diff --git a/docs/Sensor/SeeedStudio_XIAO/SeeedStudio_XIAO_ESP32S3/XIAO-ESP32S3-FreeRTOS.md b/docs/Sensor/SeeedStudio_XIAO/SeeedStudio_XIAO_ESP32S3/XIAO-ESP32S3-FreeRTOS.md
index c3aa18050a4d..29f135d41a58 100644
--- a/docs/Sensor/SeeedStudio_XIAO/SeeedStudio_XIAO_ESP32S3/XIAO-ESP32S3-FreeRTOS.md
+++ b/docs/Sensor/SeeedStudio_XIAO/SeeedStudio_XIAO_ESP32S3/XIAO-ESP32S3-FreeRTOS.md
@@ -2,12 +2,15 @@
description: XIAO ESP32S3(Sense) With FreeRTOS
title: XIAO ESP32S3(Sense) With FreeRTOS
keywords:
- - Software, FreeRtos
+ - Software
+ - FreeRtos
+ - sd
+ - camera
image: https://files.seeedstudio.com/wiki/wiki-platform/S-tempor.png
slug: /xiao-esp32s3-freertos
last_update:
- date: 09/09/2024
- author: Priyanshu_Roy
+ date: 09/14/2024
+ author: Priyanshu Roy
---
# XIAO ESP32S3(Sense) With FreeRTOS
@@ -163,7 +166,7 @@ xTaskCreate(
configMINIMAL_STACK_SIZE, /* Stack size in words, or bytes. */
NULL, /* Parameter passed into the task. */
tskIDLE_PRIORITY, /* Priority at which the task is created. */
- &task1 /* Used to pass out the created task's handle. */
+ &task /* Used to pass out the created task's handle. */
);
```
@@ -197,7 +200,7 @@ void taskFunction(void * pvParameters) {
## Visualization of tasks
-I am creating four simple task to visualize how the FreeRTOS works.
+I am creating four simple task to visualize how the FreeRTOS works.
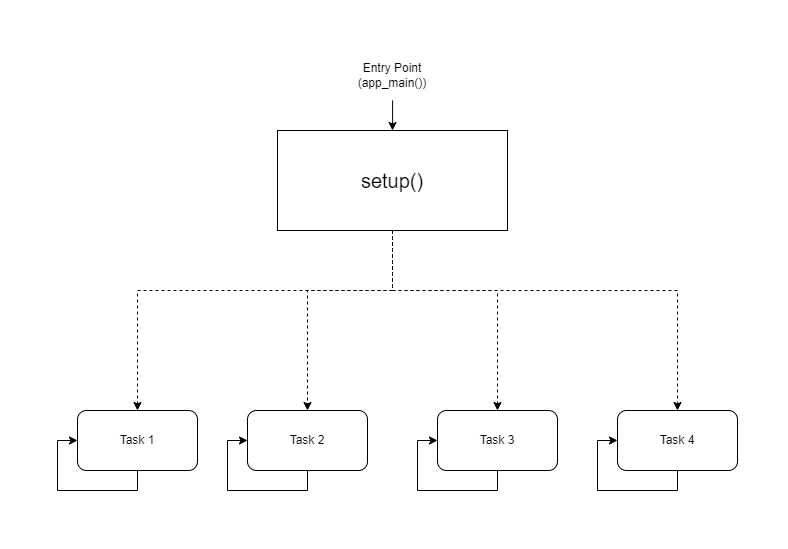
@@ -370,7 +373,7 @@ For this I am using an analog sensor [Air Quality Sensor v1.3](https://www.seeed
### Hardware Setup
-Attach the Xiao-S3 to the [Grove - Expansion Board](https://www.seeedstudio.com/Seeeduino-XIAO-Expansion-board-p-4746.html) and connect the [Air Quality Sensor v1.3](https://www.seeedstudio.com/Grove-Air-Quality-Sensor-v1-3-Arduino-Compatible.html) to the digital connector.
+Attach the Xiao-S3 to the [Grove - Expansion Board](https://www.seeedstudio.com/Seeeduino-XIAO-Expansion-board-p-4746.html) and connect the [Air Quality Sensor v1.3](https://www.seeedstudio.com/Grove-Air-Quality-Sensor-v1-3-Arduino-Compatible.html) to the analog connector.
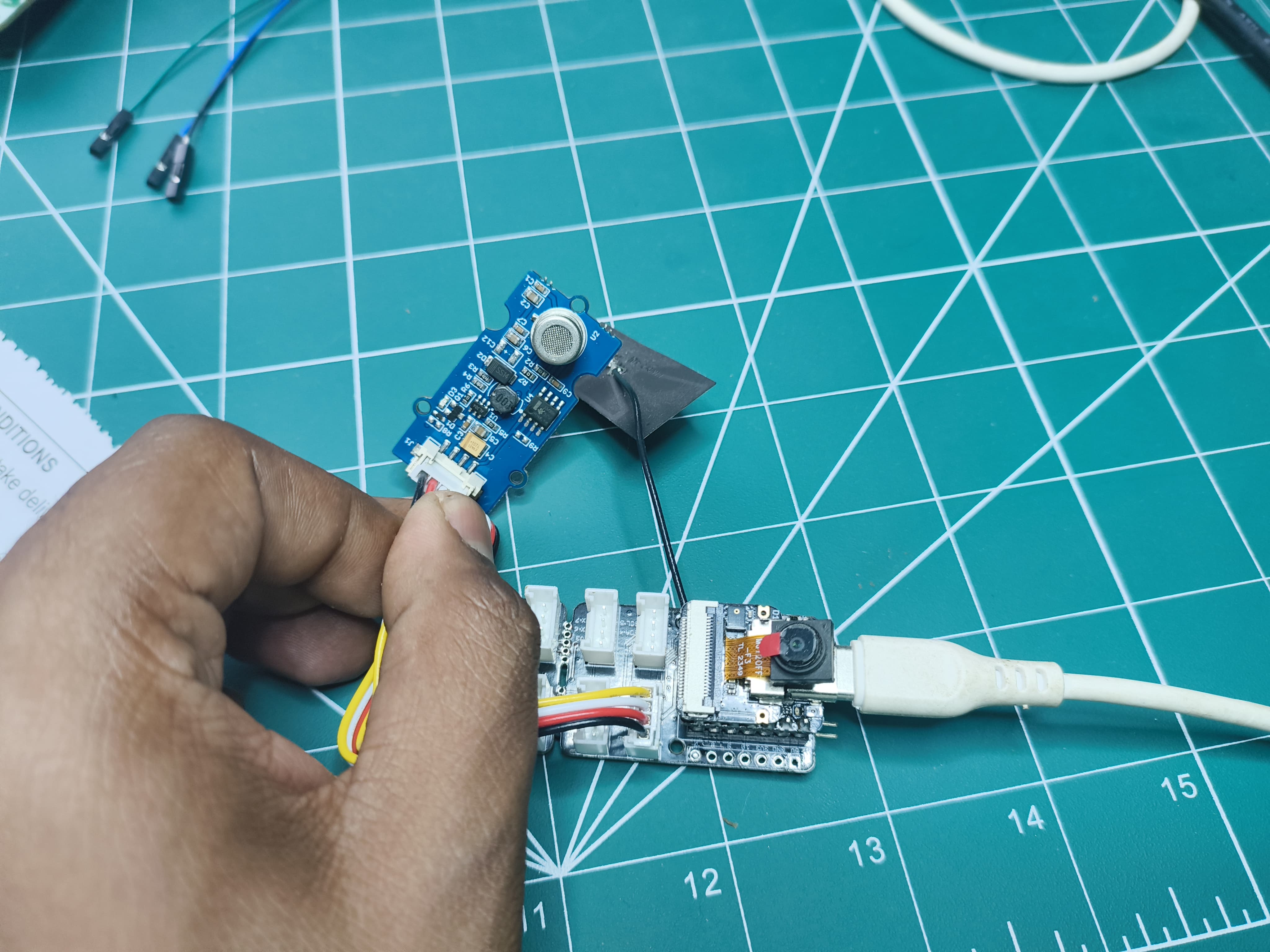
@@ -386,20 +389,91 @@ This code is designed to collect air quality data from a sensor, process the raw
#### Key Components:
- Sensor Initialization:
- - sensor_setup() function configures the sensor's I/O pins and ADC unit.
- - It attempts to initialize the sensor using initialize_air_quality_sensor().
- - If initialization is successful, the sensor is ready for data collection.
+
+```c
+air_quality_sensor_t air_quality_sensor;
+
+void sensor_setup()
+{
+ air_quality_sensor._io_num = ADC_CHANNEL_0;
+ air_quality_sensor._adc_num = ADC_UNIT_1;
+ printf("Starting Air Quality Sensor...\n");
+ if(!initialize_air_quality_sensor(&air_quality_sensor))
+ {
+ printf("Sensor ready.\n");
+ }
+ else{
+ printf("Sensor ERROR!\n");
+ }
+}
+```
+
+- sensor_setup() function configures the sensor's I/O pins and ADC unit.
+- It attempts to initialize the sensor using initialize_air_quality_sensor().
+- If initialization is successful, the sensor is ready for data collection.
- Data Collection Task:
- - poll_read_air_quality_sensor() task is created to continuously read raw data from the sensor.
- - It calls air_quality_sensor_slope() to process the raw data and calculate the slope, which is an indicator of air quality.
- - The task delays for 500 milliseconds before reading the next data point.
+
+```c
+void poll_read_air_quality_sensor(void *pvParameters)
+{
+ for (;;)
+ {
+ air_quality_sensor_slope(&air_quality_sensor);
+ vTaskDelay(500 / portTICK_PERIOD_MS);
+ }
+}
+```
+
+- poll_read_air_quality_sensor() task is created to continuously read raw data from the sensor.
+- It calls air_quality_sensor_slope() to process the raw data and calculate the slope, which is an indicator of air quality.
+- The task delays for 500 milliseconds before reading the next data point.
- Data Printing Task:
- - print_read_air_quality_sensor() task is created to periodically print the collected data and calculated air quality.
- - It retrieves the current time, slope, raw value, and air quality message using air_quality_error_to_message().
- - The task prints the data to the console in a formatted manner.
- - The task delays for 1000 milliseconds before printing the next data point.
+
+```c
+
+void print_read_air_quality_sensor(void *pvParameters)
+{
+ for (;;)
+ {
+ char buf[40];
+ air_quality_error_to_message(air_quality_sensor._air_quality,buf);
+ printf("Time : %lu\tSlope : %d\tRaw Value : %d\n%s\n", (uint32_t)esp_timer_get_time() / 1000, air_quality_sensor._air_quality, air_quality_sensor._sensor_raw_value,buf);
+ vTaskDelay(1000 / portTICK_PERIOD_MS);
+ }
+}
+```
+
+- print_read_air_quality_sensor() task is created to periodically print the collected data and calculated air quality.
+- It retrieves the current time, slope, raw value, and air quality message using air_quality_error_to_message().
+- The task prints the data to the console in a formatted manner.
+- The task delays for 1000 milliseconds before printing the next data point.
+
+```c
+
+void app_main(void)
+{
+ sensor_setup();
+ xTaskCreatePinnedToCore(
+ poll_read_air_quality_sensor, /* Function that implements the task. */
+ "poll_read_air_quality_sensor", /* Text name for the task. */
+ configMINIMAL_STACK_SIZE * 2, /* Stack size in words, not bytes. */
+ NULL, /* Parameter passed into the task. */
+ tskIDLE_PRIORITY, /* Priority at which the task is created. */
+ NULL, /* Used to pass out the created task's handle. */
+ 0); /* Core ID */
+
+ xTaskCreatePinnedToCore(
+ print_read_air_quality_sensor, /* Function that implements the task. */
+ "print_read_air_quality_sensor", /* Text name for the task. */
+ configMINIMAL_STACK_SIZE * 2, /* Stack size in words, not bytes. */
+ NULL, /* Parameter passed into the task. */
+ tskIDLE_PRIORITY + 1, /* Priority at which the task is created. */
+ NULL, /* Used to pass out the created task's handle. */
+ 0); /* Core ID */
+}
+```
### Output
@@ -436,16 +510,478 @@ Time : 51347 Slope : 3 Raw Value : 235
Fresh air.
```
+## Camera and SdCard usage in FreeRTOS
+
+For this I am using the onBoard Camera and SdCard along with ESP_IDF_v5.3.
+
+
+
+### Hardware Setup
+
+Follow the [microSD card guide](https://wiki.seeedstudio.com/xiao_esp32s3_sense_filesystem/) and [camera guide](https://wiki.seeedstudio.com/xiao_esp32s3_camera_usage/) to attach the camera and microSD card extension board to the
+
+- Format microSD card (supported up to 32Gb)
+- Attach the microSD card to the extension board
+
+The setup would look something like this :
+
+
+
+
+ Front |
+ Back |
+
+
+ |
+ |
+
+
+
+
+### Software Setup
+
+After pulling the git repository, open the folder in VSCode. Go to View->Command Palette->ESP-IDF: Add vscode Configuration Folder.
+From the bottom panel select the correct COM port, chip (ESP-S3) and build,flash and monitor.
+
+### Camera Component
+
+- Camera Configuration:
+ - Defines the GPIO pins used for various camera functions (PWDN, RESET, XCLK, SIOD, SIOC, Y9-Y2, VSYNC, HREF, PCLK, LED).
+ - Sets default values for camera parameters (e.g., clock frequency, frame buffer location, pixel format, frame size, JPEG quality, frame buffer count, grab mode).
+
+```c
+#ifndef CAMERA_CONFIG_H
+#define CAMERA_CONFIG_H
+
+#define PWDN_GPIO_NUM -1
+#define RESET_GPIO_NUM -1
+#define XCLK_GPIO_NUM 10
+#define SIOD_GPIO_NUM 40
+#define SIOC_GPIO_NUM 39
+
+#define Y9_GPIO_NUM 48
+#define Y8_GPIO_NUM 11
+#define Y7_GPIO_NUM 12
+#define Y6_GPIO_NUM 14
+#define Y5_GPIO_NUM 16
+#define Y4_GPIO_NUM 18
+#define Y3_GPIO_NUM 17
+#define Y2_GPIO_NUM 15
+#define VSYNC_GPIO_NUM 38
+#define HREF_GPIO_NUM 47
+#define PCLK_GPIO_NUM 13
+
+#define LED_GPIO_NUM 21
+
+#endif //CAMERA_CONFIG_H
+```
+
+- Camera Interface:
+ Declares functions initialize_camera() and createCameraTask().
+
+- Camera Implementation:
+
+ - Initializes the camera using the defined configuration.
+
+ ```c
+ void initialize_camera(void)
+ {
+ camera_config_t camera_config = {
+ .pin_pwdn = PWDN_GPIO_NUM,
+ .pin_reset = RESET_GPIO_NUM,
+ .pin_xclk = XCLK_GPIO_NUM,
+ .pin_sccb_sda = SIOD_GPIO_NUM,
+ .pin_sccb_scl = SIOC_GPIO_NUM,
+ .pin_d7 = Y9_GPIO_NUM,
+ .pin_d6 = Y8_GPIO_NUM,
+ .pin_d5 = Y7_GPIO_NUM,
+ .pin_d4 = Y6_GPIO_NUM,
+ .pin_d3 = Y5_GPIO_NUM,
+ .pin_d2 = Y4_GPIO_NUM,
+ .pin_d1 = Y3_GPIO_NUM,
+ .pin_d0 = Y2_GPIO_NUM,
+ .pin_vsync = VSYNC_GPIO_NUM,
+ .pin_href = HREF_GPIO_NUM,
+ .pin_pclk = PCLK_GPIO_NUM,
+
+ .xclk_freq_hz = 20000000, // The clock frequency of the image sensor
+ .fb_location = CAMERA_FB_IN_PSRAM, // Set the frame buffer storage location
+ .pixel_format = PIXFORMAT_JPEG, // The pixel format of the image: PIXFORMAT_ + YUV422|GRAYSCALE|RGB565|JPEG
+ .frame_size = FRAMESIZE_UXGA, // The resolution size of the image: FRAMESIZE_ + QVGA|CIF|VGA|SVGA|XGA|SXGA|UXGA
+ .jpeg_quality = 15, // The quality of the JPEG image, ranging from 0 to 63.
+ .fb_count = 2, // The number of frame buffers to use.
+ .grab_mode = CAMERA_GRAB_LATEST // The image capture mode.
+ };
+
+ esp_err_t ret = esp_camera_init(&camera_config);
+ if (ret == ESP_OK)
+ {
+ ESP_LOGI(cameraTag, "Camera configured successful");
+ }
+ else
+ {
+ ESP_LOGI(cameraTag, "Camera configured unsuccessful");
+ return;
+ }
+ }
+ ```
+
+ - Sets camera parameters (brightness, contrast, saturation, special effect, white balance, exposure control, AEC, AE level, AEC value, gain control, AGC gain, gain ceiling, BPC, WPC, raw GMA, LENC, hmirror, vflip, DCW, colorbar).
+
+ ```c
+ sensor_t *s = esp_camera_sensor_get();
+
+ s->set_brightness(s, 0); // -2 to 2
+ s->set_contrast(s, 0); // -2 to 2
+ s->set_saturation(s, 0); // -2 to 2
+ s->set_special_effect(s, 0); // 0 to 6 (0 - No Effect, 1 - Negative, 2 - Grayscale, 3 - Red Tint, 4 - Green Tint, 5 - Blue Tint, 6 - Sepia)
+ s->set_whitebal(s, 1); // 0 = disable , 1 = enable
+ s->set_awb_gain(s, 1); // 0 = disable , 1 = enable
+ s->set_wb_mode(s, 0); // 0 to 4 - if awb_gain enabled (0 - Auto, 1 - Sunny, 2 - Cloudy, 3 - Office, 4 - Home)
+ s->set_exposure_ctrl(s, 1); // 0 = disable , 1 = enable
+ s->set_aec2(s, 0); // 0 = disable , 1 = enable
+ s->set_ae_level(s, 0); // -2 to 2
+ s->set_aec_value(s, 300); // 0 to 1200
+ s->set_gain_ctrl(s, 1); // 0 = disable , 1 = enable
+ s->set_agc_gain(s, 0); // 0 to 30
+ s->set_gainceiling(s, (gainceiling_t)0); // 0 to 6
+ s->set_bpc(s, 0); // 0 = disable , 1 = enable
+ s->set_wpc(s, 1); // 0 = disable , 1 = enable
+ s->set_raw_gma(s, 1); // 0 = disable , 1 = enable
+ s->set_lenc(s, 1); // 0 = disable , 1 = enable
+ s->set_hmirror(s, 0); // 0 = disable , 1 = enable
+ s->set_vflip(s, 0); // 0 = disable , 1 = enable
+ s->set_dcw(s, 1); // 0 = disable , 1 = enable
+ s->set_colorbar(s, 0); // 0 = disable , 1 = enable
+ ```
+
+ - Defines a function takePicture() to capture an image and save it to SD card.
+
+ ```c
+ void takePicture()
+ {
+ ESP_LOGI(cameraTag, "Taking picture...");
+ camera_fb_t *pic = esp_camera_fb_get();
+
+ if (pic)
+ {
+ saveJpegToSdcard(pic);
+ }
+
+ ESP_LOGI(cameraTag, "Picture taken! Its size was: %zu bytes", pic->len);
+
+ esp_camera_fb_return(pic);
+ }
+ ```
+
+ - Creates a task cameraTakePicture_5_sec() to continuously take pictures every 5 seconds.
+
+ ```c
+ void cameraTakePicture_5_sec(void *pvParameters)
+ {
+ for (;;)
+ {
+ takePicture();
+ vTaskDelay(5000 / portTICK_PERIOD_MS);
+ }
+ }
+
+ void createCameraTask()
+ {
+ TaskHandle_t task;
+ xTaskCreate(
+ cameraTakePicture_5_sec, /* Function that implements the task. */
+ "cameraTakePicture_5_sec", /* Text name for the task. */
+ configMINIMAL_STACK_SIZE * 4, /* Stack size in words, or bytes. */
+ NULL, /* Parameter passed into the task. */
+ tskIDLE_PRIORITY, /* Priority at which the task is created. */
+ &task /* Used to pass out the created task's handle. */
+ );
+ }
+ ```
+
+Code Structure:
+
+- Header files (camera_config.h, camera_interface.h) and implementation files (camera_interface.c).
+- The camera_config.h file defines the camera configuration parameters.
+- The camera_interface.h file declares the functions for camera initialization and task creation.
+- The camera_interface.c file implements the camera initialization, picture-taking, and task creation logic.
+
+### SdCard Component
+
+- SD Card Configuration:
+ Defines the GPIO pins used for the SD card interface (MISO, MOSI, CLK, CS).
+
+```c
+#ifndef SDCARD_CONFIG_H
+#define SDCARD_CONFIG_H
+
+#define PIN_NUM_MISO GPIO_NUM_8
+#define PIN_NUM_MOSI GPIO_NUM_9
+#define PIN_NUM_CLK GPIO_NUM_7
+#define PIN_NUM_CS GPIO_NUM_21
+
+#endif //SDCARD_CONFIG_H
+```
+
+- SD Card Interface:
+ Declares functions initialize_sdcard(), deinitialize_sdcard(), and saveJpegToSdcard().
+
+```c
+#ifndef SDCARD_INTERFACE_H
+#define SDCARD_INTERFACE_H
+
+#include "esp_camera.h"
+
+void initialize_sdcard(void);
+void deinitialize_sdcard();
+void saveJpegToSdcard(camera_fb_t *);
+
+#endif //SDCARD_INTERFACE_H
+```
+
+- SD Card Implementation:
+
+ - Initializes the SD card using the defined configuration and mounts the SD card as a FAT filesystem.
+
+ ```c
+ sdmmc_card_t *card;
+ sdmmc_host_t host = SDSPI_HOST_DEFAULT();
+ const char mount_point[] = "/sd";
+
+ void initialize_sdcard()
+ {
+ esp_err_t ret;
+
+ // If format_if_mount_failed is set to true, SD card will be partitioned and
+ // formatted in case when mounting fails.
+ esp_vfs_fat_sdmmc_mount_config_t mount_config = {
+ #ifdef FORMAT_IF_MOUNT_FAILED
+ .format_if_mount_failed = true,
+ #else
+ .format_if_mount_failed = false,
+ #endif // EXAMPLE_FORMAT_IF_MOUNT_FAILED
+ .max_files = 5,
+ .allocation_unit_size = 32 * 1024};
+
+ ESP_LOGI(sdcardTag, "Initializing SD card");
+
+ // Use settings defined above to initialize SD card and mount FAT filesystem.
+ // Note: esp_vfs_fat_sdmmc/sdspi_mount is all-in-one convenience functions.
+ // Please check its source code and implement error recovery when developing
+ // production applications.
+ ESP_LOGI(sdcardTag, "Using SPI peripheral");
+
+ // By default, SD card frequency is initialized to SDMMC_FREQ_DEFAULT (20MHz)
+ // For setting a specific frequency, use host.max_freq_khz (range 400kHz - 20MHz for SDSPI)
+ spi_bus_config_t bus_cfg = {
+ .mosi_io_num = PIN_NUM_MOSI,
+ .miso_io_num = PIN_NUM_MISO,
+ .sclk_io_num = PIN_NUM_CLK,
+ .quadwp_io_num = -1,
+ .quadhd_io_num = -1,
+ .max_transfer_sz = host.max_freq_khz,
+ };
+ ret = spi_bus_initialize(host.slot, &bus_cfg, SDSPI_DEFAULT_DMA);
+ if (ret != ESP_OK)
+ {
+ ESP_LOGE(sdcardTag, "Failed to initialize bus.");
+ return;
+ }
+
+ // This initializes the slot without card detect (CD) and write protect (WP) signals.
+ // Modify slot_config.gpio_cd and slot_config.gpio_wp if your board has these signals.
+ sdspi_device_config_t slot_config = SDSPI_DEVICE_CONFIG_DEFAULT();
+ slot_config.gpio_cs = PIN_NUM_CS;
+ slot_config.host_id = host.slot;
+
+ ESP_LOGI(sdcardTag, "Mounting filesystem");
+ ret = esp_vfs_fat_sdspi_mount(mount_point, &host, &slot_config, &mount_config, &card);
+
+ if (ret != ESP_OK)
+ {
+ if (ret == ESP_FAIL)
+ {
+ ESP_LOGE(sdcardTag, "Failed to mount filesystem. "
+ "If you want the card to be formatted, set the FORMAT_IF_MOUNT_FAILED in sdcard_config.h");
+ }
+ else
+ {
+ ESP_LOGE(sdcardTag, "Failed to initialize the card (%s). "
+ "Make sure SD card lines have pull-up resistors in place.",
+ esp_err_to_name(ret));
+ }
+ return;
+ }
+ ESP_LOGI(sdcardTag, "Filesystem mounted");
+
+ // Card has been initialized, print its properties
+ sdmmc_card_print_info(stdout, card);
+
+ // Format FATFS
+ #ifdef FORMAT_SD_CARD
+ ret = esp_vfs_fat_sdcard_format(mount_point, card);
+ if (ret != ESP_OK)
+ {
+ ESP_LOGE(sdcardTag, "Failed to format FATFS (%s)", esp_err_to_name(ret));
+ return;
+ }
+
+ if (stat(file_foo, &st) == 0)
+ {
+ ESP_LOGI(sdcardTag, "file still exists");
+ return;
+ }
+ else
+ {
+ ESP_LOGI(sdcardTag, "file doesnt exist, format done");
+ }
+ #endif // CONFIG_EXAMPLE_FORMAT_SD_CARD
+ }
+ ```
+
+ - Provides functions to save JPEG images to the SD card.
+
+ ```c
+ uint16_t lastKnownFile = 0;
+
+ void saveJpegToSdcard(camera_fb_t *captureImage)
+ {
+ // Find the next available filename
+ char filename[32];
+
+ sprintf(filename, "%s/%u_img.jpg", mount_point, lastKnownFile++);
+
+ // Create the file and write the JPEG data
+ FILE *fp = fopen(filename, "wb");
+ if (fp != NULL)
+ {
+ fwrite(captureImage->buf, 1, captureImage->len, fp);
+ fclose(fp);
+ ESP_LOGI(sdcardTag, "JPEG saved as %s", filename);
+ }
+ else
+ {
+ ESP_LOGE(sdcardTag, "Failed to create file: %s", filename);
+ }
+ }
+ ```
+
+Component Structure:
+
+- Header files (sdcard_config.h, sdcard_interface.h) and implementation files (sdcard_interface.c).
+- The sdcard_config.h file defines the SD card configuration parameters.
+- The sdcard_interface.h file declares the functions for SD card initialization, deinitialization, and image saving.
+- The sdcard_interface.c file implements the SD card initialization, deinitialization, and image saving logic.
+
+### Main Function
+
+```c
+// main.c
+#include
+#include "camera_interface.h"
+#include "sdcard_interface.h"
+
+void initialize_drivers()
+{
+ initialize_sdcard();
+ initialize_camera();
+}
+
+void start_tasks()
+{
+ createCameraTask();
+}
+
+void app_main(void)
+{
+ initialize_drivers();
+ start_tasks();
+}
+```
+
+- Includes necessary header files for camera and SD card interfaces.
+- Initializes both the SD card and camera using the provided functions.
+- Starts the camera task to continuously take pictures
+
+### Output
+
+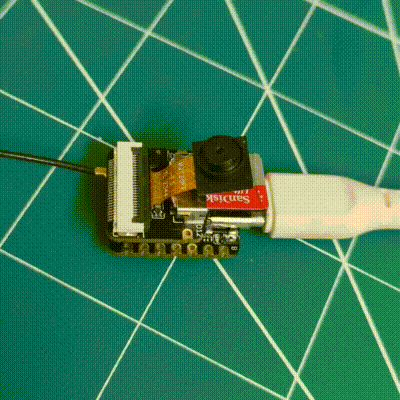
+
+#### UART Output
+
+```shell
+I (1119) main_task: Calling app_main()
+I (1123) sdcard: Initializing SD card
+I (1127) sdcard: Using SPI peripheral
+I (1132) sdcard: Mounting filesystem
+I (1137) gpio: GPIO[21]| InputEn: 0| OutputEn: 1| OpenDrain: 0| Pullup: 0| Pulldown: 0| Intr:0
+I (1146) sdspi_transaction: cmd=52, R1 response: command not supported
+I (1195) sdspi_transaction: cmd=5, R1 response: command not supported
+I (1219) sdcard: Filesystem mounted
+Name: SD32G
+Type: SDHC/SDXC
+Speed: 20.00 MHz (limit: 20.00 MHz)
+Size: 30448MB
+CSD: ver=2, sector_size=512, capacity=62357504 read_bl_len=9
+SSR: bus_width=1
+I (1226) s3 ll_cam: DMA Channel=1
+I (1230) cam_hal: cam init ok
+I (1234) sccb: pin_sda 40 pin_scl 39
+I (1238) sccb: sccb_i2c_port=1
+I (1252) camera: Detected camera at address=0x30
+I (1255) camera: Detected OV2640 camera
+I (1255) camera: Camera PID=0x26 VER=0x42 MIDL=0x7f MIDH=0xa2
+I (1344) cam_hal: buffer_size: 16384, half_buffer_size: 1024, node_buffer_size: 1024, node_cnt: 16, total_cnt: 375
+I (1344) cam_hal: Allocating 384000 Byte frame buffer in PSRAM
+I (1351) cam_hal: Allocating 384000 Byte frame buffer in PSRAM
+I (1357) cam_hal: cam config ok
+I (1361) ov2640: Set PLL: clk_2x: 0, clk_div: 0, pclk_auto: 0, pclk_div: 12
+I (1453) camera: Camera configured successful
+I (1487) main_task: Returned from app_main()
+I (1487) camera: Taking picture...
+I (1997) sdcard: JPEG saved as /sd/0_img.jpg
+I (1997) camera: Picture taken! Its size was: 45764 bytes
+I (6997) camera: Taking picture...
+I (7348) sdcard: JPEG saved as /sd/1_img.jpg
+I (7349) camera: Picture taken! Its size was: 51710 bytes
+I (12349) camera: Taking picture...
+I (12704) sdcard: JPEG saved as /sd/2_img.jpg
+I (12705) camera: Picture taken! Its size was: 51853 bytes
+I (17706) camera: Taking picture...
+I (18054) sdcard: JPEG saved as /sd/3_img.jpg
+I (18055) camera: Picture taken! Its size was: 51919 bytes
+I (23055) camera: Taking picture...
+I (23414) sdcard: JPEG saved as /sd/4_img.jpg
+I (23414) camera: Picture taken! Its size was: 51809 bytes
+I (28415) camera: Taking picture...
+I (28768) sdcard: JPEG saved as /sd/5_img.jpg
+I (28768) camera: Picture taken! Its size was: 51747 bytes
+I (33771) camera: Taking picture...
+I (34117) sdcard: JPEG saved as /sd/6_img.jpg
+I (34117) camera: Picture taken! Its size was: 51968 bytes
+```
+
+#### Output Image
+
+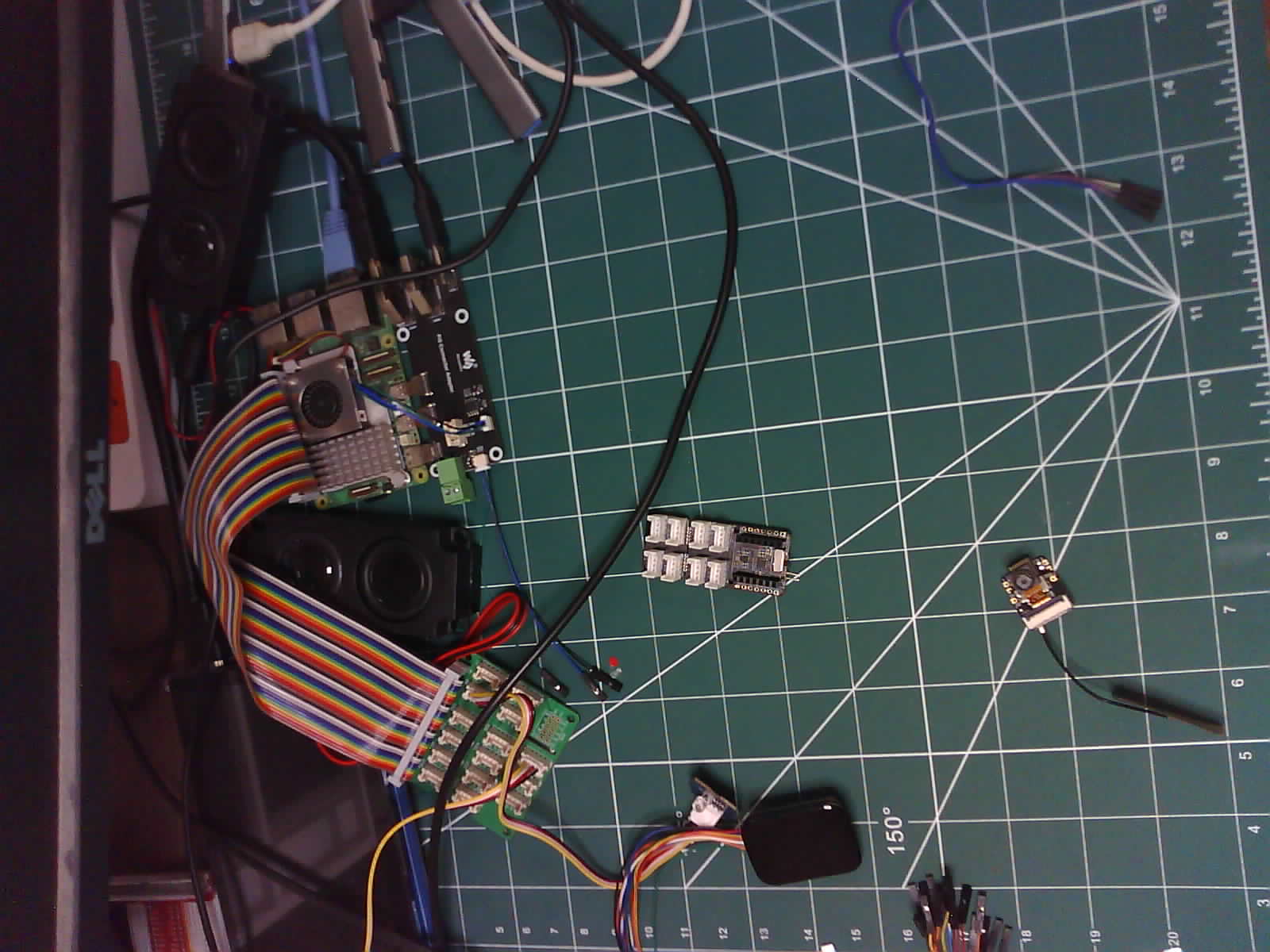
+
## FreeRtos for Arduino IDE
-FreeRtos can be used for Arduino-IDE based Xiao-S3 builds. It is similar to ESP-IDF usable but it runs on only one core and is not optimized for ESP-IDF.
+FreeRtos can be used for Arduino-IDE based XIAO-S3 builds. It is similar to ESP-IDF usable but it runs on only one core and is not optimized for ESP-IDF.
### Hardware Setup
+
Attach the Xiao-S3 to the [Grove - Expansion Board](https://www.seeedstudio.com/Seeeduino-XIAO-Expansion-board-p-4746.html) (OLED DIsplay and RTC) and connect the [Grove - Temperature, Humidity, Pressure and Gas Sensor for Arduino - BME680](https://www.seeedstudio.com/Grove-Temperature-Humidity-Pressure-and-Gas-Sensor-for-Arduino-BME680.html) to the I2c Bus.
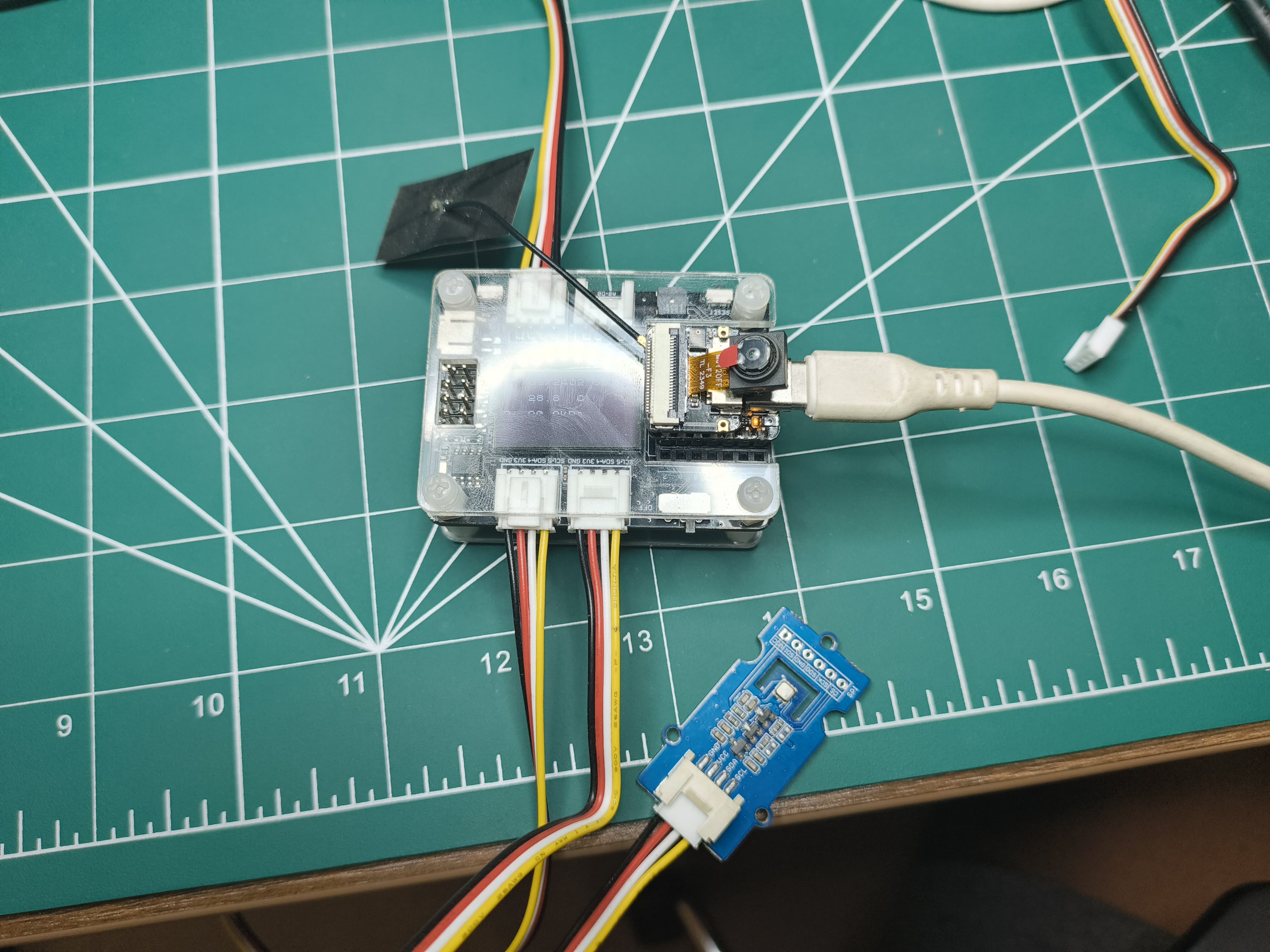
### Software Setup
+
Install the arduino libraries for [pcf8563](https://github.com/Bill2462/PCF8563-Arduino-Library), [U8x8lib](https://github.com/olikraus/U8g2_Arduino) and [bme680](https://github.com/Seeed-Studio/Seeed_Arduino_BME68x) library. Refer to [How to install library](https://wiki.seeedstudio.com/How_to_install_Arduino_Library/) to install library for Arduino.
```cpp
@@ -466,8 +1002,8 @@ PCF8563 pcf;
U8X8_SSD1306_128X64_NONAME_HW_I2C u8x8(/* clock=*/D4, /* data=*/D5, /* reset=*/U8X8_PIN_NONE); // OLEDs without Reset of the Display
// WiFi network credentials
-const char* ssid = "myCrib";
-const char* password = "8697017290";
+const char* ssid = "REPLACE_WITH_YOUR_SSID";
+const char* password = "REPLACE_WITH_YOUR_PASSWORD";
// NTP server for time synchronization
const char* ntpServer = "pool.ntp.org";
@@ -710,7 +1246,7 @@ T: 29.03 C P: 90.86 KPa H: 63.34 % G: 47.85 Kohms
## Trouble Shooting
-Some problems might encounter in the process of hardware connection, software debugging or uploading.
+Some problems might encounter in the process of hardware connection, software debugging or uploading.
## Tech Support & Product Discussion
diff --git a/docs/Sensor/SeeedStudio_XIAO/SeeedStudio_XIAO_ESP32S3_Sense/XIAO_ESP32S3_Sense_tf_and_filesystem.md b/docs/Sensor/SeeedStudio_XIAO/SeeedStudio_XIAO_ESP32S3_Sense/XIAO_ESP32S3_Sense_tf_and_filesystem.md
index 7f29d575a891..26be480a46ff 100644
--- a/docs/Sensor/SeeedStudio_XIAO/SeeedStudio_XIAO_ESP32S3_Sense/XIAO_ESP32S3_Sense_tf_and_filesystem.md
+++ b/docs/Sensor/SeeedStudio_XIAO/SeeedStudio_XIAO_ESP32S3_Sense/XIAO_ESP32S3_Sense_tf_and_filesystem.md
@@ -2,16 +2,16 @@
description: This tutorial describes how to use the microSD card and file system on the XIAO ESP32S3.
title: MicroSD card for Sense Version
keywords:
-- xiao esp32s3
-- esp32s3
-- tf
-- sd
-- file
+ - xiao esp32s3
+ - esp32s3
+ - tf
+ - sd
+ - file
image: https://files.seeedstudio.com/wiki/seeed_logo/logo_2023.png
slug: /xiao_esp32s3_sense_filesystem
last_update:
- date: 04/11/2023
- author: Citric
+ date: 09/15/2024
+ author: Priyanshu Roy
---
# File System and XIAO ESP32S3 Sense
@@ -67,6 +67,34 @@ After formatting, you can insert the microSD card into the microSD card slot. Pl
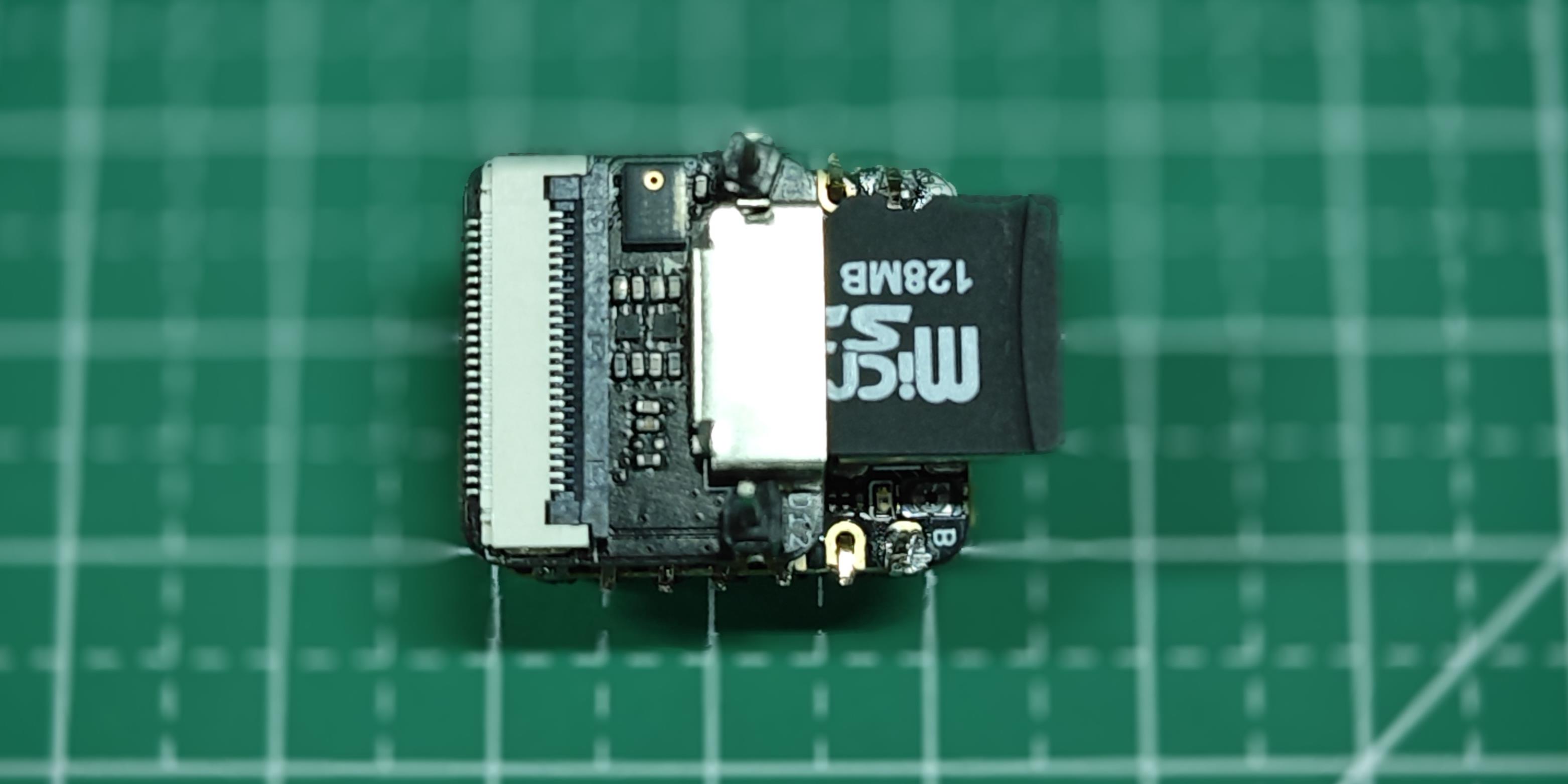
+:::tip
+If the microSD card is not being recognized by ESP32S3 but being recognized by your computer **and** error looks like :
+
+```shell
+[ 7273][E][sd_diskio.cpp:200] sdCommand(): Card Failed! cmd: 0x00
+[ 7274][E][sd_diskio.cpp:759] sdcard_mount(): f_mount failed: (3) The physical drive cannot work
+[ 7588][E][sd_diskio.cpp:200] sdCommand(): Card Failed! cmd: 0x00
+Card Mount Failed
+```
+
+Do the following steps :
+
+- Using Windows Formatter
+
+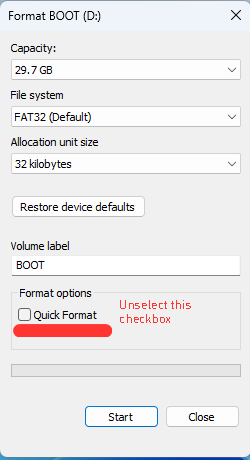
+
+- Using [SD Card Formatter](https://www.sdcard.org/downloads/formatter/) (third-Party software)
+
+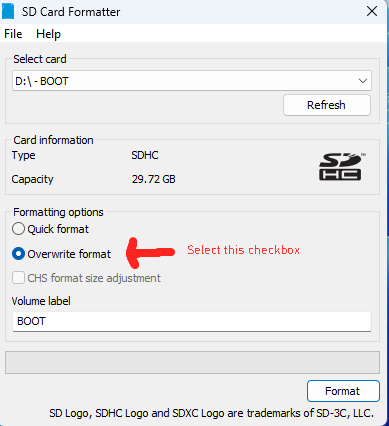
+
+**Note : **
+
+- This process will take significantly longer time than Quick Format.
+
+- These cases arises if you are reusing microSD card which has been previously used for other purposes (i.e. when microSD card containing Linux OS being reused).
+
+:::
+
### Card slot circuit design for expansion boards
The XIAO ESP32S3 Sense card slot occupies 4 GPIOs of the ESP32-S3, and the pin details of the occupancy are shown in the table below.
@@ -96,7 +124,6 @@ The XIAO ESP32S3 Sense card slot occupies 4 GPIOs of the ESP32-S3, and the pin d
-
This also means that if you choose to use the microSD card function of the expansion board, you cannot also use the SPI function of the XIAO ESP32S3. You can turn on/off the microSD card function by connecting/cutting the pads of J3.
@@ -118,7 +145,6 @@ This also means that if you choose to use the microSD card function of the expan
By default, the microSD card function is enabled after the expansion board is installed.
:::
-
## Modify the files in the microSD card
:::caution
@@ -394,7 +420,7 @@ Serial.printf("SD Card Size: %lluMB\n", cardSize);
4. The `listDir()` function lists the directories on the SD card. This function accepts as arguments the filesystem (SD), the main directory’s name, and the levels to go into the directory.
- Here’s an example of how to call this function. The `/` corresponds to the microSDcard root directory.
+ Here’s an example of how to call this function. The `/` corresponds to the microSDcard root directory.
```c
listDir(SD, "/", 0);
@@ -492,7 +518,7 @@ To complete this project, you will need to prepare the following hardware in adv
For the software, you need to install the following libraries for the Arduino IDE in advance.
-- NTPClient library forked by Taranais
+- NTPClient library forked by Taranais
@@ -584,7 +610,7 @@ void setup() {
timeClient.setTimeOffset(3600);
// Initialize SD card
- SD.begin(SD_CS);
+ SD.begin(SD_CS);
if(!SD.begin(SD_CS)) {
Serial.println("Card Mount Failed");
return;
@@ -609,7 +635,7 @@ void setup() {
writeFile(SD, "/data.txt", "Reading ID, Date, Hour, NO2, C2H5CH, VOC, CO \r\n");
}
else {
- Serial.println("File already exists");
+ Serial.println("File already exists");
}
file.close();
@@ -619,16 +645,16 @@ void setup() {
getReadings();
getTimeStamp();
logSDCard();
-
+
// Increment readingID on every new reading
readingID++;
-
+
// Start deep sleep
Serial.println("DONE! Going to sleep now.");
// Enable Timer wake_up
esp_sleep_enable_timer_wakeup(TIME_TO_SLEEP * uS_TO_S_FACTOR);
- esp_deep_sleep_start();
+ esp_deep_sleep_start();
}
void loop() {
@@ -646,13 +672,13 @@ void getReadings(){
VOC_val = gas.getGM502B();
// GM702B CO sensor
CO_val = gas.getGM702B();
-
+
Serial.print("NO2 Value is: ");
Serial.println(NO2_val);
-
+
Serial.print("C2H5CH Value is: ");
Serial.println(C2H5CH_val);
-
+
Serial.print("VOC Value is: ");
Serial.println(VOC_val);
@@ -682,8 +708,8 @@ void getTimeStamp() {
// Write the sensor readings on the SD card
void logSDCard() {
- dataMessage = String(readingID) + "," + String(dayStamp) + "," + String(timeStamp) + "," +
- String(NO2_val) + "," + String(C2H5CH_val) + "," + String(VOC_val) + "," +
+ dataMessage = String(readingID) + "," + String(dayStamp) + "," + String(timeStamp) + "," +
+ String(NO2_val) + "," + String(C2H5CH_val) + "," + String(VOC_val) + "," +
String(CO_val) + "\r\n";
Serial.print("Save data: ");
Serial.println(dataMessage);
@@ -739,9 +765,10 @@ In order to facilitate testing, the effect is shown every minute to save data, t
:::caution
There are the following things to note about this project:
+
1. The Multichannel Gas Sensor needs a period of warm-up before the values obtained are accurate. So the first few sets of data recorded can be considered to be discarded if the error is large.
2. The serial monitor will only output the saved information once, because this example uses the deep sleep function, it is equivalent to reset after waking up, that is, you need to reopen the serial port of Arduino to see the next debug information. But rest assured, if there is no problem with the card, the sensor data will be collected on time at the time you set.
-:::
+ :::
### Program annotation
@@ -787,7 +814,7 @@ Finally, the ESP32 starts the deep sleep.
```c
esp_sleep_enable_timer_wakeup(TIME_TO_SLEEP * uS_TO_S_FACTOR);
-esp_deep_sleep_start();
+esp_deep_sleep_start();
```
We recommend that you use these two functions together. Make sure that XIAO can enter deep sleep mode as soon as possible after setting the wake-up time.
@@ -834,7 +861,7 @@ Note: at the time of writing this post, the ESP32 Filesystem Uploader plugin **i
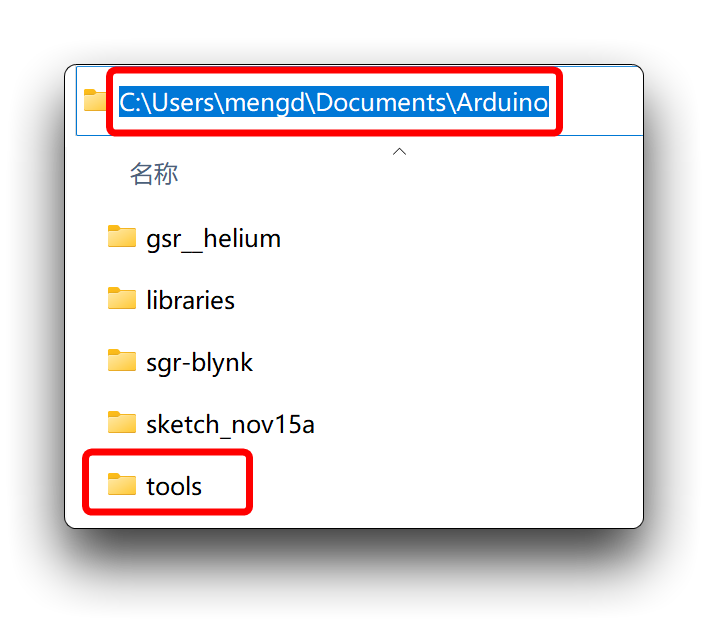
-**Step 4.** Unzip the downloaded *.zip* folder. Open it and copy the ESP32FS folder to the tools folder you created in the previous step. You should have a similar folder structure:
+**Step 4.** Unzip the downloaded _.zip_ folder. Open it and copy the ESP32FS folder to the tools folder you created in the previous step. You should have a similar folder structure:
`/tools/ESP32FS/tool/esp32fs.jar`
@@ -878,7 +905,7 @@ To upload files to the ESP32 filesystem follow the next instructions.
-**Step 9.** Inside the data folder is where you should put the files you want to save into the ESP32 filesystem. As an example, create a *.txt* file with some text called **test_example**.
+**Step 9.** Inside the data folder is where you should put the files you want to save into the ESP32 filesystem. As an example, create a _.txt_ file with some text called **test_example**.
@@ -892,18 +919,17 @@ The files were successfully uploaded to the ESP32 filesystem when you see the me
-
### Testing the Uploader
Now, let’s just check if the file was actually saved into the ESP32 filesystem. Simply upload the following code to your ESP32 board.
```cpp
#include "SPIFFS.h"
-
+
void setup() {
Serial.begin(115200);
while(!Serial);
-
+
if(!SPIFFS.begin(true)){
Serial.println("An Error has occurred while mounting SPIFFS");
return;
@@ -914,26 +940,24 @@ void setup() {
Serial.println("Failed to open file for reading");
return;
}
-
+
Serial.println("File Content:");
while(file.available()){
Serial.write(file.read());
}
file.close();
-
+
}
-
+
void loop() {
-
+
}
```
-After uploading, open the Serial Monitor at a baud rate of 115200. It should print the content of your *.txt* file on the Serial Monitor.
+After uploading, open the Serial Monitor at a baud rate of 115200. It should print the content of your _.txt_ file on the Serial Monitor.
-
-
## Flash Data Storage
:::caution
@@ -952,12 +976,11 @@ This section has been written for the XIAO ESP32C3 and is fully compatible with
- [XIAO ESP32C3 Data Permanently in different ways](https://wiki.seeedstudio.com/xiaoesp32c3-flash-storage/)
-
## Troubleshooting
## Citations & References
-This article draws on the files system content **[Random Nerd Tutorials](https://randomnerdtutorials.com/)**' on ESP32 and uses it verified on the Seeed Studio XIAO ESP32S3 Sense.
+This article draws on the files system content **[Random Nerd Tutorials](https://randomnerdtutorials.com/)**' on ESP32 and uses it verified on the Seeed Studio XIAO ESP32S3 Sense.
Special thanks to the authors of **Random Nerd Tutorials** for their hard work!
@@ -971,7 +994,6 @@ For more information about using the ESP32 development board, please read the of
- [Random Nerd Tutorials](https://randomnerdtutorials.com/)
-
## Tech Support & Product Discussion
.
@@ -987,18 +1009,3 @@ Thank you for choosing our products! We are here to provide you with different s
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-