lodash/lodash-cli for testing details.\""
+ },
+ "version": "3.0.0"
+}
diff --git a/node_modules/lodash._reevaluate/LICENSE.txt b/node_modules/lodash._reevaluate/LICENSE.txt
new file mode 100644
index 0000000..1776432
--- /dev/null
+++ b/node_modules/lodash._reevaluate/LICENSE.txt
@@ -0,0 +1,22 @@
+Copyright 2012-2015 The Dojo Foundation
+Based on Underscore.js 1.7.0, copyright 2009-2015 Jeremy Ashkenas,
+DocumentCloud and Investigative Reporters & Editors
+
+Permission is hereby granted, free of charge, to any person obtaining
+a copy of this software and associated documentation files (the
+"Software"), to deal in the Software without restriction, including
+without limitation the rights to use, copy, modify, merge, publish,
+distribute, sublicense, and/or sell copies of the Software, and to
+permit persons to whom the Software is furnished to do so, subject to
+the following conditions:
+
+The above copyright notice and this permission notice shall be
+included in all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
+EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
+MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
+NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
+LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
+OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
+WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
diff --git a/node_modules/lodash._reevaluate/README.md b/node_modules/lodash._reevaluate/README.md
new file mode 100644
index 0000000..a69b8aa
--- /dev/null
+++ b/node_modules/lodash._reevaluate/README.md
@@ -0,0 +1,20 @@
+# lodash._reevaluate v3.0.0
+
+The [modern build](https://github.com/lodash/lodash/wiki/Build-Differences) of [lodash’s](https://lodash.com/) internal `reEvaluate` exported as a [Node.js](http://nodejs.org/)/[io.js](https://iojs.org/) module.
+
+## Installation
+
+Using npm:
+
+```bash
+$ {sudo -H} npm i -g npm
+$ npm i --save lodash._reevaluate
+```
+
+In Node.js/io.js:
+
+```js
+var reEvaluate = require('lodash._reevaluate');
+```
+
+See the [package source](https://github.com/lodash/lodash/blob/3.0.0-npm-packages/lodash._reevaluate) for more details.
diff --git a/node_modules/lodash._reevaluate/index.js b/node_modules/lodash._reevaluate/index.js
new file mode 100644
index 0000000..16d7609
--- /dev/null
+++ b/node_modules/lodash._reevaluate/index.js
@@ -0,0 +1,13 @@
+/**
+ * lodash 3.0.0 (Custom Build)
+ * Build: `lodash modern modularize exports="npm" -o ./`
+ * Copyright 2012-2015 The Dojo Foundation
+ * Based on Underscore.js 1.7.0
+ * Copyright 2009-2015 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license
+ */
+
+/** Used to match template delimiters. */
+var reEvaluate = /<%([\s\S]+?)%>/g;
+
+module.exports = reEvaluate;
diff --git a/node_modules/lodash._reevaluate/package.json b/node_modules/lodash._reevaluate/package.json
new file mode 100644
index 0000000..fda09d2
--- /dev/null
+++ b/node_modules/lodash._reevaluate/package.json
@@ -0,0 +1,75 @@
+{
+ "_from": "lodash._reevaluate@^3.0.0",
+ "_id": "lodash._reevaluate@3.0.0",
+ "_inBundle": false,
+ "_integrity": "sha1-WLx0xAZklTrgsSTYBpltrKQx4u0=",
+ "_location": "/lodash._reevaluate",
+ "_phantomChildren": {},
+ "_requested": {
+ "type": "range",
+ "registry": true,
+ "raw": "lodash._reevaluate@^3.0.0",
+ "name": "lodash._reevaluate",
+ "escapedName": "lodash._reevaluate",
+ "rawSpec": "^3.0.0",
+ "saveSpec": null,
+ "fetchSpec": "^3.0.0"
+ },
+ "_requiredBy": [
+ "/gulp-util"
+ ],
+ "_resolved": "https://registry.npmjs.org/lodash._reevaluate/-/lodash._reevaluate-3.0.0.tgz",
+ "_shasum": "58bc74c40664953ae0b124d806996daca431e2ed",
+ "_spec": "lodash._reevaluate@^3.0.0",
+ "_where": "/Users/rpatra16/MyProjects/RamAndAntara/node_modules/gulp-util",
+ "author": {
+ "name": "John-David Dalton",
+ "email": "john.david.dalton@gmail.com",
+ "url": "http://allyoucanleet.com/"
+ },
+ "bugs": {
+ "url": "https://github.com/lodash/lodash/issues"
+ },
+ "bundleDependencies": false,
+ "contributors": [
+ {
+ "name": "John-David Dalton",
+ "email": "john.david.dalton@gmail.com",
+ "url": "http://allyoucanleet.com/"
+ },
+ {
+ "name": "Benjamin Tan",
+ "email": "demoneaux@gmail.com",
+ "url": "https://d10.github.io/"
+ },
+ {
+ "name": "Blaine Bublitz",
+ "email": "blaine@iceddev.com",
+ "url": "http://www.iceddev.com/"
+ },
+ {
+ "name": "Kit Cambridge",
+ "email": "github@kitcambridge.be",
+ "url": "http://kitcambridge.be/"
+ },
+ {
+ "name": "Mathias Bynens",
+ "email": "mathias@qiwi.be",
+ "url": "https://mathiasbynens.be/"
+ }
+ ],
+ "deprecated": false,
+ "description": "The modern build of lodash’s internal `reEvaluate` as a module.",
+ "homepage": "https://lodash.com/",
+ "icon": "https://lodash.com/icon.svg",
+ "license": "MIT",
+ "name": "lodash._reevaluate",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/lodash/lodash.git"
+ },
+ "scripts": {
+ "test": "echo \"See https://travis-ci.org/lodash/lodash-cli for testing details.\""
+ },
+ "version": "3.0.0"
+}
diff --git a/node_modules/lodash._reinterpolate/LICENSE.txt b/node_modules/lodash._reinterpolate/LICENSE.txt
new file mode 100644
index 0000000..1776432
--- /dev/null
+++ b/node_modules/lodash._reinterpolate/LICENSE.txt
@@ -0,0 +1,22 @@
+Copyright 2012-2015 The Dojo Foundation
+Based on Underscore.js 1.7.0, copyright 2009-2015 Jeremy Ashkenas,
+DocumentCloud and Investigative Reporters & Editors
+
+Permission is hereby granted, free of charge, to any person obtaining
+a copy of this software and associated documentation files (the
+"Software"), to deal in the Software without restriction, including
+without limitation the rights to use, copy, modify, merge, publish,
+distribute, sublicense, and/or sell copies of the Software, and to
+permit persons to whom the Software is furnished to do so, subject to
+the following conditions:
+
+The above copyright notice and this permission notice shall be
+included in all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
+EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
+MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
+NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
+LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
+OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
+WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
diff --git a/node_modules/lodash._reinterpolate/README.md b/node_modules/lodash._reinterpolate/README.md
new file mode 100644
index 0000000..1423e50
--- /dev/null
+++ b/node_modules/lodash._reinterpolate/README.md
@@ -0,0 +1,20 @@
+# lodash._reinterpolate v3.0.0
+
+The [modern build](https://github.com/lodash/lodash/wiki/Build-Differences) of [lodash’s](https://lodash.com/) internal `reInterpolate` exported as a [Node.js](http://nodejs.org/)/[io.js](https://iojs.org/) module.
+
+## Installation
+
+Using npm:
+
+```bash
+$ {sudo -H} npm i -g npm
+$ npm i --save lodash._reinterpolate
+```
+
+In Node.js/io.js:
+
+```js
+var reInterpolate = require('lodash._reinterpolate');
+```
+
+See the [package source](https://github.com/lodash/lodash/blob/3.0.0-npm-packages/lodash._reinterpolate) for more details.
diff --git a/node_modules/lodash._reinterpolate/index.js b/node_modules/lodash._reinterpolate/index.js
new file mode 100644
index 0000000..5c06abc
--- /dev/null
+++ b/node_modules/lodash._reinterpolate/index.js
@@ -0,0 +1,13 @@
+/**
+ * lodash 3.0.0 (Custom Build)
+ * Build: `lodash modern modularize exports="npm" -o ./`
+ * Copyright 2012-2015 The Dojo Foundation
+ * Based on Underscore.js 1.7.0
+ * Copyright 2009-2015 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license
+ */
+
+/** Used to match template delimiters. */
+var reInterpolate = /<%=([\s\S]+?)%>/g;
+
+module.exports = reInterpolate;
diff --git a/node_modules/lodash._reinterpolate/package.json b/node_modules/lodash._reinterpolate/package.json
new file mode 100644
index 0000000..8f96f05
--- /dev/null
+++ b/node_modules/lodash._reinterpolate/package.json
@@ -0,0 +1,77 @@
+{
+ "_from": "lodash._reinterpolate@^3.0.0",
+ "_id": "lodash._reinterpolate@3.0.0",
+ "_inBundle": false,
+ "_integrity": "sha1-DM8tiRZq8Ds2Y8eWU4t1rG4RTZ0=",
+ "_location": "/lodash._reinterpolate",
+ "_phantomChildren": {},
+ "_requested": {
+ "type": "range",
+ "registry": true,
+ "raw": "lodash._reinterpolate@^3.0.0",
+ "name": "lodash._reinterpolate",
+ "escapedName": "lodash._reinterpolate",
+ "rawSpec": "^3.0.0",
+ "saveSpec": null,
+ "fetchSpec": "^3.0.0"
+ },
+ "_requiredBy": [
+ "/gulp-util",
+ "/lodash.template",
+ "/lodash.templatesettings"
+ ],
+ "_resolved": "https://registry.npmjs.org/lodash._reinterpolate/-/lodash._reinterpolate-3.0.0.tgz",
+ "_shasum": "0ccf2d89166af03b3663c796538b75ac6e114d9d",
+ "_spec": "lodash._reinterpolate@^3.0.0",
+ "_where": "/Users/rpatra16/MyProjects/RamAndAntara/node_modules/gulp-util",
+ "author": {
+ "name": "John-David Dalton",
+ "email": "john.david.dalton@gmail.com",
+ "url": "http://allyoucanleet.com/"
+ },
+ "bugs": {
+ "url": "https://github.com/lodash/lodash/issues"
+ },
+ "bundleDependencies": false,
+ "contributors": [
+ {
+ "name": "John-David Dalton",
+ "email": "john.david.dalton@gmail.com",
+ "url": "http://allyoucanleet.com/"
+ },
+ {
+ "name": "Benjamin Tan",
+ "email": "demoneaux@gmail.com",
+ "url": "https://d10.github.io/"
+ },
+ {
+ "name": "Blaine Bublitz",
+ "email": "blaine@iceddev.com",
+ "url": "http://www.iceddev.com/"
+ },
+ {
+ "name": "Kit Cambridge",
+ "email": "github@kitcambridge.be",
+ "url": "http://kitcambridge.be/"
+ },
+ {
+ "name": "Mathias Bynens",
+ "email": "mathias@qiwi.be",
+ "url": "https://mathiasbynens.be/"
+ }
+ ],
+ "deprecated": false,
+ "description": "The modern build of lodash’s internal `reInterpolate` as a module.",
+ "homepage": "https://lodash.com/",
+ "icon": "https://lodash.com/icon.svg",
+ "license": "MIT",
+ "name": "lodash._reinterpolate",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/lodash/lodash.git"
+ },
+ "scripts": {
+ "test": "echo \"See https://travis-ci.org/lodash/lodash-cli for testing details.\""
+ },
+ "version": "3.0.0"
+}
diff --git a/node_modules/lodash._root/LICENSE b/node_modules/lodash._root/LICENSE
new file mode 100644
index 0000000..bcbe13d
--- /dev/null
+++ b/node_modules/lodash._root/LICENSE
@@ -0,0 +1,23 @@
+The MIT License (MIT)
+
+Copyright 2012-2016 The Dojo Foundation
+Based on Underscore.js, copyright 2009-2016 Jeremy Ashkenas,
+DocumentCloud and Investigative Reporters & Editors
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in all
+copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
+SOFTWARE.
diff --git a/node_modules/lodash._root/README.md b/node_modules/lodash._root/README.md
new file mode 100644
index 0000000..0329abf
--- /dev/null
+++ b/node_modules/lodash._root/README.md
@@ -0,0 +1,18 @@
+# lodash._root v3.0.1
+
+The internal [lodash](https://lodash.com/) function `root` exported as a [Node.js](https://nodejs.org/) module.
+
+## Installation
+
+Using npm:
+```bash
+$ {sudo -H} npm i -g npm
+$ npm i --save lodash._root
+```
+
+In Node.js:
+```js
+var root = require('lodash._root');
+```
+
+See the [package source](https://github.com/lodash/lodash/blob/3.0.1-npm-packages/lodash._root) for more details.
diff --git a/node_modules/lodash._root/index.js b/node_modules/lodash._root/index.js
new file mode 100644
index 0000000..2d8ba0a
--- /dev/null
+++ b/node_modules/lodash._root/index.js
@@ -0,0 +1,59 @@
+/**
+ * lodash 3.0.1 (Custom Build)
+ * Build: `lodash modularize exports="npm" -o ./`
+ * Copyright 2012-2016 The Dojo Foundation
+ * Based on Underscore.js 1.8.3
+ * Copyright 2009-2016 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license
+ */
+
+/** Used to determine if values are of the language type `Object`. */
+var objectTypes = {
+ 'function': true,
+ 'object': true
+};
+
+/** Detect free variable `exports`. */
+var freeExports = (objectTypes[typeof exports] && exports && !exports.nodeType)
+ ? exports
+ : undefined;
+
+/** Detect free variable `module`. */
+var freeModule = (objectTypes[typeof module] && module && !module.nodeType)
+ ? module
+ : undefined;
+
+/** Detect free variable `global` from Node.js. */
+var freeGlobal = checkGlobal(freeExports && freeModule && typeof global == 'object' && global);
+
+/** Detect free variable `self`. */
+var freeSelf = checkGlobal(objectTypes[typeof self] && self);
+
+/** Detect free variable `window`. */
+var freeWindow = checkGlobal(objectTypes[typeof window] && window);
+
+/** Detect `this` as the global object. */
+var thisGlobal = checkGlobal(objectTypes[typeof this] && this);
+
+/**
+ * Used as a reference to the global object.
+ *
+ * The `this` value is used if it's the global object to avoid Greasemonkey's
+ * restricted `window` object, otherwise the `window` object is used.
+ */
+var root = freeGlobal ||
+ ((freeWindow !== (thisGlobal && thisGlobal.window)) && freeWindow) ||
+ freeSelf || thisGlobal || Function('return this')();
+
+/**
+ * Checks if `value` is a global object.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {null|Object} Returns `value` if it's a global object, else `null`.
+ */
+function checkGlobal(value) {
+ return (value && value.Object === Object) ? value : null;
+}
+
+module.exports = root;
diff --git a/node_modules/lodash._root/package.json b/node_modules/lodash._root/package.json
new file mode 100644
index 0000000..1be9c0c
--- /dev/null
+++ b/node_modules/lodash._root/package.json
@@ -0,0 +1,65 @@
+{
+ "_from": "lodash._root@^3.0.0",
+ "_id": "lodash._root@3.0.1",
+ "_inBundle": false,
+ "_integrity": "sha1-+6HEUkwZ7ppfgTa0YJ8BfPTe1pI=",
+ "_location": "/lodash._root",
+ "_phantomChildren": {},
+ "_requested": {
+ "type": "range",
+ "registry": true,
+ "raw": "lodash._root@^3.0.0",
+ "name": "lodash._root",
+ "escapedName": "lodash._root",
+ "rawSpec": "^3.0.0",
+ "saveSpec": null,
+ "fetchSpec": "^3.0.0"
+ },
+ "_requiredBy": [
+ "/lodash.escape"
+ ],
+ "_resolved": "https://registry.npmjs.org/lodash._root/-/lodash._root-3.0.1.tgz",
+ "_shasum": "fba1c4524c19ee9a5f8136b4609f017cf4ded692",
+ "_spec": "lodash._root@^3.0.0",
+ "_where": "/Users/rpatra16/MyProjects/RamAndAntara/node_modules/lodash.escape",
+ "author": {
+ "name": "John-David Dalton",
+ "email": "john.david.dalton@gmail.com",
+ "url": "http://allyoucanleet.com/"
+ },
+ "bugs": {
+ "url": "https://github.com/lodash/lodash/issues"
+ },
+ "bundleDependencies": false,
+ "contributors": [
+ {
+ "name": "John-David Dalton",
+ "email": "john.david.dalton@gmail.com",
+ "url": "http://allyoucanleet.com/"
+ },
+ {
+ "name": "Blaine Bublitz",
+ "email": "blaine@iceddev.com",
+ "url": "https://github.com/phated"
+ },
+ {
+ "name": "Mathias Bynens",
+ "email": "mathias@qiwi.be",
+ "url": "https://mathiasbynens.be/"
+ }
+ ],
+ "deprecated": false,
+ "description": "The internal lodash function `root` exported as a module.",
+ "homepage": "https://lodash.com/",
+ "icon": "https://lodash.com/icon.svg",
+ "license": "MIT",
+ "name": "lodash._root",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/lodash/lodash.git"
+ },
+ "scripts": {
+ "test": "echo \"See https://travis-ci.org/lodash/lodash-cli for testing details.\""
+ },
+ "version": "3.0.1"
+}
diff --git a/node_modules/lodash.assign/LICENSE b/node_modules/lodash.assign/LICENSE
new file mode 100644
index 0000000..e0c69d5
--- /dev/null
+++ b/node_modules/lodash.assign/LICENSE
@@ -0,0 +1,47 @@
+Copyright jQuery Foundation and other contributors
+
+Based on Underscore.js, copyright Jeremy Ashkenas,
+DocumentCloud and Investigative Reporters & Editors
+
+This software consists of voluntary contributions made by many
+individuals. For exact contribution history, see the revision history
+available at https://github.com/lodash/lodash
+
+The following license applies to all parts of this software except as
+documented below:
+
+====
+
+Permission is hereby granted, free of charge, to any person obtaining
+a copy of this software and associated documentation files (the
+"Software"), to deal in the Software without restriction, including
+without limitation the rights to use, copy, modify, merge, publish,
+distribute, sublicense, and/or sell copies of the Software, and to
+permit persons to whom the Software is furnished to do so, subject to
+the following conditions:
+
+The above copyright notice and this permission notice shall be
+included in all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
+EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
+MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
+NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
+LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
+OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
+WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
+
+====
+
+Copyright and related rights for sample code are waived via CC0. Sample
+code is defined as all source code displayed within the prose of the
+documentation.
+
+CC0: http://creativecommons.org/publicdomain/zero/1.0/
+
+====
+
+Files located in the node_modules and vendor directories are externally
+maintained libraries used by this software which have their own
+licenses; we recommend you read them, as their terms may differ from the
+terms above.
diff --git a/node_modules/lodash.assign/README.md b/node_modules/lodash.assign/README.md
new file mode 100644
index 0000000..6bce2d6
--- /dev/null
+++ b/node_modules/lodash.assign/README.md
@@ -0,0 +1,18 @@
+# lodash.assign v4.2.0
+
+The [lodash](https://lodash.com/) method `_.assign` exported as a [Node.js](https://nodejs.org/) module.
+
+## Installation
+
+Using npm:
+```bash
+$ {sudo -H} npm i -g npm
+$ npm i --save lodash.assign
+```
+
+In Node.js:
+```js
+var assign = require('lodash.assign');
+```
+
+See the [documentation](https://lodash.com/docs#assign) or [package source](https://github.com/lodash/lodash/blob/4.2.0-npm-packages/lodash.assign) for more details.
diff --git a/node_modules/lodash.assign/index.js b/node_modules/lodash.assign/index.js
new file mode 100644
index 0000000..8b007bc
--- /dev/null
+++ b/node_modules/lodash.assign/index.js
@@ -0,0 +1,637 @@
+/**
+ * lodash (Custom Build)
+ * Build: `lodash modularize exports="npm" -o ./`
+ * Copyright jQuery Foundation and other contributors
+ * Released under MIT license
+ * Based on Underscore.js 1.8.3
+ * Copyright Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ */
+
+/** Used as references for various `Number` constants. */
+var MAX_SAFE_INTEGER = 9007199254740991;
+
+/** `Object#toString` result references. */
+var argsTag = '[object Arguments]',
+ funcTag = '[object Function]',
+ genTag = '[object GeneratorFunction]';
+
+/** Used to detect unsigned integer values. */
+var reIsUint = /^(?:0|[1-9]\d*)$/;
+
+/**
+ * A faster alternative to `Function#apply`, this function invokes `func`
+ * with the `this` binding of `thisArg` and the arguments of `args`.
+ *
+ * @private
+ * @param {Function} func The function to invoke.
+ * @param {*} thisArg The `this` binding of `func`.
+ * @param {Array} args The arguments to invoke `func` with.
+ * @returns {*} Returns the result of `func`.
+ */
+function apply(func, thisArg, args) {
+ switch (args.length) {
+ case 0: return func.call(thisArg);
+ case 1: return func.call(thisArg, args[0]);
+ case 2: return func.call(thisArg, args[0], args[1]);
+ case 3: return func.call(thisArg, args[0], args[1], args[2]);
+ }
+ return func.apply(thisArg, args);
+}
+
+/**
+ * The base implementation of `_.times` without support for iteratee shorthands
+ * or max array length checks.
+ *
+ * @private
+ * @param {number} n The number of times to invoke `iteratee`.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Array} Returns the array of results.
+ */
+function baseTimes(n, iteratee) {
+ var index = -1,
+ result = Array(n);
+
+ while (++index < n) {
+ result[index] = iteratee(index);
+ }
+ return result;
+}
+
+/**
+ * Creates a unary function that invokes `func` with its argument transformed.
+ *
+ * @private
+ * @param {Function} func The function to wrap.
+ * @param {Function} transform The argument transform.
+ * @returns {Function} Returns the new function.
+ */
+function overArg(func, transform) {
+ return function(arg) {
+ return func(transform(arg));
+ };
+}
+
+/** Used for built-in method references. */
+var objectProto = Object.prototype;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Used to resolve the
+ * [`toStringTag`](http://ecma-international.org/ecma-262/7.0/#sec-object.prototype.tostring)
+ * of values.
+ */
+var objectToString = objectProto.toString;
+
+/** Built-in value references. */
+var propertyIsEnumerable = objectProto.propertyIsEnumerable;
+
+/* Built-in method references for those with the same name as other `lodash` methods. */
+var nativeKeys = overArg(Object.keys, Object),
+ nativeMax = Math.max;
+
+/** Detect if properties shadowing those on `Object.prototype` are non-enumerable. */
+var nonEnumShadows = !propertyIsEnumerable.call({ 'valueOf': 1 }, 'valueOf');
+
+/**
+ * Creates an array of the enumerable property names of the array-like `value`.
+ *
+ * @private
+ * @param {*} value The value to query.
+ * @param {boolean} inherited Specify returning inherited property names.
+ * @returns {Array} Returns the array of property names.
+ */
+function arrayLikeKeys(value, inherited) {
+ // Safari 8.1 makes `arguments.callee` enumerable in strict mode.
+ // Safari 9 makes `arguments.length` enumerable in strict mode.
+ var result = (isArray(value) || isArguments(value))
+ ? baseTimes(value.length, String)
+ : [];
+
+ var length = result.length,
+ skipIndexes = !!length;
+
+ for (var key in value) {
+ if ((inherited || hasOwnProperty.call(value, key)) &&
+ !(skipIndexes && (key == 'length' || isIndex(key, length)))) {
+ result.push(key);
+ }
+ }
+ return result;
+}
+
+/**
+ * Assigns `value` to `key` of `object` if the existing value is not equivalent
+ * using [`SameValueZero`](http://ecma-international.org/ecma-262/7.0/#sec-samevaluezero)
+ * for equality comparisons.
+ *
+ * @private
+ * @param {Object} object The object to modify.
+ * @param {string} key The key of the property to assign.
+ * @param {*} value The value to assign.
+ */
+function assignValue(object, key, value) {
+ var objValue = object[key];
+ if (!(hasOwnProperty.call(object, key) && eq(objValue, value)) ||
+ (value === undefined && !(key in object))) {
+ object[key] = value;
+ }
+}
+
+/**
+ * The base implementation of `_.keys` which doesn't treat sparse arrays as dense.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the array of property names.
+ */
+function baseKeys(object) {
+ if (!isPrototype(object)) {
+ return nativeKeys(object);
+ }
+ var result = [];
+ for (var key in Object(object)) {
+ if (hasOwnProperty.call(object, key) && key != 'constructor') {
+ result.push(key);
+ }
+ }
+ return result;
+}
+
+/**
+ * The base implementation of `_.rest` which doesn't validate or coerce arguments.
+ *
+ * @private
+ * @param {Function} func The function to apply a rest parameter to.
+ * @param {number} [start=func.length-1] The start position of the rest parameter.
+ * @returns {Function} Returns the new function.
+ */
+function baseRest(func, start) {
+ start = nativeMax(start === undefined ? (func.length - 1) : start, 0);
+ return function() {
+ var args = arguments,
+ index = -1,
+ length = nativeMax(args.length - start, 0),
+ array = Array(length);
+
+ while (++index < length) {
+ array[index] = args[start + index];
+ }
+ index = -1;
+ var otherArgs = Array(start + 1);
+ while (++index < start) {
+ otherArgs[index] = args[index];
+ }
+ otherArgs[start] = array;
+ return apply(func, this, otherArgs);
+ };
+}
+
+/**
+ * Copies properties of `source` to `object`.
+ *
+ * @private
+ * @param {Object} source The object to copy properties from.
+ * @param {Array} props The property identifiers to copy.
+ * @param {Object} [object={}] The object to copy properties to.
+ * @param {Function} [customizer] The function to customize copied values.
+ * @returns {Object} Returns `object`.
+ */
+function copyObject(source, props, object, customizer) {
+ object || (object = {});
+
+ var index = -1,
+ length = props.length;
+
+ while (++index < length) {
+ var key = props[index];
+
+ var newValue = customizer
+ ? customizer(object[key], source[key], key, object, source)
+ : undefined;
+
+ assignValue(object, key, newValue === undefined ? source[key] : newValue);
+ }
+ return object;
+}
+
+/**
+ * Creates a function like `_.assign`.
+ *
+ * @private
+ * @param {Function} assigner The function to assign values.
+ * @returns {Function} Returns the new assigner function.
+ */
+function createAssigner(assigner) {
+ return baseRest(function(object, sources) {
+ var index = -1,
+ length = sources.length,
+ customizer = length > 1 ? sources[length - 1] : undefined,
+ guard = length > 2 ? sources[2] : undefined;
+
+ customizer = (assigner.length > 3 && typeof customizer == 'function')
+ ? (length--, customizer)
+ : undefined;
+
+ if (guard && isIterateeCall(sources[0], sources[1], guard)) {
+ customizer = length < 3 ? undefined : customizer;
+ length = 1;
+ }
+ object = Object(object);
+ while (++index < length) {
+ var source = sources[index];
+ if (source) {
+ assigner(object, source, index, customizer);
+ }
+ }
+ return object;
+ });
+}
+
+/**
+ * Checks if `value` is a valid array-like index.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @param {number} [length=MAX_SAFE_INTEGER] The upper bounds of a valid index.
+ * @returns {boolean} Returns `true` if `value` is a valid index, else `false`.
+ */
+function isIndex(value, length) {
+ length = length == null ? MAX_SAFE_INTEGER : length;
+ return !!length &&
+ (typeof value == 'number' || reIsUint.test(value)) &&
+ (value > -1 && value % 1 == 0 && value < length);
+}
+
+/**
+ * Checks if the given arguments are from an iteratee call.
+ *
+ * @private
+ * @param {*} value The potential iteratee value argument.
+ * @param {*} index The potential iteratee index or key argument.
+ * @param {*} object The potential iteratee object argument.
+ * @returns {boolean} Returns `true` if the arguments are from an iteratee call,
+ * else `false`.
+ */
+function isIterateeCall(value, index, object) {
+ if (!isObject(object)) {
+ return false;
+ }
+ var type = typeof index;
+ if (type == 'number'
+ ? (isArrayLike(object) && isIndex(index, object.length))
+ : (type == 'string' && index in object)
+ ) {
+ return eq(object[index], value);
+ }
+ return false;
+}
+
+/**
+ * Checks if `value` is likely a prototype object.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a prototype, else `false`.
+ */
+function isPrototype(value) {
+ var Ctor = value && value.constructor,
+ proto = (typeof Ctor == 'function' && Ctor.prototype) || objectProto;
+
+ return value === proto;
+}
+
+/**
+ * Performs a
+ * [`SameValueZero`](http://ecma-international.org/ecma-262/7.0/#sec-samevaluezero)
+ * comparison between two values to determine if they are equivalent.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to compare.
+ * @param {*} other The other value to compare.
+ * @returns {boolean} Returns `true` if the values are equivalent, else `false`.
+ * @example
+ *
+ * var object = { 'a': 1 };
+ * var other = { 'a': 1 };
+ *
+ * _.eq(object, object);
+ * // => true
+ *
+ * _.eq(object, other);
+ * // => false
+ *
+ * _.eq('a', 'a');
+ * // => true
+ *
+ * _.eq('a', Object('a'));
+ * // => false
+ *
+ * _.eq(NaN, NaN);
+ * // => true
+ */
+function eq(value, other) {
+ return value === other || (value !== value && other !== other);
+}
+
+/**
+ * Checks if `value` is likely an `arguments` object.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is an `arguments` object,
+ * else `false`.
+ * @example
+ *
+ * _.isArguments(function() { return arguments; }());
+ * // => true
+ *
+ * _.isArguments([1, 2, 3]);
+ * // => false
+ */
+function isArguments(value) {
+ // Safari 8.1 makes `arguments.callee` enumerable in strict mode.
+ return isArrayLikeObject(value) && hasOwnProperty.call(value, 'callee') &&
+ (!propertyIsEnumerable.call(value, 'callee') || objectToString.call(value) == argsTag);
+}
+
+/**
+ * Checks if `value` is classified as an `Array` object.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is an array, else `false`.
+ * @example
+ *
+ * _.isArray([1, 2, 3]);
+ * // => true
+ *
+ * _.isArray(document.body.children);
+ * // => false
+ *
+ * _.isArray('abc');
+ * // => false
+ *
+ * _.isArray(_.noop);
+ * // => false
+ */
+var isArray = Array.isArray;
+
+/**
+ * Checks if `value` is array-like. A value is considered array-like if it's
+ * not a function and has a `value.length` that's an integer greater than or
+ * equal to `0` and less than or equal to `Number.MAX_SAFE_INTEGER`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is array-like, else `false`.
+ * @example
+ *
+ * _.isArrayLike([1, 2, 3]);
+ * // => true
+ *
+ * _.isArrayLike(document.body.children);
+ * // => true
+ *
+ * _.isArrayLike('abc');
+ * // => true
+ *
+ * _.isArrayLike(_.noop);
+ * // => false
+ */
+function isArrayLike(value) {
+ return value != null && isLength(value.length) && !isFunction(value);
+}
+
+/**
+ * This method is like `_.isArrayLike` except that it also checks if `value`
+ * is an object.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is an array-like object,
+ * else `false`.
+ * @example
+ *
+ * _.isArrayLikeObject([1, 2, 3]);
+ * // => true
+ *
+ * _.isArrayLikeObject(document.body.children);
+ * // => true
+ *
+ * _.isArrayLikeObject('abc');
+ * // => false
+ *
+ * _.isArrayLikeObject(_.noop);
+ * // => false
+ */
+function isArrayLikeObject(value) {
+ return isObjectLike(value) && isArrayLike(value);
+}
+
+/**
+ * Checks if `value` is classified as a `Function` object.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a function, else `false`.
+ * @example
+ *
+ * _.isFunction(_);
+ * // => true
+ *
+ * _.isFunction(/abc/);
+ * // => false
+ */
+function isFunction(value) {
+ // The use of `Object#toString` avoids issues with the `typeof` operator
+ // in Safari 8-9 which returns 'object' for typed array and other constructors.
+ var tag = isObject(value) ? objectToString.call(value) : '';
+ return tag == funcTag || tag == genTag;
+}
+
+/**
+ * Checks if `value` is a valid array-like length.
+ *
+ * **Note:** This method is loosely based on
+ * [`ToLength`](http://ecma-international.org/ecma-262/7.0/#sec-tolength).
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a valid length, else `false`.
+ * @example
+ *
+ * _.isLength(3);
+ * // => true
+ *
+ * _.isLength(Number.MIN_VALUE);
+ * // => false
+ *
+ * _.isLength(Infinity);
+ * // => false
+ *
+ * _.isLength('3');
+ * // => false
+ */
+function isLength(value) {
+ return typeof value == 'number' &&
+ value > -1 && value % 1 == 0 && value <= MAX_SAFE_INTEGER;
+}
+
+/**
+ * Checks if `value` is the
+ * [language type](http://www.ecma-international.org/ecma-262/7.0/#sec-ecmascript-language-types)
+ * of `Object`. (e.g. arrays, functions, objects, regexes, `new Number(0)`, and `new String('')`)
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is an object, else `false`.
+ * @example
+ *
+ * _.isObject({});
+ * // => true
+ *
+ * _.isObject([1, 2, 3]);
+ * // => true
+ *
+ * _.isObject(_.noop);
+ * // => true
+ *
+ * _.isObject(null);
+ * // => false
+ */
+function isObject(value) {
+ var type = typeof value;
+ return !!value && (type == 'object' || type == 'function');
+}
+
+/**
+ * Checks if `value` is object-like. A value is object-like if it's not `null`
+ * and has a `typeof` result of "object".
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is object-like, else `false`.
+ * @example
+ *
+ * _.isObjectLike({});
+ * // => true
+ *
+ * _.isObjectLike([1, 2, 3]);
+ * // => true
+ *
+ * _.isObjectLike(_.noop);
+ * // => false
+ *
+ * _.isObjectLike(null);
+ * // => false
+ */
+function isObjectLike(value) {
+ return !!value && typeof value == 'object';
+}
+
+/**
+ * Assigns own enumerable string keyed properties of source objects to the
+ * destination object. Source objects are applied from left to right.
+ * Subsequent sources overwrite property assignments of previous sources.
+ *
+ * **Note:** This method mutates `object` and is loosely based on
+ * [`Object.assign`](https://mdn.io/Object/assign).
+ *
+ * @static
+ * @memberOf _
+ * @since 0.10.0
+ * @category Object
+ * @param {Object} object The destination object.
+ * @param {...Object} [sources] The source objects.
+ * @returns {Object} Returns `object`.
+ * @see _.assignIn
+ * @example
+ *
+ * function Foo() {
+ * this.a = 1;
+ * }
+ *
+ * function Bar() {
+ * this.c = 3;
+ * }
+ *
+ * Foo.prototype.b = 2;
+ * Bar.prototype.d = 4;
+ *
+ * _.assign({ 'a': 0 }, new Foo, new Bar);
+ * // => { 'a': 1, 'c': 3 }
+ */
+var assign = createAssigner(function(object, source) {
+ if (nonEnumShadows || isPrototype(source) || isArrayLike(source)) {
+ copyObject(source, keys(source), object);
+ return;
+ }
+ for (var key in source) {
+ if (hasOwnProperty.call(source, key)) {
+ assignValue(object, key, source[key]);
+ }
+ }
+});
+
+/**
+ * Creates an array of the own enumerable property names of `object`.
+ *
+ * **Note:** Non-object values are coerced to objects. See the
+ * [ES spec](http://ecma-international.org/ecma-262/7.0/#sec-object.keys)
+ * for more details.
+ *
+ * @static
+ * @since 0.1.0
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the array of property names.
+ * @example
+ *
+ * function Foo() {
+ * this.a = 1;
+ * this.b = 2;
+ * }
+ *
+ * Foo.prototype.c = 3;
+ *
+ * _.keys(new Foo);
+ * // => ['a', 'b'] (iteration order is not guaranteed)
+ *
+ * _.keys('hi');
+ * // => ['0', '1']
+ */
+function keys(object) {
+ return isArrayLike(object) ? arrayLikeKeys(object) : baseKeys(object);
+}
+
+module.exports = assign;
diff --git a/node_modules/lodash.assign/package.json b/node_modules/lodash.assign/package.json
new file mode 100644
index 0000000..3547aa2
--- /dev/null
+++ b/node_modules/lodash.assign/package.json
@@ -0,0 +1,113 @@
+{
+ "_args": [
+ [
+ {
+ "raw": "lodash.assign@^4.2.0",
+ "scope": null,
+ "escapedName": "lodash.assign",
+ "name": "lodash.assign",
+ "rawSpec": "^4.2.0",
+ "spec": ">=4.2.0 <5.0.0",
+ "type": "range"
+ },
+ "/Users/ramswaroop/MyProjects/RamAndAntara/node_modules/node-sass"
+ ]
+ ],
+ "_from": "lodash.assign@>=4.2.0 <5.0.0",
+ "_id": "lodash.assign@4.2.0",
+ "_inCache": true,
+ "_location": "/lodash.assign",
+ "_nodeVersion": "4.4.7",
+ "_npmOperationalInternal": {
+ "host": "packages-16-east.internal.npmjs.com",
+ "tmp": "tmp/lodash.assign-4.2.0.tgz_1471109795713_0.5842255132738501"
+ },
+ "_npmUser": {
+ "name": "jdalton",
+ "email": "john.david.dalton@gmail.com"
+ },
+ "_npmVersion": "2.15.10",
+ "_phantomChildren": {},
+ "_requested": {
+ "raw": "lodash.assign@^4.2.0",
+ "scope": null,
+ "escapedName": "lodash.assign",
+ "name": "lodash.assign",
+ "rawSpec": "^4.2.0",
+ "spec": ">=4.2.0 <5.0.0",
+ "type": "range"
+ },
+ "_requiredBy": [
+ "/node-sass"
+ ],
+ "_resolved": "https://registry.npmjs.org/lodash.assign/-/lodash.assign-4.2.0.tgz",
+ "_shasum": "0d99f3ccd7a6d261d19bdaeb9245005d285808e7",
+ "_shrinkwrap": null,
+ "_spec": "lodash.assign@^4.2.0",
+ "_where": "/Users/ramswaroop/MyProjects/RamAndAntara/node_modules/node-sass",
+ "author": {
+ "name": "John-David Dalton",
+ "email": "john.david.dalton@gmail.com",
+ "url": "http://allyoucanleet.com/"
+ },
+ "bugs": {
+ "url": "https://github.com/lodash/lodash/issues"
+ },
+ "contributors": [
+ {
+ "name": "John-David Dalton",
+ "email": "john.david.dalton@gmail.com",
+ "url": "http://allyoucanleet.com/"
+ },
+ {
+ "name": "Blaine Bublitz",
+ "email": "blaine.bublitz@gmail.com",
+ "url": "https://github.com/phated"
+ },
+ {
+ "name": "Mathias Bynens",
+ "email": "mathias@qiwi.be",
+ "url": "https://mathiasbynens.be/"
+ }
+ ],
+ "dependencies": {},
+ "description": "The lodash method `_.assign` exported as a module.",
+ "devDependencies": {},
+ "directories": {},
+ "dist": {
+ "shasum": "0d99f3ccd7a6d261d19bdaeb9245005d285808e7",
+ "tarball": "https://registry.npmjs.org/lodash.assign/-/lodash.assign-4.2.0.tgz"
+ },
+ "homepage": "https://lodash.com/",
+ "icon": "https://lodash.com/icon.svg",
+ "keywords": [
+ "lodash-modularized",
+ "assign"
+ ],
+ "license": "MIT",
+ "maintainers": [
+ {
+ "name": "jdalton",
+ "email": "john.david.dalton@gmail.com"
+ },
+ {
+ "name": "mathias",
+ "email": "mathias@qiwi.be"
+ },
+ {
+ "name": "phated",
+ "email": "blaine@iceddev.com"
+ }
+ ],
+ "name": "lodash.assign",
+ "optionalDependencies": {},
+ "readme": "ERROR: No README data found!",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/lodash/lodash.git"
+ },
+ "scripts": {
+ "test": "echo \"See https://travis-ci.org/lodash/lodash-cli for testing details.\""
+ },
+ "version": "4.2.0"
+}
diff --git a/node_modules/lodash.clonedeep/LICENSE b/node_modules/lodash.clonedeep/LICENSE
new file mode 100644
index 0000000..e0c69d5
--- /dev/null
+++ b/node_modules/lodash.clonedeep/LICENSE
@@ -0,0 +1,47 @@
+Copyright jQuery Foundation and other contributors
+
+Based on Underscore.js, copyright Jeremy Ashkenas,
+DocumentCloud and Investigative Reporters & Editors
+
+This software consists of voluntary contributions made by many
+individuals. For exact contribution history, see the revision history
+available at https://github.com/lodash/lodash
+
+The following license applies to all parts of this software except as
+documented below:
+
+====
+
+Permission is hereby granted, free of charge, to any person obtaining
+a copy of this software and associated documentation files (the
+"Software"), to deal in the Software without restriction, including
+without limitation the rights to use, copy, modify, merge, publish,
+distribute, sublicense, and/or sell copies of the Software, and to
+permit persons to whom the Software is furnished to do so, subject to
+the following conditions:
+
+The above copyright notice and this permission notice shall be
+included in all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
+EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
+MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
+NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
+LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
+OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
+WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
+
+====
+
+Copyright and related rights for sample code are waived via CC0. Sample
+code is defined as all source code displayed within the prose of the
+documentation.
+
+CC0: http://creativecommons.org/publicdomain/zero/1.0/
+
+====
+
+Files located in the node_modules and vendor directories are externally
+maintained libraries used by this software which have their own
+licenses; we recommend you read them, as their terms may differ from the
+terms above.
diff --git a/node_modules/lodash.clonedeep/README.md b/node_modules/lodash.clonedeep/README.md
new file mode 100644
index 0000000..fee48e4
--- /dev/null
+++ b/node_modules/lodash.clonedeep/README.md
@@ -0,0 +1,18 @@
+# lodash.clonedeep v4.5.0
+
+The [lodash](https://lodash.com/) method `_.cloneDeep` exported as a [Node.js](https://nodejs.org/) module.
+
+## Installation
+
+Using npm:
+```bash
+$ {sudo -H} npm i -g npm
+$ npm i --save lodash.clonedeep
+```
+
+In Node.js:
+```js
+var cloneDeep = require('lodash.clonedeep');
+```
+
+See the [documentation](https://lodash.com/docs#cloneDeep) or [package source](https://github.com/lodash/lodash/blob/4.5.0-npm-packages/lodash.clonedeep) for more details.
diff --git a/node_modules/lodash.clonedeep/index.js b/node_modules/lodash.clonedeep/index.js
new file mode 100644
index 0000000..1b0e502
--- /dev/null
+++ b/node_modules/lodash.clonedeep/index.js
@@ -0,0 +1,1748 @@
+/**
+ * lodash (Custom Build)
+ * Build: `lodash modularize exports="npm" -o ./`
+ * Copyright jQuery Foundation and other contributors
+ * Released under MIT license
+ * Based on Underscore.js 1.8.3
+ * Copyright Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ */
+
+/** Used as the size to enable large array optimizations. */
+var LARGE_ARRAY_SIZE = 200;
+
+/** Used to stand-in for `undefined` hash values. */
+var HASH_UNDEFINED = '__lodash_hash_undefined__';
+
+/** Used as references for various `Number` constants. */
+var MAX_SAFE_INTEGER = 9007199254740991;
+
+/** `Object#toString` result references. */
+var argsTag = '[object Arguments]',
+ arrayTag = '[object Array]',
+ boolTag = '[object Boolean]',
+ dateTag = '[object Date]',
+ errorTag = '[object Error]',
+ funcTag = '[object Function]',
+ genTag = '[object GeneratorFunction]',
+ mapTag = '[object Map]',
+ numberTag = '[object Number]',
+ objectTag = '[object Object]',
+ promiseTag = '[object Promise]',
+ regexpTag = '[object RegExp]',
+ setTag = '[object Set]',
+ stringTag = '[object String]',
+ symbolTag = '[object Symbol]',
+ weakMapTag = '[object WeakMap]';
+
+var arrayBufferTag = '[object ArrayBuffer]',
+ dataViewTag = '[object DataView]',
+ float32Tag = '[object Float32Array]',
+ float64Tag = '[object Float64Array]',
+ int8Tag = '[object Int8Array]',
+ int16Tag = '[object Int16Array]',
+ int32Tag = '[object Int32Array]',
+ uint8Tag = '[object Uint8Array]',
+ uint8ClampedTag = '[object Uint8ClampedArray]',
+ uint16Tag = '[object Uint16Array]',
+ uint32Tag = '[object Uint32Array]';
+
+/**
+ * Used to match `RegExp`
+ * [syntax characters](http://ecma-international.org/ecma-262/7.0/#sec-patterns).
+ */
+var reRegExpChar = /[\\^$.*+?()[\]{}|]/g;
+
+/** Used to match `RegExp` flags from their coerced string values. */
+var reFlags = /\w*$/;
+
+/** Used to detect host constructors (Safari). */
+var reIsHostCtor = /^\[object .+?Constructor\]$/;
+
+/** Used to detect unsigned integer values. */
+var reIsUint = /^(?:0|[1-9]\d*)$/;
+
+/** Used to identify `toStringTag` values supported by `_.clone`. */
+var cloneableTags = {};
+cloneableTags[argsTag] = cloneableTags[arrayTag] =
+cloneableTags[arrayBufferTag] = cloneableTags[dataViewTag] =
+cloneableTags[boolTag] = cloneableTags[dateTag] =
+cloneableTags[float32Tag] = cloneableTags[float64Tag] =
+cloneableTags[int8Tag] = cloneableTags[int16Tag] =
+cloneableTags[int32Tag] = cloneableTags[mapTag] =
+cloneableTags[numberTag] = cloneableTags[objectTag] =
+cloneableTags[regexpTag] = cloneableTags[setTag] =
+cloneableTags[stringTag] = cloneableTags[symbolTag] =
+cloneableTags[uint8Tag] = cloneableTags[uint8ClampedTag] =
+cloneableTags[uint16Tag] = cloneableTags[uint32Tag] = true;
+cloneableTags[errorTag] = cloneableTags[funcTag] =
+cloneableTags[weakMapTag] = false;
+
+/** Detect free variable `global` from Node.js. */
+var freeGlobal = typeof global == 'object' && global && global.Object === Object && global;
+
+/** Detect free variable `self`. */
+var freeSelf = typeof self == 'object' && self && self.Object === Object && self;
+
+/** Used as a reference to the global object. */
+var root = freeGlobal || freeSelf || Function('return this')();
+
+/** Detect free variable `exports`. */
+var freeExports = typeof exports == 'object' && exports && !exports.nodeType && exports;
+
+/** Detect free variable `module`. */
+var freeModule = freeExports && typeof module == 'object' && module && !module.nodeType && module;
+
+/** Detect the popular CommonJS extension `module.exports`. */
+var moduleExports = freeModule && freeModule.exports === freeExports;
+
+/**
+ * Adds the key-value `pair` to `map`.
+ *
+ * @private
+ * @param {Object} map The map to modify.
+ * @param {Array} pair The key-value pair to add.
+ * @returns {Object} Returns `map`.
+ */
+function addMapEntry(map, pair) {
+ // Don't return `map.set` because it's not chainable in IE 11.
+ map.set(pair[0], pair[1]);
+ return map;
+}
+
+/**
+ * Adds `value` to `set`.
+ *
+ * @private
+ * @param {Object} set The set to modify.
+ * @param {*} value The value to add.
+ * @returns {Object} Returns `set`.
+ */
+function addSetEntry(set, value) {
+ // Don't return `set.add` because it's not chainable in IE 11.
+ set.add(value);
+ return set;
+}
+
+/**
+ * A specialized version of `_.forEach` for arrays without support for
+ * iteratee shorthands.
+ *
+ * @private
+ * @param {Array} [array] The array to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Array} Returns `array`.
+ */
+function arrayEach(array, iteratee) {
+ var index = -1,
+ length = array ? array.length : 0;
+
+ while (++index < length) {
+ if (iteratee(array[index], index, array) === false) {
+ break;
+ }
+ }
+ return array;
+}
+
+/**
+ * Appends the elements of `values` to `array`.
+ *
+ * @private
+ * @param {Array} array The array to modify.
+ * @param {Array} values The values to append.
+ * @returns {Array} Returns `array`.
+ */
+function arrayPush(array, values) {
+ var index = -1,
+ length = values.length,
+ offset = array.length;
+
+ while (++index < length) {
+ array[offset + index] = values[index];
+ }
+ return array;
+}
+
+/**
+ * A specialized version of `_.reduce` for arrays without support for
+ * iteratee shorthands.
+ *
+ * @private
+ * @param {Array} [array] The array to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @param {*} [accumulator] The initial value.
+ * @param {boolean} [initAccum] Specify using the first element of `array` as
+ * the initial value.
+ * @returns {*} Returns the accumulated value.
+ */
+function arrayReduce(array, iteratee, accumulator, initAccum) {
+ var index = -1,
+ length = array ? array.length : 0;
+
+ if (initAccum && length) {
+ accumulator = array[++index];
+ }
+ while (++index < length) {
+ accumulator = iteratee(accumulator, array[index], index, array);
+ }
+ return accumulator;
+}
+
+/**
+ * The base implementation of `_.times` without support for iteratee shorthands
+ * or max array length checks.
+ *
+ * @private
+ * @param {number} n The number of times to invoke `iteratee`.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Array} Returns the array of results.
+ */
+function baseTimes(n, iteratee) {
+ var index = -1,
+ result = Array(n);
+
+ while (++index < n) {
+ result[index] = iteratee(index);
+ }
+ return result;
+}
+
+/**
+ * Gets the value at `key` of `object`.
+ *
+ * @private
+ * @param {Object} [object] The object to query.
+ * @param {string} key The key of the property to get.
+ * @returns {*} Returns the property value.
+ */
+function getValue(object, key) {
+ return object == null ? undefined : object[key];
+}
+
+/**
+ * Checks if `value` is a host object in IE < 9.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a host object, else `false`.
+ */
+function isHostObject(value) {
+ // Many host objects are `Object` objects that can coerce to strings
+ // despite having improperly defined `toString` methods.
+ var result = false;
+ if (value != null && typeof value.toString != 'function') {
+ try {
+ result = !!(value + '');
+ } catch (e) {}
+ }
+ return result;
+}
+
+/**
+ * Converts `map` to its key-value pairs.
+ *
+ * @private
+ * @param {Object} map The map to convert.
+ * @returns {Array} Returns the key-value pairs.
+ */
+function mapToArray(map) {
+ var index = -1,
+ result = Array(map.size);
+
+ map.forEach(function(value, key) {
+ result[++index] = [key, value];
+ });
+ return result;
+}
+
+/**
+ * Creates a unary function that invokes `func` with its argument transformed.
+ *
+ * @private
+ * @param {Function} func The function to wrap.
+ * @param {Function} transform The argument transform.
+ * @returns {Function} Returns the new function.
+ */
+function overArg(func, transform) {
+ return function(arg) {
+ return func(transform(arg));
+ };
+}
+
+/**
+ * Converts `set` to an array of its values.
+ *
+ * @private
+ * @param {Object} set The set to convert.
+ * @returns {Array} Returns the values.
+ */
+function setToArray(set) {
+ var index = -1,
+ result = Array(set.size);
+
+ set.forEach(function(value) {
+ result[++index] = value;
+ });
+ return result;
+}
+
+/** Used for built-in method references. */
+var arrayProto = Array.prototype,
+ funcProto = Function.prototype,
+ objectProto = Object.prototype;
+
+/** Used to detect overreaching core-js shims. */
+var coreJsData = root['__core-js_shared__'];
+
+/** Used to detect methods masquerading as native. */
+var maskSrcKey = (function() {
+ var uid = /[^.]+$/.exec(coreJsData && coreJsData.keys && coreJsData.keys.IE_PROTO || '');
+ return uid ? ('Symbol(src)_1.' + uid) : '';
+}());
+
+/** Used to resolve the decompiled source of functions. */
+var funcToString = funcProto.toString;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Used to resolve the
+ * [`toStringTag`](http://ecma-international.org/ecma-262/7.0/#sec-object.prototype.tostring)
+ * of values.
+ */
+var objectToString = objectProto.toString;
+
+/** Used to detect if a method is native. */
+var reIsNative = RegExp('^' +
+ funcToString.call(hasOwnProperty).replace(reRegExpChar, '\\$&')
+ .replace(/hasOwnProperty|(function).*?(?=\\\()| for .+?(?=\\\])/g, '$1.*?') + '$'
+);
+
+/** Built-in value references. */
+var Buffer = moduleExports ? root.Buffer : undefined,
+ Symbol = root.Symbol,
+ Uint8Array = root.Uint8Array,
+ getPrototype = overArg(Object.getPrototypeOf, Object),
+ objectCreate = Object.create,
+ propertyIsEnumerable = objectProto.propertyIsEnumerable,
+ splice = arrayProto.splice;
+
+/* Built-in method references for those with the same name as other `lodash` methods. */
+var nativeGetSymbols = Object.getOwnPropertySymbols,
+ nativeIsBuffer = Buffer ? Buffer.isBuffer : undefined,
+ nativeKeys = overArg(Object.keys, Object);
+
+/* Built-in method references that are verified to be native. */
+var DataView = getNative(root, 'DataView'),
+ Map = getNative(root, 'Map'),
+ Promise = getNative(root, 'Promise'),
+ Set = getNative(root, 'Set'),
+ WeakMap = getNative(root, 'WeakMap'),
+ nativeCreate = getNative(Object, 'create');
+
+/** Used to detect maps, sets, and weakmaps. */
+var dataViewCtorString = toSource(DataView),
+ mapCtorString = toSource(Map),
+ promiseCtorString = toSource(Promise),
+ setCtorString = toSource(Set),
+ weakMapCtorString = toSource(WeakMap);
+
+/** Used to convert symbols to primitives and strings. */
+var symbolProto = Symbol ? Symbol.prototype : undefined,
+ symbolValueOf = symbolProto ? symbolProto.valueOf : undefined;
+
+/**
+ * Creates a hash object.
+ *
+ * @private
+ * @constructor
+ * @param {Array} [entries] The key-value pairs to cache.
+ */
+function Hash(entries) {
+ var index = -1,
+ length = entries ? entries.length : 0;
+
+ this.clear();
+ while (++index < length) {
+ var entry = entries[index];
+ this.set(entry[0], entry[1]);
+ }
+}
+
+/**
+ * Removes all key-value entries from the hash.
+ *
+ * @private
+ * @name clear
+ * @memberOf Hash
+ */
+function hashClear() {
+ this.__data__ = nativeCreate ? nativeCreate(null) : {};
+}
+
+/**
+ * Removes `key` and its value from the hash.
+ *
+ * @private
+ * @name delete
+ * @memberOf Hash
+ * @param {Object} hash The hash to modify.
+ * @param {string} key The key of the value to remove.
+ * @returns {boolean} Returns `true` if the entry was removed, else `false`.
+ */
+function hashDelete(key) {
+ return this.has(key) && delete this.__data__[key];
+}
+
+/**
+ * Gets the hash value for `key`.
+ *
+ * @private
+ * @name get
+ * @memberOf Hash
+ * @param {string} key The key of the value to get.
+ * @returns {*} Returns the entry value.
+ */
+function hashGet(key) {
+ var data = this.__data__;
+ if (nativeCreate) {
+ var result = data[key];
+ return result === HASH_UNDEFINED ? undefined : result;
+ }
+ return hasOwnProperty.call(data, key) ? data[key] : undefined;
+}
+
+/**
+ * Checks if a hash value for `key` exists.
+ *
+ * @private
+ * @name has
+ * @memberOf Hash
+ * @param {string} key The key of the entry to check.
+ * @returns {boolean} Returns `true` if an entry for `key` exists, else `false`.
+ */
+function hashHas(key) {
+ var data = this.__data__;
+ return nativeCreate ? data[key] !== undefined : hasOwnProperty.call(data, key);
+}
+
+/**
+ * Sets the hash `key` to `value`.
+ *
+ * @private
+ * @name set
+ * @memberOf Hash
+ * @param {string} key The key of the value to set.
+ * @param {*} value The value to set.
+ * @returns {Object} Returns the hash instance.
+ */
+function hashSet(key, value) {
+ var data = this.__data__;
+ data[key] = (nativeCreate && value === undefined) ? HASH_UNDEFINED : value;
+ return this;
+}
+
+// Add methods to `Hash`.
+Hash.prototype.clear = hashClear;
+Hash.prototype['delete'] = hashDelete;
+Hash.prototype.get = hashGet;
+Hash.prototype.has = hashHas;
+Hash.prototype.set = hashSet;
+
+/**
+ * Creates an list cache object.
+ *
+ * @private
+ * @constructor
+ * @param {Array} [entries] The key-value pairs to cache.
+ */
+function ListCache(entries) {
+ var index = -1,
+ length = entries ? entries.length : 0;
+
+ this.clear();
+ while (++index < length) {
+ var entry = entries[index];
+ this.set(entry[0], entry[1]);
+ }
+}
+
+/**
+ * Removes all key-value entries from the list cache.
+ *
+ * @private
+ * @name clear
+ * @memberOf ListCache
+ */
+function listCacheClear() {
+ this.__data__ = [];
+}
+
+/**
+ * Removes `key` and its value from the list cache.
+ *
+ * @private
+ * @name delete
+ * @memberOf ListCache
+ * @param {string} key The key of the value to remove.
+ * @returns {boolean} Returns `true` if the entry was removed, else `false`.
+ */
+function listCacheDelete(key) {
+ var data = this.__data__,
+ index = assocIndexOf(data, key);
+
+ if (index < 0) {
+ return false;
+ }
+ var lastIndex = data.length - 1;
+ if (index == lastIndex) {
+ data.pop();
+ } else {
+ splice.call(data, index, 1);
+ }
+ return true;
+}
+
+/**
+ * Gets the list cache value for `key`.
+ *
+ * @private
+ * @name get
+ * @memberOf ListCache
+ * @param {string} key The key of the value to get.
+ * @returns {*} Returns the entry value.
+ */
+function listCacheGet(key) {
+ var data = this.__data__,
+ index = assocIndexOf(data, key);
+
+ return index < 0 ? undefined : data[index][1];
+}
+
+/**
+ * Checks if a list cache value for `key` exists.
+ *
+ * @private
+ * @name has
+ * @memberOf ListCache
+ * @param {string} key The key of the entry to check.
+ * @returns {boolean} Returns `true` if an entry for `key` exists, else `false`.
+ */
+function listCacheHas(key) {
+ return assocIndexOf(this.__data__, key) > -1;
+}
+
+/**
+ * Sets the list cache `key` to `value`.
+ *
+ * @private
+ * @name set
+ * @memberOf ListCache
+ * @param {string} key The key of the value to set.
+ * @param {*} value The value to set.
+ * @returns {Object} Returns the list cache instance.
+ */
+function listCacheSet(key, value) {
+ var data = this.__data__,
+ index = assocIndexOf(data, key);
+
+ if (index < 0) {
+ data.push([key, value]);
+ } else {
+ data[index][1] = value;
+ }
+ return this;
+}
+
+// Add methods to `ListCache`.
+ListCache.prototype.clear = listCacheClear;
+ListCache.prototype['delete'] = listCacheDelete;
+ListCache.prototype.get = listCacheGet;
+ListCache.prototype.has = listCacheHas;
+ListCache.prototype.set = listCacheSet;
+
+/**
+ * Creates a map cache object to store key-value pairs.
+ *
+ * @private
+ * @constructor
+ * @param {Array} [entries] The key-value pairs to cache.
+ */
+function MapCache(entries) {
+ var index = -1,
+ length = entries ? entries.length : 0;
+
+ this.clear();
+ while (++index < length) {
+ var entry = entries[index];
+ this.set(entry[0], entry[1]);
+ }
+}
+
+/**
+ * Removes all key-value entries from the map.
+ *
+ * @private
+ * @name clear
+ * @memberOf MapCache
+ */
+function mapCacheClear() {
+ this.__data__ = {
+ 'hash': new Hash,
+ 'map': new (Map || ListCache),
+ 'string': new Hash
+ };
+}
+
+/**
+ * Removes `key` and its value from the map.
+ *
+ * @private
+ * @name delete
+ * @memberOf MapCache
+ * @param {string} key The key of the value to remove.
+ * @returns {boolean} Returns `true` if the entry was removed, else `false`.
+ */
+function mapCacheDelete(key) {
+ return getMapData(this, key)['delete'](key);
+}
+
+/**
+ * Gets the map value for `key`.
+ *
+ * @private
+ * @name get
+ * @memberOf MapCache
+ * @param {string} key The key of the value to get.
+ * @returns {*} Returns the entry value.
+ */
+function mapCacheGet(key) {
+ return getMapData(this, key).get(key);
+}
+
+/**
+ * Checks if a map value for `key` exists.
+ *
+ * @private
+ * @name has
+ * @memberOf MapCache
+ * @param {string} key The key of the entry to check.
+ * @returns {boolean} Returns `true` if an entry for `key` exists, else `false`.
+ */
+function mapCacheHas(key) {
+ return getMapData(this, key).has(key);
+}
+
+/**
+ * Sets the map `key` to `value`.
+ *
+ * @private
+ * @name set
+ * @memberOf MapCache
+ * @param {string} key The key of the value to set.
+ * @param {*} value The value to set.
+ * @returns {Object} Returns the map cache instance.
+ */
+function mapCacheSet(key, value) {
+ getMapData(this, key).set(key, value);
+ return this;
+}
+
+// Add methods to `MapCache`.
+MapCache.prototype.clear = mapCacheClear;
+MapCache.prototype['delete'] = mapCacheDelete;
+MapCache.prototype.get = mapCacheGet;
+MapCache.prototype.has = mapCacheHas;
+MapCache.prototype.set = mapCacheSet;
+
+/**
+ * Creates a stack cache object to store key-value pairs.
+ *
+ * @private
+ * @constructor
+ * @param {Array} [entries] The key-value pairs to cache.
+ */
+function Stack(entries) {
+ this.__data__ = new ListCache(entries);
+}
+
+/**
+ * Removes all key-value entries from the stack.
+ *
+ * @private
+ * @name clear
+ * @memberOf Stack
+ */
+function stackClear() {
+ this.__data__ = new ListCache;
+}
+
+/**
+ * Removes `key` and its value from the stack.
+ *
+ * @private
+ * @name delete
+ * @memberOf Stack
+ * @param {string} key The key of the value to remove.
+ * @returns {boolean} Returns `true` if the entry was removed, else `false`.
+ */
+function stackDelete(key) {
+ return this.__data__['delete'](key);
+}
+
+/**
+ * Gets the stack value for `key`.
+ *
+ * @private
+ * @name get
+ * @memberOf Stack
+ * @param {string} key The key of the value to get.
+ * @returns {*} Returns the entry value.
+ */
+function stackGet(key) {
+ return this.__data__.get(key);
+}
+
+/**
+ * Checks if a stack value for `key` exists.
+ *
+ * @private
+ * @name has
+ * @memberOf Stack
+ * @param {string} key The key of the entry to check.
+ * @returns {boolean} Returns `true` if an entry for `key` exists, else `false`.
+ */
+function stackHas(key) {
+ return this.__data__.has(key);
+}
+
+/**
+ * Sets the stack `key` to `value`.
+ *
+ * @private
+ * @name set
+ * @memberOf Stack
+ * @param {string} key The key of the value to set.
+ * @param {*} value The value to set.
+ * @returns {Object} Returns the stack cache instance.
+ */
+function stackSet(key, value) {
+ var cache = this.__data__;
+ if (cache instanceof ListCache) {
+ var pairs = cache.__data__;
+ if (!Map || (pairs.length < LARGE_ARRAY_SIZE - 1)) {
+ pairs.push([key, value]);
+ return this;
+ }
+ cache = this.__data__ = new MapCache(pairs);
+ }
+ cache.set(key, value);
+ return this;
+}
+
+// Add methods to `Stack`.
+Stack.prototype.clear = stackClear;
+Stack.prototype['delete'] = stackDelete;
+Stack.prototype.get = stackGet;
+Stack.prototype.has = stackHas;
+Stack.prototype.set = stackSet;
+
+/**
+ * Creates an array of the enumerable property names of the array-like `value`.
+ *
+ * @private
+ * @param {*} value The value to query.
+ * @param {boolean} inherited Specify returning inherited property names.
+ * @returns {Array} Returns the array of property names.
+ */
+function arrayLikeKeys(value, inherited) {
+ // Safari 8.1 makes `arguments.callee` enumerable in strict mode.
+ // Safari 9 makes `arguments.length` enumerable in strict mode.
+ var result = (isArray(value) || isArguments(value))
+ ? baseTimes(value.length, String)
+ : [];
+
+ var length = result.length,
+ skipIndexes = !!length;
+
+ for (var key in value) {
+ if ((inherited || hasOwnProperty.call(value, key)) &&
+ !(skipIndexes && (key == 'length' || isIndex(key, length)))) {
+ result.push(key);
+ }
+ }
+ return result;
+}
+
+/**
+ * Assigns `value` to `key` of `object` if the existing value is not equivalent
+ * using [`SameValueZero`](http://ecma-international.org/ecma-262/7.0/#sec-samevaluezero)
+ * for equality comparisons.
+ *
+ * @private
+ * @param {Object} object The object to modify.
+ * @param {string} key The key of the property to assign.
+ * @param {*} value The value to assign.
+ */
+function assignValue(object, key, value) {
+ var objValue = object[key];
+ if (!(hasOwnProperty.call(object, key) && eq(objValue, value)) ||
+ (value === undefined && !(key in object))) {
+ object[key] = value;
+ }
+}
+
+/**
+ * Gets the index at which the `key` is found in `array` of key-value pairs.
+ *
+ * @private
+ * @param {Array} array The array to inspect.
+ * @param {*} key The key to search for.
+ * @returns {number} Returns the index of the matched value, else `-1`.
+ */
+function assocIndexOf(array, key) {
+ var length = array.length;
+ while (length--) {
+ if (eq(array[length][0], key)) {
+ return length;
+ }
+ }
+ return -1;
+}
+
+/**
+ * The base implementation of `_.assign` without support for multiple sources
+ * or `customizer` functions.
+ *
+ * @private
+ * @param {Object} object The destination object.
+ * @param {Object} source The source object.
+ * @returns {Object} Returns `object`.
+ */
+function baseAssign(object, source) {
+ return object && copyObject(source, keys(source), object);
+}
+
+/**
+ * The base implementation of `_.clone` and `_.cloneDeep` which tracks
+ * traversed objects.
+ *
+ * @private
+ * @param {*} value The value to clone.
+ * @param {boolean} [isDeep] Specify a deep clone.
+ * @param {boolean} [isFull] Specify a clone including symbols.
+ * @param {Function} [customizer] The function to customize cloning.
+ * @param {string} [key] The key of `value`.
+ * @param {Object} [object] The parent object of `value`.
+ * @param {Object} [stack] Tracks traversed objects and their clone counterparts.
+ * @returns {*} Returns the cloned value.
+ */
+function baseClone(value, isDeep, isFull, customizer, key, object, stack) {
+ var result;
+ if (customizer) {
+ result = object ? customizer(value, key, object, stack) : customizer(value);
+ }
+ if (result !== undefined) {
+ return result;
+ }
+ if (!isObject(value)) {
+ return value;
+ }
+ var isArr = isArray(value);
+ if (isArr) {
+ result = initCloneArray(value);
+ if (!isDeep) {
+ return copyArray(value, result);
+ }
+ } else {
+ var tag = getTag(value),
+ isFunc = tag == funcTag || tag == genTag;
+
+ if (isBuffer(value)) {
+ return cloneBuffer(value, isDeep);
+ }
+ if (tag == objectTag || tag == argsTag || (isFunc && !object)) {
+ if (isHostObject(value)) {
+ return object ? value : {};
+ }
+ result = initCloneObject(isFunc ? {} : value);
+ if (!isDeep) {
+ return copySymbols(value, baseAssign(result, value));
+ }
+ } else {
+ if (!cloneableTags[tag]) {
+ return object ? value : {};
+ }
+ result = initCloneByTag(value, tag, baseClone, isDeep);
+ }
+ }
+ // Check for circular references and return its corresponding clone.
+ stack || (stack = new Stack);
+ var stacked = stack.get(value);
+ if (stacked) {
+ return stacked;
+ }
+ stack.set(value, result);
+
+ if (!isArr) {
+ var props = isFull ? getAllKeys(value) : keys(value);
+ }
+ arrayEach(props || value, function(subValue, key) {
+ if (props) {
+ key = subValue;
+ subValue = value[key];
+ }
+ // Recursively populate clone (susceptible to call stack limits).
+ assignValue(result, key, baseClone(subValue, isDeep, isFull, customizer, key, value, stack));
+ });
+ return result;
+}
+
+/**
+ * The base implementation of `_.create` without support for assigning
+ * properties to the created object.
+ *
+ * @private
+ * @param {Object} prototype The object to inherit from.
+ * @returns {Object} Returns the new object.
+ */
+function baseCreate(proto) {
+ return isObject(proto) ? objectCreate(proto) : {};
+}
+
+/**
+ * The base implementation of `getAllKeys` and `getAllKeysIn` which uses
+ * `keysFunc` and `symbolsFunc` to get the enumerable property names and
+ * symbols of `object`.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @param {Function} keysFunc The function to get the keys of `object`.
+ * @param {Function} symbolsFunc The function to get the symbols of `object`.
+ * @returns {Array} Returns the array of property names and symbols.
+ */
+function baseGetAllKeys(object, keysFunc, symbolsFunc) {
+ var result = keysFunc(object);
+ return isArray(object) ? result : arrayPush(result, symbolsFunc(object));
+}
+
+/**
+ * The base implementation of `getTag`.
+ *
+ * @private
+ * @param {*} value The value to query.
+ * @returns {string} Returns the `toStringTag`.
+ */
+function baseGetTag(value) {
+ return objectToString.call(value);
+}
+
+/**
+ * The base implementation of `_.isNative` without bad shim checks.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a native function,
+ * else `false`.
+ */
+function baseIsNative(value) {
+ if (!isObject(value) || isMasked(value)) {
+ return false;
+ }
+ var pattern = (isFunction(value) || isHostObject(value)) ? reIsNative : reIsHostCtor;
+ return pattern.test(toSource(value));
+}
+
+/**
+ * The base implementation of `_.keys` which doesn't treat sparse arrays as dense.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the array of property names.
+ */
+function baseKeys(object) {
+ if (!isPrototype(object)) {
+ return nativeKeys(object);
+ }
+ var result = [];
+ for (var key in Object(object)) {
+ if (hasOwnProperty.call(object, key) && key != 'constructor') {
+ result.push(key);
+ }
+ }
+ return result;
+}
+
+/**
+ * Creates a clone of `buffer`.
+ *
+ * @private
+ * @param {Buffer} buffer The buffer to clone.
+ * @param {boolean} [isDeep] Specify a deep clone.
+ * @returns {Buffer} Returns the cloned buffer.
+ */
+function cloneBuffer(buffer, isDeep) {
+ if (isDeep) {
+ return buffer.slice();
+ }
+ var result = new buffer.constructor(buffer.length);
+ buffer.copy(result);
+ return result;
+}
+
+/**
+ * Creates a clone of `arrayBuffer`.
+ *
+ * @private
+ * @param {ArrayBuffer} arrayBuffer The array buffer to clone.
+ * @returns {ArrayBuffer} Returns the cloned array buffer.
+ */
+function cloneArrayBuffer(arrayBuffer) {
+ var result = new arrayBuffer.constructor(arrayBuffer.byteLength);
+ new Uint8Array(result).set(new Uint8Array(arrayBuffer));
+ return result;
+}
+
+/**
+ * Creates a clone of `dataView`.
+ *
+ * @private
+ * @param {Object} dataView The data view to clone.
+ * @param {boolean} [isDeep] Specify a deep clone.
+ * @returns {Object} Returns the cloned data view.
+ */
+function cloneDataView(dataView, isDeep) {
+ var buffer = isDeep ? cloneArrayBuffer(dataView.buffer) : dataView.buffer;
+ return new dataView.constructor(buffer, dataView.byteOffset, dataView.byteLength);
+}
+
+/**
+ * Creates a clone of `map`.
+ *
+ * @private
+ * @param {Object} map The map to clone.
+ * @param {Function} cloneFunc The function to clone values.
+ * @param {boolean} [isDeep] Specify a deep clone.
+ * @returns {Object} Returns the cloned map.
+ */
+function cloneMap(map, isDeep, cloneFunc) {
+ var array = isDeep ? cloneFunc(mapToArray(map), true) : mapToArray(map);
+ return arrayReduce(array, addMapEntry, new map.constructor);
+}
+
+/**
+ * Creates a clone of `regexp`.
+ *
+ * @private
+ * @param {Object} regexp The regexp to clone.
+ * @returns {Object} Returns the cloned regexp.
+ */
+function cloneRegExp(regexp) {
+ var result = new regexp.constructor(regexp.source, reFlags.exec(regexp));
+ result.lastIndex = regexp.lastIndex;
+ return result;
+}
+
+/**
+ * Creates a clone of `set`.
+ *
+ * @private
+ * @param {Object} set The set to clone.
+ * @param {Function} cloneFunc The function to clone values.
+ * @param {boolean} [isDeep] Specify a deep clone.
+ * @returns {Object} Returns the cloned set.
+ */
+function cloneSet(set, isDeep, cloneFunc) {
+ var array = isDeep ? cloneFunc(setToArray(set), true) : setToArray(set);
+ return arrayReduce(array, addSetEntry, new set.constructor);
+}
+
+/**
+ * Creates a clone of the `symbol` object.
+ *
+ * @private
+ * @param {Object} symbol The symbol object to clone.
+ * @returns {Object} Returns the cloned symbol object.
+ */
+function cloneSymbol(symbol) {
+ return symbolValueOf ? Object(symbolValueOf.call(symbol)) : {};
+}
+
+/**
+ * Creates a clone of `typedArray`.
+ *
+ * @private
+ * @param {Object} typedArray The typed array to clone.
+ * @param {boolean} [isDeep] Specify a deep clone.
+ * @returns {Object} Returns the cloned typed array.
+ */
+function cloneTypedArray(typedArray, isDeep) {
+ var buffer = isDeep ? cloneArrayBuffer(typedArray.buffer) : typedArray.buffer;
+ return new typedArray.constructor(buffer, typedArray.byteOffset, typedArray.length);
+}
+
+/**
+ * Copies the values of `source` to `array`.
+ *
+ * @private
+ * @param {Array} source The array to copy values from.
+ * @param {Array} [array=[]] The array to copy values to.
+ * @returns {Array} Returns `array`.
+ */
+function copyArray(source, array) {
+ var index = -1,
+ length = source.length;
+
+ array || (array = Array(length));
+ while (++index < length) {
+ array[index] = source[index];
+ }
+ return array;
+}
+
+/**
+ * Copies properties of `source` to `object`.
+ *
+ * @private
+ * @param {Object} source The object to copy properties from.
+ * @param {Array} props The property identifiers to copy.
+ * @param {Object} [object={}] The object to copy properties to.
+ * @param {Function} [customizer] The function to customize copied values.
+ * @returns {Object} Returns `object`.
+ */
+function copyObject(source, props, object, customizer) {
+ object || (object = {});
+
+ var index = -1,
+ length = props.length;
+
+ while (++index < length) {
+ var key = props[index];
+
+ var newValue = customizer
+ ? customizer(object[key], source[key], key, object, source)
+ : undefined;
+
+ assignValue(object, key, newValue === undefined ? source[key] : newValue);
+ }
+ return object;
+}
+
+/**
+ * Copies own symbol properties of `source` to `object`.
+ *
+ * @private
+ * @param {Object} source The object to copy symbols from.
+ * @param {Object} [object={}] The object to copy symbols to.
+ * @returns {Object} Returns `object`.
+ */
+function copySymbols(source, object) {
+ return copyObject(source, getSymbols(source), object);
+}
+
+/**
+ * Creates an array of own enumerable property names and symbols of `object`.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the array of property names and symbols.
+ */
+function getAllKeys(object) {
+ return baseGetAllKeys(object, keys, getSymbols);
+}
+
+/**
+ * Gets the data for `map`.
+ *
+ * @private
+ * @param {Object} map The map to query.
+ * @param {string} key The reference key.
+ * @returns {*} Returns the map data.
+ */
+function getMapData(map, key) {
+ var data = map.__data__;
+ return isKeyable(key)
+ ? data[typeof key == 'string' ? 'string' : 'hash']
+ : data.map;
+}
+
+/**
+ * Gets the native function at `key` of `object`.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @param {string} key The key of the method to get.
+ * @returns {*} Returns the function if it's native, else `undefined`.
+ */
+function getNative(object, key) {
+ var value = getValue(object, key);
+ return baseIsNative(value) ? value : undefined;
+}
+
+/**
+ * Creates an array of the own enumerable symbol properties of `object`.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the array of symbols.
+ */
+var getSymbols = nativeGetSymbols ? overArg(nativeGetSymbols, Object) : stubArray;
+
+/**
+ * Gets the `toStringTag` of `value`.
+ *
+ * @private
+ * @param {*} value The value to query.
+ * @returns {string} Returns the `toStringTag`.
+ */
+var getTag = baseGetTag;
+
+// Fallback for data views, maps, sets, and weak maps in IE 11,
+// for data views in Edge < 14, and promises in Node.js.
+if ((DataView && getTag(new DataView(new ArrayBuffer(1))) != dataViewTag) ||
+ (Map && getTag(new Map) != mapTag) ||
+ (Promise && getTag(Promise.resolve()) != promiseTag) ||
+ (Set && getTag(new Set) != setTag) ||
+ (WeakMap && getTag(new WeakMap) != weakMapTag)) {
+ getTag = function(value) {
+ var result = objectToString.call(value),
+ Ctor = result == objectTag ? value.constructor : undefined,
+ ctorString = Ctor ? toSource(Ctor) : undefined;
+
+ if (ctorString) {
+ switch (ctorString) {
+ case dataViewCtorString: return dataViewTag;
+ case mapCtorString: return mapTag;
+ case promiseCtorString: return promiseTag;
+ case setCtorString: return setTag;
+ case weakMapCtorString: return weakMapTag;
+ }
+ }
+ return result;
+ };
+}
+
+/**
+ * Initializes an array clone.
+ *
+ * @private
+ * @param {Array} array The array to clone.
+ * @returns {Array} Returns the initialized clone.
+ */
+function initCloneArray(array) {
+ var length = array.length,
+ result = array.constructor(length);
+
+ // Add properties assigned by `RegExp#exec`.
+ if (length && typeof array[0] == 'string' && hasOwnProperty.call(array, 'index')) {
+ result.index = array.index;
+ result.input = array.input;
+ }
+ return result;
+}
+
+/**
+ * Initializes an object clone.
+ *
+ * @private
+ * @param {Object} object The object to clone.
+ * @returns {Object} Returns the initialized clone.
+ */
+function initCloneObject(object) {
+ return (typeof object.constructor == 'function' && !isPrototype(object))
+ ? baseCreate(getPrototype(object))
+ : {};
+}
+
+/**
+ * Initializes an object clone based on its `toStringTag`.
+ *
+ * **Note:** This function only supports cloning values with tags of
+ * `Boolean`, `Date`, `Error`, `Number`, `RegExp`, or `String`.
+ *
+ * @private
+ * @param {Object} object The object to clone.
+ * @param {string} tag The `toStringTag` of the object to clone.
+ * @param {Function} cloneFunc The function to clone values.
+ * @param {boolean} [isDeep] Specify a deep clone.
+ * @returns {Object} Returns the initialized clone.
+ */
+function initCloneByTag(object, tag, cloneFunc, isDeep) {
+ var Ctor = object.constructor;
+ switch (tag) {
+ case arrayBufferTag:
+ return cloneArrayBuffer(object);
+
+ case boolTag:
+ case dateTag:
+ return new Ctor(+object);
+
+ case dataViewTag:
+ return cloneDataView(object, isDeep);
+
+ case float32Tag: case float64Tag:
+ case int8Tag: case int16Tag: case int32Tag:
+ case uint8Tag: case uint8ClampedTag: case uint16Tag: case uint32Tag:
+ return cloneTypedArray(object, isDeep);
+
+ case mapTag:
+ return cloneMap(object, isDeep, cloneFunc);
+
+ case numberTag:
+ case stringTag:
+ return new Ctor(object);
+
+ case regexpTag:
+ return cloneRegExp(object);
+
+ case setTag:
+ return cloneSet(object, isDeep, cloneFunc);
+
+ case symbolTag:
+ return cloneSymbol(object);
+ }
+}
+
+/**
+ * Checks if `value` is a valid array-like index.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @param {number} [length=MAX_SAFE_INTEGER] The upper bounds of a valid index.
+ * @returns {boolean} Returns `true` if `value` is a valid index, else `false`.
+ */
+function isIndex(value, length) {
+ length = length == null ? MAX_SAFE_INTEGER : length;
+ return !!length &&
+ (typeof value == 'number' || reIsUint.test(value)) &&
+ (value > -1 && value % 1 == 0 && value < length);
+}
+
+/**
+ * Checks if `value` is suitable for use as unique object key.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is suitable, else `false`.
+ */
+function isKeyable(value) {
+ var type = typeof value;
+ return (type == 'string' || type == 'number' || type == 'symbol' || type == 'boolean')
+ ? (value !== '__proto__')
+ : (value === null);
+}
+
+/**
+ * Checks if `func` has its source masked.
+ *
+ * @private
+ * @param {Function} func The function to check.
+ * @returns {boolean} Returns `true` if `func` is masked, else `false`.
+ */
+function isMasked(func) {
+ return !!maskSrcKey && (maskSrcKey in func);
+}
+
+/**
+ * Checks if `value` is likely a prototype object.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a prototype, else `false`.
+ */
+function isPrototype(value) {
+ var Ctor = value && value.constructor,
+ proto = (typeof Ctor == 'function' && Ctor.prototype) || objectProto;
+
+ return value === proto;
+}
+
+/**
+ * Converts `func` to its source code.
+ *
+ * @private
+ * @param {Function} func The function to process.
+ * @returns {string} Returns the source code.
+ */
+function toSource(func) {
+ if (func != null) {
+ try {
+ return funcToString.call(func);
+ } catch (e) {}
+ try {
+ return (func + '');
+ } catch (e) {}
+ }
+ return '';
+}
+
+/**
+ * This method is like `_.clone` except that it recursively clones `value`.
+ *
+ * @static
+ * @memberOf _
+ * @since 1.0.0
+ * @category Lang
+ * @param {*} value The value to recursively clone.
+ * @returns {*} Returns the deep cloned value.
+ * @see _.clone
+ * @example
+ *
+ * var objects = [{ 'a': 1 }, { 'b': 2 }];
+ *
+ * var deep = _.cloneDeep(objects);
+ * console.log(deep[0] === objects[0]);
+ * // => false
+ */
+function cloneDeep(value) {
+ return baseClone(value, true, true);
+}
+
+/**
+ * Performs a
+ * [`SameValueZero`](http://ecma-international.org/ecma-262/7.0/#sec-samevaluezero)
+ * comparison between two values to determine if they are equivalent.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to compare.
+ * @param {*} other The other value to compare.
+ * @returns {boolean} Returns `true` if the values are equivalent, else `false`.
+ * @example
+ *
+ * var object = { 'a': 1 };
+ * var other = { 'a': 1 };
+ *
+ * _.eq(object, object);
+ * // => true
+ *
+ * _.eq(object, other);
+ * // => false
+ *
+ * _.eq('a', 'a');
+ * // => true
+ *
+ * _.eq('a', Object('a'));
+ * // => false
+ *
+ * _.eq(NaN, NaN);
+ * // => true
+ */
+function eq(value, other) {
+ return value === other || (value !== value && other !== other);
+}
+
+/**
+ * Checks if `value` is likely an `arguments` object.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is an `arguments` object,
+ * else `false`.
+ * @example
+ *
+ * _.isArguments(function() { return arguments; }());
+ * // => true
+ *
+ * _.isArguments([1, 2, 3]);
+ * // => false
+ */
+function isArguments(value) {
+ // Safari 8.1 makes `arguments.callee` enumerable in strict mode.
+ return isArrayLikeObject(value) && hasOwnProperty.call(value, 'callee') &&
+ (!propertyIsEnumerable.call(value, 'callee') || objectToString.call(value) == argsTag);
+}
+
+/**
+ * Checks if `value` is classified as an `Array` object.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is an array, else `false`.
+ * @example
+ *
+ * _.isArray([1, 2, 3]);
+ * // => true
+ *
+ * _.isArray(document.body.children);
+ * // => false
+ *
+ * _.isArray('abc');
+ * // => false
+ *
+ * _.isArray(_.noop);
+ * // => false
+ */
+var isArray = Array.isArray;
+
+/**
+ * Checks if `value` is array-like. A value is considered array-like if it's
+ * not a function and has a `value.length` that's an integer greater than or
+ * equal to `0` and less than or equal to `Number.MAX_SAFE_INTEGER`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is array-like, else `false`.
+ * @example
+ *
+ * _.isArrayLike([1, 2, 3]);
+ * // => true
+ *
+ * _.isArrayLike(document.body.children);
+ * // => true
+ *
+ * _.isArrayLike('abc');
+ * // => true
+ *
+ * _.isArrayLike(_.noop);
+ * // => false
+ */
+function isArrayLike(value) {
+ return value != null && isLength(value.length) && !isFunction(value);
+}
+
+/**
+ * This method is like `_.isArrayLike` except that it also checks if `value`
+ * is an object.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is an array-like object,
+ * else `false`.
+ * @example
+ *
+ * _.isArrayLikeObject([1, 2, 3]);
+ * // => true
+ *
+ * _.isArrayLikeObject(document.body.children);
+ * // => true
+ *
+ * _.isArrayLikeObject('abc');
+ * // => false
+ *
+ * _.isArrayLikeObject(_.noop);
+ * // => false
+ */
+function isArrayLikeObject(value) {
+ return isObjectLike(value) && isArrayLike(value);
+}
+
+/**
+ * Checks if `value` is a buffer.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.3.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a buffer, else `false`.
+ * @example
+ *
+ * _.isBuffer(new Buffer(2));
+ * // => true
+ *
+ * _.isBuffer(new Uint8Array(2));
+ * // => false
+ */
+var isBuffer = nativeIsBuffer || stubFalse;
+
+/**
+ * Checks if `value` is classified as a `Function` object.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a function, else `false`.
+ * @example
+ *
+ * _.isFunction(_);
+ * // => true
+ *
+ * _.isFunction(/abc/);
+ * // => false
+ */
+function isFunction(value) {
+ // The use of `Object#toString` avoids issues with the `typeof` operator
+ // in Safari 8-9 which returns 'object' for typed array and other constructors.
+ var tag = isObject(value) ? objectToString.call(value) : '';
+ return tag == funcTag || tag == genTag;
+}
+
+/**
+ * Checks if `value` is a valid array-like length.
+ *
+ * **Note:** This method is loosely based on
+ * [`ToLength`](http://ecma-international.org/ecma-262/7.0/#sec-tolength).
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a valid length, else `false`.
+ * @example
+ *
+ * _.isLength(3);
+ * // => true
+ *
+ * _.isLength(Number.MIN_VALUE);
+ * // => false
+ *
+ * _.isLength(Infinity);
+ * // => false
+ *
+ * _.isLength('3');
+ * // => false
+ */
+function isLength(value) {
+ return typeof value == 'number' &&
+ value > -1 && value % 1 == 0 && value <= MAX_SAFE_INTEGER;
+}
+
+/**
+ * Checks if `value` is the
+ * [language type](http://www.ecma-international.org/ecma-262/7.0/#sec-ecmascript-language-types)
+ * of `Object`. (e.g. arrays, functions, objects, regexes, `new Number(0)`, and `new String('')`)
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is an object, else `false`.
+ * @example
+ *
+ * _.isObject({});
+ * // => true
+ *
+ * _.isObject([1, 2, 3]);
+ * // => true
+ *
+ * _.isObject(_.noop);
+ * // => true
+ *
+ * _.isObject(null);
+ * // => false
+ */
+function isObject(value) {
+ var type = typeof value;
+ return !!value && (type == 'object' || type == 'function');
+}
+
+/**
+ * Checks if `value` is object-like. A value is object-like if it's not `null`
+ * and has a `typeof` result of "object".
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is object-like, else `false`.
+ * @example
+ *
+ * _.isObjectLike({});
+ * // => true
+ *
+ * _.isObjectLike([1, 2, 3]);
+ * // => true
+ *
+ * _.isObjectLike(_.noop);
+ * // => false
+ *
+ * _.isObjectLike(null);
+ * // => false
+ */
+function isObjectLike(value) {
+ return !!value && typeof value == 'object';
+}
+
+/**
+ * Creates an array of the own enumerable property names of `object`.
+ *
+ * **Note:** Non-object values are coerced to objects. See the
+ * [ES spec](http://ecma-international.org/ecma-262/7.0/#sec-object.keys)
+ * for more details.
+ *
+ * @static
+ * @since 0.1.0
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the array of property names.
+ * @example
+ *
+ * function Foo() {
+ * this.a = 1;
+ * this.b = 2;
+ * }
+ *
+ * Foo.prototype.c = 3;
+ *
+ * _.keys(new Foo);
+ * // => ['a', 'b'] (iteration order is not guaranteed)
+ *
+ * _.keys('hi');
+ * // => ['0', '1']
+ */
+function keys(object) {
+ return isArrayLike(object) ? arrayLikeKeys(object) : baseKeys(object);
+}
+
+/**
+ * This method returns a new empty array.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.13.0
+ * @category Util
+ * @returns {Array} Returns the new empty array.
+ * @example
+ *
+ * var arrays = _.times(2, _.stubArray);
+ *
+ * console.log(arrays);
+ * // => [[], []]
+ *
+ * console.log(arrays[0] === arrays[1]);
+ * // => false
+ */
+function stubArray() {
+ return [];
+}
+
+/**
+ * This method returns `false`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.13.0
+ * @category Util
+ * @returns {boolean} Returns `false`.
+ * @example
+ *
+ * _.times(2, _.stubFalse);
+ * // => [false, false]
+ */
+function stubFalse() {
+ return false;
+}
+
+module.exports = cloneDeep;
diff --git a/node_modules/lodash.clonedeep/package.json b/node_modules/lodash.clonedeep/package.json
new file mode 100644
index 0000000..dafa690
--- /dev/null
+++ b/node_modules/lodash.clonedeep/package.json
@@ -0,0 +1,114 @@
+{
+ "_args": [
+ [
+ {
+ "raw": "lodash.clonedeep@^4.3.2",
+ "scope": null,
+ "escapedName": "lodash.clonedeep",
+ "name": "lodash.clonedeep",
+ "rawSpec": "^4.3.2",
+ "spec": ">=4.3.2 <5.0.0",
+ "type": "range"
+ },
+ "/Users/ramswaroop/MyProjects/RamAndAntara/node_modules/gulp-sass"
+ ]
+ ],
+ "_from": "lodash.clonedeep@>=4.3.2 <5.0.0",
+ "_id": "lodash.clonedeep@4.5.0",
+ "_inCache": true,
+ "_location": "/lodash.clonedeep",
+ "_nodeVersion": "4.4.7",
+ "_npmOperationalInternal": {
+ "host": "packages-12-west.internal.npmjs.com",
+ "tmp": "tmp/lodash.clonedeep-4.5.0.tgz_1471109839710_0.7944763591513038"
+ },
+ "_npmUser": {
+ "name": "jdalton",
+ "email": "john.david.dalton@gmail.com"
+ },
+ "_npmVersion": "2.15.10",
+ "_phantomChildren": {},
+ "_requested": {
+ "raw": "lodash.clonedeep@^4.3.2",
+ "scope": null,
+ "escapedName": "lodash.clonedeep",
+ "name": "lodash.clonedeep",
+ "rawSpec": "^4.3.2",
+ "spec": ">=4.3.2 <5.0.0",
+ "type": "range"
+ },
+ "_requiredBy": [
+ "/gulp-sass",
+ "/node-sass"
+ ],
+ "_resolved": "https://registry.npmjs.org/lodash.clonedeep/-/lodash.clonedeep-4.5.0.tgz",
+ "_shasum": "e23f3f9c4f8fbdde872529c1071857a086e5ccef",
+ "_shrinkwrap": null,
+ "_spec": "lodash.clonedeep@^4.3.2",
+ "_where": "/Users/ramswaroop/MyProjects/RamAndAntara/node_modules/gulp-sass",
+ "author": {
+ "name": "John-David Dalton",
+ "email": "john.david.dalton@gmail.com",
+ "url": "http://allyoucanleet.com/"
+ },
+ "bugs": {
+ "url": "https://github.com/lodash/lodash/issues"
+ },
+ "contributors": [
+ {
+ "name": "John-David Dalton",
+ "email": "john.david.dalton@gmail.com",
+ "url": "http://allyoucanleet.com/"
+ },
+ {
+ "name": "Blaine Bublitz",
+ "email": "blaine.bublitz@gmail.com",
+ "url": "https://github.com/phated"
+ },
+ {
+ "name": "Mathias Bynens",
+ "email": "mathias@qiwi.be",
+ "url": "https://mathiasbynens.be/"
+ }
+ ],
+ "dependencies": {},
+ "description": "The lodash method `_.cloneDeep` exported as a module.",
+ "devDependencies": {},
+ "directories": {},
+ "dist": {
+ "shasum": "e23f3f9c4f8fbdde872529c1071857a086e5ccef",
+ "tarball": "https://registry.npmjs.org/lodash.clonedeep/-/lodash.clonedeep-4.5.0.tgz"
+ },
+ "homepage": "https://lodash.com/",
+ "icon": "https://lodash.com/icon.svg",
+ "keywords": [
+ "lodash-modularized",
+ "clonedeep"
+ ],
+ "license": "MIT",
+ "maintainers": [
+ {
+ "name": "jdalton",
+ "email": "john.david.dalton@gmail.com"
+ },
+ {
+ "name": "mathias",
+ "email": "mathias@qiwi.be"
+ },
+ {
+ "name": "phated",
+ "email": "blaine@iceddev.com"
+ }
+ ],
+ "name": "lodash.clonedeep",
+ "optionalDependencies": {},
+ "readme": "ERROR: No README data found!",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/lodash/lodash.git"
+ },
+ "scripts": {
+ "test": "echo \"See https://travis-ci.org/lodash/lodash-cli for testing details.\""
+ },
+ "version": "4.5.0"
+}
diff --git a/node_modules/lodash.escape/LICENSE b/node_modules/lodash.escape/LICENSE
new file mode 100644
index 0000000..b054ca5
--- /dev/null
+++ b/node_modules/lodash.escape/LICENSE
@@ -0,0 +1,22 @@
+Copyright 2012-2016 The Dojo Foundation
+Based on Underscore.js, copyright 2009-2016 Jeremy Ashkenas,
+DocumentCloud and Investigative Reporters & Editors
+
+Permission is hereby granted, free of charge, to any person obtaining
+a copy of this software and associated documentation files (the
+"Software"), to deal in the Software without restriction, including
+without limitation the rights to use, copy, modify, merge, publish,
+distribute, sublicense, and/or sell copies of the Software, and to
+permit persons to whom the Software is furnished to do so, subject to
+the following conditions:
+
+The above copyright notice and this permission notice shall be
+included in all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
+EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
+MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
+NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
+LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
+OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
+WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
diff --git a/node_modules/lodash.escape/README.md b/node_modules/lodash.escape/README.md
new file mode 100644
index 0000000..b012def
--- /dev/null
+++ b/node_modules/lodash.escape/README.md
@@ -0,0 +1,18 @@
+# lodash.escape v3.2.0
+
+The [lodash](https://lodash.com/) method `_.escape` exported as a [Node.js](https://nodejs.org/) module.
+
+## Installation
+
+Using npm:
+```bash
+$ {sudo -H} npm i -g npm
+$ npm i --save lodash.escape
+```
+
+In Node.js:
+```js
+var escape = require('lodash.escape');
+```
+
+See the [documentation](https://lodash.com/docs#escape) or [package source](https://github.com/lodash/lodash/blob/3.2.0-npm-packages/lodash.escape) for more details.
diff --git a/node_modules/lodash.escape/index.js b/node_modules/lodash.escape/index.js
new file mode 100644
index 0000000..0971ae8
--- /dev/null
+++ b/node_modules/lodash.escape/index.js
@@ -0,0 +1,180 @@
+/**
+ * lodash 3.2.0 (Custom Build)
+ * Build: `lodash modularize exports="npm" -o ./`
+ * Copyright 2012-2016 The Dojo Foundation
+ * Based on Underscore.js 1.8.3
+ * Copyright 2009-2016 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license
+ */
+var root = require('lodash._root');
+
+/** Used as references for various `Number` constants. */
+var INFINITY = 1 / 0;
+
+/** `Object#toString` result references. */
+var symbolTag = '[object Symbol]';
+
+/** Used to match HTML entities and HTML characters. */
+var reUnescapedHtml = /[&<>"'`]/g,
+ reHasUnescapedHtml = RegExp(reUnescapedHtml.source);
+
+/** Used to map characters to HTML entities. */
+var htmlEscapes = {
+ '&': '&',
+ '<': '<',
+ '>': '>',
+ '"': '"',
+ "'": ''',
+ '`': '`'
+};
+
+/**
+ * Used by `_.escape` to convert characters to HTML entities.
+ *
+ * @private
+ * @param {string} chr The matched character to escape.
+ * @returns {string} Returns the escaped character.
+ */
+function escapeHtmlChar(chr) {
+ return htmlEscapes[chr];
+}
+
+/** Used for built-in method references. */
+var objectProto = Object.prototype;
+
+/**
+ * Used to resolve the [`toStringTag`](http://ecma-international.org/ecma-262/6.0/#sec-object.prototype.tostring)
+ * of values.
+ */
+var objectToString = objectProto.toString;
+
+/** Built-in value references. */
+var Symbol = root.Symbol;
+
+/** Used to convert symbols to primitives and strings. */
+var symbolProto = Symbol ? Symbol.prototype : undefined,
+ symbolToString = Symbol ? symbolProto.toString : undefined;
+
+/**
+ * Checks if `value` is object-like. A value is object-like if it's not `null`
+ * and has a `typeof` result of "object".
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is object-like, else `false`.
+ * @example
+ *
+ * _.isObjectLike({});
+ * // => true
+ *
+ * _.isObjectLike([1, 2, 3]);
+ * // => true
+ *
+ * _.isObjectLike(_.noop);
+ * // => false
+ *
+ * _.isObjectLike(null);
+ * // => false
+ */
+function isObjectLike(value) {
+ return !!value && typeof value == 'object';
+}
+
+/**
+ * Checks if `value` is classified as a `Symbol` primitive or object.
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is correctly classified, else `false`.
+ * @example
+ *
+ * _.isSymbol(Symbol.iterator);
+ * // => true
+ *
+ * _.isSymbol('abc');
+ * // => false
+ */
+function isSymbol(value) {
+ return typeof value == 'symbol' ||
+ (isObjectLike(value) && objectToString.call(value) == symbolTag);
+}
+
+/**
+ * Converts `value` to a string if it's not one. An empty string is returned
+ * for `null` and `undefined` values. The sign of `-0` is preserved.
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to process.
+ * @returns {string} Returns the string.
+ * @example
+ *
+ * _.toString(null);
+ * // => ''
+ *
+ * _.toString(-0);
+ * // => '-0'
+ *
+ * _.toString([1, 2, 3]);
+ * // => '1,2,3'
+ */
+function toString(value) {
+ // Exit early for strings to avoid a performance hit in some environments.
+ if (typeof value == 'string') {
+ return value;
+ }
+ if (value == null) {
+ return '';
+ }
+ if (isSymbol(value)) {
+ return Symbol ? symbolToString.call(value) : '';
+ }
+ var result = (value + '');
+ return (result == '0' && (1 / value) == -INFINITY) ? '-0' : result;
+}
+
+/**
+ * Converts the characters "&", "<", ">", '"', "'", and "\`" in `string` to
+ * their corresponding HTML entities.
+ *
+ * **Note:** No other characters are escaped. To escape additional
+ * characters use a third-party library like [_he_](https://mths.be/he).
+ *
+ * Though the ">" character is escaped for symmetry, characters like
+ * ">" and "/" don't need escaping in HTML and have no special meaning
+ * unless they're part of a tag or unquoted attribute value.
+ * See [Mathias Bynens's article](https://mathiasbynens.be/notes/ambiguous-ampersands)
+ * (under "semi-related fun fact") for more details.
+ *
+ * Backticks are escaped because in IE < 9, they can break out of
+ * attribute values or HTML comments. See [#59](https://html5sec.org/#59),
+ * [#102](https://html5sec.org/#102), [#108](https://html5sec.org/#108), and
+ * [#133](https://html5sec.org/#133) of the [HTML5 Security Cheatsheet](https://html5sec.org/)
+ * for more details.
+ *
+ * When working with HTML you should always [quote attribute values](http://wonko.com/post/html-escaping)
+ * to reduce XSS vectors.
+ *
+ * @static
+ * @memberOf _
+ * @category String
+ * @param {string} [string=''] The string to escape.
+ * @returns {string} Returns the escaped string.
+ * @example
+ *
+ * _.escape('fred, barney, & pebbles');
+ * // => 'fred, barney, & pebbles'
+ */
+function escape(string) {
+ string = toString(string);
+ return (string && reHasUnescapedHtml.test(string))
+ ? string.replace(reUnescapedHtml, escapeHtmlChar)
+ : string;
+}
+
+module.exports = escape;
diff --git a/node_modules/lodash.escape/package.json b/node_modules/lodash.escape/package.json
new file mode 100644
index 0000000..972960a
--- /dev/null
+++ b/node_modules/lodash.escape/package.json
@@ -0,0 +1,73 @@
+{
+ "_from": "lodash.escape@^3.0.0",
+ "_id": "lodash.escape@3.2.0",
+ "_inBundle": false,
+ "_integrity": "sha1-mV7g3BjBtIzJLv+ucaEKq1tIdpg=",
+ "_location": "/lodash.escape",
+ "_phantomChildren": {},
+ "_requested": {
+ "type": "range",
+ "registry": true,
+ "raw": "lodash.escape@^3.0.0",
+ "name": "lodash.escape",
+ "escapedName": "lodash.escape",
+ "rawSpec": "^3.0.0",
+ "saveSpec": null,
+ "fetchSpec": "^3.0.0"
+ },
+ "_requiredBy": [
+ "/lodash.template",
+ "/lodash.templatesettings"
+ ],
+ "_resolved": "https://registry.npmjs.org/lodash.escape/-/lodash.escape-3.2.0.tgz",
+ "_shasum": "995ee0dc18c1b48cc92effae71a10aab5b487698",
+ "_spec": "lodash.escape@^3.0.0",
+ "_where": "/Users/rpatra16/MyProjects/RamAndAntara/node_modules/lodash.template",
+ "author": {
+ "name": "John-David Dalton",
+ "email": "john.david.dalton@gmail.com",
+ "url": "http://allyoucanleet.com/"
+ },
+ "bugs": {
+ "url": "https://github.com/lodash/lodash/issues"
+ },
+ "bundleDependencies": false,
+ "contributors": [
+ {
+ "name": "John-David Dalton",
+ "email": "john.david.dalton@gmail.com",
+ "url": "http://allyoucanleet.com/"
+ },
+ {
+ "name": "Blaine Bublitz",
+ "email": "blaine@iceddev.com",
+ "url": "https://github.com/phated"
+ },
+ {
+ "name": "Mathias Bynens",
+ "email": "mathias@qiwi.be",
+ "url": "https://mathiasbynens.be/"
+ }
+ ],
+ "dependencies": {
+ "lodash._root": "^3.0.0"
+ },
+ "deprecated": false,
+ "description": "The lodash method `_.escape` exported as a module.",
+ "homepage": "https://lodash.com/",
+ "icon": "https://lodash.com/icon.svg",
+ "keywords": [
+ "lodash-modularized",
+ "escape"
+ ],
+ "license": "MIT",
+ "name": "lodash.escape",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/lodash/lodash.git"
+ },
+ "scripts": {
+ "test": "echo \"See https://travis-ci.org/lodash/lodash-cli for testing details.\""
+ },
+ "version": "3.2.0"
+}
diff --git a/node_modules/lodash.isarguments/LICENSE b/node_modules/lodash.isarguments/LICENSE
new file mode 100644
index 0000000..e0c69d5
--- /dev/null
+++ b/node_modules/lodash.isarguments/LICENSE
@@ -0,0 +1,47 @@
+Copyright jQuery Foundation and other contributors
+
+Based on Underscore.js, copyright Jeremy Ashkenas,
+DocumentCloud and Investigative Reporters & Editors
+
+This software consists of voluntary contributions made by many
+individuals. For exact contribution history, see the revision history
+available at https://github.com/lodash/lodash
+
+The following license applies to all parts of this software except as
+documented below:
+
+====
+
+Permission is hereby granted, free of charge, to any person obtaining
+a copy of this software and associated documentation files (the
+"Software"), to deal in the Software without restriction, including
+without limitation the rights to use, copy, modify, merge, publish,
+distribute, sublicense, and/or sell copies of the Software, and to
+permit persons to whom the Software is furnished to do so, subject to
+the following conditions:
+
+The above copyright notice and this permission notice shall be
+included in all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
+EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
+MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
+NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
+LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
+OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
+WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
+
+====
+
+Copyright and related rights for sample code are waived via CC0. Sample
+code is defined as all source code displayed within the prose of the
+documentation.
+
+CC0: http://creativecommons.org/publicdomain/zero/1.0/
+
+====
+
+Files located in the node_modules and vendor directories are externally
+maintained libraries used by this software which have their own
+licenses; we recommend you read them, as their terms may differ from the
+terms above.
diff --git a/node_modules/lodash.isarguments/README.md b/node_modules/lodash.isarguments/README.md
new file mode 100644
index 0000000..eb95fe1
--- /dev/null
+++ b/node_modules/lodash.isarguments/README.md
@@ -0,0 +1,18 @@
+# lodash.isarguments v3.1.0
+
+The [lodash](https://lodash.com/) method `_.isArguments` exported as a [Node.js](https://nodejs.org/) module.
+
+## Installation
+
+Using npm:
+```bash
+$ {sudo -H} npm i -g npm
+$ npm i --save lodash.isarguments
+```
+
+In Node.js:
+```js
+var isArguments = require('lodash.isarguments');
+```
+
+See the [documentation](https://lodash.com/docs#isArguments) or [package source](https://github.com/lodash/lodash/blob/3.1.0-npm-packages/lodash.isarguments) for more details.
diff --git a/node_modules/lodash.isarguments/index.js b/node_modules/lodash.isarguments/index.js
new file mode 100644
index 0000000..042dac5
--- /dev/null
+++ b/node_modules/lodash.isarguments/index.js
@@ -0,0 +1,229 @@
+/**
+ * lodash (Custom Build)
+ * Build: `lodash modularize exports="npm" -o ./`
+ * Copyright jQuery Foundation and other contributors
+ * Released under MIT license
+ * Based on Underscore.js 1.8.3
+ * Copyright Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ */
+
+/** Used as references for various `Number` constants. */
+var MAX_SAFE_INTEGER = 9007199254740991;
+
+/** `Object#toString` result references. */
+var argsTag = '[object Arguments]',
+ funcTag = '[object Function]',
+ genTag = '[object GeneratorFunction]';
+
+/** Used for built-in method references. */
+var objectProto = Object.prototype;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Used to resolve the
+ * [`toStringTag`](http://ecma-international.org/ecma-262/7.0/#sec-object.prototype.tostring)
+ * of values.
+ */
+var objectToString = objectProto.toString;
+
+/** Built-in value references. */
+var propertyIsEnumerable = objectProto.propertyIsEnumerable;
+
+/**
+ * Checks if `value` is likely an `arguments` object.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is an `arguments` object,
+ * else `false`.
+ * @example
+ *
+ * _.isArguments(function() { return arguments; }());
+ * // => true
+ *
+ * _.isArguments([1, 2, 3]);
+ * // => false
+ */
+function isArguments(value) {
+ // Safari 8.1 makes `arguments.callee` enumerable in strict mode.
+ return isArrayLikeObject(value) && hasOwnProperty.call(value, 'callee') &&
+ (!propertyIsEnumerable.call(value, 'callee') || objectToString.call(value) == argsTag);
+}
+
+/**
+ * Checks if `value` is array-like. A value is considered array-like if it's
+ * not a function and has a `value.length` that's an integer greater than or
+ * equal to `0` and less than or equal to `Number.MAX_SAFE_INTEGER`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is array-like, else `false`.
+ * @example
+ *
+ * _.isArrayLike([1, 2, 3]);
+ * // => true
+ *
+ * _.isArrayLike(document.body.children);
+ * // => true
+ *
+ * _.isArrayLike('abc');
+ * // => true
+ *
+ * _.isArrayLike(_.noop);
+ * // => false
+ */
+function isArrayLike(value) {
+ return value != null && isLength(value.length) && !isFunction(value);
+}
+
+/**
+ * This method is like `_.isArrayLike` except that it also checks if `value`
+ * is an object.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is an array-like object,
+ * else `false`.
+ * @example
+ *
+ * _.isArrayLikeObject([1, 2, 3]);
+ * // => true
+ *
+ * _.isArrayLikeObject(document.body.children);
+ * // => true
+ *
+ * _.isArrayLikeObject('abc');
+ * // => false
+ *
+ * _.isArrayLikeObject(_.noop);
+ * // => false
+ */
+function isArrayLikeObject(value) {
+ return isObjectLike(value) && isArrayLike(value);
+}
+
+/**
+ * Checks if `value` is classified as a `Function` object.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a function, else `false`.
+ * @example
+ *
+ * _.isFunction(_);
+ * // => true
+ *
+ * _.isFunction(/abc/);
+ * // => false
+ */
+function isFunction(value) {
+ // The use of `Object#toString` avoids issues with the `typeof` operator
+ // in Safari 8-9 which returns 'object' for typed array and other constructors.
+ var tag = isObject(value) ? objectToString.call(value) : '';
+ return tag == funcTag || tag == genTag;
+}
+
+/**
+ * Checks if `value` is a valid array-like length.
+ *
+ * **Note:** This method is loosely based on
+ * [`ToLength`](http://ecma-international.org/ecma-262/7.0/#sec-tolength).
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a valid length, else `false`.
+ * @example
+ *
+ * _.isLength(3);
+ * // => true
+ *
+ * _.isLength(Number.MIN_VALUE);
+ * // => false
+ *
+ * _.isLength(Infinity);
+ * // => false
+ *
+ * _.isLength('3');
+ * // => false
+ */
+function isLength(value) {
+ return typeof value == 'number' &&
+ value > -1 && value % 1 == 0 && value <= MAX_SAFE_INTEGER;
+}
+
+/**
+ * Checks if `value` is the
+ * [language type](http://www.ecma-international.org/ecma-262/7.0/#sec-ecmascript-language-types)
+ * of `Object`. (e.g. arrays, functions, objects, regexes, `new Number(0)`, and `new String('')`)
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is an object, else `false`.
+ * @example
+ *
+ * _.isObject({});
+ * // => true
+ *
+ * _.isObject([1, 2, 3]);
+ * // => true
+ *
+ * _.isObject(_.noop);
+ * // => true
+ *
+ * _.isObject(null);
+ * // => false
+ */
+function isObject(value) {
+ var type = typeof value;
+ return !!value && (type == 'object' || type == 'function');
+}
+
+/**
+ * Checks if `value` is object-like. A value is object-like if it's not `null`
+ * and has a `typeof` result of "object".
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is object-like, else `false`.
+ * @example
+ *
+ * _.isObjectLike({});
+ * // => true
+ *
+ * _.isObjectLike([1, 2, 3]);
+ * // => true
+ *
+ * _.isObjectLike(_.noop);
+ * // => false
+ *
+ * _.isObjectLike(null);
+ * // => false
+ */
+function isObjectLike(value) {
+ return !!value && typeof value == 'object';
+}
+
+module.exports = isArguments;
diff --git a/node_modules/lodash.isarguments/package.json b/node_modules/lodash.isarguments/package.json
new file mode 100644
index 0000000..0b3ccca
--- /dev/null
+++ b/node_modules/lodash.isarguments/package.json
@@ -0,0 +1,69 @@
+{
+ "_from": "lodash.isarguments@^3.0.0",
+ "_id": "lodash.isarguments@3.1.0",
+ "_inBundle": false,
+ "_integrity": "sha1-L1c9hcaiQon/AGY7SRwdM4/zRYo=",
+ "_location": "/lodash.isarguments",
+ "_phantomChildren": {},
+ "_requested": {
+ "type": "range",
+ "registry": true,
+ "raw": "lodash.isarguments@^3.0.0",
+ "name": "lodash.isarguments",
+ "escapedName": "lodash.isarguments",
+ "rawSpec": "^3.0.0",
+ "saveSpec": null,
+ "fetchSpec": "^3.0.0"
+ },
+ "_requiredBy": [
+ "/lodash.keys"
+ ],
+ "_resolved": "https://registry.npmjs.org/lodash.isarguments/-/lodash.isarguments-3.1.0.tgz",
+ "_shasum": "2f573d85c6a24289ff00663b491c1d338ff3458a",
+ "_spec": "lodash.isarguments@^3.0.0",
+ "_where": "/Users/rpatra16/MyProjects/RamAndAntara/node_modules/lodash.keys",
+ "author": {
+ "name": "John-David Dalton",
+ "email": "john.david.dalton@gmail.com",
+ "url": "http://allyoucanleet.com/"
+ },
+ "bugs": {
+ "url": "https://github.com/lodash/lodash/issues"
+ },
+ "bundleDependencies": false,
+ "contributors": [
+ {
+ "name": "John-David Dalton",
+ "email": "john.david.dalton@gmail.com",
+ "url": "http://allyoucanleet.com/"
+ },
+ {
+ "name": "Blaine Bublitz",
+ "email": "blaine.bublitz@gmail.com",
+ "url": "https://github.com/phated"
+ },
+ {
+ "name": "Mathias Bynens",
+ "email": "mathias@qiwi.be",
+ "url": "https://mathiasbynens.be/"
+ }
+ ],
+ "deprecated": false,
+ "description": "The lodash method `_.isArguments` exported as a module.",
+ "homepage": "https://lodash.com/",
+ "icon": "https://lodash.com/icon.svg",
+ "keywords": [
+ "lodash-modularized",
+ "isarguments"
+ ],
+ "license": "MIT",
+ "name": "lodash.isarguments",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/lodash/lodash.git"
+ },
+ "scripts": {
+ "test": "echo \"See https://travis-ci.org/lodash/lodash-cli for testing details.\""
+ },
+ "version": "3.1.0"
+}
diff --git a/node_modules/lodash.isarray/LICENSE b/node_modules/lodash.isarray/LICENSE
new file mode 100644
index 0000000..9cd87e5
--- /dev/null
+++ b/node_modules/lodash.isarray/LICENSE
@@ -0,0 +1,22 @@
+Copyright 2012-2015 The Dojo Foundation
+Based on Underscore.js, copyright 2009-2015 Jeremy Ashkenas,
+DocumentCloud and Investigative Reporters & Editors
+
+Permission is hereby granted, free of charge, to any person obtaining
+a copy of this software and associated documentation files (the
+"Software"), to deal in the Software without restriction, including
+without limitation the rights to use, copy, modify, merge, publish,
+distribute, sublicense, and/or sell copies of the Software, and to
+permit persons to whom the Software is furnished to do so, subject to
+the following conditions:
+
+The above copyright notice and this permission notice shall be
+included in all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
+EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
+MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
+NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
+LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
+OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
+WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
diff --git a/node_modules/lodash.isarray/README.md b/node_modules/lodash.isarray/README.md
new file mode 100644
index 0000000..ea274aa
--- /dev/null
+++ b/node_modules/lodash.isarray/README.md
@@ -0,0 +1,20 @@
+# lodash.isarray v3.0.4
+
+The [modern build](https://github.com/lodash/lodash/wiki/Build-Differences) of [lodash’s](https://lodash.com/) `_.isArray` exported as a [Node.js](http://nodejs.org/)/[io.js](https://iojs.org/) module.
+
+## Installation
+
+Using npm:
+
+```bash
+$ {sudo -H} npm i -g npm
+$ npm i --save lodash.isarray
+```
+
+In Node.js/io.js:
+
+```js
+var isArray = require('lodash.isarray');
+```
+
+See the [documentation](https://lodash.com/docs#isArray) or [package source](https://github.com/lodash/lodash/blob/3.0.4-npm-packages/lodash.isarray) for more details.
diff --git a/node_modules/lodash.isarray/index.js b/node_modules/lodash.isarray/index.js
new file mode 100644
index 0000000..dd24658
--- /dev/null
+++ b/node_modules/lodash.isarray/index.js
@@ -0,0 +1,180 @@
+/**
+ * lodash 3.0.4 (Custom Build)
+ * Build: `lodash modern modularize exports="npm" -o ./`
+ * Copyright 2012-2015 The Dojo Foundation
+ * Based on Underscore.js 1.8.3
+ * Copyright 2009-2015 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license
+ */
+
+/** `Object#toString` result references. */
+var arrayTag = '[object Array]',
+ funcTag = '[object Function]';
+
+/** Used to detect host constructors (Safari > 5). */
+var reIsHostCtor = /^\[object .+?Constructor\]$/;
+
+/**
+ * Checks if `value` is object-like.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is object-like, else `false`.
+ */
+function isObjectLike(value) {
+ return !!value && typeof value == 'object';
+}
+
+/** Used for native method references. */
+var objectProto = Object.prototype;
+
+/** Used to resolve the decompiled source of functions. */
+var fnToString = Function.prototype.toString;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Used to resolve the [`toStringTag`](http://ecma-international.org/ecma-262/6.0/#sec-object.prototype.tostring)
+ * of values.
+ */
+var objToString = objectProto.toString;
+
+/** Used to detect if a method is native. */
+var reIsNative = RegExp('^' +
+ fnToString.call(hasOwnProperty).replace(/[\\^$.*+?()[\]{}|]/g, '\\$&')
+ .replace(/hasOwnProperty|(function).*?(?=\\\()| for .+?(?=\\\])/g, '$1.*?') + '$'
+);
+
+/* Native method references for those with the same name as other `lodash` methods. */
+var nativeIsArray = getNative(Array, 'isArray');
+
+/**
+ * Used as the [maximum length](http://ecma-international.org/ecma-262/6.0/#sec-number.max_safe_integer)
+ * of an array-like value.
+ */
+var MAX_SAFE_INTEGER = 9007199254740991;
+
+/**
+ * Gets the native function at `key` of `object`.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @param {string} key The key of the method to get.
+ * @returns {*} Returns the function if it's native, else `undefined`.
+ */
+function getNative(object, key) {
+ var value = object == null ? undefined : object[key];
+ return isNative(value) ? value : undefined;
+}
+
+/**
+ * Checks if `value` is a valid array-like length.
+ *
+ * **Note:** This function is based on [`ToLength`](http://ecma-international.org/ecma-262/6.0/#sec-tolength).
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a valid length, else `false`.
+ */
+function isLength(value) {
+ return typeof value == 'number' && value > -1 && value % 1 == 0 && value <= MAX_SAFE_INTEGER;
+}
+
+/**
+ * Checks if `value` is classified as an `Array` object.
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is correctly classified, else `false`.
+ * @example
+ *
+ * _.isArray([1, 2, 3]);
+ * // => true
+ *
+ * _.isArray(function() { return arguments; }());
+ * // => false
+ */
+var isArray = nativeIsArray || function(value) {
+ return isObjectLike(value) && isLength(value.length) && objToString.call(value) == arrayTag;
+};
+
+/**
+ * Checks if `value` is classified as a `Function` object.
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is correctly classified, else `false`.
+ * @example
+ *
+ * _.isFunction(_);
+ * // => true
+ *
+ * _.isFunction(/abc/);
+ * // => false
+ */
+function isFunction(value) {
+ // The use of `Object#toString` avoids issues with the `typeof` operator
+ // in older versions of Chrome and Safari which return 'function' for regexes
+ // and Safari 8 equivalents which return 'object' for typed array constructors.
+ return isObject(value) && objToString.call(value) == funcTag;
+}
+
+/**
+ * Checks if `value` is the [language type](https://es5.github.io/#x8) of `Object`.
+ * (e.g. arrays, functions, objects, regexes, `new Number(0)`, and `new String('')`)
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is an object, else `false`.
+ * @example
+ *
+ * _.isObject({});
+ * // => true
+ *
+ * _.isObject([1, 2, 3]);
+ * // => true
+ *
+ * _.isObject(1);
+ * // => false
+ */
+function isObject(value) {
+ // Avoid a V8 JIT bug in Chrome 19-20.
+ // See https://code.google.com/p/v8/issues/detail?id=2291 for more details.
+ var type = typeof value;
+ return !!value && (type == 'object' || type == 'function');
+}
+
+/**
+ * Checks if `value` is a native function.
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a native function, else `false`.
+ * @example
+ *
+ * _.isNative(Array.prototype.push);
+ * // => true
+ *
+ * _.isNative(_);
+ * // => false
+ */
+function isNative(value) {
+ if (value == null) {
+ return false;
+ }
+ if (isFunction(value)) {
+ return reIsNative.test(fnToString.call(value));
+ }
+ return isObjectLike(value) && reIsHostCtor.test(value);
+}
+
+module.exports = isArray;
diff --git a/node_modules/lodash.isarray/package.json b/node_modules/lodash.isarray/package.json
new file mode 100644
index 0000000..93f4732
--- /dev/null
+++ b/node_modules/lodash.isarray/package.json
@@ -0,0 +1,81 @@
+{
+ "_from": "lodash.isarray@^3.0.0",
+ "_id": "lodash.isarray@3.0.4",
+ "_inBundle": false,
+ "_integrity": "sha1-eeTriMNqgSKvhvhEqpvNhRtfu1U=",
+ "_location": "/lodash.isarray",
+ "_phantomChildren": {},
+ "_requested": {
+ "type": "range",
+ "registry": true,
+ "raw": "lodash.isarray@^3.0.0",
+ "name": "lodash.isarray",
+ "escapedName": "lodash.isarray",
+ "rawSpec": "^3.0.0",
+ "saveSpec": null,
+ "fetchSpec": "^3.0.0"
+ },
+ "_requiredBy": [
+ "/lodash.keys"
+ ],
+ "_resolved": "https://registry.npmjs.org/lodash.isarray/-/lodash.isarray-3.0.4.tgz",
+ "_shasum": "79e4eb88c36a8122af86f844aa9bcd851b5fbb55",
+ "_spec": "lodash.isarray@^3.0.0",
+ "_where": "/Users/rpatra16/MyProjects/RamAndAntara/node_modules/lodash.keys",
+ "author": {
+ "name": "John-David Dalton",
+ "email": "john.david.dalton@gmail.com",
+ "url": "http://allyoucanleet.com/"
+ },
+ "bugs": {
+ "url": "https://github.com/lodash/lodash/issues"
+ },
+ "bundleDependencies": false,
+ "contributors": [
+ {
+ "name": "John-David Dalton",
+ "email": "john.david.dalton@gmail.com",
+ "url": "http://allyoucanleet.com/"
+ },
+ {
+ "name": "Benjamin Tan",
+ "email": "demoneaux@gmail.com",
+ "url": "https://d10.github.io/"
+ },
+ {
+ "name": "Blaine Bublitz",
+ "email": "blaine@iceddev.com",
+ "url": "http://www.iceddev.com/"
+ },
+ {
+ "name": "Kit Cambridge",
+ "email": "github@kitcambridge.be",
+ "url": "http://kitcambridge.be/"
+ },
+ {
+ "name": "Mathias Bynens",
+ "email": "mathias@qiwi.be",
+ "url": "https://mathiasbynens.be/"
+ }
+ ],
+ "deprecated": false,
+ "description": "The modern build of lodash’s `_.isArray` as a module.",
+ "homepage": "https://lodash.com/",
+ "icon": "https://lodash.com/icon.svg",
+ "keywords": [
+ "lodash",
+ "lodash-modularized",
+ "stdlib",
+ "util"
+ ],
+ "license": "MIT",
+ "name": "lodash.isarray",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/lodash/lodash.git"
+ },
+ "scripts": {
+ "test": "echo \"See https://travis-ci.org/lodash/lodash-cli for testing details.\""
+ },
+ "version": "3.0.4"
+}
diff --git a/node_modules/lodash.keys/LICENSE b/node_modules/lodash.keys/LICENSE
new file mode 100644
index 0000000..9cd87e5
--- /dev/null
+++ b/node_modules/lodash.keys/LICENSE
@@ -0,0 +1,22 @@
+Copyright 2012-2015 The Dojo Foundation
+Based on Underscore.js, copyright 2009-2015 Jeremy Ashkenas,
+DocumentCloud and Investigative Reporters & Editors
+
+Permission is hereby granted, free of charge, to any person obtaining
+a copy of this software and associated documentation files (the
+"Software"), to deal in the Software without restriction, including
+without limitation the rights to use, copy, modify, merge, publish,
+distribute, sublicense, and/or sell copies of the Software, and to
+permit persons to whom the Software is furnished to do so, subject to
+the following conditions:
+
+The above copyright notice and this permission notice shall be
+included in all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
+EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
+MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
+NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
+LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
+OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
+WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
diff --git a/node_modules/lodash.keys/README.md b/node_modules/lodash.keys/README.md
new file mode 100644
index 0000000..5f69a18
--- /dev/null
+++ b/node_modules/lodash.keys/README.md
@@ -0,0 +1,20 @@
+# lodash.keys v3.1.2
+
+The [modern build](https://github.com/lodash/lodash/wiki/Build-Differences) of [lodash’s](https://lodash.com/) `_.keys` exported as a [Node.js](http://nodejs.org/)/[io.js](https://iojs.org/) module.
+
+## Installation
+
+Using npm:
+
+```bash
+$ {sudo -H} npm i -g npm
+$ npm i --save lodash.keys
+```
+
+In Node.js/io.js:
+
+```js
+var keys = require('lodash.keys');
+```
+
+See the [documentation](https://lodash.com/docs#keys) or [package source](https://github.com/lodash/lodash/blob/3.1.2-npm-packages/lodash.keys) for more details.
diff --git a/node_modules/lodash.keys/index.js b/node_modules/lodash.keys/index.js
new file mode 100644
index 0000000..f4c1774
--- /dev/null
+++ b/node_modules/lodash.keys/index.js
@@ -0,0 +1,236 @@
+/**
+ * lodash 3.1.2 (Custom Build)
+ * Build: `lodash modern modularize exports="npm" -o ./`
+ * Copyright 2012-2015 The Dojo Foundation
+ * Based on Underscore.js 1.8.3
+ * Copyright 2009-2015 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license
+ */
+var getNative = require('lodash._getnative'),
+ isArguments = require('lodash.isarguments'),
+ isArray = require('lodash.isarray');
+
+/** Used to detect unsigned integer values. */
+var reIsUint = /^\d+$/;
+
+/** Used for native method references. */
+var objectProto = Object.prototype;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/* Native method references for those with the same name as other `lodash` methods. */
+var nativeKeys = getNative(Object, 'keys');
+
+/**
+ * Used as the [maximum length](http://ecma-international.org/ecma-262/6.0/#sec-number.max_safe_integer)
+ * of an array-like value.
+ */
+var MAX_SAFE_INTEGER = 9007199254740991;
+
+/**
+ * The base implementation of `_.property` without support for deep paths.
+ *
+ * @private
+ * @param {string} key The key of the property to get.
+ * @returns {Function} Returns the new function.
+ */
+function baseProperty(key) {
+ return function(object) {
+ return object == null ? undefined : object[key];
+ };
+}
+
+/**
+ * Gets the "length" property value of `object`.
+ *
+ * **Note:** This function is used to avoid a [JIT bug](https://bugs.webkit.org/show_bug.cgi?id=142792)
+ * that affects Safari on at least iOS 8.1-8.3 ARM64.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @returns {*} Returns the "length" value.
+ */
+var getLength = baseProperty('length');
+
+/**
+ * Checks if `value` is array-like.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is array-like, else `false`.
+ */
+function isArrayLike(value) {
+ return value != null && isLength(getLength(value));
+}
+
+/**
+ * Checks if `value` is a valid array-like index.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @param {number} [length=MAX_SAFE_INTEGER] The upper bounds of a valid index.
+ * @returns {boolean} Returns `true` if `value` is a valid index, else `false`.
+ */
+function isIndex(value, length) {
+ value = (typeof value == 'number' || reIsUint.test(value)) ? +value : -1;
+ length = length == null ? MAX_SAFE_INTEGER : length;
+ return value > -1 && value % 1 == 0 && value < length;
+}
+
+/**
+ * Checks if `value` is a valid array-like length.
+ *
+ * **Note:** This function is based on [`ToLength`](http://ecma-international.org/ecma-262/6.0/#sec-tolength).
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a valid length, else `false`.
+ */
+function isLength(value) {
+ return typeof value == 'number' && value > -1 && value % 1 == 0 && value <= MAX_SAFE_INTEGER;
+}
+
+/**
+ * A fallback implementation of `Object.keys` which creates an array of the
+ * own enumerable property names of `object`.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the array of property names.
+ */
+function shimKeys(object) {
+ var props = keysIn(object),
+ propsLength = props.length,
+ length = propsLength && object.length;
+
+ var allowIndexes = !!length && isLength(length) &&
+ (isArray(object) || isArguments(object));
+
+ var index = -1,
+ result = [];
+
+ while (++index < propsLength) {
+ var key = props[index];
+ if ((allowIndexes && isIndex(key, length)) || hasOwnProperty.call(object, key)) {
+ result.push(key);
+ }
+ }
+ return result;
+}
+
+/**
+ * Checks if `value` is the [language type](https://es5.github.io/#x8) of `Object`.
+ * (e.g. arrays, functions, objects, regexes, `new Number(0)`, and `new String('')`)
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is an object, else `false`.
+ * @example
+ *
+ * _.isObject({});
+ * // => true
+ *
+ * _.isObject([1, 2, 3]);
+ * // => true
+ *
+ * _.isObject(1);
+ * // => false
+ */
+function isObject(value) {
+ // Avoid a V8 JIT bug in Chrome 19-20.
+ // See https://code.google.com/p/v8/issues/detail?id=2291 for more details.
+ var type = typeof value;
+ return !!value && (type == 'object' || type == 'function');
+}
+
+/**
+ * Creates an array of the own enumerable property names of `object`.
+ *
+ * **Note:** Non-object values are coerced to objects. See the
+ * [ES spec](http://ecma-international.org/ecma-262/6.0/#sec-object.keys)
+ * for more details.
+ *
+ * @static
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the array of property names.
+ * @example
+ *
+ * function Foo() {
+ * this.a = 1;
+ * this.b = 2;
+ * }
+ *
+ * Foo.prototype.c = 3;
+ *
+ * _.keys(new Foo);
+ * // => ['a', 'b'] (iteration order is not guaranteed)
+ *
+ * _.keys('hi');
+ * // => ['0', '1']
+ */
+var keys = !nativeKeys ? shimKeys : function(object) {
+ var Ctor = object == null ? undefined : object.constructor;
+ if ((typeof Ctor == 'function' && Ctor.prototype === object) ||
+ (typeof object != 'function' && isArrayLike(object))) {
+ return shimKeys(object);
+ }
+ return isObject(object) ? nativeKeys(object) : [];
+};
+
+/**
+ * Creates an array of the own and inherited enumerable property names of `object`.
+ *
+ * **Note:** Non-object values are coerced to objects.
+ *
+ * @static
+ * @memberOf _
+ * @category Object
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the array of property names.
+ * @example
+ *
+ * function Foo() {
+ * this.a = 1;
+ * this.b = 2;
+ * }
+ *
+ * Foo.prototype.c = 3;
+ *
+ * _.keysIn(new Foo);
+ * // => ['a', 'b', 'c'] (iteration order is not guaranteed)
+ */
+function keysIn(object) {
+ if (object == null) {
+ return [];
+ }
+ if (!isObject(object)) {
+ object = Object(object);
+ }
+ var length = object.length;
+ length = (length && isLength(length) &&
+ (isArray(object) || isArguments(object)) && length) || 0;
+
+ var Ctor = object.constructor,
+ index = -1,
+ isProto = typeof Ctor == 'function' && Ctor.prototype === object,
+ result = Array(length),
+ skipIndexes = length > 0;
+
+ while (++index < length) {
+ result[index] = (index + '');
+ }
+ for (var key in object) {
+ if (!(skipIndexes && isIndex(key, length)) &&
+ !(key == 'constructor' && (isProto || !hasOwnProperty.call(object, key)))) {
+ result.push(key);
+ }
+ }
+ return result;
+}
+
+module.exports = keys;
diff --git a/node_modules/lodash.keys/package.json b/node_modules/lodash.keys/package.json
new file mode 100644
index 0000000..d8b2749
--- /dev/null
+++ b/node_modules/lodash.keys/package.json
@@ -0,0 +1,86 @@
+{
+ "_from": "lodash.keys@^3.0.0",
+ "_id": "lodash.keys@3.1.2",
+ "_inBundle": false,
+ "_integrity": "sha1-TbwEcrFWvlCgsoaFXRvQsMZWCYo=",
+ "_location": "/lodash.keys",
+ "_phantomChildren": {},
+ "_requested": {
+ "type": "range",
+ "registry": true,
+ "raw": "lodash.keys@^3.0.0",
+ "name": "lodash.keys",
+ "escapedName": "lodash.keys",
+ "rawSpec": "^3.0.0",
+ "saveSpec": null,
+ "fetchSpec": "^3.0.0"
+ },
+ "_requiredBy": [
+ "/lodash.template"
+ ],
+ "_resolved": "https://registry.npmjs.org/lodash.keys/-/lodash.keys-3.1.2.tgz",
+ "_shasum": "4dbc0472b156be50a0b286855d1bd0b0c656098a",
+ "_spec": "lodash.keys@^3.0.0",
+ "_where": "/Users/rpatra16/MyProjects/RamAndAntara/node_modules/lodash.template",
+ "author": {
+ "name": "John-David Dalton",
+ "email": "john.david.dalton@gmail.com",
+ "url": "http://allyoucanleet.com/"
+ },
+ "bugs": {
+ "url": "https://github.com/lodash/lodash/issues"
+ },
+ "bundleDependencies": false,
+ "contributors": [
+ {
+ "name": "John-David Dalton",
+ "email": "john.david.dalton@gmail.com",
+ "url": "http://allyoucanleet.com/"
+ },
+ {
+ "name": "Benjamin Tan",
+ "email": "demoneaux@gmail.com",
+ "url": "https://d10.github.io/"
+ },
+ {
+ "name": "Blaine Bublitz",
+ "email": "blaine@iceddev.com",
+ "url": "http://www.iceddev.com/"
+ },
+ {
+ "name": "Kit Cambridge",
+ "email": "github@kitcambridge.be",
+ "url": "http://kitcambridge.be/"
+ },
+ {
+ "name": "Mathias Bynens",
+ "email": "mathias@qiwi.be",
+ "url": "https://mathiasbynens.be/"
+ }
+ ],
+ "dependencies": {
+ "lodash._getnative": "^3.0.0",
+ "lodash.isarguments": "^3.0.0",
+ "lodash.isarray": "^3.0.0"
+ },
+ "deprecated": false,
+ "description": "The modern build of lodash’s `_.keys` as a module.",
+ "homepage": "https://lodash.com/",
+ "icon": "https://lodash.com/icon.svg",
+ "keywords": [
+ "lodash",
+ "lodash-modularized",
+ "stdlib",
+ "util"
+ ],
+ "license": "MIT",
+ "name": "lodash.keys",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/lodash/lodash.git"
+ },
+ "scripts": {
+ "test": "echo \"See https://travis-ci.org/lodash/lodash-cli for testing details.\""
+ },
+ "version": "3.1.2"
+}
diff --git a/node_modules/lodash.mergewith/LICENSE b/node_modules/lodash.mergewith/LICENSE
new file mode 100644
index 0000000..c6f2f61
--- /dev/null
+++ b/node_modules/lodash.mergewith/LICENSE
@@ -0,0 +1,47 @@
+Copyright JS Foundation and other contributors
+
+Based on Underscore.js, copyright Jeremy Ashkenas,
+DocumentCloud and Investigative Reporters & Editors
+
+This software consists of voluntary contributions made by many
+individuals. For exact contribution history, see the revision history
+available at https://github.com/lodash/lodash
+
+The following license applies to all parts of this software except as
+documented below:
+
+====
+
+Permission is hereby granted, free of charge, to any person obtaining
+a copy of this software and associated documentation files (the
+"Software"), to deal in the Software without restriction, including
+without limitation the rights to use, copy, modify, merge, publish,
+distribute, sublicense, and/or sell copies of the Software, and to
+permit persons to whom the Software is furnished to do so, subject to
+the following conditions:
+
+The above copyright notice and this permission notice shall be
+included in all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
+EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
+MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
+NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
+LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
+OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
+WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
+
+====
+
+Copyright and related rights for sample code are waived via CC0. Sample
+code is defined as all source code displayed within the prose of the
+documentation.
+
+CC0: http://creativecommons.org/publicdomain/zero/1.0/
+
+====
+
+Files located in the node_modules and vendor directories are externally
+maintained libraries used by this software which have their own
+licenses; we recommend you read them, as their terms may differ from the
+terms above.
diff --git a/node_modules/lodash.mergewith/README.md b/node_modules/lodash.mergewith/README.md
new file mode 100644
index 0000000..a2d8425
--- /dev/null
+++ b/node_modules/lodash.mergewith/README.md
@@ -0,0 +1,18 @@
+# lodash.mergewith v4.6.1
+
+The [Lodash](https://lodash.com/) method `_.mergeWith` exported as a [Node.js](https://nodejs.org/) module.
+
+## Installation
+
+Using npm:
+```bash
+$ {sudo -H} npm i -g npm
+$ npm i --save lodash.mergewith
+```
+
+In Node.js:
+```js
+var mergeWith = require('lodash.mergewith');
+```
+
+See the [documentation](https://lodash.com/docs#mergeWith) or [package source](https://github.com/lodash/lodash/blob/4.6.1-npm-packages/lodash.mergewith) for more details.
diff --git a/node_modules/lodash.mergewith/index.js b/node_modules/lodash.mergewith/index.js
new file mode 100644
index 0000000..2445759
--- /dev/null
+++ b/node_modules/lodash.mergewith/index.js
@@ -0,0 +1,1963 @@
+/**
+ * Lodash (Custom Build)
+ * Build: `lodash modularize exports="npm" -o ./`
+ * Copyright JS Foundation and other contributors
+ * Released under MIT license
+ * Based on Underscore.js 1.8.3
+ * Copyright Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ */
+
+/** Used as the size to enable large array optimizations. */
+var LARGE_ARRAY_SIZE = 200;
+
+/** Used to stand-in for `undefined` hash values. */
+var HASH_UNDEFINED = '__lodash_hash_undefined__';
+
+/** Used to detect hot functions by number of calls within a span of milliseconds. */
+var HOT_COUNT = 800,
+ HOT_SPAN = 16;
+
+/** Used as references for various `Number` constants. */
+var MAX_SAFE_INTEGER = 9007199254740991;
+
+/** `Object#toString` result references. */
+var argsTag = '[object Arguments]',
+ arrayTag = '[object Array]',
+ asyncTag = '[object AsyncFunction]',
+ boolTag = '[object Boolean]',
+ dateTag = '[object Date]',
+ errorTag = '[object Error]',
+ funcTag = '[object Function]',
+ genTag = '[object GeneratorFunction]',
+ mapTag = '[object Map]',
+ numberTag = '[object Number]',
+ nullTag = '[object Null]',
+ objectTag = '[object Object]',
+ proxyTag = '[object Proxy]',
+ regexpTag = '[object RegExp]',
+ setTag = '[object Set]',
+ stringTag = '[object String]',
+ undefinedTag = '[object Undefined]',
+ weakMapTag = '[object WeakMap]';
+
+var arrayBufferTag = '[object ArrayBuffer]',
+ dataViewTag = '[object DataView]',
+ float32Tag = '[object Float32Array]',
+ float64Tag = '[object Float64Array]',
+ int8Tag = '[object Int8Array]',
+ int16Tag = '[object Int16Array]',
+ int32Tag = '[object Int32Array]',
+ uint8Tag = '[object Uint8Array]',
+ uint8ClampedTag = '[object Uint8ClampedArray]',
+ uint16Tag = '[object Uint16Array]',
+ uint32Tag = '[object Uint32Array]';
+
+/**
+ * Used to match `RegExp`
+ * [syntax characters](http://ecma-international.org/ecma-262/7.0/#sec-patterns).
+ */
+var reRegExpChar = /[\\^$.*+?()[\]{}|]/g;
+
+/** Used to detect host constructors (Safari). */
+var reIsHostCtor = /^\[object .+?Constructor\]$/;
+
+/** Used to detect unsigned integer values. */
+var reIsUint = /^(?:0|[1-9]\d*)$/;
+
+/** Used to identify `toStringTag` values of typed arrays. */
+var typedArrayTags = {};
+typedArrayTags[float32Tag] = typedArrayTags[float64Tag] =
+typedArrayTags[int8Tag] = typedArrayTags[int16Tag] =
+typedArrayTags[int32Tag] = typedArrayTags[uint8Tag] =
+typedArrayTags[uint8ClampedTag] = typedArrayTags[uint16Tag] =
+typedArrayTags[uint32Tag] = true;
+typedArrayTags[argsTag] = typedArrayTags[arrayTag] =
+typedArrayTags[arrayBufferTag] = typedArrayTags[boolTag] =
+typedArrayTags[dataViewTag] = typedArrayTags[dateTag] =
+typedArrayTags[errorTag] = typedArrayTags[funcTag] =
+typedArrayTags[mapTag] = typedArrayTags[numberTag] =
+typedArrayTags[objectTag] = typedArrayTags[regexpTag] =
+typedArrayTags[setTag] = typedArrayTags[stringTag] =
+typedArrayTags[weakMapTag] = false;
+
+/** Detect free variable `global` from Node.js. */
+var freeGlobal = typeof global == 'object' && global && global.Object === Object && global;
+
+/** Detect free variable `self`. */
+var freeSelf = typeof self == 'object' && self && self.Object === Object && self;
+
+/** Used as a reference to the global object. */
+var root = freeGlobal || freeSelf || Function('return this')();
+
+/** Detect free variable `exports`. */
+var freeExports = typeof exports == 'object' && exports && !exports.nodeType && exports;
+
+/** Detect free variable `module`. */
+var freeModule = freeExports && typeof module == 'object' && module && !module.nodeType && module;
+
+/** Detect the popular CommonJS extension `module.exports`. */
+var moduleExports = freeModule && freeModule.exports === freeExports;
+
+/** Detect free variable `process` from Node.js. */
+var freeProcess = moduleExports && freeGlobal.process;
+
+/** Used to access faster Node.js helpers. */
+var nodeUtil = (function() {
+ try {
+ return freeProcess && freeProcess.binding && freeProcess.binding('util');
+ } catch (e) {}
+}());
+
+/* Node.js helper references. */
+var nodeIsTypedArray = nodeUtil && nodeUtil.isTypedArray;
+
+/**
+ * A faster alternative to `Function#apply`, this function invokes `func`
+ * with the `this` binding of `thisArg` and the arguments of `args`.
+ *
+ * @private
+ * @param {Function} func The function to invoke.
+ * @param {*} thisArg The `this` binding of `func`.
+ * @param {Array} args The arguments to invoke `func` with.
+ * @returns {*} Returns the result of `func`.
+ */
+function apply(func, thisArg, args) {
+ switch (args.length) {
+ case 0: return func.call(thisArg);
+ case 1: return func.call(thisArg, args[0]);
+ case 2: return func.call(thisArg, args[0], args[1]);
+ case 3: return func.call(thisArg, args[0], args[1], args[2]);
+ }
+ return func.apply(thisArg, args);
+}
+
+/**
+ * The base implementation of `_.times` without support for iteratee shorthands
+ * or max array length checks.
+ *
+ * @private
+ * @param {number} n The number of times to invoke `iteratee`.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @returns {Array} Returns the array of results.
+ */
+function baseTimes(n, iteratee) {
+ var index = -1,
+ result = Array(n);
+
+ while (++index < n) {
+ result[index] = iteratee(index);
+ }
+ return result;
+}
+
+/**
+ * The base implementation of `_.unary` without support for storing metadata.
+ *
+ * @private
+ * @param {Function} func The function to cap arguments for.
+ * @returns {Function} Returns the new capped function.
+ */
+function baseUnary(func) {
+ return function(value) {
+ return func(value);
+ };
+}
+
+/**
+ * Gets the value at `key` of `object`.
+ *
+ * @private
+ * @param {Object} [object] The object to query.
+ * @param {string} key The key of the property to get.
+ * @returns {*} Returns the property value.
+ */
+function getValue(object, key) {
+ return object == null ? undefined : object[key];
+}
+
+/**
+ * Creates a unary function that invokes `func` with its argument transformed.
+ *
+ * @private
+ * @param {Function} func The function to wrap.
+ * @param {Function} transform The argument transform.
+ * @returns {Function} Returns the new function.
+ */
+function overArg(func, transform) {
+ return function(arg) {
+ return func(transform(arg));
+ };
+}
+
+/**
+ * Gets the value at `key`, unless `key` is "__proto__".
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @param {string} key The key of the property to get.
+ * @returns {*} Returns the property value.
+ */
+function safeGet(object, key) {
+ return key == '__proto__'
+ ? undefined
+ : object[key];
+}
+
+/** Used for built-in method references. */
+var arrayProto = Array.prototype,
+ funcProto = Function.prototype,
+ objectProto = Object.prototype;
+
+/** Used to detect overreaching core-js shims. */
+var coreJsData = root['__core-js_shared__'];
+
+/** Used to resolve the decompiled source of functions. */
+var funcToString = funcProto.toString;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/** Used to detect methods masquerading as native. */
+var maskSrcKey = (function() {
+ var uid = /[^.]+$/.exec(coreJsData && coreJsData.keys && coreJsData.keys.IE_PROTO || '');
+ return uid ? ('Symbol(src)_1.' + uid) : '';
+}());
+
+/**
+ * Used to resolve the
+ * [`toStringTag`](http://ecma-international.org/ecma-262/7.0/#sec-object.prototype.tostring)
+ * of values.
+ */
+var nativeObjectToString = objectProto.toString;
+
+/** Used to infer the `Object` constructor. */
+var objectCtorString = funcToString.call(Object);
+
+/** Used to detect if a method is native. */
+var reIsNative = RegExp('^' +
+ funcToString.call(hasOwnProperty).replace(reRegExpChar, '\\$&')
+ .replace(/hasOwnProperty|(function).*?(?=\\\()| for .+?(?=\\\])/g, '$1.*?') + '$'
+);
+
+/** Built-in value references. */
+var Buffer = moduleExports ? root.Buffer : undefined,
+ Symbol = root.Symbol,
+ Uint8Array = root.Uint8Array,
+ allocUnsafe = Buffer ? Buffer.allocUnsafe : undefined,
+ getPrototype = overArg(Object.getPrototypeOf, Object),
+ objectCreate = Object.create,
+ propertyIsEnumerable = objectProto.propertyIsEnumerable,
+ splice = arrayProto.splice,
+ symToStringTag = Symbol ? Symbol.toStringTag : undefined;
+
+var defineProperty = (function() {
+ try {
+ var func = getNative(Object, 'defineProperty');
+ func({}, '', {});
+ return func;
+ } catch (e) {}
+}());
+
+/* Built-in method references for those with the same name as other `lodash` methods. */
+var nativeIsBuffer = Buffer ? Buffer.isBuffer : undefined,
+ nativeMax = Math.max,
+ nativeNow = Date.now;
+
+/* Built-in method references that are verified to be native. */
+var Map = getNative(root, 'Map'),
+ nativeCreate = getNative(Object, 'create');
+
+/**
+ * The base implementation of `_.create` without support for assigning
+ * properties to the created object.
+ *
+ * @private
+ * @param {Object} proto The object to inherit from.
+ * @returns {Object} Returns the new object.
+ */
+var baseCreate = (function() {
+ function object() {}
+ return function(proto) {
+ if (!isObject(proto)) {
+ return {};
+ }
+ if (objectCreate) {
+ return objectCreate(proto);
+ }
+ object.prototype = proto;
+ var result = new object;
+ object.prototype = undefined;
+ return result;
+ };
+}());
+
+/**
+ * Creates a hash object.
+ *
+ * @private
+ * @constructor
+ * @param {Array} [entries] The key-value pairs to cache.
+ */
+function Hash(entries) {
+ var index = -1,
+ length = entries == null ? 0 : entries.length;
+
+ this.clear();
+ while (++index < length) {
+ var entry = entries[index];
+ this.set(entry[0], entry[1]);
+ }
+}
+
+/**
+ * Removes all key-value entries from the hash.
+ *
+ * @private
+ * @name clear
+ * @memberOf Hash
+ */
+function hashClear() {
+ this.__data__ = nativeCreate ? nativeCreate(null) : {};
+ this.size = 0;
+}
+
+/**
+ * Removes `key` and its value from the hash.
+ *
+ * @private
+ * @name delete
+ * @memberOf Hash
+ * @param {Object} hash The hash to modify.
+ * @param {string} key The key of the value to remove.
+ * @returns {boolean} Returns `true` if the entry was removed, else `false`.
+ */
+function hashDelete(key) {
+ var result = this.has(key) && delete this.__data__[key];
+ this.size -= result ? 1 : 0;
+ return result;
+}
+
+/**
+ * Gets the hash value for `key`.
+ *
+ * @private
+ * @name get
+ * @memberOf Hash
+ * @param {string} key The key of the value to get.
+ * @returns {*} Returns the entry value.
+ */
+function hashGet(key) {
+ var data = this.__data__;
+ if (nativeCreate) {
+ var result = data[key];
+ return result === HASH_UNDEFINED ? undefined : result;
+ }
+ return hasOwnProperty.call(data, key) ? data[key] : undefined;
+}
+
+/**
+ * Checks if a hash value for `key` exists.
+ *
+ * @private
+ * @name has
+ * @memberOf Hash
+ * @param {string} key The key of the entry to check.
+ * @returns {boolean} Returns `true` if an entry for `key` exists, else `false`.
+ */
+function hashHas(key) {
+ var data = this.__data__;
+ return nativeCreate ? (data[key] !== undefined) : hasOwnProperty.call(data, key);
+}
+
+/**
+ * Sets the hash `key` to `value`.
+ *
+ * @private
+ * @name set
+ * @memberOf Hash
+ * @param {string} key The key of the value to set.
+ * @param {*} value The value to set.
+ * @returns {Object} Returns the hash instance.
+ */
+function hashSet(key, value) {
+ var data = this.__data__;
+ this.size += this.has(key) ? 0 : 1;
+ data[key] = (nativeCreate && value === undefined) ? HASH_UNDEFINED : value;
+ return this;
+}
+
+// Add methods to `Hash`.
+Hash.prototype.clear = hashClear;
+Hash.prototype['delete'] = hashDelete;
+Hash.prototype.get = hashGet;
+Hash.prototype.has = hashHas;
+Hash.prototype.set = hashSet;
+
+/**
+ * Creates an list cache object.
+ *
+ * @private
+ * @constructor
+ * @param {Array} [entries] The key-value pairs to cache.
+ */
+function ListCache(entries) {
+ var index = -1,
+ length = entries == null ? 0 : entries.length;
+
+ this.clear();
+ while (++index < length) {
+ var entry = entries[index];
+ this.set(entry[0], entry[1]);
+ }
+}
+
+/**
+ * Removes all key-value entries from the list cache.
+ *
+ * @private
+ * @name clear
+ * @memberOf ListCache
+ */
+function listCacheClear() {
+ this.__data__ = [];
+ this.size = 0;
+}
+
+/**
+ * Removes `key` and its value from the list cache.
+ *
+ * @private
+ * @name delete
+ * @memberOf ListCache
+ * @param {string} key The key of the value to remove.
+ * @returns {boolean} Returns `true` if the entry was removed, else `false`.
+ */
+function listCacheDelete(key) {
+ var data = this.__data__,
+ index = assocIndexOf(data, key);
+
+ if (index < 0) {
+ return false;
+ }
+ var lastIndex = data.length - 1;
+ if (index == lastIndex) {
+ data.pop();
+ } else {
+ splice.call(data, index, 1);
+ }
+ --this.size;
+ return true;
+}
+
+/**
+ * Gets the list cache value for `key`.
+ *
+ * @private
+ * @name get
+ * @memberOf ListCache
+ * @param {string} key The key of the value to get.
+ * @returns {*} Returns the entry value.
+ */
+function listCacheGet(key) {
+ var data = this.__data__,
+ index = assocIndexOf(data, key);
+
+ return index < 0 ? undefined : data[index][1];
+}
+
+/**
+ * Checks if a list cache value for `key` exists.
+ *
+ * @private
+ * @name has
+ * @memberOf ListCache
+ * @param {string} key The key of the entry to check.
+ * @returns {boolean} Returns `true` if an entry for `key` exists, else `false`.
+ */
+function listCacheHas(key) {
+ return assocIndexOf(this.__data__, key) > -1;
+}
+
+/**
+ * Sets the list cache `key` to `value`.
+ *
+ * @private
+ * @name set
+ * @memberOf ListCache
+ * @param {string} key The key of the value to set.
+ * @param {*} value The value to set.
+ * @returns {Object} Returns the list cache instance.
+ */
+function listCacheSet(key, value) {
+ var data = this.__data__,
+ index = assocIndexOf(data, key);
+
+ if (index < 0) {
+ ++this.size;
+ data.push([key, value]);
+ } else {
+ data[index][1] = value;
+ }
+ return this;
+}
+
+// Add methods to `ListCache`.
+ListCache.prototype.clear = listCacheClear;
+ListCache.prototype['delete'] = listCacheDelete;
+ListCache.prototype.get = listCacheGet;
+ListCache.prototype.has = listCacheHas;
+ListCache.prototype.set = listCacheSet;
+
+/**
+ * Creates a map cache object to store key-value pairs.
+ *
+ * @private
+ * @constructor
+ * @param {Array} [entries] The key-value pairs to cache.
+ */
+function MapCache(entries) {
+ var index = -1,
+ length = entries == null ? 0 : entries.length;
+
+ this.clear();
+ while (++index < length) {
+ var entry = entries[index];
+ this.set(entry[0], entry[1]);
+ }
+}
+
+/**
+ * Removes all key-value entries from the map.
+ *
+ * @private
+ * @name clear
+ * @memberOf MapCache
+ */
+function mapCacheClear() {
+ this.size = 0;
+ this.__data__ = {
+ 'hash': new Hash,
+ 'map': new (Map || ListCache),
+ 'string': new Hash
+ };
+}
+
+/**
+ * Removes `key` and its value from the map.
+ *
+ * @private
+ * @name delete
+ * @memberOf MapCache
+ * @param {string} key The key of the value to remove.
+ * @returns {boolean} Returns `true` if the entry was removed, else `false`.
+ */
+function mapCacheDelete(key) {
+ var result = getMapData(this, key)['delete'](key);
+ this.size -= result ? 1 : 0;
+ return result;
+}
+
+/**
+ * Gets the map value for `key`.
+ *
+ * @private
+ * @name get
+ * @memberOf MapCache
+ * @param {string} key The key of the value to get.
+ * @returns {*} Returns the entry value.
+ */
+function mapCacheGet(key) {
+ return getMapData(this, key).get(key);
+}
+
+/**
+ * Checks if a map value for `key` exists.
+ *
+ * @private
+ * @name has
+ * @memberOf MapCache
+ * @param {string} key The key of the entry to check.
+ * @returns {boolean} Returns `true` if an entry for `key` exists, else `false`.
+ */
+function mapCacheHas(key) {
+ return getMapData(this, key).has(key);
+}
+
+/**
+ * Sets the map `key` to `value`.
+ *
+ * @private
+ * @name set
+ * @memberOf MapCache
+ * @param {string} key The key of the value to set.
+ * @param {*} value The value to set.
+ * @returns {Object} Returns the map cache instance.
+ */
+function mapCacheSet(key, value) {
+ var data = getMapData(this, key),
+ size = data.size;
+
+ data.set(key, value);
+ this.size += data.size == size ? 0 : 1;
+ return this;
+}
+
+// Add methods to `MapCache`.
+MapCache.prototype.clear = mapCacheClear;
+MapCache.prototype['delete'] = mapCacheDelete;
+MapCache.prototype.get = mapCacheGet;
+MapCache.prototype.has = mapCacheHas;
+MapCache.prototype.set = mapCacheSet;
+
+/**
+ * Creates a stack cache object to store key-value pairs.
+ *
+ * @private
+ * @constructor
+ * @param {Array} [entries] The key-value pairs to cache.
+ */
+function Stack(entries) {
+ var data = this.__data__ = new ListCache(entries);
+ this.size = data.size;
+}
+
+/**
+ * Removes all key-value entries from the stack.
+ *
+ * @private
+ * @name clear
+ * @memberOf Stack
+ */
+function stackClear() {
+ this.__data__ = new ListCache;
+ this.size = 0;
+}
+
+/**
+ * Removes `key` and its value from the stack.
+ *
+ * @private
+ * @name delete
+ * @memberOf Stack
+ * @param {string} key The key of the value to remove.
+ * @returns {boolean} Returns `true` if the entry was removed, else `false`.
+ */
+function stackDelete(key) {
+ var data = this.__data__,
+ result = data['delete'](key);
+
+ this.size = data.size;
+ return result;
+}
+
+/**
+ * Gets the stack value for `key`.
+ *
+ * @private
+ * @name get
+ * @memberOf Stack
+ * @param {string} key The key of the value to get.
+ * @returns {*} Returns the entry value.
+ */
+function stackGet(key) {
+ return this.__data__.get(key);
+}
+
+/**
+ * Checks if a stack value for `key` exists.
+ *
+ * @private
+ * @name has
+ * @memberOf Stack
+ * @param {string} key The key of the entry to check.
+ * @returns {boolean} Returns `true` if an entry for `key` exists, else `false`.
+ */
+function stackHas(key) {
+ return this.__data__.has(key);
+}
+
+/**
+ * Sets the stack `key` to `value`.
+ *
+ * @private
+ * @name set
+ * @memberOf Stack
+ * @param {string} key The key of the value to set.
+ * @param {*} value The value to set.
+ * @returns {Object} Returns the stack cache instance.
+ */
+function stackSet(key, value) {
+ var data = this.__data__;
+ if (data instanceof ListCache) {
+ var pairs = data.__data__;
+ if (!Map || (pairs.length < LARGE_ARRAY_SIZE - 1)) {
+ pairs.push([key, value]);
+ this.size = ++data.size;
+ return this;
+ }
+ data = this.__data__ = new MapCache(pairs);
+ }
+ data.set(key, value);
+ this.size = data.size;
+ return this;
+}
+
+// Add methods to `Stack`.
+Stack.prototype.clear = stackClear;
+Stack.prototype['delete'] = stackDelete;
+Stack.prototype.get = stackGet;
+Stack.prototype.has = stackHas;
+Stack.prototype.set = stackSet;
+
+/**
+ * Creates an array of the enumerable property names of the array-like `value`.
+ *
+ * @private
+ * @param {*} value The value to query.
+ * @param {boolean} inherited Specify returning inherited property names.
+ * @returns {Array} Returns the array of property names.
+ */
+function arrayLikeKeys(value, inherited) {
+ var isArr = isArray(value),
+ isArg = !isArr && isArguments(value),
+ isBuff = !isArr && !isArg && isBuffer(value),
+ isType = !isArr && !isArg && !isBuff && isTypedArray(value),
+ skipIndexes = isArr || isArg || isBuff || isType,
+ result = skipIndexes ? baseTimes(value.length, String) : [],
+ length = result.length;
+
+ for (var key in value) {
+ if ((inherited || hasOwnProperty.call(value, key)) &&
+ !(skipIndexes && (
+ // Safari 9 has enumerable `arguments.length` in strict mode.
+ key == 'length' ||
+ // Node.js 0.10 has enumerable non-index properties on buffers.
+ (isBuff && (key == 'offset' || key == 'parent')) ||
+ // PhantomJS 2 has enumerable non-index properties on typed arrays.
+ (isType && (key == 'buffer' || key == 'byteLength' || key == 'byteOffset')) ||
+ // Skip index properties.
+ isIndex(key, length)
+ ))) {
+ result.push(key);
+ }
+ }
+ return result;
+}
+
+/**
+ * This function is like `assignValue` except that it doesn't assign
+ * `undefined` values.
+ *
+ * @private
+ * @param {Object} object The object to modify.
+ * @param {string} key The key of the property to assign.
+ * @param {*} value The value to assign.
+ */
+function assignMergeValue(object, key, value) {
+ if ((value !== undefined && !eq(object[key], value)) ||
+ (value === undefined && !(key in object))) {
+ baseAssignValue(object, key, value);
+ }
+}
+
+/**
+ * Assigns `value` to `key` of `object` if the existing value is not equivalent
+ * using [`SameValueZero`](http://ecma-international.org/ecma-262/7.0/#sec-samevaluezero)
+ * for equality comparisons.
+ *
+ * @private
+ * @param {Object} object The object to modify.
+ * @param {string} key The key of the property to assign.
+ * @param {*} value The value to assign.
+ */
+function assignValue(object, key, value) {
+ var objValue = object[key];
+ if (!(hasOwnProperty.call(object, key) && eq(objValue, value)) ||
+ (value === undefined && !(key in object))) {
+ baseAssignValue(object, key, value);
+ }
+}
+
+/**
+ * Gets the index at which the `key` is found in `array` of key-value pairs.
+ *
+ * @private
+ * @param {Array} array The array to inspect.
+ * @param {*} key The key to search for.
+ * @returns {number} Returns the index of the matched value, else `-1`.
+ */
+function assocIndexOf(array, key) {
+ var length = array.length;
+ while (length--) {
+ if (eq(array[length][0], key)) {
+ return length;
+ }
+ }
+ return -1;
+}
+
+/**
+ * The base implementation of `assignValue` and `assignMergeValue` without
+ * value checks.
+ *
+ * @private
+ * @param {Object} object The object to modify.
+ * @param {string} key The key of the property to assign.
+ * @param {*} value The value to assign.
+ */
+function baseAssignValue(object, key, value) {
+ if (key == '__proto__' && defineProperty) {
+ defineProperty(object, key, {
+ 'configurable': true,
+ 'enumerable': true,
+ 'value': value,
+ 'writable': true
+ });
+ } else {
+ object[key] = value;
+ }
+}
+
+/**
+ * The base implementation of `baseForOwn` which iterates over `object`
+ * properties returned by `keysFunc` and invokes `iteratee` for each property.
+ * Iteratee functions may exit iteration early by explicitly returning `false`.
+ *
+ * @private
+ * @param {Object} object The object to iterate over.
+ * @param {Function} iteratee The function invoked per iteration.
+ * @param {Function} keysFunc The function to get the keys of `object`.
+ * @returns {Object} Returns `object`.
+ */
+var baseFor = createBaseFor();
+
+/**
+ * The base implementation of `getTag` without fallbacks for buggy environments.
+ *
+ * @private
+ * @param {*} value The value to query.
+ * @returns {string} Returns the `toStringTag`.
+ */
+function baseGetTag(value) {
+ if (value == null) {
+ return value === undefined ? undefinedTag : nullTag;
+ }
+ return (symToStringTag && symToStringTag in Object(value))
+ ? getRawTag(value)
+ : objectToString(value);
+}
+
+/**
+ * The base implementation of `_.isArguments`.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is an `arguments` object,
+ */
+function baseIsArguments(value) {
+ return isObjectLike(value) && baseGetTag(value) == argsTag;
+}
+
+/**
+ * The base implementation of `_.isNative` without bad shim checks.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a native function,
+ * else `false`.
+ */
+function baseIsNative(value) {
+ if (!isObject(value) || isMasked(value)) {
+ return false;
+ }
+ var pattern = isFunction(value) ? reIsNative : reIsHostCtor;
+ return pattern.test(toSource(value));
+}
+
+/**
+ * The base implementation of `_.isTypedArray` without Node.js optimizations.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a typed array, else `false`.
+ */
+function baseIsTypedArray(value) {
+ return isObjectLike(value) &&
+ isLength(value.length) && !!typedArrayTags[baseGetTag(value)];
+}
+
+/**
+ * The base implementation of `_.keysIn` which doesn't treat sparse arrays as dense.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the array of property names.
+ */
+function baseKeysIn(object) {
+ if (!isObject(object)) {
+ return nativeKeysIn(object);
+ }
+ var isProto = isPrototype(object),
+ result = [];
+
+ for (var key in object) {
+ if (!(key == 'constructor' && (isProto || !hasOwnProperty.call(object, key)))) {
+ result.push(key);
+ }
+ }
+ return result;
+}
+
+/**
+ * The base implementation of `_.merge` without support for multiple sources.
+ *
+ * @private
+ * @param {Object} object The destination object.
+ * @param {Object} source The source object.
+ * @param {number} srcIndex The index of `source`.
+ * @param {Function} [customizer] The function to customize merged values.
+ * @param {Object} [stack] Tracks traversed source values and their merged
+ * counterparts.
+ */
+function baseMerge(object, source, srcIndex, customizer, stack) {
+ if (object === source) {
+ return;
+ }
+ baseFor(source, function(srcValue, key) {
+ if (isObject(srcValue)) {
+ stack || (stack = new Stack);
+ baseMergeDeep(object, source, key, srcIndex, baseMerge, customizer, stack);
+ }
+ else {
+ var newValue = customizer
+ ? customizer(safeGet(object, key), srcValue, (key + ''), object, source, stack)
+ : undefined;
+
+ if (newValue === undefined) {
+ newValue = srcValue;
+ }
+ assignMergeValue(object, key, newValue);
+ }
+ }, keysIn);
+}
+
+/**
+ * A specialized version of `baseMerge` for arrays and objects which performs
+ * deep merges and tracks traversed objects enabling objects with circular
+ * references to be merged.
+ *
+ * @private
+ * @param {Object} object The destination object.
+ * @param {Object} source The source object.
+ * @param {string} key The key of the value to merge.
+ * @param {number} srcIndex The index of `source`.
+ * @param {Function} mergeFunc The function to merge values.
+ * @param {Function} [customizer] The function to customize assigned values.
+ * @param {Object} [stack] Tracks traversed source values and their merged
+ * counterparts.
+ */
+function baseMergeDeep(object, source, key, srcIndex, mergeFunc, customizer, stack) {
+ var objValue = safeGet(object, key),
+ srcValue = safeGet(source, key),
+ stacked = stack.get(srcValue);
+
+ if (stacked) {
+ assignMergeValue(object, key, stacked);
+ return;
+ }
+ var newValue = customizer
+ ? customizer(objValue, srcValue, (key + ''), object, source, stack)
+ : undefined;
+
+ var isCommon = newValue === undefined;
+
+ if (isCommon) {
+ var isArr = isArray(srcValue),
+ isBuff = !isArr && isBuffer(srcValue),
+ isTyped = !isArr && !isBuff && isTypedArray(srcValue);
+
+ newValue = srcValue;
+ if (isArr || isBuff || isTyped) {
+ if (isArray(objValue)) {
+ newValue = objValue;
+ }
+ else if (isArrayLikeObject(objValue)) {
+ newValue = copyArray(objValue);
+ }
+ else if (isBuff) {
+ isCommon = false;
+ newValue = cloneBuffer(srcValue, true);
+ }
+ else if (isTyped) {
+ isCommon = false;
+ newValue = cloneTypedArray(srcValue, true);
+ }
+ else {
+ newValue = [];
+ }
+ }
+ else if (isPlainObject(srcValue) || isArguments(srcValue)) {
+ newValue = objValue;
+ if (isArguments(objValue)) {
+ newValue = toPlainObject(objValue);
+ }
+ else if (!isObject(objValue) || (srcIndex && isFunction(objValue))) {
+ newValue = initCloneObject(srcValue);
+ }
+ }
+ else {
+ isCommon = false;
+ }
+ }
+ if (isCommon) {
+ // Recursively merge objects and arrays (susceptible to call stack limits).
+ stack.set(srcValue, newValue);
+ mergeFunc(newValue, srcValue, srcIndex, customizer, stack);
+ stack['delete'](srcValue);
+ }
+ assignMergeValue(object, key, newValue);
+}
+
+/**
+ * The base implementation of `_.rest` which doesn't validate or coerce arguments.
+ *
+ * @private
+ * @param {Function} func The function to apply a rest parameter to.
+ * @param {number} [start=func.length-1] The start position of the rest parameter.
+ * @returns {Function} Returns the new function.
+ */
+function baseRest(func, start) {
+ return setToString(overRest(func, start, identity), func + '');
+}
+
+/**
+ * The base implementation of `setToString` without support for hot loop shorting.
+ *
+ * @private
+ * @param {Function} func The function to modify.
+ * @param {Function} string The `toString` result.
+ * @returns {Function} Returns `func`.
+ */
+var baseSetToString = !defineProperty ? identity : function(func, string) {
+ return defineProperty(func, 'toString', {
+ 'configurable': true,
+ 'enumerable': false,
+ 'value': constant(string),
+ 'writable': true
+ });
+};
+
+/**
+ * Creates a clone of `buffer`.
+ *
+ * @private
+ * @param {Buffer} buffer The buffer to clone.
+ * @param {boolean} [isDeep] Specify a deep clone.
+ * @returns {Buffer} Returns the cloned buffer.
+ */
+function cloneBuffer(buffer, isDeep) {
+ if (isDeep) {
+ return buffer.slice();
+ }
+ var length = buffer.length,
+ result = allocUnsafe ? allocUnsafe(length) : new buffer.constructor(length);
+
+ buffer.copy(result);
+ return result;
+}
+
+/**
+ * Creates a clone of `arrayBuffer`.
+ *
+ * @private
+ * @param {ArrayBuffer} arrayBuffer The array buffer to clone.
+ * @returns {ArrayBuffer} Returns the cloned array buffer.
+ */
+function cloneArrayBuffer(arrayBuffer) {
+ var result = new arrayBuffer.constructor(arrayBuffer.byteLength);
+ new Uint8Array(result).set(new Uint8Array(arrayBuffer));
+ return result;
+}
+
+/**
+ * Creates a clone of `typedArray`.
+ *
+ * @private
+ * @param {Object} typedArray The typed array to clone.
+ * @param {boolean} [isDeep] Specify a deep clone.
+ * @returns {Object} Returns the cloned typed array.
+ */
+function cloneTypedArray(typedArray, isDeep) {
+ var buffer = isDeep ? cloneArrayBuffer(typedArray.buffer) : typedArray.buffer;
+ return new typedArray.constructor(buffer, typedArray.byteOffset, typedArray.length);
+}
+
+/**
+ * Copies the values of `source` to `array`.
+ *
+ * @private
+ * @param {Array} source The array to copy values from.
+ * @param {Array} [array=[]] The array to copy values to.
+ * @returns {Array} Returns `array`.
+ */
+function copyArray(source, array) {
+ var index = -1,
+ length = source.length;
+
+ array || (array = Array(length));
+ while (++index < length) {
+ array[index] = source[index];
+ }
+ return array;
+}
+
+/**
+ * Copies properties of `source` to `object`.
+ *
+ * @private
+ * @param {Object} source The object to copy properties from.
+ * @param {Array} props The property identifiers to copy.
+ * @param {Object} [object={}] The object to copy properties to.
+ * @param {Function} [customizer] The function to customize copied values.
+ * @returns {Object} Returns `object`.
+ */
+function copyObject(source, props, object, customizer) {
+ var isNew = !object;
+ object || (object = {});
+
+ var index = -1,
+ length = props.length;
+
+ while (++index < length) {
+ var key = props[index];
+
+ var newValue = customizer
+ ? customizer(object[key], source[key], key, object, source)
+ : undefined;
+
+ if (newValue === undefined) {
+ newValue = source[key];
+ }
+ if (isNew) {
+ baseAssignValue(object, key, newValue);
+ } else {
+ assignValue(object, key, newValue);
+ }
+ }
+ return object;
+}
+
+/**
+ * Creates a function like `_.assign`.
+ *
+ * @private
+ * @param {Function} assigner The function to assign values.
+ * @returns {Function} Returns the new assigner function.
+ */
+function createAssigner(assigner) {
+ return baseRest(function(object, sources) {
+ var index = -1,
+ length = sources.length,
+ customizer = length > 1 ? sources[length - 1] : undefined,
+ guard = length > 2 ? sources[2] : undefined;
+
+ customizer = (assigner.length > 3 && typeof customizer == 'function')
+ ? (length--, customizer)
+ : undefined;
+
+ if (guard && isIterateeCall(sources[0], sources[1], guard)) {
+ customizer = length < 3 ? undefined : customizer;
+ length = 1;
+ }
+ object = Object(object);
+ while (++index < length) {
+ var source = sources[index];
+ if (source) {
+ assigner(object, source, index, customizer);
+ }
+ }
+ return object;
+ });
+}
+
+/**
+ * Creates a base function for methods like `_.forIn` and `_.forOwn`.
+ *
+ * @private
+ * @param {boolean} [fromRight] Specify iterating from right to left.
+ * @returns {Function} Returns the new base function.
+ */
+function createBaseFor(fromRight) {
+ return function(object, iteratee, keysFunc) {
+ var index = -1,
+ iterable = Object(object),
+ props = keysFunc(object),
+ length = props.length;
+
+ while (length--) {
+ var key = props[fromRight ? length : ++index];
+ if (iteratee(iterable[key], key, iterable) === false) {
+ break;
+ }
+ }
+ return object;
+ };
+}
+
+/**
+ * Gets the data for `map`.
+ *
+ * @private
+ * @param {Object} map The map to query.
+ * @param {string} key The reference key.
+ * @returns {*} Returns the map data.
+ */
+function getMapData(map, key) {
+ var data = map.__data__;
+ return isKeyable(key)
+ ? data[typeof key == 'string' ? 'string' : 'hash']
+ : data.map;
+}
+
+/**
+ * Gets the native function at `key` of `object`.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @param {string} key The key of the method to get.
+ * @returns {*} Returns the function if it's native, else `undefined`.
+ */
+function getNative(object, key) {
+ var value = getValue(object, key);
+ return baseIsNative(value) ? value : undefined;
+}
+
+/**
+ * A specialized version of `baseGetTag` which ignores `Symbol.toStringTag` values.
+ *
+ * @private
+ * @param {*} value The value to query.
+ * @returns {string} Returns the raw `toStringTag`.
+ */
+function getRawTag(value) {
+ var isOwn = hasOwnProperty.call(value, symToStringTag),
+ tag = value[symToStringTag];
+
+ try {
+ value[symToStringTag] = undefined;
+ var unmasked = true;
+ } catch (e) {}
+
+ var result = nativeObjectToString.call(value);
+ if (unmasked) {
+ if (isOwn) {
+ value[symToStringTag] = tag;
+ } else {
+ delete value[symToStringTag];
+ }
+ }
+ return result;
+}
+
+/**
+ * Initializes an object clone.
+ *
+ * @private
+ * @param {Object} object The object to clone.
+ * @returns {Object} Returns the initialized clone.
+ */
+function initCloneObject(object) {
+ return (typeof object.constructor == 'function' && !isPrototype(object))
+ ? baseCreate(getPrototype(object))
+ : {};
+}
+
+/**
+ * Checks if `value` is a valid array-like index.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @param {number} [length=MAX_SAFE_INTEGER] The upper bounds of a valid index.
+ * @returns {boolean} Returns `true` if `value` is a valid index, else `false`.
+ */
+function isIndex(value, length) {
+ var type = typeof value;
+ length = length == null ? MAX_SAFE_INTEGER : length;
+
+ return !!length &&
+ (type == 'number' ||
+ (type != 'symbol' && reIsUint.test(value))) &&
+ (value > -1 && value % 1 == 0 && value < length);
+}
+
+/**
+ * Checks if the given arguments are from an iteratee call.
+ *
+ * @private
+ * @param {*} value The potential iteratee value argument.
+ * @param {*} index The potential iteratee index or key argument.
+ * @param {*} object The potential iteratee object argument.
+ * @returns {boolean} Returns `true` if the arguments are from an iteratee call,
+ * else `false`.
+ */
+function isIterateeCall(value, index, object) {
+ if (!isObject(object)) {
+ return false;
+ }
+ var type = typeof index;
+ if (type == 'number'
+ ? (isArrayLike(object) && isIndex(index, object.length))
+ : (type == 'string' && index in object)
+ ) {
+ return eq(object[index], value);
+ }
+ return false;
+}
+
+/**
+ * Checks if `value` is suitable for use as unique object key.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is suitable, else `false`.
+ */
+function isKeyable(value) {
+ var type = typeof value;
+ return (type == 'string' || type == 'number' || type == 'symbol' || type == 'boolean')
+ ? (value !== '__proto__')
+ : (value === null);
+}
+
+/**
+ * Checks if `func` has its source masked.
+ *
+ * @private
+ * @param {Function} func The function to check.
+ * @returns {boolean} Returns `true` if `func` is masked, else `false`.
+ */
+function isMasked(func) {
+ return !!maskSrcKey && (maskSrcKey in func);
+}
+
+/**
+ * Checks if `value` is likely a prototype object.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a prototype, else `false`.
+ */
+function isPrototype(value) {
+ var Ctor = value && value.constructor,
+ proto = (typeof Ctor == 'function' && Ctor.prototype) || objectProto;
+
+ return value === proto;
+}
+
+/**
+ * This function is like
+ * [`Object.keys`](http://ecma-international.org/ecma-262/7.0/#sec-object.keys)
+ * except that it includes inherited enumerable properties.
+ *
+ * @private
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the array of property names.
+ */
+function nativeKeysIn(object) {
+ var result = [];
+ if (object != null) {
+ for (var key in Object(object)) {
+ result.push(key);
+ }
+ }
+ return result;
+}
+
+/**
+ * Converts `value` to a string using `Object.prototype.toString`.
+ *
+ * @private
+ * @param {*} value The value to convert.
+ * @returns {string} Returns the converted string.
+ */
+function objectToString(value) {
+ return nativeObjectToString.call(value);
+}
+
+/**
+ * A specialized version of `baseRest` which transforms the rest array.
+ *
+ * @private
+ * @param {Function} func The function to apply a rest parameter to.
+ * @param {number} [start=func.length-1] The start position of the rest parameter.
+ * @param {Function} transform The rest array transform.
+ * @returns {Function} Returns the new function.
+ */
+function overRest(func, start, transform) {
+ start = nativeMax(start === undefined ? (func.length - 1) : start, 0);
+ return function() {
+ var args = arguments,
+ index = -1,
+ length = nativeMax(args.length - start, 0),
+ array = Array(length);
+
+ while (++index < length) {
+ array[index] = args[start + index];
+ }
+ index = -1;
+ var otherArgs = Array(start + 1);
+ while (++index < start) {
+ otherArgs[index] = args[index];
+ }
+ otherArgs[start] = transform(array);
+ return apply(func, this, otherArgs);
+ };
+}
+
+/**
+ * Sets the `toString` method of `func` to return `string`.
+ *
+ * @private
+ * @param {Function} func The function to modify.
+ * @param {Function} string The `toString` result.
+ * @returns {Function} Returns `func`.
+ */
+var setToString = shortOut(baseSetToString);
+
+/**
+ * Creates a function that'll short out and invoke `identity` instead
+ * of `func` when it's called `HOT_COUNT` or more times in `HOT_SPAN`
+ * milliseconds.
+ *
+ * @private
+ * @param {Function} func The function to restrict.
+ * @returns {Function} Returns the new shortable function.
+ */
+function shortOut(func) {
+ var count = 0,
+ lastCalled = 0;
+
+ return function() {
+ var stamp = nativeNow(),
+ remaining = HOT_SPAN - (stamp - lastCalled);
+
+ lastCalled = stamp;
+ if (remaining > 0) {
+ if (++count >= HOT_COUNT) {
+ return arguments[0];
+ }
+ } else {
+ count = 0;
+ }
+ return func.apply(undefined, arguments);
+ };
+}
+
+/**
+ * Converts `func` to its source code.
+ *
+ * @private
+ * @param {Function} func The function to convert.
+ * @returns {string} Returns the source code.
+ */
+function toSource(func) {
+ if (func != null) {
+ try {
+ return funcToString.call(func);
+ } catch (e) {}
+ try {
+ return (func + '');
+ } catch (e) {}
+ }
+ return '';
+}
+
+/**
+ * Performs a
+ * [`SameValueZero`](http://ecma-international.org/ecma-262/7.0/#sec-samevaluezero)
+ * comparison between two values to determine if they are equivalent.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to compare.
+ * @param {*} other The other value to compare.
+ * @returns {boolean} Returns `true` if the values are equivalent, else `false`.
+ * @example
+ *
+ * var object = { 'a': 1 };
+ * var other = { 'a': 1 };
+ *
+ * _.eq(object, object);
+ * // => true
+ *
+ * _.eq(object, other);
+ * // => false
+ *
+ * _.eq('a', 'a');
+ * // => true
+ *
+ * _.eq('a', Object('a'));
+ * // => false
+ *
+ * _.eq(NaN, NaN);
+ * // => true
+ */
+function eq(value, other) {
+ return value === other || (value !== value && other !== other);
+}
+
+/**
+ * Checks if `value` is likely an `arguments` object.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is an `arguments` object,
+ * else `false`.
+ * @example
+ *
+ * _.isArguments(function() { return arguments; }());
+ * // => true
+ *
+ * _.isArguments([1, 2, 3]);
+ * // => false
+ */
+var isArguments = baseIsArguments(function() { return arguments; }()) ? baseIsArguments : function(value) {
+ return isObjectLike(value) && hasOwnProperty.call(value, 'callee') &&
+ !propertyIsEnumerable.call(value, 'callee');
+};
+
+/**
+ * Checks if `value` is classified as an `Array` object.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is an array, else `false`.
+ * @example
+ *
+ * _.isArray([1, 2, 3]);
+ * // => true
+ *
+ * _.isArray(document.body.children);
+ * // => false
+ *
+ * _.isArray('abc');
+ * // => false
+ *
+ * _.isArray(_.noop);
+ * // => false
+ */
+var isArray = Array.isArray;
+
+/**
+ * Checks if `value` is array-like. A value is considered array-like if it's
+ * not a function and has a `value.length` that's an integer greater than or
+ * equal to `0` and less than or equal to `Number.MAX_SAFE_INTEGER`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is array-like, else `false`.
+ * @example
+ *
+ * _.isArrayLike([1, 2, 3]);
+ * // => true
+ *
+ * _.isArrayLike(document.body.children);
+ * // => true
+ *
+ * _.isArrayLike('abc');
+ * // => true
+ *
+ * _.isArrayLike(_.noop);
+ * // => false
+ */
+function isArrayLike(value) {
+ return value != null && isLength(value.length) && !isFunction(value);
+}
+
+/**
+ * This method is like `_.isArrayLike` except that it also checks if `value`
+ * is an object.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is an array-like object,
+ * else `false`.
+ * @example
+ *
+ * _.isArrayLikeObject([1, 2, 3]);
+ * // => true
+ *
+ * _.isArrayLikeObject(document.body.children);
+ * // => true
+ *
+ * _.isArrayLikeObject('abc');
+ * // => false
+ *
+ * _.isArrayLikeObject(_.noop);
+ * // => false
+ */
+function isArrayLikeObject(value) {
+ return isObjectLike(value) && isArrayLike(value);
+}
+
+/**
+ * Checks if `value` is a buffer.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.3.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a buffer, else `false`.
+ * @example
+ *
+ * _.isBuffer(new Buffer(2));
+ * // => true
+ *
+ * _.isBuffer(new Uint8Array(2));
+ * // => false
+ */
+var isBuffer = nativeIsBuffer || stubFalse;
+
+/**
+ * Checks if `value` is classified as a `Function` object.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a function, else `false`.
+ * @example
+ *
+ * _.isFunction(_);
+ * // => true
+ *
+ * _.isFunction(/abc/);
+ * // => false
+ */
+function isFunction(value) {
+ if (!isObject(value)) {
+ return false;
+ }
+ // The use of `Object#toString` avoids issues with the `typeof` operator
+ // in Safari 9 which returns 'object' for typed arrays and other constructors.
+ var tag = baseGetTag(value);
+ return tag == funcTag || tag == genTag || tag == asyncTag || tag == proxyTag;
+}
+
+/**
+ * Checks if `value` is a valid array-like length.
+ *
+ * **Note:** This method is loosely based on
+ * [`ToLength`](http://ecma-international.org/ecma-262/7.0/#sec-tolength).
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a valid length, else `false`.
+ * @example
+ *
+ * _.isLength(3);
+ * // => true
+ *
+ * _.isLength(Number.MIN_VALUE);
+ * // => false
+ *
+ * _.isLength(Infinity);
+ * // => false
+ *
+ * _.isLength('3');
+ * // => false
+ */
+function isLength(value) {
+ return typeof value == 'number' &&
+ value > -1 && value % 1 == 0 && value <= MAX_SAFE_INTEGER;
+}
+
+/**
+ * Checks if `value` is the
+ * [language type](http://www.ecma-international.org/ecma-262/7.0/#sec-ecmascript-language-types)
+ * of `Object`. (e.g. arrays, functions, objects, regexes, `new Number(0)`, and `new String('')`)
+ *
+ * @static
+ * @memberOf _
+ * @since 0.1.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is an object, else `false`.
+ * @example
+ *
+ * _.isObject({});
+ * // => true
+ *
+ * _.isObject([1, 2, 3]);
+ * // => true
+ *
+ * _.isObject(_.noop);
+ * // => true
+ *
+ * _.isObject(null);
+ * // => false
+ */
+function isObject(value) {
+ var type = typeof value;
+ return value != null && (type == 'object' || type == 'function');
+}
+
+/**
+ * Checks if `value` is object-like. A value is object-like if it's not `null`
+ * and has a `typeof` result of "object".
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is object-like, else `false`.
+ * @example
+ *
+ * _.isObjectLike({});
+ * // => true
+ *
+ * _.isObjectLike([1, 2, 3]);
+ * // => true
+ *
+ * _.isObjectLike(_.noop);
+ * // => false
+ *
+ * _.isObjectLike(null);
+ * // => false
+ */
+function isObjectLike(value) {
+ return value != null && typeof value == 'object';
+}
+
+/**
+ * Checks if `value` is a plain object, that is, an object created by the
+ * `Object` constructor or one with a `[[Prototype]]` of `null`.
+ *
+ * @static
+ * @memberOf _
+ * @since 0.8.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a plain object, else `false`.
+ * @example
+ *
+ * function Foo() {
+ * this.a = 1;
+ * }
+ *
+ * _.isPlainObject(new Foo);
+ * // => false
+ *
+ * _.isPlainObject([1, 2, 3]);
+ * // => false
+ *
+ * _.isPlainObject({ 'x': 0, 'y': 0 });
+ * // => true
+ *
+ * _.isPlainObject(Object.create(null));
+ * // => true
+ */
+function isPlainObject(value) {
+ if (!isObjectLike(value) || baseGetTag(value) != objectTag) {
+ return false;
+ }
+ var proto = getPrototype(value);
+ if (proto === null) {
+ return true;
+ }
+ var Ctor = hasOwnProperty.call(proto, 'constructor') && proto.constructor;
+ return typeof Ctor == 'function' && Ctor instanceof Ctor &&
+ funcToString.call(Ctor) == objectCtorString;
+}
+
+/**
+ * Checks if `value` is classified as a typed array.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is a typed array, else `false`.
+ * @example
+ *
+ * _.isTypedArray(new Uint8Array);
+ * // => true
+ *
+ * _.isTypedArray([]);
+ * // => false
+ */
+var isTypedArray = nodeIsTypedArray ? baseUnary(nodeIsTypedArray) : baseIsTypedArray;
+
+/**
+ * Converts `value` to a plain object flattening inherited enumerable string
+ * keyed properties of `value` to own properties of the plain object.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Lang
+ * @param {*} value The value to convert.
+ * @returns {Object} Returns the converted plain object.
+ * @example
+ *
+ * function Foo() {
+ * this.b = 2;
+ * }
+ *
+ * Foo.prototype.c = 3;
+ *
+ * _.assign({ 'a': 1 }, new Foo);
+ * // => { 'a': 1, 'b': 2 }
+ *
+ * _.assign({ 'a': 1 }, _.toPlainObject(new Foo));
+ * // => { 'a': 1, 'b': 2, 'c': 3 }
+ */
+function toPlainObject(value) {
+ return copyObject(value, keysIn(value));
+}
+
+/**
+ * Creates an array of the own and inherited enumerable property names of `object`.
+ *
+ * **Note:** Non-object values are coerced to objects.
+ *
+ * @static
+ * @memberOf _
+ * @since 3.0.0
+ * @category Object
+ * @param {Object} object The object to query.
+ * @returns {Array} Returns the array of property names.
+ * @example
+ *
+ * function Foo() {
+ * this.a = 1;
+ * this.b = 2;
+ * }
+ *
+ * Foo.prototype.c = 3;
+ *
+ * _.keysIn(new Foo);
+ * // => ['a', 'b', 'c'] (iteration order is not guaranteed)
+ */
+function keysIn(object) {
+ return isArrayLike(object) ? arrayLikeKeys(object, true) : baseKeysIn(object);
+}
+
+/**
+ * This method is like `_.merge` except that it accepts `customizer` which
+ * is invoked to produce the merged values of the destination and source
+ * properties. If `customizer` returns `undefined`, merging is handled by the
+ * method instead. The `customizer` is invoked with six arguments:
+ * (objValue, srcValue, key, object, source, stack).
+ *
+ * **Note:** This method mutates `object`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.0.0
+ * @category Object
+ * @param {Object} object The destination object.
+ * @param {...Object} sources The source objects.
+ * @param {Function} customizer The function to customize assigned values.
+ * @returns {Object} Returns `object`.
+ * @example
+ *
+ * function customizer(objValue, srcValue) {
+ * if (_.isArray(objValue)) {
+ * return objValue.concat(srcValue);
+ * }
+ * }
+ *
+ * var object = { 'a': [1], 'b': [2] };
+ * var other = { 'a': [3], 'b': [4] };
+ *
+ * _.mergeWith(object, other, customizer);
+ * // => { 'a': [1, 3], 'b': [2, 4] }
+ */
+var mergeWith = createAssigner(function(object, source, srcIndex, customizer) {
+ baseMerge(object, source, srcIndex, customizer);
+});
+
+/**
+ * Creates a function that returns `value`.
+ *
+ * @static
+ * @memberOf _
+ * @since 2.4.0
+ * @category Util
+ * @param {*} value The value to return from the new function.
+ * @returns {Function} Returns the new constant function.
+ * @example
+ *
+ * var objects = _.times(2, _.constant({ 'a': 1 }));
+ *
+ * console.log(objects);
+ * // => [{ 'a': 1 }, { 'a': 1 }]
+ *
+ * console.log(objects[0] === objects[1]);
+ * // => true
+ */
+function constant(value) {
+ return function() {
+ return value;
+ };
+}
+
+/**
+ * This method returns the first argument it receives.
+ *
+ * @static
+ * @since 0.1.0
+ * @memberOf _
+ * @category Util
+ * @param {*} value Any value.
+ * @returns {*} Returns `value`.
+ * @example
+ *
+ * var object = { 'a': 1 };
+ *
+ * console.log(_.identity(object) === object);
+ * // => true
+ */
+function identity(value) {
+ return value;
+}
+
+/**
+ * This method returns `false`.
+ *
+ * @static
+ * @memberOf _
+ * @since 4.13.0
+ * @category Util
+ * @returns {boolean} Returns `false`.
+ * @example
+ *
+ * _.times(2, _.stubFalse);
+ * // => [false, false]
+ */
+function stubFalse() {
+ return false;
+}
+
+module.exports = mergeWith;
diff --git a/node_modules/lodash.mergewith/package.json b/node_modules/lodash.mergewith/package.json
new file mode 100644
index 0000000..29fd365
--- /dev/null
+++ b/node_modules/lodash.mergewith/package.json
@@ -0,0 +1,64 @@
+{
+ "_from": "lodash.mergewith@^4.6.0",
+ "_id": "lodash.mergewith@4.6.1",
+ "_inBundle": false,
+ "_integrity": "sha512-eWw5r+PYICtEBgrBE5hhlT6aAa75f411bgDz/ZL2KZqYV03USvucsxcHUIlGTDTECs1eunpI7HOV7U+WLDvNdQ==",
+ "_location": "/lodash.mergewith",
+ "_phantomChildren": {},
+ "_requested": {
+ "type": "range",
+ "registry": true,
+ "raw": "lodash.mergewith@^4.6.0",
+ "name": "lodash.mergewith",
+ "escapedName": "lodash.mergewith",
+ "rawSpec": "^4.6.0",
+ "saveSpec": null,
+ "fetchSpec": "^4.6.0"
+ },
+ "_requiredBy": [
+ "/node-sass"
+ ],
+ "_resolved": "https://registry.npmjs.org/lodash.mergewith/-/lodash.mergewith-4.6.1.tgz",
+ "_shasum": "639057e726c3afbdb3e7d42741caa8d6e4335927",
+ "_spec": "lodash.mergewith@^4.6.0",
+ "_where": "/Users/rpatra16/MyProjects/RamAndAntara/node_modules/node-sass",
+ "author": {
+ "name": "John-David Dalton",
+ "email": "john.david.dalton@gmail.com",
+ "url": "http://allyoucanleet.com/"
+ },
+ "bugs": {
+ "url": "https://github.com/lodash/lodash/issues"
+ },
+ "bundleDependencies": false,
+ "contributors": [
+ {
+ "name": "John-David Dalton",
+ "email": "john.david.dalton@gmail.com",
+ "url": "http://allyoucanleet.com/"
+ },
+ {
+ "name": "Mathias Bynens",
+ "email": "mathias@qiwi.be",
+ "url": "https://mathiasbynens.be/"
+ }
+ ],
+ "deprecated": false,
+ "description": "The Lodash method `_.mergeWith` exported as a module.",
+ "homepage": "https://lodash.com/",
+ "icon": "https://lodash.com/icon.svg",
+ "keywords": [
+ "lodash-modularized",
+ "mergewith"
+ ],
+ "license": "MIT",
+ "name": "lodash.mergewith",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/lodash/lodash.git"
+ },
+ "scripts": {
+ "test": "echo \"See https://travis-ci.org/lodash/lodash-cli for testing details.\""
+ },
+ "version": "4.6.1"
+}
diff --git a/node_modules/lodash.restparam/LICENSE.txt b/node_modules/lodash.restparam/LICENSE.txt
new file mode 100644
index 0000000..9cd87e5
--- /dev/null
+++ b/node_modules/lodash.restparam/LICENSE.txt
@@ -0,0 +1,22 @@
+Copyright 2012-2015 The Dojo Foundation
+Based on Underscore.js, copyright 2009-2015 Jeremy Ashkenas,
+DocumentCloud and Investigative Reporters & Editors
+
+Permission is hereby granted, free of charge, to any person obtaining
+a copy of this software and associated documentation files (the
+"Software"), to deal in the Software without restriction, including
+without limitation the rights to use, copy, modify, merge, publish,
+distribute, sublicense, and/or sell copies of the Software, and to
+permit persons to whom the Software is furnished to do so, subject to
+the following conditions:
+
+The above copyright notice and this permission notice shall be
+included in all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
+EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
+MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
+NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
+LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
+OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
+WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
diff --git a/node_modules/lodash.restparam/README.md b/node_modules/lodash.restparam/README.md
new file mode 100644
index 0000000..80e47a4
--- /dev/null
+++ b/node_modules/lodash.restparam/README.md
@@ -0,0 +1,20 @@
+# lodash.restparam v3.6.1
+
+The [modern build](https://github.com/lodash/lodash/wiki/Build-Differences) of [lodash’s](https://lodash.com/) `_.restParam` exported as a [Node.js](http://nodejs.org/)/[io.js](https://iojs.org/) module.
+
+## Installation
+
+Using npm:
+
+```bash
+$ {sudo -H} npm i -g npm
+$ npm i --save lodash.restparam
+```
+
+In Node.js/io.js:
+
+```js
+var restParam = require('lodash.restparam');
+```
+
+See the [documentation](https://lodash.com/docs#restParam) or [package source](https://github.com/lodash/lodash/blob/3.6.1-npm-packages/lodash.restparam) for more details.
diff --git a/node_modules/lodash.restparam/index.js b/node_modules/lodash.restparam/index.js
new file mode 100644
index 0000000..932f47a
--- /dev/null
+++ b/node_modules/lodash.restparam/index.js
@@ -0,0 +1,67 @@
+/**
+ * lodash 3.6.1 (Custom Build)
+ * Build: `lodash modern modularize exports="npm" -o ./`
+ * Copyright 2012-2015 The Dojo Foundation
+ * Based on Underscore.js 1.8.3
+ * Copyright 2009-2015 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license
+ */
+
+/** Used as the `TypeError` message for "Functions" methods. */
+var FUNC_ERROR_TEXT = 'Expected a function';
+
+/* Native method references for those with the same name as other `lodash` methods. */
+var nativeMax = Math.max;
+
+/**
+ * Creates a function that invokes `func` with the `this` binding of the
+ * created function and arguments from `start` and beyond provided as an array.
+ *
+ * **Note:** This method is based on the [rest parameter](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Functions/rest_parameters).
+ *
+ * @static
+ * @memberOf _
+ * @category Function
+ * @param {Function} func The function to apply a rest parameter to.
+ * @param {number} [start=func.length-1] The start position of the rest parameter.
+ * @returns {Function} Returns the new function.
+ * @example
+ *
+ * var say = _.restParam(function(what, names) {
+ * return what + ' ' + _.initial(names).join(', ') +
+ * (_.size(names) > 1 ? ', & ' : '') + _.last(names);
+ * });
+ *
+ * say('hello', 'fred', 'barney', 'pebbles');
+ * // => 'hello fred, barney, & pebbles'
+ */
+function restParam(func, start) {
+ if (typeof func != 'function') {
+ throw new TypeError(FUNC_ERROR_TEXT);
+ }
+ start = nativeMax(start === undefined ? (func.length - 1) : (+start || 0), 0);
+ return function() {
+ var args = arguments,
+ index = -1,
+ length = nativeMax(args.length - start, 0),
+ rest = Array(length);
+
+ while (++index < length) {
+ rest[index] = args[start + index];
+ }
+ switch (start) {
+ case 0: return func.call(this, rest);
+ case 1: return func.call(this, args[0], rest);
+ case 2: return func.call(this, args[0], args[1], rest);
+ }
+ var otherArgs = Array(start + 1);
+ index = -1;
+ while (++index < start) {
+ otherArgs[index] = args[index];
+ }
+ otherArgs[start] = rest;
+ return func.apply(this, otherArgs);
+ };
+}
+
+module.exports = restParam;
diff --git a/node_modules/lodash.restparam/package.json b/node_modules/lodash.restparam/package.json
new file mode 100644
index 0000000..40a73f0
--- /dev/null
+++ b/node_modules/lodash.restparam/package.json
@@ -0,0 +1,81 @@
+{
+ "_from": "lodash.restparam@^3.0.0",
+ "_id": "lodash.restparam@3.6.1",
+ "_inBundle": false,
+ "_integrity": "sha1-k2pOMJ7zMKdkXtQUWYbIWuWyCAU=",
+ "_location": "/lodash.restparam",
+ "_phantomChildren": {},
+ "_requested": {
+ "type": "range",
+ "registry": true,
+ "raw": "lodash.restparam@^3.0.0",
+ "name": "lodash.restparam",
+ "escapedName": "lodash.restparam",
+ "rawSpec": "^3.0.0",
+ "saveSpec": null,
+ "fetchSpec": "^3.0.0"
+ },
+ "_requiredBy": [
+ "/lodash.template"
+ ],
+ "_resolved": "https://registry.npmjs.org/lodash.restparam/-/lodash.restparam-3.6.1.tgz",
+ "_shasum": "936a4e309ef330a7645ed4145986c85ae5b20805",
+ "_spec": "lodash.restparam@^3.0.0",
+ "_where": "/Users/rpatra16/MyProjects/RamAndAntara/node_modules/lodash.template",
+ "author": {
+ "name": "John-David Dalton",
+ "email": "john.david.dalton@gmail.com",
+ "url": "http://allyoucanleet.com/"
+ },
+ "bugs": {
+ "url": "https://github.com/lodash/lodash/issues"
+ },
+ "bundleDependencies": false,
+ "contributors": [
+ {
+ "name": "John-David Dalton",
+ "email": "john.david.dalton@gmail.com",
+ "url": "http://allyoucanleet.com/"
+ },
+ {
+ "name": "Benjamin Tan",
+ "email": "demoneaux@gmail.com",
+ "url": "https://d10.github.io/"
+ },
+ {
+ "name": "Blaine Bublitz",
+ "email": "blaine@iceddev.com",
+ "url": "http://www.iceddev.com/"
+ },
+ {
+ "name": "Kit Cambridge",
+ "email": "github@kitcambridge.be",
+ "url": "http://kitcambridge.be/"
+ },
+ {
+ "name": "Mathias Bynens",
+ "email": "mathias@qiwi.be",
+ "url": "https://mathiasbynens.be/"
+ }
+ ],
+ "deprecated": false,
+ "description": "The modern build of lodash’s `_.restParam` as a module.",
+ "homepage": "https://lodash.com/",
+ "icon": "https://lodash.com/icon.svg",
+ "keywords": [
+ "lodash",
+ "lodash-modularized",
+ "stdlib",
+ "util"
+ ],
+ "license": "MIT",
+ "name": "lodash.restparam",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/lodash/lodash.git"
+ },
+ "scripts": {
+ "test": "echo \"See https://travis-ci.org/lodash/lodash-cli for testing details.\""
+ },
+ "version": "3.6.1"
+}
diff --git a/node_modules/lodash.template/LICENSE b/node_modules/lodash.template/LICENSE
new file mode 100644
index 0000000..9cd87e5
--- /dev/null
+++ b/node_modules/lodash.template/LICENSE
@@ -0,0 +1,22 @@
+Copyright 2012-2015 The Dojo Foundation
+Based on Underscore.js, copyright 2009-2015 Jeremy Ashkenas,
+DocumentCloud and Investigative Reporters & Editors
+
+Permission is hereby granted, free of charge, to any person obtaining
+a copy of this software and associated documentation files (the
+"Software"), to deal in the Software without restriction, including
+without limitation the rights to use, copy, modify, merge, publish,
+distribute, sublicense, and/or sell copies of the Software, and to
+permit persons to whom the Software is furnished to do so, subject to
+the following conditions:
+
+The above copyright notice and this permission notice shall be
+included in all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
+EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
+MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
+NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
+LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
+OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
+WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
diff --git a/node_modules/lodash.template/README.md b/node_modules/lodash.template/README.md
new file mode 100644
index 0000000..f542f71
--- /dev/null
+++ b/node_modules/lodash.template/README.md
@@ -0,0 +1,20 @@
+# lodash.template v3.6.2
+
+The [modern build](https://github.com/lodash/lodash/wiki/Build-Differences) of [lodash’s](https://lodash.com/) `_.template` exported as a [Node.js](http://nodejs.org/)/[io.js](https://iojs.org/) module.
+
+## Installation
+
+Using npm:
+
+```bash
+$ {sudo -H} npm i -g npm
+$ npm i --save lodash.template
+```
+
+In Node.js/io.js:
+
+```js
+var template = require('lodash.template');
+```
+
+See the [documentation](https://lodash.com/docs#template) or [package source](https://github.com/lodash/lodash/blob/3.6.2-npm-packages/lodash.template) for more details.
diff --git a/node_modules/lodash.template/index.js b/node_modules/lodash.template/index.js
new file mode 100644
index 0000000..e5a9629
--- /dev/null
+++ b/node_modules/lodash.template/index.js
@@ -0,0 +1,389 @@
+/**
+ * lodash 3.6.2 (Custom Build)
+ * Build: `lodash modern modularize exports="npm" -o ./`
+ * Copyright 2012-2015 The Dojo Foundation
+ * Based on Underscore.js 1.8.3
+ * Copyright 2009-2015 Jeremy Ashkenas, DocumentCloud and Investigative Reporters & Editors
+ * Available under MIT license
+ */
+var baseCopy = require('lodash._basecopy'),
+ baseToString = require('lodash._basetostring'),
+ baseValues = require('lodash._basevalues'),
+ isIterateeCall = require('lodash._isiterateecall'),
+ reInterpolate = require('lodash._reinterpolate'),
+ keys = require('lodash.keys'),
+ restParam = require('lodash.restparam'),
+ templateSettings = require('lodash.templatesettings');
+
+/** `Object#toString` result references. */
+var errorTag = '[object Error]';
+
+/** Used to match empty string literals in compiled template source. */
+var reEmptyStringLeading = /\b__p \+= '';/g,
+ reEmptyStringMiddle = /\b(__p \+=) '' \+/g,
+ reEmptyStringTrailing = /(__e\(.*?\)|\b__t\)) \+\n'';/g;
+
+/** Used to match [ES template delimiters](http://ecma-international.org/ecma-262/6.0/#sec-template-literal-lexical-components). */
+var reEsTemplate = /\$\{([^\\}]*(?:\\.[^\\}]*)*)\}/g;
+
+/** Used to ensure capturing order of template delimiters. */
+var reNoMatch = /($^)/;
+
+/** Used to match unescaped characters in compiled string literals. */
+var reUnescapedString = /['\n\r\u2028\u2029\\]/g;
+
+/** Used to escape characters for inclusion in compiled string literals. */
+var stringEscapes = {
+ '\\': '\\',
+ "'": "'",
+ '\n': 'n',
+ '\r': 'r',
+ '\u2028': 'u2028',
+ '\u2029': 'u2029'
+};
+
+/**
+ * Used by `_.template` to escape characters for inclusion in compiled string literals.
+ *
+ * @private
+ * @param {string} chr The matched character to escape.
+ * @returns {string} Returns the escaped character.
+ */
+function escapeStringChar(chr) {
+ return '\\' + stringEscapes[chr];
+}
+
+/**
+ * Checks if `value` is object-like.
+ *
+ * @private
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is object-like, else `false`.
+ */
+function isObjectLike(value) {
+ return !!value && typeof value == 'object';
+}
+
+/** Used for native method references. */
+var objectProto = Object.prototype;
+
+/** Used to check objects for own properties. */
+var hasOwnProperty = objectProto.hasOwnProperty;
+
+/**
+ * Used to resolve the [`toStringTag`](http://ecma-international.org/ecma-262/6.0/#sec-object.prototype.tostring)
+ * of values.
+ */
+var objToString = objectProto.toString;
+
+/**
+ * Used by `_.template` to customize its `_.assign` use.
+ *
+ * **Note:** This function is like `assignDefaults` except that it ignores
+ * inherited property values when checking if a property is `undefined`.
+ *
+ * @private
+ * @param {*} objectValue The destination object property value.
+ * @param {*} sourceValue The source object property value.
+ * @param {string} key The key associated with the object and source values.
+ * @param {Object} object The destination object.
+ * @returns {*} Returns the value to assign to the destination object.
+ */
+function assignOwnDefaults(objectValue, sourceValue, key, object) {
+ return (objectValue === undefined || !hasOwnProperty.call(object, key))
+ ? sourceValue
+ : objectValue;
+}
+
+/**
+ * A specialized version of `_.assign` for customizing assigned values without
+ * support for argument juggling, multiple sources, and `this` binding `customizer`
+ * functions.
+ *
+ * @private
+ * @param {Object} object The destination object.
+ * @param {Object} source The source object.
+ * @param {Function} customizer The function to customize assigned values.
+ * @returns {Object} Returns `object`.
+ */
+function assignWith(object, source, customizer) {
+ var index = -1,
+ props = keys(source),
+ length = props.length;
+
+ while (++index < length) {
+ var key = props[index],
+ value = object[key],
+ result = customizer(value, source[key], key, object, source);
+
+ if ((result === result ? (result !== value) : (value === value)) ||
+ (value === undefined && !(key in object))) {
+ object[key] = result;
+ }
+ }
+ return object;
+}
+
+/**
+ * The base implementation of `_.assign` without support for argument juggling,
+ * multiple sources, and `customizer` functions.
+ *
+ * @private
+ * @param {Object} object The destination object.
+ * @param {Object} source The source object.
+ * @returns {Object} Returns `object`.
+ */
+function baseAssign(object, source) {
+ return source == null
+ ? object
+ : baseCopy(source, keys(source), object);
+}
+
+/**
+ * Checks if `value` is an `Error`, `EvalError`, `RangeError`, `ReferenceError`,
+ * `SyntaxError`, `TypeError`, or `URIError` object.
+ *
+ * @static
+ * @memberOf _
+ * @category Lang
+ * @param {*} value The value to check.
+ * @returns {boolean} Returns `true` if `value` is an error object, else `false`.
+ * @example
+ *
+ * _.isError(new Error);
+ * // => true
+ *
+ * _.isError(Error);
+ * // => false
+ */
+function isError(value) {
+ return isObjectLike(value) && typeof value.message == 'string' && objToString.call(value) == errorTag;
+}
+
+/**
+ * Creates a compiled template function that can interpolate data properties
+ * in "interpolate" delimiters, HTML-escape interpolated data properties in
+ * "escape" delimiters, and execute JavaScript in "evaluate" delimiters. Data
+ * properties may be accessed as free variables in the template. If a setting
+ * object is provided it takes precedence over `_.templateSettings` values.
+ *
+ * **Note:** In the development build `_.template` utilizes
+ * [sourceURLs](http://www.html5rocks.com/en/tutorials/developertools/sourcemaps/#toc-sourceurl)
+ * for easier debugging.
+ *
+ * For more information on precompiling templates see
+ * [lodash's custom builds documentation](https://lodash.com/custom-builds).
+ *
+ * For more information on Chrome extension sandboxes see
+ * [Chrome's extensions documentation](https://developer.chrome.com/extensions/sandboxingEval).
+ *
+ * @static
+ * @memberOf _
+ * @category String
+ * @param {string} [string=''] The template string.
+ * @param {Object} [options] The options object.
+ * @param {RegExp} [options.escape] The HTML "escape" delimiter.
+ * @param {RegExp} [options.evaluate] The "evaluate" delimiter.
+ * @param {Object} [options.imports] An object to import into the template as free variables.
+ * @param {RegExp} [options.interpolate] The "interpolate" delimiter.
+ * @param {string} [options.sourceURL] The sourceURL of the template's compiled source.
+ * @param {string} [options.variable] The data object variable name.
+ * @param- {Object} [otherOptions] Enables the legacy `options` param signature.
+ * @returns {Function} Returns the compiled template function.
+ * @example
+ *
+ * // using the "interpolate" delimiter to create a compiled template
+ * var compiled = _.template('hello <%= user %>!');
+ * compiled({ 'user': 'fred' });
+ * // => 'hello fred!'
+ *
+ * // using the HTML "escape" delimiter to escape data property values
+ * var compiled = _.template('<%- value %> ');
+ * compiled({ 'value': '
+```
+
+Using [`npm`](http://npmjs.org/):
+
+```bash
+npm install lodash
+
+npm install -g lodash
+npm link lodash
+```
+
+To avoid potential issues, update `npm` before installing Lo-Dash:
+
+```bash
+npm install npm -g
+```
+
+In [Node.js](http://nodejs.org/) and [RingoJS v0.8.0+](http://ringojs.org/):
+
+```js
+var _ = require('lodash');
+
+// or as a drop-in replacement for Underscore
+var _ = require('lodash/lodash.underscore');
+```
+
+**Note:** If Lo-Dash is installed globally, run [`npm link lodash`](http://blog.nodejs.org/2011/03/23/npm-1-0-global-vs-local-installation/) in your project’s root directory before requiring it.
+
+In [RingoJS v0.7.0-](http://ringojs.org/):
+
+```js
+var _ = require('lodash')._;
+```
+
+In [Rhino](http://www.mozilla.org/rhino/):
+
+```js
+load('lodash.js');
+```
+
+In an AMD loader like [RequireJS](http://requirejs.org/):
+
+```js
+require({
+ 'paths': {
+ 'underscore': 'path/to/lodash'
+ }
+},
+['underscore'], function(_) {
+ console.log(_.VERSION);
+});
+```
+
+## Resources
+
+For more information check out these articles, screencasts, and other videos over Lo-Dash:
+
+ * Posts
+ - [Say “Hello” to Lo-Dash](http://kitcambridge.be/blog/say-hello-to-lo-dash/)
+
+ * Videos
+ - [Introducing Lo-Dash](https://vimeo.com/44154599)
+ - [Lo-Dash optimizations and custom builds](https://vimeo.com/44154601)
+ - [Lo-Dash’s origin and why it’s a better utility belt](https://vimeo.com/44154600)
+ - [Unit testing in Lo-Dash](https://vimeo.com/45865290)
+ - [Lo-Dash’s approach to native method use](https://vimeo.com/48576012)
+ - [CascadiaJS: Lo-Dash for a better utility belt](http://www.youtube.com/watch?v=dpPy4f_SeEk)
+
+## Features
+
+ * AMD loader support ([RequireJS](http://requirejs.org/), [curl.js](https://github.com/cujojs/curl), etc.)
+ * [_(…)](http://lodash.com/docs#_) supports intuitive chaining
+ * [_.at](http://lodash.com/docs#at) for cherry-picking collection values
+ * [_.bindKey](http://lodash.com/docs#bindKey) for binding [*“lazy”* defined](http://michaux.ca/articles/lazy-function-definition-pattern) methods
+ * [_.cloneDeep](http://lodash.com/docs#cloneDeep) for deep cloning arrays and objects
+ * [_.contains](http://lodash.com/docs#contains) accepts a `fromIndex` argument
+ * [_.forEach](http://lodash.com/docs#forEach) is chainable and supports exiting iteration early
+ * [_.forIn](http://lodash.com/docs#forIn) for iterating over an object’s own and inherited properties
+ * [_.forOwn](http://lodash.com/docs#forOwn) for iterating over an object’s own properties
+ * [_.isPlainObject](http://lodash.com/docs#isPlainObject) checks if values are created by the `Object` constructor
+ * [_.merge](http://lodash.com/docs#merge) for a deep [_.extend](http://lodash.com/docs#extend)
+ * [_.partial](http://lodash.com/docs#partial) and [_.partialRight](http://lodash.com/docs#partialRight) for partial application without `this` binding
+ * [_.template](http://lodash.com/docs#template) supports [*“imports”* options](http://lodash.com/docs#templateSettings_imports), [ES6 template delimiters](http://people.mozilla.org/~jorendorff/es6-draft.html#sec-7.8.6), and [sourceURLs](http://www.html5rocks.com/en/tutorials/developertools/sourcemaps/#toc-sourceurl)
+ * [_.where](http://lodash.com/docs#where) supports deep object comparisons
+ * [_.clone](http://lodash.com/docs#clone), [_.omit](http://lodash.com/docs#omit), [_.pick](http://lodash.com/docs#pick),
+ [and more…](http://lodash.com/docs "_.assign, _.cloneDeep, _.first, _.initial, _.isEqual, _.last, _.merge, _.rest") accept `callback` and `thisArg` arguments
+ * [_.contains](http://lodash.com/docs#contains), [_.size](http://lodash.com/docs#size), [_.toArray](http://lodash.com/docs#toArray),
+ [and more…](http://lodash.com/docs "_.at, _.countBy, _.every, _.filter, _.find, _.forEach, _.groupBy, _.invoke, _.map, _.max, _.min, _.pluck, _.reduce, _.reduceRight, _.reject, _.shuffle, _.some, _.sortBy, _.where") accept strings
+ * [_.filter](http://lodash.com/docs#filter), [_.find](http://lodash.com/docs#find), [_.map](http://lodash.com/docs#map),
+ [and more…](http://lodash.com/docs "_.countBy, _.every, _.first, _.groupBy, _.initial, _.last, _.max, _.min, _.reject, _.rest, _.some, _.sortBy, _.sortedIndex, _.uniq") support *“_.pluck”* and *“_.where”* `callback` shorthands
+
+## Support
+
+Lo-Dash has been tested in at least Chrome 5~24, Firefox 1~18, IE 6-10, Opera 9.25-12, Safari 3-6, Node.js 0.4.8-0.8.20, Narwhal 0.3.2, PhantomJS 1.8.1, RingoJS 0.9, and Rhino 1.7RC5.
diff --git a/node_modules/lodash/dist/lodash.compat.js b/node_modules/lodash/dist/lodash.compat.js
new file mode 100644
index 0000000..925e7fc
--- /dev/null
+++ b/node_modules/lodash/dist/lodash.compat.js
@@ -0,0 +1,5152 @@
+/**
+ * @license
+ * Lo-Dash 1.0.2 (Custom Build)
+ * Build: `lodash -o ./dist/lodash.compat.js`
+ * Copyright 2012-2013 The Dojo Foundation
+ * Based on Underscore.js 1.4.4
+ * Copyright 2009-2013 Jeremy Ashkenas, DocumentCloud Inc.
+ * Available under MIT license
+ */
+;(function(window, undefined) {
+
+ /** Detect free variable `exports` */
+ var freeExports = typeof exports == 'object' && exports;
+
+ /** Detect free variable `module` */
+ var freeModule = typeof module == 'object' && module && module.exports == freeExports && module;
+
+ /** Detect free variable `global` and use it as `window` */
+ var freeGlobal = typeof global == 'object' && global;
+ if (freeGlobal.global === freeGlobal) {
+ window = freeGlobal;
+ }
+
+ /** Used for array and object method references */
+ var arrayRef = [],
+ objectRef = {};
+
+ /** Used to generate unique IDs */
+ var idCounter = 0;
+
+ /** Used internally to indicate various things */
+ var indicatorObject = objectRef;
+
+ /** Used by `cachedContains` as the default size when optimizations are enabled for large arrays */
+ var largeArraySize = 30;
+
+ /** Used to restore the original `_` reference in `noConflict` */
+ var oldDash = window._;
+
+ /** Used to match HTML entities */
+ var reEscapedHtml = /&(?:amp|lt|gt|quot|#39);/g;
+
+ /** Used to match empty string literals in compiled template source */
+ var reEmptyStringLeading = /\b__p \+= '';/g,
+ reEmptyStringMiddle = /\b(__p \+=) '' \+/g,
+ reEmptyStringTrailing = /(__e\(.*?\)|\b__t\)) \+\n'';/g;
+
+ /** Used to match regexp flags from their coerced string values */
+ var reFlags = /\w*$/;
+
+ /** Used to detect if a method is native */
+ var reNative = RegExp('^' +
+ (objectRef.valueOf + '')
+ .replace(/[.*+?^${}()|[\]\\]/g, '\\$&')
+ .replace(/valueOf|for [^\]]+/g, '.+?') + '$'
+ );
+
+ /**
+ * Used to match ES6 template delimiters
+ * http://people.mozilla.org/~jorendorff/es6-draft.html#sec-7.8.6
+ */
+ var reEsTemplate = /\$\{([^\\}]*(?:\\.[^\\}]*)*)\}/g;
+
+ /** Used to match "interpolate" template delimiters */
+ var reInterpolate = /<%=([\s\S]+?)%>/g;
+
+ /** Used to ensure capturing order of template delimiters */
+ var reNoMatch = /($^)/;
+
+ /** Used to match HTML characters */
+ var reUnescapedHtml = /[&<>"']/g;
+
+ /** Used to match unescaped characters in compiled string literals */
+ var reUnescapedString = /['\n\r\t\u2028\u2029\\]/g;
+
+ /** Used to fix the JScript [[DontEnum]] bug */
+ var shadowed = [
+ 'constructor', 'hasOwnProperty', 'isPrototypeOf', 'propertyIsEnumerable',
+ 'toLocaleString', 'toString', 'valueOf'
+ ];
+
+ /** Used to make template sourceURLs easier to identify */
+ var templateCounter = 0;
+
+ /** Native method shortcuts */
+ var ceil = Math.ceil,
+ concat = arrayRef.concat,
+ floor = Math.floor,
+ getPrototypeOf = reNative.test(getPrototypeOf = Object.getPrototypeOf) && getPrototypeOf,
+ hasOwnProperty = objectRef.hasOwnProperty,
+ push = arrayRef.push,
+ toString = objectRef.toString;
+
+ /* Native method shortcuts for methods with the same name as other `lodash` methods */
+ var nativeBind = reNative.test(nativeBind = slice.bind) && nativeBind,
+ nativeIsArray = reNative.test(nativeIsArray = Array.isArray) && nativeIsArray,
+ nativeIsFinite = window.isFinite,
+ nativeIsNaN = window.isNaN,
+ nativeKeys = reNative.test(nativeKeys = Object.keys) && nativeKeys,
+ nativeMax = Math.max,
+ nativeMin = Math.min,
+ nativeRandom = Math.random;
+
+ /** `Object#toString` result shortcuts */
+ var argsClass = '[object Arguments]',
+ arrayClass = '[object Array]',
+ boolClass = '[object Boolean]',
+ dateClass = '[object Date]',
+ funcClass = '[object Function]',
+ numberClass = '[object Number]',
+ objectClass = '[object Object]',
+ regexpClass = '[object RegExp]',
+ stringClass = '[object String]';
+
+ /** Detect various environments */
+ var isIeOpera = !!window.attachEvent,
+ isV8 = nativeBind && !/\n|true/.test(nativeBind + isIeOpera);
+
+ /* Detect if `Function#bind` exists and is inferred to be fast (all but V8) */
+ var isBindFast = nativeBind && !isV8;
+
+ /* Detect if `Object.keys` exists and is inferred to be fast (IE, Opera, V8) */
+ var isKeysFast = nativeKeys && (isIeOpera || isV8);
+
+ /**
+ * Detect the JScript [[DontEnum]] bug:
+ *
+ * In IE < 9 an objects own properties, shadowing non-enumerable ones, are
+ * made non-enumerable as well.
+ */
+ var hasDontEnumBug;
+
+ /**
+ * Detect if a `prototype` properties are enumerable by default:
+ *
+ * Firefox < 3.6, Opera > 9.50 - Opera < 11.60, and Safari < 5.1
+ * (if the prototype or a property on the prototype has been set)
+ * incorrectly sets a function's `prototype` property [[Enumerable]]
+ * value to `true`.
+ */
+ var hasEnumPrototype;
+
+ /** Detect if own properties are iterated after inherited properties (IE < 9) */
+ var iteratesOwnLast;
+
+ /**
+ * Detect if `Array#shift` and `Array#splice` augment array-like objects
+ * incorrectly:
+ *
+ * Firefox < 10, IE compatibility mode, and IE < 9 have buggy Array `shift()`
+ * and `splice()` functions that fail to remove the last element, `value[0]`,
+ * of array-like objects even though the `length` property is set to `0`.
+ * The `shift()` method is buggy in IE 8 compatibility mode, while `splice()`
+ * is buggy regardless of mode in IE < 9 and buggy in compatibility mode in IE 9.
+ */
+ var hasObjectSpliceBug = (hasObjectSpliceBug = { '0': 1, 'length': 1 },
+ arrayRef.splice.call(hasObjectSpliceBug, 0, 1), hasObjectSpliceBug[0]);
+
+ /** Detect if `arguments` object indexes are non-enumerable (Firefox < 4, IE < 9, PhantomJS, Safari < 5.1) */
+ var nonEnumArgs = true;
+
+ (function() {
+ var props = [];
+ function ctor() { this.x = 1; }
+ ctor.prototype = { 'valueOf': 1, 'y': 1 };
+ for (var prop in new ctor) { props.push(prop); }
+ for (prop in arguments) { nonEnumArgs = !prop; }
+
+ hasDontEnumBug = !/valueOf/.test(props);
+ hasEnumPrototype = ctor.propertyIsEnumerable('prototype');
+ iteratesOwnLast = props[0] != 'x';
+ }(1));
+
+ /** Detect if `arguments` objects are `Object` objects (all but Opera < 10.5) */
+ var argsAreObjects = arguments.constructor == Object;
+
+ /** Detect if `arguments` objects [[Class]] is unresolvable (Firefox < 4, IE < 9) */
+ var noArgsClass = !isArguments(arguments);
+
+ /**
+ * Detect lack of support for accessing string characters by index:
+ *
+ * IE < 8 can't access characters by index and IE 8 can only access
+ * characters by index on string literals.
+ */
+ var noCharByIndex = ('x'[0] + Object('x')[0]) != 'xx';
+
+ /**
+ * Detect if a DOM node's [[Class]] is unresolvable (IE < 9)
+ * and that the JS engine won't error when attempting to coerce an object to
+ * a string without a `toString` function.
+ */
+ try {
+ var noNodeClass = toString.call(document) == objectClass && !({ 'toString': 0 } + '');
+ } catch(e) { }
+
+ /** Used to identify object classifications that `_.clone` supports */
+ var cloneableClasses = {};
+ cloneableClasses[funcClass] = false;
+ cloneableClasses[argsClass] = cloneableClasses[arrayClass] =
+ cloneableClasses[boolClass] = cloneableClasses[dateClass] =
+ cloneableClasses[numberClass] = cloneableClasses[objectClass] =
+ cloneableClasses[regexpClass] = cloneableClasses[stringClass] = true;
+
+ /** Used to lookup a built-in constructor by [[Class]] */
+ var ctorByClass = {};
+ ctorByClass[arrayClass] = Array;
+ ctorByClass[boolClass] = Boolean;
+ ctorByClass[dateClass] = Date;
+ ctorByClass[objectClass] = Object;
+ ctorByClass[numberClass] = Number;
+ ctorByClass[regexpClass] = RegExp;
+ ctorByClass[stringClass] = String;
+
+ /** Used to determine if values are of the language type Object */
+ var objectTypes = {
+ 'boolean': false,
+ 'function': true,
+ 'object': true,
+ 'number': false,
+ 'string': false,
+ 'undefined': false
+ };
+
+ /** Used to escape characters for inclusion in compiled string literals */
+ var stringEscapes = {
+ '\\': '\\',
+ "'": "'",
+ '\n': 'n',
+ '\r': 'r',
+ '\t': 't',
+ '\u2028': 'u2028',
+ '\u2029': 'u2029'
+ };
+
+ /*--------------------------------------------------------------------------*/
+
+ /**
+ * Creates a `lodash` object, that wraps the given `value`, to enable method
+ * chaining.
+ *
+ * In addition to Lo-Dash methods, wrappers also have the following `Array` methods:
+ * `concat`, `join`, `pop`, `push`, `reverse`, `shift`, `slice`, `sort`, `splice`,
+ * and `unshift`
+ *
+ * The chainable wrapper functions are:
+ * `after`, `assign`, `bind`, `bindAll`, `bindKey`, `chain`, `compact`, `compose`,
+ * `concat`, `countBy`, `debounce`, `defaults`, `defer`, `delay`, `difference`,
+ * `filter`, `flatten`, `forEach`, `forIn`, `forOwn`, `functions`, `groupBy`,
+ * `initial`, `intersection`, `invert`, `invoke`, `keys`, `map`, `max`, `memoize`,
+ * `merge`, `min`, `object`, `omit`, `once`, `pairs`, `partial`, `partialRight`,
+ * `pick`, `pluck`, `push`, `range`, `reject`, `rest`, `reverse`, `shuffle`,
+ * `slice`, `sort`, `sortBy`, `splice`, `tap`, `throttle`, `times`, `toArray`,
+ * `union`, `uniq`, `unshift`, `values`, `where`, `without`, `wrap`, and `zip`
+ *
+ * The non-chainable wrapper functions are:
+ * `clone`, `cloneDeep`, `contains`, `escape`, `every`, `find`, `has`, `identity`,
+ * `indexOf`, `isArguments`, `isArray`, `isBoolean`, `isDate`, `isElement`, `isEmpty`,
+ * `isEqual`, `isFinite`, `isFunction`, `isNaN`, `isNull`, `isNumber`, `isObject`,
+ * `isPlainObject`, `isRegExp`, `isString`, `isUndefined`, `join`, `lastIndexOf`,
+ * `mixin`, `noConflict`, `pop`, `random`, `reduce`, `reduceRight`, `result`,
+ * `shift`, `size`, `some`, `sortedIndex`, `template`, `unescape`, and `uniqueId`
+ *
+ * The wrapper functions `first` and `last` return wrapped values when `n` is
+ * passed, otherwise they return unwrapped values.
+ *
+ * @name _
+ * @constructor
+ * @category Chaining
+ * @param {Mixed} value The value to wrap in a `lodash` instance.
+ * @returns {Object} Returns a `lodash` instance.
+ */
+ function lodash(value) {
+ // exit early if already wrapped, even if wrapped by a different `lodash` constructor
+ if (value && typeof value == 'object' && value.__wrapped__) {
+ return value;
+ }
+ // allow invoking `lodash` without the `new` operator
+ if (!(this instanceof lodash)) {
+ return new lodash(value);
+ }
+ this.__wrapped__ = value;
+ }
+
+ /**
+ * By default, the template delimiters used by Lo-Dash are similar to those in
+ * embedded Ruby (ERB). Change the following template settings to use alternative
+ * delimiters.
+ *
+ * @static
+ * @memberOf _
+ * @type Object
+ */
+ lodash.templateSettings = {
+
+ /**
+ * Used to detect `data` property values to be HTML-escaped.
+ *
+ * @memberOf _.templateSettings
+ * @type RegExp
+ */
+ 'escape': /<%-([\s\S]+?)%>/g,
+
+ /**
+ * Used to detect code to be evaluated.
+ *
+ * @memberOf _.templateSettings
+ * @type RegExp
+ */
+ 'evaluate': /<%([\s\S]+?)%>/g,
+
+ /**
+ * Used to detect `data` property values to inject.
+ *
+ * @memberOf _.templateSettings
+ * @type RegExp
+ */
+ 'interpolate': reInterpolate,
+
+ /**
+ * Used to reference the data object in the template text.
+ *
+ * @memberOf _.templateSettings
+ * @type String
+ */
+ 'variable': '',
+
+ /**
+ * Used to import variables into the compiled template.
+ *
+ * @memberOf _.templateSettings
+ * @type Object
+ */
+ 'imports': {
+
+ /**
+ * A reference to the `lodash` function.
+ *
+ * @memberOf _.templateSettings.imports
+ * @type Function
+ */
+ '_': lodash
+ }
+ };
+
+ /*--------------------------------------------------------------------------*/
+
+ /**
+ * The template used to create iterator functions.
+ *
+ * @private
+ * @param {Obect} data The data object used to populate the text.
+ * @returns {String} Returns the interpolated text.
+ */
+ var iteratorTemplate = function(obj) {
+
+ var __p = 'var index, iterable = ' +
+ (obj.firstArg ) +
+ ', result = iterable;\nif (!iterable) return result;\n' +
+ (obj.top ) +
+ ';\n';
+ if (obj.arrays) {
+ __p += 'var length = iterable.length; index = -1;\nif (' +
+ (obj.arrays ) +
+ ') { ';
+ if (obj.noCharByIndex) {
+ __p += '\n if (isString(iterable)) {\n iterable = iterable.split(\'\')\n } ';
+ } ;
+ __p += '\n while (++index < length) {\n ' +
+ (obj.loop ) +
+ '\n }\n}\nelse { ';
+ } else if (obj.nonEnumArgs) {
+ __p += '\n var length = iterable.length; index = -1;\n if (length && isArguments(iterable)) {\n while (++index < length) {\n index += \'\';\n ' +
+ (obj.loop ) +
+ '\n }\n } else { ';
+ } ;
+
+ if (obj.hasEnumPrototype) {
+ __p += '\n var skipProto = typeof iterable == \'function\';\n ';
+ } ;
+
+ if (obj.isKeysFast && obj.useHas) {
+ __p += '\n var ownIndex = -1,\n ownProps = objectTypes[typeof iterable] ? nativeKeys(iterable) : [],\n length = ownProps.length;\n\n while (++ownIndex < length) {\n index = ownProps[ownIndex];\n ';
+ if (obj.hasEnumPrototype) {
+ __p += 'if (!(skipProto && index == \'prototype\')) {\n ';
+ } ;
+ __p +=
+ (obj.loop ) +
+ '';
+ if (obj.hasEnumPrototype) {
+ __p += '}\n';
+ } ;
+ __p += ' } ';
+ } else {
+ __p += '\n for (index in iterable) {';
+ if (obj.hasEnumPrototype || obj.useHas) {
+ __p += '\n if (';
+ if (obj.hasEnumPrototype) {
+ __p += '!(skipProto && index == \'prototype\')';
+ } if (obj.hasEnumPrototype && obj.useHas) {
+ __p += ' && ';
+ } if (obj.useHas) {
+ __p += 'hasOwnProperty.call(iterable, index)';
+ } ;
+ __p += ') { ';
+ } ;
+ __p +=
+ (obj.loop ) +
+ '; ';
+ if (obj.hasEnumPrototype || obj.useHas) {
+ __p += '\n }';
+ } ;
+ __p += '\n } ';
+ } ;
+
+ if (obj.hasDontEnumBug) {
+ __p += '\n\n var ctor = iterable.constructor;\n ';
+ for (var k = 0; k < 7; k++) {
+ __p += '\n index = \'' +
+ (obj.shadowed[k] ) +
+ '\';\n if (';
+ if (obj.shadowed[k] == 'constructor') {
+ __p += '!(ctor && ctor.prototype === iterable) && ';
+ } ;
+ __p += 'hasOwnProperty.call(iterable, index)) {\n ' +
+ (obj.loop ) +
+ '\n } ';
+ } ;
+
+ } ;
+
+ if (obj.arrays || obj.nonEnumArgs) {
+ __p += '\n}';
+ } ;
+ __p +=
+ (obj.bottom ) +
+ ';\nreturn result';
+
+
+ return __p
+ };
+
+ /** Reusable iterator options for `assign` and `defaults` */
+ var defaultsIteratorOptions = {
+ 'args': 'object, source, guard',
+ 'top':
+ 'var args = arguments,\n' +
+ ' argsIndex = 0,\n' +
+ " argsLength = typeof guard == 'number' ? 2 : args.length;\n" +
+ 'while (++argsIndex < argsLength) {\n' +
+ ' iterable = args[argsIndex];\n' +
+ ' if (iterable && objectTypes[typeof iterable]) {',
+ 'loop': "if (typeof result[index] == 'undefined') result[index] = iterable[index]",
+ 'bottom': ' }\n}'
+ };
+
+ /** Reusable iterator options shared by `each`, `forIn`, and `forOwn` */
+ var eachIteratorOptions = {
+ 'args': 'collection, callback, thisArg',
+ 'top': "callback = callback && typeof thisArg == 'undefined' ? callback : createCallback(callback, thisArg)",
+ 'arrays': "typeof length == 'number'",
+ 'loop': 'if (callback(iterable[index], index, collection) === false) return result'
+ };
+
+ /** Reusable iterator options for `forIn` and `forOwn` */
+ var forOwnIteratorOptions = {
+ 'top': 'if (!objectTypes[typeof iterable]) return result;\n' + eachIteratorOptions.top,
+ 'arrays': false
+ };
+
+ /*--------------------------------------------------------------------------*/
+
+ /**
+ * Creates a function optimized to search large arrays for a given `value`,
+ * starting at `fromIndex`, using strict equality for comparisons, i.e. `===`.
+ *
+ * @private
+ * @param {Array} array The array to search.
+ * @param {Mixed} value The value to search for.
+ * @param {Number} [fromIndex=0] The index to search from.
+ * @param {Number} [largeSize=30] The length at which an array is considered large.
+ * @returns {Boolean} Returns `true`, if `value` is found, else `false`.
+ */
+ function cachedContains(array, fromIndex, largeSize) {
+ fromIndex || (fromIndex = 0);
+
+ var length = array.length,
+ isLarge = (length - fromIndex) >= (largeSize || largeArraySize);
+
+ if (isLarge) {
+ var cache = {},
+ index = fromIndex - 1;
+
+ while (++index < length) {
+ // manually coerce `value` to a string because `hasOwnProperty`, in some
+ // older versions of Firefox, coerces objects incorrectly
+ var key = array[index] + '';
+ (hasOwnProperty.call(cache, key) ? cache[key] : (cache[key] = [])).push(array[index]);
+ }
+ }
+ return function(value) {
+ if (isLarge) {
+ var key = value + '';
+ return hasOwnProperty.call(cache, key) && indexOf(cache[key], value) > -1;
+ }
+ return indexOf(array, value, fromIndex) > -1;
+ }
+ }
+
+ /**
+ * Used by `_.max` and `_.min` as the default `callback` when a given
+ * `collection` is a string value.
+ *
+ * @private
+ * @param {String} value The character to inspect.
+ * @returns {Number} Returns the code unit of given character.
+ */
+ function charAtCallback(value) {
+ return value.charCodeAt(0);
+ }
+
+ /**
+ * Used by `sortBy` to compare transformed `collection` values, stable sorting
+ * them in ascending order.
+ *
+ * @private
+ * @param {Object} a The object to compare to `b`.
+ * @param {Object} b The object to compare to `a`.
+ * @returns {Number} Returns the sort order indicator of `1` or `-1`.
+ */
+ function compareAscending(a, b) {
+ var ai = a.index,
+ bi = b.index;
+
+ a = a.criteria;
+ b = b.criteria;
+
+ // ensure a stable sort in V8 and other engines
+ // http://code.google.com/p/v8/issues/detail?id=90
+ if (a !== b) {
+ if (a > b || typeof a == 'undefined') {
+ return 1;
+ }
+ if (a < b || typeof b == 'undefined') {
+ return -1;
+ }
+ }
+ return ai < bi ? -1 : 1;
+ }
+
+ /**
+ * Creates a function that, when called, invokes `func` with the `this` binding
+ * of `thisArg` and prepends any `partialArgs` to the arguments passed to the
+ * bound function.
+ *
+ * @private
+ * @param {Function|String} func The function to bind or the method name.
+ * @param {Mixed} [thisArg] The `this` binding of `func`.
+ * @param {Array} partialArgs An array of arguments to be partially applied.
+ * @param {Object} [rightIndicator] Used to indicate partially applying arguments from the right.
+ * @returns {Function} Returns the new bound function.
+ */
+ function createBound(func, thisArg, partialArgs, rightIndicator) {
+ var isFunc = isFunction(func),
+ isPartial = !partialArgs,
+ key = thisArg;
+
+ // juggle arguments
+ if (isPartial) {
+ partialArgs = thisArg;
+ }
+ if (!isFunc) {
+ thisArg = func;
+ }
+
+ function bound() {
+ // `Function#bind` spec
+ // http://es5.github.com/#x15.3.4.5
+ var args = arguments,
+ thisBinding = isPartial ? this : thisArg;
+
+ if (!isFunc) {
+ func = thisArg[key];
+ }
+ if (partialArgs.length) {
+ args = args.length
+ ? (args = slice(args), rightIndicator ? args.concat(partialArgs) : partialArgs.concat(args))
+ : partialArgs;
+ }
+ if (this instanceof bound) {
+ // ensure `new bound` is an instance of `bound` and `func`
+ noop.prototype = func.prototype;
+ thisBinding = new noop;
+ noop.prototype = null;
+
+ // mimic the constructor's `return` behavior
+ // http://es5.github.com/#x13.2.2
+ var result = func.apply(thisBinding, args);
+ return isObject(result) ? result : thisBinding;
+ }
+ return func.apply(thisBinding, args);
+ }
+ return bound;
+ }
+
+ /**
+ * Produces a callback bound to an optional `thisArg`. If `func` is a property
+ * name, the created callback will return the property value for a given element.
+ * If `func` is an object, the created callback will return `true` for elements
+ * that contain the equivalent object properties, otherwise it will return `false`.
+ *
+ * @private
+ * @param {Mixed} [func=identity] The value to convert to a callback.
+ * @param {Mixed} [thisArg] The `this` binding of the created callback.
+ * @param {Number} [argCount=3] The number of arguments the callback accepts.
+ * @returns {Function} Returns a callback function.
+ */
+ function createCallback(func, thisArg, argCount) {
+ if (func == null) {
+ return identity;
+ }
+ var type = typeof func;
+ if (type != 'function') {
+ if (type != 'object') {
+ return function(object) {
+ return object[func];
+ };
+ }
+ var props = keys(func);
+ return function(object) {
+ var length = props.length,
+ result = false;
+ while (length--) {
+ if (!(result = isEqual(object[props[length]], func[props[length]], indicatorObject))) {
+ break;
+ }
+ }
+ return result;
+ };
+ }
+ if (typeof thisArg != 'undefined') {
+ if (argCount === 1) {
+ return function(value) {
+ return func.call(thisArg, value);
+ };
+ }
+ if (argCount === 2) {
+ return function(a, b) {
+ return func.call(thisArg, a, b);
+ };
+ }
+ if (argCount === 4) {
+ return function(accumulator, value, index, object) {
+ return func.call(thisArg, accumulator, value, index, object);
+ };
+ }
+ return function(value, index, object) {
+ return func.call(thisArg, value, index, object);
+ };
+ }
+ return func;
+ }
+
+ /**
+ * Creates compiled iteration functions.
+ *
+ * @private
+ * @param {Object} [options1, options2, ...] The compile options object(s).
+ * arrays - A string of code to determine if the iterable is an array or array-like.
+ * useHas - A boolean to specify using `hasOwnProperty` checks in the object loop.
+ * args - A string of comma separated arguments the iteration function will accept.
+ * top - A string of code to execute before the iteration branches.
+ * loop - A string of code to execute in the object loop.
+ * bottom - A string of code to execute after the iteration branches.
+ *
+ * @returns {Function} Returns the compiled function.
+ */
+ function createIterator() {
+ var data = {
+ // support properties
+ 'hasDontEnumBug': hasDontEnumBug,
+ 'hasEnumPrototype': hasEnumPrototype,
+ 'isKeysFast': isKeysFast,
+ 'nonEnumArgs': nonEnumArgs,
+ 'noCharByIndex': noCharByIndex,
+ 'shadowed': shadowed,
+
+ // iterator options
+ 'arrays': 'isArray(iterable)',
+ 'bottom': '',
+ 'loop': '',
+ 'top': '',
+ 'useHas': true
+ };
+
+ // merge options into a template data object
+ for (var object, index = 0; object = arguments[index]; index++) {
+ for (var key in object) {
+ data[key] = object[key];
+ }
+ }
+ var args = data.args;
+ data.firstArg = /^[^,]+/.exec(args)[0];
+
+ // create the function factory
+ var factory = Function(
+ 'createCallback, hasOwnProperty, isArguments, isArray, isString, ' +
+ 'objectTypes, nativeKeys',
+ 'return function(' + args + ') {\n' + iteratorTemplate(data) + '\n}'
+ );
+ // return the compiled function
+ return factory(
+ createCallback, hasOwnProperty, isArguments, isArray, isString,
+ objectTypes, nativeKeys
+ );
+ }
+
+ /**
+ * A function compiled to iterate `arguments` objects, arrays, objects, and
+ * strings consistenly across environments, executing the `callback` for each
+ * element in the `collection`. The `callback` is bound to `thisArg` and invoked
+ * with three arguments; (value, index|key, collection). Callbacks may exit
+ * iteration early by explicitly returning `false`.
+ *
+ * @private
+ * @type Function
+ * @param {Array|Object|String} collection The collection to iterate over.
+ * @param {Function} [callback=identity] The function called per iteration.
+ * @param {Mixed} [thisArg] The `this` binding of `callback`.
+ * @returns {Array|Object|String} Returns `collection`.
+ */
+ var each = createIterator(eachIteratorOptions);
+
+ /**
+ * Used by `template` to escape characters for inclusion in compiled
+ * string literals.
+ *
+ * @private
+ * @param {String} match The matched character to escape.
+ * @returns {String} Returns the escaped character.
+ */
+ function escapeStringChar(match) {
+ return '\\' + stringEscapes[match];
+ }
+
+ /**
+ * Used by `escape` to convert characters to HTML entities.
+ *
+ * @private
+ * @param {String} match The matched character to escape.
+ * @returns {String} Returns the escaped character.
+ */
+ function escapeHtmlChar(match) {
+ return htmlEscapes[match];
+ }
+
+ /**
+ * Checks if `value` is a DOM node in IE < 9.
+ *
+ * @private
+ * @param {Mixed} value The value to check.
+ * @returns {Boolean} Returns `true` if the `value` is a DOM node, else `false`.
+ */
+ function isNode(value) {
+ // IE < 9 presents DOM nodes as `Object` objects except they have `toString`
+ // methods that are `typeof` "string" and still can coerce nodes to strings
+ return typeof value.toString != 'function' && typeof (value + '') == 'string';
+ }
+
+ /**
+ * A no-operation function.
+ *
+ * @private
+ */
+ function noop() {
+ // no operation performed
+ }
+
+ /**
+ * Slices the `collection` from the `start` index up to, but not including,
+ * the `end` index.
+ *
+ * Note: This function is used, instead of `Array#slice`, to support node lists
+ * in IE < 9 and to ensure dense arrays are returned.
+ *
+ * @private
+ * @param {Array|Object|String} collection The collection to slice.
+ * @param {Number} start The start index.
+ * @param {Number} end The end index.
+ * @returns {Array} Returns the new array.
+ */
+ function slice(array, start, end) {
+ start || (start = 0);
+ if (typeof end == 'undefined') {
+ end = array ? array.length : 0;
+ }
+ var index = -1,
+ length = end - start || 0,
+ result = Array(length < 0 ? 0 : length);
+
+ while (++index < length) {
+ result[index] = array[start + index];
+ }
+ return result;
+ }
+
+ /**
+ * Used by `unescape` to convert HTML entities to characters.
+ *
+ * @private
+ * @param {String} match The matched character to unescape.
+ * @returns {String} Returns the unescaped character.
+ */
+ function unescapeHtmlChar(match) {
+ return htmlUnescapes[match];
+ }
+
+ /*--------------------------------------------------------------------------*/
+
+ /**
+ * Checks if `value` is an `arguments` object.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Mixed} value The value to check.
+ * @returns {Boolean} Returns `true`, if the `value` is an `arguments` object, else `false`.
+ * @example
+ *
+ * (function() { return _.isArguments(arguments); })(1, 2, 3);
+ * // => true
+ *
+ * _.isArguments([1, 2, 3]);
+ * // => false
+ */
+ function isArguments(value) {
+ return toString.call(value) == argsClass;
+ }
+ // fallback for browsers that can't detect `arguments` objects by [[Class]]
+ if (noArgsClass) {
+ isArguments = function(value) {
+ return value ? hasOwnProperty.call(value, 'callee') : false;
+ };
+ }
+
+ /**
+ * Iterates over `object`'s own and inherited enumerable properties, executing
+ * the `callback` for each property. The `callback` is bound to `thisArg` and
+ * invoked with three arguments; (value, key, object). Callbacks may exit iteration
+ * early by explicitly returning `false`.
+ *
+ * @static
+ * @memberOf _
+ * @type Function
+ * @category Objects
+ * @param {Object} object The object to iterate over.
+ * @param {Function} [callback=identity] The function called per iteration.
+ * @param {Mixed} [thisArg] The `this` binding of `callback`.
+ * @returns {Object} Returns `object`.
+ * @example
+ *
+ * function Dog(name) {
+ * this.name = name;
+ * }
+ *
+ * Dog.prototype.bark = function() {
+ * alert('Woof, woof!');
+ * };
+ *
+ * _.forIn(new Dog('Dagny'), function(value, key) {
+ * alert(key);
+ * });
+ * // => alerts 'name' and 'bark' (order is not guaranteed)
+ */
+ var forIn = createIterator(eachIteratorOptions, forOwnIteratorOptions, {
+ 'useHas': false
+ });
+
+ /**
+ * Iterates over an object's own enumerable properties, executing the `callback`
+ * for each property. The `callback` is bound to `thisArg` and invoked with three
+ * arguments; (value, key, object). Callbacks may exit iteration early by explicitly
+ * returning `false`.
+ *
+ * @static
+ * @memberOf _
+ * @type Function
+ * @category Objects
+ * @param {Object} object The object to iterate over.
+ * @param {Function} [callback=identity] The function called per iteration.
+ * @param {Mixed} [thisArg] The `this` binding of `callback`.
+ * @returns {Object} Returns `object`.
+ * @example
+ *
+ * _.forOwn({ '0': 'zero', '1': 'one', 'length': 2 }, function(num, key) {
+ * alert(key);
+ * });
+ * // => alerts '0', '1', and 'length' (order is not guaranteed)
+ */
+ var forOwn = createIterator(eachIteratorOptions, forOwnIteratorOptions);
+
+ /**
+ * Checks if `value` is an array.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Mixed} value The value to check.
+ * @returns {Boolean} Returns `true`, if the `value` is an array, else `false`.
+ * @example
+ *
+ * (function() { return _.isArray(arguments); })();
+ * // => false
+ *
+ * _.isArray([1, 2, 3]);
+ * // => true
+ */
+ var isArray = nativeIsArray || function(value) {
+ // `instanceof` may cause a memory leak in IE 7 if `value` is a host object
+ // http://ajaxian.com/archives/working-aroung-the-instanceof-memory-leak
+ return (argsAreObjects && value instanceof Array) || toString.call(value) == arrayClass;
+ };
+
+ /**
+ * Creates an array composed of the own enumerable property names of `object`.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Object} object The object to inspect.
+ * @returns {Array} Returns a new array of property names.
+ * @example
+ *
+ * _.keys({ 'one': 1, 'two': 2, 'three': 3 });
+ * // => ['one', 'two', 'three'] (order is not guaranteed)
+ */
+ var keys = !nativeKeys ? shimKeys : function(object) {
+ if (!isObject(object)) {
+ return [];
+ }
+ if ((hasEnumPrototype && typeof object == 'function') ||
+ (nonEnumArgs && object.length && isArguments(object))) {
+ return shimKeys(object);
+ }
+ return nativeKeys(object);
+ };
+
+ /**
+ * A fallback implementation of `isPlainObject` that checks if a given `value`
+ * is an object created by the `Object` constructor, assuming objects created
+ * by the `Object` constructor have no inherited enumerable properties and that
+ * there are no `Object.prototype` extensions.
+ *
+ * @private
+ * @param {Mixed} value The value to check.
+ * @returns {Boolean} Returns `true`, if `value` is a plain object, else `false`.
+ */
+ function shimIsPlainObject(value) {
+ // avoid non-objects and false positives for `arguments` objects
+ var result = false;
+ if (!(value && typeof value == 'object') || isArguments(value)) {
+ return result;
+ }
+ // check that the constructor is `Object` (i.e. `Object instanceof Object`)
+ var ctor = value.constructor;
+ if ((!isFunction(ctor) && (!noNodeClass || !isNode(value))) || ctor instanceof ctor) {
+ // IE < 9 iterates inherited properties before own properties. If the first
+ // iterated property is an object's own property then there are no inherited
+ // enumerable properties.
+ if (iteratesOwnLast) {
+ forIn(value, function(value, key, object) {
+ result = !hasOwnProperty.call(object, key);
+ return false;
+ });
+ return result === false;
+ }
+ // In most environments an object's own properties are iterated before
+ // its inherited properties. If the last iterated property is an object's
+ // own property then there are no inherited enumerable properties.
+ forIn(value, function(value, key) {
+ result = key;
+ });
+ return result === false || hasOwnProperty.call(value, result);
+ }
+ return result;
+ }
+
+ /**
+ * A fallback implementation of `Object.keys` that produces an array of the
+ * given object's own enumerable property names.
+ *
+ * @private
+ * @param {Object} object The object to inspect.
+ * @returns {Array} Returns a new array of property names.
+ */
+ function shimKeys(object) {
+ var result = [];
+ forOwn(object, function(value, key) {
+ result.push(key);
+ });
+ return result;
+ }
+
+ /**
+ * Used to convert characters to HTML entities:
+ *
+ * Though the `>` character is escaped for symmetry, characters like `>` and `/`
+ * don't require escaping in HTML and have no special meaning unless they're part
+ * of a tag or an unquoted attribute value.
+ * http://mathiasbynens.be/notes/ambiguous-ampersands (under "semi-related fun fact")
+ */
+ var htmlEscapes = {
+ '&': '&',
+ '<': '<',
+ '>': '>',
+ '"': '"',
+ "'": '''
+ };
+
+ /** Used to convert HTML entities to characters */
+ var htmlUnescapes = invert(htmlEscapes);
+
+ /*--------------------------------------------------------------------------*/
+
+ /**
+ * Assigns own enumerable properties of source object(s) to the destination
+ * object. Subsequent sources will overwrite propery assignments of previous
+ * sources. If a `callback` function is passed, it will be executed to produce
+ * the assigned values. The `callback` is bound to `thisArg` and invoked with
+ * two arguments; (objectValue, sourceValue).
+ *
+ * @static
+ * @memberOf _
+ * @type Function
+ * @alias extend
+ * @category Objects
+ * @param {Object} object The destination object.
+ * @param {Object} [source1, source2, ...] The source objects.
+ * @param {Function} [callback] The function to customize assigning values.
+ * @param {Mixed} [thisArg] The `this` binding of `callback`.
+ * @returns {Object} Returns the destination object.
+ * @example
+ *
+ * _.assign({ 'name': 'moe' }, { 'age': 40 });
+ * // => { 'name': 'moe', 'age': 40 }
+ *
+ * var defaults = _.partialRight(_.assign, function(a, b) {
+ * return typeof a == 'undefined' ? b : a;
+ * });
+ *
+ * var food = { 'name': 'apple' };
+ * defaults(food, { 'name': 'banana', 'type': 'fruit' });
+ * // => { 'name': 'apple', 'type': 'fruit' }
+ */
+ var assign = createIterator(defaultsIteratorOptions, {
+ 'top':
+ defaultsIteratorOptions.top.replace(';',
+ ';\n' +
+ "if (argsLength > 3 && typeof args[argsLength - 2] == 'function') {\n" +
+ ' var callback = createCallback(args[--argsLength - 1], args[argsLength--], 2);\n' +
+ "} else if (argsLength > 2 && typeof args[argsLength - 1] == 'function') {\n" +
+ ' callback = args[--argsLength];\n' +
+ '}'
+ ),
+ 'loop': 'result[index] = callback ? callback(result[index], iterable[index]) : iterable[index]'
+ });
+
+ /**
+ * Creates a clone of `value`. If `deep` is `true`, nested objects will also
+ * be cloned, otherwise they will be assigned by reference. If a `callback`
+ * function is passed, it will be executed to produce the cloned values. If
+ * `callback` returns `undefined`, cloning will be handled by the method instead.
+ * The `callback` is bound to `thisArg` and invoked with one argument; (value).
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Mixed} value The value to clone.
+ * @param {Boolean} [deep=false] A flag to indicate a deep clone.
+ * @param {Function} [callback] The function to customize cloning values.
+ * @param {Mixed} [thisArg] The `this` binding of `callback`.
+ * @param- {Array} [stackA=[]] Internally used to track traversed source objects.
+ * @param- {Array} [stackB=[]] Internally used to associate clones with source counterparts.
+ * @returns {Mixed} Returns the cloned `value`.
+ * @example
+ *
+ * var stooges = [
+ * { 'name': 'moe', 'age': 40 },
+ * { 'name': 'larry', 'age': 50 }
+ * ];
+ *
+ * var shallow = _.clone(stooges);
+ * shallow[0] === stooges[0];
+ * // => true
+ *
+ * var deep = _.clone(stooges, true);
+ * deep[0] === stooges[0];
+ * // => false
+ *
+ * _.mixin({
+ * 'clone': _.partialRight(_.clone, function(value) {
+ * return _.isElement(value) ? value.cloneNode(false) : undefined;
+ * })
+ * });
+ *
+ * var clone = _.clone(document.body);
+ * clone.childNodes.length;
+ * // => 0
+ */
+ function clone(value, deep, callback, thisArg, stackA, stackB) {
+ var result = value;
+
+ // allows working with "Collections" methods without using their `callback`
+ // argument, `index|key`, for this method's `callback`
+ if (typeof deep == 'function') {
+ thisArg = callback;
+ callback = deep;
+ deep = false;
+ }
+ if (typeof callback == 'function') {
+ callback = typeof thisArg == 'undefined' ? callback : createCallback(callback, thisArg, 1);
+ result = callback(result);
+
+ var done = typeof result != 'undefined';
+ if (!done) {
+ result = value;
+ }
+ }
+ // inspect [[Class]]
+ var isObj = isObject(result);
+ if (isObj) {
+ var className = toString.call(result);
+ if (!cloneableClasses[className] || (noNodeClass && isNode(result))) {
+ return result;
+ }
+ var isArr = isArray(result);
+ }
+ // shallow clone
+ if (!isObj || !deep) {
+ return isObj && !done
+ ? (isArr ? slice(result) : assign({}, result))
+ : result;
+ }
+ var ctor = ctorByClass[className];
+ switch (className) {
+ case boolClass:
+ case dateClass:
+ return done ? result : new ctor(+result);
+
+ case numberClass:
+ case stringClass:
+ return done ? result : new ctor(result);
+
+ case regexpClass:
+ return done ? result : ctor(result.source, reFlags.exec(result));
+ }
+ // check for circular references and return corresponding clone
+ stackA || (stackA = []);
+ stackB || (stackB = []);
+
+ var length = stackA.length;
+ while (length--) {
+ if (stackA[length] == value) {
+ return stackB[length];
+ }
+ }
+ // init cloned object
+ if (!done) {
+ result = isArr ? ctor(result.length) : {};
+
+ // add array properties assigned by `RegExp#exec`
+ if (isArr) {
+ if (hasOwnProperty.call(value, 'index')) {
+ result.index = value.index;
+ }
+ if (hasOwnProperty.call(value, 'input')) {
+ result.input = value.input;
+ }
+ }
+ }
+ // add the source value to the stack of traversed objects
+ // and associate it with its clone
+ stackA.push(value);
+ stackB.push(result);
+
+ // recursively populate clone (susceptible to call stack limits)
+ (isArr ? forEach : forOwn)(done ? result : value, function(objValue, key) {
+ result[key] = clone(objValue, deep, callback, undefined, stackA, stackB);
+ });
+
+ return result;
+ }
+
+ /**
+ * Creates a deep clone of `value`. If a `callback` function is passed, it will
+ * be executed to produce the cloned values. If `callback` returns the value it
+ * was passed, cloning will be handled by the method instead. The `callback` is
+ * bound to `thisArg` and invoked with one argument; (value).
+ *
+ * Note: This function is loosely based on the structured clone algorithm. Functions
+ * and DOM nodes are **not** cloned. The enumerable properties of `arguments` objects and
+ * objects created by constructors other than `Object` are cloned to plain `Object` objects.
+ * See http://www.w3.org/TR/html5/infrastructure.html#internal-structured-cloning-algorithm.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Mixed} value The value to deep clone.
+ * @param {Function} [callback] The function to customize cloning values.
+ * @param {Mixed} [thisArg] The `this` binding of `callback`.
+ * @returns {Mixed} Returns the deep cloned `value`.
+ * @example
+ *
+ * var stooges = [
+ * { 'name': 'moe', 'age': 40 },
+ * { 'name': 'larry', 'age': 50 }
+ * ];
+ *
+ * var deep = _.cloneDeep(stooges);
+ * deep[0] === stooges[0];
+ * // => false
+ *
+ * var view = {
+ * 'label': 'docs',
+ * 'node': element
+ * };
+ *
+ * var clone = _.cloneDeep(view, function(value) {
+ * return _.isElement(value) ? value.cloneNode(true) : value;
+ * });
+ *
+ * clone.node == view.node;
+ * // => false
+ */
+ function cloneDeep(value, callback, thisArg) {
+ return clone(value, true, callback, thisArg);
+ }
+
+ /**
+ * Assigns own enumerable properties of source object(s) to the destination
+ * object for all destination properties that resolve to `undefined`. Once a
+ * property is set, additional defaults of the same property will be ignored.
+ *
+ * @static
+ * @memberOf _
+ * @type Function
+ * @category Objects
+ * @param {Object} object The destination object.
+ * @param {Object} [source1, source2, ...] The source objects.
+ * @param- {Object} [guard] Internally used to allow working with `_.reduce`
+ * without using its callback's `key` and `object` arguments as sources.
+ * @returns {Object} Returns the destination object.
+ * @example
+ *
+ * var food = { 'name': 'apple' };
+ * _.defaults(food, { 'name': 'banana', 'type': 'fruit' });
+ * // => { 'name': 'apple', 'type': 'fruit' }
+ */
+ var defaults = createIterator(defaultsIteratorOptions);
+
+ /**
+ * Creates a sorted array of all enumerable properties, own and inherited,
+ * of `object` that have function values.
+ *
+ * @static
+ * @memberOf _
+ * @alias methods
+ * @category Objects
+ * @param {Object} object The object to inspect.
+ * @returns {Array} Returns a new array of property names that have function values.
+ * @example
+ *
+ * _.functions(_);
+ * // => ['all', 'any', 'bind', 'bindAll', 'clone', 'compact', 'compose', ...]
+ */
+ function functions(object) {
+ var result = [];
+ forIn(object, function(value, key) {
+ if (isFunction(value)) {
+ result.push(key);
+ }
+ });
+ return result.sort();
+ }
+
+ /**
+ * Checks if the specified object `property` exists and is a direct property,
+ * instead of an inherited property.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Object} object The object to check.
+ * @param {String} property The property to check for.
+ * @returns {Boolean} Returns `true` if key is a direct property, else `false`.
+ * @example
+ *
+ * _.has({ 'a': 1, 'b': 2, 'c': 3 }, 'b');
+ * // => true
+ */
+ function has(object, property) {
+ return object ? hasOwnProperty.call(object, property) : false;
+ }
+
+ /**
+ * Creates an object composed of the inverted keys and values of the given `object`.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Object} object The object to invert.
+ * @returns {Object} Returns the created inverted object.
+ * @example
+ *
+ * _.invert({ 'first': 'moe', 'second': 'larry' });
+ * // => { 'moe': 'first', 'larry': 'second' } (order is not guaranteed)
+ */
+ function invert(object) {
+ var index = -1,
+ props = keys(object),
+ length = props.length,
+ result = {};
+
+ while (++index < length) {
+ var key = props[index];
+ result[object[key]] = key;
+ }
+ return result;
+ }
+
+ /**
+ * Checks if `value` is a boolean value.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Mixed} value The value to check.
+ * @returns {Boolean} Returns `true`, if the `value` is a boolean value, else `false`.
+ * @example
+ *
+ * _.isBoolean(null);
+ * // => false
+ */
+ function isBoolean(value) {
+ return value === true || value === false || toString.call(value) == boolClass;
+ }
+
+ /**
+ * Checks if `value` is a date.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Mixed} value The value to check.
+ * @returns {Boolean} Returns `true`, if the `value` is a date, else `false`.
+ * @example
+ *
+ * _.isDate(new Date);
+ * // => true
+ */
+ function isDate(value) {
+ return value instanceof Date || toString.call(value) == dateClass;
+ }
+
+ /**
+ * Checks if `value` is a DOM element.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Mixed} value The value to check.
+ * @returns {Boolean} Returns `true`, if the `value` is a DOM element, else `false`.
+ * @example
+ *
+ * _.isElement(document.body);
+ * // => true
+ */
+ function isElement(value) {
+ return value ? value.nodeType === 1 : false;
+ }
+
+ /**
+ * Checks if `value` is empty. Arrays, strings, or `arguments` objects with a
+ * length of `0` and objects with no own enumerable properties are considered
+ * "empty".
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Array|Object|String} value The value to inspect.
+ * @returns {Boolean} Returns `true`, if the `value` is empty, else `false`.
+ * @example
+ *
+ * _.isEmpty([1, 2, 3]);
+ * // => false
+ *
+ * _.isEmpty({});
+ * // => true
+ *
+ * _.isEmpty('');
+ * // => true
+ */
+ function isEmpty(value) {
+ var result = true;
+ if (!value) {
+ return result;
+ }
+ var className = toString.call(value),
+ length = value.length;
+
+ if ((className == arrayClass || className == stringClass ||
+ className == argsClass || (noArgsClass && isArguments(value))) ||
+ (className == objectClass && typeof length == 'number' && isFunction(value.splice))) {
+ return !length;
+ }
+ forOwn(value, function() {
+ return (result = false);
+ });
+ return result;
+ }
+
+ /**
+ * Performs a deep comparison between two values to determine if they are
+ * equivalent to each other. If `callback` is passed, it will be executed to
+ * compare values. If `callback` returns `undefined`, comparisons will be handled
+ * by the method instead. The `callback` is bound to `thisArg` and invoked with
+ * two arguments; (a, b).
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Mixed} a The value to compare.
+ * @param {Mixed} b The other value to compare.
+ * @param {Function} [callback] The function to customize comparing values.
+ * @param {Mixed} [thisArg] The `this` binding of `callback`.
+ * @param- {Object} [stackA=[]] Internally used track traversed `a` objects.
+ * @param- {Object} [stackB=[]] Internally used track traversed `b` objects.
+ * @returns {Boolean} Returns `true`, if the values are equvalent, else `false`.
+ * @example
+ *
+ * var moe = { 'name': 'moe', 'age': 40 };
+ * var copy = { 'name': 'moe', 'age': 40 };
+ *
+ * moe == copy;
+ * // => false
+ *
+ * _.isEqual(moe, copy);
+ * // => true
+ *
+ * var words = ['hello', 'goodbye'];
+ * var otherWords = ['hi', 'goodbye'];
+ *
+ * _.isEqual(words, otherWords, function(a, b) {
+ * var reGreet = /^(?:hello|hi)$/i,
+ * aGreet = _.isString(a) && reGreet.test(a),
+ * bGreet = _.isString(b) && reGreet.test(b);
+ *
+ * return (aGreet || bGreet) ? (aGreet == bGreet) : undefined;
+ * });
+ * // => true
+ */
+ function isEqual(a, b, callback, thisArg, stackA, stackB) {
+ // used to indicate that when comparing objects, `a` has at least the properties of `b`
+ var whereIndicator = callback === indicatorObject;
+ if (callback && !whereIndicator) {
+ callback = typeof thisArg == 'undefined' ? callback : createCallback(callback, thisArg, 2);
+ var result = callback(a, b);
+ if (typeof result != 'undefined') {
+ return !!result;
+ }
+ }
+ // exit early for identical values
+ if (a === b) {
+ // treat `+0` vs. `-0` as not equal
+ return a !== 0 || (1 / a == 1 / b);
+ }
+ var type = typeof a,
+ otherType = typeof b;
+
+ // exit early for unlike primitive values
+ if (a === a &&
+ (!a || (type != 'function' && type != 'object')) &&
+ (!b || (otherType != 'function' && otherType != 'object'))) {
+ return false;
+ }
+ // exit early for `null` and `undefined`, avoiding ES3's Function#call behavior
+ // http://es5.github.com/#x15.3.4.4
+ if (a == null || b == null) {
+ return a === b;
+ }
+ // compare [[Class]] names
+ var className = toString.call(a),
+ otherClass = toString.call(b);
+
+ if (className == argsClass) {
+ className = objectClass;
+ }
+ if (otherClass == argsClass) {
+ otherClass = objectClass;
+ }
+ if (className != otherClass) {
+ return false;
+ }
+ switch (className) {
+ case boolClass:
+ case dateClass:
+ // coerce dates and booleans to numbers, dates to milliseconds and booleans
+ // to `1` or `0`, treating invalid dates coerced to `NaN` as not equal
+ return +a == +b;
+
+ case numberClass:
+ // treat `NaN` vs. `NaN` as equal
+ return a != +a
+ ? b != +b
+ // but treat `+0` vs. `-0` as not equal
+ : (a == 0 ? (1 / a == 1 / b) : a == +b);
+
+ case regexpClass:
+ case stringClass:
+ // coerce regexes to strings (http://es5.github.com/#x15.10.6.4)
+ // treat string primitives and their corresponding object instances as equal
+ return a == b + '';
+ }
+ var isArr = className == arrayClass;
+ if (!isArr) {
+ // unwrap any `lodash` wrapped values
+ if (a.__wrapped__ || b.__wrapped__) {
+ return isEqual(a.__wrapped__ || a, b.__wrapped__ || b, callback, thisArg, stackA, stackB);
+ }
+ // exit for functions and DOM nodes
+ if (className != objectClass || (noNodeClass && (isNode(a) || isNode(b)))) {
+ return false;
+ }
+ // in older versions of Opera, `arguments` objects have `Array` constructors
+ var ctorA = !argsAreObjects && isArguments(a) ? Object : a.constructor,
+ ctorB = !argsAreObjects && isArguments(b) ? Object : b.constructor;
+
+ // non `Object` object instances with different constructors are not equal
+ if (ctorA != ctorB && !(
+ isFunction(ctorA) && ctorA instanceof ctorA &&
+ isFunction(ctorB) && ctorB instanceof ctorB
+ )) {
+ return false;
+ }
+ }
+ // assume cyclic structures are equal
+ // the algorithm for detecting cyclic structures is adapted from ES 5.1
+ // section 15.12.3, abstract operation `JO` (http://es5.github.com/#x15.12.3)
+ stackA || (stackA = []);
+ stackB || (stackB = []);
+
+ var length = stackA.length;
+ while (length--) {
+ if (stackA[length] == a) {
+ return stackB[length] == b;
+ }
+ }
+ var size = 0;
+ result = true;
+
+ // add `a` and `b` to the stack of traversed objects
+ stackA.push(a);
+ stackB.push(b);
+
+ // recursively compare objects and arrays (susceptible to call stack limits)
+ if (isArr) {
+ length = a.length;
+ size = b.length;
+
+ // compare lengths to determine if a deep comparison is necessary
+ result = size == a.length;
+ if (!result && !whereIndicator) {
+ return result;
+ }
+ // deep compare the contents, ignoring non-numeric properties
+ while (size--) {
+ var index = length,
+ value = b[size];
+
+ if (whereIndicator) {
+ while (index--) {
+ if ((result = isEqual(a[index], value, callback, thisArg, stackA, stackB))) {
+ break;
+ }
+ }
+ } else if (!(result = isEqual(a[size], value, callback, thisArg, stackA, stackB))) {
+ break;
+ }
+ }
+ return result;
+ }
+ // deep compare objects using `forIn`, instead of `forOwn`, to avoid `Object.keys`
+ // which, in this case, is more costly
+ forIn(b, function(value, key, b) {
+ if (hasOwnProperty.call(b, key)) {
+ // count the number of properties.
+ size++;
+ // deep compare each property value.
+ return (result = hasOwnProperty.call(a, key) && isEqual(a[key], value, callback, thisArg, stackA, stackB));
+ }
+ });
+
+ if (result && !whereIndicator) {
+ // ensure both objects have the same number of properties
+ forIn(a, function(value, key, a) {
+ if (hasOwnProperty.call(a, key)) {
+ // `size` will be `-1` if `a` has more properties than `b`
+ return (result = --size > -1);
+ }
+ });
+ }
+ return result;
+ }
+
+ /**
+ * Checks if `value` is, or can be coerced to, a finite number.
+ *
+ * Note: This is not the same as native `isFinite`, which will return true for
+ * booleans and empty strings. See http://es5.github.com/#x15.1.2.5.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Mixed} value The value to check.
+ * @returns {Boolean} Returns `true`, if the `value` is finite, else `false`.
+ * @example
+ *
+ * _.isFinite(-101);
+ * // => true
+ *
+ * _.isFinite('10');
+ * // => true
+ *
+ * _.isFinite(true);
+ * // => false
+ *
+ * _.isFinite('');
+ * // => false
+ *
+ * _.isFinite(Infinity);
+ * // => false
+ */
+ function isFinite(value) {
+ return nativeIsFinite(value) && !nativeIsNaN(parseFloat(value));
+ }
+
+ /**
+ * Checks if `value` is a function.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Mixed} value The value to check.
+ * @returns {Boolean} Returns `true`, if the `value` is a function, else `false`.
+ * @example
+ *
+ * _.isFunction(_);
+ * // => true
+ */
+ function isFunction(value) {
+ return typeof value == 'function';
+ }
+ // fallback for older versions of Chrome and Safari
+ if (isFunction(/x/)) {
+ isFunction = function(value) {
+ return value instanceof Function || toString.call(value) == funcClass;
+ };
+ }
+
+ /**
+ * Checks if `value` is the language type of Object.
+ * (e.g. arrays, functions, objects, regexes, `new Number(0)`, and `new String('')`)
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Mixed} value The value to check.
+ * @returns {Boolean} Returns `true`, if the `value` is an object, else `false`.
+ * @example
+ *
+ * _.isObject({});
+ * // => true
+ *
+ * _.isObject([1, 2, 3]);
+ * // => true
+ *
+ * _.isObject(1);
+ * // => false
+ */
+ function isObject(value) {
+ // check if the value is the ECMAScript language type of Object
+ // http://es5.github.com/#x8
+ // and avoid a V8 bug
+ // http://code.google.com/p/v8/issues/detail?id=2291
+ return value ? objectTypes[typeof value] : false;
+ }
+
+ /**
+ * Checks if `value` is `NaN`.
+ *
+ * Note: This is not the same as native `isNaN`, which will return `true` for
+ * `undefined` and other values. See http://es5.github.com/#x15.1.2.4.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Mixed} value The value to check.
+ * @returns {Boolean} Returns `true`, if the `value` is `NaN`, else `false`.
+ * @example
+ *
+ * _.isNaN(NaN);
+ * // => true
+ *
+ * _.isNaN(new Number(NaN));
+ * // => true
+ *
+ * isNaN(undefined);
+ * // => true
+ *
+ * _.isNaN(undefined);
+ * // => false
+ */
+ function isNaN(value) {
+ // `NaN` as a primitive is the only value that is not equal to itself
+ // (perform the [[Class]] check first to avoid errors with some host objects in IE)
+ return isNumber(value) && value != +value
+ }
+
+ /**
+ * Checks if `value` is `null`.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Mixed} value The value to check.
+ * @returns {Boolean} Returns `true`, if the `value` is `null`, else `false`.
+ * @example
+ *
+ * _.isNull(null);
+ * // => true
+ *
+ * _.isNull(undefined);
+ * // => false
+ */
+ function isNull(value) {
+ return value === null;
+ }
+
+ /**
+ * Checks if `value` is a number.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Mixed} value The value to check.
+ * @returns {Boolean} Returns `true`, if the `value` is a number, else `false`.
+ * @example
+ *
+ * _.isNumber(8.4 * 5);
+ * // => true
+ */
+ function isNumber(value) {
+ return typeof value == 'number' || toString.call(value) == numberClass;
+ }
+
+ /**
+ * Checks if a given `value` is an object created by the `Object` constructor.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Mixed} value The value to check.
+ * @returns {Boolean} Returns `true`, if `value` is a plain object, else `false`.
+ * @example
+ *
+ * function Stooge(name, age) {
+ * this.name = name;
+ * this.age = age;
+ * }
+ *
+ * _.isPlainObject(new Stooge('moe', 40));
+ * // => false
+ *
+ * _.isPlainObject([1, 2, 3]);
+ * // => false
+ *
+ * _.isPlainObject({ 'name': 'moe', 'age': 40 });
+ * // => true
+ */
+ var isPlainObject = !getPrototypeOf ? shimIsPlainObject : function(value) {
+ if (!(value && typeof value == 'object')) {
+ return false;
+ }
+ var valueOf = value.valueOf,
+ objProto = typeof valueOf == 'function' && (objProto = getPrototypeOf(valueOf)) && getPrototypeOf(objProto);
+
+ return objProto
+ ? value == objProto || (getPrototypeOf(value) == objProto && !isArguments(value))
+ : shimIsPlainObject(value);
+ };
+
+ /**
+ * Checks if `value` is a regular expression.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Mixed} value The value to check.
+ * @returns {Boolean} Returns `true`, if the `value` is a regular expression, else `false`.
+ * @example
+ *
+ * _.isRegExp(/moe/);
+ * // => true
+ */
+ function isRegExp(value) {
+ return value instanceof RegExp || toString.call(value) == regexpClass;
+ }
+
+ /**
+ * Checks if `value` is a string.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Mixed} value The value to check.
+ * @returns {Boolean} Returns `true`, if the `value` is a string, else `false`.
+ * @example
+ *
+ * _.isString('moe');
+ * // => true
+ */
+ function isString(value) {
+ return typeof value == 'string' || toString.call(value) == stringClass;
+ }
+
+ /**
+ * Checks if `value` is `undefined`.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Mixed} value The value to check.
+ * @returns {Boolean} Returns `true`, if the `value` is `undefined`, else `false`.
+ * @example
+ *
+ * _.isUndefined(void 0);
+ * // => true
+ */
+ function isUndefined(value) {
+ return typeof value == 'undefined';
+ }
+
+ /**
+ * Recursively merges own enumerable properties of the source object(s), that
+ * don't resolve to `undefined`, into the destination object. Subsequent sources
+ * will overwrite propery assignments of previous sources. If a `callback` function
+ * is passed, it will be executed to produce the merged values of the destination
+ * and source properties. If `callback` returns `undefined`, merging will be
+ * handled by the method instead. The `callback` is bound to `thisArg` and
+ * invoked with two arguments; (objectValue, sourceValue).
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Object} object The destination object.
+ * @param {Object} [source1, source2, ...] The source objects.
+ * @param {Function} [callback] The function to customize merging properties.
+ * @param {Mixed} [thisArg] The `this` binding of `callback`.
+ * @param- {Object} [deepIndicator] Internally used to indicate that `stackA`
+ * and `stackB` are arrays of traversed objects instead of source objects.
+ * @param- {Array} [stackA=[]] Internally used to track traversed source objects.
+ * @param- {Array} [stackB=[]] Internally used to associate values with their
+ * source counterparts.
+ * @returns {Object} Returns the destination object.
+ * @example
+ *
+ * var names = {
+ * 'stooges': [
+ * { 'name': 'moe' },
+ * { 'name': 'larry' }
+ * ]
+ * };
+ *
+ * var ages = {
+ * 'stooges': [
+ * { 'age': 40 },
+ * { 'age': 50 }
+ * ]
+ * };
+ *
+ * _.merge(names, ages);
+ * // => { 'stooges': [{ 'name': 'moe', 'age': 40 }, { 'name': 'larry', 'age': 50 }] }
+ *
+ * var food = {
+ * 'fruits': ['apple'],
+ * 'vegetables': ['beet']
+ * };
+ *
+ * var otherFood = {
+ * 'fruits': ['banana'],
+ * 'vegetables': ['carrot']
+ * };
+ *
+ * _.merge(food, otherFood, function(a, b) {
+ * return _.isArray(a) ? a.concat(b) : undefined;
+ * });
+ * // => { 'fruits': ['apple', 'banana'], 'vegetables': ['beet', 'carrot] }
+ */
+ function merge(object, source, deepIndicator) {
+ var args = arguments,
+ index = 0,
+ length = 2;
+
+ if (!isObject(object)) {
+ return object;
+ }
+ if (deepIndicator === indicatorObject) {
+ var callback = args[3],
+ stackA = args[4],
+ stackB = args[5];
+ } else {
+ stackA = [];
+ stackB = [];
+
+ // allows working with `_.reduce` and `_.reduceRight` without
+ // using their `callback` arguments, `index|key` and `collection`
+ if (typeof deepIndicator != 'number') {
+ length = args.length;
+ }
+ if (length > 3 && typeof args[length - 2] == 'function') {
+ callback = createCallback(args[--length - 1], args[length--], 2);
+ } else if (length > 2 && typeof args[length - 1] == 'function') {
+ callback = args[--length];
+ }
+ }
+ while (++index < length) {
+ (isArray(args[index]) ? forEach : forOwn)(args[index], function(source, key) {
+ var found,
+ isArr,
+ result = source,
+ value = object[key];
+
+ if (source && ((isArr = isArray(source)) || isPlainObject(source))) {
+ // avoid merging previously merged cyclic sources
+ var stackLength = stackA.length;
+ while (stackLength--) {
+ if ((found = stackA[stackLength] == source)) {
+ value = stackB[stackLength];
+ break;
+ }
+ }
+ if (!found) {
+ value = isArr
+ ? (isArray(value) ? value : [])
+ : (isPlainObject(value) ? value : {});
+
+ if (callback) {
+ result = callback(value, source);
+ if (typeof result != 'undefined') {
+ value = result;
+ }
+ }
+ // add `source` and associated `value` to the stack of traversed objects
+ stackA.push(source);
+ stackB.push(value);
+
+ // recursively merge objects and arrays (susceptible to call stack limits)
+ if (!callback) {
+ value = merge(value, source, indicatorObject, callback, stackA, stackB);
+ }
+ }
+ }
+ else {
+ if (callback) {
+ result = callback(value, source);
+ if (typeof result == 'undefined') {
+ result = source;
+ }
+ }
+ if (typeof result != 'undefined') {
+ value = result;
+ }
+ }
+ object[key] = value;
+ });
+ }
+ return object;
+ }
+
+ /**
+ * Creates a shallow clone of `object` excluding the specified properties.
+ * Property names may be specified as individual arguments or as arrays of
+ * property names. If a `callback` function is passed, it will be executed
+ * for each property in the `object`, omitting the properties `callback`
+ * returns truthy for. The `callback` is bound to `thisArg` and invoked
+ * with three arguments; (value, key, object).
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Object} object The source object.
+ * @param {Function|String} callback|[prop1, prop2, ...] The properties to omit
+ * or the function called per iteration.
+ * @param {Mixed} [thisArg] The `this` binding of `callback`.
+ * @returns {Object} Returns an object without the omitted properties.
+ * @example
+ *
+ * _.omit({ 'name': 'moe', 'age': 40 }, 'age');
+ * // => { 'name': 'moe' }
+ *
+ * _.omit({ 'name': 'moe', 'age': 40 }, function(value) {
+ * return typeof value == 'number';
+ * });
+ * // => { 'name': 'moe' }
+ */
+ function omit(object, callback, thisArg) {
+ var isFunc = typeof callback == 'function',
+ result = {};
+
+ if (isFunc) {
+ callback = createCallback(callback, thisArg);
+ } else {
+ var props = concat.apply(arrayRef, arguments);
+ }
+ forIn(object, function(value, key, object) {
+ if (isFunc
+ ? !callback(value, key, object)
+ : indexOf(props, key, 1) < 0
+ ) {
+ result[key] = value;
+ }
+ });
+ return result;
+ }
+
+ /**
+ * Creates a two dimensional array of the given object's key-value pairs,
+ * i.e. `[[key1, value1], [key2, value2]]`.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Object} object The object to inspect.
+ * @returns {Array} Returns new array of key-value pairs.
+ * @example
+ *
+ * _.pairs({ 'moe': 30, 'larry': 40 });
+ * // => [['moe', 30], ['larry', 40]] (order is not guaranteed)
+ */
+ function pairs(object) {
+ var index = -1,
+ props = keys(object),
+ length = props.length,
+ result = Array(length);
+
+ while (++index < length) {
+ var key = props[index];
+ result[index] = [key, object[key]];
+ }
+ return result;
+ }
+
+ /**
+ * Creates a shallow clone of `object` composed of the specified properties.
+ * Property names may be specified as individual arguments or as arrays of property
+ * names. If `callback` is passed, it will be executed for each property in the
+ * `object`, picking the properties `callback` returns truthy for. The `callback`
+ * is bound to `thisArg` and invoked with three arguments; (value, key, object).
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Object} object The source object.
+ * @param {Array|Function|String} callback|[prop1, prop2, ...] The function called
+ * per iteration or properties to pick, either as individual arguments or arrays.
+ * @param {Mixed} [thisArg] The `this` binding of `callback`.
+ * @returns {Object} Returns an object composed of the picked properties.
+ * @example
+ *
+ * _.pick({ 'name': 'moe', '_userid': 'moe1' }, 'name');
+ * // => { 'name': 'moe' }
+ *
+ * _.pick({ 'name': 'moe', '_userid': 'moe1' }, function(value, key) {
+ * return key.charAt(0) != '_';
+ * });
+ * // => { 'name': 'moe' }
+ */
+ function pick(object, callback, thisArg) {
+ var result = {};
+ if (typeof callback != 'function') {
+ var index = 0,
+ props = concat.apply(arrayRef, arguments),
+ length = isObject(object) ? props.length : 0;
+
+ while (++index < length) {
+ var key = props[index];
+ if (key in object) {
+ result[key] = object[key];
+ }
+ }
+ } else {
+ callback = createCallback(callback, thisArg);
+ forIn(object, function(value, key, object) {
+ if (callback(value, key, object)) {
+ result[key] = value;
+ }
+ });
+ }
+ return result;
+ }
+
+ /**
+ * Creates an array composed of the own enumerable property values of `object`.
+ *
+ * @static
+ * @memberOf _
+ * @category Objects
+ * @param {Object} object The object to inspect.
+ * @returns {Array} Returns a new array of property values.
+ * @example
+ *
+ * _.values({ 'one': 1, 'two': 2, 'three': 3 });
+ * // => [1, 2, 3]
+ */
+ function values(object) {
+ var index = -1,
+ props = keys(object),
+ length = props.length,
+ result = Array(length);
+
+ while (++index < length) {
+ result[index] = object[props[index]];
+ }
+ return result;
+ }
+
+ /*--------------------------------------------------------------------------*/
+
+ /**
+ * Creates an array of elements from the specified indexes, or keys, of the
+ * `collection`. Indexes may be specified as individual arguments or as arrays
+ * of indexes.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|String} collection The collection to iterate over.
+ * @param {Array|Number|String} [index1, index2, ...] The indexes of
+ * `collection` to retrieve, either as individual arguments or arrays.
+ * @returns {Array} Returns a new array of elements corresponding to the
+ * provided indexes.
+ * @example
+ *
+ * _.at(['a', 'b', 'c', 'd', 'e'], [0, 2, 4]);
+ * // => ['a', 'c', 'e']
+ *
+ * _.at(['moe', 'larry', 'curly'], 0, 2);
+ * // => ['moe', 'curly']
+ */
+ function at(collection) {
+ var index = -1,
+ props = concat.apply(arrayRef, slice(arguments, 1)),
+ length = props.length,
+ result = Array(length);
+
+ if (noCharByIndex && isString(collection)) {
+ collection = collection.split('');
+ }
+ while(++index < length) {
+ result[index] = collection[props[index]];
+ }
+ return result;
+ }
+
+ /**
+ * Checks if a given `target` element is present in a `collection` using strict
+ * equality for comparisons, i.e. `===`. If `fromIndex` is negative, it is used
+ * as the offset from the end of the collection.
+ *
+ * @static
+ * @memberOf _
+ * @alias include
+ * @category Collections
+ * @param {Array|Object|String} collection The collection to iterate over.
+ * @param {Mixed} target The value to check for.
+ * @param {Number} [fromIndex=0] The index to search from.
+ * @returns {Boolean} Returns `true` if the `target` element is found, else `false`.
+ * @example
+ *
+ * _.contains([1, 2, 3], 1);
+ * // => true
+ *
+ * _.contains([1, 2, 3], 1, 2);
+ * // => false
+ *
+ * _.contains({ 'name': 'moe', 'age': 40 }, 'moe');
+ * // => true
+ *
+ * _.contains('curly', 'ur');
+ * // => true
+ */
+ function contains(collection, target, fromIndex) {
+ var index = -1,
+ length = collection ? collection.length : 0,
+ result = false;
+
+ fromIndex = (fromIndex < 0 ? nativeMax(0, length + fromIndex) : fromIndex) || 0;
+ if (typeof length == 'number') {
+ result = (isString(collection)
+ ? collection.indexOf(target, fromIndex)
+ : indexOf(collection, target, fromIndex)
+ ) > -1;
+ } else {
+ each(collection, function(value) {
+ if (++index >= fromIndex) {
+ return !(result = value === target);
+ }
+ });
+ }
+ return result;
+ }
+
+ /**
+ * Creates an object composed of keys returned from running each element of the
+ * `collection` through the given `callback`. The corresponding value of each key
+ * is the number of times the key was returned by the `callback`. The `callback`
+ * is bound to `thisArg` and invoked with three arguments; (value, index|key, collection).
+ *
+ * If a property name is passed for `callback`, the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is passed for `callback`, the created "_.where" style callback
+ * will return `true` for elements that have the propeties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|String} collection The collection to iterate over.
+ * @param {Function|Object|String} [callback=identity] The function called per
+ * iteration. If a property name or object is passed, it will be used to create
+ * a "_.pluck" or "_.where" style callback, respectively.
+ * @param {Mixed} [thisArg] The `this` binding of `callback`.
+ * @returns {Object} Returns the composed aggregate object.
+ * @example
+ *
+ * _.countBy([4.3, 6.1, 6.4], function(num) { return Math.floor(num); });
+ * // => { '4': 1, '6': 2 }
+ *
+ * _.countBy([4.3, 6.1, 6.4], function(num) { return this.floor(num); }, Math);
+ * // => { '4': 1, '6': 2 }
+ *
+ * _.countBy(['one', 'two', 'three'], 'length');
+ * // => { '3': 2, '5': 1 }
+ */
+ function countBy(collection, callback, thisArg) {
+ var result = {};
+ callback = createCallback(callback, thisArg);
+
+ forEach(collection, function(value, key, collection) {
+ key = callback(value, key, collection) + '';
+ (hasOwnProperty.call(result, key) ? result[key]++ : result[key] = 1);
+ });
+ return result;
+ }
+
+ /**
+ * Checks if the `callback` returns a truthy value for **all** elements of a
+ * `collection`. The `callback` is bound to `thisArg` and invoked with three
+ * arguments; (value, index|key, collection).
+ *
+ * If a property name is passed for `callback`, the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is passed for `callback`, the created "_.where" style callback
+ * will return `true` for elements that have the propeties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @alias all
+ * @category Collections
+ * @param {Array|Object|String} collection The collection to iterate over.
+ * @param {Function|Object|String} [callback=identity] The function called per
+ * iteration. If a property name or object is passed, it will be used to create
+ * a "_.pluck" or "_.where" style callback, respectively.
+ * @param {Mixed} [thisArg] The `this` binding of `callback`.
+ * @returns {Boolean} Returns `true` if all elements pass the callback check,
+ * else `false`.
+ * @example
+ *
+ * _.every([true, 1, null, 'yes'], Boolean);
+ * // => false
+ *
+ * var stooges = [
+ * { 'name': 'moe', 'age': 40 },
+ * { 'name': 'larry', 'age': 50 }
+ * ];
+ *
+ * // using "_.pluck" callback shorthand
+ * _.every(stooges, 'age');
+ * // => true
+ *
+ * // using "_.where" callback shorthand
+ * _.every(stooges, { 'age': 50 });
+ * // => false
+ */
+ function every(collection, callback, thisArg) {
+ var result = true;
+ callback = createCallback(callback, thisArg);
+
+ if (isArray(collection)) {
+ var index = -1,
+ length = collection.length;
+
+ while (++index < length) {
+ if (!(result = !!callback(collection[index], index, collection))) {
+ break;
+ }
+ }
+ } else {
+ each(collection, function(value, index, collection) {
+ return (result = !!callback(value, index, collection));
+ });
+ }
+ return result;
+ }
+
+ /**
+ * Examines each element in a `collection`, returning an array of all elements
+ * the `callback` returns truthy for. The `callback` is bound to `thisArg` and
+ * invoked with three arguments; (value, index|key, collection).
+ *
+ * If a property name is passed for `callback`, the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is passed for `callback`, the created "_.where" style callback
+ * will return `true` for elements that have the propeties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @alias select
+ * @category Collections
+ * @param {Array|Object|String} collection The collection to iterate over.
+ * @param {Function|Object|String} [callback=identity] The function called per
+ * iteration. If a property name or object is passed, it will be used to create
+ * a "_.pluck" or "_.where" style callback, respectively.
+ * @param {Mixed} [thisArg] The `this` binding of `callback`.
+ * @returns {Array} Returns a new array of elements that passed the callback check.
+ * @example
+ *
+ * var evens = _.filter([1, 2, 3, 4, 5, 6], function(num) { return num % 2 == 0; });
+ * // => [2, 4, 6]
+ *
+ * var food = [
+ * { 'name': 'apple', 'organic': false, 'type': 'fruit' },
+ * { 'name': 'carrot', 'organic': true, 'type': 'vegetable' }
+ * ];
+ *
+ * // using "_.pluck" callback shorthand
+ * _.filter(food, 'organic');
+ * // => [{ 'name': 'carrot', 'organic': true, 'type': 'vegetable' }]
+ *
+ * // using "_.where" callback shorthand
+ * _.filter(food, { 'type': 'fruit' });
+ * // => [{ 'name': 'apple', 'organic': false, 'type': 'fruit' }]
+ */
+ function filter(collection, callback, thisArg) {
+ var result = [];
+ callback = createCallback(callback, thisArg);
+
+ if (isArray(collection)) {
+ var index = -1,
+ length = collection.length;
+
+ while (++index < length) {
+ var value = collection[index];
+ if (callback(value, index, collection)) {
+ result.push(value);
+ }
+ }
+ } else {
+ each(collection, function(value, index, collection) {
+ if (callback(value, index, collection)) {
+ result.push(value);
+ }
+ });
+ }
+ return result;
+ }
+
+ /**
+ * Examines each element in a `collection`, returning the first that the `callback`
+ * returns truthy for. The `callback` is bound to `thisArg` and invoked with three
+ * arguments; (value, index|key, collection).
+ *
+ * If a property name is passed for `callback`, the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is passed for `callback`, the created "_.where" style callback
+ * will return `true` for elements that have the propeties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @alias detect
+ * @category Collections
+ * @param {Array|Object|String} collection The collection to iterate over.
+ * @param {Function|Object|String} [callback=identity] The function called per
+ * iteration. If a property name or object is passed, it will be used to create
+ * a "_.pluck" or "_.where" style callback, respectively.
+ * @param {Mixed} [thisArg] The `this` binding of `callback`.
+ * @returns {Mixed} Returns the element that passed the callback check,
+ * else `undefined`.
+ * @example
+ *
+ * var even = _.find([1, 2, 3, 4, 5, 6], function(num) { return num % 2 == 0; });
+ * // => 2
+ *
+ * var food = [
+ * { 'name': 'apple', 'organic': false, 'type': 'fruit' },
+ * { 'name': 'banana', 'organic': true, 'type': 'fruit' },
+ * { 'name': 'beet', 'organic': false, 'type': 'vegetable' },
+ * { 'name': 'carrot', 'organic': true, 'type': 'vegetable' }
+ * ];
+ *
+ * // using "_.where" callback shorthand
+ * var veggie = _.find(food, { 'type': 'vegetable' });
+ * // => { 'name': 'beet', 'organic': false, 'type': 'vegetable' }
+ *
+ * // using "_.pluck" callback shorthand
+ * var healthy = _.find(food, 'organic');
+ * // => { 'name': 'banana', 'organic': true, 'type': 'fruit' }
+ */
+ function find(collection, callback, thisArg) {
+ var result;
+ callback = createCallback(callback, thisArg);
+
+ forEach(collection, function(value, index, collection) {
+ if (callback(value, index, collection)) {
+ result = value;
+ return false;
+ }
+ });
+ return result;
+ }
+
+ /**
+ * Iterates over a `collection`, executing the `callback` for each element in
+ * the `collection`. The `callback` is bound to `thisArg` and invoked with three
+ * arguments; (value, index|key, collection). Callbacks may exit iteration early
+ * by explicitly returning `false`.
+ *
+ * @static
+ * @memberOf _
+ * @alias each
+ * @category Collections
+ * @param {Array|Object|String} collection The collection to iterate over.
+ * @param {Function} [callback=identity] The function called per iteration.
+ * @param {Mixed} [thisArg] The `this` binding of `callback`.
+ * @returns {Array|Object|String} Returns `collection`.
+ * @example
+ *
+ * _([1, 2, 3]).forEach(alert).join(',');
+ * // => alerts each number and returns '1,2,3'
+ *
+ * _.forEach({ 'one': 1, 'two': 2, 'three': 3 }, alert);
+ * // => alerts each number value (order is not guaranteed)
+ */
+ function forEach(collection, callback, thisArg) {
+ if (callback && typeof thisArg == 'undefined' && isArray(collection)) {
+ var index = -1,
+ length = collection.length;
+
+ while (++index < length) {
+ if (callback(collection[index], index, collection) === false) {
+ break;
+ }
+ }
+ } else {
+ each(collection, callback, thisArg);
+ }
+ return collection;
+ }
+
+ /**
+ * Creates an object composed of keys returned from running each element of the
+ * `collection` through the `callback`. The corresponding value of each key is
+ * an array of elements passed to `callback` that returned the key. The `callback`
+ * is bound to `thisArg` and invoked with three arguments; (value, index|key, collection).
+ *
+ * If a property name is passed for `callback`, the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is passed for `callback`, the created "_.where" style callback
+ * will return `true` for elements that have the propeties of the given object,
+ * else `false`
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|String} collection The collection to iterate over.
+ * @param {Function|Object|String} [callback=identity] The function called per
+ * iteration. If a property name or object is passed, it will be used to create
+ * a "_.pluck" or "_.where" style callback, respectively.
+ * @param {Mixed} [thisArg] The `this` binding of `callback`.
+ * @returns {Object} Returns the composed aggregate object.
+ * @example
+ *
+ * _.groupBy([4.2, 6.1, 6.4], function(num) { return Math.floor(num); });
+ * // => { '4': [4.2], '6': [6.1, 6.4] }
+ *
+ * _.groupBy([4.2, 6.1, 6.4], function(num) { return this.floor(num); }, Math);
+ * // => { '4': [4.2], '6': [6.1, 6.4] }
+ *
+ * // using "_.pluck" callback shorthand
+ * _.groupBy(['one', 'two', 'three'], 'length');
+ * // => { '3': ['one', 'two'], '5': ['three'] }
+ */
+ function groupBy(collection, callback, thisArg) {
+ var result = {};
+ callback = createCallback(callback, thisArg);
+
+ forEach(collection, function(value, key, collection) {
+ key = callback(value, key, collection) + '';
+ (hasOwnProperty.call(result, key) ? result[key] : result[key] = []).push(value);
+ });
+ return result;
+ }
+
+ /**
+ * Invokes the method named by `methodName` on each element in the `collection`,
+ * returning an array of the results of each invoked method. Additional arguments
+ * will be passed to each invoked method. If `methodName` is a function, it will
+ * be invoked for, and `this` bound to, each element in the `collection`.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|String} collection The collection to iterate over.
+ * @param {Function|String} methodName The name of the method to invoke or
+ * the function invoked per iteration.
+ * @param {Mixed} [arg1, arg2, ...] Arguments to invoke the method with.
+ * @returns {Array} Returns a new array of the results of each invoked method.
+ * @example
+ *
+ * _.invoke([[5, 1, 7], [3, 2, 1]], 'sort');
+ * // => [[1, 5, 7], [1, 2, 3]]
+ *
+ * _.invoke([123, 456], String.prototype.split, '');
+ * // => [['1', '2', '3'], ['4', '5', '6']]
+ */
+ function invoke(collection, methodName) {
+ var args = slice(arguments, 2),
+ index = -1,
+ isFunc = typeof methodName == 'function',
+ length = collection ? collection.length : 0,
+ result = Array(typeof length == 'number' ? length : 0);
+
+ forEach(collection, function(value) {
+ result[++index] = (isFunc ? methodName : value[methodName]).apply(value, args);
+ });
+ return result;
+ }
+
+ /**
+ * Creates an array of values by running each element in the `collection`
+ * through the `callback`. The `callback` is bound to `thisArg` and invoked with
+ * three arguments; (value, index|key, collection).
+ *
+ * If a property name is passed for `callback`, the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is passed for `callback`, the created "_.where" style callback
+ * will return `true` for elements that have the propeties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @alias collect
+ * @category Collections
+ * @param {Array|Object|String} collection The collection to iterate over.
+ * @param {Function|Object|String} [callback=identity] The function called per
+ * iteration. If a property name or object is passed, it will be used to create
+ * a "_.pluck" or "_.where" style callback, respectively.
+ * @param {Mixed} [thisArg] The `this` binding of `callback`.
+ * @returns {Array} Returns a new array of the results of each `callback` execution.
+ * @example
+ *
+ * _.map([1, 2, 3], function(num) { return num * 3; });
+ * // => [3, 6, 9]
+ *
+ * _.map({ 'one': 1, 'two': 2, 'three': 3 }, function(num) { return num * 3; });
+ * // => [3, 6, 9] (order is not guaranteed)
+ *
+ * var stooges = [
+ * { 'name': 'moe', 'age': 40 },
+ * { 'name': 'larry', 'age': 50 }
+ * ];
+ *
+ * // using "_.pluck" callback shorthand
+ * _.map(stooges, 'name');
+ * // => ['moe', 'larry']
+ */
+ function map(collection, callback, thisArg) {
+ var index = -1,
+ length = collection ? collection.length : 0,
+ result = Array(typeof length == 'number' ? length : 0);
+
+ callback = createCallback(callback, thisArg);
+ if (isArray(collection)) {
+ while (++index < length) {
+ result[index] = callback(collection[index], index, collection);
+ }
+ } else {
+ each(collection, function(value, key, collection) {
+ result[++index] = callback(value, key, collection);
+ });
+ }
+ return result;
+ }
+
+ /**
+ * Retrieves the maximum value of an `array`. If `callback` is passed,
+ * it will be executed for each value in the `array` to generate the
+ * criterion by which the value is ranked. The `callback` is bound to
+ * `thisArg` and invoked with three arguments; (value, index, collection).
+ *
+ * If a property name is passed for `callback`, the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is passed for `callback`, the created "_.where" style callback
+ * will return `true` for elements that have the propeties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|String} collection The collection to iterate over.
+ * @param {Function|Object|String} [callback=identity] The function called per
+ * iteration. If a property name or object is passed, it will be used to create
+ * a "_.pluck" or "_.where" style callback, respectively.
+ * @param {Mixed} [thisArg] The `this` binding of `callback`.
+ * @returns {Mixed} Returns the maximum value.
+ * @example
+ *
+ * _.max([4, 2, 8, 6]);
+ * // => 8
+ *
+ * var stooges = [
+ * { 'name': 'moe', 'age': 40 },
+ * { 'name': 'larry', 'age': 50 }
+ * ];
+ *
+ * _.max(stooges, function(stooge) { return stooge.age; });
+ * // => { 'name': 'larry', 'age': 50 };
+ *
+ * // using "_.pluck" callback shorthand
+ * _.max(stooges, 'age');
+ * // => { 'name': 'larry', 'age': 50 };
+ */
+ function max(collection, callback, thisArg) {
+ var computed = -Infinity,
+ result = computed;
+
+ if (!callback && isArray(collection)) {
+ var index = -1,
+ length = collection.length;
+
+ while (++index < length) {
+ var value = collection[index];
+ if (value > result) {
+ result = value;
+ }
+ }
+ } else {
+ callback = !callback && isString(collection)
+ ? charAtCallback
+ : createCallback(callback, thisArg);
+
+ each(collection, function(value, index, collection) {
+ var current = callback(value, index, collection);
+ if (current > computed) {
+ computed = current;
+ result = value;
+ }
+ });
+ }
+ return result;
+ }
+
+ /**
+ * Retrieves the minimum value of an `array`. If `callback` is passed,
+ * it will be executed for each value in the `array` to generate the
+ * criterion by which the value is ranked. The `callback` is bound to `thisArg`
+ * and invoked with three arguments; (value, index, collection).
+ *
+ * If a property name is passed for `callback`, the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is passed for `callback`, the created "_.where" style callback
+ * will return `true` for elements that have the propeties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|String} collection The collection to iterate over.
+ * @param {Function|Object|String} [callback=identity] The function called per
+ * iteration. If a property name or object is passed, it will be used to create
+ * a "_.pluck" or "_.where" style callback, respectively.
+ * @param {Mixed} [thisArg] The `this` binding of `callback`.
+ * @returns {Mixed} Returns the minimum value.
+ * @example
+ *
+ * _.min([4, 2, 8, 6]);
+ * // => 2
+ *
+ * var stooges = [
+ * { 'name': 'moe', 'age': 40 },
+ * { 'name': 'larry', 'age': 50 }
+ * ];
+ *
+ * _.min(stooges, function(stooge) { return stooge.age; });
+ * // => { 'name': 'moe', 'age': 40 };
+ *
+ * // using "_.pluck" callback shorthand
+ * _.min(stooges, 'age');
+ * // => { 'name': 'moe', 'age': 40 };
+ */
+ function min(collection, callback, thisArg) {
+ var computed = Infinity,
+ result = computed;
+
+ if (!callback && isArray(collection)) {
+ var index = -1,
+ length = collection.length;
+
+ while (++index < length) {
+ var value = collection[index];
+ if (value < result) {
+ result = value;
+ }
+ }
+ } else {
+ callback = !callback && isString(collection)
+ ? charAtCallback
+ : createCallback(callback, thisArg);
+
+ each(collection, function(value, index, collection) {
+ var current = callback(value, index, collection);
+ if (current < computed) {
+ computed = current;
+ result = value;
+ }
+ });
+ }
+ return result;
+ }
+
+ /**
+ * Retrieves the value of a specified property from all elements in the `collection`.
+ *
+ * @static
+ * @memberOf _
+ * @type Function
+ * @category Collections
+ * @param {Array|Object|String} collection The collection to iterate over.
+ * @param {String} property The property to pluck.
+ * @returns {Array} Returns a new array of property values.
+ * @example
+ *
+ * var stooges = [
+ * { 'name': 'moe', 'age': 40 },
+ * { 'name': 'larry', 'age': 50 }
+ * ];
+ *
+ * _.pluck(stooges, 'name');
+ * // => ['moe', 'larry']
+ */
+ var pluck = map;
+
+ /**
+ * Reduces a `collection` to a value that is the accumulated result of running
+ * each element in the `collection` through the `callback`, where each successive
+ * `callback` execution consumes the return value of the previous execution.
+ * If `accumulator` is not passed, the first element of the `collection` will be
+ * used as the initial `accumulator` value. The `callback` is bound to `thisArg`
+ * and invoked with four arguments; (accumulator, value, index|key, collection).
+ *
+ * @static
+ * @memberOf _
+ * @alias foldl, inject
+ * @category Collections
+ * @param {Array|Object|String} collection The collection to iterate over.
+ * @param {Function} [callback=identity] The function called per iteration.
+ * @param {Mixed} [accumulator] Initial value of the accumulator.
+ * @param {Mixed} [thisArg] The `this` binding of `callback`.
+ * @returns {Mixed} Returns the accumulated value.
+ * @example
+ *
+ * var sum = _.reduce([1, 2, 3], function(sum, num) {
+ * return sum + num;
+ * });
+ * // => 6
+ *
+ * var mapped = _.reduce({ 'a': 1, 'b': 2, 'c': 3 }, function(result, num, key) {
+ * result[key] = num * 3;
+ * return result;
+ * }, {});
+ * // => { 'a': 3, 'b': 6, 'c': 9 }
+ */
+ function reduce(collection, callback, accumulator, thisArg) {
+ var noaccum = arguments.length < 3;
+ callback = createCallback(callback, thisArg, 4);
+
+ if (isArray(collection)) {
+ var index = -1,
+ length = collection.length;
+
+ if (noaccum) {
+ accumulator = collection[++index];
+ }
+ while (++index < length) {
+ accumulator = callback(accumulator, collection[index], index, collection);
+ }
+ } else {
+ each(collection, function(value, index, collection) {
+ accumulator = noaccum
+ ? (noaccum = false, value)
+ : callback(accumulator, value, index, collection)
+ });
+ }
+ return accumulator;
+ }
+
+ /**
+ * This method is similar to `_.reduce`, except that it iterates over a
+ * `collection` from right to left.
+ *
+ * @static
+ * @memberOf _
+ * @alias foldr
+ * @category Collections
+ * @param {Array|Object|String} collection The collection to iterate over.
+ * @param {Function} [callback=identity] The function called per iteration.
+ * @param {Mixed} [accumulator] Initial value of the accumulator.
+ * @param {Mixed} [thisArg] The `this` binding of `callback`.
+ * @returns {Mixed} Returns the accumulated value.
+ * @example
+ *
+ * var list = [[0, 1], [2, 3], [4, 5]];
+ * var flat = _.reduceRight(list, function(a, b) { return a.concat(b); }, []);
+ * // => [4, 5, 2, 3, 0, 1]
+ */
+ function reduceRight(collection, callback, accumulator, thisArg) {
+ var iterable = collection,
+ length = collection ? collection.length : 0,
+ noaccum = arguments.length < 3;
+
+ if (typeof length != 'number') {
+ var props = keys(collection);
+ length = props.length;
+ } else if (noCharByIndex && isString(collection)) {
+ iterable = collection.split('');
+ }
+ callback = createCallback(callback, thisArg, 4);
+ forEach(collection, function(value, index, collection) {
+ index = props ? props[--length] : --length;
+ accumulator = noaccum
+ ? (noaccum = false, iterable[index])
+ : callback(accumulator, iterable[index], index, collection);
+ });
+ return accumulator;
+ }
+
+ /**
+ * The opposite of `_.filter`, this method returns the elements of a
+ * `collection` that `callback` does **not** return truthy for.
+ *
+ * If a property name is passed for `callback`, the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is passed for `callback`, the created "_.where" style callback
+ * will return `true` for elements that have the propeties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|String} collection The collection to iterate over.
+ * @param {Function|Object|String} [callback=identity] The function called per
+ * iteration. If a property name or object is passed, it will be used to create
+ * a "_.pluck" or "_.where" style callback, respectively.
+ * @param {Mixed} [thisArg] The `this` binding of `callback`.
+ * @returns {Array} Returns a new array of elements that did **not** pass the
+ * callback check.
+ * @example
+ *
+ * var odds = _.reject([1, 2, 3, 4, 5, 6], function(num) { return num % 2 == 0; });
+ * // => [1, 3, 5]
+ *
+ * var food = [
+ * { 'name': 'apple', 'organic': false, 'type': 'fruit' },
+ * { 'name': 'carrot', 'organic': true, 'type': 'vegetable' }
+ * ];
+ *
+ * // using "_.pluck" callback shorthand
+ * _.reject(food, 'organic');
+ * // => [{ 'name': 'apple', 'organic': false, 'type': 'fruit' }]
+ *
+ * // using "_.where" callback shorthand
+ * _.reject(food, { 'type': 'fruit' });
+ * // => [{ 'name': 'carrot', 'organic': true, 'type': 'vegetable' }]
+ */
+ function reject(collection, callback, thisArg) {
+ callback = createCallback(callback, thisArg);
+ return filter(collection, function(value, index, collection) {
+ return !callback(value, index, collection);
+ });
+ }
+
+ /**
+ * Creates an array of shuffled `array` values, using a version of the
+ * Fisher-Yates shuffle. See http://en.wikipedia.org/wiki/Fisher-Yates_shuffle.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|String} collection The collection to shuffle.
+ * @returns {Array} Returns a new shuffled collection.
+ * @example
+ *
+ * _.shuffle([1, 2, 3, 4, 5, 6]);
+ * // => [4, 1, 6, 3, 5, 2]
+ */
+ function shuffle(collection) {
+ var index = -1,
+ length = collection ? collection.length : 0,
+ result = Array(typeof length == 'number' ? length : 0);
+
+ forEach(collection, function(value) {
+ var rand = floor(nativeRandom() * (++index + 1));
+ result[index] = result[rand];
+ result[rand] = value;
+ });
+ return result;
+ }
+
+ /**
+ * Gets the size of the `collection` by returning `collection.length` for arrays
+ * and array-like objects or the number of own enumerable properties for objects.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|String} collection The collection to inspect.
+ * @returns {Number} Returns `collection.length` or number of own enumerable properties.
+ * @example
+ *
+ * _.size([1, 2]);
+ * // => 2
+ *
+ * _.size({ 'one': 1, 'two': 2, 'three': 3 });
+ * // => 3
+ *
+ * _.size('curly');
+ * // => 5
+ */
+ function size(collection) {
+ var length = collection ? collection.length : 0;
+ return typeof length == 'number' ? length : keys(collection).length;
+ }
+
+ /**
+ * Checks if the `callback` returns a truthy value for **any** element of a
+ * `collection`. The function returns as soon as it finds passing value, and
+ * does not iterate over the entire `collection`. The `callback` is bound to
+ * `thisArg` and invoked with three arguments; (value, index|key, collection).
+ *
+ * If a property name is passed for `callback`, the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is passed for `callback`, the created "_.where" style callback
+ * will return `true` for elements that have the propeties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @alias any
+ * @category Collections
+ * @param {Array|Object|String} collection The collection to iterate over.
+ * @param {Function|Object|String} [callback=identity] The function called per
+ * iteration. If a property name or object is passed, it will be used to create
+ * a "_.pluck" or "_.where" style callback, respectively.
+ * @param {Mixed} [thisArg] The `this` binding of `callback`.
+ * @returns {Boolean} Returns `true` if any element passes the callback check,
+ * else `false`.
+ * @example
+ *
+ * _.some([null, 0, 'yes', false], Boolean);
+ * // => true
+ *
+ * var food = [
+ * { 'name': 'apple', 'organic': false, 'type': 'fruit' },
+ * { 'name': 'carrot', 'organic': true, 'type': 'vegetable' }
+ * ];
+ *
+ * // using "_.pluck" callback shorthand
+ * _.some(food, 'organic');
+ * // => true
+ *
+ * // using "_.where" callback shorthand
+ * _.some(food, { 'type': 'meat' });
+ * // => false
+ */
+ function some(collection, callback, thisArg) {
+ var result;
+ callback = createCallback(callback, thisArg);
+
+ if (isArray(collection)) {
+ var index = -1,
+ length = collection.length;
+
+ while (++index < length) {
+ if ((result = callback(collection[index], index, collection))) {
+ break;
+ }
+ }
+ } else {
+ each(collection, function(value, index, collection) {
+ return !(result = callback(value, index, collection));
+ });
+ }
+ return !!result;
+ }
+
+ /**
+ * Creates an array of elements, sorted in ascending order by the results of
+ * running each element in the `collection` through the `callback`. This method
+ * performs a stable sort, that is, it will preserve the original sort order of
+ * equal elements. The `callback` is bound to `thisArg` and invoked with three
+ * arguments; (value, index|key, collection).
+ *
+ * If a property name is passed for `callback`, the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is passed for `callback`, the created "_.where" style callback
+ * will return `true` for elements that have the propeties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|String} collection The collection to iterate over.
+ * @param {Function|Object|String} [callback=identity] The function called per
+ * iteration. If a property name or object is passed, it will be used to create
+ * a "_.pluck" or "_.where" style callback, respectively.
+ * @param {Mixed} [thisArg] The `this` binding of `callback`.
+ * @returns {Array} Returns a new array of sorted elements.
+ * @example
+ *
+ * _.sortBy([1, 2, 3], function(num) { return Math.sin(num); });
+ * // => [3, 1, 2]
+ *
+ * _.sortBy([1, 2, 3], function(num) { return this.sin(num); }, Math);
+ * // => [3, 1, 2]
+ *
+ * // using "_.pluck" callback shorthand
+ * _.sortBy(['banana', 'strawberry', 'apple'], 'length');
+ * // => ['apple', 'banana', 'strawberry']
+ */
+ function sortBy(collection, callback, thisArg) {
+ var index = -1,
+ length = collection ? collection.length : 0,
+ result = Array(typeof length == 'number' ? length : 0);
+
+ callback = createCallback(callback, thisArg);
+ forEach(collection, function(value, key, collection) {
+ result[++index] = {
+ 'criteria': callback(value, key, collection),
+ 'index': index,
+ 'value': value
+ };
+ });
+
+ length = result.length;
+ result.sort(compareAscending);
+ while (length--) {
+ result[length] = result[length].value;
+ }
+ return result;
+ }
+
+ /**
+ * Converts the `collection` to an array.
+ *
+ * @static
+ * @memberOf _
+ * @category Collections
+ * @param {Array|Object|String} collection The collection to convert.
+ * @returns {Array} Returns the new converted array.
+ * @example
+ *
+ * (function() { return _.toArray(arguments).slice(1); })(1, 2, 3, 4);
+ * // => [2, 3, 4]
+ */
+ function toArray(collection) {
+ if (collection && typeof collection.length == 'number') {
+ return noCharByIndex && isString(collection)
+ ? collection.split('')
+ : slice(collection);
+ }
+ return values(collection);
+ }
+
+ /**
+ * Examines each element in a `collection`, returning an array of all elements
+ * that have the given `properties`. When checking `properties`, this method
+ * performs a deep comparison between values to determine if they are equivalent
+ * to each other.
+ *
+ * @static
+ * @memberOf _
+ * @type Function
+ * @category Collections
+ * @param {Array|Object|String} collection The collection to iterate over.
+ * @param {Object} properties The object of property values to filter by.
+ * @returns {Array} Returns a new array of elements that have the given `properties`.
+ * @example
+ *
+ * var stooges = [
+ * { 'name': 'moe', 'age': 40 },
+ * { 'name': 'larry', 'age': 50 }
+ * ];
+ *
+ * _.where(stooges, { 'age': 40 });
+ * // => [{ 'name': 'moe', 'age': 40 }]
+ */
+ var where = filter;
+
+ /*--------------------------------------------------------------------------*/
+
+ /**
+ * Creates an array with all falsey values of `array` removed. The values
+ * `false`, `null`, `0`, `""`, `undefined` and `NaN` are all falsey.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} array The array to compact.
+ * @returns {Array} Returns a new filtered array.
+ * @example
+ *
+ * _.compact([0, 1, false, 2, '', 3]);
+ * // => [1, 2, 3]
+ */
+ function compact(array) {
+ var index = -1,
+ length = array ? array.length : 0,
+ result = [];
+
+ while (++index < length) {
+ var value = array[index];
+ if (value) {
+ result.push(value);
+ }
+ }
+ return result;
+ }
+
+ /**
+ * Creates an array of `array` elements not present in the other arrays
+ * using strict equality for comparisons, i.e. `===`.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} array The array to process.
+ * @param {Array} [array1, array2, ...] Arrays to check.
+ * @returns {Array} Returns a new array of `array` elements not present in the
+ * other arrays.
+ * @example
+ *
+ * _.difference([1, 2, 3, 4, 5], [5, 2, 10]);
+ * // => [1, 3, 4]
+ */
+ function difference(array) {
+ var index = -1,
+ length = array ? array.length : 0,
+ flattened = concat.apply(arrayRef, arguments),
+ contains = cachedContains(flattened, length),
+ result = [];
+
+ while (++index < length) {
+ var value = array[index];
+ if (!contains(value)) {
+ result.push(value);
+ }
+ }
+ return result;
+ }
+
+ /**
+ * Gets the first element of the `array`. If a number `n` is passed, the first
+ * `n` elements of the `array` are returned. If a `callback` function is passed,
+ * the first elements the `callback` returns truthy for are returned. The `callback`
+ * is bound to `thisArg` and invoked with three arguments; (value, index, array).
+ *
+ * If a property name is passed for `callback`, the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is passed for `callback`, the created "_.where" style callback
+ * will return `true` for elements that have the propeties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @alias head, take
+ * @category Arrays
+ * @param {Array} array The array to query.
+ * @param {Function|Object|Number|String} [callback|n] The function called
+ * per element or the number of elements to return. If a property name or
+ * object is passed, it will be used to create a "_.pluck" or "_.where"
+ * style callback, respectively.
+ * @param {Mixed} [thisArg] The `this` binding of `callback`.
+ * @returns {Mixed} Returns the first element(s) of `array`.
+ * @example
+ *
+ * _.first([1, 2, 3]);
+ * // => 1
+ *
+ * _.first([1, 2, 3], 2);
+ * // => [1, 2]
+ *
+ * _.first([1, 2, 3], function(num) {
+ * return num < 3;
+ * });
+ * // => [1, 2]
+ *
+ * var food = [
+ * { 'name': 'banana', 'organic': true },
+ * { 'name': 'beet', 'organic': false },
+ * ];
+ *
+ * // using "_.pluck" callback shorthand
+ * _.first(food, 'organic');
+ * // => [{ 'name': 'banana', 'organic': true }]
+ *
+ * var food = [
+ * { 'name': 'apple', 'type': 'fruit' },
+ * { 'name': 'banana', 'type': 'fruit' },
+ * { 'name': 'beet', 'type': 'vegetable' }
+ * ];
+ *
+ * // using "_.where" callback shorthand
+ * _.first(food, { 'type': 'fruit' });
+ * // => [{ 'name': 'apple', 'type': 'fruit' }, { 'name': 'banana', 'type': 'fruit' }]
+ */
+ function first(array, callback, thisArg) {
+ if (array) {
+ var n = 0,
+ length = array.length;
+
+ if (typeof callback != 'number' && callback != null) {
+ var index = -1;
+ callback = createCallback(callback, thisArg);
+ while (++index < length && callback(array[index], index, array)) {
+ n++;
+ }
+ } else {
+ n = callback;
+ if (n == null || thisArg) {
+ return array[0];
+ }
+ }
+ return slice(array, 0, nativeMin(nativeMax(0, n), length));
+ }
+ }
+
+ /**
+ * Flattens a nested array (the nesting can be to any depth). If `shallow` is
+ * truthy, `array` will only be flattened a single level.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} array The array to compact.
+ * @param {Boolean} shallow A flag to indicate only flattening a single level.
+ * @returns {Array} Returns a new flattened array.
+ * @example
+ *
+ * _.flatten([1, [2], [3, [[4]]]]);
+ * // => [1, 2, 3, 4];
+ *
+ * _.flatten([1, [2], [3, [[4]]]], true);
+ * // => [1, 2, 3, [[4]]];
+ */
+ function flatten(array, shallow) {
+ var index = -1,
+ length = array ? array.length : 0,
+ result = [];
+
+ while (++index < length) {
+ var value = array[index];
+
+ // recursively flatten arrays (susceptible to call stack limits)
+ if (isArray(value)) {
+ push.apply(result, shallow ? value : flatten(value));
+ } else {
+ result.push(value);
+ }
+ }
+ return result;
+ }
+
+ /**
+ * Gets the index at which the first occurrence of `value` is found using
+ * strict equality for comparisons, i.e. `===`. If the `array` is already
+ * sorted, passing `true` for `fromIndex` will run a faster binary search.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} array The array to search.
+ * @param {Mixed} value The value to search for.
+ * @param {Boolean|Number} [fromIndex=0] The index to search from or `true` to
+ * perform a binary search on a sorted `array`.
+ * @returns {Number} Returns the index of the matched value or `-1`.
+ * @example
+ *
+ * _.indexOf([1, 2, 3, 1, 2, 3], 2);
+ * // => 1
+ *
+ * _.indexOf([1, 2, 3, 1, 2, 3], 2, 3);
+ * // => 4
+ *
+ * _.indexOf([1, 1, 2, 2, 3, 3], 2, true);
+ * // => 2
+ */
+ function indexOf(array, value, fromIndex) {
+ var index = -1,
+ length = array ? array.length : 0;
+
+ if (typeof fromIndex == 'number') {
+ index = (fromIndex < 0 ? nativeMax(0, length + fromIndex) : fromIndex || 0) - 1;
+ } else if (fromIndex) {
+ index = sortedIndex(array, value);
+ return array[index] === value ? index : -1;
+ }
+ while (++index < length) {
+ if (array[index] === value) {
+ return index;
+ }
+ }
+ return -1;
+ }
+
+ /**
+ * Gets all but the last element of `array`. If a number `n` is passed, the
+ * last `n` elements are excluded from the result. If a `callback` function
+ * is passed, the last elements the `callback` returns truthy for are excluded
+ * from the result. The `callback` is bound to `thisArg` and invoked with three
+ * arguments; (value, index, array).
+ *
+ * If a property name is passed for `callback`, the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is passed for `callback`, the created "_.where" style callback
+ * will return `true` for elements that have the propeties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} array The array to query.
+ * @param {Function|Object|Number|String} [callback|n=1] The function called
+ * per element or the number of elements to exclude. If a property name or
+ * object is passed, it will be used to create a "_.pluck" or "_.where"
+ * style callback, respectively.
+ * @param {Mixed} [thisArg] The `this` binding of `callback`.
+ * @returns {Array} Returns a slice of `array`.
+ * @example
+ *
+ * _.initial([1, 2, 3]);
+ * // => [1, 2]
+ *
+ * _.initial([1, 2, 3], 2);
+ * // => [1]
+ *
+ * _.initial([1, 2, 3], function(num) {
+ * return num > 1;
+ * });
+ * // => [1]
+ *
+ * var food = [
+ * { 'name': 'beet', 'organic': false },
+ * { 'name': 'carrot', 'organic': true }
+ * ];
+ *
+ * // using "_.pluck" callback shorthand
+ * _.initial(food, 'organic');
+ * // => [{ 'name': 'beet', 'organic': false }]
+ *
+ * var food = [
+ * { 'name': 'banana', 'type': 'fruit' },
+ * { 'name': 'beet', 'type': 'vegetable' },
+ * { 'name': 'carrot', 'type': 'vegetable' }
+ * ];
+ *
+ * // using "_.where" callback shorthand
+ * _.initial(food, { 'type': 'vegetable' });
+ * // => [{ 'name': 'banana', 'type': 'fruit' }]
+ */
+ function initial(array, callback, thisArg) {
+ if (!array) {
+ return [];
+ }
+ var n = 0,
+ length = array.length;
+
+ if (typeof callback != 'number' && callback != null) {
+ var index = length;
+ callback = createCallback(callback, thisArg);
+ while (index-- && callback(array[index], index, array)) {
+ n++;
+ }
+ } else {
+ n = (callback == null || thisArg) ? 1 : callback || n;
+ }
+ return slice(array, 0, nativeMin(nativeMax(0, length - n), length));
+ }
+
+ /**
+ * Computes the intersection of all the passed-in arrays using strict equality
+ * for comparisons, i.e. `===`.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} [array1, array2, ...] Arrays to process.
+ * @returns {Array} Returns a new array of unique elements that are present
+ * in **all** of the arrays.
+ * @example
+ *
+ * _.intersection([1, 2, 3], [101, 2, 1, 10], [2, 1]);
+ * // => [1, 2]
+ */
+ function intersection(array) {
+ var args = arguments,
+ argsLength = args.length,
+ cache = { '0': {} },
+ index = -1,
+ length = array ? array.length : 0,
+ isLarge = length >= 100,
+ result = [],
+ seen = result;
+
+ outer:
+ while (++index < length) {
+ var value = array[index];
+ if (isLarge) {
+ var key = value + '';
+ var inited = hasOwnProperty.call(cache[0], key)
+ ? !(seen = cache[0][key])
+ : (seen = cache[0][key] = []);
+ }
+ if (inited || indexOf(seen, value) < 0) {
+ if (isLarge) {
+ seen.push(value);
+ }
+ var argsIndex = argsLength;
+ while (--argsIndex) {
+ if (!(cache[argsIndex] || (cache[argsIndex] = cachedContains(args[argsIndex], 0, 100)))(value)) {
+ continue outer;
+ }
+ }
+ result.push(value);
+ }
+ }
+ return result;
+ }
+
+ /**
+ * Gets the last element of the `array`. If a number `n` is passed, the last
+ * `n` elements of the `array` are returned. If a `callback` function is passed,
+ * the last elements the `callback` returns truthy for are returned. The `callback`
+ * is bound to `thisArg` and invoked with three arguments; (value, index, array).
+ *
+ *
+ * If a property name is passed for `callback`, the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is passed for `callback`, the created "_.where" style callback
+ * will return `true` for elements that have the propeties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} array The array to query.
+ * @param {Function|Object|Number|String} [callback|n] The function called
+ * per element or the number of elements to return. If a property name or
+ * object is passed, it will be used to create a "_.pluck" or "_.where"
+ * style callback, respectively.
+ * @param {Mixed} [thisArg] The `this` binding of `callback`.
+ * @returns {Mixed} Returns the last element(s) of `array`.
+ * @example
+ *
+ * _.last([1, 2, 3]);
+ * // => 3
+ *
+ * _.last([1, 2, 3], 2);
+ * // => [2, 3]
+ *
+ * _.last([1, 2, 3], function(num) {
+ * return num > 1;
+ * });
+ * // => [2, 3]
+ *
+ * var food = [
+ * { 'name': 'beet', 'organic': false },
+ * { 'name': 'carrot', 'organic': true }
+ * ];
+ *
+ * // using "_.pluck" callback shorthand
+ * _.last(food, 'organic');
+ * // => [{ 'name': 'carrot', 'organic': true }]
+ *
+ * var food = [
+ * { 'name': 'banana', 'type': 'fruit' },
+ * { 'name': 'beet', 'type': 'vegetable' },
+ * { 'name': 'carrot', 'type': 'vegetable' }
+ * ];
+ *
+ * // using "_.where" callback shorthand
+ * _.last(food, { 'type': 'vegetable' });
+ * // => [{ 'name': 'beet', 'type': 'vegetable' }, { 'name': 'carrot', 'type': 'vegetable' }]
+ */
+ function last(array, callback, thisArg) {
+ if (array) {
+ var n = 0,
+ length = array.length;
+
+ if (typeof callback != 'number' && callback != null) {
+ var index = length;
+ callback = createCallback(callback, thisArg);
+ while (index-- && callback(array[index], index, array)) {
+ n++;
+ }
+ } else {
+ n = callback;
+ if (n == null || thisArg) {
+ return array[length - 1];
+ }
+ }
+ return slice(array, nativeMax(0, length - n));
+ }
+ }
+
+ /**
+ * Gets the index at which the last occurrence of `value` is found using strict
+ * equality for comparisons, i.e. `===`. If `fromIndex` is negative, it is used
+ * as the offset from the end of the collection.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} array The array to search.
+ * @param {Mixed} value The value to search for.
+ * @param {Number} [fromIndex=array.length-1] The index to search from.
+ * @returns {Number} Returns the index of the matched value or `-1`.
+ * @example
+ *
+ * _.lastIndexOf([1, 2, 3, 1, 2, 3], 2);
+ * // => 4
+ *
+ * _.lastIndexOf([1, 2, 3, 1, 2, 3], 2, 3);
+ * // => 1
+ */
+ function lastIndexOf(array, value, fromIndex) {
+ var index = array ? array.length : 0;
+ if (typeof fromIndex == 'number') {
+ index = (fromIndex < 0 ? nativeMax(0, index + fromIndex) : nativeMin(fromIndex, index - 1)) + 1;
+ }
+ while (index--) {
+ if (array[index] === value) {
+ return index;
+ }
+ }
+ return -1;
+ }
+
+ /**
+ * Creates an object composed from arrays of `keys` and `values`. Pass either
+ * a single two dimensional array, i.e. `[[key1, value1], [key2, value2]]`, or
+ * two arrays, one of `keys` and one of corresponding `values`.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} keys The array of keys.
+ * @param {Array} [values=[]] The array of values.
+ * @returns {Object} Returns an object composed of the given keys and
+ * corresponding values.
+ * @example
+ *
+ * _.object(['moe', 'larry'], [30, 40]);
+ * // => { 'moe': 30, 'larry': 40 }
+ */
+ function object(keys, values) {
+ var index = -1,
+ length = keys ? keys.length : 0,
+ result = {};
+
+ while (++index < length) {
+ var key = keys[index];
+ if (values) {
+ result[key] = values[index];
+ } else {
+ result[key[0]] = key[1];
+ }
+ }
+ return result;
+ }
+
+ /**
+ * Creates an array of numbers (positive and/or negative) progressing from
+ * `start` up to but not including `end`.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Number} [start=0] The start of the range.
+ * @param {Number} end The end of the range.
+ * @param {Number} [step=1] The value to increment or descrement by.
+ * @returns {Array} Returns a new range array.
+ * @example
+ *
+ * _.range(10);
+ * // => [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
+ *
+ * _.range(1, 11);
+ * // => [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
+ *
+ * _.range(0, 30, 5);
+ * // => [0, 5, 10, 15, 20, 25]
+ *
+ * _.range(0, -10, -1);
+ * // => [0, -1, -2, -3, -4, -5, -6, -7, -8, -9]
+ *
+ * _.range(0);
+ * // => []
+ */
+ function range(start, end, step) {
+ start = +start || 0;
+ step = +step || 1;
+
+ if (end == null) {
+ end = start;
+ start = 0;
+ }
+ // use `Array(length)` so V8 will avoid the slower "dictionary" mode
+ // http://youtu.be/XAqIpGU8ZZk#t=17m25s
+ var index = -1,
+ length = nativeMax(0, ceil((end - start) / step)),
+ result = Array(length);
+
+ while (++index < length) {
+ result[index] = start;
+ start += step;
+ }
+ return result;
+ }
+
+ /**
+ * The opposite of `_.initial`, this method gets all but the first value of `array`.
+ * If a number `n` is passed, the first `n` values are excluded from the result.
+ * If a `callback` function is passed, the first elements the `callback` returns
+ * truthy for are excluded from the result. The `callback` is bound to `thisArg`
+ * and invoked with three arguments; (value, index, array).
+ *
+ * If a property name is passed for `callback`, the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is passed for `callback`, the created "_.where" style callback
+ * will return `true` for elements that have the propeties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @alias drop, tail
+ * @category Arrays
+ * @param {Array} array The array to query.
+ * @param {Function|Object|Number|String} [callback|n=1] The function called
+ * per element or the number of elements to exclude. If a property name or
+ * object is passed, it will be used to create a "_.pluck" or "_.where"
+ * style callback, respectively.
+ * @param {Mixed} [thisArg] The `this` binding of `callback`.
+ * @returns {Array} Returns a slice of `array`.
+ * @example
+ *
+ * _.rest([1, 2, 3]);
+ * // => [2, 3]
+ *
+ * _.rest([1, 2, 3], 2);
+ * // => [3]
+ *
+ * _.rest([1, 2, 3], function(num) {
+ * return num < 3;
+ * });
+ * // => [3]
+ *
+ * var food = [
+ * { 'name': 'banana', 'organic': true },
+ * { 'name': 'beet', 'organic': false },
+ * ];
+ *
+ * // using "_.pluck" callback shorthand
+ * _.rest(food, 'organic');
+ * // => [{ 'name': 'beet', 'organic': false }]
+ *
+ * var food = [
+ * { 'name': 'apple', 'type': 'fruit' },
+ * { 'name': 'banana', 'type': 'fruit' },
+ * { 'name': 'beet', 'type': 'vegetable' }
+ * ];
+ *
+ * // using "_.where" callback shorthand
+ * _.rest(food, { 'type': 'fruit' });
+ * // => [{ 'name': 'beet', 'type': 'vegetable' }]
+ */
+ function rest(array, callback, thisArg) {
+ if (typeof callback != 'number' && callback != null) {
+ var n = 0,
+ index = -1,
+ length = array ? array.length : 0;
+
+ callback = createCallback(callback, thisArg);
+ while (++index < length && callback(array[index], index, array)) {
+ n++;
+ }
+ } else {
+ n = (callback == null || thisArg) ? 1 : nativeMax(0, callback);
+ }
+ return slice(array, n);
+ }
+
+ /**
+ * Uses a binary search to determine the smallest index at which the `value`
+ * should be inserted into `array` in order to maintain the sort order of the
+ * sorted `array`. If `callback` is passed, it will be executed for `value` and
+ * each element in `array` to compute their sort ranking. The `callback` is
+ * bound to `thisArg` and invoked with one argument; (value).
+ *
+ * If a property name is passed for `callback`, the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is passed for `callback`, the created "_.where" style callback
+ * will return `true` for elements that have the propeties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} array The array to iterate over.
+ * @param {Mixed} value The value to evaluate.
+ * @param {Function|Object|String} [callback=identity] The function called per
+ * iteration. If a property name or object is passed, it will be used to create
+ * a "_.pluck" or "_.where" style callback, respectively.
+ * @param {Mixed} [thisArg] The `this` binding of `callback`.
+ * @returns {Number} Returns the index at which the value should be inserted
+ * into `array`.
+ * @example
+ *
+ * _.sortedIndex([20, 30, 50], 40);
+ * // => 2
+ *
+ * // using "_.pluck" callback shorthand
+ * _.sortedIndex([{ 'x': 20 }, { 'x': 30 }, { 'x': 50 }], { 'x': 40 }, 'x');
+ * // => 2
+ *
+ * var dict = {
+ * 'wordToNumber': { 'twenty': 20, 'thirty': 30, 'fourty': 40, 'fifty': 50 }
+ * };
+ *
+ * _.sortedIndex(['twenty', 'thirty', 'fifty'], 'fourty', function(word) {
+ * return dict.wordToNumber[word];
+ * });
+ * // => 2
+ *
+ * _.sortedIndex(['twenty', 'thirty', 'fifty'], 'fourty', function(word) {
+ * return this.wordToNumber[word];
+ * }, dict);
+ * // => 2
+ */
+ function sortedIndex(array, value, callback, thisArg) {
+ var low = 0,
+ high = array ? array.length : low;
+
+ // explicitly reference `identity` for better inlining in Firefox
+ callback = callback ? createCallback(callback, thisArg, 1) : identity;
+ value = callback(value);
+
+ while (low < high) {
+ var mid = (low + high) >>> 1;
+ callback(array[mid]) < value
+ ? low = mid + 1
+ : high = mid;
+ }
+ return low;
+ }
+
+ /**
+ * Computes the union of the passed-in arrays using strict equality for
+ * comparisons, i.e. `===`.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} [array1, array2, ...] Arrays to process.
+ * @returns {Array} Returns a new array of unique values, in order, that are
+ * present in one or more of the arrays.
+ * @example
+ *
+ * _.union([1, 2, 3], [101, 2, 1, 10], [2, 1]);
+ * // => [1, 2, 3, 101, 10]
+ */
+ function union() {
+ return uniq(concat.apply(arrayRef, arguments));
+ }
+
+ /**
+ * Creates a duplicate-value-free version of the `array` using strict equality
+ * for comparisons, i.e. `===`. If the `array` is already sorted, passing `true`
+ * for `isSorted` will run a faster algorithm. If `callback` is passed, each
+ * element of `array` is passed through a callback` before uniqueness is computed.
+ * The `callback` is bound to `thisArg` and invoked with three arguments; (value, index, array).
+ *
+ * If a property name is passed for `callback`, the created "_.pluck" style
+ * callback will return the property value of the given element.
+ *
+ * If an object is passed for `callback`, the created "_.where" style callback
+ * will return `true` for elements that have the propeties of the given object,
+ * else `false`.
+ *
+ * @static
+ * @memberOf _
+ * @alias unique
+ * @category Arrays
+ * @param {Array} array The array to process.
+ * @param {Boolean} [isSorted=false] A flag to indicate that the `array` is already sorted.
+ * @param {Function|Object|String} [callback=identity] The function called per
+ * iteration. If a property name or object is passed, it will be used to create
+ * a "_.pluck" or "_.where" style callback, respectively.
+ * @param {Mixed} [thisArg] The `this` binding of `callback`.
+ * @returns {Array} Returns a duplicate-value-free array.
+ * @example
+ *
+ * _.uniq([1, 2, 1, 3, 1]);
+ * // => [1, 2, 3]
+ *
+ * _.uniq([1, 1, 2, 2, 3], true);
+ * // => [1, 2, 3]
+ *
+ * _.uniq([1, 2, 1.5, 3, 2.5], function(num) { return Math.floor(num); });
+ * // => [1, 2, 3]
+ *
+ * _.uniq([1, 2, 1.5, 3, 2.5], function(num) { return this.floor(num); }, Math);
+ * // => [1, 2, 3]
+ *
+ * // using "_.pluck" callback shorthand
+ * _.uniq([{ 'x': 1 }, { 'x': 2 }, { 'x': 1 }], 'x');
+ * // => [{ 'x': 1 }, { 'x': 2 }]
+ */
+ function uniq(array, isSorted, callback, thisArg) {
+ var index = -1,
+ length = array ? array.length : 0,
+ result = [],
+ seen = result;
+
+ // juggle arguments
+ if (typeof isSorted == 'function') {
+ thisArg = callback;
+ callback = isSorted;
+ isSorted = false;
+ }
+ // init value cache for large arrays
+ var isLarge = !isSorted && length >= 75;
+ if (isLarge) {
+ var cache = {};
+ }
+ if (callback) {
+ seen = [];
+ callback = createCallback(callback, thisArg);
+ }
+ while (++index < length) {
+ var value = array[index],
+ computed = callback ? callback(value, index, array) : value;
+
+ if (isLarge) {
+ var key = computed + '';
+ var inited = hasOwnProperty.call(cache, key)
+ ? !(seen = cache[key])
+ : (seen = cache[key] = []);
+ }
+ if (isSorted
+ ? !index || seen[seen.length - 1] !== computed
+ : inited || indexOf(seen, computed) < 0
+ ) {
+ if (callback || isLarge) {
+ seen.push(computed);
+ }
+ result.push(value);
+ }
+ }
+ return result;
+ }
+
+ /**
+ * Creates an array with all occurrences of the passed values removed using
+ * strict equality for comparisons, i.e. `===`.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} array The array to filter.
+ * @param {Mixed} [value1, value2, ...] Values to remove.
+ * @returns {Array} Returns a new filtered array.
+ * @example
+ *
+ * _.without([1, 2, 1, 0, 3, 1, 4], 0, 1);
+ * // => [2, 3, 4]
+ */
+ function without(array) {
+ var index = -1,
+ length = array ? array.length : 0,
+ contains = cachedContains(arguments, 1),
+ result = [];
+
+ while (++index < length) {
+ var value = array[index];
+ if (!contains(value)) {
+ result.push(value);
+ }
+ }
+ return result;
+ }
+
+ /**
+ * Groups the elements of each array at their corresponding indexes. Useful for
+ * separate data sources that are coordinated through matching array indexes.
+ * For a matrix of nested arrays, `_.zip.apply(...)` can transpose the matrix
+ * in a similar fashion.
+ *
+ * @static
+ * @memberOf _
+ * @category Arrays
+ * @param {Array} [array1, array2, ...] Arrays to process.
+ * @returns {Array} Returns a new array of grouped elements.
+ * @example
+ *
+ * _.zip(['moe', 'larry'], [30, 40], [true, false]);
+ * // => [['moe', 30, true], ['larry', 40, false]]
+ */
+ function zip(array) {
+ var index = -1,
+ length = array ? max(pluck(arguments, 'length')) : 0,
+ result = Array(length);
+
+ while (++index < length) {
+ result[index] = pluck(arguments, index);
+ }
+ return result;
+ }
+
+ /*--------------------------------------------------------------------------*/
+
+ /**
+ * Creates a function that is restricted to executing `func` only after it is
+ * called `n` times. The `func` is executed with the `this` binding of the
+ * created function.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Number} n The number of times the function must be called before
+ * it is executed.
+ * @param {Function} func The function to restrict.
+ * @returns {Function} Returns the new restricted function.
+ * @example
+ *
+ * var renderNotes = _.after(notes.length, render);
+ * _.forEach(notes, function(note) {
+ * note.asyncSave({ 'success': renderNotes });
+ * });
+ * // `renderNotes` is run once, after all notes have saved
+ */
+ function after(n, func) {
+ if (n < 1) {
+ return func();
+ }
+ return function() {
+ if (--n < 1) {
+ return func.apply(this, arguments);
+ }
+ };
+ }
+
+ /**
+ * Creates a function that, when called, invokes `func` with the `this`
+ * binding of `thisArg` and prepends any additional `bind` arguments to those
+ * passed to the bound function.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Function} func The function to bind.
+ * @param {Mixed} [thisArg] The `this` binding of `func`.
+ * @param {Mixed} [arg1, arg2, ...] Arguments to be partially applied.
+ * @returns {Function} Returns the new bound function.
+ * @example
+ *
+ * var func = function(greeting) {
+ * return greeting + ' ' + this.name;
+ * };
+ *
+ * func = _.bind(func, { 'name': 'moe' }, 'hi');
+ * func();
+ * // => 'hi moe'
+ */
+ function bind(func, thisArg) {
+ // use `Function#bind` if it exists and is fast
+ // (in V8 `Function#bind` is slower except when partially applied)
+ return isBindFast || (nativeBind && arguments.length > 2)
+ ? nativeBind.call.apply(nativeBind, arguments)
+ : createBound(func, thisArg, slice(arguments, 2));
+ }
+
+ /**
+ * Binds methods on `object` to `object`, overwriting the existing method.
+ * Method names may be specified as individual arguments or as arrays of method
+ * names. If no method names are provided, all the function properties of `object`
+ * will be bound.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Object} object The object to bind and assign the bound methods to.
+ * @param {String} [methodName1, methodName2, ...] Method names on the object to bind.
+ * @returns {Object} Returns `object`.
+ * @example
+ *
+ * var view = {
+ * 'label': 'docs',
+ * 'onClick': function() { alert('clicked ' + this.label); }
+ * };
+ *
+ * _.bindAll(view);
+ * jQuery('#docs').on('click', view.onClick);
+ * // => alerts 'clicked docs', when the button is clicked
+ */
+ function bindAll(object) {
+ var funcs = concat.apply(arrayRef, arguments),
+ index = funcs.length > 1 ? 0 : (funcs = functions(object), -1),
+ length = funcs.length;
+
+ while (++index < length) {
+ var key = funcs[index];
+ object[key] = bind(object[key], object);
+ }
+ return object;
+ }
+
+ /**
+ * Creates a function that, when called, invokes the method at `object[key]`
+ * and prepends any additional `bindKey` arguments to those passed to the bound
+ * function. This method differs from `_.bind` by allowing bound functions to
+ * reference methods that will be redefined or don't yet exist.
+ * See http://michaux.ca/articles/lazy-function-definition-pattern.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Object} object The object the method belongs to.
+ * @param {String} key The key of the method.
+ * @param {Mixed} [arg1, arg2, ...] Arguments to be partially applied.
+ * @returns {Function} Returns the new bound function.
+ * @example
+ *
+ * var object = {
+ * 'name': 'moe',
+ * 'greet': function(greeting) {
+ * return greeting + ' ' + this.name;
+ * }
+ * };
+ *
+ * var func = _.bindKey(object, 'greet', 'hi');
+ * func();
+ * // => 'hi moe'
+ *
+ * object.greet = function(greeting) {
+ * return greeting + ', ' + this.name + '!';
+ * };
+ *
+ * func();
+ * // => 'hi, moe!'
+ */
+ function bindKey(object, key) {
+ return createBound(object, key, slice(arguments, 2));
+ }
+
+ /**
+ * Creates a function that is the composition of the passed functions,
+ * where each function consumes the return value of the function that follows.
+ * For example, composing the functions `f()`, `g()`, and `h()` produces `f(g(h()))`.
+ * Each function is executed with the `this` binding of the composed function.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Function} [func1, func2, ...] Functions to compose.
+ * @returns {Function} Returns the new composed function.
+ * @example
+ *
+ * var greet = function(name) { return 'hi ' + name; };
+ * var exclaim = function(statement) { return statement + '!'; };
+ * var welcome = _.compose(exclaim, greet);
+ * welcome('moe');
+ * // => 'hi moe!'
+ */
+ function compose() {
+ var funcs = arguments;
+ return function() {
+ var args = arguments,
+ length = funcs.length;
+
+ while (length--) {
+ args = [funcs[length].apply(this, args)];
+ }
+ return args[0];
+ };
+ }
+
+ /**
+ * Creates a function that will delay the execution of `func` until after
+ * `wait` milliseconds have elapsed since the last time it was invoked. Pass
+ * `true` for `immediate` to cause debounce to invoke `func` on the leading,
+ * instead of the trailing, edge of the `wait` timeout. Subsequent calls to
+ * the debounced function will return the result of the last `func` call.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Function} func The function to debounce.
+ * @param {Number} wait The number of milliseconds to delay.
+ * @param {Boolean} immediate A flag to indicate execution is on the leading
+ * edge of the timeout.
+ * @returns {Function} Returns the new debounced function.
+ * @example
+ *
+ * var lazyLayout = _.debounce(calculateLayout, 300);
+ * jQuery(window).on('resize', lazyLayout);
+ */
+ function debounce(func, wait, immediate) {
+ var args,
+ result,
+ thisArg,
+ timeoutId;
+
+ function delayed() {
+ timeoutId = null;
+ if (!immediate) {
+ result = func.apply(thisArg, args);
+ }
+ }
+ return function() {
+ var isImmediate = immediate && !timeoutId;
+ args = arguments;
+ thisArg = this;
+
+ clearTimeout(timeoutId);
+ timeoutId = setTimeout(delayed, wait);
+
+ if (isImmediate) {
+ result = func.apply(thisArg, args);
+ }
+ return result;
+ };
+ }
+
+ /**
+ * Executes the `func` function after `wait` milliseconds. Additional arguments
+ * will be passed to `func` when it is invoked.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Function} func The function to delay.
+ * @param {Number} wait The number of milliseconds to delay execution.
+ * @param {Mixed} [arg1, arg2, ...] Arguments to invoke the function with.
+ * @returns {Number} Returns the `setTimeout` timeout id.
+ * @example
+ *
+ * var log = _.bind(console.log, console);
+ * _.delay(log, 1000, 'logged later');
+ * // => 'logged later' (Appears after one second.)
+ */
+ function delay(func, wait) {
+ var args = slice(arguments, 2);
+ return setTimeout(function() { func.apply(undefined, args); }, wait);
+ }
+
+ /**
+ * Defers executing the `func` function until the current call stack has cleared.
+ * Additional arguments will be passed to `func` when it is invoked.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Function} func The function to defer.
+ * @param {Mixed} [arg1, arg2, ...] Arguments to invoke the function with.
+ * @returns {Number} Returns the `setTimeout` timeout id.
+ * @example
+ *
+ * _.defer(function() { alert('deferred'); });
+ * // returns from the function before `alert` is called
+ */
+ function defer(func) {
+ var args = slice(arguments, 1);
+ return setTimeout(function() { func.apply(undefined, args); }, 1);
+ }
+ // use `setImmediate` if it's available in Node.js
+ if (isV8 && freeModule && typeof setImmediate == 'function') {
+ defer = bind(setImmediate, window);
+ }
+
+ /**
+ * Creates a function that memoizes the result of `func`. If `resolver` is
+ * passed, it will be used to determine the cache key for storing the result
+ * based on the arguments passed to the memoized function. By default, the first
+ * argument passed to the memoized function is used as the cache key. The `func`
+ * is executed with the `this` binding of the memoized function.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Function} func The function to have its output memoized.
+ * @param {Function} [resolver] A function used to resolve the cache key.
+ * @returns {Function} Returns the new memoizing function.
+ * @example
+ *
+ * var fibonacci = _.memoize(function(n) {
+ * return n < 2 ? n : fibonacci(n - 1) + fibonacci(n - 2);
+ * });
+ */
+ function memoize(func, resolver) {
+ var cache = {};
+ return function() {
+ var key = (resolver ? resolver.apply(this, arguments) : arguments[0]) + '';
+ return hasOwnProperty.call(cache, key)
+ ? cache[key]
+ : (cache[key] = func.apply(this, arguments));
+ };
+ }
+
+ /**
+ * Creates a function that is restricted to execute `func` once. Repeat calls to
+ * the function will return the value of the first call. The `func` is executed
+ * with the `this` binding of the created function.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Function} func The function to restrict.
+ * @returns {Function} Returns the new restricted function.
+ * @example
+ *
+ * var initialize = _.once(createApplication);
+ * initialize();
+ * initialize();
+ * // `initialize` executes `createApplication` once
+ */
+ function once(func) {
+ var ran,
+ result;
+
+ return function() {
+ if (ran) {
+ return result;
+ }
+ ran = true;
+ result = func.apply(this, arguments);
+
+ // clear the `func` variable so the function may be garbage collected
+ func = null;
+ return result;
+ };
+ }
+
+ /**
+ * Creates a function that, when called, invokes `func` with any additional
+ * `partial` arguments prepended to those passed to the new function. This
+ * method is similar to `_.bind`, except it does **not** alter the `this` binding.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Function} func The function to partially apply arguments to.
+ * @param {Mixed} [arg1, arg2, ...] Arguments to be partially applied.
+ * @returns {Function} Returns the new partially applied function.
+ * @example
+ *
+ * var greet = function(greeting, name) { return greeting + ' ' + name; };
+ * var hi = _.partial(greet, 'hi');
+ * hi('moe');
+ * // => 'hi moe'
+ */
+ function partial(func) {
+ return createBound(func, slice(arguments, 1));
+ }
+
+ /**
+ * This method is similar to `_.partial`, except that `partial` arguments are
+ * appended to those passed to the new function.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Function} func The function to partially apply arguments to.
+ * @param {Mixed} [arg1, arg2, ...] Arguments to be partially applied.
+ * @returns {Function} Returns the new partially applied function.
+ * @example
+ *
+ * var defaultsDeep = _.partialRight(_.merge, _.defaults);
+ *
+ * var options = {
+ * 'variable': 'data',
+ * 'imports': { 'jq': $ }
+ * };
+ *
+ * defaultsDeep(options, _.templateSettings);
+ *
+ * options.variable
+ * // => 'data'
+ *
+ * options.imports
+ * // => { '_': _, 'jq': $ }
+ */
+ function partialRight(func) {
+ return createBound(func, slice(arguments, 1), null, indicatorObject);
+ }
+
+ /**
+ * Creates a function that, when executed, will only call the `func`
+ * function at most once per every `wait` milliseconds. If the throttled
+ * function is invoked more than once during the `wait` timeout, `func` will
+ * also be called on the trailing edge of the timeout. Subsequent calls to the
+ * throttled function will return the result of the last `func` call.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Function} func The function to throttle.
+ * @param {Number} wait The number of milliseconds to throttle executions to.
+ * @returns {Function} Returns the new throttled function.
+ * @example
+ *
+ * var throttled = _.throttle(updatePosition, 100);
+ * jQuery(window).on('scroll', throttled);
+ */
+ function throttle(func, wait) {
+ var args,
+ result,
+ thisArg,
+ timeoutId,
+ lastCalled = 0;
+
+ function trailingCall() {
+ lastCalled = new Date;
+ timeoutId = null;
+ result = func.apply(thisArg, args);
+ }
+ return function() {
+ var now = new Date,
+ remaining = wait - (now - lastCalled);
+
+ args = arguments;
+ thisArg = this;
+
+ if (remaining <= 0) {
+ clearTimeout(timeoutId);
+ timeoutId = null;
+ lastCalled = now;
+ result = func.apply(thisArg, args);
+ }
+ else if (!timeoutId) {
+ timeoutId = setTimeout(trailingCall, remaining);
+ }
+ return result;
+ };
+ }
+
+ /**
+ * Creates a function that passes `value` to the `wrapper` function as its
+ * first argument. Additional arguments passed to the function are appended
+ * to those passed to the `wrapper` function. The `wrapper` is executed with
+ * the `this` binding of the created function.
+ *
+ * @static
+ * @memberOf _
+ * @category Functions
+ * @param {Mixed} value The value to wrap.
+ * @param {Function} wrapper The wrapper function.
+ * @returns {Function} Returns the new function.
+ * @example
+ *
+ * var hello = function(name) { return 'hello ' + name; };
+ * hello = _.wrap(hello, function(func) {
+ * return 'before, ' + func('moe') + ', after';
+ * });
+ * hello();
+ * // => 'before, hello moe, after'
+ */
+ function wrap(value, wrapper) {
+ return function() {
+ var args = [value];
+ push.apply(args, arguments);
+ return wrapper.apply(this, args);
+ };
+ }
+
+ /*--------------------------------------------------------------------------*/
+
+ /**
+ * Converts the characters `&`, `<`, `>`, `"`, and `'` in `string` to their
+ * corresponding HTML entities.
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @param {String} string The string to escape.
+ * @returns {String} Returns the escaped string.
+ * @example
+ *
+ * _.escape('Moe, Larry & Curly');
+ * // => 'Moe, Larry & Curly'
+ */
+ function escape(string) {
+ return string == null ? '' : (string + '').replace(reUnescapedHtml, escapeHtmlChar);
+ }
+
+ /**
+ * This function returns the first argument passed to it.
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @param {Mixed} value Any value.
+ * @returns {Mixed} Returns `value`.
+ * @example
+ *
+ * var moe = { 'name': 'moe' };
+ * moe === _.identity(moe);
+ * // => true
+ */
+ function identity(value) {
+ return value;
+ }
+
+ /**
+ * Adds functions properties of `object` to the `lodash` function and chainable
+ * wrapper.
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @param {Object} object The object of function properties to add to `lodash`.
+ * @example
+ *
+ * _.mixin({
+ * 'capitalize': function(string) {
+ * return string.charAt(0).toUpperCase() + string.slice(1).toLowerCase();
+ * }
+ * });
+ *
+ * _.capitalize('moe');
+ * // => 'Moe'
+ *
+ * _('moe').capitalize();
+ * // => 'Moe'
+ */
+ function mixin(object) {
+ forEach(functions(object), function(methodName) {
+ var func = lodash[methodName] = object[methodName];
+
+ lodash.prototype[methodName] = function() {
+ var args = [this.__wrapped__];
+ push.apply(args, arguments);
+ return new lodash(func.apply(lodash, args));
+ };
+ });
+ }
+
+ /**
+ * Reverts the '_' variable to its previous value and returns a reference to
+ * the `lodash` function.
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @returns {Function} Returns the `lodash` function.
+ * @example
+ *
+ * var lodash = _.noConflict();
+ */
+ function noConflict() {
+ window._ = oldDash;
+ return this;
+ }
+
+ /**
+ * Produces a random number between `min` and `max` (inclusive). If only one
+ * argument is passed, a number between `0` and the given number will be returned.
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @param {Number} [min=0] The minimum possible value.
+ * @param {Number} [max=1] The maximum possible value.
+ * @returns {Number} Returns a random number.
+ * @example
+ *
+ * _.random(0, 5);
+ * // => a number between 0 and 5
+ *
+ * _.random(5);
+ * // => also a number between 0 and 5
+ */
+ function random(min, max) {
+ if (min == null && max == null) {
+ max = 1;
+ }
+ min = +min || 0;
+ if (max == null) {
+ max = min;
+ min = 0;
+ }
+ return min + floor(nativeRandom() * ((+max || 0) - min + 1));
+ }
+
+ /**
+ * Resolves the value of `property` on `object`. If `property` is a function,
+ * it will be invoked and its result returned, else the property value is
+ * returned. If `object` is falsey, then `null` is returned.
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @param {Object} object The object to inspect.
+ * @param {String} property The property to get the value of.
+ * @returns {Mixed} Returns the resolved value.
+ * @example
+ *
+ * var object = {
+ * 'cheese': 'crumpets',
+ * 'stuff': function() {
+ * return 'nonsense';
+ * }
+ * };
+ *
+ * _.result(object, 'cheese');
+ * // => 'crumpets'
+ *
+ * _.result(object, 'stuff');
+ * // => 'nonsense'
+ */
+ function result(object, property) {
+ var value = object ? object[property] : undefined;
+ return isFunction(value) ? object[property]() : value;
+ }
+
+ /**
+ * A micro-templating method that handles arbitrary delimiters, preserves
+ * whitespace, and correctly escapes quotes within interpolated code.
+ *
+ * Note: In the development build, `_.template` utilizes sourceURLs for easier
+ * debugging. See http://www.html5rocks.com/en/tutorials/developertools/sourcemaps/#toc-sourceurl
+ *
+ * Note: Lo-Dash may be used in Chrome extensions by either creating a `lodash csp`
+ * build and using precompiled templates, or loading Lo-Dash in a sandbox.
+ *
+ * For more information on precompiling templates see:
+ * http://lodash.com/#custom-builds
+ *
+ * For more information on Chrome extension sandboxes see:
+ * http://developer.chrome.com/stable/extensions/sandboxingEval.html
+ *
+ * @static
+ * @memberOf _
+ * @category Utilities
+ * @param {String} text The template text.
+ * @param {Obect} data The data object used to populate the text.
+ * @param {Object} options The options object.
+ * escape - The "escape" delimiter regexp.
+ * evaluate - The "evaluate" delimiter regexp.
+ * interpolate - The "interpolate" delimiter regexp.
+ * sourceURL - The sourceURL of the template's compiled source.
+ * variable - The data object variable name.
+ *
+ * @returns {Function|String} Returns a compiled function when no `data` object
+ * is given, else it returns the interpolated text.
+ * @example
+ *
+ * // using a compiled template
+ * var compiled = _.template('hello <%= name %>');
+ * compiled({ 'name': 'moe' });
+ * // => 'hello moe'
+ *
+ * var list = '<% _.forEach(people, function(name) { %><%= name %> <% }); %>';
+ * _.template(list, { 'people': ['moe', 'larry'] });
+ * // => 'moe larry '
+ *
+ * // using the "escape" delimiter to escape HTML in data property values
+ * _.template('<%- value %> ', { 'value': '
+```
+
+## Usage
+
+### Basic named error
+
+```javascript
+var CustomError = makeError('CustomError')
+
+// Parameters are forwarded to the super class (here Error).
+throw new CustomError('a message')
+```
+
+### Advanced error class
+
+```javascript
+function CustomError (customValue) {
+ CustomError.super.call(this, 'custom error message')
+
+ this.customValue = customValue
+}
+makeError(CustomError)
+
+// Feel free to extend the prototype.
+CustomError.prototype.myMethod = function CustomError$myMethod () {
+ console.log('CustomError.myMethod (%s, %s)', this.code, this.message)
+}
+
+//-----
+
+try {
+ throw new CustomError(42)
+} catch (error) {
+ error.myMethod()
+}
+```
+
+### Specialized error
+
+```javascript
+var SpecializedError = makeError('SpecializedError', CustomError);
+
+throw new SpecializedError(42);
+```
+
+### Inheritance
+
+> Best for ES2015+.
+
+```javascript
+import {BaseError} from 'make-error'
+
+class CustomError extends BaseError {
+ constructor () {
+ super('custom error message')
+ }
+}
+```
+
+## Related
+
+- [make-error-cause](https://www.npmjs.com/package/make-error-cause): Make your own error types, with a cause!
+
+## Contributions
+
+Contributions are *very* welcomed, either on the documentation or on
+the code.
+
+You may:
+
+- report any [issue](https://github.com/JsCommunity/make-error/issues)
+ you've encountered;
+- fork and create a pull request.
+
+## License
+
+ISC © [Julien Fontanet](http://julien.isonoe.net)
diff --git a/node_modules/make-error/dist/make-error.js b/node_modules/make-error/dist/make-error.js
new file mode 100644
index 0000000..fe0b770
--- /dev/null
+++ b/node_modules/make-error/dist/make-error.js
@@ -0,0 +1 @@
+!function(f){if("object"==typeof exports&&"undefined"!=typeof module)module.exports=f();else if("function"==typeof define&&define.amd)define([],f);else{("undefined"!=typeof window?window:"undefined"!=typeof global?global:"undefined"!=typeof self?self:this).makeError=f()}}(function(){return function e(t,n,r){function s(o,u){if(!n[o]){if(!t[o]){var a="function"==typeof require&&require;if(!u&&a)return a(o,!0);if(i)return i(o,!0);var f=new Error("Cannot find module '"+o+"'");throw f.code="MODULE_NOT_FOUND",f}var l=n[o]={exports:{}};t[o][0].call(l.exports,function(e){var n=t[o][1][e];return s(n||e)},l,l.exports,e,t,n,r)}return n[o].exports}for(var i="function"==typeof require&&require,o=0;o;
+
+/**
+ * Set the constructor prototype to `BaseError`.
+ */
+declare function makeError(super_: { new (...args: any[]): T }): makeError.Constructor;
+
+/**
+ * Create a specialized error instance.
+ */
+declare function makeError(name: string | Function, super_: { new (...args: any[]): T }): makeError.Constructor;
+
+declare module makeError {
+ /**
+ * Use with ES2015+ inheritance.
+ */
+ export class BaseError implements Error {
+ message: string;
+ name: string;
+ stack: string;
+
+ constructor(message?: string);
+ }
+
+ export interface Constructor {
+ new (message?: string): T
+ super_: any
+ prototype: T
+ }
+}
+
+export = makeError;
diff --git a/node_modules/make-error/index.js b/node_modules/make-error/index.js
new file mode 100644
index 0000000..315ab8e
--- /dev/null
+++ b/node_modules/make-error/index.js
@@ -0,0 +1,142 @@
+// ISC @ Julien Fontanet
+
+'use strict'
+
+// ===================================================================
+
+var defineProperty = Object.defineProperty
+
+// -------------------------------------------------------------------
+
+var captureStackTrace = Error.captureStackTrace
+if (!captureStackTrace) {
+ captureStackTrace = function captureStackTrace (error) {
+ var container = new Error()
+
+ defineProperty(error, 'stack', {
+ configurable: true,
+ get: function getStack () {
+ var stack = container.stack
+
+ // Replace property with value for faster future accesses.
+ defineProperty(this, 'stack', {
+ value: stack
+ })
+
+ return stack
+ },
+ set: function setStack (stack) {
+ defineProperty(error, 'stack', {
+ configurable: true,
+ value: stack,
+ writable: true
+ })
+ }
+ })
+ }
+}
+
+// -------------------------------------------------------------------
+
+function BaseError (message) {
+ if (message) {
+ defineProperty(this, 'message', {
+ configurable: true,
+ value: message,
+ writable: true
+ })
+ }
+
+ var cname = this.constructor.name
+ if (
+ cname &&
+ cname !== this.name
+ ) {
+ defineProperty(this, 'name', {
+ configurable: true,
+ value: cname,
+ writable: true
+ })
+ }
+
+ captureStackTrace(this, this.constructor)
+}
+
+BaseError.prototype = Object.create(Error.prototype, {
+ // See: https://github.com/JsCommunity/make-error/issues/4
+ constructor: {
+ configurable: true,
+ value: BaseError,
+ writable: true
+ }
+})
+
+// -------------------------------------------------------------------
+
+// Sets the name of a function if possible (depends of the JS engine).
+var setFunctionName = (function () {
+ function setFunctionName (fn, name) {
+ return defineProperty(fn, 'name', {
+ configurable: true,
+ value: name
+ })
+ }
+ try {
+ var f = function () {}
+ setFunctionName(f, 'foo')
+ if (f.name === 'foo') {
+ return setFunctionName
+ }
+ } catch (_) {}
+})()
+
+// -------------------------------------------------------------------
+
+function makeError (constructor, super_) {
+ if (super_ == null || super_ === Error) {
+ super_ = BaseError
+ } else if (typeof super_ !== 'function') {
+ throw new TypeError('super_ should be a function')
+ }
+
+ var name
+ if (typeof constructor === 'string') {
+ name = constructor
+ constructor = function () { super_.apply(this, arguments) }
+
+ // If the name can be set, do it once and for all.
+ if (setFunctionName) {
+ setFunctionName(constructor, name)
+ name = null
+ }
+ } else if (typeof constructor !== 'function') {
+ throw new TypeError('constructor should be either a string or a function')
+ }
+
+ // Also register the super constructor also as `constructor.super_` just
+ // like Node's `util.inherits()`.
+ constructor.super_ = constructor['super'] = super_
+
+ var properties = {
+ constructor: {
+ configurable: true,
+ value: constructor,
+ writable: true
+ }
+ }
+
+ // If the name could not be set on the constructor, set it on the
+ // prototype.
+ if (name != null) {
+ properties.name = {
+ configurable: true,
+ value: name,
+ writable: true
+ }
+ }
+ constructor.prototype = Object.create(super_.prototype, properties)
+
+ return constructor
+}
+exports = module.exports = makeError
+exports.BaseError = BaseError
diff --git a/node_modules/make-error/package.json b/node_modules/make-error/package.json
new file mode 100644
index 0000000..b6724aa
--- /dev/null
+++ b/node_modules/make-error/package.json
@@ -0,0 +1,117 @@
+{
+ "_args": [
+ [
+ {
+ "raw": "make-error@^1.2.0",
+ "scope": null,
+ "escapedName": "make-error",
+ "name": "make-error",
+ "rawSpec": "^1.2.0",
+ "spec": ">=1.2.0 <2.0.0",
+ "type": "range"
+ },
+ "/Users/ramswaroop/MyProjects/RamAndAntara/node_modules/make-error-cause"
+ ]
+ ],
+ "_from": "make-error@>=1.2.0 <2.0.0",
+ "_id": "make-error@1.3.0",
+ "_inCache": true,
+ "_location": "/make-error",
+ "_nodeVersion": "7.10.0",
+ "_npmOperationalInternal": {
+ "host": "s3://npm-registry-packages",
+ "tmp": "tmp/make-error-1.3.0.tgz_1495404802218_0.3306263096164912"
+ },
+ "_npmUser": {
+ "name": "julien-f",
+ "email": "julien.fontanet@isonoe.net"
+ },
+ "_npmVersion": "4.5.0",
+ "_phantomChildren": {},
+ "_requested": {
+ "raw": "make-error@^1.2.0",
+ "scope": null,
+ "escapedName": "make-error",
+ "name": "make-error",
+ "rawSpec": "^1.2.0",
+ "spec": ">=1.2.0 <2.0.0",
+ "type": "range"
+ },
+ "_requiredBy": [
+ "/make-error-cause"
+ ],
+ "_resolved": "https://registry.npmjs.org/make-error/-/make-error-1.3.0.tgz",
+ "_shasum": "52ad3a339ccf10ce62b4040b708fe707244b8b96",
+ "_shrinkwrap": null,
+ "_spec": "make-error@^1.2.0",
+ "_where": "/Users/ramswaroop/MyProjects/RamAndAntara/node_modules/make-error-cause",
+ "author": {
+ "name": "Julien Fontanet",
+ "email": "julien.fontanet@isonoe.net"
+ },
+ "bugs": {
+ "url": "https://github.com/JsCommunity/make-error/issues"
+ },
+ "dependencies": {},
+ "description": "Make your own error types!",
+ "devDependencies": {
+ "browserify": "^14.3.0",
+ "husky": "^0.13.3",
+ "jest": "^20.0.3",
+ "standard": "^10.0.2",
+ "uglify-js": "^3.0.10"
+ },
+ "directories": {},
+ "dist": {
+ "shasum": "52ad3a339ccf10ce62b4040b708fe707244b8b96",
+ "tarball": "https://registry.npmjs.org/make-error/-/make-error-1.3.0.tgz"
+ },
+ "files": [
+ "dist/",
+ "index.js",
+ "index.d.ts"
+ ],
+ "gitHead": "adac41f9d1b3bd465f845b0c9cf87f1c88d1d7f7",
+ "homepage": "https://github.com/JsCommunity/make-error",
+ "keywords": [
+ "create",
+ "custom",
+ "derive",
+ "error",
+ "errors",
+ "extend",
+ "extending",
+ "extension",
+ "factory",
+ "inherit",
+ "make",
+ "subclass"
+ ],
+ "license": "ISC",
+ "main": "index.js",
+ "maintainers": [
+ {
+ "name": "julien-f",
+ "email": "julien.fontanet@isonoe.net"
+ },
+ {
+ "name": "marsaud",
+ "email": "marsaud.fabrice@neuf.fr"
+ }
+ ],
+ "name": "make-error",
+ "optionalDependencies": {},
+ "readme": "ERROR: No README data found!",
+ "repository": {
+ "type": "git",
+ "url": "git://github.com/JsCommunity/make-error.git"
+ },
+ "scripts": {
+ "commitmsg": "yarn test",
+ "dev-test": "jest --watch",
+ "prepublish": "mkdir -p dist && browserify -s makeError index.js | uglifyjs -c > dist/make-error.js",
+ "pretest": "standard",
+ "test": "jest"
+ },
+ "version": "1.3.0"
+}
diff --git a/node_modules/make-iterator/LICENSE b/node_modules/make-iterator/LICENSE
new file mode 100644
index 0000000..d32ab44
--- /dev/null
+++ b/node_modules/make-iterator/LICENSE
@@ -0,0 +1,21 @@
+The MIT License (MIT)
+
+Copyright (c) 2014-2018, Jon Schlinkert.
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in
+all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
+THE SOFTWARE.
diff --git a/node_modules/make-iterator/README.md b/node_modules/make-iterator/README.md
new file mode 100644
index 0000000..6eb2a5d
--- /dev/null
+++ b/node_modules/make-iterator/README.md
@@ -0,0 +1,102 @@
+# make-iterator [](https://www.npmjs.com/package/make-iterator) [](https://npmjs.org/package/make-iterator) [](https://npmjs.org/package/make-iterator) [](https://travis-ci.org/jonschlinkert/make-iterator)
+
+> Convert an argument into a valid iterator. Based on the `.makeIterator()` implementation in mout https://github.com/mout/mout.
+
+Please consider following this project's author, [Jon Schlinkert](https://github.com/jonschlinkert), and consider starring the project to show your :heart: and support.
+
+## Install
+
+Install with [npm](https://www.npmjs.com/):
+
+```sh
+$ npm install --save make-iterator
+```
+
+Copyright (c) 2012, 2013 moutjs team and contributors (http://moutjs.com)
+
+## Usage
+
+```js
+var iterator = require('make-iterator');
+```
+
+**Regex**
+
+```js
+var arr = ['a', 'b', 'c', 'd', 'e', 'f'];
+var fn = iterator(/[a-c]/);
+console.log(arr.filter(fn));
+//=> ['a', 'b', 'c'];
+```
+
+**Objects**
+
+```js
+var fn = iterator({ a: 1, b: { c: 2 } });
+
+console.log(fn({ a: 1, b: { c: 2, d: 3 } }));
+//=> true
+console.log(fn({ a: 1, b: { c: 3 } }));
+//=> false
+```
+
+## About
+
+
+Contributing
+
+Pull requests and stars are always welcome. For bugs and feature requests, [please create an issue](../../issues/new).
+
+
+
+
+Running Tests
+
+Running and reviewing unit tests is a great way to get familiarized with a library and its API. You can install dependencies and run tests with the following command:
+
+```sh
+$ npm install && npm test
+```
+
+
+
+
+Building docs
+
+_(This project's readme.md is generated by [verb](https://github.com/verbose/verb-generate-readme), please don't edit the readme directly. Any changes to the readme must be made in the [.verb.md](.verb.md) readme template.)_
+
+To generate the readme, run the following command:
+
+```sh
+$ npm install -g verbose/verb#dev verb-generate-readme && verb
+```
+
+
+
+### Related projects
+
+You might also be interested in these projects:
+
+* [any](https://www.npmjs.com/package/any): Returns `true` if a value exists in the given string, array or object. | [homepage](https://github.com/jonschlinkert/any "Returns `true` if a value exists in the given string, array or object.")
+* [arr-filter](https://www.npmjs.com/package/arr-filter): Faster alternative to javascript's native filter method. | [homepage](https://github.com/jonschlinkert/arr-filter "Faster alternative to javascript's native filter method.")
+* [arr-map](https://www.npmjs.com/package/arr-map): Faster, node.js focused alternative to JavaScript's native array map. | [homepage](https://github.com/jonschlinkert/arr-map "Faster, node.js focused alternative to JavaScript's native array map.")
+* [array-every](https://www.npmjs.com/package/array-every): Returns true if the callback returns truthy for all elements in the given array. | [homepage](https://github.com/jonschlinkert/array-every "Returns true if the callback returns truthy for all elements in the given array.")
+* [collection-map](https://www.npmjs.com/package/collection-map): Returns an array of mapped values from an array or object. | [homepage](https://github.com/jonschlinkert/collection-map "Returns an array of mapped values from an array or object.")
+* [utils](https://www.npmjs.com/package/utils): Fast, generic JavaScript/node.js utility functions. | [homepage](https://github.com/jonschlinkert/utils "Fast, generic JavaScript/node.js utility functions.")
+
+### Author
+
+**Jon Schlinkert**
+
+* [LinkedIn Profile](https://linkedin.com/in/jonschlinkert)
+* [GitHub Profile](https://github.com/jonschlinkert)
+* [Twitter Profile](https://twitter.com/jonschlinkert)
+
+### License
+
+Copyright © 2018, [Jon Schlinkert](https://github.com/jonschlinkert).
+Released under the [MIT License](LICENSE).
+
+***
+
+_This file was generated by [verb-generate-readme](https://github.com/verbose/verb-generate-readme), v0.6.0, on April 08, 2018._
\ No newline at end of file
diff --git a/node_modules/make-iterator/index.js b/node_modules/make-iterator/index.js
new file mode 100644
index 0000000..15a00d0
--- /dev/null
+++ b/node_modules/make-iterator/index.js
@@ -0,0 +1,99 @@
+/*!
+ * make-iterator
+ *
+ * Copyright (c) 2014-2018, Jon Schlinkert.
+ * Released under the MIT License.
+ */
+
+'use strict';
+
+var typeOf = require('kind-of');
+
+module.exports = function makeIterator(target, thisArg) {
+ switch (typeOf(target)) {
+ case 'undefined':
+ case 'null':
+ return noop;
+ case 'function':
+ // function is the first to improve perf (most common case)
+ // also avoid using `Function#call` if not needed, which boosts
+ // perf a lot in some cases
+ return (typeof thisArg !== 'undefined') ? function(val, i, arr) {
+ return target.call(thisArg, val, i, arr);
+ } : target;
+ case 'object':
+ return function(val) {
+ return deepMatches(val, target);
+ };
+ case 'regexp':
+ return function(str) {
+ return target.test(str);
+ };
+ case 'string':
+ case 'number':
+ default: {
+ return prop(target);
+ }
+ }
+};
+
+function containsMatch(array, value) {
+ var len = array.length;
+ var i = -1;
+
+ while (++i < len) {
+ if (deepMatches(array[i], value)) {
+ return true;
+ }
+ }
+ return false;
+}
+
+function matchArray(arr, value) {
+ var len = value.length;
+ var i = -1;
+
+ while (++i < len) {
+ if (!containsMatch(arr, value[i])) {
+ return false;
+ }
+ }
+ return true;
+}
+
+function matchObject(obj, value) {
+ for (var key in value) {
+ if (value.hasOwnProperty(key)) {
+ if (deepMatches(obj[key], value[key]) === false) {
+ return false;
+ }
+ }
+ }
+ return true;
+}
+
+/**
+ * Recursively compare objects
+ */
+
+function deepMatches(val, value) {
+ if (typeOf(val) === 'object') {
+ if (Array.isArray(val) && Array.isArray(value)) {
+ return matchArray(val, value);
+ } else {
+ return matchObject(val, value);
+ }
+ } else {
+ return val === value;
+ }
+}
+
+function prop(name) {
+ return function(obj) {
+ return obj[name];
+ };
+}
+
+function noop(val) {
+ return val;
+}
diff --git a/node_modules/make-iterator/package.json b/node_modules/make-iterator/package.json
new file mode 100644
index 0000000..bedb448
--- /dev/null
+++ b/node_modules/make-iterator/package.json
@@ -0,0 +1,99 @@
+{
+ "_from": "make-iterator@^1.0.0",
+ "_id": "make-iterator@1.0.1",
+ "_inBundle": false,
+ "_integrity": "sha512-pxiuXh0iVEq7VM7KMIhs5gxsfxCux2URptUQaXo4iZZJxBAzTPOLE2BumO5dbfVYq/hBJFBR/a1mFDmOx5AGmw==",
+ "_location": "/make-iterator",
+ "_phantomChildren": {},
+ "_requested": {
+ "type": "range",
+ "registry": true,
+ "raw": "make-iterator@^1.0.0",
+ "name": "make-iterator",
+ "escapedName": "make-iterator",
+ "rawSpec": "^1.0.0",
+ "saveSpec": null,
+ "fetchSpec": "^1.0.0"
+ },
+ "_requiredBy": [
+ "/object.map"
+ ],
+ "_resolved": "https://registry.npmjs.org/make-iterator/-/make-iterator-1.0.1.tgz",
+ "_shasum": "29b33f312aa8f547c4a5e490f56afcec99133ad6",
+ "_spec": "make-iterator@^1.0.0",
+ "_where": "/Users/rpatra16/MyProjects/RamAndAntara/node_modules/object.map",
+ "author": {
+ "name": "Jon Schlinkert",
+ "url": "https://github.com/jonschlinkert"
+ },
+ "bugs": {
+ "url": "https://github.com/jonschlinkert/make-iterator/issues"
+ },
+ "bundleDependencies": false,
+ "dependencies": {
+ "kind-of": "^6.0.2"
+ },
+ "deprecated": false,
+ "description": "Convert an argument into a valid iterator. Based on the `.makeIterator()` implementation in mout https://github.com/mout/mout.",
+ "devDependencies": {
+ "gulp-format-md": "^1.0.0",
+ "mocha": "^3.5.3"
+ },
+ "engines": {
+ "node": ">=0.10.0"
+ },
+ "files": [
+ "index.js"
+ ],
+ "homepage": "https://github.com/jonschlinkert/make-iterator",
+ "keywords": [
+ "arr",
+ "array",
+ "contains",
+ "for-own",
+ "forown",
+ "forOwn",
+ "function",
+ "iterate",
+ "iterator",
+ "make"
+ ],
+ "license": "MIT",
+ "main": "index.js",
+ "name": "make-iterator",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/jonschlinkert/make-iterator.git"
+ },
+ "scripts": {
+ "test": "mocha"
+ },
+ "verb": {
+ "related": {
+ "list": [
+ "any",
+ "arr-filter",
+ "arr-map",
+ "array-every",
+ "collection-map",
+ "utils"
+ ]
+ },
+ "toc": false,
+ "layout": "default",
+ "tasks": [
+ "readme"
+ ],
+ "plugins": [
+ "gulp-format-md"
+ ],
+ "lint": {
+ "reflinks": true
+ },
+ "reflinks": [
+ "verb",
+ "verb-readme-generator"
+ ]
+ },
+ "version": "1.0.1"
+}
diff --git a/node_modules/map-cache/LICENSE b/node_modules/map-cache/LICENSE
new file mode 100644
index 0000000..1e49edf
--- /dev/null
+++ b/node_modules/map-cache/LICENSE
@@ -0,0 +1,21 @@
+The MIT License (MIT)
+
+Copyright (c) 2015-2016, Jon Schlinkert.
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in
+all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
+THE SOFTWARE.
diff --git a/node_modules/map-cache/README.md b/node_modules/map-cache/README.md
new file mode 100644
index 0000000..6260b29
--- /dev/null
+++ b/node_modules/map-cache/README.md
@@ -0,0 +1,145 @@
+# map-cache [](https://www.npmjs.com/package/map-cache) [](https://npmjs.org/package/map-cache) [](https://travis-ci.org/jonschlinkert/map-cache)
+
+Basic cache object for storing key-value pairs.
+
+## Install
+
+Install with [npm](https://www.npmjs.com/):
+
+```sh
+$ npm install map-cache --save
+```
+
+Based on MapCache in Lo-dash v3.0. [MIT License](https://github.com/lodash/lodash/blob/master/LICENSE.txt)
+
+## Usage
+
+```js
+var MapCache = require('map-cache');
+var mapCache = new MapCache();
+```
+
+## API
+
+### [MapCache](index.js#L28)
+
+Creates a cache object to store key/value pairs.
+
+**Example**
+
+```js
+var cache = new MapCache();
+```
+
+### [.set](index.js#L45)
+
+Adds `value` to `key` on the cache.
+
+**Params**
+
+* `key` **{String}**: The key of the value to cache.
+* `value` **{any}**: The value to cache.
+* `returns` **{Object}**: Returns the `Cache` object for chaining.
+
+**Example**
+
+```js
+cache.set('foo', 'bar');
+```
+
+### [.get](index.js#L65)
+
+Gets the cached value for `key`.
+
+**Params**
+
+* `key` **{String}**: The key of the value to get.
+* `returns` **{any}**: Returns the cached value.
+
+**Example**
+
+```js
+cache.get('foo');
+//=> 'bar'
+```
+
+### [.has](index.js#L82)
+
+Checks if a cached value for `key` exists.
+
+**Params**
+
+* `key` **{String}**: The key of the entry to check.
+* `returns` **{Boolean}**: Returns `true` if an entry for `key` exists, else `false`.
+
+**Example**
+
+```js
+cache.has('foo');
+//=> true
+```
+
+### [.del](index.js#L98)
+
+Removes `key` and its value from the cache.
+
+**Params**
+
+* `key` **{String}**: The key of the value to remove.
+* `returns` **{Boolean}**: Returns `true` if the entry was removed successfully, else `false`.
+
+**Example**
+
+```js
+cache.del('foo');
+```
+
+## Related projects
+
+You might also be interested in these projects:
+
+* [cache-base](https://www.npmjs.com/package/cache-base): Basic object cache with `get`, `set`, `del`, and `has` methods for node.js/javascript projects. | [homepage](https://github.com/jonschlinkert/cache-base)
+* [config-cache](https://www.npmjs.com/package/config-cache): General purpose JavaScript object storage methods. | [homepage](https://github.com/jonschlinkert/config-cache)
+* [option-cache](https://www.npmjs.com/package/option-cache): Simple API for managing options in JavaScript applications. | [homepage](https://github.com/jonschlinkert/option-cache)
+
+## Contributing
+
+Pull requests and stars are always welcome. For bugs and feature requests, [please create an issue](https://github.com/jonschlinkert/map-cache/issues/new).
+
+## Building docs
+
+Generate readme and API documentation with [verb](https://github.com/verbose/verb):
+
+```sh
+$ npm install verb && npm run docs
+```
+
+Or, if [verb](https://github.com/verbose/verb) is installed globally:
+
+```sh
+$ verb
+```
+
+## Running tests
+
+Install dev dependencies:
+
+```sh
+$ npm install -d && npm test
+```
+
+## Author
+
+**Jon Schlinkert**
+
+* [github/jonschlinkert](https://github.com/jonschlinkert)
+* [twitter/jonschlinkert](http://twitter.com/jonschlinkert)
+
+## License
+
+Copyright © 2016, [Jon Schlinkert](https://github.com/jonschlinkert).
+Released under the [MIT license](https://github.com/jonschlinkert/map-cache/blob/master/LICENSE).
+
+***
+
+_This file was generated by [verb](https://github.com/verbose/verb), v0.9.0, on May 10, 2016._
\ No newline at end of file
diff --git a/node_modules/map-cache/index.js b/node_modules/map-cache/index.js
new file mode 100644
index 0000000..f86842f
--- /dev/null
+++ b/node_modules/map-cache/index.js
@@ -0,0 +1,100 @@
+/*!
+ * map-cache
+ *
+ * Copyright (c) 2015, Jon Schlinkert.
+ * Licensed under the MIT License.
+ */
+
+'use strict';
+
+var hasOwn = Object.prototype.hasOwnProperty;
+
+/**
+ * Expose `MapCache`
+ */
+
+module.exports = MapCache;
+
+/**
+ * Creates a cache object to store key/value pairs.
+ *
+ * ```js
+ * var cache = new MapCache();
+ * ```
+ *
+ * @api public
+ */
+
+function MapCache(data) {
+ this.__data__ = data || {};
+}
+
+/**
+ * Adds `value` to `key` on the cache.
+ *
+ * ```js
+ * cache.set('foo', 'bar');
+ * ```
+ *
+ * @param {String} `key` The key of the value to cache.
+ * @param {*} `value` The value to cache.
+ * @returns {Object} Returns the `Cache` object for chaining.
+ * @api public
+ */
+
+MapCache.prototype.set = function mapSet(key, value) {
+ if (key !== '__proto__') {
+ this.__data__[key] = value;
+ }
+ return this;
+};
+
+/**
+ * Gets the cached value for `key`.
+ *
+ * ```js
+ * cache.get('foo');
+ * //=> 'bar'
+ * ```
+ *
+ * @param {String} `key` The key of the value to get.
+ * @returns {*} Returns the cached value.
+ * @api public
+ */
+
+MapCache.prototype.get = function mapGet(key) {
+ return key === '__proto__' ? undefined : this.__data__[key];
+};
+
+/**
+ * Checks if a cached value for `key` exists.
+ *
+ * ```js
+ * cache.has('foo');
+ * //=> true
+ * ```
+ *
+ * @param {String} `key` The key of the entry to check.
+ * @returns {Boolean} Returns `true` if an entry for `key` exists, else `false`.
+ * @api public
+ */
+
+MapCache.prototype.has = function mapHas(key) {
+ return key !== '__proto__' && hasOwn.call(this.__data__, key);
+};
+
+/**
+ * Removes `key` and its value from the cache.
+ *
+ * ```js
+ * cache.del('foo');
+ * ```
+ * @title .del
+ * @param {String} `key` The key of the value to remove.
+ * @returns {Boolean} Returns `true` if the entry was removed successfully, else `false`.
+ * @api public
+ */
+
+MapCache.prototype.del = function mapDelete(key) {
+ return this.has(key) && delete this.__data__[key];
+};
diff --git a/node_modules/map-cache/package.json b/node_modules/map-cache/package.json
new file mode 100644
index 0000000..b507ec3
--- /dev/null
+++ b/node_modules/map-cache/package.json
@@ -0,0 +1,92 @@
+{
+ "_from": "map-cache@^0.2.2",
+ "_id": "map-cache@0.2.2",
+ "_inBundle": false,
+ "_integrity": "sha1-wyq9C9ZSXZsFFkW7TyasXcmKDb8=",
+ "_location": "/map-cache",
+ "_phantomChildren": {},
+ "_requested": {
+ "type": "range",
+ "registry": true,
+ "raw": "map-cache@^0.2.2",
+ "name": "map-cache",
+ "escapedName": "map-cache",
+ "rawSpec": "^0.2.2",
+ "saveSpec": null,
+ "fetchSpec": "^0.2.2"
+ },
+ "_requiredBy": [
+ "/fragment-cache",
+ "/parse-filepath",
+ "/snapdragon"
+ ],
+ "_resolved": "https://registry.npmjs.org/map-cache/-/map-cache-0.2.2.tgz",
+ "_shasum": "c32abd0bd6525d9b051645bb4f26ac5dc98a0dbf",
+ "_spec": "map-cache@^0.2.2",
+ "_where": "/Users/rpatra16/MyProjects/RamAndAntara/node_modules/snapdragon",
+ "author": {
+ "name": "Jon Schlinkert",
+ "url": "https://github.com/jonschlinkert"
+ },
+ "bugs": {
+ "url": "https://github.com/jonschlinkert/map-cache/issues"
+ },
+ "bundleDependencies": false,
+ "deprecated": false,
+ "description": "Basic cache object for storing key-value pairs.",
+ "devDependencies": {
+ "gulp-format-md": "^0.1.9",
+ "should": "^8.3.1"
+ },
+ "engines": {
+ "node": ">=0.10.0"
+ },
+ "files": [
+ "index.js"
+ ],
+ "homepage": "https://github.com/jonschlinkert/map-cache",
+ "keywords": [
+ "cache",
+ "get",
+ "has",
+ "object",
+ "set",
+ "storage",
+ "store"
+ ],
+ "license": "MIT",
+ "main": "index.js",
+ "name": "map-cache",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/jonschlinkert/map-cache.git"
+ },
+ "scripts": {
+ "test": "mocha"
+ },
+ "verb": {
+ "run": true,
+ "toc": false,
+ "layout": "default",
+ "tasks": [
+ "readme"
+ ],
+ "plugins": [
+ "gulp-format-md"
+ ],
+ "related": {
+ "list": [
+ "config-cache",
+ "option-cache",
+ "cache-base"
+ ]
+ },
+ "reflinks": [
+ "verb"
+ ],
+ "lint": {
+ "reflinks": true
+ }
+ },
+ "version": "0.2.2"
+}
diff --git a/node_modules/map-obj/index.js b/node_modules/map-obj/index.js
new file mode 100644
index 0000000..8b7b4d6
--- /dev/null
+++ b/node_modules/map-obj/index.js
@@ -0,0 +1,13 @@
+'use strict';
+module.exports = function (obj, cb) {
+ var ret = {};
+ var keys = Object.keys(obj);
+
+ for (var i = 0; i < keys.length; i++) {
+ var key = keys[i];
+ var res = cb(key, obj[key], obj);
+ ret[res[0]] = res[1];
+ }
+
+ return ret;
+};
diff --git a/node_modules/map-obj/license b/node_modules/map-obj/license
new file mode 100644
index 0000000..654d0bf
--- /dev/null
+++ b/node_modules/map-obj/license
@@ -0,0 +1,21 @@
+The MIT License (MIT)
+
+Copyright (c) Sindre Sorhus (sindresorhus.com)
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in
+all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
+THE SOFTWARE.
diff --git a/node_modules/map-obj/package.json b/node_modules/map-obj/package.json
new file mode 100644
index 0000000..b913c12
--- /dev/null
+++ b/node_modules/map-obj/package.json
@@ -0,0 +1,101 @@
+{
+ "_args": [
+ [
+ {
+ "raw": "map-obj@^1.0.1",
+ "scope": null,
+ "escapedName": "map-obj",
+ "name": "map-obj",
+ "rawSpec": "^1.0.1",
+ "spec": ">=1.0.1 <2.0.0",
+ "type": "range"
+ },
+ "/Users/ramswaroop/MyProjects/RamAndAntara/node_modules/meow"
+ ]
+ ],
+ "_from": "map-obj@>=1.0.1 <2.0.0",
+ "_id": "map-obj@1.0.1",
+ "_inCache": true,
+ "_location": "/map-obj",
+ "_nodeVersion": "0.12.2",
+ "_npmUser": {
+ "name": "sindresorhus",
+ "email": "sindresorhus@gmail.com"
+ },
+ "_npmVersion": "2.7.4",
+ "_phantomChildren": {},
+ "_requested": {
+ "raw": "map-obj@^1.0.1",
+ "scope": null,
+ "escapedName": "map-obj",
+ "name": "map-obj",
+ "rawSpec": "^1.0.1",
+ "spec": ">=1.0.1 <2.0.0",
+ "type": "range"
+ },
+ "_requiredBy": [
+ "/camelcase-keys",
+ "/meow"
+ ],
+ "_resolved": "https://registry.npmjs.org/map-obj/-/map-obj-1.0.1.tgz",
+ "_shasum": "d933ceb9205d82bdcf4886f6742bdc2b4dea146d",
+ "_shrinkwrap": null,
+ "_spec": "map-obj@^1.0.1",
+ "_where": "/Users/ramswaroop/MyProjects/RamAndAntara/node_modules/meow",
+ "author": {
+ "name": "Sindre Sorhus",
+ "email": "sindresorhus@gmail.com",
+ "url": "sindresorhus.com"
+ },
+ "bugs": {
+ "url": "https://github.com/sindresorhus/map-obj/issues"
+ },
+ "dependencies": {},
+ "description": "Map object keys and values into a new object",
+ "devDependencies": {
+ "ava": "0.0.4"
+ },
+ "directories": {},
+ "dist": {
+ "shasum": "d933ceb9205d82bdcf4886f6742bdc2b4dea146d",
+ "tarball": "https://registry.npmjs.org/map-obj/-/map-obj-1.0.1.tgz"
+ },
+ "engines": {
+ "node": ">=0.10.0"
+ },
+ "files": [
+ "index.js"
+ ],
+ "gitHead": "a4f2d49ae6b5f7c0e55130b49ab0412298b797bc",
+ "homepage": "https://github.com/sindresorhus/map-obj",
+ "keywords": [
+ "map",
+ "obj",
+ "object",
+ "key",
+ "keys",
+ "value",
+ "values",
+ "val",
+ "iterate",
+ "iterator"
+ ],
+ "license": "MIT",
+ "maintainers": [
+ {
+ "name": "sindresorhus",
+ "email": "sindresorhus@gmail.com"
+ }
+ ],
+ "name": "map-obj",
+ "optionalDependencies": {},
+ "readme": "ERROR: No README data found!",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/sindresorhus/map-obj.git"
+ },
+ "scripts": {
+ "test": "node test.js"
+ },
+ "version": "1.0.1"
+}
diff --git a/node_modules/map-obj/readme.md b/node_modules/map-obj/readme.md
new file mode 100644
index 0000000..fee03d9
--- /dev/null
+++ b/node_modules/map-obj/readme.md
@@ -0,0 +1,29 @@
+# map-obj [](https://travis-ci.org/sindresorhus/map-obj)
+
+> Map object keys and values into a new object
+
+
+## Install
+
+```
+$ npm install --save map-obj
+```
+
+
+## Usage
+
+```js
+var mapObj = require('map-obj');
+
+var newObject = mapObj({foo: 'bar'}, function (key, value, object) {
+ // first element is the new key and second is the new value
+ // here we reverse the order
+ return [value, key];
+});
+//=> {bar: 'foo'}
+```
+
+
+## License
+
+MIT © [Sindre Sorhus](http://sindresorhus.com)
diff --git a/node_modules/map-visit/LICENSE b/node_modules/map-visit/LICENSE
new file mode 100644
index 0000000..83b56e7
--- /dev/null
+++ b/node_modules/map-visit/LICENSE
@@ -0,0 +1,21 @@
+The MIT License (MIT)
+
+Copyright (c) 2015-2017, Jon Schlinkert
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in
+all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
+THE SOFTWARE.
diff --git a/node_modules/map-visit/README.md b/node_modules/map-visit/README.md
new file mode 100644
index 0000000..5ab02d4
--- /dev/null
+++ b/node_modules/map-visit/README.md
@@ -0,0 +1,155 @@
+# map-visit [](https://www.npmjs.com/package/map-visit) [](https://npmjs.org/package/map-visit) [](https://npmjs.org/package/map-visit) [](https://travis-ci.org/jonschlinkert/map-visit)
+
+> Map `visit` over an array of objects.
+
+## Install
+
+Install with [npm](https://www.npmjs.com/):
+
+```sh
+$ npm install --save map-visit
+```
+
+## Usage
+
+```js
+var mapVisit = require('map-visit');
+```
+
+## What does this do?
+
+**Assign/Merge/Extend vs. Visit**
+
+Let's say you want to add a `set` method to your application that will:
+
+* set key-value pairs on a `data` object
+* extend objects onto the `data` object
+* extend arrays of objects onto the data object
+
+**Example using `extend`**
+
+Here is one way to accomplish this using Lo-Dash's `extend` (comparable to `Object.assign`):
+
+```js
+var _ = require('lodash');
+
+var obj = {
+ data: {},
+ set: function (key, value) {
+ if (Array.isArray(key)) {
+ _.extend.apply(_, [obj.data].concat(key));
+ } else if (typeof key === 'object') {
+ _.extend(obj.data, key);
+ } else {
+ obj.data[key] = value;
+ }
+ }
+};
+
+obj.set('a', 'a');
+obj.set([{b: 'b'}, {c: 'c'}]);
+obj.set({d: {e: 'f'}});
+
+console.log(obj.data);
+//=> {a: 'a', b: 'b', c: 'c', d: { e: 'f' }}
+```
+
+The above approach works fine for most use cases. However, **if you also want to emit an event** each time a property is added to the `data` object, or you want more control over what happens as the object is extended, a better approach would be to use `visit`.
+
+**Example using `visit`**
+
+In this approach:
+
+* when an array is passed to `set`, the `mapVisit` library calls the `set` method on each object in the array.
+* when an object is passed, `visit` calls `set` on each property in the object.
+
+As a result, the `data` event will be emitted every time a property is added to `data` (events are just an example, you can use this approach to perform any necessary logic every time the method is called).
+
+```js
+var mapVisit = require('map-visit');
+var visit = require('object-visit');
+
+var obj = {
+ data: {},
+ set: function (key, value) {
+ if (Array.isArray(key)) {
+ mapVisit(obj, 'set', key);
+ } else if (typeof key === 'object') {
+ visit(obj, 'set', key);
+ } else {
+ // simulate an event-emitter
+ console.log('emit', key, value);
+ obj.data[key] = value;
+ }
+ }
+};
+
+obj.set('a', 'a');
+obj.set([{b: 'b'}, {c: 'c'}]);
+obj.set({d: {e: 'f'}});
+obj.set({g: 'h', i: 'j', k: 'l'});
+
+console.log(obj.data);
+//=> {a: 'a', b: 'b', c: 'c', d: { e: 'f' }, g: 'h', i: 'j', k: 'l'}
+
+// events would look something like:
+// emit a a
+// emit b b
+// emit c c
+// emit d { e: 'f' }
+// emit g h
+// emit i j
+// emit k l
+```
+
+## About
+
+### Related projects
+
+* [collection-visit](https://www.npmjs.com/package/collection-visit): Visit a method over the items in an object, or map visit over the objects… [more](https://github.com/jonschlinkert/collection-visit) | [homepage](https://github.com/jonschlinkert/collection-visit "Visit a method over the items in an object, or map visit over the objects in an array.")
+* [object-visit](https://www.npmjs.com/package/object-visit): Call a specified method on each value in the given object. | [homepage](https://github.com/jonschlinkert/object-visit "Call a specified method on each value in the given object.")
+
+### Contributing
+
+Pull requests and stars are always welcome. For bugs and feature requests, [please create an issue](../../issues/new).
+
+### Contributors
+
+| **Commits** | **Contributor** |
+| --- | --- |
+| 15 | [jonschlinkert](https://github.com/jonschlinkert) |
+| 7 | [doowb](https://github.com/doowb) |
+
+### Building docs
+
+_(This project's readme.md is generated by [verb](https://github.com/verbose/verb-generate-readme), please don't edit the readme directly. Any changes to the readme must be made in the [.verb.md](.verb.md) readme template.)_
+
+To generate the readme, run the following command:
+
+```sh
+$ npm install -g verbose/verb#dev verb-generate-readme && verb
+```
+
+### Running tests
+
+Running and reviewing unit tests is a great way to get familiarized with a library and its API. You can install dependencies and run tests with the following command:
+
+```sh
+$ npm install && npm test
+```
+
+### Author
+
+**Jon Schlinkert**
+
+* [github/jonschlinkert](https://github.com/jonschlinkert)
+* [twitter/jonschlinkert](https://twitter.com/jonschlinkert)
+
+### License
+
+Copyright © 2017, [Jon Schlinkert](https://github.com/jonschlinkert).
+Released under the [MIT License](LICENSE).
+
+***
+
+_This file was generated by [verb-generate-readme](https://github.com/verbose/verb-generate-readme), v0.5.0, on April 09, 2017._
\ No newline at end of file
diff --git a/node_modules/map-visit/index.js b/node_modules/map-visit/index.js
new file mode 100644
index 0000000..bc54ccc
--- /dev/null
+++ b/node_modules/map-visit/index.js
@@ -0,0 +1,37 @@
+'use strict';
+
+var util = require('util');
+var visit = require('object-visit');
+
+/**
+ * Map `visit` over an array of objects.
+ *
+ * @param {Object} `collection` The context in which to invoke `method`
+ * @param {String} `method` Name of the method to call on `collection`
+ * @param {Object} `arr` Array of objects.
+ */
+
+module.exports = function mapVisit(collection, method, val) {
+ if (isObject(val)) {
+ return visit.apply(null, arguments);
+ }
+
+ if (!Array.isArray(val)) {
+ throw new TypeError('expected an array: ' + util.inspect(val));
+ }
+
+ var args = [].slice.call(arguments, 3);
+
+ for (var i = 0; i < val.length; i++) {
+ var ele = val[i];
+ if (isObject(ele)) {
+ visit.apply(null, [collection, method, ele].concat(args));
+ } else {
+ collection[method].apply(collection, [ele].concat(args));
+ }
+ }
+};
+
+function isObject(val) {
+ return val && (typeof val === 'function' || (!Array.isArray(val) && typeof val === 'object'));
+}
diff --git a/node_modules/map-visit/package.json b/node_modules/map-visit/package.json
new file mode 100644
index 0000000..0bf0adb
--- /dev/null
+++ b/node_modules/map-visit/package.json
@@ -0,0 +1,113 @@
+{
+ "_from": "map-visit@^1.0.0",
+ "_id": "map-visit@1.0.0",
+ "_inBundle": false,
+ "_integrity": "sha1-7Nyo8TFE5mDxtb1B8S80edmN+48=",
+ "_location": "/map-visit",
+ "_phantomChildren": {},
+ "_requested": {
+ "type": "range",
+ "registry": true,
+ "raw": "map-visit@^1.0.0",
+ "name": "map-visit",
+ "escapedName": "map-visit",
+ "rawSpec": "^1.0.0",
+ "saveSpec": null,
+ "fetchSpec": "^1.0.0"
+ },
+ "_requiredBy": [
+ "/collection-visit"
+ ],
+ "_resolved": "https://registry.npmjs.org/map-visit/-/map-visit-1.0.0.tgz",
+ "_shasum": "ecdca8f13144e660f1b5bd41f12f3479d98dfb8f",
+ "_spec": "map-visit@^1.0.0",
+ "_where": "/Users/rpatra16/MyProjects/RamAndAntara/node_modules/collection-visit",
+ "author": {
+ "name": "Jon Schlinkert",
+ "url": "https://github.com/jonschlinkert"
+ },
+ "bugs": {
+ "url": "https://github.com/jonschlinkert/map-visit/issues"
+ },
+ "bundleDependencies": false,
+ "contributors": [
+ {
+ "name": "Brian Woodward",
+ "email": "brian.woodward@gmail.com",
+ "url": "https://twitter.com/doowb"
+ },
+ {
+ "name": "Jon Schlinkert",
+ "email": "jon.schlinkert@sellside.com",
+ "url": "http://twitter.com/jonschlinkert"
+ }
+ ],
+ "dependencies": {
+ "object-visit": "^1.0.0"
+ },
+ "deprecated": false,
+ "description": "Map `visit` over an array of objects.",
+ "devDependencies": {
+ "clone-deep": "^0.2.4",
+ "extend-shallow": "^2.0.1",
+ "gulp-format-md": "^0.1.12",
+ "lodash": "^4.17.4",
+ "mocha": "^3.2.0"
+ },
+ "engines": {
+ "node": ">=0.10.0"
+ },
+ "files": [
+ "index.js"
+ ],
+ "homepage": "https://github.com/jonschlinkert/map-visit",
+ "keywords": [
+ "array",
+ "arrays",
+ "function",
+ "helper",
+ "invoke",
+ "key",
+ "map",
+ "method",
+ "object",
+ "objects",
+ "value",
+ "visit",
+ "visitor"
+ ],
+ "license": "MIT",
+ "main": "index.js",
+ "name": "map-visit",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/jonschlinkert/map-visit.git"
+ },
+ "scripts": {
+ "test": "mocha"
+ },
+ "verb": {
+ "toc": false,
+ "layout": "default",
+ "tasks": [
+ "readme"
+ ],
+ "plugins": [
+ "gulp-format-md"
+ ],
+ "lint": {
+ "reflinks": true
+ },
+ "related": {
+ "list": [
+ "collection-visit",
+ "object-visit"
+ ]
+ },
+ "reflinks": [
+ "verb",
+ "verb-generate-readme"
+ ]
+ },
+ "version": "1.0.0"
+}
diff --git a/node_modules/meow/index.js b/node_modules/meow/index.js
new file mode 100644
index 0000000..d7ab879
--- /dev/null
+++ b/node_modules/meow/index.js
@@ -0,0 +1,82 @@
+'use strict';
+var path = require('path');
+var minimist = require('minimist');
+var objectAssign = require('object-assign');
+var camelcaseKeys = require('camelcase-keys');
+var decamelize = require('decamelize');
+var mapObj = require('map-obj');
+var trimNewlines = require('trim-newlines');
+var redent = require('redent');
+var readPkgUp = require('read-pkg-up');
+var loudRejection = require('loud-rejection');
+var normalizePackageData = require('normalize-package-data');
+
+// get the uncached parent
+delete require.cache[__filename];
+var parentDir = path.dirname(module.parent.filename);
+
+module.exports = function (opts, minimistOpts) {
+ loudRejection();
+
+ if (Array.isArray(opts) || typeof opts === 'string') {
+ opts = {help: opts};
+ }
+
+ opts = objectAssign({
+ pkg: readPkgUp.sync({
+ cwd: parentDir,
+ normalize: false
+ }).pkg,
+ argv: process.argv.slice(2)
+ }, opts);
+
+ minimistOpts = objectAssign({}, minimistOpts);
+
+ minimistOpts.default = mapObj(minimistOpts.default || {}, function (key, value) {
+ return [decamelize(key, '-'), value];
+ });
+
+ if (Array.isArray(opts.help)) {
+ opts.help = opts.help.join('\n');
+ }
+
+ var pkg = typeof opts.pkg === 'string' ? require(path.join(parentDir, opts.pkg)) : opts.pkg;
+ var argv = minimist(opts.argv, minimistOpts);
+ var help = redent(trimNewlines(opts.help || ''), 2);
+
+ normalizePackageData(pkg);
+
+ process.title = pkg.bin ? Object.keys(pkg.bin)[0] : pkg.name;
+
+ var description = opts.description;
+ if (!description && description !== false) {
+ description = pkg.description;
+ }
+
+ help = (description ? '\n ' + description + '\n' : '') + (help ? '\n' + help : '\n');
+
+ var showHelp = function (code) {
+ console.log(help);
+ process.exit(code || 0);
+ };
+
+ if (argv.version && opts.version !== false) {
+ console.log(typeof opts.version === 'string' ? opts.version : pkg.version);
+ process.exit();
+ }
+
+ if (argv.help && opts.help !== false) {
+ showHelp();
+ }
+
+ var _ = argv._;
+ delete argv._;
+
+ return {
+ input: _,
+ flags: camelcaseKeys(argv),
+ pkg: pkg,
+ help: help,
+ showHelp: showHelp
+ };
+};
diff --git a/node_modules/meow/license b/node_modules/meow/license
new file mode 100644
index 0000000..654d0bf
--- /dev/null
+++ b/node_modules/meow/license
@@ -0,0 +1,21 @@
+The MIT License (MIT)
+
+Copyright (c) Sindre Sorhus (sindresorhus.com)
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in
+all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
+THE SOFTWARE.
diff --git a/node_modules/meow/node_modules/object-assign/index.js b/node_modules/meow/node_modules/object-assign/index.js
new file mode 100644
index 0000000..0930cf8
--- /dev/null
+++ b/node_modules/meow/node_modules/object-assign/index.js
@@ -0,0 +1,90 @@
+/*
+object-assign
+(c) Sindre Sorhus
+@license MIT
+*/
+
+'use strict';
+/* eslint-disable no-unused-vars */
+var getOwnPropertySymbols = Object.getOwnPropertySymbols;
+var hasOwnProperty = Object.prototype.hasOwnProperty;
+var propIsEnumerable = Object.prototype.propertyIsEnumerable;
+
+function toObject(val) {
+ if (val === null || val === undefined) {
+ throw new TypeError('Object.assign cannot be called with null or undefined');
+ }
+
+ return Object(val);
+}
+
+function shouldUseNative() {
+ try {
+ if (!Object.assign) {
+ return false;
+ }
+
+ // Detect buggy property enumeration order in older V8 versions.
+
+ // https://bugs.chromium.org/p/v8/issues/detail?id=4118
+ var test1 = new String('abc'); // eslint-disable-line no-new-wrappers
+ test1[5] = 'de';
+ if (Object.getOwnPropertyNames(test1)[0] === '5') {
+ return false;
+ }
+
+ // https://bugs.chromium.org/p/v8/issues/detail?id=3056
+ var test2 = {};
+ for (var i = 0; i < 10; i++) {
+ test2['_' + String.fromCharCode(i)] = i;
+ }
+ var order2 = Object.getOwnPropertyNames(test2).map(function (n) {
+ return test2[n];
+ });
+ if (order2.join('') !== '0123456789') {
+ return false;
+ }
+
+ // https://bugs.chromium.org/p/v8/issues/detail?id=3056
+ var test3 = {};
+ 'abcdefghijklmnopqrst'.split('').forEach(function (letter) {
+ test3[letter] = letter;
+ });
+ if (Object.keys(Object.assign({}, test3)).join('') !==
+ 'abcdefghijklmnopqrst') {
+ return false;
+ }
+
+ return true;
+ } catch (err) {
+ // We don't expect any of the above to throw, but better to be safe.
+ return false;
+ }
+}
+
+module.exports = shouldUseNative() ? Object.assign : function (target, source) {
+ var from;
+ var to = toObject(target);
+ var symbols;
+
+ for (var s = 1; s < arguments.length; s++) {
+ from = Object(arguments[s]);
+
+ for (var key in from) {
+ if (hasOwnProperty.call(from, key)) {
+ to[key] = from[key];
+ }
+ }
+
+ if (getOwnPropertySymbols) {
+ symbols = getOwnPropertySymbols(from);
+ for (var i = 0; i < symbols.length; i++) {
+ if (propIsEnumerable.call(from, symbols[i])) {
+ to[symbols[i]] = from[symbols[i]];
+ }
+ }
+ }
+ }
+
+ return to;
+};
diff --git a/node_modules/meow/node_modules/object-assign/license b/node_modules/meow/node_modules/object-assign/license
new file mode 100644
index 0000000..654d0bf
--- /dev/null
+++ b/node_modules/meow/node_modules/object-assign/license
@@ -0,0 +1,21 @@
+The MIT License (MIT)
+
+Copyright (c) Sindre Sorhus (sindresorhus.com)
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in
+all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
+THE SOFTWARE.
diff --git a/node_modules/meow/node_modules/object-assign/package.json b/node_modules/meow/node_modules/object-assign/package.json
new file mode 100644
index 0000000..ef405e0
--- /dev/null
+++ b/node_modules/meow/node_modules/object-assign/package.json
@@ -0,0 +1,118 @@
+{
+ "_args": [
+ [
+ {
+ "raw": "object-assign@^4.0.1",
+ "scope": null,
+ "escapedName": "object-assign",
+ "name": "object-assign",
+ "rawSpec": "^4.0.1",
+ "spec": ">=4.0.1 <5.0.0",
+ "type": "range"
+ },
+ "/Users/ramswaroop/MyProjects/RamAndAntara/node_modules/meow"
+ ]
+ ],
+ "_from": "object-assign@>=4.0.1 <5.0.0",
+ "_id": "object-assign@4.1.1",
+ "_inCache": true,
+ "_location": "/meow/object-assign",
+ "_nodeVersion": "4.6.2",
+ "_npmOperationalInternal": {
+ "host": "packages-12-west.internal.npmjs.com",
+ "tmp": "tmp/object-assign-4.1.1.tgz_1484580915042_0.07107710791751742"
+ },
+ "_npmUser": {
+ "name": "sindresorhus",
+ "email": "sindresorhus@gmail.com"
+ },
+ "_npmVersion": "2.15.11",
+ "_phantomChildren": {},
+ "_requested": {
+ "raw": "object-assign@^4.0.1",
+ "scope": null,
+ "escapedName": "object-assign",
+ "name": "object-assign",
+ "rawSpec": "^4.0.1",
+ "spec": ">=4.0.1 <5.0.0",
+ "type": "range"
+ },
+ "_requiredBy": [
+ "/meow"
+ ],
+ "_resolved": "https://registry.npmjs.org/object-assign/-/object-assign-4.1.1.tgz",
+ "_shasum": "2109adc7965887cfc05cbbd442cac8bfbb360863",
+ "_shrinkwrap": null,
+ "_spec": "object-assign@^4.0.1",
+ "_where": "/Users/ramswaroop/MyProjects/RamAndAntara/node_modules/meow",
+ "author": {
+ "name": "Sindre Sorhus",
+ "email": "sindresorhus@gmail.com",
+ "url": "sindresorhus.com"
+ },
+ "bugs": {
+ "url": "https://github.com/sindresorhus/object-assign/issues"
+ },
+ "dependencies": {},
+ "description": "ES2015 `Object.assign()` ponyfill",
+ "devDependencies": {
+ "ava": "^0.16.0",
+ "lodash": "^4.16.4",
+ "matcha": "^0.7.0",
+ "xo": "^0.16.0"
+ },
+ "directories": {},
+ "dist": {
+ "shasum": "2109adc7965887cfc05cbbd442cac8bfbb360863",
+ "tarball": "https://registry.npmjs.org/object-assign/-/object-assign-4.1.1.tgz"
+ },
+ "engines": {
+ "node": ">=0.10.0"
+ },
+ "files": [
+ "index.js"
+ ],
+ "gitHead": "a89774b252c91612203876984bbd6addbe3b5a0e",
+ "homepage": "https://github.com/sindresorhus/object-assign#readme",
+ "keywords": [
+ "object",
+ "assign",
+ "extend",
+ "properties",
+ "es2015",
+ "ecmascript",
+ "harmony",
+ "ponyfill",
+ "prollyfill",
+ "polyfill",
+ "shim",
+ "browser"
+ ],
+ "license": "MIT",
+ "maintainers": [
+ {
+ "name": "gaearon",
+ "email": "dan.abramov@gmail.com"
+ },
+ {
+ "name": "sindresorhus",
+ "email": "sindresorhus@gmail.com"
+ },
+ {
+ "name": "spicyj",
+ "email": "ben@benalpert.com"
+ }
+ ],
+ "name": "object-assign",
+ "optionalDependencies": {},
+ "readme": "ERROR: No README data found!",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/sindresorhus/object-assign.git"
+ },
+ "scripts": {
+ "bench": "matcha bench.js",
+ "test": "xo && ava"
+ },
+ "version": "4.1.1"
+}
diff --git a/node_modules/meow/node_modules/object-assign/readme.md b/node_modules/meow/node_modules/object-assign/readme.md
new file mode 100644
index 0000000..1be09d3
--- /dev/null
+++ b/node_modules/meow/node_modules/object-assign/readme.md
@@ -0,0 +1,61 @@
+# object-assign [](https://travis-ci.org/sindresorhus/object-assign)
+
+> ES2015 [`Object.assign()`](http://www.2ality.com/2014/01/object-assign.html) [ponyfill](https://ponyfill.com)
+
+
+## Use the built-in
+
+Node.js 4 and up, as well as every evergreen browser (Chrome, Edge, Firefox, Opera, Safari),
+support `Object.assign()` :tada:. If you target only those environments, then by all
+means, use `Object.assign()` instead of this package.
+
+
+## Install
+
+```
+$ npm install --save object-assign
+```
+
+
+## Usage
+
+```js
+const objectAssign = require('object-assign');
+
+objectAssign({foo: 0}, {bar: 1});
+//=> {foo: 0, bar: 1}
+
+// multiple sources
+objectAssign({foo: 0}, {bar: 1}, {baz: 2});
+//=> {foo: 0, bar: 1, baz: 2}
+
+// overwrites equal keys
+objectAssign({foo: 0}, {foo: 1}, {foo: 2});
+//=> {foo: 2}
+
+// ignores null and undefined sources
+objectAssign({foo: 0}, null, {bar: 1}, undefined);
+//=> {foo: 0, bar: 1}
+```
+
+
+## API
+
+### objectAssign(target, [source, ...])
+
+Assigns enumerable own properties of `source` objects to the `target` object and returns the `target` object. Additional `source` objects will overwrite previous ones.
+
+
+## Resources
+
+- [ES2015 spec - Object.assign](https://people.mozilla.org/~jorendorff/es6-draft.html#sec-object.assign)
+
+
+## Related
+
+- [deep-assign](https://github.com/sindresorhus/deep-assign) - Recursive `Object.assign()`
+
+
+## License
+
+MIT © [Sindre Sorhus](https://sindresorhus.com)
diff --git a/node_modules/meow/package.json b/node_modules/meow/package.json
new file mode 100644
index 0000000..1b88c7e
--- /dev/null
+++ b/node_modules/meow/package.json
@@ -0,0 +1,121 @@
+{
+ "_args": [
+ [
+ {
+ "raw": "meow@^3.7.0",
+ "scope": null,
+ "escapedName": "meow",
+ "name": "meow",
+ "rawSpec": "^3.7.0",
+ "spec": ">=3.7.0 <4.0.0",
+ "type": "range"
+ },
+ "/Users/ramswaroop/MyProjects/RamAndAntara/node_modules/node-sass"
+ ]
+ ],
+ "_from": "meow@>=3.7.0 <4.0.0",
+ "_id": "meow@3.7.0",
+ "_inCache": true,
+ "_location": "/meow",
+ "_nodeVersion": "4.2.4",
+ "_npmUser": {
+ "name": "sindresorhus",
+ "email": "sindresorhus@gmail.com"
+ },
+ "_npmVersion": "2.14.12",
+ "_phantomChildren": {},
+ "_requested": {
+ "raw": "meow@^3.7.0",
+ "scope": null,
+ "escapedName": "meow",
+ "name": "meow",
+ "rawSpec": "^3.7.0",
+ "spec": ">=3.7.0 <4.0.0",
+ "type": "range"
+ },
+ "_requiredBy": [
+ "/node-sass"
+ ],
+ "_resolved": "https://registry.npmjs.org/meow/-/meow-3.7.0.tgz",
+ "_shasum": "72cb668b425228290abbfa856892587308a801fb",
+ "_shrinkwrap": null,
+ "_spec": "meow@^3.7.0",
+ "_where": "/Users/ramswaroop/MyProjects/RamAndAntara/node_modules/node-sass",
+ "author": {
+ "name": "Sindre Sorhus",
+ "email": "sindresorhus@gmail.com",
+ "url": "sindresorhus.com"
+ },
+ "bugs": {
+ "url": "https://github.com/sindresorhus/meow/issues"
+ },
+ "dependencies": {
+ "camelcase-keys": "^2.0.0",
+ "decamelize": "^1.1.2",
+ "loud-rejection": "^1.0.0",
+ "map-obj": "^1.0.1",
+ "minimist": "^1.1.3",
+ "normalize-package-data": "^2.3.4",
+ "object-assign": "^4.0.1",
+ "read-pkg-up": "^1.0.1",
+ "redent": "^1.0.0",
+ "trim-newlines": "^1.0.0"
+ },
+ "description": "CLI app helper",
+ "devDependencies": {
+ "ava": "*",
+ "execa": "^0.1.1",
+ "indent-string": "^2.1.0",
+ "xo": "*"
+ },
+ "directories": {},
+ "dist": {
+ "shasum": "72cb668b425228290abbfa856892587308a801fb",
+ "tarball": "https://registry.npmjs.org/meow/-/meow-3.7.0.tgz"
+ },
+ "engines": {
+ "node": ">=0.10.0"
+ },
+ "files": [
+ "index.js"
+ ],
+ "gitHead": "9a5c90af79fb8f5f29c97e6b92b63f41e2df4f34",
+ "homepage": "https://github.com/sindresorhus/meow#readme",
+ "keywords": [
+ "cli",
+ "bin",
+ "util",
+ "utility",
+ "helper",
+ "argv",
+ "command",
+ "line",
+ "meow",
+ "cat",
+ "kitten",
+ "parser",
+ "option",
+ "flags",
+ "input",
+ "cmd",
+ "console"
+ ],
+ "license": "MIT",
+ "maintainers": [
+ {
+ "name": "sindresorhus",
+ "email": "sindresorhus@gmail.com"
+ }
+ ],
+ "name": "meow",
+ "optionalDependencies": {},
+ "readme": "ERROR: No README data found!",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/sindresorhus/meow.git"
+ },
+ "scripts": {
+ "test": "xo && ava"
+ },
+ "version": "3.7.0"
+}
diff --git a/node_modules/meow/readme.md b/node_modules/meow/readme.md
new file mode 100644
index 0000000..253380d
--- /dev/null
+++ b/node_modules/meow/readme.md
@@ -0,0 +1,159 @@
+# meow [](https://travis-ci.org/sindresorhus/meow)
+
+> CLI app helper
+
+
+
+
+## Features
+
+- Parses arguments using [minimist](https://github.com/substack/minimist)
+- Converts flags to [camelCase](https://github.com/sindresorhus/camelcase)
+- Outputs version when `--version`
+- Outputs description and supplied help text when `--help`
+- Makes unhandled rejected promises [fail loudly](https://github.com/sindresorhus/loud-rejection) instead of the default silent fail
+- Sets the process title to the binary name defined in package.json
+
+
+## Install
+
+```
+$ npm install --save meow
+```
+
+
+## Usage
+
+```
+$ ./foo-app.js unicorns --rainbow-cake
+```
+
+```js
+#!/usr/bin/env node
+'use strict';
+const meow = require('meow');
+const foo = require('./');
+
+const cli = meow(`
+ Usage
+ $ foo
+
+ Options
+ -r, --rainbow Include a rainbow
+
+ Examples
+ $ foo unicorns --rainbow
+ 🌈 unicorns 🌈
+`, {
+ alias: {
+ r: 'rainbow'
+ }
+});
+/*
+{
+ input: ['unicorns'],
+ flags: {rainbow: true},
+ ...
+}
+*/
+
+foo(cli.input[0], cli.flags);
+```
+
+
+## API
+
+### meow(options, [minimistOptions])
+
+Returns an object with:
+
+- `input` *(array)* - Non-flag arguments
+- `flags` *(object)* - Flags converted to camelCase
+- `pkg` *(object)* - The `package.json` object
+- `help` *(object)* - The help text used with `--help`
+- `showHelp([code=0])` *(function)* - Show the help text and exit with `code`
+
+#### options
+
+Type: `object`, `array`, `string`
+
+Can either be a string/array that is the `help` or an options object.
+
+##### description
+
+Type: `string`, `boolean`
+Default: The package.json `"description"` property
+
+A description to show above the help text.
+
+Set it to `false` to disable it altogether.
+
+##### help
+
+Type: `string`, `boolean`
+
+The help text you want shown.
+
+The input is reindented and starting/ending newlines are trimmed which means you can use a [template literal](https://developer.mozilla.org/en/docs/Web/JavaScript/Reference/template_strings) without having to care about using the correct amount of indent.
+
+If it's an array each item will be a line.
+*(Still supported, but you should use a template literal instead.)*
+
+The description will be shown above your help text automatically.
+
+Set it to `false` to disable it altogether.
+
+##### version
+
+Type: `string`, `boolean`
+Default: The package.json `"version"` property
+
+Set a custom version output.
+
+Set it to `false` to disable it altogether.
+
+##### pkg
+
+Type: `string`, `object`
+Default: Closest package.json upwards
+
+Relative path to package.json or as an object.
+
+##### argv
+
+Type: `array`
+Default: `process.argv.slice(2)`
+
+Custom arguments object.
+
+#### minimistOptions
+
+Type: `object`
+Default: `{}`
+
+Minimist [options](https://github.com/substack/minimist#var-argv--parseargsargs-opts).
+
+Keys passed to the minimist `default` option are [decamelized](https://github.com/sindresorhus/decamelize), so you can for example pass in `fooBar: 'baz'` and have it be the default for the `--foo-bar` flag.
+
+
+## Promises
+
+Meow will make unhandled rejected promises [fail loudly](https://github.com/sindresorhus/loud-rejection) instead of the default silent fail. Meaning you don't have to manually `.catch()` promises used in your CLI.
+
+
+## Tips
+
+See [`chalk`](https://github.com/chalk/chalk) if you want to colorize the terminal output.
+
+See [`get-stdin`](https://github.com/sindresorhus/get-stdin) if you want to accept input from stdin.
+
+See [`update-notifier`](https://github.com/yeoman/update-notifier) if you want update notifications.
+
+See [`configstore`](https://github.com/yeoman/configstore) if you need to persist some data.
+
+[More useful CLI utilities.](https://github.com/sindresorhus/awesome-nodejs#command-line-utilities)
+
+
+## License
+
+MIT © [Sindre Sorhus](http://sindresorhus.com)
diff --git a/node_modules/micromatch/CHANGELOG.md b/node_modules/micromatch/CHANGELOG.md
new file mode 100644
index 0000000..9d8e5ed
--- /dev/null
+++ b/node_modules/micromatch/CHANGELOG.md
@@ -0,0 +1,37 @@
+## History
+
+### key
+
+Changelog entries are classified using the following labels _(from [keep-a-changelog][]_):
+
+- `added`: for new features
+- `changed`: for changes in existing functionality
+- `deprecated`: for once-stable features removed in upcoming releases
+- `removed`: for deprecated features removed in this release
+- `fixed`: for any bug fixes
+- `bumped`: updated dependencies, only minor or higher will be listed.
+
+### [3.0.0] - 2017-04-11
+
+TODO. There should be no breaking changes. Please report any regressions. I will [reformat these release notes](https://github.com/micromatch/micromatch/pull/76) and add them to the changelog as soon as I have a chance.
+
+### [1.0.1] - 2016-12-12
+
+**Added**
+
+- Support for windows path edge cases where backslashes are used in brackets or other unusual combinations.
+
+### [1.0.0] - 2016-12-12
+
+Stable release.
+
+### [0.1.0] - 2016-10-08
+
+First release.
+
+
+[Unreleased]: https://github.com/jonschlinkert/micromatch/compare/0.1.0...HEAD
+[0.2.0]: https://github.com/jonschlinkert/micromatch/compare/0.1.0...0.2.0
+
+[keep-a-changelog]: https://github.com/olivierlacan/keep-a-changelog
+
diff --git a/node_modules/micromatch/LICENSE b/node_modules/micromatch/LICENSE
new file mode 100755
index 0000000..d32ab44
--- /dev/null
+++ b/node_modules/micromatch/LICENSE
@@ -0,0 +1,21 @@
+The MIT License (MIT)
+
+Copyright (c) 2014-2018, Jon Schlinkert.
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in
+all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
+THE SOFTWARE.
diff --git a/node_modules/micromatch/README.md b/node_modules/micromatch/README.md
new file mode 100644
index 0000000..5dfa149
--- /dev/null
+++ b/node_modules/micromatch/README.md
@@ -0,0 +1,1150 @@
+# micromatch [](https://www.npmjs.com/package/micromatch) [](https://npmjs.org/package/micromatch) [](https://npmjs.org/package/micromatch) [](https://travis-ci.org/micromatch/micromatch) [](https://ci.appveyor.com/project/micromatch/micromatch)
+
+> Glob matching for javascript/node.js. A drop-in replacement and faster alternative to minimatch and multimatch.
+
+Please consider following this project's author, [Jon Schlinkert](https://github.com/jonschlinkert), and consider starring the project to show your :heart: and support.
+
+## Table of Contents
+
+
+Details
+
+- [Install](#install)
+- [Quickstart](#quickstart)
+- [Why use micromatch?](#why-use-micromatch)
+ * [Matching features](#matching-features)
+- [Switching to micromatch](#switching-to-micromatch)
+ * [From minimatch](#from-minimatch)
+ * [From multimatch](#from-multimatch)
+- [API](#api)
+- [Options](#options)
+ * [options.basename](#optionsbasename)
+ * [options.bash](#optionsbash)
+ * [options.cache](#optionscache)
+ * [options.dot](#optionsdot)
+ * [options.failglob](#optionsfailglob)
+ * [options.ignore](#optionsignore)
+ * [options.matchBase](#optionsmatchbase)
+ * [options.nobrace](#optionsnobrace)
+ * [options.nocase](#optionsnocase)
+ * [options.nodupes](#optionsnodupes)
+ * [options.noext](#optionsnoext)
+ * [options.nonegate](#optionsnonegate)
+ * [options.noglobstar](#optionsnoglobstar)
+ * [options.nonull](#optionsnonull)
+ * [options.nullglob](#optionsnullglob)
+ * [options.snapdragon](#optionssnapdragon)
+ * [options.sourcemap](#optionssourcemap)
+ * [options.unescape](#optionsunescape)
+ * [options.unixify](#optionsunixify)
+- [Extended globbing](#extended-globbing)
+ * [extglobs](#extglobs)
+ * [braces](#braces)
+ * [regex character classes](#regex-character-classes)
+ * [regex groups](#regex-groups)
+ * [POSIX bracket expressions](#posix-bracket-expressions)
+- [Notes](#notes)
+ * [Bash 4.3 parity](#bash-43-parity)
+ * [Backslashes](#backslashes)
+- [Contributing](#contributing)
+- [Benchmarks](#benchmarks)
+ * [Running benchmarks](#running-benchmarks)
+ * [Latest results](#latest-results)
+- [About](#about)
+
+
+
+## Install
+
+Install with [npm](https://www.npmjs.com/):
+
+```sh
+$ npm install --save micromatch
+```
+
+## Quickstart
+
+```js
+var mm = require('micromatch');
+mm(list, patterns[, options]);
+```
+
+The [main export](#micromatch) takes a list of strings and one or more glob patterns:
+
+```js
+console.log(mm(['foo', 'bar', 'qux'], ['f*', 'b*']));
+//=> ['foo', 'bar']
+```
+
+Use [.isMatch()](#ismatch) to get true/false:
+
+```js
+console.log(mm.isMatch('foo', 'f*'));
+//=> true
+```
+
+[Switching](#switching-to-micromatch) from minimatch and multimatch is easy!
+
+## Why use micromatch?
+
+> micromatch is a [drop-in replacement](#switching-to-micromatch) for minimatch and multimatch
+
+* Supports all of the same matching features as [minimatch](https://github.com/isaacs/minimatch) and [multimatch](https://github.com/sindresorhus/multimatch)
+* Micromatch uses [snapdragon](https://github.com/jonschlinkert/snapdragon) for parsing and compiling globs, which provides granular control over the entire conversion process in a way that is easy to understand, reason about, and maintain.
+* More consistently accurate matching [than minimatch](https://github.com/yarnpkg/yarn/pull/3339), with more than 36,000 [test assertions](./test) to prove it.
+* More complete support for the Bash 4.3 specification than minimatch and multimatch. In fact, micromatch passes _all of the spec tests_ from bash, including some that bash still fails.
+* [Faster matching](#benchmarks), from a combination of optimized glob patterns, faster algorithms, and regex caching.
+* [Micromatch is safer](https://github.com/micromatch/braces#braces-is-safe), and is not subject to DoS with brace patterns, like minimatch and multimatch.
+* More reliable windows support than minimatch and multimatch.
+
+### Matching features
+
+* Support for multiple glob patterns (no need for wrappers like multimatch)
+* Wildcards (`**`, `*.js`)
+* Negation (`'!a/*.js'`, `'*!(b).js']`)
+* [extglobs](https://github.com/micromatch/extglob) (`+(x|y)`, `!(a|b)`)
+* [POSIX character classes](https://github.com/micromatch/expand-brackets) (`[[:alpha:][:digit:]]`)
+* [brace expansion](https://github.com/micromatch/braces) (`foo/{1..5}.md`, `bar/{a,b,c}.js`)
+* regex character classes (`foo-[1-5].js`)
+* regex logical "or" (`foo/(abc|xyz).js`)
+
+You can mix and match these features to create whatever patterns you need!
+
+## Switching to micromatch
+
+There is one notable difference between micromatch and minimatch in regards to how backslashes are handled. See [the notes about backslashes](#backslashes) for more information.
+
+### From minimatch
+
+Use [mm.isMatch()](#ismatch) instead of `minimatch()`:
+
+```js
+mm.isMatch('foo', 'b*');
+//=> false
+```
+
+Use [mm.match()](#match) instead of `minimatch.match()`:
+
+```js
+mm.match(['foo', 'bar'], 'b*');
+//=> 'bar'
+```
+
+### From multimatch
+
+Same signature:
+
+```js
+mm(['foo', 'bar', 'baz'], ['f*', '*z']);
+//=> ['foo', 'baz']
+```
+
+## API
+
+### [micromatch](index.js#L41)
+
+The main function takes a list of strings and one or more glob patterns to use for matching.
+
+**Params**
+
+* `list` **{Array}**: A list of strings to match
+* `patterns` **{String|Array}**: One or more glob patterns to use for matching.
+* `options` **{Object}**: See available [options](#options) for changing how matches are performed
+* `returns` **{Array}**: Returns an array of matches
+
+**Example**
+
+```js
+var mm = require('micromatch');
+mm(list, patterns[, options]);
+
+console.log(mm(['a.js', 'a.txt'], ['*.js']));
+//=> [ 'a.js' ]
+```
+
+### [.match](index.js#L93)
+
+Similar to the main function, but `pattern` must be a string.
+
+**Params**
+
+* `list` **{Array}**: Array of strings to match
+* `pattern` **{String}**: Glob pattern to use for matching.
+* `options` **{Object}**: See available [options](#options) for changing how matches are performed
+* `returns` **{Array}**: Returns an array of matches
+
+**Example**
+
+```js
+var mm = require('micromatch');
+mm.match(list, pattern[, options]);
+
+console.log(mm.match(['a.a', 'a.aa', 'a.b', 'a.c'], '*.a'));
+//=> ['a.a', 'a.aa']
+```
+
+### [.isMatch](index.js#L154)
+
+Returns true if the specified `string` matches the given glob `pattern`.
+
+**Params**
+
+* `string` **{String}**: String to match
+* `pattern` **{String}**: Glob pattern to use for matching.
+* `options` **{Object}**: See available [options](#options) for changing how matches are performed
+* `returns` **{Boolean}**: Returns true if the string matches the glob pattern.
+
+**Example**
+
+```js
+var mm = require('micromatch');
+mm.isMatch(string, pattern[, options]);
+
+console.log(mm.isMatch('a.a', '*.a'));
+//=> true
+console.log(mm.isMatch('a.b', '*.a'));
+//=> false
+```
+
+### [.some](index.js#L192)
+
+Returns true if some of the strings in the given `list` match any of the given glob `patterns`.
+
+**Params**
+
+* `list` **{String|Array}**: The string or array of strings to test. Returns as soon as the first match is found.
+* `patterns` **{String|Array}**: One or more glob patterns to use for matching.
+* `options` **{Object}**: See available [options](#options) for changing how matches are performed
+* `returns` **{Boolean}**: Returns true if any patterns match `str`
+
+**Example**
+
+```js
+var mm = require('micromatch');
+mm.some(list, patterns[, options]);
+
+console.log(mm.some(['foo.js', 'bar.js'], ['*.js', '!foo.js']));
+// true
+console.log(mm.some(['foo.js'], ['*.js', '!foo.js']));
+// false
+```
+
+### [.every](index.js#L228)
+
+Returns true if every string in the given `list` matches any of the given glob `patterns`.
+
+**Params**
+
+* `list` **{String|Array}**: The string or array of strings to test.
+* `patterns` **{String|Array}**: One or more glob patterns to use for matching.
+* `options` **{Object}**: See available [options](#options) for changing how matches are performed
+* `returns` **{Boolean}**: Returns true if any patterns match `str`
+
+**Example**
+
+```js
+var mm = require('micromatch');
+mm.every(list, patterns[, options]);
+
+console.log(mm.every('foo.js', ['foo.js']));
+// true
+console.log(mm.every(['foo.js', 'bar.js'], ['*.js']));
+// true
+console.log(mm.every(['foo.js', 'bar.js'], ['*.js', '!foo.js']));
+// false
+console.log(mm.every(['foo.js'], ['*.js', '!foo.js']));
+// false
+```
+
+### [.any](index.js#L260)
+
+Returns true if **any** of the given glob `patterns` match the specified `string`.
+
+**Params**
+
+* `str` **{String|Array}**: The string to test.
+* `patterns` **{String|Array}**: One or more glob patterns to use for matching.
+* `options` **{Object}**: See available [options](#options) for changing how matches are performed
+* `returns` **{Boolean}**: Returns true if any patterns match `str`
+
+**Example**
+
+```js
+var mm = require('micromatch');
+mm.any(string, patterns[, options]);
+
+console.log(mm.any('a.a', ['b.*', '*.a']));
+//=> true
+console.log(mm.any('a.a', 'b.*'));
+//=> false
+```
+
+### [.all](index.js#L308)
+
+Returns true if **all** of the given `patterns` match the specified string.
+
+**Params**
+
+* `str` **{String|Array}**: The string to test.
+* `patterns` **{String|Array}**: One or more glob patterns to use for matching.
+* `options` **{Object}**: See available [options](#options) for changing how matches are performed
+* `returns` **{Boolean}**: Returns true if any patterns match `str`
+
+**Example**
+
+```js
+var mm = require('micromatch');
+mm.all(string, patterns[, options]);
+
+console.log(mm.all('foo.js', ['foo.js']));
+// true
+
+console.log(mm.all('foo.js', ['*.js', '!foo.js']));
+// false
+
+console.log(mm.all('foo.js', ['*.js', 'foo.js']));
+// true
+
+console.log(mm.all('foo.js', ['*.js', 'f*', '*o*', '*o.js']));
+// true
+```
+
+### [.not](index.js#L340)
+
+Returns a list of strings that _**do not match any**_ of the given `patterns`.
+
+**Params**
+
+* `list` **{Array}**: Array of strings to match.
+* `patterns` **{String|Array}**: One or more glob pattern to use for matching.
+* `options` **{Object}**: See available [options](#options) for changing how matches are performed
+* `returns` **{Array}**: Returns an array of strings that **do not match** the given patterns.
+
+**Example**
+
+```js
+var mm = require('micromatch');
+mm.not(list, patterns[, options]);
+
+console.log(mm.not(['a.a', 'b.b', 'c.c'], '*.a'));
+//=> ['b.b', 'c.c']
+```
+
+### [.contains](index.js#L376)
+
+Returns true if the given `string` contains the given pattern. Similar to [.isMatch](#isMatch) but the pattern can match any part of the string.
+
+**Params**
+
+* `str` **{String}**: The string to match.
+* `patterns` **{String|Array}**: Glob pattern to use for matching.
+* `options` **{Object}**: See available [options](#options) for changing how matches are performed
+* `returns` **{Boolean}**: Returns true if the patter matches any part of `str`.
+
+**Example**
+
+```js
+var mm = require('micromatch');
+mm.contains(string, pattern[, options]);
+
+console.log(mm.contains('aa/bb/cc', '*b'));
+//=> true
+console.log(mm.contains('aa/bb/cc', '*d'));
+//=> false
+```
+
+### [.matchKeys](index.js#L432)
+
+Filter the keys of the given object with the given `glob` pattern and `options`. Does not attempt to match nested keys. If you need this feature, use [glob-object](https://github.com/jonschlinkert/glob-object) instead.
+
+**Params**
+
+* `object` **{Object}**: The object with keys to filter.
+* `patterns` **{String|Array}**: One or more glob patterns to use for matching.
+* `options` **{Object}**: See available [options](#options) for changing how matches are performed
+* `returns` **{Object}**: Returns an object with only keys that match the given patterns.
+
+**Example**
+
+```js
+var mm = require('micromatch');
+mm.matchKeys(object, patterns[, options]);
+
+var obj = { aa: 'a', ab: 'b', ac: 'c' };
+console.log(mm.matchKeys(obj, '*b'));
+//=> { ab: 'b' }
+```
+
+### [.matcher](index.js#L461)
+
+Returns a memoized matcher function from the given glob `pattern` and `options`. The returned function takes a string to match as its only argument and returns true if the string is a match.
+
+**Params**
+
+* `pattern` **{String}**: Glob pattern
+* `options` **{Object}**: See available [options](#options) for changing how matches are performed.
+* `returns` **{Function}**: Returns a matcher function.
+
+**Example**
+
+```js
+var mm = require('micromatch');
+mm.matcher(pattern[, options]);
+
+var isMatch = mm.matcher('*.!(*a)');
+console.log(isMatch('a.a'));
+//=> false
+console.log(isMatch('a.b'));
+//=> true
+```
+
+### [.capture](index.js#L536)
+
+Returns an array of matches captured by `pattern` in `string, or`null` if the pattern did not match.
+
+**Params**
+
+* `pattern` **{String}**: Glob pattern to use for matching.
+* `string` **{String}**: String to match
+* `options` **{Object}**: See available [options](#options) for changing how matches are performed
+* `returns` **{Boolean}**: Returns an array of captures if the string matches the glob pattern, otherwise `null`.
+
+**Example**
+
+```js
+var mm = require('micromatch');
+mm.capture(pattern, string[, options]);
+
+console.log(mm.capture('test/*.js', 'test/foo.js'));
+//=> ['foo']
+console.log(mm.capture('test/*.js', 'foo/bar.css'));
+//=> null
+```
+
+### [.makeRe](index.js#L571)
+
+Create a regular expression from the given glob `pattern`.
+
+**Params**
+
+* `pattern` **{String}**: A glob pattern to convert to regex.
+* `options` **{Object}**: See available [options](#options) for changing how matches are performed.
+* `returns` **{RegExp}**: Returns a regex created from the given pattern.
+
+**Example**
+
+```js
+var mm = require('micromatch');
+mm.makeRe(pattern[, options]);
+
+console.log(mm.makeRe('*.js'));
+//=> /^(?:(\.[\\\/])?(?!\.)(?=.)[^\/]*?\.js)$/
+```
+
+### [.braces](index.js#L618)
+
+Expand the given brace `pattern`.
+
+**Params**
+
+* `pattern` **{String}**: String with brace pattern to expand.
+* `options` **{Object}**: Any [options](#options) to change how expansion is performed. See the [braces](https://github.com/micromatch/braces) library for all available options.
+* `returns` **{Array}**
+
+**Example**
+
+```js
+var mm = require('micromatch');
+console.log(mm.braces('foo/{a,b}/bar'));
+//=> ['foo/(a|b)/bar']
+
+console.log(mm.braces('foo/{a,b}/bar', {expand: true}));
+//=> ['foo/(a|b)/bar']
+```
+
+### [.create](index.js#L685)
+
+Parses the given glob `pattern` and returns an array of abstract syntax trees (ASTs), with the compiled `output` and optional source `map` on each AST.
+
+**Params**
+
+* `pattern` **{String}**: Glob pattern to parse and compile.
+* `options` **{Object}**: Any [options](#options) to change how parsing and compiling is performed.
+* `returns` **{Object}**: Returns an object with the parsed AST, compiled string and optional source map.
+
+**Example**
+
+```js
+var mm = require('micromatch');
+mm.create(pattern[, options]);
+
+console.log(mm.create('abc/*.js'));
+// [{ options: { source: 'string', sourcemap: true },
+// state: {},
+// compilers:
+// { ... },
+// output: '(\\.[\\\\\\/])?abc\\/(?!\\.)(?=.)[^\\/]*?\\.js',
+// ast:
+// { type: 'root',
+// errors: [],
+// nodes:
+// [ ... ],
+// dot: false,
+// input: 'abc/*.js' },
+// parsingErrors: [],
+// map:
+// { version: 3,
+// sources: [ 'string' ],
+// names: [],
+// mappings: 'AAAA,GAAG,EAAC,kBAAC,EAAC,EAAE',
+// sourcesContent: [ 'abc/*.js' ] },
+// position: { line: 1, column: 28 },
+// content: {},
+// files: {},
+// idx: 6 }]
+```
+
+### [.parse](index.js#L732)
+
+Parse the given `str` with the given `options`.
+
+**Params**
+
+* `str` **{String}**
+* `options` **{Object}**
+* `returns` **{Object}**: Returns an AST
+
+**Example**
+
+```js
+var mm = require('micromatch');
+mm.parse(pattern[, options]);
+
+var ast = mm.parse('a/{b,c}/d');
+console.log(ast);
+// { type: 'root',
+// errors: [],
+// input: 'a/{b,c}/d',
+// nodes:
+// [ { type: 'bos', val: '' },
+// { type: 'text', val: 'a/' },
+// { type: 'brace',
+// nodes:
+// [ { type: 'brace.open', val: '{' },
+// { type: 'text', val: 'b,c' },
+// { type: 'brace.close', val: '}' } ] },
+// { type: 'text', val: '/d' },
+// { type: 'eos', val: '' } ] }
+```
+
+### [.compile](index.js#L780)
+
+Compile the given `ast` or string with the given `options`.
+
+**Params**
+
+* `ast` **{Object|String}**
+* `options` **{Object}**
+* `returns` **{Object}**: Returns an object that has an `output` property with the compiled string.
+
+**Example**
+
+```js
+var mm = require('micromatch');
+mm.compile(ast[, options]);
+
+var ast = mm.parse('a/{b,c}/d');
+console.log(mm.compile(ast));
+// { options: { source: 'string' },
+// state: {},
+// compilers:
+// { eos: [Function],
+// noop: [Function],
+// bos: [Function],
+// brace: [Function],
+// 'brace.open': [Function],
+// text: [Function],
+// 'brace.close': [Function] },
+// output: [ 'a/(b|c)/d' ],
+// ast:
+// { ... },
+// parsingErrors: [] }
+```
+
+### [.clearCache](index.js#L801)
+
+Clear the regex cache.
+
+**Example**
+
+```js
+mm.clearCache();
+```
+
+## Options
+
+* [basename](#optionsbasename)
+* [bash](#optionsbash)
+* [cache](#optionscache)
+* [dot](#optionsdot)
+* [failglob](#optionsfailglob)
+* [ignore](#optionsignore)
+* [matchBase](#optionsmatchBase)
+* [nobrace](#optionsnobrace)
+* [nocase](#optionsnocase)
+* [nodupes](#optionsnodupes)
+* [noext](#optionsnoext)
+* [noglobstar](#optionsnoglobstar)
+* [nonull](#optionsnonull)
+* [nullglob](#optionsnullglob)
+* [snapdragon](#optionssnapdragon)
+* [sourcemap](#optionssourcemap)
+* [unescape](#optionsunescape)
+* [unixify](#optionsunixify)
+
+### options.basename
+
+Allow glob patterns without slashes to match a file path based on its basename. Same behavior as [minimatch](https://github.com/isaacs/minimatch) option `matchBase`.
+
+**Type**: `Boolean`
+
+**Default**: `false`
+
+**Example**
+
+```js
+mm(['a/b.js', 'a/c.md'], '*.js');
+//=> []
+
+mm(['a/b.js', 'a/c.md'], '*.js', {matchBase: true});
+//=> ['a/b.js']
+```
+
+### options.bash
+
+Enabled by default, this option enforces bash-like behavior with stars immediately following a bracket expression. Bash bracket expressions are similar to regex character classes, but unlike regex, a star following a bracket expression **does not repeat the bracketed characters**. Instead, the star is treated the same as an other star.
+
+**Type**: `Boolean`
+
+**Default**: `true`
+
+**Example**
+
+```js
+var files = ['abc', 'ajz'];
+console.log(mm(files, '[a-c]*'));
+//=> ['abc', 'ajz']
+
+console.log(mm(files, '[a-c]*', {bash: false}));
+```
+
+### options.cache
+
+Disable regex and function memoization.
+
+**Type**: `Boolean`
+
+**Default**: `undefined`
+
+### options.dot
+
+Match dotfiles. Same behavior as [minimatch](https://github.com/isaacs/minimatch) option `dot`.
+
+**Type**: `Boolean`
+
+**Default**: `false`
+
+### options.failglob
+
+Similar to the `--failglob` behavior in Bash, throws an error when no matches are found.
+
+**Type**: `Boolean`
+
+**Default**: `undefined`
+
+### options.ignore
+
+String or array of glob patterns to match files to ignore.
+
+**Type**: `String|Array`
+
+**Default**: `undefined`
+
+### options.matchBase
+
+Alias for [options.basename](#options-basename).
+
+### options.nobrace
+
+Disable expansion of brace patterns. Same behavior as [minimatch](https://github.com/isaacs/minimatch) option `nobrace`.
+
+**Type**: `Boolean`
+
+**Default**: `undefined`
+
+See [braces](https://github.com/micromatch/braces) for more information about extended brace expansion.
+
+### options.nocase
+
+Use a case-insensitive regex for matching files. Same behavior as [minimatch](https://github.com/isaacs/minimatch).
+
+**Type**: `Boolean`
+
+**Default**: `undefined`
+
+### options.nodupes
+
+Remove duplicate elements from the result array.
+
+**Type**: `Boolean`
+
+**Default**: `undefined`
+
+**Example**
+
+Example of using the `unescape` and `nodupes` options together:
+
+```js
+mm.match(['a/b/c', 'a/b/c'], 'a/b/c');
+//=> ['a/b/c', 'a/b/c']
+
+mm.match(['a/b/c', 'a/b/c'], 'a/b/c', {nodupes: true});
+//=> ['abc']
+```
+
+### options.noext
+
+Disable extglob support, so that extglobs are regarded as literal characters.
+
+**Type**: `Boolean`
+
+**Default**: `undefined`
+
+**Examples**
+
+```js
+mm(['a/z', 'a/b', 'a/!(z)'], 'a/!(z)');
+//=> ['a/b', 'a/!(z)']
+
+mm(['a/z', 'a/b', 'a/!(z)'], 'a/!(z)', {noext: true});
+//=> ['a/!(z)'] (matches only as literal characters)
+```
+
+### options.nonegate
+
+Disallow negation (`!`) patterns, and treat leading `!` as a literal character to match.
+
+**Type**: `Boolean`
+
+**Default**: `undefined`
+
+### options.noglobstar
+
+Disable matching with globstars (`**`).
+
+**Type**: `Boolean`
+
+**Default**: `undefined`
+
+```js
+mm(['a/b', 'a/b/c', 'a/b/c/d'], 'a/**');
+//=> ['a/b', 'a/b/c', 'a/b/c/d']
+
+mm(['a/b', 'a/b/c', 'a/b/c/d'], 'a/**', {noglobstar: true});
+//=> ['a/b']
+```
+
+### options.nonull
+
+Alias for [options.nullglob](#options-nullglob).
+
+### options.nullglob
+
+If `true`, when no matches are found the actual (arrayified) glob pattern is returned instead of an empty array. Same behavior as [minimatch](https://github.com/isaacs/minimatch) option `nonull`.
+
+**Type**: `Boolean`
+
+**Default**: `undefined`
+
+### options.snapdragon
+
+Pass your own instance of [snapdragon](https://github.com/jonschlinkert/snapdragon), to customize parsers or compilers.
+
+**Type**: `Object`
+
+**Default**: `undefined`
+
+### options.sourcemap
+
+Generate a source map by enabling the `sourcemap` option with the `.parse`, `.compile`, or `.create` methods.
+
+_(Note that sourcemaps are currently not enabled for brace patterns)_
+
+**Examples**
+
+``` js
+var mm = require('micromatch');
+var pattern = '*(*(of*(a)x)z)';
+
+var res = mm.create('abc/*.js', {sourcemap: true});
+console.log(res.map);
+// { version: 3,
+// sources: [ 'string' ],
+// names: [],
+// mappings: 'AAAA,GAAG,EAAC,iBAAC,EAAC,EAAE',
+// sourcesContent: [ 'abc/*.js' ] }
+
+var ast = mm.parse('abc/**/*.js');
+var res = mm.compile(ast, {sourcemap: true});
+console.log(res.map);
+// { version: 3,
+// sources: [ 'string' ],
+// names: [],
+// mappings: 'AAAA,GAAG,EAAC,2BAAE,EAAC,iBAAC,EAAC,EAAE',
+// sourcesContent: [ 'abc/**/*.js' ] }
+
+var ast = mm.parse(pattern);
+var res = mm.compile(ast, {sourcemap: true});
+console.log(res.map);
+// { version: 3,
+// sources: [ 'string' ],
+// names: [],
+// mappings: 'AAAA,CAAE,CAAE,EAAE,CAAE,CAAC,EAAC,CAAC,EAAC,CAAC,EAAC',
+// sourcesContent: [ '*(*(of*(a)x)z)' ] }
+```
+
+### options.unescape
+
+Remove backslashes from returned matches.
+
+**Type**: `Boolean`
+
+**Default**: `undefined`
+
+**Example**
+
+In this example we want to match a literal `*`:
+
+```js
+mm.match(['abc', 'a\\*c'], 'a\\*c');
+//=> ['a\\*c']
+
+mm.match(['abc', 'a\\*c'], 'a\\*c', {unescape: true});
+//=> ['a*c']
+```
+
+### options.unixify
+
+Convert path separators on returned files to posix/unix-style forward slashes.
+
+**Type**: `Boolean`
+
+**Default**: `true` on windows, `false` everywhere else
+
+**Example**
+
+```js
+mm.match(['a\\b\\c'], 'a/**');
+//=> ['a/b/c']
+
+mm.match(['a\\b\\c'], {unixify: false});
+//=> ['a\\b\\c']
+```
+
+## Extended globbing
+
+Micromatch also supports extended globbing features.
+
+### extglobs
+
+Extended globbing, as described by the bash man page:
+
+| **pattern** | **regex equivalent** | **description** |
+| --- | --- | --- |
+| `?(pattern)` | `(pattern)?` | Matches zero or one occurrence of the given patterns |
+| `*(pattern)` | `(pattern)*` | Matches zero or more occurrences of the given patterns |
+| `+(pattern)` | `(pattern)+` | Matches one or more occurrences of the given patterns |
+| `@(pattern)` | `(pattern)` * | Matches one of the given patterns |
+| `!(pattern)` | N/A (equivalent regex is much more complicated) | Matches anything except one of the given patterns |
+
+* Note that `@` isn't a RegEx character.
+
+Powered by [extglob](https://github.com/micromatch/extglob). Visit that library for the full range of options or to report extglob related issues.
+
+### braces
+
+Brace patterns can be used to match specific ranges or sets of characters. For example, the pattern `*/{1..3}/*` would match any of following strings:
+
+```
+foo/1/bar
+foo/2/bar
+foo/3/bar
+baz/1/qux
+baz/2/qux
+baz/3/qux
+```
+
+Visit [braces](https://github.com/micromatch/braces) to see the full range of features and options related to brace expansion, or to create brace matching or expansion related issues.
+
+### regex character classes
+
+Given the list: `['a.js', 'b.js', 'c.js', 'd.js', 'E.js']`:
+
+* `[ac].js`: matches both `a` and `c`, returning `['a.js', 'c.js']`
+* `[b-d].js`: matches from `b` to `d`, returning `['b.js', 'c.js', 'd.js']`
+* `[b-d].js`: matches from `b` to `d`, returning `['b.js', 'c.js', 'd.js']`
+* `a/[A-Z].js`: matches and uppercase letter, returning `['a/E.md']`
+
+Learn about [regex character classes](http://www.regular-expressions.info/charclass.html).
+
+### regex groups
+
+Given `['a.js', 'b.js', 'c.js', 'd.js', 'E.js']`:
+
+* `(a|c).js`: would match either `a` or `c`, returning `['a.js', 'c.js']`
+* `(b|d).js`: would match either `b` or `d`, returning `['b.js', 'd.js']`
+* `(b|[A-Z]).js`: would match either `b` or an uppercase letter, returning `['b.js', 'E.js']`
+
+As with regex, parens can be nested, so patterns like `((a|b)|c)/b` will work. Although brace expansion might be friendlier to use, depending on preference.
+
+### POSIX bracket expressions
+
+POSIX brackets are intended to be more user-friendly than regex character classes. This of course is in the eye of the beholder.
+
+**Example**
+
+```js
+mm.isMatch('a1', '[[:alpha:][:digit:]]');
+//=> true
+
+mm.isMatch('a1', '[[:alpha:][:alpha:]]');
+//=> false
+```
+
+See [expand-brackets](https://github.com/jonschlinkert/expand-brackets) for more information about bracket expressions.
+
+***
+
+## Notes
+
+### Bash 4.3 parity
+
+Whenever possible matching behavior is based on behavior Bash 4.3, which is mostly consistent with minimatch.
+
+However, it's suprising how many edge cases and rabbit holes there are with glob matching, and since there is no real glob specification, and micromatch is more accurate than both Bash and minimatch, there are cases where best-guesses were made for behavior. In a few cases where Bash had no answers, we used wildmatch (used by git) as a fallback.
+
+### Backslashes
+
+There is an important, notable difference between minimatch and micromatch _in regards to how backslashes are handled_ in glob patterns.
+
+* Micromatch exclusively and explicitly reserves backslashes for escaping characters in a glob pattern, even on windows. This is consistent with bash behavior.
+* Minimatch converts all backslashes to forward slashes, which means you can't use backslashes to escape any characters in your glob patterns.
+
+We made this decision for micromatch for a couple of reasons:
+
+* consistency with bash conventions.
+* glob patterns are not filepaths. They are a type of [regular language](https://en.wikipedia.org/wiki/Regular_language) that is converted to a JavaScript regular expression. Thus, when forward slashes are defined in a glob pattern, the resulting regular expression will match windows or POSIX path separators just fine.
+
+**A note about joining paths to globs**
+
+Note that when you pass something like `path.join('foo', '*')` to micromatch, you are creating a filepath and expecting it to still work as a glob pattern. This causes problems on windows, since the `path.sep` is `\\`.
+
+In other words, since `\\` is reserved as an escape character in globs, on windows `path.join('foo', '*')` would result in `foo\\*`, which tells micromatch to match `*` as a literal character. This is the same behavior as bash.
+
+## Contributing
+
+All contributions are welcome! Please read [the contributing guide](.github/contributing.md) to get started.
+
+**Bug reports**
+
+Please create an issue if you encounter a bug or matching behavior that doesn't seem correct. If you find a matching-related issue, please:
+
+* [research existing issues first](../../issues) (open and closed)
+* visit the [GNU Bash documentation](https://www.gnu.org/software/bash/manual/) to see how Bash deals with the pattern
+* visit the [minimatch](https://github.com/isaacs/minimatch) documentation to cross-check expected behavior in node.js
+* if all else fails, since there is no real specification for globs we will probably need to discuss expected behavior and decide how to resolve it. which means any detail you can provide to help with this discussion would be greatly appreciated.
+
+**Platform issues**
+
+It's important to us that micromatch work consistently on all platforms. If you encounter any platform-specific matching or path related issues, please let us know (pull requests are also greatly appreciated).
+
+## Benchmarks
+
+### Running benchmarks
+
+Install dev dependencies:
+
+```bash
+npm i -d && npm run benchmark
+```
+
+### Latest results
+
+As of February 18, 2018 (longer bars are better):
+
+```sh
+# braces-globstar-large-list (485691 bytes)
+ micromatch ██████████████████████████████████████████████████ (517 ops/sec ±0.49%)
+ minimatch █ (18.92 ops/sec ±0.54%)
+ multimatch █ (18.94 ops/sec ±0.62%)
+
+ micromatch is faster by an avg. of 2,733%
+
+# braces-multiple (3362 bytes)
+ micromatch ██████████████████████████████████████████████████ (33,625 ops/sec ±0.45%)
+ minimatch (2.92 ops/sec ±3.26%)
+ multimatch (2.90 ops/sec ±2.76%)
+
+ micromatch is faster by an avg. of 1,156,935%
+
+# braces-range (727 bytes)
+ micromatch █████████████████████████████████████████████████ (155,220 ops/sec ±0.56%)
+ minimatch ██████ (20,186 ops/sec ±1.27%)
+ multimatch ██████ (19,809 ops/sec ±0.60%)
+
+ micromatch is faster by an avg. of 776%
+
+# braces-set (2858 bytes)
+ micromatch █████████████████████████████████████████████████ (24,354 ops/sec ±0.92%)
+ minimatch █████ (2,566 ops/sec ±0.56%)
+ multimatch ████ (2,431 ops/sec ±1.25%)
+
+ micromatch is faster by an avg. of 975%
+
+# globstar-large-list (485686 bytes)
+ micromatch █████████████████████████████████████████████████ (504 ops/sec ±0.45%)
+ minimatch ███ (33.36 ops/sec ±1.08%)
+ multimatch ███ (33.19 ops/sec ±1.35%)
+
+ micromatch is faster by an avg. of 1,514%
+
+# globstar-long-list (90647 bytes)
+ micromatch ██████████████████████████████████████████████████ (2,694 ops/sec ±1.08%)
+ minimatch ████████████████ (870 ops/sec ±1.09%)
+ multimatch ████████████████ (862 ops/sec ±0.84%)
+
+ micromatch is faster by an avg. of 311%
+
+# globstar-short-list (182 bytes)
+ micromatch ██████████████████████████████████████████████████ (328,921 ops/sec ±1.06%)
+ minimatch █████████ (64,808 ops/sec ±1.42%)
+ multimatch ████████ (57,991 ops/sec ±2.11%)
+
+ micromatch is faster by an avg. of 536%
+
+# no-glob (701 bytes)
+ micromatch █████████████████████████████████████████████████ (415,935 ops/sec ±0.36%)
+ minimatch ███████████ (92,730 ops/sec ±1.44%)
+ multimatch █████████ (81,958 ops/sec ±2.13%)
+
+ micromatch is faster by an avg. of 476%
+
+# star-basename-long (12339 bytes)
+ micromatch █████████████████████████████████████████████████ (7,963 ops/sec ±0.36%)
+ minimatch ███████████████████████████████ (5,072 ops/sec ±0.83%)
+ multimatch ███████████████████████████████ (5,028 ops/sec ±0.40%)
+
+ micromatch is faster by an avg. of 158%
+
+# star-basename-short (349 bytes)
+ micromatch ██████████████████████████████████████████████████ (269,552 ops/sec ±0.70%)
+ minimatch ██████████████████████ (122,457 ops/sec ±1.39%)
+ multimatch ████████████████████ (110,788 ops/sec ±1.99%)
+
+ micromatch is faster by an avg. of 231%
+
+# star-folder-long (19207 bytes)
+ micromatch █████████████████████████████████████████████████ (3,806 ops/sec ±0.38%)
+ minimatch ████████████████████████████ (2,204 ops/sec ±0.32%)
+ multimatch ██████████████████████████ (2,020 ops/sec ±1.07%)
+
+ micromatch is faster by an avg. of 180%
+
+# star-folder-short (551 bytes)
+ micromatch ██████████████████████████████████████████████████ (249,077 ops/sec ±0.40%)
+ minimatch ███████████ (59,431 ops/sec ±1.67%)
+ multimatch ███████████ (55,569 ops/sec ±1.43%)
+
+ micromatch is faster by an avg. of 433%
+```
+
+## About
+
+
+Contributing
+
+Pull requests and stars are always welcome. For bugs and feature requests, [please create an issue](../../issues/new).
+
+Please read the [contributing guide](.github/contributing.md) for advice on opening issues, pull requests, and coding standards.
+
+
+
+
+Running Tests
+
+Running and reviewing unit tests is a great way to get familiarized with a library and its API. You can install dependencies and run tests with the following command:
+
+```sh
+$ npm install && npm test
+```
+
+
+
+
+Building docs
+
+_(This project's readme.md is generated by [verb](https://github.com/verbose/verb-generate-readme), please don't edit the readme directly. Any changes to the readme must be made in the [.verb.md](.verb.md) readme template.)_
+
+To generate the readme, run the following command:
+
+```sh
+$ npm install -g verbose/verb#dev verb-generate-readme && verb
+```
+
+
+
+### Related projects
+
+You might also be interested in these projects:
+
+* [braces](https://www.npmjs.com/package/braces): Bash-like brace expansion, implemented in JavaScript. Safer than other brace expansion libs, with complete support… [more](https://github.com/micromatch/braces) | [homepage](https://github.com/micromatch/braces "Bash-like brace expansion, implemented in JavaScript. Safer than other brace expansion libs, with complete support for the Bash 4.3 braces specification, without sacrificing speed.")
+* [expand-brackets](https://www.npmjs.com/package/expand-brackets): Expand POSIX bracket expressions (character classes) in glob patterns. | [homepage](https://github.com/jonschlinkert/expand-brackets "Expand POSIX bracket expressions (character classes) in glob patterns.")
+* [extglob](https://www.npmjs.com/package/extglob): Extended glob support for JavaScript. Adds (almost) the expressive power of regular expressions to glob… [more](https://github.com/micromatch/extglob) | [homepage](https://github.com/micromatch/extglob "Extended glob support for JavaScript. Adds (almost) the expressive power of regular expressions to glob patterns.")
+* [fill-range](https://www.npmjs.com/package/fill-range): Fill in a range of numbers or letters, optionally passing an increment or `step` to… [more](https://github.com/jonschlinkert/fill-range) | [homepage](https://github.com/jonschlinkert/fill-range "Fill in a range of numbers or letters, optionally passing an increment or `step` to use, or create a regex-compatible range with `options.toRegex`")
+* [nanomatch](https://www.npmjs.com/package/nanomatch): Fast, minimal glob matcher for node.js. Similar to micromatch, minimatch and multimatch, but complete Bash… [more](https://github.com/micromatch/nanomatch) | [homepage](https://github.com/micromatch/nanomatch "Fast, minimal glob matcher for node.js. Similar to micromatch, minimatch and multimatch, but complete Bash 4.3 wildcard support only (no support for exglobs, posix brackets or braces)")
+
+### Contributors
+
+| **Commits** | **Contributor** |
+| --- | --- |
+| 457 | [jonschlinkert](https://github.com/jonschlinkert) |
+| 12 | [es128](https://github.com/es128) |
+| 8 | [doowb](https://github.com/doowb) |
+| 3 | [paulmillr](https://github.com/paulmillr) |
+| 2 | [TrySound](https://github.com/TrySound) |
+| 2 | [MartinKolarik](https://github.com/MartinKolarik) |
+| 2 | [charlike-old](https://github.com/charlike-old) |
+| 1 | [amilajack](https://github.com/amilajack) |
+| 1 | [mrmlnc](https://github.com/mrmlnc) |
+| 1 | [devongovett](https://github.com/devongovett) |
+| 1 | [DianeLooney](https://github.com/DianeLooney) |
+| 1 | [UltCombo](https://github.com/UltCombo) |
+| 1 | [tomByrer](https://github.com/tomByrer) |
+| 1 | [fidian](https://github.com/fidian) |
+
+### Author
+
+**Jon Schlinkert**
+
+* [linkedin/in/jonschlinkert](https://linkedin.com/in/jonschlinkert)
+* [github/jonschlinkert](https://github.com/jonschlinkert)
+* [twitter/jonschlinkert](https://twitter.com/jonschlinkert)
+
+### License
+
+Copyright © 2018, [Jon Schlinkert](https://github.com/jonschlinkert).
+Released under the [MIT License](LICENSE).
+
+***
+
+_This file was generated by [verb-generate-readme](https://github.com/verbose/verb-generate-readme), v0.6.0, on February 18, 2018._
\ No newline at end of file
diff --git a/node_modules/micromatch/index.js b/node_modules/micromatch/index.js
new file mode 100644
index 0000000..fe02f2c
--- /dev/null
+++ b/node_modules/micromatch/index.js
@@ -0,0 +1,877 @@
+'use strict';
+
+/**
+ * Module dependencies
+ */
+
+var util = require('util');
+var braces = require('braces');
+var toRegex = require('to-regex');
+var extend = require('extend-shallow');
+
+/**
+ * Local dependencies
+ */
+
+var compilers = require('./lib/compilers');
+var parsers = require('./lib/parsers');
+var cache = require('./lib/cache');
+var utils = require('./lib/utils');
+var MAX_LENGTH = 1024 * 64;
+
+/**
+ * The main function takes a list of strings and one or more
+ * glob patterns to use for matching.
+ *
+ * ```js
+ * var mm = require('micromatch');
+ * mm(list, patterns[, options]);
+ *
+ * console.log(mm(['a.js', 'a.txt'], ['*.js']));
+ * //=> [ 'a.js' ]
+ * ```
+ * @param {Array} `list` A list of strings to match
+ * @param {String|Array} `patterns` One or more glob patterns to use for matching.
+ * @param {Object} `options` See available [options](#options) for changing how matches are performed
+ * @return {Array} Returns an array of matches
+ * @summary false
+ * @api public
+ */
+
+function micromatch(list, patterns, options) {
+ patterns = utils.arrayify(patterns);
+ list = utils.arrayify(list);
+
+ var len = patterns.length;
+ if (list.length === 0 || len === 0) {
+ return [];
+ }
+
+ if (len === 1) {
+ return micromatch.match(list, patterns[0], options);
+ }
+
+ var omit = [];
+ var keep = [];
+ var idx = -1;
+
+ while (++idx < len) {
+ var pattern = patterns[idx];
+
+ if (typeof pattern === 'string' && pattern.charCodeAt(0) === 33 /* ! */) {
+ omit.push.apply(omit, micromatch.match(list, pattern.slice(1), options));
+ } else {
+ keep.push.apply(keep, micromatch.match(list, pattern, options));
+ }
+ }
+
+ var matches = utils.diff(keep, omit);
+ if (!options || options.nodupes !== false) {
+ return utils.unique(matches);
+ }
+
+ return matches;
+}
+
+/**
+ * Similar to the main function, but `pattern` must be a string.
+ *
+ * ```js
+ * var mm = require('micromatch');
+ * mm.match(list, pattern[, options]);
+ *
+ * console.log(mm.match(['a.a', 'a.aa', 'a.b', 'a.c'], '*.a'));
+ * //=> ['a.a', 'a.aa']
+ * ```
+ * @param {Array} `list` Array of strings to match
+ * @param {String} `pattern` Glob pattern to use for matching.
+ * @param {Object} `options` See available [options](#options) for changing how matches are performed
+ * @return {Array} Returns an array of matches
+ * @api public
+ */
+
+micromatch.match = function(list, pattern, options) {
+ if (Array.isArray(pattern)) {
+ throw new TypeError('expected pattern to be a string');
+ }
+
+ var unixify = utils.unixify(options);
+ var isMatch = memoize('match', pattern, options, micromatch.matcher);
+ var matches = [];
+
+ list = utils.arrayify(list);
+ var len = list.length;
+ var idx = -1;
+
+ while (++idx < len) {
+ var ele = list[idx];
+ if (ele === pattern || isMatch(ele)) {
+ matches.push(utils.value(ele, unixify, options));
+ }
+ }
+
+ // if no options were passed, uniquify results and return
+ if (typeof options === 'undefined') {
+ return utils.unique(matches);
+ }
+
+ if (matches.length === 0) {
+ if (options.failglob === true) {
+ throw new Error('no matches found for "' + pattern + '"');
+ }
+ if (options.nonull === true || options.nullglob === true) {
+ return [options.unescape ? utils.unescape(pattern) : pattern];
+ }
+ }
+
+ // if `opts.ignore` was defined, diff ignored list
+ if (options.ignore) {
+ matches = micromatch.not(matches, options.ignore, options);
+ }
+
+ return options.nodupes !== false ? utils.unique(matches) : matches;
+};
+
+/**
+ * Returns true if the specified `string` matches the given glob `pattern`.
+ *
+ * ```js
+ * var mm = require('micromatch');
+ * mm.isMatch(string, pattern[, options]);
+ *
+ * console.log(mm.isMatch('a.a', '*.a'));
+ * //=> true
+ * console.log(mm.isMatch('a.b', '*.a'));
+ * //=> false
+ * ```
+ * @param {String} `string` String to match
+ * @param {String} `pattern` Glob pattern to use for matching.
+ * @param {Object} `options` See available [options](#options) for changing how matches are performed
+ * @return {Boolean} Returns true if the string matches the glob pattern.
+ * @api public
+ */
+
+micromatch.isMatch = function(str, pattern, options) {
+ if (typeof str !== 'string') {
+ throw new TypeError('expected a string: "' + util.inspect(str) + '"');
+ }
+
+ if (isEmptyString(str) || isEmptyString(pattern)) {
+ return false;
+ }
+
+ var equals = utils.equalsPattern(options);
+ if (equals(str)) {
+ return true;
+ }
+
+ var isMatch = memoize('isMatch', pattern, options, micromatch.matcher);
+ return isMatch(str);
+};
+
+/**
+ * Returns true if some of the strings in the given `list` match any of the
+ * given glob `patterns`.
+ *
+ * ```js
+ * var mm = require('micromatch');
+ * mm.some(list, patterns[, options]);
+ *
+ * console.log(mm.some(['foo.js', 'bar.js'], ['*.js', '!foo.js']));
+ * // true
+ * console.log(mm.some(['foo.js'], ['*.js', '!foo.js']));
+ * // false
+ * ```
+ * @param {String|Array} `list` The string or array of strings to test. Returns as soon as the first match is found.
+ * @param {String|Array} `patterns` One or more glob patterns to use for matching.
+ * @param {Object} `options` See available [options](#options) for changing how matches are performed
+ * @return {Boolean} Returns true if any patterns match `str`
+ * @api public
+ */
+
+micromatch.some = function(list, patterns, options) {
+ if (typeof list === 'string') {
+ list = [list];
+ }
+ for (var i = 0; i < list.length; i++) {
+ if (micromatch(list[i], patterns, options).length === 1) {
+ return true;
+ }
+ }
+ return false;
+};
+
+/**
+ * Returns true if every string in the given `list` matches
+ * any of the given glob `patterns`.
+ *
+ * ```js
+ * var mm = require('micromatch');
+ * mm.every(list, patterns[, options]);
+ *
+ * console.log(mm.every('foo.js', ['foo.js']));
+ * // true
+ * console.log(mm.every(['foo.js', 'bar.js'], ['*.js']));
+ * // true
+ * console.log(mm.every(['foo.js', 'bar.js'], ['*.js', '!foo.js']));
+ * // false
+ * console.log(mm.every(['foo.js'], ['*.js', '!foo.js']));
+ * // false
+ * ```
+ * @param {String|Array} `list` The string or array of strings to test.
+ * @param {String|Array} `patterns` One or more glob patterns to use for matching.
+ * @param {Object} `options` See available [options](#options) for changing how matches are performed
+ * @return {Boolean} Returns true if any patterns match `str`
+ * @api public
+ */
+
+micromatch.every = function(list, patterns, options) {
+ if (typeof list === 'string') {
+ list = [list];
+ }
+ for (var i = 0; i < list.length; i++) {
+ if (micromatch(list[i], patterns, options).length !== 1) {
+ return false;
+ }
+ }
+ return true;
+};
+
+/**
+ * Returns true if **any** of the given glob `patterns`
+ * match the specified `string`.
+ *
+ * ```js
+ * var mm = require('micromatch');
+ * mm.any(string, patterns[, options]);
+ *
+ * console.log(mm.any('a.a', ['b.*', '*.a']));
+ * //=> true
+ * console.log(mm.any('a.a', 'b.*'));
+ * //=> false
+ * ```
+ * @param {String|Array} `str` The string to test.
+ * @param {String|Array} `patterns` One or more glob patterns to use for matching.
+ * @param {Object} `options` See available [options](#options) for changing how matches are performed
+ * @return {Boolean} Returns true if any patterns match `str`
+ * @api public
+ */
+
+micromatch.any = function(str, patterns, options) {
+ if (typeof str !== 'string') {
+ throw new TypeError('expected a string: "' + util.inspect(str) + '"');
+ }
+
+ if (isEmptyString(str) || isEmptyString(patterns)) {
+ return false;
+ }
+
+ if (typeof patterns === 'string') {
+ patterns = [patterns];
+ }
+
+ for (var i = 0; i < patterns.length; i++) {
+ if (micromatch.isMatch(str, patterns[i], options)) {
+ return true;
+ }
+ }
+ return false;
+};
+
+/**
+ * Returns true if **all** of the given `patterns` match
+ * the specified string.
+ *
+ * ```js
+ * var mm = require('micromatch');
+ * mm.all(string, patterns[, options]);
+ *
+ * console.log(mm.all('foo.js', ['foo.js']));
+ * // true
+ *
+ * console.log(mm.all('foo.js', ['*.js', '!foo.js']));
+ * // false
+ *
+ * console.log(mm.all('foo.js', ['*.js', 'foo.js']));
+ * // true
+ *
+ * console.log(mm.all('foo.js', ['*.js', 'f*', '*o*', '*o.js']));
+ * // true
+ * ```
+ * @param {String|Array} `str` The string to test.
+ * @param {String|Array} `patterns` One or more glob patterns to use for matching.
+ * @param {Object} `options` See available [options](#options) for changing how matches are performed
+ * @return {Boolean} Returns true if any patterns match `str`
+ * @api public
+ */
+
+micromatch.all = function(str, patterns, options) {
+ if (typeof str !== 'string') {
+ throw new TypeError('expected a string: "' + util.inspect(str) + '"');
+ }
+ if (typeof patterns === 'string') {
+ patterns = [patterns];
+ }
+ for (var i = 0; i < patterns.length; i++) {
+ if (!micromatch.isMatch(str, patterns[i], options)) {
+ return false;
+ }
+ }
+ return true;
+};
+
+/**
+ * Returns a list of strings that _**do not match any**_ of the given `patterns`.
+ *
+ * ```js
+ * var mm = require('micromatch');
+ * mm.not(list, patterns[, options]);
+ *
+ * console.log(mm.not(['a.a', 'b.b', 'c.c'], '*.a'));
+ * //=> ['b.b', 'c.c']
+ * ```
+ * @param {Array} `list` Array of strings to match.
+ * @param {String|Array} `patterns` One or more glob pattern to use for matching.
+ * @param {Object} `options` See available [options](#options) for changing how matches are performed
+ * @return {Array} Returns an array of strings that **do not match** the given patterns.
+ * @api public
+ */
+
+micromatch.not = function(list, patterns, options) {
+ var opts = extend({}, options);
+ var ignore = opts.ignore;
+ delete opts.ignore;
+
+ var unixify = utils.unixify(opts);
+ list = utils.arrayify(list).map(unixify);
+
+ var matches = utils.diff(list, micromatch(list, patterns, opts));
+ if (ignore) {
+ matches = utils.diff(matches, micromatch(list, ignore));
+ }
+
+ return opts.nodupes !== false ? utils.unique(matches) : matches;
+};
+
+/**
+ * Returns true if the given `string` contains the given pattern. Similar
+ * to [.isMatch](#isMatch) but the pattern can match any part of the string.
+ *
+ * ```js
+ * var mm = require('micromatch');
+ * mm.contains(string, pattern[, options]);
+ *
+ * console.log(mm.contains('aa/bb/cc', '*b'));
+ * //=> true
+ * console.log(mm.contains('aa/bb/cc', '*d'));
+ * //=> false
+ * ```
+ * @param {String} `str` The string to match.
+ * @param {String|Array} `patterns` Glob pattern to use for matching.
+ * @param {Object} `options` See available [options](#options) for changing how matches are performed
+ * @return {Boolean} Returns true if the patter matches any part of `str`.
+ * @api public
+ */
+
+micromatch.contains = function(str, patterns, options) {
+ if (typeof str !== 'string') {
+ throw new TypeError('expected a string: "' + util.inspect(str) + '"');
+ }
+
+ if (typeof patterns === 'string') {
+ if (isEmptyString(str) || isEmptyString(patterns)) {
+ return false;
+ }
+
+ var equals = utils.equalsPattern(patterns, options);
+ if (equals(str)) {
+ return true;
+ }
+ var contains = utils.containsPattern(patterns, options);
+ if (contains(str)) {
+ return true;
+ }
+ }
+
+ var opts = extend({}, options, {contains: true});
+ return micromatch.any(str, patterns, opts);
+};
+
+/**
+ * Returns true if the given pattern and options should enable
+ * the `matchBase` option.
+ * @return {Boolean}
+ * @api private
+ */
+
+micromatch.matchBase = function(pattern, options) {
+ if (pattern && pattern.indexOf('/') !== -1 || !options) return false;
+ return options.basename === true || options.matchBase === true;
+};
+
+/**
+ * Filter the keys of the given object with the given `glob` pattern
+ * and `options`. Does not attempt to match nested keys. If you need this feature,
+ * use [glob-object][] instead.
+ *
+ * ```js
+ * var mm = require('micromatch');
+ * mm.matchKeys(object, patterns[, options]);
+ *
+ * var obj = { aa: 'a', ab: 'b', ac: 'c' };
+ * console.log(mm.matchKeys(obj, '*b'));
+ * //=> { ab: 'b' }
+ * ```
+ * @param {Object} `object` The object with keys to filter.
+ * @param {String|Array} `patterns` One or more glob patterns to use for matching.
+ * @param {Object} `options` See available [options](#options) for changing how matches are performed
+ * @return {Object} Returns an object with only keys that match the given patterns.
+ * @api public
+ */
+
+micromatch.matchKeys = function(obj, patterns, options) {
+ if (!utils.isObject(obj)) {
+ throw new TypeError('expected the first argument to be an object');
+ }
+ var keys = micromatch(Object.keys(obj), patterns, options);
+ return utils.pick(obj, keys);
+};
+
+/**
+ * Returns a memoized matcher function from the given glob `pattern` and `options`.
+ * The returned function takes a string to match as its only argument and returns
+ * true if the string is a match.
+ *
+ * ```js
+ * var mm = require('micromatch');
+ * mm.matcher(pattern[, options]);
+ *
+ * var isMatch = mm.matcher('*.!(*a)');
+ * console.log(isMatch('a.a'));
+ * //=> false
+ * console.log(isMatch('a.b'));
+ * //=> true
+ * ```
+ * @param {String} `pattern` Glob pattern
+ * @param {Object} `options` See available [options](#options) for changing how matches are performed.
+ * @return {Function} Returns a matcher function.
+ * @api public
+ */
+
+micromatch.matcher = function matcher(pattern, options) {
+ if (Array.isArray(pattern)) {
+ return compose(pattern, options, matcher);
+ }
+
+ // if pattern is a regex
+ if (pattern instanceof RegExp) {
+ return test(pattern);
+ }
+
+ // if pattern is invalid
+ if (!utils.isString(pattern)) {
+ throw new TypeError('expected pattern to be an array, string or regex');
+ }
+
+ // if pattern is a non-glob string
+ if (!utils.hasSpecialChars(pattern)) {
+ if (options && options.nocase === true) {
+ pattern = pattern.toLowerCase();
+ }
+ return utils.matchPath(pattern, options);
+ }
+
+ // if pattern is a glob string
+ var re = micromatch.makeRe(pattern, options);
+
+ // if `options.matchBase` or `options.basename` is defined
+ if (micromatch.matchBase(pattern, options)) {
+ return utils.matchBasename(re, options);
+ }
+
+ function test(regex) {
+ var equals = utils.equalsPattern(options);
+ var unixify = utils.unixify(options);
+
+ return function(str) {
+ if (equals(str)) {
+ return true;
+ }
+
+ if (regex.test(unixify(str))) {
+ return true;
+ }
+ return false;
+ };
+ }
+
+ var fn = test(re);
+ Object.defineProperty(fn, 'result', {
+ configurable: true,
+ enumerable: false,
+ value: re.result
+ });
+ return fn;
+};
+
+/**
+ * Returns an array of matches captured by `pattern` in `string, or `null` if the pattern did not match.
+ *
+ * ```js
+ * var mm = require('micromatch');
+ * mm.capture(pattern, string[, options]);
+ *
+ * console.log(mm.capture('test/*.js', 'test/foo.js'));
+ * //=> ['foo']
+ * console.log(mm.capture('test/*.js', 'foo/bar.css'));
+ * //=> null
+ * ```
+ * @param {String} `pattern` Glob pattern to use for matching.
+ * @param {String} `string` String to match
+ * @param {Object} `options` See available [options](#options) for changing how matches are performed
+ * @return {Boolean} Returns an array of captures if the string matches the glob pattern, otherwise `null`.
+ * @api public
+ */
+
+micromatch.capture = function(pattern, str, options) {
+ var re = micromatch.makeRe(pattern, extend({capture: true}, options));
+ var unixify = utils.unixify(options);
+
+ function match() {
+ return function(string) {
+ var match = re.exec(unixify(string));
+ if (!match) {
+ return null;
+ }
+
+ return match.slice(1);
+ };
+ }
+
+ var capture = memoize('capture', pattern, options, match);
+ return capture(str);
+};
+
+/**
+ * Create a regular expression from the given glob `pattern`.
+ *
+ * ```js
+ * var mm = require('micromatch');
+ * mm.makeRe(pattern[, options]);
+ *
+ * console.log(mm.makeRe('*.js'));
+ * //=> /^(?:(\.[\\\/])?(?!\.)(?=.)[^\/]*?\.js)$/
+ * ```
+ * @param {String} `pattern` A glob pattern to convert to regex.
+ * @param {Object} `options` See available [options](#options) for changing how matches are performed.
+ * @return {RegExp} Returns a regex created from the given pattern.
+ * @api public
+ */
+
+micromatch.makeRe = function(pattern, options) {
+ if (typeof pattern !== 'string') {
+ throw new TypeError('expected pattern to be a string');
+ }
+
+ if (pattern.length > MAX_LENGTH) {
+ throw new Error('expected pattern to be less than ' + MAX_LENGTH + ' characters');
+ }
+
+ function makeRe() {
+ var result = micromatch.create(pattern, options);
+ var ast_array = [];
+ var output = result.map(function(obj) {
+ obj.ast.state = obj.state;
+ ast_array.push(obj.ast);
+ return obj.output;
+ });
+
+ var regex = toRegex(output.join('|'), options);
+ Object.defineProperty(regex, 'result', {
+ configurable: true,
+ enumerable: false,
+ value: ast_array
+ });
+ return regex;
+ }
+
+ return memoize('makeRe', pattern, options, makeRe);
+};
+
+/**
+ * Expand the given brace `pattern`.
+ *
+ * ```js
+ * var mm = require('micromatch');
+ * console.log(mm.braces('foo/{a,b}/bar'));
+ * //=> ['foo/(a|b)/bar']
+ *
+ * console.log(mm.braces('foo/{a,b}/bar', {expand: true}));
+ * //=> ['foo/(a|b)/bar']
+ * ```
+ * @param {String} `pattern` String with brace pattern to expand.
+ * @param {Object} `options` Any [options](#options) to change how expansion is performed. See the [braces][] library for all available options.
+ * @return {Array}
+ * @api public
+ */
+
+micromatch.braces = function(pattern, options) {
+ if (typeof pattern !== 'string' && !Array.isArray(pattern)) {
+ throw new TypeError('expected pattern to be an array or string');
+ }
+
+ function expand() {
+ if (options && options.nobrace === true || !/\{.*\}/.test(pattern)) {
+ return utils.arrayify(pattern);
+ }
+ return braces(pattern, options);
+ }
+
+ return memoize('braces', pattern, options, expand);
+};
+
+/**
+ * Proxy to the [micromatch.braces](#method), for parity with
+ * minimatch.
+ */
+
+micromatch.braceExpand = function(pattern, options) {
+ var opts = extend({}, options, {expand: true});
+ return micromatch.braces(pattern, opts);
+};
+
+/**
+ * Parses the given glob `pattern` and returns an array of abstract syntax
+ * trees (ASTs), with the compiled `output` and optional source `map` on
+ * each AST.
+ *
+ * ```js
+ * var mm = require('micromatch');
+ * mm.create(pattern[, options]);
+ *
+ * console.log(mm.create('abc/*.js'));
+ * // [{ options: { source: 'string', sourcemap: true },
+ * // state: {},
+ * // compilers:
+ * // { ... },
+ * // output: '(\\.[\\\\\\/])?abc\\/(?!\\.)(?=.)[^\\/]*?\\.js',
+ * // ast:
+ * // { type: 'root',
+ * // errors: [],
+ * // nodes:
+ * // [ ... ],
+ * // dot: false,
+ * // input: 'abc/*.js' },
+ * // parsingErrors: [],
+ * // map:
+ * // { version: 3,
+ * // sources: [ 'string' ],
+ * // names: [],
+ * // mappings: 'AAAA,GAAG,EAAC,kBAAC,EAAC,EAAE',
+ * // sourcesContent: [ 'abc/*.js' ] },
+ * // position: { line: 1, column: 28 },
+ * // content: {},
+ * // files: {},
+ * // idx: 6 }]
+ * ```
+ * @param {String} `pattern` Glob pattern to parse and compile.
+ * @param {Object} `options` Any [options](#options) to change how parsing and compiling is performed.
+ * @return {Object} Returns an object with the parsed AST, compiled string and optional source map.
+ * @api public
+ */
+
+micromatch.create = function(pattern, options) {
+ return memoize('create', pattern, options, function() {
+ function create(str, opts) {
+ return micromatch.compile(micromatch.parse(str, opts), opts);
+ }
+
+ pattern = micromatch.braces(pattern, options);
+ var len = pattern.length;
+ var idx = -1;
+ var res = [];
+
+ while (++idx < len) {
+ res.push(create(pattern[idx], options));
+ }
+ return res;
+ });
+};
+
+/**
+ * Parse the given `str` with the given `options`.
+ *
+ * ```js
+ * var mm = require('micromatch');
+ * mm.parse(pattern[, options]);
+ *
+ * var ast = mm.parse('a/{b,c}/d');
+ * console.log(ast);
+ * // { type: 'root',
+ * // errors: [],
+ * // input: 'a/{b,c}/d',
+ * // nodes:
+ * // [ { type: 'bos', val: '' },
+ * // { type: 'text', val: 'a/' },
+ * // { type: 'brace',
+ * // nodes:
+ * // [ { type: 'brace.open', val: '{' },
+ * // { type: 'text', val: 'b,c' },
+ * // { type: 'brace.close', val: '}' } ] },
+ * // { type: 'text', val: '/d' },
+ * // { type: 'eos', val: '' } ] }
+ * ```
+ * @param {String} `str`
+ * @param {Object} `options`
+ * @return {Object} Returns an AST
+ * @api public
+ */
+
+micromatch.parse = function(pattern, options) {
+ if (typeof pattern !== 'string') {
+ throw new TypeError('expected a string');
+ }
+
+ function parse() {
+ var snapdragon = utils.instantiate(null, options);
+ parsers(snapdragon, options);
+
+ var ast = snapdragon.parse(pattern, options);
+ utils.define(ast, 'snapdragon', snapdragon);
+ ast.input = pattern;
+ return ast;
+ }
+
+ return memoize('parse', pattern, options, parse);
+};
+
+/**
+ * Compile the given `ast` or string with the given `options`.
+ *
+ * ```js
+ * var mm = require('micromatch');
+ * mm.compile(ast[, options]);
+ *
+ * var ast = mm.parse('a/{b,c}/d');
+ * console.log(mm.compile(ast));
+ * // { options: { source: 'string' },
+ * // state: {},
+ * // compilers:
+ * // { eos: [Function],
+ * // noop: [Function],
+ * // bos: [Function],
+ * // brace: [Function],
+ * // 'brace.open': [Function],
+ * // text: [Function],
+ * // 'brace.close': [Function] },
+ * // output: [ 'a/(b|c)/d' ],
+ * // ast:
+ * // { ... },
+ * // parsingErrors: [] }
+ * ```
+ * @param {Object|String} `ast`
+ * @param {Object} `options`
+ * @return {Object} Returns an object that has an `output` property with the compiled string.
+ * @api public
+ */
+
+micromatch.compile = function(ast, options) {
+ if (typeof ast === 'string') {
+ ast = micromatch.parse(ast, options);
+ }
+
+ return memoize('compile', ast.input, options, function() {
+ var snapdragon = utils.instantiate(ast, options);
+ compilers(snapdragon, options);
+ return snapdragon.compile(ast, options);
+ });
+};
+
+/**
+ * Clear the regex cache.
+ *
+ * ```js
+ * mm.clearCache();
+ * ```
+ * @api public
+ */
+
+micromatch.clearCache = function() {
+ micromatch.cache.caches = {};
+};
+
+/**
+ * Returns true if the given value is effectively an empty string
+ */
+
+function isEmptyString(val) {
+ return String(val) === '' || String(val) === './';
+}
+
+/**
+ * Compose a matcher function with the given patterns.
+ * This allows matcher functions to be compiled once and
+ * called multiple times.
+ */
+
+function compose(patterns, options, matcher) {
+ var matchers;
+
+ return memoize('compose', String(patterns), options, function() {
+ return function(file) {
+ // delay composition until it's invoked the first time,
+ // after that it won't be called again
+ if (!matchers) {
+ matchers = [];
+ for (var i = 0; i < patterns.length; i++) {
+ matchers.push(matcher(patterns[i], options));
+ }
+ }
+
+ var len = matchers.length;
+ while (len--) {
+ if (matchers[len](file) === true) {
+ return true;
+ }
+ }
+ return false;
+ };
+ });
+}
+
+/**
+ * Memoize a generated regex or function. A unique key is generated
+ * from the `type` (usually method name), the `pattern`, and
+ * user-defined options.
+ */
+
+function memoize(type, pattern, options, fn) {
+ var key = utils.createKey(type + '=' + pattern, options);
+
+ if (options && options.cache === false) {
+ return fn(pattern, options);
+ }
+
+ if (cache.has(type, key)) {
+ return cache.get(type, key);
+ }
+
+ var val = fn(pattern, options);
+ cache.set(type, key, val);
+ return val;
+}
+
+/**
+ * Expose compiler, parser and cache on `micromatch`
+ */
+
+micromatch.compilers = compilers;
+micromatch.parsers = parsers;
+micromatch.caches = cache.caches;
+
+/**
+ * Expose `micromatch`
+ * @type {Function}
+ */
+
+module.exports = micromatch;
diff --git a/node_modules/micromatch/lib/cache.js b/node_modules/micromatch/lib/cache.js
new file mode 100644
index 0000000..fffc4c1
--- /dev/null
+++ b/node_modules/micromatch/lib/cache.js
@@ -0,0 +1 @@
+module.exports = new (require('fragment-cache'))();
diff --git a/node_modules/micromatch/lib/compilers.js b/node_modules/micromatch/lib/compilers.js
new file mode 100644
index 0000000..85cda4f
--- /dev/null
+++ b/node_modules/micromatch/lib/compilers.js
@@ -0,0 +1,77 @@
+'use strict';
+
+var nanomatch = require('nanomatch');
+var extglob = require('extglob');
+
+module.exports = function(snapdragon) {
+ var compilers = snapdragon.compiler.compilers;
+ var opts = snapdragon.options;
+
+ // register nanomatch compilers
+ snapdragon.use(nanomatch.compilers);
+
+ // get references to some specific nanomatch compilers before they
+ // are overridden by the extglob and/or custom compilers
+ var escape = compilers.escape;
+ var qmark = compilers.qmark;
+ var slash = compilers.slash;
+ var star = compilers.star;
+ var text = compilers.text;
+ var plus = compilers.plus;
+ var dot = compilers.dot;
+
+ // register extglob compilers or escape exglobs if disabled
+ if (opts.extglob === false || opts.noext === true) {
+ snapdragon.compiler.use(escapeExtglobs);
+ } else {
+ snapdragon.use(extglob.compilers);
+ }
+
+ snapdragon.use(function() {
+ this.options.star = this.options.star || function(/*node*/) {
+ return '[^\\\\/]*?';
+ };
+ });
+
+ // custom micromatch compilers
+ snapdragon.compiler
+
+ // reset referenced compiler
+ .set('dot', dot)
+ .set('escape', escape)
+ .set('plus', plus)
+ .set('slash', slash)
+ .set('qmark', qmark)
+ .set('star', star)
+ .set('text', text);
+};
+
+function escapeExtglobs(compiler) {
+ compiler.set('paren', function(node) {
+ var val = '';
+ visit(node, function(tok) {
+ if (tok.val) val += (/^\W/.test(tok.val) ? '\\' : '') + tok.val;
+ });
+ return this.emit(val, node);
+ });
+
+ /**
+ * Visit `node` with the given `fn`
+ */
+
+ function visit(node, fn) {
+ return node.nodes ? mapVisit(node.nodes, fn) : fn(node);
+ }
+
+ /**
+ * Map visit over array of `nodes`.
+ */
+
+ function mapVisit(nodes, fn) {
+ var len = nodes.length;
+ var idx = -1;
+ while (++idx < len) {
+ visit(nodes[idx], fn);
+ }
+ }
+}
diff --git a/node_modules/micromatch/lib/parsers.js b/node_modules/micromatch/lib/parsers.js
new file mode 100644
index 0000000..f80498c
--- /dev/null
+++ b/node_modules/micromatch/lib/parsers.js
@@ -0,0 +1,83 @@
+'use strict';
+
+var extglob = require('extglob');
+var nanomatch = require('nanomatch');
+var regexNot = require('regex-not');
+var toRegex = require('to-regex');
+var not;
+
+/**
+ * Characters to use in negation regex (we want to "not" match
+ * characters that are matched by other parsers)
+ */
+
+var TEXT = '([!@*?+]?\\(|\\)|\\[:?(?=.*?:?\\])|:?\\]|[*+?!^$.\\\\/])+';
+var createNotRegex = function(opts) {
+ return not || (not = textRegex(TEXT));
+};
+
+/**
+ * Parsers
+ */
+
+module.exports = function(snapdragon) {
+ var parsers = snapdragon.parser.parsers;
+
+ // register nanomatch parsers
+ snapdragon.use(nanomatch.parsers);
+
+ // get references to some specific nanomatch parsers before they
+ // are overridden by the extglob and/or parsers
+ var escape = parsers.escape;
+ var slash = parsers.slash;
+ var qmark = parsers.qmark;
+ var plus = parsers.plus;
+ var star = parsers.star;
+ var dot = parsers.dot;
+
+ // register extglob parsers
+ snapdragon.use(extglob.parsers);
+
+ // custom micromatch parsers
+ snapdragon.parser
+ .use(function() {
+ // override "notRegex" created in nanomatch parser
+ this.notRegex = /^\!+(?!\()/;
+ })
+ // reset the referenced parsers
+ .capture('escape', escape)
+ .capture('slash', slash)
+ .capture('qmark', qmark)
+ .capture('star', star)
+ .capture('plus', plus)
+ .capture('dot', dot)
+
+ /**
+ * Override `text` parser
+ */
+
+ .capture('text', function() {
+ if (this.isInside('bracket')) return;
+ var pos = this.position();
+ var m = this.match(createNotRegex(this.options));
+ if (!m || !m[0]) return;
+
+ // escape regex boundary characters and simple brackets
+ var val = m[0].replace(/([[\]^$])/g, '\\$1');
+
+ return pos({
+ type: 'text',
+ val: val
+ });
+ });
+};
+
+/**
+ * Create text regex
+ */
+
+function textRegex(pattern) {
+ var notStr = regexNot.create(pattern, {contains: true, strictClose: false});
+ var prefix = '(?:[\\^]|\\\\|';
+ return toRegex(prefix + notStr + ')', {strictClose: false});
+}
diff --git a/node_modules/micromatch/lib/utils.js b/node_modules/micromatch/lib/utils.js
new file mode 100644
index 0000000..f0ba917
--- /dev/null
+++ b/node_modules/micromatch/lib/utils.js
@@ -0,0 +1,309 @@
+'use strict';
+
+var utils = module.exports;
+var path = require('path');
+
+/**
+ * Module dependencies
+ */
+
+var Snapdragon = require('snapdragon');
+utils.define = require('define-property');
+utils.diff = require('arr-diff');
+utils.extend = require('extend-shallow');
+utils.pick = require('object.pick');
+utils.typeOf = require('kind-of');
+utils.unique = require('array-unique');
+
+/**
+ * Returns true if the platform is windows, or `path.sep` is `\\`.
+ * This is defined as a function to allow `path.sep` to be set in unit tests,
+ * or by the user, if there is a reason to do so.
+ * @return {Boolean}
+ */
+
+utils.isWindows = function() {
+ return path.sep === '\\' || process.platform === 'win32';
+};
+
+/**
+ * Get the `Snapdragon` instance to use
+ */
+
+utils.instantiate = function(ast, options) {
+ var snapdragon;
+ // if an instance was created by `.parse`, use that instance
+ if (utils.typeOf(ast) === 'object' && ast.snapdragon) {
+ snapdragon = ast.snapdragon;
+ // if the user supplies an instance on options, use that instance
+ } else if (utils.typeOf(options) === 'object' && options.snapdragon) {
+ snapdragon = options.snapdragon;
+ // create a new instance
+ } else {
+ snapdragon = new Snapdragon(options);
+ }
+
+ utils.define(snapdragon, 'parse', function(str, options) {
+ var parsed = Snapdragon.prototype.parse.apply(this, arguments);
+ parsed.input = str;
+
+ // escape unmatched brace/bracket/parens
+ var last = this.parser.stack.pop();
+ if (last && this.options.strictErrors !== true) {
+ var open = last.nodes[0];
+ var inner = last.nodes[1];
+ if (last.type === 'bracket') {
+ if (inner.val.charAt(0) === '[') {
+ inner.val = '\\' + inner.val;
+ }
+
+ } else {
+ open.val = '\\' + open.val;
+ var sibling = open.parent.nodes[1];
+ if (sibling.type === 'star') {
+ sibling.loose = true;
+ }
+ }
+ }
+
+ // add non-enumerable parser reference
+ utils.define(parsed, 'parser', this.parser);
+ return parsed;
+ });
+
+ return snapdragon;
+};
+
+/**
+ * Create the key to use for memoization. The key is generated
+ * by iterating over the options and concatenating key-value pairs
+ * to the pattern string.
+ */
+
+utils.createKey = function(pattern, options) {
+ if (utils.typeOf(options) !== 'object') {
+ return pattern;
+ }
+ var val = pattern;
+ var keys = Object.keys(options);
+ for (var i = 0; i < keys.length; i++) {
+ var key = keys[i];
+ val += ';' + key + '=' + String(options[key]);
+ }
+ return val;
+};
+
+/**
+ * Cast `val` to an array
+ * @return {Array}
+ */
+
+utils.arrayify = function(val) {
+ if (typeof val === 'string') return [val];
+ return val ? (Array.isArray(val) ? val : [val]) : [];
+};
+
+/**
+ * Return true if `val` is a non-empty string
+ */
+
+utils.isString = function(val) {
+ return typeof val === 'string';
+};
+
+/**
+ * Return true if `val` is a non-empty string
+ */
+
+utils.isObject = function(val) {
+ return utils.typeOf(val) === 'object';
+};
+
+/**
+ * Returns true if the given `str` has special characters
+ */
+
+utils.hasSpecialChars = function(str) {
+ return /(?:(?:(^|\/)[!.])|[*?+()|\[\]{}]|[+@]\()/.test(str);
+};
+
+/**
+ * Escape regex characters in the given string
+ */
+
+utils.escapeRegex = function(str) {
+ return str.replace(/[-[\]{}()^$|*+?.\\\/\s]/g, '\\$&');
+};
+
+/**
+ * Normalize slashes in the given filepath.
+ *
+ * @param {String} `filepath`
+ * @return {String}
+ */
+
+utils.toPosixPath = function(str) {
+ return str.replace(/\\+/g, '/');
+};
+
+/**
+ * Strip backslashes before special characters in a string.
+ *
+ * @param {String} `str`
+ * @return {String}
+ */
+
+utils.unescape = function(str) {
+ return utils.toPosixPath(str.replace(/\\(?=[*+?!.])/g, ''));
+};
+
+/**
+ * Strip the prefix from a filepath
+ * @param {String} `fp`
+ * @return {String}
+ */
+
+utils.stripPrefix = function(str) {
+ if (str.charAt(0) !== '.') {
+ return str;
+ }
+ var ch = str.charAt(1);
+ if (utils.isSlash(ch)) {
+ return str.slice(2);
+ }
+ return str;
+};
+
+/**
+ * Returns true if the given str is an escaped or
+ * unescaped path character
+ */
+
+utils.isSlash = function(str) {
+ return str === '/' || str === '\\/' || str === '\\' || str === '\\\\';
+};
+
+/**
+ * Returns a function that returns true if the given
+ * pattern matches or contains a `filepath`
+ *
+ * @param {String} `pattern`
+ * @return {Function}
+ */
+
+utils.matchPath = function(pattern, options) {
+ return (options && options.contains)
+ ? utils.containsPattern(pattern, options)
+ : utils.equalsPattern(pattern, options);
+};
+
+/**
+ * Returns true if the given (original) filepath or unixified path are equal
+ * to the given pattern.
+ */
+
+utils._equals = function(filepath, unixPath, pattern) {
+ return pattern === filepath || pattern === unixPath;
+};
+
+/**
+ * Returns true if the given (original) filepath or unixified path contain
+ * the given pattern.
+ */
+
+utils._contains = function(filepath, unixPath, pattern) {
+ return filepath.indexOf(pattern) !== -1 || unixPath.indexOf(pattern) !== -1;
+};
+
+/**
+ * Returns a function that returns true if the given
+ * pattern is the same as a given `filepath`
+ *
+ * @param {String} `pattern`
+ * @return {Function}
+ */
+
+utils.equalsPattern = function(pattern, options) {
+ var unixify = utils.unixify(options);
+ options = options || {};
+
+ return function fn(filepath) {
+ var equal = utils._equals(filepath, unixify(filepath), pattern);
+ if (equal === true || options.nocase !== true) {
+ return equal;
+ }
+ var lower = filepath.toLowerCase();
+ return utils._equals(lower, unixify(lower), pattern);
+ };
+};
+
+/**
+ * Returns a function that returns true if the given
+ * pattern contains a `filepath`
+ *
+ * @param {String} `pattern`
+ * @return {Function}
+ */
+
+utils.containsPattern = function(pattern, options) {
+ var unixify = utils.unixify(options);
+ options = options || {};
+
+ return function(filepath) {
+ var contains = utils._contains(filepath, unixify(filepath), pattern);
+ if (contains === true || options.nocase !== true) {
+ return contains;
+ }
+ var lower = filepath.toLowerCase();
+ return utils._contains(lower, unixify(lower), pattern);
+ };
+};
+
+/**
+ * Returns a function that returns true if the given
+ * regex matches the `filename` of a file path.
+ *
+ * @param {RegExp} `re` Matching regex
+ * @return {Function}
+ */
+
+utils.matchBasename = function(re) {
+ return function(filepath) {
+ return re.test(path.basename(filepath));
+ };
+};
+
+/**
+ * Determines the filepath to return based on the provided options.
+ * @return {any}
+ */
+
+utils.value = function(str, unixify, options) {
+ if (options && options.unixify === false) {
+ return str;
+ }
+ return unixify(str);
+};
+
+/**
+ * Returns a function that normalizes slashes in a string to forward
+ * slashes, strips `./` from beginning of paths, and optionally unescapes
+ * special characters.
+ * @return {Function}
+ */
+
+utils.unixify = function(options) {
+ options = options || {};
+ return function(filepath) {
+ if (utils.isWindows() || options.unixify === true) {
+ filepath = utils.toPosixPath(filepath);
+ }
+ if (options.stripPrefix !== false) {
+ filepath = utils.stripPrefix(filepath);
+ }
+ if (options.unescape === true) {
+ filepath = utils.unescape(filepath);
+ }
+ return filepath;
+ };
+};
diff --git a/node_modules/micromatch/package.json b/node_modules/micromatch/package.json
new file mode 100644
index 0000000..97d1349
--- /dev/null
+++ b/node_modules/micromatch/package.json
@@ -0,0 +1,216 @@
+{
+ "_from": "micromatch@^3.0.4",
+ "_id": "micromatch@3.1.10",
+ "_inBundle": false,
+ "_integrity": "sha512-MWikgl9n9M3w+bpsY3He8L+w9eF9338xRl8IAO5viDizwSzziFEyUzo2xrrloB64ADbTf8uA8vRqqttDTOmccg==",
+ "_location": "/micromatch",
+ "_phantomChildren": {},
+ "_requested": {
+ "type": "range",
+ "registry": true,
+ "raw": "micromatch@^3.0.4",
+ "name": "micromatch",
+ "escapedName": "micromatch",
+ "rawSpec": "^3.0.4",
+ "saveSpec": null,
+ "fetchSpec": "^3.0.4"
+ },
+ "_requiredBy": [
+ "/findup-sync"
+ ],
+ "_resolved": "https://registry.npmjs.org/micromatch/-/micromatch-3.1.10.tgz",
+ "_shasum": "70859bc95c9840952f359a068a3fc49f9ecfac23",
+ "_spec": "micromatch@^3.0.4",
+ "_where": "/Users/rpatra16/MyProjects/RamAndAntara/node_modules/findup-sync",
+ "author": {
+ "name": "Jon Schlinkert",
+ "url": "https://github.com/jonschlinkert"
+ },
+ "bugs": {
+ "url": "https://github.com/micromatch/micromatch/issues"
+ },
+ "bundleDependencies": false,
+ "contributors": [
+ {
+ "name": "Amila Welihinda",
+ "url": "amilajack.com"
+ },
+ {
+ "name": "Bogdan Chadkin",
+ "url": "https://github.com/TrySound"
+ },
+ {
+ "name": "Brian Woodward",
+ "url": "https://twitter.com/doowb"
+ },
+ {
+ "name": "Devon Govett",
+ "url": "http://badassjs.com"
+ },
+ {
+ "name": "Elan Shanker",
+ "url": "https://github.com/es128"
+ },
+ {
+ "name": "Fabrício Matté",
+ "url": "https://ultcombo.js.org"
+ },
+ {
+ "name": "Jon Schlinkert",
+ "url": "http://twitter.com/jonschlinkert"
+ },
+ {
+ "name": "Martin Kolárik",
+ "url": "https://kolarik.sk"
+ },
+ {
+ "name": "Olsten Larck",
+ "url": "https://i.am.charlike.online"
+ },
+ {
+ "name": "Paul Miller",
+ "url": "paulmillr.com"
+ },
+ {
+ "name": "Tom Byrer",
+ "url": "https://github.com/tomByrer"
+ },
+ {
+ "name": "Tyler Akins",
+ "url": "http://rumkin.com"
+ },
+ {
+ "url": "https://github.com/DianeLooney"
+ }
+ ],
+ "dependencies": {
+ "arr-diff": "^4.0.0",
+ "array-unique": "^0.3.2",
+ "braces": "^2.3.1",
+ "define-property": "^2.0.2",
+ "extend-shallow": "^3.0.2",
+ "extglob": "^2.0.4",
+ "fragment-cache": "^0.2.1",
+ "kind-of": "^6.0.2",
+ "nanomatch": "^1.2.9",
+ "object.pick": "^1.3.0",
+ "regex-not": "^1.0.0",
+ "snapdragon": "^0.8.1",
+ "to-regex": "^3.0.2"
+ },
+ "deprecated": false,
+ "description": "Glob matching for javascript/node.js. A drop-in replacement and faster alternative to minimatch and multimatch.",
+ "devDependencies": {
+ "bash-match": "^1.0.2",
+ "for-own": "^1.0.0",
+ "gulp": "^3.9.1",
+ "gulp-format-md": "^1.0.0",
+ "gulp-istanbul": "^1.1.3",
+ "gulp-mocha": "^5.0.0",
+ "gulp-unused": "^0.2.1",
+ "is-windows": "^1.0.2",
+ "minimatch": "^3.0.4",
+ "minimist": "^1.2.0",
+ "mocha": "^3.5.3",
+ "multimatch": "^2.1.0"
+ },
+ "engines": {
+ "node": ">=0.10.0"
+ },
+ "files": [
+ "index.js",
+ "lib"
+ ],
+ "homepage": "https://github.com/micromatch/micromatch",
+ "keywords": [
+ "bash",
+ "expand",
+ "expansion",
+ "expression",
+ "file",
+ "files",
+ "filter",
+ "find",
+ "glob",
+ "globbing",
+ "globs",
+ "globstar",
+ "match",
+ "matcher",
+ "matches",
+ "matching",
+ "micromatch",
+ "minimatch",
+ "multimatch",
+ "path",
+ "pattern",
+ "patterns",
+ "regex",
+ "regexp",
+ "regular",
+ "shell",
+ "wildcard"
+ ],
+ "license": "MIT",
+ "lintDeps": {
+ "dependencies": {
+ "options": {
+ "lock": {
+ "snapdragon": "^0.8.1"
+ }
+ }
+ },
+ "devDependencies": {
+ "files": {
+ "options": {
+ "ignore": [
+ "benchmark/**"
+ ]
+ }
+ }
+ }
+ },
+ "main": "index.js",
+ "name": "micromatch",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/micromatch/micromatch.git"
+ },
+ "scripts": {
+ "test": "mocha"
+ },
+ "verb": {
+ "toc": "collapsible",
+ "layout": "default",
+ "tasks": [
+ "readme"
+ ],
+ "plugins": [
+ "gulp-format-md"
+ ],
+ "helpers": [
+ "./benchmark/helper.js"
+ ],
+ "related": {
+ "list": [
+ "braces",
+ "expand-brackets",
+ "extglob",
+ "fill-range",
+ "nanomatch"
+ ]
+ },
+ "lint": {
+ "reflinks": true
+ },
+ "reflinks": [
+ "expand-brackets",
+ "extglob",
+ "glob-object",
+ "minimatch",
+ "multimatch",
+ "snapdragon"
+ ]
+ },
+ "version": "3.1.10"
+}
diff --git a/node_modules/mime-db/HISTORY.md b/node_modules/mime-db/HISTORY.md
new file mode 100644
index 0000000..d454eb3
--- /dev/null
+++ b/node_modules/mime-db/HISTORY.md
@@ -0,0 +1,397 @@
+1.37.0 / 2018-10-19
+===================
+
+ * Add extensions to HEIC image types
+ * Add new upstream MIME types
+
+1.36.0 / 2018-08-20
+===================
+
+ * Add Apple file extensions from IANA
+ * Add extensions from IANA for `image/*` types
+ * Add new upstream MIME types
+
+1.35.0 / 2018-07-15
+===================
+
+ * Add extension `.owl` to `application/rdf+xml`
+ * Add new upstream MIME types
+ - Removes extension `.woff` from `application/font-woff`
+
+1.34.0 / 2018-06-03
+===================
+
+ * Add extension `.csl` to `application/vnd.citationstyles.style+xml`
+ * Add extension `.es` to `application/ecmascript`
+ * Add new upstream MIME types
+ * Add `UTF-8` as default charset for `text/turtle`
+ * Mark all XML-derived types as compressible
+
+1.33.0 / 2018-02-15
+===================
+
+ * Add extensions from IANA for `message/*` types
+ * Add new upstream MIME types
+ * Fix some incorrect OOXML types
+ * Remove `application/font-woff2`
+
+1.32.0 / 2017-11-29
+===================
+
+ * Add new upstream MIME types
+ * Update `text/hjson` to registered `application/hjson`
+ * Add `text/shex` with extension `.shex`
+
+1.31.0 / 2017-10-25
+===================
+
+ * Add `application/raml+yaml` with extension `.raml`
+ * Add `application/wasm` with extension `.wasm`
+ * Add new `font` type from IANA
+ * Add new upstream font extensions
+ * Add new upstream MIME types
+ * Add extensions for JPEG-2000 images
+
+1.30.0 / 2017-08-27
+===================
+
+ * Add `application/vnd.ms-outlook`
+ * Add `application/x-arj`
+ * Add extension `.mjs` to `application/javascript`
+ * Add glTF types and extensions
+ * Add new upstream MIME types
+ * Add `text/x-org`
+ * Add VirtualBox MIME types
+ * Fix `source` records for `video/*` types that are IANA
+ * Update `font/opentype` to registered `font/otf`
+
+1.29.0 / 2017-07-10
+===================
+
+ * Add `application/fido.trusted-apps+json`
+ * Add extension `.wadl` to `application/vnd.sun.wadl+xml`
+ * Add new upstream MIME types
+ * Add `UTF-8` as default charset for `text/css`
+
+1.28.0 / 2017-05-14
+===================
+
+ * Add new upstream MIME types
+ * Add extension `.gz` to `application/gzip`
+ * Update extensions `.md` and `.markdown` to be `text/markdown`
+
+1.27.0 / 2017-03-16
+===================
+
+ * Add new upstream MIME types
+ * Add `image/apng` with extension `.apng`
+
+1.26.0 / 2017-01-14
+===================
+
+ * Add new upstream MIME types
+ * Add extension `.geojson` to `application/geo+json`
+
+1.25.0 / 2016-11-11
+===================
+
+ * Add new upstream MIME types
+
+1.24.0 / 2016-09-18
+===================
+
+ * Add `audio/mp3`
+ * Add new upstream MIME types
+
+1.23.0 / 2016-05-01
+===================
+
+ * Add new upstream MIME types
+ * Add extension `.3gpp` to `audio/3gpp`
+
+1.22.0 / 2016-02-15
+===================
+
+ * Add `text/slim`
+ * Add extension `.rng` to `application/xml`
+ * Add new upstream MIME types
+ * Fix extension of `application/dash+xml` to be `.mpd`
+ * Update primary extension to `.m4a` for `audio/mp4`
+
+1.21.0 / 2016-01-06
+===================
+
+ * Add Google document types
+ * Add new upstream MIME types
+
+1.20.0 / 2015-11-10
+===================
+
+ * Add `text/x-suse-ymp`
+ * Add new upstream MIME types
+
+1.19.0 / 2015-09-17
+===================
+
+ * Add `application/vnd.apple.pkpass`
+ * Add new upstream MIME types
+
+1.18.0 / 2015-09-03
+===================
+
+ * Add new upstream MIME types
+
+1.17.0 / 2015-08-13
+===================
+
+ * Add `application/x-msdos-program`
+ * Add `audio/g711-0`
+ * Add `image/vnd.mozilla.apng`
+ * Add extension `.exe` to `application/x-msdos-program`
+
+1.16.0 / 2015-07-29
+===================
+
+ * Add `application/vnd.uri-map`
+
+1.15.0 / 2015-07-13
+===================
+
+ * Add `application/x-httpd-php`
+
+1.14.0 / 2015-06-25
+===================
+
+ * Add `application/scim+json`
+ * Add `application/vnd.3gpp.ussd+xml`
+ * Add `application/vnd.biopax.rdf+xml`
+ * Add `text/x-processing`
+
+1.13.0 / 2015-06-07
+===================
+
+ * Add nginx as a source
+ * Add `application/x-cocoa`
+ * Add `application/x-java-archive-diff`
+ * Add `application/x-makeself`
+ * Add `application/x-perl`
+ * Add `application/x-pilot`
+ * Add `application/x-redhat-package-manager`
+ * Add `application/x-sea`
+ * Add `audio/x-m4a`
+ * Add `audio/x-realaudio`
+ * Add `image/x-jng`
+ * Add `text/mathml`
+
+1.12.0 / 2015-06-05
+===================
+
+ * Add `application/bdoc`
+ * Add `application/vnd.hyperdrive+json`
+ * Add `application/x-bdoc`
+ * Add extension `.rtf` to `text/rtf`
+
+1.11.0 / 2015-05-31
+===================
+
+ * Add `audio/wav`
+ * Add `audio/wave`
+ * Add extension `.litcoffee` to `text/coffeescript`
+ * Add extension `.sfd-hdstx` to `application/vnd.hydrostatix.sof-data`
+ * Add extension `.n-gage` to `application/vnd.nokia.n-gage.symbian.install`
+
+1.10.0 / 2015-05-19
+===================
+
+ * Add `application/vnd.balsamiq.bmpr`
+ * Add `application/vnd.microsoft.portable-executable`
+ * Add `application/x-ns-proxy-autoconfig`
+
+1.9.1 / 2015-04-19
+==================
+
+ * Remove `.json` extension from `application/manifest+json`
+ - This is causing bugs downstream
+
+1.9.0 / 2015-04-19
+==================
+
+ * Add `application/manifest+json`
+ * Add `application/vnd.micro+json`
+ * Add `image/vnd.zbrush.pcx`
+ * Add `image/x-ms-bmp`
+
+1.8.0 / 2015-03-13
+==================
+
+ * Add `application/vnd.citationstyles.style+xml`
+ * Add `application/vnd.fastcopy-disk-image`
+ * Add `application/vnd.gov.sk.xmldatacontainer+xml`
+ * Add extension `.jsonld` to `application/ld+json`
+
+1.7.0 / 2015-02-08
+==================
+
+ * Add `application/vnd.gerber`
+ * Add `application/vnd.msa-disk-image`
+
+1.6.1 / 2015-02-05
+==================
+
+ * Community extensions ownership transferred from `node-mime`
+
+1.6.0 / 2015-01-29
+==================
+
+ * Add `application/jose`
+ * Add `application/jose+json`
+ * Add `application/json-seq`
+ * Add `application/jwk+json`
+ * Add `application/jwk-set+json`
+ * Add `application/jwt`
+ * Add `application/rdap+json`
+ * Add `application/vnd.gov.sk.e-form+xml`
+ * Add `application/vnd.ims.imsccv1p3`
+
+1.5.0 / 2014-12-30
+==================
+
+ * Add `application/vnd.oracle.resource+json`
+ * Fix various invalid MIME type entries
+ - `application/mbox+xml`
+ - `application/oscp-response`
+ - `application/vwg-multiplexed`
+ - `audio/g721`
+
+1.4.0 / 2014-12-21
+==================
+
+ * Add `application/vnd.ims.imsccv1p2`
+ * Fix various invalid MIME type entries
+ - `application/vnd-acucobol`
+ - `application/vnd-curl`
+ - `application/vnd-dart`
+ - `application/vnd-dxr`
+ - `application/vnd-fdf`
+ - `application/vnd-mif`
+ - `application/vnd-sema`
+ - `application/vnd-wap-wmlc`
+ - `application/vnd.adobe.flash-movie`
+ - `application/vnd.dece-zip`
+ - `application/vnd.dvb_service`
+ - `application/vnd.micrografx-igx`
+ - `application/vnd.sealed-doc`
+ - `application/vnd.sealed-eml`
+ - `application/vnd.sealed-mht`
+ - `application/vnd.sealed-ppt`
+ - `application/vnd.sealed-tiff`
+ - `application/vnd.sealed-xls`
+ - `application/vnd.sealedmedia.softseal-html`
+ - `application/vnd.sealedmedia.softseal-pdf`
+ - `application/vnd.wap-slc`
+ - `application/vnd.wap-wbxml`
+ - `audio/vnd.sealedmedia.softseal-mpeg`
+ - `image/vnd-djvu`
+ - `image/vnd-svf`
+ - `image/vnd-wap-wbmp`
+ - `image/vnd.sealed-png`
+ - `image/vnd.sealedmedia.softseal-gif`
+ - `image/vnd.sealedmedia.softseal-jpg`
+ - `model/vnd-dwf`
+ - `model/vnd.parasolid.transmit-binary`
+ - `model/vnd.parasolid.transmit-text`
+ - `text/vnd-a`
+ - `text/vnd-curl`
+ - `text/vnd.wap-wml`
+ * Remove example template MIME types
+ - `application/example`
+ - `audio/example`
+ - `image/example`
+ - `message/example`
+ - `model/example`
+ - `multipart/example`
+ - `text/example`
+ - `video/example`
+
+1.3.1 / 2014-12-16
+==================
+
+ * Fix missing extensions
+ - `application/json5`
+ - `text/hjson`
+
+1.3.0 / 2014-12-07
+==================
+
+ * Add `application/a2l`
+ * Add `application/aml`
+ * Add `application/atfx`
+ * Add `application/atxml`
+ * Add `application/cdfx+xml`
+ * Add `application/dii`
+ * Add `application/json5`
+ * Add `application/lxf`
+ * Add `application/mf4`
+ * Add `application/vnd.apache.thrift.compact`
+ * Add `application/vnd.apache.thrift.json`
+ * Add `application/vnd.coffeescript`
+ * Add `application/vnd.enphase.envoy`
+ * Add `application/vnd.ims.imsccv1p1`
+ * Add `text/csv-schema`
+ * Add `text/hjson`
+ * Add `text/markdown`
+ * Add `text/yaml`
+
+1.2.0 / 2014-11-09
+==================
+
+ * Add `application/cea`
+ * Add `application/dit`
+ * Add `application/vnd.gov.sk.e-form+zip`
+ * Add `application/vnd.tmd.mediaflex.api+xml`
+ * Type `application/epub+zip` is now IANA-registered
+
+1.1.2 / 2014-10-23
+==================
+
+ * Rebuild database for `application/x-www-form-urlencoded` change
+
+1.1.1 / 2014-10-20
+==================
+
+ * Mark `application/x-www-form-urlencoded` as compressible.
+
+1.1.0 / 2014-09-28
+==================
+
+ * Add `application/font-woff2`
+
+1.0.3 / 2014-09-25
+==================
+
+ * Fix engine requirement in package
+
+1.0.2 / 2014-09-25
+==================
+
+ * Add `application/coap-group+json`
+ * Add `application/dcd`
+ * Add `application/vnd.apache.thrift.binary`
+ * Add `image/vnd.tencent.tap`
+ * Mark all JSON-derived types as compressible
+ * Update `text/vtt` data
+
+1.0.1 / 2014-08-30
+==================
+
+ * Fix extension ordering
+
+1.0.0 / 2014-08-30
+==================
+
+ * Add `application/atf`
+ * Add `application/merge-patch+json`
+ * Add `multipart/x-mixed-replace`
+ * Add `source: 'apache'` metadata
+ * Add `source: 'iana'` metadata
+ * Remove badly-assumed charset data
diff --git a/node_modules/mime-db/LICENSE b/node_modules/mime-db/LICENSE
new file mode 100644
index 0000000..a7ae8ee
--- /dev/null
+++ b/node_modules/mime-db/LICENSE
@@ -0,0 +1,22 @@
+
+The MIT License (MIT)
+
+Copyright (c) 2014 Jonathan Ong me@jongleberry.com
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in
+all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
+THE SOFTWARE.
diff --git a/node_modules/mime-db/README.md b/node_modules/mime-db/README.md
new file mode 100644
index 0000000..48a9e3a
--- /dev/null
+++ b/node_modules/mime-db/README.md
@@ -0,0 +1,94 @@
+# mime-db
+
+[![NPM Version][npm-version-image]][npm-url]
+[![NPM Downloads][npm-downloads-image]][npm-url]
+[![Node.js Version][node-image]][node-url]
+[![Build Status][travis-image]][travis-url]
+[![Coverage Status][coveralls-image]][coveralls-url]
+
+This is a database of all mime types.
+It consists of a single, public JSON file and does not include any logic,
+allowing it to remain as un-opinionated as possible with an API.
+It aggregates data from the following sources:
+
+- http://www.iana.org/assignments/media-types/media-types.xhtml
+- http://svn.apache.org/repos/asf/httpd/httpd/trunk/docs/conf/mime.types
+- http://hg.nginx.org/nginx/raw-file/default/conf/mime.types
+
+## Installation
+
+```bash
+npm install mime-db
+```
+
+### Database Download
+
+If you're crazy enough to use this in the browser, you can just grab the
+JSON file using [RawGit](https://rawgit.com/). It is recommended to replace
+`master` with [a release tag](https://github.com/jshttp/mime-db/tags) as the
+JSON format may change in the future.
+
+```
+https://cdn.rawgit.com/jshttp/mime-db/master/db.json
+```
+
+## Usage
+
+```js
+var db = require('mime-db');
+
+// grab data on .js files
+var data = db['application/javascript'];
+```
+
+## Data Structure
+
+The JSON file is a map lookup for lowercased mime types.
+Each mime type has the following properties:
+
+- `.source` - where the mime type is defined.
+ If not set, it's probably a custom media type.
+ - `apache` - [Apache common media types](http://svn.apache.org/repos/asf/httpd/httpd/trunk/docs/conf/mime.types)
+ - `iana` - [IANA-defined media types](http://www.iana.org/assignments/media-types/media-types.xhtml)
+ - `nginx` - [nginx media types](http://hg.nginx.org/nginx/raw-file/default/conf/mime.types)
+- `.extensions[]` - known extensions associated with this mime type.
+- `.compressible` - whether a file of this type can be gzipped.
+- `.charset` - the default charset associated with this type, if any.
+
+If unknown, every property could be `undefined`.
+
+## Contributing
+
+To edit the database, only make PRs against `src/custom.json` or
+`src/custom-suffix.json`.
+
+The `src/custom.json` file is a JSON object with the MIME type as the keys
+and the values being an object with the following keys:
+
+- `compressible` - leave out if you don't know, otherwise `true`/`false` to
+ indicate whether the data represented by the type is typically compressible.
+- `extensions` - include an array of file extensions that are associated with
+ the type.
+- `notes` - human-readable notes about the type, typically what the type is.
+- `sources` - include an array of URLs of where the MIME type and the associated
+ extensions are sourced from. This needs to be a [primary source](https://en.wikipedia.org/wiki/Primary_source);
+ links to type aggregating sites and Wikipedia are _not acceptable_.
+
+To update the build, run `npm run build`.
+
+## Adding Custom Media Types
+
+The best way to get new media types included in this library is to register
+them with the IANA. The community registration procedure is outlined in
+[RFC 6838 section 5](http://tools.ietf.org/html/rfc6838#section-5). Types
+registered with the IANA are automatically pulled into this library.
+
+[coveralls-image]: https://badgen.net/coveralls/c/github/jshttp/mime-db/master
+[coveralls-url]: https://coveralls.io/r/jshttp/mime-db?branch=master
+[node-image]: https://badgen.net/npm/node/mime-db
+[node-url]: https://nodejs.org/en/download
+[npm-downloads-image]: https://badgen.net/npm/dm/mime-db
+[npm-url]: https://npmjs.org/package/mime-db
+[npm-version-image]: https://badgen.net/npm/v/mime-db
+[travis-image]: https://badgen.net/travis/jshttp/mime-db/master
+[travis-url]: https://travis-ci.org/jshttp/mime-db
diff --git a/node_modules/mime-db/db.json b/node_modules/mime-db/db.json
new file mode 100644
index 0000000..81f614c
--- /dev/null
+++ b/node_modules/mime-db/db.json
@@ -0,0 +1,7688 @@
+{
+ "application/1d-interleaved-parityfec": {
+ "source": "iana"
+ },
+ "application/3gpdash-qoe-report+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/3gpp-ims+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/a2l": {
+ "source": "iana"
+ },
+ "application/activemessage": {
+ "source": "iana"
+ },
+ "application/activity+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/alto-costmap+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/alto-costmapfilter+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/alto-directory+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/alto-endpointcost+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/alto-endpointcostparams+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/alto-endpointprop+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/alto-endpointpropparams+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/alto-error+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/alto-networkmap+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/alto-networkmapfilter+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/aml": {
+ "source": "iana"
+ },
+ "application/andrew-inset": {
+ "source": "iana",
+ "extensions": ["ez"]
+ },
+ "application/applefile": {
+ "source": "iana"
+ },
+ "application/applixware": {
+ "source": "apache",
+ "extensions": ["aw"]
+ },
+ "application/atf": {
+ "source": "iana"
+ },
+ "application/atfx": {
+ "source": "iana"
+ },
+ "application/atom+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["atom"]
+ },
+ "application/atomcat+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["atomcat"]
+ },
+ "application/atomdeleted+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/atomicmail": {
+ "source": "iana"
+ },
+ "application/atomsvc+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["atomsvc"]
+ },
+ "application/atxml": {
+ "source": "iana"
+ },
+ "application/auth-policy+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/bacnet-xdd+zip": {
+ "source": "iana",
+ "compressible": false
+ },
+ "application/batch-smtp": {
+ "source": "iana"
+ },
+ "application/bdoc": {
+ "compressible": false,
+ "extensions": ["bdoc"]
+ },
+ "application/beep+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/calendar+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/calendar+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/call-completion": {
+ "source": "iana"
+ },
+ "application/cals-1840": {
+ "source": "iana"
+ },
+ "application/cbor": {
+ "source": "iana"
+ },
+ "application/cccex": {
+ "source": "iana"
+ },
+ "application/ccmp+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/ccxml+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["ccxml"]
+ },
+ "application/cdfx+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/cdmi-capability": {
+ "source": "iana",
+ "extensions": ["cdmia"]
+ },
+ "application/cdmi-container": {
+ "source": "iana",
+ "extensions": ["cdmic"]
+ },
+ "application/cdmi-domain": {
+ "source": "iana",
+ "extensions": ["cdmid"]
+ },
+ "application/cdmi-object": {
+ "source": "iana",
+ "extensions": ["cdmio"]
+ },
+ "application/cdmi-queue": {
+ "source": "iana",
+ "extensions": ["cdmiq"]
+ },
+ "application/cdni": {
+ "source": "iana"
+ },
+ "application/cea": {
+ "source": "iana"
+ },
+ "application/cea-2018+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/cellml+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/cfw": {
+ "source": "iana"
+ },
+ "application/clue_info+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/cms": {
+ "source": "iana"
+ },
+ "application/cnrp+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/coap-group+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/coap-payload": {
+ "source": "iana"
+ },
+ "application/commonground": {
+ "source": "iana"
+ },
+ "application/conference-info+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/cose": {
+ "source": "iana"
+ },
+ "application/cose-key": {
+ "source": "iana"
+ },
+ "application/cose-key-set": {
+ "source": "iana"
+ },
+ "application/cpl+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/csrattrs": {
+ "source": "iana"
+ },
+ "application/csta+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/cstadata+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/csvm+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/cu-seeme": {
+ "source": "apache",
+ "extensions": ["cu"]
+ },
+ "application/cwt": {
+ "source": "iana"
+ },
+ "application/cybercash": {
+ "source": "iana"
+ },
+ "application/dart": {
+ "compressible": true
+ },
+ "application/dash+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["mpd"]
+ },
+ "application/dashdelta": {
+ "source": "iana"
+ },
+ "application/davmount+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["davmount"]
+ },
+ "application/dca-rft": {
+ "source": "iana"
+ },
+ "application/dcd": {
+ "source": "iana"
+ },
+ "application/dec-dx": {
+ "source": "iana"
+ },
+ "application/dialog-info+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/dicom": {
+ "source": "iana"
+ },
+ "application/dicom+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/dicom+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/dii": {
+ "source": "iana"
+ },
+ "application/dit": {
+ "source": "iana"
+ },
+ "application/dns": {
+ "source": "iana"
+ },
+ "application/dns+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/dns-message": {
+ "source": "iana"
+ },
+ "application/docbook+xml": {
+ "source": "apache",
+ "compressible": true,
+ "extensions": ["dbk"]
+ },
+ "application/dskpp+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/dssc+der": {
+ "source": "iana",
+ "extensions": ["dssc"]
+ },
+ "application/dssc+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["xdssc"]
+ },
+ "application/dvcs": {
+ "source": "iana"
+ },
+ "application/ecmascript": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["ecma","es"]
+ },
+ "application/edi-consent": {
+ "source": "iana"
+ },
+ "application/edi-x12": {
+ "source": "iana",
+ "compressible": false
+ },
+ "application/edifact": {
+ "source": "iana",
+ "compressible": false
+ },
+ "application/efi": {
+ "source": "iana"
+ },
+ "application/emergencycalldata.comment+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/emergencycalldata.control+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/emergencycalldata.deviceinfo+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/emergencycalldata.ecall.msd": {
+ "source": "iana"
+ },
+ "application/emergencycalldata.providerinfo+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/emergencycalldata.serviceinfo+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/emergencycalldata.subscriberinfo+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/emergencycalldata.veds+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/emma+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["emma"]
+ },
+ "application/emotionml+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/encaprtp": {
+ "source": "iana"
+ },
+ "application/epp+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/epub+zip": {
+ "source": "iana",
+ "compressible": false,
+ "extensions": ["epub"]
+ },
+ "application/eshop": {
+ "source": "iana"
+ },
+ "application/exi": {
+ "source": "iana",
+ "extensions": ["exi"]
+ },
+ "application/fastinfoset": {
+ "source": "iana"
+ },
+ "application/fastsoap": {
+ "source": "iana"
+ },
+ "application/fdt+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/fhir+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/fhir+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/fido.trusted-apps+json": {
+ "compressible": true
+ },
+ "application/fits": {
+ "source": "iana"
+ },
+ "application/font-sfnt": {
+ "source": "iana"
+ },
+ "application/font-tdpfr": {
+ "source": "iana",
+ "extensions": ["pfr"]
+ },
+ "application/font-woff": {
+ "source": "iana",
+ "compressible": false
+ },
+ "application/framework-attributes+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/geo+json": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["geojson"]
+ },
+ "application/geo+json-seq": {
+ "source": "iana"
+ },
+ "application/geopackage+sqlite3": {
+ "source": "iana"
+ },
+ "application/geoxacml+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/gltf-buffer": {
+ "source": "iana"
+ },
+ "application/gml+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["gml"]
+ },
+ "application/gpx+xml": {
+ "source": "apache",
+ "compressible": true,
+ "extensions": ["gpx"]
+ },
+ "application/gxf": {
+ "source": "apache",
+ "extensions": ["gxf"]
+ },
+ "application/gzip": {
+ "source": "iana",
+ "compressible": false,
+ "extensions": ["gz"]
+ },
+ "application/h224": {
+ "source": "iana"
+ },
+ "application/held+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/hjson": {
+ "extensions": ["hjson"]
+ },
+ "application/http": {
+ "source": "iana"
+ },
+ "application/hyperstudio": {
+ "source": "iana",
+ "extensions": ["stk"]
+ },
+ "application/ibe-key-request+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/ibe-pkg-reply+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/ibe-pp-data": {
+ "source": "iana"
+ },
+ "application/iges": {
+ "source": "iana"
+ },
+ "application/im-iscomposing+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/index": {
+ "source": "iana"
+ },
+ "application/index.cmd": {
+ "source": "iana"
+ },
+ "application/index.obj": {
+ "source": "iana"
+ },
+ "application/index.response": {
+ "source": "iana"
+ },
+ "application/index.vnd": {
+ "source": "iana"
+ },
+ "application/inkml+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["ink","inkml"]
+ },
+ "application/iotp": {
+ "source": "iana"
+ },
+ "application/ipfix": {
+ "source": "iana",
+ "extensions": ["ipfix"]
+ },
+ "application/ipp": {
+ "source": "iana"
+ },
+ "application/isup": {
+ "source": "iana"
+ },
+ "application/its+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/java-archive": {
+ "source": "apache",
+ "compressible": false,
+ "extensions": ["jar","war","ear"]
+ },
+ "application/java-serialized-object": {
+ "source": "apache",
+ "compressible": false,
+ "extensions": ["ser"]
+ },
+ "application/java-vm": {
+ "source": "apache",
+ "compressible": false,
+ "extensions": ["class"]
+ },
+ "application/javascript": {
+ "source": "iana",
+ "charset": "UTF-8",
+ "compressible": true,
+ "extensions": ["js","mjs"]
+ },
+ "application/jf2feed+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/jose": {
+ "source": "iana"
+ },
+ "application/jose+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/jrd+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/json": {
+ "source": "iana",
+ "charset": "UTF-8",
+ "compressible": true,
+ "extensions": ["json","map"]
+ },
+ "application/json-patch+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/json-seq": {
+ "source": "iana"
+ },
+ "application/json5": {
+ "extensions": ["json5"]
+ },
+ "application/jsonml+json": {
+ "source": "apache",
+ "compressible": true,
+ "extensions": ["jsonml"]
+ },
+ "application/jwk+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/jwk-set+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/jwt": {
+ "source": "iana"
+ },
+ "application/kpml-request+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/kpml-response+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/ld+json": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["jsonld"]
+ },
+ "application/lgr+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/link-format": {
+ "source": "iana"
+ },
+ "application/load-control+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/lost+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["lostxml"]
+ },
+ "application/lostsync+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/lxf": {
+ "source": "iana"
+ },
+ "application/mac-binhex40": {
+ "source": "iana",
+ "extensions": ["hqx"]
+ },
+ "application/mac-compactpro": {
+ "source": "apache",
+ "extensions": ["cpt"]
+ },
+ "application/macwriteii": {
+ "source": "iana"
+ },
+ "application/mads+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["mads"]
+ },
+ "application/manifest+json": {
+ "charset": "UTF-8",
+ "compressible": true,
+ "extensions": ["webmanifest"]
+ },
+ "application/marc": {
+ "source": "iana",
+ "extensions": ["mrc"]
+ },
+ "application/marcxml+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["mrcx"]
+ },
+ "application/mathematica": {
+ "source": "iana",
+ "extensions": ["ma","nb","mb"]
+ },
+ "application/mathml+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["mathml"]
+ },
+ "application/mathml-content+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/mathml-presentation+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/mbms-associated-procedure-description+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/mbms-deregister+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/mbms-envelope+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/mbms-msk+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/mbms-msk-response+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/mbms-protection-description+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/mbms-reception-report+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/mbms-register+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/mbms-register-response+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/mbms-schedule+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/mbms-user-service-description+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/mbox": {
+ "source": "iana",
+ "extensions": ["mbox"]
+ },
+ "application/media-policy-dataset+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/media_control+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/mediaservercontrol+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["mscml"]
+ },
+ "application/merge-patch+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/metalink+xml": {
+ "source": "apache",
+ "compressible": true,
+ "extensions": ["metalink"]
+ },
+ "application/metalink4+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["meta4"]
+ },
+ "application/mets+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["mets"]
+ },
+ "application/mf4": {
+ "source": "iana"
+ },
+ "application/mikey": {
+ "source": "iana"
+ },
+ "application/mmt-usd+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/mods+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["mods"]
+ },
+ "application/moss-keys": {
+ "source": "iana"
+ },
+ "application/moss-signature": {
+ "source": "iana"
+ },
+ "application/mosskey-data": {
+ "source": "iana"
+ },
+ "application/mosskey-request": {
+ "source": "iana"
+ },
+ "application/mp21": {
+ "source": "iana",
+ "extensions": ["m21","mp21"]
+ },
+ "application/mp4": {
+ "source": "iana",
+ "extensions": ["mp4s","m4p"]
+ },
+ "application/mpeg4-generic": {
+ "source": "iana"
+ },
+ "application/mpeg4-iod": {
+ "source": "iana"
+ },
+ "application/mpeg4-iod-xmt": {
+ "source": "iana"
+ },
+ "application/mrb-consumer+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/mrb-publish+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/msc-ivr+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/msc-mixer+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/msword": {
+ "source": "iana",
+ "compressible": false,
+ "extensions": ["doc","dot"]
+ },
+ "application/mud+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/mxf": {
+ "source": "iana",
+ "extensions": ["mxf"]
+ },
+ "application/n-quads": {
+ "source": "iana"
+ },
+ "application/n-triples": {
+ "source": "iana"
+ },
+ "application/nasdata": {
+ "source": "iana"
+ },
+ "application/news-checkgroups": {
+ "source": "iana"
+ },
+ "application/news-groupinfo": {
+ "source": "iana"
+ },
+ "application/news-transmission": {
+ "source": "iana"
+ },
+ "application/nlsml+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/node": {
+ "source": "iana"
+ },
+ "application/nss": {
+ "source": "iana"
+ },
+ "application/ocsp-request": {
+ "source": "iana"
+ },
+ "application/ocsp-response": {
+ "source": "iana"
+ },
+ "application/octet-stream": {
+ "source": "iana",
+ "compressible": false,
+ "extensions": ["bin","dms","lrf","mar","so","dist","distz","pkg","bpk","dump","elc","deploy","exe","dll","deb","dmg","iso","img","msi","msp","msm","buffer"]
+ },
+ "application/oda": {
+ "source": "iana",
+ "extensions": ["oda"]
+ },
+ "application/odx": {
+ "source": "iana"
+ },
+ "application/oebps-package+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["opf"]
+ },
+ "application/ogg": {
+ "source": "iana",
+ "compressible": false,
+ "extensions": ["ogx"]
+ },
+ "application/omdoc+xml": {
+ "source": "apache",
+ "compressible": true,
+ "extensions": ["omdoc"]
+ },
+ "application/onenote": {
+ "source": "apache",
+ "extensions": ["onetoc","onetoc2","onetmp","onepkg"]
+ },
+ "application/oxps": {
+ "source": "iana",
+ "extensions": ["oxps"]
+ },
+ "application/p2p-overlay+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/parityfec": {
+ "source": "iana"
+ },
+ "application/passport": {
+ "source": "iana"
+ },
+ "application/patch-ops-error+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["xer"]
+ },
+ "application/pdf": {
+ "source": "iana",
+ "compressible": false,
+ "extensions": ["pdf"]
+ },
+ "application/pdx": {
+ "source": "iana"
+ },
+ "application/pgp-encrypted": {
+ "source": "iana",
+ "compressible": false,
+ "extensions": ["pgp"]
+ },
+ "application/pgp-keys": {
+ "source": "iana"
+ },
+ "application/pgp-signature": {
+ "source": "iana",
+ "extensions": ["asc","sig"]
+ },
+ "application/pics-rules": {
+ "source": "apache",
+ "extensions": ["prf"]
+ },
+ "application/pidf+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/pidf-diff+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/pkcs10": {
+ "source": "iana",
+ "extensions": ["p10"]
+ },
+ "application/pkcs12": {
+ "source": "iana"
+ },
+ "application/pkcs7-mime": {
+ "source": "iana",
+ "extensions": ["p7m","p7c"]
+ },
+ "application/pkcs7-signature": {
+ "source": "iana",
+ "extensions": ["p7s"]
+ },
+ "application/pkcs8": {
+ "source": "iana",
+ "extensions": ["p8"]
+ },
+ "application/pkcs8-encrypted": {
+ "source": "iana"
+ },
+ "application/pkix-attr-cert": {
+ "source": "iana",
+ "extensions": ["ac"]
+ },
+ "application/pkix-cert": {
+ "source": "iana",
+ "extensions": ["cer"]
+ },
+ "application/pkix-crl": {
+ "source": "iana",
+ "extensions": ["crl"]
+ },
+ "application/pkix-pkipath": {
+ "source": "iana",
+ "extensions": ["pkipath"]
+ },
+ "application/pkixcmp": {
+ "source": "iana",
+ "extensions": ["pki"]
+ },
+ "application/pls+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["pls"]
+ },
+ "application/poc-settings+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/postscript": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["ai","eps","ps"]
+ },
+ "application/ppsp-tracker+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/problem+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/problem+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/provenance+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/prs.alvestrand.titrax-sheet": {
+ "source": "iana"
+ },
+ "application/prs.cww": {
+ "source": "iana",
+ "extensions": ["cww"]
+ },
+ "application/prs.hpub+zip": {
+ "source": "iana",
+ "compressible": false
+ },
+ "application/prs.nprend": {
+ "source": "iana"
+ },
+ "application/prs.plucker": {
+ "source": "iana"
+ },
+ "application/prs.rdf-xml-crypt": {
+ "source": "iana"
+ },
+ "application/prs.xsf+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/pskc+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["pskcxml"]
+ },
+ "application/qsig": {
+ "source": "iana"
+ },
+ "application/raml+yaml": {
+ "compressible": true,
+ "extensions": ["raml"]
+ },
+ "application/raptorfec": {
+ "source": "iana"
+ },
+ "application/rdap+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/rdf+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["rdf","owl"]
+ },
+ "application/reginfo+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["rif"]
+ },
+ "application/relax-ng-compact-syntax": {
+ "source": "iana",
+ "extensions": ["rnc"]
+ },
+ "application/remote-printing": {
+ "source": "iana"
+ },
+ "application/reputon+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/resource-lists+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["rl"]
+ },
+ "application/resource-lists-diff+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["rld"]
+ },
+ "application/rfc+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/riscos": {
+ "source": "iana"
+ },
+ "application/rlmi+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/rls-services+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["rs"]
+ },
+ "application/route-apd+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/route-s-tsid+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/route-usd+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/rpki-ghostbusters": {
+ "source": "iana",
+ "extensions": ["gbr"]
+ },
+ "application/rpki-manifest": {
+ "source": "iana",
+ "extensions": ["mft"]
+ },
+ "application/rpki-publication": {
+ "source": "iana"
+ },
+ "application/rpki-roa": {
+ "source": "iana",
+ "extensions": ["roa"]
+ },
+ "application/rpki-updown": {
+ "source": "iana"
+ },
+ "application/rsd+xml": {
+ "source": "apache",
+ "compressible": true,
+ "extensions": ["rsd"]
+ },
+ "application/rss+xml": {
+ "source": "apache",
+ "compressible": true,
+ "extensions": ["rss"]
+ },
+ "application/rtf": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["rtf"]
+ },
+ "application/rtploopback": {
+ "source": "iana"
+ },
+ "application/rtx": {
+ "source": "iana"
+ },
+ "application/samlassertion+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/samlmetadata+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/sbml+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["sbml"]
+ },
+ "application/scaip+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/scim+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/scvp-cv-request": {
+ "source": "iana",
+ "extensions": ["scq"]
+ },
+ "application/scvp-cv-response": {
+ "source": "iana",
+ "extensions": ["scs"]
+ },
+ "application/scvp-vp-request": {
+ "source": "iana",
+ "extensions": ["spq"]
+ },
+ "application/scvp-vp-response": {
+ "source": "iana",
+ "extensions": ["spp"]
+ },
+ "application/sdp": {
+ "source": "iana",
+ "extensions": ["sdp"]
+ },
+ "application/secevent+jwt": {
+ "source": "iana"
+ },
+ "application/senml+cbor": {
+ "source": "iana"
+ },
+ "application/senml+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/senml+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/senml-exi": {
+ "source": "iana"
+ },
+ "application/sensml+cbor": {
+ "source": "iana"
+ },
+ "application/sensml+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/sensml+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/sensml-exi": {
+ "source": "iana"
+ },
+ "application/sep+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/sep-exi": {
+ "source": "iana"
+ },
+ "application/session-info": {
+ "source": "iana"
+ },
+ "application/set-payment": {
+ "source": "iana"
+ },
+ "application/set-payment-initiation": {
+ "source": "iana",
+ "extensions": ["setpay"]
+ },
+ "application/set-registration": {
+ "source": "iana"
+ },
+ "application/set-registration-initiation": {
+ "source": "iana",
+ "extensions": ["setreg"]
+ },
+ "application/sgml": {
+ "source": "iana"
+ },
+ "application/sgml-open-catalog": {
+ "source": "iana"
+ },
+ "application/shf+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["shf"]
+ },
+ "application/sieve": {
+ "source": "iana"
+ },
+ "application/simple-filter+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/simple-message-summary": {
+ "source": "iana"
+ },
+ "application/simplesymbolcontainer": {
+ "source": "iana"
+ },
+ "application/slate": {
+ "source": "iana"
+ },
+ "application/smil": {
+ "source": "iana"
+ },
+ "application/smil+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["smi","smil"]
+ },
+ "application/smpte336m": {
+ "source": "iana"
+ },
+ "application/soap+fastinfoset": {
+ "source": "iana"
+ },
+ "application/soap+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/sparql-query": {
+ "source": "iana",
+ "extensions": ["rq"]
+ },
+ "application/sparql-results+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["srx"]
+ },
+ "application/spirits-event+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/sql": {
+ "source": "iana"
+ },
+ "application/srgs": {
+ "source": "iana",
+ "extensions": ["gram"]
+ },
+ "application/srgs+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["grxml"]
+ },
+ "application/sru+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["sru"]
+ },
+ "application/ssdl+xml": {
+ "source": "apache",
+ "compressible": true,
+ "extensions": ["ssdl"]
+ },
+ "application/ssml+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["ssml"]
+ },
+ "application/stix+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/tamp-apex-update": {
+ "source": "iana"
+ },
+ "application/tamp-apex-update-confirm": {
+ "source": "iana"
+ },
+ "application/tamp-community-update": {
+ "source": "iana"
+ },
+ "application/tamp-community-update-confirm": {
+ "source": "iana"
+ },
+ "application/tamp-error": {
+ "source": "iana"
+ },
+ "application/tamp-sequence-adjust": {
+ "source": "iana"
+ },
+ "application/tamp-sequence-adjust-confirm": {
+ "source": "iana"
+ },
+ "application/tamp-status-query": {
+ "source": "iana"
+ },
+ "application/tamp-status-response": {
+ "source": "iana"
+ },
+ "application/tamp-update": {
+ "source": "iana"
+ },
+ "application/tamp-update-confirm": {
+ "source": "iana"
+ },
+ "application/tar": {
+ "compressible": true
+ },
+ "application/taxii+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/tei+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["tei","teicorpus"]
+ },
+ "application/thraud+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["tfi"]
+ },
+ "application/timestamp-query": {
+ "source": "iana"
+ },
+ "application/timestamp-reply": {
+ "source": "iana"
+ },
+ "application/timestamped-data": {
+ "source": "iana",
+ "extensions": ["tsd"]
+ },
+ "application/tlsrpt+gzip": {
+ "source": "iana"
+ },
+ "application/tlsrpt+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/tnauthlist": {
+ "source": "iana"
+ },
+ "application/trickle-ice-sdpfrag": {
+ "source": "iana"
+ },
+ "application/trig": {
+ "source": "iana"
+ },
+ "application/ttml+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/tve-trigger": {
+ "source": "iana"
+ },
+ "application/ulpfec": {
+ "source": "iana"
+ },
+ "application/urc-grpsheet+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/urc-ressheet+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/urc-targetdesc+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/urc-uisocketdesc+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vcard+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vcard+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vemmi": {
+ "source": "iana"
+ },
+ "application/vividence.scriptfile": {
+ "source": "apache"
+ },
+ "application/vnd.1000minds.decision-model+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.3gpp-prose+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.3gpp-prose-pc3ch+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.3gpp-v2x-local-service-information": {
+ "source": "iana"
+ },
+ "application/vnd.3gpp.access-transfer-events+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.3gpp.bsf+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.3gpp.gmop+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.3gpp.mc-signalling-ear": {
+ "source": "iana"
+ },
+ "application/vnd.3gpp.mcdata-payload": {
+ "source": "iana"
+ },
+ "application/vnd.3gpp.mcdata-signalling": {
+ "source": "iana"
+ },
+ "application/vnd.3gpp.mcptt-affiliation-command+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.3gpp.mcptt-floor-request+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.3gpp.mcptt-info+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.3gpp.mcptt-location-info+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.3gpp.mcptt-mbms-usage-info+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.3gpp.mcptt-signed+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.3gpp.mid-call+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.3gpp.pic-bw-large": {
+ "source": "iana",
+ "extensions": ["plb"]
+ },
+ "application/vnd.3gpp.pic-bw-small": {
+ "source": "iana",
+ "extensions": ["psb"]
+ },
+ "application/vnd.3gpp.pic-bw-var": {
+ "source": "iana",
+ "extensions": ["pvb"]
+ },
+ "application/vnd.3gpp.sms": {
+ "source": "iana"
+ },
+ "application/vnd.3gpp.sms+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.3gpp.srvcc-ext+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.3gpp.srvcc-info+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.3gpp.state-and-event-info+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.3gpp.ussd+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.3gpp2.bcmcsinfo+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.3gpp2.sms": {
+ "source": "iana"
+ },
+ "application/vnd.3gpp2.tcap": {
+ "source": "iana",
+ "extensions": ["tcap"]
+ },
+ "application/vnd.3lightssoftware.imagescal": {
+ "source": "iana"
+ },
+ "application/vnd.3m.post-it-notes": {
+ "source": "iana",
+ "extensions": ["pwn"]
+ },
+ "application/vnd.accpac.simply.aso": {
+ "source": "iana",
+ "extensions": ["aso"]
+ },
+ "application/vnd.accpac.simply.imp": {
+ "source": "iana",
+ "extensions": ["imp"]
+ },
+ "application/vnd.acucobol": {
+ "source": "iana",
+ "extensions": ["acu"]
+ },
+ "application/vnd.acucorp": {
+ "source": "iana",
+ "extensions": ["atc","acutc"]
+ },
+ "application/vnd.adobe.air-application-installer-package+zip": {
+ "source": "apache",
+ "compressible": false,
+ "extensions": ["air"]
+ },
+ "application/vnd.adobe.flash.movie": {
+ "source": "iana"
+ },
+ "application/vnd.adobe.formscentral.fcdt": {
+ "source": "iana",
+ "extensions": ["fcdt"]
+ },
+ "application/vnd.adobe.fxp": {
+ "source": "iana",
+ "extensions": ["fxp","fxpl"]
+ },
+ "application/vnd.adobe.partial-upload": {
+ "source": "iana"
+ },
+ "application/vnd.adobe.xdp+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["xdp"]
+ },
+ "application/vnd.adobe.xfdf": {
+ "source": "iana",
+ "extensions": ["xfdf"]
+ },
+ "application/vnd.aether.imp": {
+ "source": "iana"
+ },
+ "application/vnd.afpc.afplinedata": {
+ "source": "iana"
+ },
+ "application/vnd.afpc.modca": {
+ "source": "iana"
+ },
+ "application/vnd.ah-barcode": {
+ "source": "iana"
+ },
+ "application/vnd.ahead.space": {
+ "source": "iana",
+ "extensions": ["ahead"]
+ },
+ "application/vnd.airzip.filesecure.azf": {
+ "source": "iana",
+ "extensions": ["azf"]
+ },
+ "application/vnd.airzip.filesecure.azs": {
+ "source": "iana",
+ "extensions": ["azs"]
+ },
+ "application/vnd.amadeus+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.amazon.ebook": {
+ "source": "apache",
+ "extensions": ["azw"]
+ },
+ "application/vnd.amazon.mobi8-ebook": {
+ "source": "iana"
+ },
+ "application/vnd.americandynamics.acc": {
+ "source": "iana",
+ "extensions": ["acc"]
+ },
+ "application/vnd.amiga.ami": {
+ "source": "iana",
+ "extensions": ["ami"]
+ },
+ "application/vnd.amundsen.maze+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.android.package-archive": {
+ "source": "apache",
+ "compressible": false,
+ "extensions": ["apk"]
+ },
+ "application/vnd.anki": {
+ "source": "iana"
+ },
+ "application/vnd.anser-web-certificate-issue-initiation": {
+ "source": "iana",
+ "extensions": ["cii"]
+ },
+ "application/vnd.anser-web-funds-transfer-initiation": {
+ "source": "apache",
+ "extensions": ["fti"]
+ },
+ "application/vnd.antix.game-component": {
+ "source": "iana",
+ "extensions": ["atx"]
+ },
+ "application/vnd.apache.thrift.binary": {
+ "source": "iana"
+ },
+ "application/vnd.apache.thrift.compact": {
+ "source": "iana"
+ },
+ "application/vnd.apache.thrift.json": {
+ "source": "iana"
+ },
+ "application/vnd.api+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.apothekende.reservation+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.apple.installer+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["mpkg"]
+ },
+ "application/vnd.apple.keynote": {
+ "source": "iana",
+ "extensions": ["keynote"]
+ },
+ "application/vnd.apple.mpegurl": {
+ "source": "iana",
+ "extensions": ["m3u8"]
+ },
+ "application/vnd.apple.numbers": {
+ "source": "iana",
+ "extensions": ["numbers"]
+ },
+ "application/vnd.apple.pages": {
+ "source": "iana",
+ "extensions": ["pages"]
+ },
+ "application/vnd.apple.pkpass": {
+ "compressible": false,
+ "extensions": ["pkpass"]
+ },
+ "application/vnd.arastra.swi": {
+ "source": "iana"
+ },
+ "application/vnd.aristanetworks.swi": {
+ "source": "iana",
+ "extensions": ["swi"]
+ },
+ "application/vnd.artisan+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.artsquare": {
+ "source": "iana"
+ },
+ "application/vnd.astraea-software.iota": {
+ "source": "iana",
+ "extensions": ["iota"]
+ },
+ "application/vnd.audiograph": {
+ "source": "iana",
+ "extensions": ["aep"]
+ },
+ "application/vnd.autopackage": {
+ "source": "iana"
+ },
+ "application/vnd.avalon+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.avistar+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.balsamiq.bmml+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.balsamiq.bmpr": {
+ "source": "iana"
+ },
+ "application/vnd.banana-accounting": {
+ "source": "iana"
+ },
+ "application/vnd.bbf.usp.msg": {
+ "source": "iana"
+ },
+ "application/vnd.bbf.usp.msg+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.bekitzur-stech+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.bint.med-content": {
+ "source": "iana"
+ },
+ "application/vnd.biopax.rdf+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.blink-idb-value-wrapper": {
+ "source": "iana"
+ },
+ "application/vnd.blueice.multipass": {
+ "source": "iana",
+ "extensions": ["mpm"]
+ },
+ "application/vnd.bluetooth.ep.oob": {
+ "source": "iana"
+ },
+ "application/vnd.bluetooth.le.oob": {
+ "source": "iana"
+ },
+ "application/vnd.bmi": {
+ "source": "iana",
+ "extensions": ["bmi"]
+ },
+ "application/vnd.businessobjects": {
+ "source": "iana",
+ "extensions": ["rep"]
+ },
+ "application/vnd.byu.uapi+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.cab-jscript": {
+ "source": "iana"
+ },
+ "application/vnd.canon-cpdl": {
+ "source": "iana"
+ },
+ "application/vnd.canon-lips": {
+ "source": "iana"
+ },
+ "application/vnd.capasystems-pg+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.cendio.thinlinc.clientconf": {
+ "source": "iana"
+ },
+ "application/vnd.century-systems.tcp_stream": {
+ "source": "iana"
+ },
+ "application/vnd.chemdraw+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["cdxml"]
+ },
+ "application/vnd.chess-pgn": {
+ "source": "iana"
+ },
+ "application/vnd.chipnuts.karaoke-mmd": {
+ "source": "iana",
+ "extensions": ["mmd"]
+ },
+ "application/vnd.cinderella": {
+ "source": "iana",
+ "extensions": ["cdy"]
+ },
+ "application/vnd.cirpack.isdn-ext": {
+ "source": "iana"
+ },
+ "application/vnd.citationstyles.style+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["csl"]
+ },
+ "application/vnd.claymore": {
+ "source": "iana",
+ "extensions": ["cla"]
+ },
+ "application/vnd.cloanto.rp9": {
+ "source": "iana",
+ "extensions": ["rp9"]
+ },
+ "application/vnd.clonk.c4group": {
+ "source": "iana",
+ "extensions": ["c4g","c4d","c4f","c4p","c4u"]
+ },
+ "application/vnd.cluetrust.cartomobile-config": {
+ "source": "iana",
+ "extensions": ["c11amc"]
+ },
+ "application/vnd.cluetrust.cartomobile-config-pkg": {
+ "source": "iana",
+ "extensions": ["c11amz"]
+ },
+ "application/vnd.coffeescript": {
+ "source": "iana"
+ },
+ "application/vnd.collabio.xodocuments.document": {
+ "source": "iana"
+ },
+ "application/vnd.collabio.xodocuments.document-template": {
+ "source": "iana"
+ },
+ "application/vnd.collabio.xodocuments.presentation": {
+ "source": "iana"
+ },
+ "application/vnd.collabio.xodocuments.presentation-template": {
+ "source": "iana"
+ },
+ "application/vnd.collabio.xodocuments.spreadsheet": {
+ "source": "iana"
+ },
+ "application/vnd.collabio.xodocuments.spreadsheet-template": {
+ "source": "iana"
+ },
+ "application/vnd.collection+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.collection.doc+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.collection.next+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.comicbook+zip": {
+ "source": "iana",
+ "compressible": false
+ },
+ "application/vnd.comicbook-rar": {
+ "source": "iana"
+ },
+ "application/vnd.commerce-battelle": {
+ "source": "iana"
+ },
+ "application/vnd.commonspace": {
+ "source": "iana",
+ "extensions": ["csp"]
+ },
+ "application/vnd.contact.cmsg": {
+ "source": "iana",
+ "extensions": ["cdbcmsg"]
+ },
+ "application/vnd.coreos.ignition+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.cosmocaller": {
+ "source": "iana",
+ "extensions": ["cmc"]
+ },
+ "application/vnd.crick.clicker": {
+ "source": "iana",
+ "extensions": ["clkx"]
+ },
+ "application/vnd.crick.clicker.keyboard": {
+ "source": "iana",
+ "extensions": ["clkk"]
+ },
+ "application/vnd.crick.clicker.palette": {
+ "source": "iana",
+ "extensions": ["clkp"]
+ },
+ "application/vnd.crick.clicker.template": {
+ "source": "iana",
+ "extensions": ["clkt"]
+ },
+ "application/vnd.crick.clicker.wordbank": {
+ "source": "iana",
+ "extensions": ["clkw"]
+ },
+ "application/vnd.criticaltools.wbs+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["wbs"]
+ },
+ "application/vnd.ctc-posml": {
+ "source": "iana",
+ "extensions": ["pml"]
+ },
+ "application/vnd.ctct.ws+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.cups-pdf": {
+ "source": "iana"
+ },
+ "application/vnd.cups-postscript": {
+ "source": "iana"
+ },
+ "application/vnd.cups-ppd": {
+ "source": "iana",
+ "extensions": ["ppd"]
+ },
+ "application/vnd.cups-raster": {
+ "source": "iana"
+ },
+ "application/vnd.cups-raw": {
+ "source": "iana"
+ },
+ "application/vnd.curl": {
+ "source": "iana"
+ },
+ "application/vnd.curl.car": {
+ "source": "apache",
+ "extensions": ["car"]
+ },
+ "application/vnd.curl.pcurl": {
+ "source": "apache",
+ "extensions": ["pcurl"]
+ },
+ "application/vnd.cyan.dean.root+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.cybank": {
+ "source": "iana"
+ },
+ "application/vnd.d2l.coursepackage1p0+zip": {
+ "source": "iana",
+ "compressible": false
+ },
+ "application/vnd.dart": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["dart"]
+ },
+ "application/vnd.data-vision.rdz": {
+ "source": "iana",
+ "extensions": ["rdz"]
+ },
+ "application/vnd.datapackage+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.dataresource+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.debian.binary-package": {
+ "source": "iana"
+ },
+ "application/vnd.dece.data": {
+ "source": "iana",
+ "extensions": ["uvf","uvvf","uvd","uvvd"]
+ },
+ "application/vnd.dece.ttml+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["uvt","uvvt"]
+ },
+ "application/vnd.dece.unspecified": {
+ "source": "iana",
+ "extensions": ["uvx","uvvx"]
+ },
+ "application/vnd.dece.zip": {
+ "source": "iana",
+ "extensions": ["uvz","uvvz"]
+ },
+ "application/vnd.denovo.fcselayout-link": {
+ "source": "iana",
+ "extensions": ["fe_launch"]
+ },
+ "application/vnd.desmume.movie": {
+ "source": "iana"
+ },
+ "application/vnd.dir-bi.plate-dl-nosuffix": {
+ "source": "iana"
+ },
+ "application/vnd.dm.delegation+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.dna": {
+ "source": "iana",
+ "extensions": ["dna"]
+ },
+ "application/vnd.document+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.dolby.mlp": {
+ "source": "apache",
+ "extensions": ["mlp"]
+ },
+ "application/vnd.dolby.mobile.1": {
+ "source": "iana"
+ },
+ "application/vnd.dolby.mobile.2": {
+ "source": "iana"
+ },
+ "application/vnd.doremir.scorecloud-binary-document": {
+ "source": "iana"
+ },
+ "application/vnd.dpgraph": {
+ "source": "iana",
+ "extensions": ["dpg"]
+ },
+ "application/vnd.dreamfactory": {
+ "source": "iana",
+ "extensions": ["dfac"]
+ },
+ "application/vnd.drive+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.ds-keypoint": {
+ "source": "apache",
+ "extensions": ["kpxx"]
+ },
+ "application/vnd.dtg.local": {
+ "source": "iana"
+ },
+ "application/vnd.dtg.local.flash": {
+ "source": "iana"
+ },
+ "application/vnd.dtg.local.html": {
+ "source": "iana"
+ },
+ "application/vnd.dvb.ait": {
+ "source": "iana",
+ "extensions": ["ait"]
+ },
+ "application/vnd.dvb.dvbj": {
+ "source": "iana"
+ },
+ "application/vnd.dvb.esgcontainer": {
+ "source": "iana"
+ },
+ "application/vnd.dvb.ipdcdftnotifaccess": {
+ "source": "iana"
+ },
+ "application/vnd.dvb.ipdcesgaccess": {
+ "source": "iana"
+ },
+ "application/vnd.dvb.ipdcesgaccess2": {
+ "source": "iana"
+ },
+ "application/vnd.dvb.ipdcesgpdd": {
+ "source": "iana"
+ },
+ "application/vnd.dvb.ipdcroaming": {
+ "source": "iana"
+ },
+ "application/vnd.dvb.iptv.alfec-base": {
+ "source": "iana"
+ },
+ "application/vnd.dvb.iptv.alfec-enhancement": {
+ "source": "iana"
+ },
+ "application/vnd.dvb.notif-aggregate-root+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.dvb.notif-container+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.dvb.notif-generic+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.dvb.notif-ia-msglist+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.dvb.notif-ia-registration-request+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.dvb.notif-ia-registration-response+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.dvb.notif-init+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.dvb.pfr": {
+ "source": "iana"
+ },
+ "application/vnd.dvb.service": {
+ "source": "iana",
+ "extensions": ["svc"]
+ },
+ "application/vnd.dxr": {
+ "source": "iana"
+ },
+ "application/vnd.dynageo": {
+ "source": "iana",
+ "extensions": ["geo"]
+ },
+ "application/vnd.dzr": {
+ "source": "iana"
+ },
+ "application/vnd.easykaraoke.cdgdownload": {
+ "source": "iana"
+ },
+ "application/vnd.ecdis-update": {
+ "source": "iana"
+ },
+ "application/vnd.ecip.rlp": {
+ "source": "iana"
+ },
+ "application/vnd.ecowin.chart": {
+ "source": "iana",
+ "extensions": ["mag"]
+ },
+ "application/vnd.ecowin.filerequest": {
+ "source": "iana"
+ },
+ "application/vnd.ecowin.fileupdate": {
+ "source": "iana"
+ },
+ "application/vnd.ecowin.series": {
+ "source": "iana"
+ },
+ "application/vnd.ecowin.seriesrequest": {
+ "source": "iana"
+ },
+ "application/vnd.ecowin.seriesupdate": {
+ "source": "iana"
+ },
+ "application/vnd.efi.img": {
+ "source": "iana"
+ },
+ "application/vnd.efi.iso": {
+ "source": "iana"
+ },
+ "application/vnd.emclient.accessrequest+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.enliven": {
+ "source": "iana",
+ "extensions": ["nml"]
+ },
+ "application/vnd.enphase.envoy": {
+ "source": "iana"
+ },
+ "application/vnd.eprints.data+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.epson.esf": {
+ "source": "iana",
+ "extensions": ["esf"]
+ },
+ "application/vnd.epson.msf": {
+ "source": "iana",
+ "extensions": ["msf"]
+ },
+ "application/vnd.epson.quickanime": {
+ "source": "iana",
+ "extensions": ["qam"]
+ },
+ "application/vnd.epson.salt": {
+ "source": "iana",
+ "extensions": ["slt"]
+ },
+ "application/vnd.epson.ssf": {
+ "source": "iana",
+ "extensions": ["ssf"]
+ },
+ "application/vnd.ericsson.quickcall": {
+ "source": "iana"
+ },
+ "application/vnd.espass-espass+zip": {
+ "source": "iana",
+ "compressible": false
+ },
+ "application/vnd.eszigno3+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["es3","et3"]
+ },
+ "application/vnd.etsi.aoc+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.etsi.asic-e+zip": {
+ "source": "iana",
+ "compressible": false
+ },
+ "application/vnd.etsi.asic-s+zip": {
+ "source": "iana",
+ "compressible": false
+ },
+ "application/vnd.etsi.cug+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.etsi.iptvcommand+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.etsi.iptvdiscovery+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.etsi.iptvprofile+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.etsi.iptvsad-bc+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.etsi.iptvsad-cod+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.etsi.iptvsad-npvr+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.etsi.iptvservice+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.etsi.iptvsync+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.etsi.iptvueprofile+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.etsi.mcid+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.etsi.mheg5": {
+ "source": "iana"
+ },
+ "application/vnd.etsi.overload-control-policy-dataset+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.etsi.pstn+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.etsi.sci+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.etsi.simservs+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.etsi.timestamp-token": {
+ "source": "iana"
+ },
+ "application/vnd.etsi.tsl+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.etsi.tsl.der": {
+ "source": "iana"
+ },
+ "application/vnd.eudora.data": {
+ "source": "iana"
+ },
+ "application/vnd.evolv.ecig.profile": {
+ "source": "iana"
+ },
+ "application/vnd.evolv.ecig.settings": {
+ "source": "iana"
+ },
+ "application/vnd.evolv.ecig.theme": {
+ "source": "iana"
+ },
+ "application/vnd.exstream-empower+zip": {
+ "source": "iana",
+ "compressible": false
+ },
+ "application/vnd.ezpix-album": {
+ "source": "iana",
+ "extensions": ["ez2"]
+ },
+ "application/vnd.ezpix-package": {
+ "source": "iana",
+ "extensions": ["ez3"]
+ },
+ "application/vnd.f-secure.mobile": {
+ "source": "iana"
+ },
+ "application/vnd.fastcopy-disk-image": {
+ "source": "iana"
+ },
+ "application/vnd.fdf": {
+ "source": "iana",
+ "extensions": ["fdf"]
+ },
+ "application/vnd.fdsn.mseed": {
+ "source": "iana",
+ "extensions": ["mseed"]
+ },
+ "application/vnd.fdsn.seed": {
+ "source": "iana",
+ "extensions": ["seed","dataless"]
+ },
+ "application/vnd.ffsns": {
+ "source": "iana"
+ },
+ "application/vnd.filmit.zfc": {
+ "source": "iana"
+ },
+ "application/vnd.fints": {
+ "source": "iana"
+ },
+ "application/vnd.firemonkeys.cloudcell": {
+ "source": "iana"
+ },
+ "application/vnd.flographit": {
+ "source": "iana",
+ "extensions": ["gph"]
+ },
+ "application/vnd.fluxtime.clip": {
+ "source": "iana",
+ "extensions": ["ftc"]
+ },
+ "application/vnd.font-fontforge-sfd": {
+ "source": "iana"
+ },
+ "application/vnd.framemaker": {
+ "source": "iana",
+ "extensions": ["fm","frame","maker","book"]
+ },
+ "application/vnd.frogans.fnc": {
+ "source": "iana",
+ "extensions": ["fnc"]
+ },
+ "application/vnd.frogans.ltf": {
+ "source": "iana",
+ "extensions": ["ltf"]
+ },
+ "application/vnd.fsc.weblaunch": {
+ "source": "iana",
+ "extensions": ["fsc"]
+ },
+ "application/vnd.fujitsu.oasys": {
+ "source": "iana",
+ "extensions": ["oas"]
+ },
+ "application/vnd.fujitsu.oasys2": {
+ "source": "iana",
+ "extensions": ["oa2"]
+ },
+ "application/vnd.fujitsu.oasys3": {
+ "source": "iana",
+ "extensions": ["oa3"]
+ },
+ "application/vnd.fujitsu.oasysgp": {
+ "source": "iana",
+ "extensions": ["fg5"]
+ },
+ "application/vnd.fujitsu.oasysprs": {
+ "source": "iana",
+ "extensions": ["bh2"]
+ },
+ "application/vnd.fujixerox.art-ex": {
+ "source": "iana"
+ },
+ "application/vnd.fujixerox.art4": {
+ "source": "iana"
+ },
+ "application/vnd.fujixerox.ddd": {
+ "source": "iana",
+ "extensions": ["ddd"]
+ },
+ "application/vnd.fujixerox.docuworks": {
+ "source": "iana",
+ "extensions": ["xdw"]
+ },
+ "application/vnd.fujixerox.docuworks.binder": {
+ "source": "iana",
+ "extensions": ["xbd"]
+ },
+ "application/vnd.fujixerox.docuworks.container": {
+ "source": "iana"
+ },
+ "application/vnd.fujixerox.hbpl": {
+ "source": "iana"
+ },
+ "application/vnd.fut-misnet": {
+ "source": "iana"
+ },
+ "application/vnd.futoin+cbor": {
+ "source": "iana"
+ },
+ "application/vnd.futoin+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.fuzzysheet": {
+ "source": "iana",
+ "extensions": ["fzs"]
+ },
+ "application/vnd.genomatix.tuxedo": {
+ "source": "iana",
+ "extensions": ["txd"]
+ },
+ "application/vnd.geo+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.geocube+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.geogebra.file": {
+ "source": "iana",
+ "extensions": ["ggb"]
+ },
+ "application/vnd.geogebra.tool": {
+ "source": "iana",
+ "extensions": ["ggt"]
+ },
+ "application/vnd.geometry-explorer": {
+ "source": "iana",
+ "extensions": ["gex","gre"]
+ },
+ "application/vnd.geonext": {
+ "source": "iana",
+ "extensions": ["gxt"]
+ },
+ "application/vnd.geoplan": {
+ "source": "iana",
+ "extensions": ["g2w"]
+ },
+ "application/vnd.geospace": {
+ "source": "iana",
+ "extensions": ["g3w"]
+ },
+ "application/vnd.gerber": {
+ "source": "iana"
+ },
+ "application/vnd.globalplatform.card-content-mgt": {
+ "source": "iana"
+ },
+ "application/vnd.globalplatform.card-content-mgt-response": {
+ "source": "iana"
+ },
+ "application/vnd.gmx": {
+ "source": "iana",
+ "extensions": ["gmx"]
+ },
+ "application/vnd.google-apps.document": {
+ "compressible": false,
+ "extensions": ["gdoc"]
+ },
+ "application/vnd.google-apps.presentation": {
+ "compressible": false,
+ "extensions": ["gslides"]
+ },
+ "application/vnd.google-apps.spreadsheet": {
+ "compressible": false,
+ "extensions": ["gsheet"]
+ },
+ "application/vnd.google-earth.kml+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["kml"]
+ },
+ "application/vnd.google-earth.kmz": {
+ "source": "iana",
+ "compressible": false,
+ "extensions": ["kmz"]
+ },
+ "application/vnd.gov.sk.e-form+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.gov.sk.e-form+zip": {
+ "source": "iana",
+ "compressible": false
+ },
+ "application/vnd.gov.sk.xmldatacontainer+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.grafeq": {
+ "source": "iana",
+ "extensions": ["gqf","gqs"]
+ },
+ "application/vnd.gridmp": {
+ "source": "iana"
+ },
+ "application/vnd.groove-account": {
+ "source": "iana",
+ "extensions": ["gac"]
+ },
+ "application/vnd.groove-help": {
+ "source": "iana",
+ "extensions": ["ghf"]
+ },
+ "application/vnd.groove-identity-message": {
+ "source": "iana",
+ "extensions": ["gim"]
+ },
+ "application/vnd.groove-injector": {
+ "source": "iana",
+ "extensions": ["grv"]
+ },
+ "application/vnd.groove-tool-message": {
+ "source": "iana",
+ "extensions": ["gtm"]
+ },
+ "application/vnd.groove-tool-template": {
+ "source": "iana",
+ "extensions": ["tpl"]
+ },
+ "application/vnd.groove-vcard": {
+ "source": "iana",
+ "extensions": ["vcg"]
+ },
+ "application/vnd.hal+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.hal+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["hal"]
+ },
+ "application/vnd.handheld-entertainment+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["zmm"]
+ },
+ "application/vnd.hbci": {
+ "source": "iana",
+ "extensions": ["hbci"]
+ },
+ "application/vnd.hc+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.hcl-bireports": {
+ "source": "iana"
+ },
+ "application/vnd.hdt": {
+ "source": "iana"
+ },
+ "application/vnd.heroku+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.hhe.lesson-player": {
+ "source": "iana",
+ "extensions": ["les"]
+ },
+ "application/vnd.hp-hpgl": {
+ "source": "iana",
+ "extensions": ["hpgl"]
+ },
+ "application/vnd.hp-hpid": {
+ "source": "iana",
+ "extensions": ["hpid"]
+ },
+ "application/vnd.hp-hps": {
+ "source": "iana",
+ "extensions": ["hps"]
+ },
+ "application/vnd.hp-jlyt": {
+ "source": "iana",
+ "extensions": ["jlt"]
+ },
+ "application/vnd.hp-pcl": {
+ "source": "iana",
+ "extensions": ["pcl"]
+ },
+ "application/vnd.hp-pclxl": {
+ "source": "iana",
+ "extensions": ["pclxl"]
+ },
+ "application/vnd.httphone": {
+ "source": "iana"
+ },
+ "application/vnd.hydrostatix.sof-data": {
+ "source": "iana",
+ "extensions": ["sfd-hdstx"]
+ },
+ "application/vnd.hyper+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.hyper-item+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.hyperdrive+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.hzn-3d-crossword": {
+ "source": "iana"
+ },
+ "application/vnd.ibm.afplinedata": {
+ "source": "iana"
+ },
+ "application/vnd.ibm.electronic-media": {
+ "source": "iana"
+ },
+ "application/vnd.ibm.minipay": {
+ "source": "iana",
+ "extensions": ["mpy"]
+ },
+ "application/vnd.ibm.modcap": {
+ "source": "iana",
+ "extensions": ["afp","listafp","list3820"]
+ },
+ "application/vnd.ibm.rights-management": {
+ "source": "iana",
+ "extensions": ["irm"]
+ },
+ "application/vnd.ibm.secure-container": {
+ "source": "iana",
+ "extensions": ["sc"]
+ },
+ "application/vnd.iccprofile": {
+ "source": "iana",
+ "extensions": ["icc","icm"]
+ },
+ "application/vnd.ieee.1905": {
+ "source": "iana"
+ },
+ "application/vnd.igloader": {
+ "source": "iana",
+ "extensions": ["igl"]
+ },
+ "application/vnd.imagemeter.folder+zip": {
+ "source": "iana",
+ "compressible": false
+ },
+ "application/vnd.imagemeter.image+zip": {
+ "source": "iana",
+ "compressible": false
+ },
+ "application/vnd.immervision-ivp": {
+ "source": "iana",
+ "extensions": ["ivp"]
+ },
+ "application/vnd.immervision-ivu": {
+ "source": "iana",
+ "extensions": ["ivu"]
+ },
+ "application/vnd.ims.imsccv1p1": {
+ "source": "iana"
+ },
+ "application/vnd.ims.imsccv1p2": {
+ "source": "iana"
+ },
+ "application/vnd.ims.imsccv1p3": {
+ "source": "iana"
+ },
+ "application/vnd.ims.lis.v2.result+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.ims.lti.v2.toolconsumerprofile+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.ims.lti.v2.toolproxy+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.ims.lti.v2.toolproxy.id+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.ims.lti.v2.toolsettings+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.ims.lti.v2.toolsettings.simple+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.informedcontrol.rms+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.informix-visionary": {
+ "source": "iana"
+ },
+ "application/vnd.infotech.project": {
+ "source": "iana"
+ },
+ "application/vnd.infotech.project+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.innopath.wamp.notification": {
+ "source": "iana"
+ },
+ "application/vnd.insors.igm": {
+ "source": "iana",
+ "extensions": ["igm"]
+ },
+ "application/vnd.intercon.formnet": {
+ "source": "iana",
+ "extensions": ["xpw","xpx"]
+ },
+ "application/vnd.intergeo": {
+ "source": "iana",
+ "extensions": ["i2g"]
+ },
+ "application/vnd.intertrust.digibox": {
+ "source": "iana"
+ },
+ "application/vnd.intertrust.nncp": {
+ "source": "iana"
+ },
+ "application/vnd.intu.qbo": {
+ "source": "iana",
+ "extensions": ["qbo"]
+ },
+ "application/vnd.intu.qfx": {
+ "source": "iana",
+ "extensions": ["qfx"]
+ },
+ "application/vnd.iptc.g2.catalogitem+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.iptc.g2.conceptitem+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.iptc.g2.knowledgeitem+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.iptc.g2.newsitem+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.iptc.g2.newsmessage+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.iptc.g2.packageitem+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.iptc.g2.planningitem+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.ipunplugged.rcprofile": {
+ "source": "iana",
+ "extensions": ["rcprofile"]
+ },
+ "application/vnd.irepository.package+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["irp"]
+ },
+ "application/vnd.is-xpr": {
+ "source": "iana",
+ "extensions": ["xpr"]
+ },
+ "application/vnd.isac.fcs": {
+ "source": "iana",
+ "extensions": ["fcs"]
+ },
+ "application/vnd.jam": {
+ "source": "iana",
+ "extensions": ["jam"]
+ },
+ "application/vnd.japannet-directory-service": {
+ "source": "iana"
+ },
+ "application/vnd.japannet-jpnstore-wakeup": {
+ "source": "iana"
+ },
+ "application/vnd.japannet-payment-wakeup": {
+ "source": "iana"
+ },
+ "application/vnd.japannet-registration": {
+ "source": "iana"
+ },
+ "application/vnd.japannet-registration-wakeup": {
+ "source": "iana"
+ },
+ "application/vnd.japannet-setstore-wakeup": {
+ "source": "iana"
+ },
+ "application/vnd.japannet-verification": {
+ "source": "iana"
+ },
+ "application/vnd.japannet-verification-wakeup": {
+ "source": "iana"
+ },
+ "application/vnd.jcp.javame.midlet-rms": {
+ "source": "iana",
+ "extensions": ["rms"]
+ },
+ "application/vnd.jisp": {
+ "source": "iana",
+ "extensions": ["jisp"]
+ },
+ "application/vnd.joost.joda-archive": {
+ "source": "iana",
+ "extensions": ["joda"]
+ },
+ "application/vnd.jsk.isdn-ngn": {
+ "source": "iana"
+ },
+ "application/vnd.kahootz": {
+ "source": "iana",
+ "extensions": ["ktz","ktr"]
+ },
+ "application/vnd.kde.karbon": {
+ "source": "iana",
+ "extensions": ["karbon"]
+ },
+ "application/vnd.kde.kchart": {
+ "source": "iana",
+ "extensions": ["chrt"]
+ },
+ "application/vnd.kde.kformula": {
+ "source": "iana",
+ "extensions": ["kfo"]
+ },
+ "application/vnd.kde.kivio": {
+ "source": "iana",
+ "extensions": ["flw"]
+ },
+ "application/vnd.kde.kontour": {
+ "source": "iana",
+ "extensions": ["kon"]
+ },
+ "application/vnd.kde.kpresenter": {
+ "source": "iana",
+ "extensions": ["kpr","kpt"]
+ },
+ "application/vnd.kde.kspread": {
+ "source": "iana",
+ "extensions": ["ksp"]
+ },
+ "application/vnd.kde.kword": {
+ "source": "iana",
+ "extensions": ["kwd","kwt"]
+ },
+ "application/vnd.kenameaapp": {
+ "source": "iana",
+ "extensions": ["htke"]
+ },
+ "application/vnd.kidspiration": {
+ "source": "iana",
+ "extensions": ["kia"]
+ },
+ "application/vnd.kinar": {
+ "source": "iana",
+ "extensions": ["kne","knp"]
+ },
+ "application/vnd.koan": {
+ "source": "iana",
+ "extensions": ["skp","skd","skt","skm"]
+ },
+ "application/vnd.kodak-descriptor": {
+ "source": "iana",
+ "extensions": ["sse"]
+ },
+ "application/vnd.las.las+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.las.las+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["lasxml"]
+ },
+ "application/vnd.leap+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.liberty-request+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.llamagraphics.life-balance.desktop": {
+ "source": "iana",
+ "extensions": ["lbd"]
+ },
+ "application/vnd.llamagraphics.life-balance.exchange+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["lbe"]
+ },
+ "application/vnd.lotus-1-2-3": {
+ "source": "iana",
+ "extensions": ["123"]
+ },
+ "application/vnd.lotus-approach": {
+ "source": "iana",
+ "extensions": ["apr"]
+ },
+ "application/vnd.lotus-freelance": {
+ "source": "iana",
+ "extensions": ["pre"]
+ },
+ "application/vnd.lotus-notes": {
+ "source": "iana",
+ "extensions": ["nsf"]
+ },
+ "application/vnd.lotus-organizer": {
+ "source": "iana",
+ "extensions": ["org"]
+ },
+ "application/vnd.lotus-screencam": {
+ "source": "iana",
+ "extensions": ["scm"]
+ },
+ "application/vnd.lotus-wordpro": {
+ "source": "iana",
+ "extensions": ["lwp"]
+ },
+ "application/vnd.macports.portpkg": {
+ "source": "iana",
+ "extensions": ["portpkg"]
+ },
+ "application/vnd.mapbox-vector-tile": {
+ "source": "iana"
+ },
+ "application/vnd.marlin.drm.actiontoken+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.marlin.drm.conftoken+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.marlin.drm.license+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.marlin.drm.mdcf": {
+ "source": "iana"
+ },
+ "application/vnd.mason+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.maxmind.maxmind-db": {
+ "source": "iana"
+ },
+ "application/vnd.mcd": {
+ "source": "iana",
+ "extensions": ["mcd"]
+ },
+ "application/vnd.medcalcdata": {
+ "source": "iana",
+ "extensions": ["mc1"]
+ },
+ "application/vnd.mediastation.cdkey": {
+ "source": "iana",
+ "extensions": ["cdkey"]
+ },
+ "application/vnd.meridian-slingshot": {
+ "source": "iana"
+ },
+ "application/vnd.mfer": {
+ "source": "iana",
+ "extensions": ["mwf"]
+ },
+ "application/vnd.mfmp": {
+ "source": "iana",
+ "extensions": ["mfm"]
+ },
+ "application/vnd.micro+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.micrografx.flo": {
+ "source": "iana",
+ "extensions": ["flo"]
+ },
+ "application/vnd.micrografx.igx": {
+ "source": "iana",
+ "extensions": ["igx"]
+ },
+ "application/vnd.microsoft.portable-executable": {
+ "source": "iana"
+ },
+ "application/vnd.microsoft.windows.thumbnail-cache": {
+ "source": "iana"
+ },
+ "application/vnd.miele+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.mif": {
+ "source": "iana",
+ "extensions": ["mif"]
+ },
+ "application/vnd.minisoft-hp3000-save": {
+ "source": "iana"
+ },
+ "application/vnd.mitsubishi.misty-guard.trustweb": {
+ "source": "iana"
+ },
+ "application/vnd.mobius.daf": {
+ "source": "iana",
+ "extensions": ["daf"]
+ },
+ "application/vnd.mobius.dis": {
+ "source": "iana",
+ "extensions": ["dis"]
+ },
+ "application/vnd.mobius.mbk": {
+ "source": "iana",
+ "extensions": ["mbk"]
+ },
+ "application/vnd.mobius.mqy": {
+ "source": "iana",
+ "extensions": ["mqy"]
+ },
+ "application/vnd.mobius.msl": {
+ "source": "iana",
+ "extensions": ["msl"]
+ },
+ "application/vnd.mobius.plc": {
+ "source": "iana",
+ "extensions": ["plc"]
+ },
+ "application/vnd.mobius.txf": {
+ "source": "iana",
+ "extensions": ["txf"]
+ },
+ "application/vnd.mophun.application": {
+ "source": "iana",
+ "extensions": ["mpn"]
+ },
+ "application/vnd.mophun.certificate": {
+ "source": "iana",
+ "extensions": ["mpc"]
+ },
+ "application/vnd.motorola.flexsuite": {
+ "source": "iana"
+ },
+ "application/vnd.motorola.flexsuite.adsi": {
+ "source": "iana"
+ },
+ "application/vnd.motorola.flexsuite.fis": {
+ "source": "iana"
+ },
+ "application/vnd.motorola.flexsuite.gotap": {
+ "source": "iana"
+ },
+ "application/vnd.motorola.flexsuite.kmr": {
+ "source": "iana"
+ },
+ "application/vnd.motorola.flexsuite.ttc": {
+ "source": "iana"
+ },
+ "application/vnd.motorola.flexsuite.wem": {
+ "source": "iana"
+ },
+ "application/vnd.motorola.iprm": {
+ "source": "iana"
+ },
+ "application/vnd.mozilla.xul+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["xul"]
+ },
+ "application/vnd.ms-3mfdocument": {
+ "source": "iana"
+ },
+ "application/vnd.ms-artgalry": {
+ "source": "iana",
+ "extensions": ["cil"]
+ },
+ "application/vnd.ms-asf": {
+ "source": "iana"
+ },
+ "application/vnd.ms-cab-compressed": {
+ "source": "iana",
+ "extensions": ["cab"]
+ },
+ "application/vnd.ms-color.iccprofile": {
+ "source": "apache"
+ },
+ "application/vnd.ms-excel": {
+ "source": "iana",
+ "compressible": false,
+ "extensions": ["xls","xlm","xla","xlc","xlt","xlw"]
+ },
+ "application/vnd.ms-excel.addin.macroenabled.12": {
+ "source": "iana",
+ "extensions": ["xlam"]
+ },
+ "application/vnd.ms-excel.sheet.binary.macroenabled.12": {
+ "source": "iana",
+ "extensions": ["xlsb"]
+ },
+ "application/vnd.ms-excel.sheet.macroenabled.12": {
+ "source": "iana",
+ "extensions": ["xlsm"]
+ },
+ "application/vnd.ms-excel.template.macroenabled.12": {
+ "source": "iana",
+ "extensions": ["xltm"]
+ },
+ "application/vnd.ms-fontobject": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["eot"]
+ },
+ "application/vnd.ms-htmlhelp": {
+ "source": "iana",
+ "extensions": ["chm"]
+ },
+ "application/vnd.ms-ims": {
+ "source": "iana",
+ "extensions": ["ims"]
+ },
+ "application/vnd.ms-lrm": {
+ "source": "iana",
+ "extensions": ["lrm"]
+ },
+ "application/vnd.ms-office.activex+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.ms-officetheme": {
+ "source": "iana",
+ "extensions": ["thmx"]
+ },
+ "application/vnd.ms-opentype": {
+ "source": "apache",
+ "compressible": true
+ },
+ "application/vnd.ms-outlook": {
+ "compressible": false,
+ "extensions": ["msg"]
+ },
+ "application/vnd.ms-package.obfuscated-opentype": {
+ "source": "apache"
+ },
+ "application/vnd.ms-pki.seccat": {
+ "source": "apache",
+ "extensions": ["cat"]
+ },
+ "application/vnd.ms-pki.stl": {
+ "source": "apache",
+ "extensions": ["stl"]
+ },
+ "application/vnd.ms-playready.initiator+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.ms-powerpoint": {
+ "source": "iana",
+ "compressible": false,
+ "extensions": ["ppt","pps","pot"]
+ },
+ "application/vnd.ms-powerpoint.addin.macroenabled.12": {
+ "source": "iana",
+ "extensions": ["ppam"]
+ },
+ "application/vnd.ms-powerpoint.presentation.macroenabled.12": {
+ "source": "iana",
+ "extensions": ["pptm"]
+ },
+ "application/vnd.ms-powerpoint.slide.macroenabled.12": {
+ "source": "iana",
+ "extensions": ["sldm"]
+ },
+ "application/vnd.ms-powerpoint.slideshow.macroenabled.12": {
+ "source": "iana",
+ "extensions": ["ppsm"]
+ },
+ "application/vnd.ms-powerpoint.template.macroenabled.12": {
+ "source": "iana",
+ "extensions": ["potm"]
+ },
+ "application/vnd.ms-printdevicecapabilities+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.ms-printing.printticket+xml": {
+ "source": "apache",
+ "compressible": true
+ },
+ "application/vnd.ms-printschematicket+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.ms-project": {
+ "source": "iana",
+ "extensions": ["mpp","mpt"]
+ },
+ "application/vnd.ms-tnef": {
+ "source": "iana"
+ },
+ "application/vnd.ms-windows.devicepairing": {
+ "source": "iana"
+ },
+ "application/vnd.ms-windows.nwprinting.oob": {
+ "source": "iana"
+ },
+ "application/vnd.ms-windows.printerpairing": {
+ "source": "iana"
+ },
+ "application/vnd.ms-windows.wsd.oob": {
+ "source": "iana"
+ },
+ "application/vnd.ms-wmdrm.lic-chlg-req": {
+ "source": "iana"
+ },
+ "application/vnd.ms-wmdrm.lic-resp": {
+ "source": "iana"
+ },
+ "application/vnd.ms-wmdrm.meter-chlg-req": {
+ "source": "iana"
+ },
+ "application/vnd.ms-wmdrm.meter-resp": {
+ "source": "iana"
+ },
+ "application/vnd.ms-word.document.macroenabled.12": {
+ "source": "iana",
+ "extensions": ["docm"]
+ },
+ "application/vnd.ms-word.template.macroenabled.12": {
+ "source": "iana",
+ "extensions": ["dotm"]
+ },
+ "application/vnd.ms-works": {
+ "source": "iana",
+ "extensions": ["wps","wks","wcm","wdb"]
+ },
+ "application/vnd.ms-wpl": {
+ "source": "iana",
+ "extensions": ["wpl"]
+ },
+ "application/vnd.ms-xpsdocument": {
+ "source": "iana",
+ "compressible": false,
+ "extensions": ["xps"]
+ },
+ "application/vnd.msa-disk-image": {
+ "source": "iana"
+ },
+ "application/vnd.mseq": {
+ "source": "iana",
+ "extensions": ["mseq"]
+ },
+ "application/vnd.msign": {
+ "source": "iana"
+ },
+ "application/vnd.multiad.creator": {
+ "source": "iana"
+ },
+ "application/vnd.multiad.creator.cif": {
+ "source": "iana"
+ },
+ "application/vnd.music-niff": {
+ "source": "iana"
+ },
+ "application/vnd.musician": {
+ "source": "iana",
+ "extensions": ["mus"]
+ },
+ "application/vnd.muvee.style": {
+ "source": "iana",
+ "extensions": ["msty"]
+ },
+ "application/vnd.mynfc": {
+ "source": "iana",
+ "extensions": ["taglet"]
+ },
+ "application/vnd.ncd.control": {
+ "source": "iana"
+ },
+ "application/vnd.ncd.reference": {
+ "source": "iana"
+ },
+ "application/vnd.nearst.inv+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.nervana": {
+ "source": "iana"
+ },
+ "application/vnd.netfpx": {
+ "source": "iana"
+ },
+ "application/vnd.neurolanguage.nlu": {
+ "source": "iana",
+ "extensions": ["nlu"]
+ },
+ "application/vnd.nimn": {
+ "source": "iana"
+ },
+ "application/vnd.nintendo.nitro.rom": {
+ "source": "iana"
+ },
+ "application/vnd.nintendo.snes.rom": {
+ "source": "iana"
+ },
+ "application/vnd.nitf": {
+ "source": "iana",
+ "extensions": ["ntf","nitf"]
+ },
+ "application/vnd.noblenet-directory": {
+ "source": "iana",
+ "extensions": ["nnd"]
+ },
+ "application/vnd.noblenet-sealer": {
+ "source": "iana",
+ "extensions": ["nns"]
+ },
+ "application/vnd.noblenet-web": {
+ "source": "iana",
+ "extensions": ["nnw"]
+ },
+ "application/vnd.nokia.catalogs": {
+ "source": "iana"
+ },
+ "application/vnd.nokia.conml+wbxml": {
+ "source": "iana"
+ },
+ "application/vnd.nokia.conml+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.nokia.iptv.config+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.nokia.isds-radio-presets": {
+ "source": "iana"
+ },
+ "application/vnd.nokia.landmark+wbxml": {
+ "source": "iana"
+ },
+ "application/vnd.nokia.landmark+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.nokia.landmarkcollection+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.nokia.n-gage.ac+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.nokia.n-gage.data": {
+ "source": "iana",
+ "extensions": ["ngdat"]
+ },
+ "application/vnd.nokia.n-gage.symbian.install": {
+ "source": "iana",
+ "extensions": ["n-gage"]
+ },
+ "application/vnd.nokia.ncd": {
+ "source": "iana"
+ },
+ "application/vnd.nokia.pcd+wbxml": {
+ "source": "iana"
+ },
+ "application/vnd.nokia.pcd+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.nokia.radio-preset": {
+ "source": "iana",
+ "extensions": ["rpst"]
+ },
+ "application/vnd.nokia.radio-presets": {
+ "source": "iana",
+ "extensions": ["rpss"]
+ },
+ "application/vnd.novadigm.edm": {
+ "source": "iana",
+ "extensions": ["edm"]
+ },
+ "application/vnd.novadigm.edx": {
+ "source": "iana",
+ "extensions": ["edx"]
+ },
+ "application/vnd.novadigm.ext": {
+ "source": "iana",
+ "extensions": ["ext"]
+ },
+ "application/vnd.ntt-local.content-share": {
+ "source": "iana"
+ },
+ "application/vnd.ntt-local.file-transfer": {
+ "source": "iana"
+ },
+ "application/vnd.ntt-local.ogw_remote-access": {
+ "source": "iana"
+ },
+ "application/vnd.ntt-local.sip-ta_remote": {
+ "source": "iana"
+ },
+ "application/vnd.ntt-local.sip-ta_tcp_stream": {
+ "source": "iana"
+ },
+ "application/vnd.oasis.opendocument.chart": {
+ "source": "iana",
+ "extensions": ["odc"]
+ },
+ "application/vnd.oasis.opendocument.chart-template": {
+ "source": "iana",
+ "extensions": ["otc"]
+ },
+ "application/vnd.oasis.opendocument.database": {
+ "source": "iana",
+ "extensions": ["odb"]
+ },
+ "application/vnd.oasis.opendocument.formula": {
+ "source": "iana",
+ "extensions": ["odf"]
+ },
+ "application/vnd.oasis.opendocument.formula-template": {
+ "source": "iana",
+ "extensions": ["odft"]
+ },
+ "application/vnd.oasis.opendocument.graphics": {
+ "source": "iana",
+ "compressible": false,
+ "extensions": ["odg"]
+ },
+ "application/vnd.oasis.opendocument.graphics-template": {
+ "source": "iana",
+ "extensions": ["otg"]
+ },
+ "application/vnd.oasis.opendocument.image": {
+ "source": "iana",
+ "extensions": ["odi"]
+ },
+ "application/vnd.oasis.opendocument.image-template": {
+ "source": "iana",
+ "extensions": ["oti"]
+ },
+ "application/vnd.oasis.opendocument.presentation": {
+ "source": "iana",
+ "compressible": false,
+ "extensions": ["odp"]
+ },
+ "application/vnd.oasis.opendocument.presentation-template": {
+ "source": "iana",
+ "extensions": ["otp"]
+ },
+ "application/vnd.oasis.opendocument.spreadsheet": {
+ "source": "iana",
+ "compressible": false,
+ "extensions": ["ods"]
+ },
+ "application/vnd.oasis.opendocument.spreadsheet-template": {
+ "source": "iana",
+ "extensions": ["ots"]
+ },
+ "application/vnd.oasis.opendocument.text": {
+ "source": "iana",
+ "compressible": false,
+ "extensions": ["odt"]
+ },
+ "application/vnd.oasis.opendocument.text-master": {
+ "source": "iana",
+ "extensions": ["odm"]
+ },
+ "application/vnd.oasis.opendocument.text-template": {
+ "source": "iana",
+ "extensions": ["ott"]
+ },
+ "application/vnd.oasis.opendocument.text-web": {
+ "source": "iana",
+ "extensions": ["oth"]
+ },
+ "application/vnd.obn": {
+ "source": "iana"
+ },
+ "application/vnd.ocf+cbor": {
+ "source": "iana"
+ },
+ "application/vnd.oftn.l10n+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.oipf.contentaccessdownload+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.oipf.contentaccessstreaming+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.oipf.cspg-hexbinary": {
+ "source": "iana"
+ },
+ "application/vnd.oipf.dae.svg+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.oipf.dae.xhtml+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.oipf.mippvcontrolmessage+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.oipf.pae.gem": {
+ "source": "iana"
+ },
+ "application/vnd.oipf.spdiscovery+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.oipf.spdlist+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.oipf.ueprofile+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.oipf.userprofile+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.olpc-sugar": {
+ "source": "iana",
+ "extensions": ["xo"]
+ },
+ "application/vnd.oma-scws-config": {
+ "source": "iana"
+ },
+ "application/vnd.oma-scws-http-request": {
+ "source": "iana"
+ },
+ "application/vnd.oma-scws-http-response": {
+ "source": "iana"
+ },
+ "application/vnd.oma.bcast.associated-procedure-parameter+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.oma.bcast.drm-trigger+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.oma.bcast.imd+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.oma.bcast.ltkm": {
+ "source": "iana"
+ },
+ "application/vnd.oma.bcast.notification+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.oma.bcast.provisioningtrigger": {
+ "source": "iana"
+ },
+ "application/vnd.oma.bcast.sgboot": {
+ "source": "iana"
+ },
+ "application/vnd.oma.bcast.sgdd+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.oma.bcast.sgdu": {
+ "source": "iana"
+ },
+ "application/vnd.oma.bcast.simple-symbol-container": {
+ "source": "iana"
+ },
+ "application/vnd.oma.bcast.smartcard-trigger+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.oma.bcast.sprov+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.oma.bcast.stkm": {
+ "source": "iana"
+ },
+ "application/vnd.oma.cab-address-book+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.oma.cab-feature-handler+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.oma.cab-pcc+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.oma.cab-subs-invite+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.oma.cab-user-prefs+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.oma.dcd": {
+ "source": "iana"
+ },
+ "application/vnd.oma.dcdc": {
+ "source": "iana"
+ },
+ "application/vnd.oma.dd2+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["dd2"]
+ },
+ "application/vnd.oma.drm.risd+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.oma.group-usage-list+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.oma.lwm2m+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.oma.lwm2m+tlv": {
+ "source": "iana"
+ },
+ "application/vnd.oma.pal+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.oma.poc.detailed-progress-report+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.oma.poc.final-report+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.oma.poc.groups+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.oma.poc.invocation-descriptor+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.oma.poc.optimized-progress-report+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.oma.push": {
+ "source": "iana"
+ },
+ "application/vnd.oma.scidm.messages+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.oma.xcap-directory+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.omads-email+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.omads-file+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.omads-folder+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.omaloc-supl-init": {
+ "source": "iana"
+ },
+ "application/vnd.onepager": {
+ "source": "iana"
+ },
+ "application/vnd.onepagertamp": {
+ "source": "iana"
+ },
+ "application/vnd.onepagertamx": {
+ "source": "iana"
+ },
+ "application/vnd.onepagertat": {
+ "source": "iana"
+ },
+ "application/vnd.onepagertatp": {
+ "source": "iana"
+ },
+ "application/vnd.onepagertatx": {
+ "source": "iana"
+ },
+ "application/vnd.openblox.game+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openblox.game-binary": {
+ "source": "iana"
+ },
+ "application/vnd.openeye.oeb": {
+ "source": "iana"
+ },
+ "application/vnd.openofficeorg.extension": {
+ "source": "apache",
+ "extensions": ["oxt"]
+ },
+ "application/vnd.openstreetmap.data+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.custom-properties+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.customxmlproperties+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.drawing+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.drawingml.chart+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.drawingml.chartshapes+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.drawingml.diagramcolors+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.drawingml.diagramdata+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.drawingml.diagramlayout+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.drawingml.diagramstyle+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.extended-properties+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.presentationml.commentauthors+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.presentationml.comments+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.presentationml.handoutmaster+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.presentationml.notesmaster+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.presentationml.notesslide+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.presentationml.presentation": {
+ "source": "iana",
+ "compressible": false,
+ "extensions": ["pptx"]
+ },
+ "application/vnd.openxmlformats-officedocument.presentationml.presentation.main+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.presentationml.presprops+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.presentationml.slide": {
+ "source": "iana",
+ "extensions": ["sldx"]
+ },
+ "application/vnd.openxmlformats-officedocument.presentationml.slide+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.presentationml.slidelayout+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.presentationml.slidemaster+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.presentationml.slideshow": {
+ "source": "iana",
+ "extensions": ["ppsx"]
+ },
+ "application/vnd.openxmlformats-officedocument.presentationml.slideshow.main+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.presentationml.slideupdateinfo+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.presentationml.tablestyles+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.presentationml.tags+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.presentationml.template": {
+ "source": "iana",
+ "extensions": ["potx"]
+ },
+ "application/vnd.openxmlformats-officedocument.presentationml.template.main+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.presentationml.viewprops+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.spreadsheetml.calcchain+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.spreadsheetml.chartsheet+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.spreadsheetml.comments+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.spreadsheetml.connections+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.spreadsheetml.dialogsheet+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.spreadsheetml.externallink+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.spreadsheetml.pivotcachedefinition+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.spreadsheetml.pivotcacherecords+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.spreadsheetml.pivottable+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.spreadsheetml.querytable+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.spreadsheetml.revisionheaders+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.spreadsheetml.revisionlog+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.spreadsheetml.sharedstrings+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.spreadsheetml.sheet": {
+ "source": "iana",
+ "compressible": false,
+ "extensions": ["xlsx"]
+ },
+ "application/vnd.openxmlformats-officedocument.spreadsheetml.sheet.main+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.spreadsheetml.sheetmetadata+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.spreadsheetml.styles+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.spreadsheetml.table+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.spreadsheetml.tablesinglecells+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.spreadsheetml.template": {
+ "source": "iana",
+ "extensions": ["xltx"]
+ },
+ "application/vnd.openxmlformats-officedocument.spreadsheetml.template.main+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.spreadsheetml.usernames+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.spreadsheetml.volatiledependencies+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.spreadsheetml.worksheet+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.theme+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.themeoverride+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.vmldrawing": {
+ "source": "iana"
+ },
+ "application/vnd.openxmlformats-officedocument.wordprocessingml.comments+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.wordprocessingml.document": {
+ "source": "iana",
+ "compressible": false,
+ "extensions": ["docx"]
+ },
+ "application/vnd.openxmlformats-officedocument.wordprocessingml.document.glossary+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.wordprocessingml.document.main+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.wordprocessingml.endnotes+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.wordprocessingml.fonttable+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.wordprocessingml.footer+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.wordprocessingml.footnotes+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.wordprocessingml.numbering+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.wordprocessingml.settings+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.wordprocessingml.styles+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.wordprocessingml.template": {
+ "source": "iana",
+ "extensions": ["dotx"]
+ },
+ "application/vnd.openxmlformats-officedocument.wordprocessingml.template.main+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-officedocument.wordprocessingml.websettings+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-package.core-properties+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-package.digital-signature-xmlsignature+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.openxmlformats-package.relationships+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.oracle.resource+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.orange.indata": {
+ "source": "iana"
+ },
+ "application/vnd.osa.netdeploy": {
+ "source": "iana"
+ },
+ "application/vnd.osgeo.mapguide.package": {
+ "source": "iana",
+ "extensions": ["mgp"]
+ },
+ "application/vnd.osgi.bundle": {
+ "source": "iana"
+ },
+ "application/vnd.osgi.dp": {
+ "source": "iana",
+ "extensions": ["dp"]
+ },
+ "application/vnd.osgi.subsystem": {
+ "source": "iana",
+ "extensions": ["esa"]
+ },
+ "application/vnd.otps.ct-kip+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.oxli.countgraph": {
+ "source": "iana"
+ },
+ "application/vnd.pagerduty+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.palm": {
+ "source": "iana",
+ "extensions": ["pdb","pqa","oprc"]
+ },
+ "application/vnd.panoply": {
+ "source": "iana"
+ },
+ "application/vnd.paos.xml": {
+ "source": "iana"
+ },
+ "application/vnd.patentdive": {
+ "source": "iana"
+ },
+ "application/vnd.pawaafile": {
+ "source": "iana",
+ "extensions": ["paw"]
+ },
+ "application/vnd.pcos": {
+ "source": "iana"
+ },
+ "application/vnd.pg.format": {
+ "source": "iana",
+ "extensions": ["str"]
+ },
+ "application/vnd.pg.osasli": {
+ "source": "iana",
+ "extensions": ["ei6"]
+ },
+ "application/vnd.piaccess.application-licence": {
+ "source": "iana"
+ },
+ "application/vnd.picsel": {
+ "source": "iana",
+ "extensions": ["efif"]
+ },
+ "application/vnd.pmi.widget": {
+ "source": "iana",
+ "extensions": ["wg"]
+ },
+ "application/vnd.poc.group-advertisement+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.pocketlearn": {
+ "source": "iana",
+ "extensions": ["plf"]
+ },
+ "application/vnd.powerbuilder6": {
+ "source": "iana",
+ "extensions": ["pbd"]
+ },
+ "application/vnd.powerbuilder6-s": {
+ "source": "iana"
+ },
+ "application/vnd.powerbuilder7": {
+ "source": "iana"
+ },
+ "application/vnd.powerbuilder7-s": {
+ "source": "iana"
+ },
+ "application/vnd.powerbuilder75": {
+ "source": "iana"
+ },
+ "application/vnd.powerbuilder75-s": {
+ "source": "iana"
+ },
+ "application/vnd.preminet": {
+ "source": "iana"
+ },
+ "application/vnd.previewsystems.box": {
+ "source": "iana",
+ "extensions": ["box"]
+ },
+ "application/vnd.proteus.magazine": {
+ "source": "iana",
+ "extensions": ["mgz"]
+ },
+ "application/vnd.psfs": {
+ "source": "iana"
+ },
+ "application/vnd.publishare-delta-tree": {
+ "source": "iana",
+ "extensions": ["qps"]
+ },
+ "application/vnd.pvi.ptid1": {
+ "source": "iana",
+ "extensions": ["ptid"]
+ },
+ "application/vnd.pwg-multiplexed": {
+ "source": "iana"
+ },
+ "application/vnd.pwg-xhtml-print+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.qualcomm.brew-app-res": {
+ "source": "iana"
+ },
+ "application/vnd.quarantainenet": {
+ "source": "iana"
+ },
+ "application/vnd.quark.quarkxpress": {
+ "source": "iana",
+ "extensions": ["qxd","qxt","qwd","qwt","qxl","qxb"]
+ },
+ "application/vnd.quobject-quoxdocument": {
+ "source": "iana"
+ },
+ "application/vnd.radisys.moml+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.radisys.msml+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.radisys.msml-audit+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.radisys.msml-audit-conf+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.radisys.msml-audit-conn+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.radisys.msml-audit-dialog+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.radisys.msml-audit-stream+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.radisys.msml-conf+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.radisys.msml-dialog+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.radisys.msml-dialog-base+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.radisys.msml-dialog-fax-detect+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.radisys.msml-dialog-fax-sendrecv+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.radisys.msml-dialog-group+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.radisys.msml-dialog-speech+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.radisys.msml-dialog-transform+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.rainstor.data": {
+ "source": "iana"
+ },
+ "application/vnd.rapid": {
+ "source": "iana"
+ },
+ "application/vnd.rar": {
+ "source": "iana"
+ },
+ "application/vnd.realvnc.bed": {
+ "source": "iana",
+ "extensions": ["bed"]
+ },
+ "application/vnd.recordare.musicxml": {
+ "source": "iana",
+ "extensions": ["mxl"]
+ },
+ "application/vnd.recordare.musicxml+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["musicxml"]
+ },
+ "application/vnd.renlearn.rlprint": {
+ "source": "iana"
+ },
+ "application/vnd.restful+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.rig.cryptonote": {
+ "source": "iana",
+ "extensions": ["cryptonote"]
+ },
+ "application/vnd.rim.cod": {
+ "source": "apache",
+ "extensions": ["cod"]
+ },
+ "application/vnd.rn-realmedia": {
+ "source": "apache",
+ "extensions": ["rm"]
+ },
+ "application/vnd.rn-realmedia-vbr": {
+ "source": "apache",
+ "extensions": ["rmvb"]
+ },
+ "application/vnd.route66.link66+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["link66"]
+ },
+ "application/vnd.rs-274x": {
+ "source": "iana"
+ },
+ "application/vnd.ruckus.download": {
+ "source": "iana"
+ },
+ "application/vnd.s3sms": {
+ "source": "iana"
+ },
+ "application/vnd.sailingtracker.track": {
+ "source": "iana",
+ "extensions": ["st"]
+ },
+ "application/vnd.sbm.cid": {
+ "source": "iana"
+ },
+ "application/vnd.sbm.mid2": {
+ "source": "iana"
+ },
+ "application/vnd.scribus": {
+ "source": "iana"
+ },
+ "application/vnd.sealed.3df": {
+ "source": "iana"
+ },
+ "application/vnd.sealed.csf": {
+ "source": "iana"
+ },
+ "application/vnd.sealed.doc": {
+ "source": "iana"
+ },
+ "application/vnd.sealed.eml": {
+ "source": "iana"
+ },
+ "application/vnd.sealed.mht": {
+ "source": "iana"
+ },
+ "application/vnd.sealed.net": {
+ "source": "iana"
+ },
+ "application/vnd.sealed.ppt": {
+ "source": "iana"
+ },
+ "application/vnd.sealed.tiff": {
+ "source": "iana"
+ },
+ "application/vnd.sealed.xls": {
+ "source": "iana"
+ },
+ "application/vnd.sealedmedia.softseal.html": {
+ "source": "iana"
+ },
+ "application/vnd.sealedmedia.softseal.pdf": {
+ "source": "iana"
+ },
+ "application/vnd.seemail": {
+ "source": "iana",
+ "extensions": ["see"]
+ },
+ "application/vnd.sema": {
+ "source": "iana",
+ "extensions": ["sema"]
+ },
+ "application/vnd.semd": {
+ "source": "iana",
+ "extensions": ["semd"]
+ },
+ "application/vnd.semf": {
+ "source": "iana",
+ "extensions": ["semf"]
+ },
+ "application/vnd.shana.informed.formdata": {
+ "source": "iana",
+ "extensions": ["ifm"]
+ },
+ "application/vnd.shana.informed.formtemplate": {
+ "source": "iana",
+ "extensions": ["itp"]
+ },
+ "application/vnd.shana.informed.interchange": {
+ "source": "iana",
+ "extensions": ["iif"]
+ },
+ "application/vnd.shana.informed.package": {
+ "source": "iana",
+ "extensions": ["ipk"]
+ },
+ "application/vnd.shootproof+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.sigrok.session": {
+ "source": "iana"
+ },
+ "application/vnd.simtech-mindmapper": {
+ "source": "iana",
+ "extensions": ["twd","twds"]
+ },
+ "application/vnd.siren+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.smaf": {
+ "source": "iana",
+ "extensions": ["mmf"]
+ },
+ "application/vnd.smart.notebook": {
+ "source": "iana"
+ },
+ "application/vnd.smart.teacher": {
+ "source": "iana",
+ "extensions": ["teacher"]
+ },
+ "application/vnd.software602.filler.form+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.software602.filler.form-xml-zip": {
+ "source": "iana"
+ },
+ "application/vnd.solent.sdkm+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["sdkm","sdkd"]
+ },
+ "application/vnd.spotfire.dxp": {
+ "source": "iana",
+ "extensions": ["dxp"]
+ },
+ "application/vnd.spotfire.sfs": {
+ "source": "iana",
+ "extensions": ["sfs"]
+ },
+ "application/vnd.sqlite3": {
+ "source": "iana"
+ },
+ "application/vnd.sss-cod": {
+ "source": "iana"
+ },
+ "application/vnd.sss-dtf": {
+ "source": "iana"
+ },
+ "application/vnd.sss-ntf": {
+ "source": "iana"
+ },
+ "application/vnd.stardivision.calc": {
+ "source": "apache",
+ "extensions": ["sdc"]
+ },
+ "application/vnd.stardivision.draw": {
+ "source": "apache",
+ "extensions": ["sda"]
+ },
+ "application/vnd.stardivision.impress": {
+ "source": "apache",
+ "extensions": ["sdd"]
+ },
+ "application/vnd.stardivision.math": {
+ "source": "apache",
+ "extensions": ["smf"]
+ },
+ "application/vnd.stardivision.writer": {
+ "source": "apache",
+ "extensions": ["sdw","vor"]
+ },
+ "application/vnd.stardivision.writer-global": {
+ "source": "apache",
+ "extensions": ["sgl"]
+ },
+ "application/vnd.stepmania.package": {
+ "source": "iana",
+ "extensions": ["smzip"]
+ },
+ "application/vnd.stepmania.stepchart": {
+ "source": "iana",
+ "extensions": ["sm"]
+ },
+ "application/vnd.street-stream": {
+ "source": "iana"
+ },
+ "application/vnd.sun.wadl+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["wadl"]
+ },
+ "application/vnd.sun.xml.calc": {
+ "source": "apache",
+ "extensions": ["sxc"]
+ },
+ "application/vnd.sun.xml.calc.template": {
+ "source": "apache",
+ "extensions": ["stc"]
+ },
+ "application/vnd.sun.xml.draw": {
+ "source": "apache",
+ "extensions": ["sxd"]
+ },
+ "application/vnd.sun.xml.draw.template": {
+ "source": "apache",
+ "extensions": ["std"]
+ },
+ "application/vnd.sun.xml.impress": {
+ "source": "apache",
+ "extensions": ["sxi"]
+ },
+ "application/vnd.sun.xml.impress.template": {
+ "source": "apache",
+ "extensions": ["sti"]
+ },
+ "application/vnd.sun.xml.math": {
+ "source": "apache",
+ "extensions": ["sxm"]
+ },
+ "application/vnd.sun.xml.writer": {
+ "source": "apache",
+ "extensions": ["sxw"]
+ },
+ "application/vnd.sun.xml.writer.global": {
+ "source": "apache",
+ "extensions": ["sxg"]
+ },
+ "application/vnd.sun.xml.writer.template": {
+ "source": "apache",
+ "extensions": ["stw"]
+ },
+ "application/vnd.sus-calendar": {
+ "source": "iana",
+ "extensions": ["sus","susp"]
+ },
+ "application/vnd.svd": {
+ "source": "iana",
+ "extensions": ["svd"]
+ },
+ "application/vnd.swiftview-ics": {
+ "source": "iana"
+ },
+ "application/vnd.symbian.install": {
+ "source": "apache",
+ "extensions": ["sis","sisx"]
+ },
+ "application/vnd.syncml+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["xsm"]
+ },
+ "application/vnd.syncml.dm+wbxml": {
+ "source": "iana",
+ "extensions": ["bdm"]
+ },
+ "application/vnd.syncml.dm+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["xdm"]
+ },
+ "application/vnd.syncml.dm.notification": {
+ "source": "iana"
+ },
+ "application/vnd.syncml.dmddf+wbxml": {
+ "source": "iana"
+ },
+ "application/vnd.syncml.dmddf+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.syncml.dmtnds+wbxml": {
+ "source": "iana"
+ },
+ "application/vnd.syncml.dmtnds+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.syncml.ds.notification": {
+ "source": "iana"
+ },
+ "application/vnd.tableschema+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.tao.intent-module-archive": {
+ "source": "iana",
+ "extensions": ["tao"]
+ },
+ "application/vnd.tcpdump.pcap": {
+ "source": "iana",
+ "extensions": ["pcap","cap","dmp"]
+ },
+ "application/vnd.think-cell.ppttc+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.tmd.mediaflex.api+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.tml": {
+ "source": "iana"
+ },
+ "application/vnd.tmobile-livetv": {
+ "source": "iana",
+ "extensions": ["tmo"]
+ },
+ "application/vnd.tri.onesource": {
+ "source": "iana"
+ },
+ "application/vnd.trid.tpt": {
+ "source": "iana",
+ "extensions": ["tpt"]
+ },
+ "application/vnd.triscape.mxs": {
+ "source": "iana",
+ "extensions": ["mxs"]
+ },
+ "application/vnd.trueapp": {
+ "source": "iana",
+ "extensions": ["tra"]
+ },
+ "application/vnd.truedoc": {
+ "source": "iana"
+ },
+ "application/vnd.ubisoft.webplayer": {
+ "source": "iana"
+ },
+ "application/vnd.ufdl": {
+ "source": "iana",
+ "extensions": ["ufd","ufdl"]
+ },
+ "application/vnd.uiq.theme": {
+ "source": "iana",
+ "extensions": ["utz"]
+ },
+ "application/vnd.umajin": {
+ "source": "iana",
+ "extensions": ["umj"]
+ },
+ "application/vnd.unity": {
+ "source": "iana",
+ "extensions": ["unityweb"]
+ },
+ "application/vnd.uoml+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["uoml"]
+ },
+ "application/vnd.uplanet.alert": {
+ "source": "iana"
+ },
+ "application/vnd.uplanet.alert-wbxml": {
+ "source": "iana"
+ },
+ "application/vnd.uplanet.bearer-choice": {
+ "source": "iana"
+ },
+ "application/vnd.uplanet.bearer-choice-wbxml": {
+ "source": "iana"
+ },
+ "application/vnd.uplanet.cacheop": {
+ "source": "iana"
+ },
+ "application/vnd.uplanet.cacheop-wbxml": {
+ "source": "iana"
+ },
+ "application/vnd.uplanet.channel": {
+ "source": "iana"
+ },
+ "application/vnd.uplanet.channel-wbxml": {
+ "source": "iana"
+ },
+ "application/vnd.uplanet.list": {
+ "source": "iana"
+ },
+ "application/vnd.uplanet.list-wbxml": {
+ "source": "iana"
+ },
+ "application/vnd.uplanet.listcmd": {
+ "source": "iana"
+ },
+ "application/vnd.uplanet.listcmd-wbxml": {
+ "source": "iana"
+ },
+ "application/vnd.uplanet.signal": {
+ "source": "iana"
+ },
+ "application/vnd.uri-map": {
+ "source": "iana"
+ },
+ "application/vnd.valve.source.material": {
+ "source": "iana"
+ },
+ "application/vnd.vcx": {
+ "source": "iana",
+ "extensions": ["vcx"]
+ },
+ "application/vnd.vd-study": {
+ "source": "iana"
+ },
+ "application/vnd.vectorworks": {
+ "source": "iana"
+ },
+ "application/vnd.vel+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.verimatrix.vcas": {
+ "source": "iana"
+ },
+ "application/vnd.vidsoft.vidconference": {
+ "source": "iana"
+ },
+ "application/vnd.visio": {
+ "source": "iana",
+ "extensions": ["vsd","vst","vss","vsw"]
+ },
+ "application/vnd.visionary": {
+ "source": "iana",
+ "extensions": ["vis"]
+ },
+ "application/vnd.vividence.scriptfile": {
+ "source": "iana"
+ },
+ "application/vnd.vsf": {
+ "source": "iana",
+ "extensions": ["vsf"]
+ },
+ "application/vnd.wap.sic": {
+ "source": "iana"
+ },
+ "application/vnd.wap.slc": {
+ "source": "iana"
+ },
+ "application/vnd.wap.wbxml": {
+ "source": "iana",
+ "extensions": ["wbxml"]
+ },
+ "application/vnd.wap.wmlc": {
+ "source": "iana",
+ "extensions": ["wmlc"]
+ },
+ "application/vnd.wap.wmlscriptc": {
+ "source": "iana",
+ "extensions": ["wmlsc"]
+ },
+ "application/vnd.webturbo": {
+ "source": "iana",
+ "extensions": ["wtb"]
+ },
+ "application/vnd.wfa.p2p": {
+ "source": "iana"
+ },
+ "application/vnd.wfa.wsc": {
+ "source": "iana"
+ },
+ "application/vnd.windows.devicepairing": {
+ "source": "iana"
+ },
+ "application/vnd.wmc": {
+ "source": "iana"
+ },
+ "application/vnd.wmf.bootstrap": {
+ "source": "iana"
+ },
+ "application/vnd.wolfram.mathematica": {
+ "source": "iana"
+ },
+ "application/vnd.wolfram.mathematica.package": {
+ "source": "iana"
+ },
+ "application/vnd.wolfram.player": {
+ "source": "iana",
+ "extensions": ["nbp"]
+ },
+ "application/vnd.wordperfect": {
+ "source": "iana",
+ "extensions": ["wpd"]
+ },
+ "application/vnd.wqd": {
+ "source": "iana",
+ "extensions": ["wqd"]
+ },
+ "application/vnd.wrq-hp3000-labelled": {
+ "source": "iana"
+ },
+ "application/vnd.wt.stf": {
+ "source": "iana",
+ "extensions": ["stf"]
+ },
+ "application/vnd.wv.csp+wbxml": {
+ "source": "iana"
+ },
+ "application/vnd.wv.csp+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.wv.ssp+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.xacml+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.xara": {
+ "source": "iana",
+ "extensions": ["xar"]
+ },
+ "application/vnd.xfdl": {
+ "source": "iana",
+ "extensions": ["xfdl"]
+ },
+ "application/vnd.xfdl.webform": {
+ "source": "iana"
+ },
+ "application/vnd.xmi+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vnd.xmpie.cpkg": {
+ "source": "iana"
+ },
+ "application/vnd.xmpie.dpkg": {
+ "source": "iana"
+ },
+ "application/vnd.xmpie.plan": {
+ "source": "iana"
+ },
+ "application/vnd.xmpie.ppkg": {
+ "source": "iana"
+ },
+ "application/vnd.xmpie.xlim": {
+ "source": "iana"
+ },
+ "application/vnd.yamaha.hv-dic": {
+ "source": "iana",
+ "extensions": ["hvd"]
+ },
+ "application/vnd.yamaha.hv-script": {
+ "source": "iana",
+ "extensions": ["hvs"]
+ },
+ "application/vnd.yamaha.hv-voice": {
+ "source": "iana",
+ "extensions": ["hvp"]
+ },
+ "application/vnd.yamaha.openscoreformat": {
+ "source": "iana",
+ "extensions": ["osf"]
+ },
+ "application/vnd.yamaha.openscoreformat.osfpvg+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["osfpvg"]
+ },
+ "application/vnd.yamaha.remote-setup": {
+ "source": "iana"
+ },
+ "application/vnd.yamaha.smaf-audio": {
+ "source": "iana",
+ "extensions": ["saf"]
+ },
+ "application/vnd.yamaha.smaf-phrase": {
+ "source": "iana",
+ "extensions": ["spf"]
+ },
+ "application/vnd.yamaha.through-ngn": {
+ "source": "iana"
+ },
+ "application/vnd.yamaha.tunnel-udpencap": {
+ "source": "iana"
+ },
+ "application/vnd.yaoweme": {
+ "source": "iana"
+ },
+ "application/vnd.yellowriver-custom-menu": {
+ "source": "iana",
+ "extensions": ["cmp"]
+ },
+ "application/vnd.youtube.yt": {
+ "source": "iana"
+ },
+ "application/vnd.zul": {
+ "source": "iana",
+ "extensions": ["zir","zirz"]
+ },
+ "application/vnd.zzazz.deck+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["zaz"]
+ },
+ "application/voicexml+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["vxml"]
+ },
+ "application/voucher-cms+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/vq-rtcpxr": {
+ "source": "iana"
+ },
+ "application/wasm": {
+ "compressible": true,
+ "extensions": ["wasm"]
+ },
+ "application/watcherinfo+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/webpush-options+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/whoispp-query": {
+ "source": "iana"
+ },
+ "application/whoispp-response": {
+ "source": "iana"
+ },
+ "application/widget": {
+ "source": "iana",
+ "extensions": ["wgt"]
+ },
+ "application/winhlp": {
+ "source": "apache",
+ "extensions": ["hlp"]
+ },
+ "application/wita": {
+ "source": "iana"
+ },
+ "application/wordperfect5.1": {
+ "source": "iana"
+ },
+ "application/wsdl+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["wsdl"]
+ },
+ "application/wspolicy+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["wspolicy"]
+ },
+ "application/x-7z-compressed": {
+ "source": "apache",
+ "compressible": false,
+ "extensions": ["7z"]
+ },
+ "application/x-abiword": {
+ "source": "apache",
+ "extensions": ["abw"]
+ },
+ "application/x-ace-compressed": {
+ "source": "apache",
+ "extensions": ["ace"]
+ },
+ "application/x-amf": {
+ "source": "apache"
+ },
+ "application/x-apple-diskimage": {
+ "source": "apache",
+ "extensions": ["dmg"]
+ },
+ "application/x-arj": {
+ "compressible": false,
+ "extensions": ["arj"]
+ },
+ "application/x-authorware-bin": {
+ "source": "apache",
+ "extensions": ["aab","x32","u32","vox"]
+ },
+ "application/x-authorware-map": {
+ "source": "apache",
+ "extensions": ["aam"]
+ },
+ "application/x-authorware-seg": {
+ "source": "apache",
+ "extensions": ["aas"]
+ },
+ "application/x-bcpio": {
+ "source": "apache",
+ "extensions": ["bcpio"]
+ },
+ "application/x-bdoc": {
+ "compressible": false,
+ "extensions": ["bdoc"]
+ },
+ "application/x-bittorrent": {
+ "source": "apache",
+ "extensions": ["torrent"]
+ },
+ "application/x-blorb": {
+ "source": "apache",
+ "extensions": ["blb","blorb"]
+ },
+ "application/x-bzip": {
+ "source": "apache",
+ "compressible": false,
+ "extensions": ["bz"]
+ },
+ "application/x-bzip2": {
+ "source": "apache",
+ "compressible": false,
+ "extensions": ["bz2","boz"]
+ },
+ "application/x-cbr": {
+ "source": "apache",
+ "extensions": ["cbr","cba","cbt","cbz","cb7"]
+ },
+ "application/x-cdlink": {
+ "source": "apache",
+ "extensions": ["vcd"]
+ },
+ "application/x-cfs-compressed": {
+ "source": "apache",
+ "extensions": ["cfs"]
+ },
+ "application/x-chat": {
+ "source": "apache",
+ "extensions": ["chat"]
+ },
+ "application/x-chess-pgn": {
+ "source": "apache",
+ "extensions": ["pgn"]
+ },
+ "application/x-chrome-extension": {
+ "extensions": ["crx"]
+ },
+ "application/x-cocoa": {
+ "source": "nginx",
+ "extensions": ["cco"]
+ },
+ "application/x-compress": {
+ "source": "apache"
+ },
+ "application/x-conference": {
+ "source": "apache",
+ "extensions": ["nsc"]
+ },
+ "application/x-cpio": {
+ "source": "apache",
+ "extensions": ["cpio"]
+ },
+ "application/x-csh": {
+ "source": "apache",
+ "extensions": ["csh"]
+ },
+ "application/x-deb": {
+ "compressible": false
+ },
+ "application/x-debian-package": {
+ "source": "apache",
+ "extensions": ["deb","udeb"]
+ },
+ "application/x-dgc-compressed": {
+ "source": "apache",
+ "extensions": ["dgc"]
+ },
+ "application/x-director": {
+ "source": "apache",
+ "extensions": ["dir","dcr","dxr","cst","cct","cxt","w3d","fgd","swa"]
+ },
+ "application/x-doom": {
+ "source": "apache",
+ "extensions": ["wad"]
+ },
+ "application/x-dtbncx+xml": {
+ "source": "apache",
+ "compressible": true,
+ "extensions": ["ncx"]
+ },
+ "application/x-dtbook+xml": {
+ "source": "apache",
+ "compressible": true,
+ "extensions": ["dtb"]
+ },
+ "application/x-dtbresource+xml": {
+ "source": "apache",
+ "compressible": true,
+ "extensions": ["res"]
+ },
+ "application/x-dvi": {
+ "source": "apache",
+ "compressible": false,
+ "extensions": ["dvi"]
+ },
+ "application/x-envoy": {
+ "source": "apache",
+ "extensions": ["evy"]
+ },
+ "application/x-eva": {
+ "source": "apache",
+ "extensions": ["eva"]
+ },
+ "application/x-font-bdf": {
+ "source": "apache",
+ "extensions": ["bdf"]
+ },
+ "application/x-font-dos": {
+ "source": "apache"
+ },
+ "application/x-font-framemaker": {
+ "source": "apache"
+ },
+ "application/x-font-ghostscript": {
+ "source": "apache",
+ "extensions": ["gsf"]
+ },
+ "application/x-font-libgrx": {
+ "source": "apache"
+ },
+ "application/x-font-linux-psf": {
+ "source": "apache",
+ "extensions": ["psf"]
+ },
+ "application/x-font-pcf": {
+ "source": "apache",
+ "extensions": ["pcf"]
+ },
+ "application/x-font-snf": {
+ "source": "apache",
+ "extensions": ["snf"]
+ },
+ "application/x-font-speedo": {
+ "source": "apache"
+ },
+ "application/x-font-sunos-news": {
+ "source": "apache"
+ },
+ "application/x-font-type1": {
+ "source": "apache",
+ "extensions": ["pfa","pfb","pfm","afm"]
+ },
+ "application/x-font-vfont": {
+ "source": "apache"
+ },
+ "application/x-freearc": {
+ "source": "apache",
+ "extensions": ["arc"]
+ },
+ "application/x-futuresplash": {
+ "source": "apache",
+ "extensions": ["spl"]
+ },
+ "application/x-gca-compressed": {
+ "source": "apache",
+ "extensions": ["gca"]
+ },
+ "application/x-glulx": {
+ "source": "apache",
+ "extensions": ["ulx"]
+ },
+ "application/x-gnumeric": {
+ "source": "apache",
+ "extensions": ["gnumeric"]
+ },
+ "application/x-gramps-xml": {
+ "source": "apache",
+ "extensions": ["gramps"]
+ },
+ "application/x-gtar": {
+ "source": "apache",
+ "extensions": ["gtar"]
+ },
+ "application/x-gzip": {
+ "source": "apache"
+ },
+ "application/x-hdf": {
+ "source": "apache",
+ "extensions": ["hdf"]
+ },
+ "application/x-httpd-php": {
+ "compressible": true,
+ "extensions": ["php"]
+ },
+ "application/x-install-instructions": {
+ "source": "apache",
+ "extensions": ["install"]
+ },
+ "application/x-iso9660-image": {
+ "source": "apache",
+ "extensions": ["iso"]
+ },
+ "application/x-java-archive-diff": {
+ "source": "nginx",
+ "extensions": ["jardiff"]
+ },
+ "application/x-java-jnlp-file": {
+ "source": "apache",
+ "compressible": false,
+ "extensions": ["jnlp"]
+ },
+ "application/x-javascript": {
+ "compressible": true
+ },
+ "application/x-latex": {
+ "source": "apache",
+ "compressible": false,
+ "extensions": ["latex"]
+ },
+ "application/x-lua-bytecode": {
+ "extensions": ["luac"]
+ },
+ "application/x-lzh-compressed": {
+ "source": "apache",
+ "extensions": ["lzh","lha"]
+ },
+ "application/x-makeself": {
+ "source": "nginx",
+ "extensions": ["run"]
+ },
+ "application/x-mie": {
+ "source": "apache",
+ "extensions": ["mie"]
+ },
+ "application/x-mobipocket-ebook": {
+ "source": "apache",
+ "extensions": ["prc","mobi"]
+ },
+ "application/x-mpegurl": {
+ "compressible": false
+ },
+ "application/x-ms-application": {
+ "source": "apache",
+ "extensions": ["application"]
+ },
+ "application/x-ms-shortcut": {
+ "source": "apache",
+ "extensions": ["lnk"]
+ },
+ "application/x-ms-wmd": {
+ "source": "apache",
+ "extensions": ["wmd"]
+ },
+ "application/x-ms-wmz": {
+ "source": "apache",
+ "extensions": ["wmz"]
+ },
+ "application/x-ms-xbap": {
+ "source": "apache",
+ "extensions": ["xbap"]
+ },
+ "application/x-msaccess": {
+ "source": "apache",
+ "extensions": ["mdb"]
+ },
+ "application/x-msbinder": {
+ "source": "apache",
+ "extensions": ["obd"]
+ },
+ "application/x-mscardfile": {
+ "source": "apache",
+ "extensions": ["crd"]
+ },
+ "application/x-msclip": {
+ "source": "apache",
+ "extensions": ["clp"]
+ },
+ "application/x-msdos-program": {
+ "extensions": ["exe"]
+ },
+ "application/x-msdownload": {
+ "source": "apache",
+ "extensions": ["exe","dll","com","bat","msi"]
+ },
+ "application/x-msmediaview": {
+ "source": "apache",
+ "extensions": ["mvb","m13","m14"]
+ },
+ "application/x-msmetafile": {
+ "source": "apache",
+ "extensions": ["wmf","wmz","emf","emz"]
+ },
+ "application/x-msmoney": {
+ "source": "apache",
+ "extensions": ["mny"]
+ },
+ "application/x-mspublisher": {
+ "source": "apache",
+ "extensions": ["pub"]
+ },
+ "application/x-msschedule": {
+ "source": "apache",
+ "extensions": ["scd"]
+ },
+ "application/x-msterminal": {
+ "source": "apache",
+ "extensions": ["trm"]
+ },
+ "application/x-mswrite": {
+ "source": "apache",
+ "extensions": ["wri"]
+ },
+ "application/x-netcdf": {
+ "source": "apache",
+ "extensions": ["nc","cdf"]
+ },
+ "application/x-ns-proxy-autoconfig": {
+ "compressible": true,
+ "extensions": ["pac"]
+ },
+ "application/x-nzb": {
+ "source": "apache",
+ "extensions": ["nzb"]
+ },
+ "application/x-perl": {
+ "source": "nginx",
+ "extensions": ["pl","pm"]
+ },
+ "application/x-pilot": {
+ "source": "nginx",
+ "extensions": ["prc","pdb"]
+ },
+ "application/x-pkcs12": {
+ "source": "apache",
+ "compressible": false,
+ "extensions": ["p12","pfx"]
+ },
+ "application/x-pkcs7-certificates": {
+ "source": "apache",
+ "extensions": ["p7b","spc"]
+ },
+ "application/x-pkcs7-certreqresp": {
+ "source": "apache",
+ "extensions": ["p7r"]
+ },
+ "application/x-rar-compressed": {
+ "source": "apache",
+ "compressible": false,
+ "extensions": ["rar"]
+ },
+ "application/x-redhat-package-manager": {
+ "source": "nginx",
+ "extensions": ["rpm"]
+ },
+ "application/x-research-info-systems": {
+ "source": "apache",
+ "extensions": ["ris"]
+ },
+ "application/x-sea": {
+ "source": "nginx",
+ "extensions": ["sea"]
+ },
+ "application/x-sh": {
+ "source": "apache",
+ "compressible": true,
+ "extensions": ["sh"]
+ },
+ "application/x-shar": {
+ "source": "apache",
+ "extensions": ["shar"]
+ },
+ "application/x-shockwave-flash": {
+ "source": "apache",
+ "compressible": false,
+ "extensions": ["swf"]
+ },
+ "application/x-silverlight-app": {
+ "source": "apache",
+ "extensions": ["xap"]
+ },
+ "application/x-sql": {
+ "source": "apache",
+ "extensions": ["sql"]
+ },
+ "application/x-stuffit": {
+ "source": "apache",
+ "compressible": false,
+ "extensions": ["sit"]
+ },
+ "application/x-stuffitx": {
+ "source": "apache",
+ "extensions": ["sitx"]
+ },
+ "application/x-subrip": {
+ "source": "apache",
+ "extensions": ["srt"]
+ },
+ "application/x-sv4cpio": {
+ "source": "apache",
+ "extensions": ["sv4cpio"]
+ },
+ "application/x-sv4crc": {
+ "source": "apache",
+ "extensions": ["sv4crc"]
+ },
+ "application/x-t3vm-image": {
+ "source": "apache",
+ "extensions": ["t3"]
+ },
+ "application/x-tads": {
+ "source": "apache",
+ "extensions": ["gam"]
+ },
+ "application/x-tar": {
+ "source": "apache",
+ "compressible": true,
+ "extensions": ["tar"]
+ },
+ "application/x-tcl": {
+ "source": "apache",
+ "extensions": ["tcl","tk"]
+ },
+ "application/x-tex": {
+ "source": "apache",
+ "extensions": ["tex"]
+ },
+ "application/x-tex-tfm": {
+ "source": "apache",
+ "extensions": ["tfm"]
+ },
+ "application/x-texinfo": {
+ "source": "apache",
+ "extensions": ["texinfo","texi"]
+ },
+ "application/x-tgif": {
+ "source": "apache",
+ "extensions": ["obj"]
+ },
+ "application/x-ustar": {
+ "source": "apache",
+ "extensions": ["ustar"]
+ },
+ "application/x-virtualbox-hdd": {
+ "compressible": true,
+ "extensions": ["hdd"]
+ },
+ "application/x-virtualbox-ova": {
+ "compressible": true,
+ "extensions": ["ova"]
+ },
+ "application/x-virtualbox-ovf": {
+ "compressible": true,
+ "extensions": ["ovf"]
+ },
+ "application/x-virtualbox-vbox": {
+ "compressible": true,
+ "extensions": ["vbox"]
+ },
+ "application/x-virtualbox-vbox-extpack": {
+ "compressible": false,
+ "extensions": ["vbox-extpack"]
+ },
+ "application/x-virtualbox-vdi": {
+ "compressible": true,
+ "extensions": ["vdi"]
+ },
+ "application/x-virtualbox-vhd": {
+ "compressible": true,
+ "extensions": ["vhd"]
+ },
+ "application/x-virtualbox-vmdk": {
+ "compressible": true,
+ "extensions": ["vmdk"]
+ },
+ "application/x-wais-source": {
+ "source": "apache",
+ "extensions": ["src"]
+ },
+ "application/x-web-app-manifest+json": {
+ "compressible": true,
+ "extensions": ["webapp"]
+ },
+ "application/x-www-form-urlencoded": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/x-x509-ca-cert": {
+ "source": "apache",
+ "extensions": ["der","crt","pem"]
+ },
+ "application/x-xfig": {
+ "source": "apache",
+ "extensions": ["fig"]
+ },
+ "application/x-xliff+xml": {
+ "source": "apache",
+ "compressible": true,
+ "extensions": ["xlf"]
+ },
+ "application/x-xpinstall": {
+ "source": "apache",
+ "compressible": false,
+ "extensions": ["xpi"]
+ },
+ "application/x-xz": {
+ "source": "apache",
+ "extensions": ["xz"]
+ },
+ "application/x-zmachine": {
+ "source": "apache",
+ "extensions": ["z1","z2","z3","z4","z5","z6","z7","z8"]
+ },
+ "application/x400-bp": {
+ "source": "iana"
+ },
+ "application/xacml+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/xaml+xml": {
+ "source": "apache",
+ "compressible": true,
+ "extensions": ["xaml"]
+ },
+ "application/xcap-att+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/xcap-caps+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/xcap-diff+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["xdf"]
+ },
+ "application/xcap-el+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/xcap-error+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/xcap-ns+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/xcon-conference-info+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/xcon-conference-info-diff+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/xenc+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["xenc"]
+ },
+ "application/xhtml+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["xhtml","xht"]
+ },
+ "application/xhtml-voice+xml": {
+ "source": "apache",
+ "compressible": true
+ },
+ "application/xliff+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["xml","xsl","xsd","rng"]
+ },
+ "application/xml-dtd": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["dtd"]
+ },
+ "application/xml-external-parsed-entity": {
+ "source": "iana"
+ },
+ "application/xml-patch+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/xmpp+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/xop+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["xop"]
+ },
+ "application/xproc+xml": {
+ "source": "apache",
+ "compressible": true,
+ "extensions": ["xpl"]
+ },
+ "application/xslt+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["xslt"]
+ },
+ "application/xspf+xml": {
+ "source": "apache",
+ "compressible": true,
+ "extensions": ["xspf"]
+ },
+ "application/xv+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["mxml","xhvml","xvml","xvm"]
+ },
+ "application/yang": {
+ "source": "iana",
+ "extensions": ["yang"]
+ },
+ "application/yang-data+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/yang-data+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/yang-patch+json": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/yang-patch+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "application/yin+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["yin"]
+ },
+ "application/zip": {
+ "source": "iana",
+ "compressible": false,
+ "extensions": ["zip"]
+ },
+ "application/zlib": {
+ "source": "iana"
+ },
+ "application/zstd": {
+ "source": "iana"
+ },
+ "audio/1d-interleaved-parityfec": {
+ "source": "iana"
+ },
+ "audio/32kadpcm": {
+ "source": "iana"
+ },
+ "audio/3gpp": {
+ "source": "iana",
+ "compressible": false,
+ "extensions": ["3gpp"]
+ },
+ "audio/3gpp2": {
+ "source": "iana"
+ },
+ "audio/aac": {
+ "source": "iana"
+ },
+ "audio/ac3": {
+ "source": "iana"
+ },
+ "audio/adpcm": {
+ "source": "apache",
+ "extensions": ["adp"]
+ },
+ "audio/amr": {
+ "source": "iana"
+ },
+ "audio/amr-wb": {
+ "source": "iana"
+ },
+ "audio/amr-wb+": {
+ "source": "iana"
+ },
+ "audio/aptx": {
+ "source": "iana"
+ },
+ "audio/asc": {
+ "source": "iana"
+ },
+ "audio/atrac-advanced-lossless": {
+ "source": "iana"
+ },
+ "audio/atrac-x": {
+ "source": "iana"
+ },
+ "audio/atrac3": {
+ "source": "iana"
+ },
+ "audio/basic": {
+ "source": "iana",
+ "compressible": false,
+ "extensions": ["au","snd"]
+ },
+ "audio/bv16": {
+ "source": "iana"
+ },
+ "audio/bv32": {
+ "source": "iana"
+ },
+ "audio/clearmode": {
+ "source": "iana"
+ },
+ "audio/cn": {
+ "source": "iana"
+ },
+ "audio/dat12": {
+ "source": "iana"
+ },
+ "audio/dls": {
+ "source": "iana"
+ },
+ "audio/dsr-es201108": {
+ "source": "iana"
+ },
+ "audio/dsr-es202050": {
+ "source": "iana"
+ },
+ "audio/dsr-es202211": {
+ "source": "iana"
+ },
+ "audio/dsr-es202212": {
+ "source": "iana"
+ },
+ "audio/dv": {
+ "source": "iana"
+ },
+ "audio/dvi4": {
+ "source": "iana"
+ },
+ "audio/eac3": {
+ "source": "iana"
+ },
+ "audio/encaprtp": {
+ "source": "iana"
+ },
+ "audio/evrc": {
+ "source": "iana"
+ },
+ "audio/evrc-qcp": {
+ "source": "iana"
+ },
+ "audio/evrc0": {
+ "source": "iana"
+ },
+ "audio/evrc1": {
+ "source": "iana"
+ },
+ "audio/evrcb": {
+ "source": "iana"
+ },
+ "audio/evrcb0": {
+ "source": "iana"
+ },
+ "audio/evrcb1": {
+ "source": "iana"
+ },
+ "audio/evrcnw": {
+ "source": "iana"
+ },
+ "audio/evrcnw0": {
+ "source": "iana"
+ },
+ "audio/evrcnw1": {
+ "source": "iana"
+ },
+ "audio/evrcwb": {
+ "source": "iana"
+ },
+ "audio/evrcwb0": {
+ "source": "iana"
+ },
+ "audio/evrcwb1": {
+ "source": "iana"
+ },
+ "audio/evs": {
+ "source": "iana"
+ },
+ "audio/fwdred": {
+ "source": "iana"
+ },
+ "audio/g711-0": {
+ "source": "iana"
+ },
+ "audio/g719": {
+ "source": "iana"
+ },
+ "audio/g722": {
+ "source": "iana"
+ },
+ "audio/g7221": {
+ "source": "iana"
+ },
+ "audio/g723": {
+ "source": "iana"
+ },
+ "audio/g726-16": {
+ "source": "iana"
+ },
+ "audio/g726-24": {
+ "source": "iana"
+ },
+ "audio/g726-32": {
+ "source": "iana"
+ },
+ "audio/g726-40": {
+ "source": "iana"
+ },
+ "audio/g728": {
+ "source": "iana"
+ },
+ "audio/g729": {
+ "source": "iana"
+ },
+ "audio/g7291": {
+ "source": "iana"
+ },
+ "audio/g729d": {
+ "source": "iana"
+ },
+ "audio/g729e": {
+ "source": "iana"
+ },
+ "audio/gsm": {
+ "source": "iana"
+ },
+ "audio/gsm-efr": {
+ "source": "iana"
+ },
+ "audio/gsm-hr-08": {
+ "source": "iana"
+ },
+ "audio/ilbc": {
+ "source": "iana"
+ },
+ "audio/ip-mr_v2.5": {
+ "source": "iana"
+ },
+ "audio/isac": {
+ "source": "apache"
+ },
+ "audio/l16": {
+ "source": "iana"
+ },
+ "audio/l20": {
+ "source": "iana"
+ },
+ "audio/l24": {
+ "source": "iana",
+ "compressible": false
+ },
+ "audio/l8": {
+ "source": "iana"
+ },
+ "audio/lpc": {
+ "source": "iana"
+ },
+ "audio/melp": {
+ "source": "iana"
+ },
+ "audio/melp1200": {
+ "source": "iana"
+ },
+ "audio/melp2400": {
+ "source": "iana"
+ },
+ "audio/melp600": {
+ "source": "iana"
+ },
+ "audio/midi": {
+ "source": "apache",
+ "extensions": ["mid","midi","kar","rmi"]
+ },
+ "audio/mobile-xmf": {
+ "source": "iana"
+ },
+ "audio/mp3": {
+ "compressible": false,
+ "extensions": ["mp3"]
+ },
+ "audio/mp4": {
+ "source": "iana",
+ "compressible": false,
+ "extensions": ["m4a","mp4a"]
+ },
+ "audio/mp4a-latm": {
+ "source": "iana"
+ },
+ "audio/mpa": {
+ "source": "iana"
+ },
+ "audio/mpa-robust": {
+ "source": "iana"
+ },
+ "audio/mpeg": {
+ "source": "iana",
+ "compressible": false,
+ "extensions": ["mpga","mp2","mp2a","mp3","m2a","m3a"]
+ },
+ "audio/mpeg4-generic": {
+ "source": "iana"
+ },
+ "audio/musepack": {
+ "source": "apache"
+ },
+ "audio/ogg": {
+ "source": "iana",
+ "compressible": false,
+ "extensions": ["oga","ogg","spx"]
+ },
+ "audio/opus": {
+ "source": "iana"
+ },
+ "audio/parityfec": {
+ "source": "iana"
+ },
+ "audio/pcma": {
+ "source": "iana"
+ },
+ "audio/pcma-wb": {
+ "source": "iana"
+ },
+ "audio/pcmu": {
+ "source": "iana"
+ },
+ "audio/pcmu-wb": {
+ "source": "iana"
+ },
+ "audio/prs.sid": {
+ "source": "iana"
+ },
+ "audio/qcelp": {
+ "source": "iana"
+ },
+ "audio/raptorfec": {
+ "source": "iana"
+ },
+ "audio/red": {
+ "source": "iana"
+ },
+ "audio/rtp-enc-aescm128": {
+ "source": "iana"
+ },
+ "audio/rtp-midi": {
+ "source": "iana"
+ },
+ "audio/rtploopback": {
+ "source": "iana"
+ },
+ "audio/rtx": {
+ "source": "iana"
+ },
+ "audio/s3m": {
+ "source": "apache",
+ "extensions": ["s3m"]
+ },
+ "audio/silk": {
+ "source": "apache",
+ "extensions": ["sil"]
+ },
+ "audio/smv": {
+ "source": "iana"
+ },
+ "audio/smv-qcp": {
+ "source": "iana"
+ },
+ "audio/smv0": {
+ "source": "iana"
+ },
+ "audio/sp-midi": {
+ "source": "iana"
+ },
+ "audio/speex": {
+ "source": "iana"
+ },
+ "audio/t140c": {
+ "source": "iana"
+ },
+ "audio/t38": {
+ "source": "iana"
+ },
+ "audio/telephone-event": {
+ "source": "iana"
+ },
+ "audio/tone": {
+ "source": "iana"
+ },
+ "audio/uemclip": {
+ "source": "iana"
+ },
+ "audio/ulpfec": {
+ "source": "iana"
+ },
+ "audio/usac": {
+ "source": "iana"
+ },
+ "audio/vdvi": {
+ "source": "iana"
+ },
+ "audio/vmr-wb": {
+ "source": "iana"
+ },
+ "audio/vnd.3gpp.iufp": {
+ "source": "iana"
+ },
+ "audio/vnd.4sb": {
+ "source": "iana"
+ },
+ "audio/vnd.audiokoz": {
+ "source": "iana"
+ },
+ "audio/vnd.celp": {
+ "source": "iana"
+ },
+ "audio/vnd.cisco.nse": {
+ "source": "iana"
+ },
+ "audio/vnd.cmles.radio-events": {
+ "source": "iana"
+ },
+ "audio/vnd.cns.anp1": {
+ "source": "iana"
+ },
+ "audio/vnd.cns.inf1": {
+ "source": "iana"
+ },
+ "audio/vnd.dece.audio": {
+ "source": "iana",
+ "extensions": ["uva","uvva"]
+ },
+ "audio/vnd.digital-winds": {
+ "source": "iana",
+ "extensions": ["eol"]
+ },
+ "audio/vnd.dlna.adts": {
+ "source": "iana"
+ },
+ "audio/vnd.dolby.heaac.1": {
+ "source": "iana"
+ },
+ "audio/vnd.dolby.heaac.2": {
+ "source": "iana"
+ },
+ "audio/vnd.dolby.mlp": {
+ "source": "iana"
+ },
+ "audio/vnd.dolby.mps": {
+ "source": "iana"
+ },
+ "audio/vnd.dolby.pl2": {
+ "source": "iana"
+ },
+ "audio/vnd.dolby.pl2x": {
+ "source": "iana"
+ },
+ "audio/vnd.dolby.pl2z": {
+ "source": "iana"
+ },
+ "audio/vnd.dolby.pulse.1": {
+ "source": "iana"
+ },
+ "audio/vnd.dra": {
+ "source": "iana",
+ "extensions": ["dra"]
+ },
+ "audio/vnd.dts": {
+ "source": "iana",
+ "extensions": ["dts"]
+ },
+ "audio/vnd.dts.hd": {
+ "source": "iana",
+ "extensions": ["dtshd"]
+ },
+ "audio/vnd.dvb.file": {
+ "source": "iana"
+ },
+ "audio/vnd.everad.plj": {
+ "source": "iana"
+ },
+ "audio/vnd.hns.audio": {
+ "source": "iana"
+ },
+ "audio/vnd.lucent.voice": {
+ "source": "iana",
+ "extensions": ["lvp"]
+ },
+ "audio/vnd.ms-playready.media.pya": {
+ "source": "iana",
+ "extensions": ["pya"]
+ },
+ "audio/vnd.nokia.mobile-xmf": {
+ "source": "iana"
+ },
+ "audio/vnd.nortel.vbk": {
+ "source": "iana"
+ },
+ "audio/vnd.nuera.ecelp4800": {
+ "source": "iana",
+ "extensions": ["ecelp4800"]
+ },
+ "audio/vnd.nuera.ecelp7470": {
+ "source": "iana",
+ "extensions": ["ecelp7470"]
+ },
+ "audio/vnd.nuera.ecelp9600": {
+ "source": "iana",
+ "extensions": ["ecelp9600"]
+ },
+ "audio/vnd.octel.sbc": {
+ "source": "iana"
+ },
+ "audio/vnd.presonus.multitrack": {
+ "source": "iana"
+ },
+ "audio/vnd.qcelp": {
+ "source": "iana"
+ },
+ "audio/vnd.rhetorex.32kadpcm": {
+ "source": "iana"
+ },
+ "audio/vnd.rip": {
+ "source": "iana",
+ "extensions": ["rip"]
+ },
+ "audio/vnd.rn-realaudio": {
+ "compressible": false
+ },
+ "audio/vnd.sealedmedia.softseal.mpeg": {
+ "source": "iana"
+ },
+ "audio/vnd.vmx.cvsd": {
+ "source": "iana"
+ },
+ "audio/vnd.wave": {
+ "compressible": false
+ },
+ "audio/vorbis": {
+ "source": "iana",
+ "compressible": false
+ },
+ "audio/vorbis-config": {
+ "source": "iana"
+ },
+ "audio/wav": {
+ "compressible": false,
+ "extensions": ["wav"]
+ },
+ "audio/wave": {
+ "compressible": false,
+ "extensions": ["wav"]
+ },
+ "audio/webm": {
+ "source": "apache",
+ "compressible": false,
+ "extensions": ["weba"]
+ },
+ "audio/x-aac": {
+ "source": "apache",
+ "compressible": false,
+ "extensions": ["aac"]
+ },
+ "audio/x-aiff": {
+ "source": "apache",
+ "extensions": ["aif","aiff","aifc"]
+ },
+ "audio/x-caf": {
+ "source": "apache",
+ "compressible": false,
+ "extensions": ["caf"]
+ },
+ "audio/x-flac": {
+ "source": "apache",
+ "extensions": ["flac"]
+ },
+ "audio/x-m4a": {
+ "source": "nginx",
+ "extensions": ["m4a"]
+ },
+ "audio/x-matroska": {
+ "source": "apache",
+ "extensions": ["mka"]
+ },
+ "audio/x-mpegurl": {
+ "source": "apache",
+ "extensions": ["m3u"]
+ },
+ "audio/x-ms-wax": {
+ "source": "apache",
+ "extensions": ["wax"]
+ },
+ "audio/x-ms-wma": {
+ "source": "apache",
+ "extensions": ["wma"]
+ },
+ "audio/x-pn-realaudio": {
+ "source": "apache",
+ "extensions": ["ram","ra"]
+ },
+ "audio/x-pn-realaudio-plugin": {
+ "source": "apache",
+ "extensions": ["rmp"]
+ },
+ "audio/x-realaudio": {
+ "source": "nginx",
+ "extensions": ["ra"]
+ },
+ "audio/x-tta": {
+ "source": "apache"
+ },
+ "audio/x-wav": {
+ "source": "apache",
+ "extensions": ["wav"]
+ },
+ "audio/xm": {
+ "source": "apache",
+ "extensions": ["xm"]
+ },
+ "chemical/x-cdx": {
+ "source": "apache",
+ "extensions": ["cdx"]
+ },
+ "chemical/x-cif": {
+ "source": "apache",
+ "extensions": ["cif"]
+ },
+ "chemical/x-cmdf": {
+ "source": "apache",
+ "extensions": ["cmdf"]
+ },
+ "chemical/x-cml": {
+ "source": "apache",
+ "extensions": ["cml"]
+ },
+ "chemical/x-csml": {
+ "source": "apache",
+ "extensions": ["csml"]
+ },
+ "chemical/x-pdb": {
+ "source": "apache"
+ },
+ "chemical/x-xyz": {
+ "source": "apache",
+ "extensions": ["xyz"]
+ },
+ "font/collection": {
+ "source": "iana",
+ "extensions": ["ttc"]
+ },
+ "font/otf": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["otf"]
+ },
+ "font/sfnt": {
+ "source": "iana"
+ },
+ "font/ttf": {
+ "source": "iana",
+ "extensions": ["ttf"]
+ },
+ "font/woff": {
+ "source": "iana",
+ "extensions": ["woff"]
+ },
+ "font/woff2": {
+ "source": "iana",
+ "extensions": ["woff2"]
+ },
+ "image/aces": {
+ "source": "iana",
+ "extensions": ["exr"]
+ },
+ "image/apng": {
+ "compressible": false,
+ "extensions": ["apng"]
+ },
+ "image/avci": {
+ "source": "iana"
+ },
+ "image/avcs": {
+ "source": "iana"
+ },
+ "image/bmp": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["bmp"]
+ },
+ "image/cgm": {
+ "source": "iana",
+ "extensions": ["cgm"]
+ },
+ "image/dicom-rle": {
+ "source": "iana",
+ "extensions": ["drle"]
+ },
+ "image/emf": {
+ "source": "iana",
+ "extensions": ["emf"]
+ },
+ "image/fits": {
+ "source": "iana",
+ "extensions": ["fits"]
+ },
+ "image/g3fax": {
+ "source": "iana",
+ "extensions": ["g3"]
+ },
+ "image/gif": {
+ "source": "iana",
+ "compressible": false,
+ "extensions": ["gif"]
+ },
+ "image/heic": {
+ "source": "iana",
+ "extensions": ["heic"]
+ },
+ "image/heic-sequence": {
+ "source": "iana",
+ "extensions": ["heics"]
+ },
+ "image/heif": {
+ "source": "iana",
+ "extensions": ["heif"]
+ },
+ "image/heif-sequence": {
+ "source": "iana",
+ "extensions": ["heifs"]
+ },
+ "image/ief": {
+ "source": "iana",
+ "extensions": ["ief"]
+ },
+ "image/jls": {
+ "source": "iana",
+ "extensions": ["jls"]
+ },
+ "image/jp2": {
+ "source": "iana",
+ "compressible": false,
+ "extensions": ["jp2","jpg2"]
+ },
+ "image/jpeg": {
+ "source": "iana",
+ "compressible": false,
+ "extensions": ["jpeg","jpg","jpe"]
+ },
+ "image/jpm": {
+ "source": "iana",
+ "compressible": false,
+ "extensions": ["jpm"]
+ },
+ "image/jpx": {
+ "source": "iana",
+ "compressible": false,
+ "extensions": ["jpx","jpf"]
+ },
+ "image/ktx": {
+ "source": "iana",
+ "extensions": ["ktx"]
+ },
+ "image/naplps": {
+ "source": "iana"
+ },
+ "image/pjpeg": {
+ "compressible": false
+ },
+ "image/png": {
+ "source": "iana",
+ "compressible": false,
+ "extensions": ["png"]
+ },
+ "image/prs.btif": {
+ "source": "iana",
+ "extensions": ["btif"]
+ },
+ "image/prs.pti": {
+ "source": "iana",
+ "extensions": ["pti"]
+ },
+ "image/pwg-raster": {
+ "source": "iana"
+ },
+ "image/sgi": {
+ "source": "apache",
+ "extensions": ["sgi"]
+ },
+ "image/svg+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["svg","svgz"]
+ },
+ "image/t38": {
+ "source": "iana",
+ "extensions": ["t38"]
+ },
+ "image/tiff": {
+ "source": "iana",
+ "compressible": false,
+ "extensions": ["tif","tiff"]
+ },
+ "image/tiff-fx": {
+ "source": "iana",
+ "extensions": ["tfx"]
+ },
+ "image/vnd.adobe.photoshop": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["psd"]
+ },
+ "image/vnd.airzip.accelerator.azv": {
+ "source": "iana",
+ "extensions": ["azv"]
+ },
+ "image/vnd.cns.inf2": {
+ "source": "iana"
+ },
+ "image/vnd.dece.graphic": {
+ "source": "iana",
+ "extensions": ["uvi","uvvi","uvg","uvvg"]
+ },
+ "image/vnd.djvu": {
+ "source": "iana",
+ "extensions": ["djvu","djv"]
+ },
+ "image/vnd.dvb.subtitle": {
+ "source": "iana",
+ "extensions": ["sub"]
+ },
+ "image/vnd.dwg": {
+ "source": "iana",
+ "extensions": ["dwg"]
+ },
+ "image/vnd.dxf": {
+ "source": "iana",
+ "extensions": ["dxf"]
+ },
+ "image/vnd.fastbidsheet": {
+ "source": "iana",
+ "extensions": ["fbs"]
+ },
+ "image/vnd.fpx": {
+ "source": "iana",
+ "extensions": ["fpx"]
+ },
+ "image/vnd.fst": {
+ "source": "iana",
+ "extensions": ["fst"]
+ },
+ "image/vnd.fujixerox.edmics-mmr": {
+ "source": "iana",
+ "extensions": ["mmr"]
+ },
+ "image/vnd.fujixerox.edmics-rlc": {
+ "source": "iana",
+ "extensions": ["rlc"]
+ },
+ "image/vnd.globalgraphics.pgb": {
+ "source": "iana"
+ },
+ "image/vnd.microsoft.icon": {
+ "source": "iana",
+ "extensions": ["ico"]
+ },
+ "image/vnd.mix": {
+ "source": "iana"
+ },
+ "image/vnd.mozilla.apng": {
+ "source": "iana"
+ },
+ "image/vnd.ms-modi": {
+ "source": "iana",
+ "extensions": ["mdi"]
+ },
+ "image/vnd.ms-photo": {
+ "source": "apache",
+ "extensions": ["wdp"]
+ },
+ "image/vnd.net-fpx": {
+ "source": "iana",
+ "extensions": ["npx"]
+ },
+ "image/vnd.radiance": {
+ "source": "iana"
+ },
+ "image/vnd.sealed.png": {
+ "source": "iana"
+ },
+ "image/vnd.sealedmedia.softseal.gif": {
+ "source": "iana"
+ },
+ "image/vnd.sealedmedia.softseal.jpg": {
+ "source": "iana"
+ },
+ "image/vnd.svf": {
+ "source": "iana"
+ },
+ "image/vnd.tencent.tap": {
+ "source": "iana",
+ "extensions": ["tap"]
+ },
+ "image/vnd.valve.source.texture": {
+ "source": "iana",
+ "extensions": ["vtf"]
+ },
+ "image/vnd.wap.wbmp": {
+ "source": "iana",
+ "extensions": ["wbmp"]
+ },
+ "image/vnd.xiff": {
+ "source": "iana",
+ "extensions": ["xif"]
+ },
+ "image/vnd.zbrush.pcx": {
+ "source": "iana",
+ "extensions": ["pcx"]
+ },
+ "image/webp": {
+ "source": "apache",
+ "extensions": ["webp"]
+ },
+ "image/wmf": {
+ "source": "iana",
+ "extensions": ["wmf"]
+ },
+ "image/x-3ds": {
+ "source": "apache",
+ "extensions": ["3ds"]
+ },
+ "image/x-cmu-raster": {
+ "source": "apache",
+ "extensions": ["ras"]
+ },
+ "image/x-cmx": {
+ "source": "apache",
+ "extensions": ["cmx"]
+ },
+ "image/x-freehand": {
+ "source": "apache",
+ "extensions": ["fh","fhc","fh4","fh5","fh7"]
+ },
+ "image/x-icon": {
+ "source": "apache",
+ "compressible": true,
+ "extensions": ["ico"]
+ },
+ "image/x-jng": {
+ "source": "nginx",
+ "extensions": ["jng"]
+ },
+ "image/x-mrsid-image": {
+ "source": "apache",
+ "extensions": ["sid"]
+ },
+ "image/x-ms-bmp": {
+ "source": "nginx",
+ "compressible": true,
+ "extensions": ["bmp"]
+ },
+ "image/x-pcx": {
+ "source": "apache",
+ "extensions": ["pcx"]
+ },
+ "image/x-pict": {
+ "source": "apache",
+ "extensions": ["pic","pct"]
+ },
+ "image/x-portable-anymap": {
+ "source": "apache",
+ "extensions": ["pnm"]
+ },
+ "image/x-portable-bitmap": {
+ "source": "apache",
+ "extensions": ["pbm"]
+ },
+ "image/x-portable-graymap": {
+ "source": "apache",
+ "extensions": ["pgm"]
+ },
+ "image/x-portable-pixmap": {
+ "source": "apache",
+ "extensions": ["ppm"]
+ },
+ "image/x-rgb": {
+ "source": "apache",
+ "extensions": ["rgb"]
+ },
+ "image/x-tga": {
+ "source": "apache",
+ "extensions": ["tga"]
+ },
+ "image/x-xbitmap": {
+ "source": "apache",
+ "extensions": ["xbm"]
+ },
+ "image/x-xcf": {
+ "compressible": false
+ },
+ "image/x-xpixmap": {
+ "source": "apache",
+ "extensions": ["xpm"]
+ },
+ "image/x-xwindowdump": {
+ "source": "apache",
+ "extensions": ["xwd"]
+ },
+ "message/cpim": {
+ "source": "iana"
+ },
+ "message/delivery-status": {
+ "source": "iana"
+ },
+ "message/disposition-notification": {
+ "source": "iana",
+ "extensions": [
+ "disposition-notification"
+ ]
+ },
+ "message/external-body": {
+ "source": "iana"
+ },
+ "message/feedback-report": {
+ "source": "iana"
+ },
+ "message/global": {
+ "source": "iana",
+ "extensions": ["u8msg"]
+ },
+ "message/global-delivery-status": {
+ "source": "iana",
+ "extensions": ["u8dsn"]
+ },
+ "message/global-disposition-notification": {
+ "source": "iana",
+ "extensions": ["u8mdn"]
+ },
+ "message/global-headers": {
+ "source": "iana",
+ "extensions": ["u8hdr"]
+ },
+ "message/http": {
+ "source": "iana",
+ "compressible": false
+ },
+ "message/imdn+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "message/news": {
+ "source": "iana"
+ },
+ "message/partial": {
+ "source": "iana",
+ "compressible": false
+ },
+ "message/rfc822": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["eml","mime"]
+ },
+ "message/s-http": {
+ "source": "iana"
+ },
+ "message/sip": {
+ "source": "iana"
+ },
+ "message/sipfrag": {
+ "source": "iana"
+ },
+ "message/tracking-status": {
+ "source": "iana"
+ },
+ "message/vnd.si.simp": {
+ "source": "iana"
+ },
+ "message/vnd.wfa.wsc": {
+ "source": "iana",
+ "extensions": ["wsc"]
+ },
+ "model/3mf": {
+ "source": "iana"
+ },
+ "model/gltf+json": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["gltf"]
+ },
+ "model/gltf-binary": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["glb"]
+ },
+ "model/iges": {
+ "source": "iana",
+ "compressible": false,
+ "extensions": ["igs","iges"]
+ },
+ "model/mesh": {
+ "source": "iana",
+ "compressible": false,
+ "extensions": ["msh","mesh","silo"]
+ },
+ "model/stl": {
+ "source": "iana"
+ },
+ "model/vnd.collada+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["dae"]
+ },
+ "model/vnd.dwf": {
+ "source": "iana",
+ "extensions": ["dwf"]
+ },
+ "model/vnd.flatland.3dml": {
+ "source": "iana"
+ },
+ "model/vnd.gdl": {
+ "source": "iana",
+ "extensions": ["gdl"]
+ },
+ "model/vnd.gs-gdl": {
+ "source": "apache"
+ },
+ "model/vnd.gs.gdl": {
+ "source": "iana"
+ },
+ "model/vnd.gtw": {
+ "source": "iana",
+ "extensions": ["gtw"]
+ },
+ "model/vnd.moml+xml": {
+ "source": "iana",
+ "compressible": true
+ },
+ "model/vnd.mts": {
+ "source": "iana",
+ "extensions": ["mts"]
+ },
+ "model/vnd.opengex": {
+ "source": "iana"
+ },
+ "model/vnd.parasolid.transmit.binary": {
+ "source": "iana"
+ },
+ "model/vnd.parasolid.transmit.text": {
+ "source": "iana"
+ },
+ "model/vnd.rosette.annotated-data-model": {
+ "source": "iana"
+ },
+ "model/vnd.usdz+zip": {
+ "source": "iana",
+ "compressible": false
+ },
+ "model/vnd.valve.source.compiled-map": {
+ "source": "iana"
+ },
+ "model/vnd.vtu": {
+ "source": "iana",
+ "extensions": ["vtu"]
+ },
+ "model/vrml": {
+ "source": "iana",
+ "compressible": false,
+ "extensions": ["wrl","vrml"]
+ },
+ "model/x3d+binary": {
+ "source": "apache",
+ "compressible": false,
+ "extensions": ["x3db","x3dbz"]
+ },
+ "model/x3d+fastinfoset": {
+ "source": "iana"
+ },
+ "model/x3d+vrml": {
+ "source": "apache",
+ "compressible": false,
+ "extensions": ["x3dv","x3dvz"]
+ },
+ "model/x3d+xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["x3d","x3dz"]
+ },
+ "model/x3d-vrml": {
+ "source": "iana"
+ },
+ "multipart/alternative": {
+ "source": "iana",
+ "compressible": false
+ },
+ "multipart/appledouble": {
+ "source": "iana"
+ },
+ "multipart/byteranges": {
+ "source": "iana"
+ },
+ "multipart/digest": {
+ "source": "iana"
+ },
+ "multipart/encrypted": {
+ "source": "iana",
+ "compressible": false
+ },
+ "multipart/form-data": {
+ "source": "iana",
+ "compressible": false
+ },
+ "multipart/header-set": {
+ "source": "iana"
+ },
+ "multipart/mixed": {
+ "source": "iana",
+ "compressible": false
+ },
+ "multipart/multilingual": {
+ "source": "iana"
+ },
+ "multipart/parallel": {
+ "source": "iana"
+ },
+ "multipart/related": {
+ "source": "iana",
+ "compressible": false
+ },
+ "multipart/report": {
+ "source": "iana"
+ },
+ "multipart/signed": {
+ "source": "iana",
+ "compressible": false
+ },
+ "multipart/vnd.bint.med-plus": {
+ "source": "iana"
+ },
+ "multipart/voice-message": {
+ "source": "iana"
+ },
+ "multipart/x-mixed-replace": {
+ "source": "iana"
+ },
+ "text/1d-interleaved-parityfec": {
+ "source": "iana"
+ },
+ "text/cache-manifest": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["appcache","manifest"]
+ },
+ "text/calendar": {
+ "source": "iana",
+ "extensions": ["ics","ifb"]
+ },
+ "text/calender": {
+ "compressible": true
+ },
+ "text/cmd": {
+ "compressible": true
+ },
+ "text/coffeescript": {
+ "extensions": ["coffee","litcoffee"]
+ },
+ "text/css": {
+ "source": "iana",
+ "charset": "UTF-8",
+ "compressible": true,
+ "extensions": ["css"]
+ },
+ "text/csv": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["csv"]
+ },
+ "text/csv-schema": {
+ "source": "iana"
+ },
+ "text/directory": {
+ "source": "iana"
+ },
+ "text/dns": {
+ "source": "iana"
+ },
+ "text/ecmascript": {
+ "source": "iana"
+ },
+ "text/encaprtp": {
+ "source": "iana"
+ },
+ "text/enriched": {
+ "source": "iana"
+ },
+ "text/fwdred": {
+ "source": "iana"
+ },
+ "text/grammar-ref-list": {
+ "source": "iana"
+ },
+ "text/html": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["html","htm","shtml"]
+ },
+ "text/jade": {
+ "extensions": ["jade"]
+ },
+ "text/javascript": {
+ "source": "iana",
+ "compressible": true
+ },
+ "text/jcr-cnd": {
+ "source": "iana"
+ },
+ "text/jsx": {
+ "compressible": true,
+ "extensions": ["jsx"]
+ },
+ "text/less": {
+ "extensions": ["less"]
+ },
+ "text/markdown": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["markdown","md"]
+ },
+ "text/mathml": {
+ "source": "nginx",
+ "extensions": ["mml"]
+ },
+ "text/mizar": {
+ "source": "iana"
+ },
+ "text/n3": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["n3"]
+ },
+ "text/parameters": {
+ "source": "iana"
+ },
+ "text/parityfec": {
+ "source": "iana"
+ },
+ "text/plain": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["txt","text","conf","def","list","log","in","ini"]
+ },
+ "text/provenance-notation": {
+ "source": "iana"
+ },
+ "text/prs.fallenstein.rst": {
+ "source": "iana"
+ },
+ "text/prs.lines.tag": {
+ "source": "iana",
+ "extensions": ["dsc"]
+ },
+ "text/prs.prop.logic": {
+ "source": "iana"
+ },
+ "text/raptorfec": {
+ "source": "iana"
+ },
+ "text/red": {
+ "source": "iana"
+ },
+ "text/rfc822-headers": {
+ "source": "iana"
+ },
+ "text/richtext": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["rtx"]
+ },
+ "text/rtf": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["rtf"]
+ },
+ "text/rtp-enc-aescm128": {
+ "source": "iana"
+ },
+ "text/rtploopback": {
+ "source": "iana"
+ },
+ "text/rtx": {
+ "source": "iana"
+ },
+ "text/sgml": {
+ "source": "iana",
+ "extensions": ["sgml","sgm"]
+ },
+ "text/shex": {
+ "extensions": ["shex"]
+ },
+ "text/slim": {
+ "extensions": ["slim","slm"]
+ },
+ "text/strings": {
+ "source": "iana"
+ },
+ "text/stylus": {
+ "extensions": ["stylus","styl"]
+ },
+ "text/t140": {
+ "source": "iana"
+ },
+ "text/tab-separated-values": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["tsv"]
+ },
+ "text/troff": {
+ "source": "iana",
+ "extensions": ["t","tr","roff","man","me","ms"]
+ },
+ "text/turtle": {
+ "source": "iana",
+ "charset": "UTF-8",
+ "extensions": ["ttl"]
+ },
+ "text/ulpfec": {
+ "source": "iana"
+ },
+ "text/uri-list": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["uri","uris","urls"]
+ },
+ "text/vcard": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["vcard"]
+ },
+ "text/vnd.a": {
+ "source": "iana"
+ },
+ "text/vnd.abc": {
+ "source": "iana"
+ },
+ "text/vnd.ascii-art": {
+ "source": "iana"
+ },
+ "text/vnd.curl": {
+ "source": "iana",
+ "extensions": ["curl"]
+ },
+ "text/vnd.curl.dcurl": {
+ "source": "apache",
+ "extensions": ["dcurl"]
+ },
+ "text/vnd.curl.mcurl": {
+ "source": "apache",
+ "extensions": ["mcurl"]
+ },
+ "text/vnd.curl.scurl": {
+ "source": "apache",
+ "extensions": ["scurl"]
+ },
+ "text/vnd.debian.copyright": {
+ "source": "iana"
+ },
+ "text/vnd.dmclientscript": {
+ "source": "iana"
+ },
+ "text/vnd.dvb.subtitle": {
+ "source": "iana",
+ "extensions": ["sub"]
+ },
+ "text/vnd.esmertec.theme-descriptor": {
+ "source": "iana"
+ },
+ "text/vnd.fly": {
+ "source": "iana",
+ "extensions": ["fly"]
+ },
+ "text/vnd.fmi.flexstor": {
+ "source": "iana",
+ "extensions": ["flx"]
+ },
+ "text/vnd.gml": {
+ "source": "iana"
+ },
+ "text/vnd.graphviz": {
+ "source": "iana",
+ "extensions": ["gv"]
+ },
+ "text/vnd.hgl": {
+ "source": "iana"
+ },
+ "text/vnd.in3d.3dml": {
+ "source": "iana",
+ "extensions": ["3dml"]
+ },
+ "text/vnd.in3d.spot": {
+ "source": "iana",
+ "extensions": ["spot"]
+ },
+ "text/vnd.iptc.newsml": {
+ "source": "iana"
+ },
+ "text/vnd.iptc.nitf": {
+ "source": "iana"
+ },
+ "text/vnd.latex-z": {
+ "source": "iana"
+ },
+ "text/vnd.motorola.reflex": {
+ "source": "iana"
+ },
+ "text/vnd.ms-mediapackage": {
+ "source": "iana"
+ },
+ "text/vnd.net2phone.commcenter.command": {
+ "source": "iana"
+ },
+ "text/vnd.radisys.msml-basic-layout": {
+ "source": "iana"
+ },
+ "text/vnd.si.uricatalogue": {
+ "source": "iana"
+ },
+ "text/vnd.sun.j2me.app-descriptor": {
+ "source": "iana",
+ "extensions": ["jad"]
+ },
+ "text/vnd.trolltech.linguist": {
+ "source": "iana"
+ },
+ "text/vnd.wap.si": {
+ "source": "iana"
+ },
+ "text/vnd.wap.sl": {
+ "source": "iana"
+ },
+ "text/vnd.wap.wml": {
+ "source": "iana",
+ "extensions": ["wml"]
+ },
+ "text/vnd.wap.wmlscript": {
+ "source": "iana",
+ "extensions": ["wmls"]
+ },
+ "text/vtt": {
+ "charset": "UTF-8",
+ "compressible": true,
+ "extensions": ["vtt"]
+ },
+ "text/x-asm": {
+ "source": "apache",
+ "extensions": ["s","asm"]
+ },
+ "text/x-c": {
+ "source": "apache",
+ "extensions": ["c","cc","cxx","cpp","h","hh","dic"]
+ },
+ "text/x-component": {
+ "source": "nginx",
+ "extensions": ["htc"]
+ },
+ "text/x-fortran": {
+ "source": "apache",
+ "extensions": ["f","for","f77","f90"]
+ },
+ "text/x-gwt-rpc": {
+ "compressible": true
+ },
+ "text/x-handlebars-template": {
+ "extensions": ["hbs"]
+ },
+ "text/x-java-source": {
+ "source": "apache",
+ "extensions": ["java"]
+ },
+ "text/x-jquery-tmpl": {
+ "compressible": true
+ },
+ "text/x-lua": {
+ "extensions": ["lua"]
+ },
+ "text/x-markdown": {
+ "compressible": true,
+ "extensions": ["mkd"]
+ },
+ "text/x-nfo": {
+ "source": "apache",
+ "extensions": ["nfo"]
+ },
+ "text/x-opml": {
+ "source": "apache",
+ "extensions": ["opml"]
+ },
+ "text/x-org": {
+ "compressible": true,
+ "extensions": ["org"]
+ },
+ "text/x-pascal": {
+ "source": "apache",
+ "extensions": ["p","pas"]
+ },
+ "text/x-processing": {
+ "compressible": true,
+ "extensions": ["pde"]
+ },
+ "text/x-sass": {
+ "extensions": ["sass"]
+ },
+ "text/x-scss": {
+ "extensions": ["scss"]
+ },
+ "text/x-setext": {
+ "source": "apache",
+ "extensions": ["etx"]
+ },
+ "text/x-sfv": {
+ "source": "apache",
+ "extensions": ["sfv"]
+ },
+ "text/x-suse-ymp": {
+ "compressible": true,
+ "extensions": ["ymp"]
+ },
+ "text/x-uuencode": {
+ "source": "apache",
+ "extensions": ["uu"]
+ },
+ "text/x-vcalendar": {
+ "source": "apache",
+ "extensions": ["vcs"]
+ },
+ "text/x-vcard": {
+ "source": "apache",
+ "extensions": ["vcf"]
+ },
+ "text/xml": {
+ "source": "iana",
+ "compressible": true,
+ "extensions": ["xml"]
+ },
+ "text/xml-external-parsed-entity": {
+ "source": "iana"
+ },
+ "text/yaml": {
+ "extensions": ["yaml","yml"]
+ },
+ "video/1d-interleaved-parityfec": {
+ "source": "iana"
+ },
+ "video/3gpp": {
+ "source": "iana",
+ "extensions": ["3gp","3gpp"]
+ },
+ "video/3gpp-tt": {
+ "source": "iana"
+ },
+ "video/3gpp2": {
+ "source": "iana",
+ "extensions": ["3g2"]
+ },
+ "video/bmpeg": {
+ "source": "iana"
+ },
+ "video/bt656": {
+ "source": "iana"
+ },
+ "video/celb": {
+ "source": "iana"
+ },
+ "video/dv": {
+ "source": "iana"
+ },
+ "video/encaprtp": {
+ "source": "iana"
+ },
+ "video/h261": {
+ "source": "iana",
+ "extensions": ["h261"]
+ },
+ "video/h263": {
+ "source": "iana",
+ "extensions": ["h263"]
+ },
+ "video/h263-1998": {
+ "source": "iana"
+ },
+ "video/h263-2000": {
+ "source": "iana"
+ },
+ "video/h264": {
+ "source": "iana",
+ "extensions": ["h264"]
+ },
+ "video/h264-rcdo": {
+ "source": "iana"
+ },
+ "video/h264-svc": {
+ "source": "iana"
+ },
+ "video/h265": {
+ "source": "iana"
+ },
+ "video/iso.segment": {
+ "source": "iana"
+ },
+ "video/jpeg": {
+ "source": "iana",
+ "extensions": ["jpgv"]
+ },
+ "video/jpeg2000": {
+ "source": "iana"
+ },
+ "video/jpm": {
+ "source": "apache",
+ "extensions": ["jpm","jpgm"]
+ },
+ "video/mj2": {
+ "source": "iana",
+ "extensions": ["mj2","mjp2"]
+ },
+ "video/mp1s": {
+ "source": "iana"
+ },
+ "video/mp2p": {
+ "source": "iana"
+ },
+ "video/mp2t": {
+ "source": "iana",
+ "extensions": ["ts"]
+ },
+ "video/mp4": {
+ "source": "iana",
+ "compressible": false,
+ "extensions": ["mp4","mp4v","mpg4"]
+ },
+ "video/mp4v-es": {
+ "source": "iana"
+ },
+ "video/mpeg": {
+ "source": "iana",
+ "compressible": false,
+ "extensions": ["mpeg","mpg","mpe","m1v","m2v"]
+ },
+ "video/mpeg4-generic": {
+ "source": "iana"
+ },
+ "video/mpv": {
+ "source": "iana"
+ },
+ "video/nv": {
+ "source": "iana"
+ },
+ "video/ogg": {
+ "source": "iana",
+ "compressible": false,
+ "extensions": ["ogv"]
+ },
+ "video/parityfec": {
+ "source": "iana"
+ },
+ "video/pointer": {
+ "source": "iana"
+ },
+ "video/quicktime": {
+ "source": "iana",
+ "compressible": false,
+ "extensions": ["qt","mov"]
+ },
+ "video/raptorfec": {
+ "source": "iana"
+ },
+ "video/raw": {
+ "source": "iana"
+ },
+ "video/rtp-enc-aescm128": {
+ "source": "iana"
+ },
+ "video/rtploopback": {
+ "source": "iana"
+ },
+ "video/rtx": {
+ "source": "iana"
+ },
+ "video/smpte291": {
+ "source": "iana"
+ },
+ "video/smpte292m": {
+ "source": "iana"
+ },
+ "video/ulpfec": {
+ "source": "iana"
+ },
+ "video/vc1": {
+ "source": "iana"
+ },
+ "video/vc2": {
+ "source": "iana"
+ },
+ "video/vnd.cctv": {
+ "source": "iana"
+ },
+ "video/vnd.dece.hd": {
+ "source": "iana",
+ "extensions": ["uvh","uvvh"]
+ },
+ "video/vnd.dece.mobile": {
+ "source": "iana",
+ "extensions": ["uvm","uvvm"]
+ },
+ "video/vnd.dece.mp4": {
+ "source": "iana"
+ },
+ "video/vnd.dece.pd": {
+ "source": "iana",
+ "extensions": ["uvp","uvvp"]
+ },
+ "video/vnd.dece.sd": {
+ "source": "iana",
+ "extensions": ["uvs","uvvs"]
+ },
+ "video/vnd.dece.video": {
+ "source": "iana",
+ "extensions": ["uvv","uvvv"]
+ },
+ "video/vnd.directv.mpeg": {
+ "source": "iana"
+ },
+ "video/vnd.directv.mpeg-tts": {
+ "source": "iana"
+ },
+ "video/vnd.dlna.mpeg-tts": {
+ "source": "iana"
+ },
+ "video/vnd.dvb.file": {
+ "source": "iana",
+ "extensions": ["dvb"]
+ },
+ "video/vnd.fvt": {
+ "source": "iana",
+ "extensions": ["fvt"]
+ },
+ "video/vnd.hns.video": {
+ "source": "iana"
+ },
+ "video/vnd.iptvforum.1dparityfec-1010": {
+ "source": "iana"
+ },
+ "video/vnd.iptvforum.1dparityfec-2005": {
+ "source": "iana"
+ },
+ "video/vnd.iptvforum.2dparityfec-1010": {
+ "source": "iana"
+ },
+ "video/vnd.iptvforum.2dparityfec-2005": {
+ "source": "iana"
+ },
+ "video/vnd.iptvforum.ttsavc": {
+ "source": "iana"
+ },
+ "video/vnd.iptvforum.ttsmpeg2": {
+ "source": "iana"
+ },
+ "video/vnd.motorola.video": {
+ "source": "iana"
+ },
+ "video/vnd.motorola.videop": {
+ "source": "iana"
+ },
+ "video/vnd.mpegurl": {
+ "source": "iana",
+ "extensions": ["mxu","m4u"]
+ },
+ "video/vnd.ms-playready.media.pyv": {
+ "source": "iana",
+ "extensions": ["pyv"]
+ },
+ "video/vnd.nokia.interleaved-multimedia": {
+ "source": "iana"
+ },
+ "video/vnd.nokia.mp4vr": {
+ "source": "iana"
+ },
+ "video/vnd.nokia.videovoip": {
+ "source": "iana"
+ },
+ "video/vnd.objectvideo": {
+ "source": "iana"
+ },
+ "video/vnd.radgamettools.bink": {
+ "source": "iana"
+ },
+ "video/vnd.radgamettools.smacker": {
+ "source": "iana"
+ },
+ "video/vnd.sealed.mpeg1": {
+ "source": "iana"
+ },
+ "video/vnd.sealed.mpeg4": {
+ "source": "iana"
+ },
+ "video/vnd.sealed.swf": {
+ "source": "iana"
+ },
+ "video/vnd.sealedmedia.softseal.mov": {
+ "source": "iana"
+ },
+ "video/vnd.uvvu.mp4": {
+ "source": "iana",
+ "extensions": ["uvu","uvvu"]
+ },
+ "video/vnd.vivo": {
+ "source": "iana",
+ "extensions": ["viv"]
+ },
+ "video/vp8": {
+ "source": "iana"
+ },
+ "video/webm": {
+ "source": "apache",
+ "compressible": false,
+ "extensions": ["webm"]
+ },
+ "video/x-f4v": {
+ "source": "apache",
+ "extensions": ["f4v"]
+ },
+ "video/x-fli": {
+ "source": "apache",
+ "extensions": ["fli"]
+ },
+ "video/x-flv": {
+ "source": "apache",
+ "compressible": false,
+ "extensions": ["flv"]
+ },
+ "video/x-m4v": {
+ "source": "apache",
+ "extensions": ["m4v"]
+ },
+ "video/x-matroska": {
+ "source": "apache",
+ "compressible": false,
+ "extensions": ["mkv","mk3d","mks"]
+ },
+ "video/x-mng": {
+ "source": "apache",
+ "extensions": ["mng"]
+ },
+ "video/x-ms-asf": {
+ "source": "apache",
+ "extensions": ["asf","asx"]
+ },
+ "video/x-ms-vob": {
+ "source": "apache",
+ "extensions": ["vob"]
+ },
+ "video/x-ms-wm": {
+ "source": "apache",
+ "extensions": ["wm"]
+ },
+ "video/x-ms-wmv": {
+ "source": "apache",
+ "compressible": false,
+ "extensions": ["wmv"]
+ },
+ "video/x-ms-wmx": {
+ "source": "apache",
+ "extensions": ["wmx"]
+ },
+ "video/x-ms-wvx": {
+ "source": "apache",
+ "extensions": ["wvx"]
+ },
+ "video/x-msvideo": {
+ "source": "apache",
+ "extensions": ["avi"]
+ },
+ "video/x-sgi-movie": {
+ "source": "apache",
+ "extensions": ["movie"]
+ },
+ "video/x-smv": {
+ "source": "apache",
+ "extensions": ["smv"]
+ },
+ "x-conference/x-cooltalk": {
+ "source": "apache",
+ "extensions": ["ice"]
+ },
+ "x-shader/x-fragment": {
+ "compressible": true
+ },
+ "x-shader/x-vertex": {
+ "compressible": true
+ }
+}
diff --git a/node_modules/mime-db/index.js b/node_modules/mime-db/index.js
new file mode 100644
index 0000000..551031f
--- /dev/null
+++ b/node_modules/mime-db/index.js
@@ -0,0 +1,11 @@
+/*!
+ * mime-db
+ * Copyright(c) 2014 Jonathan Ong
+ * MIT Licensed
+ */
+
+/**
+ * Module exports.
+ */
+
+module.exports = require('./db.json')
diff --git a/node_modules/mime-db/package.json b/node_modules/mime-db/package.json
new file mode 100644
index 0000000..b526832
--- /dev/null
+++ b/node_modules/mime-db/package.json
@@ -0,0 +1,100 @@
+{
+ "_from": "mime-db@~1.37.0",
+ "_id": "mime-db@1.37.0",
+ "_inBundle": false,
+ "_integrity": "sha512-R3C4db6bgQhlIhPU48fUtdVmKnflq+hRdad7IyKhtFj06VPNVdk2RhiYL3UjQIlso8L+YxAtFkobT0VK+S/ybg==",
+ "_location": "/mime-db",
+ "_phantomChildren": {},
+ "_requested": {
+ "type": "range",
+ "registry": true,
+ "raw": "mime-db@~1.37.0",
+ "name": "mime-db",
+ "escapedName": "mime-db",
+ "rawSpec": "~1.37.0",
+ "saveSpec": null,
+ "fetchSpec": "~1.37.0"
+ },
+ "_requiredBy": [
+ "/mime-types"
+ ],
+ "_resolved": "https://registry.npmjs.org/mime-db/-/mime-db-1.37.0.tgz",
+ "_shasum": "0b6a0ce6fdbe9576e25f1f2d2fde8830dc0ad0d8",
+ "_spec": "mime-db@~1.37.0",
+ "_where": "/Users/rpatra16/MyProjects/RamAndAntara/node_modules/mime-types",
+ "bugs": {
+ "url": "https://github.com/jshttp/mime-db/issues"
+ },
+ "bundleDependencies": false,
+ "contributors": [
+ {
+ "name": "Douglas Christopher Wilson",
+ "email": "doug@somethingdoug.com"
+ },
+ {
+ "name": "Jonathan Ong",
+ "email": "me@jongleberry.com",
+ "url": "http://jongleberry.com"
+ },
+ {
+ "name": "Robert Kieffer",
+ "email": "robert@broofa.com",
+ "url": "http://github.com/broofa"
+ }
+ ],
+ "deprecated": false,
+ "description": "Media Type Database",
+ "devDependencies": {
+ "bluebird": "3.5.2",
+ "co": "4.6.0",
+ "cogent": "1.0.1",
+ "csv-parse": "2.5.0",
+ "eslint": "5.7.0",
+ "eslint-config-standard": "12.0.0",
+ "eslint-plugin-import": "2.14.0",
+ "eslint-plugin-node": "7.0.1",
+ "eslint-plugin-promise": "4.0.1",
+ "eslint-plugin-standard": "4.0.0",
+ "gnode": "0.1.2",
+ "mocha": "5.2.0",
+ "nyc": "13.1.0",
+ "raw-body": "2.3.3",
+ "stream-to-array": "2.3.0"
+ },
+ "engines": {
+ "node": ">= 0.6"
+ },
+ "files": [
+ "HISTORY.md",
+ "LICENSE",
+ "README.md",
+ "db.json",
+ "index.js"
+ ],
+ "homepage": "https://github.com/jshttp/mime-db#readme",
+ "keywords": [
+ "mime",
+ "db",
+ "type",
+ "types",
+ "database",
+ "charset",
+ "charsets"
+ ],
+ "license": "MIT",
+ "name": "mime-db",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/jshttp/mime-db.git"
+ },
+ "scripts": {
+ "build": "node scripts/build",
+ "fetch": "node scripts/fetch-apache && gnode scripts/fetch-iana && node scripts/fetch-nginx",
+ "lint": "eslint .",
+ "test": "mocha --reporter spec --bail --check-leaks test/",
+ "test-cov": "nyc --reporter=html --reporter=text npm test",
+ "test-travis": "nyc --reporter=text npm test",
+ "update": "npm run fetch && npm run build"
+ },
+ "version": "1.37.0"
+}
diff --git a/node_modules/mime-types/HISTORY.md b/node_modules/mime-types/HISTORY.md
new file mode 100644
index 0000000..dd7f4f8
--- /dev/null
+++ b/node_modules/mime-types/HISTORY.md
@@ -0,0 +1,285 @@
+2.1.21 / 2018-10-19
+===================
+
+ * deps: mime-db@~1.37.0
+ - Add extensions to HEIC image types
+ - Add new upstream MIME types
+
+2.1.20 / 2018-08-26
+===================
+
+ * deps: mime-db@~1.36.0
+ - Add Apple file extensions from IANA
+ - Add extensions from IANA for `image/*` types
+ - Add new upstream MIME types
+
+2.1.19 / 2018-07-17
+===================
+
+ * deps: mime-db@~1.35.0
+ - Add extension `.csl` to `application/vnd.citationstyles.style+xml`
+ - Add extension `.es` to `application/ecmascript`
+ - Add extension `.owl` to `application/rdf+xml`
+ - Add new upstream MIME types
+ - Add UTF-8 as default charset for `text/turtle`
+
+2.1.18 / 2018-02-16
+===================
+
+ * deps: mime-db@~1.33.0
+ - Add `application/raml+yaml` with extension `.raml`
+ - Add `application/wasm` with extension `.wasm`
+ - Add `text/shex` with extension `.shex`
+ - Add extensions for JPEG-2000 images
+ - Add extensions from IANA for `message/*` types
+ - Add new upstream MIME types
+ - Update font MIME types
+ - Update `text/hjson` to registered `application/hjson`
+
+2.1.17 / 2017-09-01
+===================
+
+ * deps: mime-db@~1.30.0
+ - Add `application/vnd.ms-outlook`
+ - Add `application/x-arj`
+ - Add extension `.mjs` to `application/javascript`
+ - Add glTF types and extensions
+ - Add new upstream MIME types
+ - Add `text/x-org`
+ - Add VirtualBox MIME types
+ - Fix `source` records for `video/*` types that are IANA
+ - Update `font/opentype` to registered `font/otf`
+
+2.1.16 / 2017-07-24
+===================
+
+ * deps: mime-db@~1.29.0
+ - Add `application/fido.trusted-apps+json`
+ - Add extension `.wadl` to `application/vnd.sun.wadl+xml`
+ - Add extension `.gz` to `application/gzip`
+ - Add new upstream MIME types
+ - Update extensions `.md` and `.markdown` to be `text/markdown`
+
+2.1.15 / 2017-03-23
+===================
+
+ * deps: mime-db@~1.27.0
+ - Add new mime types
+ - Add `image/apng`
+
+2.1.14 / 2017-01-14
+===================
+
+ * deps: mime-db@~1.26.0
+ - Add new mime types
+
+2.1.13 / 2016-11-18
+===================
+
+ * deps: mime-db@~1.25.0
+ - Add new mime types
+
+2.1.12 / 2016-09-18
+===================
+
+ * deps: mime-db@~1.24.0
+ - Add new mime types
+ - Add `audio/mp3`
+
+2.1.11 / 2016-05-01
+===================
+
+ * deps: mime-db@~1.23.0
+ - Add new mime types
+
+2.1.10 / 2016-02-15
+===================
+
+ * deps: mime-db@~1.22.0
+ - Add new mime types
+ - Fix extension of `application/dash+xml`
+ - Update primary extension for `audio/mp4`
+
+2.1.9 / 2016-01-06
+==================
+
+ * deps: mime-db@~1.21.0
+ - Add new mime types
+
+2.1.8 / 2015-11-30
+==================
+
+ * deps: mime-db@~1.20.0
+ - Add new mime types
+
+2.1.7 / 2015-09-20
+==================
+
+ * deps: mime-db@~1.19.0
+ - Add new mime types
+
+2.1.6 / 2015-09-03
+==================
+
+ * deps: mime-db@~1.18.0
+ - Add new mime types
+
+2.1.5 / 2015-08-20
+==================
+
+ * deps: mime-db@~1.17.0
+ - Add new mime types
+
+2.1.4 / 2015-07-30
+==================
+
+ * deps: mime-db@~1.16.0
+ - Add new mime types
+
+2.1.3 / 2015-07-13
+==================
+
+ * deps: mime-db@~1.15.0
+ - Add new mime types
+
+2.1.2 / 2015-06-25
+==================
+
+ * deps: mime-db@~1.14.0
+ - Add new mime types
+
+2.1.1 / 2015-06-08
+==================
+
+ * perf: fix deopt during mapping
+
+2.1.0 / 2015-06-07
+==================
+
+ * Fix incorrectly treating extension-less file name as extension
+ - i.e. `'path/to/json'` will no longer return `application/json`
+ * Fix `.charset(type)` to accept parameters
+ * Fix `.charset(type)` to match case-insensitive
+ * Improve generation of extension to MIME mapping
+ * Refactor internals for readability and no argument reassignment
+ * Prefer `application/*` MIME types from the same source
+ * Prefer any type over `application/octet-stream`
+ * deps: mime-db@~1.13.0
+ - Add nginx as a source
+ - Add new mime types
+
+2.0.14 / 2015-06-06
+===================
+
+ * deps: mime-db@~1.12.0
+ - Add new mime types
+
+2.0.13 / 2015-05-31
+===================
+
+ * deps: mime-db@~1.11.0
+ - Add new mime types
+
+2.0.12 / 2015-05-19
+===================
+
+ * deps: mime-db@~1.10.0
+ - Add new mime types
+
+2.0.11 / 2015-05-05
+===================
+
+ * deps: mime-db@~1.9.1
+ - Add new mime types
+
+2.0.10 / 2015-03-13
+===================
+
+ * deps: mime-db@~1.8.0
+ - Add new mime types
+
+2.0.9 / 2015-02-09
+==================
+
+ * deps: mime-db@~1.7.0
+ - Add new mime types
+ - Community extensions ownership transferred from `node-mime`
+
+2.0.8 / 2015-01-29
+==================
+
+ * deps: mime-db@~1.6.0
+ - Add new mime types
+
+2.0.7 / 2014-12-30
+==================
+
+ * deps: mime-db@~1.5.0
+ - Add new mime types
+ - Fix various invalid MIME type entries
+
+2.0.6 / 2014-12-30
+==================
+
+ * deps: mime-db@~1.4.0
+ - Add new mime types
+ - Fix various invalid MIME type entries
+ - Remove example template MIME types
+
+2.0.5 / 2014-12-29
+==================
+
+ * deps: mime-db@~1.3.1
+ - Fix missing extensions
+
+2.0.4 / 2014-12-10
+==================
+
+ * deps: mime-db@~1.3.0
+ - Add new mime types
+
+2.0.3 / 2014-11-09
+==================
+
+ * deps: mime-db@~1.2.0
+ - Add new mime types
+
+2.0.2 / 2014-09-28
+==================
+
+ * deps: mime-db@~1.1.0
+ - Add new mime types
+ - Add additional compressible
+ - Update charsets
+
+2.0.1 / 2014-09-07
+==================
+
+ * Support Node.js 0.6
+
+2.0.0 / 2014-09-02
+==================
+
+ * Use `mime-db`
+ * Remove `.define()`
+
+1.0.2 / 2014-08-04
+==================
+
+ * Set charset=utf-8 for `text/javascript`
+
+1.0.1 / 2014-06-24
+==================
+
+ * Add `text/jsx` type
+
+1.0.0 / 2014-05-12
+==================
+
+ * Return `false` for unknown types
+ * Set charset=utf-8 for `application/json`
+
+0.1.0 / 2014-05-02
+==================
+
+ * Initial release
diff --git a/node_modules/mime-types/LICENSE b/node_modules/mime-types/LICENSE
new file mode 100644
index 0000000..0616607
--- /dev/null
+++ b/node_modules/mime-types/LICENSE
@@ -0,0 +1,23 @@
+(The MIT License)
+
+Copyright (c) 2014 Jonathan Ong
+Copyright (c) 2015 Douglas Christopher Wilson
+
+Permission is hereby granted, free of charge, to any person obtaining
+a copy of this software and associated documentation files (the
+'Software'), to deal in the Software without restriction, including
+without limitation the rights to use, copy, modify, merge, publish,
+distribute, sublicense, and/or sell copies of the Software, and to
+permit persons to whom the Software is furnished to do so, subject to
+the following conditions:
+
+The above copyright notice and this permission notice shall be
+included in all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND,
+EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
+MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
+IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY
+CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT,
+TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE
+SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
diff --git a/node_modules/mime-types/README.md b/node_modules/mime-types/README.md
new file mode 100644
index 0000000..b68b52e
--- /dev/null
+++ b/node_modules/mime-types/README.md
@@ -0,0 +1,107 @@
+# mime-types
+
+[![NPM Version][npm-version-image]][npm-url]
+[![NPM Downloads][npm-downloads-image]][npm-url]
+[![Node.js Version][node-version-image]][node-version-url]
+[![Build Status][travis-image]][travis-url]
+[![Test Coverage][coveralls-image]][coveralls-url]
+
+The ultimate javascript content-type utility.
+
+Similar to [the `mime@1.x` module](https://www.npmjs.com/package/mime), except:
+
+- __No fallbacks.__ Instead of naively returning the first available type,
+ `mime-types` simply returns `false`, so do
+ `var type = mime.lookup('unrecognized') || 'application/octet-stream'`.
+- No `new Mime()` business, so you could do `var lookup = require('mime-types').lookup`.
+- No `.define()` functionality
+- Bug fixes for `.lookup(path)`
+
+Otherwise, the API is compatible with `mime` 1.x.
+
+## Install
+
+This is a [Node.js](https://nodejs.org/en/) module available through the
+[npm registry](https://www.npmjs.com/). Installation is done using the
+[`npm install` command](https://docs.npmjs.com/getting-started/installing-npm-packages-locally):
+
+```sh
+$ npm install mime-types
+```
+
+## Adding Types
+
+All mime types are based on [mime-db](https://www.npmjs.com/package/mime-db),
+so open a PR there if you'd like to add mime types.
+
+## API
+
+```js
+var mime = require('mime-types')
+```
+
+All functions return `false` if input is invalid or not found.
+
+### mime.lookup(path)
+
+Lookup the content-type associated with a file.
+
+```js
+mime.lookup('json') // 'application/json'
+mime.lookup('.md') // 'text/markdown'
+mime.lookup('file.html') // 'text/html'
+mime.lookup('folder/file.js') // 'application/javascript'
+mime.lookup('folder/.htaccess') // false
+
+mime.lookup('cats') // false
+```
+
+### mime.contentType(type)
+
+Create a full content-type header given a content-type or extension.
+
+```js
+mime.contentType('markdown') // 'text/x-markdown; charset=utf-8'
+mime.contentType('file.json') // 'application/json; charset=utf-8'
+
+// from a full path
+mime.contentType(path.extname('/path/to/file.json')) // 'application/json; charset=utf-8'
+```
+
+### mime.extension(type)
+
+Get the default extension for a content-type.
+
+```js
+mime.extension('application/octet-stream') // 'bin'
+```
+
+### mime.charset(type)
+
+Lookup the implied default charset of a content-type.
+
+```js
+mime.charset('text/markdown') // 'UTF-8'
+```
+
+### var type = mime.types[extension]
+
+A map of content-types by extension.
+
+### [extensions...] = mime.extensions[type]
+
+A map of extensions by content-type.
+
+## License
+
+[MIT](LICENSE)
+
+[coveralls-image]: https://badgen.net/coveralls/c/github/jshttp/mime-types/master
+[coveralls-url]: https://coveralls.io/r/jshttp/mime-types?branch=master
+[node-version-image]: https://badgen.net/npm/node/mime-types
+[node-version-url]: https://nodejs.org/en/download
+[npm-downloads-image]: https://badgen.net/npm/dm/mime-types
+[npm-url]: https://npmjs.org/package/mime-types
+[npm-version-image]: https://badgen.net/npm/v/mime-types
+[travis-image]: https://badgen.net/travis/jshttp/mime-types/master
+[travis-url]: https://travis-ci.org/jshttp/mime-types
diff --git a/node_modules/mime-types/index.js b/node_modules/mime-types/index.js
new file mode 100644
index 0000000..b9f34d5
--- /dev/null
+++ b/node_modules/mime-types/index.js
@@ -0,0 +1,188 @@
+/*!
+ * mime-types
+ * Copyright(c) 2014 Jonathan Ong
+ * Copyright(c) 2015 Douglas Christopher Wilson
+ * MIT Licensed
+ */
+
+'use strict'
+
+/**
+ * Module dependencies.
+ * @private
+ */
+
+var db = require('mime-db')
+var extname = require('path').extname
+
+/**
+ * Module variables.
+ * @private
+ */
+
+var EXTRACT_TYPE_REGEXP = /^\s*([^;\s]*)(?:;|\s|$)/
+var TEXT_TYPE_REGEXP = /^text\//i
+
+/**
+ * Module exports.
+ * @public
+ */
+
+exports.charset = charset
+exports.charsets = { lookup: charset }
+exports.contentType = contentType
+exports.extension = extension
+exports.extensions = Object.create(null)
+exports.lookup = lookup
+exports.types = Object.create(null)
+
+// Populate the extensions/types maps
+populateMaps(exports.extensions, exports.types)
+
+/**
+ * Get the default charset for a MIME type.
+ *
+ * @param {string} type
+ * @return {boolean|string}
+ */
+
+function charset (type) {
+ if (!type || typeof type !== 'string') {
+ return false
+ }
+
+ // TODO: use media-typer
+ var match = EXTRACT_TYPE_REGEXP.exec(type)
+ var mime = match && db[match[1].toLowerCase()]
+
+ if (mime && mime.charset) {
+ return mime.charset
+ }
+
+ // default text/* to utf-8
+ if (match && TEXT_TYPE_REGEXP.test(match[1])) {
+ return 'UTF-8'
+ }
+
+ return false
+}
+
+/**
+ * Create a full Content-Type header given a MIME type or extension.
+ *
+ * @param {string} str
+ * @return {boolean|string}
+ */
+
+function contentType (str) {
+ // TODO: should this even be in this module?
+ if (!str || typeof str !== 'string') {
+ return false
+ }
+
+ var mime = str.indexOf('/') === -1
+ ? exports.lookup(str)
+ : str
+
+ if (!mime) {
+ return false
+ }
+
+ // TODO: use content-type or other module
+ if (mime.indexOf('charset') === -1) {
+ var charset = exports.charset(mime)
+ if (charset) mime += '; charset=' + charset.toLowerCase()
+ }
+
+ return mime
+}
+
+/**
+ * Get the default extension for a MIME type.
+ *
+ * @param {string} type
+ * @return {boolean|string}
+ */
+
+function extension (type) {
+ if (!type || typeof type !== 'string') {
+ return false
+ }
+
+ // TODO: use media-typer
+ var match = EXTRACT_TYPE_REGEXP.exec(type)
+
+ // get extensions
+ var exts = match && exports.extensions[match[1].toLowerCase()]
+
+ if (!exts || !exts.length) {
+ return false
+ }
+
+ return exts[0]
+}
+
+/**
+ * Lookup the MIME type for a file path/extension.
+ *
+ * @param {string} path
+ * @return {boolean|string}
+ */
+
+function lookup (path) {
+ if (!path || typeof path !== 'string') {
+ return false
+ }
+
+ // get the extension ("ext" or ".ext" or full path)
+ var extension = extname('x.' + path)
+ .toLowerCase()
+ .substr(1)
+
+ if (!extension) {
+ return false
+ }
+
+ return exports.types[extension] || false
+}
+
+/**
+ * Populate the extensions and types maps.
+ * @private
+ */
+
+function populateMaps (extensions, types) {
+ // source preference (least -> most)
+ var preference = ['nginx', 'apache', undefined, 'iana']
+
+ Object.keys(db).forEach(function forEachMimeType (type) {
+ var mime = db[type]
+ var exts = mime.extensions
+
+ if (!exts || !exts.length) {
+ return
+ }
+
+ // mime -> extensions
+ extensions[type] = exts
+
+ // extension -> mime
+ for (var i = 0; i < exts.length; i++) {
+ var extension = exts[i]
+
+ if (types[extension]) {
+ var from = preference.indexOf(db[types[extension]].source)
+ var to = preference.indexOf(mime.source)
+
+ if (types[extension] !== 'application/octet-stream' &&
+ (from > to || (from === to && types[extension].substr(0, 12) === 'application/'))) {
+ // skip the remapping
+ continue
+ }
+ }
+
+ // set the extension -> mime
+ types[extension] = type
+ }
+ })
+}
diff --git a/node_modules/mime-types/package.json b/node_modules/mime-types/package.json
new file mode 100644
index 0000000..fda82ae
--- /dev/null
+++ b/node_modules/mime-types/package.json
@@ -0,0 +1,87 @@
+{
+ "_from": "mime-types@~2.1.19",
+ "_id": "mime-types@2.1.21",
+ "_inBundle": false,
+ "_integrity": "sha512-3iL6DbwpyLzjR3xHSFNFeb9Nz/M8WDkX33t1GFQnFOllWk8pOrh/LSrB5OXlnlW5P9LH73X6loW/eogc+F5lJg==",
+ "_location": "/mime-types",
+ "_phantomChildren": {},
+ "_requested": {
+ "type": "range",
+ "registry": true,
+ "raw": "mime-types@~2.1.19",
+ "name": "mime-types",
+ "escapedName": "mime-types",
+ "rawSpec": "~2.1.19",
+ "saveSpec": null,
+ "fetchSpec": "~2.1.19"
+ },
+ "_requiredBy": [
+ "/form-data",
+ "/request"
+ ],
+ "_resolved": "https://registry.npmjs.org/mime-types/-/mime-types-2.1.21.tgz",
+ "_shasum": "28995aa1ecb770742fe6ae7e58f9181c744b3f96",
+ "_spec": "mime-types@~2.1.19",
+ "_where": "/Users/rpatra16/MyProjects/RamAndAntara/node_modules/request",
+ "bugs": {
+ "url": "https://github.com/jshttp/mime-types/issues"
+ },
+ "bundleDependencies": false,
+ "contributors": [
+ {
+ "name": "Douglas Christopher Wilson",
+ "email": "doug@somethingdoug.com"
+ },
+ {
+ "name": "Jeremiah Senkpiel",
+ "email": "fishrock123@rocketmail.com",
+ "url": "https://searchbeam.jit.su"
+ },
+ {
+ "name": "Jonathan Ong",
+ "email": "me@jongleberry.com",
+ "url": "http://jongleberry.com"
+ }
+ ],
+ "dependencies": {
+ "mime-db": "~1.37.0"
+ },
+ "deprecated": false,
+ "description": "The ultimate javascript content-type utility.",
+ "devDependencies": {
+ "eslint": "5.7.0",
+ "eslint-config-standard": "12.0.0",
+ "eslint-plugin-import": "2.14.0",
+ "eslint-plugin-node": "7.0.1",
+ "eslint-plugin-promise": "4.0.1",
+ "eslint-plugin-standard": "4.0.0",
+ "mocha": "5.2.0",
+ "nyc": "13.1.0"
+ },
+ "engines": {
+ "node": ">= 0.6"
+ },
+ "files": [
+ "HISTORY.md",
+ "LICENSE",
+ "index.js"
+ ],
+ "homepage": "https://github.com/jshttp/mime-types#readme",
+ "keywords": [
+ "mime",
+ "types"
+ ],
+ "license": "MIT",
+ "name": "mime-types",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/jshttp/mime-types.git"
+ },
+ "scripts": {
+ "lint": "eslint .",
+ "test": "mocha --reporter spec test/test.js",
+ "test-cov": "nyc --reporter=html --reporter=text npm test",
+ "test-travis": "nyc --reporter=text npm test"
+ },
+ "version": "2.1.21"
+}
diff --git a/node_modules/minimatch/LICENSE b/node_modules/minimatch/LICENSE
new file mode 100644
index 0000000..19129e3
--- /dev/null
+++ b/node_modules/minimatch/LICENSE
@@ -0,0 +1,15 @@
+The ISC License
+
+Copyright (c) Isaac Z. Schlueter and Contributors
+
+Permission to use, copy, modify, and/or distribute this software for any
+purpose with or without fee is hereby granted, provided that the above
+copyright notice and this permission notice appear in all copies.
+
+THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES
+WITH REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF
+MERCHANTABILITY AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR
+ANY SPECIAL, DIRECT, INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES
+WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS, WHETHER IN AN
+ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS ACTION, ARISING OUT OF OR
+IN CONNECTION WITH THE USE OR PERFORMANCE OF THIS SOFTWARE.
diff --git a/node_modules/minimatch/README.md b/node_modules/minimatch/README.md
new file mode 100644
index 0000000..d458bc2
--- /dev/null
+++ b/node_modules/minimatch/README.md
@@ -0,0 +1,216 @@
+# minimatch
+
+A minimal matching utility.
+
+[](http://travis-ci.org/isaacs/minimatch)
+
+
+This is the matching library used internally by npm.
+
+It works by converting glob expressions into JavaScript `RegExp`
+objects.
+
+## Usage
+
+```javascript
+var minimatch = require("minimatch")
+
+minimatch("bar.foo", "*.foo") // true!
+minimatch("bar.foo", "*.bar") // false!
+minimatch("bar.foo", "*.+(bar|foo)", { debug: true }) // true, and noisy!
+```
+
+## Features
+
+Supports these glob features:
+
+* Brace Expansion
+* Extended glob matching
+* "Globstar" `**` matching
+
+See:
+
+* `man sh`
+* `man bash`
+* `man 3 fnmatch`
+* `man 5 gitignore`
+
+## Minimatch Class
+
+Create a minimatch object by instanting the `minimatch.Minimatch` class.
+
+```javascript
+var Minimatch = require("minimatch").Minimatch
+var mm = new Minimatch(pattern, options)
+```
+
+### Properties
+
+* `pattern` The original pattern the minimatch object represents.
+* `options` The options supplied to the constructor.
+* `set` A 2-dimensional array of regexp or string expressions.
+ Each row in the
+ array corresponds to a brace-expanded pattern. Each item in the row
+ corresponds to a single path-part. For example, the pattern
+ `{a,b/c}/d` would expand to a set of patterns like:
+
+ [ [ a, d ]
+ , [ b, c, d ] ]
+
+ If a portion of the pattern doesn't have any "magic" in it
+ (that is, it's something like `"foo"` rather than `fo*o?`), then it
+ will be left as a string rather than converted to a regular
+ expression.
+
+* `regexp` Created by the `makeRe` method. A single regular expression
+ expressing the entire pattern. This is useful in cases where you wish
+ to use the pattern somewhat like `fnmatch(3)` with `FNM_PATH` enabled.
+* `negate` True if the pattern is negated.
+* `comment` True if the pattern is a comment.
+* `empty` True if the pattern is `""`.
+
+### Methods
+
+* `makeRe` Generate the `regexp` member if necessary, and return it.
+ Will return `false` if the pattern is invalid.
+* `match(fname)` Return true if the filename matches the pattern, or
+ false otherwise.
+* `matchOne(fileArray, patternArray, partial)` Take a `/`-split
+ filename, and match it against a single row in the `regExpSet`. This
+ method is mainly for internal use, but is exposed so that it can be
+ used by a glob-walker that needs to avoid excessive filesystem calls.
+
+All other methods are internal, and will be called as necessary.
+
+## Functions
+
+The top-level exported function has a `cache` property, which is an LRU
+cache set to store 100 items. So, calling these methods repeatedly
+with the same pattern and options will use the same Minimatch object,
+saving the cost of parsing it multiple times.
+
+### minimatch(path, pattern, options)
+
+Main export. Tests a path against the pattern using the options.
+
+```javascript
+var isJS = minimatch(file, "*.js", { matchBase: true })
+```
+
+### minimatch.filter(pattern, options)
+
+Returns a function that tests its
+supplied argument, suitable for use with `Array.filter`. Example:
+
+```javascript
+var javascripts = fileList.filter(minimatch.filter("*.js", {matchBase: true}))
+```
+
+### minimatch.match(list, pattern, options)
+
+Match against the list of
+files, in the style of fnmatch or glob. If nothing is matched, and
+options.nonull is set, then return a list containing the pattern itself.
+
+```javascript
+var javascripts = minimatch.match(fileList, "*.js", {matchBase: true}))
+```
+
+### minimatch.makeRe(pattern, options)
+
+Make a regular expression object from the pattern.
+
+## Options
+
+All options are `false` by default.
+
+### debug
+
+Dump a ton of stuff to stderr.
+
+### nobrace
+
+Do not expand `{a,b}` and `{1..3}` brace sets.
+
+### noglobstar
+
+Disable `**` matching against multiple folder names.
+
+### dot
+
+Allow patterns to match filenames starting with a period, even if
+the pattern does not explicitly have a period in that spot.
+
+Note that by default, `a/**/b` will **not** match `a/.d/b`, unless `dot`
+is set.
+
+### noext
+
+Disable "extglob" style patterns like `+(a|b)`.
+
+### nocase
+
+Perform a case-insensitive match.
+
+### nonull
+
+When a match is not found by `minimatch.match`, return a list containing
+the pattern itself if this option is set. When not set, an empty list
+is returned if there are no matches.
+
+### matchBase
+
+If set, then patterns without slashes will be matched
+against the basename of the path if it contains slashes. For example,
+`a?b` would match the path `/xyz/123/acb`, but not `/xyz/acb/123`.
+
+### nocomment
+
+Suppress the behavior of treating `#` at the start of a pattern as a
+comment.
+
+### nonegate
+
+Suppress the behavior of treating a leading `!` character as negation.
+
+### flipNegate
+
+Returns from negate expressions the same as if they were not negated.
+(Ie, true on a hit, false on a miss.)
+
+
+## Comparisons to other fnmatch/glob implementations
+
+While strict compliance with the existing standards is a worthwhile
+goal, some discrepancies exist between minimatch and other
+implementations, and are intentional.
+
+If the pattern starts with a `!` character, then it is negated. Set the
+`nonegate` flag to suppress this behavior, and treat leading `!`
+characters normally. This is perhaps relevant if you wish to start the
+pattern with a negative extglob pattern like `!(a|B)`. Multiple `!`
+characters at the start of a pattern will negate the pattern multiple
+times.
+
+If a pattern starts with `#`, then it is treated as a comment, and
+will not match anything. Use `\#` to match a literal `#` at the
+start of a line, or set the `nocomment` flag to suppress this behavior.
+
+The double-star character `**` is supported by default, unless the
+`noglobstar` flag is set. This is supported in the manner of bsdglob
+and bash 4.1, where `**` only has special significance if it is the only
+thing in a path part. That is, `a/**/b` will match `a/x/y/b`, but
+`a/**b` will not.
+
+If an escaped pattern has no matches, and the `nonull` flag is set,
+then minimatch.match returns the pattern as-provided, rather than
+interpreting the character escapes. For example,
+`minimatch.match([], "\\*a\\?")` will return `"\\*a\\?"` rather than
+`"*a?"`. This is akin to setting the `nullglob` option in bash, except
+that it does not resolve escaped pattern characters.
+
+If brace expansion is not disabled, then it is performed before any
+other interpretation of the glob pattern. Thus, a pattern like
+`+(a|{b),c)}`, which would not be valid in bash or zsh, is expanded
+**first** into the set of `+(a|b)` and `+(a|c)`, and those patterns are
+checked for validity. Since those two are valid, matching proceeds.
diff --git a/node_modules/minimatch/browser.js b/node_modules/minimatch/browser.js
new file mode 100644
index 0000000..7d05159
--- /dev/null
+++ b/node_modules/minimatch/browser.js
@@ -0,0 +1,1159 @@
+(function(f){if(typeof exports==="object"&&typeof module!=="undefined"){module.exports=f()}else if(typeof define==="function"&&define.amd){define([],f)}else{var g;if(typeof window!=="undefined"){g=window}else if(typeof global!=="undefined"){g=global}else if(typeof self!=="undefined"){g=self}else{g=this}g.minimatch = f()}})(function(){var define,module,exports;return (function e(t,n,r){function s(o,u){if(!n[o]){if(!t[o]){var a=typeof require=="function"&&require;if(!u&&a)return a(o,!0);if(i)return i(o,!0);var f=new Error("Cannot find module '"+o+"'");throw f.code="MODULE_NOT_FOUND",f}var l=n[o]={exports:{}};t[o][0].call(l.exports,function(e){var n=t[o][1][e];return s(n?n:e)},l,l.exports,e,t,n,r)}return n[o].exports}var i=typeof require=="function"&&require;for(var o=0;o any number of characters
+var star = qmark + '*?'
+
+// ** when dots are allowed. Anything goes, except .. and .
+// not (^ or / followed by one or two dots followed by $ or /),
+// followed by anything, any number of times.
+var twoStarDot = '(?:(?!(?:\\\/|^)(?:\\.{1,2})($|\\\/)).)*?'
+
+// not a ^ or / followed by a dot,
+// followed by anything, any number of times.
+var twoStarNoDot = '(?:(?!(?:\\\/|^)\\.).)*?'
+
+// characters that need to be escaped in RegExp.
+var reSpecials = charSet('().*{}+?[]^$\\!')
+
+// "abc" -> { a:true, b:true, c:true }
+function charSet (s) {
+ return s.split('').reduce(function (set, c) {
+ set[c] = true
+ return set
+ }, {})
+}
+
+// normalizes slashes.
+var slashSplit = /\/+/
+
+minimatch.filter = filter
+function filter (pattern, options) {
+ options = options || {}
+ return function (p, i, list) {
+ return minimatch(p, pattern, options)
+ }
+}
+
+function ext (a, b) {
+ a = a || {}
+ b = b || {}
+ var t = {}
+ Object.keys(b).forEach(function (k) {
+ t[k] = b[k]
+ })
+ Object.keys(a).forEach(function (k) {
+ t[k] = a[k]
+ })
+ return t
+}
+
+minimatch.defaults = function (def) {
+ if (!def || !Object.keys(def).length) return minimatch
+
+ var orig = minimatch
+
+ var m = function minimatch (p, pattern, options) {
+ return orig.minimatch(p, pattern, ext(def, options))
+ }
+
+ m.Minimatch = function Minimatch (pattern, options) {
+ return new orig.Minimatch(pattern, ext(def, options))
+ }
+
+ return m
+}
+
+Minimatch.defaults = function (def) {
+ if (!def || !Object.keys(def).length) return Minimatch
+ return minimatch.defaults(def).Minimatch
+}
+
+function minimatch (p, pattern, options) {
+ if (typeof pattern !== 'string') {
+ throw new TypeError('glob pattern string required')
+ }
+
+ if (!options) options = {}
+
+ // shortcut: comments match nothing.
+ if (!options.nocomment && pattern.charAt(0) === '#') {
+ return false
+ }
+
+ // "" only matches ""
+ if (pattern.trim() === '') return p === ''
+
+ return new Minimatch(pattern, options).match(p)
+}
+
+function Minimatch (pattern, options) {
+ if (!(this instanceof Minimatch)) {
+ return new Minimatch(pattern, options)
+ }
+
+ if (typeof pattern !== 'string') {
+ throw new TypeError('glob pattern string required')
+ }
+
+ if (!options) options = {}
+ pattern = pattern.trim()
+
+ // windows support: need to use /, not \
+ if (path.sep !== '/') {
+ pattern = pattern.split(path.sep).join('/')
+ }
+
+ this.options = options
+ this.set = []
+ this.pattern = pattern
+ this.regexp = null
+ this.negate = false
+ this.comment = false
+ this.empty = false
+
+ // make the set of regexps etc.
+ this.make()
+}
+
+Minimatch.prototype.debug = function () {}
+
+Minimatch.prototype.make = make
+function make () {
+ // don't do it more than once.
+ if (this._made) return
+
+ var pattern = this.pattern
+ var options = this.options
+
+ // empty patterns and comments match nothing.
+ if (!options.nocomment && pattern.charAt(0) === '#') {
+ this.comment = true
+ return
+ }
+ if (!pattern) {
+ this.empty = true
+ return
+ }
+
+ // step 1: figure out negation, etc.
+ this.parseNegate()
+
+ // step 2: expand braces
+ var set = this.globSet = this.braceExpand()
+
+ if (options.debug) this.debug = console.error
+
+ this.debug(this.pattern, set)
+
+ // step 3: now we have a set, so turn each one into a series of path-portion
+ // matching patterns.
+ // These will be regexps, except in the case of "**", which is
+ // set to the GLOBSTAR object for globstar behavior,
+ // and will not contain any / characters
+ set = this.globParts = set.map(function (s) {
+ return s.split(slashSplit)
+ })
+
+ this.debug(this.pattern, set)
+
+ // glob --> regexps
+ set = set.map(function (s, si, set) {
+ return s.map(this.parse, this)
+ }, this)
+
+ this.debug(this.pattern, set)
+
+ // filter out everything that didn't compile properly.
+ set = set.filter(function (s) {
+ return s.indexOf(false) === -1
+ })
+
+ this.debug(this.pattern, set)
+
+ this.set = set
+}
+
+Minimatch.prototype.parseNegate = parseNegate
+function parseNegate () {
+ var pattern = this.pattern
+ var negate = false
+ var options = this.options
+ var negateOffset = 0
+
+ if (options.nonegate) return
+
+ for (var i = 0, l = pattern.length
+ ; i < l && pattern.charAt(i) === '!'
+ ; i++) {
+ negate = !negate
+ negateOffset++
+ }
+
+ if (negateOffset) this.pattern = pattern.substr(negateOffset)
+ this.negate = negate
+}
+
+// Brace expansion:
+// a{b,c}d -> abd acd
+// a{b,}c -> abc ac
+// a{0..3}d -> a0d a1d a2d a3d
+// a{b,c{d,e}f}g -> abg acdfg acefg
+// a{b,c}d{e,f}g -> abdeg acdeg abdeg abdfg
+//
+// Invalid sets are not expanded.
+// a{2..}b -> a{2..}b
+// a{b}c -> a{b}c
+minimatch.braceExpand = function (pattern, options) {
+ return braceExpand(pattern, options)
+}
+
+Minimatch.prototype.braceExpand = braceExpand
+
+function braceExpand (pattern, options) {
+ if (!options) {
+ if (this instanceof Minimatch) {
+ options = this.options
+ } else {
+ options = {}
+ }
+ }
+
+ pattern = typeof pattern === 'undefined'
+ ? this.pattern : pattern
+
+ if (typeof pattern === 'undefined') {
+ throw new Error('undefined pattern')
+ }
+
+ if (options.nobrace ||
+ !pattern.match(/\{.*\}/)) {
+ // shortcut. no need to expand.
+ return [pattern]
+ }
+
+ return expand(pattern)
+}
+
+// parse a component of the expanded set.
+// At this point, no pattern may contain "/" in it
+// so we're going to return a 2d array, where each entry is the full
+// pattern, split on '/', and then turned into a regular expression.
+// A regexp is made at the end which joins each array with an
+// escaped /, and another full one which joins each regexp with |.
+//
+// Following the lead of Bash 4.1, note that "**" only has special meaning
+// when it is the *only* thing in a path portion. Otherwise, any series
+// of * is equivalent to a single *. Globstar behavior is enabled by
+// default, and can be disabled by setting options.noglobstar.
+Minimatch.prototype.parse = parse
+var SUBPARSE = {}
+function parse (pattern, isSub) {
+ var options = this.options
+
+ // shortcuts
+ if (!options.noglobstar && pattern === '**') return GLOBSTAR
+ if (pattern === '') return ''
+
+ var re = ''
+ var hasMagic = !!options.nocase
+ var escaping = false
+ // ? => one single character
+ var patternListStack = []
+ var negativeLists = []
+ var plType
+ var stateChar
+ var inClass = false
+ var reClassStart = -1
+ var classStart = -1
+ // . and .. never match anything that doesn't start with .,
+ // even when options.dot is set.
+ var patternStart = pattern.charAt(0) === '.' ? '' // anything
+ // not (start or / followed by . or .. followed by / or end)
+ : options.dot ? '(?!(?:^|\\\/)\\.{1,2}(?:$|\\\/))'
+ : '(?!\\.)'
+ var self = this
+
+ function clearStateChar () {
+ if (stateChar) {
+ // we had some state-tracking character
+ // that wasn't consumed by this pass.
+ switch (stateChar) {
+ case '*':
+ re += star
+ hasMagic = true
+ break
+ case '?':
+ re += qmark
+ hasMagic = true
+ break
+ default:
+ re += '\\' + stateChar
+ break
+ }
+ self.debug('clearStateChar %j %j', stateChar, re)
+ stateChar = false
+ }
+ }
+
+ for (var i = 0, len = pattern.length, c
+ ; (i < len) && (c = pattern.charAt(i))
+ ; i++) {
+ this.debug('%s\t%s %s %j', pattern, i, re, c)
+
+ // skip over any that are escaped.
+ if (escaping && reSpecials[c]) {
+ re += '\\' + c
+ escaping = false
+ continue
+ }
+
+ switch (c) {
+ case '/':
+ // completely not allowed, even escaped.
+ // Should already be path-split by now.
+ return false
+
+ case '\\':
+ clearStateChar()
+ escaping = true
+ continue
+
+ // the various stateChar values
+ // for the "extglob" stuff.
+ case '?':
+ case '*':
+ case '+':
+ case '@':
+ case '!':
+ this.debug('%s\t%s %s %j <-- stateChar', pattern, i, re, c)
+
+ // all of those are literals inside a class, except that
+ // the glob [!a] means [^a] in regexp
+ if (inClass) {
+ this.debug(' in class')
+ if (c === '!' && i === classStart + 1) c = '^'
+ re += c
+ continue
+ }
+
+ // if we already have a stateChar, then it means
+ // that there was something like ** or +? in there.
+ // Handle the stateChar, then proceed with this one.
+ self.debug('call clearStateChar %j', stateChar)
+ clearStateChar()
+ stateChar = c
+ // if extglob is disabled, then +(asdf|foo) isn't a thing.
+ // just clear the statechar *now*, rather than even diving into
+ // the patternList stuff.
+ if (options.noext) clearStateChar()
+ continue
+
+ case '(':
+ if (inClass) {
+ re += '('
+ continue
+ }
+
+ if (!stateChar) {
+ re += '\\('
+ continue
+ }
+
+ plType = stateChar
+ patternListStack.push({
+ type: plType,
+ start: i - 1,
+ reStart: re.length
+ })
+ // negation is (?:(?!js)[^/]*)
+ re += stateChar === '!' ? '(?:(?!(?:' : '(?:'
+ this.debug('plType %j %j', stateChar, re)
+ stateChar = false
+ continue
+
+ case ')':
+ if (inClass || !patternListStack.length) {
+ re += '\\)'
+ continue
+ }
+
+ clearStateChar()
+ hasMagic = true
+ re += ')'
+ var pl = patternListStack.pop()
+ plType = pl.type
+ // negation is (?:(?!js)[^/]*)
+ // The others are (?:)
+ switch (plType) {
+ case '!':
+ negativeLists.push(pl)
+ re += ')[^/]*?)'
+ pl.reEnd = re.length
+ break
+ case '?':
+ case '+':
+ case '*':
+ re += plType
+ break
+ case '@': break // the default anyway
+ }
+ continue
+
+ case '|':
+ if (inClass || !patternListStack.length || escaping) {
+ re += '\\|'
+ escaping = false
+ continue
+ }
+
+ clearStateChar()
+ re += '|'
+ continue
+
+ // these are mostly the same in regexp and glob
+ case '[':
+ // swallow any state-tracking char before the [
+ clearStateChar()
+
+ if (inClass) {
+ re += '\\' + c
+ continue
+ }
+
+ inClass = true
+ classStart = i
+ reClassStart = re.length
+ re += c
+ continue
+
+ case ']':
+ // a right bracket shall lose its special
+ // meaning and represent itself in
+ // a bracket expression if it occurs
+ // first in the list. -- POSIX.2 2.8.3.2
+ if (i === classStart + 1 || !inClass) {
+ re += '\\' + c
+ escaping = false
+ continue
+ }
+
+ // handle the case where we left a class open.
+ // "[z-a]" is valid, equivalent to "\[z-a\]"
+ if (inClass) {
+ // split where the last [ was, make sure we don't have
+ // an invalid re. if so, re-walk the contents of the
+ // would-be class to re-translate any characters that
+ // were passed through as-is
+ // TODO: It would probably be faster to determine this
+ // without a try/catch and a new RegExp, but it's tricky
+ // to do safely. For now, this is safe and works.
+ var cs = pattern.substring(classStart + 1, i)
+ try {
+ RegExp('[' + cs + ']')
+ } catch (er) {
+ // not a valid class!
+ var sp = this.parse(cs, SUBPARSE)
+ re = re.substr(0, reClassStart) + '\\[' + sp[0] + '\\]'
+ hasMagic = hasMagic || sp[1]
+ inClass = false
+ continue
+ }
+ }
+
+ // finish up the class.
+ hasMagic = true
+ inClass = false
+ re += c
+ continue
+
+ default:
+ // swallow any state char that wasn't consumed
+ clearStateChar()
+
+ if (escaping) {
+ // no need
+ escaping = false
+ } else if (reSpecials[c]
+ && !(c === '^' && inClass)) {
+ re += '\\'
+ }
+
+ re += c
+
+ } // switch
+ } // for
+
+ // handle the case where we left a class open.
+ // "[abc" is valid, equivalent to "\[abc"
+ if (inClass) {
+ // split where the last [ was, and escape it
+ // this is a huge pita. We now have to re-walk
+ // the contents of the would-be class to re-translate
+ // any characters that were passed through as-is
+ cs = pattern.substr(classStart + 1)
+ sp = this.parse(cs, SUBPARSE)
+ re = re.substr(0, reClassStart) + '\\[' + sp[0]
+ hasMagic = hasMagic || sp[1]
+ }
+
+ // handle the case where we had a +( thing at the *end*
+ // of the pattern.
+ // each pattern list stack adds 3 chars, and we need to go through
+ // and escape any | chars that were passed through as-is for the regexp.
+ // Go through and escape them, taking care not to double-escape any
+ // | chars that were already escaped.
+ for (pl = patternListStack.pop(); pl; pl = patternListStack.pop()) {
+ var tail = re.slice(pl.reStart + 3)
+ // maybe some even number of \, then maybe 1 \, followed by a |
+ tail = tail.replace(/((?:\\{2})*)(\\?)\|/g, function (_, $1, $2) {
+ if (!$2) {
+ // the | isn't already escaped, so escape it.
+ $2 = '\\'
+ }
+
+ // need to escape all those slashes *again*, without escaping the
+ // one that we need for escaping the | character. As it works out,
+ // escaping an even number of slashes can be done by simply repeating
+ // it exactly after itself. That's why this trick works.
+ //
+ // I am sorry that you have to see this.
+ return $1 + $1 + $2 + '|'
+ })
+
+ this.debug('tail=%j\n %s', tail, tail)
+ var t = pl.type === '*' ? star
+ : pl.type === '?' ? qmark
+ : '\\' + pl.type
+
+ hasMagic = true
+ re = re.slice(0, pl.reStart) + t + '\\(' + tail
+ }
+
+ // handle trailing things that only matter at the very end.
+ clearStateChar()
+ if (escaping) {
+ // trailing \\
+ re += '\\\\'
+ }
+
+ // only need to apply the nodot start if the re starts with
+ // something that could conceivably capture a dot
+ var addPatternStart = false
+ switch (re.charAt(0)) {
+ case '.':
+ case '[':
+ case '(': addPatternStart = true
+ }
+
+ // Hack to work around lack of negative lookbehind in JS
+ // A pattern like: *.!(x).!(y|z) needs to ensure that a name
+ // like 'a.xyz.yz' doesn't match. So, the first negative
+ // lookahead, has to look ALL the way ahead, to the end of
+ // the pattern.
+ for (var n = negativeLists.length - 1; n > -1; n--) {
+ var nl = negativeLists[n]
+
+ var nlBefore = re.slice(0, nl.reStart)
+ var nlFirst = re.slice(nl.reStart, nl.reEnd - 8)
+ var nlLast = re.slice(nl.reEnd - 8, nl.reEnd)
+ var nlAfter = re.slice(nl.reEnd)
+
+ nlLast += nlAfter
+
+ // Handle nested stuff like *(*.js|!(*.json)), where open parens
+ // mean that we should *not* include the ) in the bit that is considered
+ // "after" the negated section.
+ var openParensBefore = nlBefore.split('(').length - 1
+ var cleanAfter = nlAfter
+ for (i = 0; i < openParensBefore; i++) {
+ cleanAfter = cleanAfter.replace(/\)[+*?]?/, '')
+ }
+ nlAfter = cleanAfter
+
+ var dollar = ''
+ if (nlAfter === '' && isSub !== SUBPARSE) {
+ dollar = '$'
+ }
+ var newRe = nlBefore + nlFirst + nlAfter + dollar + nlLast
+ re = newRe
+ }
+
+ // if the re is not "" at this point, then we need to make sure
+ // it doesn't match against an empty path part.
+ // Otherwise a/* will match a/, which it should not.
+ if (re !== '' && hasMagic) {
+ re = '(?=.)' + re
+ }
+
+ if (addPatternStart) {
+ re = patternStart + re
+ }
+
+ // parsing just a piece of a larger pattern.
+ if (isSub === SUBPARSE) {
+ return [re, hasMagic]
+ }
+
+ // skip the regexp for non-magical patterns
+ // unescape anything in it, though, so that it'll be
+ // an exact match against a file etc.
+ if (!hasMagic) {
+ return globUnescape(pattern)
+ }
+
+ var flags = options.nocase ? 'i' : ''
+ var regExp = new RegExp('^' + re + '$', flags)
+
+ regExp._glob = pattern
+ regExp._src = re
+
+ return regExp
+}
+
+minimatch.makeRe = function (pattern, options) {
+ return new Minimatch(pattern, options || {}).makeRe()
+}
+
+Minimatch.prototype.makeRe = makeRe
+function makeRe () {
+ if (this.regexp || this.regexp === false) return this.regexp
+
+ // at this point, this.set is a 2d array of partial
+ // pattern strings, or "**".
+ //
+ // It's better to use .match(). This function shouldn't
+ // be used, really, but it's pretty convenient sometimes,
+ // when you just want to work with a regex.
+ var set = this.set
+
+ if (!set.length) {
+ this.regexp = false
+ return this.regexp
+ }
+ var options = this.options
+
+ var twoStar = options.noglobstar ? star
+ : options.dot ? twoStarDot
+ : twoStarNoDot
+ var flags = options.nocase ? 'i' : ''
+
+ var re = set.map(function (pattern) {
+ return pattern.map(function (p) {
+ return (p === GLOBSTAR) ? twoStar
+ : (typeof p === 'string') ? regExpEscape(p)
+ : p._src
+ }).join('\\\/')
+ }).join('|')
+
+ // must match entire pattern
+ // ending in a * or ** will make it less strict.
+ re = '^(?:' + re + ')$'
+
+ // can match anything, as long as it's not this.
+ if (this.negate) re = '^(?!' + re + ').*$'
+
+ try {
+ this.regexp = new RegExp(re, flags)
+ } catch (ex) {
+ this.regexp = false
+ }
+ return this.regexp
+}
+
+minimatch.match = function (list, pattern, options) {
+ options = options || {}
+ var mm = new Minimatch(pattern, options)
+ list = list.filter(function (f) {
+ return mm.match(f)
+ })
+ if (mm.options.nonull && !list.length) {
+ list.push(pattern)
+ }
+ return list
+}
+
+Minimatch.prototype.match = match
+function match (f, partial) {
+ this.debug('match', f, this.pattern)
+ // short-circuit in the case of busted things.
+ // comments, etc.
+ if (this.comment) return false
+ if (this.empty) return f === ''
+
+ if (f === '/' && partial) return true
+
+ var options = this.options
+
+ // windows: need to use /, not \
+ if (path.sep !== '/') {
+ f = f.split(path.sep).join('/')
+ }
+
+ // treat the test path as a set of pathparts.
+ f = f.split(slashSplit)
+ this.debug(this.pattern, 'split', f)
+
+ // just ONE of the pattern sets in this.set needs to match
+ // in order for it to be valid. If negating, then just one
+ // match means that we have failed.
+ // Either way, return on the first hit.
+
+ var set = this.set
+ this.debug(this.pattern, 'set', set)
+
+ // Find the basename of the path by looking for the last non-empty segment
+ var filename
+ var i
+ for (i = f.length - 1; i >= 0; i--) {
+ filename = f[i]
+ if (filename) break
+ }
+
+ for (i = 0; i < set.length; i++) {
+ var pattern = set[i]
+ var file = f
+ if (options.matchBase && pattern.length === 1) {
+ file = [filename]
+ }
+ var hit = this.matchOne(file, pattern, partial)
+ if (hit) {
+ if (options.flipNegate) return true
+ return !this.negate
+ }
+ }
+
+ // didn't get any hits. this is success if it's a negative
+ // pattern, failure otherwise.
+ if (options.flipNegate) return false
+ return this.negate
+}
+
+// set partial to true to test if, for example,
+// "/a/b" matches the start of "/*/b/*/d"
+// Partial means, if you run out of file before you run
+// out of pattern, then that's fine, as long as all
+// the parts match.
+Minimatch.prototype.matchOne = function (file, pattern, partial) {
+ var options = this.options
+
+ this.debug('matchOne',
+ { 'this': this, file: file, pattern: pattern })
+
+ this.debug('matchOne', file.length, pattern.length)
+
+ for (var fi = 0,
+ pi = 0,
+ fl = file.length,
+ pl = pattern.length
+ ; (fi < fl) && (pi < pl)
+ ; fi++, pi++) {
+ this.debug('matchOne loop')
+ var p = pattern[pi]
+ var f = file[fi]
+
+ this.debug(pattern, p, f)
+
+ // should be impossible.
+ // some invalid regexp stuff in the set.
+ if (p === false) return false
+
+ if (p === GLOBSTAR) {
+ this.debug('GLOBSTAR', [pattern, p, f])
+
+ // "**"
+ // a/**/b/**/c would match the following:
+ // a/b/x/y/z/c
+ // a/x/y/z/b/c
+ // a/b/x/b/x/c
+ // a/b/c
+ // To do this, take the rest of the pattern after
+ // the **, and see if it would match the file remainder.
+ // If so, return success.
+ // If not, the ** "swallows" a segment, and try again.
+ // This is recursively awful.
+ //
+ // a/**/b/**/c matching a/b/x/y/z/c
+ // - a matches a
+ // - doublestar
+ // - matchOne(b/x/y/z/c, b/**/c)
+ // - b matches b
+ // - doublestar
+ // - matchOne(x/y/z/c, c) -> no
+ // - matchOne(y/z/c, c) -> no
+ // - matchOne(z/c, c) -> no
+ // - matchOne(c, c) yes, hit
+ var fr = fi
+ var pr = pi + 1
+ if (pr === pl) {
+ this.debug('** at the end')
+ // a ** at the end will just swallow the rest.
+ // We have found a match.
+ // however, it will not swallow /.x, unless
+ // options.dot is set.
+ // . and .. are *never* matched by **, for explosively
+ // exponential reasons.
+ for (; fi < fl; fi++) {
+ if (file[fi] === '.' || file[fi] === '..' ||
+ (!options.dot && file[fi].charAt(0) === '.')) return false
+ }
+ return true
+ }
+
+ // ok, let's see if we can swallow whatever we can.
+ while (fr < fl) {
+ var swallowee = file[fr]
+
+ this.debug('\nglobstar while', file, fr, pattern, pr, swallowee)
+
+ // XXX remove this slice. Just pass the start index.
+ if (this.matchOne(file.slice(fr), pattern.slice(pr), partial)) {
+ this.debug('globstar found match!', fr, fl, swallowee)
+ // found a match.
+ return true
+ } else {
+ // can't swallow "." or ".." ever.
+ // can only swallow ".foo" when explicitly asked.
+ if (swallowee === '.' || swallowee === '..' ||
+ (!options.dot && swallowee.charAt(0) === '.')) {
+ this.debug('dot detected!', file, fr, pattern, pr)
+ break
+ }
+
+ // ** swallows a segment, and continue.
+ this.debug('globstar swallow a segment, and continue')
+ fr++
+ }
+ }
+
+ // no match was found.
+ // However, in partial mode, we can't say this is necessarily over.
+ // If there's more *pattern* left, then
+ if (partial) {
+ // ran out of file
+ this.debug('\n>>> no match, partial?', file, fr, pattern, pr)
+ if (fr === fl) return true
+ }
+ return false
+ }
+
+ // something other than **
+ // non-magic patterns just have to match exactly
+ // patterns with magic have been turned into regexps.
+ var hit
+ if (typeof p === 'string') {
+ if (options.nocase) {
+ hit = f.toLowerCase() === p.toLowerCase()
+ } else {
+ hit = f === p
+ }
+ this.debug('string match', p, f, hit)
+ } else {
+ hit = f.match(p)
+ this.debug('pattern match', p, f, hit)
+ }
+
+ if (!hit) return false
+ }
+
+ // Note: ending in / means that we'll get a final ""
+ // at the end of the pattern. This can only match a
+ // corresponding "" at the end of the file.
+ // If the file ends in /, then it can only match a
+ // a pattern that ends in /, unless the pattern just
+ // doesn't have any more for it. But, a/b/ should *not*
+ // match "a/b/*", even though "" matches against the
+ // [^/]*? pattern, except in partial mode, where it might
+ // simply not be reached yet.
+ // However, a/b/ should still satisfy a/*
+
+ // now either we fell off the end of the pattern, or we're done.
+ if (fi === fl && pi === pl) {
+ // ran out of pattern and filename at the same time.
+ // an exact hit!
+ return true
+ } else if (fi === fl) {
+ // ran out of file, but still had pattern left.
+ // this is ok if we're doing the match as part of
+ // a glob fs traversal.
+ return partial
+ } else if (pi === pl) {
+ // ran out of pattern, still have file left.
+ // this is only acceptable if we're on the very last
+ // empty segment of a file with a trailing slash.
+ // a/* should match a/b/
+ var emptyFileEnd = (fi === fl - 1) && (file[fi] === '')
+ return emptyFileEnd
+ }
+
+ // should be unreachable.
+ throw new Error('wtf?')
+}
+
+// replace stuff like \* with *
+function globUnescape (s) {
+ return s.replace(/\\(.)/g, '$1')
+}
+
+function regExpEscape (s) {
+ return s.replace(/[-[\]{}()*+?.,\\^$|#\s]/g, '\\$&')
+}
+
+},{"brace-expansion":2,"path":undefined}],2:[function(require,module,exports){
+var concatMap = require('concat-map');
+var balanced = require('balanced-match');
+
+module.exports = expandTop;
+
+var escSlash = '\0SLASH'+Math.random()+'\0';
+var escOpen = '\0OPEN'+Math.random()+'\0';
+var escClose = '\0CLOSE'+Math.random()+'\0';
+var escComma = '\0COMMA'+Math.random()+'\0';
+var escPeriod = '\0PERIOD'+Math.random()+'\0';
+
+function numeric(str) {
+ return parseInt(str, 10) == str
+ ? parseInt(str, 10)
+ : str.charCodeAt(0);
+}
+
+function escapeBraces(str) {
+ return str.split('\\\\').join(escSlash)
+ .split('\\{').join(escOpen)
+ .split('\\}').join(escClose)
+ .split('\\,').join(escComma)
+ .split('\\.').join(escPeriod);
+}
+
+function unescapeBraces(str) {
+ return str.split(escSlash).join('\\')
+ .split(escOpen).join('{')
+ .split(escClose).join('}')
+ .split(escComma).join(',')
+ .split(escPeriod).join('.');
+}
+
+
+// Basically just str.split(","), but handling cases
+// where we have nested braced sections, which should be
+// treated as individual members, like {a,{b,c},d}
+function parseCommaParts(str) {
+ if (!str)
+ return [''];
+
+ var parts = [];
+ var m = balanced('{', '}', str);
+
+ if (!m)
+ return str.split(',');
+
+ var pre = m.pre;
+ var body = m.body;
+ var post = m.post;
+ var p = pre.split(',');
+
+ p[p.length-1] += '{' + body + '}';
+ var postParts = parseCommaParts(post);
+ if (post.length) {
+ p[p.length-1] += postParts.shift();
+ p.push.apply(p, postParts);
+ }
+
+ parts.push.apply(parts, p);
+
+ return parts;
+}
+
+function expandTop(str) {
+ if (!str)
+ return [];
+
+ var expansions = expand(escapeBraces(str));
+ return expansions.filter(identity).map(unescapeBraces);
+}
+
+function identity(e) {
+ return e;
+}
+
+function embrace(str) {
+ return '{' + str + '}';
+}
+function isPadded(el) {
+ return /^-?0\d/.test(el);
+}
+
+function lte(i, y) {
+ return i <= y;
+}
+function gte(i, y) {
+ return i >= y;
+}
+
+function expand(str) {
+ var expansions = [];
+
+ var m = balanced('{', '}', str);
+ if (!m || /\$$/.test(m.pre)) return [str];
+
+ var isNumericSequence = /^-?\d+\.\.-?\d+(?:\.\.-?\d+)?$/.test(m.body);
+ var isAlphaSequence = /^[a-zA-Z]\.\.[a-zA-Z](?:\.\.-?\d+)?$/.test(m.body);
+ var isSequence = isNumericSequence || isAlphaSequence;
+ var isOptions = /^(.*,)+(.+)?$/.test(m.body);
+ if (!isSequence && !isOptions) {
+ // {a},b}
+ if (m.post.match(/,.*}/)) {
+ str = m.pre + '{' + m.body + escClose + m.post;
+ return expand(str);
+ }
+ return [str];
+ }
+
+ var n;
+ if (isSequence) {
+ n = m.body.split(/\.\./);
+ } else {
+ n = parseCommaParts(m.body);
+ if (n.length === 1) {
+ // x{{a,b}}y ==> x{a}y x{b}y
+ n = expand(n[0]).map(embrace);
+ if (n.length === 1) {
+ var post = m.post.length
+ ? expand(m.post)
+ : [''];
+ return post.map(function(p) {
+ return m.pre + n[0] + p;
+ });
+ }
+ }
+ }
+
+ // at this point, n is the parts, and we know it's not a comma set
+ // with a single entry.
+
+ // no need to expand pre, since it is guaranteed to be free of brace-sets
+ var pre = m.pre;
+ var post = m.post.length
+ ? expand(m.post)
+ : [''];
+
+ var N;
+
+ if (isSequence) {
+ var x = numeric(n[0]);
+ var y = numeric(n[1]);
+ var width = Math.max(n[0].length, n[1].length)
+ var incr = n.length == 3
+ ? Math.abs(numeric(n[2]))
+ : 1;
+ var test = lte;
+ var reverse = y < x;
+ if (reverse) {
+ incr *= -1;
+ test = gte;
+ }
+ var pad = n.some(isPadded);
+
+ N = [];
+
+ for (var i = x; test(i, y); i += incr) {
+ var c;
+ if (isAlphaSequence) {
+ c = String.fromCharCode(i);
+ if (c === '\\')
+ c = '';
+ } else {
+ c = String(i);
+ if (pad) {
+ var need = width - c.length;
+ if (need > 0) {
+ var z = new Array(need + 1).join('0');
+ if (i < 0)
+ c = '-' + z + c.slice(1);
+ else
+ c = z + c;
+ }
+ }
+ }
+ N.push(c);
+ }
+ } else {
+ N = concatMap(n, function(el) { return expand(el) });
+ }
+
+ for (var j = 0; j < N.length; j++) {
+ for (var k = 0; k < post.length; k++) {
+ expansions.push([pre, N[j], post[k]].join(''))
+ }
+ }
+
+ return expansions;
+}
+
+
+},{"balanced-match":3,"concat-map":4}],3:[function(require,module,exports){
+module.exports = balanced;
+function balanced(a, b, str) {
+ var bal = 0;
+ var m = {};
+ var ended = false;
+
+ for (var i = 0; i < str.length; i++) {
+ if (a == str.substr(i, a.length)) {
+ if (!('start' in m)) m.start = i;
+ bal++;
+ }
+ else if (b == str.substr(i, b.length) && 'start' in m) {
+ ended = true;
+ bal--;
+ if (!bal) {
+ m.end = i;
+ m.pre = str.substr(0, m.start);
+ m.body = (m.end - m.start > 1)
+ ? str.substring(m.start + a.length, m.end)
+ : '';
+ m.post = str.slice(m.end + b.length);
+ return m;
+ }
+ }
+ }
+
+ // if we opened more than we closed, find the one we closed
+ if (bal && ended) {
+ var start = m.start + a.length;
+ m = balanced(a, b, str.substr(start));
+ if (m) {
+ m.start += start;
+ m.end += start;
+ m.pre = str.slice(0, start) + m.pre;
+ }
+ return m;
+ }
+}
+
+},{}],4:[function(require,module,exports){
+module.exports = function (xs, fn) {
+ var res = [];
+ for (var i = 0; i < xs.length; i++) {
+ var x = fn(xs[i], i);
+ if (Array.isArray(x)) res.push.apply(res, x);
+ else res.push(x);
+ }
+ return res;
+};
+
+},{}]},{},[1])(1)
+});
\ No newline at end of file
diff --git a/node_modules/minimatch/minimatch.js b/node_modules/minimatch/minimatch.js
new file mode 100644
index 0000000..ec4c05c
--- /dev/null
+++ b/node_modules/minimatch/minimatch.js
@@ -0,0 +1,912 @@
+module.exports = minimatch
+minimatch.Minimatch = Minimatch
+
+var path = { sep: '/' }
+try {
+ path = require('path')
+} catch (er) {}
+
+var GLOBSTAR = minimatch.GLOBSTAR = Minimatch.GLOBSTAR = {}
+var expand = require('brace-expansion')
+
+// any single thing other than /
+// don't need to escape / when using new RegExp()
+var qmark = '[^/]'
+
+// * => any number of characters
+var star = qmark + '*?'
+
+// ** when dots are allowed. Anything goes, except .. and .
+// not (^ or / followed by one or two dots followed by $ or /),
+// followed by anything, any number of times.
+var twoStarDot = '(?:(?!(?:\\\/|^)(?:\\.{1,2})($|\\\/)).)*?'
+
+// not a ^ or / followed by a dot,
+// followed by anything, any number of times.
+var twoStarNoDot = '(?:(?!(?:\\\/|^)\\.).)*?'
+
+// characters that need to be escaped in RegExp.
+var reSpecials = charSet('().*{}+?[]^$\\!')
+
+// "abc" -> { a:true, b:true, c:true }
+function charSet (s) {
+ return s.split('').reduce(function (set, c) {
+ set[c] = true
+ return set
+ }, {})
+}
+
+// normalizes slashes.
+var slashSplit = /\/+/
+
+minimatch.filter = filter
+function filter (pattern, options) {
+ options = options || {}
+ return function (p, i, list) {
+ return minimatch(p, pattern, options)
+ }
+}
+
+function ext (a, b) {
+ a = a || {}
+ b = b || {}
+ var t = {}
+ Object.keys(b).forEach(function (k) {
+ t[k] = b[k]
+ })
+ Object.keys(a).forEach(function (k) {
+ t[k] = a[k]
+ })
+ return t
+}
+
+minimatch.defaults = function (def) {
+ if (!def || !Object.keys(def).length) return minimatch
+
+ var orig = minimatch
+
+ var m = function minimatch (p, pattern, options) {
+ return orig.minimatch(p, pattern, ext(def, options))
+ }
+
+ m.Minimatch = function Minimatch (pattern, options) {
+ return new orig.Minimatch(pattern, ext(def, options))
+ }
+
+ return m
+}
+
+Minimatch.defaults = function (def) {
+ if (!def || !Object.keys(def).length) return Minimatch
+ return minimatch.defaults(def).Minimatch
+}
+
+function minimatch (p, pattern, options) {
+ if (typeof pattern !== 'string') {
+ throw new TypeError('glob pattern string required')
+ }
+
+ if (!options) options = {}
+
+ // shortcut: comments match nothing.
+ if (!options.nocomment && pattern.charAt(0) === '#') {
+ return false
+ }
+
+ // "" only matches ""
+ if (pattern.trim() === '') return p === ''
+
+ return new Minimatch(pattern, options).match(p)
+}
+
+function Minimatch (pattern, options) {
+ if (!(this instanceof Minimatch)) {
+ return new Minimatch(pattern, options)
+ }
+
+ if (typeof pattern !== 'string') {
+ throw new TypeError('glob pattern string required')
+ }
+
+ if (!options) options = {}
+ pattern = pattern.trim()
+
+ // windows support: need to use /, not \
+ if (path.sep !== '/') {
+ pattern = pattern.split(path.sep).join('/')
+ }
+
+ this.options = options
+ this.set = []
+ this.pattern = pattern
+ this.regexp = null
+ this.negate = false
+ this.comment = false
+ this.empty = false
+
+ // make the set of regexps etc.
+ this.make()
+}
+
+Minimatch.prototype.debug = function () {}
+
+Minimatch.prototype.make = make
+function make () {
+ // don't do it more than once.
+ if (this._made) return
+
+ var pattern = this.pattern
+ var options = this.options
+
+ // empty patterns and comments match nothing.
+ if (!options.nocomment && pattern.charAt(0) === '#') {
+ this.comment = true
+ return
+ }
+ if (!pattern) {
+ this.empty = true
+ return
+ }
+
+ // step 1: figure out negation, etc.
+ this.parseNegate()
+
+ // step 2: expand braces
+ var set = this.globSet = this.braceExpand()
+
+ if (options.debug) this.debug = console.error
+
+ this.debug(this.pattern, set)
+
+ // step 3: now we have a set, so turn each one into a series of path-portion
+ // matching patterns.
+ // These will be regexps, except in the case of "**", which is
+ // set to the GLOBSTAR object for globstar behavior,
+ // and will not contain any / characters
+ set = this.globParts = set.map(function (s) {
+ return s.split(slashSplit)
+ })
+
+ this.debug(this.pattern, set)
+
+ // glob --> regexps
+ set = set.map(function (s, si, set) {
+ return s.map(this.parse, this)
+ }, this)
+
+ this.debug(this.pattern, set)
+
+ // filter out everything that didn't compile properly.
+ set = set.filter(function (s) {
+ return s.indexOf(false) === -1
+ })
+
+ this.debug(this.pattern, set)
+
+ this.set = set
+}
+
+Minimatch.prototype.parseNegate = parseNegate
+function parseNegate () {
+ var pattern = this.pattern
+ var negate = false
+ var options = this.options
+ var negateOffset = 0
+
+ if (options.nonegate) return
+
+ for (var i = 0, l = pattern.length
+ ; i < l && pattern.charAt(i) === '!'
+ ; i++) {
+ negate = !negate
+ negateOffset++
+ }
+
+ if (negateOffset) this.pattern = pattern.substr(negateOffset)
+ this.negate = negate
+}
+
+// Brace expansion:
+// a{b,c}d -> abd acd
+// a{b,}c -> abc ac
+// a{0..3}d -> a0d a1d a2d a3d
+// a{b,c{d,e}f}g -> abg acdfg acefg
+// a{b,c}d{e,f}g -> abdeg acdeg abdeg abdfg
+//
+// Invalid sets are not expanded.
+// a{2..}b -> a{2..}b
+// a{b}c -> a{b}c
+minimatch.braceExpand = function (pattern, options) {
+ return braceExpand(pattern, options)
+}
+
+Minimatch.prototype.braceExpand = braceExpand
+
+function braceExpand (pattern, options) {
+ if (!options) {
+ if (this instanceof Minimatch) {
+ options = this.options
+ } else {
+ options = {}
+ }
+ }
+
+ pattern = typeof pattern === 'undefined'
+ ? this.pattern : pattern
+
+ if (typeof pattern === 'undefined') {
+ throw new Error('undefined pattern')
+ }
+
+ if (options.nobrace ||
+ !pattern.match(/\{.*\}/)) {
+ // shortcut. no need to expand.
+ return [pattern]
+ }
+
+ return expand(pattern)
+}
+
+// parse a component of the expanded set.
+// At this point, no pattern may contain "/" in it
+// so we're going to return a 2d array, where each entry is the full
+// pattern, split on '/', and then turned into a regular expression.
+// A regexp is made at the end which joins each array with an
+// escaped /, and another full one which joins each regexp with |.
+//
+// Following the lead of Bash 4.1, note that "**" only has special meaning
+// when it is the *only* thing in a path portion. Otherwise, any series
+// of * is equivalent to a single *. Globstar behavior is enabled by
+// default, and can be disabled by setting options.noglobstar.
+Minimatch.prototype.parse = parse
+var SUBPARSE = {}
+function parse (pattern, isSub) {
+ var options = this.options
+
+ // shortcuts
+ if (!options.noglobstar && pattern === '**') return GLOBSTAR
+ if (pattern === '') return ''
+
+ var re = ''
+ var hasMagic = !!options.nocase
+ var escaping = false
+ // ? => one single character
+ var patternListStack = []
+ var negativeLists = []
+ var plType
+ var stateChar
+ var inClass = false
+ var reClassStart = -1
+ var classStart = -1
+ // . and .. never match anything that doesn't start with .,
+ // even when options.dot is set.
+ var patternStart = pattern.charAt(0) === '.' ? '' // anything
+ // not (start or / followed by . or .. followed by / or end)
+ : options.dot ? '(?!(?:^|\\\/)\\.{1,2}(?:$|\\\/))'
+ : '(?!\\.)'
+ var self = this
+
+ function clearStateChar () {
+ if (stateChar) {
+ // we had some state-tracking character
+ // that wasn't consumed by this pass.
+ switch (stateChar) {
+ case '*':
+ re += star
+ hasMagic = true
+ break
+ case '?':
+ re += qmark
+ hasMagic = true
+ break
+ default:
+ re += '\\' + stateChar
+ break
+ }
+ self.debug('clearStateChar %j %j', stateChar, re)
+ stateChar = false
+ }
+ }
+
+ for (var i = 0, len = pattern.length, c
+ ; (i < len) && (c = pattern.charAt(i))
+ ; i++) {
+ this.debug('%s\t%s %s %j', pattern, i, re, c)
+
+ // skip over any that are escaped.
+ if (escaping && reSpecials[c]) {
+ re += '\\' + c
+ escaping = false
+ continue
+ }
+
+ switch (c) {
+ case '/':
+ // completely not allowed, even escaped.
+ // Should already be path-split by now.
+ return false
+
+ case '\\':
+ clearStateChar()
+ escaping = true
+ continue
+
+ // the various stateChar values
+ // for the "extglob" stuff.
+ case '?':
+ case '*':
+ case '+':
+ case '@':
+ case '!':
+ this.debug('%s\t%s %s %j <-- stateChar', pattern, i, re, c)
+
+ // all of those are literals inside a class, except that
+ // the glob [!a] means [^a] in regexp
+ if (inClass) {
+ this.debug(' in class')
+ if (c === '!' && i === classStart + 1) c = '^'
+ re += c
+ continue
+ }
+
+ // if we already have a stateChar, then it means
+ // that there was something like ** or +? in there.
+ // Handle the stateChar, then proceed with this one.
+ self.debug('call clearStateChar %j', stateChar)
+ clearStateChar()
+ stateChar = c
+ // if extglob is disabled, then +(asdf|foo) isn't a thing.
+ // just clear the statechar *now*, rather than even diving into
+ // the patternList stuff.
+ if (options.noext) clearStateChar()
+ continue
+
+ case '(':
+ if (inClass) {
+ re += '('
+ continue
+ }
+
+ if (!stateChar) {
+ re += '\\('
+ continue
+ }
+
+ plType = stateChar
+ patternListStack.push({
+ type: plType,
+ start: i - 1,
+ reStart: re.length
+ })
+ // negation is (?:(?!js)[^/]*)
+ re += stateChar === '!' ? '(?:(?!(?:' : '(?:'
+ this.debug('plType %j %j', stateChar, re)
+ stateChar = false
+ continue
+
+ case ')':
+ if (inClass || !patternListStack.length) {
+ re += '\\)'
+ continue
+ }
+
+ clearStateChar()
+ hasMagic = true
+ re += ')'
+ var pl = patternListStack.pop()
+ plType = pl.type
+ // negation is (?:(?!js)[^/]*)
+ // The others are (?:)
+ switch (plType) {
+ case '!':
+ negativeLists.push(pl)
+ re += ')[^/]*?)'
+ pl.reEnd = re.length
+ break
+ case '?':
+ case '+':
+ case '*':
+ re += plType
+ break
+ case '@': break // the default anyway
+ }
+ continue
+
+ case '|':
+ if (inClass || !patternListStack.length || escaping) {
+ re += '\\|'
+ escaping = false
+ continue
+ }
+
+ clearStateChar()
+ re += '|'
+ continue
+
+ // these are mostly the same in regexp and glob
+ case '[':
+ // swallow any state-tracking char before the [
+ clearStateChar()
+
+ if (inClass) {
+ re += '\\' + c
+ continue
+ }
+
+ inClass = true
+ classStart = i
+ reClassStart = re.length
+ re += c
+ continue
+
+ case ']':
+ // a right bracket shall lose its special
+ // meaning and represent itself in
+ // a bracket expression if it occurs
+ // first in the list. -- POSIX.2 2.8.3.2
+ if (i === classStart + 1 || !inClass) {
+ re += '\\' + c
+ escaping = false
+ continue
+ }
+
+ // handle the case where we left a class open.
+ // "[z-a]" is valid, equivalent to "\[z-a\]"
+ if (inClass) {
+ // split where the last [ was, make sure we don't have
+ // an invalid re. if so, re-walk the contents of the
+ // would-be class to re-translate any characters that
+ // were passed through as-is
+ // TODO: It would probably be faster to determine this
+ // without a try/catch and a new RegExp, but it's tricky
+ // to do safely. For now, this is safe and works.
+ var cs = pattern.substring(classStart + 1, i)
+ try {
+ RegExp('[' + cs + ']')
+ } catch (er) {
+ // not a valid class!
+ var sp = this.parse(cs, SUBPARSE)
+ re = re.substr(0, reClassStart) + '\\[' + sp[0] + '\\]'
+ hasMagic = hasMagic || sp[1]
+ inClass = false
+ continue
+ }
+ }
+
+ // finish up the class.
+ hasMagic = true
+ inClass = false
+ re += c
+ continue
+
+ default:
+ // swallow any state char that wasn't consumed
+ clearStateChar()
+
+ if (escaping) {
+ // no need
+ escaping = false
+ } else if (reSpecials[c]
+ && !(c === '^' && inClass)) {
+ re += '\\'
+ }
+
+ re += c
+
+ } // switch
+ } // for
+
+ // handle the case where we left a class open.
+ // "[abc" is valid, equivalent to "\[abc"
+ if (inClass) {
+ // split where the last [ was, and escape it
+ // this is a huge pita. We now have to re-walk
+ // the contents of the would-be class to re-translate
+ // any characters that were passed through as-is
+ cs = pattern.substr(classStart + 1)
+ sp = this.parse(cs, SUBPARSE)
+ re = re.substr(0, reClassStart) + '\\[' + sp[0]
+ hasMagic = hasMagic || sp[1]
+ }
+
+ // handle the case where we had a +( thing at the *end*
+ // of the pattern.
+ // each pattern list stack adds 3 chars, and we need to go through
+ // and escape any | chars that were passed through as-is for the regexp.
+ // Go through and escape them, taking care not to double-escape any
+ // | chars that were already escaped.
+ for (pl = patternListStack.pop(); pl; pl = patternListStack.pop()) {
+ var tail = re.slice(pl.reStart + 3)
+ // maybe some even number of \, then maybe 1 \, followed by a |
+ tail = tail.replace(/((?:\\{2})*)(\\?)\|/g, function (_, $1, $2) {
+ if (!$2) {
+ // the | isn't already escaped, so escape it.
+ $2 = '\\'
+ }
+
+ // need to escape all those slashes *again*, without escaping the
+ // one that we need for escaping the | character. As it works out,
+ // escaping an even number of slashes can be done by simply repeating
+ // it exactly after itself. That's why this trick works.
+ //
+ // I am sorry that you have to see this.
+ return $1 + $1 + $2 + '|'
+ })
+
+ this.debug('tail=%j\n %s', tail, tail)
+ var t = pl.type === '*' ? star
+ : pl.type === '?' ? qmark
+ : '\\' + pl.type
+
+ hasMagic = true
+ re = re.slice(0, pl.reStart) + t + '\\(' + tail
+ }
+
+ // handle trailing things that only matter at the very end.
+ clearStateChar()
+ if (escaping) {
+ // trailing \\
+ re += '\\\\'
+ }
+
+ // only need to apply the nodot start if the re starts with
+ // something that could conceivably capture a dot
+ var addPatternStart = false
+ switch (re.charAt(0)) {
+ case '.':
+ case '[':
+ case '(': addPatternStart = true
+ }
+
+ // Hack to work around lack of negative lookbehind in JS
+ // A pattern like: *.!(x).!(y|z) needs to ensure that a name
+ // like 'a.xyz.yz' doesn't match. So, the first negative
+ // lookahead, has to look ALL the way ahead, to the end of
+ // the pattern.
+ for (var n = negativeLists.length - 1; n > -1; n--) {
+ var nl = negativeLists[n]
+
+ var nlBefore = re.slice(0, nl.reStart)
+ var nlFirst = re.slice(nl.reStart, nl.reEnd - 8)
+ var nlLast = re.slice(nl.reEnd - 8, nl.reEnd)
+ var nlAfter = re.slice(nl.reEnd)
+
+ nlLast += nlAfter
+
+ // Handle nested stuff like *(*.js|!(*.json)), where open parens
+ // mean that we should *not* include the ) in the bit that is considered
+ // "after" the negated section.
+ var openParensBefore = nlBefore.split('(').length - 1
+ var cleanAfter = nlAfter
+ for (i = 0; i < openParensBefore; i++) {
+ cleanAfter = cleanAfter.replace(/\)[+*?]?/, '')
+ }
+ nlAfter = cleanAfter
+
+ var dollar = ''
+ if (nlAfter === '' && isSub !== SUBPARSE) {
+ dollar = '$'
+ }
+ var newRe = nlBefore + nlFirst + nlAfter + dollar + nlLast
+ re = newRe
+ }
+
+ // if the re is not "" at this point, then we need to make sure
+ // it doesn't match against an empty path part.
+ // Otherwise a/* will match a/, which it should not.
+ if (re !== '' && hasMagic) {
+ re = '(?=.)' + re
+ }
+
+ if (addPatternStart) {
+ re = patternStart + re
+ }
+
+ // parsing just a piece of a larger pattern.
+ if (isSub === SUBPARSE) {
+ return [re, hasMagic]
+ }
+
+ // skip the regexp for non-magical patterns
+ // unescape anything in it, though, so that it'll be
+ // an exact match against a file etc.
+ if (!hasMagic) {
+ return globUnescape(pattern)
+ }
+
+ var flags = options.nocase ? 'i' : ''
+ var regExp = new RegExp('^' + re + '$', flags)
+
+ regExp._glob = pattern
+ regExp._src = re
+
+ return regExp
+}
+
+minimatch.makeRe = function (pattern, options) {
+ return new Minimatch(pattern, options || {}).makeRe()
+}
+
+Minimatch.prototype.makeRe = makeRe
+function makeRe () {
+ if (this.regexp || this.regexp === false) return this.regexp
+
+ // at this point, this.set is a 2d array of partial
+ // pattern strings, or "**".
+ //
+ // It's better to use .match(). This function shouldn't
+ // be used, really, but it's pretty convenient sometimes,
+ // when you just want to work with a regex.
+ var set = this.set
+
+ if (!set.length) {
+ this.regexp = false
+ return this.regexp
+ }
+ var options = this.options
+
+ var twoStar = options.noglobstar ? star
+ : options.dot ? twoStarDot
+ : twoStarNoDot
+ var flags = options.nocase ? 'i' : ''
+
+ var re = set.map(function (pattern) {
+ return pattern.map(function (p) {
+ return (p === GLOBSTAR) ? twoStar
+ : (typeof p === 'string') ? regExpEscape(p)
+ : p._src
+ }).join('\\\/')
+ }).join('|')
+
+ // must match entire pattern
+ // ending in a * or ** will make it less strict.
+ re = '^(?:' + re + ')$'
+
+ // can match anything, as long as it's not this.
+ if (this.negate) re = '^(?!' + re + ').*$'
+
+ try {
+ this.regexp = new RegExp(re, flags)
+ } catch (ex) {
+ this.regexp = false
+ }
+ return this.regexp
+}
+
+minimatch.match = function (list, pattern, options) {
+ options = options || {}
+ var mm = new Minimatch(pattern, options)
+ list = list.filter(function (f) {
+ return mm.match(f)
+ })
+ if (mm.options.nonull && !list.length) {
+ list.push(pattern)
+ }
+ return list
+}
+
+Minimatch.prototype.match = match
+function match (f, partial) {
+ this.debug('match', f, this.pattern)
+ // short-circuit in the case of busted things.
+ // comments, etc.
+ if (this.comment) return false
+ if (this.empty) return f === ''
+
+ if (f === '/' && partial) return true
+
+ var options = this.options
+
+ // windows: need to use /, not \
+ if (path.sep !== '/') {
+ f = f.split(path.sep).join('/')
+ }
+
+ // treat the test path as a set of pathparts.
+ f = f.split(slashSplit)
+ this.debug(this.pattern, 'split', f)
+
+ // just ONE of the pattern sets in this.set needs to match
+ // in order for it to be valid. If negating, then just one
+ // match means that we have failed.
+ // Either way, return on the first hit.
+
+ var set = this.set
+ this.debug(this.pattern, 'set', set)
+
+ // Find the basename of the path by looking for the last non-empty segment
+ var filename
+ var i
+ for (i = f.length - 1; i >= 0; i--) {
+ filename = f[i]
+ if (filename) break
+ }
+
+ for (i = 0; i < set.length; i++) {
+ var pattern = set[i]
+ var file = f
+ if (options.matchBase && pattern.length === 1) {
+ file = [filename]
+ }
+ var hit = this.matchOne(file, pattern, partial)
+ if (hit) {
+ if (options.flipNegate) return true
+ return !this.negate
+ }
+ }
+
+ // didn't get any hits. this is success if it's a negative
+ // pattern, failure otherwise.
+ if (options.flipNegate) return false
+ return this.negate
+}
+
+// set partial to true to test if, for example,
+// "/a/b" matches the start of "/*/b/*/d"
+// Partial means, if you run out of file before you run
+// out of pattern, then that's fine, as long as all
+// the parts match.
+Minimatch.prototype.matchOne = function (file, pattern, partial) {
+ var options = this.options
+
+ this.debug('matchOne',
+ { 'this': this, file: file, pattern: pattern })
+
+ this.debug('matchOne', file.length, pattern.length)
+
+ for (var fi = 0,
+ pi = 0,
+ fl = file.length,
+ pl = pattern.length
+ ; (fi < fl) && (pi < pl)
+ ; fi++, pi++) {
+ this.debug('matchOne loop')
+ var p = pattern[pi]
+ var f = file[fi]
+
+ this.debug(pattern, p, f)
+
+ // should be impossible.
+ // some invalid regexp stuff in the set.
+ if (p === false) return false
+
+ if (p === GLOBSTAR) {
+ this.debug('GLOBSTAR', [pattern, p, f])
+
+ // "**"
+ // a/**/b/**/c would match the following:
+ // a/b/x/y/z/c
+ // a/x/y/z/b/c
+ // a/b/x/b/x/c
+ // a/b/c
+ // To do this, take the rest of the pattern after
+ // the **, and see if it would match the file remainder.
+ // If so, return success.
+ // If not, the ** "swallows" a segment, and try again.
+ // This is recursively awful.
+ //
+ // a/**/b/**/c matching a/b/x/y/z/c
+ // - a matches a
+ // - doublestar
+ // - matchOne(b/x/y/z/c, b/**/c)
+ // - b matches b
+ // - doublestar
+ // - matchOne(x/y/z/c, c) -> no
+ // - matchOne(y/z/c, c) -> no
+ // - matchOne(z/c, c) -> no
+ // - matchOne(c, c) yes, hit
+ var fr = fi
+ var pr = pi + 1
+ if (pr === pl) {
+ this.debug('** at the end')
+ // a ** at the end will just swallow the rest.
+ // We have found a match.
+ // however, it will not swallow /.x, unless
+ // options.dot is set.
+ // . and .. are *never* matched by **, for explosively
+ // exponential reasons.
+ for (; fi < fl; fi++) {
+ if (file[fi] === '.' || file[fi] === '..' ||
+ (!options.dot && file[fi].charAt(0) === '.')) return false
+ }
+ return true
+ }
+
+ // ok, let's see if we can swallow whatever we can.
+ while (fr < fl) {
+ var swallowee = file[fr]
+
+ this.debug('\nglobstar while', file, fr, pattern, pr, swallowee)
+
+ // XXX remove this slice. Just pass the start index.
+ if (this.matchOne(file.slice(fr), pattern.slice(pr), partial)) {
+ this.debug('globstar found match!', fr, fl, swallowee)
+ // found a match.
+ return true
+ } else {
+ // can't swallow "." or ".." ever.
+ // can only swallow ".foo" when explicitly asked.
+ if (swallowee === '.' || swallowee === '..' ||
+ (!options.dot && swallowee.charAt(0) === '.')) {
+ this.debug('dot detected!', file, fr, pattern, pr)
+ break
+ }
+
+ // ** swallows a segment, and continue.
+ this.debug('globstar swallow a segment, and continue')
+ fr++
+ }
+ }
+
+ // no match was found.
+ // However, in partial mode, we can't say this is necessarily over.
+ // If there's more *pattern* left, then
+ if (partial) {
+ // ran out of file
+ this.debug('\n>>> no match, partial?', file, fr, pattern, pr)
+ if (fr === fl) return true
+ }
+ return false
+ }
+
+ // something other than **
+ // non-magic patterns just have to match exactly
+ // patterns with magic have been turned into regexps.
+ var hit
+ if (typeof p === 'string') {
+ if (options.nocase) {
+ hit = f.toLowerCase() === p.toLowerCase()
+ } else {
+ hit = f === p
+ }
+ this.debug('string match', p, f, hit)
+ } else {
+ hit = f.match(p)
+ this.debug('pattern match', p, f, hit)
+ }
+
+ if (!hit) return false
+ }
+
+ // Note: ending in / means that we'll get a final ""
+ // at the end of the pattern. This can only match a
+ // corresponding "" at the end of the file.
+ // If the file ends in /, then it can only match a
+ // a pattern that ends in /, unless the pattern just
+ // doesn't have any more for it. But, a/b/ should *not*
+ // match "a/b/*", even though "" matches against the
+ // [^/]*? pattern, except in partial mode, where it might
+ // simply not be reached yet.
+ // However, a/b/ should still satisfy a/*
+
+ // now either we fell off the end of the pattern, or we're done.
+ if (fi === fl && pi === pl) {
+ // ran out of pattern and filename at the same time.
+ // an exact hit!
+ return true
+ } else if (fi === fl) {
+ // ran out of file, but still had pattern left.
+ // this is ok if we're doing the match as part of
+ // a glob fs traversal.
+ return partial
+ } else if (pi === pl) {
+ // ran out of pattern, still have file left.
+ // this is only acceptable if we're on the very last
+ // empty segment of a file with a trailing slash.
+ // a/* should match a/b/
+ var emptyFileEnd = (fi === fl - 1) && (file[fi] === '')
+ return emptyFileEnd
+ }
+
+ // should be unreachable.
+ throw new Error('wtf?')
+}
+
+// replace stuff like \* with *
+function globUnescape (s) {
+ return s.replace(/\\(.)/g, '$1')
+}
+
+function regExpEscape (s) {
+ return s.replace(/[-[\]{}()*+?.,\\^$|#\s]/g, '\\$&')
+}
diff --git a/node_modules/minimatch/package.json b/node_modules/minimatch/package.json
new file mode 100644
index 0000000..953009e
--- /dev/null
+++ b/node_modules/minimatch/package.json
@@ -0,0 +1,66 @@
+{
+ "_from": "minimatch@^2.0.1",
+ "_id": "minimatch@2.0.10",
+ "_inBundle": false,
+ "_integrity": "sha1-jQh8OcazjAAbl/ynzm0OHoCvusc=",
+ "_location": "/minimatch",
+ "_phantomChildren": {},
+ "_requested": {
+ "type": "range",
+ "registry": true,
+ "raw": "minimatch@^2.0.1",
+ "name": "minimatch",
+ "escapedName": "minimatch",
+ "rawSpec": "^2.0.1",
+ "saveSpec": null,
+ "fetchSpec": "^2.0.1"
+ },
+ "_requiredBy": [
+ "/glob",
+ "/glob-stream"
+ ],
+ "_resolved": "https://registry.npmjs.org/minimatch/-/minimatch-2.0.10.tgz",
+ "_shasum": "8d087c39c6b38c001b97fca7ce6d0e1e80afbac7",
+ "_spec": "minimatch@^2.0.1",
+ "_where": "/Users/rpatra16/MyProjects/RamAndAntara/node_modules/glob-stream",
+ "author": {
+ "name": "Isaac Z. Schlueter",
+ "email": "i@izs.me",
+ "url": "http://blog.izs.me"
+ },
+ "bugs": {
+ "url": "https://github.com/isaacs/minimatch/issues"
+ },
+ "bundleDependencies": false,
+ "dependencies": {
+ "brace-expansion": "^1.0.0"
+ },
+ "deprecated": "Please update to minimatch 3.0.2 or higher to avoid a RegExp DoS issue",
+ "description": "a glob matcher in javascript",
+ "devDependencies": {
+ "browserify": "^9.0.3",
+ "standard": "^3.7.2",
+ "tap": "^1.2.0"
+ },
+ "engines": {
+ "node": "*"
+ },
+ "files": [
+ "minimatch.js",
+ "browser.js"
+ ],
+ "homepage": "https://github.com/isaacs/minimatch#readme",
+ "license": "ISC",
+ "main": "minimatch.js",
+ "name": "minimatch",
+ "repository": {
+ "type": "git",
+ "url": "git://github.com/isaacs/minimatch.git"
+ },
+ "scripts": {
+ "posttest": "standard minimatch.js test/*.js",
+ "prepublish": "browserify -o browser.js -e minimatch.js -s minimatch --bare",
+ "test": "tap test/*.js"
+ },
+ "version": "2.0.10"
+}
diff --git a/node_modules/minimist/.travis.yml b/node_modules/minimist/.travis.yml
new file mode 100644
index 0000000..74c57bf
--- /dev/null
+++ b/node_modules/minimist/.travis.yml
@@ -0,0 +1,8 @@
+language: node_js
+node_js:
+ - "0.8"
+ - "0.10"
+ - "0.12"
+ - "iojs"
+before_install:
+ - npm install -g npm@~1.4.6
diff --git a/node_modules/minimist/LICENSE b/node_modules/minimist/LICENSE
new file mode 100644
index 0000000..ee27ba4
--- /dev/null
+++ b/node_modules/minimist/LICENSE
@@ -0,0 +1,18 @@
+This software is released under the MIT license:
+
+Permission is hereby granted, free of charge, to any person obtaining a copy of
+this software and associated documentation files (the "Software"), to deal in
+the Software without restriction, including without limitation the rights to
+use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of
+the Software, and to permit persons to whom the Software is furnished to do so,
+subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in all
+copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS
+FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR
+COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER
+IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN
+CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
diff --git a/node_modules/minimist/example/parse.js b/node_modules/minimist/example/parse.js
new file mode 100644
index 0000000..abff3e8
--- /dev/null
+++ b/node_modules/minimist/example/parse.js
@@ -0,0 +1,2 @@
+var argv = require('../')(process.argv.slice(2));
+console.dir(argv);
diff --git a/node_modules/minimist/index.js b/node_modules/minimist/index.js
new file mode 100644
index 0000000..6a0559d
--- /dev/null
+++ b/node_modules/minimist/index.js
@@ -0,0 +1,236 @@
+module.exports = function (args, opts) {
+ if (!opts) opts = {};
+
+ var flags = { bools : {}, strings : {}, unknownFn: null };
+
+ if (typeof opts['unknown'] === 'function') {
+ flags.unknownFn = opts['unknown'];
+ }
+
+ if (typeof opts['boolean'] === 'boolean' && opts['boolean']) {
+ flags.allBools = true;
+ } else {
+ [].concat(opts['boolean']).filter(Boolean).forEach(function (key) {
+ flags.bools[key] = true;
+ });
+ }
+
+ var aliases = {};
+ Object.keys(opts.alias || {}).forEach(function (key) {
+ aliases[key] = [].concat(opts.alias[key]);
+ aliases[key].forEach(function (x) {
+ aliases[x] = [key].concat(aliases[key].filter(function (y) {
+ return x !== y;
+ }));
+ });
+ });
+
+ [].concat(opts.string).filter(Boolean).forEach(function (key) {
+ flags.strings[key] = true;
+ if (aliases[key]) {
+ flags.strings[aliases[key]] = true;
+ }
+ });
+
+ var defaults = opts['default'] || {};
+
+ var argv = { _ : [] };
+ Object.keys(flags.bools).forEach(function (key) {
+ setArg(key, defaults[key] === undefined ? false : defaults[key]);
+ });
+
+ var notFlags = [];
+
+ if (args.indexOf('--') !== -1) {
+ notFlags = args.slice(args.indexOf('--')+1);
+ args = args.slice(0, args.indexOf('--'));
+ }
+
+ function argDefined(key, arg) {
+ return (flags.allBools && /^--[^=]+$/.test(arg)) ||
+ flags.strings[key] || flags.bools[key] || aliases[key];
+ }
+
+ function setArg (key, val, arg) {
+ if (arg && flags.unknownFn && !argDefined(key, arg)) {
+ if (flags.unknownFn(arg) === false) return;
+ }
+
+ var value = !flags.strings[key] && isNumber(val)
+ ? Number(val) : val
+ ;
+ setKey(argv, key.split('.'), value);
+
+ (aliases[key] || []).forEach(function (x) {
+ setKey(argv, x.split('.'), value);
+ });
+ }
+
+ function setKey (obj, keys, value) {
+ var o = obj;
+ keys.slice(0,-1).forEach(function (key) {
+ if (o[key] === undefined) o[key] = {};
+ o = o[key];
+ });
+
+ var key = keys[keys.length - 1];
+ if (o[key] === undefined || flags.bools[key] || typeof o[key] === 'boolean') {
+ o[key] = value;
+ }
+ else if (Array.isArray(o[key])) {
+ o[key].push(value);
+ }
+ else {
+ o[key] = [ o[key], value ];
+ }
+ }
+
+ function aliasIsBoolean(key) {
+ return aliases[key].some(function (x) {
+ return flags.bools[x];
+ });
+ }
+
+ for (var i = 0; i < args.length; i++) {
+ var arg = args[i];
+
+ if (/^--.+=/.test(arg)) {
+ // Using [\s\S] instead of . because js doesn't support the
+ // 'dotall' regex modifier. See:
+ // http://stackoverflow.com/a/1068308/13216
+ var m = arg.match(/^--([^=]+)=([\s\S]*)$/);
+ var key = m[1];
+ var value = m[2];
+ if (flags.bools[key]) {
+ value = value !== 'false';
+ }
+ setArg(key, value, arg);
+ }
+ else if (/^--no-.+/.test(arg)) {
+ var key = arg.match(/^--no-(.+)/)[1];
+ setArg(key, false, arg);
+ }
+ else if (/^--.+/.test(arg)) {
+ var key = arg.match(/^--(.+)/)[1];
+ var next = args[i + 1];
+ if (next !== undefined && !/^-/.test(next)
+ && !flags.bools[key]
+ && !flags.allBools
+ && (aliases[key] ? !aliasIsBoolean(key) : true)) {
+ setArg(key, next, arg);
+ i++;
+ }
+ else if (/^(true|false)$/.test(next)) {
+ setArg(key, next === 'true', arg);
+ i++;
+ }
+ else {
+ setArg(key, flags.strings[key] ? '' : true, arg);
+ }
+ }
+ else if (/^-[^-]+/.test(arg)) {
+ var letters = arg.slice(1,-1).split('');
+
+ var broken = false;
+ for (var j = 0; j < letters.length; j++) {
+ var next = arg.slice(j+2);
+
+ if (next === '-') {
+ setArg(letters[j], next, arg)
+ continue;
+ }
+
+ if (/[A-Za-z]/.test(letters[j]) && /=/.test(next)) {
+ setArg(letters[j], next.split('=')[1], arg);
+ broken = true;
+ break;
+ }
+
+ if (/[A-Za-z]/.test(letters[j])
+ && /-?\d+(\.\d*)?(e-?\d+)?$/.test(next)) {
+ setArg(letters[j], next, arg);
+ broken = true;
+ break;
+ }
+
+ if (letters[j+1] && letters[j+1].match(/\W/)) {
+ setArg(letters[j], arg.slice(j+2), arg);
+ broken = true;
+ break;
+ }
+ else {
+ setArg(letters[j], flags.strings[letters[j]] ? '' : true, arg);
+ }
+ }
+
+ var key = arg.slice(-1)[0];
+ if (!broken && key !== '-') {
+ if (args[i+1] && !/^(-|--)[^-]/.test(args[i+1])
+ && !flags.bools[key]
+ && (aliases[key] ? !aliasIsBoolean(key) : true)) {
+ setArg(key, args[i+1], arg);
+ i++;
+ }
+ else if (args[i+1] && /true|false/.test(args[i+1])) {
+ setArg(key, args[i+1] === 'true', arg);
+ i++;
+ }
+ else {
+ setArg(key, flags.strings[key] ? '' : true, arg);
+ }
+ }
+ }
+ else {
+ if (!flags.unknownFn || flags.unknownFn(arg) !== false) {
+ argv._.push(
+ flags.strings['_'] || !isNumber(arg) ? arg : Number(arg)
+ );
+ }
+ if (opts.stopEarly) {
+ argv._.push.apply(argv._, args.slice(i + 1));
+ break;
+ }
+ }
+ }
+
+ Object.keys(defaults).forEach(function (key) {
+ if (!hasKey(argv, key.split('.'))) {
+ setKey(argv, key.split('.'), defaults[key]);
+
+ (aliases[key] || []).forEach(function (x) {
+ setKey(argv, x.split('.'), defaults[key]);
+ });
+ }
+ });
+
+ if (opts['--']) {
+ argv['--'] = new Array();
+ notFlags.forEach(function(key) {
+ argv['--'].push(key);
+ });
+ }
+ else {
+ notFlags.forEach(function(key) {
+ argv._.push(key);
+ });
+ }
+
+ return argv;
+};
+
+function hasKey (obj, keys) {
+ var o = obj;
+ keys.slice(0,-1).forEach(function (key) {
+ o = (o[key] || {});
+ });
+
+ var key = keys[keys.length - 1];
+ return key in o;
+}
+
+function isNumber (x) {
+ if (typeof x === 'number') return true;
+ if (/^0x[0-9a-f]+$/i.test(x)) return true;
+ return /^[-+]?(?:\d+(?:\.\d*)?|\.\d+)(e[-+]?\d+)?$/.test(x);
+}
+
diff --git a/node_modules/minimist/package.json b/node_modules/minimist/package.json
new file mode 100644
index 0000000..94e8451
--- /dev/null
+++ b/node_modules/minimist/package.json
@@ -0,0 +1,75 @@
+{
+ "_from": "minimist@1.2.0",
+ "_id": "minimist@1.2.0",
+ "_inBundle": false,
+ "_integrity": "sha1-o1AIsg9BOD7sH7kU9M1d95omQoQ=",
+ "_location": "/minimist",
+ "_phantomChildren": {},
+ "_requested": {
+ "type": "version",
+ "registry": true,
+ "raw": "minimist@1.2.0",
+ "name": "minimist",
+ "escapedName": "minimist",
+ "rawSpec": "1.2.0",
+ "saveSpec": null,
+ "fetchSpec": "1.2.0"
+ },
+ "_requiredBy": [
+ "/gulp",
+ "/gulp-util",
+ "/meow"
+ ],
+ "_resolved": "http://registry.npmjs.org/minimist/-/minimist-1.2.0.tgz",
+ "_shasum": "a35008b20f41383eec1fb914f4cd5df79a264284",
+ "_spec": "minimist@1.2.0",
+ "_where": "/Users/rpatra16/MyProjects/RamAndAntara/node_modules/gulp",
+ "author": {
+ "name": "James Halliday",
+ "email": "mail@substack.net",
+ "url": "http://substack.net"
+ },
+ "bugs": {
+ "url": "https://github.com/substack/minimist/issues"
+ },
+ "bundleDependencies": false,
+ "deprecated": false,
+ "description": "parse argument options",
+ "devDependencies": {
+ "covert": "^1.0.0",
+ "tap": "~0.4.0",
+ "tape": "^3.5.0"
+ },
+ "homepage": "https://github.com/substack/minimist",
+ "keywords": [
+ "argv",
+ "getopt",
+ "parser",
+ "optimist"
+ ],
+ "license": "MIT",
+ "main": "index.js",
+ "name": "minimist",
+ "repository": {
+ "type": "git",
+ "url": "git://github.com/substack/minimist.git"
+ },
+ "scripts": {
+ "coverage": "covert test/*.js",
+ "test": "tap test/*.js"
+ },
+ "testling": {
+ "files": "test/*.js",
+ "browsers": [
+ "ie/6..latest",
+ "ff/5",
+ "firefox/latest",
+ "chrome/10",
+ "chrome/latest",
+ "safari/5.1",
+ "safari/latest",
+ "opera/12"
+ ]
+ },
+ "version": "1.2.0"
+}
diff --git a/node_modules/minimist/readme.markdown b/node_modules/minimist/readme.markdown
new file mode 100644
index 0000000..30a74cf
--- /dev/null
+++ b/node_modules/minimist/readme.markdown
@@ -0,0 +1,91 @@
+# minimist
+
+parse argument options
+
+This module is the guts of optimist's argument parser without all the
+fanciful decoration.
+
+[](http://ci.testling.com/substack/minimist)
+
+[](http://travis-ci.org/substack/minimist)
+
+# example
+
+``` js
+var argv = require('minimist')(process.argv.slice(2));
+console.dir(argv);
+```
+
+```
+$ node example/parse.js -a beep -b boop
+{ _: [], a: 'beep', b: 'boop' }
+```
+
+```
+$ node example/parse.js -x 3 -y 4 -n5 -abc --beep=boop foo bar baz
+{ _: [ 'foo', 'bar', 'baz' ],
+ x: 3,
+ y: 4,
+ n: 5,
+ a: true,
+ b: true,
+ c: true,
+ beep: 'boop' }
+```
+
+# methods
+
+``` js
+var parseArgs = require('minimist')
+```
+
+## var argv = parseArgs(args, opts={})
+
+Return an argument object `argv` populated with the array arguments from `args`.
+
+`argv._` contains all the arguments that didn't have an option associated with
+them.
+
+Numeric-looking arguments will be returned as numbers unless `opts.string` or
+`opts.boolean` is set for that argument name.
+
+Any arguments after `'--'` will not be parsed and will end up in `argv._`.
+
+options can be:
+
+* `opts.string` - a string or array of strings argument names to always treat as
+strings
+* `opts.boolean` - a boolean, string or array of strings to always treat as
+booleans. if `true` will treat all double hyphenated arguments without equal signs
+as boolean (e.g. affects `--foo`, not `-f` or `--foo=bar`)
+* `opts.alias` - an object mapping string names to strings or arrays of string
+argument names to use as aliases
+* `opts.default` - an object mapping string argument names to default values
+* `opts.stopEarly` - when true, populate `argv._` with everything after the
+first non-option
+* `opts['--']` - when true, populate `argv._` with everything before the `--`
+and `argv['--']` with everything after the `--`. Here's an example:
+* `opts.unknown` - a function which is invoked with a command line parameter not
+defined in the `opts` configuration object. If the function returns `false`, the
+unknown option is not added to `argv`.
+
+```
+> require('./')('one two three -- four five --six'.split(' '), { '--': true })
+{ _: [ 'one', 'two', 'three' ],
+ '--': [ 'four', 'five', '--six' ] }
+```
+
+Note that with `opts['--']` set, parsing for arguments still stops after the
+`--`.
+
+# install
+
+With [npm](https://npmjs.org) do:
+
+```
+npm install minimist
+```
+
+# license
+
+MIT
diff --git a/node_modules/minimist/test/all_bool.js b/node_modules/minimist/test/all_bool.js
new file mode 100644
index 0000000..ac83548
--- /dev/null
+++ b/node_modules/minimist/test/all_bool.js
@@ -0,0 +1,32 @@
+var parse = require('../');
+var test = require('tape');
+
+test('flag boolean true (default all --args to boolean)', function (t) {
+ var argv = parse(['moo', '--honk', 'cow'], {
+ boolean: true
+ });
+
+ t.deepEqual(argv, {
+ honk: true,
+ _: ['moo', 'cow']
+ });
+
+ t.deepEqual(typeof argv.honk, 'boolean');
+ t.end();
+});
+
+test('flag boolean true only affects double hyphen arguments without equals signs', function (t) {
+ var argv = parse(['moo', '--honk', 'cow', '-p', '55', '--tacos=good'], {
+ boolean: true
+ });
+
+ t.deepEqual(argv, {
+ honk: true,
+ tacos: 'good',
+ p: 55,
+ _: ['moo', 'cow']
+ });
+
+ t.deepEqual(typeof argv.honk, 'boolean');
+ t.end();
+});
diff --git a/node_modules/minimist/test/bool.js b/node_modules/minimist/test/bool.js
new file mode 100644
index 0000000..14b0717
--- /dev/null
+++ b/node_modules/minimist/test/bool.js
@@ -0,0 +1,166 @@
+var parse = require('../');
+var test = require('tape');
+
+test('flag boolean default false', function (t) {
+ var argv = parse(['moo'], {
+ boolean: ['t', 'verbose'],
+ default: { verbose: false, t: false }
+ });
+
+ t.deepEqual(argv, {
+ verbose: false,
+ t: false,
+ _: ['moo']
+ });
+
+ t.deepEqual(typeof argv.verbose, 'boolean');
+ t.deepEqual(typeof argv.t, 'boolean');
+ t.end();
+
+});
+
+test('boolean groups', function (t) {
+ var argv = parse([ '-x', '-z', 'one', 'two', 'three' ], {
+ boolean: ['x','y','z']
+ });
+
+ t.deepEqual(argv, {
+ x : true,
+ y : false,
+ z : true,
+ _ : [ 'one', 'two', 'three' ]
+ });
+
+ t.deepEqual(typeof argv.x, 'boolean');
+ t.deepEqual(typeof argv.y, 'boolean');
+ t.deepEqual(typeof argv.z, 'boolean');
+ t.end();
+});
+test('boolean and alias with chainable api', function (t) {
+ var aliased = [ '-h', 'derp' ];
+ var regular = [ '--herp', 'derp' ];
+ var opts = {
+ herp: { alias: 'h', boolean: true }
+ };
+ var aliasedArgv = parse(aliased, {
+ boolean: 'herp',
+ alias: { h: 'herp' }
+ });
+ var propertyArgv = parse(regular, {
+ boolean: 'herp',
+ alias: { h: 'herp' }
+ });
+ var expected = {
+ herp: true,
+ h: true,
+ '_': [ 'derp' ]
+ };
+
+ t.same(aliasedArgv, expected);
+ t.same(propertyArgv, expected);
+ t.end();
+});
+
+test('boolean and alias with options hash', function (t) {
+ var aliased = [ '-h', 'derp' ];
+ var regular = [ '--herp', 'derp' ];
+ var opts = {
+ alias: { 'h': 'herp' },
+ boolean: 'herp'
+ };
+ var aliasedArgv = parse(aliased, opts);
+ var propertyArgv = parse(regular, opts);
+ var expected = {
+ herp: true,
+ h: true,
+ '_': [ 'derp' ]
+ };
+ t.same(aliasedArgv, expected);
+ t.same(propertyArgv, expected);
+ t.end();
+});
+
+test('boolean and alias array with options hash', function (t) {
+ var aliased = [ '-h', 'derp' ];
+ var regular = [ '--herp', 'derp' ];
+ var alt = [ '--harp', 'derp' ];
+ var opts = {
+ alias: { 'h': ['herp', 'harp'] },
+ boolean: 'h'
+ };
+ var aliasedArgv = parse(aliased, opts);
+ var propertyArgv = parse(regular, opts);
+ var altPropertyArgv = parse(alt, opts);
+ var expected = {
+ harp: true,
+ herp: true,
+ h: true,
+ '_': [ 'derp' ]
+ };
+ t.same(aliasedArgv, expected);
+ t.same(propertyArgv, expected);
+ t.same(altPropertyArgv, expected);
+ t.end();
+});
+
+test('boolean and alias using explicit true', function (t) {
+ var aliased = [ '-h', 'true' ];
+ var regular = [ '--herp', 'true' ];
+ var opts = {
+ alias: { h: 'herp' },
+ boolean: 'h'
+ };
+ var aliasedArgv = parse(aliased, opts);
+ var propertyArgv = parse(regular, opts);
+ var expected = {
+ herp: true,
+ h: true,
+ '_': [ ]
+ };
+
+ t.same(aliasedArgv, expected);
+ t.same(propertyArgv, expected);
+ t.end();
+});
+
+// regression, see https://github.com/substack/node-optimist/issues/71
+test('boolean and --x=true', function(t) {
+ var parsed = parse(['--boool', '--other=true'], {
+ boolean: 'boool'
+ });
+
+ t.same(parsed.boool, true);
+ t.same(parsed.other, 'true');
+
+ parsed = parse(['--boool', '--other=false'], {
+ boolean: 'boool'
+ });
+
+ t.same(parsed.boool, true);
+ t.same(parsed.other, 'false');
+ t.end();
+});
+
+test('boolean --boool=true', function (t) {
+ var parsed = parse(['--boool=true'], {
+ default: {
+ boool: false
+ },
+ boolean: ['boool']
+ });
+
+ t.same(parsed.boool, true);
+ t.end();
+});
+
+test('boolean --boool=false', function (t) {
+ var parsed = parse(['--boool=false'], {
+ default: {
+ boool: true
+ },
+ boolean: ['boool']
+ });
+
+ t.same(parsed.boool, false);
+ t.end();
+});
diff --git a/node_modules/minimist/test/dash.js b/node_modules/minimist/test/dash.js
new file mode 100644
index 0000000..5a4fa5b
--- /dev/null
+++ b/node_modules/minimist/test/dash.js
@@ -0,0 +1,31 @@
+var parse = require('../');
+var test = require('tape');
+
+test('-', function (t) {
+ t.plan(5);
+ t.deepEqual(parse([ '-n', '-' ]), { n: '-', _: [] });
+ t.deepEqual(parse([ '-' ]), { _: [ '-' ] });
+ t.deepEqual(parse([ '-f-' ]), { f: '-', _: [] });
+ t.deepEqual(
+ parse([ '-b', '-' ], { boolean: 'b' }),
+ { b: true, _: [ '-' ] }
+ );
+ t.deepEqual(
+ parse([ '-s', '-' ], { string: 's' }),
+ { s: '-', _: [] }
+ );
+});
+
+test('-a -- b', function (t) {
+ t.plan(3);
+ t.deepEqual(parse([ '-a', '--', 'b' ]), { a: true, _: [ 'b' ] });
+ t.deepEqual(parse([ '--a', '--', 'b' ]), { a: true, _: [ 'b' ] });
+ t.deepEqual(parse([ '--a', '--', 'b' ]), { a: true, _: [ 'b' ] });
+});
+
+test('move arguments after the -- into their own `--` array', function(t) {
+ t.plan(1);
+ t.deepEqual(
+ parse([ '--name', 'John', 'before', '--', 'after' ], { '--': true }),
+ { name: 'John', _: [ 'before' ], '--': [ 'after' ] });
+});
diff --git a/node_modules/minimist/test/default_bool.js b/node_modules/minimist/test/default_bool.js
new file mode 100644
index 0000000..780a311
--- /dev/null
+++ b/node_modules/minimist/test/default_bool.js
@@ -0,0 +1,35 @@
+var test = require('tape');
+var parse = require('../');
+
+test('boolean default true', function (t) {
+ var argv = parse([], {
+ boolean: 'sometrue',
+ default: { sometrue: true }
+ });
+ t.equal(argv.sometrue, true);
+ t.end();
+});
+
+test('boolean default false', function (t) {
+ var argv = parse([], {
+ boolean: 'somefalse',
+ default: { somefalse: false }
+ });
+ t.equal(argv.somefalse, false);
+ t.end();
+});
+
+test('boolean default to null', function (t) {
+ var argv = parse([], {
+ boolean: 'maybe',
+ default: { maybe: null }
+ });
+ t.equal(argv.maybe, null);
+ var argv = parse(['--maybe'], {
+ boolean: 'maybe',
+ default: { maybe: null }
+ });
+ t.equal(argv.maybe, true);
+ t.end();
+
+})
diff --git a/node_modules/minimist/test/dotted.js b/node_modules/minimist/test/dotted.js
new file mode 100644
index 0000000..d8b3e85
--- /dev/null
+++ b/node_modules/minimist/test/dotted.js
@@ -0,0 +1,22 @@
+var parse = require('../');
+var test = require('tape');
+
+test('dotted alias', function (t) {
+ var argv = parse(['--a.b', '22'], {default: {'a.b': 11}, alias: {'a.b': 'aa.bb'}});
+ t.equal(argv.a.b, 22);
+ t.equal(argv.aa.bb, 22);
+ t.end();
+});
+
+test('dotted default', function (t) {
+ var argv = parse('', {default: {'a.b': 11}, alias: {'a.b': 'aa.bb'}});
+ t.equal(argv.a.b, 11);
+ t.equal(argv.aa.bb, 11);
+ t.end();
+});
+
+test('dotted default with no alias', function (t) {
+ var argv = parse('', {default: {'a.b': 11}});
+ t.equal(argv.a.b, 11);
+ t.end();
+});
diff --git a/node_modules/minimist/test/kv_short.js b/node_modules/minimist/test/kv_short.js
new file mode 100644
index 0000000..f813b30
--- /dev/null
+++ b/node_modules/minimist/test/kv_short.js
@@ -0,0 +1,16 @@
+var parse = require('../');
+var test = require('tape');
+
+test('short -k=v' , function (t) {
+ t.plan(1);
+
+ var argv = parse([ '-b=123' ]);
+ t.deepEqual(argv, { b: 123, _: [] });
+});
+
+test('multi short -k=v' , function (t) {
+ t.plan(1);
+
+ var argv = parse([ '-a=whatever', '-b=robots' ]);
+ t.deepEqual(argv, { a: 'whatever', b: 'robots', _: [] });
+});
diff --git a/node_modules/minimist/test/long.js b/node_modules/minimist/test/long.js
new file mode 100644
index 0000000..5d3a1e0
--- /dev/null
+++ b/node_modules/minimist/test/long.js
@@ -0,0 +1,31 @@
+var test = require('tape');
+var parse = require('../');
+
+test('long opts', function (t) {
+ t.deepEqual(
+ parse([ '--bool' ]),
+ { bool : true, _ : [] },
+ 'long boolean'
+ );
+ t.deepEqual(
+ parse([ '--pow', 'xixxle' ]),
+ { pow : 'xixxle', _ : [] },
+ 'long capture sp'
+ );
+ t.deepEqual(
+ parse([ '--pow=xixxle' ]),
+ { pow : 'xixxle', _ : [] },
+ 'long capture eq'
+ );
+ t.deepEqual(
+ parse([ '--host', 'localhost', '--port', '555' ]),
+ { host : 'localhost', port : 555, _ : [] },
+ 'long captures sp'
+ );
+ t.deepEqual(
+ parse([ '--host=localhost', '--port=555' ]),
+ { host : 'localhost', port : 555, _ : [] },
+ 'long captures eq'
+ );
+ t.end();
+});
diff --git a/node_modules/minimist/test/num.js b/node_modules/minimist/test/num.js
new file mode 100644
index 0000000..2cc77f4
--- /dev/null
+++ b/node_modules/minimist/test/num.js
@@ -0,0 +1,36 @@
+var parse = require('../');
+var test = require('tape');
+
+test('nums', function (t) {
+ var argv = parse([
+ '-x', '1234',
+ '-y', '5.67',
+ '-z', '1e7',
+ '-w', '10f',
+ '--hex', '0xdeadbeef',
+ '789'
+ ]);
+ t.deepEqual(argv, {
+ x : 1234,
+ y : 5.67,
+ z : 1e7,
+ w : '10f',
+ hex : 0xdeadbeef,
+ _ : [ 789 ]
+ });
+ t.deepEqual(typeof argv.x, 'number');
+ t.deepEqual(typeof argv.y, 'number');
+ t.deepEqual(typeof argv.z, 'number');
+ t.deepEqual(typeof argv.w, 'string');
+ t.deepEqual(typeof argv.hex, 'number');
+ t.deepEqual(typeof argv._[0], 'number');
+ t.end();
+});
+
+test('already a number', function (t) {
+ var argv = parse([ '-x', 1234, 789 ]);
+ t.deepEqual(argv, { x : 1234, _ : [ 789 ] });
+ t.deepEqual(typeof argv.x, 'number');
+ t.deepEqual(typeof argv._[0], 'number');
+ t.end();
+});
diff --git a/node_modules/minimist/test/parse.js b/node_modules/minimist/test/parse.js
new file mode 100644
index 0000000..7b4a2a1
--- /dev/null
+++ b/node_modules/minimist/test/parse.js
@@ -0,0 +1,197 @@
+var parse = require('../');
+var test = require('tape');
+
+test('parse args', function (t) {
+ t.deepEqual(
+ parse([ '--no-moo' ]),
+ { moo : false, _ : [] },
+ 'no'
+ );
+ t.deepEqual(
+ parse([ '-v', 'a', '-v', 'b', '-v', 'c' ]),
+ { v : ['a','b','c'], _ : [] },
+ 'multi'
+ );
+ t.end();
+});
+
+test('comprehensive', function (t) {
+ t.deepEqual(
+ parse([
+ '--name=meowmers', 'bare', '-cats', 'woo',
+ '-h', 'awesome', '--multi=quux',
+ '--key', 'value',
+ '-b', '--bool', '--no-meep', '--multi=baz',
+ '--', '--not-a-flag', 'eek'
+ ]),
+ {
+ c : true,
+ a : true,
+ t : true,
+ s : 'woo',
+ h : 'awesome',
+ b : true,
+ bool : true,
+ key : 'value',
+ multi : [ 'quux', 'baz' ],
+ meep : false,
+ name : 'meowmers',
+ _ : [ 'bare', '--not-a-flag', 'eek' ]
+ }
+ );
+ t.end();
+});
+
+test('flag boolean', function (t) {
+ var argv = parse([ '-t', 'moo' ], { boolean: 't' });
+ t.deepEqual(argv, { t : true, _ : [ 'moo' ] });
+ t.deepEqual(typeof argv.t, 'boolean');
+ t.end();
+});
+
+test('flag boolean value', function (t) {
+ var argv = parse(['--verbose', 'false', 'moo', '-t', 'true'], {
+ boolean: [ 't', 'verbose' ],
+ default: { verbose: true }
+ });
+
+ t.deepEqual(argv, {
+ verbose: false,
+ t: true,
+ _: ['moo']
+ });
+
+ t.deepEqual(typeof argv.verbose, 'boolean');
+ t.deepEqual(typeof argv.t, 'boolean');
+ t.end();
+});
+
+test('newlines in params' , function (t) {
+ var args = parse([ '-s', "X\nX" ])
+ t.deepEqual(args, { _ : [], s : "X\nX" });
+
+ // reproduce in bash:
+ // VALUE="new
+ // line"
+ // node program.js --s="$VALUE"
+ args = parse([ "--s=X\nX" ])
+ t.deepEqual(args, { _ : [], s : "X\nX" });
+ t.end();
+});
+
+test('strings' , function (t) {
+ var s = parse([ '-s', '0001234' ], { string: 's' }).s;
+ t.equal(s, '0001234');
+ t.equal(typeof s, 'string');
+
+ var x = parse([ '-x', '56' ], { string: 'x' }).x;
+ t.equal(x, '56');
+ t.equal(typeof x, 'string');
+ t.end();
+});
+
+test('stringArgs', function (t) {
+ var s = parse([ ' ', ' ' ], { string: '_' })._;
+ t.same(s.length, 2);
+ t.same(typeof s[0], 'string');
+ t.same(s[0], ' ');
+ t.same(typeof s[1], 'string');
+ t.same(s[1], ' ');
+ t.end();
+});
+
+test('empty strings', function(t) {
+ var s = parse([ '-s' ], { string: 's' }).s;
+ t.equal(s, '');
+ t.equal(typeof s, 'string');
+
+ var str = parse([ '--str' ], { string: 'str' }).str;
+ t.equal(str, '');
+ t.equal(typeof str, 'string');
+
+ var letters = parse([ '-art' ], {
+ string: [ 'a', 't' ]
+ });
+
+ t.equal(letters.a, '');
+ t.equal(letters.r, true);
+ t.equal(letters.t, '');
+
+ t.end();
+});
+
+
+test('string and alias', function(t) {
+ var x = parse([ '--str', '000123' ], {
+ string: 's',
+ alias: { s: 'str' }
+ });
+
+ t.equal(x.str, '000123');
+ t.equal(typeof x.str, 'string');
+ t.equal(x.s, '000123');
+ t.equal(typeof x.s, 'string');
+
+ var y = parse([ '-s', '000123' ], {
+ string: 'str',
+ alias: { str: 's' }
+ });
+
+ t.equal(y.str, '000123');
+ t.equal(typeof y.str, 'string');
+ t.equal(y.s, '000123');
+ t.equal(typeof y.s, 'string');
+ t.end();
+});
+
+test('slashBreak', function (t) {
+ t.same(
+ parse([ '-I/foo/bar/baz' ]),
+ { I : '/foo/bar/baz', _ : [] }
+ );
+ t.same(
+ parse([ '-xyz/foo/bar/baz' ]),
+ { x : true, y : true, z : '/foo/bar/baz', _ : [] }
+ );
+ t.end();
+});
+
+test('alias', function (t) {
+ var argv = parse([ '-f', '11', '--zoom', '55' ], {
+ alias: { z: 'zoom' }
+ });
+ t.equal(argv.zoom, 55);
+ t.equal(argv.z, argv.zoom);
+ t.equal(argv.f, 11);
+ t.end();
+});
+
+test('multiAlias', function (t) {
+ var argv = parse([ '-f', '11', '--zoom', '55' ], {
+ alias: { z: [ 'zm', 'zoom' ] }
+ });
+ t.equal(argv.zoom, 55);
+ t.equal(argv.z, argv.zoom);
+ t.equal(argv.z, argv.zm);
+ t.equal(argv.f, 11);
+ t.end();
+});
+
+test('nested dotted objects', function (t) {
+ var argv = parse([
+ '--foo.bar', '3', '--foo.baz', '4',
+ '--foo.quux.quibble', '5', '--foo.quux.o_O',
+ '--beep.boop'
+ ]);
+
+ t.same(argv.foo, {
+ bar : 3,
+ baz : 4,
+ quux : {
+ quibble : 5,
+ o_O : true
+ }
+ });
+ t.same(argv.beep, { boop : true });
+ t.end();
+});
diff --git a/node_modules/minimist/test/parse_modified.js b/node_modules/minimist/test/parse_modified.js
new file mode 100644
index 0000000..ab620dc
--- /dev/null
+++ b/node_modules/minimist/test/parse_modified.js
@@ -0,0 +1,9 @@
+var parse = require('../');
+var test = require('tape');
+
+test('parse with modifier functions' , function (t) {
+ t.plan(1);
+
+ var argv = parse([ '-b', '123' ], { boolean: 'b' });
+ t.deepEqual(argv, { b: true, _: [123] });
+});
diff --git a/node_modules/minimist/test/short.js b/node_modules/minimist/test/short.js
new file mode 100644
index 0000000..d513a1c
--- /dev/null
+++ b/node_modules/minimist/test/short.js
@@ -0,0 +1,67 @@
+var parse = require('../');
+var test = require('tape');
+
+test('numeric short args', function (t) {
+ t.plan(2);
+ t.deepEqual(parse([ '-n123' ]), { n: 123, _: [] });
+ t.deepEqual(
+ parse([ '-123', '456' ]),
+ { 1: true, 2: true, 3: 456, _: [] }
+ );
+});
+
+test('short', function (t) {
+ t.deepEqual(
+ parse([ '-b' ]),
+ { b : true, _ : [] },
+ 'short boolean'
+ );
+ t.deepEqual(
+ parse([ 'foo', 'bar', 'baz' ]),
+ { _ : [ 'foo', 'bar', 'baz' ] },
+ 'bare'
+ );
+ t.deepEqual(
+ parse([ '-cats' ]),
+ { c : true, a : true, t : true, s : true, _ : [] },
+ 'group'
+ );
+ t.deepEqual(
+ parse([ '-cats', 'meow' ]),
+ { c : true, a : true, t : true, s : 'meow', _ : [] },
+ 'short group next'
+ );
+ t.deepEqual(
+ parse([ '-h', 'localhost' ]),
+ { h : 'localhost', _ : [] },
+ 'short capture'
+ );
+ t.deepEqual(
+ parse([ '-h', 'localhost', '-p', '555' ]),
+ { h : 'localhost', p : 555, _ : [] },
+ 'short captures'
+ );
+ t.end();
+});
+
+test('mixed short bool and capture', function (t) {
+ t.same(
+ parse([ '-h', 'localhost', '-fp', '555', 'script.js' ]),
+ {
+ f : true, p : 555, h : 'localhost',
+ _ : [ 'script.js' ]
+ }
+ );
+ t.end();
+});
+
+test('short and long', function (t) {
+ t.deepEqual(
+ parse([ '-h', 'localhost', '-fp', '555', 'script.js' ]),
+ {
+ f : true, p : 555, h : 'localhost',
+ _ : [ 'script.js' ]
+ }
+ );
+ t.end();
+});
diff --git a/node_modules/minimist/test/stop_early.js b/node_modules/minimist/test/stop_early.js
new file mode 100644
index 0000000..bdf9fbc
--- /dev/null
+++ b/node_modules/minimist/test/stop_early.js
@@ -0,0 +1,15 @@
+var parse = require('../');
+var test = require('tape');
+
+test('stops parsing on the first non-option when stopEarly is set', function (t) {
+ var argv = parse(['--aaa', 'bbb', 'ccc', '--ddd'], {
+ stopEarly: true
+ });
+
+ t.deepEqual(argv, {
+ aaa: 'bbb',
+ _: ['ccc', '--ddd']
+ });
+
+ t.end();
+});
diff --git a/node_modules/minimist/test/unknown.js b/node_modules/minimist/test/unknown.js
new file mode 100644
index 0000000..462a36b
--- /dev/null
+++ b/node_modules/minimist/test/unknown.js
@@ -0,0 +1,102 @@
+var parse = require('../');
+var test = require('tape');
+
+test('boolean and alias is not unknown', function (t) {
+ var unknown = [];
+ function unknownFn(arg) {
+ unknown.push(arg);
+ return false;
+ }
+ var aliased = [ '-h', 'true', '--derp', 'true' ];
+ var regular = [ '--herp', 'true', '-d', 'true' ];
+ var opts = {
+ alias: { h: 'herp' },
+ boolean: 'h',
+ unknown: unknownFn
+ };
+ var aliasedArgv = parse(aliased, opts);
+ var propertyArgv = parse(regular, opts);
+
+ t.same(unknown, ['--derp', '-d']);
+ t.end();
+});
+
+test('flag boolean true any double hyphen argument is not unknown', function (t) {
+ var unknown = [];
+ function unknownFn(arg) {
+ unknown.push(arg);
+ return false;
+ }
+ var argv = parse(['--honk', '--tacos=good', 'cow', '-p', '55'], {
+ boolean: true,
+ unknown: unknownFn
+ });
+ t.same(unknown, ['--tacos=good', 'cow', '-p']);
+ t.same(argv, {
+ honk: true,
+ _: []
+ });
+ t.end();
+});
+
+test('string and alias is not unknown', function (t) {
+ var unknown = [];
+ function unknownFn(arg) {
+ unknown.push(arg);
+ return false;
+ }
+ var aliased = [ '-h', 'hello', '--derp', 'goodbye' ];
+ var regular = [ '--herp', 'hello', '-d', 'moon' ];
+ var opts = {
+ alias: { h: 'herp' },
+ string: 'h',
+ unknown: unknownFn
+ };
+ var aliasedArgv = parse(aliased, opts);
+ var propertyArgv = parse(regular, opts);
+
+ t.same(unknown, ['--derp', '-d']);
+ t.end();
+});
+
+test('default and alias is not unknown', function (t) {
+ var unknown = [];
+ function unknownFn(arg) {
+ unknown.push(arg);
+ return false;
+ }
+ var aliased = [ '-h', 'hello' ];
+ var regular = [ '--herp', 'hello' ];
+ var opts = {
+ default: { 'h': 'bar' },
+ alias: { 'h': 'herp' },
+ unknown: unknownFn
+ };
+ var aliasedArgv = parse(aliased, opts);
+ var propertyArgv = parse(regular, opts);
+
+ t.same(unknown, []);
+ t.end();
+ unknownFn(); // exercise fn for 100% coverage
+});
+
+test('value following -- is not unknown', function (t) {
+ var unknown = [];
+ function unknownFn(arg) {
+ unknown.push(arg);
+ return false;
+ }
+ var aliased = [ '--bad', '--', 'good', 'arg' ];
+ var opts = {
+ '--': true,
+ unknown: unknownFn
+ };
+ var argv = parse(aliased, opts);
+
+ t.same(unknown, ['--bad']);
+ t.same(argv, {
+ '--': ['good', 'arg'],
+ '_': []
+ })
+ t.end();
+});
diff --git a/node_modules/minimist/test/whitespace.js b/node_modules/minimist/test/whitespace.js
new file mode 100644
index 0000000..8a52a58
--- /dev/null
+++ b/node_modules/minimist/test/whitespace.js
@@ -0,0 +1,8 @@
+var parse = require('../');
+var test = require('tape');
+
+test('whitespace should be whitespace' , function (t) {
+ t.plan(1);
+ var x = parse([ '-x', '\t' ]).x;
+ t.equal(x, '\t');
+});
diff --git a/node_modules/mixin-deep/LICENSE b/node_modules/mixin-deep/LICENSE
new file mode 100644
index 0000000..99c9369
--- /dev/null
+++ b/node_modules/mixin-deep/LICENSE
@@ -0,0 +1,21 @@
+The MIT License (MIT)
+
+Copyright (c) 2014-2015, 2017, Jon Schlinkert.
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in
+all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
+THE SOFTWARE.
diff --git a/node_modules/mixin-deep/README.md b/node_modules/mixin-deep/README.md
new file mode 100644
index 0000000..111bde0
--- /dev/null
+++ b/node_modules/mixin-deep/README.md
@@ -0,0 +1,80 @@
+# mixin-deep [](https://www.npmjs.com/package/mixin-deep) [](https://npmjs.org/package/mixin-deep) [](https://npmjs.org/package/mixin-deep) [](https://travis-ci.org/jonschlinkert/mixin-deep)
+
+> Deeply mix the properties of objects into the first object. Like merge-deep, but doesn't clone.
+
+Please consider following this project's author, [Jon Schlinkert](https://github.com/jonschlinkert), and consider starring the project to show your :heart: and support.
+
+## Install
+
+Install with [npm](https://www.npmjs.com/):
+
+```sh
+$ npm install --save mixin-deep
+```
+
+## Usage
+
+```js
+var mixinDeep = require('mixin-deep');
+
+mixinDeep({a: {aa: 'aa'}}, {a: {bb: 'bb'}}, {a: {cc: 'cc'}});
+//=> { a: { aa: 'aa', bb: 'bb', cc: 'cc' } }
+```
+
+## About
+
+
+Contributing
+
+Pull requests and stars are always welcome. For bugs and feature requests, [please create an issue](../../issues/new).
+
+
+
+
+Running Tests
+
+Running and reviewing unit tests is a great way to get familiarized with a library and its API. You can install dependencies and run tests with the following command:
+
+```sh
+$ npm install && npm test
+```
+
+
+
+Building docs
+
+_(This project's readme.md is generated by [verb](https://github.com/verbose/verb-generate-readme), please don't edit the readme directly. Any changes to the readme must be made in the [.verb.md](.verb.md) readme template.)_
+
+To generate the readme, run the following command:
+
+```sh
+$ npm install -g verbose/verb#dev verb-generate-readme && verb
+```
+
+
+
+### Related projects
+
+You might also be interested in these projects:
+
+* [defaults-deep](https://www.npmjs.com/package/defaults-deep): Like `extend` but recursively copies only the missing properties/values to the target object. | [homepage](https://github.com/jonschlinkert/defaults-deep "Like `extend` but recursively copies only the missing properties/values to the target object.")
+* [extend-shallow](https://www.npmjs.com/package/extend-shallow): Extend an object with the properties of additional objects. node.js/javascript util. | [homepage](https://github.com/jonschlinkert/extend-shallow "Extend an object with the properties of additional objects. node.js/javascript util.")
+* [merge-deep](https://www.npmjs.com/package/merge-deep): Recursively merge values in a javascript object. | [homepage](https://github.com/jonschlinkert/merge-deep "Recursively merge values in a javascript object.")
+* [mixin-object](https://www.npmjs.com/package/mixin-object): Mixin the own and inherited properties of other objects onto the first object. Pass an… [more](https://github.com/jonschlinkert/mixin-object) | [homepage](https://github.com/jonschlinkert/mixin-object "Mixin the own and inherited properties of other objects onto the first object. Pass an empty object as the first arg to shallow clone.")
+
+### Author
+
+**Jon Schlinkert**
+
+* [linkedin/in/jonschlinkert](https://linkedin.com/in/jonschlinkert)
+* [github/jonschlinkert](https://github.com/jonschlinkert)
+* [twitter/jonschlinkert](https://twitter.com/jonschlinkert)
+
+### License
+
+Copyright © 2017, [Jon Schlinkert](https://github.com/jonschlinkert).
+Released under the [MIT License](LICENSE).
+
+***
+
+_This file was generated by [verb-generate-readme](https://github.com/verbose/verb-generate-readme), v0.6.0, on December 09, 2017._
\ No newline at end of file
diff --git a/node_modules/mixin-deep/index.js b/node_modules/mixin-deep/index.js
new file mode 100644
index 0000000..909fbef
--- /dev/null
+++ b/node_modules/mixin-deep/index.js
@@ -0,0 +1,53 @@
+'use strict';
+
+var isExtendable = require('is-extendable');
+var forIn = require('for-in');
+
+function mixinDeep(target, objects) {
+ var len = arguments.length, i = 0;
+ while (++i < len) {
+ var obj = arguments[i];
+ if (isObject(obj)) {
+ forIn(obj, copy, target);
+ }
+ }
+ return target;
+}
+
+/**
+ * Copy properties from the source object to the
+ * target object.
+ *
+ * @param {*} `val`
+ * @param {String} `key`
+ */
+
+function copy(val, key) {
+ if (key === '__proto__') {
+ return;
+ }
+
+ var obj = this[key];
+ if (isObject(val) && isObject(obj)) {
+ mixinDeep(obj, val);
+ } else {
+ this[key] = val;
+ }
+}
+
+/**
+ * Returns true if `val` is an object or function.
+ *
+ * @param {any} val
+ * @return {Boolean}
+ */
+
+function isObject(val) {
+ return isExtendable(val) && !Array.isArray(val);
+}
+
+/**
+ * Expose `mixinDeep`
+ */
+
+module.exports = mixinDeep;
diff --git a/node_modules/mixin-deep/node_modules/is-extendable/LICENSE b/node_modules/mixin-deep/node_modules/is-extendable/LICENSE
new file mode 100644
index 0000000..c0d7f13
--- /dev/null
+++ b/node_modules/mixin-deep/node_modules/is-extendable/LICENSE
@@ -0,0 +1,21 @@
+The MIT License (MIT)
+
+Copyright (c) 2015-2017, Jon Schlinkert.
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in
+all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
+THE SOFTWARE.
\ No newline at end of file
diff --git a/node_modules/mixin-deep/node_modules/is-extendable/README.md b/node_modules/mixin-deep/node_modules/is-extendable/README.md
new file mode 100644
index 0000000..875b56a
--- /dev/null
+++ b/node_modules/mixin-deep/node_modules/is-extendable/README.md
@@ -0,0 +1,88 @@
+# is-extendable [](https://www.npmjs.com/package/is-extendable) [](https://npmjs.org/package/is-extendable) [](https://npmjs.org/package/is-extendable) [](https://travis-ci.org/jonschlinkert/is-extendable)
+
+> Returns true if a value is a plain object, array or function.
+
+## Install
+
+Install with [npm](https://www.npmjs.com/):
+
+```sh
+$ npm install --save is-extendable
+```
+
+## Usage
+
+```js
+var isExtendable = require('is-extendable');
+```
+
+Returns true if the value is any of the following:
+
+* array
+* plain object
+* function
+
+## Notes
+
+All objects in JavaScript can have keys, but it's a pain to check for this, since we ether need to verify that the value is not `null` or `undefined` and:
+
+* the value is not a primitive, or
+* that the object is a plain object, function or array
+
+Also note that an `extendable` object is not the same as an [extensible object](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object/isExtensible), which is one that (in es6) is not sealed, frozen, or marked as non-extensible using `preventExtensions`.
+
+## Release history
+
+### v1.0.0 - 2017/07/20
+
+**Breaking changes**
+
+* No longer considers date, regex or error objects to be extendable
+
+## About
+
+### Related projects
+
+* [assign-deep](https://www.npmjs.com/package/assign-deep): Deeply assign the enumerable properties and/or es6 Symbol properies of source objects to the target… [more](https://github.com/jonschlinkert/assign-deep) | [homepage](https://github.com/jonschlinkert/assign-deep "Deeply assign the enumerable properties and/or es6 Symbol properies of source objects to the target (first) object.")
+* [is-equal-shallow](https://www.npmjs.com/package/is-equal-shallow): Does a shallow comparison of two objects, returning false if the keys or values differ. | [homepage](https://github.com/jonschlinkert/is-equal-shallow "Does a shallow comparison of two objects, returning false if the keys or values differ.")
+* [is-plain-object](https://www.npmjs.com/package/is-plain-object): Returns true if an object was created by the `Object` constructor. | [homepage](https://github.com/jonschlinkert/is-plain-object "Returns true if an object was created by the `Object` constructor.")
+* [isobject](https://www.npmjs.com/package/isobject): Returns true if the value is an object and not an array or null. | [homepage](https://github.com/jonschlinkert/isobject "Returns true if the value is an object and not an array or null.")
+* [kind-of](https://www.npmjs.com/package/kind-of): Get the native type of a value. | [homepage](https://github.com/jonschlinkert/kind-of "Get the native type of a value.")
+
+### Contributing
+
+Pull requests and stars are always welcome. For bugs and feature requests, [please create an issue](../../issues/new).
+
+### Building docs
+
+_(This project's readme.md is generated by [verb](https://github.com/verbose/verb-generate-readme), please don't edit the readme directly. Any changes to the readme must be made in the [.verb.md](.verb.md) readme template.)_
+
+To generate the readme, run the following command:
+
+```sh
+$ npm install -g verbose/verb#dev verb-generate-readme && verb
+```
+
+### Running tests
+
+Running and reviewing unit tests is a great way to get familiarized with a library and its API. You can install dependencies and run tests with the following command:
+
+```sh
+$ npm install && npm test
+```
+
+### Author
+
+**Jon Schlinkert**
+
+* [github/jonschlinkert](https://github.com/jonschlinkert)
+* [twitter/jonschlinkert](https://twitter.com/jonschlinkert)
+
+### License
+
+Copyright © 2017, [Jon Schlinkert](https://github.com/jonschlinkert).
+Released under the [MIT License](LICENSE).
+
+***
+
+_This file was generated by [verb-generate-readme](https://github.com/verbose/verb-generate-readme), v0.6.0, on July 20, 2017._
\ No newline at end of file
diff --git a/node_modules/mixin-deep/node_modules/is-extendable/index.d.ts b/node_modules/mixin-deep/node_modules/is-extendable/index.d.ts
new file mode 100644
index 0000000..b96d507
--- /dev/null
+++ b/node_modules/mixin-deep/node_modules/is-extendable/index.d.ts
@@ -0,0 +1,5 @@
+export = isExtendable;
+
+declare function isExtendable(val: any): boolean;
+
+declare namespace isExtendable {}
diff --git a/node_modules/mixin-deep/node_modules/is-extendable/index.js b/node_modules/mixin-deep/node_modules/is-extendable/index.js
new file mode 100644
index 0000000..a8b26ad
--- /dev/null
+++ b/node_modules/mixin-deep/node_modules/is-extendable/index.js
@@ -0,0 +1,14 @@
+/*!
+ * is-extendable
+ *
+ * Copyright (c) 2015-2017, Jon Schlinkert.
+ * Released under the MIT License.
+ */
+
+'use strict';
+
+var isPlainObject = require('is-plain-object');
+
+module.exports = function isExtendable(val) {
+ return isPlainObject(val) || typeof val === 'function' || Array.isArray(val);
+};
diff --git a/node_modules/mixin-deep/node_modules/is-extendable/package.json b/node_modules/mixin-deep/node_modules/is-extendable/package.json
new file mode 100644
index 0000000..3c2c17a
--- /dev/null
+++ b/node_modules/mixin-deep/node_modules/is-extendable/package.json
@@ -0,0 +1,98 @@
+{
+ "_from": "is-extendable@^1.0.1",
+ "_id": "is-extendable@1.0.1",
+ "_inBundle": false,
+ "_integrity": "sha512-arnXMxT1hhoKo9k1LZdmlNyJdDDfy2v0fXjFlmok4+i8ul/6WlbVge9bhM74OpNPQPMGUToDtz+KXa1PneJxOA==",
+ "_location": "/mixin-deep/is-extendable",
+ "_phantomChildren": {},
+ "_requested": {
+ "type": "range",
+ "registry": true,
+ "raw": "is-extendable@^1.0.1",
+ "name": "is-extendable",
+ "escapedName": "is-extendable",
+ "rawSpec": "^1.0.1",
+ "saveSpec": null,
+ "fetchSpec": "^1.0.1"
+ },
+ "_requiredBy": [
+ "/mixin-deep"
+ ],
+ "_resolved": "https://registry.npmjs.org/is-extendable/-/is-extendable-1.0.1.tgz",
+ "_shasum": "a7470f9e426733d81bd81e1155264e3a3507cab4",
+ "_spec": "is-extendable@^1.0.1",
+ "_where": "/Users/rpatra16/MyProjects/RamAndAntara/node_modules/mixin-deep",
+ "author": {
+ "name": "Jon Schlinkert",
+ "url": "https://github.com/jonschlinkert"
+ },
+ "bugs": {
+ "url": "https://github.com/jonschlinkert/is-extendable/issues"
+ },
+ "bundleDependencies": false,
+ "dependencies": {
+ "is-plain-object": "^2.0.4"
+ },
+ "deprecated": false,
+ "description": "Returns true if a value is a plain object, array or function.",
+ "devDependencies": {
+ "gulp-format-md": "^1.0.0",
+ "mocha": "^3.4.2"
+ },
+ "engines": {
+ "node": ">=0.10.0"
+ },
+ "files": [
+ "index.js",
+ "index.d.ts"
+ ],
+ "homepage": "https://github.com/jonschlinkert/is-extendable",
+ "keywords": [
+ "array",
+ "assign",
+ "check",
+ "date",
+ "extend",
+ "extendable",
+ "extensible",
+ "function",
+ "is",
+ "object",
+ "regex",
+ "test"
+ ],
+ "license": "MIT",
+ "main": "index.js",
+ "name": "is-extendable",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/jonschlinkert/is-extendable.git"
+ },
+ "scripts": {
+ "test": "mocha"
+ },
+ "types": "index.d.ts",
+ "verb": {
+ "related": {
+ "list": [
+ "assign-deep",
+ "is-equal-shallow",
+ "is-plain-object",
+ "isobject",
+ "kind-of"
+ ]
+ },
+ "toc": false,
+ "layout": "default",
+ "tasks": [
+ "readme"
+ ],
+ "plugins": [
+ "gulp-format-md"
+ ],
+ "lint": {
+ "reflinks": true
+ }
+ },
+ "version": "1.0.1"
+}
diff --git a/node_modules/mixin-deep/package.json b/node_modules/mixin-deep/package.json
new file mode 100644
index 0000000..5c2ea7e
--- /dev/null
+++ b/node_modules/mixin-deep/package.json
@@ -0,0 +1,98 @@
+{
+ "_from": "mixin-deep@^1.2.0",
+ "_id": "mixin-deep@1.3.1",
+ "_inBundle": false,
+ "_integrity": "sha512-8ZItLHeEgaqEvd5lYBXfm4EZSFCX29Jb9K+lAHhDKzReKBQKj3R+7NOF6tjqYi9t4oI8VUfaWITJQm86wnXGNQ==",
+ "_location": "/mixin-deep",
+ "_phantomChildren": {
+ "is-plain-object": "2.0.4"
+ },
+ "_requested": {
+ "type": "range",
+ "registry": true,
+ "raw": "mixin-deep@^1.2.0",
+ "name": "mixin-deep",
+ "escapedName": "mixin-deep",
+ "rawSpec": "^1.2.0",
+ "saveSpec": null,
+ "fetchSpec": "^1.2.0"
+ },
+ "_requiredBy": [
+ "/base"
+ ],
+ "_resolved": "https://registry.npmjs.org/mixin-deep/-/mixin-deep-1.3.1.tgz",
+ "_shasum": "a49e7268dce1a0d9698e45326c5626df3543d0fe",
+ "_spec": "mixin-deep@^1.2.0",
+ "_where": "/Users/rpatra16/MyProjects/RamAndAntara/node_modules/base",
+ "author": {
+ "name": "Jon Schlinkert",
+ "url": "https://github.com/jonschlinkert"
+ },
+ "bugs": {
+ "url": "https://github.com/jonschlinkert/mixin-deep/issues"
+ },
+ "bundleDependencies": false,
+ "dependencies": {
+ "for-in": "^1.0.2",
+ "is-extendable": "^1.0.1"
+ },
+ "deprecated": false,
+ "description": "Deeply mix the properties of objects into the first object. Like merge-deep, but doesn't clone.",
+ "devDependencies": {
+ "gulp-format-md": "^1.0.0",
+ "mocha": "^3.5.3",
+ "should": "^13.1.3"
+ },
+ "engines": {
+ "node": ">=0.10.0"
+ },
+ "files": [
+ "index.js"
+ ],
+ "homepage": "https://github.com/jonschlinkert/mixin-deep",
+ "keywords": [
+ "deep",
+ "extend",
+ "key",
+ "keys",
+ "merge",
+ "mixin",
+ "object",
+ "prop",
+ "properties",
+ "util",
+ "values"
+ ],
+ "license": "MIT",
+ "main": "index.js",
+ "name": "mixin-deep",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/jonschlinkert/mixin-deep.git"
+ },
+ "scripts": {
+ "test": "mocha"
+ },
+ "verb": {
+ "toc": false,
+ "layout": "default",
+ "tasks": [
+ "readme"
+ ],
+ "plugins": [
+ "gulp-format-md"
+ ],
+ "related": {
+ "list": [
+ "defaults-deep",
+ "extend-shallow",
+ "merge-deep",
+ "mixin-object"
+ ]
+ },
+ "lint": {
+ "reflinks": true
+ }
+ },
+ "version": "1.3.1"
+}
diff --git a/node_modules/mkdirp/.travis.yml b/node_modules/mkdirp/.travis.yml
new file mode 100644
index 0000000..74c57bf
--- /dev/null
+++ b/node_modules/mkdirp/.travis.yml
@@ -0,0 +1,8 @@
+language: node_js
+node_js:
+ - "0.8"
+ - "0.10"
+ - "0.12"
+ - "iojs"
+before_install:
+ - npm install -g npm@~1.4.6
diff --git a/node_modules/mkdirp/LICENSE b/node_modules/mkdirp/LICENSE
new file mode 100644
index 0000000..432d1ae
--- /dev/null
+++ b/node_modules/mkdirp/LICENSE
@@ -0,0 +1,21 @@
+Copyright 2010 James Halliday (mail@substack.net)
+
+This project is free software released under the MIT/X11 license:
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in
+all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
+THE SOFTWARE.
diff --git a/node_modules/mkdirp/bin/cmd.js b/node_modules/mkdirp/bin/cmd.js
new file mode 100755
index 0000000..d95de15
--- /dev/null
+++ b/node_modules/mkdirp/bin/cmd.js
@@ -0,0 +1,33 @@
+#!/usr/bin/env node
+
+var mkdirp = require('../');
+var minimist = require('minimist');
+var fs = require('fs');
+
+var argv = minimist(process.argv.slice(2), {
+ alias: { m: 'mode', h: 'help' },
+ string: [ 'mode' ]
+});
+if (argv.help) {
+ fs.createReadStream(__dirname + '/usage.txt').pipe(process.stdout);
+ return;
+}
+
+var paths = argv._.slice();
+var mode = argv.mode ? parseInt(argv.mode, 8) : undefined;
+
+(function next () {
+ if (paths.length === 0) return;
+ var p = paths.shift();
+
+ if (mode === undefined) mkdirp(p, cb)
+ else mkdirp(p, mode, cb)
+
+ function cb (err) {
+ if (err) {
+ console.error(err.message);
+ process.exit(1);
+ }
+ else next();
+ }
+})();
diff --git a/node_modules/mkdirp/bin/usage.txt b/node_modules/mkdirp/bin/usage.txt
new file mode 100644
index 0000000..f952aa2
--- /dev/null
+++ b/node_modules/mkdirp/bin/usage.txt
@@ -0,0 +1,12 @@
+usage: mkdirp [DIR1,DIR2..] {OPTIONS}
+
+ Create each supplied directory including any necessary parent directories that
+ don't yet exist.
+
+ If the directory already exists, do nothing.
+
+OPTIONS are:
+
+ -m, --mode If a directory needs to be created, set the mode as an octal
+ permission string.
+
diff --git a/node_modules/mkdirp/examples/pow.js b/node_modules/mkdirp/examples/pow.js
new file mode 100644
index 0000000..e692421
--- /dev/null
+++ b/node_modules/mkdirp/examples/pow.js
@@ -0,0 +1,6 @@
+var mkdirp = require('mkdirp');
+
+mkdirp('/tmp/foo/bar/baz', function (err) {
+ if (err) console.error(err)
+ else console.log('pow!')
+});
diff --git a/node_modules/mkdirp/index.js b/node_modules/mkdirp/index.js
new file mode 100644
index 0000000..6ce241b
--- /dev/null
+++ b/node_modules/mkdirp/index.js
@@ -0,0 +1,98 @@
+var path = require('path');
+var fs = require('fs');
+var _0777 = parseInt('0777', 8);
+
+module.exports = mkdirP.mkdirp = mkdirP.mkdirP = mkdirP;
+
+function mkdirP (p, opts, f, made) {
+ if (typeof opts === 'function') {
+ f = opts;
+ opts = {};
+ }
+ else if (!opts || typeof opts !== 'object') {
+ opts = { mode: opts };
+ }
+
+ var mode = opts.mode;
+ var xfs = opts.fs || fs;
+
+ if (mode === undefined) {
+ mode = _0777 & (~process.umask());
+ }
+ if (!made) made = null;
+
+ var cb = f || function () {};
+ p = path.resolve(p);
+
+ xfs.mkdir(p, mode, function (er) {
+ if (!er) {
+ made = made || p;
+ return cb(null, made);
+ }
+ switch (er.code) {
+ case 'ENOENT':
+ mkdirP(path.dirname(p), opts, function (er, made) {
+ if (er) cb(er, made);
+ else mkdirP(p, opts, cb, made);
+ });
+ break;
+
+ // In the case of any other error, just see if there's a dir
+ // there already. If so, then hooray! If not, then something
+ // is borked.
+ default:
+ xfs.stat(p, function (er2, stat) {
+ // if the stat fails, then that's super weird.
+ // let the original error be the failure reason.
+ if (er2 || !stat.isDirectory()) cb(er, made)
+ else cb(null, made);
+ });
+ break;
+ }
+ });
+}
+
+mkdirP.sync = function sync (p, opts, made) {
+ if (!opts || typeof opts !== 'object') {
+ opts = { mode: opts };
+ }
+
+ var mode = opts.mode;
+ var xfs = opts.fs || fs;
+
+ if (mode === undefined) {
+ mode = _0777 & (~process.umask());
+ }
+ if (!made) made = null;
+
+ p = path.resolve(p);
+
+ try {
+ xfs.mkdirSync(p, mode);
+ made = made || p;
+ }
+ catch (err0) {
+ switch (err0.code) {
+ case 'ENOENT' :
+ made = sync(path.dirname(p), opts, made);
+ sync(p, opts, made);
+ break;
+
+ // In the case of any other error, just see if there's a dir
+ // there already. If so, then hooray! If not, then something
+ // is borked.
+ default:
+ var stat;
+ try {
+ stat = xfs.statSync(p);
+ }
+ catch (err1) {
+ throw err0;
+ }
+ if (!stat.isDirectory()) throw err0;
+ break;
+ }
+ }
+
+ return made;
+};
diff --git a/node_modules/mkdirp/node_modules/minimist/.travis.yml b/node_modules/mkdirp/node_modules/minimist/.travis.yml
new file mode 100644
index 0000000..cc4dba2
--- /dev/null
+++ b/node_modules/mkdirp/node_modules/minimist/.travis.yml
@@ -0,0 +1,4 @@
+language: node_js
+node_js:
+ - "0.8"
+ - "0.10"
diff --git a/node_modules/mkdirp/node_modules/minimist/LICENSE b/node_modules/mkdirp/node_modules/minimist/LICENSE
new file mode 100644
index 0000000..ee27ba4
--- /dev/null
+++ b/node_modules/mkdirp/node_modules/minimist/LICENSE
@@ -0,0 +1,18 @@
+This software is released under the MIT license:
+
+Permission is hereby granted, free of charge, to any person obtaining a copy of
+this software and associated documentation files (the "Software"), to deal in
+the Software without restriction, including without limitation the rights to
+use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of
+the Software, and to permit persons to whom the Software is furnished to do so,
+subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in all
+copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS
+FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR
+COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER
+IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN
+CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
diff --git a/node_modules/mkdirp/node_modules/minimist/example/parse.js b/node_modules/mkdirp/node_modules/minimist/example/parse.js
new file mode 100644
index 0000000..abff3e8
--- /dev/null
+++ b/node_modules/mkdirp/node_modules/minimist/example/parse.js
@@ -0,0 +1,2 @@
+var argv = require('../')(process.argv.slice(2));
+console.dir(argv);
diff --git a/node_modules/mkdirp/node_modules/minimist/index.js b/node_modules/mkdirp/node_modules/minimist/index.js
new file mode 100644
index 0000000..584f551
--- /dev/null
+++ b/node_modules/mkdirp/node_modules/minimist/index.js
@@ -0,0 +1,187 @@
+module.exports = function (args, opts) {
+ if (!opts) opts = {};
+
+ var flags = { bools : {}, strings : {} };
+
+ [].concat(opts['boolean']).filter(Boolean).forEach(function (key) {
+ flags.bools[key] = true;
+ });
+
+ [].concat(opts.string).filter(Boolean).forEach(function (key) {
+ flags.strings[key] = true;
+ });
+
+ var aliases = {};
+ Object.keys(opts.alias || {}).forEach(function (key) {
+ aliases[key] = [].concat(opts.alias[key]);
+ aliases[key].forEach(function (x) {
+ aliases[x] = [key].concat(aliases[key].filter(function (y) {
+ return x !== y;
+ }));
+ });
+ });
+
+ var defaults = opts['default'] || {};
+
+ var argv = { _ : [] };
+ Object.keys(flags.bools).forEach(function (key) {
+ setArg(key, defaults[key] === undefined ? false : defaults[key]);
+ });
+
+ var notFlags = [];
+
+ if (args.indexOf('--') !== -1) {
+ notFlags = args.slice(args.indexOf('--')+1);
+ args = args.slice(0, args.indexOf('--'));
+ }
+
+ function setArg (key, val) {
+ var value = !flags.strings[key] && isNumber(val)
+ ? Number(val) : val
+ ;
+ setKey(argv, key.split('.'), value);
+
+ (aliases[key] || []).forEach(function (x) {
+ setKey(argv, x.split('.'), value);
+ });
+ }
+
+ for (var i = 0; i < args.length; i++) {
+ var arg = args[i];
+
+ if (/^--.+=/.test(arg)) {
+ // Using [\s\S] instead of . because js doesn't support the
+ // 'dotall' regex modifier. See:
+ // http://stackoverflow.com/a/1068308/13216
+ var m = arg.match(/^--([^=]+)=([\s\S]*)$/);
+ setArg(m[1], m[2]);
+ }
+ else if (/^--no-.+/.test(arg)) {
+ var key = arg.match(/^--no-(.+)/)[1];
+ setArg(key, false);
+ }
+ else if (/^--.+/.test(arg)) {
+ var key = arg.match(/^--(.+)/)[1];
+ var next = args[i + 1];
+ if (next !== undefined && !/^-/.test(next)
+ && !flags.bools[key]
+ && (aliases[key] ? !flags.bools[aliases[key]] : true)) {
+ setArg(key, next);
+ i++;
+ }
+ else if (/^(true|false)$/.test(next)) {
+ setArg(key, next === 'true');
+ i++;
+ }
+ else {
+ setArg(key, flags.strings[key] ? '' : true);
+ }
+ }
+ else if (/^-[^-]+/.test(arg)) {
+ var letters = arg.slice(1,-1).split('');
+
+ var broken = false;
+ for (var j = 0; j < letters.length; j++) {
+ var next = arg.slice(j+2);
+
+ if (next === '-') {
+ setArg(letters[j], next)
+ continue;
+ }
+
+ if (/[A-Za-z]/.test(letters[j])
+ && /-?\d+(\.\d*)?(e-?\d+)?$/.test(next)) {
+ setArg(letters[j], next);
+ broken = true;
+ break;
+ }
+
+ if (letters[j+1] && letters[j+1].match(/\W/)) {
+ setArg(letters[j], arg.slice(j+2));
+ broken = true;
+ break;
+ }
+ else {
+ setArg(letters[j], flags.strings[letters[j]] ? '' : true);
+ }
+ }
+
+ var key = arg.slice(-1)[0];
+ if (!broken && key !== '-') {
+ if (args[i+1] && !/^(-|--)[^-]/.test(args[i+1])
+ && !flags.bools[key]
+ && (aliases[key] ? !flags.bools[aliases[key]] : true)) {
+ setArg(key, args[i+1]);
+ i++;
+ }
+ else if (args[i+1] && /true|false/.test(args[i+1])) {
+ setArg(key, args[i+1] === 'true');
+ i++;
+ }
+ else {
+ setArg(key, flags.strings[key] ? '' : true);
+ }
+ }
+ }
+ else {
+ argv._.push(
+ flags.strings['_'] || !isNumber(arg) ? arg : Number(arg)
+ );
+ }
+ }
+
+ Object.keys(defaults).forEach(function (key) {
+ if (!hasKey(argv, key.split('.'))) {
+ setKey(argv, key.split('.'), defaults[key]);
+
+ (aliases[key] || []).forEach(function (x) {
+ setKey(argv, x.split('.'), defaults[key]);
+ });
+ }
+ });
+
+ notFlags.forEach(function(key) {
+ argv._.push(key);
+ });
+
+ return argv;
+};
+
+function hasKey (obj, keys) {
+ var o = obj;
+ keys.slice(0,-1).forEach(function (key) {
+ o = (o[key] || {});
+ });
+
+ var key = keys[keys.length - 1];
+ return key in o;
+}
+
+function setKey (obj, keys, value) {
+ var o = obj;
+ keys.slice(0,-1).forEach(function (key) {
+ if (o[key] === undefined) o[key] = {};
+ o = o[key];
+ });
+
+ var key = keys[keys.length - 1];
+ if (o[key] === undefined || typeof o[key] === 'boolean') {
+ o[key] = value;
+ }
+ else if (Array.isArray(o[key])) {
+ o[key].push(value);
+ }
+ else {
+ o[key] = [ o[key], value ];
+ }
+}
+
+function isNumber (x) {
+ if (typeof x === 'number') return true;
+ if (/^0x[0-9a-f]+$/i.test(x)) return true;
+ return /^[-+]?(?:\d+(?:\.\d*)?|\.\d+)(e[-+]?\d+)?$/.test(x);
+}
+
+function longest (xs) {
+ return Math.max.apply(null, xs.map(function (x) { return x.length }));
+}
diff --git a/node_modules/mkdirp/node_modules/minimist/package.json b/node_modules/mkdirp/node_modules/minimist/package.json
new file mode 100644
index 0000000..86ea510
--- /dev/null
+++ b/node_modules/mkdirp/node_modules/minimist/package.json
@@ -0,0 +1,71 @@
+{
+ "_from": "minimist@0.0.8",
+ "_id": "minimist@0.0.8",
+ "_inBundle": false,
+ "_integrity": "sha1-hX/Kv8M5fSYluCKCYuhqp6ARsF0=",
+ "_location": "/mkdirp/minimist",
+ "_phantomChildren": {},
+ "_requested": {
+ "type": "version",
+ "registry": true,
+ "raw": "minimist@0.0.8",
+ "name": "minimist",
+ "escapedName": "minimist",
+ "rawSpec": "0.0.8",
+ "saveSpec": null,
+ "fetchSpec": "0.0.8"
+ },
+ "_requiredBy": [
+ "/mkdirp"
+ ],
+ "_resolved": "http://registry.npmjs.org/minimist/-/minimist-0.0.8.tgz",
+ "_shasum": "857fcabfc3397d2625b8228262e86aa7a011b05d",
+ "_spec": "minimist@0.0.8",
+ "_where": "/Users/rpatra16/MyProjects/RamAndAntara/node_modules/mkdirp",
+ "author": {
+ "name": "James Halliday",
+ "email": "mail@substack.net",
+ "url": "http://substack.net"
+ },
+ "bugs": {
+ "url": "https://github.com/substack/minimist/issues"
+ },
+ "bundleDependencies": false,
+ "deprecated": false,
+ "description": "parse argument options",
+ "devDependencies": {
+ "tap": "~0.4.0",
+ "tape": "~1.0.4"
+ },
+ "homepage": "https://github.com/substack/minimist",
+ "keywords": [
+ "argv",
+ "getopt",
+ "parser",
+ "optimist"
+ ],
+ "license": "MIT",
+ "main": "index.js",
+ "name": "minimist",
+ "repository": {
+ "type": "git",
+ "url": "git://github.com/substack/minimist.git"
+ },
+ "scripts": {
+ "test": "tap test/*.js"
+ },
+ "testling": {
+ "files": "test/*.js",
+ "browsers": [
+ "ie/6..latest",
+ "ff/5",
+ "firefox/latest",
+ "chrome/10",
+ "chrome/latest",
+ "safari/5.1",
+ "safari/latest",
+ "opera/12"
+ ]
+ },
+ "version": "0.0.8"
+}
diff --git a/node_modules/mkdirp/node_modules/minimist/readme.markdown b/node_modules/mkdirp/node_modules/minimist/readme.markdown
new file mode 100644
index 0000000..c256353
--- /dev/null
+++ b/node_modules/mkdirp/node_modules/minimist/readme.markdown
@@ -0,0 +1,73 @@
+# minimist
+
+parse argument options
+
+This module is the guts of optimist's argument parser without all the
+fanciful decoration.
+
+[](http://ci.testling.com/substack/minimist)
+
+[](http://travis-ci.org/substack/minimist)
+
+# example
+
+``` js
+var argv = require('minimist')(process.argv.slice(2));
+console.dir(argv);
+```
+
+```
+$ node example/parse.js -a beep -b boop
+{ _: [], a: 'beep', b: 'boop' }
+```
+
+```
+$ node example/parse.js -x 3 -y 4 -n5 -abc --beep=boop foo bar baz
+{ _: [ 'foo', 'bar', 'baz' ],
+ x: 3,
+ y: 4,
+ n: 5,
+ a: true,
+ b: true,
+ c: true,
+ beep: 'boop' }
+```
+
+# methods
+
+``` js
+var parseArgs = require('minimist')
+```
+
+## var argv = parseArgs(args, opts={})
+
+Return an argument object `argv` populated with the array arguments from `args`.
+
+`argv._` contains all the arguments that didn't have an option associated with
+them.
+
+Numeric-looking arguments will be returned as numbers unless `opts.string` or
+`opts.boolean` is set for that argument name.
+
+Any arguments after `'--'` will not be parsed and will end up in `argv._`.
+
+options can be:
+
+* `opts.string` - a string or array of strings argument names to always treat as
+strings
+* `opts.boolean` - a string or array of strings to always treat as booleans
+* `opts.alias` - an object mapping string names to strings or arrays of string
+argument names to use as aliases
+* `opts.default` - an object mapping string argument names to default values
+
+# install
+
+With [npm](https://npmjs.org) do:
+
+```
+npm install minimist
+```
+
+# license
+
+MIT
diff --git a/node_modules/mkdirp/node_modules/minimist/test/dash.js b/node_modules/mkdirp/node_modules/minimist/test/dash.js
new file mode 100644
index 0000000..8b034b9
--- /dev/null
+++ b/node_modules/mkdirp/node_modules/minimist/test/dash.js
@@ -0,0 +1,24 @@
+var parse = require('../');
+var test = require('tape');
+
+test('-', function (t) {
+ t.plan(5);
+ t.deepEqual(parse([ '-n', '-' ]), { n: '-', _: [] });
+ t.deepEqual(parse([ '-' ]), { _: [ '-' ] });
+ t.deepEqual(parse([ '-f-' ]), { f: '-', _: [] });
+ t.deepEqual(
+ parse([ '-b', '-' ], { boolean: 'b' }),
+ { b: true, _: [ '-' ] }
+ );
+ t.deepEqual(
+ parse([ '-s', '-' ], { string: 's' }),
+ { s: '-', _: [] }
+ );
+});
+
+test('-a -- b', function (t) {
+ t.plan(3);
+ t.deepEqual(parse([ '-a', '--', 'b' ]), { a: true, _: [ 'b' ] });
+ t.deepEqual(parse([ '--a', '--', 'b' ]), { a: true, _: [ 'b' ] });
+ t.deepEqual(parse([ '--a', '--', 'b' ]), { a: true, _: [ 'b' ] });
+});
diff --git a/node_modules/mkdirp/node_modules/minimist/test/default_bool.js b/node_modules/mkdirp/node_modules/minimist/test/default_bool.js
new file mode 100644
index 0000000..f0041ee
--- /dev/null
+++ b/node_modules/mkdirp/node_modules/minimist/test/default_bool.js
@@ -0,0 +1,20 @@
+var test = require('tape');
+var parse = require('../');
+
+test('boolean default true', function (t) {
+ var argv = parse([], {
+ boolean: 'sometrue',
+ default: { sometrue: true }
+ });
+ t.equal(argv.sometrue, true);
+ t.end();
+});
+
+test('boolean default false', function (t) {
+ var argv = parse([], {
+ boolean: 'somefalse',
+ default: { somefalse: false }
+ });
+ t.equal(argv.somefalse, false);
+ t.end();
+});
diff --git a/node_modules/mkdirp/node_modules/minimist/test/dotted.js b/node_modules/mkdirp/node_modules/minimist/test/dotted.js
new file mode 100644
index 0000000..ef0ae34
--- /dev/null
+++ b/node_modules/mkdirp/node_modules/minimist/test/dotted.js
@@ -0,0 +1,16 @@
+var parse = require('../');
+var test = require('tape');
+
+test('dotted alias', function (t) {
+ var argv = parse(['--a.b', '22'], {default: {'a.b': 11}, alias: {'a.b': 'aa.bb'}});
+ t.equal(argv.a.b, 22);
+ t.equal(argv.aa.bb, 22);
+ t.end();
+});
+
+test('dotted default', function (t) {
+ var argv = parse('', {default: {'a.b': 11}, alias: {'a.b': 'aa.bb'}});
+ t.equal(argv.a.b, 11);
+ t.equal(argv.aa.bb, 11);
+ t.end();
+});
diff --git a/node_modules/mkdirp/node_modules/minimist/test/long.js b/node_modules/mkdirp/node_modules/minimist/test/long.js
new file mode 100644
index 0000000..5d3a1e0
--- /dev/null
+++ b/node_modules/mkdirp/node_modules/minimist/test/long.js
@@ -0,0 +1,31 @@
+var test = require('tape');
+var parse = require('../');
+
+test('long opts', function (t) {
+ t.deepEqual(
+ parse([ '--bool' ]),
+ { bool : true, _ : [] },
+ 'long boolean'
+ );
+ t.deepEqual(
+ parse([ '--pow', 'xixxle' ]),
+ { pow : 'xixxle', _ : [] },
+ 'long capture sp'
+ );
+ t.deepEqual(
+ parse([ '--pow=xixxle' ]),
+ { pow : 'xixxle', _ : [] },
+ 'long capture eq'
+ );
+ t.deepEqual(
+ parse([ '--host', 'localhost', '--port', '555' ]),
+ { host : 'localhost', port : 555, _ : [] },
+ 'long captures sp'
+ );
+ t.deepEqual(
+ parse([ '--host=localhost', '--port=555' ]),
+ { host : 'localhost', port : 555, _ : [] },
+ 'long captures eq'
+ );
+ t.end();
+});
diff --git a/node_modules/mkdirp/node_modules/minimist/test/parse.js b/node_modules/mkdirp/node_modules/minimist/test/parse.js
new file mode 100644
index 0000000..8a90646
--- /dev/null
+++ b/node_modules/mkdirp/node_modules/minimist/test/parse.js
@@ -0,0 +1,318 @@
+var parse = require('../');
+var test = require('tape');
+
+test('parse args', function (t) {
+ t.deepEqual(
+ parse([ '--no-moo' ]),
+ { moo : false, _ : [] },
+ 'no'
+ );
+ t.deepEqual(
+ parse([ '-v', 'a', '-v', 'b', '-v', 'c' ]),
+ { v : ['a','b','c'], _ : [] },
+ 'multi'
+ );
+ t.end();
+});
+
+test('comprehensive', function (t) {
+ t.deepEqual(
+ parse([
+ '--name=meowmers', 'bare', '-cats', 'woo',
+ '-h', 'awesome', '--multi=quux',
+ '--key', 'value',
+ '-b', '--bool', '--no-meep', '--multi=baz',
+ '--', '--not-a-flag', 'eek'
+ ]),
+ {
+ c : true,
+ a : true,
+ t : true,
+ s : 'woo',
+ h : 'awesome',
+ b : true,
+ bool : true,
+ key : 'value',
+ multi : [ 'quux', 'baz' ],
+ meep : false,
+ name : 'meowmers',
+ _ : [ 'bare', '--not-a-flag', 'eek' ]
+ }
+ );
+ t.end();
+});
+
+test('nums', function (t) {
+ var argv = parse([
+ '-x', '1234',
+ '-y', '5.67',
+ '-z', '1e7',
+ '-w', '10f',
+ '--hex', '0xdeadbeef',
+ '789'
+ ]);
+ t.deepEqual(argv, {
+ x : 1234,
+ y : 5.67,
+ z : 1e7,
+ w : '10f',
+ hex : 0xdeadbeef,
+ _ : [ 789 ]
+ });
+ t.deepEqual(typeof argv.x, 'number');
+ t.deepEqual(typeof argv.y, 'number');
+ t.deepEqual(typeof argv.z, 'number');
+ t.deepEqual(typeof argv.w, 'string');
+ t.deepEqual(typeof argv.hex, 'number');
+ t.deepEqual(typeof argv._[0], 'number');
+ t.end();
+});
+
+test('flag boolean', function (t) {
+ var argv = parse([ '-t', 'moo' ], { boolean: 't' });
+ t.deepEqual(argv, { t : true, _ : [ 'moo' ] });
+ t.deepEqual(typeof argv.t, 'boolean');
+ t.end();
+});
+
+test('flag boolean value', function (t) {
+ var argv = parse(['--verbose', 'false', 'moo', '-t', 'true'], {
+ boolean: [ 't', 'verbose' ],
+ default: { verbose: true }
+ });
+
+ t.deepEqual(argv, {
+ verbose: false,
+ t: true,
+ _: ['moo']
+ });
+
+ t.deepEqual(typeof argv.verbose, 'boolean');
+ t.deepEqual(typeof argv.t, 'boolean');
+ t.end();
+});
+
+test('flag boolean default false', function (t) {
+ var argv = parse(['moo'], {
+ boolean: ['t', 'verbose'],
+ default: { verbose: false, t: false }
+ });
+
+ t.deepEqual(argv, {
+ verbose: false,
+ t: false,
+ _: ['moo']
+ });
+
+ t.deepEqual(typeof argv.verbose, 'boolean');
+ t.deepEqual(typeof argv.t, 'boolean');
+ t.end();
+
+});
+
+test('boolean groups', function (t) {
+ var argv = parse([ '-x', '-z', 'one', 'two', 'three' ], {
+ boolean: ['x','y','z']
+ });
+
+ t.deepEqual(argv, {
+ x : true,
+ y : false,
+ z : true,
+ _ : [ 'one', 'two', 'three' ]
+ });
+
+ t.deepEqual(typeof argv.x, 'boolean');
+ t.deepEqual(typeof argv.y, 'boolean');
+ t.deepEqual(typeof argv.z, 'boolean');
+ t.end();
+});
+
+test('newlines in params' , function (t) {
+ var args = parse([ '-s', "X\nX" ])
+ t.deepEqual(args, { _ : [], s : "X\nX" });
+
+ // reproduce in bash:
+ // VALUE="new
+ // line"
+ // node program.js --s="$VALUE"
+ args = parse([ "--s=X\nX" ])
+ t.deepEqual(args, { _ : [], s : "X\nX" });
+ t.end();
+});
+
+test('strings' , function (t) {
+ var s = parse([ '-s', '0001234' ], { string: 's' }).s;
+ t.equal(s, '0001234');
+ t.equal(typeof s, 'string');
+
+ var x = parse([ '-x', '56' ], { string: 'x' }).x;
+ t.equal(x, '56');
+ t.equal(typeof x, 'string');
+ t.end();
+});
+
+test('stringArgs', function (t) {
+ var s = parse([ ' ', ' ' ], { string: '_' })._;
+ t.same(s.length, 2);
+ t.same(typeof s[0], 'string');
+ t.same(s[0], ' ');
+ t.same(typeof s[1], 'string');
+ t.same(s[1], ' ');
+ t.end();
+});
+
+test('empty strings', function(t) {
+ var s = parse([ '-s' ], { string: 's' }).s;
+ t.equal(s, '');
+ t.equal(typeof s, 'string');
+
+ var str = parse([ '--str' ], { string: 'str' }).str;
+ t.equal(str, '');
+ t.equal(typeof str, 'string');
+
+ var letters = parse([ '-art' ], {
+ string: [ 'a', 't' ]
+ });
+
+ t.equal(letters.a, '');
+ t.equal(letters.r, true);
+ t.equal(letters.t, '');
+
+ t.end();
+});
+
+
+test('slashBreak', function (t) {
+ t.same(
+ parse([ '-I/foo/bar/baz' ]),
+ { I : '/foo/bar/baz', _ : [] }
+ );
+ t.same(
+ parse([ '-xyz/foo/bar/baz' ]),
+ { x : true, y : true, z : '/foo/bar/baz', _ : [] }
+ );
+ t.end();
+});
+
+test('alias', function (t) {
+ var argv = parse([ '-f', '11', '--zoom', '55' ], {
+ alias: { z: 'zoom' }
+ });
+ t.equal(argv.zoom, 55);
+ t.equal(argv.z, argv.zoom);
+ t.equal(argv.f, 11);
+ t.end();
+});
+
+test('multiAlias', function (t) {
+ var argv = parse([ '-f', '11', '--zoom', '55' ], {
+ alias: { z: [ 'zm', 'zoom' ] }
+ });
+ t.equal(argv.zoom, 55);
+ t.equal(argv.z, argv.zoom);
+ t.equal(argv.z, argv.zm);
+ t.equal(argv.f, 11);
+ t.end();
+});
+
+test('nested dotted objects', function (t) {
+ var argv = parse([
+ '--foo.bar', '3', '--foo.baz', '4',
+ '--foo.quux.quibble', '5', '--foo.quux.o_O',
+ '--beep.boop'
+ ]);
+
+ t.same(argv.foo, {
+ bar : 3,
+ baz : 4,
+ quux : {
+ quibble : 5,
+ o_O : true
+ }
+ });
+ t.same(argv.beep, { boop : true });
+ t.end();
+});
+
+test('boolean and alias with chainable api', function (t) {
+ var aliased = [ '-h', 'derp' ];
+ var regular = [ '--herp', 'derp' ];
+ var opts = {
+ herp: { alias: 'h', boolean: true }
+ };
+ var aliasedArgv = parse(aliased, {
+ boolean: 'herp',
+ alias: { h: 'herp' }
+ });
+ var propertyArgv = parse(regular, {
+ boolean: 'herp',
+ alias: { h: 'herp' }
+ });
+ var expected = {
+ herp: true,
+ h: true,
+ '_': [ 'derp' ]
+ };
+
+ t.same(aliasedArgv, expected);
+ t.same(propertyArgv, expected);
+ t.end();
+});
+
+test('boolean and alias with options hash', function (t) {
+ var aliased = [ '-h', 'derp' ];
+ var regular = [ '--herp', 'derp' ];
+ var opts = {
+ alias: { 'h': 'herp' },
+ boolean: 'herp'
+ };
+ var aliasedArgv = parse(aliased, opts);
+ var propertyArgv = parse(regular, opts);
+ var expected = {
+ herp: true,
+ h: true,
+ '_': [ 'derp' ]
+ };
+ t.same(aliasedArgv, expected);
+ t.same(propertyArgv, expected);
+ t.end();
+});
+
+test('boolean and alias using explicit true', function (t) {
+ var aliased = [ '-h', 'true' ];
+ var regular = [ '--herp', 'true' ];
+ var opts = {
+ alias: { h: 'herp' },
+ boolean: 'h'
+ };
+ var aliasedArgv = parse(aliased, opts);
+ var propertyArgv = parse(regular, opts);
+ var expected = {
+ herp: true,
+ h: true,
+ '_': [ ]
+ };
+
+ t.same(aliasedArgv, expected);
+ t.same(propertyArgv, expected);
+ t.end();
+});
+
+// regression, see https://github.com/substack/node-optimist/issues/71
+test('boolean and --x=true', function(t) {
+ var parsed = parse(['--boool', '--other=true'], {
+ boolean: 'boool'
+ });
+
+ t.same(parsed.boool, true);
+ t.same(parsed.other, 'true');
+
+ parsed = parse(['--boool', '--other=false'], {
+ boolean: 'boool'
+ });
+
+ t.same(parsed.boool, true);
+ t.same(parsed.other, 'false');
+ t.end();
+});
diff --git a/node_modules/mkdirp/node_modules/minimist/test/parse_modified.js b/node_modules/mkdirp/node_modules/minimist/test/parse_modified.js
new file mode 100644
index 0000000..21851b0
--- /dev/null
+++ b/node_modules/mkdirp/node_modules/minimist/test/parse_modified.js
@@ -0,0 +1,9 @@
+var parse = require('../');
+var test = require('tape');
+
+test('parse with modifier functions' , function (t) {
+ t.plan(1);
+
+ var argv = parse([ '-b', '123' ], { boolean: 'b' });
+ t.deepEqual(argv, { b: true, _: ['123'] });
+});
diff --git a/node_modules/mkdirp/node_modules/minimist/test/short.js b/node_modules/mkdirp/node_modules/minimist/test/short.js
new file mode 100644
index 0000000..d513a1c
--- /dev/null
+++ b/node_modules/mkdirp/node_modules/minimist/test/short.js
@@ -0,0 +1,67 @@
+var parse = require('../');
+var test = require('tape');
+
+test('numeric short args', function (t) {
+ t.plan(2);
+ t.deepEqual(parse([ '-n123' ]), { n: 123, _: [] });
+ t.deepEqual(
+ parse([ '-123', '456' ]),
+ { 1: true, 2: true, 3: 456, _: [] }
+ );
+});
+
+test('short', function (t) {
+ t.deepEqual(
+ parse([ '-b' ]),
+ { b : true, _ : [] },
+ 'short boolean'
+ );
+ t.deepEqual(
+ parse([ 'foo', 'bar', 'baz' ]),
+ { _ : [ 'foo', 'bar', 'baz' ] },
+ 'bare'
+ );
+ t.deepEqual(
+ parse([ '-cats' ]),
+ { c : true, a : true, t : true, s : true, _ : [] },
+ 'group'
+ );
+ t.deepEqual(
+ parse([ '-cats', 'meow' ]),
+ { c : true, a : true, t : true, s : 'meow', _ : [] },
+ 'short group next'
+ );
+ t.deepEqual(
+ parse([ '-h', 'localhost' ]),
+ { h : 'localhost', _ : [] },
+ 'short capture'
+ );
+ t.deepEqual(
+ parse([ '-h', 'localhost', '-p', '555' ]),
+ { h : 'localhost', p : 555, _ : [] },
+ 'short captures'
+ );
+ t.end();
+});
+
+test('mixed short bool and capture', function (t) {
+ t.same(
+ parse([ '-h', 'localhost', '-fp', '555', 'script.js' ]),
+ {
+ f : true, p : 555, h : 'localhost',
+ _ : [ 'script.js' ]
+ }
+ );
+ t.end();
+});
+
+test('short and long', function (t) {
+ t.deepEqual(
+ parse([ '-h', 'localhost', '-fp', '555', 'script.js' ]),
+ {
+ f : true, p : 555, h : 'localhost',
+ _ : [ 'script.js' ]
+ }
+ );
+ t.end();
+});
diff --git a/node_modules/mkdirp/node_modules/minimist/test/whitespace.js b/node_modules/mkdirp/node_modules/minimist/test/whitespace.js
new file mode 100644
index 0000000..8a52a58
--- /dev/null
+++ b/node_modules/mkdirp/node_modules/minimist/test/whitespace.js
@@ -0,0 +1,8 @@
+var parse = require('../');
+var test = require('tape');
+
+test('whitespace should be whitespace' , function (t) {
+ t.plan(1);
+ var x = parse([ '-x', '\t' ]).x;
+ t.equal(x, '\t');
+});
diff --git a/node_modules/mkdirp/package.json b/node_modules/mkdirp/package.json
new file mode 100644
index 0000000..9ba9149
--- /dev/null
+++ b/node_modules/mkdirp/package.json
@@ -0,0 +1,65 @@
+{
+ "_from": "mkdirp@0.5.1",
+ "_id": "mkdirp@0.5.1",
+ "_inBundle": false,
+ "_integrity": "sha1-MAV0OOrGz3+MR2fzhkjWaX11yQM=",
+ "_location": "/mkdirp",
+ "_phantomChildren": {},
+ "_requested": {
+ "type": "version",
+ "registry": true,
+ "raw": "mkdirp@0.5.1",
+ "name": "mkdirp",
+ "escapedName": "mkdirp",
+ "rawSpec": "0.5.1",
+ "saveSpec": null,
+ "fetchSpec": "0.5.1"
+ },
+ "_requiredBy": [
+ "/fstream",
+ "/node-gyp",
+ "/node-sass",
+ "/vinyl-fs"
+ ],
+ "_resolved": "http://registry.npmjs.org/mkdirp/-/mkdirp-0.5.1.tgz",
+ "_shasum": "30057438eac6cf7f8c4767f38648d6697d75c903",
+ "_spec": "mkdirp@0.5.1",
+ "_where": "/Users/rpatra16/MyProjects/RamAndAntara/node_modules/vinyl-fs",
+ "author": {
+ "name": "James Halliday",
+ "email": "mail@substack.net",
+ "url": "http://substack.net"
+ },
+ "bin": {
+ "mkdirp": "bin/cmd.js"
+ },
+ "bugs": {
+ "url": "https://github.com/substack/node-mkdirp/issues"
+ },
+ "bundleDependencies": false,
+ "dependencies": {
+ "minimist": "0.0.8"
+ },
+ "deprecated": false,
+ "description": "Recursively mkdir, like `mkdir -p`",
+ "devDependencies": {
+ "mock-fs": "2 >=2.7.0",
+ "tap": "1"
+ },
+ "homepage": "https://github.com/substack/node-mkdirp#readme",
+ "keywords": [
+ "mkdir",
+ "directory"
+ ],
+ "license": "MIT",
+ "main": "index.js",
+ "name": "mkdirp",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/substack/node-mkdirp.git"
+ },
+ "scripts": {
+ "test": "tap test/*.js"
+ },
+ "version": "0.5.1"
+}
diff --git a/node_modules/mkdirp/readme.markdown b/node_modules/mkdirp/readme.markdown
new file mode 100644
index 0000000..3cc1315
--- /dev/null
+++ b/node_modules/mkdirp/readme.markdown
@@ -0,0 +1,100 @@
+# mkdirp
+
+Like `mkdir -p`, but in node.js!
+
+[](http://travis-ci.org/substack/node-mkdirp)
+
+# example
+
+## pow.js
+
+```js
+var mkdirp = require('mkdirp');
+
+mkdirp('/tmp/foo/bar/baz', function (err) {
+ if (err) console.error(err)
+ else console.log('pow!')
+});
+```
+
+Output
+
+```
+pow!
+```
+
+And now /tmp/foo/bar/baz exists, huzzah!
+
+# methods
+
+```js
+var mkdirp = require('mkdirp');
+```
+
+## mkdirp(dir, opts, cb)
+
+Create a new directory and any necessary subdirectories at `dir` with octal
+permission string `opts.mode`. If `opts` is a non-object, it will be treated as
+the `opts.mode`.
+
+If `opts.mode` isn't specified, it defaults to `0777 & (~process.umask())`.
+
+`cb(err, made)` fires with the error or the first directory `made`
+that had to be created, if any.
+
+You can optionally pass in an alternate `fs` implementation by passing in
+`opts.fs`. Your implementation should have `opts.fs.mkdir(path, mode, cb)` and
+`opts.fs.stat(path, cb)`.
+
+## mkdirp.sync(dir, opts)
+
+Synchronously create a new directory and any necessary subdirectories at `dir`
+with octal permission string `opts.mode`. If `opts` is a non-object, it will be
+treated as the `opts.mode`.
+
+If `opts.mode` isn't specified, it defaults to `0777 & (~process.umask())`.
+
+Returns the first directory that had to be created, if any.
+
+You can optionally pass in an alternate `fs` implementation by passing in
+`opts.fs`. Your implementation should have `opts.fs.mkdirSync(path, mode)` and
+`opts.fs.statSync(path)`.
+
+# usage
+
+This package also ships with a `mkdirp` command.
+
+```
+usage: mkdirp [DIR1,DIR2..] {OPTIONS}
+
+ Create each supplied directory including any necessary parent directories that
+ don't yet exist.
+
+ If the directory already exists, do nothing.
+
+OPTIONS are:
+
+ -m, --mode If a directory needs to be created, set the mode as an octal
+ permission string.
+
+```
+
+# install
+
+With [npm](http://npmjs.org) do:
+
+```
+npm install mkdirp
+```
+
+to get the library, or
+
+```
+npm install -g mkdirp
+```
+
+to get the command.
+
+# license
+
+MIT
diff --git a/node_modules/mkdirp/test/chmod.js b/node_modules/mkdirp/test/chmod.js
new file mode 100644
index 0000000..6a404b9
--- /dev/null
+++ b/node_modules/mkdirp/test/chmod.js
@@ -0,0 +1,41 @@
+var mkdirp = require('../').mkdirp;
+var path = require('path');
+var fs = require('fs');
+var test = require('tap').test;
+var _0777 = parseInt('0777', 8);
+var _0755 = parseInt('0755', 8);
+var _0744 = parseInt('0744', 8);
+
+var ps = [ '', 'tmp' ];
+
+for (var i = 0; i < 25; i++) {
+ var dir = Math.floor(Math.random() * Math.pow(16,4)).toString(16);
+ ps.push(dir);
+}
+
+var file = ps.join('/');
+
+test('chmod-pre', function (t) {
+ var mode = _0744
+ mkdirp(file, mode, function (er) {
+ t.ifError(er, 'should not error');
+ fs.stat(file, function (er, stat) {
+ t.ifError(er, 'should exist');
+ t.ok(stat && stat.isDirectory(), 'should be directory');
+ t.equal(stat && stat.mode & _0777, mode, 'should be 0744');
+ t.end();
+ });
+ });
+});
+
+test('chmod', function (t) {
+ var mode = _0755
+ mkdirp(file, mode, function (er) {
+ t.ifError(er, 'should not error');
+ fs.stat(file, function (er, stat) {
+ t.ifError(er, 'should exist');
+ t.ok(stat && stat.isDirectory(), 'should be directory');
+ t.end();
+ });
+ });
+});
diff --git a/node_modules/mkdirp/test/clobber.js b/node_modules/mkdirp/test/clobber.js
new file mode 100644
index 0000000..2433b9a
--- /dev/null
+++ b/node_modules/mkdirp/test/clobber.js
@@ -0,0 +1,38 @@
+var mkdirp = require('../').mkdirp;
+var path = require('path');
+var fs = require('fs');
+var test = require('tap').test;
+var _0755 = parseInt('0755', 8);
+
+var ps = [ '', 'tmp' ];
+
+for (var i = 0; i < 25; i++) {
+ var dir = Math.floor(Math.random() * Math.pow(16,4)).toString(16);
+ ps.push(dir);
+}
+
+var file = ps.join('/');
+
+// a file in the way
+var itw = ps.slice(0, 3).join('/');
+
+
+test('clobber-pre', function (t) {
+ console.error("about to write to "+itw)
+ fs.writeFileSync(itw, 'I AM IN THE WAY, THE TRUTH, AND THE LIGHT.');
+
+ fs.stat(itw, function (er, stat) {
+ t.ifError(er)
+ t.ok(stat && stat.isFile(), 'should be file')
+ t.end()
+ })
+})
+
+test('clobber', function (t) {
+ t.plan(2);
+ mkdirp(file, _0755, function (err) {
+ t.ok(err);
+ t.equal(err.code, 'ENOTDIR');
+ t.end();
+ });
+});
diff --git a/node_modules/mkdirp/test/mkdirp.js b/node_modules/mkdirp/test/mkdirp.js
new file mode 100644
index 0000000..eaa8921
--- /dev/null
+++ b/node_modules/mkdirp/test/mkdirp.js
@@ -0,0 +1,28 @@
+var mkdirp = require('../');
+var path = require('path');
+var fs = require('fs');
+var exists = fs.exists || path.exists;
+var test = require('tap').test;
+var _0777 = parseInt('0777', 8);
+var _0755 = parseInt('0755', 8);
+
+test('woo', function (t) {
+ t.plan(5);
+ var x = Math.floor(Math.random() * Math.pow(16,4)).toString(16);
+ var y = Math.floor(Math.random() * Math.pow(16,4)).toString(16);
+ var z = Math.floor(Math.random() * Math.pow(16,4)).toString(16);
+
+ var file = '/tmp/' + [x,y,z].join('/');
+
+ mkdirp(file, _0755, function (err) {
+ t.ifError(err);
+ exists(file, function (ex) {
+ t.ok(ex, 'file created');
+ fs.stat(file, function (err, stat) {
+ t.ifError(err);
+ t.equal(stat.mode & _0777, _0755);
+ t.ok(stat.isDirectory(), 'target not a directory');
+ })
+ })
+ });
+});
diff --git a/node_modules/mkdirp/test/opts_fs.js b/node_modules/mkdirp/test/opts_fs.js
new file mode 100644
index 0000000..97186b6
--- /dev/null
+++ b/node_modules/mkdirp/test/opts_fs.js
@@ -0,0 +1,29 @@
+var mkdirp = require('../');
+var path = require('path');
+var test = require('tap').test;
+var mockfs = require('mock-fs');
+var _0777 = parseInt('0777', 8);
+var _0755 = parseInt('0755', 8);
+
+test('opts.fs', function (t) {
+ t.plan(5);
+
+ var x = Math.floor(Math.random() * Math.pow(16,4)).toString(16);
+ var y = Math.floor(Math.random() * Math.pow(16,4)).toString(16);
+ var z = Math.floor(Math.random() * Math.pow(16,4)).toString(16);
+
+ var file = '/beep/boop/' + [x,y,z].join('/');
+ var xfs = mockfs.fs();
+
+ mkdirp(file, { fs: xfs, mode: _0755 }, function (err) {
+ t.ifError(err);
+ xfs.exists(file, function (ex) {
+ t.ok(ex, 'created file');
+ xfs.stat(file, function (err, stat) {
+ t.ifError(err);
+ t.equal(stat.mode & _0777, _0755);
+ t.ok(stat.isDirectory(), 'target not a directory');
+ });
+ });
+ });
+});
diff --git a/node_modules/mkdirp/test/opts_fs_sync.js b/node_modules/mkdirp/test/opts_fs_sync.js
new file mode 100644
index 0000000..6c370aa
--- /dev/null
+++ b/node_modules/mkdirp/test/opts_fs_sync.js
@@ -0,0 +1,27 @@
+var mkdirp = require('../');
+var path = require('path');
+var test = require('tap').test;
+var mockfs = require('mock-fs');
+var _0777 = parseInt('0777', 8);
+var _0755 = parseInt('0755', 8);
+
+test('opts.fs sync', function (t) {
+ t.plan(4);
+
+ var x = Math.floor(Math.random() * Math.pow(16,4)).toString(16);
+ var y = Math.floor(Math.random() * Math.pow(16,4)).toString(16);
+ var z = Math.floor(Math.random() * Math.pow(16,4)).toString(16);
+
+ var file = '/beep/boop/' + [x,y,z].join('/');
+ var xfs = mockfs.fs();
+
+ mkdirp.sync(file, { fs: xfs, mode: _0755 });
+ xfs.exists(file, function (ex) {
+ t.ok(ex, 'created file');
+ xfs.stat(file, function (err, stat) {
+ t.ifError(err);
+ t.equal(stat.mode & _0777, _0755);
+ t.ok(stat.isDirectory(), 'target not a directory');
+ });
+ });
+});
diff --git a/node_modules/mkdirp/test/perm.js b/node_modules/mkdirp/test/perm.js
new file mode 100644
index 0000000..fbce44b
--- /dev/null
+++ b/node_modules/mkdirp/test/perm.js
@@ -0,0 +1,32 @@
+var mkdirp = require('../');
+var path = require('path');
+var fs = require('fs');
+var exists = fs.exists || path.exists;
+var test = require('tap').test;
+var _0777 = parseInt('0777', 8);
+var _0755 = parseInt('0755', 8);
+
+test('async perm', function (t) {
+ t.plan(5);
+ var file = '/tmp/' + (Math.random() * (1<<30)).toString(16);
+
+ mkdirp(file, _0755, function (err) {
+ t.ifError(err);
+ exists(file, function (ex) {
+ t.ok(ex, 'file created');
+ fs.stat(file, function (err, stat) {
+ t.ifError(err);
+ t.equal(stat.mode & _0777, _0755);
+ t.ok(stat.isDirectory(), 'target not a directory');
+ })
+ })
+ });
+});
+
+test('async root perm', function (t) {
+ mkdirp('/tmp', _0755, function (err) {
+ if (err) t.fail(err);
+ t.end();
+ });
+ t.end();
+});
diff --git a/node_modules/mkdirp/test/perm_sync.js b/node_modules/mkdirp/test/perm_sync.js
new file mode 100644
index 0000000..398229f
--- /dev/null
+++ b/node_modules/mkdirp/test/perm_sync.js
@@ -0,0 +1,36 @@
+var mkdirp = require('../');
+var path = require('path');
+var fs = require('fs');
+var exists = fs.exists || path.exists;
+var test = require('tap').test;
+var _0777 = parseInt('0777', 8);
+var _0755 = parseInt('0755', 8);
+
+test('sync perm', function (t) {
+ t.plan(4);
+ var file = '/tmp/' + (Math.random() * (1<<30)).toString(16) + '.json';
+
+ mkdirp.sync(file, _0755);
+ exists(file, function (ex) {
+ t.ok(ex, 'file created');
+ fs.stat(file, function (err, stat) {
+ t.ifError(err);
+ t.equal(stat.mode & _0777, _0755);
+ t.ok(stat.isDirectory(), 'target not a directory');
+ });
+ });
+});
+
+test('sync root perm', function (t) {
+ t.plan(3);
+
+ var file = '/tmp';
+ mkdirp.sync(file, _0755);
+ exists(file, function (ex) {
+ t.ok(ex, 'file created');
+ fs.stat(file, function (err, stat) {
+ t.ifError(err);
+ t.ok(stat.isDirectory(), 'target not a directory');
+ })
+ });
+});
diff --git a/node_modules/mkdirp/test/race.js b/node_modules/mkdirp/test/race.js
new file mode 100644
index 0000000..b0b9e18
--- /dev/null
+++ b/node_modules/mkdirp/test/race.js
@@ -0,0 +1,37 @@
+var mkdirp = require('../').mkdirp;
+var path = require('path');
+var fs = require('fs');
+var exists = fs.exists || path.exists;
+var test = require('tap').test;
+var _0777 = parseInt('0777', 8);
+var _0755 = parseInt('0755', 8);
+
+test('race', function (t) {
+ t.plan(10);
+ var ps = [ '', 'tmp' ];
+
+ for (var i = 0; i < 25; i++) {
+ var dir = Math.floor(Math.random() * Math.pow(16,4)).toString(16);
+ ps.push(dir);
+ }
+ var file = ps.join('/');
+
+ var res = 2;
+ mk(file);
+
+ mk(file);
+
+ function mk (file, cb) {
+ mkdirp(file, _0755, function (err) {
+ t.ifError(err);
+ exists(file, function (ex) {
+ t.ok(ex, 'file created');
+ fs.stat(file, function (err, stat) {
+ t.ifError(err);
+ t.equal(stat.mode & _0777, _0755);
+ t.ok(stat.isDirectory(), 'target not a directory');
+ });
+ })
+ });
+ }
+});
diff --git a/node_modules/mkdirp/test/rel.js b/node_modules/mkdirp/test/rel.js
new file mode 100644
index 0000000..4ddb342
--- /dev/null
+++ b/node_modules/mkdirp/test/rel.js
@@ -0,0 +1,32 @@
+var mkdirp = require('../');
+var path = require('path');
+var fs = require('fs');
+var exists = fs.exists || path.exists;
+var test = require('tap').test;
+var _0777 = parseInt('0777', 8);
+var _0755 = parseInt('0755', 8);
+
+test('rel', function (t) {
+ t.plan(5);
+ var x = Math.floor(Math.random() * Math.pow(16,4)).toString(16);
+ var y = Math.floor(Math.random() * Math.pow(16,4)).toString(16);
+ var z = Math.floor(Math.random() * Math.pow(16,4)).toString(16);
+
+ var cwd = process.cwd();
+ process.chdir('/tmp');
+
+ var file = [x,y,z].join('/');
+
+ mkdirp(file, _0755, function (err) {
+ t.ifError(err);
+ exists(file, function (ex) {
+ t.ok(ex, 'file created');
+ fs.stat(file, function (err, stat) {
+ t.ifError(err);
+ process.chdir(cwd);
+ t.equal(stat.mode & _0777, _0755);
+ t.ok(stat.isDirectory(), 'target not a directory');
+ })
+ })
+ });
+});
diff --git a/node_modules/mkdirp/test/return.js b/node_modules/mkdirp/test/return.js
new file mode 100644
index 0000000..bce68e5
--- /dev/null
+++ b/node_modules/mkdirp/test/return.js
@@ -0,0 +1,25 @@
+var mkdirp = require('../');
+var path = require('path');
+var fs = require('fs');
+var test = require('tap').test;
+
+test('return value', function (t) {
+ t.plan(4);
+ var x = Math.floor(Math.random() * Math.pow(16,4)).toString(16);
+ var y = Math.floor(Math.random() * Math.pow(16,4)).toString(16);
+ var z = Math.floor(Math.random() * Math.pow(16,4)).toString(16);
+
+ var file = '/tmp/' + [x,y,z].join('/');
+
+ // should return the first dir created.
+ // By this point, it would be profoundly surprising if /tmp didn't
+ // already exist, since every other test makes things in there.
+ mkdirp(file, function (err, made) {
+ t.ifError(err);
+ t.equal(made, '/tmp/' + x);
+ mkdirp(file, function (err, made) {
+ t.ifError(err);
+ t.equal(made, null);
+ });
+ });
+});
diff --git a/node_modules/mkdirp/test/return_sync.js b/node_modules/mkdirp/test/return_sync.js
new file mode 100644
index 0000000..7c222d3
--- /dev/null
+++ b/node_modules/mkdirp/test/return_sync.js
@@ -0,0 +1,24 @@
+var mkdirp = require('../');
+var path = require('path');
+var fs = require('fs');
+var test = require('tap').test;
+
+test('return value', function (t) {
+ t.plan(2);
+ var x = Math.floor(Math.random() * Math.pow(16,4)).toString(16);
+ var y = Math.floor(Math.random() * Math.pow(16,4)).toString(16);
+ var z = Math.floor(Math.random() * Math.pow(16,4)).toString(16);
+
+ var file = '/tmp/' + [x,y,z].join('/');
+
+ // should return the first dir created.
+ // By this point, it would be profoundly surprising if /tmp didn't
+ // already exist, since every other test makes things in there.
+ // Note that this will throw on failure, which will fail the test.
+ var made = mkdirp.sync(file);
+ t.equal(made, '/tmp/' + x);
+
+ // making the same file again should have no effect.
+ made = mkdirp.sync(file);
+ t.equal(made, null);
+});
diff --git a/node_modules/mkdirp/test/root.js b/node_modules/mkdirp/test/root.js
new file mode 100644
index 0000000..9e7d079
--- /dev/null
+++ b/node_modules/mkdirp/test/root.js
@@ -0,0 +1,19 @@
+var mkdirp = require('../');
+var path = require('path');
+var fs = require('fs');
+var test = require('tap').test;
+var _0755 = parseInt('0755', 8);
+
+test('root', function (t) {
+ // '/' on unix, 'c:/' on windows.
+ var file = path.resolve('/');
+
+ mkdirp(file, _0755, function (err) {
+ if (err) throw err
+ fs.stat(file, function (er, stat) {
+ if (er) throw er
+ t.ok(stat.isDirectory(), 'target is a directory');
+ t.end();
+ })
+ });
+});
diff --git a/node_modules/mkdirp/test/sync.js b/node_modules/mkdirp/test/sync.js
new file mode 100644
index 0000000..8c8dc93
--- /dev/null
+++ b/node_modules/mkdirp/test/sync.js
@@ -0,0 +1,32 @@
+var mkdirp = require('../');
+var path = require('path');
+var fs = require('fs');
+var exists = fs.exists || path.exists;
+var test = require('tap').test;
+var _0777 = parseInt('0777', 8);
+var _0755 = parseInt('0755', 8);
+
+test('sync', function (t) {
+ t.plan(4);
+ var x = Math.floor(Math.random() * Math.pow(16,4)).toString(16);
+ var y = Math.floor(Math.random() * Math.pow(16,4)).toString(16);
+ var z = Math.floor(Math.random() * Math.pow(16,4)).toString(16);
+
+ var file = '/tmp/' + [x,y,z].join('/');
+
+ try {
+ mkdirp.sync(file, _0755);
+ } catch (err) {
+ t.fail(err);
+ return t.end();
+ }
+
+ exists(file, function (ex) {
+ t.ok(ex, 'file created');
+ fs.stat(file, function (err, stat) {
+ t.ifError(err);
+ t.equal(stat.mode & _0777, _0755);
+ t.ok(stat.isDirectory(), 'target not a directory');
+ });
+ });
+});
diff --git a/node_modules/mkdirp/test/umask.js b/node_modules/mkdirp/test/umask.js
new file mode 100644
index 0000000..2033c63
--- /dev/null
+++ b/node_modules/mkdirp/test/umask.js
@@ -0,0 +1,28 @@
+var mkdirp = require('../');
+var path = require('path');
+var fs = require('fs');
+var exists = fs.exists || path.exists;
+var test = require('tap').test;
+var _0777 = parseInt('0777', 8);
+var _0755 = parseInt('0755', 8);
+
+test('implicit mode from umask', function (t) {
+ t.plan(5);
+ var x = Math.floor(Math.random() * Math.pow(16,4)).toString(16);
+ var y = Math.floor(Math.random() * Math.pow(16,4)).toString(16);
+ var z = Math.floor(Math.random() * Math.pow(16,4)).toString(16);
+
+ var file = '/tmp/' + [x,y,z].join('/');
+
+ mkdirp(file, function (err) {
+ t.ifError(err);
+ exists(file, function (ex) {
+ t.ok(ex, 'file created');
+ fs.stat(file, function (err, stat) {
+ t.ifError(err);
+ t.equal(stat.mode & _0777, _0777 & (~process.umask()));
+ t.ok(stat.isDirectory(), 'target not a directory');
+ });
+ })
+ });
+});
diff --git a/node_modules/mkdirp/test/umask_sync.js b/node_modules/mkdirp/test/umask_sync.js
new file mode 100644
index 0000000..11a7614
--- /dev/null
+++ b/node_modules/mkdirp/test/umask_sync.js
@@ -0,0 +1,32 @@
+var mkdirp = require('../');
+var path = require('path');
+var fs = require('fs');
+var exists = fs.exists || path.exists;
+var test = require('tap').test;
+var _0777 = parseInt('0777', 8);
+var _0755 = parseInt('0755', 8);
+
+test('umask sync modes', function (t) {
+ t.plan(4);
+ var x = Math.floor(Math.random() * Math.pow(16,4)).toString(16);
+ var y = Math.floor(Math.random() * Math.pow(16,4)).toString(16);
+ var z = Math.floor(Math.random() * Math.pow(16,4)).toString(16);
+
+ var file = '/tmp/' + [x,y,z].join('/');
+
+ try {
+ mkdirp.sync(file);
+ } catch (err) {
+ t.fail(err);
+ return t.end();
+ }
+
+ exists(file, function (ex) {
+ t.ok(ex, 'file created');
+ fs.stat(file, function (err, stat) {
+ t.ifError(err);
+ t.equal(stat.mode & _0777, (_0777 & (~process.umask())));
+ t.ok(stat.isDirectory(), 'target not a directory');
+ });
+ });
+});
diff --git a/node_modules/ms/index.js b/node_modules/ms/index.js
new file mode 100644
index 0000000..6a522b1
--- /dev/null
+++ b/node_modules/ms/index.js
@@ -0,0 +1,152 @@
+/**
+ * Helpers.
+ */
+
+var s = 1000;
+var m = s * 60;
+var h = m * 60;
+var d = h * 24;
+var y = d * 365.25;
+
+/**
+ * Parse or format the given `val`.
+ *
+ * Options:
+ *
+ * - `long` verbose formatting [false]
+ *
+ * @param {String|Number} val
+ * @param {Object} [options]
+ * @throws {Error} throw an error if val is not a non-empty string or a number
+ * @return {String|Number}
+ * @api public
+ */
+
+module.exports = function(val, options) {
+ options = options || {};
+ var type = typeof val;
+ if (type === 'string' && val.length > 0) {
+ return parse(val);
+ } else if (type === 'number' && isNaN(val) === false) {
+ return options.long ? fmtLong(val) : fmtShort(val);
+ }
+ throw new Error(
+ 'val is not a non-empty string or a valid number. val=' +
+ JSON.stringify(val)
+ );
+};
+
+/**
+ * Parse the given `str` and return milliseconds.
+ *
+ * @param {String} str
+ * @return {Number}
+ * @api private
+ */
+
+function parse(str) {
+ str = String(str);
+ if (str.length > 100) {
+ return;
+ }
+ var match = /^((?:\d+)?\.?\d+) *(milliseconds?|msecs?|ms|seconds?|secs?|s|minutes?|mins?|m|hours?|hrs?|h|days?|d|years?|yrs?|y)?$/i.exec(
+ str
+ );
+ if (!match) {
+ return;
+ }
+ var n = parseFloat(match[1]);
+ var type = (match[2] || 'ms').toLowerCase();
+ switch (type) {
+ case 'years':
+ case 'year':
+ case 'yrs':
+ case 'yr':
+ case 'y':
+ return n * y;
+ case 'days':
+ case 'day':
+ case 'd':
+ return n * d;
+ case 'hours':
+ case 'hour':
+ case 'hrs':
+ case 'hr':
+ case 'h':
+ return n * h;
+ case 'minutes':
+ case 'minute':
+ case 'mins':
+ case 'min':
+ case 'm':
+ return n * m;
+ case 'seconds':
+ case 'second':
+ case 'secs':
+ case 'sec':
+ case 's':
+ return n * s;
+ case 'milliseconds':
+ case 'millisecond':
+ case 'msecs':
+ case 'msec':
+ case 'ms':
+ return n;
+ default:
+ return undefined;
+ }
+}
+
+/**
+ * Short format for `ms`.
+ *
+ * @param {Number} ms
+ * @return {String}
+ * @api private
+ */
+
+function fmtShort(ms) {
+ if (ms >= d) {
+ return Math.round(ms / d) + 'd';
+ }
+ if (ms >= h) {
+ return Math.round(ms / h) + 'h';
+ }
+ if (ms >= m) {
+ return Math.round(ms / m) + 'm';
+ }
+ if (ms >= s) {
+ return Math.round(ms / s) + 's';
+ }
+ return ms + 'ms';
+}
+
+/**
+ * Long format for `ms`.
+ *
+ * @param {Number} ms
+ * @return {String}
+ * @api private
+ */
+
+function fmtLong(ms) {
+ return plural(ms, d, 'day') ||
+ plural(ms, h, 'hour') ||
+ plural(ms, m, 'minute') ||
+ plural(ms, s, 'second') ||
+ ms + ' ms';
+}
+
+/**
+ * Pluralization helper.
+ */
+
+function plural(ms, n, name) {
+ if (ms < n) {
+ return;
+ }
+ if (ms < n * 1.5) {
+ return Math.floor(ms / n) + ' ' + name;
+ }
+ return Math.ceil(ms / n) + ' ' + name + 's';
+}
diff --git a/node_modules/ms/license.md b/node_modules/ms/license.md
new file mode 100644
index 0000000..69b6125
--- /dev/null
+++ b/node_modules/ms/license.md
@@ -0,0 +1,21 @@
+The MIT License (MIT)
+
+Copyright (c) 2016 Zeit, Inc.
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in all
+copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
+SOFTWARE.
diff --git a/node_modules/ms/package.json b/node_modules/ms/package.json
new file mode 100644
index 0000000..e808db6
--- /dev/null
+++ b/node_modules/ms/package.json
@@ -0,0 +1,69 @@
+{
+ "_from": "ms@2.0.0",
+ "_id": "ms@2.0.0",
+ "_inBundle": false,
+ "_integrity": "sha1-VgiurfwAvmwpAd9fmGF4jeDVl8g=",
+ "_location": "/ms",
+ "_phantomChildren": {},
+ "_requested": {
+ "type": "version",
+ "registry": true,
+ "raw": "ms@2.0.0",
+ "name": "ms",
+ "escapedName": "ms",
+ "rawSpec": "2.0.0",
+ "saveSpec": null,
+ "fetchSpec": "2.0.0"
+ },
+ "_requiredBy": [
+ "/debug"
+ ],
+ "_resolved": "https://registry.npmjs.org/ms/-/ms-2.0.0.tgz",
+ "_shasum": "5608aeadfc00be6c2901df5f9861788de0d597c8",
+ "_spec": "ms@2.0.0",
+ "_where": "/Users/rpatra16/MyProjects/RamAndAntara/node_modules/debug",
+ "bugs": {
+ "url": "https://github.com/zeit/ms/issues"
+ },
+ "bundleDependencies": false,
+ "deprecated": false,
+ "description": "Tiny milisecond conversion utility",
+ "devDependencies": {
+ "eslint": "3.19.0",
+ "expect.js": "0.3.1",
+ "husky": "0.13.3",
+ "lint-staged": "3.4.1",
+ "mocha": "3.4.1"
+ },
+ "eslintConfig": {
+ "extends": "eslint:recommended",
+ "env": {
+ "node": true,
+ "es6": true
+ }
+ },
+ "files": [
+ "index.js"
+ ],
+ "homepage": "https://github.com/zeit/ms#readme",
+ "license": "MIT",
+ "lint-staged": {
+ "*.js": [
+ "npm run lint",
+ "prettier --single-quote --write",
+ "git add"
+ ]
+ },
+ "main": "./index",
+ "name": "ms",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/zeit/ms.git"
+ },
+ "scripts": {
+ "lint": "eslint lib/* bin/*",
+ "precommit": "lint-staged",
+ "test": "mocha tests.js"
+ },
+ "version": "2.0.0"
+}
diff --git a/node_modules/ms/readme.md b/node_modules/ms/readme.md
new file mode 100644
index 0000000..84a9974
--- /dev/null
+++ b/node_modules/ms/readme.md
@@ -0,0 +1,51 @@
+# ms
+
+[](https://travis-ci.org/zeit/ms)
+[](https://zeit.chat/)
+
+Use this package to easily convert various time formats to milliseconds.
+
+## Examples
+
+```js
+ms('2 days') // 172800000
+ms('1d') // 86400000
+ms('10h') // 36000000
+ms('2.5 hrs') // 9000000
+ms('2h') // 7200000
+ms('1m') // 60000
+ms('5s') // 5000
+ms('1y') // 31557600000
+ms('100') // 100
+```
+
+### Convert from milliseconds
+
+```js
+ms(60000) // "1m"
+ms(2 * 60000) // "2m"
+ms(ms('10 hours')) // "10h"
+```
+
+### Time format written-out
+
+```js
+ms(60000, { long: true }) // "1 minute"
+ms(2 * 60000, { long: true }) // "2 minutes"
+ms(ms('10 hours'), { long: true }) // "10 hours"
+```
+
+## Features
+
+- Works both in [node](https://nodejs.org) and in the browser.
+- If a number is supplied to `ms`, a string with a unit is returned.
+- If a string that contains the number is supplied, it returns it as a number (e.g.: it returns `100` for `'100'`).
+- If you pass a string with a number and a valid unit, the number of equivalent ms is returned.
+
+## Caught a bug?
+
+1. [Fork](https://help.github.com/articles/fork-a-repo/) this repository to your own GitHub account and then [clone](https://help.github.com/articles/cloning-a-repository/) it to your local device
+2. Link the package to the global module directory: `npm link`
+3. Within the module you want to test your local development instance of ms, just link it to the dependencies: `npm link ms`. Instead of the default one from npm, node will now use your clone of ms!
+
+As always, you can run the tests using: `npm test`
diff --git a/node_modules/multipipe/.npmignore b/node_modules/multipipe/.npmignore
new file mode 100644
index 0000000..3c3629e
--- /dev/null
+++ b/node_modules/multipipe/.npmignore
@@ -0,0 +1 @@
+node_modules
diff --git a/node_modules/multipipe/.travis.yml b/node_modules/multipipe/.travis.yml
new file mode 100644
index 0000000..6e5919d
--- /dev/null
+++ b/node_modules/multipipe/.travis.yml
@@ -0,0 +1,3 @@
+language: node_js
+node_js:
+ - "0.10"
diff --git a/node_modules/multipipe/History.md b/node_modules/multipipe/History.md
new file mode 100644
index 0000000..52f6e85
--- /dev/null
+++ b/node_modules/multipipe/History.md
@@ -0,0 +1,25 @@
+
+0.1.1 / 2014-06-01
+==================
+
+ * update duplexer2 dep
+
+0.1.0 / 2014-05-24
+==================
+
+ * add optional callback
+
+0.0.2 / 2014-02-20
+==================
+
+ * fix infinite loop
+
+0.0.1 / 2014-01-15
+==================
+
+* fix error bubbling
+
+0.0.0 / 2014-01-13
+==================
+
+* initial release
diff --git a/node_modules/multipipe/Makefile b/node_modules/multipipe/Makefile
new file mode 100644
index 0000000..d1df0ab
--- /dev/null
+++ b/node_modules/multipipe/Makefile
@@ -0,0 +1,10 @@
+
+node_modules: package.json
+ @npm install
+
+test:
+ @./node_modules/.bin/mocha \
+ --reporter spec \
+ --timeout 100
+
+.PHONY: test
\ No newline at end of file
diff --git a/node_modules/multipipe/Readme.md b/node_modules/multipipe/Readme.md
new file mode 100644
index 0000000..fe8c221
--- /dev/null
+++ b/node_modules/multipipe/Readme.md
@@ -0,0 +1,102 @@
+# multipipe
+
+A better `Stream#pipe` that creates duplex streams and lets you handle errors in one place.
+
+[](http://travis-ci.org/segmentio/multipipe)
+
+## Example
+
+```js
+var pipe = require('multipipe');
+
+// pipe streams
+var stream = pipe(streamA, streamB, streamC);
+
+// centralized error handling
+stream.on('error', fn);
+
+// creates a new stream
+source.pipe(stream).pipe(dest);
+
+// optional callback on finish or error
+pipe(streamA, streamB, streamC, function(err){
+ // ...
+});
+```
+
+## Duplex streams
+
+ Write to the pipe and you'll really write to the first stream, read from the pipe and you'll read from the last stream.
+
+```js
+var stream = pipe(a, b, c);
+
+source
+ .pipe(stream)
+ .pipe(destination);
+```
+
+ In this example the flow of data is:
+
+ * source ->
+ * a ->
+ * b ->
+ * c ->
+ * destination
+
+## Error handling
+
+ Each `pipe` forwards the errors the streams it wraps emit, so you have one central place to handle errors:
+
+```js
+var stream = pipe(a, b, c);
+
+stream.on('error', function(err){
+ // called three times
+});
+
+a.emit('error', new Error);
+b.emit('error', new Error);
+c.emit('error', new Error);
+```
+
+## API
+
+### pipe(stream, ...)
+
+Pass a variable number of streams and each will be piped to the next one.
+
+A stream will be returned that wraps passed in streams in a way that errors will be forwarded and you can write to and/or read from it.
+
+Pass a function as last argument to be called on `error` or `finish` of the last stream.
+
+## Installation
+
+```bash
+$ npm install multipipe
+```
+
+## License
+
+The MIT License (MIT)
+
+Copyright (c) 2014 Segment.io Inc. <friends@segment.io>
+Copyright (c) 2014 Julian Gruber <julian@juliangruber.com>
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in
+all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
+THE SOFTWARE.
diff --git a/node_modules/multipipe/index.js b/node_modules/multipipe/index.js
new file mode 100644
index 0000000..5e406db
--- /dev/null
+++ b/node_modules/multipipe/index.js
@@ -0,0 +1,72 @@
+
+/**
+ * Module dependencies.
+ */
+
+var duplexer = require('duplexer2');
+var Stream = require('stream');
+
+/**
+ * Slice reference.
+ */
+
+var slice = [].slice;
+
+/**
+ * Duplexer options.
+ */
+
+var opts = {
+ bubbleErrors: false
+};
+
+/**
+ * Expose `pipe`.
+ */
+
+module.exports = pipe;
+
+/**
+ * Pipe.
+ *
+ * @param {Stream,...,[Function]}
+ * @return {Stream}
+ * @api public
+ */
+
+function pipe(){
+ if (arguments.length == 1) return arguments[0];
+ var streams = slice.call(arguments);
+ var cb;
+ if ('function' == typeof streams[streams.length - 1]) {
+ cb = streams.splice(-1)[0];
+ }
+ var first = streams[0];
+ var last = streams[streams.length - 1];
+ var ret;
+
+ if (first.writable && last.readable) ret = duplexer(opts, first, last);
+ else if (first.writable) ret = first;
+ else if (last.readable) ret = last;
+ else ret = new Stream;
+
+ streams.forEach(function(stream, i){
+ var next = streams[i+1];
+ if (next) stream.pipe(next);
+ if (stream != ret) stream.on('error', ret.emit.bind(ret, 'error'));
+ });
+
+ if (cb) {
+ var ended = false;
+ ret.on('error', end);
+ last.on('finish', end);
+ function end(err){
+ if (ended) return;
+ ended = true;
+ cb(err);
+ }
+ }
+
+ return ret;
+}
+
diff --git a/node_modules/multipipe/package.json b/node_modules/multipipe/package.json
new file mode 100644
index 0000000..2b0a7f3
--- /dev/null
+++ b/node_modules/multipipe/package.json
@@ -0,0 +1,48 @@
+{
+ "_from": "multipipe@^0.1.2",
+ "_id": "multipipe@0.1.2",
+ "_inBundle": false,
+ "_integrity": "sha1-Ko8t33Du1WTf8tV/HhoTfZ8FB4s=",
+ "_location": "/multipipe",
+ "_phantomChildren": {},
+ "_requested": {
+ "type": "range",
+ "registry": true,
+ "raw": "multipipe@^0.1.2",
+ "name": "multipipe",
+ "escapedName": "multipipe",
+ "rawSpec": "^0.1.2",
+ "saveSpec": null,
+ "fetchSpec": "^0.1.2"
+ },
+ "_requiredBy": [
+ "/gulp-util"
+ ],
+ "_resolved": "https://registry.npmjs.org/multipipe/-/multipipe-0.1.2.tgz",
+ "_shasum": "2a8f2ddf70eed564dff2d57f1e1a137d9f05078b",
+ "_spec": "multipipe@^0.1.2",
+ "_where": "/Users/rpatra16/MyProjects/RamAndAntara/node_modules/gulp-util",
+ "bugs": {
+ "url": "https://github.com/juliangruber/multipipe/issues"
+ },
+ "bundleDependencies": false,
+ "dependencies": {
+ "duplexer2": "0.0.2"
+ },
+ "deprecated": false,
+ "description": "pipe streams with centralized error handling",
+ "devDependencies": {
+ "mocha": "~1.17.0"
+ },
+ "homepage": "https://github.com/juliangruber/multipipe#readme",
+ "license": "MIT",
+ "name": "multipipe",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/juliangruber/multipipe.git"
+ },
+ "scripts": {
+ "test": "make test"
+ },
+ "version": "0.1.2"
+}
diff --git a/node_modules/multipipe/test/multipipe.js b/node_modules/multipipe/test/multipipe.js
new file mode 100644
index 0000000..078d2e0
--- /dev/null
+++ b/node_modules/multipipe/test/multipipe.js
@@ -0,0 +1,141 @@
+var assert = require('assert');
+var pipe = require('..');
+var Stream = require('stream');
+
+describe('pipe(a)', function(){
+ it('should return a', function(){
+ var readable = Readable();
+ var stream = pipe(readable);
+ assert.equal(stream, readable);
+ });
+});
+
+describe('pipe(a, b, c)', function(){
+ it('should pipe internally', function(done){
+ pipe(Readable(), Transform(), Writable(done));
+ });
+
+ it('should be writable', function(done){
+ var stream = pipe(Transform(), Writable(done));
+ assert(stream.writable);
+ Readable().pipe(stream);
+ });
+
+ it('should be readable', function(done){
+ var stream = pipe(Readable(), Transform());
+ assert(stream.readable);
+ stream.pipe(Writable(done));
+ });
+
+ it('should be readable and writable', function(done){
+ var stream = pipe(Transform(), Transform());
+ assert(stream.readable);
+ assert(stream.writable);
+ Readable()
+ .pipe(stream)
+ .pipe(Writable(done));
+ });
+
+ describe('errors', function(){
+ it('should reemit', function(done){
+ var a = Transform();
+ var b = Transform();
+ var c = Transform();
+ var stream = pipe(a, b, c);
+ var err = new Error;
+ var i = 0;
+
+ stream.on('error', function(_err){
+ i++;
+ assert.equal(_err, err);
+ assert(i <= 3);
+ if (i == 3) done();
+ });
+
+ a.emit('error', err);
+ b.emit('error', err);
+ c.emit('error', err);
+ });
+
+ it('should not reemit endlessly', function(done){
+ var a = Transform();
+ var b = Transform();
+ var c = Transform();
+ c.readable = false;
+ var stream = pipe(a, b, c);
+ var err = new Error;
+ var i = 0;
+
+ stream.on('error', function(_err){
+ i++;
+ assert.equal(_err, err);
+ assert(i <= 3);
+ if (i == 3) done();
+ });
+
+ a.emit('error', err);
+ b.emit('error', err);
+ c.emit('error', err);
+ });
+ });
+});
+
+describe('pipe(a, b, c, fn)', function(){
+ it('should call on finish', function(done){
+ var finished = false;
+ var a = Readable();
+ var b = Transform();
+ var c = Writable(function(){
+ finished = true;
+ });
+
+ pipe(a, b, c, function(err){
+ assert(!err);
+ assert(finished);
+ done();
+ });
+ });
+
+ it('should call with error once', function(done){
+ var a = Readable();
+ var b = Transform();
+ var c = Writable();
+ var err = new Error;
+
+ pipe(a, b, c, function(err){
+ assert(err);
+ done();
+ });
+
+ a.emit('error', err);
+ b.emit('error', err);
+ c.emit('error', err);
+ });
+});
+
+function Readable(){
+ var readable = new Stream.Readable({ objectMode: true });
+ readable._read = function(){
+ this.push('a');
+ this.push(null);
+ };
+ return readable;
+}
+
+function Transform(){
+ var transform = new Stream.Transform({ objectMode: true });
+ transform._transform = function(chunk, _, done){
+ done(null, chunk.toUpperCase());
+ };
+ return transform;
+}
+
+function Writable(cb){
+ var writable = new Stream.Writable({ objectMode: true });
+ writable._write = function(chunk, _, done){
+ assert.equal(chunk, 'A');
+ done();
+ cb();
+ };
+ return writable;
+}
diff --git a/node_modules/nan/CHANGELOG.md b/node_modules/nan/CHANGELOG.md
new file mode 100644
index 0000000..10d3ee6
--- /dev/null
+++ b/node_modules/nan/CHANGELOG.md
@@ -0,0 +1,503 @@
+# NAN ChangeLog
+
+**Version 2.11.1: current Node 10.11.0, Node 0.12: 0.12.18, Node 0.10: 0.10.48, iojs: 3.3.1**
+
+### 2.11.1 Sep 29 2018
+
+- Fix: adapt to V8 7.0 24a22c3b25eeeec2016c6ec239bdd6169e985447
+
+### 2.11.0 Aug 25 2018
+
+ - Removal: remove `FunctionCallbackInfo::Callee` for nodejs `>= 10` 1a56c0a6efd4fac944cb46c30912a8e023bda7d4
+ - Bugfix: Fix `AsyncProgressWorkerBase::WorkProgress` sends invalid data b0c764d1dab11e9f8b37ffb81e2560a4498aad5e
+ - Feature: Introduce `GetCurrentEventLoop` b4911b0bb1f6d47d860e10ec014d941c51efac5e
+ - Feature: Add `NAN_MODULE_WORKER_ENABLED` macro as a replacement for `NAN_MODULE` b058fb047d18a58250e66ae831444441c1f2ac7a
+
+### 2.10.0 Mar 16 2018
+
+ - Deprecation: Deprecate `MakeCallback` 5e92b19a59e194241d6a658bd6ff7bfbda372950
+ - Feature: add `Nan::Call` overload 4482e1242fe124d166fc1a5b2be3c1cc849fe452
+ - Feature: add more `Nan::Call` overloads 8584e63e6d04c7d2eb8c4a664e4ef57d70bf672b
+ - Feature: Fix deprecation warnings for Node 10 1caf258243b0602ed56922bde74f1c91b0cbcb6a
+
+### 2.9.2 Feb 22 2018
+
+ - Bugfix: Bandaid for async hooks 212bd2f849be14ef1b02fc85010b053daa24252b
+
+### 2.9.1 Feb 22 2018
+
+ - Bugfix: Avoid deprecation warnings in deprecated `Nan::Callback::operator()` 372b14d91289df4604b0f81780709708c45a9aa4
+ - Bugfix: Avoid deprecation warnings in `Nan::JSON` 3bc294bce0b7d0a3ee4559926303e5ed4866fda2
+
+### 2.9.0 Feb 22 2018
+
+ - Deprecation: Deprecate legacy `Callback::Call` 6dd5fa690af61ca3523004b433304c581b3ea309
+ - Feature: introduce `AsyncResource` class 90c0a179c0d8cb5fd26f1a7d2b1d6231eb402d48o
+ - Feature: Add context aware `Nan::Callback::Call` functions 7169e09fb088418b6e388222e88b4c13f07ebaee
+ - Feature: Make `AsyncWorker` context aware 066ba21a6fb9e2b5230c9ed3a6fc51f1211736a4
+ - Feature: add `Callback` overload to `Nan::Call` 5328daf66e202658c1dc0d916c3aaba99b3cc606
+ - Bugfix: fix warning: suggest parentheses around `&&` within `||` b2bb63d68b8ae623a526b542764e1ac82319cb2c
+ - Bugfix: Fix compilation on io.js 3 d06114dba0a522fb436f0c5f47b994210968cd7b
+
+### 2.8.0 Nov 15 2017
+
+ - Deprecation: Deprecate `Nan::ForceSet` in favor of `Nan::DefineOwnProperty()` 95cbb976d6fbbba88ba0f86dd188223a8591b4e7
+ - Feature: Add `Nan::AsyncProgressQueueWorker` a976636ecc2ef617d1b061ce4a6edf39923691cb
+ - Feature: Add `Nan::DefineOwnProperty()` 95cbb976d6fbbba88ba0f86dd188223a8591b4e7
+ - Bugfix: Fix compiling on io.js 1 & 2 82705a64503ce60c62e98df5bd02972bba090900
+ - Bugfix: Use DefineOwnProperty instead of ForceSet 95cbb976d6fbbba88ba0f86dd188223a8591b4e7
+
+### 2.7.0 Aug 30 2017
+
+ - Feature: Add `Nan::To()` overload. b93280670c9f6da42ed4cf6cbf085ffdd87bd65b
+ - Bugfix: Fix ternary in `Nan::MaybeLocal::FromMaybe()`. 79a26f7d362e756a9524e672a82c3d603b542867
+
+### 2.6.2 Apr 12 2017
+
+ - Bugfix: Fix v8::JSON::Parse() deprecation warning. 87f6a3c65815fa062296a994cc863e2fa124867d
+
+### 2.6.1 Apr 6 2017
+
+ - Bugfix: nan_json.h: fix build breakage in Node 6 ac8d47dc3c10bfbf3f15a6b951633120c0ee6d51
+
+### 2.6.0 Apr 6 2017
+
+ - Feature: nan: add support for JSON::Parse & Stringify b533226c629cce70e1932a873bb6f849044a56c5
+
+### 2.5.1 Jan 23 2017
+
+ - Bugfix: Fix disappearing handle for private value 6a80995694f162ef63dbc9948fbefd45d4485aa0
+ - Bugfix: Add missing scopes a93b8bae6bc7d32a170db6e89228b7f60ee57112
+ - Bugfix: Use string::data instead of string::front in NewOneByteString d5f920371e67e1f3b268295daee6e83af86b6e50
+
+### 2.5.0 Dec 21 2016
+
+ - Feature: Support Private accessors a86255cb357e8ad8ccbf1f6a4a901c921e39a178
+ - Bugfix: Abort in delete operators that shouldn't be called 0fe38215ff8581703967dfd26c12793feb960018
+
+### 2.4.0 Jul 10 2016
+
+ - Feature: Rewrite Callback to add Callback::Reset c4cf44d61f8275cd5f7b0c911d7a806d4004f649
+ - Feature: AsyncProgressWorker: add template types for .send 1242c9a11a7ed481c8f08ec06316385cacc513d0
+ - Bugfix: Add constness to old Persistent comparison operators bd43cb9982c7639605d60fd073efe8cae165d9b2
+
+### 2.3.5 May 31 2016
+
+ - Bugfix: Replace NAN_INLINE with 'inline' keyword. 71819d8725f822990f439479c9aba3b240804909
+
+### 2.3.4 May 31 2016
+
+ - Bugfix: Remove V8 deprecation warnings 0592fb0a47f3a1c7763087ebea8e1138829f24f9
+ - Bugfix: Fix new versions not to use WeakCallbackInfo::IsFirstPass 615c19d9e03d4be2049c10db0151edbc3b229246
+ - Bugfix: Make ObjectWrap::handle() const d19af99595587fe7a26bd850af6595c2a7145afc
+ - Bugfix: Fix compilation errors related to 0592fb0a47f3a1c7763087ebea8e1138829f24f9 e9191c525b94f652718325e28610a1adcf90fed8
+
+### 2.3.3 May 4 2016
+
+ - Bugfix: Refactor SetMethod() to deal with v8::Templates (#566) b9083cf6d5de6ebe6bcb49c7502fbb7c0d9ddda8
+
+### 2.3.2 Apr 27 2016
+
+ - Bugfix: Fix compilation on outdated versions due to Handle removal f8b7c875d04d425a41dfd4f3f8345bc3a11e6c52
+
+### 2.3.1 Apr 27 2016
+
+ - Bugfix: Don't use deprecated v8::Template::Set() in SetMethod a90951e9ea70fa1b3836af4b925322919159100e
+
+### 2.3.0 Apr 27 2016
+
+ - Feature: added Signal() for invoking async callbacks without sending data from AsyncProgressWorker d8adba45f20e077d00561b20199133620c990b38
+ - Bugfix: Don't use deprecated v8::Template::Set() 00dacf0a4b86027415867fa7f1059acc499dcece
+
+### 2.2.1 Mar 29 2016
+
+ - Bugfix: Use NewFromUnsigned in ReturnValue::Set(uint32_t i) for pre_12 3a18f9bdce29826e0e4c217854bc476918241a58
+ - Performance: Remove unneeeded nullptr checks b715ef44887931c94f0d1605b3b1a4156eebece9
+
+### 2.2.0 Jan 9 2016
+
+ - Feature: Add Function::Call wrapper 4c157474dacf284d125c324177b45aa5dabc08c6
+ - Feature: Rename GC*logueCallback to GCCallback for > 4.0 3603435109f981606d300eb88004ca101283acec
+ - Bugfix: Fix Global::Pass for old versions 367e82a60fbaa52716232cc89db1cc3f685d77d9
+ - Bugfix: Remove weird MaybeLocal wrapping of what already is a MaybeLocal 23b4590db10c2ba66aee2338aebe9751c4cb190b
+
+### 2.1.0 Oct 8 2015
+
+ - Deprecation: Deprecate NanErrnoException in favor of ErrnoException 0af1ca4cf8b3f0f65ed31bc63a663ab3319da55c
+ - Feature: added helper class for accessing contents of typedarrays 17b51294c801e534479d5463697a73462d0ca555
+ - Feature: [Maybe types] Add MakeMaybe(...) 48d7b53d9702b0c7a060e69ea10fea8fb48d814d
+ - Feature: new: allow utf16 string with length 66ac6e65c8ab9394ef588adfc59131b3b9d8347b
+ - Feature: Introduce SetCallHandler and SetCallAsFunctionHandler 7764a9a115d60ba10dc24d86feb0fbc9b4f75537
+ - Bugfix: Enable creating Locals from Globals under Node 0.10. 9bf9b8b190821af889790fdc18ace57257e4f9ff
+ - Bugfix: Fix issue #462 where PropertyCallbackInfo data is not stored safely. 55f50adedd543098526c7b9f4fffd607d3f9861f
+
+### 2.0.9 Sep 8 2015
+
+ - Bugfix: EscapableHandleScope in Nan::NewBuffer for Node 0.8 and 0.10 b1654d7
+
+### 2.0.8 Aug 28 2015
+
+ - Work around duplicate linking bug in clang 11902da
+
+### 2.0.7 Aug 26 2015
+
+ - Build: Repackage
+
+### 2.0.6 Aug 26 2015
+
+ - Bugfix: Properly handle null callback in FunctionTemplate factory 6e99cb1
+ - Bugfix: Remove unused static std::map instances 525bddc
+ - Bugfix: Make better use of maybe versions of APIs bfba85b
+ - Bugfix: Fix shadowing issues with handle in ObjectWrap 0a9072d
+
+### 2.0.5 Aug 10 2015
+
+ - Bugfix: Reimplement weak callback in ObjectWrap 98d38c1
+ - Bugfix: Make sure callback classes are not assignable, copyable or movable 81f9b1d
+
+### 2.0.4 Aug 6 2015
+
+ - Build: Repackage
+
+### 2.0.3 Aug 6 2015
+
+ - Bugfix: Don't use clang++ / g++ syntax extension. 231450e
+
+### 2.0.2 Aug 6 2015
+
+ - Build: Repackage
+
+### 2.0.1 Aug 6 2015
+
+ - Bugfix: Add workaround for missing REPLACE_INVALID_UTF8 60d6687
+ - Bugfix: Reimplement ObjectWrap from scratch to prevent memory leaks 6484601
+ - Bugfix: Fix Persistent leak in FunctionCallbackInfo and PropertyCallbackInfo 641ef5f
+ - Bugfix: Add missing overload for Nan::NewInstance that takes argc/argv 29450ed
+
+### 2.0.0 Jul 31 2015
+
+ - Change: Renamed identifiers with leading underscores b5932b4
+ - Change: Replaced NanObjectWrapHandle with class NanObjectWrap 464f1e1
+ - Change: Replace NanScope and NanEscpableScope macros with classes 47751c4
+ - Change: Rename NanNewBufferHandle to NanNewBuffer 6745f99
+ - Change: Rename NanBufferUse to NanNewBuffer 3e8b0a5
+ - Change: Rename NanNewBuffer to NanCopyBuffer d6af78d
+ - Change: Remove Nan prefix from all names 72d1f67
+ - Change: Update Buffer API for new upstream changes d5d3291
+ - Change: Rename Scope and EscapableScope to HandleScope and EscapableHandleScope 21a7a6a
+ - Change: Get rid of Handles e6c0daf
+ - Feature: Support io.js 3 with V8 4.4
+ - Feature: Introduce NanPersistent 7fed696
+ - Feature: Introduce NanGlobal 4408da1
+ - Feature: Added NanTryCatch 10f1ca4
+ - Feature: Update for V8 v4.3 4b6404a
+ - Feature: Introduce NanNewOneByteString c543d32
+ - Feature: Introduce namespace Nan 67ed1b1
+ - Removal: Remove NanLocker and NanUnlocker dd6e401
+ - Removal: Remove string converters, except NanUtf8String, which now follows the node implementation b5d00a9
+ - Removal: Remove NanReturn* macros d90a25c
+ - Removal: Remove HasInstance e8f84fe
+
+
+### 1.9.0 Jul 31 2015
+
+ - Feature: Added `NanFatalException` 81d4a2c
+ - Feature: Added more error types 4265f06
+ - Feature: Added dereference and function call operators to NanCallback c4b2ed0
+ - Feature: Added indexed GetFromPersistent and SaveToPersistent edd510c
+ - Feature: Added more overloads of SaveToPersistent and GetFromPersistent 8b1cef6
+ - Feature: Added NanErrnoException dd87d9e
+ - Correctness: Prevent assign, copy, and move for classes that do not support it 1f55c59, 4b808cb, c96d9b2, fba4a29, 3357130
+ - Deprecation: Deprecate `NanGetPointerSafe` and `NanSetPointerSafe` 81d4a2c
+ - Deprecation: Deprecate `NanBooleanOptionValue` and `NanUInt32OptionValue` 0ad254b
+
+### 1.8.4 Apr 26 2015
+
+ - Build: Repackage
+
+### 1.8.3 Apr 26 2015
+
+ - Bugfix: Include missing header 1af8648
+
+### 1.8.2 Apr 23 2015
+
+ - Build: Repackage
+
+### 1.8.1 Apr 23 2015
+
+ - Bugfix: NanObjectWrapHandle should take a pointer 155f1d3
+
+### 1.8.0 Apr 23 2015
+
+ - Feature: Allow primitives with NanReturnValue 2e4475e
+ - Feature: Added comparison operators to NanCallback 55b075e
+ - Feature: Backport thread local storage 15bb7fa
+ - Removal: Remove support for signatures with arguments 8a2069d
+ - Correcteness: Replaced NanObjectWrapHandle macro with function 0bc6d59
+
+### 1.7.0 Feb 28 2015
+
+ - Feature: Made NanCallback::Call accept optional target 8d54da7
+ - Feature: Support atom-shell 0.21 0b7f1bb
+
+### 1.6.2 Feb 6 2015
+
+ - Bugfix: NanEncode: fix argument type for node::Encode on io.js 2be8639
+
+### 1.6.1 Jan 23 2015
+
+ - Build: version bump
+
+### 1.5.3 Jan 23 2015
+
+ - Build: repackage
+
+### 1.6.0 Jan 23 2015
+
+ - Deprecated `NanNewContextHandle` in favor of `NanNew` 49259af
+ - Support utility functions moved in newer v8 versions (Node 0.11.15, io.js 1.0) a0aa179
+ - Added `NanEncode`, `NanDecodeBytes` and `NanDecodeWrite` 75e6fb9
+
+### 1.5.2 Jan 23 2015
+
+ - Bugfix: Fix non-inline definition build error with clang++ 21d96a1, 60fadd4
+ - Bugfix: Readded missing String constructors 18d828f
+ - Bugfix: Add overload handling NanNew(..) 5ef813b
+ - Bugfix: Fix uv_work_cb versioning 997e4ae
+ - Bugfix: Add function factory and test 4eca89c
+ - Bugfix: Add object template factory and test cdcb951
+ - Correctness: Lifted an io.js related typedef c9490be
+ - Correctness: Make explicit downcasts of String lengths 00074e6
+ - Windows: Limit the scope of disabled warning C4530 83d7deb
+
+### 1.5.1 Jan 15 2015
+
+ - Build: version bump
+
+### 1.4.3 Jan 15 2015
+
+ - Build: version bump
+
+### 1.4.2 Jan 15 2015
+
+ - Feature: Support io.js 0dbc5e8
+
+### 1.5.0 Jan 14 2015
+
+ - Feature: Support io.js b003843
+ - Correctness: Improved NanNew internals 9cd4f6a
+ - Feature: Implement progress to NanAsyncWorker 8d6a160
+
+### 1.4.1 Nov 8 2014
+
+ - Bugfix: Handle DEBUG definition correctly
+ - Bugfix: Accept int as Boolean
+
+### 1.4.0 Nov 1 2014
+
+ - Feature: Added NAN_GC_CALLBACK 6a5c245
+ - Performance: Removed unnecessary local handle creation 18a7243, 41fe2f8
+ - Correctness: Added constness to references in NanHasInstance 02c61cd
+ - Warnings: Fixed spurious warnings from -Wundef and -Wshadow, 541b122, 99d8cb6
+ - Windoze: Shut Visual Studio up when compiling 8d558c1
+ - License: Switch to plain MIT from custom hacked MIT license 11de983
+ - Build: Added test target to Makefile e232e46
+ - Performance: Removed superfluous scope in NanAsyncWorker f4b7821
+ - Sugar/Feature: Added NanReturnThis() and NanReturnHolder() shorthands 237a5ff, d697208
+ - Feature: Added suitable overload of NanNew for v8::Integer::NewFromUnsigned b27b450
+
+### 1.3.0 Aug 2 2014
+
+ - Added NanNew(std::string)
+ - Added NanNew(std::string&)
+ - Added NanAsciiString helper class
+ - Added NanUtf8String helper class
+ - Added NanUcs2String helper class
+ - Deprecated NanRawString()
+ - Deprecated NanCString()
+ - Added NanGetIsolateData(v8::Isolate *isolate)
+ - Added NanMakeCallback(v8::Handle target, v8::Handle func, int argc, v8::Handle* argv)
+ - Added NanMakeCallback(v8::Handle target, v8::Handle symbol, int argc, v8::Handle* argv)
+ - Added NanMakeCallback(v8::Handle target, const char* method, int argc, v8::Handle* argv)
+ - Added NanSetTemplate(v8::Handle templ, v8::Handle name , v8::Handle value, v8::PropertyAttribute attributes)
+ - Added NanSetPrototypeTemplate(v8::Local templ, v8::Handle name, v8::Handle value, v8::PropertyAttribute attributes)
+ - Added NanSetInstanceTemplate(v8::Local templ, const char *name, v8::Handle value)
+ - Added NanSetInstanceTemplate(v8::Local templ, v8::Handle name, v8::Handle value, v8::PropertyAttribute attributes)
+
+### 1.2.0 Jun 5 2014
+
+ - Add NanSetPrototypeTemplate
+ - Changed NAN_WEAK_CALLBACK internals, switched _NanWeakCallbackData to class,
+ introduced _NanWeakCallbackDispatcher
+ - Removed -Wno-unused-local-typedefs from test builds
+ - Made test builds Windows compatible ('Sleep()')
+
+### 1.1.2 May 28 2014
+
+ - Release to fix more stuff-ups in 1.1.1
+
+### 1.1.1 May 28 2014
+
+ - Release to fix version mismatch in nan.h and lack of changelog entry for 1.1.0
+
+### 1.1.0 May 25 2014
+
+ - Remove nan_isolate, use v8::Isolate::GetCurrent() internally instead
+ - Additional explicit overloads for NanNew(): (char*,int), (uint8_t*[,int]),
+ (uint16_t*[,int), double, int, unsigned int, bool, v8::String::ExternalStringResource*,
+ v8::String::ExternalAsciiStringResource*
+ - Deprecate NanSymbol()
+ - Added SetErrorMessage() and ErrorMessage() to NanAsyncWorker
+
+### 1.0.0 May 4 2014
+
+ - Heavy API changes for V8 3.25 / Node 0.11.13
+ - Use cpplint.py
+ - Removed NanInitPersistent
+ - Removed NanPersistentToLocal
+ - Removed NanFromV8String
+ - Removed NanMakeWeak
+ - Removed NanNewLocal
+ - Removed NAN_WEAK_CALLBACK_OBJECT
+ - Removed NAN_WEAK_CALLBACK_DATA
+ - Introduce NanNew, replaces NanNewLocal, NanPersistentToLocal, adds many overloaded typed versions
+ - Introduce NanUndefined, NanNull, NanTrue and NanFalse
+ - Introduce NanEscapableScope and NanEscapeScope
+ - Introduce NanMakeWeakPersistent (requires a special callback to work on both old and new node)
+ - Introduce NanMakeCallback for node::MakeCallback
+ - Introduce NanSetTemplate
+ - Introduce NanGetCurrentContext
+ - Introduce NanCompileScript and NanRunScript
+ - Introduce NanAdjustExternalMemory
+ - Introduce NanAddGCEpilogueCallback, NanAddGCPrologueCallback, NanRemoveGCEpilogueCallback, NanRemoveGCPrologueCallback
+ - Introduce NanGetHeapStatistics
+ - Rename NanAsyncWorker#SavePersistent() to SaveToPersistent()
+
+### 0.8.0 Jan 9 2014
+
+ - NanDispose -> NanDisposePersistent, deprecate NanDispose
+ - Extract _NAN_*_RETURN_TYPE, pull up NAN_*()
+
+### 0.7.1 Jan 9 2014
+
+ - Fixes to work against debug builds of Node
+ - Safer NanPersistentToLocal (avoid reinterpret_cast)
+ - Speed up common NanRawString case by only extracting flattened string when necessary
+
+### 0.7.0 Dec 17 2013
+
+ - New no-arg form of NanCallback() constructor.
+ - NanCallback#Call takes Handle rather than Local
+ - Removed deprecated NanCallback#Run method, use NanCallback#Call instead
+ - Split off _NAN_*_ARGS_TYPE from _NAN_*_ARGS
+ - Restore (unofficial) Node 0.6 compatibility at NanCallback#Call()
+ - Introduce NanRawString() for char* (or appropriate void*) from v8::String
+ (replacement for NanFromV8String)
+ - Introduce NanCString() for null-terminated char* from v8::String
+
+### 0.6.0 Nov 21 2013
+
+ - Introduce NanNewLocal(v8::Handle value) for use in place of
+ v8::Local::New(...) since v8 started requiring isolate in Node 0.11.9
+
+### 0.5.2 Nov 16 2013
+
+ - Convert SavePersistent and GetFromPersistent in NanAsyncWorker from protected and public
+
+### 0.5.1 Nov 12 2013
+
+ - Use node::MakeCallback() instead of direct v8::Function::Call()
+
+### 0.5.0 Nov 11 2013
+
+ - Added @TooTallNate as collaborator
+ - New, much simpler, "include_dirs" for binding.gyp
+ - Added full range of NAN_INDEX_* macros to match NAN_PROPERTY_* macros
+
+### 0.4.4 Nov 2 2013
+
+ - Isolate argument from v8::Persistent::MakeWeak removed for 0.11.8+
+
+### 0.4.3 Nov 2 2013
+
+ - Include node_object_wrap.h, removed from node.h for Node 0.11.8.
+
+### 0.4.2 Nov 2 2013
+
+ - Handle deprecation of v8::Persistent::Dispose(v8::Isolate* isolate)) for
+ Node 0.11.8 release.
+
+### 0.4.1 Sep 16 2013
+
+ - Added explicit `#include ` as it was removed from node.h for v0.11.8
+
+### 0.4.0 Sep 2 2013
+
+ - Added NAN_INLINE and NAN_DEPRECATED and made use of them
+ - Added NanError, NanTypeError and NanRangeError
+ - Cleaned up code
+
+### 0.3.2 Aug 30 2013
+
+ - Fix missing scope declaration in GetFromPersistent() and SaveToPersistent
+ in NanAsyncWorker
+
+### 0.3.1 Aug 20 2013
+
+ - fix "not all control paths return a value" compile warning on some platforms
+
+### 0.3.0 Aug 19 2013
+
+ - Made NAN work with NPM
+ - Lots of fixes to NanFromV8String, pulling in features from new Node core
+ - Changed node::encoding to Nan::Encoding in NanFromV8String to unify the API
+ - Added optional error number argument for NanThrowError()
+ - Added NanInitPersistent()
+ - Added NanReturnNull() and NanReturnEmptyString()
+ - Added NanLocker and NanUnlocker
+ - Added missing scopes
+ - Made sure to clear disposed Persistent handles
+ - Changed NanAsyncWorker to allocate error messages on the heap
+ - Changed NanThrowError(Local) to NanThrowError(Handle)
+ - Fixed leak in NanAsyncWorker when errmsg is used
+
+### 0.2.2 Aug 5 2013
+
+ - Fixed usage of undefined variable with node::BASE64 in NanFromV8String()
+
+### 0.2.1 Aug 5 2013
+
+ - Fixed 0.8 breakage, node::BUFFER encoding type not available in 0.8 for
+ NanFromV8String()
+
+### 0.2.0 Aug 5 2013
+
+ - Added NAN_PROPERTY_GETTER, NAN_PROPERTY_SETTER, NAN_PROPERTY_ENUMERATOR,
+ NAN_PROPERTY_DELETER, NAN_PROPERTY_QUERY
+ - Extracted _NAN_METHOD_ARGS, _NAN_GETTER_ARGS, _NAN_SETTER_ARGS,
+ _NAN_PROPERTY_GETTER_ARGS, _NAN_PROPERTY_SETTER_ARGS,
+ _NAN_PROPERTY_ENUMERATOR_ARGS, _NAN_PROPERTY_DELETER_ARGS,
+ _NAN_PROPERTY_QUERY_ARGS
+ - Added NanGetInternalFieldPointer, NanSetInternalFieldPointer
+ - Added NAN_WEAK_CALLBACK, NAN_WEAK_CALLBACK_OBJECT,
+ NAN_WEAK_CALLBACK_DATA, NanMakeWeak
+ - Renamed THROW_ERROR to _NAN_THROW_ERROR
+ - Added NanNewBufferHandle(char*, size_t, node::smalloc::FreeCallback, void*)
+ - Added NanBufferUse(char*, uint32_t)
+ - Added NanNewContextHandle(v8::ExtensionConfiguration*,
+ v8::Handle, v8::Handle)
+ - Fixed broken NanCallback#GetFunction()
+ - Added optional encoding and size arguments to NanFromV8String()
+ - Added NanGetPointerSafe() and NanSetPointerSafe()
+ - Added initial test suite (to be expanded)
+ - Allow NanUInt32OptionValue to convert any Number object
+
+### 0.1.0 Jul 21 2013
+
+ - Added `NAN_GETTER`, `NAN_SETTER`
+ - Added `NanThrowError` with single Local argument
+ - Added `NanNewBufferHandle` with single uint32_t argument
+ - Added `NanHasInstance(Persistent&, Handle)`
+ - Added `Local NanCallback#GetFunction()`
+ - Added `NanCallback#Call(int, Local[])`
+ - Deprecated `NanCallback#Run(int, Local[])` in favour of Call
diff --git a/node_modules/nan/LICENSE.md b/node_modules/nan/LICENSE.md
new file mode 100644
index 0000000..dddd13d
--- /dev/null
+++ b/node_modules/nan/LICENSE.md
@@ -0,0 +1,13 @@
+The MIT License (MIT)
+=====================
+
+Copyright (c) 2018 NAN contributors
+-----------------------------------
+
+*NAN contributors listed at *
+
+Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
diff --git a/node_modules/nan/README.md b/node_modules/nan/README.md
new file mode 100644
index 0000000..d002fe1
--- /dev/null
+++ b/node_modules/nan/README.md
@@ -0,0 +1,456 @@
+Native Abstractions for Node.js
+===============================
+
+**A header file filled with macro and utility goodness for making add-on development for Node.js easier across versions 0.8, 0.10, 0.12, 1, 2, 3, 4, 5, 6, 7, 8, 9 and 10.**
+
+***Current version: 2.11.1***
+
+*(See [CHANGELOG.md](https://github.com/nodejs/nan/blob/master/CHANGELOG.md) for complete ChangeLog)*
+
+[](https://nodei.co/npm/nan/) [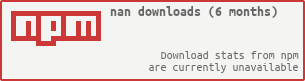](https://nodei.co/npm/nan/)
+
+[](http://travis-ci.org/nodejs/nan)
+[](https://ci.appveyor.com/project/RodVagg/nan)
+
+Thanks to the crazy changes in V8 (and some in Node core), keeping native addons compiling happily across versions, particularly 0.10 to 0.12 to 4.0, is a minor nightmare. The goal of this project is to store all logic necessary to develop native Node.js addons without having to inspect `NODE_MODULE_VERSION` and get yourself into a macro-tangle.
+
+This project also contains some helper utilities that make addon development a bit more pleasant.
+
+ * **[News & Updates](#news)**
+ * **[Usage](#usage)**
+ * **[Example](#example)**
+ * **[API](#api)**
+ * **[Tests](#tests)**
+ * **[Knowns issues](#issues)**
+ * **[Governance & Contributing](#governance)**
+
+
+
+## News & Updates
+
+
+
+## Usage
+
+Simply add **NAN** as a dependency in the *package.json* of your Node addon:
+
+``` bash
+$ npm install --save nan
+```
+
+Pull in the path to **NAN** in your *binding.gyp* so that you can use `#include ` in your *.cpp* files:
+
+``` python
+"include_dirs" : [
+ "` when compiling your addon.
+
+
+
+## Example
+
+Just getting started with Nan? Take a look at the **[Node Add-on Examples](https://github.com/nodejs/node-addon-examples)**.
+
+Refer to a [quick-start **Nan** Boilerplate](https://github.com/fcanas/node-native-boilerplate) for a ready-to-go project that utilizes basic Nan functionality.
+
+For a simpler example, see the **[async pi estimation example](https://github.com/nodejs/nan/tree/master/examples/async_pi_estimate)** in the examples directory for full code and an explanation of what this Monte Carlo Pi estimation example does. Below are just some parts of the full example that illustrate the use of **NAN**.
+
+Yet another example is **[nan-example-eol](https://github.com/CodeCharmLtd/nan-example-eol)**. It shows newline detection implemented as a native addon.
+
+Also take a look at our comprehensive **[C++ test suite](https://github.com/nodejs/nan/tree/master/test/cpp)** which has a plethora of code snippets for your pasting pleasure.
+
+
+
+## API
+
+Additional to the NAN documentation below, please consult:
+
+* [The V8 Getting Started * Guide](https://github.com/v8/v8/wiki/Getting%20Started%20with%20Embedding)
+* [The V8 Embedders * Guide](https://github.com/v8/v8/wiki/Embedder%27s%20Guide)
+* [V8 API Documentation](http://v8docs.nodesource.com/)
+* [Node Add-on Documentation](https://nodejs.org/api/addons.html)
+
+
+
+### JavaScript-accessible methods
+
+A _template_ is a blueprint for JavaScript functions and objects in a context. You can use a template to wrap C++ functions and data structures within JavaScript objects so that they can be manipulated from JavaScript. See the V8 Embedders Guide section on [Templates](https://github.com/v8/v8/wiki/Embedder%27s-Guide#templates) for further information.
+
+In order to expose functionality to JavaScript via a template, you must provide it to V8 in a form that it understands. Across the versions of V8 supported by NAN, JavaScript-accessible method signatures vary widely, NAN fully abstracts method declaration and provides you with an interface that is similar to the most recent V8 API but is backward-compatible with older versions that still use the now-deceased `v8::Argument` type.
+
+* **Method argument types**
+ - Nan::FunctionCallbackInfo
+ - Nan::PropertyCallbackInfo
+ - Nan::ReturnValue
+* **Method declarations**
+ - Method declaration
+ - Getter declaration
+ - Setter declaration
+ - Property getter declaration
+ - Property setter declaration
+ - Property enumerator declaration
+ - Property deleter declaration
+ - Property query declaration
+ - Index getter declaration
+ - Index setter declaration
+ - Index enumerator declaration
+ - Index deleter declaration
+ - Index query declaration
+* Method and template helpers
+ - Nan::SetMethod()
+ - Nan::SetPrototypeMethod()
+ - Nan::SetAccessor()
+ - Nan::SetNamedPropertyHandler()
+ - Nan::SetIndexedPropertyHandler()
+ - Nan::SetTemplate()
+ - Nan::SetPrototypeTemplate()
+ - Nan::SetInstanceTemplate()
+ - Nan::SetCallHandler()
+ - Nan::SetCallAsFunctionHandler()
+
+### Scopes
+
+A _local handle_ is a pointer to an object. All V8 objects are accessed using handles, they are necessary because of the way the V8 garbage collector works.
+
+A handle scope can be thought of as a container for any number of handles. When you've finished with your handles, instead of deleting each one individually you can simply delete their scope.
+
+The creation of `HandleScope` objects is different across the supported versions of V8. Therefore, NAN provides its own implementations that can be used safely across these.
+
+ - Nan::HandleScope
+ - Nan::EscapableHandleScope
+
+Also see the V8 Embedders Guide section on [Handles and Garbage Collection](https://github.com/v8/v8/wiki/Embedder%27s%20Guide#handles-and-garbage-collection).
+
+### Persistent references
+
+An object reference that is independent of any `HandleScope` is a _persistent_ reference. Where a `Local` handle only lives as long as the `HandleScope` in which it was allocated, a `Persistent` handle remains valid until it is explicitly disposed.
+
+Due to the evolution of the V8 API, it is necessary for NAN to provide a wrapper implementation of the `Persistent` classes to supply compatibility across the V8 versions supported.
+
+ - Nan::PersistentBase & v8::PersistentBase
+ - Nan::NonCopyablePersistentTraits & v8::NonCopyablePersistentTraits
+ - Nan::CopyablePersistentTraits & v8::CopyablePersistentTraits
+ - Nan::Persistent
+ - Nan::Global
+ - Nan::WeakCallbackInfo
+ - Nan::WeakCallbackType
+
+Also see the V8 Embedders Guide section on [Handles and Garbage Collection](https://developers.google.com/v8/embed#handles).
+
+### New
+
+NAN provides a `Nan::New()` helper for the creation of new JavaScript objects in a way that's compatible across the supported versions of V8.
+
+ - Nan::New()
+ - Nan::Undefined()
+ - Nan::Null()
+ - Nan::True()
+ - Nan::False()
+ - Nan::EmptyString()
+
+
+### Converters
+
+NAN contains functions that convert `v8::Value`s to other `v8::Value` types and native types. Since type conversion is not guaranteed to succeed, they return `Nan::Maybe` types. These converters can be used in place of `value->ToX()` and `value->XValue()` (where `X` is one of the types, e.g. `Boolean`) in a way that provides a consistent interface across V8 versions. Newer versions of V8 use the new `v8::Maybe` and `v8::MaybeLocal` types for these conversions, older versions don't have this functionality so it is provided by NAN.
+
+ - Nan::To()
+
+### Maybe Types
+
+The `Nan::MaybeLocal` and `Nan::Maybe` types are monads that encapsulate `v8::Local` handles that _may be empty_.
+
+* **Maybe Types**
+ - Nan::MaybeLocal
+ - Nan::Maybe
+ - Nan::Nothing
+ - Nan::Just
+* **Maybe Helpers**
+ - Nan::Call()
+ - Nan::ToDetailString()
+ - Nan::ToArrayIndex()
+ - Nan::Equals()
+ - Nan::NewInstance()
+ - Nan::GetFunction()
+ - Nan::Set()
+ - Nan::DefineOwnProperty()
+ - Nan::ForceSet()
+ - Nan::Get()
+ - Nan::GetPropertyAttributes()
+ - Nan::Has()
+ - Nan::Delete()
+ - Nan::GetPropertyNames()
+ - Nan::GetOwnPropertyNames()
+ - Nan::SetPrototype()
+ - Nan::ObjectProtoToString()
+ - Nan::HasOwnProperty()
+ - Nan::HasRealNamedProperty()
+ - Nan::HasRealIndexedProperty()
+ - Nan::HasRealNamedCallbackProperty()
+ - Nan::GetRealNamedPropertyInPrototypeChain()
+ - Nan::GetRealNamedProperty()
+ - Nan::CallAsFunction()
+ - Nan::CallAsConstructor()
+ - Nan::GetSourceLine()
+ - Nan::GetLineNumber()
+ - Nan::GetStartColumn()
+ - Nan::GetEndColumn()
+ - Nan::CloneElementAt()
+ - Nan::HasPrivate()
+ - Nan::GetPrivate()
+ - Nan::SetPrivate()
+ - Nan::DeletePrivate()
+ - Nan::MakeMaybe()
+
+### Script
+
+NAN provides a `v8::Script` helpers as the API has changed over the supported versions of V8.
+
+ - Nan::CompileScript()
+ - Nan::RunScript()
+
+
+### JSON
+
+The _JSON_ object provides the c++ versions of the methods offered by the `JSON` object in javascript. V8 exposes these methods via the `v8::JSON` object.
+
+ - Nan::JSON.Parse
+ - Nan::JSON.Stringify
+
+Refer to the V8 JSON object in the [V8 documentation](https://v8docs.nodesource.com/node-7.4/da/d6f/classv8_1_1_j_s_o_n.html) for more information about these methods and their arguments.
+
+### Errors
+
+NAN includes helpers for creating, throwing and catching Errors as much of this functionality varies across the supported versions of V8 and must be abstracted.
+
+Note that an Error object is simply a specialized form of `v8::Value`.
+
+Also consult the V8 Embedders Guide section on [Exceptions](https://developers.google.com/v8/embed#exceptions) for more information.
+
+ - Nan::Error()
+ - Nan::RangeError()
+ - Nan::ReferenceError()
+ - Nan::SyntaxError()
+ - Nan::TypeError()
+ - Nan::ThrowError()
+ - Nan::ThrowRangeError()
+ - Nan::ThrowReferenceError()
+ - Nan::ThrowSyntaxError()
+ - Nan::ThrowTypeError()
+ - Nan::FatalException()
+ - Nan::ErrnoException()
+ - Nan::TryCatch
+
+
+### Buffers
+
+NAN's `node::Buffer` helpers exist as the API has changed across supported Node versions. Use these methods to ensure compatibility.
+
+ - Nan::NewBuffer()
+ - Nan::CopyBuffer()
+ - Nan::FreeCallback()
+
+### Nan::Callback
+
+`Nan::Callback` makes it easier to use `v8::Function` handles as callbacks. A class that wraps a `v8::Function` handle, protecting it from garbage collection and making it particularly useful for storage and use across asynchronous execution.
+
+ - Nan::Callback
+
+### Asynchronous work helpers
+
+`Nan::AsyncWorker`, `Nan::AsyncProgressWorker` and `Nan::AsyncProgressQueueWorker` are helper classes that make working with asynchronous code easier.
+
+ - Nan::AsyncWorker
+ - Nan::AsyncProgressWorkerBase & Nan::AsyncProgressWorker
+ - Nan::AsyncProgressQueueWorker
+ - Nan::AsyncQueueWorker
+
+### Strings & Bytes
+
+Miscellaneous string & byte encoding and decoding functionality provided for compatibility across supported versions of V8 and Node. Implemented by NAN to ensure that all encoding types are supported, even for older versions of Node where they are missing.
+
+ - Nan::Encoding
+ - Nan::Encode()
+ - Nan::DecodeBytes()
+ - Nan::DecodeWrite()
+
+
+### Object Wrappers
+
+The `ObjectWrap` class can be used to make wrapped C++ objects and a factory of wrapped objects.
+
+ - Nan::ObjectWrap
+
+
+### V8 internals
+
+The hooks to access V8 internals—including GC and statistics—are different across the supported versions of V8, therefore NAN provides its own hooks that call the appropriate V8 methods.
+
+ - NAN_GC_CALLBACK()
+ - Nan::AddGCEpilogueCallback()
+ - Nan::RemoveGCEpilogueCallback()
+ - Nan::AddGCPrologueCallback()
+ - Nan::RemoveGCPrologueCallback()
+ - Nan::GetHeapStatistics()
+ - Nan::SetCounterFunction()
+ - Nan::SetCreateHistogramFunction()
+ - Nan::SetAddHistogramSampleFunction()
+ - Nan::IdleNotification()
+ - Nan::LowMemoryNotification()
+ - Nan::ContextDisposedNotification()
+ - Nan::GetInternalFieldPointer()
+ - Nan::SetInternalFieldPointer()
+ - Nan::AdjustExternalMemory()
+
+
+### Miscellaneous V8 Helpers
+
+ - Nan::Utf8String
+ - Nan::GetCurrentContext()
+ - Nan::SetIsolateData()
+ - Nan::GetIsolateData()
+ - Nan::TypedArrayContents
+
+
+### Miscellaneous Node Helpers
+
+ - Nan::AsyncResource
+ - Nan::MakeCallback()
+ - NAN_MODULE_INIT()
+ - Nan::Export()
+
+
+
+
+
+
+### Tests
+
+To run the NAN tests do:
+
+``` sh
+npm install
+npm run-script rebuild-tests
+npm test
+```
+
+Or just:
+
+``` sh
+npm install
+make test
+```
+
+
+
+## Known issues
+
+### Compiling against Node.js 0.12 on OSX
+
+With new enough compilers available on OSX, the versions of V8 headers corresponding to Node.js 0.12
+do not compile anymore. The error looks something like:
+
+```
+❯ CXX(target) Release/obj.target/accessors/cpp/accessors.o
+In file included from ../cpp/accessors.cpp:9:
+In file included from ../../nan.h:51:
+In file included from /Users/ofrobots/.node-gyp/0.12.18/include/node/node.h:61:
+/Users/ofrobots/.node-gyp/0.12.18/include/node/v8.h:5800:54: error: 'CreateHandle' is a protected member of 'v8::HandleScope'
+ return Handle(reinterpret_cast(HandleScope::CreateHandle(
+ ~~~~~~~~~~~~~^~~~~~~~~~~~
+```
+
+This can be worked around by patching your local versions of v8.h corresponding to Node 0.12 to make
+`v8::Handle` a friend of `v8::HandleScope`. Since neither Node.js not V8 support this release line anymore
+this patch cannot be released by either project in an official release.
+
+For this reason, we do not test against Node.js 0.12 on OSX in this project's CI. If you need to support
+that configuration, you will need to either get an older compiler, or apply a source patch to the version
+of V8 headers as a workaround.
+
+
+
+## Governance & Contributing
+
+NAN is governed by the [Node.js Addon API Working Group](https://github.com/nodejs/CTC/blob/master/WORKING_GROUPS.md#addon-api)
+
+### Addon API Working Group (WG)
+
+The NAN project is jointly governed by a Working Group which is responsible for high-level guidance of the project.
+
+Members of the WG are also known as Collaborators, there is no distinction between the two, unlike other Node.js projects.
+
+The WG has final authority over this project including:
+
+* Technical direction
+* Project governance and process (including this policy)
+* Contribution policy
+* GitHub repository hosting
+* Maintaining the list of additional Collaborators
+
+For the current list of WG members, see the project [README.md](./README.md#collaborators).
+
+Individuals making significant and valuable contributions are made members of the WG and given commit-access to the project. These individuals are identified by the WG and their addition to the WG is discussed via GitHub and requires unanimous consensus amongst those WG members participating in the discussion with a quorum of 50% of WG members required for acceptance of the vote.
+
+_Note:_ If you make a significant contribution and are not considered for commit-access log an issue or contact a WG member directly.
+
+For the current list of WG members / Collaborators, see the project [README.md](./README.md#collaborators).
+
+### Consensus Seeking Process
+
+The WG follows a [Consensus Seeking](http://en.wikipedia.org/wiki/Consensus-seeking_decision-making) decision making model.
+
+Modifications of the contents of the NAN repository are made on a collaborative basis. Anybody with a GitHub account may propose a modification via pull request and it will be considered by the WG. All pull requests must be reviewed and accepted by a WG member with sufficient expertise who is able to take full responsibility for the change. In the case of pull requests proposed by an existing WG member, an additional WG member is required for sign-off. Consensus should be sought if additional WG members participate and there is disagreement around a particular modification.
+
+If a change proposal cannot reach a consensus, a WG member can call for a vote amongst the members of the WG. Simple majority wins.
+
+
+
+## Developer's Certificate of Origin 1.1
+
+By making a contribution to this project, I certify that:
+
+* (a) The contribution was created in whole or in part by me and I
+ have the right to submit it under the open source license
+ indicated in the file; or
+
+* (b) The contribution is based upon previous work that, to the best
+ of my knowledge, is covered under an appropriate open source
+ license and I have the right under that license to submit that
+ work with modifications, whether created in whole or in part
+ by me, under the same open source license (unless I am
+ permitted to submit under a different license), as indicated
+ in the file; or
+
+* (c) The contribution was provided directly to me by some other
+ person who certified (a), (b) or (c) and I have not modified
+ it.
+
+* (d) I understand and agree that this project and the contribution
+ are public and that a record of the contribution (including all
+ personal information I submit with it, including my sign-off) is
+ maintained indefinitely and may be redistributed consistent with
+ this project or the open source license(s) involved.
+
+
+
+### WG Members / Collaborators
+
+
+
+## Licence & copyright
+
+Copyright (c) 2018 NAN WG Members / Collaborators (listed above).
+
+Native Abstractions for Node.js is licensed under an MIT license. All rights not explicitly granted in the MIT license are reserved. See the included LICENSE file for more details.
diff --git a/node_modules/nan/doc/asyncworker.md b/node_modules/nan/doc/asyncworker.md
new file mode 100644
index 0000000..239de80
--- /dev/null
+++ b/node_modules/nan/doc/asyncworker.md
@@ -0,0 +1,146 @@
+## Asynchronous work helpers
+
+`Nan::AsyncWorker`, `Nan::AsyncProgressWorker` and `Nan::AsyncProgressQueueWorker` are helper classes that make working with asynchronous code easier.
+
+ - Nan::AsyncWorker
+ - Nan::AsyncProgressWorkerBase & Nan::AsyncProgressWorker
+ - Nan::AsyncProgressQueueWorker
+ - Nan::AsyncQueueWorker
+
+
+### Nan::AsyncWorker
+
+`Nan::AsyncWorker` is an _abstract_ class that you can subclass to have much of the annoying asynchronous queuing and handling taken care of for you. It can even store arbitrary V8 objects for you and have them persist while the asynchronous work is in progress.
+
+This class internally handles the details of creating an [`AsyncResource`][AsyncResource], and running the callback in the
+correct async context. To be able to identify the async resources created by this class in async-hooks, provide a
+`resource_name` to the constructor. It is recommended that the module name be used as a prefix to the `resource_name` to avoid
+collisions in the names. For more details see [`AsyncResource`][AsyncResource] documentation. The `resource_name` needs to stay valid for the lifetime of the worker instance.
+
+Definition:
+
+```c++
+class AsyncWorker {
+ public:
+ explicit AsyncWorker(Callback *callback_, const char* resource_name = "nan:AsyncWorker");
+
+ virtual ~AsyncWorker();
+
+ virtual void WorkComplete();
+
+ void SaveToPersistent(const char *key, const v8::Local &value);
+
+ void SaveToPersistent(const v8::Local &key,
+ const v8::Local &value);
+
+ void SaveToPersistent(uint32_t index,
+ const v8::Local &value);
+
+ v8::Local GetFromPersistent(const char *key) const;
+
+ v8::Local GetFromPersistent(const v8::Local &key) const;
+
+ v8::Local GetFromPersistent(uint32_t index) const;
+
+ virtual void Execute() = 0;
+
+ uv_work_t request;
+
+ virtual void Destroy();
+
+ protected:
+ Persistent persistentHandle;
+
+ Callback *callback;
+
+ virtual void HandleOKCallback();
+
+ virtual void HandleErrorCallback();
+
+ void SetErrorMessage(const char *msg);
+
+ const char* ErrorMessage();
+};
+```
+
+
+### Nan::AsyncProgressWorkerBase & Nan::AsyncProgressWorker
+
+`Nan::AsyncProgressWorkerBase` is an _abstract_ class template that extends `Nan::AsyncWorker` and adds additional progress reporting callbacks that can be used during the asynchronous work execution to provide progress data back to JavaScript.
+
+Previously the definiton of `Nan::AsyncProgressWorker` only allowed sending `const char` data. Now extending `Nan::AsyncProgressWorker` will yield an instance of the implicit `Nan::AsyncProgressWorkerBase` template with type `` for compatibility.
+
+`Nan::AsyncProgressWorkerBase` & `Nan::AsyncProgressWorker` is intended for best-effort delivery of nonessential progress messages, e.g. a progress bar. The last event sent before the main thread is woken will be delivered.
+
+Definition:
+
+```c++
+template
+class AsyncProgressWorkerBase : public AsyncWorker {
+ public:
+ explicit AsyncProgressWorkerBase(Callback *callback_, const char* resource_name = ...);
+
+ virtual ~AsyncProgressWorkerBase();
+
+ void WorkProgress();
+
+ class ExecutionProgress {
+ public:
+ void Signal() const;
+ void Send(const T* data, size_t count) const;
+ };
+
+ virtual void Execute(const ExecutionProgress& progress) = 0;
+
+ virtual void HandleProgressCallback(const T *data, size_t count) = 0;
+
+ virtual void Destroy();
+};
+
+typedef AsyncProgressWorkerBase AsyncProgressWorker;
+```
+
+
+### Nan::AsyncProgressQueueWorker
+
+`Nan::AsyncProgressQueueWorker` is an _abstract_ class template that extends `Nan::AsyncWorker` and adds additional progress reporting callbacks that can be used during the asynchronous work execution to provide progress data back to JavaScript.
+
+`Nan::AsyncProgressQueueWorker` behaves exactly the same as `Nan::AsyncProgressWorker`, except all events are queued and delivered to the main thread.
+
+Definition:
+
+```c++
+template
+class AsyncProgressQueueWorker : public AsyncWorker {
+ public:
+ explicit AsyncProgressQueueWorker(Callback *callback_, const char* resource_name = "nan:AsyncProgressQueueWorker");
+
+ virtual ~AsyncProgressQueueWorker();
+
+ void WorkProgress();
+
+ class ExecutionProgress {
+ public:
+ void Send(const T* data, size_t count) const;
+ };
+
+ virtual void Execute(const ExecutionProgress& progress) = 0;
+
+ virtual void HandleProgressCallback(const T *data, size_t count) = 0;
+
+ virtual void Destroy();
+};
+```
+
+
+### Nan::AsyncQueueWorker
+
+`Nan::AsyncQueueWorker` will run a `Nan::AsyncWorker` asynchronously via libuv. Both the `execute` and `after_work` steps are taken care of for you. Most of the logic for this is embedded in `Nan::AsyncWorker`.
+
+Definition:
+
+```c++
+void AsyncQueueWorker(AsyncWorker *);
+```
+
+[AsyncResource]: "node_misc.html#api_nan_asyncresource"
diff --git a/node_modules/nan/doc/buffers.md b/node_modules/nan/doc/buffers.md
new file mode 100644
index 0000000..8d8d25c
--- /dev/null
+++ b/node_modules/nan/doc/buffers.md
@@ -0,0 +1,54 @@
+## Buffers
+
+NAN's `node::Buffer` helpers exist as the API has changed across supported Node versions. Use these methods to ensure compatibility.
+
+ - Nan::NewBuffer()
+ - Nan::CopyBuffer()
+ - Nan::FreeCallback()
+
+
+### Nan::NewBuffer()
+
+Allocate a new `node::Buffer` object with the specified size and optional data. Calls `node::Buffer::New()`.
+
+Note that when creating a `Buffer` using `Nan::NewBuffer()` and an existing `char*`, it is assumed that the ownership of the pointer is being transferred to the new `Buffer` for management.
+When a `node::Buffer` instance is garbage collected and a `FreeCallback` has not been specified, `data` will be disposed of via a call to `free()`.
+You _must not_ free the memory space manually once you have created a `Buffer` in this way.
+
+Signature:
+
+```c++
+Nan::MaybeLocal Nan::NewBuffer(uint32_t size)
+Nan::MaybeLocal Nan::NewBuffer(char* data, uint32_t size)
+Nan::MaybeLocal Nan::NewBuffer(char *data,
+ size_t length,
+ Nan::FreeCallback callback,
+ void *hint)
+```
+
+
+
+### Nan::CopyBuffer()
+
+Similar to [`Nan::NewBuffer()`](#api_nan_new_buffer) except that an implicit memcpy will occur within Node. Calls `node::Buffer::Copy()`.
+
+Management of the `char*` is left to the user, you should manually free the memory space if necessary as the new `Buffer` will have its own copy.
+
+Signature:
+
+```c++
+Nan::MaybeLocal Nan::CopyBuffer(const char *data, uint32_t size)
+```
+
+
+
+### Nan::FreeCallback()
+
+A free callback that can be provided to [`Nan::NewBuffer()`](#api_nan_new_buffer).
+The supplied callback will be invoked when the `Buffer` undergoes garbage collection.
+
+Signature:
+
+```c++
+typedef void (*FreeCallback)(char *data, void *hint);
+```
diff --git a/node_modules/nan/doc/callback.md b/node_modules/nan/doc/callback.md
new file mode 100644
index 0000000..f7af0bf
--- /dev/null
+++ b/node_modules/nan/doc/callback.md
@@ -0,0 +1,76 @@
+## Nan::Callback
+
+`Nan::Callback` makes it easier to use `v8::Function` handles as callbacks. A class that wraps a `v8::Function` handle, protecting it from garbage collection and making it particularly useful for storage and use across asynchronous execution.
+
+ - Nan::Callback
+
+
+### Nan::Callback
+
+```c++
+class Callback {
+ public:
+ Callback();
+
+ explicit Callback(const v8::Local &fn);
+
+ ~Callback();
+
+ bool operator==(const Callback &other) const;
+
+ bool operator!=(const Callback &other) const;
+
+ v8::Local operator*() const;
+
+ MaybeLocal operator()(AsyncResource* async_resource,
+ v8::Local target,
+ int argc = 0,
+ v8::Local argv[] = 0) const;
+
+ MaybeLocal operator()(AsyncResource* async_resource,
+ int argc = 0,
+ v8::Local argv[] = 0) const;
+
+ void SetFunction(const v8::Local &fn);
+
+ v8::Local GetFunction() const;
+
+ bool IsEmpty() const;
+
+ void Reset(const v8::Local &fn);
+
+ void Reset();
+
+ MaybeLocal Call(v8::Local target,
+ int argc,
+ v8::Local argv[],
+ AsyncResource* async_resource) const;
+ MaybeLocal Call(int argc,
+ v8::Local argv[],
+ AsyncResource* async_resource) const;
+
+ // Deprecated versions. Use the versions that accept an async_resource instead
+ // as they run the callback in the correct async context as specified by the
+ // resource. If you want to call a synchronous JS function (i.e. on a
+ // non-empty JS stack), you can use Nan::Call instead.
+ v8::Local operator()(v8::Local target,
+ int argc = 0,
+ v8::Local argv[] = 0) const;
+
+ v8::Local operator()(int argc = 0,
+ v8::Local argv[] = 0) const;
+ v8::Local Call(v8::Local target,
+ int argc,
+ v8::Local argv[]) const;
+
+ v8::Local Call(int argc, v8::Local argv[]) const;
+};
+```
+
+Example usage:
+
+```c++
+v8::Local function;
+Nan::Callback callback(function);
+callback.Call(0, 0);
+```
diff --git a/node_modules/nan/doc/converters.md b/node_modules/nan/doc/converters.md
new file mode 100644
index 0000000..d20861b
--- /dev/null
+++ b/node_modules/nan/doc/converters.md
@@ -0,0 +1,41 @@
+## Converters
+
+NAN contains functions that convert `v8::Value`s to other `v8::Value` types and native types. Since type conversion is not guaranteed to succeed, they return `Nan::Maybe` types. These converters can be used in place of `value->ToX()` and `value->XValue()` (where `X` is one of the types, e.g. `Boolean`) in a way that provides a consistent interface across V8 versions. Newer versions of V8 use the new `v8::Maybe` and `v8::MaybeLocal` types for these conversions, older versions don't have this functionality so it is provided by NAN.
+
+ - Nan::To()
+
+
+### Nan::To()
+
+Converts a `v8::Local` to a different subtype of `v8::Value` or to a native data type. Returns a `Nan::MaybeLocal<>` or a `Nan::Maybe<>` accordingly.
+
+See [maybe_types.md](./maybe_types.md) for more information on `Nan::Maybe` types.
+
+Signatures:
+
+```c++
+// V8 types
+Nan::MaybeLocal Nan::To(v8::Local val);
+Nan::MaybeLocal Nan::To(v8::Local val);
+Nan::MaybeLocal Nan::To(v8::Local val);
+Nan::MaybeLocal Nan::To(v8::Local val);
+Nan::MaybeLocal Nan::To(v8::Local val);
+Nan::MaybeLocal Nan::To(v8::Local val);
+Nan::MaybeLocal Nan::To(v8::Local val);
+
+// Native types
+Nan::Maybe Nan::To(v8::Local val);
+Nan::Maybe