name="q"
placeholder="Type To Search..."
type="search"
- hx-get="{% url 'search-projects' %}"
+ hx-get="{% url 'api-search-projects' %}"
hx-indicator=".htmx-indicator"
hx-swap="none"
hx-target="#search-results"
diff --git a/backend/apps/slack/__init__.py b/backend/apps/slack/__init__.py
new file mode 100644
index 000000000..e5d45429a
--- /dev/null
+++ b/backend/apps/slack/__init__.py
@@ -0,0 +1 @@
+from apps.slack.commands import * # noqa: F403
diff --git a/backend/apps/slack/apps.py b/backend/apps/slack/apps.py
new file mode 100644
index 000000000..636a92ea0
--- /dev/null
+++ b/backend/apps/slack/apps.py
@@ -0,0 +1,10 @@
+from django.apps import AppConfig
+from django.conf import settings
+from slack_bolt import App
+
+
+class SlackConfig(AppConfig):
+ default_auto_field = "django.db.models.BigAutoField"
+ name = "apps.slack"
+
+ app = App(token=settings.SLACK_BOT_TOKEN) if settings.SLACK_BOT_TOKEN != "None" else None # noqa: S105
diff --git a/backend/apps/slack/blocks.py b/backend/apps/slack/blocks.py
new file mode 100644
index 000000000..9be99061b
--- /dev/null
+++ b/backend/apps/slack/blocks.py
@@ -0,0 +1,14 @@
+"""Slack blocks."""
+
+
+def divider():
+ """Return divider block."""
+ return {"type": "divider"}
+
+
+def markdown(text):
+ """Return markdown block."""
+ return {
+ "type": "section",
+ "text": {"type": "mrkdwn", "text": text},
+ }
diff --git a/backend/apps/slack/commands/__init__.py b/backend/apps/slack/commands/__init__.py
new file mode 100644
index 000000000..662515c11
--- /dev/null
+++ b/backend/apps/slack/commands/__init__.py
@@ -0,0 +1 @@
+from apps.slack.commands.contribute import contribute
diff --git a/backend/apps/slack/commands/contribute.py b/backend/apps/slack/commands/contribute.py
new file mode 100644
index 000000000..edae78537
--- /dev/null
+++ b/backend/apps/slack/commands/contribute.py
@@ -0,0 +1,63 @@
+"""Slack bot contribute command."""
+
+from apps.common.utils import get_absolute_url
+from apps.slack.apps import SlackConfig
+from apps.slack.blocks import divider, markdown
+from apps.slack.constants import OWASP_PROJECT_NEST_CHANNEL_ID
+
+
+def contribute(ack, say, command):
+ """Slack /contribute command handler."""
+ from apps.github.models.issue import Issue
+ from apps.owasp.api.search.issue import get_issues
+ from apps.owasp.models.project import Project
+
+ ack()
+
+ # TODO(arkid15r): consider adding escaping for the user's input.
+ search_query = command["text"]
+ blocks = [
+ divider(),
+ markdown(f'*No results found for "{search_query}"*\n'),
+ ]
+ issues = get_issues(search_query, distinct=True, limit=10)
+
+ if issues:
+ blocks = [
+ divider(),
+ markdown(
+ (
+ f"\n*Here are top 10 most relevant issues (1 issue per project) "
+ f"that I found for*\n `/contribute {search_query}`:\n"
+ )
+ if search_query
+ else (
+ "\n*Here are top 10 most recent issues (1 issue per project):*\n"
+ "You can refine the results by using a more specific query, e.g.\n"
+ "`/contribute python good first issue`"
+ )
+ ),
+ ]
+ for idx, issue in enumerate(issues):
+ blocks.append(
+ markdown(
+ f"\n{idx + 1}. {issue['idx_project_name']}\n"
+ f"<{issue['idx_url']}|{issue['idx_title']}>\n"
+ ),
+ )
+
+ blocks.append(
+ markdown(
+ f"⚠️ *Extended search over {Issue.open_issues_count()} open issues in "
+ f"{Project.active_projects_count()} OWASP projects is available at "
+ f"<{get_absolute_url('project-issues')}>*\n"
+ "You can share feedback on your search experience "
+ f"in the <#{OWASP_PROJECT_NEST_CHANNEL_ID}|project-nest> channel."
+ ),
+ )
+
+ say(blocks=blocks)
+
+
+if SlackConfig.app:
+ contribute = SlackConfig.app.command("/contribute")(contribute)
diff --git a/backend/apps/slack/constants.py b/backend/apps/slack/constants.py
new file mode 100644
index 000000000..a6b50d370
--- /dev/null
+++ b/backend/apps/slack/constants.py
@@ -0,0 +1,3 @@
+"""Slack app constants."""
+
+OWASP_PROJECT_NEST_CHANNEL_ID = "C07JLLG2GFQ"
diff --git a/backend/apps/slack/management/__init__.py b/backend/apps/slack/management/__init__.py
new file mode 100644
index 000000000..e69de29bb
diff --git a/backend/apps/slack/management/commands/__init__.py b/backend/apps/slack/management/commands/__init__.py
new file mode 100644
index 000000000..e69de29bb
diff --git a/backend/apps/slack/management/commands/run_slack_bot.py b/backend/apps/slack/management/commands/run_slack_bot.py
new file mode 100644
index 000000000..cd9aeb74d
--- /dev/null
+++ b/backend/apps/slack/management/commands/run_slack_bot.py
@@ -0,0 +1,19 @@
+"""A command to start Slack bot."""
+
+import logging
+
+from django.conf import settings
+from django.core.management.base import BaseCommand
+from slack_bolt.adapter.socket_mode import SocketModeHandler
+
+from apps.slack.apps import SlackConfig
+
+logger = logging.getLogger(__name__)
+
+
+class Command(BaseCommand):
+ help = "Runs Slack bot application."
+
+ def handle(self, *args, **options):
+ if settings.SLACK_APP_TOKEN != "None": # noqa: S105
+ SocketModeHandler(SlackConfig.app, settings.SLACK_APP_TOKEN).start()
diff --git a/backend/data/nest.json b/backend/data/nest.json
index 67da3728f..d3c174573 100644
--- a/backend/data/nest.json
+++ b/backend/data/nest.json
@@ -4,10 +4,11 @@
"pk": 1,
"fields": {
"nest_created_at": "2024-09-11T19:06:59.693Z",
- "nest_updated_at": "2024-09-11T19:06:59.693Z",
+ "nest_updated_at": "2024-09-13T14:54:00.231Z",
"node_id": "I_kwDOMl6dl86V22oI",
"title": "Hardcoded username and endless loop tests didn't work ",
"body": "Hi there!\r\nI tried ABAP-Code-Analyzer with the \"python main.py ABAPTeste02ListagemdeOV.txt\" command. ABAPTeste02ListagemdeOV.txt ( attached) file was in the same directory than ABAP-Code-Analyzer.\r\nThe xls output file was generated with no lines (attached).\r\nI had understood ABAP-Code-Analyzer should \"caught\" hardcode user name and endless loop...\r\nThanks!\r\n[abap_security_scan_report.xlsx](https://github.com/user-attachments/files/16932297/abap_security_scan_report.xlsx)\r\n[ABAPTeste02ListagemdeOV.txt](https://github.com/user-attachments/files/16932299/ABAPTeste02ListagemdeOV.txt)\r\n",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/abap-code-scanner/issues/1",
@@ -30,10 +31,11 @@
"pk": 2,
"fields": {
"nest_created_at": "2024-09-11T19:10:10.587Z",
- "nest_updated_at": "2024-09-11T19:10:10.587Z",
+ "nest_updated_at": "2024-09-13T14:56:28.200Z",
"node_id": "I_kwDOLYZa3c6BGmff",
"title": "SDRF Working Group Meetings",
"body": "OWASP SDRF Weekly Meeting\r\n**First Meeting**: Monday, 4 March · 5:00 – 6:00pm\r\n**Time zone**: Australia/Sydney\r\n**URL**: https://meet.google.com/yvq-phpg-viy\r\n\r\nWill re-occur weekly on Mondays 5PM AEDT. ",
+ "summary": "A weekly meeting for the OWASP SDRF Working Group is scheduled to take place every Monday at 5:00 PM AEDT, starting on March 4. Participants can join via the provided Google Meet link. It may be helpful to set reminders for these recurring meetings.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-secure-development-and-release-framework/issues/1",
@@ -56,10 +58,11 @@
"pk": 3,
"fields": {
"nest_created_at": "2024-09-11T19:10:47.813Z",
- "nest_updated_at": "2024-09-11T19:10:47.813Z",
+ "nest_updated_at": "2024-09-13T14:56:57.808Z",
"node_id": "I_kwDOLD0lt86ByuLx",
"title": "Please add a description to this project",
"body": "",
+ "summary": "The issue requests the addition of a project description. This will help clarify the project's purpose and improve understanding for users and contributors. It is suggested to draft a concise summary that highlights the project's goals, features, and potential use cases.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-cybersecurity-certification-course/issues/1",
@@ -82,10 +85,11 @@
"pk": 4,
"fields": {
"nest_created_at": "2024-09-11T19:10:51.794Z",
- "nest_updated_at": "2024-09-11T19:10:51.794Z",
+ "nest_updated_at": "2024-09-13T14:57:01.156Z",
"node_id": "I_kwDOLD0cZ859uhUf",
"title": "Solana Top Common Smart Contract Issues - QuillAudits",
"body": "**### 1] Integer Overflow** \r\n**Rust offers different integer types with varying ranges:**\r\nu8 (8 bits): 0 to 255\r\nu16 (16 bits): 0 to 65535\r\nu32 (32 bits): 0 to 4294967295\r\nu64 (64 bits): 0 to 18446744073709551615\r\nu128 (128 bits): 0 to a very large positive number\r\n\r\nInteger overflow occurs when an arithmetic operation results in a value that exceeds the maximum representable value of the chosen integer data type. \r\nFor example, a = a + 1 — if an u8 integer a is changed to a value outside of its range, say 256 or -1, then an overflow/underflow will occur.\r\nThis can lead to unexpected behavior in smart contracts, as the value wraps around to the minimum value instead of throwing an error.\r\n\r\n**Example:-**\r\n```\r\n\r\nub fn pretty_time(t: u64) -> String { \r\nlet seconds = t % 60;\r\n let minutes = (t / 60) % 60;\r\n let hours = (t / (60 * 60)) % 24;\r\n let days = t / (60 * 60 * 24);\r\n\r\n\r\npub fn calculate_fee_from_amount(amount: u64, percentage: f32) -> u64 {\r\n if percentage <= 0.0 {\r\n return 0\r\n }\r\n let precision_factor: f32 = 1000000.0;\r\n let factor = (percentage / 100.0 * precision_factor) as u128; //largest it can get is 10^4\r\n (amount as u128 * factor / precision_factor as u128) as u64 // this does not fit if amount\r\n // itself cannot fit into u64\r\n\r\n```\r\n\r\n`Description`:\r\nIn the above functions mentioned, we are doing some calculations using the `*` and `/` operators, which can cause an overflow/underflow. If one uses the debug, build, it can cause a panic; however, in the release build, it will underflow silently.\r\n\r\n`Remediation`\r\n1. Instead of using the `*` operator, we recommend using the `checked_mul` function to prevent an overflow.\r\n2. Instead of using the `/` operator, we recommend using the `checked_div` function to prevent an underflow.\r\n\r\n\r\n### 2]Missing Account Verification \r\nThe \"Missing Account Verification\" issue in Rust smart contracts on the Solana blockchain typically arises when a smart contract fails to verify or check the ownership and structure of an account, potentially leading to vulnerabilities. This can occur due to various reasons, such as incorrect constructor arguments, failed contract verification, or lack of signature validation. This can lead to several problems, including Unauthorized Access, Denial-of-Service Attacks, and Loss of Funds.\r\n\r\n**Example:-**\r\n ```\r\n if !acc.sender.is_signer || !acc.metadata.is_signer {\r\n return Err(ProgramError::MissingRequiredSignature)\r\n }\r\n\r\ncreate.rs - Line 121:\r\n if a.rent.key != &sysvar::rent::id() ||\r\n a.token_program.key != &spl_token::id() ||\r\n a.associated_token_program.key != &spl_associated_token_account::id() ||\r\n a.system_program.key != &system_program::id()\r\n {\r\n return Err(ProgramError::InvalidAccountData)\r\n }\r\n\r\n```\r\n```\r\ncreate.rs - Line 85:\r\n if !a.sender.is_writable || //fee payer\r\n !a.sender_tokens.is_writable || //debtor\r\n !a.recipient_tokens.is_writable || //might be created\r\n !a.metadata.is_writable || //will be created\r\n !a.escrow_tokens.is_writable || //creditor\r\n !a.streamflow_treasury_tokens.is_writable || //might be created\r\n !a.partner_tokens.is_writable\r\n //might be created\r\n // || !a.liquidator.is_writable //creditor (tx fees)\r\n {\r\n return Err(SfError::AccountsNotWritable.into())\r\n }\r\n```\r\n\r\n```\r\ncreate.rs - Line 80:\r\n if !a.escrow_tokens.data_is_empty() || !a.metadata.data_is_empty() {\r\n return Err(ProgramError::AccountAlreadyInitialized)\r\n }\r\n\r\n```\r\n```\r\ncreate.rs - Line 99:\r\n let strm_treasury_pubkey = Pubkey::from_str(STRM_TREASURY).unwrap();\r\n let withdrawor_pubkey = Pubkey::from_str(WITHDRAWOR_ADDRESS).unwrap();\r\n let strm_treasury_tokens = get_associated_token_address(&strm_treasury_pubkey, a.mint.key);\r\n let sender_tokens = get_associated_token_address(a.sender.key, a.mint.key);\r\n let recipient_tokens = get_associated_token_address(a.recipient.key, a.mint.key);\r\n let partner_tokens = get_associated_token_address(a.partner.key, a.mint.key);\r\n\r\n\r\n if a.streamflow_treasury.key != &strm_treasury_pubkey ||\r\n a.streamflow_treasury_tokens.key != &strm_treasury_tokens ||\r\n a.withdrawor.key != &withdrawor_pubkey\r\n {\r\n return Err(SfError::InvalidTreasury.into())\r\n }\r\n```\r\n\r\n`Description`: Account verification is critical in Solana programs. Signed and writable accounts, as recommended, are to be verified before the business logic implementation, Also, the accounts data is verified to match the business logic requirements.\r\n\r\n\r\n### 3]Missing Signer check\r\nIf the account provided as a signer is not included as a signer in the transaction, Solana will throw a MissingRequiredSignature error. This helps prevent the unauthorized execution of instructions.\r\n\r\n**Example:-**\r\n\r\n```\r\n let init_vesting_account = create_account(\r\n &payer.key,\r\n &vesting_account_key,\r\n rent.minimum_balance(state_size),\r\n state_size as u64,\r\n &program_id,\r\n );\r\n\r\n```\r\n`Description`: In the above example, An instruction should only be available to a restricted set of entities; the program should verify that the call has been signed by the appropriate entity.\r\n\r\n`Remediation`: Verify that the payer account is a signer using the is_signer() function.\r\n\r\n\r\n### 4]Arithmetic Accuracy Deviation \r\nArithmetic accuracy deviations in Rust smart contracts on Solana can occur due to various factors, leading to unintended behaviour and potential security vulnerabilities. These deviations refer to situations where the outcome of mathematical operations within a smart contract differs from the expected result.\r\n\r\n**Example:-**\r\n \r\n```\r\nlet x = args.num_nfts_in_bucket;\r\n let y = args.num_nfts_in_lockers;\r\n let x_plus_y = (y as u64).checked_add(x as u64).unwrap();\r\n\r\n let numerator = args\r\n .locker_duration\r\n .checked_mul(y as u64)\r\n .unwrap()\r\n .checked_mul(100)\r\n .unwrap()\r\n .checked_mul(LAMPORTS_PER_DROPLET)\r\n .unwrap();\r\n\r\nlet denominator = args.max_locker_duration.checked_mul(x_plus_y).unwrap();\r\n let raw_interest = numerator.checked_div(denominator).unwrap();\r\n\r\n```\r\n`Description`: In the above example,Consider a scenario in which x = 1000, y = 1, and max_duration = t ∗ 116 sec.\r\n• By those above conditions, a user can skip t seconds of duration for interest calculation. \r\n• Letlocker_duration=6, andtis6sec.\r\n• Interest=(6∗1∗100∗108)/(6∗116∗8640∗1001)=(int)0.997765=0\r\n\r\n`Remediation`: It is recommended to perform a ceiling division when calculating interest owed.\r\n\r\n\r\n\r\n### 5]Arbitrary signed program invocation \r\nThe Arbitrary Signed Program Invocation issue in Solana refers to a vulnerability in the token instruction code that allows invoking an arbitrary program instead of the real SPL-token program. This issue can lead to security risks and potential attacks on the Solana blockchain.\r\n\r\nIn Solana, a transaction consists of one or more instructions, an array of accounts to read and write data from, and one or more signatures. Instructions are the smallest execution logic on Solana and invoke programs that make calls to the Solana runtime to update the state. Programs on Solana don't store data/state; rather, data/state is stored in accounts. When a Solana program invokes another program, the callee's program ID is supplied to the call typically through one of the following two functions: invoke or invoke_signed. The program ID is the first parameter of the instruction. In the case of the token instruction, there was a vulnerability that allowed invoking an arbitrary program, which could lead to security risks and potential attacks.\r\n\r\n**Example:-**\r\n```\r\n#[program]\r\npub mod Issue_ASPI {\r\n use super::*;\r\n pub fn transfer_tokens(ctx: Context, amount: u64) -> ProgramResult {\r\n let token_program = ctx.accounts.token_program.clone();\r\n\r\n // Vulnerable: Allowing arbitrary program invocation\r\n invoke(&token_program, &ctx.accounts.token_account, &[amount.to_le_bytes().as_ref()]);\r\n Ok(())\r\n }\r\n}\r\n```\r\n`Description`: In The above example, The code invokes the token_program without validating its program ID. An attacker could supply a malicious program instead, leading to unintended execution.\r\nExploitation:\r\nThe attacker supplies a malicious program ID as token_program.\r\nYour program invokes the malicious program, potentially granting it unintended control over tokens or other assets.\r\n\r\n`Remediation`: To avoid such vulnerabilities and attacks in general, it is essential to ensure that the program ID is correctly checked and validated before executing any instructions\r\n\r\n### 6] Solana account confusions \r\nIn your Solana smart contracts, remember that users can provide any type of account as input. Don't assume ownership alone guarantees the account matches your expectations. Always verify the account's data type to avoid security vulnerabilities. Solana programs often involve multiple account types for data storage. Checking account ownership isn't enough. Validate every provided account's data type against your intended use.\r\n\r\n**Example:-**\r\n```\r\nprocessor.rs - Line 44\r\n let vesting_account_key = Pubkey::create_program_address(&[&seeds], &program_id).unwrap();\r\n if vesting_account_key != *vesting_account.key {\r\n msg!(\"Provided vesting account is invalid\");\r\n return Err(ProgramError::InvalidArgument);\r\n }\r\n```\r\n\r\n`Description`: In the above example,The address generated using create_program_address is not guaranteed to be a valid program address off the curve. Program addresses do not lie on the ed25519 curve and, therefore, have no valid private key associated with them, and thus, generating a signature for it is impossible. There is about a 50/50 chance of this happening for a given collection of seeds and program ID.\r\n\r\n`Remediation`: To generate a valid program address using a specific seed, use find_program_address function, which iterates through multiple bump seeds until a valid combination that does not lie on the curve is found.\r\n\r\n### 7]Error not handled \r\nUnhandled errors in Rust Solana programs pose a significant threat to their security and functionality. These errors can lead to unexpected behavior, program crashes, and even potential financial losses. When an error occurs, the program can either handle it gracefully or leave it unhandled. Unhandled errors are those that the program doesn't explicitly handle and recover from.\r\n\r\n**Example:-**\r\n\r\n```\r\npub mod my_program {\r\n use super::*;\r\n\r\n pub fn transfer_tokens(ctx: Context, amount: u64) -> ProgramResult {\r\n // Validate inputs\r\n if amount == 0 {\r\n return Err(ProgramError::InvalidInput); // Explicit error for invalid amount\r\n }\r\n // ... (rest of the transfer logic)\r\n // Handle potential errors from other operations\r\n let transfer_result = spl_token::transfer(\r\n /* ... transfer parameters ... */\r\n );\r\n match transfer_result {\r\n Ok(()) => {\r\n // Transfer successful\r\n }\r\n Err(error) => {\r\n // Handle transfer error appropriately\r\n return Err(error);\r\n }\r\n }\r\n\r\n Ok(())\r\n }\r\n```\r\n\r\n`Description`: In the Above Example, there's a potential \"error not handled\" issue. After calling spl_token::transfer, which presumably performs a token transfer operation, the code checks the result with a match statement. If the transfer operation fails, an error is caught, but the error is simply returned without any further handling or logging.\r\n\r\n`Remediation`: In order to mitigate the \"error not handled\" issue, it's recommended to include proper error handling mechanisms, such as logging the error, rolling back any state changes, or taking appropriate actions based on the specific error. Simply returning the error without any additional handling may lead to undesired behavior and make it difficult to identify and debug issues during the execution of the program.\r\n\r\n\r\n",
+ "summary": "The issue outlines several common vulnerabilities found in Solana smart contracts, each accompanied by a description, examples, and remediation suggestions. \n\n1. **Integer Overflow**: Arithmetic operations can lead to overflow/underflow errors. Use `checked_mul` and `checked_div` functions for safe calculations.\n\n2. **Missing Account Verification**: Failing to verify account ownership can lead to unauthorized access and vulnerabilities. Ensure all accounts are properly verified before executing business logic.\n\n3. **Missing Signer Check**: An account not included as a signer can trigger an error. Always check that the required accounts are signers using the `is_signer()` function.\n\n4. **Arithmetic Accuracy Deviation**: Mathematical operations might yield unexpected results due to erroneous calculations. It is recommended to perform ceiling division for accurate interest calculations.\n\n5. **Arbitrary Signed Program Invocation**: Invoking a program without validating its ID can lead to attacks. Always validate the program ID before invocation.\n\n6. **Solana Account Confusions**: Verify not just the ownership of accounts but also their data types to prevent security vulnerabilities.\n\n7. **Error Not Handled**: Unhandled errors can cause crashes or unintended behavior. Implement proper error handling to manage and log errors adequately.\n\nTo address these issues, developers should adopt safer coding practices, validate inputs thoroughly, and implement robust error handling.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-solana-programs-top-10/issues/1",
@@ -108,10 +112,11 @@
"pk": 5,
"fields": {
"nest_created_at": "2024-09-11T19:10:55.756Z",
- "nest_updated_at": "2024-09-11T19:10:55.756Z",
+ "nest_updated_at": "2024-09-13T14:57:04.466Z",
"node_id": "I_kwDOLD0PbM6ByuKa",
"title": "Please add a description to this project",
"body": "",
+ "summary": "The issue requests the addition of a project description to provide clarity and context. It suggests that a concise summary outlining the project's purpose, features, and goals would enhance understanding for potential users and contributors. The next step would be to draft and include a clear and informative description in the project's documentation.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-pryingdeep/issues/2",
@@ -134,10 +139,11 @@
"pk": 6,
"fields": {
"nest_created_at": "2024-09-11T19:11:09.490Z",
- "nest_updated_at": "2024-09-11T19:11:09.490Z",
+ "nest_updated_at": "2024-09-13T14:57:15.402Z",
"node_id": "I_kwDOLDUp6s6ByuIQ",
"title": "Please add a description to this project",
"body": "",
+ "summary": "The issue requests the addition of a project description to provide clarity and context. It suggests that a clear and concise description would enhance understanding and engagement with the project. To address this, a suitable project description should be drafted and added.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-de-addiction/issues/1",
@@ -160,10 +166,11 @@
"pk": 7,
"fields": {
"nest_created_at": "2024-09-11T19:11:13.632Z",
- "nest_updated_at": "2024-09-11T19:11:13.632Z",
+ "nest_updated_at": "2024-09-13T14:57:18.709Z",
"node_id": "I_kwDOLDUacM6ByuFI",
"title": "Please add a description to this project",
"body": "",
+ "summary": "The issue requests the addition of a project description to provide clarity and context for users. To address this, a concise and informative description should be crafted and added to the project's documentation.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-appsec-contract-builder/issues/1",
@@ -186,10 +193,11 @@
"pk": 8,
"fields": {
"nest_created_at": "2024-09-11T19:11:17.662Z",
- "nest_updated_at": "2024-09-11T19:11:17.662Z",
+ "nest_updated_at": "2024-09-13T14:57:21.987Z",
"node_id": "I_kwDOLDUMl86ByuDS",
"title": "Please add a description to this project",
"body": "",
+ "summary": "The issue requests the addition of a project description to provide clarity and context. It is suggested to draft a concise overview that outlines the project's purpose, functionality, and any key features.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-naivesystems-analyze/issues/2",
@@ -212,10 +220,11 @@
"pk": 9,
"fields": {
"nest_created_at": "2024-09-11T19:11:21.832Z",
- "nest_updated_at": "2024-09-11T19:11:21.832Z",
+ "nest_updated_at": "2024-09-13T14:57:25.220Z",
"node_id": "I_kwDOLDUMEs6ByuCI",
"title": "Please add a description to this project",
"body": "",
+ "summary": "The issue requests the addition of a project description. This would help provide context and clarify the project's purpose and goals. It is suggested to draft a concise overview that highlights key features and objectives.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-sapkiln/issues/1",
@@ -242,6 +251,7 @@
"node_id": "I_kwDOLDUCls6J0ix2",
"title": "[Feature] We should spell check the ASVS PDF before it goes out",
"body": "Related to this PR: #3 \r\n\r\nDue to technical jargon, this might be tricky in a workflow.",
+ "summary": "The issue highlights the need for a spell check of the ASVS PDF before its release. It points out that the presence of technical jargon could complicate the spell-checking process. To address this, a careful review and possibly an adjustment of the workflow may be necessary to ensure accuracy.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-thick-client-application-security-verification-standard/issues/4",
@@ -270,6 +280,7 @@
"node_id": "I_kwDOLDUCls6J1Mmp",
"title": "[Feature] Fix documents coming from v0.1",
"body": "when we are happy with our v**0**.x, we should rework the github action and folder structure to come from v**1**.x",
+ "summary": "The issue highlights the need to address documents originating from version 0.1. It suggests that once the team is satisfied with version 0.x, they should revise the GitHub action and reorganize the folder structure to align with version 1.x. A rework is necessary to ensure a smooth transition to the new version.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-thick-client-application-security-verification-standard/issues/5",
@@ -294,10 +305,11 @@
"pk": 12,
"fields": {
"nest_created_at": "2024-09-11T19:11:28.496Z",
- "nest_updated_at": "2024-09-11T19:11:28.496Z",
+ "nest_updated_at": "2024-09-13T14:57:28.619Z",
"node_id": "I_kwDOLDUCls6VlrRN",
"title": "Should DLL Hijacking be a single item?",
"body": "as suggested in #6 by @matreurai \r\n\r\n> Note : I've seen in TASVS-STORAGE a category specific to a specific vulnerability (DLL Hijacking). I think this should be modified as the testing standard is not made for specific vulnerabilities in my opinion. Additionally, these items are explaining a type of attack and do not provide any guidance to what to test or how to prevent/mitigate risks of such attacks. The equivalent in the Web Application Security Verification Standard would be to add an item as such:\r\n> \"Cross-Site Scripting Category - Blind cross-site scripting (XSS) is a variant of stored XSS where the malicious payload is executed in a different context or application than where it was originally injected.\".\r\n> I don't think this make sense in the context of a Verification Standard.",
+ "summary": "The issue discusses whether DLL Hijacking should be treated as a standalone item within the testing standard, as it currently appears in the TASVS-STORAGE category. The suggestion is to modify this approach since the existing items primarily describe the attack type without providing actionable guidance on testing or risk mitigation. The comparison is made to the Web Application Security Verification Standard, where specific attack variants are better integrated. It may be beneficial to revise the structure to enhance clarity and usability in verifying security standards.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-thick-client-application-security-verification-standard/issues/7",
@@ -329,6 +341,7 @@
"node_id": "I_kwDOLDUCls6VoUff",
"title": "Consider bringing in new items from V5 web ASVS for crypto ",
"body": "We could expand our list, for example nonces/IV is one I'd really like to bring in: https://github.com/OWASP/ASVS/blob/master/5.0/en/0x14-V6-Cryptography.md",
+ "summary": "The issue suggests incorporating new items from the V5 web ASVS related to cryptography into the existing framework. Specifically, the author expresses interest in adding concepts like nonces and initialization vectors (IVs). It would be beneficial to review the linked ASVS document and determine which additional cryptographic items can be integrated into the current standards.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-thick-client-application-security-verification-standard/issues/8",
@@ -355,10 +368,11 @@
"pk": 14,
"fields": {
"nest_created_at": "2024-09-11T19:12:15.554Z",
- "nest_updated_at": "2024-09-11T19:12:15.554Z",
+ "nest_updated_at": "2024-09-13T14:58:05.082Z",
"node_id": "I_kwDOKrhCTc6Byt6F",
"title": "Please add a description to this project",
"body": "",
+ "summary": "The issue requests the addition of a project description to provide clarity and context. It would be beneficial to outline the project's purpose, features, and any relevant details to enhance understanding for potential users and contributors.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-antiforensics-project/issues/1",
@@ -381,10 +395,11 @@
"pk": 15,
"fields": {
"nest_created_at": "2024-09-11T19:12:19.748Z",
- "nest_updated_at": "2024-09-11T19:12:19.748Z",
+ "nest_updated_at": "2024-09-13T14:58:08.330Z",
"node_id": "I_kwDOKrgTWc6Byt17",
"title": "Please add a description to this project",
"body": "",
+ "summary": "The issue requests the addition of a project description. Providing a clear and concise description will help users understand the purpose and functionality of the project. It is recommended to draft a brief overview that outlines the main features and goals of the project.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-top-10-in-xr/issues/1",
@@ -411,6 +426,7 @@
"node_id": "I_kwDOKYMc786H6did",
"title": "False negative related to SQL Injection",
"body": "The endpoint: `https://brokencrystals.com/api/testimonials/count?query=%27` is vulnerable to an SQL injection\r\n\r\nThe endpoint does NOT return `50X` error when the SQL injection occurs, thus: `STATUS_CODE_FILTER` doesn't catch it\r\n\r\nI believe it would be a smart idea to look for common SQL errors such as:\r\n`' - unterminated quoted string at or near \"'\"`\r\n\r\nOther errors are listed here:\r\nhttps://owasp.org/www-project-web-security-testing-guide/latest/4-Web_Application_Security_Testing/07-Input_Validation_Testing/05-Testing_for_SQL_Injection\r\n\r\nI can't easily find one 'list' that has all the SQL errors",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OFFAT/issues/100",
@@ -437,6 +453,7 @@
"node_id": "I_kwDOKYMc786IlNBg",
"title": "Add automated tests",
"body": "Currently each and every PR raised need to be tested manually. Add automated tests using pytest/unittest library which can test PR before merging using Github actions.",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OFFAT/issues/106",
@@ -463,6 +480,7 @@
"node_id": "I_kwDOKYMc786MIhVC",
"title": "Support for HTTP/2",
"body": "If I understand correctly, OFFAT does not currently work for HTTP/2? I tried to fuzz some API that uses HTTP/2, but OFFAT produces \r\n\r\n`RemoteDisconnected('Remote end closed connection without response')`\r\n\r\nIs support for this planned in the future?",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OFFAT/issues/116",
@@ -487,10 +505,11 @@
"pk": 19,
"fields": {
"nest_created_at": "2024-09-11T19:13:31.551Z",
- "nest_updated_at": "2024-09-11T19:13:31.551Z",
+ "nest_updated_at": "2024-09-13T14:59:01.356Z",
"node_id": "I_kwDOKYMc786S-W9h",
"title": "Option to bypass host availability check",
"body": "If the server now returns a 502 on the root request, the testing will stop. Some servers only reply to valid paths for request. It would be nice if we can have a flag to bypass this \"check whether host is available\". Further, it appears that this host check request is not passed through the provided http proxy using -p http://127.0.0.1:8080.",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OFFAT/issues/131",
@@ -517,10 +536,11 @@
"pk": 20,
"fields": {
"nest_created_at": "2024-09-11T19:14:15.683Z",
- "nest_updated_at": "2024-09-11T19:14:15.683Z",
+ "nest_updated_at": "2024-09-13T14:59:15.920Z",
"node_id": "I_kwDOKSOWo86Byt0T",
"title": "Please add a description to this project",
"body": "",
+ "summary": "The issue requests the addition of a project description to provide clarity and context. It would be helpful to create a concise summary that outlines the project's purpose, features, and any relevant details for potential users or contributors.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-ai-top-ten/issues/1",
@@ -543,10 +563,11 @@
"pk": 21,
"fields": {
"nest_created_at": "2024-09-11T19:14:29.608Z",
- "nest_updated_at": "2024-09-11T19:14:29.608Z",
+ "nest_updated_at": "2024-09-13T14:59:26.954Z",
"node_id": "I_kwDOKLCek86Bytxf",
"title": "Please add a description to this project",
"body": "",
+ "summary": "The issue requests the addition of a project description to provide clarity and context about its purpose and functionality. To address this, a concise and informative description should be drafted and added to the project documentation.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-memory-safety/issues/1",
@@ -573,6 +594,7 @@
"node_id": "I_kwDOKLCYV85zp7QN",
"title": "Bootloader Test Cases",
"body": "A bootloader section would provide test case coverages at lower lever components that create chains of trust that secure devices at boot and their identity",
+ "summary": "The issue discusses the need for a dedicated section that outlines test cases for bootloaders. These test cases should focus on lower-level components that establish chains of trust, ensuring device security during boot and maintaining their identity. To address this issue, it would be beneficial to develop and document comprehensive test cases that cover these aspects.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/owasp-istg/issues/2",
@@ -601,6 +623,7 @@
"node_id": "I_kwDOKLCYV85005W_",
"title": "ATT&CK ICS, D3FEND & ISTG",
"body": "Folks have asked about relation to adjacent frameworks like ATT&CK ICS, D3FEND (links below) and overlap of them with ISTG. \r\n\r\nhttps://attack.mitre.org/techniques/ics/\r\nhttps://attack.mitre.org/matrices/ics/ \r\nhttps://d3fend.mitre.org/\r\n\r\nCurious if the project has a perspective to share and if there are opportunities to partner or collaborate on mappings in the future?",
+ "summary": "The issue discusses the relationship between the ATT&CK ICS, D3FEND frameworks, and their overlap with ISTG. There is a request for insights on how these frameworks interrelate and an interest in exploring potential collaboration or mapping opportunities in the future. It may be beneficial to outline any existing connections or propose a plan for collaboration on mappings.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/owasp-istg/issues/3",
@@ -629,6 +652,7 @@
"node_id": "I_kwDOKLCYV853SzuL",
"title": "Create ISTG cover art and align on look/feel with MSTG where possible",
"body": "MSTG is a mature flagship OWASP project with a large following and a steady flow of contributors maintaining the guide. Until ISTG has a similar maturity and following, it'll be challenging to keep up with MSTG. Although, we should aim to align where it make sense and build relationships with testing guide project leaders for support. \r\n\r\nObservations and opportunities to align\r\n- ISTG should have a similar cover to [MSTG's]( https://github.com/OWASP/owasp-mastg/blob/master/Document/Images/mstg_cover.png) \r\n- GitHub pages theme ([material](https://github.com/OWASP/owasp-istg)) should be similar \r\n- Add a download link to the checklist and other formats like PDF \r\n- Input validation category abbreviation detailed in https://github.com/OWASP/owasp-istg/issues/4",
+ "summary": "The issue discusses the need to create cover art for the ISTG (Mobile Security Testing Guide) that aligns in look and feel with the MSTG (Mobile Security Testing Guide). It highlights that while MSTG is a well-established project, ISTG should strive to align its branding and resources where feasible to foster relationships with MSTG project leaders for support.\n\nKey observations include:\n- Designing ISTG cover art to resemble the MSTG cover.\n- Ensuring the GitHub pages theme for ISTG is consistent with MSTG’s.\n- Adding a download link for the checklist and other formats like PDF.\n- Addressing a specific detail related to input validation category abbreviations.\n\nTo proceed, the team should focus on creating the cover art and aligning the GitHub pages theme accordingly. Additionally, they should implement the suggested download link and follow up on the input validation category issue.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/owasp-istg/issues/6",
@@ -655,6 +679,7 @@
"node_id": "I_kwDOKLCYV86ASsrT",
"title": "Test objective for analyzing build flags of compiled binaries",
"body": "Add a test objective that analyzes compiled binaries build flags for PIE/ASLR, NX, etc. that aid in decompilation strategies for reversing.\r\n\r\nReferring to:\r\n\r\n- [ISTG-MEM-INFO-001](https://github.com/OWASP/owasp-istg/tree/main/src/03_test_cases/memory#disclosure-of-source-code-and-binaries-istg-mem-info-001)\r\n- [ISTG-FW-INFO-001](https://github.com/OWASP/owasp-istg/blob/main/src/03_test_cases/firmware/README.md#disclosure-of-source-code-and-binaries-istg-fw-info-001)\r\n",
+ "summary": "The issue suggests adding a test objective focused on analyzing the build flags of compiled binaries, specifically looking for attributes like Position Independent Execution (PIE), Address Space Layout Randomization (ASLR), and Non-Executable (NX) bits. These elements are crucial for developing decompilation strategies in reverse engineering efforts. To address this, it may be beneficial to reference existing test cases related to the disclosure of source code and binaries from the provided links.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/owasp-istg/issues/8",
@@ -679,10 +704,11 @@
"pk": 26,
"fields": {
"nest_created_at": "2024-09-11T19:14:51.096Z",
- "nest_updated_at": "2024-09-11T19:14:51.096Z",
+ "nest_updated_at": "2024-09-13T14:59:40.273Z",
"node_id": "I_kwDOKHixBs6Bytup",
"title": "Please add a description to this project",
"body": "",
+ "summary": "The project currently lacks a description, which is essential for understanding its purpose and functionality. It is suggested to create a clear and concise overview that outlines the project's objectives, features, and usage instructions. This will help potential users and contributors better engage with the project.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-untrust/issues/1",
@@ -705,10 +731,11 @@
"pk": 27,
"fields": {
"nest_created_at": "2024-09-11T19:15:24.498Z",
- "nest_updated_at": "2024-09-11T19:15:24.498Z",
+ "nest_updated_at": "2024-09-13T15:00:06.635Z",
"node_id": "I_kwDOJ_RyPc6Bytpb",
"title": "Please add a description to this project",
"body": "",
+ "summary": "The issue requests the addition of a project description to provide clarity and context. It is important to create a concise and informative summary that outlines the project's purpose, features, and goals.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-api-governance/issues/1",
@@ -731,10 +758,11 @@
"pk": 28,
"fields": {
"nest_created_at": "2024-09-11T19:15:28.512Z",
- "nest_updated_at": "2024-09-11T19:15:28.512Z",
+ "nest_updated_at": "2024-09-13T15:00:10.085Z",
"node_id": "I_kwDOJ8-yTs6NkH0A",
"title": "Need to know the status",
"body": "Hi Team,\r\n\r\nAny update on this project and where are we standing !. Let me know if need any help !\r\n\r\nThanks,\r\nJanibasha",
+ "summary": "The issue seeks an update on the project's current status. It indicates that assistance may be offered if needed. To move forward, a response detailing the project's progress and any required support would be beneficial.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-llm-prompt-hacking/issues/1",
@@ -757,10 +785,11 @@
"pk": 29,
"fields": {
"nest_created_at": "2024-09-11T19:15:58.522Z",
- "nest_updated_at": "2024-09-11T19:15:58.522Z",
+ "nest_updated_at": "2024-09-13T15:00:33.944Z",
"node_id": "I_kwDOJx_i6s6Bytkp",
"title": "Please add a description to this project",
"body": "",
+ "summary": "The issue requests the addition of a project description to provide clarity and context. It would be beneficial to draft a concise overview that outlines the project's purpose, features, and any relevant details to enhance understanding for users and contributors.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-kubefim/issues/1",
@@ -783,10 +812,11 @@
"pk": 30,
"fields": {
"nest_created_at": "2024-09-11T19:16:09.384Z",
- "nest_updated_at": "2024-09-11T19:16:09.384Z",
+ "nest_updated_at": "2024-09-13T15:00:42.697Z",
"node_id": "I_kwDOJsDs1M6Bytiv",
"title": "Please add a description to this project",
"body": "",
+ "summary": "The issue requests the addition of a project description to provide clarity and context. To address this, a concise and informative description should be crafted, outlining the project's purpose, features, and any relevant details that would help users understand its functionality.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-open-security-information-base/issues/2",
@@ -813,6 +843,7 @@
"node_id": "I_kwDOJjxT185tlC3F",
"title": "Missing app layers",
"body": "One of the key ways LLMs/ generative aI is used is through chaining/ agents. Agents put in one of the biggest risks for applications based on LLM/ Generative AI. There are issues with users allowing machine access to agents to perform tasks and agents seem to work autonomously creating security holes.",
+ "summary": "The issue highlights concerns regarding the security risks associated with using agents in applications based on large language models (LLMs) and generative AI. Specifically, it points out that allowing machine access to these agents could lead to autonomous behavior that may create vulnerabilities. To address this, a review of the current implementation of agent access and security measures is necessary to mitigate potential risks.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/issues/117",
@@ -830,8 +861,8 @@
16
],
"labels": [
- 7,
- 8
+ 8,
+ 7
]
}
},
@@ -844,6 +875,7 @@
"node_id": "I_kwDOJjxT185to50E",
"title": "Remove LLM05: Supply Chain Vulnerabilities in Favor of LLM03: Training Data Poisoning and LLM07: Insecure Plugin Design",
"body": "# **Background:**\r\n- As per published [v1.0](https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/tree/main/1_0_vulns) of the OWASP Top 10 for Large Language Model Applications project, I believe we have a duplicate entry within `LLM05: Supply Chain Vulnerabilities`\r\n - The [LLM-05](https://llmtop10.com/llm05/) `LLM05: Supply Chain Vulnerabilities` entry largely overlaps with [LLM-03](https://llmtop10.com/llm03/) `LLM03: Training Data Poisoning`\r\n - The [LLM-05](https://llmtop10.com/llm05/) `LLM05: Supply Chain Vulnerabilities` entry also overlaps with the topic of plugins in [LLM07: Insecure Plugin Design](https://llmtop10.com/llm07/) `LLM07: Insecure Plugin Design`\r\n- This is causing confusion, inconsistency and leaves us not fully utilizing the power of the Top 10 list to include any new vulnerabilities surfaced or if we decide to look at some of the [vulnerables < v1.0](https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/tree/main/Archive) which didn't make the cut when we revise [v.1.0.1](https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/tree/main/1_0_1_vulns) of the project\r\n\r\n- If we also take the foundation of the OWASP Top 10 lists - [OWASP Top 10 API Security Risks – 2023](https://owasp.org/www-project-top-ten/) as an example, then it is clear (or at least ot a security professional) that even though Supply Chain is not listed as a vulnerability but an organization referencing and basing their Web Application Security stack on the OWASP Top 10, certainly still need to take the whole concept of Supply Chain (via SBOM, however) is still extremely important to that software stack.\r\n- When we threat model an LLM Application, we have to ensure that are some pre-requisites taken into consideration which make them out of scope for us to focus specifically on risks and vulnerabilities present in the area we are assessing. These may be explicitly stated or inherently understood and is not listed as a top risk during the exercise.\r\n\r\n# **Proposed Changes**\r\n- Whilst I agree Supply Chain is an important topic in any security considerations, only the important **true top 10 vulnerabilities** require each subset referencing the supply-chain if it is valid in that specific area - I.E, Training Data Poisoning, Plugins etc. \r\n - I do not believe we need an entry soley dedicated to Supply Chain itself and each entry that has a Supply Chain as an example attack scenario should reference the supply chain topic here",
+ "summary": "The issue raises concerns about the redundancy of the entry \"LLM05: Supply Chain Vulnerabilities\" within the OWASP Top 10 for Large Language Model Applications. It argues that this entry overlaps significantly with \"LLM03: Training Data Poisoning\" and \"LLM07: Insecure Plugin Design,\" leading to confusion and inconsistency. The author suggests that while supply chain vulnerabilities are important, they should be referenced within relevant entries rather than having a separate category. \n\nTo address this, the proposed change is to remove the redundant supply chain entry and ensure that any mention of supply chain vulnerabilities is integrated into the other related categories. This would streamline the list and enhance clarity regarding the top vulnerabilities in LLM applications.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/issues/119",
@@ -879,6 +911,7 @@
"node_id": "I_kwDOJjxT185wOkr7",
"title": "Sensitive Information Disclosure",
"body": "In summary, this “vulnerability” is problematic because it mostly doesn’t represent a root cause, but a result or symptom. In the 2021 OWASP Top 10, they reoriented from symptoms to root causes. They actually renamed sensitive information exposure to cryptographic failure to focus on root causes.\r\n\r\nThere is, however, one aspect of this vulnerability that seems to be a true root cause. Regardless of the input it gets, large language model outputs are unpredictable. That unpredictability doesn’t necessarily come from training data poisoning, third-party data sources, or prompt injection. It comes from the stochastic nature and natural language processing capabilities of the large language model as well as ambiguities and inaccuracies inherent in natural language. I would argue that this aspect is a key point mentioned in this section that should be carried forward, however, **I believe it makes more sense in Overreliance**. \r\n",
+ "summary": "The issue discusses the classification of a vulnerability related to sensitive information disclosure, emphasizing that it is more of a symptom rather than a root cause. The author references the OWASP Top 10's shift in focus from symptoms to root causes, suggesting that the unpredictability of large language model outputs is a significant root cause. This unpredictability stems from the stochastic nature of these models and the inherent ambiguities in natural language, which may not be directly linked to typical concerns like data poisoning or prompt injection. It is proposed that this aspect should be integrated into the discussion on Overreliance instead. \n\nTo address this issue, it may be necessary to reevaluate how vulnerabilities are categorized, particularly in relation to the unpredictability of language models.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/issues/140",
@@ -910,6 +943,7 @@
"node_id": "I_kwDOJjxT185w0GmG",
"title": "Recommended Updates to Excessive Agency",
"body": "Recommend \r\ntrialed instead of trialled\r\n\r\nfavor instead of favour\r\n\r\netc. instead of etc\r\n\r\nAdd 'to' to this phrase: Limit the permissions that LLM plugins/tools are granted to other systems the minimum necessary\r\nSo it reads: Limit the permissions that LLM plugins/tools are granted to other systems to the minimum necessary\r\n\r\nConsider reworking the following:\r\nExcessive Agency is the vulnerability that enables damaging actions to be performed in response to unexpected/ambiguous outputs from an LLM (regardless of what is causing the LLM to malfunction; be it hallucination/confabulation, direct/indirect prompt injection, malicious plugin, poorly-engineered benign prompts, or just a poorly-performing model). The root cause of Excessive Agency is typically one or more of: excessive functionality, excessive permissions or excessive autonomy.\r\n\r\nTo be:\r\nExcessive Agency is the vulnerability that enables damaging actions to be performed in response to unexpected/ambiguous outputs from an LLM (regardless of what is causing the LLM to malfunction; be it hallucination/confabulation, direct/indirect prompt injection, malicious plugin, poorly-engineered benign prompts, or just a poorly-performing model). Excessive agency is a vulnerability of excessive functionality, permissions, and/ or autonomy. This differs from Insecure Output Handling which is concerned with insufficient scrutiny of LLM outputs.\r\n \r\n \r\nConsider these adjustments to Common Examples of Vulnerability\r\n1. Excessive Functionality: An LLM agent has access to plugins which include functions that are not needed for the intended operation of the system. For example, a developer needs to grant an LLM agent the ability to read documents from a repository, but the 3rd-party plugin they choose to use also includes the ability to modify and delete documents.\r\n2. Excessive Functionality: A plugin that was trialed during the development phase was dropped in favor of a better alternative, but the original plugin remains available to the LLM agent.\r\n3. Excessive Permissions: An LLM plugin has permissions on other systems that are not needed for the intended operation of the application. E.g., a plugin intended to read data connects to a database server using an identity that not only has SELECT permissions, but also UPDATE, INSERT and DELETE permissions.\r\n4. Excessive Permissions: An LLM plugin that is designed to perform operations on behalf of a user accesses downstream systems with a generic high-privileged identity. E.g., a plugin to read the current user's document store connects to the document repository with a privileged account that has access to all users' files.\r\n5. Excessive Autonomy: An LLM-based application or plugin fails to independently verify and approve high-impact actions. E.g., a plugin that allows a user's documents to be deleted performs deletions without any confirmation from the user.\r\n\r\n\r\n\r\n\r\n",
+ "summary": "The issue suggests several updates to the documentation regarding Excessive Agency, including language changes and clarifications. \n\nRecommendations include replacing \"trialled\" with \"trialed,\" \"favour\" with \"favor,\" and adding \"to\" in a specified phrase for clarity. Additionally, a reworking of the definition of Excessive Agency is proposed to better differentiate it from Insecure Output Handling, emphasizing the role of excessive functionality, permissions, and autonomy as root causes.\n\nFurther adjustments are suggested for the section on Common Examples of Vulnerability, providing clearer examples of excessive functionality, permissions, and autonomy in LLM plugins. \n\nTo address this issue, implement the recommended edits and enhancements to the documentation.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/issues/172",
@@ -940,6 +974,7 @@
"node_id": "I_kwDOJjxT185w0Ix7",
"title": "Consider changes/updates to insecure plugin design",
"body": "Addition to the intro:\r\nSince plugins are, under normal circumstances, accessed only by the LLM, exploitation is typically a result of another vulnerability such as excessive agency or direct or indirect prompt injection. However, plugins are still responsible for protecting themselves since side-channel attacks can still occur.\r\n\r\n\r\nPossibly updates to Common Examples:\r\n1. A plugin accepts its input parameters in a single text field instead of distinct input parameters that can be validated and sanitized.\r\n3. A plugin accepts raw SQL or programming statements, which are more difficult to validate than distinct parameters.\r\n5. A plugin adheres to inadequate fine grained authorization controls. \r\n6. A plugin blindly trusts that the LLM output, which is the input to the plugin, correctly represents the expected output for the initial prompt. \r\n7. A plugin treats all LLM content as being created entirely by the user and performs any requested actions without requiring additional authorization.\r\n\r\nPossiblr changes to attack scenarios\r\n2. A plugin used to retrieve embeddings from a vector store accepts configuration parameters as a connection string without any validation. This allows an attacker to experiment and access other vector stores by changing names or host parameters and exfiltrate embeddings they should not have access to. \r\n3. A plugin accepts SQL WHERE clauses as advanced filters, which are then appended to the filtering SQL. This allows an attacker to stage a SQL attack.\r\n4. An attacker uses indirect prompt injection to exploit an insecure code management plugin that has no input validation and weak access control to transfer repository ownership and lock out the user from their repositories.",
+ "summary": "The issue discusses the need for updates to the design of insecure plugins, emphasizing the importance of self-protection against potential vulnerabilities. It suggests enhancing the introduction to highlight that while plugins are mainly accessed by the LLM, they must still safeguard against side-channel attacks.\n\nThe issue proposes revisions to the \"Common Examples\" section, focusing on various insecure practices such as inadequate input validation, reliance on raw SQL statements, insufficient authorization controls, and assumptions regarding the trustworthiness of LLM output.\n\nAdditionally, it outlines potential attack scenarios, emphasizing risks associated with accepting unvalidated configuration parameters, SQL injection vulnerabilities, and the dangers of indirect prompt injection leading to unauthorized actions, like repository ownership transfer.\n\nTo address these concerns, it may be necessary to implement stricter validation and sanitization measures for plugin inputs, enhance authorization controls, and ensure that plugins do not blindly trust LLM outputs.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/issues/174",
@@ -970,6 +1005,7 @@
"node_id": "I_kwDOJjxT185xxQ2q",
"title": "Change from Vulnerabilities to Risks",
"body": "With the realization that the top 10 focuses on the risks that vulnerabilities, I recommend changing our template and the content of the Top 10 to match.\r\n\r\nThe TL;DR \r\n\r\nFo to the [OWASP Top 10](https://owasp.org/www-project-top-ten/) page and the first 2 sentences read:\r\n> The OWASP Top 10 is a standard awareness document for developers and web application security. It represents a broad consensus about the most critical security risks to web applications.\r\n\r\nMy detailed reasoning is in this document: [risks-vs-vulnerabilities.md](https://github.com/Bobsimonoff/LLM-4-Applications-Commentary/blob/main/docs/risks-vs-vulnerabilities.md)",
+ "summary": "The issue suggests changing the terminology from \"Vulnerabilities\" to \"Risks\" in the context of the OWASP Top 10, as the focus is more on the risks associated with vulnerabilities. The author points to the OWASP Top 10 page, which defines it as a document highlighting critical security risks to web applications. A detailed rationale is provided in an accompanying document. \n\nTo address this issue, the template and content of the Top 10 should be updated to reflect this focus on risks rather than vulnerabilities.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/issues/188",
@@ -987,8 +1023,8 @@
20
],
"labels": [
- 7,
- 12
+ 12,
+ 7
]
}
},
@@ -1001,6 +1037,7 @@
"node_id": "I_kwDOJjxT185yPKW0",
"title": "(2) Website Typos + Proposed Fixes",
"body": "# Issue 1\r\n**# Where:** https://llmtop10.com/\r\n**# What:** In LLM06's \"feature box\" there is a typo inconsistent with other assets / grammar.\r\n**# Current:** \r\n\r\n>“***LLM’s*** may reveal confidential data...”\r\n\r\n**# Proposed:** \r\n>***LLMs*** (no apostrophe) may reveal confidential data...\r\n\r\n**# Justification:** \r\nGrammar / continuity (to match the PDF, Whitepaper, and Google Doc which all contain \"LLMs\"; the plural of LLM, without an apostrophe, for LLM06.\r\n\r\n# Issue 2 \r\n**# Where:** https://llmtop10.com/intro/\r\n**# What:** In the 1st sentence there is a typo.\r\n**# Current:** \r\n> “The frenzy ***of*** interest ***of*** Large Language Models (LLMs) following ***of*** mass-market pre- trained chatbots in late 2022 has been remarkable.”\r\n\r\n**# Proposed:** \r\n> The frenzy of interest in Large Language Models (LLMs) following the mass marketing of pretrained chatbots in late 2022 has been remarkable.\r\n\r\n**# Justification:** \r\nTypo impacting clarity.\r\n\r\n# Notes:\r\n1. **Potential labels:** \"bug\" + \"website\" **—PS:** It's my understanding I can't assign a \"Label\" because I am not a repo \"Contributor\", or else I'd add the labels; please let me know if that's not the case.\r\n2. Great project / community, hope this helps. :heart:",
+ "summary": "The issue highlights two typos found on the website https://llmtop10.com/. \n\n**Issue 1:** In the feature box for LLM06, \"LLM’s\" should be corrected to \"LLMs\" to ensure grammatical consistency with other materials. \n\n**Issue 2:** On the introduction page, the first sentence contains multiple occurrences of \"of\" that disrupt clarity. It should be revised to read: \"The frenzy of interest in Large Language Models (LLMs) following the mass marketing of pretrained chatbots in late 2022 has been remarkable.\"\n\nFor resolution, it is suggested to correct the identified typos on the website. Additionally, appropriate labels such as \"bug\" and \"website\" could be added to the issue for better tracking.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/issues/196",
@@ -1030,6 +1067,7 @@
"node_id": "I_kwDOJjxT185yc-Fn",
"title": "Overreliance: Prevention could include Human in the loop",
"body": "Since overreliance occurs with humans or systems overly rely on LLM outputs, I think human in the loop is still valid as a how to prevent measure. ",
+ "summary": "The issue discusses the concern of overreliance on large language model (LLM) outputs by humans or systems. It suggests that incorporating a human in the loop could be an effective preventive measure. To address this, it may be beneficial to explore strategies for integrating human oversight into workflows that utilize LLMs.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/issues/199",
@@ -1060,6 +1098,7 @@
"node_id": "I_kwDOJjxT185ydD7j",
"title": "template for example attack scenarios change for consistency",
"body": "\r\nCondensing the template a bit to demonstrate the issue. I think the Example Attack Scenarios should change .... see below:\r\n\r\n### Common Examples of Risk\r\n\r\n1. Example 1: Specific instance or type of this risk.\r\n\r\n### Prevention and Mitigation Strategies\r\n\r\n1. Prevention Step 1: A step or strategy that can be used to prevent the risk or mitigate its effects.\r\n\r\n### Example Attack Scenarios\r\n\r\nold\r\n> Scenario #1: A detailed scenario illustrating how an attacker could potentially exploit this risk, including the attacker's actions and the potential outcomes.\r\n\r\nnew \r\n> 1. Scenario #1: A detailed scenario illustrating how an attacker could potentially exploit this risk, including the attacker's actions and the potential outcomes.\r\n",
+ "summary": "The issue discusses the need for consistency in the formatting of the \"Example Attack Scenarios\" section of a template. The suggestion is to modify the existing format by changing the numbering style for scenarios to align with the rest of the template. The proposed change is to replace the old format with a new one that begins the scenarios with a numerical listing. To implement this, the formatting across the template should be reviewed and adjusted accordingly.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/issues/200",
@@ -1090,6 +1129,7 @@
"node_id": "I_kwDOJjxT1850jeQz",
"title": "Merge summary file into the Vulnerabilities files",
"body": "Currently the summary of each risk is in a single file that is separate from the actual risk details. This causes a disconnect when the risk is updated. Above description in the template for each risk I would like to see the summary section. Then at production time all the summary sections can be grabbed and put into a single file for PDF generation. ",
+ "summary": "The issue highlights the need to integrate the summary sections of risk descriptions into the Vulnerabilities files to ensure coherence and ease of updates. The proposed solution is to modify the template for each risk to include the summary section, allowing for the aggregation of all summaries into a single file for PDF generation during production. Implementing this change will improve the accessibility and usability of the risk documentation.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/issues/225",
@@ -1120,6 +1160,7 @@
"node_id": "I_kwDOJjxT18509gbo",
"title": "Enhance the OWASP LLM Applications Project with a Related Patterns Functionality",
"body": "IMO, we should look to provide a glossary or [`CAPEC`](https://capec.mitre.org/) approach to the OWASP LLM Application vulnerabilities - Similar to the way it is done with The [OWASP Web Application](https://owasp.org/Top10/) standards framework, see \"[OWASP Related Patterns](https://owasp.org/www-community/attacks/)\"\r\n\r\nA typical CAPEC entry includes a detailed Execution Flow. This consists of 3 sections:\r\n\r\n- **Explore** – Guidance on what to look for that may indicate that the software may be vulnerable to this attack.\r\n- **Experiment** – Guidance on possible ways to test if the software may be vulnerable to this attack.\r\n- **Exploit** – Summary of possible ways to exploit the software if your experiments are successful.\r\nIn many of the CAPEC entries, there will also be an external mapping to one of three possible other data sources:\r\n\r\n[WASC Threat Classification 2.0](http://projects.webappsec.org/w/page/13246978/Threat%20Classification) – A comprehensive framework from The Web Application Security Consortium that categorizes and organizes key security threats to web applications to facilitate standardizing threat reporting and response.\r\n[ATT&CK Related Patterns](https://attack.mitre.org/techniques/enterprise/) – A curated set of adversary behavior descriptors collected by MITRE, providing invaluable insights into the techniques used by threat actors to compromise and maneuver within systems.\r\n[OWASP Related Patterns](https://owasp.org/www-community/attacks/) – A set of techniques that attackers use to exploit the vulnerabilities in applications.\r\n\r\nKudos to **SilverStr** for the awesome [blog post](https://danaepp.com/adversarial-thinking-for-bug-hunters) which triggered my inspiration for us to adopt this",
+ "summary": "The issue suggests enhancing the OWASP LLM Applications Project by incorporating a Related Patterns functionality, akin to the CAPEC framework. This enhancement would involve creating a glossary that outlines vulnerabilities in a structured manner, featuring sections on exploration, experimentation, and exploitation, similar to CAPEC entries. It also proposes external mappings to other relevant threat classification systems, such as WASC and MITRE's ATT&CK. To move forward, the project team should consider developing a detailed framework that aligns with these suggestions.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/issues/230",
@@ -1151,6 +1192,7 @@
"node_id": "I_kwDOJjxT18513f0Q",
"title": "Enhancement Suggestion: Add RAG to the main diagram",
"body": "Retrieval augmented generation (RAG) is technique to enrich LLMs with own data. It has become very popular as it lowers the complexity entry to enriching input in LLM apps, allows for better access controls as opposed to fine tuning, and is known to reduce hallucination (see https://www.securityweek.com/vector-embeddings-antidote-to-psychotic-llms-and-a-cure-for-alert-fatigue/) see also the excellent [Samsung paper on enterprise use of GenAI and the role of RAG](https://arxiv.org/ftp/arxiv/papers/2309/2309.01105.pdf).\r\n\r\nRAG creates its own security risks and adds to the attack surface. yet, the diagram only includes fine tuning, **We should add explicitly RAG as part of our diagram and annotate it with related LLM items**. \r\nSome useful links:\r\n**architectural approaches**\r\nAzure: https://github.com/Azure/GPT-RAG \r\nAWS SageMaker: https://docs.aws.amazon.com/sagemaker/latest/dg/jumpstart-foundation-models-customize-rag.html\r\nAWS Bedrock RAG workshop: https://github.com/aws-samples/amazon-bedrock-rag-workshop\r\n\r\n**security concerns:**\r\n[Security of AI Embeddings explained](https://ironcorelabs.com/ai-encryption/)\r\n[Anonymity at Risk? Assessing Re-Identification Capabilities of Large Language Models](https://arxiv.org/abs/2308.11103)\r\n[Embedding Layer: AI (Brace For These Hidden GPT Dangers)](https://predictivethought.com/embedding-layer-ai-brace-for-these-hidden-gpt-dangers/)\r\n",
+ "summary": "The issue suggests an enhancement to include Retrieval Augmented Generation (RAG) in the main diagram, as it is a valuable technique for enriching LLMs with data. RAG can lower the complexity of using LLM applications, improve access control compared to fine-tuning, and help mitigate hallucination issues. However, it also introduces new security risks.\n\nCurrently, the diagram only reflects fine-tuning, and the proposal is to explicitly integrate RAG, along with annotations related to LLMs. Several resources provided include architectural approaches from Azure and AWS, as well as security concerns related to AI embeddings. \n\nTo address this issue, the main task would be to update the diagram to incorporate RAG and its associated implications.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/issues/240",
@@ -1182,6 +1224,7 @@
"node_id": "I_kwDOJjxT18513jts",
"title": "Create Threat Model and Discuss RAG with its security risks for LLM",
"body": "Retrieval augmented generation (RAG) is a technique to enrich LLMs with the apps/org own data. It has become very popular as it lowers the complexity entry to enriching input in LLM apps, allows for better access controls as opposed to fine-tuning, and is known to reduce hallucination (see https://www.securityweek.com/vector-embeddings-antidote-to-psychotic-llms-and-a-cure-for-alert-fatigue/) see also the excellent [Samsung paper on enterprise use of GenAI and the role of RAG](https://arxiv.org/ftp/arxiv/papers/2309/2309.01105.pdf).\r\n\r\n**Currently, we have occasional references to RAG but we should create a threat model and discuss this on its own and the impact on the LLM top** 10. As RAG becomes a distinct enterprise PATTERN it also creates its own security risks and expands the attack surface with:\r\nThis includes \r\n- a second process of generating embeddings that can vary in complexity and can be using the main or secondary LLM\r\n- vector database\r\n- the use of embeddings in prompts and responses \r\n- use of other services (eg Azure Cognitive Services) or specialised plugins, for instance, the OpenAI upsert retrieval plugin for semantic search plugin(https://github.com/openai/chatgpt-retrieval-plugin)\r\n\r\n\r\n### _Some useful links_**\r\n**architectural approaches**\r\nAzure: https://github.com/Azure/GPT-RAG\r\nAWS SageMaker: https://docs.aws.amazon.com/sagemaker/latest/dg/jumpstart-foundation-models-customize-rag.html\r\nAWS Bedrock RAG workshop: https://github.com/aws-samples/amazon-bedrock-rag-workshop\r\n\r\n**security concerns**\r\n[Security of AI Embeddings explained](https://ironcorelabs.com/ai-encryption/)\r\n[Anonymity at Risk? Assessing Re-Identification Capabilities of Large Language Models](https://arxiv.org/abs/2308.11103)\r\n[Embedding Layer: AI (Brace For These Hidden GPT Dangers)](https://predictivethought.com/embedding-layer-ai-brace-for-these-hidden-gpt-dangers/)",
+ "summary": "The issue discusses the need to create a comprehensive threat model for Retrieval Augmented Generation (RAG), a technique that enhances large language models (LLMs) with organizational data. Despite its benefits in simplifying access to data, improving access controls, and reducing hallucinations, RAG introduces unique security risks and expands the attack surface. Key concerns include the complexity of generating embeddings, the security of vector databases, and the integration of external services and plugins.\n\nTo address these issues, it is recommended to systematically evaluate the security implications of RAG and outline a detailed threat model that considers its impact on LLMs. This would involve analyzing the security of architectural approaches and reviewing existing literature on potential vulnerabilities related to AI embeddings.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/issues/241",
@@ -1199,9 +1242,9 @@
17
],
"labels": [
- 7,
+ 19,
12,
- 19
+ 7
]
}
},
@@ -1214,6 +1257,7 @@
"node_id": "I_kwDOJjxT18516Vr_",
"title": "LLM07 - Insecure Plugin Design - Mitigation/How to Prevent Enhancements",
"body": "I believe [LLM07](https://llmtop10.com/llm07/) could benefit from some or all of these mitigation methods to be included in the vulnerability:\r\n\r\n- Human in the Loop: A plugin should not be able to invoke another plugin (by default), especially plugins with high stakes operations and to ensure that generated content meets quality and ethical standards.\r\n- It should be transparent to the user which plugin will be invoked, and what data is sent to it. Possibly even allow modifying the data before its sent.\r\n- A security contract and threat model for plugins should be created, so we can have a secure and open infrastructure where all parties know what their security responsibilities are. \r\n- An LLM application must assume plugins cannot be trusted (e.g. direct or indirect prompt injection), and similarly plugins cannot blindly trust LLM application invocations (example: confused deputy attack)\r\n- Regularly perform red teaming and model serialization attacks with thorough benchmarking and reporting of input and outputs.\r\n- Plugins that handle PII and/or impersonate the user are high stakes.\r\n- Isolation. Discussed before that follows a Kernel LLM vs. Sandbox LLMs could help. \r\n\r\nThese mitigation techniques are primarily focused towards combatting indirect prompt injection, but should pretty much be a defacto standard. I also think there should be some sort of statement or wording such as \"**Plugins should never be inheriently trusted**\".\r\n\r\nResource and inspiration kudos to [embracethered](https://embracethered.com/blog/posts/2023/chatgpt-cross-plugin-request-forgery-and-prompt-injection./).",
+ "summary": "The issue discusses potential enhancements for mitigating vulnerabilities related to the LLM07 - Insecure Plugin Design category. Key suggestions include:\n\n- Implementing a \"Human in the Loop\" approach to prevent plugins from invoking one another by default, especially for high-stakes operations.\n- Ensuring transparency regarding which plugins are invoked and what data is transmitted, allowing users to modify this data.\n- Establishing a security contract and threat model to clarify security responsibilities among all parties involved.\n- Assuming that plugins cannot be fully trusted, and vice versa, to prevent issues like prompt injection and confused deputy attacks.\n- Conducting regular red teaming and model serialization attacks with comprehensive benchmarking and reporting.\n- Recognizing that plugins dealing with Personally Identifiable Information (PII) or impersonation are particularly high-risk.\n- Considering isolation strategies, such as separating Kernel LLMs from Sandbox LLMs.\n\nThese measures primarily address indirect prompt injection and should be adopted as standard practice. A proposed statement emphasizes that \"Plugins should never be inherently trusted.\" Further exploration and implementation of these techniques are encouraged.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/issues/242",
@@ -1244,6 +1288,7 @@
"node_id": "I_kwDOJjxT1852ArkM",
"title": "Enhancement Suggestion: Enhance Diagram to Include LLM Architecture Types and Technologies",
"body": "To enhance our project and the next diagram artifact version we added for v1.1, I think we should include information about traditional REST API and websocket architecture into the diagram and how this interoperates with LLM state within Generative AI.\r\n\r\nThis architecture can be recon'd by an adversary in attempt to perform prompt injection or other techniques to exploit vulnerabilities within the LLM application.\r\n\r\n**Reference Examples:**\r\n\r\n- [amigoscode](https://amigoscode.com/)\r\n\r\n- https://livebook.manning.com/book/building-chatbots-with-microsoft-bot-framework-and-nodejs/chapter-6/v-4/",
+ "summary": "The issue proposes an enhancement for the project's next diagram artifact version by incorporating information on traditional REST API and websocket architecture. This addition aims to illustrate how these architectures interact with the LLM state within Generative AI, highlighting potential vulnerabilities, such as prompt injection. It suggests referencing existing examples to inform these enhancements. To address this, the team may need to analyze and integrate relevant architecture types and their implications for security in the upcoming diagram version.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/issues/243",
@@ -1275,6 +1320,7 @@
"node_id": "I_kwDOJjxT1852Kpmq",
"title": "Add the definitions to the documents/site",
"body": "The definitions currently live in the wiki, and aren't included in either the PDF or the website. We should fix that. ",
+ "summary": "The issue highlights the need to incorporate definitions from the wiki into the official documents and website, as they are currently missing from both the PDF and online content. To address this, the definitions should be extracted from the wiki and integrated into the relevant sections of the documents and website.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/issues/244",
@@ -1305,6 +1351,7 @@
"node_id": "I_kwDOJjxT1852i51h",
"title": "LLM-01: Adding example of Prompt injection in ReAct agents",
"body": "I propose to add a prompt injection attack scenario specific to organizations that are looking into implementing autonomous ReAct based agents to expose to customers, such as those that can be be built with Langchain. In these agents, it is possible to leverage prompt injection to inject Thoughts, Actions and Observations that alter the behaviour of the agent, but not via a direct jailbreak (i.e.: deviation from the system prompt) - instead they do so by altering the external reality of the agent.\r\n\r\n**Reference and attack example:**\r\nhttps://labs.withsecure.com/publications/llm-agent-prompt-injection\r\n\r\nProbably better understood in action in this short 30-sec video: https://www.linkedin.com/posts/withsecure_prompt-injection-for-react-llm-agents-ugcPost-7125756992341618688-ZFQ5\r\n\r\nSuggested text for the example to add: \"_An autonomous LLM agent on a web shop assists users in managining orders. It can access order details and issue refunds by using a set of tools via the ReAct (Reason+Act) framework. An attacker injects forged Thoughts, Actions and Observations into the LLM context via prompt injection. This tricks the LLM into thinking that the user has ordered an item they have not and that this item is eligible for a refund. Under these false premises, the agent proceeds to issue a refund to the malicious user for an order they never placed._\"\r\n\r\nIt's debatable though where such a scenario would fit: it's an injection attack, but not a direct jail break. The \"LLM08: Excessive Agency\" vulnerability definitely has an overlap, so does the the \"LLM07: Insecure Plugin Design\" to an extent. In this scenario mentioned by the article the agent should not be able to issue a refund if the original order doesn't exist or if information doesn't match the records.\r\n\r\n\r\n",
+ "summary": "The issue proposes adding an example of a prompt injection attack tailored for organizations implementing autonomous ReAct-based agents, particularly those built with Langchain. The attack involves manipulating the agent's behavior by altering its understanding of external reality rather than using a direct jailbreak. An illustrative scenario is provided where an attacker injects false information into a web shop's order management agent, leading it to issue a refund for a non-existent order. The discussion raises questions about the appropriate categorization of this attack, noting potential overlaps with existing vulnerabilities. To enhance security, it is suggested that agents should validate information against actual records before executing actions like refunds. \n\nIt may be helpful to incorporate this example into the documentation or relevant vulnerability discussions to better illustrate the risks associated with prompt injection in ReAct agents.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/issues/246",
@@ -1335,6 +1382,7 @@
"node_id": "I_kwDOJjxT1855Ah2v",
"title": "LLM05 - dead link",
"body": "**Description**, there is a dead link (https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/blob/main/1_1_vulns/InsecurePluginDesign.md) Insecure Plugin Design.\r\n\r\n**Prevention and Mitigation Strategies**, **item number 7**, there is a dead link (https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/blob/main/1_0_vulns/Training_Data_Poisoning.md) Training Data Poisoning.",
+ "summary": "The issue reports dead links in the documentation for \"Insecure Plugin Design\" and \"Training Data Poisoning.\" The links provided are non-functional, which likely hinders access to important information. To resolve the issue, the links should be updated or fixed to direct users to the correct resources.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/issues/254",
@@ -1367,6 +1415,7 @@
"node_id": "I_kwDOJjxT1856-VBt",
"title": "LLM10 - Additional bullet point that doesn't exist in Markdown files",
"body": "In LLM10 in the Common Examples of Vulnerability section of LLM10 there is an entire bullet point in there that doesn't exist in the markdown files for v1.1\r\n\r\n\r\n",
+ "summary": "The issue highlights a discrepancy where a bullet point present in the \"Common Examples of Vulnerability\" section of LLM10 is missing from the markdown files for version 1.1. To address this, it may be necessary to update the markdown files to include the missing bullet point to ensure consistency.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/issues/258",
@@ -1399,6 +1448,7 @@
"node_id": "I_kwDOJjxT1856-VSh",
"title": "LLM03 - Numbering of Prevention and Mitigation Strategies in PDF does not match Markdown",
"body": "The Prevention and Mitigation Strategies in the Markdown file for LLM10 goes up to higher however in the PDF it only goes up to 7 and has more sub points. \r\n\r\nhttps://github.com/OWASP/www-project-top-10-for-large-language-model-applications/blob/main/1_1_vulns/LLM03_TrainingDataPoisoning.md\r\n\r\n\r\n",
+ "summary": "The issue highlights a discrepancy between the numbering of Prevention and Mitigation Strategies in the Markdown file and the corresponding PDF for LLM03. The Markdown document lists strategies beyond number 7, while the PDF only goes up to 7 and includes additional sub points. To resolve this, the numbering in the PDF should be aligned with the Markdown file to ensure consistency.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/issues/259",
@@ -1431,6 +1481,7 @@
"node_id": "I_kwDOJjxT1858fhgF",
"title": "Add CloudBorne and CloudJacking Attacks to LLM-05 Supply Chain - CVE-2023-4969",
"body": "Remember, an issue is not the place to ask questions. You can use our [Slack channel](https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/wiki) for that, or you may want to start a discussion on the [Discussion Board](https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/discussions).\r\n\r\n### When reporting an issue, please be sure to include the following:\r\n- [X ] Before you open an issue, please check if a similar issue already exists or has been closed before.\r\n- [X ] A descriptive title and apply the specific **LLM-0-10** label relative to the entry. See our [available labels](https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/labels).\r\n- [X ] A description of the problem you're trying to solve, including *why* you think this is a problem\r\n- [X ] If the enhancement changes current behavior, reasons why your solution is better\r\n- [ ] What artifact and version of the project you're referencing, and the location (I.E OWASP site, llmtop10.com, repo)\r\n- [ ] The behavior you expect to see, and the actual behavior\r\n\r\n#### Steps to Reproduce\r\n-------------------------------------------\r\n\r\nNA\r\n\r\n#### What happens?\r\n-------------\r\n\r\nNA\r\n\r\n#### What were you expecting to happen?\r\n----------------------------------\r\n\r\nWithin the current [LLM entry](https://llmtop10.com/llm05/), I think supply-chain needs to cover cloudborne and cloudjacking attacks, IE GPU and cloud providers in further detail which the entry currently does not cover and is applicable to all companies and AI developers who depend on cloud resources.\r\nI added an article of interest below which I feel would back up this entry' resources section.\r\n\r\n#### Any logs, error output, etc?\r\n----------------------------\r\n\r\nNA\r\n\r\n#### Any other comments?\r\n-------------------\r\n[LeftoverLocals: Listening to LLM responses through leaked GPU local memory](https://blog.trailofbits.com/2024/01/16/leftoverlocals-listening-to-llm-responses-through-leaked-gpu-local-memory/)\r\n\r\n- [NA] Slack post link (if relevant)\r\n\r\n#### What versions of hardware and software are you using?\r\n----------------------------------------\r\n\r\nNA",
+ "summary": "The issue suggests that the current LLM-05 Supply Chain entry (CVE-2023-4969) should be updated to include details about CloudBorne and CloudJacking attacks, specifically in the context of GPU and cloud providers. This addition is deemed important for all companies and AI developers relying on cloud resources, as the topic is currently underrepresented. The submitter has provided a supportive article to enhance the resources section. To address this, the relevant updates and references should be incorporated into the existing entry.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/issues/264",
@@ -1448,8 +1499,8 @@
22
],
"labels": [
- 10,
- 17
+ 17,
+ 10
]
}
},
@@ -1462,6 +1513,7 @@
"node_id": "I_kwDOJjxT186DHV8Y",
"title": "Updating Prompt's logo + others",
"body": "Update the Prompt Security logo on the footer across all the site with the attached one\r\nOn this page https://llmtop10.com/contributors/, under 'Core Team' add Prompt Security as the company associated with Itamar Golan\r\nOn this page https://llmtop10.com/contributors/, under 'Contributors' add Prompt Security as the company associated with Lior Drihem\r\nOn this page https://llmtop10.com/contributors/, under 'Organizations', update Prompt's logo as well with the attached one\r\n\r\n\r\n",
+ "summary": "The issue involves several updates related to the Prompt Security logo and its association with team members on the contributors page of the website. Specifically, the tasks include: \n\n1. Updating the Prompt Security logo in the footer across the entire site with the provided new logo.\n2. Adding Prompt Security as the company associated with Itamar Golan under the 'Core Team' section on the contributors page.\n3. Adding Prompt Security as the company associated with Lior Drihem under the 'Contributors' section on the same page.\n4. Updating the organization section with the new Prompt logo as well.\n\nTo address this, the necessary updates to the logo and contributor associations need to be implemented on the specified pages.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/issues/277",
@@ -1479,8 +1531,8 @@
28
],
"labels": [
- 14,
- 21
+ 21,
+ 14
]
}
},
@@ -1493,6 +1545,7 @@
"node_id": "I_kwDOJjxT186EeoHH",
"title": "[suggestion enhancement] references of prompt injection -> jailbreaking, call out Many-Shot Jailbreaking explicitly",
"body": "Remember, an issue is not the place to ask questions. You can use our [Slack channel](https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/wiki) for that, or you may want to start a discussion on the [Discussion Board](https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/discussions).\r\n\r\n### When reporting an issue, please be sure to include the following:\r\n- [X] Before you open an issue, please check if a similar issue already exists or has been closed before.\r\n- [X] A descriptive title and apply the specific **LLM-0-10** label relative to the entry. See our [available labels](https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/labels).\r\n- [X] A description of the problem you're trying to solve, including *why* you think this is a problem\r\n- [X] If the enhancement changes current behavior, reasons why your solution is better\r\n- [X] What artifact and version of the project you're referencing, and the location (I.E OWASP site, llmtop10.com, repo)\r\n- [X] The behavior you expect to see, and the actual behavior\r\n\r\n#### Steps to Reproduce\r\n-------------------------------------------\r\n1. NA\r\n2. …\r\n3. …\r\n\r\n#### What happens?\r\n-------------\r\n\r\n`2_0_vulns/LLM01_PromptInjection.md` [here](https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/blob/main/2_0_vulns/LLM01_PromptInjection.md)\r\n\r\ni think we should segregate [Basics of prompt injection](https://hiddenlayer.com/research/prompt-injection-attacks-on-llms/#Basics-of-prompt-injection), [Jailbreaking](https://hiddenlayer.com/research/prompt-injection-attacks-on-llms/#Jailbreaking), [Prompt Leaking](https://hiddenlayer.com/research/prompt-injection-attacks-on-llms/#Prompt-Leaking), [Prompt Hijacking](https://hiddenlayer.com/research/prompt-injection-attacks-on-llms/#Prompt-Hijacking) and [Indirect Injections](https://hiddenlayer.com/research/prompt-injection-attacks-on-llms/#Indirect-Injections) as separate entities\r\n\r\nsince context windows are now longer and more powerful, Many-Shot Jailbreaking is a common technique which was not the case back in `v1.0` of our project and therefore i think it should be called out as a technique or at least a reference\r\n\r\n#### What were you expecting to happen?\r\n----------------------------------\r\n\r\n#### Any logs, error output, etc?\r\n----------------------------\r\n\r\n#### Any other comments?\r\n-------------------\r\n\r\nPosted in #team-llm-promptinjection [here](https://owasp.slack.com/archives/C05FHTB7QH0/p1712142327925279)\r\n\r\nSolid references:\r\n- https://hiddenlayer.com/research/prompt-injection-attacks-on-llms/\r\n- https://www.anthropic.com/research/many-shot-jailbreaking\r\n\r\n\r\n",
+ "summary": "The issue suggests enhancing the documentation by explicitly separating different types of prompt injection attacks, including Basics of Prompt Injection, Jailbreaking, Prompt Leaking, Prompt Hijacking, and Indirect Injections. It emphasizes the importance of recognizing Many-Shot Jailbreaking as a significant technique due to advancements in context windows. To address this, the documentation should be updated to acknowledge and detail this technique as a distinct reference. \n\nAction items may include revising the relevant documentation to incorporate these suggestions and ensure clarity on the different attack types.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/issues/281",
@@ -1521,6 +1574,7 @@
"node_id": "I_kwDOJjxT186FNc3W",
"title": "Extend LLM-04: RAG poisoning with glitch tokens causes DoS",
"body": "Remember, an issue is not the place to ask questions. You can use our [Slack channel](https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/wiki) for that, or you may want to start a discussion on the [Discussion Board](https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/discussions).\r\n\r\n### When reporting an issue, please be sure to include the following:\r\n- [x] Before you open an issue, please check if a similar issue already exists or has been closed before.\r\n- [x] A descriptive title and apply the specific **LLM-0-10** label relative to the entry. See our [available labels](https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/labels).\r\n- [x] A description of the problem you're trying to solve, including *why* you think this is a problem\r\n- [x] If the enhancement changes current behavior, reasons why your solution is better\r\n- [x] What artifact and version of the project you're referencing, and the location (I.E OWASP site, llmtop10.com, repo)\r\n- [x] The behavior you expect to see, and the actual behavior\r\n\r\n#### Steps to Reproduce\r\n-------------------------------------------\r\n1. NA\r\n2. …\r\n3. …\r\n\r\n#### What happens?\r\n-------------\r\n`2_0_vulns/LLM04_ModelDoS.md` does not refer to DoS through glitch tokens injected into the prompt from a RAG solution. If a malicious actor is able to introduce broadly relevant information to the RAG database which includes glitch tokens for the given model that is used they are able to effectively run a denial of service attack for all users of the LLM.\r\n\r\nThe main issue is that there is no clear indication which token caused the model to glitch so there is no obvious way to automatically remediate such issues. Enterprises build large RAG databases from (mostly) user generated content (i.e. Confluence / SharePoint / ... ) and also update this content frequently. A malicious actor could therefore easily introduce new content to the RAG database, including but not limited to glitch tokens which effectively cause a Denial of Service situation for all end users of the application.\r\n\r\n\r\n#### What were you expecting to happen?\r\n----------------------------------\r\n…\r\n\r\n#### Any logs, error output, etc?\r\n----------------------------\r\n\r\n\r\n#### Any other comments?\r\n-------------------\r\nA \"normal\" glitch token attack doesn't pose a significant threat as it only renders the current user session / context unusable. However through a poisoned RAG database a malicious user can inject these tokens into some/many/most/ if not all conversations, thus causing a broad service outage.\r\n\r\n#### Sources talking about the issue\r\n-------------------\r\n1. https://www.youtube.com/watch?v=WO2X3oZEJOA\r\n2. https://www.lesswrong.com/posts/aPeJE8bSo6rAFoLqg/solidgoldmagikarp-plus-prompt-generation\r\n3. https://www.lesswrong.com/posts/8viQEp8KBg2QSW4Yc/solidgoldmagikarp-iii-glitch-token-archaeology\r\n\r\n- [x] Slack post link (if relevant)\r\nhttps://owasp.slack.com/archives/C05FN2GDUE7/p1712761308419699\r\n\r\n#### What versions of hardware and software are you using?\r\n----------------------------------------\r\n**Operating System:** …\r\n**Browser:** …\r\n\r\n- [x] Chrome\r\n- [x] Firefox\r\n- [x] Edge\r\n- [x] Safari 11\r\n- [x] Safari 10\r\n- [x] IE 11\r\n",
+ "summary": "The issue discusses the vulnerability related to the LLM-04 model, specifically how RAG poisoning with glitch tokens can lead to a denial of service (DoS) for all users. It highlights that malicious actors can inject glitch tokens into the RAG database, which can disrupt the model's functionality across multiple user sessions. The current documentation does not address this potential exploitation, leaving a gap in understanding how to identify or remediate such attacks effectively. It suggests the need for enhancements to the documentation and possibly the model to mitigate these risks. Addressing this vulnerability is crucial to safeguard enterprise applications that rely on user-generated content for their RAG databases.",
"state": "open",
"state_reason": "reopened",
"url": "https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/issues/283",
@@ -1538,8 +1592,8 @@
30
],
"labels": [
- 17,
- 24
+ 24,
+ 17
]
}
},
@@ -1552,6 +1606,7 @@
"node_id": "I_kwDOJjxT186FWvRN",
"title": "setup automation for triage of issues against the project board",
"body": "Remember, an issue is not the place to ask questions. You can use our [Slack channel](https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/wiki) for that, or you may want to start a discussion on the [Discussion Board](https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/discussions).\r\n\r\n### When reporting an issue, please be sure to include the following:\r\n- [X] Before you open an issue, please check if a similar issue already exists or has been closed before.\r\n- [X] A descriptive title and apply the specific **LLM-0-10** label relative to the entry. See our [available labels](https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/labels).\r\n- [X] A description of the problem you're trying to solve, including *why* you think this is a problem\r\n- [X] If the enhancement changes current behavior, reasons why your solution is better\r\n- [X] What artifact and version of the project you're referencing, and the location (I.E OWASP site, llmtop10.com, repo)\r\n- [X] The behavior you expect to see, and the actual behavior\r\n\r\n#### Steps to Reproduce\r\n-------------------------------------------\r\n1. NA\r\n2. …\r\n3. …\r\n\r\n#### What happens?\r\n-------------\r\nright now, we currently don't have any automation for triage against our issue board which is slowing down inefficiency, productivity and not working to its full capability\r\n\r\n#### What were you expecting to happen?\r\n----------------------------------\r\ni'm going to setup automation (ie assignment of issues based on labels) to members of the core team to enhance efficiency\r\n\r\n#### Any logs, error output, etc?\r\n----------------------------\r\nNA\r\n\r\n#### Any other comments?\r\n-------------------\r\nhere is my prior PR for the GH issue template\r\n- https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/pull/256\r\n\r\n- [X] Slack post link (if relevant)\r\nhttps://owasp.slack.com/archives/C26MQBZ0B/p1712754527768819\r\nhttps://owasp.slack.com/archives/C05CM6DUZ1D/p1712754555606069\r\nneed github PAT/fine-grained token from OWASP org admins\r\n\r\n\r\n",
+ "summary": "The issue highlights the lack of automation for triaging issues on the project board, which is currently hindering efficiency and productivity. The author plans to implement automation, specifically by assigning issues based on labels to core team members. To proceed, there is a need for a GitHub Personal Access Token (PAT) or fine-grained token from OWASP organization administrators to enable the automation setup. It is suggested to address the automation requirement and obtain the necessary permissions.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/issues/285",
@@ -1580,6 +1635,7 @@
"node_id": "I_kwDOJjxT186FfGU9",
"title": "LLM-01 - Add canary tokens to Prevention and Mitigation Strategies",
"body": "Remember, an issue is not the place to ask questions. You can use our [Slack channel](https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/wiki) for that, or you may want to start a discussion on the [Discussion Board](https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/discussions).\r\n\r\n### When reporting an issue, please be sure to include the following:\r\n- [x] Before you open an issue, please check if a similar issue already exists or has been closed before.\r\n- [x] A descriptive title and apply the specific **LLM-0-10** label relative to the entry. See our [available labels](https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/labels).\r\n- [x] A description of the problem you're trying to solve, including *why* you think this is a problem\r\n- [x] If the enhancement changes current behavior, reasons why your solution is better\r\n- [x] What artifact and version of the project you're referencing, and the location (I.E OWASP site, llmtop10.com, repo)\r\n- [x] The behavior you expect to see, and the actual behavior\r\n\r\n#### Steps to Reproduce\r\n-------------------------------------------\r\n1. …\r\n2. …\r\n3. …\r\n\r\n#### What happens?\r\n-------------\r\nNot included\r\n\r\n#### What were you expecting to happen?\r\n----------------------------------\r\nInclude usage of canary tokens as a recommended mitigation strategy for prompt injection. Canary tokens can be used to detect system prompt leakage and to verify system prompt effectiveness / adherence.\r\n\r\n##### Proposed language\r\n--------------------------\r\n```\r\n6. Dynamically add a synthetic canary token to the model's system prompt and scan the models output for the canary token. If the token is leaked in the generation output this is a strong indicator for prompt injection that aims to dump the system prompt itself.\r\n7. Dynamically add a synthetic canary token to the model's system prompt and specifically instruct the model to include this token (must not be the same token as in 6.) at the end of every response. Check if the token is included in the models output message and remove the token before sending the response to the front-end component. If the token is not present, the model's system prompt was most likely overridden through means of prompt injection.\r\n```\r\n\r\n#### Any logs, error output, etc?\r\n----------------------------\r\n\r\n\r\n#### Any other comments?\r\n-------------------\r\n…\r\n\r\n- [x] Slack post link (if relevant)\r\nhttps://owasp.slack.com/archives/C05FHTB7QH0/p1712913754686959",
+ "summary": "The issue suggests adding canary tokens to the Prevention and Mitigation Strategies for prompt injection attacks. The proposal includes two main strategies: dynamically adding a synthetic canary token to the model's system prompt to detect prompt leakage, and instructing the model to include a different token in every response to verify the integrity of the system prompt. If the tokens are leaked or absent, it indicates potential prompt injection. To address this, the implementation of these canary tokens as a recommended mitigation strategy should be explored.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/issues/288",
@@ -1608,6 +1664,7 @@
"node_id": "I_kwDOJjxT186FnBJr",
"title": "Drive standard spelling from Terminology glossary",
"body": "Applicable to https://llmtop10.com/ and **Security & Governance Checklist** (from [here](https://owasp.org/www-project-top-10-for-large-language-model-applications/llm-top-10-governance-doc/LLM_AI_Security_and_Governance_Checklist-v1.1.pdf)).\r\n\r\nKey terms are not expressed consistently. Suggestion to establish a canonical spelling. Consider that first use might be different than subsequent use (for example, perhaps might want \"Large Language Model (LLM)\" for first use then \"LLM\" for subsequent use in varying scopes). \r\n\r\n**From the Top 10 website:**\r\n\r\n\"LLM\" variants include at least the following:\r\n1. Large Language Model in LLM01 and LLM04\r\n2. LLM in LLM02 and LLM10\r\n3. LLM's (for plural, with apostrophe) in LLM06 \r\n4. LLMs (for plural, no apostrophe) in LLM09\r\n \r\n**From the Security & Governance Checklist:**\r\n\r\n\"LLM\" variants include at least the following:\r\n1. Large Language Models (LLMs)\r\n2. LLMs (Large Language Models)\r\n3. LLM\r\n4. large language model\r\n6. Large Language Model\r\n\r\nThe varied use of LLM as a term is but one example. Non-exhaustive additional examples include: \r\n1. GenAI vs generative artificial intelligence (GenAI) vs generative AI\r\n3. AIML vs AI/ML\r\n\r\n~The terminology section could establish a preferred style. This need not preclude reestablishing terminology in context as makes sense, but suggesting it simply guide the preferred style among the many possibilities.~ Per comment from @GangGreenTemperTatum, there is already a [terminology section](https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/wiki/Definitions) on the wiki. So the remaining point is that terms are expressed inconsistently.",
+ "summary": "The issue highlights the inconsistency in the usage of key terms related to \"Large Language Models\" (LLM) across the LLM Top 10 website and the Security & Governance Checklist. It suggests establishing a canonical spelling for these terms, noting that the first use may differ from subsequent mentions (e.g., \"Large Language Model (LLM)\" initially, followed by \"LLM\"). Specific examples of inconsistencies are provided, including variations in spelling and phrasing for \"LLM\" and related terms like GenAI and AIML. The suggestion is to create a preferred style guide within the existing terminology section to ensure consistency in term usage. It is recommended that a review of the terminology section be conducted to standardize expressions across the documentation.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/issues/291",
@@ -1637,6 +1694,7 @@
"node_id": "I_kwDOJjxT186FoHkV",
"title": "ads test from https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/pull/292",
"body": "ads test from https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/pull/292",
+ "summary": "The issue discusses the implementation of an ads test related to a previous pull request. It appears that there may be a need for clarification or further development of the test to ensure it aligns with the project's objectives. To address this, reviewing the relevant pull request and evaluating the current testing framework could be beneficial.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/issues/293",
@@ -1665,6 +1723,7 @@
"node_id": "I_kwDOJjxT186IrVLb",
"title": "Translation Effort - Language-specific links to other OWASP resources",
"body": "While translating the LLM Top 10 into German, @johannhartmann and I noticed that there are a few links embedded within the text (not just the references).\r\n\r\nFor example, LLM05 links to [A06:2021 - Vulnerable and Outdated Components](https://owasp.org/Top10/A06_2021-Vulnerable_and_Outdated_Components/). It also links to https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/blob/main/1_0_vulns/Training_Data_Poisoning.md. Furthermore, LLM06 links to the wiki at https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/wiki/Definitions.\r\n\r\nThis raises two questions:\r\n\r\n- Should links to other OWASP documents point to their English originals or to the translations (where available)?\r\n- Are there canonical links, or do we just point to the latest/current version? As some of them contain versions, this is a bit unclear.\r\n\r\nI couldn't find any guidance in the documentation regarding a common approach. It seems like the Spanish translation has kept the links as they are in the English version.\r\n\r\nHow should we proceed so that it is consistent across all translations? \r\n\r\n(Original post in [Slack](https://owasp.slack.com/archives/C05956H7R8R/p1715608819205709))",
+ "summary": "The issue highlights the need for clarity regarding the handling of links to other OWASP resources while translating the LLM Top 10 into German. It raises two main questions: whether links should point to the original English documents or available translations, and whether to use canonical links or the latest version of the documents. There’s a lack of guidance in the documentation on this matter, and the Spanish translation has maintained the original English links. A consistent approach across all translations is necessary. \n\nTo resolve this, a decision needs to be made on the linking strategy for translations.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/issues/310",
@@ -1679,8 +1738,8 @@
"author": 32,
"repository": 151,
"assignees": [
- 22,
- 26
+ 26,
+ 22
],
"labels": []
}
@@ -1690,10 +1749,11 @@
"pk": 60,
"fields": {
"nest_created_at": "2024-09-11T19:17:10.526Z",
- "nest_updated_at": "2024-09-11T19:17:10.526Z",
+ "nest_updated_at": "2024-09-13T15:00:46.205Z",
"node_id": "I_kwDOJjxT186QMuv1",
"title": "cannot access editable source of v2 diagram - www-project-top-10-for-large-language-model-applications/2_0_vulns/artifacts /v2.0 - OWASP Top 10 for LLM Applications and Generative AI - LLM Application HLD - Presentation DLD.jpeg",
"body": "Remember, an issue is not the place to ask questions. You can use our [Slack channel](https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/wiki) for that, or you may want to consult the following Slack channels:\r\n\r\n- [#project-top10-for-llm](https://owasp.slack.com/archives/C05956H7R8R)\r\n- [#team-llm-web](https://owasp.slack.com/archives/C06RXVCQB1C)\r\n- [#team-llm_diagrams_and_visuals](https://owasp.slack.com/archives/C05L7TW8VCY)\r\n- Each vulnerabilities has its own dedicated Slack channel for discussions in the format of `#team-llm0X`, I.E ([#team-llm03_data_and_model_poisoning](https://owasp.slack.com/archives/C05F7JWFYBU))\r\n\r\n### When reporting an issue, please be sure to include the following:\r\n- [ ] Before you open an issue, please check if a similar issue already exists or has been closed before.\r\n- [ ] A descriptive title and apply the specific **LLM-0-10** label relative to the entry. See our [available labels](https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/labels).\r\n- [ ] A description of the problem you're trying to solve, including *why* you think this is a problem\r\n- [ ] If the enhancement changes current behavior, reasons why your solution is better\r\n- [ ] What artifact and version of the project you're referencing, and the location (I.E OWASP site, llmtop10.com, repo)\r\n- [ ] The behavior you expect to see, and the actual behavior\r\n\r\n#### Steps to Reproduce\r\n-------------------------------------------\r\n1. …\r\n2. …\r\n3. …\r\n\r\n#### What happens?\r\n-------------\r\n…\r\n\r\n#### What were you expecting to happen?\r\n----------------------------------\r\n…\r\n\r\n#### Any logs, error output, etc?\r\n----------------------------\r\n\r\n\r\n#### Any other comments?\r\n-------------------\r\n…\r\n\r\n- [ ] Slack post link (if relevant)\r\n\r\n#### What versions of hardware and software are you using?\r\n----------------------------------------\r\n**Operating System:** …\r\n**Browser:** …\r\n\r\n- [ ] Chrome\r\n- [ ] Firefox\r\n- [ ] Edge\r\n- [ ] Safari 11\r\n- [ ] Safari 10\r\n- [ ] IE 11\r\n",
+ "summary": "The issue reports an inability to access the editable source of a specific diagram related to the OWASP Top 10 for LLM Applications. The user is directed to use Slack channels for questions and to follow specific guidelines for reporting issues, including ensuring similar issues haven't been previously addressed, providing descriptive titles and labels, and detailing the problem, expected behavior, and any relevant logs.\n\nTo address this issue, it may be necessary to check the accessibility of the diagram's source or provide clearer instructions on how to access the editable version.",
"state": "open",
"state_reason": "reopened",
"url": "https://github.com/OWASP/www-project-top-10-for-large-language-model-applications/issues/388",
@@ -1718,10 +1778,11 @@
"pk": 61,
"fields": {
"nest_created_at": "2024-09-11T19:17:38.472Z",
- "nest_updated_at": "2024-09-11T19:17:38.472Z",
+ "nest_updated_at": "2024-09-13T15:01:08.504Z",
"node_id": "I_kwDOJZbJH86L0MUq",
"title": "ERROR: No matching distribution found for pywin==306",
"body": "Hello,\r\n\r\nI had an error while installing requirements.txt, see the screenshot below:\r\n\r\n\r\n\r\nhow can I solve it?",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/SAPKiln/issues/1",
@@ -1744,10 +1805,11 @@
"pk": 62,
"fields": {
"nest_created_at": "2024-09-11T19:17:45.802Z",
- "nest_updated_at": "2024-09-11T19:17:45.802Z",
+ "nest_updated_at": "2024-09-13T15:01:14.786Z",
"node_id": "I_kwDOJZXuOs6Bythd",
"title": "Please add a description to this project",
"body": "",
+ "summary": "The issue requests the addition of a project description to provide clarity and context. It suggests that including a detailed description will improve understanding for users and contributors. To address this, a clear and concise project description should be drafted and added to the repository.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-blockchain-appsec-standard/issues/1",
@@ -1770,10 +1832,11 @@
"pk": 63,
"fields": {
"nest_created_at": "2024-09-11T19:18:21.161Z",
- "nest_updated_at": "2024-09-11T19:18:21.161Z",
+ "nest_updated_at": "2024-09-13T15:01:39.679Z",
"node_id": "I_kwDOJTHMcM6Bytfk",
"title": "Please add a description to this project",
"body": "",
+ "summary": "The issue requests the addition of a project description. To address this, it would be helpful to draft a clear and concise overview of the project's purpose, features, and goals.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-minefield/issues/2",
@@ -1796,10 +1859,11 @@
"pk": 64,
"fields": {
"nest_created_at": "2024-09-11T19:18:41.667Z",
- "nest_updated_at": "2024-09-11T19:18:41.667Z",
+ "nest_updated_at": "2024-09-13T15:01:56.557Z",
"node_id": "I_kwDOJNCEE86Byta2",
"title": "Please add a description to this project",
"body": "",
+ "summary": "A request has been made to include a project description. It would be helpful to provide a clear and concise overview of the project's purpose, features, and functionality.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-cognito-catastrophe/issues/2",
@@ -1826,6 +1890,7 @@
"node_id": "I_kwDOJJEsKM5qX4p_",
"title": "Support VSCode editor",
"body": "### Plugin release for VSCode\r\nIDE-Vulscanner is projected to be ide agonistic and supports the IntelliJ plugin for now. Given developers mostly use VSCode and IntelliJ ide's for day jobs it would be a good idea to release a VSCode plugin. ",
+ "summary": "The issue suggests developing a VSCode plugin for IDE-Vulscanner, which currently only supports IntelliJ. Since many developers primarily use VSCode, creating a plugin for this editor would enhance its usability. The next steps involve assessing the requirements and implementing a VSCode-compatible version of the plugin.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-ide-vulscanner/issues/17",
@@ -1857,6 +1922,7 @@
"node_id": "I_kwDOJJEsKM5qX4-F",
"title": "Support multi language scan",
"body": "### Enhance the plugin for multi-language scan \r\nAs of now plugin supports maven-based project scans and the ask is to support well-known program languages like Go, Python, Java, Gradle-based projects, etc.",
+ "summary": "The issue requests an enhancement of the plugin to enable multi-language scanning capabilities. Currently, the plugin only supports Maven-based projects, and the proposal is to extend support to include popular programming languages such as Go, Python, Java, and Gradle-based projects. To address this, the development of additional scanning features for these languages is needed.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-ide-vulscanner/issues/18",
@@ -1888,6 +1954,7 @@
"node_id": "I_kwDOJJEsKM5qX5On",
"title": "Support vulnerable code linting",
"body": "### Vul code linting\r\nThe bigger picture of this plugin is to support compile time linting of any code which might cause vulnerability to overall software.",
+ "summary": "The issue discusses the need for a plugin that enables compile-time linting to identify potential vulnerabilities in code. The goal is to enhance software security by catching issues early in the development process. To address this, the plugin should be developed to effectively analyze code for vulnerabilities during compilation.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-ide-vulscanner/issues/19",
@@ -1915,10 +1982,11 @@
"pk": 68,
"fields": {
"nest_created_at": "2024-09-11T19:18:58.756Z",
- "nest_updated_at": "2024-09-11T19:18:58.756Z",
+ "nest_updated_at": "2024-09-13T15:02:05.333Z",
"node_id": "I_kwDOJJEsKM6Gx0SR",
"title": "Add IntelliJ 2024.1 support",
"body": "I would really love to also use the plugin in IntelliJ 2024.1\r\n\r\nCurrently it's not listed as available for this version. Haven't tried installing it manually tho.",
+ "summary": "The issue requests support for the plugin in IntelliJ 2024.1, as it is currently not listed as compatible with that version. It might be beneficial to test whether manual installation works, but official support would be ideal. Consider updating the plugin to ensure compatibility with IntelliJ 2024.1.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-ide-vulscanner/issues/24",
@@ -1941,10 +2009,11 @@
"pk": 69,
"fields": {
"nest_created_at": "2024-09-11T19:19:15.530Z",
- "nest_updated_at": "2024-09-11T19:19:15.530Z",
+ "nest_updated_at": "2024-09-13T15:02:19.017Z",
"node_id": "I_kwDOI9Ahm85r2IXl",
"title": "Please consider Oracle manipulation",
"body": "Hi there,\r\n\r\nThanks for this great repo. After reading the top 10 list, IMHO, please consider to add Oracle manipulation into this (some hacks happened).\r\n\r\nAbout Oracle manipulation:\r\n\r\n# Oracle manipulation\r\n## Desciption\r\nOracle manipulation is an attack that smart contract rely off-chain information on other services called oracles (eg. price). If the data was wrong it might lead to abnormal behavior.\r\n\r\n## Impact\r\nMight drain the pool from Defi protocol.\r\n\r\n## Steps to fix\r\nUse a decentralized oracle network, or time-weighted average price feed.\r\n\r\n## Example\r\nThe lending contract use the price from dex oracle, attacker make flash loan and manipulate the token price (drain one asset from the pool). After price manipulation, attacker can make loan from lending pool if the token price source is dex oracle and not validated properly.",
+ "summary": "The issue discusses the need to address Oracle manipulation in the repository. It highlights that this type of attack can exploit vulnerabilities in smart contracts that rely on off-chain data from oracles, potentially leading to significant losses in DeFi protocols. To mitigate this risk, it suggests implementing a decentralized oracle network or utilizing time-weighted average price feeds. A specific example is provided, illustrating how an attacker could exploit a lending contract by manipulating token prices through a decentralized exchange oracle. It is recommended to consider adding protections against such manipulation.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-smart-contract-top-10/issues/4",
@@ -1973,6 +2042,7 @@
"node_id": "I_kwDOI1zPn85hrZIL",
"title": "Have a vulnerable AI project :-)?",
"body": "Hi there!\r\nThis is a great project! Thank you for documenting it so nicely!\r\nTo make it more practical for those that might have trouble understanding some of the sources referenced: will there ever be a “vulnerable AI” project where we can make some of the attacks described more practical :-)?",
+ "summary": "The issue expresses appreciation for the project and its documentation while suggesting the creation of a \"vulnerable AI\" project. This would allow users to practically engage with the attacks described in the existing documentation. To address this, the team could consider developing a dedicated project focused on showcasing these vulnerabilities in a hands-on manner.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-ai-security-and-privacy-guide/issues/6",
@@ -1999,6 +2069,7 @@
"node_id": "I_kwDOI1zPn85pBZOf",
"title": "Process podcast to see if anything new",
"body": "https://www.devseccon.com/the-secure-developer-podcast/ep-134-the-five-pillars-of-mlsecops-with-ian-swanson",
+ "summary": "The issue discusses the need to process a specific podcast episode to extract any new insights or relevant information. It suggests reviewing the content of the episode titled \"The Five Pillars of MLSecOps\" to identify key takeaways that may be beneficial for the project. To address this, one should listen to the episode and summarize the main points or actionable ideas presented.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-ai-security-and-privacy-guide/issues/10",
@@ -2025,6 +2096,7 @@
"node_id": "I_kwDOI1zPn85pBZRG",
"title": "Process article by Susanna Cox on ML ops controls",
"body": "",
+ "summary": "The issue discusses the need to process an article on ML ops controls. It highlights the importance of implementing proper controls in machine learning operations to ensure efficiency and compliance. To move forward, the article should be reviewed and analyzed for key insights that can be incorporated into the existing framework or practices.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-ai-security-and-privacy-guide/issues/11",
@@ -2051,6 +2123,7 @@
"node_id": "I_kwDOI1zPn85pDIJy",
"title": "Process ENISA presentations of AI security conference June 2023",
"body": "eg 2.2-isabel-praca-enisa_aicybersecurityconference_v2",
+ "summary": "The issue pertains to the need for processing presentations from the ENISA AI Security Conference held in June 2023. Specifically, it references a particular presentation file (2.2-isabel-praca-enisa_aicybersecurityconference_v2) that requires attention. The next steps involve reviewing and possibly summarizing or extracting key insights from the mentioned presentation as part of the overall processing effort.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-ai-security-and-privacy-guide/issues/12",
@@ -2077,6 +2150,7 @@
"node_id": "I_kwDOI1zPn85rBCXC",
"title": "Do gap analysis with responsible AI guide",
"body": "https://www.considerati.com/static/default/files/Responsible%20AI%20guide.pdf",
+ "summary": "The issue discusses the need to conduct a gap analysis using the Responsible AI guide linked in the description. The goal is to identify discrepancies between current practices and the standards outlined in the guide. To address this, a detailed assessment of existing AI practices against the guidelines provided in the document should be performed. This will help in improving adherence to responsible AI principles.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-ai-security-and-privacy-guide/issues/14",
@@ -2099,10 +2173,11 @@
"pk": 75,
"fields": {
"nest_created_at": "2024-09-11T19:19:53.240Z",
- "nest_updated_at": "2024-09-11T19:19:53.240Z",
+ "nest_updated_at": "2024-09-13T03:39:12.472Z",
"node_id": "I_kwDOI1zPn858tKig",
"title": "Controls in table format",
"body": "Do we have risks and controls mapping described in table format? ",
+ "summary": "The issue inquires about the existence of a mapping of risks and controls presented in a table format. It suggests that such a format would be beneficial for clarity and organization. To address this, it may be necessary to create or find a detailed table that outlines the risks alongside their corresponding controls.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-ai-security-and-privacy-guide/issues/35",
@@ -2125,10 +2200,11 @@
"pk": 76,
"fields": {
"nest_created_at": "2024-09-11T19:20:48.056Z",
- "nest_updated_at": "2024-09-11T22:50:23.514Z",
+ "nest_updated_at": "2024-09-13T17:08:17.896Z",
"node_id": "I_kwDOIhSPhM5YsL-r",
"title": "Getting specific containers that fail the checker rule.",
"body": "If any container violates the checker rule, the culprit must be busted. So, better to create a ContainerCheck Flow that will help identify the culprit. \r\n\r\nSteps \r\n1. Creating a Container Check Class\r\n2. Passing the Parent Workload via TinyDB Query Lambdas\r\n3. Collect all the Parent Workloads violation and child containers in a Static Variable or Otherwise. \r\n4. Ignore the TinyDB result and prioritize the ContainerCheck Class output",
+ "summary": "The issue discusses the need for a system to identify containers that violate a specific checker rule. The proposed solution involves creating a ContainerCheck Flow to pinpoint these violations. Key steps include developing a Container Check Class, utilizing TinyDB Query Lambdas to pass the Parent Workload, and collecting violations of Parent Workloads along with their child containers in a static variable. Additionally, it suggests that the output from the ContainerCheck Class should take precedence over the TinyDB results. Implementation of these steps is necessary to enhance the detection of rule violations.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/KubeLight/issues/2",
@@ -2158,6 +2234,7 @@
"node_id": "I_kwDOIhSPhM5YsMce",
"title": "Read Me improvement ",
"body": "1. Adding CI badges\r\n2. Adding Sqube Badge - CodeQuality and Security\r\n3. Adding Sponsor badge after permission. \r\n4. Add Documentation link [docs.kubelight.com](docs.kubelight.com)",
+ "summary": "The issue suggests several improvements for the README file, including the addition of CI badges, a Sqube badge for code quality and security, a sponsor badge pending permission, and a documentation link to docs.kubelight.com. To address this, the specified badges and links should be integrated into the README as outlined.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/KubeLight/issues/3",
@@ -2186,6 +2263,7 @@
"node_id": "I_kwDOIhSPhM5YtOYI",
"title": "Benchmark Module for Kubelight",
"body": "### Description \r\nKubelight requires a new benchmark module that helps to run various benchmarks on cluster configuration.\r\n\r\n#### To Do \r\n- [ ] Create a List of possible benchmarking rules\r\n\r\n\r\n\r\n",
+ "summary": "The issue discusses the need for a new benchmark module for Kubelight to facilitate running various benchmarks on cluster configurations. The proposed task involves creating a list of possible benchmarking rules to guide the development of this module.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/KubeLight/issues/4",
@@ -2214,6 +2292,7 @@
"node_id": "I_kwDOIhSPhM5YydY1",
"title": "Kubernetes & Container Security",
"body": "",
+ "summary": "The issue discusses concerns related to security in Kubernetes and container environments. It highlights potential vulnerabilities and the need for better security practices to protect applications running in these settings. Suggested actions may include implementing stricter access controls, regular security audits, and adopting best practices for container image management. Addressing these points can enhance the overall security posture of Kubernetes deployments.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/KubeLight/issues/5",
@@ -2240,6 +2319,7 @@
"node_id": "I_kwDOIhSPhM5Y0ZR0",
"title": "Exempting rules from default namespaces",
"body": "Exempt to run rules in default namespaces \r\nex - default, kube-system. ",
+ "summary": "The issue discusses the need to exempt certain rules from being applied in default namespaces, specifically the \"default\" and \"kube-system\" namespaces. The proposed solution involves implementing a mechanism that allows these namespaces to bypass the standard rule application. To address this, the implementation of exclusion criteria for these specific namespaces should be considered.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/KubeLight/issues/6",
@@ -2266,6 +2346,7 @@
"node_id": "I_kwDOIhSPhM5Y0nti",
"title": "Decorator for exception handling in Checker",
"body": "1. Add exception handling to all the methods of Container Checker\r\n2. Add exception handling so that flow will not be disrupted because of one issue. \r\n3. Global exception is not recommended, still over the remove it, add comment for the same. ",
+ "summary": "The issue discusses the need to enhance the Container Checker by implementing exception handling across all its methods. The goal is to ensure that the overall flow remains intact even if a single method encounters an error. It also mentions that while global exception handling is not preferred, it should be removed and accompanied by a comment explaining the rationale. \n\nTo address this, the implementation of individual exception handling for each method is required, along with documentation regarding the decision against global exception handling.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/KubeLight/issues/7",
@@ -2297,6 +2378,7 @@
"node_id": "I_kwDOIhSPhM5Y5s4p",
"title": "Memory and CPU Requests/Limit Check to be user input",
"body": "As of now, we only check if the memory or CPU limit is set or not, better would be \r\n1. To take user input to validate the memory and CPU limits.\r\n2. Translate \"M\" and \"m\" for CPU and G, Gi, Mi, and M for RAM. Better to use 1000 conversion units for both.",
+ "summary": "The issue proposes enhancements to the current memory and CPU limit checks. Specifically, it suggests allowing user input for validating these limits and improving the unit translation process. The proposal includes using consistent conversion units: \"M\" and \"m\" for CPU, and \"G\", \"Gi\", \"Mi\", and \"M\" for RAM, with a preference for 1000 as the conversion standard. Implementation of these changes could improve usability and clarity.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/KubeLight/issues/8",
@@ -2321,10 +2403,11 @@
"pk": 83,
"fields": {
"nest_created_at": "2024-09-11T19:21:03.949Z",
- "nest_updated_at": "2024-09-11T19:21:03.949Z",
+ "nest_updated_at": "2024-09-13T15:03:32.572Z",
"node_id": "I_kwDOIbsMfc6BytVG",
"title": "Please add a description to this project",
"body": "",
+ "summary": "The issue requests the addition of a project description. To address this, a concise and informative description outlining the project's purpose, features, and usage should be created and included.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-application-security-monitoring-standard/issues/1",
@@ -2347,10 +2430,11 @@
"pk": 84,
"fields": {
"nest_created_at": "2024-09-11T19:21:27.404Z",
- "nest_updated_at": "2024-09-11T19:21:27.404Z",
+ "nest_updated_at": "2024-09-13T15:03:51.410Z",
"node_id": "I_kwDOITh-Lc6FWu_t",
"title": "Associated Github Repo no longer exists",
"body": "https://github.com/anil-yelken/Vulnerable-Flask-App\r\n\r\nNo longer exists.",
+ "summary": "The GitHub issue reports that the repository for the \"Vulnerable Flask App\" is no longer available. It may be necessary to either find an alternative repository for similar content or restore the original repository if possible.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-vulnerable-flask-app/issues/1",
@@ -2377,6 +2461,7 @@
"node_id": "I_kwDOH7x8X85TQF_x",
"title": "Create documentation",
"body": "- [x] Depends on https://github.com/commjoen/wrongsecrets-ctf-party/issues/86 and on https://github.com/commjoen/wrongsecrets/issues/453 \r\n\r\nTodos:\r\n- [ ] have github pages in place\r\n- [ ] have helm release process in place\r\n- [ ] Have supportive Docker release scripts in place (both tagged&latest)\r\n- [ ] cleanup the guides to be limited on what we actually have (k8s, Azure, GCP & AWS)\r\n- [ ] document the details required to run K8s\r\n- [ ] document the details required to run AWS\r\n- [ ] document the details required to run GCP\r\n- [ ] document the details required to run Azure\r\n- [ ] document CTFD setup (Automated and manual!)\r\n",
+ "summary": "Create comprehensive documentation for the project. Follow these steps:\n\n1. Ensure that dependencies from the linked issues are resolved.\n2. Set up GitHub Pages for hosting the documentation.\n3. Establish a Helm release process to manage deployments effectively.\n4. Create Docker release scripts for both tagged and latest images to streamline container management.\n5. Clean up existing guides to focus on supported platforms: Kubernetes (K8s), Azure, Google Cloud Platform (GCP), and Amazon Web Services (AWS).\n6. Document specific configurations and requirements for running Kubernetes.\n7. Provide detailed instructions for setting up AWS.\n8. Document GCP setup requirements.\n9. Outline Azure setup instructions.\n10. Include a section on setting up CTFD, covering both automated and manual processes.\n\nBegin by creating a structure for the documentation and outlining the information needed for each platform. Then, tackle the GitHub Pages setup and Helm processes.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/wrongsecrets-ctf-party/issues/79",
@@ -2407,6 +2492,7 @@
"node_id": "I_kwDOH7x8X85hW_Qg",
"title": "Replace Request with something better maintained",
"body": "Given 2 Security alerts regarding the NPM package `Request` we need to change it to something else.\r\nSteps to take: \r\n- [ ] Wait for kubernetes-client to be ready (See below for tracking)\r\n- [ ] migrate to the latest version of the kubernetes-client (this will include giving typed requests/responses to the k8s API)\r\n- [ ] see if we have Request anywhere else and remove it from there (start by uninstalling it ;-))",
+ "summary": "Replace the `Request` NPM package with a better-maintained alternative due to two security alerts. Follow these steps:\n\n1. **Monitor Kubernetes Client Development**:\n - Track the readiness of the `kubernetes-client` library to ensure compatibility and support.\n\n2. **Migrate to Latest Version**:\n - Update the project to utilize the latest version of the `kubernetes-client`.\n - Implement typed requests and responses for the Kubernetes API to enhance type safety and reduce errors.\n\n3. **Audit and Remove Request Usage**:\n - Search the codebase for any other instances of the `Request` package.\n - Uninstall the package from the project once identified and ensure all calls to it are replaced with the new alternative.\n\nStart by checking the current version of `kubernetes-client` and its release notes for breaking changes or migration guides. After that, proceed with the migration and code adjustments.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/wrongsecrets-ctf-party/issues/212",
@@ -2435,6 +2521,7 @@
"node_id": "I_kwDOH7x8X85mb_ev",
"title": "Embed vault server in safe container",
"body": "We want to open the vault challenges to the ctf by adding a vault dev server to the teamnamespace by means of javascript. This requires:\r\n- [ ] vault container with deployment and service\r\n- [ ] Rbac extension for player to describe and read logs of pods/deployment\r\n- [ ] Nsp extension to make vault, desktop, and WrongSecrets talk to eachbother in same namespace\r\n- [ ] extend balancer to proxy to vault\r\n- [ ] Add initialisation JavaScript to give vault the right policies and contents\r\n- [ ] Extend tests\r\n- [ ] have a PVC for just vault isolated for each NS",
+ "summary": "Embed a Vault server in a safe container for the CTF. Follow these steps:\n\n1. **Create Vault Container**: Develop a deployment and service configuration for the Vault container within the team namespace. Ensure it adheres to best practices for container security.\n\n2. **Implement RBAC Extension**: Establish Role-Based Access Control (RBAC) permissions for players. This should allow them to describe and read logs of the pods and deployments related to the Vault server.\n\n3. **Develop NSP Extension**: Create a Network Service Proxy (NSP) extension that enables the Vault, desktop, and WrongSecrets applications to communicate within the same namespace effectively.\n\n4. **Extend Load Balancer**: Modify the existing load balancer to include functionality for proxying requests to the Vault server, ensuring it can handle traffic appropriately.\n\n5. **Initialize Vault with JavaScript**: Write initialization JavaScript that configures the Vault with necessary policies and preloads required contents for the CTF environment.\n\n6. **Enhance Testing**: Expand the testing framework to include tests specifically for the Vault server integration, ensuring all components function correctly together.\n\n7. **Set Up Persistent Volume Claim (PVC)**: Create a Persistent Volume Claim dedicated to the Vault, ensuring it is isolated for each namespace to maintain data integrity and security.\n\n**First Steps**:\n- Start by designing the deployment and service for the Vault container.\n- Set up the RBAC permissions structure.\n- Begin drafting the JavaScript initialization script to configure Vault correctly.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/wrongsecrets-ctf-party/issues/250",
@@ -2463,6 +2550,7 @@
"node_id": "I_kwDOH7x8X85m7_X3",
"title": "Create proxy and websocket configuration configmap for wrongsecrets-balancer",
"body": "Given we will have to upgrade the webtop often, we need to be able to easily adjust the requierd proxying in the wrongsecrets-balancer. That is why we need a configmap that contains a mapping for:\r\n- which urls should end up to a websocket upgrade to which service\r\n- which urls need to be proxied to which address\r\n\r\nso, something like:\r\n- websocket\r\n - servicename1:8080:\r\n incomingurl1\r\n incomingurl2\r\n incomingurl3\r\n - serviname2:3000\r\n incomingurl4\r\n incomingurl5\r\n - proxy\r\n - servicename1:8080:\r\n incomingurl6\r\n incomingurl7\r\n - servicename2:3000\r\n incomingurl8withwildcard\r\n - servicename3(CTFD)\r\n incomingurl9\r\n incomingurl10\r\n - servicename4(grafana)\r\n incomingurl10\r\n\r\nthe deployment should mount the configmap in so that the balancer its code should be able to read it. both the deployment and balancer code need to be adjusted.\r\nhttps://github.com/OWASP/wrongsecrets-ctf-party/blob/main/wrongsecrets-balancer/src/proxy/proxy.js#L106 should no longer be hardcoded but usse a configmap to load like above",
+ "summary": "Create a ConfigMap for the wrongsecrets-balancer to facilitate dynamic proxying and WebSocket upgrades. Define a structure in the ConfigMap that maps URLs to services for both WebSocket and proxy configurations.\n\n1. Define the ConfigMap structure:\n - Create entries for WebSocket upgrades:\n - Map incoming URLs to service names and ports.\n - Create entries for proxying:\n - Map incoming URLs to service names and ports, including wildcard support.\n\nExample structure:\n```yaml\napiVersion: v1\nkind: ConfigMap\nmetadata:\n name: wrongsecrets-balancer-config\ndata:\n websocket: |\n servicename1:8080:\n - incomingurl1\n - incomingurl2\n - incomingurl3\n servicename2:3000:\n - incomingurl4\n - incomingurl5\n proxy: |\n servicename1:8080:\n - incomingurl6\n - incomingurl7\n servicename2:3000:\n - incomingurl8withwildcard\n servicename3(CTFD):\n - incomingurl9\n - incomingurl10\n servicename4(grafana):\n - incomingurl10\n```\n\n2. Adjust the deployment:\n - Mount the ConfigMap into the deployment so the balancer can access it.\n - Ensure the correct file path is used for the balancer code to read the configuration.\n\n3. Update the balancer code:\n - Refactor the hardcoded logic in `proxy.js` to read from the mounted ConfigMap instead of using hardcoded values.\n\n4. Test the implementation:\n - Verify that the application correctly reads the ConfigMap and routes requests as defined.\n\nBy following these steps, ensure seamless upgrades and modifications to the proxying behavior without changing the codebase each time.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/wrongsecrets-ctf-party/issues/252",
@@ -2491,6 +2579,7 @@
"node_id": "I_kwDOH7x8X85qXfmQ",
"title": "Create cypres test for full round trip (webtop &wrongsecrets)",
"body": "We need to build cypress tests that check based on a Minikube env whether:\r\n- [ ] creating a team works: you can register a team, and then end up with having 2 buttons, that you can click to see the webtop and the wrongsecrets instance\r\n- [ ] joining a team works: you can join the same team as in the previous test again by giving the passscode and that end up with having 2 buttons, that you can click to see the webtop and the wrongsecrets instance\r\n- [ ] admin interface works",
+ "summary": "Create Cypress tests for full round trip in Minikube environment. Focus on the following functionalities:\n\n1. **Test Team Creation**:\n - Simulate team registration.\n - Verify that two buttons appear to access the webtop and wrongsecrets instances.\n\n2. **Test Team Joining**:\n - Join the previously created team using the passcode.\n - Ensure that the same two buttons for webtop and wrongsecrets instances are visible.\n\n3. **Test Admin Interface**:\n - Validate that the admin interface is functional and accessible.\n\n**First Steps**:\n- Set up a Minikube environment and ensure Cypress is installed.\n- Write test cases using Cypress commands to automate the above scenarios.\n- Use Cypress commands like `cy.visit()` to navigate to the necessary pages and `cy.get()` to interact with buttons.\n- Implement assertions to check for the existence of buttons and validate correct behavior of the admin interface.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/wrongsecrets-ctf-party/issues/284",
@@ -2521,6 +2610,7 @@
"node_id": "I_kwDOH7x8X85z20rW",
"title": "Upgrade to latest webtop ",
"body": "Given that everything changed in the new KasmVNC setup for the latest Linux [webtop](https://docs.linuxserver.io/images/docker-webtop), we need to upgrade the balancer again.\r\nTasks in this ticket:\r\n- [ ] pin to a very recent version of the webtop\r\n- [ ] update the balancer to be compatible with the webtop",
+ "summary": "Upgrade to the latest webtop version. \n\n1. Pin the project to the most recent version of the webtop as specified in the [LinuxServer documentation](https://docs.linuxserver.io/images/docker-webtop).\n2. Review the changes in the new KasmVNC setup to understand the differences from the previous version.\n3. Update the load balancer configuration to ensure compatibility with the new webtop features and requirements.\n4. Test the integration of the updated balancer with the webtop to verify functionality.\n5. Document any changes made during the upgrade process for future reference.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/wrongsecrets-ctf-party/issues/371",
@@ -2545,10 +2635,11 @@
"pk": 91,
"fields": {
"nest_created_at": "2024-09-11T19:22:13.123Z",
- "nest_updated_at": "2024-09-11T22:53:47.233Z",
+ "nest_updated_at": "2024-09-13T17:07:25.631Z",
"node_id": "I_kwDOH7x8X86SdOo1",
"title": "Upgrade to the latest wrongsecrets (1.9.1)",
"body": "- [x] update k8s to 1.30: https://github.com/OWASP/wrongsecrets-ctf-party/pull/666\r\n- [x] update all deps\r\n- [ ] enable the new challenge in javascript (e.g. add sealed-secrets items) and upgrade to 1.9.1 for wrongsecrets image\r\n - [ ] make 1.9.x where challenge 48 is enabled in CTF mode and update vault challenge CTF settings and vault-challenges can be temporary disabled via env-vars.\r\n - [ ] create javascript for ctf-party with new tests\r\n - [ ] release new balancer image\r\n- [ ] fix https://github.com/OWASP/wrongsecrets-ctf-party/issues/665\r\n- [ ] fix https://github.com/OWASP/wrongsecrets-ctf-party/pull/630\r\n- [ ] fix https://github.com/OWASP/wrongsecrets-ctf-party/pull/642\r\n- [ ] update and fix CTFd everywhere",
+ "summary": "Upgrade wrongsecrets to version 1.9.1. \n\n1. Ensure Kubernetes is updated to version 1.30 as per pull request #666.\n2. Update all dependencies to their latest versions.\n3. Enable the new JavaScript challenge by integrating sealed-secrets items and upgrading to the wrongsecrets image version 1.9.1.\n - Implement challenge 48 in CTF mode in version 1.9.x, modifying vault challenge settings. Allow temporary disabling of vault challenges through environment variables.\n - Develop new JavaScript tests for the CTF party.\n - Release an updated balancer image.\n4. Address and resolve the following issues:\n - Fix issue #665.\n - Resolve pull request #630.\n - Address pull request #642.\n5. Update and fix CTFd across the board.\n\nBegin by updating Kubernetes and dependencies, then proceed to implement the new JavaScript challenge and tackle the listed issues sequentially.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/wrongsecrets-ctf-party/issues/664",
@@ -2578,6 +2669,7 @@
"node_id": "I_kwDOH7x8X86SdPL2",
"title": "Fix bug in admin interface",
"body": "when a namespace is loaded and we want to manage it via the admin env we get various errors in the ui. these need to be fixed.",
+ "summary": "Fix the bug in the admin interface that occurs when loading a namespace for management in the admin environment. Identify the specific errors displayed in the UI during this process. \n\n1. Review the admin interface code, particularly the components responsible for loading and managing namespaces.\n2. Check for any error messages in the console and correlate them with the user interface issues.\n3. Investigate the data flow and state management related to namespace loading; ensure that the correct data is being passed to the UI components.\n4. Implement error handling to provide clearer feedback in the UI.\n5. Test the changes thoroughly in different scenarios to ensure that loading namespaces does not produce errors.\n\nBegin by isolating the error source and replicating the issue in a development environment.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/wrongsecrets-ctf-party/issues/665",
@@ -2602,10 +2694,11 @@
"pk": 93,
"fields": {
"nest_created_at": "2024-09-11T19:23:40.415Z",
- "nest_updated_at": "2024-09-11T19:23:40.415Z",
+ "nest_updated_at": "2024-09-13T15:05:15.591Z",
"node_id": "I_kwDOHpaoic6BytQC",
"title": "Please add a description to this project",
"body": "",
+ "summary": "The issue requests the addition of a project description. To address this, a concise and informative description needs to be created that outlines the project's purpose, features, and any relevant details for potential users or contributors.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-application-security-playbook/issues/1",
@@ -2632,6 +2725,7 @@
"node_id": "I_kwDOHeRoz85gnpT4",
"title": "Implement semgrep for all langs",
"body": "Create a github action that runs the free version of codeQL and Semgrep for all languages.",
+ "summary": "Implement Semgrep integration for all supported programming languages. \n\n1. Create a GitHub Action workflow file in the `.github/workflows` directory.\n2. Define triggers for the workflow, such as `push` and `pull_request`.\n3. Set up a job that runs on the latest version of Ubuntu.\n4. Install Semgrep by adding a step to run `pip install semgrep`.\n5. Configure the CodeQL action by adding `actions/setup-codeql@v1` and specifying the languages to analyze.\n6. Add steps to run Semgrep rules for each language, ensuring to include relevant rules for analysis.\n7. Collect the results from both Semgrep and CodeQL and output them in a usable format, such as JSON or SARIF.\n8. Test the GitHub Action in a repository with sample code to validate the integration.\n\nBy following these steps, ensure that the action runs efficiently and captures any issues across all languages.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/wrongsecrets-binaries/issues/17",
@@ -2660,6 +2754,7 @@
"node_id": "I_kwDOHeRoz85nH_c5",
"title": "Create CTF versions on generation",
"body": "Create CTF versions on compile of the files during github action and quickbuild.sh, these versions of the binaries should have:\r\n- spoiling disabled\r\n- randomize the actual secret",
+ "summary": "Implement CTF versions during the file generation and compilation processes in GitHub Actions and quickbuild.sh. Ensure that these binary versions have the following specifications:\n\n1. Disable spoiling in the compilation settings.\n2. Introduce randomness in the actual secret used within the binaries.\n\n**First Steps:**\n\n1. Modify the GitHub Actions workflow file to include a new job for creating CTF binaries.\n2. Update the quickbuild.sh script to add an option for generating CTF versions during the build process.\n3. Configure compiler flags or options to disable spoiling when compiling the binaries.\n4. Implement a function to randomize the secret used in the binary, ensuring it is unique for each build.\n5. Test the new CTF binaries to verify that spoiling is indeed disabled and that the secrets are randomized as intended.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/wrongsecrets-binaries/issues/23",
@@ -2684,10 +2779,11 @@
"pk": 96,
"fields": {
"nest_created_at": "2024-09-11T19:24:04.083Z",
- "nest_updated_at": "2024-09-11T22:53:40.828Z",
+ "nest_updated_at": "2024-09-13T17:07:19.825Z",
"node_id": "I_kwDOHeRoz85nH_o0",
"title": "Add pre-commit",
"body": "for this we need to setup precommit that works at least on macos/linux/windows.\r\n- [ ] Add pre-commit configuration (docs, language related stuff?)\r\n- [ ] onboard wrongsecrets-binaries to automated pre-commit corrections for c/c++\r\n- [ ] onboard wrongsecrets-binaries to automated pre-commit corrections for Rust\r\n- [ ] onboard wrongsecrets-binaries to automated pre-commit corrections for Golang",
+ "summary": "Implement pre-commit hooks for cross-platform compatibility (macOS, Linux, Windows). \n\n1. Set up a pre-commit configuration file (e.g., `.pre-commit-config.yaml`) in the repository to define hooks and their environments.\n2. Include documentation and relevant language specifications that detail how to use and customize pre-commit hooks.\n3. Integrate automated pre-commit corrections for `wrongsecrets-binaries` in the following languages:\n - C/C++\n - Research existing pre-commit hooks for C/C++.\n - Configure hooks to automatically correct `wrongsecrets-binaries`.\n - Rust\n - Identify Rust-specific pre-commit hooks.\n - Set up necessary hooks for Rust to handle `wrongsecrets-binaries`.\n - Golang\n - Explore Golang pre-commit options.\n - Ensure hooks for Golang also address `wrongsecrets-binaries`.\n\nBegin by creating the `.pre-commit-config.yaml` file and testing it with basic hooks for one language, then expand to others.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/wrongsecrets-binaries/issues/24",
@@ -2712,10 +2808,11 @@
"pk": 97,
"fields": {
"nest_created_at": "2024-09-11T19:24:05.309Z",
- "nest_updated_at": "2024-09-11T22:53:42.074Z",
+ "nest_updated_at": "2024-09-13T17:07:20.900Z",
"node_id": "I_kwDOHeRoz85sFR3D",
"title": "Add notary for MacOS",
"body": "We need to sign the macos binaries:\r\nhttps://github.com/marketplace/actions/code-sign-action ",
+ "summary": "Implement code signing for MacOS binaries. Utilize the GitHub Action available at [code-sign-action](https://github.com/marketplace/actions/code-sign-action) to automate the signing process.\n\n**First Steps:**\n1. Integrate the code-sign-action into your GitHub workflow file.\n2. Generate or obtain a valid MacOS Developer ID certificate.\n3. Store the certificate securely in GitHub Secrets for access during the build process.\n4. Update the workflow configuration to include the necessary parameters for the code-sign-action, such as the certificate and entitlements file.\n5. Test the workflow to ensure binaries are signed correctly and verify the signature after the build.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/wrongsecrets-binaries/issues/36",
@@ -2738,10 +2835,11 @@
"pk": 98,
"fields": {
"nest_created_at": "2024-09-11T19:25:01.635Z",
- "nest_updated_at": "2024-09-11T19:25:01.635Z",
+ "nest_updated_at": "2024-09-13T15:06:18.772Z",
"node_id": "I_kwDOHGUcT855-cLX",
"title": "Unnecessary use of LIST permission example of attack does not work",
"body": "I tired to replicate the Unnecessary use of LIST permission example attack but it does not work. I think the problem is that in the K8s version before 1.24, every time we would create a service account, a non-expiring secret token (Mountable secrets & Tokens) was created by default. However, from version 1.24 onwards, it was disbanded and no secret token is created by default when we create a service account.\r\n\r\nWhen i tried to access to http://127.0.0.1:8001/api/v1/namespaces/default/secrets/abcd link i can see the \"secretAuthToken\": \"dmVyeVNlY3VyZTEyMw==\"\r\n",
+ "summary": "The issue discusses the failure to replicate a specific attack example related to the unnecessary use of LIST permissions. The author notes that the attack's context relies on the presence of a non-expiring secret token associated with service accounts, which was standard practice in Kubernetes versions prior to 1.24. However, this behavior has changed in version 1.24, where no secret token is created by default for new service accounts. The author successfully accessed a secret token at a given URL, indicating that while the attack example may not function as intended in newer versions, the existence of the secret token can still be observed.\n\nTo address this issue, it may be necessary to update the attack example to reflect the changes in Kubernetes version 1.24 and clarify the conditions under which it can be replicated.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-kubernetes-top-ten/issues/53",
@@ -2764,10 +2862,11 @@
"pk": 99,
"fields": {
"nest_created_at": "2024-09-11T19:25:06.040Z",
- "nest_updated_at": "2024-09-11T19:25:06.040Z",
+ "nest_updated_at": "2024-09-13T15:06:22.019Z",
"node_id": "I_kwDOHGSr786BytOw",
"title": "Please add a description to this project",
"body": "",
+ "summary": "The issue requests the addition of a project description. It suggests that a clear and concise explanation of the project's purpose and functionality would be beneficial. To address this, a brief overview outlining the project's goals, features, and usage should be developed and included in the project documentation.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-toctourex/issues/1",
@@ -2790,10 +2889,11 @@
"pk": 100,
"fields": {
"nest_created_at": "2024-09-11T19:25:13.295Z",
- "nest_updated_at": "2024-09-11T19:25:13.295Z",
+ "nest_updated_at": "2024-09-13T15:06:27.918Z",
"node_id": "I_kwDOHGPq186BytNV",
"title": "Please add a description to this project",
"body": "",
+ "summary": "The issue requests the addition of a project description to improve clarity and provide context for users. To address this, a concise and informative description should be drafted and included in the project documentation.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-supplychaingoat/issues/1",
@@ -2820,6 +2920,7 @@
"node_id": "I_kwDOG95VyM5Fl4uZ",
"title": "Add Initial Wavenet Logic for generating Text To Speech.",
"body": "Add a folder TTS, in the repo, which will have the logic to convert Text to Speech, add text file for testing as well. \r\n\r\nYou can either use:\r\n1. Wavenets for Text to Speech Conversion. \r\n2. Google or any Open Source API ",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/SKF-VideoEditorAPI/issues/3",
@@ -2842,10 +2943,11 @@
"pk": 102,
"fields": {
"nest_created_at": "2024-09-11T19:25:31.779Z",
- "nest_updated_at": "2024-09-11T19:25:31.779Z",
+ "nest_updated_at": "2024-09-13T15:06:42.169Z",
"node_id": "I_kwDOG95VyM5Gn28w",
"title": "ADD CI/CD for the package ",
"body": "We need CI/CD pipeline for the package for following proper SDLC",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/SKF-VideoEditorAPI/issues/14",
@@ -2874,6 +2976,7 @@
"node_id": "I_kwDOG95VyM5Gn3N2",
"title": "Update README with all info about the package and how we can run it",
"body": "",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/SKF-VideoEditorAPI/issues/15",
@@ -2900,6 +3003,7 @@
"node_id": "I_kwDOG95VyM5HIdxV",
"title": "ADD Unit Test for the existing APIs",
"body": "",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/SKF-VideoEditorAPI/issues/20",
@@ -2928,6 +3032,7 @@
"node_id": "I_kwDOG95VyM5H45vv",
"title": "Adding OTP Verification API",
"body": "",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/SKF-VideoEditorAPI/issues/24",
@@ -2954,6 +3059,7 @@
"node_id": "I_kwDOG95PUM5Fl5Ks",
"title": "Create UI In Figma and ADD design.md file",
"body": "Figma Design for the APP will be highly appreciate, please ADD design.md consisting of every component url and screenshot in the repo as well.",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/SKF-VideoEditorUI/issues/4",
@@ -2978,10 +3084,11 @@
"pk": 107,
"fields": {
"nest_created_at": "2024-09-11T19:25:41.059Z",
- "nest_updated_at": "2024-09-11T19:25:41.059Z",
+ "nest_updated_at": "2024-09-13T15:06:46.179Z",
"node_id": "I_kwDOG95PUM5H45x_",
"title": "Adding OTP Verification UI",
"body": "",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/SKF-VideoEditorUI/issues/8",
@@ -3004,10 +3111,11 @@
"pk": 108,
"fields": {
"nest_created_at": "2024-09-11T19:25:58.098Z",
- "nest_updated_at": "2024-09-11T19:25:58.098Z",
+ "nest_updated_at": "2024-09-13T15:06:59.645Z",
"node_id": "I_kwDOG2VVl86BytMG",
"title": "Please add a description to this project",
"body": "",
+ "summary": "A request has been made to include a project description. It would be beneficial to provide an overview that outlines the project's purpose, features, and how to get started.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-chaingoat/issues/1",
@@ -3030,10 +3138,11 @@
"pk": 109,
"fields": {
"nest_created_at": "2024-09-11T19:26:10.299Z",
- "nest_updated_at": "2024-09-11T19:26:10.299Z",
+ "nest_updated_at": "2024-09-13T15:07:08.955Z",
"node_id": "I_kwDOGwIlcs6TvxGu",
"title": "Dependency Dashboard",
"body": "This issue lists Renovate updates and detected dependencies. Read the [Dependency Dashboard](https://docs.renovatebot.com/key-concepts/dashboard/) docs to learn more.\n\nThis repository currently has no open or pending branches.\n\n## Detected dependencies\n\nregex\n
\n\n\n---\n\n- [ ] Check this box to trigger a request for Renovate to run again on this repository\n\n",
+ "summary": "Implement a Dependency Dashboard for the repository. Utilize Renovate to monitor and manage updates for dependencies. Refer to the [Dependency Dashboard documentation](https://docs.renovatebot.com/key-concepts/dashboard/) for guidance.\n\n1. Note that the repository currently has no open or pending branches.\n2. Identify the detected dependency: `corazawaf/coraza` version `3.2.1`.\n3. To trigger Renovate to run again, check the provided box. \n\nBegin by setting up Renovate if it isn't already configured, and ensure it has access to the repository's dependencies. After that, actively monitor the dashboard for updates and manage them accordingly.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-coraza-web-application-firewall/issues/9",
@@ -3056,10 +3165,11 @@
"pk": 110,
"fields": {
"nest_created_at": "2024-09-11T19:26:14.303Z",
- "nest_updated_at": "2024-09-11T19:26:14.303Z",
+ "nest_updated_at": "2024-09-13T15:07:12.280Z",
"node_id": "I_kwDOGwIRCM5Rx9gw",
"title": "Supply Chain Management section doesn't focus on the \"management\"",
"body": "As a small improvement, I believe the supply chain management section should look into the \"management\" of the supply chain, more than the continuous scanning that is mentioned in the implementation phase. This section _should_ focus more around the inventory of applications and services that are deployed/being-used/etc (*BOMs are the direct example here) and the ability to go through them to understand what you have, and what you can filter whenever a need comes up (you need to search for a CVE, you need to understand how many apps use a specific library, etc.)",
+ "summary": "The GitHub issue suggests that the Supply Chain Management section lacks emphasis on the \"management\" aspect of supply chains. It recommends that this section should concentrate on inventorying applications and services, such as BOMs, to enhance understanding of deployed resources. The focus should include the ability to filter and search for specific needs, such as identifying CVEs or tracking library usage across applications. Consider revising the section to address these points for improved clarity and functionality.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-application-security-awareness-campaigns/issues/1",
@@ -3082,10 +3192,11 @@
"pk": 111,
"fields": {
"nest_created_at": "2024-09-11T19:26:40.955Z",
- "nest_updated_at": "2024-09-11T19:26:40.955Z",
+ "nest_updated_at": "2024-09-13T15:07:33.453Z",
"node_id": "I_kwDOGdcO-85AVcdr",
"title": "Layout issue",
"body": "The page build failed for the `main` branch with the following error:\r\n\r\nA file was included in `/_layouts/col-sidebar.html` that is a symlink or does not exist in your `_includes` directory. \r\n\r\n\r\nThe page build failed for the `main` branch with the following error:\r\n\r\nThe tag `https` on line 52 in `index.md` is not a recognized Liquid tag",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-chapter-kanchipuram/issues/1",
@@ -3112,6 +3223,7 @@
"node_id": "I_kwDOGDmZaM5HOG_e",
"title": "Build an Operation that will allow running arbitrary commands",
"body": "Sometimes it's useful to run an arbitrary command or external script when receiving a response.",
+ "summary": "Implement an Operation to execute arbitrary commands or external scripts upon receiving a response. \n\n1. Define the operation interface to accept command strings and necessary parameters. \n2. Utilize a command execution library compatible with the target environment (e.g., `subprocess` in Python).\n3. Ensure proper handling of input and output streams to capture command results or errors.\n4. Implement error handling for command execution failure, including logging and response handling.\n5. Create a configuration option to enable or disable this feature for security considerations.\n6. Write unit tests to validate command execution with various inputs and ensure safe handling of commands.\n\nBegin by setting up the project structure and identifying the language/framework used. Then, create a prototype of the command execution function using the chosen execution library.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/21",
@@ -3143,6 +3255,7 @@
"node_id": "I_kwDOGDmZaM5HOHLM",
"title": "Add a JWT parser",
"body": "JWT is used in many places already, Raider needs a way to inspect a JWT object",
+ "summary": "Implement a JWT parser for Raider to enable inspection of JWT objects. \n\n1. Research existing JWT libraries available for the programming language used in Raider. Consider libraries like `jsonwebtoken` for JavaScript or `pyjwt` for Python.\n \n2. Create a new module or class specifically for JWT parsing. Define functions to decode and verify JWT tokens, ensuring to handle different signing algorithms (e.g., HS256, RS256).\n\n3. Implement error handling for invalid tokens and provide meaningful error messages.\n\n4. Write unit tests for the JWT parser to ensure its reliability and correctness. Test various cases, including valid tokens, expired tokens, and malformed tokens.\n\n5. Update the Raider documentation to include usage examples and integration instructions for the new JWT parser.\n\n6. Open a pull request to merge the JWT parser into the main branch, allowing for code review and further enhancements.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/22",
@@ -3174,6 +3287,7 @@
"node_id": "I_kwDOGDmZaM5HOIm5",
"title": "Write a tutorial with a demo on access control",
"body": "Write a technical blog post detailing instructions on how to set up and run access control automatically using Raider.",
+ "summary": "Create a comprehensive tutorial that demonstrates access control using Raider. Include step-by-step instructions to set up and run the access control system automatically. \n\n1. **Define Access Control**: Start the tutorial by explaining what access control is and its importance in securing applications.\n\n2. **Set Up Raider**: \n - Install Raider on your system by cloning the repository from GitHub.\n - Navigate to the Raider directory and run the installation commands specific to your environment.\n\n3. **Configure Access Control**:\n - Describe how to configure access control settings in Raider. Include examples of JSON or YAML configuration files that specify user roles and permissions.\n\n4. **Implement Access Control Logic**: \n - Write code snippets showing how to integrate access control checks within your application. Use Raider's API to manage user permissions effectively.\n\n5. **Test the Implementation**: \n - Provide instructions for running tests to ensure that the access control mechanism works as intended. Use unit tests to validate role-based access.\n\n6. **Demo Application**: \n - Create a simple demo application that showcases the access control features. Walk through the steps needed to run the application and test different user roles.\n\n7. **Troubleshooting**: \n - Include a section on common issues and solutions when setting up access control with Raider.\n\n8. **Conclusion**: \n - Summarize the benefits of implementing access control and encourage users to explore further customizations with Raider.\n\nBy following these steps, ensure the tutorial is detailed, clear, and provides a hands-on experience for the reader.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/24",
@@ -3205,6 +3319,7 @@
"node_id": "I_kwDOGDmZaM5HOI5Q",
"title": "Update documentation for all operations",
"body": "Progress:\r\n- [ ] NextStage\r\n- [ ] Print\r\n- [ ] Save\r\n- [ ] Error\r\n- [ ] Http\r\n- [ ] Grep\r\n- [ ] Writing custom operations",
+ "summary": "Update documentation for all operations listed below:\n\n1. **NextStage**: Specify usage scenarios, parameters, and examples. Include error handling procedures.\n2. **Print**: Detail the output formatting options and potential use cases. Provide code snippets for clarity.\n3. **Save**: Explain the different save options and formats available, along with examples of how to implement them.\n4. **Error**: Document error types, common error messages, and troubleshooting tips. Create a section for best practices.\n5. **Http**: Outline the HTTP methods supported, authentication mechanisms, and error handling. Include examples of API calls.\n6. **Grep**: Clarify the syntax, options available, and typical use cases. Provide sample commands for various scenarios.\n7. **Writing custom operations**: Provide guidelines on how to create custom operations, including structure, naming conventions, and integration with existing operations.\n\n**First Steps**:\n- Review current documentation for each operation.\n- Gather examples and use cases from existing code and user feedback.\n- Define a consistent format for documentation updates.\n- Assign team members to specific operations for faster progress.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/26",
@@ -3236,6 +3351,7 @@
"node_id": "I_kwDOGDmZaM5HOI89",
"title": "Update documentation for all Plugins",
"body": "Progress:\r\n- [X] Common\r\n - [X] Plugin\r\n - [X] Parser\r\n - [X] Processor\r\n - [X] Empty\r\n- [X] Basic\r\n - [X] Variable\r\n - [X] Prompt\r\n - [X] Cookie\r\n - [X] Header\r\n - [X] File\r\n - [X] Command\r\n - [X] Regex\r\n - [X] Html\r\n - [ ] Json\r\n- [ ] Modifiers\r\n - [ ] Alter\r\n - [ ] Combine\r\n- [ ] Parsers\r\n - [ ] Urlparser\r\n- [ ] Processors\r\n - [ ] Urlencode\r\n - [ ] Urldecode\r\n - [ ] B64encode\r\n - [ ] B64decode\r\n- [ ] Writing custom plugins",
+ "summary": "Update the documentation for all plugins. Ensure that the following sections are completed:\n\n1. **Common**\n - Confirm the following are documented: \n - Plugin\n - Parser\n - Processor\n - Empty\n\n2. **Basic**\n - Document the following plugins:\n - Variable\n - Prompt\n - Cookie\n - Header\n - File\n - Command\n - Regex\n - Html\n - Json (incomplete)\n\n3. **Modifiers**\n - Complete documentation for:\n - Alter\n - Combine\n\n4. **Parsers**\n - Document the Urlparser.\n\n5. **Processors**\n - Document the following processors:\n - Urlencode\n - Urldecode\n - B64encode\n - B64decode\n\n6. **Writing Custom Plugins**\n - Provide comprehensive guidelines for writing custom plugins.\n\n**First Steps:**\n- Review existing documentation for the completed sections to ensure accuracy.\n- Identify the key features and functionalities of the plugins that require documentation.\n- Draft documentation for the incomplete entries, focusing on clear examples and usage scenarios.\n- Collaborate with other developers to gather insights and feedback on the custom plugins section.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/27",
@@ -3267,6 +3383,7 @@
"node_id": "I_kwDOGDmZaM5HOLcN",
"title": "Build a simple mechanism to run bruteforce attacks",
"body": "",
+ "summary": "Implement a simple bruteforce attack mechanism. \n\n1. Define the target system and the authentication method (e.g., username/password, API tokens).\n2. Create a list of potential usernames and passwords. Use dictionaries or generate combinations programmatically.\n3. Choose a programming language suitable for network requests (e.g., Python, JavaScript).\n4. Utilize libraries for handling HTTP requests (e.g., `requests` in Python).\n5. Set up a loop to iterate through the usernames and passwords, sending requests to the target system.\n6. Implement error handling to capture failed attempts and check responses for successful authentication.\n7. Consider adding delays between requests to avoid detection and rate limiting.\n\nBegin by testing against a controlled environment to ensure compliance and avoid legal issues.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/29",
@@ -3284,9 +3401,9 @@
59
],
"labels": [
- 35,
40,
- 41
+ 41,
+ 35
]
}
},
@@ -3299,6 +3416,7 @@
"node_id": "I_kwDOGDmZaM5HOLpE",
"title": "Fuzz more than one input at a time",
"body": "",
+ "summary": "Implement a feature to allow fuzzing of multiple inputs concurrently. Focus on modifying the fuzzing engine to handle multiple inputs in parallel without conflicts. \n\n1. Analyze the current architecture to understand how inputs are processed sequentially.\n2. Identify potential race conditions or shared resource conflicts when inputs are fuzzed simultaneously.\n3. Refactor the input handling mechanism to support concurrent processing, possibly using threads or asynchronous I/O.\n4. Update the input generation logic to create and manage multiple input streams.\n5. Ensure proper logging and error handling for each input to track the results effectively.\n6. Test the implementation with various input combinations to validate stability and performance.\n\nConsider reviewing existing fuzzing frameworks for inspiration and best practices in concurrent input handling.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/30",
@@ -3316,10 +3434,10 @@
59
],
"labels": [
- 35,
40,
41,
- 42
+ 42,
+ 35
]
}
},
@@ -3332,6 +3450,7 @@
"node_id": "I_kwDOGDmZaM5HOMDA",
"title": "Add shortcuts for common fuzzing payloads",
"body": "Add shortcuts to generate random integers, characters, etc... for fuzzing purposes",
+ "summary": "Implement shortcuts for generating common fuzzing payloads, such as random integers and characters. \n\n1. Define a list of commonly used payload types for fuzzing, including:\n - Random integers (specify range)\n - Random characters (specify character set)\n - Random strings (specify length and character set)\n - Special characters or control sequences\n\n2. Create a utility function or class to generate these payloads efficiently. Ensure it handles edge cases and provides options for customization.\n\n3. Integrate the shortcuts into the existing fuzzing framework, allowing users to easily access these functionalities.\n\n4. Update the documentation to include usage examples and instructions on how to utilize the new shortcuts for fuzzing.\n\n5. Consider implementing a configuration file where users can define their preferences for payload generation.\n\nStart by reviewing the current codebase for existing payload generation methods, and identify areas where shortcuts can be implemented or improved.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/31",
@@ -3349,9 +3468,9 @@
59
],
"labels": [
- 35,
40,
- 41
+ 41,
+ 35
]
}
},
@@ -3364,6 +3483,7 @@
"node_id": "I_kwDOGDmZaM5HOMRw",
"title": "Make fuzzing faster with threads",
"body": "Currently fuzzing runs slow because it's using a single thread.",
+ "summary": "Enhance fuzzing performance by implementing multithreading. Analyze the current single-threaded fuzzing process to identify bottlenecks. \n\n1. Investigate the existing codebase to understand how the fuzzing loop operates. \n2. Refactor the code to divide the fuzzing workload across multiple threads.\n3. Utilize a thread pool to manage the threads efficiently and avoid frequent creation and destruction of thread instances.\n4. Ensure proper synchronization mechanisms are in place to handle shared resources and prevent race conditions.\n5. Test the modified fuzzing process to confirm that multithreading improves performance without sacrificing accuracy. \n\nBegin by setting up a development environment where you can safely test changes to the fuzzing implementation.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/32",
@@ -3381,9 +3501,9 @@
59
],
"labels": [
- 35,
40,
- 41
+ 41,
+ 35
]
}
},
@@ -3396,6 +3516,7 @@
"node_id": "I_kwDOGDmZaM5HOM_f",
"title": "Document fuzzing",
"body": "",
+ "summary": "Implement comprehensive fuzzing documentation. \n\n1. Define fuzzing: Explain what fuzzing is and its importance in software testing.\n2. Outline fuzzing techniques: Describe different types of fuzzing, such as mutation-based, generation-based, and protocol-based fuzzing.\n3. Detail setup instructions: Provide step-by-step guidelines for setting up a fuzzing environment, including necessary tools and dependencies.\n4. Include usage examples: Offer practical examples of how to run fuzzers on specific applications or codebases.\n5. Highlight best practices: List best practices for effective fuzzing, such as targeting specific inputs, monitoring application behavior, and interpreting results.\n\nFirst steps:\n- Research existing fuzzing frameworks and tools like AFL, LibFuzzer, or Honggfuzz.\n- Create a basic tutorial for setting up one of these tools in a sample project.\n- Collect common pitfalls and troubleshooting tips from community discussions.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/33",
@@ -3413,9 +3534,9 @@
59
],
"labels": [
- 38,
40,
- 41
+ 41,
+ 38
]
}
},
@@ -3428,6 +3549,7 @@
"node_id": "I_kwDOGDmZaM5Th9LM",
"title": "Document command line interface",
"body": "Add documentation for the command line interface",
+ "summary": "Document the command line interface (CLI). Include detailed instructions on usage, commands, options, and examples. Start by identifying all the available commands and their functionalities. \n\n1. List all CLI commands and describe their purpose.\n2. For each command, specify required and optional arguments.\n3. Provide usage examples for clarity, demonstrating common scenarios.\n4. Include error handling information and troubleshooting tips.\n5. Organize the documentation into sections for easy navigation.\n\nBegin by gathering existing code comments and any informal documentation. Review the CLI codebase to ensure accuracy and completeness.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/76",
@@ -3445,8 +3567,8 @@
59
],
"labels": [
- 38,
- 43
+ 43,
+ 38
]
}
},
@@ -3459,6 +3581,7 @@
"node_id": "I_kwDOGDmZaM5Th-8Z",
"title": "Add example showing how to write custom Operations/Plugins",
"body": "",
+ "summary": "Create an example demonstrating the process of writing custom Operations/Plugins. \n\n1. Define the purpose of the custom operation/plugin.\n2. Set up the development environment, ensuring all dependencies are installed.\n3. Create a new file for the custom operation/plugin in the appropriate directory.\n4. Implement the core functionality, adhering to the existing code structure and conventions.\n5. Write unit tests to validate the custom operation/plugin.\n6. Document the code, explaining how to use the custom operation/plugin effectively.\n7. Test the implementation in a sample project to ensure compatibility and performance.\n8. Submit a pull request with the new example, including a clear description of the changes made. \n\nFocus on providing clear, concise code snippets and explanations in the example for better understanding.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/77",
@@ -3476,9 +3599,9 @@
59
],
"labels": [
- 38,
- 37,
36,
+ 37,
+ 38,
44,
45
]
@@ -3493,6 +3616,7 @@
"node_id": "I_kwDOGDmZaM5TiFlr",
"title": "Add attack examples to documentation",
"body": "- [ ] Fuzzing username/password\r\n - [ ] Bruteforce\r\n - [ ] User enumeration\r\n - [ ] User registration\r\n - [ ] Password reset\r\n- [ ] Multi-factor authentication\r\n - [ ] Bruteforcing MFA code\r\n - [ ] Bypassing MFA checks\r\n- [ ] Session management\r\n - [ ] Session fixation\r\n - [ ] Session hijacking\r\n - [ ] Improper session termination\r\n- [ ] Authentication bypass\r\n - [ ] Replay attack\r\n - [ ] Missing critical authentication step\r\n - [ ] Using alternate step to bypass security restrictions\r\n - [ ] Missing authentication for critical functions\r\n- [ ] OAuth2.0\r\n - [ ] bruteforcing client_id/client_secret\r\n - [ ] redirect_uri vulnerabilities\r\n - [ ] abusing OAuth grant types\r\n - [ ] PKCE downgrade\r\n - [ ] bruteforce available scopes\r\n - [ ] automate username/password bruteforce attacks on OAuth\r\n - [ ] CSRF attacks\r\n - [ ] leaking authorization code\r\n - [ ] leaking access/refresh tokens\r\n - [ ] improper token invalidation ",
+ "summary": "Add attack examples to the documentation. Include specific details for each category:\n\n1. **Fuzzing Username/Password:**\n - Document approaches for:\n - Bruteforce attacks\n - User enumeration tactics\n - Exploiting user registration processes\n - Attacking password reset functionalities\n\n2. **Multi-Factor Authentication (MFA):**\n - Provide examples for:\n - Bruteforcing MFA codes\n - Bypassing MFA checks through various means\n\n3. **Session Management:**\n - Illustrate vulnerabilities related to:\n - Session fixation attacks\n - Session hijacking methodologies\n - Improper session termination scenarios\n\n4. **Authentication Bypass:**\n - Detail techniques such as:\n - Conducting replay attacks\n - Identifying missing critical authentication steps\n - Utilizing alternate steps to bypass security restrictions\n - Recognizing functions lacking authentication\n\n5. **OAuth 2.0 Vulnerabilities:**\n - Explain risks associated with:\n - Bruteforcing client_id and client_secret\n - Redirect_uri vulnerabilities\n - Abusing various OAuth grant types\n - PKCE downgrade attacks\n - Bruteforcing available scopes\n - Automating username/password bruteforce attacks on OAuth\n - CSRF attack scenarios\n - Leaking authorization codes\n - Leaking access and refresh tokens\n - Improper token invalidation issues\n\n**First Steps:**\n- Gather real-world attack scenarios and examples for each category.\n- Collaborate with security experts to validate the examples.\n- Prepare detailed write-ups for each attack, including potential mitigations.\n- Update the documentation repository with the new content and ensure it is easily accessible.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/78",
@@ -3510,9 +3634,9 @@
59
],
"labels": [
- 38,
40,
- 45
+ 45,
+ 38
]
}
},
@@ -3525,6 +3649,7 @@
"node_id": "I_kwDOGDmZaM5Tj54i",
"title": "Write tutorials with sample authentications on authenticationtest.com",
"body": "",
+ "summary": "Create tutorials for authentication methods used on authenticationtest.com. \n\n1. Identify key authentication methods to cover, such as OAuth, JWT, and Basic Authentication.\n2. Develop sample code snippets for each method, ensuring clarity and simplicity.\n3. Include step-by-step instructions on how to implement each authentication method.\n4. Provide explanations of security considerations and best practices associated with each method.\n5. Develop a section for troubleshooting common issues that users may encounter during implementation.\n6. Format the tutorials for easy readability and accessibility on the website.\n\nBegin by gathering documentation from authenticationtest.com to ensure accuracy. Then, outline the structure of each tutorial before diving into coding and writing.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/80",
@@ -3542,9 +3667,9 @@
60
],
"labels": [
+ 46,
38,
- 39,
- 46
+ 39
]
}
},
@@ -3557,6 +3682,7 @@
"node_id": "I_kwDOGDmZaM5Tj59e",
"title": "Write a tutorial on testing OAuth",
"body": "Using Keycloak should work",
+ "summary": "Create a tutorial on testing OAuth with Keycloak. Begin by outlining the prerequisites: ensure you have Keycloak installed and running, and access to a web application or API that requires OAuth authentication.\n\n1. **Set Up Keycloak**:\n - Download and install Keycloak.\n - Start the Keycloak server.\n - Access the Keycloak admin console (typically at `http://localhost:8080/auth`).\n\n2. **Configure a Realm**:\n - Create a new realm in Keycloak.\n - Define the realm settings as needed for your application.\n\n3. **Create a Client**:\n - In the created realm, navigate to \"Clients\" and create a new client.\n - Set the client ID and configure the client settings (e.g., access type as `confidential` or `public`).\n - Note the client secret if using a confidential client.\n\n4. **Set Up Roles and Users**:\n - Define roles within the realm to control access.\n - Create users and assign them the necessary roles.\n\n5. **Test OAuth Flow**:\n - Use Postman or a similar tool to initiate the OAuth flow.\n - Request an authorization code by navigating to the authorization endpoint.\n - Exchange the authorization code for an access token by calling the token endpoint.\n\n6. **Validate the Access Token**:\n - Decode the access token using a JWT library.\n - Verify claims such as expiration and audience.\n\n7. **Test API Access**:\n - Use the obtained access token to make requests to your API.\n - Ensure that the API correctly validates the token and responds based on the user's roles.\n\n8. **Automate the Testing**:\n - Write scripts using testing frameworks (e.g., Jest, Mocha) to automate the OAuth flow and API access tests.\n - Include tests for valid and invalid tokens.\n\n9. **Document the Process**:\n - Clearly document each step, providing code snippets and configuration details.\n - Include troubleshooting tips for common issues.\n\nBy following these steps, you will create a comprehensive tutorial for testing OAuth using Keycloak.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/81",
@@ -3574,9 +3700,9 @@
59
],
"labels": [
+ 47,
38,
- 39,
- 47
+ 39
]
}
},
@@ -3589,6 +3715,7 @@
"node_id": "I_kwDOGDmZaM5Tj6Op",
"title": "Write tutorials on how to exploit various JuiceShop issues",
"body": "Use [OWASP Juiceshop](https://owasp.org/www-project-juice-shop/) to demo some ways to exploit issues using raider",
+ "summary": "Create tutorials demonstrating the exploitation of various OWASP Juice Shop vulnerabilities using Raider. \n\n1. **Set Up Environment**:\n - Clone the OWASP Juice Shop repository.\n - Install Raider by following the documentation on its GitHub page.\n\n2. **Identify Vulnerabilities**:\n - Review the Juice Shop documentation to understand its known vulnerabilities.\n - Use tools like OWASP ZAP or Burp Suite to scan the Juice Shop instance for security issues.\n\n3. **Develop Tutorials**:\n - For each identified vulnerability, write a detailed tutorial that includes:\n - **Description**: Explain the vulnerability and its impact.\n - **Demonstration**: Show how to exploit the vulnerability using Raider.\n - **Mitigation**: Provide suggestions on how to fix the issue in a real application.\n\n4. **Organize Content**:\n - Structure the tutorials in a clear format, using headings, code snippets, and screenshots where applicable.\n\n5. **Publish and Share**:\n - Create a dedicated branch in the GitHub repository for the tutorials.\n - Use Markdown for formatting and ensure the content is accessible and easy to understand.\n\nBy following these steps, effectively educate users on exploiting and securing applications against vulnerabilities through practical demonstrations.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/83",
@@ -3620,6 +3747,7 @@
"node_id": "I_kwDOGDmZaM5Tj7Vk",
"title": "Build a complete CLI",
"body": "Current command line interface is still lacking in features. Following commands are planned:\r\n\r\n- [ ] show\r\n - [x] projects\r\n - [x] hyfiles\r\n - [x] graphs\r\n - [x] flows\r\n - [ ] plugins\r\n - [ ] inputs\r\n - [ ] outputs\r\n - [ ] operations\r\n- [x] config\r\n - [x] proxy\r\n - [x] verify\r\n - [x] loglevel\r\n - [x] user agent\r\n - [x] active project\r\n- [ ] new\r\n - [x] project\r\n - [x] hyfile\r\n - [ ] user\r\n - [ ] copy project\r\n- [x] delete\r\n - [x] project\r\n - [x] hyfiles\r\n- [ ] edit\r\n - [ ] rename project\r\n - [ ] rename hyfile\r\n - [x] edit hyfile by filename\r\n - [ ] edit hyfile by Flow/FlowGraph name\r\n- [ ] inspect\r\n - [ ] inspect HTTP requests/responses\r\n - [ ] show inputs/outputs\r\n- [ ] run\r\n - [X] single Flow\r\n - [X] FlowGraph\r\n - [X] Chain Flows/FlowGraphs\r\n - [ ] choose a User\r\n- [ ] fuzz\r\n - [ ] fuzz single flow\r\n - [ ] fuzz plugin in a flowgraph\r\n- [ ] shell\r\n - [ ] run ipython to debug configuration\r\n - [ ] run hy to debug configuration\r\n",
+ "summary": "Enhance the command line interface (CLI) by implementing the following planned commands and their associated features. \n\n1. **Show Command**: \n - Implement `show plugins`, `show inputs`, `show outputs`, and `show operations` functionalities to complete this command.\n \n2. **New Command**: \n - Finalize `new user` and `new copy project` features, which are currently incomplete.\n\n3. **Edit Command**:\n - Develop `rename project`, `rename hyfile`, and `edit hyfile by Flow/FlowGraph name` functionalities to extend this command.\n\n4. **Inspect Command**:\n - Introduce `inspect HTTP requests/responses` and `show inputs/outputs` features to enhance the inspection capabilities of the CLI.\n\n5. **Run Command**:\n - Add the ability to `choose a User` for running flows, which is currently missing.\n\n6. **Fuzz Command**:\n - Create functionalities for `fuzz single flow` and `fuzz plugin in a flowgraph` to allow fuzz testing options.\n\n7. **Shell Command**:\n - Implement options to `run ipython` and `run hy` for debugging configuration issues.\n\n**First Steps**:\n- Begin by prioritizing the implementation of the `show`, `new`, and `edit` commands since they have multiple incomplete functionalities.\n- Set up a development environment and create feature branches for each command.\n- Write unit tests for each new feature to ensure reliability.\n- Review existing codebase for any reusable components that can be utilized in the new implementations.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/85",
@@ -3637,8 +3765,8 @@
59
],
"labels": [
- 35,
- 43
+ 43,
+ 35
]
}
},
@@ -3651,6 +3779,7 @@
"node_id": "I_kwDOGDmZaM5Tj737",
"title": "Build a way to debug hyfiles",
"body": "- [ ] print hylang code of flows in logs\r\n- [ ] run an interactive hy shell\r\n- [ ] run an interactive python shell\r\n- [ ] run scripts from CLI\r\n- [ ] interact with plugins\r\n - [ ] breakpoint on plugin used as input\r\n - [ ] show current plugin value\r\n - [ ] send the request with new value\r\n - [ ] receive the response \r\n - [ ] runs operations\r\n - [ ] continue next flow in the graph\r\n - [ ] set up NextStage\r\n- [ ] interact with flows\r\n - [ ] breakpoint before a flow's request is sent\r\n - [ ] or run just a flow ignoring previous flows in the graph\r\n - [ ] show current request inputs (including missing ones)\r\n - [ ] asks the user to change any value\r\n - [ ] supply missing values\r\n - [ ] sends new request\r\n - [ ] receives the response\r\n - [ ] run operations\r\n - [ ] continue to next flow\r\n - [ ] or manually set the NextStage \r\n",
+ "summary": "Implement a debugging system for hyfiles by following these steps:\n\n1. **Log Hylang Code**: Ensure that the Hylang code of flows is printed in the logs for easy reference.\n \n2. **Interactive Shells**: \n - Integrate an interactive Hy shell for executing Hylang commands.\n - Integrate an interactive Python shell for executing Python commands.\n\n3. **CLI Script Execution**: Enable the ability to run scripts directly from the command-line interface (CLI).\n\n4. **Plugin Interaction**: \n - Set breakpoints on plugins used as input to monitor their execution.\n - Display the current value of the plugin.\n - Implement functionality to send requests with modified values.\n - Handle the response received from the plugin.\n - Allow for various operations to be executed post-response.\n - Enable continuation to the next flow in the graph or set up the NextStage.\n\n5. **Flow Interaction**: \n - Introduce breakpoints before a flow's request is dispatched.\n - Allow execution of a single flow while bypassing prior flows in the graph.\n - Display current request inputs, highlighting any missing values.\n - Prompt the user to modify values as needed.\n - Supply any missing values and resend the request.\n - Capture and process the response, allowing for subsequent operations.\n - Continue to the next flow or manually set the NextStage as necessary.\n\nBy addressing these tasks, create a robust debugging framework that enhances the development and troubleshooting of hyfiles. Start by establishing logging mechanisms and integrating interactive shells, then progressively implement the plugin and flow interaction features.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/86",
@@ -3682,6 +3811,7 @@
"node_id": "I_kwDOGDmZaM5Tj9tU",
"title": "Update user agent version in common.hy",
"body": "Currently raider doesn't update its version so the one used in _common.hy will only be used even if newer version is installed",
+ "summary": "Update the user agent version in `common.hy`. Ensure that the raider updates its version correctly to reflect any newer versions installed. \n\n1. Locate the section in `common.hy` where the user agent version is defined.\n2. Modify the logic to check for the installed version of the raider and update the user agent accordingly.\n3. Implement a version-checking mechanism that compares the current version with the installed version.\n4. Test the changes to verify that the user agent reflects the latest installed version. \n5. Document the changes made for clarity and future reference.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/87",
@@ -3699,8 +3829,8 @@
59
],
"labels": [
- 46,
- 48
+ 48,
+ 46
]
}
},
@@ -3713,6 +3843,7 @@
"node_id": "I_kwDOGDmZaM5Tj91t",
"title": "Add a new hyfile next to common.hy that isn't changed by raider",
"body": "Some raider settings might be desired to be set by the user globally and read upon starting raider. A new file is needed for that, since common.hy is changed by raider automatically.",
+ "summary": "Create a new configuration file, named `user_settings.hy`, situated alongside `common.hy`. Ensure that this file is not modified by the raider tool during its operations. \n\n1. Define the purpose of `user_settings.hy` to allow users to set global raider settings that persist across sessions.\n2. Implement functionality within the raider startup process to read configurations from `user_settings.hy`.\n3. Ensure that modifications to `common.hy` do not affect or overwrite the settings defined in `user_settings.hy`.\n4. Document the format and options available for user settings in the new file for clarity.\n5. Test the integration by verifying that settings in `user_settings.hy` are correctly loaded at startup and do not conflict with any modifications made to `common.hy`.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/88",
@@ -3730,8 +3861,8 @@
59
],
"labels": [
- 35,
42,
+ 35,
44
]
}
@@ -3745,6 +3876,7 @@
"node_id": "I_kwDOGDmZaM5Tj-Dd",
"title": "Build a mechanism to print the diff between responses",
"body": "Often during pentesting one changes the request input then compares the 2 responses to check for differences. Raider needs a mechanism to print the diff of 2 responses when using different values as the input of a plugin.",
+ "summary": "Implement a diff mechanism for comparing responses in Raider. \n\n1. Create a function that captures responses from plugin input variations.\n2. Utilize a diffing library (e.g., `difflib` in Python) to compare the two response outputs.\n3. Format the diff output for clarity, highlighting additions, deletions, and modifications.\n4. Integrate this function into the existing plugin workflow, ensuring it triggers automatically when multiple responses are generated.\n5. Test the implementation with various input scenarios to validate accuracy and performance.\n\nBegin by reviewing the current response handling in Raider, then proceed to set up the diffing functionality.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/89",
@@ -3762,9 +3894,9 @@
59
],
"labels": [
- 35,
40,
- 42
+ 42,
+ 35
]
}
},
@@ -3777,6 +3909,7 @@
"node_id": "I_kwDOGDmZaM5Tj-TX",
"title": "Add an easier way to encode/decode and get the hashes of inputs",
"body": "Currently the user needs to import libraries in hyfiles when they want for example to calculate the md5 hash of some string before sending it. Since this is common, it would make sense to import them automatically so the user has to write less code to actually use those.",
+ "summary": "Implement an automatic import feature for commonly used encoding, decoding, and hashing libraries in hyfiles. This enhancement will simplify the user experience by allowing direct access to functions like MD5 hash calculations without manual imports.\n\n1. Identify the most commonly used libraries for encoding, decoding, and hashing (e.g., `hashlib` for MD5).\n2. Modify the hyfiles initialization process to include these libraries automatically.\n3. Create utility functions for encoding and decoding, as well as for calculating hashes, to streamline user interaction.\n4. Update documentation to reflect the new automatic imports and usage examples.\n5. Consider backward compatibility to ensure existing code remains functional without requiring changes.\n\nBegin by investigating the current import process in hyfiles and design the implementation plan for automatic imports.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/90",
@@ -3794,8 +3927,8 @@
59
],
"labels": [
- 35,
42,
+ 35,
44
]
}
@@ -3809,6 +3942,7 @@
"node_id": "I_kwDOGDmZaM5Tj-jK",
"title": "Work with sessions in raider",
"body": "Raider would benefit from saving the history of all generated inputs/received outputs in a session file in _project.hy. To do this we need:\r\n\r\n- [ ] Saving all plugin inputs\r\n- [ ] Saving all plugin outputs ",
+ "summary": "Implement session management in Raider. Modify the project to save the history of all generated inputs and received outputs in a session file named `_project.hy`. \n\n1. **Save Plugin Inputs**: \n - Create a mechanism to log all inputs provided to the plugins during a session.\n - Ensure that this data is saved in a structured format within the session file.\n \n2. **Save Plugin Outputs**: \n - Capture the outputs generated by the plugins after processing the inputs.\n - Store this information alongside the corresponding inputs in the session file for easy tracking.\n\nBegin by defining the data structure for the session file. Then, implement functions to write inputs and outputs to the file during the plugin execution. Test this functionality to ensure that all relevant data is being captured and saved correctly.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/91",
@@ -3826,10 +3960,10 @@
59
],
"labels": [
- 35,
- 38,
+ 43,
42,
- 43
+ 35,
+ 38
]
}
},
@@ -3842,6 +3976,7 @@
"node_id": "I_kwDOGDmZaM5Tj_KO",
"title": "Traverse the graphs to find vulnerabilities",
"body": "Similar to [Modeling and Discovering Vulnerabilities with Code Property Graphs\r\n](https://www.sec.cs.tu-bs.de/pubs/2014-ieeesp.pdf) it should be possible to traverse graphs to identify vulnerabilities in authentication systems. The linked paper is about finding linux kernel vulnerabilities having the source code available. When testing authentication, we usually don't have the source code, so the graph instead is built using raider Flow objects, each with its own inputs, outputs, and a way to conditionally decide what the next stage is. At the moment of writing this, the graph architecture still isn't fully implemented so it's not yet possible to start experimenting with this. I wrote this ticket to keep track of the research done towards this goal, and to have a place to discuss the progress towards it.",
+ "summary": "Implement graph traversal techniques to identify vulnerabilities in authentication systems. Reference the methodology from the paper \"Modeling and Discovering Vulnerabilities with Code Property Graphs.\" Since direct source code access is typically unavailable, utilize raider Flow objects to construct the graph, which includes inputs, outputs, and conditional pathways for traversal.\n\nTo begin tackling this problem:\n\n1. Complete the implementation of the graph architecture to facilitate experimentation.\n2. Develop algorithms to traverse the constructed graphs systematically.\n3. Identify key patterns and vulnerabilities specific to authentication systems that can be mapped onto the graph structure.\n4. Document findings and discuss progress within this GitHub issue for collaborative research and development.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/92",
@@ -3874,6 +4009,7 @@
"node_id": "I_kwDOGDmZaM5Tj_YZ",
"title": "Integrate raider with other tools",
"body": "Some tool would benefit from having an integration allowing to easily authenticate anywhere. I created this issue to keep an eye on what integrations would be worth considering:\r\n\r\n- [ ] ZAProxy\r\n- [ ] BurpSuite\r\n- [ ] mitmproxy\r\n- [ ] browser extensions (to generate hyfiles from HTTP requests/responses)\r\n- [ ] emacs (hey, it's LISP!)",
+ "summary": "Integrate Raider with essential tools for improved authentication. Focus on the following integrations:\n\n- Implement support for ZAProxy.\n- Develop a connection with BurpSuite.\n- Enable compatibility with mitmproxy.\n- Create browser extensions to generate hyfiles from HTTP requests/responses.\n- Explore integration with Emacs.\n\nStart by researching the APIs and authentication methods used by each tool. Assess the feasibility of each integration based on existing Raider architecture. Prioritize integrations based on user demand and technical complexity. Create a roadmap for implementation and assign tasks for each integration.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/93",
@@ -3891,8 +4027,8 @@
59
],
"labels": [
- 35,
- 42
+ 42,
+ 35
]
}
},
@@ -3905,6 +4041,7 @@
"node_id": "I_kwDOGDmZaM5Tj_2X",
"title": "Fix github actions",
"body": "Currently some github actions don't work as desired:\r\n\r\n- [ ] mypy action doesn't really work\r\n- [ ] what needs to run on dev and what on main\r\n - [ ] decide what events need to trigger actions\r\n - [ ] choose which branch to monitor for what action\r\n- [ ] create an action to publish raider to PyPi",
+ "summary": "Fix GitHub Actions by addressing the following tasks:\n\n1. **Resolve Mypy Action Issues**:\n - Investigate the current configuration of the mypy action in the workflow YAML file.\n - Ensure that the mypy configuration file (`mypy.ini` or `setup.cfg`) is correctly specified and located.\n - Check for any dependencies or installation issues that might prevent mypy from running.\n\n2. **Determine Action Triggers**:\n - Analyze the project's workflow to decide which actions should run on the `dev` branch versus the `main` branch.\n - List the events that should trigger specific actions (e.g., `push`, `pull_request`, `release`).\n - Document the rationale for choosing certain events for triggering actions to maintain clarity.\n\n3. **Select Branch Monitoring**:\n - Define which branches should be monitored for each action.\n - Update the workflow file to reflect these branch settings, using the `on` directive in the YAML configuration.\n\n4. **Create PyPi Publishing Action**:\n - Develop a new workflow that automates the process of publishing the package to PyPi.\n - Utilize the `twine` tool for uploading distributions, ensuring that credentials are securely managed through GitHub Secrets.\n - Define the events that should trigger this publishing action, typically on releases or merges to `main`.\n\n**First Steps**:\n- Start with reviewing the existing actions in the `.github/workflows` directory.\n- Test the mypy action locally to identify specific error messages.\n- Draft a new YAML configuration for the publishing action, focusing on the necessary steps to build and upload the package.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/94",
@@ -3935,6 +4072,7 @@
"node_id": "I_kwDOGDmZaM5TkAqC",
"title": "Create an easy way to generate hyfiles from within python",
"body": "Since more people are comfortable with Python than Lisp, it would be great to have an easy way to generate those configuration files by working with Python objects directly. Since hyfiles just import those python objects to be used, it shouldn't be too hard, and if achieved, more people will feel comfortable using raider.",
+ "summary": "Implement a Python-based solution for generating hyfiles. Focus on creating a straightforward interface that allows users to work with Python objects directly, facilitating the conversion to hyfiles. \n\n1. Analyze the current hyfile structure and identify how Python objects can be represented.\n2. Develop a Python module that allows users to define configurations using Python syntax.\n3. Implement a function to serialize these Python objects into the required hyfile format.\n4. Ensure that the generated hyfiles correctly import the Python objects as intended.\n5. Test the implementation with various use cases to guarantee compatibility and reliability.\n\nBy simplifying the process of hyfile generation, increase accessibility for users unfamiliar with Lisp, ultimately enhancing the usability of the raider tool.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/96",
@@ -3952,8 +4090,8 @@
59
],
"labels": [
- 35,
- 42
+ 42,
+ 35
]
}
},
@@ -3966,6 +4104,7 @@
"node_id": "I_kwDOGDmZaM5TkA5L",
"title": "Split operations into groups",
"body": "Like Plugins have been split into related groups, it would make sense to do the same to Operations, to make it easier to manage them. ",
+ "summary": "Split operations into related groups for improved management. Analyze existing operations and categorize them based on functionality or purpose, similar to how plugins are organized. \n\nFirst steps:\n1. Review the current list of operations to identify common themes or functionalities.\n2. Create a proposed structure for grouping operations, ensuring it aligns with user needs and enhances usability.\n3. Implement the grouping in the codebase, ensuring backward compatibility with existing functionality.\n4. Update documentation to reflect the new organization of operations. \n5. Test the changes thoroughly to verify that operations function correctly within their new groups.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/97",
@@ -3983,9 +4122,9 @@
59
],
"labels": [
+ 42,
35,
- 38,
- 42
+ 38
]
}
},
@@ -3998,6 +4137,7 @@
"node_id": "I_kwDOGDmZaM5TrAG2",
"title": "Create a custom dark mode theme for the docs",
"body": "I got some feedback from someone who looked at the docs that it would be nice to have a dark mode option. I suggest we quickly create a simple color scheme to differentiate the Raider docs from default Sphinx settings and provide a dark mode option.",
+ "summary": "Implement a custom dark mode theme for the documentation. \n\n1. Analyze the current Sphinx default theme and identify the color palette used.\n2. Design a new color scheme that enhances readability in low-light environments, focusing on contrasting text and background colors.\n3. Create a new CSS file for the dark mode styles, modifying background colors, text colors, and link colors as necessary.\n4. Update the Sphinx configuration file (`conf.py`) to include the new dark mode CSS file.\n5. Develop a toggle mechanism (e.g., a button or switch) to allow users to switch between light and dark themes.\n6. Test the new theme across different devices and browsers to ensure compatibility and usability.\n7. Gather user feedback to refine the dark mode experience. \n\nBegin with step 1 by reviewing the existing theme and experimenting with potential color combinations.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/99",
@@ -4028,6 +4168,7 @@
"node_id": "I_kwDOGDmZaM5XKtQ9",
"title": "Store Next links as igraph vertices",
"body": "For now igraph is barely being used, only the Flows are stored as nodes. To make use of igraph, the links between nodes (Next) need to be somehow stored as vertices.",
+ "summary": "Implement storage of Next links as igraph vertices. Currently, only Flows are represented as nodes in the igraph structure. Extend the igraph model to include Next links, treating them as vertices to enhance the graph's utility.\n\n1. Review the existing implementation of Flows in igraph to understand how nodes are currently structured.\n2. Define the attributes and properties of the Next links that will be stored as vertices.\n3. Modify the data model to accommodate the new vertex type for Next links.\n4. Update the igraph initialization and graph construction logic to include Next links as vertices.\n5. Test the updated implementation to ensure that Next links are correctly integrated and function as expected within the graph structure.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/105",
@@ -4045,8 +4186,8 @@
59
],
"labels": [
- 35,
- 42
+ 42,
+ 35
]
}
},
@@ -4059,6 +4200,7 @@
"node_id": "I_kwDOGDmZaM5XvTZR",
"title": "Create XPath Plugin",
"body": "Add a plugin to extract data using XPath",
+ "summary": "Create an XPath Plugin to enable data extraction via XPath queries. \n\n1. Define the plugin architecture to support XPath functionality.\n2. Implement core functionality to parse XML documents and evaluate XPath expressions.\n3. Utilize libraries like `lxml` or `xml.etree.ElementTree` for XML parsing and XPath support.\n4. Develop a user interface component for users to input XPath expressions.\n5. Add error handling to manage invalid XPath queries and provide user feedback.\n6. Write unit tests to ensure the plugin correctly extracts data based on various XPath scenarios.\n7. Document the plugin usage and provide examples for common XPath queries.\n\nBegin by setting up a basic plugin structure and integrating an XML parsing library. Then, implement a simple XPath evaluation function to validate the approach.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/109",
@@ -4089,6 +4231,7 @@
"node_id": "I_kwDOGDmZaM5X319D",
"title": "Create a Wait Operation",
"body": "Build an Operation that will wait either for a specified amount of time (sleep) or until user manually continues the process",
+ "summary": "Create a new Wait Operation that allows for two modes of operation: a timed wait and a manual wait. Implement a function that takes an input parameter for the duration of the sleep in seconds. Use built-in threading or asynchronous features to pause execution for the specified time.\n\nFor the manual continuation, design an interface or command prompt that allows the user to resume the process when ready. Utilize event listeners or signaling mechanisms to capture the user's input effectively.\n\nStart by:\n1. Defining the operation interface with parameters for time duration and mode (sleep/manual).\n2. Implementing the timed wait using `time.sleep()` or `asyncio.sleep()` for asynchronous operations.\n3. Setting up a loop or listener for manual continuation, using input() or GUI elements depending on the application context.\n4. Testing the operation in both modes to ensure correct functionality and user experience.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/111",
@@ -4119,6 +4262,7 @@
"node_id": "I_kwDOGDmZaM5X6y-4",
"title": "Fix broken PlantUML diagrams",
"body": "After upgrading documentation dependencies, the diagrams broke and aren't displayed.",
+ "summary": "Fix broken PlantUML diagrams. \n\nFirst, identify the specific dependencies that were upgraded and check their release notes for any breaking changes related to PlantUML. \n\nNext, verify the configuration settings in the documentation framework to ensure they align with the latest versions of the dependencies. \n\nInvestigate any changes in syntax or required settings for PlantUML that might have occurred due to the upgrade. \n\nRun tests to reproduce the issue and confirm that diagrams are not displaying. \n\nConsider rolling back to the previous versions of the dependencies temporarily to assess if this resolves the issue. \n\nFinally, update the PlantUML rendering process if necessary, and ensure that all diagram files are correctly formatted and accessible within the project structure.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/113",
@@ -4136,8 +4280,8 @@
59
],
"labels": [
- 38,
- 48
+ 48,
+ 38
]
}
},
@@ -4150,6 +4294,7 @@
"node_id": "I_kwDOGDmZaM5Yny49",
"title": "Remove the need to use :name parameter in Plugins",
"body": "Plugins need to have an unique name, and yet it shouldn't be the user's responsibility to give it that name, it could be the variable's name used when defining Plugins, or some automatically generated unique id if it's not set to a variable.",
+ "summary": "Remove the requirement for the `:name` parameter in Plugins. Ensure that each Plugin has a unique identifier automatically generated from the variable name used during Plugin definition, or create a fallback mechanism that generates a unique ID if no variable name is provided.\n\n1. Investigate the current implementation of Plugin registration and how the `:name` parameter is utilized.\n2. Refactor the code to extract the variable name from the context where the Plugin is defined.\n3. Implement logic to generate a unique ID for Plugins when the variable name is not available.\n4. Update the documentation to reflect these changes and guide users on the new Plugin definition process.\n5. Write unit tests to verify that unique identifiers are correctly assigned to Plugins without the `:name` parameter.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/117",
@@ -4167,8 +4312,8 @@
59
],
"labels": [
- 37,
- 42
+ 42,
+ 37
]
}
},
@@ -4181,6 +4326,7 @@
"node_id": "I_kwDOGDmZaM5Yn1HA",
"title": "Fix multiple users setup",
"body": "At the moment after the latest architecture changes, it's not possible anymore to work with multiple users. New CLI option to chose users needs to be implemented too.",
+ "summary": "Implement support for multiple user setups following recent architecture changes. Address the inability to work with multiple users in the current system. Develop a new CLI option that allows users to select their profiles. \n\nFirst steps:\n1. Review the recent architecture changes to identify breaking points affecting multi-user functionality.\n2. Define the requirements for the new CLI option, including how users will be listed and selected.\n3. Create a prototype for the CLI option, ensuring it integrates well with the existing system.\n4. Test the multi-user functionality to verify that users can switch profiles without issues.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/118",
@@ -4198,8 +4344,8 @@
59
],
"labels": [
- 42,
- 48
+ 48,
+ 42
]
}
},
@@ -4212,6 +4358,7 @@
"node_id": "I_kwDOGDmZaM5Yn3tI",
"title": "Fix logging for Plugins",
"body": "Plugins aren't logging information correctly at the moment, it doesn't use the same logger rest of raider does.",
+ "summary": "Fix the logging mechanism for plugins in the Raider application. Ensure that plugins utilize the same logging framework as the rest of the Raider codebase. \n\n1. Identify the current logging implementation used in plugins and compare it with the standard logging approach used elsewhere in Raider.\n2. Refactor the plugin logging code to adopt the common logging framework, ensuring consistency across the application.\n3. Replace any custom logging functions in plugins with the standardized logger calls.\n4. Test the logging functionality of plugins to verify that logs are recorded correctly and consistently with the rest of the application.\n5. Update documentation to reflect the changes made to the logging system for plugins. \n\nInitiate this process by reviewing the existing logging code in at least one plugin and familiarize yourself with the main Raider logging setup.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/119",
@@ -4229,8 +4376,8 @@
59
],
"labels": [
- 42,
- 48
+ 48,
+ 42
]
}
},
@@ -4243,6 +4390,7 @@
"node_id": "I_kwDOGDmZaM5Yn5yw",
"title": "Store multiple proxies in the common.hy",
"body": "Having multiple proxies stored would be useful, and accessing them by their aliases, for example 'burpsuite': 'localhost:8080' and 'zaproxy':'localhost:8081' and switching between them by the alias. http:// prefix shouldn't be a requirement, HTTP proxy is only supported type now.",
+ "summary": "Implement storage for multiple proxies in `common.hy`. Define a structure to hold proxies with aliases, such as:\n\n```python\nproxies = {\n 'burpsuite': 'localhost:8080',\n 'zaproxy': 'localhost:8081'\n}\n```\n\nEnsure that accessing proxies by their aliases is straightforward, allowing users to switch between them easily without needing an `http://` prefix. \n\nBegin by:\n\n1. Modifying the `common.hy` file to include a dictionary for proxy storage.\n2. Creating functions to add, remove, and retrieve proxies by alias.\n3. Updating existing code to utilize the new proxy structure for connections. \n4. Writing unit tests to verify that adding, retrieving, and switching proxies works correctly.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/120",
@@ -4260,8 +4408,8 @@
59
],
"labels": [
- 35,
- 42
+ 42,
+ 35
]
}
},
@@ -4274,6 +4422,7 @@
"node_id": "I_kwDOGDmZaM5Yn6fi",
"title": "Add macros and document creating own macros",
"body": "Add more macros to make configuration easier and document them.",
+ "summary": "Implement additional macros to simplify configuration processes. Ensure thorough documentation for creating custom macros is provided.\n\n**First Steps:**\n1. Identify key areas within the codebase where macros can enhance configuration.\n2. Develop new macros that address these areas, focusing on improving usability and efficiency.\n3. Create a comprehensive guide that details the steps for users to create their own macros, including examples and best practices.\n4. Review existing macros to ensure consistency in naming conventions and functionality.\n5. Test the new macros and documentation for clarity and effectiveness.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/121",
@@ -4305,6 +4454,7 @@
"node_id": "I_kwDOGDmZaM5YoLYQ",
"title": "Use consistent CamelCase across the codebase",
"body": "Some identifiers don't use CamelCase so it's confusing when having to guess which case it's in.",
+ "summary": "Identify inconsistencies in identifier naming conventions throughout the codebase. Ensure all identifiers adhere to CamelCase formatting. \n\n1. Scan the codebase for identifiers that do not follow CamelCase, such as variable names, function names, and class names.\n2. Create a list of all affected identifiers for easy reference.\n3. Implement a script or use automated tools to detect and highlight non-CamelCase identifiers.\n4. Refactor the identified identifiers to conform to CamelCase standards, ensuring to update any references throughout the code.\n5. Run tests to confirm no functionality is broken due to the renaming.\n6. Document the new naming convention in the project's contribution guidelines to promote consistency among future contributions.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/123",
@@ -4335,6 +4485,7 @@
"node_id": "I_kwDOGDmZaM5YuD93",
"title": "Fix issues reported by pylint",
"body": "After latest architecture changes the new code hasn't been tested with pylint, and it returns many issues for now that is worth looking at.",
+ "summary": "Fix pylint issues resulting from recent architecture changes. Run pylint on the new codebase to identify all reported issues. Review the output and categorize problems based on severity and type (e.g., syntax errors, style violations, potential bugs). \n\nStart with the following steps:\n1. Install pylint if not already done using `pip install pylint`.\n2. Execute pylint on the project directory: `pylint `.\n3. Document the issues reported, prioritizing them for resolution.\n4. Begin resolving the most critical issues first, such as syntax errors and major style violations.\n5. Commit changes and re-run pylint to ensure issues are addressed and no new problems are introduced. \n6. Continue this process iteratively until all issues are resolved.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/126",
@@ -4365,6 +4516,7 @@
"node_id": "I_kwDOGDmZaM5Y7thA",
"title": "Write hylang/LISP case study",
"body": "Explain why LISP was the best choice and why macros are useful:\r\n- [ ] Why LISP\r\n- [ ] Why hylang\r\n- [ ] What are Macros\r\n- [ ] How to use Macros",
+ "summary": "Create a case study focusing on Hylang and LISP. Address the following points:\n\n1. **Justify the Choice of LISP**: Explain its historical significance, expressiveness, and suitability for symbolic computation and rapid prototyping.\n\n2. **Highlight the Advantages of Hylang**: Discuss its integration with Python, ease of use for Python developers, and its Lisp-like syntax that enhances productivity.\n\n3. **Define Macros**: Clarify what macros are in LISP, emphasizing their role in code transformation and metaprogramming.\n\n4. **Demonstrate Macro Usage**: Provide examples of how to define and use macros in Hylang, showcasing their ability to extend the language and create domain-specific languages.\n\n**First Steps to Tackle the Problem**:\n- Research the historical context and unique features of LISP.\n- Compare Hylang with traditional LISP dialects and other programming languages.\n- Explore the macro system in LISP and Hylang, including syntax and common patterns.\n- Compile illustrative examples to demonstrate macro capabilities in practice.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/128",
@@ -4395,6 +4547,7 @@
"node_id": "I_kwDOGDmZaM5Y7t9H",
"title": "Write a HOWTO on writing macros",
"body": "Write a short introduction about how to use macros with raider.",
+ "summary": "Create a HOWTO document on writing macros for Raider. Start with a brief introduction explaining the purpose of macros in Raider, emphasizing their utility in automating repetitive tasks and enhancing workflow efficiency.\n\nOutline the following steps for writing macros:\n\n1. **Set Up Raider Environment**: Ensure Raider is properly installed and configured on your system.\n\n2. **Access Macro Editor**: Open Raider and navigate to the macro editor interface. Familiarize yourself with the layout and available options.\n\n3. **Define Macro Functions**: Use the provided syntax to define macro functions. Include examples of common functions, such as triggering events or executing commands.\n\n4. **Record a Macro**: Demonstrate how to record a macro by performing actions within Raider. Explain how to start, pause, and stop the recording process.\n\n5. **Edit a Macro**: Show how to edit the recorded macro for optimization. Discuss the importance of commenting within the code for clarity.\n\n6. **Test the Macro**: Guide users on how to test the macro in a controlled environment to ensure it works as intended, and how to troubleshoot common issues.\n\n7. **Save and Share Macros**: Explain how to save macros for future use and share them with other users, if applicable.\n\n8. **Best Practices**: Conclude with best practices for writing efficient and maintainable macros, such as keeping them modular and avoiding hard-coded values.\n\nEncourage readers to explore further customization options and contribute to the Raider community by sharing their macro creations.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/129",
@@ -4425,6 +4578,7 @@
"node_id": "I_kwDOGDmZaM5Y7uYz",
"title": "Document existing raider macros",
"body": "Add documentation explaining how existing raider macros work",
+ "summary": "Document existing raider macros. Include detailed explanations of the functionality and usage of each macro. Break down the components of the macros, including any parameters, return values, and examples of how they can be utilized in different scenarios. \n\nStart by identifying all existing raider macros in the codebase. Create a structured format for the documentation, such as a markdown file or wiki page, to ensure consistency. For each macro, provide a high-level overview, followed by a technical breakdown.\n\nConsider implementing the following steps:\n1. Review the current codebase to locate all macros related to raider functionality.\n2. Analyze the code to understand how each macro operates and its purpose.\n3. Write clear and concise documentation for each macro, including code snippets.\n4. Create a README or index page that links to the documentation for easy navigation.\n5. Review the documentation for clarity and accuracy before finalizing.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/130",
@@ -4455,6 +4609,7 @@
"node_id": "I_kwDOGDmZaM5Y7uzJ",
"title": "Add docstrings to all new code",
"body": "The new code has no docstrings yet, to build a complete documentation those needs to be set up.",
+ "summary": "Add docstrings to all new code to ensure comprehensive documentation. Review each function, class, and module to provide clear descriptions of their purpose, parameters, return values, and any exceptions raised. \n\nFirst, identify all new code files that lack docstrings. Next, establish a consistent format for docstrings, following the conventions of the project (e.g., Google style or NumPy style). Then, incrementally add docstrings to each identified code element, ensuring clarity and completeness. Finally, run documentation generation tools (like Sphinx) to verify that the new docstrings are incorporated correctly into the overall project documentation.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/131",
@@ -4485,6 +4640,7 @@
"node_id": "I_kwDOGDmZaM5ZPBx9",
"title": "Fix logging for utils",
"body": "Like Plugins, utils isn't using the logger properly so no log messages are being shown for example when reading hyfiles",
+ "summary": "Fix the logging implementation in the utils module. Ensure that the logging framework is correctly integrated to display log messages, particularly during the reading of hyfiles. \n\n1. Review the current logging setup in the utils module.\n2. Identify areas where logging calls are missing or incorrectly implemented.\n3. Implement appropriate logging calls at key points in the code, such as before and after reading hyfiles.\n4. Test the logging functionality to confirm that messages are displayed as expected.\n5. Update documentation to reflect any changes made in the logging process.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/136",
@@ -4502,8 +4658,8 @@
59
],
"labels": [
- 44,
- 48
+ 48,
+ 44
]
}
},
@@ -4516,6 +4672,7 @@
"node_id": "I_kwDOGDmZaM5Zm8cj",
"title": "Create a way to use GraphQL within hylang files",
"body": "Either some macros or plugins will be needed to improve the hyfiles when dealing with graphql",
+ "summary": "Implement GraphQL support in hylang files by developing macros or plugins. This will enhance the handling of GraphQL queries and mutations within Hy syntax.\n\n1. Analyze the current Hy syntax and identify key areas where GraphQL integration is necessary.\n2. Design macros that can simplify the construction of GraphQL queries, ensuring they translate properly into the underlying Python code.\n3. Consider creating a plugin that can manage GraphQL schemas, types, and resolvers, making it easier for developers to work with GraphQL in Hy.\n4. Develop unit tests to ensure the reliability of the new macros or plugins, particularly focusing on edge cases in GraphQL operations.\n5. Document the usage of the new features clearly for users of hylang, providing examples of common GraphQL operations in Hy syntax.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/141",
@@ -4533,8 +4690,8 @@
59
],
"labels": [
- 37,
- 44
+ 44,
+ 37
]
}
},
@@ -4547,6 +4704,7 @@
"node_id": "I_kwDOGDmZaM5ZsajZ",
"title": "Create a raider REPL",
"body": "To preserve a session across runs, raider would need to have a REPL, and the user should have the ability to inspect and manipulate all individual elements.",
+ "summary": "Implement a Raider REPL to enable session preservation across runs. Ensure the REPL allows users to inspect and modify individual elements within the session.\n\n1. Define the REPL interface and establish how users will interact with it.\n2. Integrate session management functionality to save and load session states.\n3. Implement inspection capabilities, allowing users to view current elements and their states.\n4. Add manipulation features to enable users to modify elements dynamically.\n5. Test the REPL for user experience and ensure all functionalities work as intended.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/raider/issues/142",
@@ -4564,8 +4722,8 @@
59
],
"labels": [
- 35,
- 42
+ 42,
+ 35
]
}
},
@@ -4578,6 +4736,7 @@
"node_id": "I_kwDOGDKlFs5mtsUw",
"title": "migrate content to www-project repo",
"body": "There is a trend in OWASP have the documentation projects accessible via the project web pages, and it may be that this repo could be combined into the [www-project-threat-modeling-playbook](https://github.com/OWASP/www-project-threat-modeling-playbook) repo\r\n\r\nwe could then browse the pages in a similar manner to the [Secure Coding Practices](https://owasp.org/www-project-secure-coding-practices-quick-reference-guide/stable-en/) document",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-modeling-playbook/issues/1",
@@ -4600,10 +4759,11 @@
"pk": 160,
"fields": {
"nest_created_at": "2024-09-11T19:29:59.579Z",
- "nest_updated_at": "2024-09-11T19:29:59.579Z",
+ "nest_updated_at": "2024-09-13T15:08:50.458Z",
"node_id": "I_kwDOGDKlFs5mts0i",
"title": "provide pdf and epub",
"body": "We provide pdf and epub versions of the document in the [OWASP Developer Guide](https://owasp.org/www-project-developer-guide/) as part of the commit workflow\r\n\r\nThis can be done for the OTMP as well if this is useful",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-modeling-playbook/issues/2",
@@ -4630,6 +4790,7 @@
"node_id": "MDU6SXNzdWU5ODI3MjEwNDk=",
"title": "make frontend show Graph",
"body": "there's an experimental \"show CREs in a graph\" frontend page, hook it up to the relevant rest calls",
+ "summary": "The issue discusses the need to connect an experimental frontend page that displays CREs in a graph format with the appropriate REST API calls. To address this, the relevant integration between the frontend and the backend needs to be established.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/7",
@@ -4658,6 +4819,7 @@
"node_id": "MDU6SXNzdWU5ODI3NDE1NjE=",
"title": "Add pre-commit hooks",
"body": "add pre commit hooks for\r\n` black . `\r\n`mypy --strict --exclude venv/ . `\r\n`FLASK_APP=cre.py FLASK_CONFIG=test flask test | grep -i FAIL ` # there has to be a better way to ensure tests pass\r\n` if [[ $(FLASK_APP=cre.py FLASK_CONFIG=test flask test --coverage | grep TOTAL | awk '{print $6}' | tr -d \"%\" ) -lt 70 ]] then \r\n exit 1 \r\nfi` # there has to be a better way to ensure coverage \r\n\r\n",
+ "summary": "The issue discusses the need to implement pre-commit hooks for several tasks, including formatting code with `black`, type checking with `mypy`, running Flask tests, and checking test coverage. The author expresses a desire for more efficient methods to ensure tests pass and to verify code coverage meets a minimum threshold of 70%. To address this issue, the relevant pre-commit hooks should be created and optimized for the mentioned tasks.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/13",
@@ -4689,6 +4851,7 @@
"node_id": "MDU6SXNzdWU5ODI3Nzk4ODQ=",
"title": "Create production docker container",
"body": "start with distroless\r\nhttps://medium.com/@luke_perry_dev/dockerizing-with-distroless-f3b84ae10f3a",
+ "summary": "The issue requests the creation of a production Docker container using a distroless image. A reference article is provided for guidance on the process. To address this, one needs to follow the outlined steps in the article to properly configure and build the Docker container.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/15",
@@ -4715,6 +4878,7 @@
"node_id": "MDU6SXNzdWU5ODgzNDA2MzQ=",
"title": "Get rid of default LinkType:Same",
"body": "with then new linktypes introduced in issue: 29 there is no need for Same to be the default anymore, remove it",
+ "summary": "The issue suggests removing the default LinkType \"Same\" since new link types have been introduced that make it unnecessary. It is implied that adjustments to the code or configuration are needed to eliminate \"Same\" as the default option.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/34",
@@ -4741,6 +4905,7 @@
"node_id": "MDU6SXNzdWU5OTIwODMzNzE=",
"title": "Setup email forwarding in the domain to the whole team and change contact info on the landing page to info@opencre.org",
"body": "",
+ "summary": "The issue requests the setup of email forwarding for the entire team using the domain and updating the contact information on the landing page to reflect the new email address info@opencre.org. To address this, the necessary configurations for email forwarding should be implemented, and the landing page content should be updated accordingly.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/46",
@@ -4771,6 +4936,7 @@
"node_id": "MDU6SXNzdWU5OTIxNjEzMjI=",
"title": "Data: complete standard hyperlinks and names",
"body": "After having decided between v2 and v3:\r\nadd urls for CWE, OPC, NIST(Torsten) and WSTG, and nice names for the cheat sheets\r\n",
+ "summary": "The issue discusses the need to finalize the choice between v2 and v3 and subsequently add complete standard hyperlinks and appropriate names for various resources, including CWE, OPC, NIST, and WSTG cheat sheets. The next steps involve selecting the version and then updating the documentation with the required URLs and names.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/49",
@@ -4801,6 +4967,7 @@
"node_id": "MDU6SXNzdWU5OTM5MzQwMjQ=",
"title": "Docker Improvements",
"body": "build frontend\r\ninstall deps for python without building them from scratch\r\n\r\noffer production option where application runs in a distroless container\r\nimprove docker entrypoint to optionally run migrations on launch\r\ndocument required env vars to point to database outside container\r\n",
+ "summary": "The issue discusses several proposed improvements for the Docker setup of a project. Key points include building the frontend more efficiently, installing Python dependencies without building them from scratch, and offering a production option that utilizes a distroless container. Additionally, it suggests enhancing the Docker entrypoint to support optional migration execution on launch and documenting the necessary environment variables for connecting to an external database. Addressing these points will likely improve the overall efficiency and usability of the Docker implementation.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/65",
@@ -4831,6 +4998,7 @@
"node_id": "I_kwDOF9wO7c474T0Z",
"title": "Switch to new css framework",
"body": "## Issue\r\n\r\nCurrently, [Semantic-ui](https://semantic-ui.com/) is used as the main CSS framework. The library hasn't seen a new release/update since 2018. Not anything with priority but would be better to use a styling framework that is still supported and known, so a wider community has the ability to give the CRE project a boost :) .",
+ "summary": "The issue discusses the need to transition from the outdated Semantic-ui CSS framework, which has not been updated since 2018, to a more current and supported styling framework. This change would enhance community engagement and support for the project. It is suggested that switching to a well-known framework could benefit the project's development. Consider researching and evaluating alternative CSS frameworks for this transition.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/105",
@@ -4857,6 +5025,7 @@
"node_id": "I_kwDOF9wO7c480wFK",
"title": "Provide ability to link directly to a standard item through the url",
"body": "Provide ability to link directly to a standard item through the url, in two ways:\r\n1. eg opencre.org/123-456&deeplink=ASVS\r\nThis forwards the user from opencre, without rendering a CRE page, to the hyperlink that belongs to that standard\r\n2. eg opencre.org/bypasslink/asvs/v6.1.1/encrypt%20personal%data%at%rest\r\nThis also forwards to that asvs page based on our database AND it asks for a description of the topic. We collect those, so we can see what standard entries we apparently have no topic for (which would be the only reason to use method 2 instead of method 1. Stil that last argument is optional. ",
+ "summary": "The issue requests the implementation of a feature that allows users to link directly to a standard item via a URL in two distinct ways. The first method involves a simple forwarding to the standard's hyperlink without rendering a page. The second method not only forwards to a specific page but also prompts the user for a description of the topic, which can help identify gaps in the existing topic entries. To address this issue, the development team should consider implementing both linking methods as described.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/116",
@@ -4885,6 +5054,7 @@
"node_id": "I_kwDOF9wO7c482_D1",
"title": "Node.js API wrapper",
"body": "## User Story\r\n\r\n**As the** lead developer of OWASP Juice Shop\r\n**I want** to include a Node.js module that wraps the CRE API into a programmatically usable library\r\n**so that** I do not have to worry about putting together API calls manually\r\n**and** do not need to manually crawl JSON responses for the links I am interested in.\r\n\r\n### Expected Behaviour\r\n\r\nModule will be kept up-to-date with API changes.",
+ "summary": "The issue outlines a request to create a Node.js module that serves as a wrapper for the CRE API, enabling easier and more efficient interaction with the API by eliminating the need for manual API calls and JSON response parsing. The module should also be maintained to ensure it remains current with any changes to the API. To address this, a development plan for building and maintaining the module should be formulated.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/117",
@@ -4911,6 +5081,7 @@
"node_id": "I_kwDOF9wO7c4_DNE2",
"title": "Ingest owasp metadata for projects and make CRE mappings",
"body": "## Issue\r\nCRE data is nice for finding out what is the problem and what regulations it solves\r\n but it doesn't show how to test for it or actually solve it.\r\nMeanwhile owasp has exported data that tells you which projects exist and mostly, what they do.\r\nlet's combine the two\r\n\r\n### Expected Behaviour\r\nmake document type project\r\nmake frontend page project\r\ningest data from here https://raw.githubusercontent.com/OWASP/owasp.github.io/main/_data/projects.json\r\nassign to CREs\r\n????\r\nproffit\r\n\r\n### Actual Behaviour\r\nthe aboe doesn't exist\r\n",
+ "summary": "The issue discusses the need to integrate OWASP metadata for various projects with existing CRE (Common Vulnerabilities and Exposures) mappings. While CRE data helps identify problems and relevant regulations, it lacks guidance on testing and resolution. The proposal is to create a document type for projects, develop a frontend page, and ingest data from the provided OWASP projects JSON file to assign it to CREs. Currently, this functionality does not exist. \n\nTo move forward, the following tasks should be addressed: creating the project document type, developing the frontend page, and implementing the data ingestion and assignment process.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/126",
@@ -4942,6 +5113,7 @@
"node_id": "I_kwDOF9wO7c5CL1yw",
"title": "add SKF data",
"body": "## Issue\r\n\r\n### **What is the issue?**\r\n\r\nSKF has a knowledge base and code examples\r\nwe could add the relevant SKF knowledge base items (MASVS, ASVS and custom descriptions) to CRE.\r\nLet's do this ",
+ "summary": "The issue proposes adding relevant SKF knowledge base items, including MASVS, ASVS, and custom descriptions, to the CRE platform. To address this, the necessary SKF content should be identified and integrated into the system.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/145",
@@ -4970,6 +5142,7 @@
"node_id": "I_kwDOF9wO7c5C7XxK",
"title": "Auto data health checks",
"body": "once the auto-backups are in place\r\ncreate an action that downloads the latest database from heroku and compares it to the latest known good state.\r\nIf the known good state data isn't equivalent to the heroku ones, fail.\r\n\r\nThis might be possible by just dumping dbs, filtering out heroku custom things and checking for string equivalence",
+ "summary": "The issue discusses implementing automated data health checks following the establishment of auto-backups. The proposed solution involves creating an action that downloads the latest database from Heroku and compares it to the latest known good state. If discrepancies are found between the known good state and the Heroku data, the process should fail. It suggests that this could potentially be achieved by dumping the databases, filtering out Heroku-specific elements, and checking for string equivalence. \n\nTo move forward, the task would involve developing the action to facilitate this comparison and failure mechanism.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/149",
@@ -5000,6 +5173,7 @@
"node_id": "I_kwDOF9wO7c5D-qSb",
"title": "Browse topics feature is too hidden",
"body": "### **What is the issue?**\r\n\r\nThe show high level topics link is hidden in the text of the front page, whereas it should be a prominent feature - perhaps left of the search text with a button that says Browse topics, or perhaps just 'Topics'\r\nhttps://www.opencre.org/search/%3E%3E\r\n\r\nPerhaps we want to make it faster first (#154 )\r\n\r\n",
+ "summary": "The \"Browse topics\" feature is currently not prominently displayed on the front page, making it difficult for users to find. It is suggested that this feature be made more visible, possibly by placing it to the left of the search text with a clear button labeled \"Browse topics\" or simply \"Topics.\" Additionally, there is a mention of the need to improve speed, which may be addressed in a related issue. \n\nTo enhance user experience, consider redesigning the placement of the \"Browse topics\" link and ensuring it stands out on the page.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/155",
@@ -5026,6 +5200,7 @@
"node_id": "I_kwDOF9wO7c5EjnQx",
"title": "Add export functionality",
"body": "Based on OWASP/www-project-integration-standards#25:\r\n\r\n> Given a REST call, add the ability to export the results into a google sheets spreadsheet which allows humans to get the mappings into a CSV format. This is already partly implemented (`application/utils/spreadsheet.py`) but we haven't implemented any API plumbing for it.\r\n\r\nIn the FE, that could be implemented as a Button (see below).\r\nIt should handle CRE pages, standard pages and generic search pages.\r\n\r\n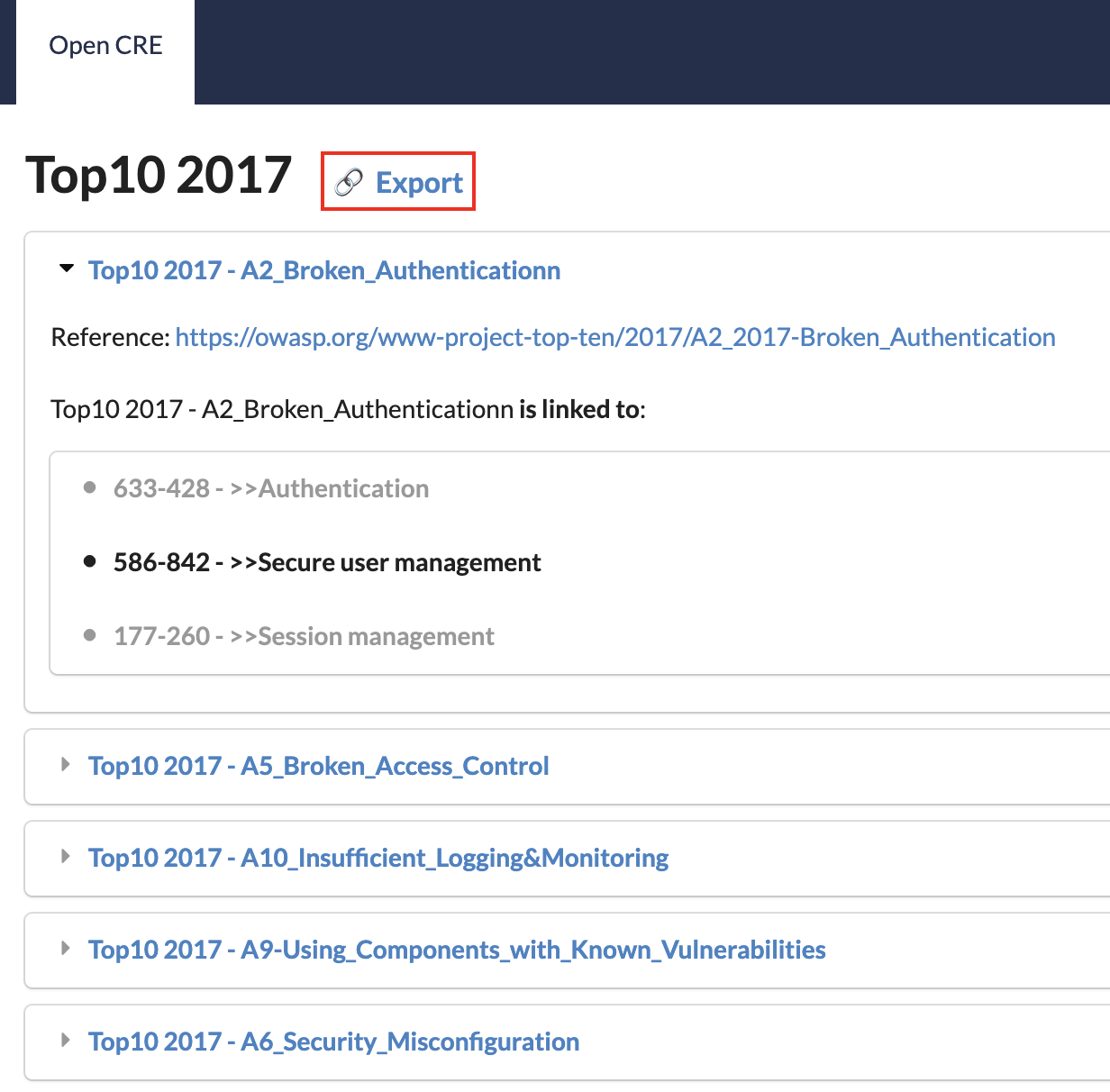",
+ "summary": "The issue requests the implementation of an export functionality that allows users to export REST call results into a Google Sheets spreadsheet, facilitating the conversion into CSV format. While there is partial implementation in `application/utils/spreadsheet.py`, additional API integration is needed. The front end should include a button to trigger this functionality, applicable for CRE pages, standard pages, and generic search pages. The next steps involve completing the API plumbing and designing the button for the user interface.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/161",
@@ -5052,6 +5227,7 @@
"node_id": "I_kwDOF9wO7c5F-pVX",
"title": "Add a browse button",
"body": "Add a browse button with the same styling as the search button and with a little bullet list as an icon. Put it right of the \"Open CRE\" tab and let it link to the root CREs - when that one is fixed.",
+ "summary": "The issue requests the addition of a browse button styled similarly to the search button, featuring a bullet list icon. This button should be positioned to the right of the \"Open CRE\" tab and should link to the root CREs once resolved. Implementation of this feature is needed.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/180",
@@ -5080,6 +5256,7 @@
"node_id": "I_kwDOF9wO7c5Gig7y",
"title": "Clean up topic page and make more clear",
"body": "Based on analysis and interviews, the following low hanging fruit has been identified to make the topics page more clear and lean:\r\n-Remove “tags: “line\r\n-Remove “CRE:xxx-xxx”: at the beginning of every line\r\n-Also remove “CRE:” prefix (instead of “CRE: >>Secure data storage” say “Secure data storage” because we should not call topics “CRE’s”. It’s not forbidden to do so, but it’s jargon.\r\n-Move “bla bla contains” block more to the top, below the “is linked to” block\r\n-For topic pages: change “is linked to” to “refers to”\r\n-For standard pages: change “is linked to” to “is referenced by”\r\n-Remove xxx-xxx Before every hyperlink to a CRE topic: Instead of “163-776 Backups”, write “Backups”\r\n-If easy to do: indent all border blocks so that titles stand out more clear as separators of content: “Bla bla related to”\r\n-By default: collapse topics that are ‘related to’\r\n-Change “is part of” to “is child of”:\r\n-Add a hyperlink icon next to references on a topic page, so you get taken directly to the content",
+ "summary": "The issue suggests several improvements to enhance clarity and simplicity on the topics page. Key recommendations include removing unnecessary prefixes and jargon, adjusting the placement of certain blocks, and changing specific terminology for consistency. Additionally, it proposes visual adjustments like indenting border blocks and collapsing related topics by default. Finally, adding a hyperlink icon next to references is also suggested for easier navigation. Implementing these changes would lead to a more user-friendly experience.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/186",
@@ -5108,6 +5285,7 @@
"node_id": "I_kwDOF9wO7c5Gkk9r",
"title": "Add topic descriptions",
"body": "Add a description field to topics in the UI and in the data. example: HTTP security headers: \"Configuration of the web server putting special information in the http communication, for example content security policies\"",
+ "summary": "The issue proposes adding a description field to topics within the user interface and data. This would provide additional context for each topic, like the example given for HTTP security headers, which explains its purpose and configuration. Implementing this feature would enhance user understanding of various topics.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/187",
@@ -5136,6 +5314,7 @@
"node_id": "I_kwDOF9wO7c5Gl-qa",
"title": "'Wrong secrets' seems empty because doesn't expand",
"body": "When arriving at the Wrong secrets project, the title doesn't expand, so the hyperlink is not shown and users may believe they are at a dead end.\r\nhttps://www.opencre.org/node/tool/OWASP%20WrongSecrets/",
+ "summary": "The issue highlights that the \"Wrong secrets\" project title does not expand, resulting in the hyperlink not being visible. This could lead to users thinking they have reached a dead end. To address this, it is suggested to ensure that the project title is properly expandable to display the hyperlink.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/188",
@@ -5166,6 +5345,7 @@
"node_id": "I_kwDOF9wO7c5HJZZs",
"title": "CRE does not contain the Att&ck framework",
"body": "## Issue\r\n\r\n### **What is the issue?**\r\n\r\nWe are missing the Att&ck framework, it could be used to add more CREs and easily map more tools, code snippets etc.\r\nShould be relatively easy to import via https://attack.mitre.org/resources/working-with-attack/",
+ "summary": "The issue highlights the absence of the ATT&CK framework within the current system, which limits the ability to incorporate additional CREs and effectively map tools and code snippets. To address this, it is suggested to import the framework using the resources available on the MITRE ATT&CK website.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/192",
@@ -5192,6 +5372,7 @@
"node_id": "I_kwDOF9wO7c5HJZ2a",
"title": "CRE does not contain the def3nd framework",
"body": "## Issue\r\n\r\n### **What is the issue?**\r\nwhat the title says",
+ "summary": "The issue states that the CRE does not include the def3nd framework. To address this, the framework needs to be integrated into the CRE.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/193",
@@ -5218,6 +5399,7 @@
"node_id": "I_kwDOF9wO7c5Hct5v",
"title": "Provide Trusted Contributors with the ability to import data in opencre.org",
"body": "## Issue\r\n\r\n### **What is the issue?**\r\n\r\nCurrently, new data in opencre.org can be inputted by way of a code-maintainer running an import script.\r\nThis is inefficient as it greatly restricts who can import data and forces a hard coupling between code maintainers and data authors.\r\n\r\n\r\n### Expected Behaviour\r\n\r\nData authors should have a strongly authenticated UI which allows them to easily mass-import data into opencre.org\r\nThey should also be given the freedom to either manipulate or replace individual mappings.\r\nHowever, special care should be taken to preserve CRE immutability.\r\n\r\n### Possible implementations:\r\n#### Admin panel connected to an idp\r\n\r\nThere is an admin page which uses open id connect and an external identity provider (e.g. okta) to authenticate a data author.\r\nData authors could provide an email address which when added to an identity provider solution gives them access to said admin panel, from there they can either provide the url of a spreadsheet that fits the [mapping template](https://docs.google.com/spreadsheets/d/1f47aZydJ47n-iGb0fkmu880wSaFyYDM-zdkgs6oMV7I/edit?usp=sharing) and the backend runs the equivalent of `python cre.py --add --from-spreadsheet `\r\n\r\nPros: industry standards solution, on-prem users can hook their own idp\r\nCons: needs an external idp solution, maintenance burden.\r\n\r\n\r\n",
+ "summary": "The issue outlines the need to enhance the data import process for opencre.org by allowing Trusted Contributors to import data directly through a user-friendly interface. Currently, only code maintainers can run the import script, which limits flexibility and creates unnecessary dependency between maintainers and data authors.\n\nThe expected behavior is to create a secure, authenticated UI for data authors to mass-import data, with options to manipulate or replace individual mappings while ensuring the integrity of CRE immutability.\n\nOne proposed implementation involves developing an admin panel that integrates with an identity provider (IDP) for authentication. Data authors could access this panel and submit a spreadsheet URL that adheres to a specified mapping template, enabling automated data import.\n\nTo move forward, additional implementation alternatives are needed that might address the cons of using an external IDP while still meeting the project's requirements.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/194",
@@ -5244,6 +5426,7 @@
"node_id": "I_kwDOF9wO7c5Hdy_x",
"title": "Create an automated parser for the core rule set",
"body": "## Issue\r\nThere is no automatic way to import core rule set rules\r\nCRS project exports their rules in JSON .\r\nWe should be parsing this and auto-exporting them.\r\n",
+ "summary": "The issue highlights the absence of an automated method to import the core rule set rules, which are currently exported in JSON format. The task involves creating a parser that can read these JSON files and facilitate the auto-exporting of the rules. A link to the CRS project is mentioned for reference. Development of this parser is essential to streamline the import process.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/196",
@@ -5272,6 +5455,7 @@
"node_id": "I_kwDOF9wO7c5Imsfi",
"title": " Make frontend show gap analysis",
"body": "## Issue\r\nWe have a functional gap analysis feature in the backend, let's build a frontend page which can show it.\r\nThis will need significant thinking and design on presentation before we get to building\r\n",
+ "summary": "The issue involves creating a frontend page to display the existing functional gap analysis feature from the backend. It requires careful consideration and design regarding how the information will be presented before proceeding with the development. A design plan should be drafted to guide the implementation.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/203",
@@ -5300,6 +5484,7 @@
"node_id": "I_kwDOF9wO7c5ImtCH",
"title": "Make frontned able to import from spreadsheet template.",
"body": "## Issue\r\nchild of #194 , the trusted contributor import page should allow contributors to import from a spreadsheet template for mappings that don't have parsers yet",
+ "summary": "The issue discusses enhancing the frontend to enable contributors to import data using a spreadsheet template. This feature is aimed at facilitating the import process for mappings that currently lack parsers. To address this, the implementation of a user-friendly import function for the specified template is needed.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/204",
@@ -5326,6 +5511,7 @@
"node_id": "I_kwDOF9wO7c5ImtM8",
"title": "make pagination also for tag results",
"body": "## Issue\r\nget by tag is not paginated, we don't have a lot of tags yet so for now its performant, but paginate it so that it's faster with more data",
+ "summary": "The issue addresses the lack of pagination for results retrieved by tags. While current performance is acceptable due to the limited number of tags, it is suggested to implement pagination to enhance efficiency as the data grows. The recommended action is to add pagination functionality for tag results.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/205",
@@ -5352,6 +5538,7 @@
"node_id": "I_kwDOF9wO7c5LRihE",
"title": "auto parse CWE",
"body": "## Issue\r\n\r\n### **What is the issue?**\r\nCWE is a framework which is meant to be parsed automatically, however, we add the mappings manually from a spreadsheet.\r\nParse CWE from here https://cwe.mitre.org/data/downloads.html\r\n",
+ "summary": "The issue revolves around the need to automate the parsing of the Common Weakness Enumeration (CWE) framework instead of manually adding mappings from a spreadsheet. The proposed solution is to retrieve and parse the CWE data from the provided link. Action is required to implement this automated parsing process.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/219",
@@ -5380,6 +5567,7 @@
"node_id": "I_kwDOF9wO7c5XVVeu",
"title": "Get User Feedback and perform usability research for the frontend",
"body": "Our frontend works but that's about it. We are presenting quite a lot of data in a not very organised way.\r\nWe could benefit from data-presentation architecture.\r\n\r\nA good way to do this would be to talk to potential users and get feedback on what a good frontend would look like",
+ "summary": "The issue highlights the need for improved organization and presentation of data in the frontend. To enhance usability, it suggests conducting user feedback sessions to gather insights on what an effective frontend should entail. Engaging with potential users will help identify specific areas for improvement and inform the design of a more user-friendly interface. It is recommended to initiate user interviews or surveys to collect this valuable feedback.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/241",
@@ -5406,6 +5594,7 @@
"node_id": "I_kwDOF9wO7c5XVVg9",
"title": "Redesign frontend based on user feedback",
"body": "after #241 is done we should action this user feedback by redesigning our frontend appropriately",
+ "summary": "The issue discusses the need to redesign the frontend in response to user feedback, which should be addressed after the completion of a related task (#241). Actioning the feedback will involve making appropriate changes to enhance the user interface.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/242",
@@ -5432,6 +5621,7 @@
"node_id": "I_kwDOF9wO7c5XVWac",
"title": "add filtering to the frontend",
"body": "currently, the only way to do filtering is to know the exact url you need to input, which is not great ux, a good idea would be to add a drop down next to the search button that somehow allows constructing the filtering query from the frontend",
+ "summary": "The issue suggests enhancing the user experience by adding a filtering feature to the frontend. Currently, users must know the exact URL for filtering, which is not user-friendly. The proposed solution is to implement a dropdown next to the search button that allows users to construct filtering queries more intuitively. It would be beneficial to explore options for this dropdown implementation.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/243",
@@ -5458,6 +5648,7 @@
"node_id": "I_kwDOF9wO7c5XVWgP",
"title": "Add gap analysis capability to the frontend",
"body": "currently, our backend allows for performing gap analysis exercises between any two standards we support.\r\nwe should:\r\n1. revisit the implementation with some GRC people and validate that our implementation of gap analysis is correct.\r\n2. add a frontend feature that allows for gap analysis to be performed from the frontend",
+ "summary": "The issue suggests enhancing the frontend to include gap analysis capabilities, which are currently available only through the backend. The proposed steps include validating the existing gap analysis implementation with GRC experts and developing a frontend feature to enable users to perform gap analysis directly from the user interface.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/244",
@@ -5486,6 +5677,7 @@
"node_id": "I_kwDOF9wO7c5ktiub",
"title": "Create an API endpoint which given a Standard JSON, suggests which CREs are the most likely mappings for this standard section",
"body": "As an opencre or standard maintainer, I want to be able to import new standards or other security information very quickly in the CRE graph.\r\nGiven I have this information in a [CRE Standard](https://github.com/OWASP/common-requirement-enumeration/blob/main/application/defs/cre_defs.py#L384) format, I need to find which CRE is best suited for linking with a specific section of my standard.\r\nIn order to do that I need an API call POST [opencre.org/rest/v1/link/suggest](http://opencre.org/rest/v1/link/suggest) where the body of the api call is a CRE Standard in json format.\r\nOpenCRE then needs to reply with a json array of CREs that are best suited to match my standard.\r\ne.g.\r\n POST [opencre.org/rest/v1/link/suggest](http://opencre.org/rest/v1/link/suggest)\r\n\r\n```{\"doctype\":\"Standard\",\"hyperlink\":\"https://github.com/OWASP/ASVS/blob/v4.0.2/4.0/en/0x11-V2-Authentication.md\",\"links\":[],\"name\":\"ASVS\",\"section\":\"Verify that the challenge nonce is at least 64 bits in length, and statistically unique or unique over the lifetime of the cryptographic device.\",\"sectionID\":\"V2.9.2\"}]```\r\n\r\nResponse:\r\n\r\n``` [{\"doctype\":\"CRE\",\"id\":\"287-251\",\"name\":\"Use a unique challenge nonce of sufficient size\",\"tags\":[\"Cryptography\"]},{\"doctype\":\"CRE\",\"id\":\"287-252\",\"name\":\"Some other CRE\",\"tags\":[\"Cryptography\"]}] ```\r\n\r\n",
+ "summary": "The issue requests the creation of an API endpoint that takes a standard JSON object and suggests the most suitable Common Requirement Enumerations (CREs) for a specific section of that standard. The endpoint should be a POST request to `opencre.org/rest/v1/link/suggest`, with the body containing the CRE Standard in JSON format. The expected response is a JSON array of recommended CREs that align with the provided standard section. Implementation of this functionality would facilitate quicker integration of new standards into the CRE graph.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/275",
@@ -5512,6 +5704,7 @@
"node_id": "I_kwDOF9wO7c5k3WTA",
"title": "By default: collapse linked sources if they contain more than x (say 5) entries",
"body": "By default collapsed linked sources if they contain more than x (say 5) entries\r\nTo avoid clutter",
+ "summary": "The issue suggests implementing a feature to automatically collapse linked sources when they contain more than a specified number of entries (e.g., 5) to reduce visual clutter. A possible action would be to set up a default threshold for collapsing these sources within the application.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/283",
@@ -5544,6 +5737,7 @@
"node_id": "I_kwDOF9wO7c5l1vzb",
"title": "create api call which takes a standard object and suggests which CRE it should match to",
"body": "currently adding mappings requires the new standard to either map to an existing standard or manual mapping.\r\nthis is inefficient, we can use embeddings to automatically find which CRE each standard section links to .\r\n\r\ndesign an api call which when you POST a standard document with it's section content, it suggests which CRE it should map to\r\n\r\n(preparation for ability to add mappings)",
+ "summary": "The issue discusses the need to create an API call that takes a standard object and suggests the appropriate CRE (Common Regulatory Element) it should match to. Currently, the process of adding mappings is inefficient, requiring either a link to an existing standard or manual input. The proposal involves utilizing embeddings to automatically identify connections between standard section content and CREs. The task is to design an API endpoint that, when a standard document is posted, will provide suggestions for mapping to the relevant CRE. This development is crucial for streamlining the mapping process.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/285",
@@ -5574,6 +5768,7 @@
"node_id": "I_kwDOF9wO7c5oZWJq",
"title": "create swagger or .proto definition api",
"body": "## Issue\r\n\r\n### **What is the issue?**\r\n\r\nas opencre.org is getting adoption we receive an increasing number of requests for SDKs, Clients or other API integrations.\r\nWe currently do not have any way of integrating OpenCRE with the outside world other than its undocumented API or the frontend. If we document our API we will be able to use Open APIs code generation capabilities to create clients.\r\n\r\n### Expected Behaviour\r\n\r\nThere is a formal definition of our api in either .proto or swagger files.\r\nThese files can be used to generate clients.\r\n",
+ "summary": "The issue highlights the need for a formal definition of the OpenCRE API, as the current undocumented API limits external integration and client generation. To address this, the suggestion is to create either Swagger or .proto files for the API. This documentation will enable the generation of SDKs and clients, facilitating improved adoption and integration with OpenCRE. \n\nNext steps involve defining the API specifications in the chosen format (Swagger or .proto).",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/295",
@@ -5605,6 +5800,7 @@
"node_id": "I_kwDOF9wO7c5rqXY7",
"title": "[BUG] pci dss repeats section id in section",
"body": "the parser for the pci dss standard repeats the section id in the section name field",
+ "summary": "There is a bug in the PCI DSS parser where the section ID is being duplicated in the section name field. To resolve this issue, the parsing logic should be reviewed and adjusted to ensure that the section ID is not repeated in the output.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/328",
@@ -5635,6 +5831,7 @@
"node_id": "I_kwDOF9wO7c5s4wVF",
"title": "[Feature request] Create a flexible endpoint for documents",
"body": "**Current behaviour**\r\n\r\nThere is currently an endpoint `/rest/v1/id/xxx-xxx` in the application that is responsible for a particular CRE instance. Such an instance has a unique identifier, which is a pair of three-digit numbers separated by a dash. Within the body of this instance there are links to other CREs and documents. If we want to get any linked CRE instance, we can easily get it using the above mentioned endpoint via its id.\r\n\r\n**Problem**\r\n\r\nThis approach allows us to easily move from parent to child CRE instances and vice versa, but it doesn't include other many-to-many relationships, i.e. between CRE and linked document. It doesn't allow us to get a lot of important information, such as\r\n- documents of a certain type for a given CRE\r\n- all CREs linked to this document\r\netc.\r\n\r\n**Feature Request**\r\n\r\nUsing queryset parameters, we could easily solve both problems with the following requests:\r\n`/rest/v1/document/?CRE=xxx-xxx` solves the first problem and\r\n`/rest/v1/document/?CRE=xxx-xxx&doctype=Standard` solves the second. \r\n\r\nSo the request is to combine all documents within an endpoint and add the ability to filter document instances using the queryset parameter.\r\nThis would solve the problem mentioned in the https://github.com/OWASP/common-requirement-enumeration/issues/285 ticket and add other important functionality. ",
+ "summary": "The issue discusses the limitations of the current endpoint `/rest/v1/id/xxx-xxx`, which allows for easy navigation between CRE instances but does not support many-to-many relationships with linked documents. It highlights the need for a more flexible endpoint that can provide access to documents related to a specific CRE as well as the CREs associated with a particular document. \n\nThe proposed solution is to create a new endpoint `/rest/v1/document/` that utilizes queryset parameters to filter documents by CRE and document type. Implementing this feature would enhance functionality and address the concerns raised in a related issue.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/334",
@@ -5661,6 +5858,7 @@
"node_id": "I_kwDOF9wO7c5w-BEQ",
"title": "Better inform users on chatbot privacy",
"body": "Better inform users on chatbot privacy -\r\nwhile users log in, before they get shown the login, and when using it should be clear that:\r\n-we only need them to login to maximize the number of queries per minute per unique user\r\n-their account is not used to authenticate with the Large Language Model\r\n-only their prompt is sent\r\n-see the info on the chatbot page on this\r\n-maybe we should link to the privacy policy of the PALM LLM\r\n-we also need to update our privacy policy to reflect this info. The google SSO refers to it\r\n\r\nIt's best to first show a page when people arrive at /chatbot and have no session: describing the above, saying welcome to OpenCRE chat, and then a link to login, taking you to google SSO.\r\n\r\nSo basically a piece of text to show on that landing page, in the privacy policy and on the chatbot page.\r\n\r\nThen somehow we need to deal with what google says in the SSO: \"To continue, Google will share your name, email address, language preference, and profile picture with opencre.org\" Either we need to change some settings, change that text, or refer to it in OUR text: despite that google sends us your name and mail address, we don't store it. Preferably we pick an SSO method that does not send it al all, or rather an alternative to google sso?",
+ "summary": "The issue highlights the need for improved user communication regarding chatbot privacy. It suggests that users should be informed about the login process and the data being handled, specifically that login is only for query management, no user account data is used for authentication with the Large Language Model, and only user prompts are sent. Recommendations include creating a dedicated landing page for first-time users, updating the privacy policy, and linking to the PALM LLM privacy policy. Additionally, the issue raises concerns about the information shared by Google during the SSO process and suggests considering alternative authentication methods that do not share personal data. To address these points, a clear informational text should be developed for the landing page, chatbot page, and privacy policy.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/375",
@@ -5689,6 +5887,7 @@
"node_id": "I_kwDOF9wO7c5xBKXd",
"title": "Have a separate UI element to enter language request, to prevent requests in the prompt to bother the matching",
"body": "Have a separate UI element to enter language request, to prevent requests in the prompt to bother the matching.\r\nIf you ask 'Answer in Chinese how I should prevent command injection' you get a worse opencre match than when you ask 'How should I prevent command injection'.\r\nProbably because we calculate the embedding over the entire user prompt.\r\nWe can do two things:\r\n1. add a text box with the label 'Language of Answer' and put English in it by default, then use that in constructing the final prompt\r\n2. add a text box with the label 'Instructions' and put there by default 'Anwer in English'. People can change that to 'Answer in layman terms in German' for example. This is the nicest I believe",
+ "summary": "The issue discusses the need for a separate UI element to specify language requests in order to avoid interfering with the main prompt used for matching. Currently, including language requests within the prompt negatively impacts the quality of the matching. The proposed solutions include adding a dedicated text box labeled 'Language of Answer', defaulting to English, or creating a text box labeled 'Instructions' with a default prompt of 'Answer in English', allowing users to customize their requests. Implementing one of these solutions could improve the matching process.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/376",
@@ -5717,6 +5916,7 @@
"node_id": "I_kwDOF9wO7c5xN7v9",
"title": "List supported standards and documents",
"body": "## Issue\r\n[From Sam Stepanyan]\r\nIt would be convenient to know which standards and in general documents are supported by opencre\r\nthis helps both with chatbot queries but also general knowledge of what OpenCRE supports\r\n\r\nImplementation wise this is a simple query to select unique names from nodes and a frontend page to present this somehow",
+ "summary": "The issue suggests the need for a comprehensive list of supported standards and documents by OpenCRE to enhance user knowledge and assist with chatbot queries. It proposes a straightforward implementation involving a query to select unique names from nodes, followed by the creation of a frontend page for presentation. To move forward, the team should focus on developing this query and designing the frontend display.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/383",
@@ -5743,6 +5943,7 @@
"node_id": "I_kwDOF9wO7c5zHX82",
"title": "Create a Product Security Wayfinder component",
"body": "## Issue\r\nWe created the first Wayfinder manually here https://owasp.org/projects/\r\nIt's cool, the community has embraced it but it's manual and currently sits in a shared drive.\r\n\r\n\r\n### Expected Behaviour\r\n\r\nAs an OpenCRE.org user I can browse a graphical way of exploring tools and standards known to OpenCRE by grouping them on which part of the SDLC they map to. Similar to the wayfinder above.\r\n\r\nI can filter the new wayfinder by selecting supporting organizations, licenses or any other Key from the metadata fields.\r\n\r\n### Actual Behaviour\r\n\r\nWe have the data to automate the creation of the wayfinder, let's do so.\r\n\r\nTo achieve this we need to\r\n* grab unique names from every non-cre node in our database \r\n* enrich them with metadata such as SDLC steps they fit into\r\n* find a way to automatically provide this info on import (e.g. by modifying our importers or creating a static map to start and then require projects to add this info in their self contained links)\r\n* present this info in a frontend in a nice way allowing for filtering based on supporting org, license, and other metadata we may have\r\n",
+ "summary": "The issue discusses the need to create an automated Product Security Wayfinder component, which would enhance the current manual version hosted on a shared drive. The expected functionality includes a graphical interface for users to explore tools and standards related to OpenCRE, organized by their relevance in the Software Development Life Cycle (SDLC). Users should be able to filter options based on metadata such as supporting organizations and licenses.\n\nTo implement this, the following steps are proposed:\n- Extract unique names from non-CRE nodes in the database.\n- Enrich this data with relevant metadata.\n- Automate the information import process, possibly by modifying existing importers or creating a static initial mapping.\n- Develop a user-friendly frontend to present the data and allow for filtering based on various metadata attributes.\n\nThe next steps should focus on gathering the necessary data and developing the automated processes for the Wayfinder.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/426",
@@ -5769,6 +5970,7 @@
"node_id": "I_kwDOF9wO7c52k3nR",
"title": "Mapping issue: NIST SSDF to NIST SP 800-53",
"body": "## Issue\r\n\r\n### **What is the issue?**\r\n\r\nThe mapping from NIST SSDF PO.1.2 (Identify and document all security requirements) to SP800-53 gives SC-18 Mobile Code as the only Direct mapping. This doesn't seem correct.\r\n\r\n### Expected Behaviour\r\n\r\nI don't have extensive knowledge of 800-53, but I would think SA-8 is a closer match for instance.\r\n",
+ "summary": "The issue raised concerns the mapping of NIST SSDF PO.1.2, which focuses on identifying and documenting all security requirements, to NIST SP 800-53. Currently, SC-18 Mobile Code is the only direct mapping provided, which is being questioned as potentially incorrect. The expectation is that SA-8 might be a more appropriate match. \n\nTo address this, a review of the mappings between SSDF and SP 800-53 is necessary to ensure accuracy and completeness.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/446",
@@ -5797,6 +5999,7 @@
"node_id": "I_kwDOF9wO7c52q6ox",
"title": "Mapping issue: Missing a reference to the OWASP DoS cheat sheet",
"body": "## Issue\r\n\r\n### **What is the issue?**\r\n\r\nWhen I type \"denial of service\" in the search field on opencre.org I cannot find a reference to the OWASP Denial of Service cheat sheet - https://cheatsheetseries.owasp.org/cheatsheets/Denial_of_Service_Cheat_Sheet.html\r\n\r\n### Expected Behaviour\r\n\r\nThe OWASP Denial of Service cheat sheet link should appear as a reference.\r\n\r\n### Actual Behaviour\r\n\r\nSeveral other references appear on the results page (e.g. ASVS, other OWASP cheat sheets, CAPEC, NIST, CWE, etc) but no link to the OWASP DoS cheat sheet.\r\n\r\n### Steps to reproduce\r\n\r\nVisit opencre.org and type in \"denial of service\" in the search field and then click Search.",
+ "summary": "A mapping issue has been identified regarding the absence of a reference to the OWASP Denial of Service (DoS) cheat sheet when searching for \"denial of service\" on opencre.org. Currently, other relevant references appear in the search results, but the specific link to the OWASP DoS cheat sheet is missing. To address this issue, the OWASP DoS cheat sheet should be added to the search results to ensure it is accessible to users.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/449",
@@ -5823,6 +6026,7 @@
"node_id": "I_kwDOF9wO7c53ZL51",
"title": "Use 'Requirement' instead of CRE",
"body": "New visitors don't know what a CRE is. Besides it's common requirement ENUMERATION. So it should in fact read CR. Let's call it Requirement everywhere. To start with at the places where we say \"Which contains CREs\" and \"Which is related to CREs\"",
+ "summary": "The issue suggests replacing the term \"CRE\" with \"Requirement\" for clarity, as new visitors may not understand what CRE means. It proposes to use \"Requirement\" consistently throughout the documentation, starting with locations that reference \"Which contains CREs\" and \"Which is related to CREs.\" The necessary action includes updating these specific phrases in the documentation to reflect the new terminology.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/453",
@@ -5849,6 +6053,7 @@
"node_id": "I_kwDOF9wO7c537Y2P",
"title": "Add arguments to cre endpoint to support the standard integration and metrics",
"body": "When a standard like WSTG links to OpenCRE's, we at least can ask them to add an argument saying 'source=bla' where bla needs to be some recognizable name for the standard - perhaps an encoded name, or something else that may be less likely to change over time.\r\nThat way we can keep count.\r\nAlso: do we need more info that will help us in parsing? I guess not because for WSTG we'll use the content of the page to parse the title and reconstruct the hyperlink, right?",
+ "summary": "The issue suggests enhancing the 'cre' endpoint by adding an argument for tracking integration with standards like WSTG. Specifically, it proposes including a 'source' parameter with a recognizable name for the standard to facilitate counting. Additionally, it questions whether more information is needed for parsing, noting that for WSTG, the page content will be used to parse the title and reconstruct hyperlinks. To address this issue, the team should consider implementing the 'source' argument and evaluate if additional parsing information is necessary.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/455",
@@ -5877,6 +6082,7 @@
"node_id": "I_kwDOF9wO7c55zvZ8",
"title": "Map Analysis UI Clunkiness",
"body": "## Issue\r\n\r\n### **What is the issue?**\r\n\r\nWhen browsing a list of standards that map to a single standard and hovering over one, the info box that pops up covers the next few in the list. This makes quickly browsing through standards in the same section clunky as you need to move the mouse away until the box disappears before you can hover over the next standard. \r\n\r\n\r\n\r\n### Expected Behaviour\r\n\r\nIdeally the hover-over info box would be offset to the right/left so that the following or preceding standards aren't covered.\r\n\r\n### Actual Behaviour\r\n\r\nDescribed above.\r\n\r\n### Steps to reproduce\r\n\r\nDescribed above.",
+ "summary": "The issue pertains to the clunkiness of the Map Analysis UI, specifically regarding the hover-over info box that appears when browsing standards. The current implementation causes the info box to obstruct subsequent items in the list, hindering quick navigation. The expected behavior is for the info box to be offset to prevent it from covering adjacent standards. To resolve this, adjustments need to be made to the positioning of the hover-over info box.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/456",
@@ -5905,6 +6111,7 @@
"node_id": "I_kwDOF9wO7c57bO9U",
"title": "Add CWE titles",
"body": "Currently we only have CWE ids. For users it is often handy to see what weakness is linked without having to click on it. In Mapanalysis especially.\r\nWe have titles (Sectionnames) for many standards.\r\nI think we used to have the titles.\r\n",
+ "summary": "The issue highlights the need to add Common Weakness Enumeration (CWE) titles alongside the existing CWE IDs to enhance user experience, particularly in the Mapanalysis tool. It suggests that having the titles readily visible would eliminate the need for users to click to find out what each weakness refers to. It is also noted that titles for many standards are already available, implying that reinstating the CWE titles should be feasible. To address this, the implementation of CWE titles should be considered.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/468",
@@ -5931,6 +6138,7 @@
"node_id": "I_kwDOF9wO7c57bPiM",
"title": "Add OpenCRE itself to mapanalysis",
"body": "It would be good to have opencre be one of the standards in the map analyis. it can be helpful to have a opencre-samm overview for example. \r\n",
+ "summary": "The issue suggests incorporating OpenCRE into the map analysis framework, highlighting its potential as a standard tool. It specifically mentions the need for an overview of OpenCRE in relation to SAMM. To address this, the implementation of OpenCRE within the map analysis system is recommended.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/469",
@@ -5959,6 +6167,7 @@
"node_id": "I_kwDOF9wO7c57bP3e",
"title": "Return hyperlinks from mapanalysis REST endpoint",
"body": "See title",
+ "summary": "The issue requests the implementation of returning hyperlinks from the mapanalysis REST endpoint. To address this, the development team should consider modifying the existing endpoint to include the necessary hyperlink data in its response.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/470",
@@ -5985,6 +6194,7 @@
"node_id": "I_kwDOF9wO7c57bQO0",
"title": "Mapping: to more owasp resources",
"body": "Link to resources such as https://owasp.org/www-community/attacks/Path_Traversal ",
+ "summary": "The issue suggests enhancing the current documentation by adding links to more OWASP resources, specifically mentioning the Path Traversal attack page. It would be beneficial to identify additional relevant OWASP resources to include in the mapping for improved guidance.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/471",
@@ -6013,6 +6223,7 @@
"node_id": "I_kwDOF9wO7c57bQhL",
"title": "Mapping: Map to the rest of CWE",
"body": "6Could we map the rest of CWE, such as 652-query injection linking to injection?",
+ "summary": "The issue discusses the need to map additional Common Weakness Enumeration (CWE) entries, specifically suggesting the inclusion of CWE-652 (query injection) under the broader category of injection vulnerabilities. The action required involves expanding the current mapping to ensure all relevant CWEs are properly linked.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/472",
@@ -6041,6 +6252,7 @@
"node_id": "I_kwDOF9wO7c5-pggV",
"title": "Make smartlink go to CRE directly",
"body": "If you smartlink for example with CWE 611 and there's only one CRE connected, it should jump straight to that CRE and show the wealth of information. That CRE page has all the information that the current smartlink landing page has, so.\r\n[https://www.opencre.org/smartlink/standard/CWE/611](https://www.opencre.org/smartlink/standard/CWE/611)",
+ "summary": "The issue requests that the smartlink feature be updated so that when there is only one connected CRE (Common Related Entity) for a specific CWE (Common Weakness Enumeration), it should redirect users directly to that CRE's page. The current landing page for smartlinks contains the same information as the CRE page, making this direct navigation more efficient. To resolve this, the functionality for smartlink should be modified to check the number of connected CREs and redirect accordingly.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/486",
@@ -6069,6 +6281,7 @@
"node_id": "I_kwDOF9wO7c6BB3rY",
"title": "Try alternative graph database",
"body": "## Issue\r\n\r\n### **What is the issue?**\r\n\r\nGap analysis calculation takes a few hours if we run it on a beefy machine. The bottleneck is neo4j being slow.\r\nWe should investigate alternatives.\r\nAn alternative that seems promising and works with our existing database is [Apache Age](https://age.apache.org/age-manual/master/intro/setup.html)\r\n\r\nAge runs on top of our current database (postgres) and allows for Cypher queries on top of postgres.\r\nI'm being told it is faster than neo",
+ "summary": "The issue highlights that the gap analysis calculation is significantly slow when using Neo4j, taking several hours on a powerful machine. As a potential solution, the suggestion is to explore alternatives, particularly Apache Age, which operates on PostgreSQL and supports Cypher queries. It is reported to be faster than Neo4j. The next step involves investigating the feasibility and performance of implementing Apache Age as a replacement for Neo4j to improve the calculation speed.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/488",
@@ -6098,6 +6311,7 @@
"node_id": "I_kwDOF9wO7c6Cg4z-",
"title": "Numpy 1.23 does not support python-3.11",
"body": "\r\n### Numpy and Python version mismatch\r\n\r\nNumpy 1.23 does not support python 3.11 so when running `pip install -r requirements.txt` the process fails.\r\nDocs: https://numpy.org/devdocs/release/1.23.1-notes.htmlhttps://numpy.org/devdocs/release/1.23.1-notes.html (the 1.23.0 notes don't mention explicitly that supported version but I don't expect it to support more than the 1.23.1 version)\r\n\r\n### Expected Behaviour\r\n\r\nCan install packages with recommended Python version.\r\n\r\n### Actual Behaviour\r\n\r\n```\r\npip install -r requirements.txt\r\n...\r\nnote: This error originates from a subprocess, and is likely not a problem with pip.\r\n ERROR: Failed building wheel for numpy\r\nFailed to build numpy\r\nERROR: Could not build wheels for numpy, which is required to install pyproject.toml-based projects\r\n```\r\n\r\n### Steps to reproduce\r\n\r\nCreate a fresh python venv with python 3.11.7 and then run:\r\n```\r\npip install -r requirements.txt\r\n```",
+ "summary": "There is an issue with Numpy 1.23, as it does not support Python 3.11. When attempting to run `pip install -r requirements.txt`, the installation fails due to an inability to build the Numpy wheel, resulting in error messages related to the subprocess. The expected behavior is to install the packages compatible with the recommended Python version. To resolve this, it is suggested to either downgrade Python to a supported version or upgrade Numpy to a version that is compatible with Python 3.11.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/490",
@@ -6124,6 +6338,7 @@
"node_id": "I_kwDOF9wO7c6FB7lJ",
"title": "[explorer] add graph debugging information",
"body": "## Issue\r\n\r\nboth the original explorer and the cre version do not show WHY the graph is the shape it is, for the sake of debugging we should be albe to know why the graph has the specific shape it has (which are the root cres, the ones after that etc)",
+ "summary": "The issue highlights the lack of debugging information in both the original explorer and the cre version regarding the shape of the graph. It suggests that users should have access to details explaining the reasons behind the graph's specific configuration, including information about root nodes and subsequent elements. To address this, the implementation of a feature that provides insights into the graph structure for debugging purposes is recommended.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/493",
@@ -6150,6 +6365,7 @@
"node_id": "I_kwDOF9wO7c6FB8Ap",
"title": "[explorer] improve graph visuals",
"body": "## Issue\r\n\r\nimprove the CRE graph visuals by making them as responsive and cool as the originals",
+ "summary": "The issue discusses the need to enhance the visuals of the CRE graph to ensure they are as responsive and visually appealing as the original versions. To address this, improvements in design and responsiveness should be implemented.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/494",
@@ -6176,6 +6392,7 @@
"node_id": "I_kwDOF9wO7c6GDN0H",
"title": "[api] add ability to export results as CycloneDX attestations",
"body": "## Issue\r\n\r\n### **What is the issue?**\r\nCycloneDX supports attestations. This is their way to do standards and they have native CRE support in their attestations.\r\n\r\nTime for us to also support CycloneDX\r\n\r\nFind a way to make OpenCRE dump attestation documents https://cyclonedx-python-library.readthedocs.io/en/latest/autoapi/cyclonedx/model/index.html#cyclonedx.model.ExternalReferenceType.ATTESTATION\r\n\r\nhttps://github.com/CycloneDX/guides/blob/main/Attestations/en/0x30-Making-Attestations.md",
+ "summary": "The issue highlights the need to add functionality for exporting results as CycloneDX attestations, which are a part of the CycloneDX standard. The goal is to implement support for this format to enable OpenCRE to generate attestation documents. Reference materials include the CycloneDX Python library documentation and a guide on making attestations. To address this, the development team should explore how to integrate CycloneDX's attestation capabilities into the existing system.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/499",
@@ -6204,6 +6421,7 @@
"node_id": "I_kwDOF9wO7c6JGXzk",
"title": "Prune map analysis search to save time and memory",
"body": "Map analysis takes days to calculate and gigabytes to store, but we're only interested in the top matches from standard to standard. It's no use to find 100 weakly linked topics if you have a couple of direct links and a number of strong links.\r\nThe idea therefore is to prune the search we do for map analysis, by stopping search early if we know that we're looking\r\n1. design a strategy for pruning. First stab: don't search for average and weak links if we have direct and strong links. Don't search for weak links if we have direct, strong or average links'.\r\n2. update the search algorithm. This will require some smartness, depending on how it is implemented. \r\n\r\nBy the way, I notice we don't seem to have weak links anymore: should we change our categorization and make 3-6 average and 7+ weak?\r\n\r\nFirst step: find expertise in Neo4J. Rob ",
+ "summary": "The issue highlights the inefficiencies in the current map analysis process, which takes an excessive amount of time and storage space due to the inclusion of less relevant link types. The proposal suggests implementing a pruning strategy to optimize the search. This includes:\n\n1. Designing a pruning strategy to avoid searching for average and weak links when direct and strong links are present.\n2. Updating the search algorithm to incorporate this strategy effectively.\n\nAdditionally, there is a consideration of redefining link categories, as weak links may no longer be applicable. The first action suggested is to consult with an expert in Neo4J to assist with these changes.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/506",
@@ -6232,6 +6450,7 @@
"node_id": "I_kwDOF9wO7c6L31xX",
"title": "Explorer is incomplete",
"body": "opencre.com/explorer is missing a lot of branches, for example everything under input and output protection.\r\nIf adding those branches makes building the tree up slower, the build up probably will need to be optimized. ",
+ "summary": "The Explorer on opencre.com is lacking several branches, particularly those related to input and output protection. To address this, it is suggested that these branches be added, and if their inclusion slows down the tree-building process, optimization may be necessary to improve performance.",
"state": "open",
"state_reason": "reopened",
"url": "https://github.com/OWASP/OpenCRE/issues/514",
@@ -6263,6 +6482,7 @@
"node_id": "I_kwDOF9wO7c6OrUtU",
"title": "fix e2e tests",
"body": "## Issue\r\n\r\n### **What is the issue?**\r\ne2e tests are failing, we should fix them\r\n",
+ "summary": "The end-to-end (e2e) tests are currently failing and require attention to ensure they function correctly. The task involves diagnosing the issues causing the failures and implementing fixes accordingly.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/523",
@@ -6295,6 +6515,7 @@
"node_id": "I_kwDOF9wO7c6P2GHC",
"title": "cp: cannot stat 'cres/db.sqlite': No such file or directory",
"body": "## Issue\r\ncp: cannot stat 'cres/db.sqlite': No such file or directory\r\n\r\n### **What is the issue?**\r\n\r\nI am new to the project and trying to get it installed on my Ubuntu machine. \r\n\r\nHave cloned the repository and on step 2 which states:\r\n**Copy sqlite database to required location\r\ncp cres/db.sqlite standards_cache.sqlite**\r\n\r\nI am getting this error: **cp: cannot stat 'cres/db.sqlite': No such file or directory**\r\n\r\nI have searched the repository for db.sqlite but have not been able to locate it.",
+ "summary": "The issue reported involves an error when attempting to copy a SQLite database file during the installation process of a project. The user is encountering the message \"cp: cannot stat 'cres/db.sqlite': No such file or directory\" after following the installation steps, specifically when trying to copy the file from the 'cres' directory to 'standards_cache.sqlite'. \n\nIt seems that the required 'db.sqlite' file is missing from the repository. To resolve this, it may be necessary to check if the file should be included in the repository or if there are any instructions on how to generate or obtain this database file.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/527",
@@ -6321,6 +6542,7 @@
"node_id": "I_kwDOF9wO7c6P3CKZ",
"title": "zeljkoobrenovic.github.io - reported as unsafe",
"body": "## Issue\r\n\r\nThis site has been reported as unsafe\r\nHosted by zeljkoobrenovic.github.io\r\nMicrosoft recommends you don't continue to this site. It has been reported to Microsoft for containing phishing threats which may try to steal personal or financial information.\r\n\r\n### **What is the issue?**\r\n\r\nWhen clicking \"Explore our catalog in one list\" on the homepage that links to this page: [zeljkoobrenovic.github.io](url) am getting a Microsoft warning page.\r\n\r\n### Expected Behaviour\r\n\r\nWhat should have happened?\r\n\r\nShould link to a safe page.\r\n\r\n### Actual Behaviour\r\n\r\nWhat actually happened?\r\n\r\nLinks to a page that has been flagged as containing phishing threats.\r\n\r\n### Steps to reproduce\r\n\r\nHow can we reproduce the error?\r\n\r\n- Go to homepage of opencre\r\n- Click **Explore**",
+ "summary": "The issue reported involves the site zeljkoobrenovic.github.io being flagged as unsafe due to phishing threats, specifically when users click on the \"Explore our catalog in one list\" link on the homepage. Users receive a warning from Microsoft advising against continuing to the site. The expected behavior is for the link to direct to a safe page, but instead, it leads to a flagged page. \n\nTo resolve this issue, it is necessary to investigate the content of the linked page and address any potential security concerns to ensure it is safe for users.",
"state": "open",
"state_reason": "reopened",
"url": "https://github.com/OWASP/OpenCRE/issues/528",
@@ -6351,6 +6573,7 @@
"node_id": "I_kwDOF9wO7c6QU3Ea",
"title": "make `--upstream_sync` also sync the gap analysis graph progressively",
"body": "## Issue\r\n\r\n### **What is the issue?**\r\n\r\nCurrent gap analysis graph is 9Gb which makes it very hard to download reliably when running locally (also you shouldn't need 9Gb of data to run the project)\r\n\r\n### Expected Behaviour\r\n\r\nUpstream sync should either download the data necessary in order to run(graph structure).\r\nFor the Gap Analysis graph it should either grab it from a place suitable for this sort of data (e.g. google public dataset) or it should load it from upstream opportunistically and then save it in the local database\r\n",
+ "summary": "The issue addresses the challenge of managing the large 9GB gap analysis graph, which complicates local downloads. The expected behavior is for the `--upstream_sync` command to facilitate a more efficient download process by either obtaining the necessary data from a more suitable source, like a Google public dataset, or by opportunistically loading it from upstream and saving it in the local database. To resolve this, modifications to the syncing process may be needed to enhance data management and reduce the local storage burden.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/534",
@@ -6381,6 +6604,7 @@
"node_id": "I_kwDOF9wO7c6Rf2Oi",
"title": "Explorer search removes all standards",
"body": "The amazing new native explorer looks great. I observed that when you enter a search term such as session, that the linked standards are no longer displayed. I doubt this is by design. Can it be fixed?",
+ "summary": "The issue reported concerns the native explorer's search functionality, which fails to display linked standards when a search term is entered. It seems this behavior is unintended, and a fix is needed to ensure that standards remain visible during searches.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/544",
@@ -6410,6 +6634,7 @@
"node_id": "I_kwDOF9wO7c6SOSiO",
"title": "Whitespaces in URLs from Chatbot - 'You can find more information about this section of CWE on its OpenCRE page'",
"body": "## Issue\r\n\r\n### **What is the issue?**\r\n\r\nWhen clicking from the Chatbot the following link white spaces appear in the URL: 'You can find more information about this section of CWE on its OpenCRE page'\r\n\r\n### Expected Behaviour\r\n\r\nWhat should have happened?\r\n\r\nHyphens should be used.\r\n\r\n### Actual Behaviour\r\n\r\nWhat actually happened?\r\n\r\nWhite spaces in URL result in %20 and could cause issues with different browsers and a potentially poor user experience.\r\n\r\n### Steps to reproduce\r\n\r\nHow can we reproduce the error?\r\n\r\nAsk the chatbot a question and click on 'You can find more information about this section of CWE on its OpenCRE page' at the bottom of the response.",
+ "summary": "The issue reported involves the presence of white spaces in URLs generated by the Chatbot, specifically in the link text: 'You can find more information about this section of CWE on its OpenCRE page'. Instead of using hyphens, the white spaces are encoded as %20, which may lead to compatibility issues across different browsers and negatively impact user experience. \n\nTo resolve this, the link formatting should be adjusted to replace white spaces with hyphens in the URLs.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/545",
@@ -6436,6 +6661,7 @@
"node_id": "I_kwDOF9wO7c6SXAXN",
"title": "ISO control numbers interpreted wrongfully",
"body": "Spyros we have a recurring problem with the ISO numbers. there are two 7.1's and two 8.1's etc. I was pointed to it by a user. Last time this happened because 7.10 was interpreted as a value and was turned into 7.1. It should be treated as a string. I recall you fixing this.\r\nGood to see that folks are noticing.",
+ "summary": "The issue involves the incorrect interpretation of ISO control numbers, specifically with versions like 7.1 and 8.1 being duplicated due to numerical misinterpretation (e.g., 7.10 being treated as 7.1). This misinterpretation needs to be addressed by ensuring that these numbers are treated as strings rather than numeric values. A potential solution may involve revisiting previous fixes that addressed similar problems.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/546",
@@ -6464,6 +6690,7 @@
"node_id": "I_kwDOF9wO7c6Tszn8",
"title": "python cre.py --upstream_sync - ModuleNotFoundError: No module named 'click'",
"body": "## Issue\r\n\r\n### **What is the issue?**\r\n\r\nAfter **make install** should there be an instruction to run **pip install -r requirements.txt** ?\r\n\r\n### Expected Behaviour\r\n\r\nWhat should have happened?\r\n\r\nRequirements should be installed and the command **python cre.py --upstream_sync** should not produce an error.\r\n\r\n### Actual Behaviour\r\n\r\nWhat actually happened?\r\n\r\nWhen running \r\n\r\nGetting: **ModuleNotFoundError: No module named 'click'**\r\n\r\nHow can we reproduce the error?\r\n\r\nSet up a new project and follow steps as outlined in README",
+ "summary": "The issue reported involves a `ModuleNotFoundError` for the 'click' module when executing the command `python cre.py --upstream_sync` after performing a `make install`. The expected behavior is that all necessary requirements should be installed automatically, preventing such errors. However, it seems that the required packages may not have been installed, leading to the error when running the command. \n\nTo resolve this, it may be necessary to include an instruction to run `pip install -r requirements.txt` after the `make install` step in the setup documentation.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/551",
@@ -6486,10 +6713,11 @@
"pk": 228,
"fields": {
"nest_created_at": "2024-09-11T19:31:56.826Z",
- "nest_updated_at": "2024-09-12T00:16:07.817Z",
+ "nest_updated_at": "2024-09-13T17:00:20.264Z",
"node_id": "I_kwDOF9wO7c6VpBSs",
"title": "[Import] Existing graph contains cycle",
"body": "## Issue\r\n\r\n### **What is the issue?**\r\n\r\nAn error happens when trying to import a mapping after populating the database from upstream \r\n\r\n### Expected Behaviour\r\n\r\nWhen I run the import on an empty database, it imports correctly\r\n\r\nCommand to clean the database:\r\n```\r\n~/OpenCRE$ rm -rf standards_cache.sqlite; make migrate-upgrade\r\n```\r\n\r\nCommand to start the import:\r\n```\r\n~$ curl -X POST http://CRE-LOCAL-SERVER:5000/rest/v1/cre_csv_import -F \"cre_csv=@cra_cre.csv\"\r\n{\"new_cres\":[\"347-352\",\"028-254\",\"820-878\",\"007-274\",\"286-500\",\"732-148\",\"002-202\",\"841-757\",\"240-274\",\"261-010\",\"766-162\",\"731-120\",\"227-045\",\"571-640\"],\"new_standards\":7,\"status\":\"success\"}\r\n```\r\n\r\nResult from the server side:\r\n```\r\nINFO:application.cmd.cre_main:Registering resource CSA CCM of length 1\r\nINFO:application.database.db:knew of node CSA CCM:IVS-06:Vulnerability Remediation:https://cloudsecurityalliance.org/research/cloud-c\r\n controls-matrix/ ,updating\r\nINFO:werkzeug:161.218.188.108 - - [06/Sep/2024 10:13:14] \"POST /rest/v1/cre_csv_import HTTP/1.1\" 200 -\r\n```\r\n\r\n### Actual Behaviour\r\n\r\nCommand to clean the database and populate from upstream:\r\n```\r\n~/OpenCRE$ rm -rf standards_cache.sqlite; make migrate-upgrade; python cre.py --upstream_sync\r\n```\r\n\r\nCommand to start the import:\r\n```\r\n~$ curl -X POST http://CRE-LOCAL-SERVER:5000/rest/v1/cre_csv_import -F \"cre_csv=@cra_cre.csv\"\r\n\r\n\r\n500 Internal Server Error\r\n
Internal Server Error
\r\n
The server encountered an internal error and was unable to complete your request. Either the server is overloaded or there is an error in the application.
\r\n```\r\n\r\nResult from the server side:\r\n```\r\n return cors_after_request(app.make_response(f(*args, **kwargs)))\r\n File \"/home/csi/OpenCRE/venv/lib/python3.10/site-packages/flask/app.py\", line 880, in full_dispatch_request\r\n rv = self.dispatch_request()\r\n File \"/home/csi/OpenCRE/venv/lib/python3.10/site-packages/flask/app.py\", line 865, in dispatch_request\r\n return self.ensure_sync(self.view_functions[rule.endpoint])(**view_args) # type: ignore[no-any-return]\r\n File \"/home/csi/OpenCRE/application/web/web_main.py\", line 743, in import_from_cre_csv\r\n new_cre, exists = cre_main.register_cre(cre, database)\r\n File \"/home/csi/OpenCRE/application/cmd/cre_main.py\", line 122, in register_cre\r\n collection.add_internal_link(\r\n File \"/home/csi/OpenCRE/application/database/db.py\", line 1597, in add_internal_link\r\n cycle = self.__introduces_cycle(f\"CRE: {higher.id}\", f\"CRE: {lower.id}\")\r\n File \"/home/csi/OpenCRE/application/database/db.py\", line 766, in __introduces_cycle\r\n raise ValueError(\r\nValueError: Existing graph contains cycle,this not a recoverable error, manual database actions are required [('CRE: 155-155', 'CRE:\r\n 546-564'), ('CRE: 546-564', 'CRE: 155-155')]\r\nINFO:werkzeug:161.218.188.108 - - [06/Sep/2024 10:08:39] \"POST /rest/v1/cre_csv_import HTTP/1.1\" 500 -\r\n```\r\n\r\n### Steps to reproduce\r\n\r\nFirst clean the database and populate from upstream:\r\n```\r\n~/OpenCRE$ rm -rf standards_cache.sqlite; make migrate-upgrade; python cre.py --upstream_sync\r\n```\r\n\r\nSecond have a CSV file in the correct format to import (name the file cra_cre.csv with the following content)\r\n```\r\nCRE 0,CRE 1,CRE 2,CRE 3,CRE 4,Cyber Resiliency Act|name,Cyber Resiliency Act|id,Cyber Resiliency Act|hyperlink,NIST 800-53 v5|name,NIST 800-53 v5|id,NIST 800-53 v5|hyperlink,ISO/IEC 27001:2013|name,ISO/IEC 27001:2013|id,ISO/IEC 27001:2013|hyperlink,ASVS|name,ASVS|id,ASVS|hyperlink,CIS v8|name,CIS v8|id,CIS v8|hyperlink,PCI DSS|name,PCI DSS|id,PCI DSS|hyperlink,CSA CCM|name,CSA CCM|id,CSA CCM|hyperlink\r\n,,347-352|Set and confirm integrity of security deployment configuration,,,Designed; developed; and produced to ensure cybersecurity,1.1,https://www.european-cyber-resilience-act.com/Cyber_Resilience_Act_Annex_1.html,,,,,,,,,,,,,,,,,,\r\n,,028-254|Secure auto-updates over full stack,,,Address and remediate vulnerabilities,2.2,https://www.european-cyber-resilience-act.com/Cyber_Resilience_Act_Annex_1.html,,,,,,,,,,,,,,,,,,\r\n,,820-878|Document all trust boundaries and significant data flows,,,Designed; developed; and produced to ensure cybersecurity,1.1,https://www.european-cyber-resilience-act.com/Cyber_Resilience_Act_Annex_1.html,,,,,,,,,,,,,,,,,,\r\n,,,007-274|Patching and updating system components,,Address and remediate vulnerabilities,2.2,https://www.european-cyber-resilience-act.com/Cyber_Resilience_Act_Annex_1.html,,,,,,,,,,,,,,,,,,\r\n,,,286-500|OS security,,Designed; developed; and produced to ensure cybersecurity,1.1,https://www.european-cyber-resilience-act.com/Cyber_Resilience_Act_Annex_1.html,,,,,,,,,,,,,,,,,,\r\n,,732-148|Vulnerability management,,,Address and remediate vulnerabilities,2.2,https://www.european-cyber-resilience-act.com/Cyber_Resilience_Act_Annex_1.html,,,,,,,,,,,,,,,,,,\r\n,,,002-202|Address and remediate,,Address and remediate vulnerabilities,2.2,https://www.european-cyber-resilience-act.com/Cyber_Resilience_Act_Annex_1.html,Flaw Remediation,SI-2,https://csrc.nist.gov/publications/detail/sp/800-53/rev-5/final,Management of technical vulnerabilities,A.12.6.1,https://www.iso.org/standard/54534.html,Security Update Verification,V14.4,https://owasp.org/www-project-application-security-verification-standard/,Continuous Vulnerability Management,Control 7,https://www.cisecurity.org/controls/v8/,Ensure that all system components and software are protected from known vulnerabilities by installing applicable vendor-supplied security patches.,6.2,https://www.pcisecuritystandards.org/documents/PCI_DSS_v3-2-1.pdf,Vulnerability Remediation,IVS-06,https://cloudsecurityalliance.org/research/cloud-controls-matrix/\r\n,,,,\"841-757|Use approved cryptographic algorithms in generation, seeding and verification of OTPs\",Designed; developed; and produced to ensure cybersecurity,1.1,https://www.european-cyber-resilience-act.com/Cyber_Resilience_Act_Annex_1.html,,,,,,,,,,,,,,,,,,\r\n,,,240-274|Log only non-sensitive data,,Designed; developed; and produced to ensure cybersecurity,1.1,https://www.european-cyber-resilience-act.com/Cyber_Resilience_Act_Annex_1.html,,,,,,,,,,,,,,,,,,\r\n,,,261-010|Program management for secure software development,,Designed; developed; and produced to ensure cybersecurity,1.1,https://www.european-cyber-resilience-act.com/Cyber_Resilience_Act_Annex_1.html,,,,,,,,,,,,,,,,,,\r\n,766-162|Security Analysis and documentation,,,,Address and remediate vulnerabilities,2.2,https://www.european-cyber-resilience-act.com/Cyber_Resilience_Act_Annex_1.html,,,,,,,,,,,,,,,,,,\r\n,,,,731-120|Document requirements for (data) protection levels,Designed; developed; and produced to ensure cybersecurity,1.1,https://www.european-cyber-resilience-act.com/Cyber_Resilience_Act_Annex_1.html,,,,,,,,,,,,,,,,,,\r\n,,,,227-045|Identify sensitive data and subject it to a policy,Designed; developed; and produced to ensure cybersecurity,1.1,https://www.european-cyber-resilience-act.com/Cyber_Resilience_Act_Annex_1.html,,,,,,,,,,,,,,,,,,\r\n,,,571-640|Personal data handling management,,Designed; developed; and produced to ensure cybersecurity,1.1,https://www.european-cyber-resilience-act.com/Cyber_Resilience_Act_Annex_1.html,,,,,,,,,,,,,,,,,,\r\n```\r\n\r\n\r\nThird run the command to start the import:\r\n```\r\n~$ curl -X POST http://CRE-LOCAL-SERVER:5000/rest/v1/cre_csv_import -F \"cre_csv=@cra_cre.csv\"\r\n```\r\n\r\n",
+ "summary": "The issue reported involves an error that occurs when attempting to import a mapping into a database that has been populated from an upstream source. While the import works correctly on an empty database, it fails with a \"500 Internal Server Error\" when the database contains data. The underlying problem seems to be related to a cycle in the existing graph structure, which prevents the import from completing successfully. \n\nTo resolve this, manual database actions are required to fix the cycle issue identified in the error message. It may involve reviewing the relationships between nodes to eliminate any cycles before attempting another import.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OpenCRE/issues/552",
@@ -6516,10 +6744,11 @@
"pk": 229,
"fields": {
"nest_created_at": "2024-09-11T19:32:01.930Z",
- "nest_updated_at": "2024-09-11T19:32:01.930Z",
+ "nest_updated_at": "2024-09-13T15:09:18.379Z",
"node_id": "I_kwDOF9sgec4852Zl",
"title": "images",
"body": "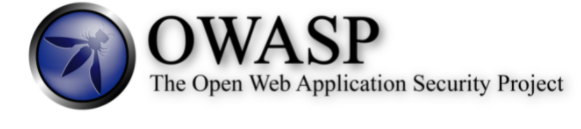\r\n",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-chapter-gujarat-technological-university/issues/2",
@@ -6546,6 +6775,7 @@
"node_id": "I_kwDOF8qHOc5f-TgD",
"title": "Add descriptions for business users",
"body": "## Context\r\n\r\nThe language of OWASP documents speaks to security, operations, IT and engineering professionals. The introduction of low-code/no-code has lowered the bar to become a digital builder. This is the root of why this technology is so important. However, it also means that we cannot assume the same level of technical or security accoman from all low-code/no-code developers.\r\n\r\nTo enable usage of the OWASP Low-Code/No-Code Top 10 by everyone, no matter their technical background, we would need to remove our assumptions and describe risks in a common language that everyone can understand. This should not replace the technical or security oriented language which is a golden standard of an OWASP project, but come as a parallel view on the same issues.\r\n\r\n## Proposal Description\r\n\r\nThe current template for a risk category is as follows:\r\n\r\n- Risk Rating\r\n- The Gist\r\n- Description\r\n- Example Attack Scenarios\r\n - Scenario 1\r\n - Scenario 2\r\n - ...\r\n- How to Prevent\r\n- References\r\n\r\nWe propose the following additions:\r\n- A new section between _Risk Rating_ and _The Gist_ describing the category in simple terms\r\n- A new subsection for each _Scenario_ describing it in simple terms",
+ "summary": "The issue highlights the need for clearer descriptions in OWASP documentation to accommodate business users and those with varying technical expertise, particularly in the context of low-code/no-code development. It suggests that the existing risk category template be enhanced by adding a new section that explains each risk category in simpler terms, as well as a simplified explanation for each attack scenario. This approach aims to make the information more accessible while maintaining the technical depth for expert users. \n\nTo implement this, it would be necessary to revise the template accordingly, ensuring that the new sections are clear and straightforward.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-top-10-low-code-no-code-security-risks/issues/48",
@@ -6576,6 +6806,7 @@
"node_id": "I_kwDOF8qHOc5f-U7M",
"title": "Add product-specific examples",
"body": "## Context\r\n\r\nLow-Code/No-Code [can mean many different things](https://www.gartner.com/en/newsroom/press-releases/2022-12-13-gartner-forecasts-worldwide-low-code-development-technologies-market-to-grow-20-percent-in-2023). Tools can differ in technology, users, developers, use cases and more. For example, Low-Code Application Platforms (LCAP) is used to build web and mobile applications while Robotic Process Automation (RPA) is used to build bots. These technologies are ever-changing and are in the process of merging with eachother, so its still important to cover them in a single project. However, we should also emphasize where they differ, allowing people to focus on the risks relevant to a particular technology.\r\n\r\n## Proposal Description\r\n\r\nThe current template for a risk category is as follows:\r\n\r\n- Risk Rating\r\n- The Gist\r\n- Description\r\n- Example Attack Scenarios\r\n - Scenario 1\r\n - Scenario 2\r\n - ...\r\n- How to Prevent\r\n- References\r\n\r\nWe propose the following additions:\r\n- A new subsection under _Risk Rating_, where we provide different ratings for each Low-Code development technologies\r\n- Label each _Scenario_ with the relevant technologies. A scenario can apply to multiple technologies.\r\n\r\nLow-Code development technologies to distinguish:\r\n- Low-Code Application Platforms (LCAP)\r\n- Robotic Process Automation (RPA)\r\n- Integration Platform as a Service (iPaaS)",
+ "summary": "The issue discusses the need to enhance the current risk assessment template for Low-Code/No-Code technologies by incorporating product-specific examples. It highlights the diversity in Low-Code tools and the importance of distinguishing between different technologies, such as Low-Code Application Platforms (LCAP), Robotic Process Automation (RPA), and Integration Platform as a Service (iPaaS). \n\nThe proposal suggests adding a subsection under the Risk Rating to provide specific ratings for each technology and to label attack scenarios with the relevant technologies, allowing for a clearer understanding of risks associated with each. \n\nTo address this, the template should be updated to include these new subsections and categorizations for improved clarity and relevance.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-top-10-low-code-no-code-security-risks/issues/49",
@@ -6604,6 +6835,7 @@
"node_id": "I_kwDOF8qHOc5qYGEn",
"title": "LCNC-SEC-04 - Scenario 2 (Example Attack Scenarios)",
"body": "Consider (re)moving Scenario 2\r\n\r\n I know this is unrelated and is not part of the change, but scenario 2 doesn't really fit here, right?\r\n\r\n_Originally posted by @mbrg in https://github.com/OWASP/www-project-top-10-low-code-no-code-security-risks/pull/56#discussion_r1182454750_\r\n ",
+ "summary": "The issue raises a concern about the relevance of Scenario 2 in the context of the project. It suggests that Scenario 2 may not be appropriate for inclusion in the current documentation. A review of the content and context of Scenario 2 is needed to determine whether it should be removed or revised to better align with the project's objectives.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-top-10-low-code-no-code-security-risks/issues/67",
@@ -6630,6 +6862,7 @@
"node_id": "I_kwDOF8qHOc5qYGWm",
"title": "LCNC-SEC-03 - Clarify \"unintended consequences\"",
"body": "\"Unintended consequences\" may be too nebulous, it's coverage is broad and vague. Clarity in the description and impact for what \"unintended consequences\" means in relation to data leakage is required, or consider a different title.\r\n\r\n@mbrg ",
+ "summary": "The issue raises concerns about the term \"unintended consequences,\" suggesting it is too vague and broad in its current usage. It calls for a clearer definition and description of its implications, specifically regarding data leakage. Alternatively, it proposes considering a different title to enhance clarity. To address this, a more precise formulation or rephrasing of the term is needed.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-top-10-low-code-no-code-security-risks/issues/68",
@@ -6652,10 +6885,11 @@
"pk": 234,
"fields": {
"nest_created_at": "2024-09-11T19:32:31.845Z",
- "nest_updated_at": "2024-09-11T19:32:31.845Z",
+ "nest_updated_at": "2024-09-13T03:46:09.791Z",
"node_id": "I_kwDOF8qHOc5qYHG1",
"title": "LCNC-SEC-10 - Consider moving attack scenario to LCNC-SEC-08",
"body": " I know that this was part of current available version, but should we move this risk of having too verbose logs to LCNC-SEC-08?\r\n\r\n_Originally posted by @mbrg in https://github.com/OWASP/www-project-top-10-low-code-no-code-security-risks/pull/56#discussion_r1182467102_\r\n ",
+ "summary": "The issue discusses the possibility of relocating an attack scenario related to verbose logs from its current position to a different section, LCNC-SEC-08. While it acknowledges that the scenario is part of the current available version, it raises the question of whether this change would be beneficial. To address this, a review of both sections and their relevance to the scenario should be conducted to determine if the move is warranted.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-top-10-low-code-no-code-security-risks/issues/69",
@@ -6678,10 +6912,11 @@
"pk": 235,
"fields": {
"nest_created_at": "2024-09-11T19:35:23.243Z",
- "nest_updated_at": "2024-09-11T19:35:23.243Z",
+ "nest_updated_at": "2024-09-13T15:11:54.036Z",
"node_id": "I_kwDOFOM8vs56Di1G",
"title": "Hold MTG #22: 2024/10",
"body": "- [x] fix the date\n- [ ] announce the meeting on the page\n- [ ] make connpass event\n- [ ] spread the word\n- [ ] hold the meeting\n- [ ] archive the announcement\n",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-chapter-saitama/issues/79",
@@ -6708,6 +6943,7 @@
"node_id": "I_kwDOFOM8vs56Di2H",
"title": "Hold MTG #23: 2024/12",
"body": "- [ ] fix the date\n- [ ] announce the meeting on the page\n- [ ] make connpass event\n- [ ] spread the word\n- [ ] hold the meeting\n- [ ] archive the announcement\n",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-chapter-saitama/issues/80",
@@ -6730,10 +6966,11 @@
"pk": 237,
"fields": {
"nest_created_at": "2024-09-11T19:35:34.620Z",
- "nest_updated_at": "2024-09-11T19:35:34.620Z",
+ "nest_updated_at": "2024-09-13T15:12:02.613Z",
"node_id": "I_kwDOFLkrvc55lLR7",
"title": "2 typos \"prvilege requried \" -> \"privilege required \"",
"body": "In line \"Note: Let’s call the Critical/High vulnerabilities with no prvilege requried and minimal user interaction as ‘OneClick’.\"\r\n\r\nhttps://github.com/OWASP/www-project-desktop-app-security-top-10/blob/435a699e590c8440e5592d1bfe32c682fd9b68d0/tab_severitybasedranking.md?plain=1#L16",
+ "summary": "The issue identifies two typographical errors in the text where \"prvilege requried\" should be corrected to \"privilege required.\" The line in question refers to Critical/High vulnerabilities that require no privilege and minimal user interaction, labeled as ‘OneClick’. The suggested action is to update the text in the specified line to reflect the correct spelling.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-desktop-app-security-top-10/issues/4",
@@ -6760,6 +6997,7 @@
"node_id": "I_kwDOFAM8Ps5LY-F4",
"title": "Vehicleid for existing users not showing up",
"body": "When accessing the forum, a GET request to /community/api/v2/community/posts/recent is done to receive information about posts and comments. There are 3 existing posts made by the 3 default users, however there is no information about their vehicles.\r\n\r\n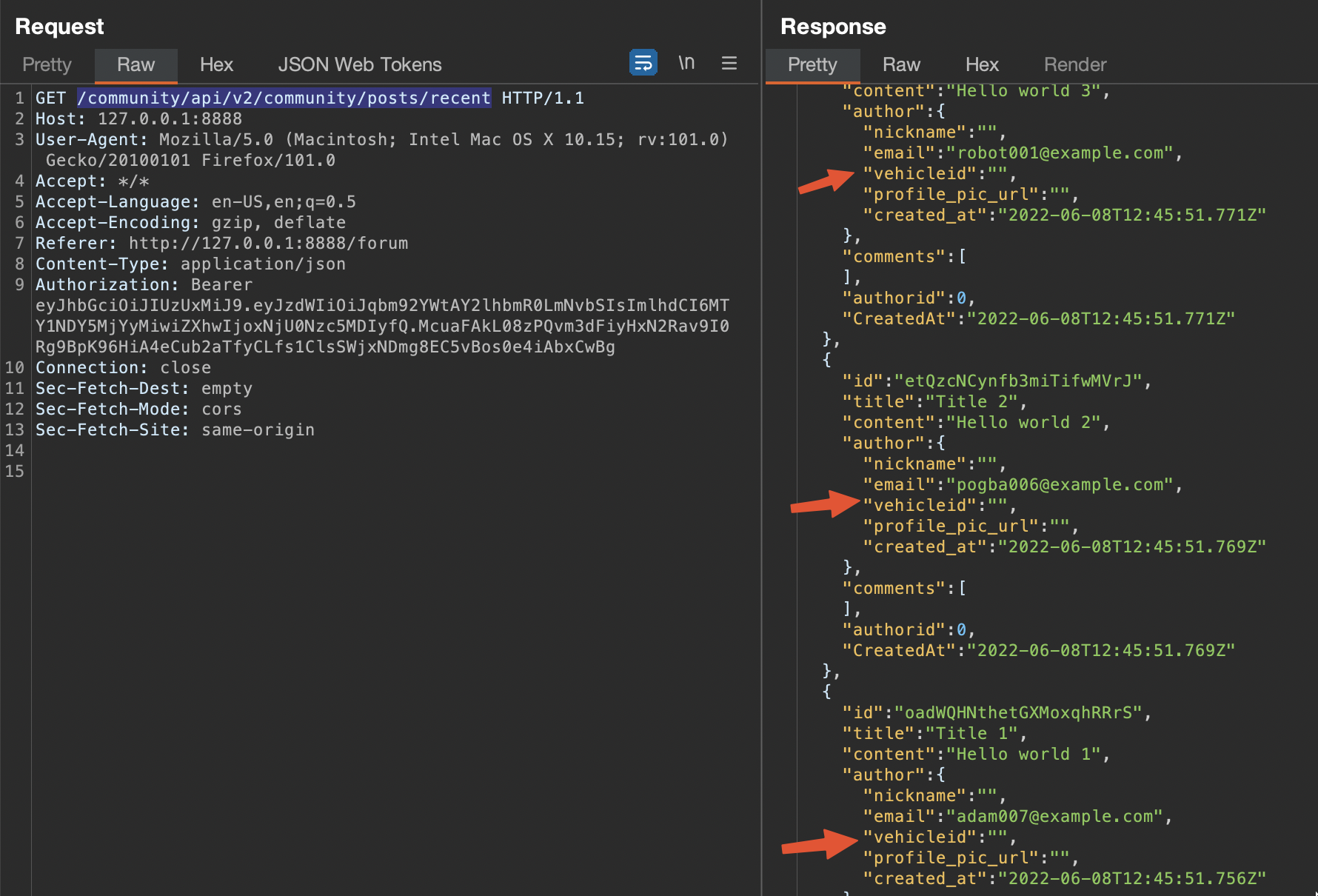\r\n\r\nWhich is honestly not a big deal, you can simply create two users, post something and use their vehicle guids for the challenges. However, I think it would be nice to already have some pre-built vehicleids so the challenge progress feels more natural. I think the vehicles are already created because there is mechanic reports for the default users, so I'm not sure if this is the expected behaviour.\r\n\r\nBoth last stable and development versions have this behaviour. Environment: Macbook M1, macOS Monterey 12.4, Docker 4.7.1 (77678).\r\n",
+ "summary": "Fix the issue of vehicle IDs not displaying for existing users in the forum's recent posts API. Ensure that when a GET request is made to `/community/api/v2/community/posts/recent`, the response includes vehicle information for the three default users who have made posts.\n\n1. Investigate the API endpoint to confirm if vehicle IDs are being fetched correctly from the database.\n2. Check the database to ensure that vehicle records exist for the default users, and verify that the mechanic reports are correctly linked to these users.\n3. Review the data serialization process in the API to ensure that vehicle information is included in the response.\n4. Test the changes in both stable and development versions to confirm the fix.\n\nConsider adding pre-built vehicle IDs to enhance user experience in challenges.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/crAPI/issues/75",
@@ -6789,6 +7027,7 @@
"node_id": "I_kwDOFAM8Ps5Mpxef",
"title": "Community Author Information is a \"Snapshot\" rather than live",
"body": "**Is your feature request related to a problem? Please describe.**\r\nIf you make a post in the community, your current avatar, email address, nickname, etc are all saved in Mongo at that moment. This causes a few issues:\r\n\r\n* If the author modifies any of their information, this isn't reflect in the community\r\n* Saving the image data in mongo creates overhead + the UI is less responsive when returning base64encoded image data.\r\n\r\n**Describe the solution you'd like**\r\nChange author information to be return dynamically. Additionally, don't save any image information in mongo or return image data as base64encoded info. Instead add a new endpoint that dynamically returns the correct user's avatar. Less overhead, less storage, plus caching rules can be set up for the images that the browser can handle.",
+ "summary": "Implement dynamic retrieval of community author information instead of storing it as a snapshot in MongoDB. \n\n1. Identify and modify the existing schema where author data (avatar, email, nickname) is currently saved.\n2. Create a new API endpoint that fetches the current author details dynamically from the user profile database, ensuring real-time accuracy.\n3. Remove the logic that saves image data in MongoDB. Instead, serve images directly from the user profile storage.\n4. Establish caching rules for image responses to enhance UI responsiveness and reduce server load.\n5. Test the new endpoint to ensure it correctly reflects any changes made to the author’s information in real-time. \n\nBegin by reviewing the current data retrieval methods in the community post functionality and plan the necessary changes to the backend architecture.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/crAPI/issues/80",
@@ -6818,6 +7057,7 @@
"node_id": "I_kwDOFAM8Ps5PmTnc",
"title": "Good to provide solution doc for all challenges",
"body": "**Is your feature request related to a problem? Please describe.**\r\nPeople can explore the challenges but always good to provide final solutions as well.\r\n\r\n**Describe the solution you'd like**\r\nSome doc or youtube videos will definitely help for novice member like me\r\n\r\n**Describe alternatives if any you've considered**\r\nDoc or Video reference\r\n\r\n**Additional context**\r\nAdd any other context/screenshots/rough sketchs about the feature request here.",
+ "summary": "Create a comprehensive solution documentation for all challenges. Include both written guides and video tutorials to aid novice members in understanding the solutions.\n\n1. **Identify Challenges**: Compile a list of existing challenges that require documentation.\n2. **Document Solutions**: For each challenge, write clear, step-by-step solutions. Use code snippets where necessary to illustrate key points.\n3. **Create Video Content**: Record video tutorials that walk through the solutions, highlighting important concepts and providing visual aids.\n4. **Organize Content**: Structure the documentation in an easily navigable format, categorizing challenges by difficulty or topic.\n5. **Solicit Feedback**: After initial documentation is created, gather feedback from users to improve clarity and usefulness.\n\nBy following these steps, enhance the learning experience for all users engaging with the challenges.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/crAPI/issues/95",
@@ -6847,6 +7087,7 @@
"node_id": "I_kwDOFAM8Ps5QJsda",
"title": "Does crAPI's configuration allow it to be used as a Capture-the-flag (CTF) event?",
"body": "**Is your feature request related to a problem? Please describe.**\r\nCurious to know if crAPI's configuration allows it to be used as Capture-the-flag (CTF) event\r\n\r\n**Describe the solution you'd like**\r\nA way to configure crAPI to return (e.g in the HTTP response body) a user configured custom flag when particular challenges are solved\r\n\r\n**Describe alternatives if any you've considered**\r\nNone at the moment..\r\n\r\n**Additional context**\r\nAdd any other context/screenshots/rough sketchs about the feature request here.",
+ "summary": "Evaluate the feasibility of using crAPI for Capture-the-Flag (CTF) events. Assess the current configuration capabilities of crAPI to determine if it can be adapted to return user-defined flags in HTTP responses upon solving specific challenges.\n\n1. Review the current crAPI configuration settings and documentation to identify how responses are generated.\n2. Determine if the API can be modified or extended to include custom payloads in response bodies.\n3. Implement a prototype that allows for configurable flags to be set and returned based on challenge completion.\n4. Test the prototype with various challenge scenarios to ensure flags are triggered correctly.\n\nAdditionally, consider potential security implications and ensure that the implementation does not expose sensitive data or vulnerabilities.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/crAPI/issues/97",
@@ -6876,6 +7117,7 @@
"node_id": "I_kwDOFAM8Ps5TNXg8",
"title": "Add GRPC service in crAPI ",
"body": "**Is your feature request related to a problem? Please describe.**\r\nCurrently, crAPI only supports REST endpoints. We can only identify and demo REST vulnerabilities with crAPI. We should add a GRPC service which will make it easier to demo GRPC vulnerabilities. \r\n\r\n**Describe the solution you'd like**\r\n@piyushroshan, can you please add more context on a plausible solution here?",
+ "summary": "Implement a gRPC service in crAPI to enhance vulnerability demonstration capabilities. \n\n1. Analyze the current architecture of crAPI to understand how REST endpoints are integrated.\n2. Research gRPC framework and its integration with existing services.\n3. Create a new gRPC service definition using Protocol Buffers (.proto files) that mirrors the REST endpoints.\n4. Develop the gRPC server-side implementation, ensuring it adheres to the same logic as the REST endpoints.\n5. Implement client-side functionality to call the gRPC service, enabling users to demo vulnerabilities.\n6. Test the new gRPC service to ensure functionality and compatibility with existing tools.\n7. Update documentation to include instructions for using the new gRPC service.\n\nEngage with @piyushroshan for additional insights on the solution design and integration specifics.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/crAPI/issues/110",
@@ -6907,6 +7149,7 @@
"node_id": "I_kwDOFAM8Ps5TNYvH",
"title": "Add GraphQL service in crAPI",
"body": "**Is your feature request related to a problem? Please describe.**\r\nCurrently, crAPI only supports REST endpoints. We can only identify and demo REST vulnerabilities with crAPI. We should add a GraphQL service which will make it easier to demo GraphQL vulnerabilities.\r\n\r\n**Describe the solution you'd like**\r\nA GraphQL API using which we can demonstrate Authorization, Introspection, Batching, Injection, CSRF and other GraphQL attacks. \r\n",
+ "summary": "Implement GraphQL service in crAPI to extend its capabilities beyond REST endpoints. \n\n1. **Identify Requirements**: Determine the specific GraphQL vulnerabilities to demonstrate, including Authorization, Introspection, Batching, Injection, and CSRF.\n\n2. **Set Up GraphQL Schema**: Create a GraphQL schema that defines types, queries, and mutations relevant to the identified vulnerabilities.\n\n3. **Develop GraphQL Resolvers**: Implement resolvers for the defined queries and mutations, ensuring they are designed to simulate vulnerabilities effectively.\n\n4. **Integrate with crAPI**: Ensure the new GraphQL service is integrated into the existing crAPI framework, allowing users to seamlessly switch between REST and GraphQL endpoints.\n\n5. **Create Documentation**: Document the usage of the new GraphQL service, including examples of how to trigger various vulnerabilities.\n\n6. **Testing**: Conduct thorough testing to ensure the GraphQL service operates as intended and effectively demonstrates the targeted vulnerabilities.\n\nBy following these steps, enhance crAPI's functionality to include a robust GraphQL service for vulnerability demonstration.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/crAPI/issues/111",
@@ -6939,6 +7182,7 @@
"node_id": "I_kwDOFAM8Ps5TNbJk",
"title": "Document all the vulnerabilities which currently exist in crAPI",
"body": "**Is your feature request related to a problem? Please describe.**\r\nA documentation page around all the vulnerabilities that currently exist in crAPI will be very helpful. This will provide an easy way to evaluate if crAPI is right for our use cases. \r\n\r\n**Describe the solution you'd like**\r\nA table in README or a separate markdown file that lists down all the vulnerabilities crAPI has and maps them to OWASP API Top 10, OWASP Top 10 and CWEs. \r\n\r\n**Additional context**\r\nNot needed",
+ "summary": "Document all existing vulnerabilities in crAPI. Create a comprehensive documentation page that lists vulnerabilities and maps them to relevant standards such as OWASP API Top 10, OWASP Top 10, and Common Weakness Enumerations (CWEs).\n\n**First Steps:**\n1. Conduct a thorough security assessment of crAPI to identify all vulnerabilities.\n2. Categorize each vulnerability according to OWASP API Top 10 and CWEs.\n3. Draft a table format for the README or create a separate markdown file to present the findings clearly.\n4. Ensure that the documentation is easy to navigate and understand for potential users evaluating crAPI.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/crAPI/issues/112",
@@ -6953,8 +7197,8 @@
"author": 84,
"repository": 406,
"assignees": [
- 87,
- 88
+ 88,
+ 87
],
"labels": [
58,
@@ -6973,6 +7217,7 @@
"node_id": "I_kwDOFAM8Ps5TNckV",
"title": "Up-to-date postman collection as per all the supported vulnerabilities",
"body": "**Is your feature request related to a problem? Please describe.**\r\nThe current crAPI postman collection is 5 months old and there have been some enhancements afterward. Some of the changes might be in API specs and will need updates in API specs as well as postman collections. \r\n\r\n**Describe the solution you'd like**\r\nCan we have a GitHub workflow which runs on every PR or every week, checks for a diff of postman collection, and updates the postman collection if necessary? ",
+ "summary": "Update the crAPI Postman collection to reflect all supported vulnerabilities as per recent enhancements. The existing collection is outdated by five months and requires synchronization with the latest API specifications.\n\nImplement a GitHub workflow that triggers on every pull request or on a weekly basis. This workflow should:\n\n1. Compare the current Postman collection with the latest version.\n2. Identify any differences or updates needed in the API specifications.\n3. Automatically update the Postman collection if discrepancies are found.\n\nFirst steps to tackle this problem:\n\n- Review the latest API specifications to identify enhancements made over the last five months.\n- Create a GitHub Actions workflow that runs a script to check for differences in the Postman collection.\n- Use a diff tool to compare the existing collection against the updated version.\n- Develop a mechanism to automatically update the Postman collection based on the results of the comparison, ensuring that any changes are properly committed back to the repository.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/crAPI/issues/113",
@@ -7004,6 +7249,7 @@
"node_id": "I_kwDOFAM8Ps5TNlp7",
"title": "Monitoring for crAPI",
"body": "**Is your feature request related to a problem? Please describe.**\r\nProvide an easy way to set up crAPI along with monitoring setup. For example, with Kubernetes setup, I should be able to monitor golden signals for my Kubernetes setup with Kube-state metrics. \r\n\r\n**Describe the solution you'd like**\r\nWay to deploy an application with Prometheus and Grafana. The user should be able to create a Grafana dashboard out of the infra metrics from crAPI. We can also provide a dashboard template. \r\n",
+ "summary": "Implement a monitoring solution for crAPI to facilitate easy deployment and monitoring of Kubernetes setups. \n\n1. **Set Up Deployment**: Create a guide for deploying crAPI alongside Prometheus and Grafana. Include Kubernetes configuration examples for deploying these tools in a cluster.\n\n2. **Integrate Kube-State Metrics**: Ensure that crAPI can collect kube-state metrics for monitoring golden signals. Configure Prometheus to scrape these metrics from the Kubernetes environment.\n\n3. **Develop Grafana Dashboard Templates**: Provide pre-configured Grafana dashboard templates that visualize the infrastructure metrics collected by crAPI. \n\n4. **Document Monitoring Setup**: Create detailed documentation on how to set up monitoring, including how to deploy crAPI with Prometheus and Grafana, and how to use the dashboard templates effectively.\n\n5. **Test the Setup**: Validate the entire monitoring setup by deploying a test application and ensuring that metrics are collected and displayed correctly on the Grafana dashboards.\n\nStart by creating a detailed deployment guide that outlines the necessary configurations and steps for users to follow.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/crAPI/issues/118",
@@ -7033,6 +7279,7 @@
"node_id": "I_kwDOFAM8Ps5TOJMB",
"title": "Document Happy Path for crAPI",
"body": "**Is your feature request related to a problem? Please describe.**\r\nCurrently, when we start crAPI, we don't know where to click or where to look to generate specific vulnerabilities. We should document a \"happy path\" or principal workflow which will help people to actually understand the working of the crAPI application. \r\n\r\n**Describe the solution you'd like**\r\nA document with \"happy path\" and link it in main README. \r\n\r\n**Describe alternatives if any you've considered**\r\nNA\r\n\r\n**Additional context**\r\nNA",
+ "summary": "Document the \"happy path\" for crAPI to improve user experience. Create a detailed guide outlining the primary workflow for generating specific vulnerabilities within the application. \n\n1. Identify key features of crAPI that users need to navigate.\n2. Outline steps to access these features, including any prerequisites for setup.\n3. Include screenshots or diagrams to visually guide users through the process.\n4. Ensure the document is clear and concise, emphasizing crucial actions and decisions.\n5. Link the completed document in the main README for easy access.\n\nStart by gathering information from existing users about their experiences and challenges. Use this feedback to structure the document effectively.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/crAPI/issues/120",
@@ -7065,6 +7312,7 @@
"node_id": "I_kwDOFAM8Ps5TOKJS",
"title": "Add direct command Injection vulnerability (CWE-77, OWASP API 8)",
"body": "**Is your feature request related to a problem? Please describe.**\r\nCurrently, we can use crAPI to demonstrate indirect command injection but we also want to add capabilities to demonstrate direct command injection. \r\n\r\n**Describe the solution you'd like**\r\n@piyushroshan , can you guide us here for a solution?\r\n\r\n",
+ "summary": "Implement direct command injection vulnerability (CWE-77, OWASP API 8) in crAPI. This enhancement should allow users to demonstrate both indirect and direct command injection attacks.\n\n**First Steps:**\n1. Research direct command injection techniques and identify common payloads.\n2. Create a new endpoint in crAPI specifically designed to showcase direct command injection.\n3. Ensure the endpoint accepts user input that can be executed as a command in the underlying system.\n4. Implement appropriate safeguards in testing environments to prevent any unauthorized access or system damage.\n5. Document the new feature, detailing the payloads used and their expected outcomes for educational purposes.\n6. Collaborate with @piyushroshan for guidance on best practices and integration into the existing framework.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/crAPI/issues/121",
@@ -7097,6 +7345,7 @@
"node_id": "I_kwDOFAM8Ps5TOM1k",
"title": "Add Security Misconfiguration vulnerabilities in crAPI",
"body": "**Is your feature request related to a problem? Please describe.**\r\nWe should be able to demo security misconfiguration vulnerabilities with crAPI. Security misconfiguration falls under [API7:2019 Security Misconfiguration](https://github.com/OWASP/API-Security/blob/master/2019/en/src/0xa7-security-misconfiguration.md). \r\n\r\n\r\n\r\n**Describe the solution you'd like**\r\nThere are CWEs which fall under security misconfigs:\r\n\r\n- [CWE-2: Environmental Security Flaws](https://cwe.mitre.org/data/definitions/2.html)\r\n- [CWE-16: Configuration](https://cwe.mitre.org/data/definitions/16.html)\r\n- [CWE-388: Error Handling](https://cwe.mitre.org/data/definitions/388.html)\r\n\r\nWe want to add capability in crAPI to be able to demo all three of them. \r\n\r\n**Describe alternatives if any you've considered**\r\nNA\r\n\r\n**Additional context**\r\nNA",
+ "summary": "Add Security Misconfiguration Vulnerabilities to crAPI.\n\n1. Implement demo capabilities for security misconfiguration vulnerabilities, specifically targeting API7:2019 Security Misconfiguration.\n2. Focus on incorporating the following Common Weakness Enumerations (CWEs):\n - CWE-2: Environmental Security Flaws\n - CWE-16: Configuration\n - CWE-388: Error Handling\n3. Create test cases and scenarios that effectively demonstrate each of the identified CWEs within the crAPI framework.\n\n**First Steps:**\n- Research and gather detailed information on the security misconfigurations represented by the specified CWEs.\n- Design and outline the architecture for integrating these vulnerabilities into crAPI.\n- Develop initial proof-of-concept implementations for each CWE to validate the approach.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/crAPI/issues/122",
@@ -7126,6 +7375,7 @@
"node_id": "I_kwDOFAM8Ps5TOPPy",
"title": "Add a way to demonstrate insufficient logging and monitoring vulnerabilities in crAPI",
"body": "**Is your feature request related to a problem? Please describe.**\r\ncrAPI doesn't have the capability to demo insufficient logging and monitoring vulnerability which is [OWASP API 10:2019](https://github.com/OWASP/API-Security/blob/master/2019/en/src/0xaa-insufficient-logging-monitoring.md). \r\n\r\n**Describe the solution you'd like**\r\n[API10:2019 Insufficient Logging & Monitoring](https://github.com/OWASP/API-Security/blob/master/2019/en/src/0xaa-insufficient-logging-monitoring.md) includes two CWEs:\r\n\r\n- [CWE-223: Omission of Security-relevant Information](https://cwe.mitre.org/data/definitions/223.html)\r\n- [CWE-778: Insufficient Logging](https://cwe.mitre.org/data/definitions/778.html)\r\n\r\nWe want to add the capability to demonstrate both of them. \r\n\r\n**Describe alternatives if any you've considered**\r\nNA\r\n\r\n**Additional context**\r\nNA",
+ "summary": "Implement a demonstration of insufficient logging and monitoring vulnerabilities in crAPI. Address the absence of capability to showcase vulnerabilities related to [OWASP API 10:2019](https://github.com/OWASP/API-Security/blob/master/2019/en/src/0xaa-insufficient-logging-monitoring.md).\n\n1. Analyze the OWASP documentation for Insufficient Logging and Monitoring, focusing on the two relevant CWEs: \n - [CWE-223: Omission of Security-relevant Information](https://cwe.mitre.org/data/definitions/223.html)\n - [CWE-778: Insufficient Logging](https://cwe.mitre.org/data/definitions/778.html)\n\n2. Design an architecture that allows the simulation of these vulnerabilities within the crAPI environment.\n\n3. Develop logging features that intentionally omit critical security information to represent CWE-223.\n\n4. Create scenarios where logging is minimal or absent entirely to demonstrate CWE-778.\n\n5. Test the implementation to ensure it effectively showcases the vulnerabilities as intended.\n\n6. Document the process and findings to facilitate understanding and education on the vulnerabilities.\n\n7. Consider integrating automated tests to verify the presence of these vulnerabilities in future builds.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/crAPI/issues/123",
@@ -7155,6 +7405,7 @@
"node_id": "I_kwDOFAM8Ps5Tfute",
"title": "Easy mode and Challenge mode for using crAPI",
"body": "**Is your feature request related to a problem? Please describe.**\r\nThere should be 2 ways to use crAPI. \r\n- Easy mode would have an easily accessible swagger file. \r\n- Challenge mode would not.\r\n\r\n**Describe the solution you'd like**\r\nAs above.",
+ "summary": "Implement two usage modes for crAPI: Easy mode and Challenge mode. \n\n1. **Easy Mode**: Create an easily accessible Swagger file that provides a user-friendly interface for API exploration. Ensure that the documentation is clear and includes examples for common use cases.\n\n2. **Challenge Mode**: Remove the Swagger file and require users to interact with the API through direct HTTP requests, encouraging a deeper understanding of the API's functionality.\n\n**First Steps**:\n- Review the current crAPI documentation and identify the modifications needed to create the Swagger file for Easy mode.\n- Set up a separate branch for the Challenge mode implementation.\n- Evaluate the current API endpoints to ensure they are well-documented and can be effectively used without Swagger.\n- Gather user feedback on preferred features for both modes to guide development.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/crAPI/issues/127",
@@ -7184,6 +7435,7 @@
"node_id": "I_kwDOFAM8Ps5TfywR",
"title": "Video upload functionality is not eminent",
"body": "**Is your feature request related to a problem? Please describe.**\r\nCurrently, an upload video functionality is not eminent on the edit profile screen. Here's how it looks like:\r\n\r\n\r\n**Describe the solution you'd like**\r\nWe can add a rectangular upload box to make it prominent. \r\n\r\n**Additional context**\r\n\r\n",
+ "summary": "Implement a prominent video upload functionality on the edit profile screen. \n\n1. Add a rectangular upload box to enhance visibility.\n2. Ensure the upload box is clearly labeled to guide users.\n3. Use appropriate styling to differentiate the upload box from other elements on the screen.\n\n**First Steps:**\n- Review the current layout of the edit profile screen.\n- Design a mockup of the upload box with dimensions and positioning.\n- Update the front-end code to integrate the new upload box, using HTML and CSS.\n- Implement JavaScript functionality to handle video uploads, including file type validation and size limits.\n- Test the feature across different devices and browsers for compatibility.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/crAPI/issues/129",
@@ -7215,6 +7467,7 @@
"node_id": "I_kwDOFAM8Ps5ccaC_",
"title": "Docker cannot run services in this part of the web, but other services are available",
"body": "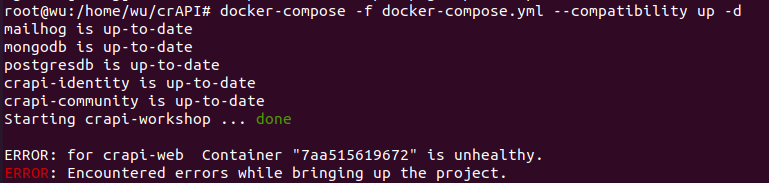\r\n",
+ "summary": "Investigate the issue where Docker cannot run services in the specified area of the web, despite other services functioning correctly. \n\n1. Check Docker's network configuration to ensure it is not restricted from accessing the required web services.\n2. Verify the firewall settings on the host machine to rule out any blocking of Docker's outbound connections.\n3. Examine the Docker logs for any error messages or warnings that may indicate the root cause of the problem.\n4. Test the connectivity from within a Docker container using tools like `curl` or `ping` to diagnose network issues.\n5. Review Docker's version and configuration to ensure compatibility with the services being accessed.\n\nBegin troubleshooting by running a simple container and attempting to reach the problematic services to gather more information.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/crAPI/issues/167",
@@ -7241,6 +7494,7 @@
"node_id": "I_kwDOFAM8Ps5eUVoL",
"title": "ERROR: Encountered errors while bringing up the project.",
"body": "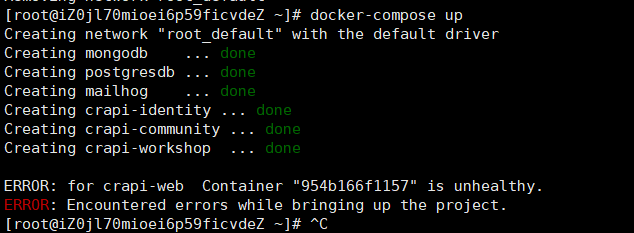\r\n",
+ "summary": "Investigate and resolve the project startup errors indicated in the GitHub issue. Review the error message from the screenshot for specific details on what is failing during the project launch. \n\nFirst steps:\n1. Check the project dependencies and ensure they are correctly defined in the configuration files (e.g., `package.json`, `requirements.txt`).\n2. Verify that all required services (e.g., databases, caches) are running and accessible.\n3. Review the Docker configuration files (e.g., `Dockerfile`, `docker-compose.yml`) for any misconfigurations that might prevent the services from starting.\n4. Run the project in a local development environment to reproduce the error and gather more information.\n5. Check version compatibility of all software components involved (e.g., libraries, frameworks) and update if necessary. \n6. Look for any recent changes in the project that might have introduced this issue, and consider rolling back to a previous stable version.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/crAPI/issues/171",
@@ -7269,6 +7523,7 @@
"node_id": "I_kwDOFAM8Ps5ekgdL",
"title": "dependency failed to start: container crapi-workshop exited",
"body": "**Describe the bug**\r\nAfter running \"docker compose -f docker-compose.yml --compatibility up -d\" I receive the error: dependency failed to start: container crapi-workshop exited (0)\r\n\r\n\r\n**To Reproduce**\r\nSteps to reproduce the behavior.\r\n1. Use the commands listed on github:\r\n (curl -o docker-compose.yml https://raw.githubusercontent.com/OWASP/crAPI/main/deploy/docker/docker- compose.yml\r\n docker compose pull\r\n docker compose -f docker-compose.yml --compatibility up -d) with Network of the VM set to NAT. Behaviour of crapi: all containers start and no errors are received.\r\n2. Shutdown the VM, change Network to internal network, and start VM. Use the last command and you'll receive the error.\r\n\r\n**Expected behavior**\r\nAll containers should start.\r\n\r\n**Runtime Environment**\r\nSytem/Environemnt information (e.g Output of docker -v and uname -a)\r\n-Debian 11 running in VirtualBox 7\r\n-Network of VM changed from the default NAT to Internal Network\r\n-Docker Engine - Community Version: 23.0.1\r\n-Docker Compose version v2.16.0\r\n[crapi-workshop log.txt](https://github.com/OWASP/crAPI/files/10748201/crapi-workshop.log.txt)\r\n",
+ "summary": "Investigate the \"dependency failed to start: container crapi-workshop exited\" error when running the Docker Compose setup. \n\n**Steps to Reproduce:**\n1. Execute the following commands to set up the environment:\n - Download the Docker Compose file:\n ```bash\n curl -o docker-compose.yml https://raw.githubusercontent.com/OWASP/crAPI/main/deploy/docker/docker-compose.yml\n ```\n - Pull the necessary images:\n ```bash\n docker compose pull\n ```\n - Start the containers:\n ```bash\n docker compose -f docker-compose.yml --compatibility up -d\n ```\n2. Initially, run this in a Virtual Machine (VM) with the network set to NAT; all containers should start without errors.\n3. Shut down the VM, change the network setting to Internal Network, and restart the VM. Re-run the last command to see the error.\n\n**Expected Outcome:**\nAll containers should start successfully regardless of the network configuration.\n\n**Environment Details:**\n- Debian 11 in VirtualBox 7\n- Docker Engine - Community Version: 23.0.1\n- Docker Compose version: v2.16.0\n\n**First Steps to Tackle the Problem:**\n1. Check the logs of the `crapi-workshop` container for specific error messages. Examine the provided log file for any relevant details.\n2. Verify network configurations and ensure that all necessary ports are open for communication between containers in the Internal Network setup.\n3. Review the Docker Compose file for any environment variables or services that might be affected by the network mode change.\n4. Test if other containers can communicate properly in the new network setup to rule out broader networking issues.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/crAPI/issues/172",
@@ -7297,6 +7552,7 @@
"node_id": "I_kwDOFAM8Ps5fAZhY",
"title": "Correct password requirements",
"body": "# Description\r\n\r\nThe guide to create a password at the sign up says \"Password should contain at least one digit, one small letter and one capital letter and should at least contain 8 characters.\", however, there is one requirement that is not described here. The password must have an special character.\r\n\r\n## To Reproduce\r\n\r\nJust go to the sign up page and type a valid password according to the description such as \"1234567qW\", but the password will be invalid, then try adding a special character like \"1234567qW@\", and the password will be accepted.\r\n\r\n### Expected behavior\r\n\r\nExpected that the password description would be correct, like \"Password should contain at least one digit, one special charater, one small letter and one capital letter and should at least contain 8 characters.\"\r\n\r\n\r\n#### Runtime Environment\r\n\r\nDocker version 20.10.13 on Ubuntu 20 x86_64\r\n",
+ "summary": "Update password requirements in the sign-up guide. Ensure the description clearly states that a password must include at least one special character, in addition to one digit, one lowercase letter, one uppercase letter, and a minimum of 8 characters.\n\n### Steps to Address:\n\n1. Review the current sign-up page for the password requirements text.\n2. Modify the password requirements section to include special character stipulation.\n3. Test the sign-up functionality with various password combinations to confirm that the validation logic aligns with the updated requirements.\n4. Update any related documentation or user guides to reflect the new password requirements.\n5. Deploy the changes and verify on the staging environment before pushing to production.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/crAPI/issues/173",
@@ -7325,6 +7581,7 @@
"node_id": "I_kwDOFAM8Ps5fNAX4",
"title": "crapi-workshop is unhealthy",
"body": "",
+ "summary": "Investigate the unhealthy status of the crapi-workshop. Examine the provided image for error messages or indicators of failure. \n\nCheck the health checks configured for the service and verify if they are correctly set up. Review the logs for any recent errors or anomalies that could point to the root cause of the issue.\n\nEnsure that all required services and dependencies are running and accessible. Check for resource constraints such as memory or CPU limits that may be affecting the service's performance. \n\nStart by:\n1. Reviewing the health check configuration and logs.\n2. Verifying service dependencies are operational.\n3. Monitoring resource usage to identify potential bottlenecks. \n\nDocument findings and propose fixes based on the gathered information.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/crAPI/issues/175",
@@ -7353,6 +7610,7 @@
"node_id": "I_kwDOFAM8Ps5fUmBQ",
"title": "localhost:8888 is unreachable",
"body": "I can't reach localhost:8888 but I can reach localhost:8025. what is the solution to this problem?\r\n\r\nAlso when I checked the health of the containers, I am seeing that crapi identity is exited 1 minute ago\r\nIs there anyone who experienced this trouble?\r\n\r\nI checked the crapi-identity container's logs and the first issue is PostgreSQL FATAL: database \"crapi\" does not exist.\r\nIn addition: I have got 3 containers that are alive.\r\n",
+ "summary": "Check connectivity to localhost:8888. Since localhost:8025 is accessible, investigate configuration settings related to the service running on port 8888.\n\nExamine the crapi-identity container, which has exited recently. Review logs to confirm the issue: \"PostgreSQL FATAL: database 'crapi' does not exist.\" \n\nTo tackle the problem:\n\n1. **Verify Database Creation**: Ensure that the PostgreSQL database named \"crapi\" is created. If it doesn't exist, create it using the appropriate SQL command or configuration.\n\n2. **Inspect Container Networking**: Confirm that the crapi-identity container is correctly configured to connect to PostgreSQL. Check environment variables and service links.\n\n3. **Check Docker Compose Configuration**: Review your Docker Compose file (if applicable) for any misconfigurations related to the crapi-identity service and PostgreSQL service.\n\n4. **Restart Containers**: After confirming the database exists and configurations are correct, restart the containers.\n\n5. **Monitor Logs**: Continuously monitor the logs of crapi-identity and PostgreSQL to ensure all services are functioning properly.\n\n6. **Test the Endpoint**: After addressing the database issue, test the localhost:8888 endpoint again to verify accessibility.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/crAPI/issues/176",
@@ -7381,6 +7639,7 @@
"node_id": "I_kwDOFAM8Ps5gA2xK",
"title": "crapi-web Container is unhealthy",
"body": "crapi-web log:\r\nCreating test database for alias 'default'...\r\nCreating test database for alias 'mongodb'...\r\nroot ERROR /workshop/api/mechanic/signup - {'name': 'MechRaju', 'email': 'mechraju@crapi.com', 'mechanic_code': 'TRAC_MEC_3', 'number': '9123456708'} - 400 -{'password': [ErrorDetail(string='This field is required.', code='required')]}\r\ndjango.request WARNING Bad Request: /workshop/api/mechanic/signup\r\n.django.request WARNING Bad Request: /workshop/api/mechanic/signup\r\n.django.request WARNING Bad Request: /workshop/api/mechanic/signup\r\n.django.request WARNING Unauthorized: /workshop/api/mechanic/\r\ndjango.request WARNING Unauthorized: /workshop/api/mechanic/\r\n.django.request WARNING Unauthorized: /workshop/api/mechanic/\r\n..root ERROR /workshop/api/merchant/contact_mechanic - {'mechanic_api': 'https://www.google.com', 'number_of_repeats': 5, 'mechanic_code': 'TRAC_MEC_3', 'vin': '9NFXO86WBWA082766', 'problem_details': 'My Car is not working'} - 400 -{'repeat_request_if_failed': [ErrorDetail(string='This field is required.', code='required')]}\r\ndjango.request WARNING Bad Request: /workshop/api/merchant/contact_mechanic\r\n.root INFO Repeat count: 0\r\nroot INFO Repeat count: 1\r\nroot INFO Repeat count: 2\r\nroot INFO Repeat count: 3\r\nroot INFO Repeat count: 4\r\nroot INFO Repeat count: 5\r\ndjango.request WARNING Bad Request: /workshop/api/merchant/contact_mechanic\r\n....................\r\n\r\nRuntime Environment\r\nDocker version 20.10.12, build 20.10.12-0ubuntu4\r\ndocker-compose version 1.29.2, build unknown\r\nubuntu-desktop 22.04 amd64 \r\n",
+ "summary": "Investigate the unhealthy state of the crapi-web container. Analyze logs indicating multiple Bad Request (400) errors for the `/workshop/api/mechanic/signup` and `/workshop/api/merchant/contact_mechanic` endpoints. \n\nIdentify the missing required fields in the requests:\n- For signup, ensure the `password` field is included in the payload.\n- For contact mechanic, ensure `repeat_request_if_failed` is present.\n\nCheck the server's response to unauthorized access attempts, suggesting potential issues with authentication or token validation for the `/workshop/api/mechanic/` endpoint.\n\nExamine the Docker and docker-compose versions for compatibility with the application. \n\nPossible first steps:\n1. Modify the request payloads to include the missing required fields.\n2. Verify the authentication mechanism and ensure valid tokens are being used.\n3. Restart the crapi-web container and monitor logs for changes in health status.\n4. Test the API endpoints manually or with a tool like Postman to confirm they respond correctly with the expected payloads.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/crAPI/issues/178",
@@ -7409,6 +7668,7 @@
"node_id": "I_kwDOFAM8Ps5iA6Ep",
"title": "Can't reach localhost:8888 after suspending my virtual machine",
"body": "**Describe the bug**\r\nI successfully downloaded crAPI and it was running on my Kali virtual machine but when I suspended my host and started it again localhost:8888 was not opening. It kept on loading.\r\n\r\n**Expected behavior**\r\nIt should load every time i try opening 127.0.0.1:8888 \r\n\r\n **Environment**\r\nSytem/Environemnt information \r\n\r\ndocker-compose version 1.29.2, built unknown \r\ndocker-py version : 5.0.3\r\nCPython version : 3.11.2\r\nOpenSSL version : OpenSSL 3.0.8 7 Feb 2023\r\nOperating system : Kali Linux Debian 6.1.20-kali1",
+ "summary": "- Investigate the issue of not reaching localhost:8888 after suspending and resuming the virtual machine.\n- Ensure that the crAPI service is still running in the Docker container after the VM resumes. \n- Check the status of the Docker containers by running `docker ps` to confirm that the crAPI container is active.\n- If the crAPI container is not running, restart it using `docker-compose up -d` from the directory containing the docker-compose.yml file.\n- Verify that the port 8888 is correctly mapped in the docker-compose.yml file.\n- If the service is running but still inaccessible, check for any network issues or firewall settings that may be affecting the connection.\n- Consider restarting Docker service with `sudo systemctl restart docker` to reset any network configurations.\n- Monitor the Docker logs for any errors using `docker-compose logs` to identify potential issues.\n- Test accessing crAPI using `curl http://127.0.0.1:8888` from the terminal to isolate the problem further. \n- If issues persist, consider reconfiguring the network settings of the VM or Docker to ensure proper communication.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/crAPI/issues/182",
@@ -7437,6 +7697,7 @@
"node_id": "I_kwDOFAM8Ps5jEoZ7",
"title": "Coupon and Coupons are different collections ?",
"body": "https://github.com/OWASP/crAPI/blob/df1be10efa127a9cf1e0f9479836bedc980b9f36/services/community/api/models/coupon.go#L61\r\n\r\nDoes it intentional typo or not, because I can create a coupon but can't use that.\r\n\r\nPay attention on the validate coupon func and a collection name that used.\r\n\r\nI recommend to use constants for table/collection names if you'll pass this manually in every calls.",
+ "summary": "Clarify whether \"Coupon\" and \"Coupons\" represent different collections in the codebase. Check the implementation of the `validate coupon` function and the collection name utilized within it to understand the discrepancy. Investigate if the inability to use a created coupon is due to a naming inconsistency.\n\nTo tackle this issue:\n\n1. Review the definition of both \"Coupon\" and \"Coupons\" in the `coupon.go` file.\n2. Examine the `validate coupon` function for how it references the collection names.\n3. Test the creation and validation of a coupon to reproduce the issue.\n4. Propose the implementation of constants for collection/table names to ensure consistency across the codebase. This will help prevent similar issues in the future.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/crAPI/issues/186",
@@ -7463,6 +7724,7 @@
"node_id": "I_kwDOFAM8Ps5mQX4w",
"title": "Traceback (most recent call last): crapi-workshop | File \"/app/crapi/merchant/tests.py\", line 134, in test_contact_mechanic",
"body": "Hi, I install by docker,but find a bug in startuping! \r\n\r\n\r\n\r\nThe information in this screenshot appears several times and may be helpful for you to debug.\r\n\r\n\r\n\r\n\r\n\r\n",
+ "summary": "Investigate the bug occurring during Docker startup for the crAPI project. The traceback indicates an issue in the `test_contact_mechanic` function located in `merchant/tests.py` at line 134. Review the error messages shown in the provided screenshots, as they may contain critical information for debugging.\n\nFirst steps to tackle the problem:\n\n1. Reproduce the issue by running the Docker container and executing the relevant tests.\n2. Analyze the traceback to identify the root cause of the failure in the `test_contact_mechanic` function.\n3. Check for any missing dependencies or configuration issues in the Docker setup that could lead to this error.\n4. Review recent changes in the codebase that might have affected the functionality being tested.\n5. Consult the documentation or community forums for similar issues encountered by other users.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/crAPI/issues/200",
@@ -7491,6 +7753,7 @@
"node_id": "I_kwDOFAM8Ps5nM_ig",
"title": " Install crapi for the first time(ERROR: for crapi-web Container \"28eac0bf7569\" is unhealthy.)",
"body": "**Describe the bug**\r\n\r\n```\r\n$: sudo docker-compose -f docker-compose.yml --compatibility up -d\r\nmongodb is up-to-date\r\nmailhog is up-to-date\r\napi.crapi.io is up-to-date\r\npostgresdb is up-to-date\r\ncrapi-identity is up-to-date\r\ncrapi-community is up-to-date\r\nStarting crapi-workshop ... done\r\n\r\nERROR: for crapi-web Container \"28eac0bf7569\" is unhealthy.\r\nERROR: Encountered errors while bringing up the project.\r\n\r\n```\r\n\r\n**Runtime Environment**\r\n \r\n\r\n$: uname -a\r\n```\r\nLinux wg-virtual-machine 5.19.0-41-generic #42~22.04.1-Ubuntu SMP PREEMPT_DYNAMIC Tue Apr 18 17:40:00 UTC 2 x86_64 x86_64 x86_64 GNU/Linux\r\n```\r\n\r\n```\r\n$: sudo docker version\r\n\r\nClient: Docker Engine - Community\r\n Version: 24.0.2\r\n API version: 1.43\r\n Go version: go1.20.4\r\n Git commit: cb74dfc\r\n Built: Thu May 25 21:51:00 2023\r\n OS/Arch: linux/amd64\r\n Context: default\r\n\r\nServer: Docker Engine - Community\r\n Engine:\r\n Version: 24.0.2\r\n API version: 1.43 (minimum version 1.12)\r\n Go version: go1.20.4\r\n Git commit: 659604f\r\n Built: Thu May 25 21:51:00 2023\r\n OS/Arch: linux/amd64\r\n Experimental: false\r\n containerd:\r\n Version: 1.6.21\r\n GitCommit: 3dce8eb055cbb6872793272b4f20ed16117344f8\r\n runc:\r\n Version: 1.1.7\r\n GitCommit: v1.1.7-0-g860f061\r\n docker-init:\r\n Version: 0.19.0\r\n```\r\n\r\n```\r\nsudo docker-compose version\r\n\r\ndocker-compose version 1.29.2, build unknown\r\ndocker-py version: 5.0.3\r\nCPython version: 3.10.6\r\n```\r\n\r\n",
+ "summary": "Investigate the \"unhealthy\" status of the `crapi-web` container during the initial installation of crapi. Perform the following steps:\n\n1. **Check Container Logs**: Run `sudo docker logs crapi-web` to examine the logs for any errors or issues that may indicate why the container is unhealthy.\n\n2. **Inspect Health Check Configuration**: Review the Dockerfile or docker-compose.yml for `crapi-web` to identify the health check command and ensure it is correctly configured. \n\n3. **Validate Dependencies**: Ensure all dependencies and services required by `crapi-web` are running correctly. Confirm the statuses of `mongodb`, `postgresdb`, and other related services.\n\n4. **Increase Health Check Timeout**: If the health check command requires more time to respond, adjust the timeout settings in the health check configuration.\n\n5. **Resource Allocation**: Verify that the system has sufficient resources (CPU, memory) allocated to Docker. Use `docker stats` to monitor resource usage.\n\n6. **Network Configuration**: Ensure that there are no network-related issues preventing `crapi-web` from communicating with its dependencies.\n\n7. **Update Docker and Docker Compose**: Ensure that you are running the latest stable versions of Docker and Docker Compose. Upgrade if necessary.\n\n8. **Rebuild the Image**: If the problem persists, consider rebuilding the `crapi-web` image using `sudo docker-compose build crapi-web` and then restart the services.\n\n9. **Consult Documentation**: Refer to the official crapi documentation or GitHub repository for any specific troubleshooting tips related to the `crapi-web` container.\n\nBy following these steps, you should be able to diagnose and potentially resolve the \"unhealthy\" status of the `crapi-web` container.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/crAPI/issues/204",
@@ -7519,6 +7782,7 @@
"node_id": "I_kwDOFAM8Ps5oDh58",
"title": "Container Mongodb error ",
"body": "this is a result of an error that I have been facing for 2 days now and if the mongodb is healhty the crapi_community shows an error \r\nI have just to run this command and wait which one gonna show an error \r\n$sudo VERSION=develop docker-compose -f docker-compose.yml --compatibility up -d[+] Building 0.0s (0/0) \r\n[+] Running 6/6\r\n ✔ Container api.crapi.io Running 0.0s \r\n ✔ Container postgresdb Healthy 0.5s \r\n ✘ Container mongodb Error 13.6s \r\n ✔ Container mailhog Running 0.0s \r\n ✔ Container crapi-identity Created 0.0s \r\n ✔ Container crapi-community Created\r\n\r\n\r\n\r\n\r\n\r\ndocker logs mongo db\r\n\r\nWARNING: MongoDB 5.0+ requires a CPU with AVX support, and your current system does not appear to have that!\r\n see https://jira.mongodb.org/browse/SERVER-54407\r\n see also https://www.mongodb.com/community/forums/t/mongodb-5-0-cpu-intel-g4650-compatibility/116610/2\r\n see also https://github.com/docker-library/mongo/issues/485#issuecomment-891991814\r\n\r\nabout to fork child process, waiting until server is ready for connections.\r\nforked process: 30\r\n\r\n{\"t\":{\"$date\":\"2023-06-07T10:12:15.470+00:00\"},\"s\":\"I\", \"c\":\"CONTROL\", \"id\":20698, \"ctx\":\"main\",\"msg\":\"***** SERVER RESTARTED *****\"}\r\n{\"t\":{\"$date\":\"2023-06-07T10:12:15.472+00:00\"},\"s\":\"I\", \"c\":\"CONTROL\", \"id\":23285, \"ctx\":\"main\",\"msg\":\"Automatically disabling TLS 1.0, to force-enable TLS 1.0 specify --sslDisabledProtocols 'none'\"}\r\n{\"t\":{\"$date\":\"2023-06-07T10:12:15.474+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":4648601, \"ctx\":\"main\",\"msg\":\"Implicit TCP FastOpen unavailable. If TCP FastOpen is required, set tcpFastOpenServer, tcpFastOpenClient, and tcpFastOpenQueueSize.\"}\r\n{\"t\":{\"$date\":\"2023-06-07T10:12:15.475+00:00\"},\"s\":\"I\", \"c\":\"STORAGE\", \"id\":4615611, \"ctx\":\"initandlisten\",\"msg\":\"MongoDB starting\",\"attr\":{\"pid\":30,\"port\":27017,\"dbPath\":\"/data/db\",\"architecture\":\"64-bit\",\"host\":\"a636cf2cec8a\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T10:12:15.475+00:00\"},\"s\":\"W\", \"c\":\"CONTROL\", \"id\":20720, \"ctx\":\"initandlisten\",\"msg\":\"Available memory is less than system memory\",\"attr\":{\"availableMemSizeMB\":128,\"systemMemSizeMB\":3927}}\r\n{\"t\":{\"$date\":\"2023-06-07T10:12:15.475+00:00\"},\"s\":\"I\", \"c\":\"CONTROL\", \"id\":23403, \"ctx\":\"initandlisten\",\"msg\":\"Build Info\",\"attr\":{\"buildInfo\":{\"version\":\"4.4.22\",\"gitVersion\":\"fc832685b99221cffb1f5bb5a4ff5ad3e1c416b2\",\"openSSLVersion\":\"OpenSSL 1.1.1f 31 Mar 2020\",\"modules\":[],\"allocator\":\"tcmalloc\",\"environment\":{\"distmod\":\"ubuntu2004\",\"distarch\":\"x86_64\",\"target_arch\":\"x86_64\"}}}}\r\n{\"t\":{\"$date\":\"2023-06-07T10:12:15.475+00:00\"},\"s\":\"I\", \"c\":\"CONTROL\", \"id\":51765, \"ctx\":\"initandlisten\",\"msg\":\"Operating System\",\"attr\":{\"os\":{\"name\":\"Ubuntu\",\"version\":\"20.04\"}}}\r\n{\"t\":{\"$date\":\"2023-06-07T10:12:15.475+00:00\"},\"s\":\"I\", \"c\":\"CONTROL\", \"id\":21951, \"ctx\":\"initandlisten\",\"msg\":\"Options set by command line\",\"attr\":{\"options\":{\"net\":{\"bindIp\":\"127.0.0.1\",\"port\":27017,\"tls\":{\"mode\":\"disabled\"}},\"processManagement\":{\"fork\":true,\"pidFilePath\":\"/tmp/docker-entrypoint-temp-mongod.pid\"},\"systemLog\":{\"destination\":\"file\",\"logAppend\":true,\"path\":\"/proc/1/fd/1\"}}}}\r\n{\"t\":{\"$date\":\"2023-06-07T10:12:15.476+00:00\"},\"s\":\"I\", \"c\":\"STORAGE\", \"id\":22300, \"ctx\":\"initandlisten\",\"msg\":\"The configured WiredTiger cache size is more than 80% of available RAM. See http://dochub.mongodb.org/core/faq-memory-diagnostics-wt\",\"tags\":[\"startupWarnings\"]}\r\n{\"t\":{\"$date\":\"2023-06-07T10:12:15.476+00:00\"},\"s\":\"I\", \"c\":\"STORAGE\", \"id\":22315, \"ctx\":\"initandlisten\",\"msg\":\"Opening WiredTiger\",\"attr\":{\"config\":\"create,cache_size=256M,session_max=33000,eviction=(threads_min=4,threads_max=4),config_base=false,statistics=(fast),log=(enabled=true,archive=true,path=journal,compressor=snappy),file_manager=(close_idle_time=100000,close_scan_interval=10,close_handle_minimum=250),statistics_log=(wait=0),verbose=[recovery_progress,checkpoint_progress,compact_progress],\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T10:12:16.241+00:00\"},\"s\":\"I\", \"c\":\"STORAGE\", \"id\":22430, \"ctx\":\"initandlisten\",\"msg\":\"WiredTiger message\",\"attr\":{\"message\":\"[1686132736:241776][30:0x7fc634e35cc0], txn-recover: [WT_VERB_RECOVERY | WT_VERB_RECOVERY_PROGRESS] Set global recovery timestamp: (0, 0)\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T10:12:16.241+00:00\"},\"s\":\"I\", \"c\":\"STORAGE\", \"id\":22430, \"ctx\":\"initandlisten\",\"msg\":\"WiredTiger message\",\"attr\":{\"message\":\"[1686132736:241884][30:0x7fc634e35cc0], txn-recover: [WT_VERB_RECOVERY | WT_VERB_RECOVERY_PROGRESS] Set global oldest timestamp: (0, 0)\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T10:12:16.259+00:00\"},\"s\":\"I\", \"c\":\"STORAGE\", \"id\":4795906, \"ctx\":\"initandlisten\",\"msg\":\"WiredTiger opened\",\"attr\":{\"durationMillis\":783}}\r\n{\"t\":{\"$date\":\"2023-06-07T10:12:16.259+00:00\"},\"s\":\"I\", \"c\":\"RECOVERY\", \"id\":23987, \"ctx\":\"initandlisten\",\"msg\":\"WiredTiger recoveryTimestamp\",\"attr\":{\"recoveryTimestamp\":{\"$timestamp\":{\"t\":0,\"i\":0}}}}\r\n{\"t\":{\"$date\":\"2023-06-07T10:12:16.341+00:00\"},\"s\":\"I\", \"c\":\"STORAGE\", \"id\":22262, \"ctx\":\"initandlisten\",\"msg\":\"Timestamp monitor starting\"}\r\n{\"t\":{\"$date\":\"2023-06-07T10:12:16.357+00:00\"},\"s\":\"W\", \"c\":\"CONTROL\", \"id\":22120, \"ctx\":\"initandlisten\",\"msg\":\"Access control is not enabled for the database. Read and write access to data and configuration is unrestricted\",\"tags\":[\"startupWarnings\"]}\r\n{\"t\":{\"$date\":\"2023-06-07T10:12:16.357+00:00\"},\"s\":\"W\", \"c\":\"CONTROL\", \"id\":22178, \"ctx\":\"initandlisten\",\"msg\":\"/sys/kernel/mm/transparent_hugepage/enabled is 'always'. We suggest setting it to 'never'\",\"tags\":[\"startupWarnings\"]}\r\n{\"t\":{\"$date\":\"2023-06-07T10:12:16.359+00:00\"},\"s\":\"I\", \"c\":\"STORAGE\", \"id\":20320, \"ctx\":\"initandlisten\",\"msg\":\"createCollection\",\"attr\":{\"namespace\":\"admin.system.version\",\"uuidDisposition\":\"provided\",\"uuid\":{\"uuid\":{\"$uuid\":\"6963ed1a-f25c-4956-a0c4-19f00a0e8fd1\"}},\"options\":{\"uuid\":{\"$uuid\":\"6963ed1a-f25c-4956-a0c4-19f00a0e8fd1\"}}}}\r\n{\"t\":{\"$date\":\"2023-06-07T10:12:16.788+00:00\"},\"s\":\"I\", \"c\":\"INDEX\", \"id\":20345, \"ctx\":\"initandlisten\",\"msg\":\"Index build: done building\",\"attr\":{\"buildUUID\":null,\"namespace\":\"admin.system.version\",\"index\":\"_id_\",\"commitTimestamp\":{\"$timestamp\":{\"t\":0,\"i\":0}}}}\r\n{\"t\":{\"$date\":\"2023-06-07T10:12:16.788+00:00\"},\"s\":\"I\", \"c\":\"COMMAND\", \"id\":20459, \"ctx\":\"initandlisten\",\"msg\":\"Setting featureCompatibilityVersion\",\"attr\":{\"newVersion\":\"4.4\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T10:12:16.788+00:00\"},\"s\":\"I\", \"c\":\"STORAGE\", \"id\":20536, \"ctx\":\"initandlisten\",\"msg\":\"Flow Control is enabled on this deployment\"}\r\n{\"t\":{\"$date\":\"2023-06-07T10:12:16.790+00:00\"},\"s\":\"I\", \"c\":\"STORAGE\", \"id\":20320, \"ctx\":\"initandlisten\",\"msg\":\"createCollection\",\"attr\":{\"namespace\":\"local.startup_log\",\"uuidDisposition\":\"generated\",\"uuid\":{\"uuid\":{\"$uuid\":\"f0272e6b-f2fc-40ed-990f-3957e3e132eb\"}},\"options\":{\"capped\":true,\"size\":10485760}}}\r\n{\"t\":{\"$date\":\"2023-06-07T10:12:16.830+00:00\"},\"s\":\"I\", \"c\":\"INDEX\", \"id\":20345, \"ctx\":\"initandlisten\",\"msg\":\"Index build: done building\",\"attr\":{\"buildUUID\":null,\"namespace\":\"local.startup_log\",\"index\":\"_id_\",\"commitTimestamp\":{\"$timestamp\":{\"t\":0,\"i\":0}}}}\r\n{\"t\":{\"$date\":\"2023-06-07T10:12:16.831+00:00\"},\"s\":\"I\", \"c\":\"FTDC\", \"id\":20625, \"ctx\":\"initandlisten\",\"msg\":\"Initializing full-time diagnostic data capture\",\"attr\":{\"dataDirectory\":\"/data/db/diagnostic.data\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T10:12:16.831+00:00\"},\"s\":\"I\", \"c\":\"REPL\", \"id\":6015317, \"ctx\":\"initandlisten\",\"msg\":\"Setting new configuration state\",\"attr\":{\"newState\":\"ConfigReplicationDisabled\",\"oldState\":\"ConfigPreStart\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T10:12:16.897+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":23015, \"ctx\":\"listener\",\"msg\":\"Listening on\",\"attr\":{\"address\":\"/tmp/mongodb-27017.sock\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T10:12:16.897+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":23015, \"ctx\":\"listener\",\"msg\":\"Listening on\",\"attr\":{\"address\":\"127.0.0.1\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T10:12:16.897+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":23016, \"ctx\":\"listener\",\"msg\":\"Waiting for connections\",\"attr\":{\"port\":27017,\"ssl\":\"off\"}}\r\nchild process started successfully, parent exiting\r\n{\"t\":{\"$date\":\"2023-06-07T10:12:17.574+00:00\"},\"s\":\"I\", \"c\":\"COMMAND\", \"id\":51803, \"ctx\":\"LogicalSessionCacheRefresh\",\"msg\":\"Slow query\",\"attr\":{\"type\":\"command\",\"ns\":\"config.system.sessions\",\"command\":{\"listIndexes\":\"system.sessions\",\"cursor\":{},\"$db\":\"config\"},\"numYields\":0,\"ok\":0,\"errMsg\":\"ns does not exist: config.system.sessions\",\"errName\":\"NamespaceNotFound\",\"errCode\":26,\"reslen\":134,\"locks\":{\"FeatureCompatibilityVersion\":{\"acquireCount\":{\"r\":1}},\"ReplicationStateTransition\":{\"acquireCount\":{\"w\":1}},\"Global\":{\"acquireCount\":{\"r\":1}},\"Database\":{\"acquireCount\":{\"r\":1}},\"Collection\":{\"acquireCount\":{\"r\":1}},\"Mutex\":{\"acquireCount\":{\"r\":1}}},\"protocol\":\"op_msg\",\"durationMillis\":677}}\r\n{\"t\":{\"$date\":\"2023-06-07T10:12:17.581+00:00\"},\"s\":\"I\", \"c\":\"COMMAND\", \"id\":51803, \"ctx\":\"LogicalSessionCacheReap\",\"msg\":\"Slow query\",\"attr\":{\"type\":\"command\",\"ns\":\"config.system.sessions\",\"command\":{\"listIndexes\":\"system.sessions\",\"cursor\":{},\"$db\":\"config\"},\"numYields\":0,\"ok\":0,\"errMsg\":\"ns does not exist: config.system.sessions\",\"errName\":\"NamespaceNotFound\",\"errCode\":26,\"reslen\":134,\"locks\":{\"FeatureCompatibilityVersion\":{\"acquireCount\":{\"r\":1}},\"ReplicationStateTransition\":{\"acquireCount\":{\"w\":1}},\"Global\":{\"acquireCount\":{\"r\":1}},\"Database\":{\"acquireCount\":{\"r\":1}},\"Collection\":{\"acquireCount\":{\"r\":1}},\"Mutex\":{\"acquireCount\":{\"r\":1}}},\"protocol\":\"op_msg\",\"durationMillis\":684}}\r\n{\"t\":{\"$date\":\"2023-06-07T10:12:17.668+00:00\"},\"s\":\"I\", \"c\":\"STORAGE\", \"id\":20320, \"ctx\":\"LogicalSessionCacheRefresh\",\"msg\":\"createCollection\",\"attr\":{\"namespace\":\"config.system.sessions\",\"uuidDisposition\":\"generated\",\"uuid\":{\"uuid\":{\"$uuid\":\"611d3916-8a82-4f2e-9c68-b54e0a1481fb\"}},\"options\":{}}}\r\n{\"t\":{\"$date\":\"2023-06-07T10:12:17.887+00:00\"},\"s\":\"I\", \"c\":\"CONTROL\", \"id\":20712, \"ctx\":\"LogicalSessionCacheReap\",\"msg\":\"Sessions collection is not set up; waiting until next sessions reap interval\",\"attr\":{\"error\":\"NamespaceNotFound: config.system.sessions does not exist\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T10:12:18.672+00:00\"},\"s\":\"I\", \"c\":\"INDEX\", \"id\":20345, \"ctx\":\"LogicalSessionCacheRefresh\",\"msg\":\"Index build: done building\",\"attr\":{\"buildUUID\":null,\"namespace\":\"config.system.sessions\",\"index\":\"_id_\",\"commitTimestamp\":{\"$timestamp\":{\"t\":0,\"i\":0}}}}\r\n{\"t\":{\"$date\":\"2023-06-07T10:12:18.672+00:00\"},\"s\":\"I\", \"c\":\"INDEX\", \"id\":20345, \"ctx\":\"LogicalSessionCacheRefresh\",\"msg\":\"Index build: done building\",\"attr\":{\"buildUUID\":null,\"namespace\":\"config.system.sessions\",\"index\":\"lsidTTLIndex\",\"commitTimestamp\":{\"$timestamp\":{\"t\":0,\"i\":0}}}}\r\n{\"t\":{\"$date\":\"2023-06-07T10:12:18.672+00:00\"},\"s\":\"I\", \"c\":\"COMMAND\", \"id\":51803, \"ctx\":\"LogicalSessionCacheRefresh\",\"msg\":\"Slow query\",\"attr\":{\"type\":\"command\",\"ns\":\"config.system.sessions\",\"command\":{\"createIndexes\":\"system.sessions\",\"indexes\":[{\"key\":{\"lastUse\":1},\"name\":\"lsidTTLIndex\",\"expireAfterSeconds\":1800}],\"writeConcern\":{},\"$db\":\"config\"},\"numYields\":0,\"reslen\":114,\"locks\":{\"ParallelBatchWriterMode\":{\"acquireCount\":{\"r\":5}},\"FeatureCompatibilityVersion\":{\"acquireCount\":{\"r\":2,\"w\":3}},\"ReplicationStateTransition\":{\"acquireCount\":{\"w\":5}},\"Global\":{\"acquireCount\":{\"r\":2,\"w\":3}},\"Database\":{\"acquireCount\":{\"r\":2,\"w\":3}},\"Collection\":{\"acquireCount\":{\"r\":3,\"w\":2}},\"Mutex\":{\"acquireCount\":{\"r\":6}}},\"flowControl\":{\"acquireCount\":1,\"timeAcquiringMicros\":2},\"storage\":{},\"protocol\":\"op_msg\",\"durationMillis\":1004}}\r\n{\"t\":{\"$date\":\"2023-06-07T10:12:28.466+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":22943, \"ctx\":\"listener\",\"msg\":\"Connection accepted\",\"attr\":{\"remote\":\"127.0.0.1:45044\",\"connectionId\":1,\"connectionCount\":1}}\r\n{\"t\":{\"$date\":\"2023-06-07T10:12:33.367+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":51800, \"ctx\":\"conn1\",\"msg\":\"client metadata\",\"attr\":{\"remote\":\"127.0.0.1:45044\",\"client\":\"conn1\",\"doc\":{\"application\":{\"name\":\"MongoDB Shell\"},\"driver\":{\"name\":\"MongoDB Internal Client\",\"version\":\"4.4.22\"},\"os\":{\"type\":\"Linux\",\"name\":\"Ubuntu\",\"architecture\":\"x86_64\",\"version\":\"20.04\"}}}}\r\n{\"t\":{\"$date\":\"2023-06-07T10:12:36.472+00:00\"},\"s\":\"I\", \"c\":\"COMMAND\", \"id\":51803, \"ctx\":\"conn1\",\"msg\":\"Slow query\",\"attr\":{\"type\":\"command\",\"ns\":\"admin.$cmd\",\"appName\":\"MongoDB Shell\",\"command\":{\"whatsmyuri\":1,\"$db\":\"admin\"},\"numYields\":0,\"reslen\":63,\"locks\":{},\"protocol\":\"op_msg\",\"durationMillis\":253}}\r\n{\"t\":{\"$date\":\"2023-06-07T10:12:41.278+00:00\"},\"s\":\"I\", \"c\":\"COMMAND\", \"id\":51803, \"ctx\":\"conn1\",\"msg\":\"Slow query\",\"attr\":{\"type\":\"command\",\"ns\":\"admin.$cmd\",\"appName\":\"MongoDB Shell\",\"command\":{\"buildinfo\":1.0,\"$db\":\"admin\"},\"numYields\":0,\"reslen\":1811,\"locks\":{},\"protocol\":\"op_msg\",\"durationMillis\":2311}}\r\n{\"t\":{\"$date\":\"2023-06-07T10:12:46.870+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":22944, \"ctx\":\"conn1\",\"msg\":\"Connection ended\",\"attr\":{\"remote\":\"127.0.0.1:45044\",\"connectionId\":1,\"connectionCount\":0}}\r\n{\"t\":{\"$date\":\"2023-06-07T10:13:37.506+00:00\"},\"s\":\"I\", \"c\":\"STORAGE\", \"id\":22430, \"ctx\":\"WTCheckpointThread\",\"msg\":\"WiredTiger message\",\"attr\":{\"message\":\"[1686132815:869508][30:0x7fc62de26700], WT_SESSION.checkpoint: [WT_VERB_CHECKPOINT_PROGRESS] saving checkpoint snapshot min: 34, snapshot max: 34 snapshot count: 0, oldest timestamp: (0, 0) , meta checkpoint timestamp: (0, 0) base write gen: 1\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T10:13:37.486+00:00\"},\"s\":\"I\", \"c\":\"COMMAND\", \"id\":23099, \"ctx\":\"PeriodicTaskRunner\",\"msg\":\"Task finished\",\"attr\":{\"taskName\":\"DBConnectionPool-cleaner\",\"durationMillis\":8031}}\r\n{\"t\":{\"$date\":\"2023-06-07T10:13:57.468+00:00\"},\"s\":\"I\", \"c\":\"COMMAND\", \"id\":23099, \"ctx\":\"PeriodicTaskRunner\",\"msg\":\"Task finished\",\"attr\":{\"taskName\":\"DBConnectionPool-cleaner\",\"durationMillis\":4043}}\r\n{\"t\":{\"$date\":\"2023-06-07T10:15:36.268+00:00\"},\"s\":\"I\", \"c\":\"COMMAND\", \"id\":23099, \"ctx\":\"PeriodicTaskRunner\",\"msg\":\"Task finished\",\"attr\":{\"taskName\":\"DBConnectionPool-cleaner\",\"durationMillis\":14701}}\r\n/usr/local/bin/docker-entrypoint.sh: line 416: 81 Killed \"${mongo[@]}\" \"$rootAuthDatabase\" <<-EOJS\r\ndb.createUser({\r\nuser: $(_js_escape \"$MONGO_INITDB_ROOT_USERNAME\"),\r\npwd: $(_js_escape \"$MONGO_INITDB_ROOT_PASSWORD\"),\r\nroles: [ { role: 'root', db: $(_js_escape \"$rootAuthDatabase\") } ]\r\n})\r\nEOJS\r\n\r\n\r\nWARNING: MongoDB 5.0+ requires a CPU with AVX support, and your current system does not appear to have that!\r\n see https://jira.mongodb.org/browse/SERVER-54407\r\n see also https://www.mongodb.com/community/forums/t/mongodb-5-0-cpu-intel-g4650-compatibility/116610/2\r\n see also https://github.com/docker-library/mongo/issues/485#issuecomment-891991814\r\n\r\n{\"t\":{\"$date\":\"2023-06-07T11:04:23.700+00:00\"},\"s\":\"I\", \"c\":\"CONTROL\", \"id\":23285, \"ctx\":\"main\",\"msg\":\"Automatically disabling TLS 1.0, to force-enable TLS 1.0 specify --sslDisabledProtocols 'none'\"}\r\n{\"t\":{\"$date\":\"2023-06-07T11:04:23.703+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":4648601, \"ctx\":\"main\",\"msg\":\"Implicit TCP FastOpen unavailable. If TCP FastOpen is required, set tcpFastOpenServer, tcpFastOpenClient, and tcpFastOpenQueueSize.\"}\r\n{\"t\":{\"$date\":\"2023-06-07T11:04:23.704+00:00\"},\"s\":\"I\", \"c\":\"STORAGE\", \"id\":4615611, \"ctx\":\"initandlisten\",\"msg\":\"MongoDB starting\",\"attr\":{\"pid\":1,\"port\":27017,\"dbPath\":\"/data/db\",\"architecture\":\"64-bit\",\"host\":\"a636cf2cec8a\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:04:23.704+00:00\"},\"s\":\"W\", \"c\":\"CONTROL\", \"id\":20720, \"ctx\":\"initandlisten\",\"msg\":\"Available memory is less than system memory\",\"attr\":{\"availableMemSizeMB\":128,\"systemMemSizeMB\":3927}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:04:23.704+00:00\"},\"s\":\"I\", \"c\":\"CONTROL\", \"id\":23403, \"ctx\":\"initandlisten\",\"msg\":\"Build Info\",\"attr\":{\"buildInfo\":{\"version\":\"4.4.22\",\"gitVersion\":\"fc832685b99221cffb1f5bb5a4ff5ad3e1c416b2\",\"openSSLVersion\":\"OpenSSL 1.1.1f 31 Mar 2020\",\"modules\":[],\"allocator\":\"tcmalloc\",\"environment\":{\"distmod\":\"ubuntu2004\",\"distarch\":\"x86_64\",\"target_arch\":\"x86_64\"}}}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:04:23.704+00:00\"},\"s\":\"I\", \"c\":\"CONTROL\", \"id\":51765, \"ctx\":\"initandlisten\",\"msg\":\"Operating System\",\"attr\":{\"os\":{\"name\":\"Ubuntu\",\"version\":\"20.04\"}}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:04:23.704+00:00\"},\"s\":\"I\", \"c\":\"CONTROL\", \"id\":21951, \"ctx\":\"initandlisten\",\"msg\":\"Options set by command line\",\"attr\":{\"options\":{\"net\":{\"bindIp\":\"*\"},\"security\":{\"authorization\":\"enabled\"}}}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:04:23.706+00:00\"},\"s\":\"W\", \"c\":\"STORAGE\", \"id\":22271, \"ctx\":\"initandlisten\",\"msg\":\"Detected unclean shutdown - Lock file is not empty\",\"attr\":{\"lockFile\":\"/data/db/mongod.lock\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:04:23.706+00:00\"},\"s\":\"I\", \"c\":\"STORAGE\", \"id\":22270, \"ctx\":\"initandlisten\",\"msg\":\"Storage engine to use detected by data files\",\"attr\":{\"dbpath\":\"/data/db\",\"storageEngine\":\"wiredTiger\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:04:23.706+00:00\"},\"s\":\"W\", \"c\":\"STORAGE\", \"id\":22302, \"ctx\":\"initandlisten\",\"msg\":\"Recovering data from the last clean checkpoint.\"}\r\n{\"t\":{\"$date\":\"2023-06-07T11:04:23.706+00:00\"},\"s\":\"I\", \"c\":\"STORAGE\", \"id\":22300, \"ctx\":\"initandlisten\",\"msg\":\"The configured WiredTiger cache size is more than 80% of available RAM. See http://dochub.mongodb.org/core/faq-memory-diagnostics-wt\",\"tags\":[\"startupWarnings\"]}\r\n{\"t\":{\"$date\":\"2023-06-07T11:04:23.706+00:00\"},\"s\":\"I\", \"c\":\"STORAGE\", \"id\":22315, \"ctx\":\"initandlisten\",\"msg\":\"Opening WiredTiger\",\"attr\":{\"config\":\"create,cache_size=256M,session_max=33000,eviction=(threads_min=4,threads_max=4),config_base=false,statistics=(fast),log=(enabled=true,archive=true,path=journal,compressor=snappy),file_manager=(close_idle_time=100000,close_scan_interval=10,close_handle_minimum=250),statistics_log=(wait=0),verbose=[recovery_progress,checkpoint_progress,compact_progress],\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:04:24.147+00:00\"},\"s\":\"I\", \"c\":\"STORAGE\", \"id\":22430, \"ctx\":\"initandlisten\",\"msg\":\"WiredTiger message\",\"attr\":{\"message\":\"[1686135864:147357][1:0x7f0929c1dcc0], txn-recover: [WT_VERB_RECOVERY_PROGRESS] Recovering log 1 through 2\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:04:24.988+00:00\"},\"s\":\"I\", \"c\":\"STORAGE\", \"id\":22430, \"ctx\":\"initandlisten\",\"msg\":\"WiredTiger message\",\"attr\":{\"message\":\"[1686135864:988764][1:0x7f0929c1dcc0], txn-recover: [WT_VERB_RECOVERY_PROGRESS] Recovering log 2 through 2\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:04:25.791+00:00\"},\"s\":\"I\", \"c\":\"STORAGE\", \"id\":22430, \"ctx\":\"initandlisten\",\"msg\":\"WiredTiger message\",\"attr\":{\"message\":\"[1686135865:791543][1:0x7f0929c1dcc0], txn-recover: [WT_VERB_RECOVERY | WT_VERB_RECOVERY_PROGRESS] Main recovery loop: starting at 1/21504 to 2/256\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:04:25.792+00:00\"},\"s\":\"I\", \"c\":\"STORAGE\", \"id\":22430, \"ctx\":\"initandlisten\",\"msg\":\"WiredTiger message\",\"attr\":{\"message\":\"[1686135865:792677][1:0x7f0929c1dcc0], txn-recover: [WT_VERB_RECOVERY_PROGRESS] Recovering log 1 through 2\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:04:26.874+00:00\"},\"s\":\"I\", \"c\":\"STORAGE\", \"id\":22430, \"ctx\":\"initandlisten\",\"msg\":\"WiredTiger message\",\"attr\":{\"message\":\"[1686135866:874734][1:0x7f0929c1dcc0], file:sizeStorer.wt, txn-recover: [WT_VERB_RECOVERY_PROGRESS] Recovering log 2 through 2\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:04:27.780+00:00\"},\"s\":\"I\", \"c\":\"STORAGE\", \"id\":22430, \"ctx\":\"initandlisten\",\"msg\":\"WiredTiger message\",\"attr\":{\"message\":\"[1686135867:780713][1:0x7f0929c1dcc0], file:sizeStorer.wt, txn-recover: [WT_VERB_RECOVERY | WT_VERB_RECOVERY_PROGRESS] Set global recovery timestamp: (0, 0)\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:04:27.781+00:00\"},\"s\":\"I\", \"c\":\"STORAGE\", \"id\":22430, \"ctx\":\"initandlisten\",\"msg\":\"WiredTiger message\",\"attr\":{\"message\":\"[1686135867:781022][1:0x7f0929c1dcc0], file:sizeStorer.wt, txn-recover: [WT_VERB_RECOVERY | WT_VERB_RECOVERY_PROGRESS] Set global oldest timestamp: (0, 0)\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:04:28.079+00:00\"},\"s\":\"I\", \"c\":\"STORAGE\", \"id\":22430, \"ctx\":\"initandlisten\",\"msg\":\"WiredTiger message\",\"attr\":{\"message\":\"[1686135868:79249][1:0x7f0929c1dcc0], WT_SESSION.checkpoint: [WT_VERB_CHECKPOINT_PROGRESS] saving checkpoint snapshot min: 16, snapshot max: 16 snapshot count: 0, oldest timestamp: (0, 0) , meta checkpoint timestamp: (0, 0) base write gen: 7\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:04:28.160+00:00\"},\"s\":\"I\", \"c\":\"STORAGE\", \"id\":4795906, \"ctx\":\"initandlisten\",\"msg\":\"WiredTiger opened\",\"attr\":{\"durationMillis\":4454}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:04:28.160+00:00\"},\"s\":\"I\", \"c\":\"RECOVERY\", \"id\":23987, \"ctx\":\"initandlisten\",\"msg\":\"WiredTiger recoveryTimestamp\",\"attr\":{\"recoveryTimestamp\":{\"$timestamp\":{\"t\":0,\"i\":0}}}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:04:28.669+00:00\"},\"s\":\"I\", \"c\":\"STORAGE\", \"id\":22262, \"ctx\":\"initandlisten\",\"msg\":\"Timestamp monitor starting\"}\r\n{\"t\":{\"$date\":\"2023-06-07T11:04:28.704+00:00\"},\"s\":\"W\", \"c\":\"CONTROL\", \"id\":22178, \"ctx\":\"initandlisten\",\"msg\":\"/sys/kernel/mm/transparent_hugepage/enabled is 'always'. We suggest setting it to 'never'\",\"tags\":[\"startupWarnings\"]}\r\n{\"t\":{\"$date\":\"2023-06-07T11:04:29.372+00:00\"},\"s\":\"I\", \"c\":\"STORAGE\", \"id\":20536, \"ctx\":\"initandlisten\",\"msg\":\"Flow Control is enabled on this deployment\"}\r\n{\"t\":{\"$date\":\"2023-06-07T11:04:29.676+00:00\"},\"s\":\"I\", \"c\":\"FTDC\", \"id\":20625, \"ctx\":\"initandlisten\",\"msg\":\"Initializing full-time diagnostic data capture\",\"attr\":{\"dataDirectory\":\"/data/db/diagnostic.data\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:04:29.681+00:00\"},\"s\":\"I\", \"c\":\"REPL\", \"id\":6015317, \"ctx\":\"initandlisten\",\"msg\":\"Setting new configuration state\",\"attr\":{\"newState\":\"ConfigReplicationDisabled\",\"oldState\":\"ConfigPreStart\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:04:29.973+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":23015, \"ctx\":\"listener\",\"msg\":\"Listening on\",\"attr\":{\"address\":\"/tmp/mongodb-27017.sock\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:04:29.974+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":23015, \"ctx\":\"listener\",\"msg\":\"Listening on\",\"attr\":{\"address\":\"0.0.0.0\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:04:29.974+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":23016, \"ctx\":\"listener\",\"msg\":\"Waiting for connections\",\"attr\":{\"port\":27017,\"ssl\":\"off\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:04:30.057+00:00\"},\"s\":\"I\", \"c\":\"FTDC\", \"id\":20631, \"ctx\":\"ftdc\",\"msg\":\"Unclean full-time diagnostic data capture shutdown detected, found interim file, some metrics may have been lost\",\"attr\":{\"error\":{\"code\":0,\"codeName\":\"OK\"}}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:04:39.257+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":22943, \"ctx\":\"listener\",\"msg\":\"Connection accepted\",\"attr\":{\"remote\":\"172.18.0.3:38144\",\"connectionId\":1,\"connectionCount\":1}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:04:39.258+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":51800, \"ctx\":\"conn1\",\"msg\":\"client metadata\",\"attr\":{\"remote\":\"172.18.0.3:38144\",\"client\":\"conn1\",\"doc\":{\"application\":{\"name\":\"MongoDB Shell\"},\"driver\":{\"name\":\"MongoDB Internal Client\",\"version\":\"4.4.22\"},\"os\":{\"type\":\"Linux\",\"name\":\"Ubuntu\",\"architecture\":\"x86_64\",\"version\":\"20.04\"}}}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:04:40.995+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":22944, \"ctx\":\"conn1\",\"msg\":\"Connection ended\",\"attr\":{\"remote\":\"172.18.0.3:38144\",\"connectionId\":1,\"connectionCount\":0}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:04:59.469+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":22943, \"ctx\":\"listener\",\"msg\":\"Connection accepted\",\"attr\":{\"remote\":\"172.18.0.3:48658\",\"connectionId\":2,\"connectionCount\":1}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:04:59.496+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":51800, \"ctx\":\"conn2\",\"msg\":\"client metadata\",\"attr\":{\"remote\":\"172.18.0.3:48658\",\"client\":\"conn2\",\"doc\":{\"application\":{\"name\":\"MongoDB Shell\"},\"driver\":{\"name\":\"MongoDB Internal Client\",\"version\":\"4.4.22\"},\"os\":{\"type\":\"Linux\",\"name\":\"Ubuntu\",\"architecture\":\"x86_64\",\"version\":\"20.04\"}}}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:04:59.679+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":22944, \"ctx\":\"conn2\",\"msg\":\"Connection ended\",\"attr\":{\"remote\":\"172.18.0.3:48658\",\"connectionId\":2,\"connectionCount\":0}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:05:16.191+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":22943, \"ctx\":\"listener\",\"msg\":\"Connection accepted\",\"attr\":{\"remote\":\"172.18.0.3:41614\",\"connectionId\":3,\"connectionCount\":1}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:05:16.191+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":51800, \"ctx\":\"conn3\",\"msg\":\"client metadata\",\"attr\":{\"remote\":\"172.18.0.3:41614\",\"client\":\"conn3\",\"doc\":{\"application\":{\"name\":\"MongoDB Shell\"},\"driver\":{\"name\":\"MongoDB Internal Client\",\"version\":\"4.4.22\"},\"os\":{\"type\":\"Linux\",\"name\":\"Ubuntu\",\"architecture\":\"x86_64\",\"version\":\"20.04\"}}}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:05:16.382+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":22944, \"ctx\":\"conn3\",\"msg\":\"Connection ended\",\"attr\":{\"remote\":\"172.18.0.3:41614\",\"connectionId\":3,\"connectionCount\":0}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:05:28.707+00:00\"},\"s\":\"I\", \"c\":\"STORAGE\", \"id\":22430, \"ctx\":\"WTCheckpointThread\",\"msg\":\"WiredTiger message\",\"attr\":{\"message\":\"[1686135928:707384][1:0x7f0922c0e700], WT_SESSION.checkpoint: [WT_VERB_CHECKPOINT_PROGRESS] saving checkpoint snapshot min: 18, snapshot max: 18 snapshot count: 0, oldest timestamp: (0, 0) , meta checkpoint timestamp: (0, 0) base write gen: 7\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:05:31.571+00:00\"},\"s\":\"I\", \"c\":\"COMMAND\", \"id\":23099, \"ctx\":\"PeriodicTaskRunner\",\"msg\":\"Task finished\",\"attr\":{\"taskName\":\"DBConnectionPool-cleaner\",\"durationMillis\":1702}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:05:33.168+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":22943, \"ctx\":\"listener\",\"msg\":\"Connection accepted\",\"attr\":{\"remote\":\"172.18.0.3:46880\",\"connectionId\":4,\"connectionCount\":1}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:05:33.169+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":51800, \"ctx\":\"conn4\",\"msg\":\"client metadata\",\"attr\":{\"remote\":\"172.18.0.3:46880\",\"client\":\"conn4\",\"doc\":{\"application\":{\"name\":\"MongoDB Shell\"},\"driver\":{\"name\":\"MongoDB Internal Client\",\"version\":\"4.4.22\"},\"os\":{\"type\":\"Linux\",\"name\":\"Ubuntu\",\"architecture\":\"x86_64\",\"version\":\"20.04\"}}}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:05:33.366+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":22944, \"ctx\":\"conn4\",\"msg\":\"Connection ended\",\"attr\":{\"remote\":\"172.18.0.3:46880\",\"connectionId\":4,\"connectionCount\":0}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:05:50.005+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":22943, \"ctx\":\"listener\",\"msg\":\"Connection accepted\",\"attr\":{\"remote\":\"172.18.0.3:47756\",\"connectionId\":5,\"connectionCount\":1}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:05:50.006+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":51800, \"ctx\":\"conn5\",\"msg\":\"client metadata\",\"attr\":{\"remote\":\"172.18.0.3:47756\",\"client\":\"conn5\",\"doc\":{\"application\":{\"name\":\"MongoDB Shell\"},\"driver\":{\"name\":\"MongoDB Internal Client\",\"version\":\"4.4.22\"},\"os\":{\"type\":\"Linux\",\"name\":\"Ubuntu\",\"architecture\":\"x86_64\",\"version\":\"20.04\"}}}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:05:50.106+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":22944, \"ctx\":\"conn5\",\"msg\":\"Connection ended\",\"attr\":{\"remote\":\"172.18.0.3:47756\",\"connectionId\":5,\"connectionCount\":0}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:05:56.926+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":22943, \"ctx\":\"listener\",\"msg\":\"Connection accepted\",\"attr\":{\"remote\":\"172.18.0.7:34006\",\"connectionId\":6,\"connectionCount\":1}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:05:57.585+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":51800, \"ctx\":\"conn6\",\"msg\":\"client metadata\",\"attr\":{\"remote\":\"172.18.0.7:34006\",\"client\":\"conn6\",\"doc\":{\"driver\":{\"name\":\"mongo-go-driver\",\"version\":\"v1.3.5\"},\"os\":{\"type\":\"linux\",\"architecture\":\"amd64\"},\"platform\":\"go1.20.4\"}}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:05:57.601+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":22943, \"ctx\":\"listener\",\"msg\":\"Connection accepted\",\"attr\":{\"remote\":\"172.18.0.7:34020\",\"connectionId\":7,\"connectionCount\":2}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:05:57.619+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":51800, \"ctx\":\"conn7\",\"msg\":\"client metadata\",\"attr\":{\"remote\":\"172.18.0.7:34020\",\"client\":\"conn7\",\"doc\":{\"driver\":{\"name\":\"mongo-go-driver\",\"version\":\"v1.3.5\"},\"os\":{\"type\":\"linux\",\"architecture\":\"amd64\"},\"platform\":\"go1.20.4\"}}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:05:59.871+00:00\"},\"s\":\"I\", \"c\":\"ACCESS\", \"id\":20251, \"ctx\":\"conn7\",\"msg\":\"Supported SASL mechanisms requested for unknown user\",\"attr\":{\"user\":\"admin@admin\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:06:01.399+00:00\"},\"s\":\"I\", \"c\":\"COMMAND\", \"id\":51803, \"ctx\":\"conn7\",\"msg\":\"Slow query\",\"attr\":{\"type\":\"command\",\"ns\":\"admin.$cmd\",\"command\":{\"isMaster\":1,\"saslSupportedMechs\":\"admin.admin\",\"compression\":[],\"client\":{\"driver\":{\"name\":\"mongo-go-driver\",\"version\":\"v1.3.5\"},\"os\":{\"type\":\"linux\",\"architecture\":\"amd64\"},\"platform\":\"go1.20.4\"},\"$readPreference\":{\"mode\":\"secondaryPreferred\"},\"$db\":\"admin\"},\"numYields\":0,\"reslen\":319,\"locks\":{},\"protocol\":\"op_query\",\"durationMillis\":2792}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:06:01.779+00:00\"},\"s\":\"I\", \"c\":\"ACCESS\", \"id\":20249, \"ctx\":\"conn7\",\"msg\":\"Authentication failed\",\"attr\":{\"mechanism\":\"SCRAM-SHA-1\",\"speculative\":false,\"principalName\":\"admin\",\"authenticationDatabase\":\"admin\",\"remote\":\"172.18.0.7:34020\",\"extraInfo\":{},\"error\":\"UserNotFound: Could not find user \\\"admin\\\" for db \\\"admin\\\"\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:06:01.795+00:00\"},\"s\":\"I\", \"c\":\"COMMAND\", \"id\":51803, \"ctx\":\"conn7\",\"msg\":\"Slow query\",\"attr\":{\"type\":\"command\",\"ns\":\"admin.$cmd\",\"command\":{\"saslStart\":1,\"mechanism\":\"SCRAM-SHA-1\",\"payload\":\"xxx\",\"$db\":\"admin\"},\"numYields\":0,\"ok\":0,\"errMsg\":\"Authentication failed.\",\"errName\":\"AuthenticationFailed\",\"errCode\":18,\"reslen\":118,\"locks\":{},\"protocol\":\"op_msg\",\"durationMillis\":106}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:06:01.796+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":22944, \"ctx\":\"conn7\",\"msg\":\"Connection ended\",\"attr\":{\"remote\":\"172.18.0.7:34020\",\"connectionId\":7,\"connectionCount\":1}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:06:01.833+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":22944, \"ctx\":\"conn6\",\"msg\":\"Connection ended\",\"attr\":{\"remote\":\"172.18.0.7:34006\",\"connectionId\":6,\"connectionCount\":0}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:06:06.303+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":22943, \"ctx\":\"listener\",\"msg\":\"Connection accepted\",\"attr\":{\"remote\":\"172.18.0.3:54760\",\"connectionId\":8,\"connectionCount\":1}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:06:06.304+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":51800, \"ctx\":\"conn8\",\"msg\":\"client metadata\",\"attr\":{\"remote\":\"172.18.0.3:54760\",\"client\":\"conn8\",\"doc\":{\"application\":{\"name\":\"MongoDB Shell\"},\"driver\":{\"name\":\"MongoDB Internal Client\",\"version\":\"4.4.22\"},\"os\":{\"type\":\"Linux\",\"name\":\"Ubuntu\",\"architecture\":\"x86_64\",\"version\":\"20.04\"}}}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:06:06.498+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":22944, \"ctx\":\"conn8\",\"msg\":\"Connection ended\",\"attr\":{\"remote\":\"172.18.0.3:54760\",\"connectionId\":8,\"connectionCount\":0}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:06:22.268+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":22943, \"ctx\":\"listener\",\"msg\":\"Connection accepted\",\"attr\":{\"remote\":\"172.18.0.3:33884\",\"connectionId\":9,\"connectionCount\":1}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:06:22.269+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":51800, \"ctx\":\"conn9\",\"msg\":\"client metadata\",\"attr\":{\"remote\":\"172.18.0.3:33884\",\"client\":\"conn9\",\"doc\":{\"application\":{\"name\":\"MongoDB Shell\"},\"driver\":{\"name\":\"MongoDB Internal Client\",\"version\":\"4.4.22\"},\"os\":{\"type\":\"Linux\",\"name\":\"Ubuntu\",\"architecture\":\"x86_64\",\"version\":\"20.04\"}}}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:06:22.380+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":22944, \"ctx\":\"conn9\",\"msg\":\"Connection ended\",\"attr\":{\"remote\":\"172.18.0.3:33884\",\"connectionId\":9,\"connectionCount\":0}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:06:28.764+00:00\"},\"s\":\"I\", \"c\":\"STORAGE\", \"id\":22430, \"ctx\":\"WTCheckpointThread\",\"msg\":\"WiredTiger message\",\"attr\":{\"message\":\"[1686135988:764217][1:0x7f0922c0e700], WT_SESSION.checkpoint: [WT_VERB_CHECKPOINT_PROGRESS] saving checkpoint snapshot min: 21, snapshot max: 21 snapshot count: 0, oldest timestamp: (0, 0) , meta checkpoint timestamp: (0, 0) base write gen: 7\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:06:38.378+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":22943, \"ctx\":\"listener\",\"msg\":\"Connection accepted\",\"attr\":{\"remote\":\"172.18.0.3:33064\",\"connectionId\":10,\"connectionCount\":1}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:06:38.386+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":51800, \"ctx\":\"conn10\",\"msg\":\"client metadata\",\"attr\":{\"remote\":\"172.18.0.3:33064\",\"client\":\"conn10\",\"doc\":{\"application\":{\"name\":\"MongoDB Shell\"},\"driver\":{\"name\":\"MongoDB Internal Client\",\"version\":\"4.4.22\"},\"os\":{\"type\":\"Linux\",\"name\":\"Ubuntu\",\"architecture\":\"x86_64\",\"version\":\"20.04\"}}}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:06:38.579+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":22944, \"ctx\":\"conn10\",\"msg\":\"Connection ended\",\"attr\":{\"remote\":\"172.18.0.3:33064\",\"connectionId\":10,\"connectionCount\":0}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:06:40.387+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":22943, \"ctx\":\"listener\",\"msg\":\"Connection accepted\",\"attr\":{\"remote\":\"172.18.0.7:35868\",\"connectionId\":11,\"connectionCount\":1}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:06:40.476+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":51800, \"ctx\":\"conn11\",\"msg\":\"client metadata\",\"attr\":{\"remote\":\"172.18.0.7:35868\",\"client\":\"conn11\",\"doc\":{\"driver\":{\"name\":\"mongo-go-driver\",\"version\":\"v1.3.5\"},\"os\":{\"type\":\"linux\",\"architecture\":\"amd64\"},\"platform\":\"go1.20.4\"}}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:06:40.513+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":22943, \"ctx\":\"listener\",\"msg\":\"Connection accepted\",\"attr\":{\"remote\":\"172.18.0.7:35876\",\"connectionId\":12,\"connectionCount\":2}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:06:40.513+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":51800, \"ctx\":\"conn12\",\"msg\":\"client metadata\",\"attr\":{\"remote\":\"172.18.0.7:35876\",\"client\":\"conn12\",\"doc\":{\"driver\":{\"name\":\"mongo-go-driver\",\"version\":\"v1.3.5\"},\"os\":{\"type\":\"linux\",\"architecture\":\"amd64\"},\"platform\":\"go1.20.4\"}}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:06:40.524+00:00\"},\"s\":\"I\", \"c\":\"ACCESS\", \"id\":20251, \"ctx\":\"conn12\",\"msg\":\"Supported SASL mechanisms requested for unknown user\",\"attr\":{\"user\":\"admin@admin\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:06:40.525+00:00\"},\"s\":\"I\", \"c\":\"ACCESS\", \"id\":20249, \"ctx\":\"conn12\",\"msg\":\"Authentication failed\",\"attr\":{\"mechanism\":\"SCRAM-SHA-1\",\"speculative\":false,\"principalName\":\"admin\",\"authenticationDatabase\":\"admin\",\"remote\":\"172.18.0.7:35876\",\"extraInfo\":{},\"error\":\"UserNotFound: Could not find user \\\"admin\\\" for db \\\"admin\\\"\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:06:40.526+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":22944, \"ctx\":\"conn12\",\"msg\":\"Connection ended\",\"attr\":{\"remote\":\"172.18.0.7:35876\",\"connectionId\":12,\"connectionCount\":1}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:06:40.567+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":22944, \"ctx\":\"conn11\",\"msg\":\"Connection ended\",\"attr\":{\"remote\":\"172.18.0.7:35868\",\"connectionId\":11,\"connectionCount\":0}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:06:54.472+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":22943, \"ctx\":\"listener\",\"msg\":\"Connection accepted\",\"attr\":{\"remote\":\"172.18.0.3:41320\",\"connectionId\":13,\"connectionCount\":1}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:06:54.474+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":51800, \"ctx\":\"conn13\",\"msg\":\"client metadata\",\"attr\":{\"remote\":\"172.18.0.3:41320\",\"client\":\"conn13\",\"doc\":{\"application\":{\"name\":\"MongoDB Shell\"},\"driver\":{\"name\":\"MongoDB Internal Client\",\"version\":\"4.4.22\"},\"os\":{\"type\":\"Linux\",\"name\":\"Ubuntu\",\"architecture\":\"x86_64\",\"version\":\"20.04\"}}}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:06:54.569+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":22944, \"ctx\":\"conn13\",\"msg\":\"Connection ended\",\"attr\":{\"remote\":\"172.18.0.3:41320\",\"connectionId\":13,\"connectionCount\":0}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:07:10.569+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":22943, \"ctx\":\"listener\",\"msg\":\"Connection accepted\",\"attr\":{\"remote\":\"172.18.0.3:44538\",\"connectionId\":14,\"connectionCount\":1}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:07:10.570+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":51800, \"ctx\":\"conn14\",\"msg\":\"client metadata\",\"attr\":{\"remote\":\"172.18.0.3:44538\",\"client\":\"conn14\",\"doc\":{\"application\":{\"name\":\"MongoDB Shell\"},\"driver\":{\"name\":\"MongoDB Internal Client\",\"version\":\"4.4.22\"},\"os\":{\"type\":\"Linux\",\"name\":\"Ubuntu\",\"architecture\":\"x86_64\",\"version\":\"20.04\"}}}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:07:10.668+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":22944, \"ctx\":\"conn14\",\"msg\":\"Connection ended\",\"attr\":{\"remote\":\"172.18.0.3:44538\",\"connectionId\":14,\"connectionCount\":0}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:07:27.069+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":22943, \"ctx\":\"listener\",\"msg\":\"Connection accepted\",\"attr\":{\"remote\":\"172.18.0.3:56014\",\"connectionId\":15,\"connectionCount\":1}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:07:27.070+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":51800, \"ctx\":\"conn15\",\"msg\":\"client metadata\",\"attr\":{\"remote\":\"172.18.0.3:56014\",\"client\":\"conn15\",\"doc\":{\"application\":{\"name\":\"MongoDB Shell\"},\"driver\":{\"name\":\"MongoDB Internal Client\",\"version\":\"4.4.22\"},\"os\":{\"type\":\"Linux\",\"name\":\"Ubuntu\",\"architecture\":\"x86_64\",\"version\":\"20.04\"}}}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:07:27.172+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":22944, \"ctx\":\"conn15\",\"msg\":\"Connection ended\",\"attr\":{\"remote\":\"172.18.0.3:56014\",\"connectionId\":15,\"connectionCount\":0}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:07:28.818+00:00\"},\"s\":\"I\", \"c\":\"STORAGE\", \"id\":22430, \"ctx\":\"WTCheckpointThread\",\"msg\":\"WiredTiger message\",\"attr\":{\"message\":\"[1686136048:818265][1:0x7f0922c0e700], WT_SESSION.checkpoint: [WT_VERB_CHECKPOINT_PROGRESS] saving checkpoint snapshot min: 23, snapshot max: 23 snapshot count: 0, oldest timestamp: (0, 0) , meta checkpoint timestamp: (0, 0) base write gen: 7\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:07:42.870+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":22943, \"ctx\":\"listener\",\"msg\":\"Connection accepted\",\"attr\":{\"remote\":\"172.18.0.3:58318\",\"connectionId\":16,\"connectionCount\":1}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:07:42.871+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":51800, \"ctx\":\"conn16\",\"msg\":\"client metadata\",\"attr\":{\"remote\":\"172.18.0.3:58318\",\"client\":\"conn16\",\"doc\":{\"application\":{\"name\":\"MongoDB Shell\"},\"driver\":{\"name\":\"MongoDB Internal Client\",\"version\":\"4.4.22\"},\"os\":{\"type\":\"Linux\",\"name\":\"Ubuntu\",\"architecture\":\"x86_64\",\"version\":\"20.04\"}}}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:07:42.994+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":22944, \"ctx\":\"conn16\",\"msg\":\"Connection ended\",\"attr\":{\"remote\":\"172.18.0.3:58318\",\"connectionId\":16,\"connectionCount\":0}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:07:58.492+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":22943, \"ctx\":\"listener\",\"msg\":\"Connection accepted\",\"attr\":{\"remote\":\"172.18.0.3:38162\",\"connectionId\":17,\"connectionCount\":1}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:07:58.566+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":51800, \"ctx\":\"conn17\",\"msg\":\"client metadata\",\"attr\":{\"remote\":\"172.18.0.3:38162\",\"client\":\"conn17\",\"doc\":{\"application\":{\"name\":\"MongoDB Shell\"},\"driver\":{\"name\":\"MongoDB Internal Client\",\"version\":\"4.4.22\"},\"os\":{\"type\":\"Linux\",\"name\":\"Ubuntu\",\"architecture\":\"x86_64\",\"version\":\"20.04\"}}}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:07:58.778+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":22944, \"ctx\":\"conn17\",\"msg\":\"Connection ended\",\"attr\":{\"remote\":\"172.18.0.3:38162\",\"connectionId\":17,\"connectionCount\":0}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:08:14.394+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":22943, \"ctx\":\"listener\",\"msg\":\"Connection accepted\",\"attr\":{\"remote\":\"172.18.0.3:33518\",\"connectionId\":18,\"connectionCount\":1}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:08:14.395+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":51800, \"ctx\":\"conn18\",\"msg\":\"client metadata\",\"attr\":{\"remote\":\"172.18.0.3:33518\",\"client\":\"conn18\",\"doc\":{\"application\":{\"name\":\"MongoDB Shell\"},\"driver\":{\"name\":\"MongoDB Internal Client\",\"version\":\"4.4.22\"},\"os\":{\"type\":\"Linux\",\"name\":\"Ubuntu\",\"architecture\":\"x86_64\",\"version\":\"20.04\"}}}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:08:14.484+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":22944, \"ctx\":\"conn18\",\"msg\":\"Connection ended\",\"attr\":{\"remote\":\"172.18.0.3:33518\",\"connectionId\":18,\"connectionCount\":0}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:08:28.908+00:00\"},\"s\":\"I\", \"c\":\"STORAGE\", \"id\":22430, \"ctx\":\"WTCheckpointThread\",\"msg\":\"WiredTiger message\",\"attr\":{\"message\":\"[1686136108:908044][1:0x7f0922c0e700], WT_SESSION.checkpoint: [WT_VERB_CHECKPOINT_PROGRESS] saving checkpoint snapshot min: 25, snapshot max: 25 snapshot count: 0, oldest timestamp: (0, 0) , meta checkpoint timestamp: (0, 0) base write gen: 7\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:08:49.778+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":22943, \"ctx\":\"listener\",\"msg\":\"Connection accepted\",\"attr\":{\"remote\":\"172.18.0.3:47224\",\"connectionId\":19,\"connectionCount\":1}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:08:49.817+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":51800, \"ctx\":\"conn19\",\"msg\":\"client metadata\",\"attr\":{\"remote\":\"172.18.0.3:47224\",\"client\":\"conn19\",\"doc\":{\"application\":{\"name\":\"MongoDB Shell\"},\"driver\":{\"name\":\"MongoDB Internal Client\",\"version\":\"4.4.22\"},\"os\":{\"type\":\"Linux\",\"name\":\"Ubuntu\",\"architecture\":\"x86_64\",\"version\":\"20.04\"}}}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:08:51.268+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":22944, \"ctx\":\"conn19\",\"msg\":\"Connection ended\",\"attr\":{\"remote\":\"172.18.0.3:47224\",\"connectionId\":19,\"connectionCount\":0}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:09:11.107+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":22943, \"ctx\":\"listener\",\"msg\":\"Connection accepted\",\"attr\":{\"remote\":\"172.18.0.3:47016\",\"connectionId\":20,\"connectionCount\":1}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:09:12.077+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":51800, \"ctx\":\"conn20\",\"msg\":\"client metadata\",\"attr\":{\"remote\":\"172.18.0.3:47016\",\"client\":\"conn20\",\"doc\":{\"application\":{\"name\":\"MongoDB Shell\"},\"driver\":{\"name\":\"MongoDB Internal Client\",\"version\":\"4.4.22\"},\"os\":{\"type\":\"Linux\",\"name\":\"Ubuntu\",\"architecture\":\"x86_64\",\"version\":\"20.04\"}}}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:09:24.584+00:00\"},\"s\":\"I\", \"c\":\"COMMAND\", \"id\":51803, \"ctx\":\"conn20\",\"msg\":\"Slow query\",\"attr\":{\"type\":\"command\",\"ns\":\"admin.$cmd\",\"appName\":\"MongoDB Shell\",\"command\":{\"isMaster\":1,\"client\":{\"application\":{\"name\":\"MongoDB Shell\"},\"driver\":{\"name\":\"MongoDB Internal Client\",\"version\":\"4.4.22\"},\"os\":{\"type\":\"Linux\",\"name\":\"Ubuntu\",\"architecture\":\"x86_64\",\"version\":\"20.04\"}},\"$db\":\"admin\"},\"numYields\":0,\"reslen\":319,\"locks\":{},\"protocol\":\"op_query\",\"durationMillis\":695}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:09:29.833+00:00\"},\"s\":\"I\", \"c\":\"COMMAND\", \"id\":51803, \"ctx\":\"conn20\",\"msg\":\"Slow query\",\"attr\":{\"type\":\"command\",\"ns\":\"test.$cmd\",\"appName\":\"MongoDB Shell\",\"command\":{\"isMaster\":1.0,\"forShell\":1.0,\"$db\":\"test\"},\"numYields\":0,\"reslen\":304,\"locks\":{},\"protocol\":\"op_msg\",\"durationMillis\":239}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:09:38.679+00:00\"},\"s\":\"I\", \"c\":\"COMMAND\", \"id\":20499, \"ctx\":\"ftdc\",\"msg\":\"serverStatus was very slow\",\"attr\":{\"timeStats\":{\"after basic\":8,\"after asserts\":8,\"after connections\":8,\"after electionMetrics\":8,\"after extra_info\":8,\"after flowControl\":8,\"after globalLock\":118,\"after indexBulkBuilder\":118,\"after locks\":118,\"after logicalSessionRecordCache\":7021,\"after mirroredReads\":7021,\"after network\":7021,\"after opLatencies\":7021,\"after opReadConcernCounters\":7021,\"after opcounters\":7021,\"after opcountersRepl\":7021,\"after oplog\":7021,\"after oplogTruncation\":7119,\"after repl\":7119,\"after scramCache\":7119,\"after security\":7119,\"after storageEngine\":7119,\"after tcmalloc\":7119,\"after trafficRecording\":7119,\"after transactions\":7119,\"after transportSecurity\":7119,\"after twoPhaseCommitCoordinator\":7119,\"after wiredTiger\":7527,\"at end\":8600}}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:09:41.471+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":22944, \"ctx\":\"conn20\",\"msg\":\"Connection ended\",\"attr\":{\"remote\":\"172.18.0.3:47016\",\"connectionId\":20,\"connectionCount\":0}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:09:47.168+00:00\"},\"s\":\"I\", \"c\":\"COMMAND\", \"id\":20499, \"ctx\":\"ftdc\",\"msg\":\"serverStatus was very slow\",\"attr\":{\"timeStats\":{\"after basic\":0,\"after asserts\":0,\"after connections\":0,\"after electionMetrics\":0,\"after extra_info\":423,\"after flowControl\":423,\"after globalLock\":423,\"after indexBulkBuilder\":423,\"after locks\":423,\"after logicalSessionRecordCache\":423,\"after mirroredReads\":423,\"after network\":423,\"after opLatencies\":423,\"after opReadConcernCounters\":423,\"after opcounters\":423,\"after opcountersRepl\":423,\"after oplog\":423,\"after oplogTruncation\":550,\"after repl\":550,\"after scramCache\":550,\"after security\":550,\"after storageEngine\":550,\"after tcmalloc\":550,\"after trafficRecording\":550,\"after transactions\":550,\"after transportSecurity\":550,\"after twoPhaseCommitCoordinator\":550,\"after wiredTiger\":550,\"at end\":1110}}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:09:54.376+00:00\"},\"s\":\"I\", \"c\":\"COMMAND\", \"id\":51803, \"ctx\":\"LogicalSessionCacheReap\",\"msg\":\"Slow query\",\"attr\":{\"type\":\"command\",\"ns\":\"config.system.sessions\",\"command\":{\"listIndexes\":\"system.sessions\",\"cursor\":{},\"$db\":\"config\"},\"numYields\":0,\"reslen\":245,\"locks\":{\"FeatureCompatibilityVersion\":{\"acquireCount\":{\"r\":1}},\"ReplicationStateTransition\":{\"acquireCount\":{\"w\":1}},\"Global\":{\"acquireCount\":{\"r\":1}},\"Database\":{\"acquireCount\":{\"r\":1}},\"Collection\":{\"acquireCount\":{\"r\":1}},\"Mutex\":{\"acquireCount\":{\"r\":1}}},\"storage\":{},\"protocol\":\"op_msg\",\"durationMillis\":10399}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:09:54.675+00:00\"},\"s\":\"I\", \"c\":\"STORAGE\", \"id\":22430, \"ctx\":\"WTCheckpointThread\",\"msg\":\"WiredTiger message\",\"attr\":{\"message\":\"[1686136194:675072][1:0x7f0922c0e700], WT_SESSION.checkpoint: [WT_VERB_CHECKPOINT_PROGRESS] saving checkpoint snapshot min: 27, snapshot max: 27 snapshot count: 0, oldest timestamp: (0, 0) , meta checkpoint timestamp: (0, 0) base write gen: 7\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:09:56.492+00:00\"},\"s\":\"I\", \"c\":\"COMMAND\", \"id\":20499, \"ctx\":\"ftdc\",\"msg\":\"serverStatus was very slow\",\"attr\":{\"timeStats\":{\"after basic\":869,\"after asserts\":869,\"after connections\":869,\"after electionMetrics\":869,\"after extra_info\":1042,\"after flowControl\":1042,\"after globalLock\":1042,\"after indexBulkBuilder\":1042,\"after locks\":1042,\"after logicalSessionRecordCache\":1042,\"after mirroredReads\":1042,\"after network\":1042,\"after opLatencies\":1042,\"after opReadConcernCounters\":1042,\"after opcounters\":1042,\"after opcountersRepl\":1042,\"after oplog\":1042,\"after oplogTruncation\":1237,\"after repl\":1237,\"after scramCache\":1237,\"after security\":1237,\"after storageEngine\":1237,\"after tcmalloc\":1237,\"after trafficRecording\":1237,\"after transactions\":1237,\"after transportSecurity\":1237,\"after twoPhaseCommitCoordinator\":1237,\"after wiredTiger\":1238,\"at end\":1414}}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:09:59.266+00:00\"},\"s\":\"I\", \"c\":\"COMMAND\", \"id\":51803, \"ctx\":\"LogicalSessionCacheRefresh\",\"msg\":\"Slow query\",\"attr\":{\"type\":\"command\",\"ns\":\"config.system.sessions\",\"command\":{\"listIndexes\":\"system.sessions\",\"cursor\":{},\"$db\":\"config\"},\"numYields\":0,\"reslen\":245,\"locks\":{\"FeatureCompatibilityVersion\":{\"acquireCount\":{\"r\":1}},\"ReplicationStateTransition\":{\"acquireCount\":{\"w\":1}},\"Global\":{\"acquireCount\":{\"r\":1}},\"Database\":{\"acquireCount\":{\"r\":1}},\"Collection\":{\"acquireCount\":{\"r\":1}},\"Mutex\":{\"acquireCount\":{\"r\":1}}},\"storage\":{},\"protocol\":\"op_msg\",\"durationMillis\":15195}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:09:59.614+00:00\"},\"s\":\"I\", \"c\":\"COMMAND\", \"id\":51803, \"ctx\":\"LogicalSessionCacheReap\",\"msg\":\"Slow query\",\"attr\":{\"type\":\"command\",\"ns\":\"config.transactions\",\"command\":{\"find\":\"transactions\",\"filter\":{\"lastWriteDate\":{\"$lt\":{\"$date\":\"2023-06-07T10:39:54.489Z\"}}},\"projection\":{\"_id\":1},\"sort\":{\"_id\":1},\"readConcern\":{},\"$db\":\"config\"},\"planSummary\":\"EOF\",\"keysExamined\":0,\"docsExamined\":0,\"cursorExhausted\":true,\"numYields\":0,\"nreturned\":0,\"reslen\":108,\"locks\":{\"FeatureCompatibilityVersion\":{\"acquireCount\":{\"r\":1}},\"ReplicationStateTransition\":{\"acquireCount\":{\"w\":1}},\"Global\":{\"acquireCount\":{\"r\":1}},\"Database\":{\"acquireCount\":{\"r\":1}},\"Collection\":{\"acquireCount\":{\"r\":2}},\"Mutex\":{\"acquireCount\":{\"r\":1}}},\"readConcern\":{\"provenance\":\"clientSupplied\"},\"storage\":{},\"protocol\":\"op_msg\",\"durationMillis\":5113}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:10:31.918+00:00\"},\"s\":\"I\", \"c\":\"COMMAND\", \"id\":20499, \"ctx\":\"ftdc\",\"msg\":\"serverStatus was very slow\",\"attr\":{\"timeStats\":{\"after basic\":201,\"after asserts\":201,\"after connections\":201,\"after electionMetrics\":201,\"after extra_info\":534,\"after flowControl\":534,\"after globalLock\":534,\"after indexBulkBuilder\":534,\"after locks\":534,\"after logicalSessionRecordCache\":534,\"after mirroredReads\":534,\"after network\":534,\"after opLatencies\":534,\"after opReadConcernCounters\":534,\"after opcounters\":534,\"after opcountersRepl\":534,\"after oplog\":534,\"after oplogTruncation\":534,\"after repl\":534,\"after scramCache\":534,\"after security\":534,\"after storageEngine\":534,\"after tcmalloc\":534,\"after trafficRecording\":534,\"after transactions\":534,\"after transportSecurity\":534,\"after twoPhaseCommitCoordinator\":534,\"after wiredTiger\":534,\"at end\":1222}}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:10:51.873+00:00\"},\"s\":\"I\", \"c\":\"COMMAND\", \"id\":23099, \"ctx\":\"PeriodicTaskRunner\",\"msg\":\"Task finished\",\"attr\":{\"taskName\":\"DBConnectionPool-cleaner\",\"durationMillis\":4405}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:10:56.066+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":22943, \"ctx\":\"listener\",\"msg\":\"Connection accepted\",\"attr\":{\"remote\":\"172.18.0.3:60452\",\"connectionId\":21,\"connectionCount\":1}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:10:57.267+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":22943, \"ctx\":\"listener\",\"msg\":\"Connection accepted\",\"attr\":{\"remote\":\"172.18.0.3:60458\",\"connectionId\":22,\"connectionCount\":2}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:10:58.669+00:00\"},\"s\":\"I\", \"c\":\"COMMAND\", \"id\":23099, \"ctx\":\"PeriodicTaskRunner\",\"msg\":\"Task finished\",\"attr\":{\"taskName\":\"UnusedLockCleaner\",\"durationMillis\":6795}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:11:03.580+00:00\"},\"s\":\"I\", \"c\":\"COMMAND\", \"id\":20499, \"ctx\":\"ftdc\",\"msg\":\"serverStatus was very slow\",\"attr\":{\"timeStats\":{\"after basic\":100,\"after asserts\":100,\"after connections\":100,\"after electionMetrics\":100,\"after extra_info\":403,\"after flowControl\":403,\"after globalLock\":403,\"after indexBulkBuilder\":403,\"after locks\":905,\"after logicalSessionRecordCache\":905,\"after mirroredReads\":905,\"after network\":905,\"after opLatencies\":905,\"after opReadConcernCounters\":905,\"after opcounters\":905,\"after opcountersRepl\":905,\"after oplog\":905,\"after oplogTruncation\":907,\"after repl\":907,\"after scramCache\":907,\"after security\":907,\"after storageEngine\":907,\"after tcmalloc\":907,\"after trafficRecording\":907,\"after transactions\":907,\"after transportSecurity\":907,\"after twoPhaseCommitCoordinator\":907,\"after wiredTiger\":907,\"at end\":1313}}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:11:05.968+00:00\"},\"s\":\"I\", \"c\":\"STORAGE\", \"id\":22430, \"ctx\":\"WTCheckpointThread\",\"msg\":\"WiredTiger message\",\"attr\":{\"message\":\"[1686136265:968123][1:0x7f0922c0e700], WT_SESSION.checkpoint: [WT_VERB_CHECKPOINT_PROGRESS] saving checkpoint snapshot min: 29, snapshot max: 29 snapshot count: 0, oldest timestamp: (0, 0) , meta checkpoint timestamp: (0, 0) base write gen: 7\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:11:19.069+00:00\"},\"s\":\"I\", \"c\":\"COMMAND\", \"id\":20499, \"ctx\":\"ftdc\",\"msg\":\"serverStatus was very slow\",\"attr\":{\"timeStats\":{\"after basic\":0,\"after asserts\":0,\"after connections\":0,\"after electionMetrics\":0,\"after extra_info\":460,\"after flowControl\":460,\"after globalLock\":460,\"after indexBulkBuilder\":460,\"after locks\":460,\"after logicalSessionRecordCache\":460,\"after mirroredReads\":460,\"after network\":460,\"after opLatencies\":460,\"after opReadConcernCounters\":460,\"after opcounters\":460,\"after opcountersRepl\":460,\"after oplog\":460,\"after oplogTruncation\":466,\"after repl\":466,\"after scramCache\":466,\"after security\":466,\"after storageEngine\":466,\"after tcmalloc\":466,\"after trafficRecording\":466,\"after transactions\":466,\"after transportSecurity\":466,\"after twoPhaseCommitCoordinator\":466,\"after wiredTiger\":466,\"at end\":1062}}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:11:14.168+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":51800, \"ctx\":\"conn21\",\"msg\":\"client metadata\",\"attr\":{\"remote\":\"172.18.0.3:60452\",\"client\":\"conn21\",\"doc\":{\"application\":{\"name\":\"MongoDB Shell\"},\"driver\":{\"name\":\"MongoDB Internal Client\",\"version\":\"4.4.22\"},\"os\":{\"type\":\"Linux\",\"name\":\"Ubuntu\",\"architecture\":\"x86_64\",\"version\":\"20.04\"}}}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:11:14.168+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":51800, \"ctx\":\"conn22\",\"msg\":\"client metadata\",\"attr\":{\"remote\":\"172.18.0.3:60458\",\"client\":\"conn22\",\"doc\":{\"application\":{\"name\":\"MongoDB Shell\"},\"driver\":{\"name\":\"MongoDB Internal Client\",\"version\":\"4.4.22\"},\"os\":{\"type\":\"Linux\",\"name\":\"Ubuntu\",\"architecture\":\"x86_64\",\"version\":\"20.04\"}}}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:11:42.178+00:00\"},\"s\":\"I\", \"c\":\"COMMAND\", \"id\":51803, \"ctx\":\"conn22\",\"msg\":\"Slow query\",\"attr\":{\"type\":\"command\",\"ns\":\"admin.$cmd\",\"appName\":\"MongoDB Shell\",\"command\":{\"isMaster\":1,\"client\":{\"application\":{\"name\":\"MongoDB Shell\"},\"driver\":{\"name\":\"MongoDB Internal Client\",\"version\":\"4.4.22\"},\"os\":{\"type\":\"Linux\",\"name\":\"Ubuntu\",\"architecture\":\"x86_64\",\"version\":\"20.04\"}},\"$db\":\"admin\"},\"numYields\":0,\"reslen\":319,\"locks\":{},\"protocol\":\"op_query\",\"durationMillis\":26393}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:11:42.170+00:00\"},\"s\":\"I\", \"c\":\"COMMAND\", \"id\":51803, \"ctx\":\"conn21\",\"msg\":\"Slow query\",\"attr\":{\"type\":\"command\",\"ns\":\"admin.$cmd\",\"appName\":\"MongoDB Shell\",\"command\":{\"isMaster\":1,\"client\":{\"application\":{\"name\":\"MongoDB Shell\"},\"driver\":{\"name\":\"MongoDB Internal Client\",\"version\":\"4.4.22\"},\"os\":{\"type\":\"Linux\",\"name\":\"Ubuntu\",\"architecture\":\"x86_64\",\"version\":\"20.04\"}},\"$db\":\"admin\"},\"numYields\":0,\"reslen\":319,\"locks\":{},\"protocol\":\"op_query\",\"durationMillis\":26999}}\r\n\r\nWARNING: MongoDB 5.0+ requires a CPU with AVX support, and your current system does not appear to have that!\r\n see https://jira.mongodb.org/browse/SERVER-54407\r\n see also https://www.mongodb.com/community/forums/t/mongodb-5-0-cpu-intel-g4650-compatibility/116610/2\r\n see also https://github.com/docker-library/mongo/issues/485#issuecomment-891991814\r\n\r\n{\"t\":{\"$date\":\"2023-06-07T11:52:17.003+00:00\"},\"s\":\"I\", \"c\":\"CONTROL\", \"id\":23285, \"ctx\":\"main\",\"msg\":\"Automatically disabling TLS 1.0, to force-enable TLS 1.0 specify --sslDisabledProtocols 'none'\"}\r\n{\"t\":{\"$date\":\"2023-06-07T11:52:17.014+00:00\"},\"s\":\"I\", \"c\":\"NETWORK\", \"id\":4648601, \"ctx\":\"main\",\"msg\":\"Implicit TCP FastOpen unavailable. If TCP FastOpen is required, set tcpFastOpenServer, tcpFastOpenClient, and tcpFastOpenQueueSize.\"}\r\n{\"t\":{\"$date\":\"2023-06-07T11:52:17.016+00:00\"},\"s\":\"I\", \"c\":\"STORAGE\", \"id\":4615611, \"ctx\":\"initandlisten\",\"msg\":\"MongoDB starting\",\"attr\":{\"pid\":1,\"port\":27017,\"dbPath\":\"/data/db\",\"architecture\":\"64-bit\",\"host\":\"a636cf2cec8a\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:52:17.021+00:00\"},\"s\":\"W\", \"c\":\"CONTROL\", \"id\":20720, \"ctx\":\"initandlisten\",\"msg\":\"Available memory is less than system memory\",\"attr\":{\"availableMemSizeMB\":128,\"systemMemSizeMB\":3927}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:52:17.021+00:00\"},\"s\":\"I\", \"c\":\"CONTROL\", \"id\":23403, \"ctx\":\"initandlisten\",\"msg\":\"Build Info\",\"attr\":{\"buildInfo\":{\"version\":\"4.4.22\",\"gitVersion\":\"fc832685b99221cffb1f5bb5a4ff5ad3e1c416b2\",\"openSSLVersion\":\"OpenSSL 1.1.1f 31 Mar 2020\",\"modules\":[],\"allocator\":\"tcmalloc\",\"environment\":{\"distmod\":\"ubuntu2004\",\"distarch\":\"x86_64\",\"target_arch\":\"x86_64\"}}}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:52:17.021+00:00\"},\"s\":\"I\", \"c\":\"CONTROL\", \"id\":51765, \"ctx\":\"initandlisten\",\"msg\":\"Operating System\",\"attr\":{\"os\":{\"name\":\"Ubuntu\",\"version\":\"20.04\"}}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:52:17.021+00:00\"},\"s\":\"I\", \"c\":\"CONTROL\", \"id\":21951, \"ctx\":\"initandlisten\",\"msg\":\"Options set by command line\",\"attr\":{\"options\":{\"net\":{\"bindIp\":\"*\"},\"security\":{\"authorization\":\"enabled\"}}}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:52:17.023+00:00\"},\"s\":\"W\", \"c\":\"STORAGE\", \"id\":22271, \"ctx\":\"initandlisten\",\"msg\":\"Detected unclean shutdown - Lock file is not empty\",\"attr\":{\"lockFile\":\"/data/db/mongod.lock\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:52:17.023+00:00\"},\"s\":\"I\", \"c\":\"STORAGE\", \"id\":22270, \"ctx\":\"initandlisten\",\"msg\":\"Storage engine to use detected by data files\",\"attr\":{\"dbpath\":\"/data/db\",\"storageEngine\":\"wiredTiger\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:52:17.023+00:00\"},\"s\":\"W\", \"c\":\"STORAGE\", \"id\":22302, \"ctx\":\"initandlisten\",\"msg\":\"Recovering data from the last clean checkpoint.\"}\r\n{\"t\":{\"$date\":\"2023-06-07T11:52:17.023+00:00\"},\"s\":\"I\", \"c\":\"STORAGE\", \"id\":22300, \"ctx\":\"initandlisten\",\"msg\":\"The configured WiredTiger cache size is more than 80% of available RAM. See http://dochub.mongodb.org/core/faq-memory-diagnostics-wt\",\"tags\":[\"startupWarnings\"]}\r\n{\"t\":{\"$date\":\"2023-06-07T11:52:17.024+00:00\"},\"s\":\"I\", \"c\":\"STORAGE\", \"id\":22315, \"ctx\":\"initandlisten\",\"msg\":\"Opening WiredTiger\",\"attr\":{\"config\":\"create,cache_size=256M,session_max=33000,eviction=(threads_min=4,threads_max=4),config_base=false,statistics=(fast),log=(enabled=true,archive=true,path=journal,compressor=snappy),file_manager=(close_idle_time=100000,close_scan_interval=10,close_handle_minimum=250),statistics_log=(wait=0),verbose=[recovery_progress,checkpoint_progress,compact_progress],\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:52:20.997+00:00\"},\"s\":\"I\", \"c\":\"STORAGE\", \"id\":22430, \"ctx\":\"initandlisten\",\"msg\":\"WiredTiger message\",\"attr\":{\"message\":\"[1686138740:997479][1:0x7f75326efcc0], txn-recover: [WT_VERB_RECOVERY_PROGRESS] Recovering log 2 through 3\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:52:30.394+00:00\"},\"s\":\"I\", \"c\":\"STORAGE\", \"id\":22430, \"ctx\":\"initandlisten\",\"msg\":\"WiredTiger message\",\"attr\":{\"message\":\"[1686138750:394043][1:0x7f75326efcc0], txn-recover: [WT_VERB_RECOVERY_PROGRESS] Recovering log 3 through 3\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:52:42.413+00:00\"},\"s\":\"I\", \"c\":\"STORAGE\", \"id\":22430, \"ctx\":\"initandlisten\",\"msg\":\"WiredTiger message\",\"attr\":{\"message\":\"[1686138762:413345][1:0x7f75326efcc0], txn-recover: [WT_VERB_RECOVERY | WT_VERB_RECOVERY_PROGRESS] Main recovery loop: starting at 2/8192 to 3/256\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:52:43.283+00:00\"},\"s\":\"I\", \"c\":\"STORAGE\", \"id\":22430, \"ctx\":\"initandlisten\",\"msg\":\"WiredTiger message\",\"attr\":{\"message\":\"[1686138763:283850][1:0x7f75326efcc0], txn-recover: [WT_VERB_RECOVERY_PROGRESS] Recovering log 2 through 3\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:53:01.955+00:00\"},\"s\":\"I\", \"c\":\"STORAGE\", \"id\":22430, \"ctx\":\"initandlisten\",\"msg\":\"WiredTiger message\",\"attr\":{\"message\":\"[1686138781:955463][1:0x7f75326efcc0], txn-recover: [WT_VERB_RECOVERY_PROGRESS] Recovering log 3 through 3\"}}\r\n{\"t\":{\"$date\":\"2023-06-07T11:54:12.205+00:00\"},\"s\":\"I\", \"c\":\"STORAGE\", \"id\":22430, \"ctx\":\"initandlisten\",\"msg\":\"WiredTiger message\",\"attr\":{\"message\":\"[1686138849:906448][1:0x7f75326efcc0], txn-recover: [WT_VERB_RECOVERY | WT_VERB_RECOVERY_PROGRESS] Set global recovery timestamp: (0, 0)\"}}\r\n**Describe the bug**\r\nA clear and concise description of what the bug is.\r\n\r\n**To Reproduce**\r\nSteps to reproduce the behavior.\r\nIf applicable, add screenshots to help explain your problem.\r\n\r\n**Expected behavior**\r\nA clear and concise description of what you expected to happen.\r\n\r\n**Runtime Environment**\r\nSytem/Environemnt information (e.g Output of docker -v and uname -a)\r\n\r\n",
+ "summary": "**Summarize the MongoDB Container Error Issue**\n\n1. **Identify the issue**: Address the error occurring when starting the MongoDB container using Docker. The command run is:\n ```\n sudo VERSION=develop docker-compose -f docker-compose.yml --compatibility up -d\n ```\n The MongoDB container shows an error while others run without issues.\n\n2. **Examine logs**: Review the MongoDB container logs using:\n ```\n docker logs mongodb\n ```\n Key warnings include:\n - MongoDB 5.0+ requires CPU with AVX support, which your system lacks. Reference: [JIRA SERVER-54407](https://jira.mongodb.org/browse/SERVER-54407).\n - Available memory is significantly lower than the system memory.\n - The configured WiredTiger cache size exceeds 80% of available RAM.\n\n3. **Possible causes**:\n - Lack of AVX support in the CPU.\n - Insufficient available memory for MongoDB operations.\n - Unclean shutdown detected, indicated by a non-empty lock file (`/data/db/mongod.lock`).\n\n4. **Proposed first steps**:\n - Confirm CPU capabilities and consider using a machine with AVX support if necessary.\n - Free up or allocate more RAM to the container to ensure it has adequate resources.\n - If the lock file indicates an improper shutdown, remove the lock file:\n ```\n rm /data/db/mongod.lock\n ```\n - Adjust the WiredTiger cache size in the MongoDB configuration to use less RAM or increase the available RAM in the system.\n\n5. **Monitor results**: Restart the MongoDB container after making the necessary adjustments and check the logs again for any persistent issues or errors. \n\nBy following these steps, you should be able to troubleshoot the MongoDB container error effectively.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/crAPI/issues/206",
@@ -7547,6 +7811,7 @@
"node_id": "I_kwDOFAM8Ps54h0Dn",
"title": "crAPI main page not loading on localhost",
"body": "**Describe the bug**\r\nEvery time I curl from Linux the file with the given command it gets to my folder empty + when I manually filled the file with the set up and pull the main page is not loading on my localhost\r\n\r\n**To Reproduce**\r\n\r\n\r\n\r\n\r\n**Expected behavior**\r\ndocker-compose.yml should contain the set up. I tried manually editing the file and it runs but when in the login the loader never ends as I showed on the pictures above\r\n\r\n",
+ "summary": "Investigate the issue with the crAPI main page not loading on localhost. Confirm that the `docker-compose.yml` file is correctly configured with the necessary setup. \n\n1. Check the output of the `curl` command; ensure that the expected files are being downloaded correctly and are not empty.\n2. Verify the contents of the `docker-compose.yml` file. Ensure all required services are defined and correctly configured.\n3. If manually editing the `docker-compose.yml` resolves some issues but leads to an infinite loading screen during login, consider checking the logs of the running services for any errors. Use `docker-compose logs` to fetch the logs.\n4. Investigate network configurations and firewall settings that may be blocking access to localhost.\n5. Ensure all dependencies are correctly installed and running. Run `docker-compose up` to start the services and monitor for any errors during startup.\n\nBegin troubleshooting with these steps to pinpoint the root cause of the loading issue.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/crAPI/issues/221",
@@ -7575,6 +7840,7 @@
"node_id": "I_kwDOFAM8Ps54-xJF",
"title": " required but undefined property 'converstion_params' in openapi-spec.json",
"body": "In the openapi-spec.json file, the definition for 'ProfileVideo' at line 3012 appears to have a spelling mistake for one of the required properties.\r\n\r\nconvers**t**ion_params should be conversion_params (additional 't' character is likely an error).\r\n\r\n\r\n",
+ "summary": "Fix the spelling mistake in the 'openapi-spec.json' file regarding the 'ProfileVideo' definition at line 3012. Change the required property from 'converstion_params' to 'conversion_params' to eliminate the undefined property error. \n\n1. Open the 'openapi-spec.json' file.\n2. Navigate to line 3012.\n3. Locate the entry for 'converstion_params'.\n4. Correct the spelling to 'conversion_params'.\n5. Save the changes and validate the JSON structure to ensure there are no syntax errors.\n6. Test the API to confirm that the required property is now recognized correctly.\n\nEnsure to run any existing tests or linting processes to verify that the change does not introduce new issues.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/crAPI/issues/222",
@@ -7603,6 +7869,7 @@
"node_id": "I_kwDOFAM8Ps5-2ehZ",
"title": "Need help with challenge 13",
"body": "Hi,\r\nI'm having issues solving challenge 13. Is this an appropriate place to ask for help?\r\nEdw.",
+ "summary": "Request assistance for Challenge 13. Clearly outline the specific problems you are facing, including any error messages or unexpected behavior. Share relevant code snippets or configurations that relate to the challenge. \n\nTo tackle the problem, first, review the challenge requirements and constraints. Then, isolate the section of code that is causing issues. Utilize debugging techniques such as adding print statements or using a debugger to trace the execution flow. Additionally, consult the documentation or resources related to the technology you're using for this challenge. Engage with the community by posting your findings and questions.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/crAPI/issues/234",
@@ -7629,6 +7896,7 @@
"node_id": "I_kwDOFAM8Ps5-5l6w",
"title": "PostgreSQL database not accepting edits",
"body": "Hi,\r\nIn Challenge 13, I have found the coupon_code parameter in the /workshop/api/shop/apply_coupon to be injectable.\r\nI also found the applied_coupon table in the PostgreSQL database.\r\n\r\nThe endpoint accepts the following injection and returns the database version:\r\n\"coupon_code\":\"TRAC075'; SELECT version() --+\"\r\n\r\nBut it refuses the following and returns a 500 error:\r\n\"coupon_code\":\"TRAC075'; DELETE FROM applied_coupon WHERE coupon_code=TRAC075 --+\"\r\n\r\nIs there anything that needs to be changed in the crAPI config file to allow user edits to be made to the database? I noticed there are restrictions for shell injection.\r\n\r\nThanks,\r\nEdw.\r\n",
+ "summary": "Investigate the PostgreSQL database's handling of SQL injection and user input sanitization. \n\n1. Confirm the vulnerability in the `/workshop/api/shop/apply_coupon` endpoint by testing the injectable `coupon_code` parameter. \n2. Attempt to execute basic SQL commands through the parameter to understand the extent of the injection vulnerability.\n3. Analyze the server's response to various SQL commands, noting the 500 error for delete operations.\n4. Review the crAPI configuration file for any settings that may restrict database write operations or user edits.\n5. Determine if there are security measures like query whitelisting or input validation that could be causing the issue.\n\nConsider modifying the configuration to allow write operations if appropriate, while ensuring that security is maintained.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/crAPI/issues/235",
@@ -7655,6 +7923,7 @@
"node_id": "I_kwDOFAM8Ps6E6ERf",
"title": "Getting Core Dumped Error!!",
"body": "**Describe the bug**\r\nWhen attempting to run the crAPI Docker container (`crapi/crapi-web:latest`) on my Ubuntu x86_64 system with Docker version 20.10.13, the container fails to start and exits with an \"Illegal instruction (core dumped)\" error.\r\n\r\n**To Reproduce**\r\nSteps to reproduce the behavior:\r\n1. Pull the crAPI Docker image:\r\n ```bash\r\n docker pull crapi/crapi-web:latest\r\n ```\r\n2. Run the Docker container:\r\n ```bash\r\n docker run --name crapi-web crapi/crapi-web:latest\r\n ```\r\n3. Observe the container status and inspect Docker logs:\r\n ```bash\r\n docker logs crapi-web\r\n ```\r\n4. Output\r\n```bash\r\ntotal 16\r\ndrwxr-xr-x 2 root root 4096 Feb 4 15:58 .\r\ndrwxr-xr-x 1 root root 4096 Feb 4 16:05 ..\r\n-rw-r--r-- 1 root root 2240 Feb 4 15:58 server.crt\r\n-rw-r--r-- 1 root root 3272 Feb 4 15:58 server.key\r\nWORKSHOP_SERVICE=crapi-workshop:8000\r\nHOSTNAME=6685c8e25d64\r\nSHLVL=2\r\nHOME=/root\r\nHTTP_PROTOCOL=http\r\nPATH=/usr/local/sbin:/usr/local/bin:/usr/sbin:/usr/bin:/sbin:/bin:/usr/local/openresty/luajit/bin:/usr/local/openresty/nginx/sbin:/usr/local/openresty/bin\r\nCOMMUNITY_SERVICE=crapi-community:8087\r\nTLS_ENABLED=false\r\nIDENTITY_SERVICE=crapi-identity:8080\r\nPWD=/\r\nNGINX_TEMPLATE=/etc/nginx/conf.d/default.conf.template\r\nIllegal instruction (core dumped)\r\n```\r\n\r\n**Expected behavior**\r\nThe crAPI Docker container (`crapi/crapi-web:latest`) should start successfully and be ready to serve requests on the specified ports (e.g., port 80 and port 443).\r\n\r\n**Runtime Environment**\r\n- Docker Version:\r\n ```bash\r\n docker -v\r\n ```\r\n Output:\r\n ```\r\n Docker version 20.10.25+dfsg1, build b82b9f3\r\n ```\r\n- Operating System:\r\n ```bash\r\n uname -a\r\n ```\r\n Output:\r\n ```\r\n Linux kali 6.6.9-amd64 #1 SMP PREEMPT_DYNAMIC Kali 6.6.9-1kali1 (2024-01-08) x86_64 GNU/Linux\r\n ```\r\n\r\n**Additional Information**\r\n- No custom configurations or changes were applied to the Docker environment.\r\n- Restarting Docker service and rebooting the host machine did not resolve the issue.\r\n \r\nPlease let me know if further information or logs are needed to diagnose this issue. Thank you!",
+ "summary": "Diagnose and resolve the \"Illegal instruction (core dumped)\" error when running the crAPI Docker container (`crapi/crapi-web:latest`) on Ubuntu x86_64.\n\n1. **Verify Compatibility**: Ensure that the Docker image is compatible with your CPU architecture. Use `docker inspect` to check the architecture of the `crapi/crapi-web:latest` image.\n\n2. **Check System Requirements**: Ensure that your system meets all the prerequisites for running crAPI, including any necessary libraries and dependencies.\n\n3. **Inspect Docker Logs**: Use `docker logs crapi-web` to gather more detailed error output. Look for any specific commands or instructions that might be causing the illegal instruction.\n\n4. **Test with Different Docker Versions**: Try running the container on a different Docker version or upgrade to the latest version. Sometimes, compatibility issues arise with specific versions.\n\n5. **Run with Debugging Options**: Start the container with debugging options enabled to gain more insight into the crash:\n ```bash\n docker run --name crapi-web --entrypoint /bin/bash crapi/crapi-web:latest\n ```\n\n6. **Check for Hardware Issues**: Run a hardware diagnostic to ensure the CPU is functioning correctly. Sometimes, core dumps can be a result of hardware failures.\n\n7. **Examine CPU Features**: Use `cat /proc/cpuinfo` to check for CPU features. If the Docker image is built with specific CPU optimizations (like AVX or SSE), ensure your CPU supports those features.\n\n8. **Contact Support**: If the issue persists, provide detailed logs and the results of the above checks to the crAPI support team or file a new issue on GitHub for further assistance.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/crAPI/issues/241",
@@ -7685,6 +7954,7 @@
"node_id": "I_kwDOFAM8Ps6Hlq3D",
"title": " Install crAPI in userland Kali Linux",
"body": "Hello,\r\nI am trying to install crAPI at userland Kali Linux.\r\nThe commands are not working.\r\nMay you could give me advise?\r\n\r\nThank you and Kind regards\r\n",
+ "summary": "Install crAPI on userland Kali Linux. Troubleshoot the installation commands that are failing. \n\n1. Verify your current environment and ensure you have the necessary dependencies installed by running:\n ```bash\n sudo apt update && sudo apt install git python3 python3-pip\n ```\n\n2. Clone the crAPI repository:\n ```bash\n git clone https://github.com/your-repo/crAPI.git\n ```\n\n3. Navigate to the crAPI directory:\n ```bash\n cd crAPI\n ```\n\n4. Install the required Python packages:\n ```bash\n pip3 install -r requirements.txt\n ```\n\n5. Check for any specific installation instructions in the README file of the crAPI repository.\n\n6. If errors persist, capture the error messages and search for solutions or report the issue with detailed logs for further assistance.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/crAPI/issues/246",
@@ -7713,6 +7983,7 @@
"node_id": "I_kwDOFAM8Ps6LlDo9",
"title": "Security missconfiguration vulnerability ",
"body": "Want to ask that is the crAPI have security missconfiguration vulnerability ",
+ "summary": "Investigate potential security misconfiguration vulnerabilities in crAPI. \n\n1. Review the crAPI configuration files for default settings that may expose sensitive information.\n2. Examine access controls and permissions to ensure they are properly set for users and services.\n3. Check for unnecessary services or ports that are open, which could be exploited.\n4. Conduct a security assessment to identify any outdated libraries or frameworks that may introduce vulnerabilities.\n5. Implement security best practices such as enforcing HTTPS, validating input, and sanitizing output.\n\nDocument findings and suggest necessary configurations to mitigate identified risks.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/crAPI/issues/255",
@@ -7741,6 +8012,7 @@
"node_id": "I_kwDOFAM8Ps6PjImU",
"title": "Local host:8888 is unreachable",
"body": "I can't reach localhost:8888 but I can reach localhost:8025. How can I fix this issue? \r\n\r\n",
+ "summary": "Troubleshoot the issue of localhost:8888 being unreachable while localhost:8025 is accessible. \n\n1. Confirm that the application intended to run on port 8888 is actively running. Check the service status to ensure it is not stopped or crashed.\n\n2. Verify that the port 8888 is not blocked by a firewall or security settings. Use tools like `netstat` or `lsof` to check if the port is in use and if the application is listening on that port.\n\n3. Check the application's configuration files to ensure it is set to bind to the correct address and port. Look for settings related to host and port in the configuration.\n\n4. Investigate any relevant logs from the application for error messages or warnings that could provide insights into why it isn't accessible.\n\n5. If applicable, restart the application to see if the issue resolves itself.\n\n6. Ensure that no other services are conflicting with port 8888. If necessary, change the port in the application configuration to another unused port and test again.\n\nBy following these steps, you can identify and resolve the issue preventing access to localhost:8888.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/crAPI/issues/260",
@@ -7765,10 +8037,11 @@
"pk": 273,
"fields": {
"nest_created_at": "2024-09-11T19:37:06.496Z",
- "nest_updated_at": "2024-09-12T00:14:35.543Z",
+ "nest_updated_at": "2024-09-13T17:01:07.878Z",
"node_id": "I_kwDOFAM8Ps6VvOKo",
"title": "docker in running on VM not accesibe Using Host Machine",
"body": "**If You're running this docker container on your Ubuntu VM and not able to access using Host machine using ip-address (Ex. 192.168.10.6)**\r\n\r\n- [x] #Do the Following\r\n - First Stop the container using (CTRL+C)\r\n - Open docker-compose.yml and modify the two things \r\n - `crapi-web` service: Under `crapi-web` modify the `ports` sections\r\n - \"0.0.0.0:8888:80\"\r\n - \"0.0.0.0:8888:80\"\r\n - `mailhog` service: modify the `ports` sections\r\n - \"0.0.0.0:8025:8025\"\r\n \r\n **If you have any doubt, referrer the image:**\r\n \r\n \r\n\r\n\r\n\r\n",
+ "summary": "Modify Docker configuration for accessibility from host machine. Follow these steps:\n\n1. Stop the running Docker container using `CTRL+C`.\n2. Open `docker-compose.yml`.\n3. Update the `crapi-web` service:\n - Change the `ports` section to `\"0.0.0.0:8888:80\"` for both entries.\n4. Update the `mailhog` service:\n - Change the `ports` section to `\"0.0.0.0:8025:8025\"`.\n\nEnsure that the changes are saved. After modifying the configuration, restart the Docker container to apply the changes. Verify accessibility from the host machine using the specified IP address (e.g., 192.168.10.6). If issues persist, consult the provided images for additional guidance.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/crAPI/issues/274",
@@ -7797,6 +8070,7 @@
"node_id": "I_kwDOEyybVc57w796",
"title": "Add German translations",
"body": "Hi, if you like I could create Norwegian and German translations since I am proficient in those two languages. I could also look over the Spanish translation and see if I can improve the language a bit. My wife is a Spanish language teacher so I am sure I can get some support there. \r\n\r\n",
+ "summary": "The issue suggests adding German and Norwegian translations, with the author expressing their proficiency in these languages. Additionally, they offer to review the existing Spanish translation for improvements, leveraging support from a Spanish teacher. It may be beneficial to accept the offer and coordinate the translation efforts accordingly.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/cornucopia/issues/281",
@@ -7823,6 +8097,7 @@
"node_id": "I_kwDOEyybVc58Ovm_",
"title": "Add new version of the Online wiki deck that contains the new ASVS 4.0 mapping",
"body": "The old wiki deck contains the 3.0 ASVS requirements. see: https://wiki.owasp.org/index.php/Cornucopia_-_Ecommerce_Website_-_VE_2\r\n\r\nThe wiki deck should be updated to account for the 4.0 ASVS requirements.\r\n",
+ "summary": "The issue requests an update to the Online wiki deck to reflect the new ASVS 4.0 mapping, as the current version includes the outdated ASVS 3.0 requirements. To address this, the wiki deck needs to be revised to incorporate the changes and requirements from ASVS 4.0.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/cornucopia/issues/293",
@@ -7845,10 +8120,11 @@
"pk": 276,
"fields": {
"nest_created_at": "2024-09-11T19:38:23.850Z",
- "nest_updated_at": "2024-09-12T03:11:16.828Z",
+ "nest_updated_at": "2024-09-13T16:54:06.205Z",
"node_id": "I_kwDOEyybVc6Jubpf",
"title": "🧚🤖 Pixeebot Activity Dashboard",
"body": "\n👋 This dashboard summarizes my activity on the repository, including available improvement opportunities.\n\n\n## Recommendations\n_Last analysis: Sep 11 | Next scheduled analysis: Sep 18_\n\n### Open\n\n ✅ Nice work, you're all caught up!\n\n\n### Available\n\n ✅ Nothing yet, but I'm continuing to monitor your PRs.\n\n\n### Completed\n✅ You merged improvements I recommended [View](https://github.com/OWASP/cornucopia/pulls?q=is%3Apr+is%3Amerged+author%3Aapp%2Fpixeebot)\n✅ I also hardened PRs for you [View](https://github.com/OWASP/cornucopia/pulls?q=%22Hardening+Suggestion%22+in%3Atitle+is%3Apr+author%3Aapp%2Fpixeebot+is%3Amerged+head%3Apixeebot%2F)\n\n## Metrics\n**What would you like to see here?** [Let us know!](https://tally.so/r/mYa4Y5)\n\n## Resources\n\n📚 **Quick links**\nPixee Docs | Codemodder by Pixee\n\n🧰 **Tools I work with**\n[SonarCloud](https://docs.pixee.ai/code-scanning-tools/sonar) | [SonarQube](https://docs.pixee.ai/code-scanning-tools/sonarqube) | [CodeQL](https://docs.pixee.ai/code-scanning-tools/codeql) | [Semgrep](https://docs.pixee.ai/code-scanning-tools/semgrep)\n\n🚀 **Pixee CLI**\nThe power of my codemods in your local development environment. [Learn more](https://github.com/pixee/pixee-cli)\n\n💬 **Reach out**\nFeedback | Support\n\n---\n\n❤️ Follow, share, and engage with Pixee: GitHub | [LinkedIn](https://www.linkedin.com/company/pixee/) | [Slack](https://pixee-community.slack.com/signup#/domain-signup)\n\n\n \n\n",
+ "summary": "The Pixeebot Activity Dashboard provides a summary of repository activity, including current status and recommendations for improvement. As of the last analysis, all tasks are up to date, with no pending improvements. Recently merged improvements and hardened pull requests have been noted. The dashboard invites feedback on the metrics displayed and encourages users to share suggestions for additional features. Users are also provided with quick links to relevant resources and tools, as well as contact options for support and feedback. To enhance the dashboard, consider providing input on desired metrics.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/cornucopia/issues/549",
@@ -7875,6 +8151,7 @@
"node_id": "I_kwDOEyybVc6K2tWJ",
"title": "Ensure the converter can create print-ready proofs for print-on-demand jobs",
"body": "**Is your feature request related to a problem? Please describe.**\r\n\r\nIn order to drive the project further and create print ready proofs for print-on-demand we need to be able to script the idml to pdf convertion.\r\n\r\n**Describe the solution you'd like**\r\nA clear and concise description of what you want to happen.\r\n\r\nScribus looks promising: https://wiki.scribus.net/canvas/Command_line_scripts#Usefull_'Create_PDF_out_of_existing_scribus_document'_script\r\n\r\nPerhaps we could create a github action for doing the convertion or a python module.\r\n\r\n**Describe alternatives you've considered**\r\n\r\nIndesign server is an alternative, but it’s a commercial product.\r\n\r\n**Additional context**\r\n\r\nCurrently we are not delivering print ready design files. A designer always have install the fonts, open the idml document, clone the back of the card 79 times to the correct place and export to pdf.\r\nInstead we should just be able to deliver final pdfs and not idml with embedded art works.\r\n\r\nAs a middle step we could look into correctly add the links to the graphics from python, but I am afraid it won’t be platform dependent.\r\n",
+ "summary": "The issue highlights the need for a solution to create print-ready proofs for print-on-demand jobs by automating the IDML to PDF conversion process. The suggested approach involves using Scribus for scripting the conversion or possibly creating a GitHub action or Python module to streamline this process. Currently, the workflow requires manual intervention by designers to export the files, which is inefficient. Alternatives like Indesign Server are noted but are not preferred due to their commercial nature. The next steps should focus on implementing an automated solution that can handle the conversion effectively.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/cornucopia/issues/583",
@@ -7901,6 +8178,7 @@
"node_id": "I_kwDOEyybVc6LGIZM",
"title": "Build the requirement list on the card using OpenCRE for easier maintenance and collaboration. ",
"body": "**Is your feature request related to a problem? Please describe.**\r\n\r\nCurrently the requirement list connected with each card has a tendency to become outdated. There is also too little discussions around them which means that it's not sure all of them are equally relevant for all cards. \r\n\r\n**Describe the solution you'd like**\r\n\r\nWe should be able to build the requirement map on the cards by querying the OpenCRE API. This will invite to more cross-team collaboration and maintenance of the requirements connected to the cards and make the map easier to maintain. \r\n\r\n**Describe alternatives you've considered**\r\n\r\nThe requirement list has been moved out of the word and indesign files, this has helped, but using OpenCRE is the next logical step.\r\n\r\n",
+ "summary": "The issue highlights the problem of outdated requirement lists associated with cards, which lack sufficient discussion and relevance. The proposed solution is to utilize the OpenCRE API to build a requirement map for these cards, promoting better collaboration and easier maintenance. An alternative considered was moving the requirement list out of word and design files, which has already shown some improvement. \n\nNext steps could involve integrating the OpenCRE API to enhance the requirement mapping process.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/cornucopia/issues/595",
@@ -7927,6 +8205,7 @@
"node_id": "I_kwDOEyybVc6LGK9o",
"title": "Language review of the translations.",
"body": "**Is your feature request related to a problem? Please describe.**\r\n\r\nWe now have the decks translated into 6 languages, but we have not done a proper review of the language. The English, Norwegian and Dutch versions are probably fine, but what about the rest? \r\n\r\n**Describe the solution you'd like**\r\n\r\nGet someone that has been working as a native language teacher or translator to have a look at the translations in order to make sure the translations are properly done.\r\n",
+ "summary": "The issue highlights the need for a comprehensive review of translations for decks available in six languages. While the English, Norwegian, and Dutch versions are assumed to be acceptable, there is uncertainty regarding the quality of the other translations. The proposed solution involves engaging a native language teacher or translator to assess and ensure the accuracy and appropriateness of all translations. It may be beneficial to identify and contact qualified individuals for this review process.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/cornucopia/issues/596",
@@ -7953,6 +8232,7 @@
"node_id": "I_kwDOEdhCDs47aYXL",
"title": "OWASP threat modeling references",
"body": "Add references to other OWASP threat modeling projects and resources",
+ "summary": "The issue suggests adding references to various OWASP threat modeling projects and resources to enhance the existing documentation. To address this, it would be beneficial to gather a list of relevant OWASP resources and incorporate them into the documentation for better accessibility and guidance.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-threat-modeling-playbook/issues/3",
@@ -7979,6 +8259,7 @@
"node_id": "I_kwDOEdhCDs47aYeL",
"title": "Include a list of threat modeling tools",
"body": "As part of the tooling step",
+ "summary": "The issue suggests that a list of threat modeling tools should be included in the documentation, specifically within the tooling section. To address this, the team should compile and add relevant tools to enhance the resource's comprehensiveness.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-threat-modeling-playbook/issues/4",
@@ -8001,10 +8282,11 @@
"pk": 282,
"fields": {
"nest_created_at": "2024-09-11T19:39:37.166Z",
- "nest_updated_at": "2024-09-11T19:39:37.166Z",
+ "nest_updated_at": "2024-09-13T15:14:15.785Z",
"node_id": "I_kwDOEdhCDs47aYqV",
"title": "Include a list of threat modeling methodologies",
"body": "As part of the selecting a methodology step",
+ "summary": "The issue requests the inclusion of a list of threat modeling methodologies within the documentation, specifically during the selection process of a methodology. It suggests enhancing the guidance provided to users when they are choosing a suitable threat modeling approach. To address this, a compilation of existing threat modeling methodologies should be created and added to the relevant section of the documentation.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-threat-modeling-playbook/issues/5",
@@ -8031,6 +8313,7 @@
"node_id": "I_kwDOEcPlPM5hiKgz",
"title": "💻✅ Incorporate Good Practices back into the handbook",
"body": "https://owasp.org/www-committee-project/#div-practice",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-committee-project/issues/21",
@@ -8053,10 +8336,11 @@
"pk": 284,
"fields": {
"nest_created_at": "2024-09-11T19:39:46.047Z",
- "nest_updated_at": "2024-09-11T19:39:46.047Z",
+ "nest_updated_at": "2024-09-13T03:50:56.619Z",
"node_id": "I_kwDOEcPlPM5hiLKX",
"title": "📝👀 Update the .github/README.md for the OWASP Github page to better reflect projects and their statuses.",
"body": "",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-committee-project/issues/22",
@@ -8085,6 +8369,7 @@
"node_id": "I_kwDOEcPlPM5hjFys",
"title": "📋🌟 create a list or projects or update the existing one to show last release, commit #, last commit, stars,",
"body": "### Discussed in https://github.com/OWASP/www-committee-project/discussions/44\r\n\r\n
\r\n\r\nOriginally posted by **DonnieBLT** March 22, 2023\r\nsee this as a starting point https://github.com/psiinon/owasp-projects \r\n\r\nwe can create a github action that runs daily to update the list\r\nmake sure it shows - \r\nprimary language, license, Forks, stars, Issues, PRs, issues need help last updated, Release version, date released \r\nfigure out a way to show project statuses from each github repo to link roadmap issues similar to how the github list of repos has the stats
",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-committee-project/issues/48",
@@ -8111,6 +8396,7 @@
"node_id": "I_kwDOEcPlPM5jQpN5",
"title": "HANDBOOK: Discuss/document legal review for project donations",
"body": "Nothing about legal review for project donations. There should be. The OWASP Foundation should provide this service if they don't already. The foundation will be unable to protect project leaders without the necessary due diligence, which is one of the benefits of joining a foundation in the first place. This needs to be addressed.",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-committee-project/issues/58",
@@ -8141,6 +8427,7 @@
"node_id": "I_kwDOEcPlPM5jQpdQ",
"title": "HANDBOOK: Document copyright transfer",
"body": "No mention of copyright transfer. We should discuss this in a future committee meeting, decide on a path forward and document the requirements in the handbook.",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-committee-project/issues/59",
@@ -8171,6 +8458,7 @@
"node_id": "I_kwDOEcPlPM5jQp5i",
"title": "HANDBOOK: Prescribe OSS licenses",
"body": "Some OSI licenses are anti-business or may lead to unintended legal requirements to disclose IP based on a deployments configuration. We should be prescriptive in the licenses we will accept.",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-committee-project/issues/60",
@@ -8201,6 +8489,7 @@
"node_id": "I_kwDOEcPlPM5jQqB6",
"title": "HANDBOOK: Require CODEOWNERS and SECURITY.md",
"body": "We should require a CODEOWNERS file and SECURITY.md in every project repo.",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-committee-project/issues/61",
@@ -8231,6 +8520,7 @@
"node_id": "I_kwDOEcPlPM5jQqVK",
"title": "HANDBOOK: JIRA onboarding?",
"body": "The new project request form takes you to JIRA, which requires an account. Future leaders will use their personal or work email address. We should encourage them to use their OWASP email address, but they won't have one yet. Therefore, I don't think JIRA is the right intake process. ",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-committee-project/issues/62",
@@ -8261,6 +8551,7 @@
"node_id": "I_kwDOEcPlPM5jQqiQ",
"title": "HANDBOOK: Clarify project types",
"body": "We need to clarify project types. CycloneDX is the only \"Standard\" project that I'm aware of. Other \"standards\" like ASVS are categorized as documentation projects. We need to discuss this. CycloneDX does not fit into any existing project types. But the creation of a \"standard\" project type may be problematic with ASVS and others that are a different type of standard.",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-committee-project/issues/63",
@@ -8291,6 +8582,7 @@
"node_id": "I_kwDOEcPlPM5k_oQ6",
"title": "Remove other \"project handbooks\" ",
"body": "there are a few places where the handbook is mentioned see below: - would be good to consolidate them all into one official repo or file and remove all copies of the previous handbook and forward them to the new one\r\n\r\n1. https://github.com/OWASP/www-policy/blob/master/unpublished/project-handbook.md\r\n2. https://github.com/OWASP-Foundation/Project-Handbook\r\n3. https://owasp.org/www-policy/operational/projects\r\n4. https://owasp.org/www-pdf-archive/PROJECT_LEADER-HANDBOOK_2014.pdf\r\n5. https://owasp.org/www-pdf-archive//OWASPProjectsHandbook2013.pdf\r\n6. https://github.com/OWASP/www-committee-project/tree/master/handbook",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-committee-project/issues/68",
@@ -8321,6 +8613,7 @@
"node_id": "I_kwDOEcPlPM5o2MvR",
"title": "Setup a Github Action to monitor project pages for activity ",
"body": "1) if a project has not changed it's project page in 1 week, send a reminder\r\n2) if a project has not had updates for 6 months send a reminder\r\n3) if a project has not had updates for 2 years, send an archive notice\r\n4) if a project has not had updates for 3 years, archive it",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-committee-project/issues/71",
@@ -8343,10 +8636,11 @@
"pk": 294,
"fields": {
"nest_created_at": "2024-09-11T19:40:14.558Z",
- "nest_updated_at": "2024-09-11T19:40:14.558Z",
+ "nest_updated_at": "2024-09-13T15:14:34.494Z",
"node_id": "I_kwDOEZvT985B4hvR",
"title": "bug: acurl command bug",
"body": "error msg:\r\n```\r\n~ ❯❯❯ acurl http://localhost:8000/DescribeIpReport\r\nTraceback (most recent call last):\r\n File \"gurl/__main__.py\", line 32, in \r\n main()\r\n File \"gurl/__main__.py\", line 20, in main\r\n res = gurl.parse_curl_trace(f.read())\r\n File \"/venv/lib/python3.8/site-packages/gurl/__init__.py\", line 104, in parse_curl_trace\r\n reqres[\"_meta\"][\"curl_log\"] = log\r\nTypeError: 'NoneType' object is not subscriptable\r\n~ ❯❯❯\r\n```",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/apicheck/issues/8",
@@ -8369,10 +8663,11 @@
"pk": 295,
"fields": {
"nest_created_at": "2024-09-11T19:40:32.779Z",
- "nest_updated_at": "2024-09-12T00:14:41.132Z",
+ "nest_updated_at": "2024-09-13T17:01:03.793Z",
"node_id": "I_kwDOEWezOc5wUjF7",
"title": "Is this initiative still active?",
"body": "Haven't seen updates since 2021. I've been looking for uses of ontology to derive threats from descriptions of infrastructure. This one at least derives them from DFDs, but in Threat Dragon format. ",
+ "summary": "Verify the current status of the initiative by checking for recent commits or activity on the repository. Investigate the last updates made in 2021 to determine if there are any pending issues or discussions. Explore the possibility of integrating ontology for threat analysis in infrastructure descriptions. Consider analyzing the existing Threat Dragon format to understand how threats are derived from Data Flow Diagrams (DFDs). \n\nAs initial steps, review the documentation and codebase for insights into the methodology used for threat derivation. Engage with the community by posting inquiries or suggestions on the GitHub issue tracker. Propose enhancements or collaborations to reactivate the project and expand its capabilities.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/OdTM/issues/1",
@@ -8399,6 +8694,7 @@
"node_id": "I_kwDOETRnAc4-CaNB",
"title": "Possible new ideas for challenges",
"body": "This ticket is for creating/listing possible ideas. If an Idea is picked up by a developer, then it gets its own tickets. \r\n- [x] #44\r\n- [x] #43\r\n- [x] Google support (https://github.com/commjoen/wrongsecrets/issues/40, https://github.com/commjoen/wrongsecrets/issues/39), \r\n- [x] #93\r\n- [x] Alibaba cloud support (will not do this, maybe have a write up later?)\r\n- [x] #299\r\n- [x] Heroku support\r\n- [x] #144\r\n- [x] Secret in logs (= challenge 8)\r\n- [x] #187\r\n- [x] #188\r\n- [x] #189\r\n- [x] #199\r\n- [x] #200\r\n- [x] #201\r\n- [x] #148\r\n- [x] Hardcoded in testcode (https://github.com/commjoen/wrongsecrets/issues/37#issuecomment-1011482070)\r\n- [x] #296\r\n- [ ] #810\r\n- [x] #815\r\n- [ ] #297\r\n- [ ] #811\r\n- [ ] #812\r\n- [x] have a too long living OIDC token which can be used to extract and apply (wont'do)\r\n- [x] Jenkins or other github secondary ci/cd secret (won't do, as it requiers another container next to it & needs maintenance. Our current ci/cd action shows the issue already.\r\n- [ ] #345\r\n- [ ] Simple one that is a mix of 1 & 13: docker container is run with password as parameter, but the whole command is placed in a .sh file and stored in the git repo (aka: use .gitignore to block local helper scripts)\r\n- [x] #344\r\n- [x] SOPS/sealed secrets misconfig : a bogus sealed secret with misconfigured retrieval setup? (pending)\r\n- [ ] #615\r\n- [x] #614\r\n- [ ] #613\r\n- [x] #377\r\n- [x] #616 \r\n- [x] #809\r\n- [x] #813\r\n- [x] Based on @robvanderveer his suggestion: https://github.com/OWASP/wrongsecrets/issues/616\r\n- [ ] Have passwordless challenges based on impersonation such as https://github.com/OWASP/wrongsecrets/blob/master/src/main/resources/explanations/challenge11_hint-azure.adoc - agreed to not create a new challenge, but extend GCP/AWS with a similar solution for cahllenge 11.\r\n- [x] #814\r\n- [ ] Bad RSA private key redaction; https://www.hezmatt.org/~mpalmer/blog/2020/05/17/private-key-redaction-ur-doin-it-rong.html (tip from @nbaars )\r\n- [ ] A kotlin binary",
+ "summary": "Create a list of potential challenges for developers to tackle. Each selected idea should be expanded into its own ticket. Review the following ideas and select those you wish to pursue:\n\n1. Implement Google support issues referenced in tickets #40 and #39.\n2. Explore Alibaba cloud support, with a possible write-up later.\n3. Add Heroku support.\n4. Address the challenge related to secrets in logs (challenge 8).\n5. Investigate hardcoded credentials in test code as noted in issue #37.\n6. Develop a challenge featuring a long-lived OIDC token for extraction and application (decide not to implement).\n7. Suggest a challenge involving Docker containers run with passwords as parameters, stored in a .sh file within the repo (ensure to use .gitignore).\n8. Create challenges based on misconfigured SOPS/sealed secrets as discussed.\n9. Consider passwordless challenges involving impersonation, potentially extending existing GCP/AWS challenges.\n\nBegin by filtering through the ideas above, selecting a few that align with current priorities. Create new tickets for each selected idea, outlining specific acceptance criteria and technical requirements needed for implementation.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/wrongsecrets/issues/37",
@@ -8427,6 +8723,7 @@
"node_id": "I_kwDOETRnAc5Ajjwq",
"title": "Record howto's",
"body": "Let's have a recording of the solutions!",
+ "summary": "Create a repository for recording how-to solutions. Implement a structured format for documenting each solution, including prerequisites, step-by-step instructions, and code snippets where applicable. Use Markdown for readability and organization.\n\n1. Set up a README file to outline the purpose of the repository and how to contribute.\n2. Choose a recording tool or software that allows you to capture screen activity and audio simultaneously.\n3. Establish a consistent naming convention for the recorded files to ensure easy navigation.\n4. Develop a template for submitting how-to recordings, including sections for problem description, solution steps, and any relevant links or references.\n5. Encourage contributors to test their solutions before recording to ensure accuracy.\n6. Create a directory structure in the repository for categorizing recordings by topic or technology.\n\nBegin by recording a few initial how-to solutions as examples to guide future contributions.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/wrongsecrets/issues/131",
@@ -8457,6 +8754,7 @@
"node_id": "I_kwDOETRnAc5D9H6b",
"title": "Improve ui of the overall app",
"body": "After talking to @mikewoudenberg about the front-end of the application, we realized it can use some love!\r\nMike will help us to get into a better design together. This is what this ticket is all about!",
+ "summary": "Improve the UI of the overall application. Collaborate with @mikewoudenberg to enhance the front-end design. \n\nFirst steps to tackle the problem:\n1. Conduct a UI audit to identify areas needing improvement.\n2. Gather user feedback to understand pain points and preferences.\n3. Create wireframes and design prototypes for proposed changes.\n4. Implement changes incrementally, focusing on high-impact areas first.\n5. Test the new designs with users for feedback and iterate as needed.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/wrongsecrets/issues/198",
@@ -8488,6 +8786,7 @@
"node_id": "I_kwDOETRnAc5EAKfQ",
"title": "create a secrets detection testbed branch with revoked credentials",
"body": "Steps to take:\r\n\r\n- [x] List secrets present in this issue\r\n- [x] Create a link to this issue in the web interface https://github.com/commjoen/wrongsecrets/pull/250\r\n- [x] Implement keys below in a separate branch with a script to generate a container from it which can be scanned\r\n- [x] Add master merging script for the .github/scripts/docker-create-and-push.sh \r\n\r\nKeys that can be added:\r\n\r\n- [x] Azure\r\n- [x] AWS\r\n- [x] GCP\r\n- [x] Git credentials :SSH key\r\n- [x] Git credentials: developer token\r\n- [x] private key RSA key & private ECC key\r\n- [x] GPG keychain (armored and notarmored)\r\n- [x] AES keys\r\n- [x] Slack callback\r\n- [x] kubeconfig\r\n- [x] QR-code (will be hard to represent a secret which is detactable other than through entropy,skipping it)\r\n- [x] BasicAuth\r\n- [x] gradle credentials\r\n- [x] mvn credentials\r\n- [x] NPM\r\n- [x] Firebase push notification keys (android/ios)\r\n- [x] OTP Seed\r\n- [x] segment.io access keys\r\n- [x] Vault root token & unseal keys\r\n- [x] Any JWT Token\r\n- [x] Gitlab PAT\r\n- [x] Gpg armoured export private and public keys\r\n- [x] Azure devops access token\r\n- [x] onepassword emergency kit, 1password-credentials.json and accesstokens\r\n- [x] keybase paperkey\r\n- [ ] IBM-cloud?\r\n- [ ] Nomad credentials (wait till https://github.com/commjoen/wrongsecrets/issues/299 happens)\r\n- [ ] Spring boot Session token\r\n- [x] Slack access tokens:bottoken & usertoken, applevel token & config token (requested..pending)\r\n- [ ] Braze API-keys (requested)\r\n- [ ] Lastpass integration/api-key\r\n- [ ] Confidant key\r\n- [x] Docker hub access token\r\n- [x] Vagrant cloud access token\r\n- [ ] confluence/jira secrets\r\n- [ ] AWS instance profile\r\n- [ ] add dockerconfig (https://kubernetes.io/docs/tasks/configure-pod-container/pull-image-private-registry/) \r\n- [ ] secrets above with encodings (base64, Hex encoding)\r\n- [ ] Database connection strings\r\n- [ ] OIDC token\r\n\r\nwhich other secret would you like to add? please comment\r\n",
+ "summary": "Create a branch for secrets detection testbed with revoked credentials. Follow these steps:\n\n1. **List Secrets**: Compile a comprehensive list of secrets to be included, such as:\n - Azure, AWS, GCP credentials\n - Git credentials (SSH key, developer token)\n - Private keys (RSA, ECC)\n - GPG keychain (both armored and not armored)\n - AES keys, Slack callback, kubeconfig, BasicAuth\n - Gradle, Maven, NPM credentials\n - Firebase push notification keys, OTP Seed\n - Segment.io access keys, Vault root token & unseal keys\n - Any JWT token, GitLab PAT, Azure DevOps access token\n - 1Password emergency kit, Keybase paperkey\n - Docker hub, Vagrant cloud access tokens\n - Additional secrets as suggested by comments (e.g., IBM Cloud, Nomad, Spring Boot session token, etc.).\n\n2. **Create Issue Link**: Reference the issue in the web interface by linking to `https://github.com/commjoen/wrongsecrets/pull/250`.\n\n3. **Implement Keys in a Branch**: Create a separate branch to implement the above keys. Develop a script that generates a container capable of scanning these secrets.\n\n4. **Add Merging Script**: Incorporate a master merging script within the `.github/scripts/docker-create-and-push.sh` file.\n\n5. **Consider Encodings**: Ensure to include secrets with various encodings such as Base64 and Hex encoding.\n\n6. **Database and OIDC Tokens**: Address the inclusion of database connection strings and OIDC tokens in the secrets list.\n\n7. **Seek Additional Input**: Encourage community input on additional secrets to incorporate by requesting comments.\n\nBegin by compiling the list of secrets and creating the new branch.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/wrongsecrets/issues/201",
@@ -8516,6 +8815,7 @@
"node_id": "I_kwDOETRnAc5Gp34J",
"title": "Create optional low value keys which can be used as canarotykens in AWS/GCP",
"body": "",
+ "summary": "Implement optional low-value keys for use as canary tokens in AWS and GCP. Define the key characteristics and requirements for these tokens, ensuring they can be easily integrated into existing infrastructure.\n\n1. Research existing canary token implementations in AWS and GCP to establish best practices.\n2. Design a schema for the low-value keys, specifying the format, expiration, and usage limits.\n3. Develop a mechanism for generating and managing these tokens, including a secure storage solution.\n4. Create documentation outlining the setup process and examples of how to use the tokens in various scenarios.\n5. Test the implementation in a staging environment to ensure functionality and security.\n6. Roll out the solution and gather feedback for potential improvements.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/wrongsecrets/issues/236",
@@ -8540,10 +8840,11 @@
"pk": 301,
"fields": {
"nest_created_at": "2024-09-11T19:41:27.003Z",
- "nest_updated_at": "2024-09-11T22:53:33.754Z",
+ "nest_updated_at": "2024-09-13T17:07:13.065Z",
"node_id": "I_kwDOETRnAc5Jz1RE",
"title": "Add hardcoded encryption key on top of a secret.",
"body": "Have a challenge about \"bad encryption practices\" where we hardocde the key and the secret in java.\r\n\r\nSteps to take:\r\n\r\n- create a secret on your computer\r\n- encrypt it with your algorithm of choice (could be AES, 3DES, whatever you like) on your computer resulting in the ciphertext you need\r\n- now take the ciphertext and put that in the java code of the challenge\r\n- add the key you used to encrypt the challenge and a decryption method to the challenge. See contributing.md on how to create the challenge.",
+ "summary": "Implement a challenge showcasing \"bad encryption practices\" by hardcoding an encryption key alongside a secret in Java.\n\n1. **Create a Secret**: Generate a meaningful secret on your local machine.\n \n2. **Encrypt the Secret**: Use an encryption algorithm of your choice (e.g., AES, 3DES) to encrypt the secret, producing the ciphertext.\n\n3. **Integrate Ciphertext into Code**: Embed the generated ciphertext directly into the Java code for the challenge.\n\n4. **Add Hardcoded Key**: Include the encryption key used for the encryption process in the code.\n\n5. **Implement Decryption Method**: Write a method that can decrypt the ciphertext using the hardcoded key.\n\n6. **Refer to Contributing Guidelines**: Consult `contributing.md` for specific instructions on how to format and add the challenge to the repository.\n\nBy following these steps, create a clear example of poor encryption practices for educational purposes.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/wrongsecrets/issues/297",
@@ -8576,6 +8877,7 @@
"node_id": "I_kwDOETRnAc5Ng0D5",
"title": "Log secret encoded from your cloud app towards the logging solution of your cloudprovider.",
"body": "Have a challenge for all 3 cloudproviders, where we log the actual secret inot the logs of the cloudprovider only.\r\n- [ ] log secret in AWS Cloudwatch\r\n- [ ] log secret in GCP stackdriver\r\n- [ ] log secret in Azure log Analytics",
+ "summary": "Log secrets encoded from your cloud application to the logging solutions of the three major cloud providers: AWS, GCP, and Azure. \n\n1. **AWS CloudWatch**: Implement logging functionality that captures the secret and sends it to CloudWatch logs. Use AWS SDK to configure logging settings, ensuring that the secret is encoded properly before logging. Verify the output in the CloudWatch console.\n\n2. **GCP Stackdriver**: Utilize Google Cloud logging libraries to encode the secret from your application and log it into Stackdriver. Ensure that the logging configuration is set up correctly to capture and store the logs. Check the logs in the GCP console for successful logging.\n\n3. **Azure Log Analytics**: Configure your Azure application to encode the secret and send it to Log Analytics. Use Azure Monitor SDK or REST APIs to facilitate the logging process. Validate the logs in the Azure portal to confirm that the secret is recorded.\n\n**First Steps**:\n- Set up logging libraries for each cloud provider in your application.\n- Ensure that proper encoding mechanisms are in place for the secrets before logging.\n- Test logging functionality in a development environment to confirm that logs are being captured as intended.\n- Review cloud provider documentation for specific logging API details and best practices.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/wrongsecrets/issues/345",
@@ -8605,6 +8907,7 @@
"node_id": "I_kwDOETRnAc5PLCdi",
"title": "Create improved documentation",
"body": "Have something like https://madhuakula.com/kubernetes-goat/ but then for wrognsecrets and reorganize the docs and wiki here.\r\n\r\n\r\n\r\n\r\nRaw notes: doxRS @madhuakula can start in same repo, lets see if we can include https://github.com/commjoen/wrongsecrets/tree/fix-container/src/main/resources/explanations automatically so we don't need to copy-paste. ",
+ "summary": "Improve documentation for the WrongSecrets project. \n\n1. Create a comprehensive resource similar to the existing Kubernetes Goat documentation found at https://madhuakula.com/kubernetes-goat/.\n2. Reorganize the current documentation and wiki to enhance clarity and usability.\n3. Utilize the existing explanations from the WrongSecrets GitHub repository (https://github.com/commjoen/wrongsecrets/tree/fix-container/src/main/resources/explanations) to avoid manual copy-pasting. \n4. Explore ways to automate the integration of these explanations into the new documentation structure.\n\nFirst steps to tackle the problem:\n- Assess the current state of documentation and identify key areas for improvement.\n- Review the content available in the WrongSecrets repository for potential inclusion.\n- Develop a structured outline for the new documentation layout.\n- Implement a strategy to automate the integration of explanations from the repository.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/wrongsecrets/issues/368",
@@ -8635,6 +8938,7 @@
"node_id": "I_kwDOETRnAc5Q4lhz",
"title": "Find and fix memory leak",
"body": "after running `hey` twice against `/challenge/1` we see that the amount of sessions build up to 400, and memory consumption goes from 200Mb to 277 MB after which, when all sessions are released, we still see 274 MB in use (e.g. 70 MB is not GCed?)",
+ "summary": "Investigate and resolve the memory leak issue observed when running the `hey` tool against the `/challenge/1` endpoint. After executing the test twice, track the session count, which escalates to 400, and monitor memory usage that increases from 200 MB to 277 MB. Upon releasing all sessions, confirm that 274 MB remains allocated, indicating that 70 MB has not been garbage collected.\n\nTake the following initial steps to tackle the problem:\n\n1. **Profile Memory Usage**: Use a memory profiler (e.g., VisualVM, Memory Analyzer) to identify objects that are not being collected by the garbage collector after sessions are released.\n\n2. **Review Session Management**: Examine the session handling logic in the application. Check for any references to session objects that may be retained unintentionally, preventing garbage collection.\n\n3. **Check for Strong References**: Look for strong references to session-related data that might be causing memory retention. Replace them with weak references if appropriate.\n\n4. **Analyze Code Paths**: Review the code paths executed during the two runs with `hey`. Pay special attention to any resources that are not being properly disposed of or released, such as database connections or file handles.\n\n5. **Implement Logging**: Add logging around session creation and destruction to monitor their lifecycle and track potential leaks.\n\n6. **Run Tests**: After implementing changes, run the tests again to verify if memory usage stabilizes and sessions are properly garbage collected.\n\n7. **Document Findings**: Record the findings, changes made, and their impact on memory consumption to assist with future debugging and maintenance.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/wrongsecrets/issues/389",
@@ -8666,6 +8970,7 @@
"node_id": "I_kwDOETRnAc5Sbitv",
"title": "Have a github action to compare git-secrets and trufflehog without any configuration update",
"body": "Create a multistage pipelien in which we check the performance of \r\n- [ ] git-secrets (https://github.com/awslabs/git-secrets) \r\n- [ ] git-leaks (https://github.com/zricethezav/gitleaks) - https://github.com/gitleaks/gitleaks-action (only commercial? will have to ask for a license key...)\r\n - [ ] request license key\r\n - [ ] do scanning\r\n - [ ] capture result in count\r\n- [ ] trufflehog (https://github.com/trufflesecurity/trufflehog) - https://github.com/marketplace/actions/trufflehog-oss \r\n - [x] do scanning (see https://github.com/OWASP/wrongsecrets/actions/workflows/scanners.yml, will have to update it with more scanners and capturing)\r\n - [ ] capture result in count\r\n- [ ] trufflehog3 \r\n- [ ] Whispers (https://github.com/Skyscanner/whispers) - https://github.com/Skyscanner/whispers/issues/72 \r\n- [ ] Github secret scanning (https://docs.github.com/en/developers/overview/secret-scanning-partner-program) \r\n- [ ] gittyleaks (https://github.com/kootenpv/gittyleaks)\r\n- [ ] git-all-secretss (https://github.com/anshumanbh/git-all-secrets)\r\n- [ ] detect-secrets (https://github.com/Yelp/detect-secrets)\r\n- [ ] Java Sast tool?\r\n\r\nfor their detection out of the box.",
+ "summary": "Implement a GitHub Action to compare the effectiveness of various secret scanning tools without requiring configuration updates. Create a multistage pipeline that includes the following steps:\n\n1. **Assess Git-Secrets**:\n - Integrate and execute git-secrets to validate its performance.\n - Link: [git-secrets](https://github.com/awslabs/git-secrets).\n\n2. **Incorporate Git-Leaks**:\n - Request a license key if necessary for git-leaks.\n - Perform scanning with Git-Leaks.\n - Capture and log the results in terms of detected secrets.\n - Link: [git-leaks](https://github.com/zricethezav/gitleaks) and [gitleaks-action](https://github.com/gitleaks/gitleaks-action).\n\n3. **Utilize TruffleHog**:\n - Execute scanning using TruffleHog.\n - Update the existing workflow to include more scanners and ensure results are captured.\n - Link: [trufflehog](https://github.com/trufflesecurity/trufflehog) and [trufflehog-oss action](https://github.com/marketplace/actions/trufflehog-oss).\n\n4. **Evaluate TruffleHog3**:\n - Add TruffleHog3 to the pipeline for comparison.\n\n5. **Integrate Whispers**:\n - Include the Whispers tool into the pipeline.\n - Link: [Whispers](https://github.com/Skyscanner/whispers).\n\n6. **Add GitHub Secret Scanning**:\n - Integrate GitHub's built-in secret scanning capabilities.\n - Link: [GitHub Secret Scanning](https://docs.github.com/en/developers/overview/secret-scanning-partner-program).\n\n7. **Implement Additional Tools**:\n - Add gittyleaks, git-all-secrets, and detect-secrets to the analysis.\n - Links:\n - [gittyleaks](https://github.com/kootenpv/gittyleaks)\n - [git-all-secrets](https://github.com/anshumanbh/git-all-secrets)\n - [detect-secrets](https://github.com/Yelp/detect-secrets)\n\n8. **Consider Java SAST Tools**:\n - Explore Java static application security testing (SAST) tools for further analysis.\n\n**First Steps**:\n- Set up a new GitHub Action workflow file.\n- Define the pipeline stages and include each tool as a separate job.\n- Start with integrating git-secrets and trufflehog to establish a baseline.\n- Ensure each tool’s results are captured and logged effectively.\n- Gradually add more tools and functionalities to the pipeline based on initial performance metrics.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/wrongsecrets/issues/424",
@@ -8694,6 +8999,7 @@
"node_id": "I_kwDOETRnAc5SbjNS",
"title": "Create a separate repo where you allow actions to run, so you can get the secret out",
"body": "Create a separate repo where you allow actions to run, so you can get the secret out. The idea would be that you have a challenge with a forkable action+Secret, so anyone forking this repository should get access to the given secret.\r\nTODO:\r\n- [ ] Have the Github secret forkable\r\n- [ ] Create a github action using it\r\n- [ ] Create the actual challenge with the secret encrypted as part of the java code (See contributing.md on how to create the challenge)",
+ "summary": "Create a separate GitHub repository to enable actions to run and expose a secret. Ensure that the repository allows forking so that any user who forks the repo can access the designated secret. \n\n**Technical Steps:**\n1. **Configure GitHub Secrets:** Set up the repository to include a GitHub secret that is forkable.\n2. **Develop GitHub Action:** Create a GitHub Action that utilizes the forkable secret.\n3. **Design Challenge:** Implement the challenge by encrypting the secret within the Java code. Refer to `contributing.md` for detailed instructions on how to structure the challenge.\n\n**First Steps:**\n- Set up a new repository on GitHub.\n- Research GitHub's handling of secrets in forked repositories to ensure the secret is accessible.\n- Draft the initial version of the GitHub Action and test its functionality with a sample secret.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/wrongsecrets/issues/425",
@@ -8722,6 +9028,7 @@
"node_id": "I_kwDOETRnAc5aLa_f",
"title": "Create challengecreatorcli",
"body": "In order to speed up challengecreation we can best create a cli which creates the required class file and asciidoc. It only needs options like:\r\n- type (cloud/k8s/docker)\r\n- Title (Freeform)\r\nAnd then generate the required challenge file and the asciidoc ",
+ "summary": "Create a command-line interface (CLI) named `challengecreatorcli` to streamline challenge creation. The CLI should accept the following options:\n\n- **Type**: Specify the type of challenge (options include `cloud`, `k8s`, or `docker`).\n- **Title**: Provide a freeform title for the challenge.\n\nUpon execution, the CLI must perform the following actions:\n\n1. Generate the required class file based on the specified type.\n2. Create an AsciiDoc file that includes the challenge title and relevant content.\n\nTo tackle this problem:\n\n1. Set up a new CLI project using a suitable programming language (e.g., Python, Node.js).\n2. Implement argument parsing to handle the `type` and `title` options.\n3. Define templates for the class file and AsciiDoc to ensure consistent formatting.\n4. Write functions to generate the class file and AsciiDoc file based on the user inputs.\n5. Test the CLI with various inputs to ensure it creates files correctly.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/wrongsecrets/issues/555",
@@ -8752,6 +9059,7 @@
"node_id": "I_kwDOETRnAc5eRsJe",
"title": "Have a secret in .gitignore /.ssh",
"body": "- [ ] Create a branch with added secret in .gitignore and a secret in .ssh\r\n- [ ] Create the challenges for both where the secrets for both files are having their own separate challenge with encryption of the secrets to hide them from detection engines.",
+ "summary": "1. Create a new branch dedicated to secrets management.\n2. Add a secret file to the `.gitignore` to prevent it from being tracked.\n3. Place an additional secret in the `.ssh` directory, ensuring it is also included in the `.gitignore`.\n4. Develop separate challenges for each secret. \n5. Implement encryption methods for the secrets to obscure them from detection engines. \n6. Investigate suitable encryption algorithms (e.g., AES, RSA) for securing the secrets.\n7. Test the implementation to confirm that the secrets are encrypted properly and remain undetected.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/wrongsecrets/issues/613",
@@ -8784,6 +9092,7 @@
"node_id": "I_kwDOETRnAc5eRsLw",
"title": "Native languages round 2: SWift! ",
"body": "This challenge is about finding hardcoded secrets in binaries in Swift! With this we want to explain to our users that no language or binary is safe to just put the secret in offline. For this you need to:\r\n\r\n- [ ] Add a swift binary to the [wrongsecrets/binaries ](https://github.com/OWASP/wrongsecrets-binaries) repowith crosscompiling for the various OSes\r\n- [ ] Add a challenge here that uses the binary (See contributing.md and the code of the other binary challenges).",
+ "summary": "Create a Swift binary to demonstrate the challenge of finding hardcoded secrets within binaries. Follow these steps:\n\n1. **Add Swift Binary**: Implement a Swift binary and place it in the [wrongsecrets/binaries](https://github.com/OWASP/wrongsecrets-binaries) repository. Ensure the binary supports cross-compilation for various operating systems.\n\n2. **Develop Challenge**: Create a corresponding challenge that utilizes the new binary. Refer to the guidelines in `contributing.md` and examine the code of existing binary challenges for structure and style.\n\n3. **Technical Details**:\n - Use Swift's features to hardcode secrets in the binary.\n - Ensure the binary can be built for macOS, Linux, and Windows.\n - Document the build process for each OS in the repository.\n\n4. **First Steps**:\n - Set up your Swift development environment.\n - Create a simple Swift application that includes hardcoded secrets.\n - Test cross-compilation for different operating systems.\n - Draft the challenge description and expected outcomes for users engaging with the binary.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/wrongsecrets/issues/615",
@@ -8815,6 +9124,7 @@
"node_id": "I_kwDOETRnAc5f-HcJ",
"title": "aad-pod-identity is no longer supported & K8S namespace does not enforce restricted policy in Azure",
"body": "In order to complete the migration to Kubernetes 1.25 on AKS and enforce the `restricted` psa in the default namespace, we need to migrate from `aad-pod-identity` to https://azure.github.io/azure-workload-identity/docs/\r\n\r\nIssue is caused by #652 #646\r\n\r\nPlease note that: if you want to pick up this issue, you have to have experience with:\r\n- terraform\r\n- helm\r\n- Azure AKS\r\n- Azure workload identity",
+ "summary": "Migrate from `aad-pod-identity` to Azure Workload Identity for Kubernetes 1.25 on AKS. Enforce the `restricted` Pod Security Admission (PSA) policy in the default namespace. \n\nAddress the issues caused by #652 and #646. \n\nImplement the following first steps:\n1. Review the Azure Workload Identity documentation to understand the migration process.\n2. Assess the current setup of `aad-pod-identity` in your AKS environment.\n3. Plan the migration strategy, ensuring minimal downtime and compatibility with existing workloads.\n4. Update Terraform scripts to use Azure Workload Identity configurations.\n5. Modify Helm charts to integrate with the new identity mechanism.\n6. Test the migration in a staging environment before deploying to production. \n\nEnsure you have experience with Terraform, Helm, Azure AKS, and Azure Workload Identity before proceeding.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/wrongsecrets/issues/670",
@@ -8845,6 +9155,7 @@
"node_id": "I_kwDOETRnAc5g53rq",
"title": "DAST scan - Investigate & fix if required results of the ZAP scan",
"body": "\r\nA ZAP baseline scan demonstrates several issues. Investigate each of these issues and create a fix if required, or leave on ignore if not relevant. Full ZAP report can be found in GitHub Actions.\r\n\r\nIssues:\r\n- [ ] Information Disclosure - Suspicious Comments [10027]\r\n- [ ] User Controllable HTML Element Attribute (Potential XSS) [10031]\r\n- [ ] Non-Storable Content [10049]\r\n- [ ] Cookie without SameSite Attribute [10054]\r\n- [ ] CSP: Wildcard Directive [10055]\r\n- [ ] Permissions Policy Header Not Set [10063]\r\n- [ ] Modern Web Application [10109]\r\n- [ ] Dangerous JS Functions [10110]\r\n- [ ] Loosely Scoped Cookie [90033]\r\n\r\n### Please provide relevant logs\r\n\r\n```\r\nIGNORE: Information Disclosure - Suspicious Comments [10027] x 14 \r\n\thttp://localhost:8080/webjars/bootstrap/5.2.3/js/bootstrap.bundle.min.js (200 OK)\r\n\thttp://localhost:8080/webjars/datatables/1.13.2/js/dataTables.bootstrap5.min.js (200 OK)\r\n\thttp://localhost:8080/webjars/datatables/1.13.2/js/jquery.dataTables.min.js (200 OK)\r\n\thttp://localhost:8080/webjars/github-buttons/2.14.1/dist/buttons.js (200 OK)\r\n\thttp://localhost:8080/webjars/jquery/3.6.3/jquery.js (200 OK)\r\nIGNORE: User Controllable HTML Element Attribute (Potential XSS) [10031] x 6 \r\n\thttp://localhost:8080/challenge/0 (200 OK)\r\n\thttp://localhost:8080/challenge/0 (200 OK)\r\n\thttp://localhost:8080/challenge/0 (200 OK)\r\n\thttp://localhost:8080/challenge/2 (200 OK)\r\n\thttp://localhost:8080/challenge/2 (200 OK)\r\nIGNORE: Non-Storable Content [10049] x 11 \r\n\thttp://localhost:8080 (200 OK)\r\n\thttp://localhost:8080/ (200 OK)\r\n\thttp://localhost:8080/challenge/0 (200 OK)\r\n\thttp://localhost:8080/challenge/1 (200 OK)\r\n\thttp://localhost:8080/challenge/2 (200 OK)\r\nIGNORE: Cookie without SameSite Attribute [10054] x 1 \r\n\thttp://localhost:8080/challenge/0 (200 OK)\r\nIGNORE: CSP: Wildcard Directive [10055] x 12 \r\n\thttp://localhost:8080 (200 OK)\r\n\thttp://localhost:8080/challenge/0 (200 OK)\r\n\thttp://localhost:8080/robots.txt (404 Not Found)\r\n\thttp://localhost:8080/sitemap.xml (404 Not Found)\r\n\thttp://localhost:8080 (200 OK)\r\nIGNORE: Permissions Policy Header Not Set [10063] x 11 \r\n\thttp://localhost:8080 (200 OK)\r\n\thttp://localhost:8080/ (200 OK)\r\n\thttp://localhost:8080/challenge/0 (200 OK)\r\n\thttp://localhost:8080/challenge/1 (200 OK)\r\n\thttp://localhost:8080/challenge/2 (200 OK)\r\nIGNORE: Modern Web Application [10109] x 11 \r\n\thttp://localhost:8080 (200 OK)\r\n\thttp://localhost:8080/ (200 OK)\r\n\thttp://localhost:8080/challenge/0 (200 OK)\r\n\thttp://localhost:8080/challenge/1 (200 OK)\r\n\thttp://localhost:8080/challenge/2 (200 OK)\r\nIGNORE: Dangerous JS Functions [10110] x 1 \r\n\thttp://localhost:8080/webjars/jquery/3.6.3/jquery.js (200 OK)\r\nIGNORE: Loosely Scoped Cookie [90033] x 1 \r\n\thttp://localhost:8080/challenge/0 (200 OK)\r\n```\r\n\r\n### Any possible solutions?\r\n\r\nNeeds further investigation per issue.\r\n\r\n### If the bug is confirmed, would you be willing to submit a PR?\r\n\r\nYes\r\n",
+ "summary": "Investigate and address the findings from the ZAP baseline scan. Review the full ZAP report located in GitHub Actions. Each identified issue requires examination to determine if a fix is necessary or if it can be ignored.\n\n### Issues to Address:\n- **Information Disclosure - Suspicious Comments [10027]**: Review comments in the listed JavaScript files. Determine if sensitive information is being exposed.\n- **User Controllable HTML Element Attribute (Potential XSS) [10031]**: Analyze the specified endpoints for potential XSS vulnerabilities due to user input affecting HTML attributes.\n- **Non-Storable Content [10049]**: Assess the content that cannot be stored and evaluate if it impacts functionality or security.\n- **Cookie without SameSite Attribute [10054]**: Ensure cookies have the SameSite attribute set to prevent cross-site request forgery attacks.\n- **CSP: Wildcard Directive [10055]**: Modify Content Security Policy to eliminate wildcard directives for better security.\n- **Permissions Policy Header Not Set [10063]**: Implement the Permissions-Policy header to restrict features in the application.\n- **Modern Web Application [10109]**: Review compliance with modern web standards and practices.\n- **Dangerous JS Functions [10110]**: Identify and mitigate any risks associated with the use of dangerous JavaScript functions.\n- **Loosely Scoped Cookie [90033]**: Tighten cookie scope to enhance security.\n\n### Technical Steps:\n1. Access the ZAP report in GitHub Actions.\n2. For each issue, reproduce the findings locally for further analysis.\n3. Document the findings for each issue, noting whether a fix is required or if it can be ignored.\n4. Implement necessary fixes or updates in the application code.\n5. Test changes to ensure vulnerabilities are resolved.\n6. Prepare a pull request (PR) with all modifications for review.\n\nConfirm your willingness to submit a PR upon verification of the issues.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/wrongsecrets/issues/709",
@@ -8869,11 +9180,12 @@
"model": "github.issue",
"pk": 312,
"fields": {
- "nest_created_at": "2024-09-11T19:41:47.000Z",
- "nest_updated_at": "2024-09-11T19:41:47.000Z",
+ "nest_created_at": "2024-09-11T19:41:47Z",
+ "nest_updated_at": "2024-09-11T19:41:47Z",
"node_id": "I_kwDOETRnAc5hKLH7",
"title": "New Challenge: use weak KDF to protect a secret",
"body": "### Context\r\n\r\nThis is a Docker challenge focused on using the wrong KDF to protect a secret. \r\nIn crypto-js there is an AES encryption mechanism, which uses MD5 as its KDF. This library is often used on mobile for encryption in hybrid apps. So what if we make a challenge in which the user has to find the right \"pin\"to be able to decrypt a secret offered on screen? (E.g. a 4-8 digit pin with md5 based KDF, and a secret fitting in 128 bytes.\r\nWe need to relate it to the MSTG on how to use (P)KDF with additional entropy and contextual binding.\r\n\r\n### Did you encounter this in real life? Could you tell us more about the scenario?\r\nSee https://github.com/brix/crypto-js/blob/c8a2312474ae60c823f3c00b4d7aac2da460bbfc/test/config-test.js for test defaults.\r\n\r\n",
+ "summary": "Create a Docker challenge that utilizes a weak Key Derivation Function (KDF) to protect a secret. Implement AES encryption using the crypto-js library, which employs MD5 as its KDF. The challenge should require users to discover the correct \"pin\" (a 4-8 digit numeric code) to decrypt a secret displayed on the screen, ensuring the secret does not exceed 128 bytes.\n\n1. Set up a Docker environment that includes the necessary dependencies for crypto-js.\n2. Implement the encryption mechanism using MD5 as the KDF for AES encryption.\n3. Generate a secret and encrypt it using the established mechanism.\n4. Design a user interface that prompts participants to input their guessed pin.\n5. Validate the pin against the KDF and provide feedback on success or failure.\n6. Reference the Mobile Security Testing Guide (MSTG) for guidelines on utilizing (P)KDF with additional entropy and contextual binding to deepen the challenge's complexity.\n\nFocus on ensuring that the challenge clearly illustrates the pitfalls of using weak KDFs in cryptographic implementations.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/wrongsecrets/issues/713",
@@ -8904,6 +9216,7 @@
"node_id": "I_kwDOETRnAc5hQIIF",
"title": "Optimize Github actions",
"body": "In order to become more efficient in CLI time there are a bunch of things we can simplify:\r\n- [ ] 1. create 1 clean-install with all the sskips in place materials to have the jar file\r\n- [ ] 2. Change swagger action to depend on 1\r\n- [ ] 3. Change DAST to depend on 1\r\n- [ ] 4. Change Docker container creation action to depend on1\r\n- [ ] Optimize many of the github actions to just run once instead of twice during a PR.\r\n- [ ] quickfail container test if something is wrong...",
+ "summary": "Optimize GitHub Actions for increased efficiency in CLI time. Follow these steps:\n\n1. **Create a Clean Install**: Implement a single clean installation action that incorporates all necessary skips to generate the JAR file efficiently.\n \n2. **Modify Swagger Action**: Adjust the Swagger action to depend on the newly created clean install action to streamline the process.\n\n3. **Revise DAST Dependencies**: Update the Dynamic Application Security Testing (DAST) action to rely on the clean install action, ensuring it runs only after the successful creation of the JAR file.\n\n4. **Refactor Docker Container Creation**: Change the Docker container creation action to depend on the clean install, minimizing redundant builds.\n\n5. **Optimize Action Runs**: Review and modify existing GitHub actions to ensure they execute only once during a Pull Request instead of twice, reducing unnecessary processing time.\n\n6. **Implement Quick Fail for Container Tests**: Enhance the container testing process to include a quick fail mechanism that halts the test if any issues are detected early on.\n\nInitiate the optimization by prioritizing the creation of the clean install action, followed by updating dependencies for Swagger, DAST, and Docker actions. Then, assess the current action runs and implement the quick fail feature in the container tests.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/wrongsecrets/issues/714",
@@ -8934,6 +9247,7 @@
"node_id": "I_kwDOETRnAc5lTn1o",
"title": "Nexus deployment credentials in settings.xml",
"body": "This challenge is about packing and explaining why you should not have nexus deployment credentials in your github project hardcoded.\r\nHowever, we will not have an actual nexus setup right now. \r\n\r\nTODO's:\r\n\r\n- [x] - Create a settings.xml for Maven to be able to connect to an imaginary Nexus repo See https://github.com/sonatype/nexus-book-examples/blob/master/maven/settings/settings.xml as an example.\r\n- [x] - Implement a challenge that reads the actual challenge secret value from the settings.xml (see contributing.md for more details)",
+ "summary": "Create a `settings.xml` file for Maven to connect to a hypothetical Nexus repository without hardcoding deployment credentials in your GitHub project. Reference the example from the Sonatype Nexus book [here](https://github.com/sonatype/nexus-book-examples/blob/master/maven/settings/settings.xml). \n\nImplement a challenge that securely reads the challenge secret value from `settings.xml`. Follow the guidelines in `contributing.md` for specifics on reading the secret value.\n\nFirst steps to tackle the problem:\n1. Design the `settings.xml` structure, ensuring it contains placeholders for credentials that are not included directly in the GitHub repository.\n2. Develop a mechanism to read the secret credential values from the `settings.xml` during the build process, potentially utilizing environment variables or secure storage solutions like GitHub Secrets.\n3. Write documentation explaining the importance of not hardcoding sensitive information in source code, focusing on security best practices.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/wrongsecrets/issues/810",
@@ -8965,6 +9279,7 @@
"node_id": "I_kwDOETRnAc5lTp8Y",
"title": "Add misconfiguration of docker secret in code (See for docker compose: https://docs.docker.com/engine/swarm/secrets/)",
"body": "Create a challenge for docker compose setup, where the compose secret is hardcoded inside teh docker compose yml\r\n\r\nTODO:\r\n- [ ] Implement a docker-compose setup for wrongsecrets with the secret as an env var, add the compose file to the repo\r\n- [ ] implement the challenge according to contributing.md, but make sure we read the challenge from the file so we can play the challenge without the need to actually use teh docker-compose setup.",
+ "summary": "Create a Docker Compose challenge by misconfiguring Docker secrets in the `docker-compose.yml` file. Specifically, hardcode the secret as an environment variable within the Docker Compose setup. \n\nFollow these steps:\n\n1. **Implement Docker Compose Setup:**\n - Create a `docker-compose.yml` file that includes a service with a secret improperly defined as an environment variable.\n - Reference the Docker documentation for secrets to ensure the setup is clear: [Docker Secrets Documentation](https://docs.docker.com/engine/swarm/secrets/).\n\n2. **Add Compose File to Repository:**\n - Commit the `docker-compose.yml` file containing the misconfiguration to the GitHub repository.\n\n3. **Implement the Challenge:**\n - Follow the guidelines outlined in `contributing.md` for creating challenges.\n - Ensure the challenge can be executed without the need for the Docker Compose setup by reading the challenge from a file.\n\n4. **Test the Challenge:**\n - Verify that the challenge accurately reflects the misconfiguration situation and is playable as intended.\n\nThis will provide an educational challenge for users to learn about Docker secrets and their correct implementation.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/wrongsecrets/issues/811",
@@ -8996,6 +9311,7 @@
"node_id": "I_kwDOETRnAc5lTqhP",
"title": "Add misconfiguration for mounting in secret in during build: https://docs.docker.com/engine/reference/commandline/buildx_build/",
"body": "This challenge is about using docker secrets from docker buildx buildpacks:\r\n\r\nUse the --secret, but then with a hardcoded value referenced in the shell script to publish the docker container and explain that using --secret is a good idea, but not with a hardcoded call in a git-comitted buildscript.\r\n\r\nTodo:\r\n- [ ] Embed the secret variable in https://github.com/OWASP/wrongsecrets/blob/master/.github/scripts/docker-create.sh and make sure it lands in a file in the docker container\r\n- [ ] create a challenge that reads the secret from that file and teaches why this is a bad idea (See contributing.md)",
+ "summary": "Implement misconfiguration for mounting secrets during Docker build with buildx. Reference the Docker build documentation for proper usage of the `--secret` flag.\n\n1. Modify the script located at `https://github.com/OWASP/wrongsecrets/blob/master/.github/scripts/docker-create.sh` to embed a secret variable using the `--secret` option.\n2. Ensure that the secret is written to a file inside the Docker container during the build process.\n3. Create a challenge that reads the secret from the specified file, demonstrating the risks associated with hardcoding secrets in version-controlled build scripts.\n\nFirst steps:\n- Review the current script to identify where secrets are being handled.\n- Integrate the `--secret` flag into the build command.\n- Create a temporary file in the Docker container to store the secret.\n- Develop a separate challenge that educates users on the dangers of hardcoding secrets, in accordance with the guidelines outlined in `contributing.md`.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/wrongsecrets/issues/812",
@@ -9025,6 +9341,7 @@
"node_id": "I_kwDOETRnAc5wlGJO",
"title": "Have a challenge with a backup bucket containing the secret",
"body": "### Context\r\n\r\n- What should the challenge scenario be like?\r\nHave a backup s3/storage bucket with a private ed25519 key publicly exposed \r\n- What should the participant learn from completing the challenge?\r\nSecure your backup at all cost\r\n- For what category would the challenge be? (e.g. Docker, K8s, binary)\r\nDocker/cloud depending on how we implement the backup solution\r\n\r\nActions:\r\n- [ ] create separate Terraform folder to have an S3 bucket (in our AWS folder) under the name \"backupchallenge\"\r\n- [ ] have the key copying logic in a shell script using AWS CLI as part of the backupchallenge folder\r\n- [ ] implement the challenge according to contributing.md and make sure you hide the key in your classfile.\r\n",
+ "summary": "**Create a Backup Challenge for Secure Storage of Secrets**\n\n1. **Define the Challenge Scenario**: Set up a backup S3/storage bucket that contains a private ed25519 key, which is intentionally exposed to test security practices.\n\n2. **Establish Learning Objectives**: Ensure participants understand the importance of securing backup data and implementing best practices for secret management.\n\n3. **Categorize the Challenge**: Classify the challenge under Docker/cloud technologies, based on the implementation of the backup solution.\n\n**Next Steps**:\n\n- **Create Infrastructure**: \n - Set up a new folder in Terraform specifically for this challenge.\n - In this folder, create an S3 bucket named \"backupchallenge\" within the existing AWS folder structure.\n\n- **Implement Backup Logic**:\n - Develop a shell script that utilizes the AWS CLI to handle the logic for copying the private key into the S3 bucket as part of the backup process.\n\n- **Follow Contribution Guidelines**: \n - Implement the challenge as outlined in the contributing.md document.\n - Ensure that the private key is hidden in your class file to prevent exposure.\n\nBy following these steps, you will create a valuable learning experience focused on securing sensitive information in cloud storage.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/wrongsecrets/issues/982",
@@ -9054,6 +9371,7 @@
"node_id": "I_kwDOETRnAc54_uwV",
"title": "performance for the main screen load",
"body": "Improve the performance for the main screen loading by:\r\n\r\n- [ ] pre-compiling the content for the table as much as possible\r\n- [ ] Have a minification step for all the js/css offered (see https://pagespeed.web.dev/analysis/https-wrongsecrets-herokuapp-com/xltdvc76x1?form_factor=mobile)\r\n- [ ] find a way to strip css for bootstrap that we will never use?\r\n- [ ] load all computed integers from cache (max score)\r\n- [ ] stop blocking of rendering\r\n- [ ] enable virtual threads",
+ "summary": "Enhance the main screen loading performance by implementing the following steps:\n\n1. Pre-compile content for the table to reduce rendering time. Investigate the use of static site generation or server-side rendering techniques to deliver pre-rendered HTML.\n\n2. Implement a minification step for all JavaScript and CSS files. Utilize tools like UglifyJS for JS and CSSNano for CSS to minimize file sizes, which can decrease loading times. Reference the PageSpeed insights for further optimization.\n\n3. Analyze Bootstrap usage and identify unused CSS. Employ tools such as PurgeCSS to remove unused styles from the production build, thereby reducing the overall CSS footprint.\n\n4. Optimize caching strategies for computed integers. Investigate caching mechanisms (e.g., Redis or in-memory caching) to store frequently accessed data and minimize computation on subsequent loads.\n\n5. Review the rendering pipeline to eliminate any blocking resources that hinder page rendering. Consider deferring non-essential JavaScript and utilizing asynchronous loading techniques.\n\n6. Explore enabling virtual threads to improve concurrency and resource management within the application. Investigate the compatibility of your current environment with Project Loom or similar threading models.\n\nBegin by profiling the existing loading performance using tools such as Google Lighthouse or WebPageTest to establish a baseline and identify specific bottlenecks. Then, prioritize the above tasks based on their potential impact on performance.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/wrongsecrets/issues/1115",
@@ -9083,6 +9401,7 @@
"node_id": "I_kwDOETRnAc5-lrGO",
"title": "Kubernetes ephemeral container to extract secret cached by subprocess",
"body": "### Context\r\n\r\n- What should the challenge scenario be like?\r\nWe're interfacing with a secrets mgmt system to keep our secret safe, but we don't want to make a network call every time we use the secret. We've built an abstraction to handle interfacing with the system, and for performance reasons, we're caching the secret in memory using a spawned subprocess (or in memory). Using an appropriate container with debugging tools (jmap?), we can extract the secret from the subprocess/the heap!\r\n\r\n- What should the participant learn from completing the challenge?\r\nBeing able to exec in prod can harm even relatively safe secrets. Also, be careful with debug modes 🤡 \r\n\r\n- For what category would the challenge be? (e.g. Docker, K8s, binary)\r\nK8s\r\n\r\n### Did you encounter this in real life? Could you tell us more about the scenario?\r\n\r\nI had to attach a debug ephemeral container to a running one, which had multiple debugging tools installed.\r\n\r\n### If the challenge request is approved, would you be willing to submit a PR?\r\n\r\nYes\r\n",
+ "summary": "Create a Kubernetes ephemeral container to extract a secret cached by a subprocess. \n\n### Technical Details\n- Design a challenge scenario where a secrets management system is interfaced to retrieve secrets, avoiding multiple network calls by caching secrets in a subprocess.\n- Implement an ephemeral container equipped with debugging tools (e.g., `jmap`) to access the subprocess's memory and extract the cached secret.\n- Highlight the risks of executing commands in a production environment, emphasizing the potential exposure of even safe secrets and the implications of enabling debug modes.\n\n### First Steps\n1. Define the requirements for the ephemeral container, including necessary debugging tools.\n2. Set up a Kubernetes environment to deploy the application with the caching mechanism.\n3. Enable ephemeral containers in the Kubernetes cluster configuration.\n4. Develop a procedure to attach the ephemeral container to the running application pod.\n5. Test the extraction of the cached secret using the debugging tools from the ephemeral container.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/wrongsecrets/issues/1236",
@@ -9113,6 +9432,7 @@
"node_id": "I_kwDOETRnAc5_7_Qc",
"title": "Have a .net dll based RE challenge!",
"body": "### Context\r\n\r\nDLLs and exes can be hard to debug. What about hidden secrets over there?\r\n\r\nCan we have a challenge whiich is cross-compiled using .Net runtime and a few DLLs to have a secret inside similar like the golang/rust challenges?\r\n\r\n- [x] Add .net based binary to the [wrongsecrets/binaries ](https://github.com/OWASP/wrongsecrets-binaries)repowith crosscompiling for the various OSes\r\n- [ ] Add a challenge here that uses the binary (See contributing.md and the code of the other binary challenges).",
+ "summary": "Create a .NET DLL-based reverse engineering challenge. \n\n1. Cross-compile a .NET binary using the .NET runtime for multiple operating systems.\n2. Integrate the compiled DLL into the [wrongsecrets/binaries](https://github.com/OWASP/wrongsecrets-binaries) repository.\n3. Develop a challenge that utilizes the binary, following the guidelines in contributing.md and examining the structure of existing binary challenges.\n\nFirst steps:\n- Set up a development environment with .NET SDK.\n- Use `dotnet publish` to cross-compile the binary for different OSes.\n- Create a new challenge file that references the compiled DLL and includes the challenge description and solution.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/wrongsecrets/issues/1243",
@@ -9145,6 +9465,7 @@
"node_id": "I_kwDOETRnAc6GX1Qz",
"title": "Goerli testnet is being deprecated: help us migrate to another testnet!",
"body": "Challenge 25, 26, and 27 are based on the Goerli testnet, which is being deprecated. We need to redo these challenges on another testnet.",
+ "summary": "Migrate challenges 25, 26, and 27 from the deprecated Goerli testnet to a new testnet. \n\n1. Identify a suitable alternative testnet, such as Sepolia or Mumbai.\n2. Update the smart contracts and deployment scripts to be compatible with the chosen testnet.\n3. Modify any relevant configurations in the project to point to the new testnet.\n4. Test the deployment and functionality of the challenges on the new testnet.\n5. Document the migration process for future reference. \n\nEnsure all dependencies and libraries are compatible with the selected testnet during the migration.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/wrongsecrets/issues/1360",
@@ -9171,10 +9492,11 @@
"pk": 322,
"fields": {
"nest_created_at": "2024-09-11T19:43:35.817Z",
- "nest_updated_at": "2024-09-12T00:14:58.204Z",
+ "nest_updated_at": "2024-09-13T17:00:49.923Z",
"node_id": "I_kwDOERPzhs6EXuue",
"title": "Field \"Vulnerability Mapping\"",
"body": "Hi,\r\n\r\nIt is normal that I do not found the field **Vulnerability Mapping** in the JSON returned?\r\n\r\n👀 View from the web:\r\n\r\n\r\n👀 View from the JSON:\r\n\r\n\r\n📖 JSON file:\r\n[cwe.json](https://github.com/OWASP/cwe-tool/files/14839275/cwe.json)\r\n\r\n💻 Version used:\r\n\r\n\r\n🤔 Did I miss something?\r\n\r\n😉 Thanks a lot in advance for your help.\r\n\r\n\r\n",
+ "summary": "The issue raised concerns the absence of the \"Vulnerability Mapping\" field in the JSON response compared to what is displayed on the web interface. The user provided screenshots for both views and referenced a specific JSON file for further context. They are seeking clarification on whether this omission is expected or if there is something they might have overlooked. \n\nTo resolve this, it may be necessary to investigate the JSON structure and confirm if the \"Vulnerability Mapping\" field should indeed be included or if there are updates required to align the JSON output with the web display.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/cwe-tool/issues/29",
@@ -9197,10 +9519,11 @@
"pk": 323,
"fields": {
"nest_created_at": "2024-09-11T19:44:18.875Z",
- "nest_updated_at": "2024-09-11T19:44:18.875Z",
+ "nest_updated_at": "2024-09-13T15:15:55.661Z",
"node_id": "I_kwDOEQbdus5_PwOh",
"title": "Website shows ancient WIP Draft Chapter Policy",
"body": "When browsing on the OWASP website (owasp.org) and you browse to chapter policies\r\n\r\nAbout->Policies\r\n\r\nThen select [Chapters Policy](https://owasp.org/www-policy/operational/chapters)\r\n\r\nYou will notice on the top right a \"next\" selection entitled \"Chapters Policy - Draft (WIP)\"\r\n\r\nWhen selecting this entry you will be sent to a page that is out of date and states:\r\n\r\n\"Members are invited to provide feedback on this draft policy until January 22, 1970\"\r\n\r\nThis page needs to be removed or at least updated.",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-committee-chapter/issues/24",
@@ -9227,6 +9550,7 @@
"node_id": "MDU6SXNzdWU4OTE5Nzg5MzE=",
"title": "Integrate threat engine with Cornucopia / EoP cards",
"body": "TD suggests STRIDE when adding threats to the data flow diagram, and one idea is that when one of STRIDE categories is suggested by TD, then the default description could have a link to the specific EoP suit (so for example if it is Repudiation then we could link to the EoP Repudiation suit).\r\n\r\nThis would need the EoP to be split out into the individual suits (Spoofing, Tampering, Repudiation, Information disclosure, Denial of service, Elevation of privilege), subject of https://github.com/adamshostack/eop/issues/3",
+ "summary": "Integrate the threat engine with Cornucopia and EoP cards. Use STRIDE methodology for adding threats to the data flow diagram. When a STRIDE category is identified by the threat detection (TD), implement a default description that links to the corresponding EoP suit. For instance, if the threat is Repudiation, link it to the EoP Repudiation suit.\n\nFirst steps to tackle the problem:\n\n1. Review STRIDE categories and their definitions.\n2. Identify the EoP suits (Spoofing, Tampering, Repudiation, Information Disclosure, Denial of Service, Elevation of Privilege).\n3. Split the EoP content into individual suits as mentioned in issue #3 of the EoP repository.\n4. Develop a mapping mechanism that connects STRIDE threats to the appropriate EoP suits.\n5. Update the data flow diagram generation logic to include these links in the default descriptions.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/140",
@@ -9241,13 +9565,13 @@
"author": 43,
"repository": 502,
"assignees": [
- 43,
- 113
+ 113,
+ 43
],
"labels": [
- 71,
72,
- 73
+ 73,
+ 71
]
}
},
@@ -9260,6 +9584,7 @@
"node_id": "MDU6SXNzdWU5Mzk1NjU3NzY=",
"title": "Add templates to the list of sample threat models",
"body": "Add feature to import a threat template or a list of pre defined threats. Seems ... chaotic to have to re-add threats for every model that you design. especially if designing a lot of threat models. When reusing components/elements, you will encounter the same threats... this tool should scale to reuse predefined components/elements and threats. Process of generating threat list is way too manual.",
+ "summary": "Add templates for sample threat models to streamline threat modeling. Implement an import feature for threat templates or predefined threat lists to reduce redundancy in threat definitions across multiple models. \n\n1. Analyze the current threat modeling process to identify repetitive tasks related to threat addition.\n2. Design a user-friendly interface for importing threat templates.\n3. Develop a backend mechanism to store and retrieve these templates efficiently.\n4. Ensure the system allows for easy integration of predefined threats with existing models.\n5. Create documentation to guide users on how to utilize the new import feature and templates.\n\nFocus on enhancing scalability and reducing manual effort in generating threat lists.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/220",
@@ -9275,8 +9600,8 @@
"repository": 502,
"assignees": [],
"labels": [
- 71,
- 73
+ 73,
+ 71
]
}
},
@@ -9289,6 +9614,7 @@
"node_id": "MDU6SXNzdWU4OTE5Nzc3MTI=",
"title": "Threat generation for more than one element",
"body": "**Is your feature request related to a problem? Please describe.**\r\nIt's frustrating that you have to choose each element and generate threats for each and everyone of them.\r\n\r\n**Describe the solution you'd like**\r\nAbility to choose more than one, possibly all elements and generate threats. That way I could generate the standard threats, which should be put in an editable library btw, and then get the responsible developers to do a review. In that way it's a great tool for awareness and learning as well.\r\n\r\n**Describe alternatives you've considered**\r\nAt the moment I've made a list of all standard mitigations and \"threat descriptions\" in the form of CAPEC and/or CWE references in the project. I draw the model, go through all of the elements and generate standard threats, then go through the json file to manually search and replace.\r\n\r\n**Additional context**\r\nNope\r\n\r\n",
+ "summary": "Implement a feature that allows users to select multiple elements simultaneously for threat generation. \n\n1. Identify the current process of selecting individual elements and generating threats for each.\n2. Develop a multi-selection interface in the user interface to allow bulk selection of elements.\n3. Modify the threat generation algorithm to process multiple elements at once, producing a comprehensive list of threats.\n4. Create an editable library for standard threats so users can customize and categorize threats as needed.\n5. Implement a review mechanism for developers to evaluate the generated threats against their specific elements.\n\nConsider creating a prototype for the multi-selection UI and testing it with a subset of elements to refine the user experience.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/132",
@@ -9304,9 +9630,9 @@
"repository": 502,
"assignees": [],
"labels": [
- 71,
72,
- 73
+ 73,
+ 71
]
}
},
@@ -9315,10 +9641,11 @@
"pk": 327,
"fields": {
"nest_created_at": "2024-09-11T20:08:07.376Z",
- "nest_updated_at": "2024-09-11T20:08:07.376Z",
+ "nest_updated_at": "2024-09-13T03:53:38.188Z",
"node_id": "MDU6SXNzdWU4NjYyODA3OTU=",
"title": "Support Azure OAuth",
"body": "**Describe what problem your feature request solves**\r\nAdd ability to use multiple passport connectors, such as Azure AD.\r\n\r\n**Describe the solution you'd like**\r\nI'd like to add the Azure AD Passport connector for authentication/authorization. I _think_ it would make the most sense to have this be enabled via configuration, and also allow for multiple passport strategies. For example: `export PASSPORT_STRATEGIES=\"['github', 'azuread-openidconnect']\"` or `export PASSPORT_STRATEGIES=\"['github']\"`\r\nThis would open up the possibility of adding other passport strategies/connectors in the future.\r\n\r\n\r\n**Additional context**\r\nSome questions I have:\r\n- Because Azure AD requires a bunch more config options, would it make sense to implement something like [dotenv](https://github.com/motdotla/dotenv) to make configuration a bit easier?\r\n - If so, should that be a separate feature / PR to keep the scope somewhat limited?\r\n- Does it make sense to restructure how the passport authentication is configured to make it easier to add other authentication types in the future?\r\n\r\nI'm eager to add this functionality, but wanted to see if it's a desired feature, and what the appetite is as far as the size and scope of pull requests.",
+ "summary": "Add Azure OAuth support by implementing the Azure AD Passport connector for authentication and authorization. Enable configuration for multiple passport strategies, allowing for flexibility in authentication methods. Use environment variable `PASSPORT_STRATEGIES` to specify active strategies, e.g., `export PASSPORT_STRATEGIES=\"['github', 'azuread-openidconnect']\"`.\n\nConsider the following steps to tackle the problem:\n\n1. **Research Azure AD Integration**: Review Azure AD documentation to understand required configuration and authentication flows.\n \n2. **Implement Configuration Management**: Decide whether to integrate a solution like `dotenv` for easier configuration management. If chosen, create a separate issue or PR to maintain focus.\n\n3. **Refactor Passport Authentication Setup**: Analyze the current passport authentication setup and restructure it to facilitate the addition of new strategies in the future.\n\n4. **Develop and Test**: Code the Azure AD Passport connector, ensuring it adheres to existing frameworks and conventions. Conduct thorough testing for both Azure AD and existing strategies.\n\n5. **Documentation**: Update documentation to include instructions for configuring Azure AD and using multiple passport strategies.\n\nEngage with the community to gauge interest and feedback on the feature's scope before proceeding with the implementation.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/61",
@@ -9329,16 +9656,13 @@
"comments_count": 14,
"closed_at": null,
"created_at": "2021-04-23T16:55:51Z",
- "updated_at": "2024-03-23T18:47:48Z",
+ "updated_at": "2024-09-12T08:01:18Z",
"author": 136,
"repository": 502,
- "assignees": [
- 137
- ],
+ "assignees": [],
"labels": [
71,
- 74,
- 75
+ 74
]
}
},
@@ -9351,6 +9675,7 @@
"node_id": "MDU6SXNzdWU4NzA5MDAxMjE=",
"title": "provide API for CI/CD Pipelines",
"body": "**Describe what problem your feature request solves**\r\nProvide an API for CI/CD pipelines\r\n\r\n**Describe the solution you'd like**\r\nProvide an API for CI/CD pipelines, [see here](https://github.com/bbachi/vuejs-nodejs-example/tree/master/api) for an example\r\n\r\n**Additional context**\r\n- What functions are exposed for this API?\r\n- How do we handle authentication/authorization?\r\n- What do we use to document the API? (main github docs, auto-generated via swagger or apidoc?)\r\n",
+ "summary": "Implement an API for CI/CD pipelines. \n\n1. Define the problem of lacking an API for seamless CI/CD integration.\n2. Reference the provided example API [here](https://github.com/bbachi/vuejs-nodejs-example/tree/master/api) for guidance on structure and functionality.\n3. Identify the essential functions to expose through the API, such as triggering builds, retrieving build statuses, and managing pipeline configurations.\n4. Determine the approach for handling authentication and authorization—consider OAuth2 or API tokens for secure access.\n5. Decide on documentation methods. Evaluate options like using the main GitHub documentation, or auto-generating documentation with tools like Swagger or Apidoc.\n\nFirst steps:\n- Conduct a requirements analysis to finalize the API endpoints.\n- Set up a project structure and choose a technology stack (e.g., Node.js, Express).\n- Implement basic authentication and authorization mechanisms.\n- Begin developing the API endpoints, starting with the most critical functionalities.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/88",
@@ -9366,8 +9691,8 @@
"repository": 502,
"assignees": [],
"labels": [
- 71,
- 76
+ 76,
+ 71
]
}
},
@@ -9380,6 +9705,7 @@
"node_id": "MDU6SXNzdWU5Mjc4NDAyMTg=",
"title": "Text Search",
"body": "**Describe what problem your feature request solves**\r\nAbility to search for elements based on user-defined text (element type, description, etc)\r\n\r\n**Describe the solution you'd like**\r\nWhen viewing a diagram, I'd like a search option to be able to find only UI elements that include the given search term. \r\n\r\n**Additional context**\r\nTo start with, this can be a simple text search that searches all element types and all fields but expanded on later for more \"advanced\" search features (only certain types, fields, etc).\r\n\r\nThis improvement was suggested by Simon S \r\n\r\nIt would be good to be able to search for any text within the model\r\nNote that the search box has been temporarily removed via merge #502 \r\n",
+ "summary": "Implement a text search feature for diagrams to enable users to locate UI elements based on user-defined text criteria such as element type and description. \n\n1. Create a search input field within the diagram view.\n2. Implement a basic search function that scans all element types and fields for the specified search term.\n3. Ensure the search results highlight or filter the UI elements that match the search criteria.\n4. Consider future enhancements, such as limiting searches to specific element types or fields for a more refined search experience.\n\nNote that the search box is currently absent due to merge #502, so address any compatibility issues during implementation.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/194",
@@ -9395,8 +9721,8 @@
"repository": 502,
"assignees": [],
"labels": [
- 71,
- 75
+ 75,
+ 71
]
}
},
@@ -9409,6 +9735,7 @@
"node_id": "I_kwDOEAWEP85DBN_r",
"title": "MITRE Att&ck integration with STRIDE threats",
"body": "Describe what problem your feature request solves:\r\nCurrently the tool does a good job for decomposing the architecture and applying threats according to the STRIDE framework. This still leaves some gaps to determine the cybersecurity requirements that the process needs to adhere to remediate identified threats.\r\n\r\nDescribe the solution you'd like:\r\nThe solution I am proposing is that we can create an additional layer of taxonomy of threats under STRIDE to identify the attackers tactics and techniques which might be used to identify the right defenses. These can become the cybersecurity requirements for the process that we are threat modelling.\r\n\r\nThis combined process for threat modeling can be like:\r\nThe first step is to identify the process, map out the dataflows and interactions between them and the trust boundaries.[Threat dragon is capable of this]\r\n\r\nSecond, for each of the subsystems, enumerate a STRIDE matrix listing the mnemonics. \r\nThird, the 12 ATT&CK tactics are tallied. Enumerated tactics are:\r\n• Initial Access\r\n• Execution\r\n• Persistence\r\n• Privilege Escalation\r\n• Defense Evasion\r\n• Credential Access\r\n• Discovery\r\n• Lateral Movement\r\n• Collection\r\n• Command and Control\r\n• Exfiltration\r\n• Impact\r\n\r\nIn Step 4, for each of the tactics within each of the STRIDE mnemonics, the applicable techniques are evaluated. For instance, for the STRIDE mnemonic of spoofing, the 12 tactics are evaluated for ATT&CK threat techniques that could result in spoofing against authenticity. In other words, Steps 2 through 4 are a process of elimination. \r\n\r\nAdvantages:\r\n1. Using consistent semantics and vocabulary to communicate threats.\r\n2. Understanding the adversary tactics which helps visualize the defenses to those threats.\r\n3. Use open source framework created by MITRE to educate the development teams for various threats and associated remediations.\r\n4. Identify cybersecurity requirements that help defend against multiple threats. [e.g] Preventing Initial access can remediate other threats that are not directly related. \r\n\r\n\r\nReferences:\r\nhttps://blog.isc2.org/isc2_blog/2020/02/under-attack-how-mitres-methodology-to-find-threats-and-embed-counter-measures-might-work-in-your-or.html\r\n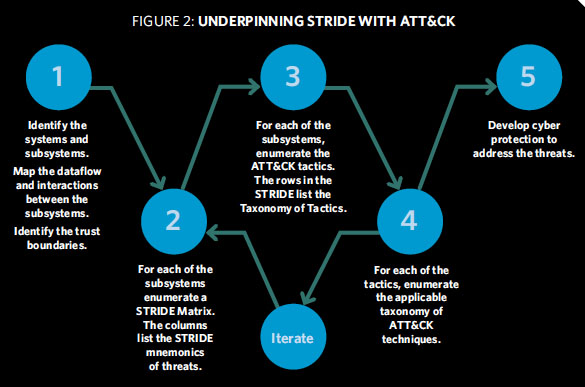\r\n\r\n\r\n",
+ "summary": "Integrate MITRE ATT&CK with STRIDE threats to enhance threat modeling capabilities. Address the gaps in identifying cybersecurity requirements for remediating threats by proposing an additional taxonomy layer under STRIDE.\n\n1. Identify the process and map dataflows and interactions, including trust boundaries. Utilize Threat Dragon for this task.\n2. Enumerate a STRIDE matrix for each subsystem, listing relevant mnemonics.\n3. Tally the 12 ATT&CK tactics: Initial Access, Execution, Persistence, Privilege Escalation, Defense Evasion, Credential Access, Discovery, Lateral Movement, Collection, Command and Control, Exfiltration, and Impact.\n4. Evaluate applicable techniques for each tactic within the STRIDE mnemonics, focusing on techniques that could lead to specific threats, such as spoofing.\n\nAdvantages include:\n- Establishing a consistent vocabulary for discussing threats.\n- Enhancing understanding of adversary tactics and aligning defenses.\n- Utilizing the MITRE framework to educate development teams on threats and remediations.\n- Identifying cybersecurity requirements that provide broader defense mechanisms.\n\nStart by mapping current processes and identifying areas where the integration can be implemented effectively.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/361",
@@ -9424,8 +9751,8 @@
"repository": 502,
"assignees": [],
"labels": [
- 71,
- 77
+ 77,
+ 71
]
}
},
@@ -9438,6 +9765,7 @@
"node_id": "I_kwDOEAWEP85DVzt0",
"title": "desktop command line interface",
"body": "**Describe what problem your feature request solves**\r\nVersion 1.x of Threat Dragon has a CLI - this needs to be migrated to version 2.0\r\n\r\n**Describe the solution you'd like**\r\nImplement CLI for version 2.0 using version 1.x as an example\r\n\r\n**Additional context**\r\nVersion 1.x used the [yargs package](https://www.npmjs.com/package/yargs) to supply the CLI\r\n",
+ "summary": "Migrate the Threat Dragon CLI from version 1.x to version 2.0. Utilize the existing CLI implementation in version 1.x as a reference for development.\n\n1. Review the CLI structure and commands in Threat Dragon version 1.x.\n2. Install the `yargs` package in version 2.0 to manage command-line arguments.\n3. Define the command structure and options for version 2.0, ensuring compatibility with new features.\n4. Implement the CLI commands, leveraging the existing logic from version 1.x where applicable.\n5. Test the new CLI implementation thoroughly to ensure all commands function as intended.\n\nConsider potential changes in the underlying architecture of version 2.0 that may affect the CLI functionality and address them during implementation.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/372",
@@ -9453,10 +9781,10 @@
"repository": 502,
"assignees": [],
"labels": [
- 71,
- 78,
+ 80,
79,
- 80
+ 78,
+ 71
]
}
},
@@ -9469,6 +9797,7 @@
"node_id": "I_kwDOEAWEP85DzfMt",
"title": "Resize Diagram or Export to PNG",
"body": "**Describe what problem your feature request solves**\r\nI don't currently have a way to share a large threat model with non-git stakeholders.\r\n\r\n**Describe the solution you'd like**\r\nAs a Cloud Security Engineer, I would like the ability to export a threat model outside of threat dragon so that I can share a diagram with stakeholders that are not currently using threat dragon.\r\n\r\n**Additional context**\r\nMVP - Desktop version could use a resize function \r\nLong term - Exports options for different formats\r\n",
+ "summary": "Implement a feature to resize diagrams or export them as PNG files in Threat Dragon. This addresses the need for Cloud Security Engineers to share large threat models with non-Git stakeholders effectively.\n\n1. Develop a resizing function for diagrams in the desktop version of Threat Dragon, allowing users to adjust dimensions for better visibility and usability.\n2. Create an export feature that enables saving diagrams in PNG format, ensuring high-quality sharing options for stakeholders.\n3. Consider long-term enhancements by exploring additional export options for various formats (e.g., PDF, SVG) to cater to different user needs.\n\nBegin by reviewing the existing diagram rendering codebase to identify how resizing can be implemented. Create a prototype for the resizing functionality, then integrate the export feature for PNG format, ensuring compatibility with current diagram structures. Test the implementation thoroughly before deploying.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/385",
@@ -9484,8 +9813,8 @@
"repository": 502,
"assignees": [],
"labels": [
- 71,
- 77
+ 77,
+ 71
]
}
},
@@ -9494,10 +9823,11 @@
"pk": 333,
"fields": {
"nest_created_at": "2024-09-11T20:08:19.449Z",
- "nest_updated_at": "2024-09-11T20:08:19.450Z",
+ "nest_updated_at": "2024-09-13T15:17:01.472Z",
"node_id": "I_kwDOEAWEP85Hd-OR",
"title": "provide dark mode for desktop",
"body": "**Describe what problem your feature request solves**\r\nIt would be good to allow the use of Dark Mode in the desktop. This is already provided in the web application by the browser\r\n\r\n**Describe the solution you'd like**\r\nFollow this tutorial : https://www.electronjs.org/docs/latest/tutorial/dark-mode\r\n\r\n**Additional context**\r\n",
+ "summary": "Implement dark mode for the desktop application. This feature will enhance user experience by providing a visually comfortable interface, similar to what is already available in the web application.\n\n1. Reference the Electron documentation on dark mode: https://www.electronjs.org/docs/latest/tutorial/dark-mode.\n2. Investigate how to integrate dark mode support into the existing desktop application.\n3. Update the application settings to allow users to toggle dark mode on and off.\n4. Test the implementation across different operating systems to ensure compatibility.\n5. Gather user feedback to refine and improve the dark mode feature.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/404",
@@ -9508,12 +9838,10 @@
"comments_count": 4,
"closed_at": null,
"created_at": "2022-04-10T13:44:39Z",
- "updated_at": "2024-07-08T17:01:39Z",
+ "updated_at": "2024-09-12T08:02:59Z",
"author": 43,
"repository": 502,
- "assignees": [
- 130
- ],
+ "assignees": [],
"labels": [
71,
78
@@ -9529,6 +9857,7 @@
"node_id": "I_kwDOEAWEP85H7-Or",
"title": "desktop about-box is MacOS only",
"body": "**Describe what problem your feature request solves**\r\nThe desktop drop down menu role: 'about' is probably MacOS only\r\n\r\n**Describe the solution you'd like**\r\nAdd in code like this, to make it cross platform:\r\n```\r\n {\r\n label: 'about Electron',\r\n // Set menu roles\r\n role: 'about', // about, this value is only for Mac OS X systems\r\n // Click event is invalid when the role attribute of click event can be recognized\r\n click: () => {\r\n var aboutWin = new BrowserWindow({ width: 300, height: 200, parent: win, modal: true });\r\n aboutWin.loadFile('about.html');\r\n }\r\n },\r\n```\r\n\r\n**Additional context**\r\n",
+ "summary": "Implement cross-platform support for the desktop drop-down menu role: 'about'. Currently, this feature is exclusive to MacOS.\n\n**Proposed Solution:**\nIntegrate the following code snippet to ensure compatibility across different operating systems:\n\n```javascript\n{\n label: 'about Electron',\n role: 'about', // Note: This role is only recognized on MacOS\n click: () => {\n var aboutWin = new BrowserWindow({ width: 300, height: 200, parent: win, modal: true });\n aboutWin.loadFile('about.html');\n }\n},\n```\n\n**First Steps to Tackle the Problem:**\n1. Review the current implementation of the 'about' role in the Electron desktop application.\n2. Modify the menu creation logic to include a conditional check for the operating system.\n3. Create an `about.html` file if it doesn't exist, ensuring it contains relevant information about the application.\n4. Test the implementation on both MacOS and other operating systems to confirm functionality.\n5. Document the changes in the project’s README or relevant documentation to guide future contributions.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/421",
@@ -9544,10 +9873,10 @@
"repository": 502,
"assignees": [],
"labels": [
- 71,
- 78,
81,
- 82
+ 82,
+ 78,
+ 71
]
}
},
@@ -9560,6 +9889,7 @@
"node_id": "I_kwDOEAWEP85JpJwG",
"title": "Support storage backends other than github",
"body": "**Describe what problem your feature request solves**\r\nInstead of using github repositories for storage of threat models, I'd like to store them all in an alternative centralized storage mechanism (I have a strong affinity to AWS, so s3 would be my preference)\r\n\r\n**Describe the solution you'd like**\r\nIntroduce a storage mechanism configuration and support options like which cloud provider (aws, azure, google) and any relevant configuration options for each one (for AWS, this would probably just be bucket name and region). Of course, this introduces a new dependency on IAM credentials for the service, which I'd say is an exercise left to the reader (I'd personally launch threat-dragon as a containerized workload in AWS and attach an IAM role, but others assumedly would want to set AWS_ACCESS_KEY_ID etc.)\r\n",
+ "summary": "Implement support for alternative storage backends beyond GitHub for threat models. Focus on integrating centralized storage solutions, specifically targeting AWS S3 as a primary option.\n\n1. **Identify Requirements**: Define the necessary configuration options for various cloud providers, including AWS, Azure, and Google Cloud. For AWS, specify parameters like bucket name and region.\n\n2. **Develop Configuration Interface**: Create a configuration interface that allows users to select their preferred cloud provider and input required settings. Ensure this interface can handle varying configurations based on the selected provider.\n\n3. **Manage IAM Credentials**: Address the dependency on IAM credentials. Offer guidance on best practices for credential management, such as using IAM roles for containerized deployments on AWS or environment variables for local setups.\n\n4. **Implement Storage Logic**: Develop the backend logic to interact with the selected cloud storage solution. This includes creating, reading, updating, and deleting threat models stored in S3 or other supported providers.\n\n5. **Testing and Validation**: Test the new storage backends thoroughly to ensure reliability and performance. Validate that the configurations work seamlessly across different cloud environments.\n\n6. **Documentation**: Update documentation to include instructions on how to configure and use the new storage options, including examples for each supported provider.\n\nBegin by reviewing the existing storage implementation, then outline the architectural changes needed to support multiple cloud providers.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/426",
@@ -9577,9 +9907,9 @@
141
],
"labels": [
- 71,
74,
- 75
+ 75,
+ 71
]
}
},
@@ -9588,10 +9918,11 @@
"pk": 336,
"fields": {
"nest_created_at": "2024-09-11T20:08:26.685Z",
- "nest_updated_at": "2024-09-11T20:08:26.685Z",
+ "nest_updated_at": "2024-09-13T03:53:41.018Z",
"node_id": "I_kwDOEAWEP85NKG0E",
"title": "Replace veux with pinia",
"body": "**Describe what problem your feature request solves**\r\n[Vuex](https://vuex.vuejs.org/) is now in maintenance mode. It still works, but will no longer receive new features. It is recommended to use Pinia for new applications.\r\n\r\n**Describe the solution you'd like**\r\nReplace Veux with Pinia\r\n\r\n**Additional context**\r\nhttps://vuejs.org/guide/scaling-up/state-management.html#pinia\r\n",
+ "summary": "Replace Veux with Pinia to modernize state management in the application. Vuex is currently in maintenance mode and will not receive new features, making it imperative to transition to Pinia for ongoing support and enhancements.\n\n**First Steps:**\n1. Install Pinia via npm:\n ```bash\n npm install pinia\n ```\n2. Set up Pinia in your main application file, typically `main.js` or `main.ts`:\n ```javascript\n import { createPinia } from 'pinia';\n import { createApp } from 'vue';\n\n const app = createApp(App);\n const pinia = createPinia();\n app.use(pinia);\n app.mount('#app');\n ```\n3. Migrate existing Vuex store modules to Pinia stores. For each Vuex module, create a corresponding Pinia store using the `defineStore` function:\n ```javascript\n import { defineStore } from 'pinia';\n\n export const useMyStore = defineStore('myStore', {\n state: () => ({\n // state properties\n }),\n actions: {\n // action methods\n },\n getters: {\n // getter methods\n },\n });\n ```\n4. Replace Vuex store calls with Pinia store calls in your components. Update state access, actions, and getters accordingly.\n\n5. Test the application thoroughly to ensure that the migration did not introduce any regressions.\n\nBy following these steps, transition to Pinia will enhance performance and future-proof the application's state management.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/442",
@@ -9602,15 +9933,12 @@
"comments_count": 2,
"closed_at": null,
"created_at": "2022-07-05T16:06:24Z",
- "updated_at": "2024-08-23T09:49:54Z",
+ "updated_at": "2024-09-12T08:01:48Z",
"author": 43,
"repository": 502,
- "assignees": [
- 142
- ],
+ "assignees": [],
"labels": [
- 71,
- 73
+ 71
]
}
},
@@ -9619,10 +9947,11 @@
"pk": 337,
"fields": {
"nest_created_at": "2024-09-11T20:08:28.727Z",
- "nest_updated_at": "2024-09-11T20:08:28.727Z",
+ "nest_updated_at": "2024-09-13T03:53:42.053Z",
"node_id": "I_kwDOEAWEP85NKI8Q",
"title": "Replace Vue CLI with Vite",
"body": "**Describe what problem your feature request solves**\r\n[Vue CLI](https://cli.vuejs.org/) is the official webpack-based toolchain for Vue. It is now in maintenance mode and ideally should be replaced with [Vite](https://vitejs.dev/) which will provide superior developer experience in most cases.\r\n\r\n**Describe the solution you'd like**\r\nReplace Vue CLI with Vite\r\n\r\n**Additional context**\r\nhttps://vuejs.org/guide/scaling-up/tooling.html#vue-cli\r\n",
+ "summary": "Replace Vue CLI with Vite to enhance the developer experience and leverage Vite's modern features. \n\n1. Assess the current project setup using Vue CLI, including configuration files, scripts, and dependencies.\n2. Install Vite by running `npm install vite --save-dev`.\n3. Update project structure to align with Vite's requirements. Create a `vite.config.js` file tailored for the project setup.\n4. Migrate existing Vue CLI configurations and plugins to Vite equivalents. This may involve replacing Webpack-specific code with Vite-compatible configurations.\n5. Adjust npm scripts in `package.json` to use Vite commands, replacing any Vue CLI commands.\n6. Test the application thoroughly to ensure all functionalities work post-migration.\n7. Document the migration process and update any relevant project documentation. \n\nRefer to the [Vite documentation](https://vitejs.dev/) for detailed guidance on configuration and setup.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/443",
@@ -9633,15 +9962,12 @@
"comments_count": 0,
"closed_at": null,
"created_at": "2022-07-05T16:15:04Z",
- "updated_at": "2024-08-23T09:49:54Z",
+ "updated_at": "2024-09-12T07:59:47Z",
"author": 43,
"repository": 502,
- "assignees": [
- 142
- ],
+ "assignees": [],
"labels": [
- 71,
- 73
+ 71
]
}
},
@@ -9650,10 +9976,11 @@
"pk": 338,
"fields": {
"nest_created_at": "2024-09-11T20:08:30.910Z",
- "nest_updated_at": "2024-09-11T20:08:30.910Z",
+ "nest_updated_at": "2024-09-13T03:53:43.063Z",
"node_id": "I_kwDOEAWEP85NKNAl",
"title": "Migrate to Vue 3",
- "body": "**Describe what problem your feature request solves**\r\nVue 3 is the current, latest major version of Vue. It contains new features that are not present in Vue 2 (most notably Composition API), and also contains breaking changes that makes it incompatible with Vue 2.\r\nIn general, Vue 3 provides smaller bundle sizes, better performance, better scalability, and better TypeScript / IDE support. If starting a new project today, Vue 3 is the recommended choice.\r\n\r\n**Describe the solution you'd like**\r\n[Migrate to Vue 3](https://v3-migration.vuejs.org/)\r\nAlso make sure that vue-router is also updated (to something like ^4.1.0)\r\n\r\n**Additional context**\r\nhttps://vuejs.org/about/faq.html#what-s-the-difference-between-vue-2-and-vue-3\r\nPresent version of Vue is 2.6.11\r\n\r\nI18N will need to change: use the Vue I18n v9 [documentation](https://vue-i18n.intlify.dev/) instead.\r\n",
+ "body": "**Describe what problem your feature request solves**\r\nVue 3 is the current, latest major version of Vue. It contains new features that are not present in Vue 2 (most notably Composition API), and also contains breaking changes that makes it incompatible with Vue 2.\r\nIn general, Vue 3 provides smaller bundle sizes, better performance, better scalability, and better TypeScript / IDE support. If starting a new project today, Vue 3 is the recommended choice.\r\n\r\n**Describe the solution you'd like**\r\n[Migrate to Vue 3](https://v3-migration.vuejs.org/)\r\nAlso make sure that vue-router is also updated (to something like ^4.1.0)\r\n\r\n**Additional context**\r\nhttps://vuejs.org/about/faq.html#what-s-the-difference-between-vue-2-and-vue-3\r\nPresent version of Vue is 2.6.11\r\n\r\npackage `vue-template-compiler` will need to be updated\r\n\r\nI18N will need to change: use the Vue I18n v9 [documentation](https://vue-i18n.intlify.dev/) instead.\r\n",
+ "summary": "**Migrate to Vue 3**\n\n1. Upgrade the project to Vue 3 from the current version 2.6.11.\n2. Implement the Composition API for better scalability and performance.\n3. Update `vue-router` to version ^4.1.0 to ensure compatibility with Vue 3.\n4. Replace `vue-template-compiler` with the appropriate version that supports Vue 3.\n5. Transition I18N setup to use Vue I18n v9 as per the updated documentation.\n\n**First Steps:**\n\n- Review the [Vue 3 Migration Guide](https://v3-migration.vuejs.org/) to understand breaking changes and new features.\n- Assess current project structure and identify components that need refactoring to utilize the Composition API.\n- Update dependencies in `package.json`, specifically `vue`, `vue-router`, and `vue-template-compiler`.\n- Test the application extensively to identify any issues arising from the migration.\n- Update I18N implementation according to Vue I18n v9 documentation.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/444",
@@ -9664,12 +9991,10 @@
"comments_count": 1,
"closed_at": null,
"created_at": "2022-07-05T16:32:39Z",
- "updated_at": "2024-08-23T09:49:54Z",
+ "updated_at": "2024-09-12T07:50:54Z",
"author": 43,
"repository": 502,
- "assignees": [
- 142
- ],
+ "assignees": [],
"labels": [
71,
73
@@ -9685,6 +10010,7 @@
"node_id": "I_kwDOEAWEP85NhyZK",
"title": "Provide text Search and Replace",
"body": "**Describe what problem your feature request solves**\r\nSometimes there is a lot of renaming to be done on a threat project, for example if the namespace changes\r\n\r\n**Describe the solution you'd like**\r\nProvide text 'Search and Replace' functionality to the Text Search feature\r\n\r\n**Additional context**\r\nUser feedback \"_a mass replace functionality in addition to search. Because renamings happen_\"\r\n",
+ "summary": "Implement a 'Search and Replace' feature for text in the project.\n\n**Problem:** Address the need for mass renaming, particularly when namespaces change, by facilitating an efficient way to perform bulk edits across the codebase.\n\n**Solution:** Introduce a 'Search and Replace' functionality that enhances the existing text search feature. This should allow users to specify a search term and provide a replacement term, applying changes across the entire project or within selected files.\n\n**Technical Details:**\n- Extend the current search functionality to include a replace option.\n- Ensure the feature supports regular expressions for flexible search patterns.\n- Implement safeguards to prevent unintended replacements, such as a preview of changes before committing.\n- Consider integrating version control features to allow users to revert changes easily.\n\n**First Steps:**\n1. Analyze the current text search implementation to determine how to best integrate the search and replace functionality.\n2. Define the user interface changes needed for the 'Replace' option.\n3. Develop the core logic for replacing text, including handling edge cases like overlapping matches.\n4. Create unit tests to validate the search and replace functionality.\n5. Gather user feedback on the implementation to refine the feature further.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/454",
@@ -9713,6 +10039,7 @@
"node_id": "I_kwDOEAWEP85NiD1X",
"title": "View multiple diagrams",
"body": "**Describe what problem your feature request solves**\r\nSome projects have more than one diagram, but only one can be viewed at any one time\r\n\r\n**Describe the solution you'd like**\r\nprovide ability to view multiple diagrams at the same time\r\n\r\n**Additional context**\r\nUser request \"_Can I open multiple diagrams in their own windows so that I can see them side by side? We have up to 4 diagrams_\"\r\n",
+ "summary": "Implement functionality to view multiple diagrams simultaneously. Address the limitation where only one diagram is viewable at a time, which hinders users managing projects with multiple diagrams.\n\n1. **Analyze Current Diagram Rendering**: Review the existing code for how diagrams are rendered and displayed. Identify the rendering engine or component responsible for displaying a single diagram.\n\n2. **Design Multi-Window Interface**: Create a user interface that allows users to open multiple windows or tabs for diagrams. Ensure that the interface maintains usability and clarity.\n\n3. **Modify State Management**: Adjust the state management to handle multiple diagram instances. This includes ensuring that each diagram can be independently manipulated without affecting others.\n\n4. **Implement Window Management**: Develop the logic for opening, closing, and arranging multiple windows. Consider using a library or framework that facilitates window management if needed.\n\n5. **Test Feature**: Conduct thorough testing with various numbers of diagrams (up to 4 as mentioned) to ensure stability and performance.\n\n6. **Gather User Feedback**: After implementation, collect feedback from users to refine the feature further.",
"state": "open",
"state_reason": "reopened",
"url": "https://github.com/OWASP/threat-dragon/issues/458",
@@ -9728,8 +10055,8 @@
"repository": 502,
"assignees": [],
"labels": [
- 71,
- 77
+ 77,
+ 71
]
}
},
@@ -9742,6 +10069,7 @@
"node_id": "I_kwDOEAWEP85NiFMr",
"title": "add moveable labels for data flows and trust boundaries",
"body": "**Describe what problem your feature request solves**\r\nThe labels for data flows and trust boundaries can not be moved, and may conflict on the diagram\r\n\r\n**Describe the solution you'd like**\r\nAbility to move the data flow and trust boundary labels, as they may coincide\r\n\r\n**Additional context**\r\nUser feedback \"_Labels for flows and boundaries are not movable, for example it can be not clear whether the label applies to a dataflow or a boundary_\"\r\n",
+ "summary": "Implement moveable labels for data flows and trust boundaries to enhance diagram clarity. \n\n**Problem to solve:**\nCurrent labels for data flows and trust boundaries are static, leading to potential overlap and confusion in diagrams.\n\n**Proposed solution:**\n1. Develop functionality to enable users to click and drag labels for data flows and trust boundaries to desired positions.\n2. Ensure that labels can be clearly associated with their respective elements to avoid ambiguity.\n\n**Technical details:**\n- Modify the diagram rendering logic to include a draggable property for labels.\n- Update the event handling to capture mouse events for label movement.\n- Implement collision detection to prevent labels from overlapping with other elements unless explicitly allowed.\n- Maintain the current association of labels to their respective data flows or trust boundaries during movement.\n\n**First steps:**\n1. Review the current implementation of the label rendering system.\n2. Identify the event listeners associated with label interactions.\n3. Begin prototyping the drag-and-drop functionality for labels.\n4. Test the movement feature for usability and clarity in various diagram scenarios.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/459",
@@ -9757,8 +10085,8 @@
"repository": 502,
"assignees": [],
"labels": [
- 71,
- 83
+ 83,
+ 71
]
}
},
@@ -9771,6 +10099,7 @@
"node_id": "I_kwDOEAWEP85NiNTX",
"title": "PDF report sections and ToC",
"body": "**Describe what problem your feature request solves**\r\nIt can be difficult to find the diagrams in reports with multiple diagrams\r\n\r\n**Describe the solution you'd like**\r\nProvide sections and a Table of Contents for the diagrams in the PDF report\r\n\r\n**Additional context**\r\nUser feedback \"_Would be great to have PDF create sections for each diagram. Our models use multiple diagrams (up to 4) and it takes a while to manually find diagrams in a PDF report_\"\r\n",
+ "summary": "Implement a feature to add sections and a Table of Contents (ToC) for diagrams in PDF reports. This will enhance navigation in reports containing multiple diagrams, making it easier for users to locate specific diagrams quickly.\n\n**Technical Steps to Address This:**\n1. **Analyze Current PDF Generation Process**: Review the existing functionality used to generate PDF reports and how diagrams are currently included.\n2. **Define Data Structure**: Create a structure to store metadata for each diagram, including titles and page numbers, which will be used to generate the ToC.\n3. **Modify PDF Layout**: Update the PDF layout code to include sections for each diagram, ensuring each section is clearly labeled.\n4. **Generate Table of Contents**: Implement a method to automatically compile the ToC from the defined metadata, listing all diagrams with corresponding links to their sections.\n5. **Testing**: Conduct thorough testing with reports containing varying numbers of diagrams to ensure the ToC and sections function correctly and improve user navigation.\n6. **Gather User Feedback**: After implementation, solicit feedback from users to refine and enhance the feature.\n\nBy following these steps, improve the usability of PDF reports containing multiple diagrams and streamline the user experience.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/461",
@@ -9786,9 +10115,9 @@
"repository": 502,
"assignees": [],
"labels": [
- 71,
73,
- 84
+ 84,
+ 71
]
}
},
@@ -9801,6 +10130,7 @@
"node_id": "I_kwDOEAWEP85NiQpG",
"title": "Warn when dataflows dangle",
"body": "**Describe what problem your feature request solves**\r\nA dataflow needs to be connected at both ends, otherwise it does not make sense\r\n\r\n**Describe the solution you'd like**\r\nThe dataflow colour or shape should signify that the dataflow is not connected at both ends\r\n\r\n**Additional context**\r\nUser request \"_There should be visual identification for dataflows that are not connected to objects on one or more sides (MS TMT used to list them)_\"\r\n",
+ "summary": "Implement a warning system for dangling dataflows. Ensure that each dataflow is visually identifiable when it is not connected at both ends. Modify the color or shape of the dataflow to indicate its unconnected status. \n\n### Technical Details:\n1. **Identify Dataflow Status**: Create a function that checks the connection status of each dataflow. This function should verify if both ends are linked to objects.\n2. **Define Visual Indicators**: Decide on specific colors or shapes to represent unconnected dataflows. For instance, use red color or dashed lines for unconnected flows.\n3. **Integrate into UI**: Modify the user interface to apply the visual changes dynamically as dataflows are created or modified.\n4. **User Feedback**: Implement a tooltip or message that provides additional information when a user hovers over a dangling dataflow.\n\n### First Steps:\n1. Review the existing dataflow structure and connection logic.\n2. Develop the function to check for connection status.\n3. Prototype the visual changes and gather user feedback.\n4. Test the implementation across various scenarios to ensure reliability.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/463",
@@ -9829,6 +10159,7 @@
"node_id": "I_kwDOEAWEP85Pic6-",
"title": "Provide timeouts / reporting for login failures",
"body": "**Describe what problem your feature request solves**\r\nIf there is a problem logging in, specifically the 'Login with Github', then either a blank dashboard page is presented to the user, or a constantly spinning wait\r\n\r\n**Describe the solution you'd like**\r\nIf the login fails, present the user with an error message and return to the homepage\r\n\r\n**Additional context**\r\nWhen canceling login to github:\r\n\r\n\r\n\r\nLog of cancelled login to Github:\r\n\r\n```\r\ndebug: controllers/auth.js: API login request: [object Object] {\"service\":\"threat-dragon\",\"timestamp\":\"2022-08-10 11:10:02\"}\r\ndebug: controllers/auth.js: API oauthReturn request: [object Object] {\"service\":\"threat-dragon\",\"timestamp\":\"2022-08-10 11:10:04\"}\r\ndebug: controllers/auth.js: API completeLogin request: [object Object] {\"service\":\"threat-dragon\",\"timestamp\":\"2022-08-10 11:10:04\"}\r\nerror: controllers/auth.js: {\"service\":\"threat-dragon\",\"timestamp\":\"2022-08-10 11:10:05\"}\r\nerror: Requires authentication {\"body\":{\"documentation_url\":\"https://docs.github.com/rest/reference/users#get-the-authenticated-user\",\"message\":\"Requires authentication\"},\"headers\":{\"access-control-allow-origin\":\"*\",\"access-control-expose-headers\":\"ETag, Link, Location, Retry-After, X-GitHub-OTP, X-RateLimit-Limit, X-RateLimit-Remaining, X-RateLimit-Used, X-RateLimit-Resource, X-RateLimit-Reset, X-OAuth-Scopes, X-Accepted-OAuth-Scopes, X-Poll-Interval, X-GitHub-Media-Type, X-GitHub-SSO, X-GitHub-Request-Id, Deprecation, Sunset\",\"connection\":\"close\",\"content-length\":\"131\",\"content-security-policy\":\"default-src 'none'\",\"content-type\":\"application/json; charset=utf-8\",\"date\":\"Wed, 10 Aug 2022 10:10:05 GMT\",\"referrer-policy\":\"origin-when-cross-origin, strict-origin-when-cross-origin\",\"server\":\"GitHub.com\",\"strict-transport-security\":\"max-age=31536000; includeSubdomains; preload\",\"vary\":\"Accept-Encoding, Accept, X-Requested-With\",\"x-content-type-options\":\"nosniff\",\"x-frame-options\":\"deny\",\"x-github-media-type\":\"github.v3; format=json\",\"x-github-request-id\":\"DCD8:9FDC:C3D6C6:CE492A:62F383FD\",\"x-ratelimit-limit\":\"60\",\"x-ratelimit-remaining\":\"58\",\"x-ratelimit-reset\":\"1660129699\",\"x-ratelimit-resource\":\"core\",\"x-ratelimit-used\":\"2\",\"x-xss-protection\":\"0\"},\"service\":\"threat-dragon\",\"statusCode\":401,\"timestamp\":\"2022-08-10 11:10:05\"}\r\nerror: undefined {\"service\":\"threat-dragon\",\"timestamp\":\"2022-08-10 11:10:05\"}\r\ndebug: controllers/auth.js: Returning error to client: {\"status\":500,\"message\":\"Internal Server Error\",\"details\":\"Internal Server Error\"} {\"service\":\"threat-dragon\",\"timestamp\":\"2022-08-10 11:10:05\"}\r\n```\r\nWhen protocol mismatch occurs (HTTPS certs not valid):\r\n\r\n\r\n",
+ "summary": "Implement timeouts and error reporting for login failures.\n\n1. Identify the issue: Users encounter a blank dashboard or a spinning wait icon when the \"Login with GitHub\" feature fails.\n\n2. Propose a solution: Upon a login failure, display an error message to the user and redirect them back to the homepage.\n\n3. Review existing error logs for understanding:\n - Analyze the log entries related to login requests to identify failure points. For instance, check for HTTP status codes like 401 (authentication required) or 500 (internal server error) as shown in the example logs.\n\n4. Design the error handling process:\n - Create a timeout mechanism for the login process. If the login attempt exceeds a specified duration without a response, trigger an error state.\n - Implement a user-friendly error message that specifies the nature of the failure (e.g., \"Login Failed: Please check your credentials or try again later.\").\n\n5. Redirect users: Ensure that after displaying the error message, the application navigates users back to the homepage to provide a seamless experience.\n\n6. First steps to tackle the problem:\n - Review the authentication controller code to locate where the login requests are processed.\n - Implement a timeout function that cancels the login attempt if it takes too long.\n - Add error handling logic to manage different types of errors (e.g., authentication errors, protocol mismatches).\n - Test the implementation thoroughly to ensure that users receive appropriate feedback on login failures.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/481",
@@ -9857,6 +10188,7 @@
"node_id": "I_kwDOEAWEP85QK_81",
"title": "console.log redirected to log file",
"body": "**Describe what problem your feature request solves**\r\nWe have some places where the console is used to log messages, which do not appear in the log file\r\n\r\n**Describe the solution you'd like**\r\nIt would be good to make sure that all console.log messages also appear in the log file. This has been done for electron logs, so would be good for server and vue logs\r\n\r\n**Additional context**\r\nAt the same time ensure that no tokens or PII is being logged\r\n",
+ "summary": "Implement a solution to redirect `console.log` messages to a log file. Ensure all logging mechanisms, including those used in server and Vue environments, capture console outputs.\n\n1. **Investigate Existing Logging Mechanisms**: Review how logging is currently implemented for Electron to identify potential methods for redirecting console output to files.\n\n2. **Modify Console Methods**: Override `console.log`, `console.warn`, and other relevant console methods to include file writing functionality. Utilize a logging library or Node.js' `fs` module to append logs to a designated log file.\n\n3. **Implement Filtering for Sensitive Data**: Integrate a mechanism to filter out any tokens or Personally Identifiable Information (PII) from the logs before writing them to the log file. Use regular expressions or a predefined list of sensitive keywords.\n\n4. **Test the Implementation**: Create unit tests to ensure that all console messages are captured in the log file and that no sensitive data is logged.\n\n5. **Document the Changes**: Update project documentation to reflect the new logging behavior, including examples of how messages will appear in the log file and any configurations needed for sensitive data filtering.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/493",
@@ -9872,8 +10204,8 @@
"repository": 502,
"assignees": [],
"labels": [
- 71,
- 85
+ 85,
+ 71
]
}
},
@@ -9886,6 +10218,7 @@
"node_id": "I_kwDOEAWEP85bOYiH",
"title": "Update jekyll versions for docs ",
"body": "**Describe what problem your feature request solves**\r\nThe ruby gem files reference out of date versions\r\n\r\n**Describe the solution you'd like**\r\nupdate docs gem files for up to date versions\r\n\r\n**Additional context**\r\n\r\n",
+ "summary": "Update Jekyll versions for documentation. \n\nIdentify outdated Ruby gem files in the documentation. Replace current references with the latest stable versions of Jekyll and its dependencies. \n\nFirst steps: \n1. Check the current Jekyll version in the Gemfile and Gemfile.lock files.\n2. Visit the official Jekyll repository or RubyGems to find the latest versions.\n3. Update the Gemfile with the new version numbers.\n4. Run `bundle update` to ensure all dependencies are resolved.\n5. Test the documentation site locally to confirm that updates do not introduce any issues. \n6. Commit the changes and submit a pull request for review.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/584",
@@ -9901,9 +10234,9 @@
"repository": 502,
"assignees": [],
"labels": [
- 71,
85,
- 86
+ 86,
+ 71
]
}
},
@@ -9916,6 +10249,7 @@
"node_id": "I_kwDOEAWEP85eq-qF",
"title": "Support for more granular threats using threat trees",
"body": "**Describe what problem your feature request solves**\r\nWhile documenting specific threats, it would be helpful if we could select more granular threats in the Edit Threat modal. For example, LINDDUN and STRIDE both have threat trees corresponding to each threat. The frameworks refer to these trees in their mitigation strategies section, so having this feature would be useful to quickly find the corresponding mitigations. More context is provided below.\r\n\r\n**Describe the solution you'd like**\r\nCurrently we can select the type of threat in the Edit Threat modal. This type corresponds to the root node of a threat tree. Perhaps there could be an additional drop-down menu below this with options for each of the child nodes in the corresponding threat trees?\r\n\r\n**Additional context**\r\nFor example, the Linkability threat in LINDDUN has the threat tree shown below. On selecting Linkability in the Edit Threat modal, another dropdown below could have options corresponding to the child nodes in this tree. \r\n\r\n\r\n\r\nSource: [Linkability Threat Trees](https://www.linddun.org/linkability)\r\n\r\nHaving this information could then allow us to find the corresponding mitigation strategies from a table like this one:\r\n\r\n\r\n\r\nSource: [LINDDUN Mitigation Strategies](https://www.linddun.org/mitigation-strategies-and-solutions)\r\n\r\nThank you!",
+ "summary": "Implement support for granular threats using threat trees in the Edit Threat modal. Enable selection of child nodes corresponding to threat trees like LINDDUN and STRIDE. \n\n1. Modify the Edit Threat modal to include a new dropdown menu beneath the existing threat type selection.\n2. Populate this dropdown with options for each child node from the selected threat tree.\n3. Ensure that selecting a child node allows users to easily reference associated mitigation strategies.\n4. Review the Linkability threat tree from LINDDUN as a primary example for implementation, ensuring the structure aligns with existing frameworks.\n5. Test the feature to confirm that it accurately displays child nodes and links to relevant mitigation strategies.\n\nThese steps will enhance usability and speed up the threat documentation process.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/607",
@@ -9933,8 +10267,8 @@
143
],
"labels": [
- 71,
- 77
+ 77,
+ 71
]
}
},
@@ -9947,6 +10281,7 @@
"node_id": "I_kwDOEAWEP85gb7yz",
"title": "Allow user to select multiple threat types",
"body": "**Describe what problem your feature request solves**\r\nAt the moment when selecting a threat type, I can only select one of the available options. For example, I can indicate a threat covers Confidentiality **or** Integrity **or** Availability, but cannot indicate it affects multiple of them. This creates a problem when discussing threats that legitimately affect multiple areas—for instance, a service crash that creates Availability issues and may disclose information while crashing that threatens Confidentiality.\r\n\r\n**Describe the solution you'd like**\r\nThreat types should be multi-select, perhaps with check-boxes or the Option-key-to-select-multiple solution. This enables users to select one or multiple depending on the context of the threat.",
+ "summary": "Implement multi-select functionality for threat types. \n\n**Problem Addressed**: Currently, users can only select a single threat type (Confidentiality, Integrity, Availability), which limits the ability to accurately represent threats affecting multiple areas, such as a service crash impacting both Availability and Confidentiality.\n\n**Proposed Solution**: Introduce check-boxes or an Option-key mechanism to allow users to select multiple threat types simultaneously. This will improve threat categorization and facilitate better discussions around multi-faceted threats.\n\n**First Steps to Tackle the Problem**:\n1. Analyze the current UI component handling threat type selection.\n2. Modify the selection component to support multiple selections (consider using a library or framework that handles check-boxes).\n3. Update the backend logic to accept and process multiple threat types.\n4. Perform testing to ensure that both selection and data handling work as expected.\n5. Collect user feedback on the new feature for further refinements.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/620",
@@ -9962,9 +10297,9 @@
"repository": 502,
"assignees": [],
"labels": [
- 71,
72,
- 73
+ 73,
+ 71
]
}
},
@@ -9977,6 +10312,7 @@
"node_id": "I_kwDOEAWEP85hGL0h",
"title": "Flatpak/flathub support",
"body": "**Describe what problem your feature request solves**\r\nA [Flatpak](https://flatpak.org/) install would be a great alternative to Snaps and Appimages, since this allows most users to download threat-dragon straight from their software store. Would people be open for this? I could look into it myself but I want to see if there is an appetite for it.\r\n\r\nFlatpack [instructions](https://github.com/flatpak/flatpak) and [flathub](https://flathub.org/)\r\nalong with [electron and flatpak](https://docs.flatpak.org/en/latest/electron.html)",
+ "summary": "Implement Flatpak support for Threat Dragon. \n\nEvaluate the benefits of offering a Flatpak installation option as an alternative to Snaps and AppImages. Focus on the convenience for users in downloading Threat Dragon directly from their software store.\n\nResearch Flatpak and Flathub documentation thoroughly. Review the Flatpak [instructions](https://github.com/flatpak/flatpak) and explore Flathub [guidelines](https://flathub.org/). \n\nInvestigate how to package the application using Electron within Flatpak. Consult the [Electron and Flatpak documentation](https://docs.flatpak.org/en/latest/electron.html) for specific requirements.\n\nBegin by:\n1. Setting up a Flatpak development environment.\n2. Creating a Flatpak manifest file for the Threat Dragon application.\n3. Testing the build process locally to ensure functionality.\n4. Engaging with the community to gauge interest in this feature.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/626",
@@ -9995,10 +10331,10 @@
146
],
"labels": [
- 71,
- 78,
80,
- 82
+ 82,
+ 78,
+ 71
]
}
},
@@ -10011,6 +10347,7 @@
"node_id": "I_kwDOEAWEP85jHNdW",
"title": "Use RAAML for threat models",
"body": "**Describe what problem your feature request solves**\r\n\r\n\r\nThe Risk Analysis and Assessment Modeling Language (RAAML) specification is a sysml compliant format that would allow integration with other modeling capabilities such as simulation. It is also a component UAFML. \r\n\r\n**Describe the solution you'd like**\r\n\r\n\r\nImplement modeling capabilities to support RAAML either as default or as content import/export.\r\n\r\n**Additional context**\r\n\r\n\r\nhttps://www.omg.org/spec/RAAML/1.0/Beta2/About-RAAML\r\nhttps://emfjson.github.io/projects/ecorejs/latest/",
+ "summary": "Implement RAAML support for threat models. Utilize the Risk Analysis and Assessment Modeling Language (RAAML) specification to facilitate integration with simulation tools and enhance modeling capabilities. Ensure compatibility with UAFML and SysML standards.\n\n**First Steps:**\n1. Review the RAAML specification at the provided OMG link to understand its structure and requirements.\n2. Analyze current modeling capabilities and identify integration points for RAAML.\n3. Design a framework for importing and exporting RAAML content.\n4. Develop a prototype that demonstrates basic RAAML modeling capabilities.\n5. Test the prototype with existing threat models to validate functionality.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/639",
@@ -10026,9 +10363,9 @@
"repository": 502,
"assignees": [],
"labels": [
- 71,
73,
- 77
+ 77,
+ 71
]
}
},
@@ -10041,6 +10378,7 @@
"node_id": "I_kwDOEAWEP85jNXUB",
"title": "Make the API OSLC compliant",
"body": "**Describe what problem your feature request solves**\r\n\r\n\r\nModeling tools have their own interoperability specification called Open Services for Lifecycle Collaboration (OSLC). It would be nice if Threat Dragon could provide OSLC compatibility to facilitate ALM / PLM integration.\r\n\r\n**Describe the solution you'd like**\r\n\r\n\r\nProvide OSLC compatible API and documentation with OpenAPI.\r\n\r\n**Additional context**\r\n\r\n\r\nhttps://open-services.net/\r\nhttps://www.ibm.com/docs/en/elms/elm/6.0.4?topic=integrating-oslc-integrations\r\nhttps://docs.oasis-open-projects.org/oslc-op/qm/v2.1/os/quality-management-spec.html\r\n",
+ "summary": "Implement OSLC Compliance for API\n\n- Address the need for interoperability among modeling tools by making the Threat Dragon API OSLC compliant.\n- Ensure compatibility with Open Services for Lifecycle Collaboration (OSLC) to enable integration with Application Lifecycle Management (ALM) and Product Lifecycle Management (PLM) systems.\n- Develop an OSLC-compatible API that adheres to the specifications outlined on the OSLC website and related documentation.\n\nFirst Steps:\n1. Review the OSLC specifications and guidelines available at https://open-services.net/ to understand the requirements for compliance.\n2. Analyze the current Threat Dragon API structure and identify necessary modifications to align with OSLC standards.\n3. Create a design plan for the new OSLC-compatible API, including endpoints and data formats.\n4. Implement the API changes, ensuring to include detailed documentation using OpenAPI for ease of use and integration by third parties.\n5. Test the new API for compliance with OSLC standards and validate functionality with ALM/PLM tools.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/641",
@@ -10056,9 +10394,9 @@
"repository": 502,
"assignees": [],
"labels": [
- 71,
73,
- 87
+ 87,
+ 71
]
}
},
@@ -10071,6 +10409,7 @@
"node_id": "I_kwDOEAWEP85jhf0_",
"title": "Use the new File System Access API on Chromium browsers",
"body": "**Describe what problem your feature request solves**\r\nThe actual way of handling local threat models could generate a lot of files because a new file is generated each time a user saves the working threat model.\r\n\r\n**Describe the solution you'd like**\r\nOn chromium based browsers the new File System Access API should be used (it's the one used in https://vscode.dev/)\r\n\r\n**Additional context**\r\nBlog presenting the API: https://developer.chrome.com/articles/file-system-access/ \r\n",
+ "summary": "Implement the File System Access API for Chromium browsers to improve local threat model file handling. \n\n**Problem to Solve:**\nCurrent file management generates excessive files by creating a new file each time a user saves a threat model, leading to clutter and inefficiency.\n\n**Proposed Solution:**\nUtilize the File System Access API to allow direct access and manipulation of files in the user's local file system. This will streamline the saving process, enabling users to overwrite existing files or manage their local files more effectively.\n\n**Technical Steps to Tackle the Problem:**\n1. Research the File System Access API and understand its capabilities and limitations.\n2. Update the codebase to replace current file handling methods with the File System Access API.\n3. Implement a file picker UI to allow users to select existing files to overwrite or choose locations for new files.\n4. Test the integration across different Chromium-based browsers to ensure compatibility and performance.\n5. Document the changes and provide user guidance on the new file handling process.\n\n**Resources:**\nRefer to the detailed blog on the File System Access API for implementation guidelines: https://developer.chrome.com/articles/file-system-access/",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/643",
@@ -10088,8 +10427,8 @@
43
],
"labels": [
- 71,
- 80
+ 80,
+ 71
]
}
},
@@ -10102,6 +10441,7 @@
"node_id": "I_kwDOEAWEP85pxCwC",
"title": "Provide demo versions of 1.x and 2.x to combined site",
"body": "**Describe what problem your feature request solves**:\r\nIt would be good to have demos of both versions 1.x and 2.x on a combined site alongside pytm\r\n\r\n**Describe the solution you'd like**:\r\n@izar has offered to provide access to [threatmodeling.dev](http://threatmodeling.dev/) site so that demos of pytm and Threat Dragon can be alongside each other\r\n\r\n**Additional context**:\r\nControl of the site would also be with OWASP along with @izar, and this will guarantee long term continuity\r\n",
+ "summary": "Create demo versions of both 1.x and 2.x on a combined site with pytm. Ensure that the demos are accessible through the threatmodeling.dev platform. \n\n1. Collaborate with @izar to set up the environment on the threatmodeling.dev site.\n2. Establish a version control process to manage updates for both 1.x and 2.x demos.\n3. Ensure that OWASP maintains control over the site for long-term support and continuity, involving @izar in the management.\n4. Develop a user-friendly interface to allow easy navigation between the demos of pytm and Threat Dragon.\n5. Test the demos thoroughly to ensure they function correctly and are user-friendly before launch. \n\nBy implementing these steps, you will provide users with an integrated experience of the different versions available.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/684",
@@ -10117,9 +10457,9 @@
"repository": 502,
"assignees": [],
"labels": [
- 71,
+ 87,
79,
- 87
+ 71
]
}
},
@@ -10132,6 +10472,7 @@
"node_id": "I_kwDOEAWEP85qAOP3",
"title": "Integration with OpenAI API",
"body": "**Describe what problem your feature request solves**:\r\nIt would be good to investigate Threat Dragon integration with OpenAI API\r\n\r\n**Describe the solution you'd like**:\r\nDescription of what it would take and roughly how long\r\n\r\n**Additional context**:\r\n@cvlucian asks: _\"research into Threat Dragon being integrated with OpenAI API? Endless possibilities probably to populate threat models models based on technical spec docs or simple input, AI assessment of risks etc.\"_\r\n",
+ "summary": "Investigate integration of Threat Dragon with OpenAI API. Focus on leveraging OpenAI's capabilities to enhance threat modeling processes by populating models using technical specifications or simple inputs. Assess potential for AI-driven risk evaluations.\n\nOutline the required steps for integration:\n1. Research OpenAI API documentation to understand its functionalities and limitations.\n2. Define use cases for Threat Dragon, such as:\n - Automatically generating threat models from input data.\n - Conducting AI-based assessments of identified risks.\n3. Evaluate data inputs and outputs necessary for seamless integration.\n4. Create a prototype to test API interactions with Threat Dragon.\n5. Estimate implementation time based on complexity of integration tasks.\n\nCollaborate with @cvlucian and other contributors to refine use cases and gather additional insights on practical applications. Prioritize identifying any potential challenges related to data privacy and compliance when utilizing AI models for sensitive information.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/685",
@@ -10160,6 +10501,7 @@
"node_id": "I_kwDOEAWEP85twZey",
"title": "Enable threats to be reassigned and/or duplicated to other components",
"body": "**Describe what problem your feature request solves**:\r\n\r\nOnce a threat is created, it is not possible to duplicate it or move it to other components. You need to manually repeat the whole process or delete a threat and add new one in different component.\r\n\r\n**Describe the solution you'd like**:\r\n\r\nIt would be really useful to be able to move threats from one component to another and to duplicate once created threat in other component. \r\n\r\n**Additional context**:\r\n\r\nThere are threats applicable to many components and it is time-consuming to create the same threat in every one of them. Also, once the component is created and threats are added to them, if you want to split it into two components you need to recreate every threat from the original one.\r\n",
+ "summary": "Implement functionality to enable reassignment and duplication of threats across components. \n\n**Problem Statement**: \nCurrently, threats can only be created manually for each component, leading to inefficiencies. Users must duplicate efforts by recreating threats or deleting and re-adding them to different components, which is time-consuming.\n\n**Proposed Solution**: \nIntroduce features that allow users to:\n1. Move a threat from one component to another.\n2. Duplicate an existing threat to another component directly.\n\n**Technical Considerations**:\n- Modify the threat management system to include options for 'Move' and 'Duplicate' actions.\n- Ensure that moving a threat updates all relevant relationships and dependencies associated with that threat.\n- Implement a user interface that allows easy selection of target components for duplication or reassignment.\n- Consider the impact on data integrity and ensure that threats remain consistent across components.\n\n**Next Steps**:\n1. Analyze the current database schema to understand how threats are linked to components.\n2. Design the user interface for moving and duplicating threats.\n3. Develop the backend logic for moving threats, ensuring proper handling of existing references.\n4. Test the new functionalities thoroughly to avoid data inconsistencies.\n5. Document the changes and provide user guidelines for utilizing the new features.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/716",
@@ -10175,8 +10517,8 @@
"repository": 502,
"assignees": [],
"labels": [
- 71,
- 73
+ 73,
+ 71
]
}
},
@@ -10189,6 +10531,7 @@
"node_id": "I_kwDOEAWEP85uU5Sc",
"title": "Upgrade to latest antv/x6 drawing package",
"body": "**Describe what problem your feature request solves**:\r\nwe have a lot of niggles with the drawing package, and this may be because we are using an old version :\r\n\r\n```\r\n \"@antv/x6\": \"^1.32.7\",\r\n \"@antv/x6-vue-shape\": \"^1.4.0\",\r\n```\r\n\r\n**Describe the solution you'd like**:\r\nupgrade to latest version 2.x - note that this is a breaking change:\r\n\r\n```\r\n \"@antv/x6\": \"^2.13.1\",\r\n \"@antv/x6-vue-shape\": \"^2.1.1\",\r\n```\r\n\r\n**Additional context**:\r\n\r\n",
+ "summary": "Upgrade the antv/x6 drawing package to the latest version to resolve existing issues with the current implementation. The current dependencies are:\n\n```\n \"@antv/x6\": \"^1.32.7\",\n \"@antv/x6-vue-shape\": \"^1.4.0\",\n```\n\nTransition to the following versions, noting that this is a breaking change:\n\n```\n \"@antv/x6\": \"^2.13.1\",\n \"@antv/x6-vue-shape\": \"^2.1.1\",\n```\n\n### Steps to Tackle the Problem:\n\n1. **Review Release Notes**: Examine the release notes for versions 2.x to identify breaking changes and new features.\n \n2. **Update Dependencies**: Modify the `package.json` to reflect the new versions.\n\n3. **Run Tests**: Execute existing unit and integration tests to identify breaking changes or issues introduced by the upgrade.\n\n4. **Refactor Code**: Address any deprecated methods or properties within the codebase that may have changed in the new version.\n\n5. **Test Functionality**: Manually test the application to ensure all drawing functionalities work as expected with the upgraded packages.\n\n6. **Document Changes**: Update documentation to reflect any changes in usage due to the upgrade.\n\n7. **Monitor for Bugs**: After deployment, monitor the application for any new issues that may arise from the upgrade.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/722",
@@ -10206,10 +10549,10 @@
43
],
"labels": [
- 71,
- 75,
83,
- 87
+ 75,
+ 87,
+ 71
]
}
},
@@ -10222,6 +10565,7 @@
"node_id": "I_kwDOEAWEP85zLzuV",
"title": "provide pipeline tests for utilities",
"body": "**Describe what problem your feature request solves**:\r\nWe are not sure if the utilities still run OK, and these should be checked in one of the pipelines\r\n\r\n**Describe the solution you'd like**:\r\nCheck that the utilities still run in the CI pipeline\r\n\r\n**Additional context**:\r\nUtilities:\r\n* DragonExtractor\r\n* threat-mvp\r\n* TMT2TD\r\nand possibly [DragonGPT](https://github.com/LuizBoina/dragon-gpt)\r\n\r\n",
+ "summary": "Implement pipeline tests for utilities to ensure functionality. Verify that the following utilities execute correctly within the CI pipeline:\n\n1. DragonExtractor\n2. threat-mvp\n3. TMT2TD\n4. Potentially include DragonGPT\n\nBegin by creating test cases for each utility. Use unit tests to validate core functionalities and integration tests for interactions with other components. \n\nSteps to tackle the problem:\n1. Review the existing utility code to identify key functions and expected outputs.\n2. Develop unit tests for each utility, covering edge cases and typical use cases using a testing framework like pytest or unittest.\n3. Integrate these tests into the CI pipeline configuration, ensuring they run on each commit or pull request.\n4. Monitor test results and adjust as necessary to resolve any failures or issues.\n5. Document the testing process and update README files where appropriate to guide future contributions.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/762",
@@ -10237,8 +10581,8 @@
"repository": 502,
"assignees": [],
"labels": [
- 71,
- 79
+ 79,
+ 71
]
}
},
@@ -10251,6 +10595,7 @@
"node_id": "I_kwDOEAWEP8524btK",
"title": "Clicking on data flow does not open it in properties",
"body": "**Describe the bug**:\r\nClicking on an existing data flow does not set its properties into the properties section. A double click is needed, but it results in an addition of a new point on the data flow.\r\n\r\n**Expected behaviour**:\r\nA single click on the data flow makes its properties accessible in the properties section\r\n\r\n**Environment**:\r\n\r\n- Version: 2.1.1\r\n- Platform: Desktop App and Web App\r\n- OS: MAC OS / Windows / Linux\r\n- Browser: All\r\n\r\n**To Reproduce**:\r\n1. Add a data flow\r\n2. Select another component on the diagram\r\n3. Click on the data flow just created\r\n\r\n",
+ "summary": "Fix the issue where clicking on a data flow does not open its properties. Ensure that a single click on the data flow correctly populates the properties section instead of requiring a double click, which inadvertently adds a new point to the data flow.\n\n**Technical Details**:\n- Investigate the event handling for mouse clicks on data flow components within the properties section.\n- Check the logic that differentiates between single and double click actions.\n- Update the event listener for the data flow to ensure that it sets the properties on a single click.\n\n**First Steps**:\n1. Review the code responsible for handling clicks on data flow components.\n2. Identify the function that currently manages the properties section update.\n3. Modify the click event listener to trigger the properties update on a single click.\n4. Test the changes across different platforms (Mac OS, Windows, Linux) and in various browsers to ensure consistent behavior.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/785",
@@ -10284,6 +10629,7 @@
"node_id": "I_kwDOEAWEP853bZp0",
"title": "Add the full database of PLOT4ai threats to Threat Dragon",
"body": "**Describe what problem your feature request solves**:\r\nPLOT4ai contains a database of 86 threats. Threat Dragon recently added support for the categories, similar to the other frameworks, but it would be nice if the threats under each category would become available from a drop-down menu as well.\r\nThe way threats are organized under the categories can be observed here: https://plot4.ai/library\r\n\r\n**Describe the solution you'd like**:\r\nWhen you add a threat (or edit one), you can already select the threat type, which corresponds to the plot4ai category.\r\nPLOT4ai has a [json file](https://github.com/PLOT4ai/plot4ai-library/blob/main/deck.json) available with all the threats; based on this json file and the selected type/category, the relevant threats could be loaded and used to populate a drop-down box called 'threat'. The user could then select the applicable threat.\r\nAdditionally, the information in the _explanation_ field could be used to prefill the Description-field in Threat Dragon and the information in the _recommendations_ field could be used to prefill the Mitigation field in Threat Dragon\r\n\r\n**Additional context**:\r\nPLOT4ai is in it's setup very similar to LINDDUN, which is also part of Threat Dragon. Adding this functionality would also open the possibility to add the threats from LINDDUN to threat dragon.\r\n\r\nThe additions of 'Development Phase' and 'Threat' to the threat dialog should only be visible when the diagram type is 'PLOT4ai', and possibly also for 'LINDDUN' diagram type.\r\n",
+ "summary": "Implement the full database of PLOT4ai threats in Threat Dragon. \n\n**Problem to solve**: Integrate 86 PLOT4ai threats into Threat Dragon, utilizing a user-friendly drop-down menu to enhance user experience when selecting threats.\n\n**Proposed solution**:\n1. Utilize the existing PLOT4ai [JSON file](https://github.com/PLOT4ai/plot4ai-library/blob/main/deck.json) to extract threat data.\n2. Modify the threat addition/editing interface to include a drop-down menu for selecting threats based on the chosen PLOT4ai category.\n3. Prefill the Description field in Threat Dragon with the information from the _explanation_ field of the JSON and the Mitigation field with data from the _recommendations_ field.\n4. Ensure that these new fields ('Development Phase' and 'Threat') appear only when the diagram type is set to 'PLOT4ai' or 'LINDDUN'.\n\n**First steps**:\n- Review the structure of the PLOT4ai JSON file to understand how threats are categorized.\n- Modify the front-end code of Threat Dragon to create a drop-down menu that dynamically populates with threats based on the selected category.\n- Implement the functionality to prefill the Description and Mitigation fields from the corresponding JSON data.\n- Test the integration to ensure that the drop-down menu and prefilled fields work correctly within the Threat Dragon interface.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/796",
@@ -10301,9 +10647,9 @@
150
],
"labels": [
- 71,
72,
- 73
+ 73,
+ 71
]
}
},
@@ -10316,6 +10662,7 @@
"node_id": "I_kwDOEAWEP853bcDN",
"title": "Add the full database of LINDDUN threats to Threat Dragon",
"body": "**Describe what problem your feature request solves**:\r\nLINDDUN contains a database of 35 threats. Threat Dragon already has support for the categories, similar to the other frameworks, but it would be nice if the threats under each category would become available from a drop-down menu as well.\r\nThe way threats are organized under the categories can be observed here: https://downloads.linddun.org/linddun-go/default/v20230802/go.pdf\r\n\r\n**Describe the solution you'd like**:\r\nWhen you add a threat (or edit one), you can already select the threat type, which corresponds to the linddun category.\r\nIf the linddun cards were to be digitalized in json format - similar to plot4ai - then this could serve as a database; then, based on this json file and the selected type/category, the relevant threats could be loaded and used to populate a drop-down box called 'threat'. The user could then select the applicable threat.\r\nAdditionally, the rest of the information on the linddun cards could be used to prefill the Description-field in Threat Dragon.\r\n\r\n**Additional context**:\r\nLINDDUN is very similar to PLOT4ai, which was also recently added to Threat Dragon. Adding this functionality would also open the possibility to add the threats from PLOT4ai to threat dragon.",
+ "summary": "Implement full integration of the LINDDUN threat database into Threat Dragon. \n\n**Problem**: Enhance the usability of Threat Dragon by allowing users to select specific LINDDUN threats from a drop-down menu, improving threat identification in threat modeling.\n\n**Solution**: \n1. Digitalize the LINDDUN threat cards into a JSON format, similar to the PLOT4ai integration.\n2. Update the Threat Dragon interface to include a new drop-down menu for threats, linked to the category selected by the user.\n3. Populate the drop-down based on the JSON data, allowing users to select relevant threats based on their chosen category.\n4. Use additional details from the LINDDUN cards to automatically fill the Description field in Threat Dragon when a threat is selected.\n\n**First Steps**:\n- Gather the JSON format of the LINDDUN threat cards from the provided PDF link.\n- Develop a mapping of the threat categories to the existing Threat Dragon structure.\n- Implement the front-end changes to introduce a new drop-down menu for threat selection.\n- Test the integration with sample data to ensure smooth functionality and accurate threat population.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/797",
@@ -10333,9 +10680,9 @@
150
],
"labels": [
- 71,
72,
- 73
+ 73,
+ 71
]
}
},
@@ -10344,10 +10691,11 @@
"pk": 361,
"fields": {
"nest_created_at": "2024-09-11T20:09:18.224Z",
- "nest_updated_at": "2024-09-11T20:09:18.224Z",
+ "nest_updated_at": "2024-09-13T03:53:44.409Z",
"node_id": "I_kwDOEAWEP854AXzc",
"title": "Isolate Authentication and Repository",
"body": "**Describe what problem your feature request solves**:\r\nIn reviewing #629, #426 and #1 I believe we need to decouple Authentication from the Repository, in cases where it is sensible.\r\n\r\n| Authentication | Repository | Status |\r\n| ------------- | ------------- |---------|\r\n| Github | Github Repo | Implemented |\r\n| Bitbucket | Bitbucket Repo | Implemented |\r\n| AWS IAM | S3 | #426 requests |\r\n| AWS IAM | AWS SQL | New |\r\n| Azure | Azure Blob | New |\r\n| Azure | Azure SQL | New |\r\n(Note - I am not proposing to build all of these combos!)\r\n\r\n\r\n**Describe the solution you'd like**:\r\n- [ ] Enable either intermediate screens between Provider selection and Repo selection for choice of Repository (where appropriate) or rely upon property files only.\r\n- [ ] Isolate the Provider and Repository coupling in Node - at present the authentication mechanism sets the repository, and they are 1-2-1.\r\n\r\n**Key Questions**:\r\n1. Is there a valid use case here to have a single Authentication Provider enable access to multiple types of repository? (I am not anticipating multiple repositories within a threat dragon instance)\r\n2. Do we want end users/modellers, not deployers, to select between repository options themselves or defer this to config in deployment?\r\n\r\nAs always, happy to be told this is beyond the scope or vision of the platform. ",
+ "summary": "Isolate Authentication from Repository\n\n**Problem Solving**: Decouple Authentication from Repository to allow flexible integration with various repository types without direct coupling.\n\n**Proposed Solution**:\n1. Implement intermediate screens or use property files to allow users to choose the repository type after selecting the authentication provider.\n2. Refactor Node to eliminate the 1-2-1 relationship between authentication mechanisms and repositories, enabling a single authentication provider to access multiple repository types.\n\n**Key Questions to Address**:\n- Validate the use case for a single Authentication Provider accessing multiple repository types.\n- Determine if end users should choose repository options or if this decision should be made during deployment configuration.\n\n**First Steps**:\n1. Review existing code to identify where authentication and repository coupling occurs.\n2. Design a prototype for intermediate screens or property file management.\n3. Assess the implications of refactoring authentication and repository interaction on current implementations.\n4. Gather feedback on use cases from stakeholders to guide the development process.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/805",
@@ -10358,12 +10706,10 @@
"comments_count": 1,
"closed_at": null,
"created_at": "2023-11-28T00:24:25Z",
- "updated_at": "2024-07-15T09:23:02Z",
+ "updated_at": "2024-09-12T07:58:30Z",
"author": 141,
"repository": 502,
- "assignees": [
- 141
- ],
+ "assignees": [],
"labels": [
71,
74
@@ -10379,6 +10725,7 @@
"node_id": "I_kwDOEAWEP857QYJr",
"title": "Desktop should show indication when loading",
"body": "**Describe what problem your feature request solves**:\r\nThe desktop application can take a long time to load, and the spinner in App.vue does not show while loading\r\n\r\n**Describe the solution you'd like**:\r\nDesktop app shows indication of loading\r\n\r\n**Additional context**:\r\nsteps to reproduce:\r\n1. download the installer for a new version of Threat Dragon\r\n2. install the new version\r\n3. invoke the new version and wait up to 30s before getting a window appearing\r\n",
+ "summary": "Implement a loading indicator for the desktop application during startup. \n\n**Problem to Solve**: The desktop application currently lacks a visual indication when it is loading, leading to user confusion during the delay, which can last up to 30 seconds.\n\n**Solution**: \n1. Modify the App.vue component to display a loading spinner during the initialization phase of the application.\n2. Ensure the spinner is visible until the application fully loads and the main window appears.\n\n**Technical Steps**:\n1. Identify the lifecycle hooks in App.vue responsible for application startup.\n2. Introduce a reactive property (e.g., `isLoading`) to manage the loading state.\n3. Set `isLoading` to `true` at the beginning of the startup process and change it to `false` once the application is fully loaded.\n4. Update the template in App.vue to conditionally render the loading spinner based on the `isLoading` property.\n5. Test the implementation by installing a new version of Threat Dragon and verifying that the loading spinner appears during startup.\n\n**Next Steps**:\n- Review the current initialization code in App.vue.\n- Create a simple loading spinner component if none exists.\n- Implement the state management for loading indication.\n- Conduct user testing to ensure the loading experience is improved.",
"state": "open",
"state_reason": "reopened",
"url": "https://github.com/OWASP/threat-dragon/issues/823",
@@ -10394,9 +10741,9 @@
"repository": 502,
"assignees": [],
"labels": [
- 71,
75,
- 78
+ 78,
+ 71
]
}
},
@@ -10405,10 +10752,11 @@
"pk": 363,
"fields": {
"nest_created_at": "2024-09-11T20:09:22.413Z",
- "nest_updated_at": "2024-09-11T20:09:22.413Z",
+ "nest_updated_at": "2024-09-13T03:53:45.749Z",
"node_id": "I_kwDOEAWEP857ZVYL",
"title": "PDF report generation froze the application",
"body": "**Describe the bug**:\r\nGenerating a PDF report halted the desktop application\r\n\r\n**Expected behaviour**:\r\nApplication should not freeze\r\n\r\n**Environment**:\r\n\r\n- Version: 2.1.2\r\n- Platform: Desktop App\r\n- OS: Windows\r\n- Browser: electron\r\n\r\n**To Reproduce**:\r\n\r\n**Any additional context, screenshots, etc**:\r\n@mikkolehtisalo reports \"the Windows Server we run the tool on acted differently from ordinary desktop operating systems. I ended up moving the data to Excel/Word and finishing the report by hand.\"\r\n",
+ "summary": "Investigate the application freeze during PDF report generation. Ensure the application remains responsive while generating reports.\n\n**Technical Details**:\n- Version: 2.1.2\n- Platform: Desktop App\n- OS: Windows (specifically mentioned issues on Windows Server)\n- Browser: Electron\n\n**Steps to Reproduce**:\n1. Open the application.\n2. Initiate PDF report generation.\n\n**First Steps to Tackle the Problem**:\n1. Analyze the report generation code for synchronous operations that could block the main thread.\n2. Implement asynchronous processing for PDF generation to prevent UI freeze.\n3. Test the application on both regular desktop and Windows Server environments to replicate the issue.\n4. Utilize logging to capture any errors or performance metrics during report generation. \n5. Consider using a worker thread or a background process for handling PDF generation tasks.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/826",
@@ -10419,16 +10767,14 @@
"comments_count": 5,
"closed_at": null,
"created_at": "2024-01-08T11:16:52Z",
- "updated_at": "2024-07-18T18:54:57Z",
+ "updated_at": "2024-09-12T07:56:10Z",
"author": 43,
"repository": 502,
- "assignees": [
- 151
- ],
+ "assignees": [],
"labels": [
+ 88,
78,
- 81,
- 88
+ 81
]
}
},
@@ -10441,6 +10787,7 @@
"node_id": "I_kwDOEAWEP857ZbP2",
"title": "Generic git support",
"body": "**Describe what problem your feature request solves**:\r\nNot everyone uses github or bitbucket for git repo access, it would be good to provide for these organizations\r\n\r\n**Describe the solution you'd like**:\r\nProvide support for git, not specific to github or bitbucket\r\n\r\n**Additional context**:\r\n@mikkolehtisalo reports \"the application has capability to save into github. However configuring internal git that is not github would require code changes. More generic git support would be super.\"\r\n",
+ "summary": "Implement generic Git support to enable repository access beyond GitHub and Bitbucket. \n\n1. Assess current integration capabilities and identify hard-coded dependencies on GitHub and Bitbucket within the application.\n2. Develop a flexible configuration system that allows users to input their own Git repository details (e.g., URL, authentication).\n3. Refactor the codebase to utilize standard Git commands and APIs, ensuring compatibility with various Git hosting services.\n4. Test the implementation with multiple Git providers to validate functionality and stability.\n5. Update documentation to guide users on how to configure their own Git repositories.\n\nStart by analyzing the existing code that handles Git interactions and outline a plan for abstraction to accommodate different Git providers.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/828",
@@ -10456,9 +10803,9 @@
"repository": 502,
"assignees": [],
"labels": [
- 71,
74,
- 75
+ 75,
+ 71
]
}
},
@@ -10471,6 +10818,7 @@
"node_id": "I_kwDOEAWEP857rNmj",
"title": "Revise data model of threat + mitigations",
"body": "**Describe what problem your feature request solves**:\r\nMultiple mitigations for a threat are possible, and also multiple threats per mitigations\r\n\r\n**Describe the solution you'd like**:\r\nrevise data model of threat and mitigations to be more flexible\r\n\r\n**Additional context**:\r\nUser reports:\r\n\"_The data model for threat mitigations is in my opinion simply incorrrect. One threat may have many mitigations, which has different implementation status. At this moment the tool seems to allow tracking only one mitigation per threat._\r\n\r\n_Not being able to copy or move threats makes impossible to refactor the environment image later on. I would take even \"make a copy of this threat to there\" without any linking of contents to fix this. Without this I had to redraw the environment and do a lot of manual work to get the final report cleaned up._\"\r\n\r\n",
+ "summary": "Revise the data model for threats and mitigations to enhance flexibility. \n\n**Address the following issues**:\n1. Allow multiple mitigations for each threat, accommodating various implementation statuses.\n2. Enable multiple threats to be associated with each mitigation.\n\n**Implement a solution**:\n1. Redesign the database schema to support a many-to-many relationship between threats and mitigations, possibly using a join table.\n2. Update the application logic to handle the new relationships, ensuring that users can add, edit, and view multiple mitigations per threat and vice versa.\n\n**Consider additional features**:\n1. Implement functionality to copy or move threats, allowing users to refactor environment images without redundant manual work.\n\n**First steps**:\n1. Analyze the current data model structure and identify necessary changes.\n2. Create a prototype of the new data model and gather feedback from stakeholders.\n3. Begin implementing the database schema changes and update the application code accordingly.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/834",
@@ -10486,8 +10834,8 @@
"repository": 502,
"assignees": [],
"labels": [
- 71,
- 75
+ 75,
+ 71
]
}
},
@@ -10500,6 +10848,7 @@
"node_id": "I_kwDOEAWEP859hs3c",
"title": "winget support would be great",
"body": "**Describe what problem your feature request solves**:\r\n\r\nIt would make the installation and upgrading the software on modern Windows very very easy.\r\n\r\n**Describe the solution you'd like**:\r\n\r\nI'd love to be able to say `winget install --id OWASP.ThreatDragon` or similar -- and of course subsequently `winget upgrade --id OWASP.ThreatDragon` -- in order to install and upgrade the software.\r\n\r\nPS: I think there is a way to integrate this with GitHub automation, but I need to dig that up again and will comment once I find it. That way if the assets in your releases follow a particular schema a PR will automatically go to microsoft/winget-pkgs.",
+ "summary": "Implement winget support to simplify software installation and upgrades on modern Windows systems. \n\n1. Enable users to install software using commands like `winget install --id OWASP.ThreatDragon` and upgrade with `winget upgrade --id OWASP.ThreatDragon`.\n2. Investigate integration with GitHub automation to streamline the process; ensure that release assets conform to a specified schema for automatic PR submissions to microsoft/winget-pkgs.\n\nFirst steps:\n- Research the winget CLI documentation to understand command usage and requirements.\n- Develop a manifest file for OWASP ThreatDragon that adheres to winget's specifications.\n- Test the installation and upgrade commands locally to verify functionality.\n- Explore GitHub Actions to automate the PR creation process for updates.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/848",
@@ -10518,10 +10867,10 @@
153
],
"labels": [
- 71,
- 78,
80,
- 81
+ 81,
+ 78,
+ 71
]
}
},
@@ -10534,6 +10883,7 @@
"node_id": "I_kwDOEAWEP8596XGk",
"title": "Long term file format",
"body": "**Describe what problem your feature request solves**:\r\nThe Threat Dragon file format / JSON schema uses two related but incompatible versions for 1.x and 2.x, and neither of these is a format other tools can use\r\n\r\n**Describe the solution you'd like**:\r\nThreat Dragon version 3.x should use a standard file format instead of the existing incompatible versions 1.x and versions 2.x formats\r\n[Open Threat Model](https://github.com/iriusrisk/OpenThreatModel) file format has been released and could be considered alongside CycloneDx\r\n\r\n**Additional context**:\r\n\r\n* [CycloneDx](https://cyclonedx.org/) is an [ECMA standard 424](https://ecma-international.org/publications-and-standards/standards/ecma-424/)\r\n* CycloneDx can provide the definition of the threat model file, as a TMBOM (Threat Model Bill of Materials)\r\n* There is also a [CyclonDX github issue](https://github.com/CycloneDX/specification/issues/462) for tracking this work\r\n* The TMBOM fields need to be defined in the [CycloneDx Property Taxonomy](https://github.com/CycloneDX/cyclonedx-property-taxonomy)\r\n* The closest CycloneDX BOM to a TMBOM is the [example of an HBOM](https://github.com/CycloneDX/bom-examples/blob/master/HBOM/PCIe-SATA-adapter-board/bom.json) (Hardware BOM)\r\n* An informal workstream (#[workstream-threat-model](https://cyclonedx.slack.com/archives/C073KMCQMUG)) has been set up on the OWASP CycloneDX Slack which meets regularly\r\n* [Agenda and minutes are available](https://docs.google.com/document/d/1OxM2KDAKmkY6Z-K0Qe5Gce7jMWWnCU1Osd3P4V8t8yQ/) and [TMBOM Use Cases and Requirements](https://docs.google.com/document/d/1gwfk0GHafuUE06GRc31kkQ-NTB2w8ZatYGKUm7Ah-5Q/)\r\n\r\n",
+ "summary": "Create a standardized file format for Threat Dragon version 3.x to replace the incompatible 1.x and 2.x JSON schemas. Evaluate the Open Threat Model file format and CycloneDX as potential solutions.\n\n1. Review the CycloneDX specification, particularly ECMA standard 424, which outlines the Threat Model Bill of Materials (TMBOM) structure.\n2. Analyze the TMBOM fields and their definitions in the CycloneDX Property Taxonomy.\n3. Compare the TMBOM requirements with the existing CycloneDX BOM examples, specifically the HBOM for reference.\n4. Participate in the informal workstream on OWASP CycloneDX Slack to gather insights and collaborate with others involved in this effort.\n5. Consult the provided agenda and minutes for ongoing discussions and document any relevant use cases and requirements for the TMBOM. \n\nBy standardizing the file format, improve interoperability with other tools and enhance usability for users of Threat Dragon.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/850",
@@ -10551,9 +10901,9 @@
43
],
"labels": [
- 71,
+ 89,
75,
- 89
+ 71
]
}
},
@@ -10566,6 +10916,7 @@
"node_id": "I_kwDOEAWEP85-aL-p",
"title": "Refactor Providers to use standard model",
"body": "The existing providers have been maintained against the initial Github schema. A refactor to maintain a standard repository schema is necessary to simplify the code base.",
+ "summary": "Refactor the Providers to conform to the standard repository schema. Analyze the current implementation of the providers that are based on the initial GitHub schema. Identify discrepancies and areas for improvement to align with the new model.\n\n1. Review the standard repository schema and document the differences between it and the current provider implementations.\n2. Update data structures and methods in the providers to match the standard schema requirements.\n3. Ensure that all existing functionality remains intact by writing unit tests for the current behavior.\n4. Gradually replace the old schema references in the codebase with the new standard model.\n5. Conduct thorough testing to validate that all providers function correctly after the refactor.\n\nBy following these steps, enhance the maintainability and clarity of the codebase, which will streamline future development efforts.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/860",
@@ -10583,8 +10934,8 @@
141
],
"labels": [
- 74,
- 88
+ 88,
+ 74
]
}
},
@@ -10597,6 +10948,7 @@
"node_id": "I_kwDOEAWEP85-mY1C",
"title": "Feature Request: OPSEC Threat Modeling",
"body": "**Describe what problem your feature request solves**:\r\nThere is no OPSEC based threat modeling unless its in context SDLC\r\n\r\n**Describe the solution you'd like**:\r\nI would like an option to use Threat-Dragon to create OPSEC or Operation Security based threat model\r\n\r\n**Additional context**:\r\nMost threat models are defined in terms of software developers life cycle or IT. OPSEC applies to IT and all domains especially physical. I could find no OPSEC threat models and think this could easily be added to Threat-Dragon and increase the user baser. The ability to easily create OPSEC threat models and display them especially for the physical domain has a lot of value. See an good example here: https://old.reddit.com/r/opsec/comments/eh6uvc/want_to_learn_opsec_as_a_total_beginner_start_here/\r\n\r\n\r\n",
+ "summary": "Implement OPSEC Threat Modeling feature in Threat-Dragon. \n\n1. Identify the current limitations in Threat-Dragon regarding OPSEC-based threat modeling. \n2. Research existing OPSEC frameworks and methodologies to incorporate relevant aspects into the tool.\n3. Develop a user interface option for creating OPSEC threat models, ensuring it accommodates both IT and physical domains.\n4. Create templates or guidelines for users to easily build OPSEC threat models.\n5. Validate the feature with potential users to gather feedback and improve functionality.\n\nThis enhancement will broaden Threat-Dragon’s applicability and attract a wider user base by addressing a gap in existing threat modeling tools.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/861",
@@ -10612,8 +10964,8 @@
"repository": 502,
"assignees": [],
"labels": [
- 71,
- 75
+ 75,
+ 71
]
}
},
@@ -10626,6 +10978,7 @@
"node_id": "I_kwDOEAWEP85-5wp2",
"title": "Create AppVeyor pipeline for signing Windows installer",
"body": "**Describe what problem your feature request solves**:\r\nAs of July 2023 the Certificate Authority/Brower Forum’s [CA/B Forum](https://cabforum.org/baseline-requirements-code-signing/) requires all code signing private keys be stored on secure hardware.\r\nThe cost is prohibitive, $175 to $250 per year\r\n\r\n**Describe the solution you'd like**:\r\nWindows installer signed\r\n\r\n**Additional context**:\r\nThe [How to Sign a Windows App in Electron Builder](https://codesigningstore.com/how-to-sign-a-windows-app-in-electron-builder) describes what needs to be done to sign the Threat Dragon application.\r\nThe existing certificate runs out on 20th February 2024\r\n",
+ "summary": "Create an AppVeyor pipeline for signing the Windows installer for the Threat Dragon application. \n\n1. Store code signing private keys on secure hardware to comply with CA/B Forum requirements effective July 2023. \n2. Evaluate and select a cost-effective hardware security module (HSM) solution, considering expenses between $175 to $250 per year.\n3. Implement the signing process using the guidance from the \"How to Sign a Windows App in Electron Builder\" documentation.\n4. Configure the AppVeyor pipeline to automate the signing of the Windows installer.\n5. Ensure that the current certificate is renewed before it expires on February 20, 2024.\n\nFirst steps:\n- Research and choose an appropriate HSM provider.\n- Set up an AppVeyor account and create a new pipeline configuration.\n- Write and test the script for the signing process within the pipeline.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/872",
@@ -10641,9 +10994,9 @@
"repository": 502,
"assignees": [],
"labels": [
- 71,
+ 90,
87,
- 90
+ 71
]
}
},
@@ -10656,6 +11009,7 @@
"node_id": "I_kwDOEAWEP85_m2Mp",
"title": "Support Github/Bitbucket/Gitlab in Desktop",
"body": "I am unclear why we would not support the various repositories in Desktop mode, so proposing enabling.\r\n\r\nWelcome feedback.",
+ "summary": "Enable support for GitHub, Bitbucket, and GitLab in Desktop mode. Assess the current architecture of the Desktop application to identify integration points for these repositories. \n\nFirst, gather information on the existing APIs for GitHub, Bitbucket, and GitLab. Review the authentication methods currently used in Desktop mode and determine if they can accommodate the additional services. \n\nNext, implement a plan to create a user interface for repository selection, ensuring that it allows users to authenticate and manage repositories seamlessly. Consider leveraging existing libraries or SDKs for each service to streamline the integration process. \n\nFinally, solicit feedback from users and developers to refine the functionality and address any potential issues that arise during the implementation phase.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/883",
@@ -10671,9 +11025,9 @@
"repository": 502,
"assignees": [],
"labels": [
- 71,
+ 91,
78,
- 91
+ 71
]
}
},
@@ -10686,6 +11040,7 @@
"node_id": "I_kwDOEAWEP86BTduO",
"title": "Provide translation for Demo Model Page",
"body": "**Describe what problem your feature request solves**:\r\nCurrently , In the [Select Demo Page] User can't access the Demo models name in any language other than English as shown below\r\n\r\n**Describe the solution you'd like**:\r\nFor Better User Experience the Demo models should also have used i18n \r\n\r\n**Additional context**:\r\n\r\n\r\n",
+ "summary": "Implement internationalization (i18n) for the Demo Model Page. \n\n1. Identify the current implementation of the Demo Model names that restricts access to English only.\n2. Analyze the existing codebase to locate where the Demo Model names are defined and rendered.\n3. Create a localization file that includes translations for the Demo Model names in multiple languages.\n4. Modify the rendering logic to pull the appropriate translation based on the user's selected language.\n5. Test the changes to ensure that the Demo Model names display correctly in different languages.\n6. Update the documentation to reflect the new i18n implementation.\n\nEnhance user experience by enabling multilingual support for Demo Model names.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/902",
@@ -10701,8 +11056,8 @@
"repository": 502,
"assignees": [],
"labels": [
- 71,
- 73
+ 73,
+ 71
]
}
},
@@ -10711,10 +11066,11 @@
"pk": 373,
"fields": {
"nest_created_at": "2024-09-11T20:09:44.344Z",
- "nest_updated_at": "2024-09-11T20:09:44.344Z",
+ "nest_updated_at": "2024-09-13T15:17:02.857Z",
"node_id": "I_kwDOEAWEP86BdDcU",
"title": "Conform to OCSF schema",
"body": "**Describe what problem your feature request solves**:\r\nThe Open Cybersecurity Schema Framework ([OCSF](https://schema.ocsf.io/1.1.0/)) provides categories to organize event classes, which can be consumed by various operational monitoring tools.\r\n\r\n**Describe the solution you'd like**:\r\nProvide fields in the threat that conform to OCSF schema [Vulnerability Details object](https://schema.ocsf.io/1.1.0/objects/vulnerability) which is part of the [Vulnerability Finding Class](https://schema.ocsf.io/1.1.0/classes/vulnerability_finding)\r\n\r\n**Additional context**:\r\n\r\n",
+ "summary": "Conform to the OCSF schema by implementing fields in the threat data that adhere to the OCSF Vulnerability Details object. This enhancement will enable better organization and categorization of event classes, facilitating integration with various operational monitoring tools.\n\n1. Review the OCSF schema documentation, specifically the [Vulnerability Details object](https://schema.ocsf.io/1.1.0/objects/vulnerability) and the [Vulnerability Finding Class](https://schema.ocsf.io/1.1.0/classes/vulnerability_finding).\n2. Identify the necessary fields from the Vulnerability Details object that need to be implemented in the existing threat data structure.\n3. Modify the data model to include these fields, ensuring compatibility with the OCSF schema.\n4. Test the changes to ensure that the new fields are correctly populated and formatted according to the OCSF specifications.\n5. Update documentation to reflect the new schema conformance and provide examples of the updated threat data structure.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/904",
@@ -10722,15 +11078,16 @@
"sequence_id": 2171877140,
"is_locked": false,
"lock_reason": "",
- "comments_count": 0,
+ "comments_count": 1,
"closed_at": null,
"created_at": "2024-03-06T16:08:53Z",
- "updated_at": "2024-03-06T16:08:54Z",
+ "updated_at": "2024-09-13T07:01:18Z",
"author": 43,
"repository": 502,
"assignees": [],
"labels": [
- 71
+ 71,
+ 1115
]
}
},
@@ -10743,6 +11100,7 @@
"node_id": "I_kwDOEAWEP86Bdofc",
"title": "Running threat dragon behind nginx",
"body": "**Describe the bug**:\r\nWhen deploying Threat Dragon to Kubernetes, I can't get the Github Oauth to work. I guess it could be a nginx config needed?\r\n\r\n**Expected behaviour**:\r\nLogin with Github Oauth should work behind a proxy.\r\n\r\n**Logs**:\r\n\r\n```\r\nerror: controllers/auth.js: {\"service\":\"threat-dragon\",\"timestamp\":\"2024-03-06 17:05:09\"}\r\nerror: Unexpected token ' in JSON at position 0 {\"service\":\"threat-dragon\",\"stack\":\"SyntaxError: Unexpected token ' in JSON at position 0\\n at JSON.parse ()\\n at getPrimaryKey (/app/td.server/dist/helpers/encryption.helper.js:23:19)\\n at Object.encryptPromise (/app/td.server/dist/helpers/encryption.helper.js:99:13)\\n at _callee$ (/app/td.server/dist/helpers/jwt.helper.js:21:47)\\n at tryCatch (/app/td.server/node_modules/@babel/runtime/helpers/regeneratorRuntime.js:45:16)\\n at Generator. (/app/td.server/node_modules/@babel/runtime/helpers/regeneratorRuntime.js:133:17)\\n at Generator.next (/app/td.server/node_modules/@babel/runtime/helpers/regeneratorRuntime.js:74:21)\\n at asyncGeneratorStep (/app/td.server/node_modules/@babel/runtime/helpers/asyncToGenerator.js:3:24)\\n at _next (/app/td.server/node_modules/@babel/runtime/helpers/asyncToGenerator.js:22:9)\\n at /app/td.server/node_modules/@babel/runtime/helpers/asyncToGenerator.js:27:7\\n at new Promise ()\\n at Object. (/app/td.server/node_modules/@babel/runtime/helpers/asyncToGenerator.js:19:12)\\n at Object.createAsync (/app/td.server/dist/helpers/jwt.helper.js:49:17)\\n at _callee$ (/app/td.server/dist/controllers/auth.js:55:42)\\n at tryCatch (/app/td.server/node_modules/@babel/runtime/helpers/regeneratorRuntime.js:45:16)\\n at Generator. (/app/td.server/node_modules/@babel/runtime/helpers/regeneratorRuntime.js:133:17)\",\"timestamp\":\"2024-03-06 17:05:09\"}\r\nerror: undefined {\"service\":\"threat-dragon\",\"timestamp\":\"2024-03-06 17:05:09\"}\r\n```\r\n",
+ "summary": "Investigate GitHub OAuth issue in Threat Dragon behind Nginx. \n\n1. Review Nginx configuration to ensure proper handling of OAuth callbacks. Confirm that the proxy settings are correctly forwarding requests, especially the Authorization header and any required cookies.\n\n2. Check the application's environment variables related to GitHub OAuth (e.g., `GITHUB_CLIENT_ID`, `GITHUB_CLIENT_SECRET`, `GITHUB_CALLBACK_URL`). Ensure they are correctly set and match the settings in the GitHub OAuth application.\n\n3. Analyze error logs for JSON parsing issues. Specifically, look at the error `Unexpected token ' in JSON at position 0`. This suggests malformed JSON is being processed. Trace back to where the JSON is generated or received to identify potential issues.\n\n4. Test the OAuth flow directly without Nginx to determine if the problem lies with the application or the proxy configuration.\n\n5. If issues persist, enable detailed logging in both Threat Dragon and Nginx to capture more information during the OAuth flow.\n\n6. Consult Threat Dragon and Nginx documentation for additional configuration settings that may be necessary for OAuth to function correctly behind a proxy. \n\nStart with validating Nginx settings and examining the application's environment variables for correctness.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/905",
@@ -10758,8 +11116,8 @@
"repository": 502,
"assignees": [],
"labels": [
- 73,
- 88
+ 88,
+ 73
]
}
},
@@ -10772,6 +11130,7 @@
"node_id": "I_kwDOEAWEP86BspRR",
"title": "GITHUB_ENTERPRISE_PORT incorrect placement due to bug in octonode",
"body": "**Describe the bug**:\r\nThreat Dragon is improperly constructing GitHub Enterprise (GHE) URLs (after initial OAuth login), leading to `404` errors on GHE, with Threat Dragon then returning `500` errors.\r\n\r\nIt appears to start here:\r\n- https://github.com/OWASP/threat-dragon/blob/622338453c5dca4d01aa78646d1a9f382f3a0e8b/td.server/src/repositories/githubrepo.js#L9-L10\r\n\r\nThe inclusion of both the base path (`/api/v3`) and the `port` when constructing the URL for octonode causes the URL to be invalid.\r\n\r\nUsing `github.company.com` as the example...\r\n\r\n- this is what `enterpriseOpts` is set to, then:\r\n\r\n`enterpriseOpts { hostname: 'github.company.com/api/v3', port: 443, protocol: 'https' }`\r\n\r\n- So, then in [Octonode](https://github.com/pksunkara/octonode/blob/v0.10.2/lib/octonode/client.js#L221-L229):\r\n\r\n```javascript\r\n _url = url.format({\r\n protocol: urlFromPath.protocol || this.options && this.options.protocol || \"https:\",\r\n auth: urlFromPath.auth || (this.token && this.token.username && this.token.password ? this.token.username + \":\" + this.token.password : ''),\r\n hostname: urlFromPath.hostname || this.options && this.options.hostname || \"api.github.com\",\r\n port: urlFromPath.port || this.options && this.options.port,\r\n pathname: urlFromPath.pathname,\r\n query: query\r\n });\r\n```\r\n\r\n- and `this.options.hostname` is `http://github.company.com/api/v3`, so `_url` ends up being\r\n`https://github.company.com/api/v3:443/user`\r\n\r\n**Expected behaviour**:\r\nThreat Dragon should properly construct the GHE URL.\r\n\r\n**Environment**:\r\n\r\n- Version: v2.2.0\r\n- Platform: Web App\r\n- OS: Linux\r\n- Browser: N/A\r\n\r\n**To Reproduce**:\r\n- Enable use of GitHub Enterprise by setting `GITHUB_ENTERPRISE_HOSTNAME` in Threat Dragon's env\r\n- Login with GitHub in Threat Dragon UI\r\n- OAuth flow completes, but fetching `/api/v3/user` returns an error\r\n- Observe `td.server` logs, displaying error similar to below:\r\n\r\n```\r\nerror: Not Found {\r\n \"body\": {\r\n \"documentation_url\": \"https://docs.github.com/enterprise-server@3.8/rest\",\r\n \"message\": \"Not Found\"\r\n },\r\n \"headers\": {\r\n \"access-control-allow-origin\": \"*\",\r\n \"access-control-expose-headers\": \"ETag, Link, Location, Retry-After, X-GitHub-OTP, X-RateLimit-Limit, X-RateLimit-Remaining, X-RateLimit-Used, X-RateLimit-Resource, X-RateLimit-Reset, X-OAuth-Scopes, X-Accepted-OAuth-Scopes, X-Poll-Interval, X-GitHub-Media-Type, X-GitHub-SSO, X-GitHub-Request-Id, Deprecation, Sunset\",\r\n \"connection\": \"close\",\r\n \"content-length\": \"96\",\r\n \"content-security-policy\": \"default-src 'none'\",\r\n \"content-type\": \"application/json; charset=utf-8\",\r\n \"date\": \"Fri, 08 Mar 2024 12:18:19 GMT\",\r\n \"referrer-policy\": \"origin-when-cross-origin, strict-origin-when-cross-origin\",\r\n \"server\": \"nginx/1.14.2\",\r\n \"strict-transport-security\": \"max-age=31536000; includeSubdomains\",\r\n \"x-accepted-oauth-scopes\": \"repo\",\r\n \"x-content-type-options\": \"nosniff\",\r\n \"x-frame-options\": \"deny\",\r\n \"x-github-enterprise-version\": \"3.8.11\",\r\n \"x-github-media-type\": \"github.v3; format=json\",\r\n \"x-github-request-id\": \"8527aaa6-62b5-460d-aebc-a0093eadff93\",\r\n \"x-oauth-client-id\": \"b72b631276646ddd4635\",\r\n \"x-oauth-scopes\": \"repo\",\r\n \"x-ratelimit-limit\": \"14000\",\r\n \"x-ratelimit-remaining\": \"13999\",\r\n \"x-ratelimit-reset\": \"1709903899\",\r\n \"x-ratelimit-resource\": \"core\",\r\n \"x-ratelimit-used\": \"1\",\r\n \"x-runtime-rack\": \"0.031289\",\r\n \"x-xss-protection\": \"0\"\r\n },\r\n \"service\": \"threat-dragon\",\r\n \"statusCode\": 404,\r\n \"timestamp\": \"2024-03-08 07:18:19\"\r\n}\r\n```\r\n\r\n- Checking the GHE side, we see that the URL is actually:\r\n`https://github.company.com/api/v3:443/user`",
+ "summary": "Fix the incorrect construction of GitHub Enterprise (GHE) URLs in Threat Dragon due to a bug in octonode. The issue arises from combining the base path (`/api/v3`) with the hostname, resulting in malformed URLs such as `https://github.company.com/api/v3:443/user`, leading to `404` errors on GHE and subsequent `500` errors in Threat Dragon.\n\n**Steps to tackle the problem:**\n\n1. **Identify the Source of the Bug:**\n - Review the code in `githubrepo.js`, specifically lines 9-10, where `enterpriseOpts` is constructed. Ensure the `hostname` does not include the base path.\n\n2. **Modify `enterpriseOpts`:**\n - Adjust the construction of `enterpriseOpts` to separate the `hostname` from the API version. For instance, set `hostname` to `github.company.com` and handle the API version separately.\n\n3. **Verify URL Construction in Octonode:**\n - Examine how octonode constructs the URL in `client.js` (lines 221-229). Ensure that the `hostname` and `port` are set correctly without the base path. \n\n4. **Test the Fix:**\n - Enable GitHub Enterprise in Threat Dragon and perform the OAuth login to verify that the constructed URL is now valid (e.g., `https://github.company.com/user` instead of using the incorrect format).\n\n5. **Update Documentation:**\n - Document the expected format of `GITHUB_ENTERPRISE_HOSTNAME` in Threat Dragon's environment settings to prevent future issues.\n\nBy implementing these steps, ensure Threat Dragon properly constructs GHE URLs and resolves the current errors.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/907",
@@ -10789,8 +11148,8 @@
157
],
"labels": [
- 75,
- 88
+ 88,
+ 75
]
}
},
@@ -10799,10 +11158,11 @@
"pk": 376,
"fields": {
"nest_created_at": "2024-09-11T20:09:49.356Z",
- "nest_updated_at": "2024-09-11T20:09:49.356Z",
+ "nest_updated_at": "2024-09-13T03:53:47.416Z",
"node_id": "I_kwDOEAWEP86E-6UZ",
"title": "Optional entity properties are not shown in reports",
"body": "**Describe what problem your feature request solves**:\r\n\r\nSome of the optional entity properties (such as `protocol`, `isEncrypted`, `isPublicNetwork`) can be edited in the diagram editing page but they do not appear in the report page.\r\n\r\n**Describe the solution you'd like**:\r\n\r\nEntity properties are listed with the entity, perhaps between the description and the list of threats.\r\n\r\n**Additional context**:\r\n\r\nHere's a screenshot of something I hacked together showing the properties of one of the data flow entities of the V2 demo model:\r\n\r\n\r\n\r\nIf it looks promising, I can finish it off and open a pull request.",
+ "summary": "Implement a feature to display optional entity properties in reports. \n\nIdentify the missing optional properties, such as `protocol`, `isEncrypted`, and `isPublicNetwork`, that are editable on the diagram editing page but not shown in the report. Modify the report generation logic to include these properties, positioning them between the entity description and the list of threats.\n\nBegin by:\n\n1. Reviewing the current report generation code to locate where entity properties are fetched and displayed.\n2. Adding logic to retrieve and display the optional properties for each entity.\n3. Testing the changes to ensure that the new properties are correctly rendered in the report.\n4. Creating a pull request with the updates for further review and integration. \n\nConsider user interface implications and ensure that the report remains clear and informative with the added details.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/937",
@@ -10813,17 +11173,15 @@
"comments_count": 2,
"closed_at": null,
"created_at": "2024-04-08T12:33:50Z",
- "updated_at": "2024-08-23T09:29:52Z",
+ "updated_at": "2024-09-12T07:51:58Z",
"author": 158,
"repository": 502,
- "assignees": [
- 158
- ],
+ "assignees": [],
"labels": [
71,
- 80,
84,
- 87
+ 87,
+ 80
]
}
},
@@ -10836,6 +11194,7 @@
"node_id": "I_kwDOEAWEP86GGHeM",
"title": "server CSP",
"body": "**Describe what problem your feature request solves**:\r\nThe server needs to implement a content security policy\r\n\r\n**Describe the solution you'd like**:\r\nuse the package [express-csp-header](https://www.npmjs.com/package/express-csp-header)\r\nor alternatively extend helmet: `td.server/src/config/securityheaders.config.js`\r\n\r\n**Additional context**:\r\nIt would be good to add NONCE\r\nSome incomplete work was done to add CSP in pull request #1040 \r\n",
+ "summary": "Implement a Content Security Policy (CSP) for the server. Utilize the `express-csp-header` package or enhance the existing security headers configuration in `td.server/src/config/securityheaders.config.js` with CSP features. \n\n1. Assess the current state of CSP implementation from pull request #1040.\n2. Choose between implementing `express-csp-header` or extending Helmet for CSP functionality.\n3. If using `express-csp-header`, install the package and configure it in the server setup, ensuring to define appropriate CSP directives.\n4. If extending Helmet, modify the security headers configuration file to include CSP directives and consider adding a NONCE for inline scripts.\n5. Test the implementation thoroughly to ensure that it meets the desired security standards and does not break existing functionalities.",
"state": "open",
"state_reason": "reopened",
"url": "https://github.com/OWASP/threat-dragon/issues/950",
@@ -10851,8 +11210,8 @@
"repository": 502,
"assignees": [],
"labels": [
- 71,
- 80
+ 80,
+ 71
]
}
},
@@ -10865,6 +11224,7 @@
"node_id": "I_kwDOEAWEP86G4-d-",
"title": "Implement mousewheel button dragging for graph panning",
"body": "**Describe what problem your feature request solves**:\r\nThe current graph panning method requires a combination of keyboard and mouse inputs, and mousewheel button is redundant with left mouse button.\r\n\r\n**Describe the solution you'd like**:\r\nUsers should be able to press down the mousewheel button and move the mouse to pan around the graph.",
+ "summary": "Implement mousewheel button dragging for graph panning. \n\n**Problem to solve**: Eliminate the need for combining keyboard and mouse inputs for graph panning, as the current method is cumbersome and the mousewheel button is underutilized.\n\n**Proposed solution**: Enable users to click and hold the mousewheel button to drag and pan the graph. \n\n**Technical details**:\n1. Capture the mousewheel button press event.\n2. Track mouse movement while the button is pressed.\n3. Update the graph's view based on the relative mouse movement.\n4. Ensure smooth panning experience, possibly by implementing a throttling mechanism to limit the update frequency.\n\n**First steps**:\n1. Modify the event listener to include mousewheel button down and up events.\n2. Implement logic to track mouse position changes while the button is held down.\n3. Adjust the graph rendering logic to respond to these position changes accordingly.\n4. Test functionality across different browsers and devices to ensure compatibility.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/954",
@@ -10880,9 +11240,9 @@
"repository": 502,
"assignees": [],
"labels": [
- 71,
+ 83,
75,
- 83
+ 71
]
}
},
@@ -10895,6 +11255,7 @@
"node_id": "I_kwDOEAWEP86Jqphs",
"title": "Translate to Farsi",
"body": "**Describe what problem your feature request solves**:\r\nIt would be good to have the translation to Farsi\r\n\r\n**Describe the solution you'd like**:\r\nTranslate into a new file using the pull request #925 'Vue I18n English Localization to Malay (MS)' as an example of what files need to be modified\r\n\r\n**Additional context**:\r\n\r\n",
+ "summary": "Translate the feature request into Farsi. \n\n**Problem to Solve**: Provide Farsi translation for better accessibility and user experience.\n\n**Proposed Solution**: Create a new file for Farsi translation. Use pull request #925, which details the translation of Vue I18n English Localization to Malay (MS), as a reference for identifying necessary file modifications.\n\n**Next Steps**:\n1. Review pull request #925 to understand the file structure and changes made.\n2. Identify the original language files that require translation to Farsi.\n3. Create a new Farsi localization file based on the identified files.\n4. Implement the translations, ensuring adherence to localization standards.\n5. Submit a pull request with your changes for review.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/962",
@@ -10923,6 +11284,7 @@
"node_id": "I_kwDOEAWEP86K606F",
"title": "Two undo/redo methods do not join up",
"body": "**Describe the bug**:\r\nThere is a pull down menu item which can undo/redo text\r\nand there is also a undo/redo for the components on the diagram\r\n\r\n**Expected behaviour**:\r\nwhen redo/undo is selected from the pull down menu, if the drawing is in focus then the drawing package undo/redo buttons should be applied to the drawing components. If drawing component's attributes are changed then this should have redo/undo applied from the drawing package buttons\r\n\r\n**Environment**:\r\n\r\n- Version: 2.x\r\n- Platform: Desktop App\r\n- OS: any\r\n- Browser: electron\r\n\r\n**To Reproduce**:\r\n\r\n1. open a diagram in the desktop app\r\n2. observe undo/redo from within the drawing package for a component\r\n3. modify the name of the component, observe redo/undo from pull down\r\n4. use undo/redo from within the drawing package and observe node name changing, but not text box\r\n5. observe that pull down menu redo/undo does not apply to movement + changes to drawing components\r\n\r\n**Any additional context, screenshots, etc**:\r\n",
+ "summary": "Fix the disconnect between the two undo/redo methods in the application. The current implementation has separate undo/redo functionalities for text and diagram components, which leads to inconsistent behavior.\n\n1. Ensure that when the drawing area is in focus, the pull-down menu's undo/redo actions apply to the drawing components. \n2. When modifying component attributes (e.g., name changes), ensure that these actions are properly captured and can be undone/redone through both the drawing package buttons and the pull-down menu options.\n\n### Steps to Tackle the Problem:\n\n- **Review Existing Code**: Analyze the current implementations of both undo/redo functionalities to identify how they manage state changes and what triggers their respective actions.\n- **Design Unified Logic**: Create a cohesive logic that links the two undo/redo systems. Investigate how to trigger the drawing package's undo/redo methods when the pull-down menu is used and vice versa.\n- **Implement Event Listeners**: Add event listeners or hooks that detect when an action is performed in one system and trigger the corresponding action in the other.\n- **Test Scenarios**: Create test cases covering various scenarios, such as component modifications, movement actions, and interactions with both undo/redo systems to ensure consistent behavior.\n- **Documentation**: Update the documentation to reflect the new behavior and guide users on how to use the unified undo/redo functionality effectively. \n\nThis will enhance the user experience by ensuring that changes made in the drawing components are consistently managed across both methods.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/972",
@@ -10941,8 +11303,8 @@
],
"labels": [
80,
- 87,
- 88
+ 88,
+ 87
]
}
},
@@ -10955,6 +11317,7 @@
"node_id": "I_kwDOEAWEP86LNCuo",
"title": "Allow user to specify a commit message to be used",
"body": "**Describe what problem your feature request solves**:\r\n\"Created by OWASP Threat Dragon\" and \"Updated by OWASP Threat Dragon\" are fine for playing around, but are not enough for a real production use.\r\n\r\n**Describe the solution you'd like**:\r\nI would like to be able to write a commit message myself while saving changes in TD. The feature should be disabled by default but has 2 modi if activated via ENV: `enabled` (you can write your own message) and `forced` (you have to write your own message).\r\n",
+ "summary": "Implement a feature to allow users to specify custom commit messages when saving changes in OWASP Threat Dragon.\n\n1. Identify the current commit message generation logic in the codebase.\n2. Introduce an environment variable (ENV) to control the feature:\n - Set the default to disabled.\n - Implement two modes: \n - `enabled`: Allow users to input their own commit messages.\n - `forced`: Mandate that users provide a custom commit message.\n3. Create a user interface element (e.g., a text input field) for entering custom commit messages.\n4. Update the save functionality to include the user-defined commit message when committing changes.\n5. Ensure backward compatibility with the existing commit message system.\n6. Write unit tests to validate the new functionality and modes.\n7. Document the new feature in the README or relevant documentation files. \n\nStart by reviewing the existing saving and commit message code to understand how to integrate the new feature effectively.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/973",
@@ -10970,8 +11333,8 @@
"repository": 502,
"assignees": [],
"labels": [
- 71,
- 73
+ 73,
+ 71
]
}
},
@@ -10984,6 +11347,7 @@
"node_id": "I_kwDOEAWEP86Pi4OO",
"title": "Add new PLOT4ai diagram components",
"body": "**Describe what problem your feature request solves**:\r\nPLOT4ai has a need for other diagram components as well as the existing Process, Acotr and Store components\r\n\r\n**Describe the solution you'd like**:\r\nIf the diagram is of type 'PLOT4ai' then two new component types become available for the diagram, 'Model' and 'Result'\r\n\r\n**Additional context**:\r\n\r\nImage taken from a presentation at ThreatModCon 2024 Lisbon:\r\n\r\n\r\n",
+ "summary": "Add new components 'Model' and 'Result' to PLOT4ai diagrams. \n\n1. Identify the existing diagram structure in the PLOT4ai codebase, focusing on how components like Process, Actor, and Store are defined and rendered.\n2. Implement the logic for new component types 'Model' and 'Result' to ensure they integrate seamlessly with the current diagram functionality.\n3. Update the diagram type check to enable these new components only when the diagram type is 'PLOT4ai'.\n4. Create necessary UI elements for adding and manipulating these new components within the diagram interface.\n5. Test the integration to confirm that the new components function correctly and do not introduce any regressions. \n\nRefer to the presentation from ThreatModCon 2024 for additional context and design inspiration.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/1018",
@@ -11001,9 +11365,9 @@
150
],
"labels": [
- 71,
73,
- 83
+ 83,
+ 71
]
}
},
@@ -11016,6 +11380,7 @@
"node_id": "I_kwDOEAWEP86P1TUl",
"title": "PDF generation from web app",
"body": "**Describe what problem your feature request solves**:\r\nThe desktop app allows saving the report as PDF.\r\nFor the web app, I only found the option to print the report.\r\n\r\nIt would be nice to have a \"download PDF\" option in the web app.\r\n\r\n**Describe the solution you'd like**:\r\nNext to the \"print\" button could be a \"download\" button that auto generates and downloads a PDF file.\r\n",
+ "summary": "Implement a \"download PDF\" feature in the web app to enhance user experience. This feature should allow users to generate and download reports as PDF files, similar to the functionality available in the desktop app.\n\n1. Investigate existing print functionality to understand the current implementation.\n2. Choose a PDF generation library compatible with the web app's technology stack (e.g., jsPDF, html2pdf.js).\n3. Add a \"download\" button next to the \"print\" button in the report interface.\n4. Write a function that captures the report content and converts it to a PDF format using the selected library.\n5. Trigger the PDF generation when the \"download\" button is clicked and initiate the download process.\n6. Test the PDF output to ensure it matches the report format and retains all necessary data.\n\nBy following these steps, you can successfully implement the requested feature for the web application.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/1022",
@@ -11044,6 +11409,7 @@
"node_id": "I_kwDOEAWEP86QE7bD",
"title": "apply for Best Practices badge",
"body": "**Describe what problem your feature request solves**:\r\nthe OpenSSF run a [Best Practices Badge Program](https://www.bestpractices.dev/en), it is very likely that Threat Dragon follows these best practices\r\n\r\n**Describe the solution you'd like**:\r\nInspect the criteria for [gaining the badge](https://www.bestpractices.dev/en/criteria/0) and apply\r\n\r\n**Additional context**:\r\nConsider the OWASP Project Committee [Good Practice](https://owasp.org/www-committee-project/#div-practice)\r\nConsider linking directly to OWASP from the initial home page\r\nConsider applying for [OWASP Production status](https://owasp.org/www-committee-project/#div-promotions)\r\n",
+ "summary": "Apply for the Best Practices badge for Threat Dragon by following these steps:\n\n1. **Inspect Badge Criteria**: Review the criteria outlined for the Best Practices Badge on the official [Best Practices website](https://www.bestpractices.dev/en/criteria/0). Ensure that Threat Dragon adheres to these standards.\n\n2. **Perform Assessment**: Conduct a thorough assessment of Threat Dragon against the criteria. Document areas of compliance and identify any gaps that need addressing.\n\n3. **Implement Improvements**: Address any identified gaps to align Threat Dragon with the best practices. Focus on coding standards, security measures, documentation, and community engagement as specified in the criteria.\n\n4. **Link OWASP Resources**: Consider incorporating direct links to OWASP resources from Threat Dragon’s homepage to enhance visibility and adherence to security best practices.\n\n5. **Apply for OWASP Production Status**: Explore the requirements for achieving OWASP Production status. Prepare necessary documentation and improvements to meet these requirements.\n\n6. **Submit Application**: Once all criteria are met, formally submit an application for the Best Practices badge through the official process outlined on the Best Practices website.\n\nBy following these steps, enhance Threat Dragon’s credibility and ensure it meets industry standards for security and best practices.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/1027",
@@ -11073,6 +11439,7 @@
"node_id": "I_kwDOEAWEP86RGrZY",
"title": "Canvas reverts to small view area on save",
"body": "**Describe the bug**:\r\nWhen a diagram is saved, Antv/X6 drawing package reverts the canvas size to the size of the container view \r\nThe user is left with a confusing diagram and has to drag components to get them back into view\r\nPossible that updating to antv/x6 version 2.x will solve this, see #722 \r\n\r\n**Expected behaviour**:\r\nwhen opening a diagram, the canvas size and viewing area should match the previous sizes\r\n\r\n**Environment**:\r\n\r\n- Version: after commit 6b8f3e3\r\n- Platform: both Web App and Desktop App\r\n- OS: all of MacOS / Windows / Linux\r\n- Browser: all of chrome, firefox, safari\r\n\r\n**To Reproduce**:\r\n\r\n1. Open the example diagram\r\n2. drag a component so that the diagram canvas resizes\r\n3. save the diagram\r\n4. only a portion of the canvas is now displayed\r\n\r\n**Any additional context, screenshots, etc**:\r\n\r\nThe canvas size needs to be saved and restored when redrawn. Here is debug:\r\n\r\n```\r\ntd.vue/src/service/x6/graph/events.js\r\n@@ -8,6 +8,10 @@ import { CELL_SELECTED, CELL_UNSELECTED } from '@/store/actions/cell.js';\r\n import { THREATMODEL_MODIFIED } from '@/store/actions/threatmodel.js';\r\n import shapes from '@/service/x6/shapes/index.js';\r\n \r\n+const canvasResized = ({ width, height }) => {\r\n+ console.debug('canvas resized to width ', width, ' height ', height);\r\n+};\r\n+\r\n const edgeConnected = ({ isNew, edge }) => {\r\n\r\n@@ -142,6 +146,7 @@ const nodeAddFlow = (graph) => ({ node }) => {\r\n };\r\n \r\n const listen = (graph) => {\r\n+ graph.on('resize', canvasResized);\r\n graph.on('edge:connected', edgeConnected);\r\n\r\n@@ -157,6 +162,7 @@ const listen = (graph) => {\r\n };\r\n \r\n const removeListeners = (graph) => {\r\n+ graph.off('resize', canvasResized);\r\n graph.off('edge:connected', edgeConnected);\r\n```\r\n",
+ "summary": "Reproduce the bug where the canvas reverts to a small view area upon saving a diagram using the Antv/X6 drawing package. \n\n**Steps to tackle the problem**:\n\n1. **Analyze the current behavior**:\n - Confirm that when a diagram is saved, the canvas size reverts to the container's size instead of retaining the user's modifications.\n\n2. **Check the version**:\n - Consider updating Antv/X6 to version 2.x as indicated in issue #722. Ensure that this version addresses the canvas resizing issue.\n\n3. **Modify the event listeners**:\n - Investigate the event handling in `td.vue/src/service/x6/graph/events.js`.\n - Implement logic to save the canvas size before saving the diagram. This might involve adding a new listener that captures the canvas dimensions whenever it is resized.\n\n4. **Implement canvas size restoration**:\n - After saving the diagram, ensure that the previously saved canvas size is restored when the diagram is reloaded. This may involve adjusting the state management or storage mechanism for the diagram's properties.\n\n5. **Test across environments**:\n - Verify the solution on all specified platforms (MacOS, Windows, Linux) and browsers (Chrome, Firefox, Safari) to ensure consistent behavior.\n\n6. **Document the changes**:\n - Update the codebase and document the changes made to handle canvas resizing and restoration for future reference and maintenance.\n\nFollowing these steps should lead to a resolution of the canvas resizing issue after saving diagrams.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/1041",
@@ -11090,11 +11457,11 @@
43
],
"labels": [
- 88,
- 92,
- 87,
80,
- 83
+ 83,
+ 87,
+ 88,
+ 92
]
}
},
@@ -11107,6 +11474,7 @@
"node_id": "I_kwDOEAWEP86T0tja",
"title": "PDF Report dialog does not show existing PDF files",
"body": "**Describe the bug**:\r\n\r\nExisting PDF files are not shown in file save dialog (probably wrong file type filter, i.e., two dots before the PDF extension).\r\n\r\n**Expected behaviour**:\r\n\r\nExisting PDF files are shown in file save dialog.\r\n\r\n**Environment**:\r\nVersion 2.2.0\r\nDesktop App\r\nWindows 11\r\n\r\n**To Reproduce**:\r\n\r\nExport PDF file into directory which contains PDF files.\r\n\r\n**Any additional context, screenshots, etc**:\r\n\r\n\r\n\r\n",
+ "summary": "Fix the PDF report dialog to display existing PDF files. The issue stems from a likely misconfiguration in the file type filter, which may be using an incorrect format (e.g., two dots before the PDF extension).\n\n**Steps to address the issue:**\n\n1. Investigate the file type filter settings in the PDF export dialog.\n2. Correct the file extension filter for PDF files to ensure it properly recognizes existing files (e.g., change from `..pdf` to `.pdf`).\n3. Test the file dialog after making changes to confirm that existing PDF files are displayed correctly.\n4. Review the code for other file type filters to ensure consistency and prevent similar issues.\n\n**Check environment:**\n- Confirm the bug exists in version 2.2.0 on Windows 11. \n\n**Document findings and update the issue tracker with progress.**",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/1062",
@@ -11123,8 +11491,8 @@
"assignees": [],
"labels": [
80,
- 84,
- 88
+ 88,
+ 84
]
}
},
@@ -11137,6 +11505,7 @@
"node_id": "I_kwDOEAWEP86T0wOO",
"title": "\"Reason for out of scope\" missing in PDF report",
"body": "**Describe the bug**:\r\n\r\nPDF report does not contain \"Reason for out of scope\" for \"Out of Scope\" elements.\r\n\r\n**Expected behaviour**:\r\n\r\nPDF report contains \"Reason for out of scope\" which is important for documentation purposes.\r\n\r\n**Environment**:\r\n\r\n- Version: 2.2\r\n- Platform: Desktop App\r\n- OS: Windows 11\r\n\r\n**To Reproduce**:\r\n\r\nHave element in DFD with \"Out of Scope\" checkbox checked and \"Reason for out of scope\" filled.\r\nDo PDF export.\r\n\r\n**Any additional context, screenshots, etc**:\r\n\r\n\r\n",
+ "summary": "Add \"Reason for out of scope\" to PDF report for \"Out of Scope\" elements. \n\n1. Identify the section in the PDF generation code where report data is compiled.\n2. Check if the \"Reason for out of scope\" field is being retrieved from the data model.\n3. Ensure this field is included in the data being passed to the PDF generation function.\n4. Update the PDF template to accommodate and display the \"Reason for out of scope\" appropriately.\n5. Test the PDF export functionality after implementing changes to verify that the reason appears as expected.\n\nReview the PDF generation library to confirm it supports the necessary formatting for the new content. Ensure compatibility with version 2.2 on Windows 11 desktop app.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/1063",
@@ -11153,8 +11522,8 @@
"assignees": [],
"labels": [
80,
- 84,
- 88
+ 88,
+ 84
]
}
},
@@ -11167,6 +11536,7 @@
"node_id": "I_kwDOEAWEP86T1sB7",
"title": "Allow resizing of canvas area",
"body": "**Describe what problem your feature request solves**:\r\n\r\nI cannot increase the canvas size. I can only increase the app's window size.\r\n\r\n**Environment**\r\nVersion 2.2\r\nWindows 11\r\nDesktop App\r\n4K Monitor\r\n\r\n**Describe the solution you'd like**:\r\n\r\nI want to be able to increase the canvas size to have a larger area for modeling.\r\n\r\n**Additional context**:\r\n\r\n\r\n",
+ "summary": "Implement a feature to allow resizing of the canvas area within the application. \n\n**Problem to Solve**: The current limitation prevents users from increasing the canvas size, restricting modeling space despite the ability to resize the app's window. This is particularly problematic for users working on 4K monitors who require more modeling area for detailed work.\n\n**Proposed Solution**: Enable users to adjust the canvas size independently from the application window size. This could involve adding a resize handle or a settings option to specify canvas dimensions.\n\n**Technical Steps**:\n1. Investigate the existing canvas rendering logic to identify where size constraints are applied.\n2. Modify the canvas initialization parameters to accept dynamic width and height inputs.\n3. Implement event listeners for mouse or touch events to allow users to drag to resize the canvas.\n4. Ensure the canvas can maintain aspect ratio or allow for freeform resizing based on user preferences.\n5. Update the application's UI to reflect the new canvas size and ensure compatibility with existing features.\n6. Test the resizing feature on various resolutions and screen sizes, particularly focusing on 4K displays.\n\nStart by reviewing the canvas rendering code and identifying the current limitations in size settings.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/1064",
@@ -11182,8 +11552,8 @@
"repository": 502,
"assignees": [],
"labels": [
- 71,
- 73
+ 73,
+ 71
]
}
},
@@ -11196,6 +11566,7 @@
"node_id": "I_kwDOEAWEP86T1vq7",
"title": "Improve Drag and Drop of Components",
"body": "**Describe what problem your feature request solves**:\r\n\r\nI have to select the _text_ of a component in the components view in order to drag and drop it into the canvas.\r\nIt is not possible to drag and drop by clicking other parts of the component.\r\n\r\n**Environment**\r\nVersion 2.2\r\nWindows 11\r\nDesktop App\r\n4K Monitor\r\n\r\n**Describe the solution you'd like**:\r\n\r\nI want to select any part of the component for drag and drop.\r\n\r\n**Additional context**:\r\n\r\n\r\n\r\n",
+ "summary": "Improve the drag and drop functionality for components in the desktop app. Currently, users must click on the text of a component in the components view to initiate a drag and drop action. This limitation hinders usability, as users should be able to click anywhere on the component to perform the drag and drop.\n\n**Technical Details:**\n- Examine the current event listeners associated with the component's drag-and-drop functionality.\n- Identify the areas in the UI where click events are registered and modify them to include the entire area of the component, not just the text.\n- Ensure that the drag-and-drop behavior is consistent across different components and does not interfere with other UI interactions.\n\n**First Steps:**\n1. Review the existing code handling the drag-and-drop feature, focusing on event listeners for mouse interactions.\n2. Implement a test case that allows for dragging from different parts of the component.\n3. Conduct usability testing to ensure that the improved functionality meets user expectations.\n4. Document the changes made and update any relevant UI guidelines.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/1065",
@@ -11211,9 +11582,9 @@
"repository": 502,
"assignees": [],
"labels": [
- 71,
73,
- 83
+ 83,
+ 71
]
}
},
@@ -11222,10 +11593,11 @@
"pk": 390,
"fields": {
"nest_created_at": "2024-09-11T20:10:18.569Z",
- "nest_updated_at": "2024-09-11T20:10:18.569Z",
+ "nest_updated_at": "2024-09-13T16:57:38.012Z",
"node_id": "I_kwDOEAWEP86T1yQ7",
"title": "Add keyboard shortcut for save [CTRL + S]",
"body": "**Describe what problem your feature request solves**:\r\n\r\nI have to use the UI button in order to save.\r\n\r\n**Describe the solution you'd like**:\r\n\r\nI would like to simply hit CTRL + S.\r\n\r\n**Additional context**:\r\n\r\n\r\n\r\n",
+ "summary": "Implement a keyboard shortcut for saving files using CTRL + S. \n\n**Problem**: Users are currently required to click a UI button to save, which is inefficient and disrupts workflow.\n\n**Solution**: Enable the CTRL + S keyboard shortcut to trigger the save functionality.\n\n**Technical Details**:\n1. Identify the area of the codebase where the save function is implemented.\n2. Create an event listener for keyboard events that detects when CTRL + S is pressed.\n3. Prevent the default action of the event to avoid browser behavior (like opening the save dialog).\n4. Call the existing save function when the shortcut is activated.\n\n**First Steps**:\n1. Review the existing save function implementation.\n2. Locate the appropriate module or component where keyboard event listeners can be added.\n3. Implement the event listener for CTRL + S.\n4. Test the functionality to ensure it works as intended across different browsers.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/1066",
@@ -11236,10 +11608,12 @@
"comments_count": 1,
"closed_at": null,
"created_at": "2024-08-22T09:56:14Z",
- "updated_at": "2024-08-23T10:02:36Z",
+ "updated_at": "2024-09-13T14:12:50Z",
"author": 162,
"repository": 502,
- "assignees": [],
+ "assignees": [
+ 136
+ ],
"labels": [
71,
80,
@@ -11256,6 +11630,7 @@
"node_id": "I_kwDOEAWEP86T2dE0",
"title": "Improve selection of edges and creation of bendpoints",
"body": "**Describe what problem your feature request solves**:\r\n\r\nCurrently, it does not feel intuitive to select a data flow edge or a trust boundary. I have to select the text or the endpoint in order to access the properties. If i click anywhere else on the line, a bend point is immediately created. Often, I don't want to create a bend point but just select the data flow / trust boundary to edit properties.\r\n\r\n**Describe the solution you'd like**:\r\n\r\n I would prefer a UX similar to Miro. When clicking the edge, the properties are shown. When clicking on the circle between two bend points, a new bend point is created.\r\n\r\n**Additional context**:\r\n\r\n\r\n- In Threat Dragon, clicking on line immediately creates bend point.\r\n\r\n\r\n- In Miro, clicking on line selects the line first and enables to edit properties. Bend points can then be created afterwards.\r\n\r\n\r\n",
+ "summary": "Enhance edge selection and bend point creation in Threat Dragon.\n\n**Identify the problem**: \nModify the current selection mechanism for data flow edges and trust boundaries to improve user experience. Currently, users must click on text or endpoints to access properties, while clicking anywhere else on the line creates an unintended bend point.\n\n**Proposed solution**: \nImplement a selection system similar to Miro. Enable property editing by clicking on the edge itself, while allowing bend points to be added only when clicking on designated areas between bend points.\n\n**Technical details**:\n1. **Event Handling**: Adjust the mouse click event listeners for edge elements to differentiate between selecting the edge and creating bend points.\n2. **Hit Testing**: Implement hit testing to identify if the click is on the edge or a specific area between bend points. Use bounding box calculations to determine click areas.\n3. **UI Feedback**: Provide visual feedback when the edge is selected (e.g., highlight or change cursor) to indicate that the properties can be edited.\n4. **Property Panel**: Ensure that the properties panel updates with the correct edge information when an edge is selected.\n\n**First steps**:\n1. Review the current event handling code for edges and bend points.\n2. Define the clickable areas for edges and bend points in the SVG or Canvas rendering.\n3. Implement hit testing logic to distinguish between a selection and a bend point creation.\n4. Test the new interaction model for usability and functionality across different scenarios.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/1067",
@@ -11271,10 +11646,10 @@
"repository": 502,
"assignees": [],
"labels": [
- 71,
73,
83,
- 87
+ 87,
+ 71
]
}
},
@@ -11287,6 +11662,7 @@
"node_id": "I_kwDOEAWEP86T2fPJ",
"title": "I have to click undo five times to actually undo the action",
"body": "**Describe the bug**:\r\n\r\nWhen creating a data flow by double clicking a process, I have to execute undo five times in order to actually undo the action.\r\n\r\n**Expected behaviour**:\r\n\r\nOnly clicking once should be enough.\r\n\r\n**Environment**:\r\n\r\n- Version: 2.2\r\n- Platform: Desktop App\r\n- OS: Windows 11\r\n\r\n\r\n**To Reproduce**:\r\n\r\n\r\n1. Create Process Element.\r\n2. Double click on it.\r\n3. Click on undo five times.\r\n\r\n**Any additional context, screenshots, etc**:\r\n\r\n\r\n\r\n",
+ "summary": "Investigate the undo functionality in version 2.2 of the desktop app on Windows 11. \n\n**Bug Overview**: \nWhen a user creates a process element and double-clicks it, the undo action requires five clicks to revert the last action, whereas it should only require one click.\n\n**Steps to Tackle the Problem**:\n\n1. **Review Undo Logic**: Examine the code responsible for the undo stack management. Ensure it tracks actions correctly and that each action corresponds to a single undo step.\n \n2. **Debug the Undo Function**: Use debugging tools to trace the execution of the undo function after creating a process element. Check how many actions are being pushed onto the undo stack upon the double-click event.\n\n3. **Check Event Listeners**: Verify if multiple event listeners are attached to the double-click event, leading to multiple actions being recorded.\n\n4. **Test with Different Actions**: Create different process elements using variations (single-click, different elements) to confirm if the issue persists across all scenarios.\n\n5. **Update Documentation**: If a solution is found, update the documentation to reflect the correct usage of the undo function.\n\nImplement these steps to identify and resolve the undo action issue effectively.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/1068",
@@ -11303,8 +11679,8 @@
"assignees": [],
"labels": [
80,
- 83,
- 88
+ 88,
+ 83
]
}
},
@@ -11313,10 +11689,11 @@
"pk": 393,
"fields": {
"nest_created_at": "2024-09-11T20:10:25.378Z",
- "nest_updated_at": "2024-09-11T20:10:25.378Z",
+ "nest_updated_at": "2024-09-13T16:57:40.085Z",
"node_id": "I_kwDOEAWEP86T2iJI",
"title": "Undo does not bring back data flows",
"body": "**Describe the bug**:\r\n\r\nHave two processes connected by data flow. When deleting the process, the data flows are deleted, too. When undoing the action, only the process is brought back. The data flows are gone.\r\n\r\n**Expected behaviour**:\r\n\r\nUndo should bring back all deleted elements.\r\n\r\n**Environment**:\r\n\r\n- Version: 2.2\r\n- Platform: Desktop App\r\n- OS: Windows 11\r\n\r\n**To Reproduce**:\r\n\r\n\r\n1. Create two processes\r\n2. Connect them with data flow\r\n3. Delete one process\r\n4. Click undo\r\n\r\n**Any additional context, screenshots, etc**:\r\n\r\n\r\n\r\n",
+ "summary": "**Fix the Undo Functionality for Data Flows** \n\n**Issue**: When deleting a process connected by data flow, the data flows are also removed. Upon using the undo command, only the process is restored, leaving the data flows missing.\n\n**Expected Behavior**: Ensure that the undo action restores all deleted elements, including processes and their associated data flows.\n\n**Environment Details**:\n- Version: 2.2\n- Platform: Desktop App\n- OS: Windows 11\n\n**Reproduction Steps**:\n1. Create two processes.\n2. Connect the processes with a data flow.\n3. Delete one of the processes.\n4. Click the undo button.\n\n**Action Items**:\n1. Investigate the current implementation of the undo functionality to identify why data flows are not restored.\n2. Modify the undo logic to track and restore both processes and their associated data flows.\n3. Implement unit tests to verify that the undo action behaves as expected in scenarios involving multiple connected elements.\n4. Test the functionality across different scenarios to ensure reliability.\n\n**Next Steps**:\n- Review the current data structure handling for processes and data flows.\n- Design a solution to link data flows to their respective processes to ensure they can be restored together.\n- Document the changes and monitor subsequent user feedback for further improvements.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/1069",
@@ -11324,17 +11701,17 @@
"sequence_id": 2480546376,
"is_locked": false,
"lock_reason": "",
- "comments_count": 3,
+ "comments_count": 4,
"closed_at": null,
"created_at": "2024-08-22T11:28:13Z",
- "updated_at": "2024-08-23T11:47:50Z",
+ "updated_at": "2024-09-13T16:33:40Z",
"author": 162,
"repository": 502,
"assignees": [],
"labels": [
+ 88,
73,
- 83,
- 88
+ 83
]
}
},
@@ -11347,6 +11724,7 @@
"node_id": "I_kwDOEAWEP86UHzLm",
"title": "move threatdragon.com to owasp domain",
"body": "**Describe what problem your feature request solves**:\r\n\r\nAt present we have the demo and docs hosted by heroku at threatdragon.com\r\nIt would be good that this is moved to threatdragon.owasp.org, in a similar way to mas.owasp.org for example\r\n\r\n**Describe the solution you'd like**:\r\nmove to threatdragon.owasp.org\r\n\r\n**Additional context**:\r\nthe index page for mas.owasp.org is here: https://github.com/OWASP/owasp-mastg/blob/master/docs/index.md?plain=1\r\n",
+ "summary": "Move threatdragon.com to the OWASP domain. \n\n**Problem to Solve**: Currently, the demo and documentation for Threat Dragon are hosted on threatdragon.com via Heroku. Migrating to the OWASP domain (threatdragon.owasp.org) will streamline hosting and enhance visibility within the OWASP community, similar to the setup for mas.owasp.org.\n\n**Proposed Solution**: Transition the hosting from threatdragon.com to threatdragon.owasp.org.\n\n**Technical Steps to Tackle the Problem**:\n1. Set up a subdomain for Threat Dragon on the OWASP domain (threatdragon.owasp.org).\n2. Transfer the existing content from threatdragon.com to the new subdomain.\n3. Ensure that all links and references in documentation are updated to point to the new domain.\n4. Configure DNS settings to direct traffic from threatdragon.com to threatdragon.owasp.org.\n5. Verify that all features function properly on the new hosting environment.\n6. Redirect traffic from threatdragon.com to the new domain to maintain access and SEO.\n\n**Additional Context**: Refer to the index page for mas.owasp.org for structure and layout guidance: https://github.com/OWASP/owasp-mastg/blob/master/docs/index.md?plain=1.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/1073",
@@ -11375,6 +11753,7 @@
"node_id": "I_kwDOEAWEP86VwNCQ",
"title": "Migrate to Bootstrap v5.x",
"body": "**Describe what problem your feature request solves**:\r\nThreat Dragon is stuck on Bootstrap version 4.6.1 because of breaking changes going to Bootstrap v5.x\r\nSee https://getbootstrap.com/docs/5.0/migration/\r\n\r\n**Describe the solution you'd like**:\r\nprovide Bootstrap 5.3.3 in file `td.vue/package.json`\r\n\r\n**Additional context**:\r\nMoving to Bootstrap 5.3.3 was tried in commit 37a1734906767b5db265064244222f3ce3c2e90f\r\n",
+ "summary": "Migrate Threat Dragon to Bootstrap v5.x to resolve issues stemming from reliance on Bootstrap 4.6.1 due to breaking changes. \n\n1. Update the `td.vue/package.json` file to include Bootstrap version 5.3.3.\n2. Review the migration guide at https://getbootstrap.com/docs/5.0/migration/ to identify breaking changes and necessary adjustments.\n3. Test the application thoroughly to ensure compatibility and fix any issues arising from the migration.\n\nBegin by creating a branch for the migration, updating the package version, and conducting an initial test to identify immediate breaking changes.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/1080",
@@ -11390,8 +11769,8 @@
"repository": 502,
"assignees": [],
"labels": [
- 87,
- 93
+ 93,
+ 87
]
}
},
@@ -11400,10 +11779,11 @@
"pk": 396,
"fields": {
"nest_created_at": "2024-09-11T20:10:30.404Z",
- "nest_updated_at": "2024-09-12T01:08:50.312Z",
+ "nest_updated_at": "2024-09-13T03:53:49.468Z",
"node_id": "I_kwDOEAWEP86WD248",
"title": "Can't move ends of line objects inside of Trust Boundary frame",
"body": "**Describe the bug**:\r\nWhen line objects (i.e., Data Flow, Trust Boundary lines) are inside of a Trust Boundary frame, manipulation of the line ends causes the manipulated end to be placed at a position other than where the cursor was on release of the left mouse button.\r\n\r\n**Expected behaviour**:\r\nLine ends are placed where the mouse cursor is at the time that the left mouse button is released.\r\n\r\n**Environment**:\r\n\r\n- Version: 2.2.0.0\r\n- Platform: Desktop App\r\n- OS: Windows\r\n\r\n**To Reproduce**:\r\n1) On a STRIDE diagram, drag a Data Flow line to the canvas.\r\n2) Drag a Trust Boundary frame to the canvas and resize it so that the Data Flow line is fully enclosed in the Trust Boundary.\r\n3) Click on one end of the Data Flow line and drag it slightly to another position *within* the Trust Boundary.\r\n4) Note that the Trust Boundary highlights yellow (as if something were being anchored to it) once the line end begins to be repositioned.\r\n5) Note that - upon release of the line end - the end will position somewhere else (it looks like the middle of the frame) within the Trust Boundary.\r\n5) Note that selecting only the Trust Boundary and deleting it will delete both objects.\r\n",
+ "summary": "**Fix the issue with line object manipulation inside Trust Boundary frames**.\n\n**Identify the bug**: When manipulating the ends of line objects within a Trust Boundary frame, the endpoint is not positioned correctly upon mouse release. Instead of aligning with the cursor, it appears to default to a central position within the frame.\n\n**Expected functionality**: Ensure that the endpoints of lines are accurately placed according to the mouse cursor's position at the moment of releasing the left mouse button.\n\n**Technical details**:\n\n1. Investigate the mouse event handling, particularly the logic that determines the final position of line endpoints upon mouse release.\n2. Check for any conditional logic that might be influencing the positioning when lines are within a Trust Boundary frame.\n3. Review any anchoring or snapping features that may interfere with line endpoint placement.\n\n**First Steps**:\n\n1. Set up a development environment using the specified version (2.2.0.0) on Windows.\n2. Reproduce the issue by following the steps outlined in the \"To Reproduce\" section of the bug report.\n3. Add debugging statements to log the coordinates of the mouse cursor during the drag action and the coordinates received upon mouse release.\n4. Analyze how the Trust Boundary frame’s properties affect the line endpoints during manipulation.\n5. Modify the event handling code to ensure that the endpoint's final position reflects the cursor's last known position instead of an arbitrary location.\n\n**Next Actions**: Implement the code changes and test extensively to confirm that the issue is resolved across various scenarios involving Trust Boundary frames and line objects.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/threat-dragon/issues/1082",
@@ -11419,10 +11799,10 @@
"repository": 502,
"assignees": [],
"labels": [
- 80,
- 83,
+ 88,
87,
- 88
+ 80,
+ 83
]
}
},
@@ -11431,10 +11811,11 @@
"pk": 397,
"fields": {
"nest_created_at": "2024-09-11T20:10:57.810Z",
- "nest_updated_at": "2024-09-11T20:10:57.810Z",
+ "nest_updated_at": "2024-09-13T15:17:12.248Z",
"node_id": "MDU6SXNzdWU3ODY1NjgzODA=",
"title": "Participation request",
"body": "I'm interested to take part in this amazing initiative. \r\nCould you please guide?\r\nThanks!",
+ "summary": "A user has expressed interest in participating in the initiative and is seeking guidance on how to get involved. It would be helpful to provide instructions or resources for participation.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-kubernetes-security-testing-guide/issues/4",
@@ -11465,6 +11846,7 @@
"node_id": "I_kwDOD7_kCs5MnZj6",
"title": "Pipeline Tampering Risks & Prevention",
"body": "Hi folks,\r\nAs a DevSecOps practitioner for many sizes of development, there is a critical one for maintaining DevSecOps Pipeline to prevent integrity violation and DRY principle with the pipeline consuming\r\n\r\nAbstraction Ideas:\r\n- Pipeline definition store as separate repos\r\n- Consuming pipeline as git sub-modules\r\n- Pipeline call should be visible and measured\r\n\r\nBenefits:\r\n\r\n- [ ] Pipeline enforcement\r\n- [ ] Pipeline integrity\r\n- [ ] Pipeline scalability\r\n\r\nI'm happy to help but not so sure which category should we put it on",
+ "summary": "The issue discusses the risks of pipeline tampering in DevSecOps and suggests methods for prevention, including storing pipeline definitions in separate repositories, using git sub-modules, and ensuring visibility and measurability of pipeline calls. The proposed changes aim to enhance pipeline enforcement, integrity, and scalability. It also requests guidance on the appropriate category for these suggestions. To move forward, it would be beneficial to categorize the issue and outline actionable steps for implementing the proposed ideas.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/DevSecOpsGuideline/issues/47",
@@ -11482,9 +11864,9 @@
165
],
"labels": [
- 95,
96,
- 97
+ 97,
+ 95
]
}
},
@@ -11497,6 +11879,7 @@
"node_id": "I_kwDOD7_kCs5MuBHN",
"title": "Software Composition Analysis & Supply Chain Risk Management",
"body": "Hey!\r\n\r\nI see that the SCA is a little bit less developed than other parts of the doc, so I'd be happy to expand on this to include various techniques, technologies, tools, and workflows on how this is done in a real-world scenario. Let me know if that's what you're interested in. I also gave a talk about it [here](https://mostafa.dev/practical-cybersecurity-supply-chain-risk-management-7a54ed7d9cc7).",
+ "summary": "There is a request to enhance the Software Composition Analysis (SCA) section of the documentation, as it currently lacks depth compared to other areas. The suggestion includes adding various techniques, technologies, tools, and workflows relevant to real-world scenarios. It's also noted that a relevant talk was given on the topic. Expanding this section would improve the overall quality and comprehensiveness of the documentation.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/DevSecOpsGuideline/issues/48",
@@ -11514,8 +11897,8 @@
166
],
"labels": [
- 95,
- 96
+ 96,
+ 95
]
}
},
@@ -11528,6 +11911,7 @@
"node_id": "I_kwDOD7_kCs5M0c-2",
"title": "Access Controls in pipeline config",
"body": "Hey All,\r\nI can provide some content about accessing to pipeline configs, user types and branch protection to secure pipeline config files.\r\nIs this topic sound valuable for you?\r\nThnks.",
+ "summary": "The issue discusses the need for content related to access controls in pipeline configurations, including user types and branch protection for securing these files. The author is seeking feedback on the value of this topic. It may be beneficial to respond with thoughts on the importance of this information and possibly outline specific areas to cover.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/DevSecOpsGuideline/issues/50",
@@ -11545,9 +11929,9 @@
167
],
"labels": [
- 95,
96,
- 97
+ 97,
+ 95
]
}
},
@@ -11560,6 +11944,7 @@
"node_id": "I_kwDOD7_kCs5M561T",
"title": "Add open source solution ",
"body": "Gitlab security pipelines\r\nCan be found: https://gitlab.com/whitespots-public/pipelines\r\n\r\nWith Security stage integrated into your team's pipelines, on, let's say, every release, Security stage is run and trigger Security pipelines to do the job. Security stage itself doesn't affect your time-to-market. \r\nSecurity pipelines combine different types of security scanners in one \"Security\" stage. \r\nScans reports are sent to DefectDojo, where triage begins. \r\n\r\nPipelines integration instructions: https://www.youtube.com/watch?v=DLN1kNh_Ha0 \r\nSSDLC based approach is described here: https://www.youtube.com/watch?v=6FGV4OcrIB8\r\nHow to work with DefectDojo tutorial: https://www.youtube.com/watch?v=_uFOIf1BUwU\r\n\r\nWe are contributing to this project for about 2 years and are ready to share. Hope it worth being added here. ",
+ "summary": "The issue proposes adding an open-source solution for integrating security pipelines within GitLab, specifically for use during release processes. The integration allows for running a security stage that triggers various security scanners, without impacting the time-to-market. The results of these scans are sent to DefectDojo for triaging. \n\nThe author has provided links to video instructions for pipeline integration, a secure software development lifecycle (SSDLC) approach, and a tutorial on working with DefectDojo. The contribution has been developed over two years, indicating a robust solution worth considering for inclusion. \n\nTo address this issue, it may be necessary to evaluate the proposed solution and assess its compatibility with existing systems before proceeding with the integration.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/DevSecOpsGuideline/issues/52",
@@ -11588,6 +11973,7 @@
"node_id": "I_kwDOD7_kCs5a2wd4",
"title": "Add source of schemas to assests folder",
"body": "Hi, can u add the source scheme file of [image](https://github.com/OWASP/DevSecOpsGuideline/blob/master/document/assets/images/Pipeline-view.png) to assests folder.\r\nThis will allow other users to change the images.",
+ "summary": "The issue requests the addition of the source scheme file for a specific image to the assets folder. This would enable other users to modify the images as needed. To resolve this, the relevant source file should be located and added to the assets folder.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/DevSecOpsGuideline/issues/65",
@@ -11603,8 +11989,8 @@
"repository": 513,
"assignees": [],
"labels": [
- 95,
- 97
+ 97,
+ 95
]
}
},
@@ -11617,6 +12003,7 @@
"node_id": "I_kwDOD7_kCs5o53SY",
"title": "Dependency Security Management & Continuous Dependency Remediation",
"body": "In the modern AppSec program, it's necessary to \"shift-left\" security & governance for dependency from the `Code` to the `Plan` stage.\r\n\r\n# Conceptual approach\r\n\r\n## Plan phrase:\r\n\r\n### For OSS Dependency:\r\n- Benchmark OSS dependency project with [OpenSFF Scorecard](https://github.com/ossf/scorecard)\r\n\r\n### For vendor and third-party dependency:\r\n- Involve SBOM as artifacts release manifest in order to be aware of downstream dependencies. The benefits of the SBOM approach allow the security team to perform security assessments without the need for source code - might not available with third-party\r\n\r\nBuilding private dependencies registry to secure store and sign-off for dependency to prevent availability and tampering issues from upstream maintainers\r\n\r\n## Code phrase:\r\n- Setup proper dependency security scanning tool in CI/CD pipeline\r\n- Setup Dependency Vulnerability Assessment to continuously scan and alerts for new finding developers\r\n",
+ "summary": "The issue discusses the need for enhanced security management for dependencies within modern application security programs by shifting the focus from the coding phase to the planning stage. \n\nKey recommendations include utilizing the OpenSFF Scorecard to benchmark open-source dependencies and incorporating Software Bill of Materials (SBOM) for vendor and third-party dependencies to facilitate security assessments. Furthermore, the establishment of a private dependencies registry is suggested to secure and manage dependencies effectively, mitigating risks associated with availability and tampering.\n\nIn the coding phase, the implementation of dependency security scanning tools in the CI/CD pipeline and continuous vulnerability assessments to alert developers of new findings is emphasized. \n\nAction items may involve integrating the recommended tools and frameworks into the existing security processes to strengthen dependency management and remediation.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/DevSecOpsGuideline/issues/75",
@@ -11643,6 +12030,7 @@
"node_id": "I_kwDOD7_kCs6K0HXa",
"title": "Provenance",
"body": "Add steps with provenance generating on Build and provenance check on Deploy",
+ "summary": "The issue requests the addition of steps for generating provenance during the build process and checking provenance during deployment. This implies that enhancements are needed in the build and deployment workflows to incorporate provenance tracking and verification. Implementing these steps will help ensure the integrity and traceability of the builds.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/DevSecOpsGuideline/issues/86",
@@ -11657,12 +12045,12 @@
"author": 169,
"repository": 513,
"assignees": [
- 166,
- 169
+ 169,
+ 166
],
"labels": [
- 95,
- 96
+ 96,
+ 95
]
}
},
@@ -11675,6 +12063,7 @@
"node_id": "I_kwDOD7_kCs6M_uJB",
"title": "Diagram as code",
"body": "Would there be a significant interest in moving toward diagram as code for the various schema in this repository? \n\nI have used `mermaid` personally with significant success to either document workflow, git history and a few others common diagram.\n\nIt fosters diagram evolution given everything is \"as code\". \n\n\nNot certain if they would render properly on the web but it does in GitHub markdown. ",
+ "summary": "The issue discusses the potential interest in adopting \"diagram as code\" for the schemas in the repository, highlighting the success of using `mermaid` for documenting workflows and other diagrams. The author notes that this approach supports the evolution of diagrams since they are managed as code. There is uncertainty about whether these diagrams would render correctly on the web, although they do work in GitHub markdown. \n\nTo move forward, it may be beneficial to evaluate the feasibility of integrating `mermaid` or similar tools into the repository's documentation process.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/DevSecOpsGuideline/issues/89",
@@ -11697,10 +12086,11 @@
"pk": 406,
"fields": {
"nest_created_at": "2024-09-11T20:11:45.076Z",
- "nest_updated_at": "2024-09-12T00:15:03.994Z",
+ "nest_updated_at": "2024-09-13T17:00:41.837Z",
"node_id": "I_kwDOD7_kCs6Vsx-L",
"title": "Translating DevSecOps Guideline to \"Farsi\"",
"body": "A clear and technical translation with simplicity is needed for the valuable set of recommendations presented in this project for the Persian speaking community, and we intend to translate it.",
+ "summary": "The issue discusses the need for a clear and technically accurate translation of the DevSecOps Guideline into Farsi, aimed at making the recommendations accessible to the Persian-speaking community. The task involves ensuring that the translation is both straightforward and retains the original intent of the guidelines. It would be beneficial to initiate the translation process while considering these aspects.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/DevSecOpsGuideline/issues/91",
@@ -11727,6 +12117,7 @@
"node_id": "I_kwDOD7JcLc5XKQof",
"title": "Feature Request -- FIM Stats on each run",
"body": "It would be helpful to have a report on the same line that reports the time required for the cycle.\r\nAn example is below:\r\n\r\n Total time consumed in this round file integrity checking = 1006506ms (1006.506s) Number of files Scanned = 10003 Number of files in the DB = 10040\r\n\r\nLatest Round: TimeConsumed=1006506ms (1006.506s) FilesScanned=10003 CurrentFilesInDB=10045\r\n",
+ "summary": "Implement a feature to display performance statistics for each file integrity monitoring (FIM) run. Include a report that details the total cycle time, number of files scanned, and current files in the database. \n\nStructure the output as follows:\n- Total time consumed in this round of file integrity checking: `Total time consumed = {time}ms ({time in seconds}s)`\n- Include counts for:\n - Number of files scanned\n - Number of files in the database\n\nFor example:\n```\nTotal time consumed in this round of file integrity checking = 1006506ms (1006.506s)\nNumber of files scanned = 10003\nNumber of files in the DB = 10040\n\nLatest Round: TimeConsumed=1006506ms (1006.506s) FilesScanned=10003 CurrentFilesInDB=10045\n```\n\n**First Steps:**\n1. Identify the section of the code responsible for executing the FIM runs.\n2. Measure the execution time using a suitable timer method (e.g., `System.currentTimeMillis()` in Java).\n3. Gather statistics on the number of files scanned and those present in the database during each run.\n4. Format and output the collected data in the specified report format after each run.\n5. Test the implementation to ensure accuracy and performance impact.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-winfim.net/issues/8",
@@ -11753,6 +12144,7 @@
"node_id": "I_kwDOD7JcLc5Y841u",
"title": "Newline on the StopMessage String",
"body": "There is a carriage return on the stop message string located in the Controller.cs:\r\n\r\n string stopMessage = \"Total time consumed in this round file integrity checking = \" +\r\n watch.ElapsedMilliseconds + \"ms (\" +\r\n Math.Round(Convert.ToDouble(watch.ElapsedMilliseconds) / 1000, 3)\r\n .ToString(CultureInfo.InvariantCulture) + \"s).\\n\" +\r\n LogHelper.GetRemoteConnections();\r\n\r\nThis newline creates havoc with Excel importing CSV. More specifically, the cell looks blank.\r\n",
+ "summary": "Remove the newline character from the stop message string in `Controller.cs`. The current implementation introduces a carriage return that disrupts the CSV format, causing Excel to misinterpret the data, resulting in blank cells.\n\n**Technical Steps to Address the Issue:**\n\n1. Locate the `stopMessage` string declaration in `Controller.cs`.\n2. Eliminate the newline character (`\\n`) at the end of the string.\n - Change:\n ```csharp\n string stopMessage = \"Total time consumed in this round file integrity checking = \" +\n watch.ElapsedMilliseconds + \"ms (\" +\n Math.Round(Convert.ToDouble(watch.ElapsedMilliseconds) / 1000, 3)\n .ToString(CultureInfo.InvariantCulture) + \"s).\\n\" +\n LogHelper.GetRemoteConnections();\n ```\n - To:\n ```csharp\n string stopMessage = \"Total time consumed in this round file integrity checking = \" +\n watch.ElapsedMilliseconds + \"ms (\" +\n Math.Round(Convert.ToDouble(watch.ElapsedMilliseconds) / 1000, 3)\n .ToString(CultureInfo.InvariantCulture) + \"s).\" +\n LogHelper.GetRemoteConnections();\n ```\n\n3. Test the output to ensure the string formats correctly without causing issues in Excel.\n4. Validate the CSV import functionality to confirm that the changes resolve the blank cell issue in Excel.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-winfim.net/issues/9",
@@ -11779,6 +12171,7 @@
"node_id": "I_kwDOD7JcLc5Y8-P5",
"title": "Improper Date Time Representation",
"body": "Within Service.cs and Controller.cs there are multiple places where the log format is set to yyyy/dd/MM.\r\nIt Should be yyyy/MM/dd\r\n",
+ "summary": "Correct the date time representation in Service.cs and Controller.cs. Replace all instances of the log format set to `yyyy/dd/MM` with `yyyy/MM/dd`. \n\n1. Open Service.cs and Controller.cs files.\n2. Search for occurrences of `yyyy/dd/MM`.\n3. Update each occurrence to `yyyy/MM/dd`.\n4. Test the logging functionality to ensure the date format is displayed correctly after the changes. \n5. Commit the changes and create a pull request for review.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-winfim.net/issues/10",
@@ -11805,6 +12198,7 @@
"node_id": "I_kwDOD7JcLc5aN67s",
"title": "Windows Defender spikes CPU usage when WinFIM.NET service is running",
"body": "Hello,\r\n\r\nWe are testing your tool on Windows Server 2019. Somehow, when the WinFIM.NET service is running, it spikes up Windows Defender CPU usage. Even when we do not have any files in the monitoring folder (C:/Temp currently). We tried to set couple exclusions in Windows Defender, but no luck. Also, the tool is set to run with parameter 0 in scheduler file.\r\n\r\nAny idea how to set this properly? Or how to set the exclusions properly?\r\n\r\nThanks.",
+ "summary": "Investigate high CPU usage by Windows Defender when WinFIM.NET service is active on Windows Server 2019. \n\n1. Confirm that the WinFIM.NET service is running with parameter 0 as intended.\n2. Attempt to add specific exclusions for the WinFIM.NET service in Windows Defender:\n - Open Windows Security settings.\n - Navigate to \"Virus & threat protection.\"\n - Select \"Manage settings\" under \"Virus & threat protection settings.\"\n - Scroll down to \"Exclusions\" and add C:/Temp and any relevant executables related to WinFIM.NET.\n3. Monitor CPU usage after applying exclusions to determine if the issue persists.\n4. Check for any other processes that may interact with WinFIM.NET that could also be causing CPU spikes.\n5. Review the Windows Event Viewer for any logs that might indicate conflicts or issues with the WinFIM.NET service or Windows Defender. \n\nDocument any changes made and their effects on CPU usage for further troubleshooting if necessary.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-winfim.net/issues/12",
@@ -11831,6 +12225,7 @@
"node_id": "I_kwDOD7JcLc5u6IBN",
"title": "No owner info for who delete or modify files",
"body": "Hi,\r\n\r\nIf somebody deletes or modify the files, from the FIM logs, we don't know who did it. How to add feature to track who delete or modify files? ",
+ "summary": "Implement a feature to track file deletions and modifications by logging user information in FIM (File Integrity Monitoring) logs. \n\n1. Analyze the current FIM logging system to identify how file changes are recorded.\n2. Modify the logging mechanism to capture and store the user ID or username associated with file modifications and deletions.\n3. Ensure that the system can retrieve user information from the operating environment (e.g., leveraging audit logs or filesystem events).\n4. Update documentation to reflect new logging capabilities and instructions for monitoring file changes.\n5. Test the implementation in a controlled environment to verify that user information is correctly logged and retrievable. \n6. Deploy the changes to the production environment and monitor for any issues.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-winfim.net/issues/15",
@@ -11853,10 +12248,11 @@
"pk": 412,
"fields": {
"nest_created_at": "2024-09-11T20:12:16.371Z",
- "nest_updated_at": "2024-09-11T20:12:16.371Z",
+ "nest_updated_at": "2024-09-13T15:17:59.316Z",
"node_id": "I_kwDOD7JcLc6PiR9W",
"title": "No support for UTF-8 characters in path",
"body": "WinFIM throws 7773 errors whenever a word in the path to a file contains a non en_US character.\r\nThe problematic letters are replaced in the path which logically makes it wrong as the log detail shows:\r\n`[...] could be renamed / deleted during the hash calculation. This file is ignored in this checking cycle - Can't find a part of the path`\r\n\r\nIt would be nice to add UTF-8 support or at least give a procedure on how to enable it.",
+ "summary": "Enhance WinFIM to support UTF-8 characters in file paths. Currently, the application throws errors (7773) when encountering non-English characters in paths, leading to incorrect file handling during hash calculations. The logs indicate that files with non-en_US characters are ignored, causing issues like: `[...] could be renamed / deleted during the hash calculation. This file is ignored in this checking cycle - Can't find a part of the path`.\n\nTo tackle this problem:\n\n1. **Review Current Encoding Methods**: Investigate how WinFIM currently handles file paths and identify the areas where character encoding is limited to en_US.\n\n2. **Implement UTF-8 Encoding**: Modify the file path handling code to support UTF-8 encoding. Ensure that all I/O operations (reading, writing, hashing) correctly interpret and process UTF-8 characters.\n\n3. **Test with Various Encodings**: Create test cases that include file paths with a range of UTF-8 characters. Verify that the application can handle these paths without errors.\n\n4. **Update Documentation**: If a straightforward method to enable UTF-8 support is not feasible, document the steps required to configure the application for UTF-8 processing.\n\n5. **Seek Community Feedback**: Engage with users to gather insights on their experiences and any additional requirements concerning file path handling.\n\nBy following these steps, ensure that WinFIM can robustly handle file paths containing UTF-8 characters, improving its usability for a broader audience.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-winfim.net/issues/16",
@@ -11879,10 +12275,11 @@
"pk": 413,
"fields": {
"nest_created_at": "2024-09-11T20:13:00.030Z",
- "nest_updated_at": "2024-09-11T20:13:00.030Z",
+ "nest_updated_at": "2024-09-13T15:18:33.473Z",
"node_id": "I_kwDOD5sfqM6BytKM",
"title": "Please add a description to this project",
"body": "",
+ "summary": "The issue requests the addition of a project description to provide clarity and context for users. To address this, a concise and informative description outlining the project's purpose, features, and usage should be created and added.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-jvmxray/issues/3",
@@ -11905,10 +12302,11 @@
"pk": 414,
"fields": {
"nest_created_at": "2024-09-11T20:13:07.406Z",
- "nest_updated_at": "2024-09-11T20:13:07.406Z",
+ "nest_updated_at": "2024-09-13T15:18:39.442Z",
"node_id": "I_kwDOD5sGHc6BytI9",
"title": "Please add a description to this project",
"body": "",
+ "summary": "The issue requests the addition of a project description to provide clarity and context. It suggests that including a concise overview of the project's purpose and functionality would enhance understanding for potential users and contributors. To address this, a brief and informative description should be drafted and added to the project documentation.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-redteam-toolkit/issues/2",
@@ -11931,10 +12329,11 @@
"pk": 415,
"fields": {
"nest_created_at": "2024-09-11T20:13:21.779Z",
- "nest_updated_at": "2024-09-11T20:13:21.779Z",
+ "nest_updated_at": "2024-09-13T15:18:50.605Z",
"node_id": "MDU6SXNzdWU2NjEwMTIyMTI=",
"title": "Create OWASP conference table package",
"body": "During the July Outreach Committee call we agreed that we could setup a suggested conference package that the foundation could ship in support of OWASP tables in conferences.\r\n\r\nIn order to close this ticket we need:\r\n\r\nList of suggested artwork\r\nList of suggested swag and formats\r\nA suggested relevant playbook for ordering shipping and expensing the \"conference package\" per world region.\r\nA suggested slide-deck/talking points(?)\r\nSince, to my understanding, OWASP cannot promote commercial distributors, the list of suggested distributors can be private\r\n\r\nThe purpose of this action is to enable volunteers to represent OWASP in events with tables",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-committee-outreach/issues/8",
@@ -11963,6 +12362,7 @@
"node_id": "MDU6SXNzdWU3OTY5MDk0OTg=",
"title": "Fix numbering before release",
"body": "We have to make sure to fix the numbering of requirements before release. ",
+ "summary": "The issue highlights the need to correct the numbering of requirements prior to the release. It suggests that a review and update of the current numbering system is necessary to ensure accuracy.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/IoT-Security-Verification-Standard-ISVS/issues/55",
@@ -11991,6 +12391,7 @@
"node_id": "MDU6SXNzdWU4MDg4MjgxODk=",
"title": "Add info on how to deal with non conformities in \"using the ISVS\" chapter",
"body": "The ISVS currently does not address that not implementing a security control and/or accepting a failed security control/vulnerability is a effort vs risk based decision. We could add something to the using the ISVS chapter. \r\n",
+ "summary": "The issue suggests enhancing the \"using the ISVS\" chapter by including guidance on managing non-conformities, particularly regarding the decision-making process for not implementing security controls or accepting vulnerabilities. It emphasizes the need for a discussion on the effort versus risk-based decisions in these scenarios. To address this, additional content outlining best practices or frameworks for handling such situations could be beneficial.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/IoT-Security-Verification-Standard-ISVS/issues/63",
@@ -12017,10 +12418,11 @@
"pk": 418,
"fields": {
"nest_created_at": "2024-09-11T20:13:50.765Z",
- "nest_updated_at": "2024-09-12T00:15:08.880Z",
+ "nest_updated_at": "2024-09-13T16:59:56.501Z",
"node_id": "MDU6SXNzdWU4MTUxODk0Mzg=",
"title": "Suggestions to improve consistency - Update \"Using ISVS\" Figure",
"body": "There are several areas which I believe could be more consistent:\r\n\r\n1. Wi-Fi and Bluetooth\r\nThese are very specific protocols. What if the device uses LoRaWAN or Zigbee?\r\nIt could be more beneficial to separate LAN and WAN communications as the threat model is different.\r\n\r\n2. Figure used in https://github.com/OWASP/IoT-Security-Verification-Standard-ISVS/blob/master/en/Using_ISVS.md#the-isvs-security-model\r\nThe blocks used in the figure are confusing: they mix components and features (e.g. Wi-Fi or software updates) with processes (e.g. design, secure development).\r\nI would suggest to:\r\n- Regroup all processes in one box.\r\n- Simplify the device \"design\" with components and features in boxes representing a physical device. For example: \"internal hardware\", \"external hardware\", \"software\".\r\n\r\n",
+ "summary": "The issue discusses the need for improved consistency in the \"Using ISVS\" figure. It highlights two main areas for enhancement: \n\n1. The distinction between communication protocols, suggesting a separation of LAN and WAN communications to address different threat models.\n \n2. The organization of elements in the figure, recommending that components and processes be clearly differentiated. It suggests regrouping all processes into one box and simplifying the device design by categorizing components and features into boxes representing a physical device.\n\nTo address these points, it would be beneficial to revise the figure to enhance clarity and consistency.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/IoT-Security-Verification-Standard-ISVS/issues/65",
@@ -12035,12 +12437,12 @@
"author": 177,
"repository": 547,
"assignees": [
- 12,
- 176
+ 176,
+ 12
],
"labels": [
- 98,
- 99
+ 99,
+ 98
]
}
},
@@ -12053,6 +12455,7 @@
"node_id": "MDU6SXNzdWU4MzAxNjE2MTA=",
"title": "Missing Freeze and Mix & Match attack cases",
"body": "The firmware update chapter currently explicitly covers roll-back attacks. The Freeze and Mix & Match attack cases are not (explicitly) covered. \r\n- Freeze attacks: an attacker tricks the device / ecosystem in believing the device is up to date while in reality it is not. \r\n- Mix & Match attacks: an attacker supplies combinations of packages or package metadata that never existed in the upstream repository. Can occur if for example individual packages are signed, but package indexes are not. \r\n\r\nThese, together with others that we potentially overlooked, can be found here: https://theupdateframework.io/security/ \r\n\r\n",
+ "summary": "The issue highlights the absence of coverage for Freeze and Mix & Match attack cases in the firmware update chapter, which currently only addresses roll-back attacks. Freeze attacks involve misleading the device into thinking it is updated when it is not, while Mix & Match attacks consist of supplying non-existent combinations of packages or metadata. The discussion suggests reviewing additional attack scenarios listed on the provided resource link to ensure comprehensive coverage of security threats. It may be beneficial to update the documentation to include these attack cases.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/IoT-Security-Verification-Standard-ISVS/issues/80",
@@ -12081,6 +12484,7 @@
"node_id": "MDU6SXNzdWU4MzQ0MTUxNDg=",
"title": "L3 requirements for Bluetooth and Wifi aren't high enough",
"body": "The suggestions for Bluetooth and Wifi are reasonable but for L3 I think they need to go further:\r\n\r\n- WPA3 for Wifi.\r\n- Minimum of Bluetooth 4.2 - improved security over 4.1 with Secure Connections.\r\n-- I can't find any reference to improved security for 5, 5.1 and 5.2.\r\n- Recommendations on the different pairing models. I'm still researching this, but it seems the 6 digit PIN in SSP (Secure Simple Pairing) isn't particularly strong - ~~see tools like https://github.com/mikeryan/crackle/~~ _Crackle only works on Legacy Pairing, not Secure Connections, see https://github.com/mikeryan/crackle/blob/master/FAQ.md#is-my-device-using-le-legacy-pairing-or-le-secure-connections_\r\n",
+ "summary": "The issue highlights that the L3 requirements for Bluetooth and Wifi need to be enhanced. Specifically, it suggests implementing WPA3 for Wifi security and setting a minimum requirement of Bluetooth 4.2, which offers better security than 4.1. Additionally, there is a call for recommendations regarding different pairing models, particularly addressing concerns about the strength of the 6-digit PIN in Secure Simple Pairing. Further research into these security aspects is necessary to improve the overall requirements. It would be beneficial to update the L3 requirements to include these enhancements.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/IoT-Security-Verification-Standard-ISVS/issues/82",
@@ -12110,6 +12514,7 @@
"node_id": "I_kwDODpWfz847V6-W",
"title": "Detect & Response set of requirements missing",
"body": "The ISVS currently does not cover security requirements related to detecting and responding to security incidents. \r\n\r\nExample requirement that's missing: Verify that an appropriate response strategy is in place in case an end device's root keys are compromised, given that root keys cannot be remotely updated. \r\n\r\nOther example of a requirement that exists but could be generalized: \r\n| **4.5.8** | Verify that users can obtain an overview of paired devices to validate that they are legitimate (for example, by comparing the MAC addresses of connected devices to the expected ones). | | | ✓ |",
+ "summary": "The issue highlights a gap in the current ISVS regarding the absence of security requirements focused on detecting and responding to security incidents. A specific example provided is the need to ensure an appropriate response strategy for compromised root keys, which cannot be updated remotely. Additionally, it suggests that some existing requirements, like user validation of paired devices, could benefit from being generalized. To address this, the requirements for incident detection and response need to be developed and integrated into the ISVS framework.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/IoT-Security-Verification-Standard-ISVS/issues/86",
@@ -12136,6 +12541,7 @@
"node_id": "I_kwDODpWfz849BY3K",
"title": "V2: Missing anti-bruteforce & reauthentication requirements in authentication section",
"body": "Hi! \r\n\r\nI noticed that V2 doesn't currently contain any requirements for: \r\n\r\n- anti-bruteforce protection of authentication mechanisms \r\n- re-authentication in regular intervals (so that users and/or devices do not remain authenticated forever). \r\n\r\nDo you think it would be a good idea to add these? Some example (draft) requirements could be: \r\n\r\n- Verify that authentication mechanisms have sufficient protection against brute force attacks.\r\n- Verify that re-authentication of users and devices is required at regular intervals. The interval duration should depend on the security criticality of the application or functionality. ",
+ "summary": "The issue highlights the absence of anti-bruteforce protection and reauthentication requirements in the V2 authentication section. It suggests that these elements should be included to enhance security. Proposed requirements include ensuring authentication mechanisms are robust against brute force attacks and mandating regular re-authentication based on the application's security needs. It would be beneficial to consider adding these requirements to strengthen the authentication framework.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/IoT-Security-Verification-Standard-ISVS/issues/88",
@@ -12162,6 +12568,7 @@
"node_id": "I_kwDODpWfz85CVeDF",
"title": "3.2.9\t RAM scrambling?",
"body": "Hi,\r\n\r\n3.2.9 on OS configuration says that one should:\r\n Verify the embedded OS provides protection against unauthorized access to RAM (e.g. RAM scrambling).\r\n\r\nI'm somewhat confused about this as I thought RAM scrambling was a transparent hardware security measure. Could u please list some examples on OS's where you can effectively configure RAM scrambling or similar measures? Is there anything in Linux for that? \r\n\r\nThanks,\r\nAttila\r\n\r\n",
+ "summary": "The issue raises a question about the requirement in OS configuration 3.2.9 regarding RAM scrambling and its role in protecting against unauthorized access to RAM. The user seeks clarification on whether RAM scrambling is a hardware feature and requests examples of operating systems that allow for effective configuration of RAM scrambling or similar security measures, particularly looking for Linux solutions. To address this, the issue could benefit from providing specific examples and guidance on configuring RAM scrambling in various operating systems, including Linux.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/IoT-Security-Verification-Standard-ISVS/issues/89",
@@ -12188,6 +12595,7 @@
"node_id": "I_kwDODhZs8M6DQdbY",
"title": "Update: 07-implementation/00-toc to include a section on SAST",
"body": "**Describe what change you would like** : \r\nIt would be good to have a section on static analysis in the implementation section, and add semgrep to the new section\r\n\r\n**Context** : \r\nSection: 07-implementation/00-toc\r\n\r\nsemgrep: https://semgrep.dev/docs/getting-started/quickstart-oss/\r\n\r\n",
+ "summary": "The issue requests the addition of a section on static analysis in the implementation section, specifically within the 07-implementation/00-toc. The suggestion includes incorporating information about semgrep, a static analysis tool. It would be beneficial to update the table of contents to reflect this new section.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-developer-guide/issues/179",
@@ -12212,10 +12620,11 @@
"pk": 425,
"fields": {
"nest_created_at": "2024-09-11T20:14:55.441Z",
- "nest_updated_at": "2024-09-11T20:14:55.441Z",
+ "nest_updated_at": "2024-09-13T15:19:55.206Z",
"node_id": "I_kwDODhZs8M6HbWkI",
"title": "Create OpenAI Agent backed by this repo & the Wayfinder",
"body": "**Describe what content should be added** : \r\n\r\n\r\nCreate an OpenAI chatbot backed by an OS LLM trained with the knowledge from this repo, the Wayfinder project (OWASP Integration Standards), SKF (?)\r\n\r\n**Context** : \r\nAll sections \r\n\r\n\r\n",
+ "summary": "The issue requests the creation of an OpenAI chatbot that utilizes an open-source language model (LLM) trained with knowledge from a specific repository and the Wayfinder project, which relates to OWASP Integration Standards. It suggests that the implementation should encompass all sections of the existing content. To proceed, further exploration of the current repository and integration of the relevant knowledge from Wayfinder and SKF may be necessary.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-developer-guide/issues/196",
@@ -12248,6 +12657,7 @@
"node_id": "I_kwDODhZs8M6PN9Sy",
"title": "Provide cross references to cornucopia",
"body": "**Describe what content should be added** : \r\nIt would be good to link across to the cornucopia cards from the checklist\r\nThe first step to cross referencing to cornucopia is provide identifiers to the checklist (done in #225 )\r\nthen the checklists can link across to the cornucopia cards API\r\n\r\n**Context** : \r\nSection: 06-design/02-web-app-checklist/\r\n\r\nFrom @sydseter 's comment in Threat dragon issue #140 '[Integrate threat engine with Cornucopia / EoP cards](https://github.com/OWASP/threat-dragon/issues/140)', we have : \r\nFull yaml source can be found at https://github.com/OWASP/cornucopia/tree/master/source\r\nWe also have endpoints to the OWASP Cornucopia Website App and Mobile App editions.\r\n\r\nOWASP Cornucopia Website App Edition:\r\n\r\nhttps://copi.securedelivery.io/cards/2.00/DV2\r\nhttps://copi.securedelivery.io/cards/2.00/AC2\r\nhttps://copi.securedelivery.io/cards/2.00/SM2\r\nhttps://copi.securedelivery.io/cards/2.00/AZ2\r\nhttps://copi.securedelivery.io/cards/2.00/CR2\r\nhttps://copi.securedelivery.io/cards/2.00/CO2\r\n\r\nOWASP Cornucopia Mobile App Edition:\r\n\r\nhttps://copi.securedelivery.io/cards/1.00/CO2\r\nhttps://copi.securedelivery.io/cards/1.00/PC2\r\nhttps://copi.securedelivery.io/cards/1.00/AA2\r\nhttps://copi.securedelivery.io/cards/1.00/NS2\r\nhttps://copi.securedelivery.io/cards/1.00/RS2\r\nhttps://copi.securedelivery.io/cards/1.00/CRM2\r\nhttps://copi.securedelivery.io/cards/1.00/COM2\r\n",
+ "summary": "The issue discusses the need to create cross-references between the checklist and the Cornucopia cards. The first step involves adding identifiers to the checklist, which has already been addressed in a previous issue. Following this, the checklists should be linked to the Cornucopia cards API for better integration and accessibility.\n\nTo move forward, it will be necessary to implement the linking mechanism between the checklist and the Cornucopia cards using the provided API endpoints.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-developer-guide/issues/231",
@@ -12277,6 +12687,7 @@
"node_id": "I_kwDODhZs8M6QTlxM",
"title": "Translate DevGuide to Spanish",
"body": "**Describe what change you would like** : \r\nThe Developer Guide needs translations, so it would be great if the DevGuide could be translated\r\nSpanish is the first choice because of its wide use across countries/continents\r\n\r\n**Context** : \r\nSection: release-es/*\r\n\r\nContact @jgadsden for advice on how to approach this",
+ "summary": "The issue requests the translation of the Developer Guide into Spanish due to its extensive use across various regions. It suggests focusing on the release-es/* sections and indicates that advice can be sought from a specified contact for guidance on how to proceed with the translation effort.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-developer-guide/issues/239",
@@ -12292,8 +12703,8 @@
"repository": 563,
"assignees": [],
"labels": [
- 102,
- 107
+ 107,
+ 102
]
}
},
@@ -12306,6 +12717,7 @@
"node_id": "I_kwDODhZs8M6QVeyd",
"title": "Translations to other langauges",
"body": "**Describe what change you would like** : \r\nThe Developer Guide needs translations to reach a wider audience. Suggested languages are:\r\n\r\n- Arabic 'العربية'\r\n- German 'Deutsch'\r\n- Greek 'Ελληνικά'\r\n- English\r\n- Finnish 'Suomi'\r\n- French 'Français'\r\n- Hindi 'हिंदी'\r\n- Bahasa Indonesia\r\n- Japanese '日本語'\r\n- Malay\r\n- Chinese '中文'\r\n\r\nSpanish translation is subject of separate issue #239 \r\nPortuguese 'Português' - translation subject of separate issue #267 \r\n\r\n**Context** : \r\nSection: all sections\r\n\r\n",
+ "summary": "The issue highlights the need for translating the Developer Guide into multiple languages to make it accessible to a broader audience. The suggested languages include Arabic, German, Greek, Finnish, French, Hindi, Bahasa Indonesia, Japanese, Malay, and Chinese. It notes that Spanish and Portuguese translations are being addressed in separate issues. To move forward, translations for the listed languages should be prioritized.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-developer-guide/issues/240",
@@ -12321,8 +12733,8 @@
"repository": 563,
"assignees": [],
"labels": [
- 102,
- 107
+ 107,
+ 102
]
}
},
@@ -12335,6 +12747,7 @@
"node_id": "I_kwDODhZs8M6R4zQ-",
"title": "Add OWASP MASWE",
"body": "**Describe what change you would like** : \r\nThere is a new enumeration for the MAS project, [MASWE](https://mas.owasp.org/MASWE)\r\nIt would be good to provide a page on this\r\n\r\n**Context** : \r\nSection: not sure\r\n",
+ "summary": "The issue requests the addition of a new enumeration for the OWASP MAS project, specifically for MASWE. The suggestion is to create a dedicated page to provide information about this enumeration. It is unclear which section of the project this should be added to, indicating that further clarification may be needed. To address this, the appropriate section for the new page should be determined and the content for MASWE created.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-developer-guide/issues/246",
@@ -12365,6 +12778,7 @@
"node_id": "I_kwDODhZs8M6TTjZv",
"title": "Add OWASP OpenCRE from Wayfinder project",
"body": "**Describe what change you would like** : \r\nThe Open Common Requirement Enumeration, OpenCRE, is part of the Wayfinder project and it would be good to have a page in the Developer Guide for this\r\n\r\n**Context** : \r\nSection: new section `05-requirements/03-opencre.md`\r\n`05-requirements/03-skf.md` moved to `05-requirements/07-skf.md`\r\n",
+ "summary": "A request has been made to include a new page in the Developer Guide for the Open Common Requirement Enumeration (OpenCRE) from the Wayfinder project. This would involve creating a new section titled `05-requirements/03-opencre.md` and relocating the existing `05-requirements/03-skf.md` to `05-requirements/07-skf.md`. It is suggested that the necessary documentation and content for OpenCRE be developed and added accordingly.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-developer-guide/issues/258",
@@ -12396,6 +12810,7 @@
"node_id": "I_kwDODhZs8M6U2T8-",
"title": "Translate DevGuide to Portuguese Brazil",
"body": "**Describe what change you would like** : \r\nProvide the DevGuide translation to Brazil Portuguese\r\nA new fileset has been created under `release-pt-br` and the ToC created in `_data/release-pt-br.yaml`\r\n\r\n**Context** : \r\nSection: `release-pt-br` and `_data/release-pt-br.yaml`\r\n",
+ "summary": "The issue requests the translation of the DevGuide into Brazilian Portuguese. A new fileset has been established under `release-pt-br`, and the Table of Contents is available in `_data/release-pt-br.yaml`. To address this, the necessary translation work needs to be completed for the DevGuide.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-developer-guide/issues/267",
@@ -12427,6 +12842,7 @@
"node_id": "MDU6SXNzdWU1NTk3ODMzMzc=",
"title": "OWASP Fragmentation Document ",
"body": "**What is required?**\r\nDeliver a document showcasing how the OWASP projects are fragmented across the SDLC, what are some best practices to implementing the tools, and what are some bad use cases.",
+ "summary": "The issue requests the creation of a document that highlights the fragmentation of OWASP projects throughout the Software Development Life Cycle (SDLC). It should include best practices for implementing these tools as well as examples of poor use cases. To address this, a comprehensive analysis of OWASP tools and their integration into various stages of the SDLC is needed, along with practical recommendations and cautionary examples.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-integration-standards/issues/4",
@@ -12453,6 +12869,7 @@
"node_id": "MDU6SXNzdWU1NTk3ODU4MDM=",
"title": "Projects Integration Discussions with Project Leaders",
"body": "**What is required?**\r\nDiscuss with the major projects that are interested in integrating with other projects and see how they would benefit best from other projects, and what they can deliver for other projects as well.",
+ "summary": "The issue highlights the need for discussions with major projects interested in integration. The goal is to explore mutual benefits and contributions between these projects. To move forward, it is suggested to initiate conversations with project leaders to identify potential collaboration opportunities and define how each project can support the other.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-integration-standards/issues/6",
@@ -12479,6 +12896,7 @@
"node_id": "MDU6SXNzdWU1NjE1OTE4NjE=",
"title": "Create Roadmap",
"body": "**Summary:** Create a one-page document listing where this project is going.\r\n**Current State:** An external observer cannot find goals and targets of the project\r\n**Acceptance Criteria:** There is a one page document listing deliverables and problems to be tackled for the next semester",
+ "summary": "The issue requests the creation of a one-page roadmap document that outlines the project's goals and targets. Currently, it is difficult for external observers to understand the project's direction. The completion of this task requires a concise document that lists the deliverables and challenges to be addressed in the upcoming semester.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-integration-standards/issues/7",
@@ -12505,6 +12923,7 @@
"node_id": "MDU6SXNzdWU1NjE2MzUyNzk=",
"title": "Automated Way of Showing Info about OWASP Projects",
"body": "**Summary**: OWASP has a ton of projects which are very fragmented across both org repos and random github repos. Each project performs it's own tasks but there's no centralized way of finding what each project does and how it connects to the rest.\r\nThis can be solved by adding a small metadata file to each project root.\r\nThen we can use Github's api to either search for specific strings in the metadata files or, grab the metadata files from the owasp org and statically map owasp projects not under the org.\r\nFrom there a script can extract metadata and populate a template with:\r\n\r\n- State of SDLC each project fits in,\r\n- Links to github and docs\r\n- Builders Breakers Defenders designation\r\n\r\nThis allows central presentation of all owasp projects and ease of finding new ones.\r\n\r\nMetadata file format TBD.\r\n\r\n**Acceptance Criteria:** \r\n\r\n* There's an automated way to gather info about owasp projects and presenting them.\r\n* There is a way to see info about flagship projects in one place.\r\n",
+ "summary": "The issue highlights the fragmentation of OWASP projects across various repositories, making it difficult to understand their functions and interconnections. A proposed solution involves adding a metadata file to each project root, which can be utilized alongside GitHub's API to gather information on all OWASP projects, including those not under the organization’s main repository. The extracted metadata would include details such as the SDLC stage of each project, relevant links, and designations like Builders, Breakers, and Defenders. \n\nTo move forward, the format for the metadata file needs to be determined, and an automated system for collecting and presenting this information should be developed, ensuring flagship projects are easily accessible in one location.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-integration-standards/issues/8",
@@ -12533,6 +12952,7 @@
"node_id": "MDU6SXNzdWU2ODY5OTQ1NjU=",
"title": "Projects Update in the Diagram",
"body": "Requirements:\r\n- SCVS\r\n\r\nImplementation -> Secure Libraries:\r\n- OWASP Java Encoder\r\n- OWASP Java HTML Sanitizer\r\n\r\nVerification -> Tools:\r\n- OWASP O-Saft\r\n\r\nOperations:\r\n- Dependency Track\r\n\r\nEdit 1: Add O-Saft",
+ "summary": "The issue discusses updates needed in a project diagram, specifically focusing on the Secure Libraries and Verification sections. The requirements include implementing the OWASP Java Encoder and OWASP Java HTML Sanitizer for secure libraries. For verification, the addition of OWASP O-Saft is requested, along with the mention of Dependency Track in operations. An edit indicates the necessity to add O-Saft to the diagram. It appears that the next steps involve incorporating these elements into the project diagram accordingly.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-integration-standards/issues/24",
@@ -12559,6 +12979,7 @@
"node_id": "MDU6SXNzdWU3MTMwODk0MzE=",
"title": "add a csv/google sheets front end to the cre rest api",
"body": "There's currently no way to import/export data to/from the REST API.\r\nThis ticket has two parts:\r\n* Given a REST call, add the ability to export the results into a google sheets spreadsheet which allows humans to get the mappings into a CSV format. This is already partly implemented (utils/spreadsheet_utils.py) but we haven't implemented any API plumbing for it.\r\n\r\n* There's a way for Authorissed users to submit a spreadsheet that follows the [template](https://docs.google.com/spreadsheets/d/1f47aZydJ47n-iGb0fkmu880wSaFyYDM-zdkgs6oMV7I/edit#gid=1841715588) and import mappings from it.\r\nThis involves some openidconnect integration and some way of allowing which users can submit mappings + plumbing for the parsers to receive a spreadsheet url. This functionality already exists via the cmd api but haven't managed to plumb the openid connect stuff.",
+ "summary": "The issue highlights the need to enhance the existing REST API by adding functionality for importing and exporting data through CSV or Google Sheets. It consists of two main tasks: \n\n1. Implementing the capability to export results from a REST call into a Google Sheets format, utilizing the partially developed features in `utils/spreadsheet_utils.py`, and establishing the necessary API integration.\n \n2. Enabling authorized users to submit spreadsheets that follow a specified template for importing mappings. This requires integrating OpenID Connect for user verification, along with the necessary infrastructure to process the submitted spreadsheet URLs and connect to the existing command-line API functionality.\n\nTo address the issue, the focus should be on completing the API integrations and ensuring proper user authorization for spreadsheet submissions.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-integration-standards/issues/25",
@@ -12585,6 +13006,7 @@
"node_id": "I_kwDODUeCys48d35_",
"title": "Consider to use the 'Open Security Information Base' as OWASP driven ID",
"body": "The OWASP Top10 team has developed an open structure to store information about OWASP projects and links to external IT-security standards: 'Open Security Information Base'. Regarding the discussions with @robvanderveer we have compiled a YAML structure accepting names and numbers (as alias) to browse through the structure.\r\nAn alpha structure, showing an example for ASVS 4.0.2 is on OWASP's slack channel ['# project-integration'](https://owasp.slack.com/archives/CPMEWT342/p1633279131013100), for now.\r\nWe should consider to bring together this development with the CRE project.",
+ "summary": "The issue suggests integrating the 'Open Security Information Base' with the CRE project, as developed by the OWASP Top10 team. A YAML structure has been created to organize information about OWASP projects and relevant IT-security standards. An example structure related to ASVS 4.0.2 is available on OWASP's Slack channel. The next steps would involve assessing the potential for collaboration between these two initiatives.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-integration-standards/issues/43",
@@ -12607,10 +13029,11 @@
"pk": 439,
"fields": {
"nest_created_at": "2024-09-11T20:16:21.820Z",
- "nest_updated_at": "2024-09-11T20:16:21.820Z",
+ "nest_updated_at": "2024-09-13T03:57:34.405Z",
"node_id": "I_kwDODUeCys52RKj2",
"title": "Rename 'Policy Gap Evaluation' in the Wayfinder diagram?",
"body": "The [OWASP Developer Guide](https://owasp.org/www-project-developer-guide/) uses the Wayfinder diagram as the structure of the document, so that sections are Requrements, Design etc. One of the sections is 'Policy Gap Evaluation', because of this area of the Wayfinder diagram.\r\n\r\nWhat does 'Policy Gap Evaluation' actually refer to? After some thought it seems to be concerned with 'Security Gap Analysis' used in management systems such as ISO 27001, so the Developer Guide has renamed 'Policy Gap Evaluation' to 'Security Gap Analysis'.\r\n\r\nCould the Wayfinder diagram have 'Policy Gap Evaluation' renamed to 'Security Gap Analysis', but if I have this wrong the Developer Guide section could be named back to 'Policy Gap Evaluation' to align with the Wayfinder diagram.",
+ "summary": "The issue discusses the need to clarify and potentially rename the 'Policy Gap Evaluation' section in the Wayfinder diagram, as it is currently causing confusion. It suggests that this term may be more accurately represented as 'Security Gap Analysis', aligning with terminology used in the OWASP Developer Guide. The proposed action is to either rename the Wayfinder diagram section to 'Security Gap Analysis' or revert the Developer Guide section back to 'Policy Gap Evaluation' for consistency.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-integration-standards/issues/51",
@@ -12633,10 +13056,11 @@
"pk": 440,
"fields": {
"nest_created_at": "2024-09-11T20:16:59.421Z",
- "nest_updated_at": "2024-09-11T20:16:59.421Z",
+ "nest_updated_at": "2024-09-13T15:21:20.364Z",
"node_id": "I_kwDODQO5YM5FJXdo",
"title": "Page Must Remove Wiki References",
"body": "The wiki should no longer be referenced. Please either move content to these pages or else remove the content. Be advised, regarding the wiki content, I received the following ticket:\r\n------------------\r\nHi , \r\nUnder the link https://wiki.owasp.org/index.php/OWASP_Internet_of_Things_Project#tab=Firmware_Analysis\r\n\r\nIn the table:\r\nOn the \"Device Firmware Tools\" row: \r\nİtem: \r\nBinwalk firmware analysis tool ----> https://binwalk.org/ \r\n\r\nleads to bitcoin gambling website \r\n\r\nI checked this with multiple DNS servers. Firefox DOH, Google 8.8.8.8. I think it is not DNS poisoning.\r\n\r\n------------------\r\n\r\nThe above is what led me to find that you are referencing outdated content as I WAS going to direct them here for up-to-date content.",
+ "summary": "The issue highlights the need to remove references to the wiki across the project due to outdated content, specifically pointing out that a link to a firmware analysis tool leads to an unrelated website. It suggests either relocating relevant content to the project's pages or eliminating it entirely. Action is required to update or remove the wiki references to ensure accuracy and relevance.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-internet-of-things/issues/8",
@@ -12665,6 +13089,7 @@
"node_id": "MDU6SXNzdWU1OTUzMTY1ODE=",
"title": "sim swapping",
"body": "I read more and more articles about the dangers of sim swapping. Would be nice to have some guidelines on how to prevent such attacks.\r\n\r\nExample article https://www.vice.com/en_us/article/pke9zk/paypal-and-venmo-are-letting-sim-swappers-hijack-accounts",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-community/issues/103",
@@ -12696,6 +13121,7 @@
"node_id": "MDU6SXNzdWU3MTU0ODIxNTM=",
"title": "Broken Links",
"body": "Was going through pages, found plenty of broken references. Ran a [broken URL checker](https://www.deadlinkchecker.com/website-dead-link-checker.asp) against the website, found a lot of broken URLs. Fixed some of them in #290 . Some URLs remain broken:\r\n\r\n```\r\nhttps://support.google.com/mail/forum/AAAAK7un8RU3J3r2JqFNTw/discussion/?hl=en&gpf=d/topic/gmail/3J3r2JqFNTw/discussion\r\nhttps://www.javaworld.com/javaworld/javaqa/2003-05/01-qa-0509-jcrypt.html?page=2\r\nhttp://www.php-security.org/downloads/rips.pdf\r\nhttp://www.seclab.tuwien.ac.at/papers/pixy.pdf\r\nhttp://w2spconf.com/2010/papers/p27.pdf\r\nhttps://www.codemagi.com/blog/post/194\r\nhttps://www.itu.int/rec/T-REC-X.690-200811-I/en\r\nhttps://www.ietf.org/id/draft-ietf-websec-key-pinning-09.txt\r\nhttps://github.com/andresriancho/w3af/blob/master/plugins/grep/csp.py\r\nhttp://blog.php-security.org/archives/76-Holes-in-most-preg_match-filters.html\r\nhttp://www.webapptest.org/ms-access-sql-injection-cheat-sheet-EN.html\r\nhttp://labs.idefense.com/intelligence/vulnerabilities/display.php?id=77\r\nhttp://www.ruxcon.org.au/files/2008/Attacking_Rich_Internet_Applications.pdf\r\nhttp://yehg.net/lab/pr0js/files.php/inspath.zip\r\nhttp://yehg.net/lab/pr0js/files.php/php_brute_force_detect.zip\r\nhttp://www.comptechdoc.org/independent/web/cgi/ssimanual/ssiexamples.html\r\nhttp://www.iss.net/security_center/advice/Exploits/TCP/session_hijacking/default.htm\r\nhttp://www.derkeiler.com/pdf/Mailing-Lists/Securiteam/2002-12/0099.pdf\r\nhttp://archives.neohapsis.com/archives/bugtraq/2002-05/0118.html\r\nhttp://hacker-eliminator.com/trojansymptoms.html\r\nhttp://www.microsoft.com/technet/security/bulletin/MS00-078.mspx\r\nhttps://www.checkmarx.com/Demo/XSHM.aspx\r\nhttps://blog.watchfire.com/wfblog/2008/06/javascript-code.html\r\nhttp://shlang.com/netkill/netkill.html\r\nhttps://cirt.net/code/nikto.shtml\r\nhttps://addons.mozilla.org/en-US/firefox/addon/heartbleed-checker/\r\nhttps://www.ecrimelabs.com/tools/webroot/WebRoot.txt\r\nhttps://www.cs.rice.edu/~scrosby/hash/slides/USENIX-RegexpWIP.2.ppt\r\nhttps://www.checkmarx.com/NewsDetails.aspx?id=23&cat=3\r\nhttps://owasp.org/index.php/Dhiraj_Mishra\r\nhttp://puzzlemall.googlecode.com/files/Session\r\nhttps://owasp.org/index.php/Image:RequestRodeo-MartinJohns.pdf\r\nhttp://windows.stanford.edu/docs/IISsecchecklist.htm\r\nhttp://www.net-security.org/dl/articles/php-file-upload.pdf\r\nhttp://www.windowsitpro.com/Files/18/27072/Webtable_01.pdf\r\nhttps://www.imperva.com/404?aspxerrorpath=/application_defense_center/glossary/forceful_browsing.html\r\nhttp://info.sen.ca.gov/pub/01-02/bill/sen/sb_1351-1400/sb_1386_bill_20020926_chaptered.html\r\nhttps://blog.shapesecurity.com/heartbleed-bug-places-encrypted-user-data-and-webservers-at-risk\r\nhttps://www.mitre.org/sites/default/files/publications/pr-18-2417-deliver-uncompromised-MITRE-study-8AUG2018.pdf\r\nhttp://www.microsoft.com/technet/security/bulletin/ms04-028.mspx\r\nhttp://www.digitaldwarf.be/products/mangle.c\r\nhttp://projects.info-pull.com/mokb/\r\nhttp://www.bonsai-sec.com/en/research/untidy-xml-fuzzer.php\r\nhttps://support.snyk.io/snyk-cli/how-can-i-set-a-snyk-cli-project-as-open-source\r\nhttp://www.rubcast.rub.de/index2.php?id=1009\r\nhttp://nvlpubs.nist.gov/nistpubs/SpecialPublications/NIST.SP.800-53r4.pdf\r\nhttp://aeditor.rubyforge.org/ruby_cplusplus/index.html\r\nhttps://owasp-skf.gitbook.io/asvs-write-ups/filename-injection\r\nhttp://tomcat.apache.org/tomcat-6.0-doc/config/context.html\r\nhttps://blog.48bits.com/2010/09/28/iis6-asp-file-upload-for-fun-and-profit/\r\nhttp://palisade.plynt.com/issues/2006Jun/injection-stored-procedures/\r\nhttp://www.bindshell.net/tools/odysseus\r\nhttp://www.ntobjectives.com/products/firewater/\r\nhttp://home.intekom.com/rdawes/exodus.html\r\nhttp://www.wastelands.gen.nz/odysseus/index.php\r\nhttp://www.webcohort.com/web_application_security/research/tools.html\r\nhttp://www.rsasecurity.com/standards/ssl/basics.html\r\nhttp://palisade.plynt.com/issues/2005Aug/page-tokens/\r\nhttp://www.microsoft.com/mspress/books/toc/5612.asp\r\nhttp://www.seczone.cn/2018/06/27/codesec源代码安全检测平台/\r\n```\r\n\r\nIf anyone wants to go through these, `grep --color=always -nr -Ff broken_urls_left.txt|grep --color=always -v \"broken_\"|sort` will show where those URLs are specifically (might miss some of these, though). Could probably also find a lot of broken internal references by looking for `\"wikilink\"`.",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-community/issues/292",
@@ -12726,6 +13152,7 @@
"node_id": "MDU6SXNzdWU3Mzg2MTMyNTc=",
"title": "HttpOnly needs updates",
"body": "I just finished dealing with `auto-migrated` issues for this article, it could definitely use some content updates:\r\nhttps://github.com/OWASP/www-community/blob/master/pages/HttpOnly.md it still talks about old versions of IE and Opera. \r\n\r\nThis article includes an extensive table that needs re-working after the auto-migration as well (which I did not tackle).\r\n\r\nIs Opera even relevant in 2020? Do we still care about IE with Edge/Edge Chrome?",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-community/issues/314",
@@ -12757,6 +13184,7 @@
"node_id": "I_kwDODQOsk84_TJQD",
"title": "Suggested CSV Injection mitigation does not survive saving and re-opening in Excel",
"body": "https://github.com/OWASP/www-community/blob/master/pages/attacks/CSV_Injection.md\r\n\r\nExcel is commonly used to edit CSV files. Unfortunately when saving CSVs Excel strips out some of the characters which are inserted to prevent the CSV injection. This is unfortunate behaviour from Excel, and should really be fixed there, but I'd like to be able to prevent formulas from being inserted into CSVs and run on my user's computers.\r\n\r\nFor most outputs it's possible to completely disallow cells starting with \"=\", and \"@\", irrespective of quoting. But \"-\" is obviously required for numbers.\r\n\r\nOne suggestion for solving this is inserting an extra tab character, which prevents Excel from removing the quotes.\r\n\r\nhttp://georgemauer.net/2017/10/07/csv-injection.html\r\n\r\nReproduction:\r\n\r\nConsider the following CSV:\r\n```csv\r\na,b\r\n,\"'=1+2\"\r\n```\r\n\r\nOpen the CSV, focus on the cell with the formula, and then move the focus away. Save the CSV, it is saved as:\r\n```\r\na,b\r\n,=1+2\r\n```\r\n\r\nOpen the CSV again, the formula is executed and \"3\" is shown in the cell.",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-community/issues/517",
@@ -12783,6 +13211,7 @@
"node_id": "I_kwDODQOsk85f89NH",
"title": "\"Poor Logging Practice\" page is incomplete",
"body": "The page \"[Poor Logging Practice](https://owasp.org/www-community/vulnerabilities/Poor_Logging_Practice)\" is incomplete and is missing information:\r\n- \"Risk Factors\" says \"TBD\"\r\n- A lot of sections contain dummy values, e.g. \"Examples\", \"Related Attacks\", ...\r\n- The sections say \"good practice\" / \"poor practice\" without ever explaining _why_ it is considered good or bad; this is not very helpful\r\n- Given that this is an OWASP page, and the URL path even includes `.../vulnerabilities/...`, the page never properly mentions what the security aspects are. The only vague security related statement it contains is:\r\n > It can also cause log messages accidentally returned to the end users, revealing internal information to attackers.\r\n \r\n > When the use of system output streams is jumbled together with the code that uses loggers properly, the result is often a well-kept log that is missing critical information. In addition, using system output streams can also cause log messages accidentally returned to end users, revealing application internal information to attackers.\r\n \r\n (I am not completely sure though what \"cause log messages accidentally returned to the end users\" is supposed to mean here, is that about log injection (that has its [own page already](https://owasp.org/www-community/attacks/Log_Injection))?)\r\n\r\nIf there are really security aspects to this, then it would be good if they are more clearly highlighted (especially for non-`static final` loggers and multiple loggers). Otherwise if this is just general programming advice maybe this should not be part of the OWASP articles?\r\n",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-community/issues/734",
@@ -12809,6 +13238,7 @@
"node_id": "I_kwDODQOsk85-u1K1",
"title": "Add to \"Changing Registered Email Address For An Account\" page to describe guidelines for System accounts with multiple email addresses",
"body": "> The other thing that bothers me about this - and it absolutely depends on context, what type of app, etc - this flow seems to assume/expect there to be only a single, authoritative email address for the user. Not just identification, but the identity itself. Contrast that to apps (e.g. like Github) wherein you can add multiple email addresses to a single user account. It is an architectural consideration to be sure, but that changes a lot of perspective as well. \r\n> E.g. just adding a 2nd email address to an existing account, you would simply reauthenticate the user, and then send notification-only email to all the other existing addresses on the account (as you do note that Google does), with an option to react or cancel (eg something like \"If this wasnt you, let us know\"). \r\nAnd cannot remove an address or change the primary, until the 2nd is approved. \r\n>\r\n> I would like to make a suggestion, to review other large / reputable / reliable sites to compare this proposed flow with what they actually do, and their existing threat model for that flow. E.g. you mentioned Google, I mentioned Github - worth digging in a bit. My assertion is that we'll find that very few actually go through all this. \r\nThat said, to repeat my earlier comment: it really depends on context and type of app :-)\r\n\r\n_Originally posted by @avidouglen in https://github.com/OWASP/www-community/issues/843#issuecomment-1878447314_\r\n ",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-community/issues/874",
@@ -12835,6 +13265,7 @@
"node_id": "I_kwDODQOsk85_mgs8",
"title": "device cookie lockout list storage advice?",
"body": "On the device cookies idea: https://owasp.org/www-community/Slow_Down_Online_Guessing_Attacks_with_Device_Cookies\r\n\r\n\r\nHow would you store the lockout list entries?\r\n\r\nThe individual entries expire, no?\r\n\r\nPlus you might want a quick reference to \"device cookie\" -> banned(bool), or \"IP\" -> limited(bool), or \"username\" -> limited(bool)\r\n\r\nIs it meant to be stored as an in-memory KV? or stored in Redis for clustering?\r\n\r\nWhat would an ideal table layout be?",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-community/issues/885",
@@ -12861,6 +13292,7 @@
"node_id": "I_kwDODQOsk85_zRGz",
"title": "Broken images on Session Fixation Page",
"body": "Image links are broken on https://github.com/OWASP/www-community/blob/master/pages/attacks/Session_fixation.md\r\nURL: https://owasp.org/www-community/attacks/Session_fixation",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-community/issues/888",
@@ -12892,6 +13324,7 @@
"node_id": "I_kwDODQOsk86BUlda",
"title": "Combining Password Hash Plus Limited Preimage Data To Improve Password Hash Security",
"body": "Hi All,\r\n\r\nI am posting this question here with the hope that web security pros can chime in. \r\n\r\nThe question was prompted by [my effort to deal](https://github.com/jetty/jetty.project/issues/11489) with weak password hashing in the Jetty application container's default web app security options.\r\n\r\n- Password hashes protect against plain passwords, meaning access to a credential database doesn't automatically mean the ability to use those credentials to access corresponding accounts. \r\n\r\n- In doing so however, we are then faced with the issue of pre-computed hashes, whose preimage string could be used to access user accounts even when a user's actual password differs, because of hash collision. There are rainbow tables with such pre-computed hashes.\r\n\r\n- The mitigations are now a number of hashing schemes intended to make it expensive for an attacker to compute these hashes thus dissuade someone from trying.\r\n\r\n**_In addition to existing mitigations, has there been any consideration given to the idea of pairing hashes with limited password data to counteract hash exploits?_** \r\n\r\nWhat if the option is added to include a limited set of randomly extracted characters from the source password (preimage) along with the position of said characters, then store that information along with the hash. In other words, in addition to what we do now: MD5:PASSWORD_HASH (jetty example), the developer would optionally append one or more sequences of: **PasswordCharacterPasswordCharacterPosition**.\r\n\r\nBelow is an example of what the resulting password hash entry could look like. The hash length is fixed so we know the **t** at the end is the first source password(preimage) data character, the integer after is the position of that character within the source password, the **:** is a delimiter for the limited password data sequence.\r\n\r\n`MD5:3bcc3177cb5efa4d7341d42af8f06418t2:%7`\r\n\r\nWhen a user attempts to authenticate, you validate the hash per usual then additionally validate that those randomly saved password characters are indeed present in the password at the corresponding positions. This would largely nuke the utility of rainbow tables since exploiting hashes is now coupled with the need to in essence know something about the actual source password that was used to create it.\r\n\r\nOf course the password data does reveal some bit of information about the password for anyone who has access to the database, but with a strong enough password this should be a non-issue I think (correct me on this). I imagine there is some scheme that can indicate by how much a password is weaken if you reveal characters and their corresponding position?\r\n\r\n\r\nNow rubbing my Darwin beard as I wait for the take down :)\r\n",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-community/issues/901",
@@ -12918,6 +13351,7 @@
"node_id": "I_kwDODQOsk86CKkHQ",
"title": "Community Events page has a difficult to understand format",
"body": "The code that generates a list of community events from Meetup:\r\n\r\npages/social/community_events.md\r\n\r\ngenerates output like:\r\n\r\n\r\n\r\nThis is confusing, as the end time is not 4 pm, but another time entirely. This is actually saying the time zone in the image. \r\n\r\nCould the time zone be separated out and made more obvious?\r\n\r\nTime: 10:30 (-4 America/Toronto) \r\n\r\nor just\r\n\r\nTime: 10:30 am (America/Toronto)",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-community/issues/905",
@@ -12933,8 +13367,8 @@
"repository": 596,
"assignees": [],
"labels": [
- 108,
- 112
+ 112,
+ 108
]
}
},
@@ -12943,10 +13377,11 @@
"pk": 451,
"fields": {
"nest_created_at": "2024-09-11T20:17:22.147Z",
- "nest_updated_at": "2024-09-11T20:17:22.147Z",
+ "nest_updated_at": "2024-09-13T03:58:11.863Z",
"node_id": "I_kwDODQOsk86V3tch",
"title": "Would OWASP be interested in publishing a guide on how to do cross-organization mTLS?",
"body": "I've been working with cross-organization mTLS for quite a while and the standard guidance (just do whatever you want) is remarkably terrible. \r\n\r\nWould OWASP be interested in publishing a guide on how to do it right that focuses on security, operations, and not emailing certificates around?",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-community/issues/991",
@@ -12973,6 +13408,7 @@
"node_id": "I_kwDODQOii85DhMco",
"title": "Regional Chapter Naming",
"body": "From: John DiLeo @johndileo\r\n\r\nWhat is the intended meaning of the statement \"Branches should follow the naming rules of City chapters\"? Is it intended that branches have no naming to indicate they're part of a Regional Chapter, and that their naming make them indistinguishable from City Chapters? It has been our convention to include each branch's city after the name of the chapter (e.g., \"OWASP New Zealand - Auckland\"). If I'm reading the proposed policy correctly, we would be required to rename our branches to \"OWASP Auckland,\" etc., eliminating their identity as part of our Regional Chapter.",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-policy/issues/57",
@@ -13003,6 +13439,7 @@
"node_id": "I_kwDODQOii85DhNnx",
"title": "Chapter and Branch Leader Limits",
"body": "From: John DiLeo @johndileo\r\n\r\nPlease clarify the limits on Regional Chapter and Regional Branch leaders' recognition as OWASP Leaders. Is it intended that OWASP grant leader benefits to up to five (5) Regional Chapter Leaders, plus up to five (5) branch leaders, as the draft policy seems to state?\r\n\r\nIf 'yes,' I recommend adjusting the Branch Leader limit to the greater of five (5), or one (1) per Branch, in addition to the five (5) Regional Chapter leaders.\r\n\r\nIf 'no,' I recommend adjusting the overall limit to the greater of five (5), or three (3) plus one (1) per Branch (e.g., for OWASP New Zealand, the initial limit would be six)\"",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-policy/issues/59",
@@ -13032,6 +13469,7 @@
"node_id": "I_kwDODQOii85DhPVD",
"title": "Reference Meeting Platform Consistently",
"body": "From: Lisa Jones @latf6711 \r\n\r\nWe need to be consistent when referring to Meetup in the Policy. Under shared services, we call it a chapter scheduling service for meetings and local events. Under meeting and activity requirements, it is called the OWASP meeting platform.",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-policy/issues/61",
@@ -13061,6 +13499,7 @@
"node_id": "I_kwDODQOii85DhQLn",
"title": "Remove Soft Requirement for Listed Events",
"body": "From: Lisa Jones @latf6711 \r\n\r\nIs this a requirement? If not, I would suggest it be removed.\r\n\r\nNB: Per the Policy above, if you misconfigure or do not use the above automation, you will need to do this step manually. Once your event is complete, please add it to the past events tab. For a more detailed example, see [https://owasp.org/www-projectchapter-example/]",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-policy/issues/62",
@@ -13090,6 +13529,7 @@
"node_id": "I_kwDODQOii85DhRH5",
"title": "Audit Operations Should Remain With Staff",
"body": "From: Lisa Jones @latf6711 \r\n\r\nThe chapter committee is going to complete operational audit tasks on Regional Chapters? How is this going to be accomplished?",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-policy/issues/63",
@@ -13119,6 +13559,7 @@
"node_id": "I_kwDODQOii85DhRvn",
"title": "Nonresponsive Leaders",
"body": "From: Lisa Jones @latf6711 \r\n\r\nThere needs to be a recourse for leaders not responding. We give them an email address, increased response time to nine days, and the email can be forwarded to any other platform. ",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-policy/issues/64",
@@ -13148,6 +13589,7 @@
"node_id": "I_kwDODQOii85DhSYy",
"title": "Soft Requirement for Monitoring",
"body": "From: Lisa Jones @latf6711 \r\n\r\nThis statement does not make sense to me? \r\n**Leaders should** monitor and participate in the OWASP Leaders List, other Google groups, and the Slack platform.",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-policy/issues/65",
@@ -13177,6 +13619,7 @@
"node_id": "I_kwDODQOii85DhYZk",
"title": "Use of OWASP Trademarks on Chapter Social Media",
"body": "From: Lisa Jones @latf6711 \r\n\r\nChapters are currently allowed to use the OWASP name and logo in various places such as social media accounts as they wish with no guidelines or consequences. This needs to be reviewed. OWASP® is now a registered trademark of the Foundation. ",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-policy/issues/66",
@@ -13205,6 +13648,7 @@
"node_id": "I_kwDODQOii85Dhabp",
"title": "Number of Accounts",
"body": "From: Lisa Jones @latf6711 \r\n\r\nShared Services\r\nHow many accounts are city/student/regional/branch chapters entitled to have?\r\n",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-policy/issues/67",
@@ -13235,6 +13679,7 @@
"node_id": "I_kwDODQOii85Dhaqp",
"title": "Restrict Advertising Events/Activities not from OWASP",
"body": "From: Lisa Jones @latf6711 \r\n\r\nThe use of other organizations' tools and platforms is displayed on the chapter page connected to a URL that needs to be restricted. The promoting of OTHER organizations' events and activities on the OWASP website and OWASP provided tools needs to be prohibited.",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-policy/issues/68",
@@ -13263,6 +13708,7 @@
"node_id": "I_kwDODQOii85DhdQT",
"title": "City Chapters are not restricted to the City Named",
"body": "From: Lisa Jones @latf6711 \r\n\r\n`City chapters are defined for a single city only.` This is an incorrect statement. City chapters were named after the primary meeting place of the chapter. City chapters were never defined strictly to that city.\r\n\r\nDefinition of defined: having a definite outline or specification; precisely marked or stated. ",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-policy/issues/70",
@@ -13292,6 +13738,7 @@
"node_id": "I_kwDODQOii85Dhdz9",
"title": "Chapter Leader Residency Requirements",
"body": "From: Lisa Jones @latf6711 \r\n\r\n`City chapter leaders should reside in the same country as their city chapter.` \r\n\r\nA leader could possibly need to take a plane to get to the city of the chapter in some countries. The above statement was added to the chapter policy. This defeats the building of community in that city and could eliminate the physical participation and assist in holding the meeting. This will lead to leaders in name only to fulfill the 2 leader minimum. ",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-policy/issues/71",
@@ -13320,6 +13767,7 @@
"node_id": "I_kwDODQOii85Dhekz",
"title": "Student Chapters Requirements not Operationally Feasible",
"body": "From: Lisa Jones @latf6711 \r\n\r\n`Student chapters do not need to meet minimum student leadership requirements during the semester or year breaks. Still, they must come back into compliance within 30 days of starting a new semester or year.`\r\n\r\nWho will keep track of the different schools semester dates and calendar breaks? The person requesting the chapter should still need to create the page within 30 days of approval. Access to the OWASP GitHub page is not governed by each school's breaks. There are only 3 meeting activities required over a 12 month period. I am not sure why the statement was added. It is not necessary and creates more confusion. ",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-policy/issues/72",
@@ -13349,6 +13797,7 @@
"node_id": "I_kwDODQOii85Dhl8J",
"title": "Leaders Without Benefits not Operationally Feasible",
"body": "From: Lisa Jones @latf6711 \r\n\r\n```\r\nRegional Chapter Leadership\r\nRegional Leadership management is the same as per City chapters;\r\nRegional chapters must have at least three and usually a maximum of five OWASP Foundation-recognized leaders. If the region has more than five associated locations, it can have up to seven (7) leaders.\r\n```\r\n\r\nThe two statements above contradict each other and should be eliminated. If regional chapters are allowed to have 7 leaders but only 5 are eligible for leader benefits. This can not be managed as is. ",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-policy/issues/73",
@@ -13378,6 +13827,7 @@
"node_id": "I_kwDODQOii85DhmyP",
"title": "Force Requirement to Accept Github Invitation For All",
"body": "From: Lisa Jones @latf6711 \r\n\r\n`At least one leader, but preferably all leaders, must accept the GitHub invite before it expires. A leader with chapter repository admin rights can grant other leaders admin access.\r\n\r\nThis statement is creating a single point of failure. This is why we ask for a minimum of 2 leaders. This contradicts the requirements in the request ticket and the statement in Share Services. \r\n",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-policy/issues/74",
@@ -13407,6 +13857,7 @@
"node_id": "I_kwDODQOii85DhorL",
"title": "How to Validate Starting a New City Chapter Requirements",
"body": "From: Lisa Jones @latf6711 \r\n\r\nRegarding regional chapters...\r\n\r\n`All “Starting a New City chapter” requirements must be met`\r\n\r\nHow will the this be validated?",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-policy/issues/75",
@@ -13436,6 +13887,7 @@
"node_id": "I_kwDODQOii85DhpWy",
"title": "Operational Overhead and Clarification - City/Regional Chapter Agreement ",
"body": "From: Lisa Jones @latf6711 \r\n\r\n`All City chapter leaders within the defined region must agree to the creation of the new Regional Chapter, and there must be no dissenting City chapter leaders within the region. `\r\n\r\nThe agreement needs to be documented and kept on file in case of future disagreements. No verbal agreement will be accepted. \r\n\r\nFrom Me: And does that mean any new city chapters in the region get to have a say after the regional is formed?",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-policy/issues/76",
@@ -13465,6 +13917,7 @@
"node_id": "I_kwDODQOii85DhqAo",
"title": "Regional Approval Process",
"body": "From: Lisa Jones @latf6711 \r\n\r\n`The OWASP Foundation will only create regional Chapters with the approval of the Chapter Committee after confirming regional policy requirements are met. `\r\n\r\nWhat is the process for this to be completed? How will the chapter committee confirm that the requirements have been met? How often will they review and respond?",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-policy/issues/77",
@@ -13494,6 +13947,7 @@
"node_id": "I_kwDODQOii85DhrzZ",
"title": "Operational Functionality Should not be Handled By Committee",
"body": "From: Lisa Jones @latf6711 \r\n\r\nWho will oversee that the Chapter Committee is fulfilling and completing their responsibilities that are outlined in the policy?\r\nIf they are not completed in a reasonable timeframe or there is an issue who should we forward the inquiries to?",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-policy/issues/79",
@@ -13523,6 +13977,7 @@
"node_id": "I_kwDODQOii85Dhwa9",
"title": "Emphasize Enabling the Community",
"body": "From: Dirk Wetter @drwetter\r\n\r\nThe additional sentence emphasizes enabling the community. \r\n\r\n**Current:** `Chapters are central to OWASP’s mission of building a community of appsec professionals and developers around the world. `\r\n\r\n**Suggested**: `As OWASP's mission is bringing software security to the people, it is essential that the OWASP foundation is enabling the community and supporting them wherever possible. Chapters are central to OWASP’s mission of building a community of appsec professionals and developers around the world.\r\n\r\n`",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-policy/issues/80",
@@ -13552,6 +14007,7 @@
"node_id": "I_kwDODQOii85DhzHR",
"title": "Committees Operational Monitoring and Communication Clarification",
"body": "From: Dirk Wetter @drwetter \r\n\r\n**Current:** `The Chapter Committee will additionally monitor this yearly.`\r\n\r\n**Suggested:** `The Chapter Committee will additionally monitor this yearly and discuss deficiencies with the Regional Chapter if necessary.`\r\n\r\nThe reasoning is to establish communication before taking action.",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-policy/issues/83",
@@ -13577,21 +14033,22 @@
"pk": 473,
"fields": {
"nest_created_at": "2024-09-11T20:18:02.645Z",
- "nest_updated_at": "2024-09-11T20:18:02.645Z",
+ "nest_updated_at": "2024-09-13T15:21:31.443Z",
"node_id": "I_kwDODQOii85Or3Xy",
"title": "Bylaws reconcilliation - 15% everywhere",
"body": "Let's make all splits/admin overhead 15% throughout all policies.\r\n",
- "state": "open",
- "state_reason": "",
+ "summary": "",
+ "state": "closed",
+ "state_reason": "completed",
"url": "https://github.com/OWASP/www-policy/issues/104",
"number": 104,
"sequence_id": 1320121842,
"is_locked": false,
"lock_reason": "",
"comments_count": 1,
- "closed_at": null,
+ "closed_at": "2024-09-13T12:28:52Z",
"created_at": "2022-07-27T21:06:14Z",
- "updated_at": "2022-07-28T12:53:26Z",
+ "updated_at": "2024-09-13T12:28:52Z",
"author": 198,
"repository": 597,
"assignees": [
@@ -13607,21 +14064,22 @@
"pk": 474,
"fields": {
"nest_created_at": "2024-09-11T20:18:04.368Z",
- "nest_updated_at": "2024-09-11T20:18:04.368Z",
+ "nest_updated_at": "2024-09-13T15:21:32.824Z",
"node_id": "I_kwDODQOii85Or3vH",
"title": "Bylaw Reconcilliation - Update Membership Policy for new bylaws",
"body": "- Individual members - all forms of \r\n- Complimentary members - no vote\r\n- Leaders - must be a financial member\r\n- Leadership requirements",
- "state": "open",
- "state_reason": "",
+ "summary": "",
+ "state": "closed",
+ "state_reason": "completed",
"url": "https://github.com/OWASP/www-policy/issues/105",
"number": 105,
"sequence_id": 1320123335,
"is_locked": false,
"lock_reason": "",
"comments_count": 0,
- "closed_at": null,
+ "closed_at": "2024-09-13T12:28:52Z",
"created_at": "2022-07-27T21:08:05Z",
- "updated_at": "2022-07-27T21:08:05Z",
+ "updated_at": "2024-09-13T12:28:52Z",
"author": 198,
"repository": 597,
"assignees": [
@@ -13637,21 +14095,22 @@
"pk": 475,
"fields": {
"nest_created_at": "2024-09-11T20:18:05.983Z",
- "nest_updated_at": "2024-09-11T20:18:05.983Z",
+ "nest_updated_at": "2024-09-13T15:21:34.189Z",
"node_id": "I_kwDODQOii85Ouk7Z",
"title": "Bylaw Policy Reconcilliation - spell check",
"body": "",
- "state": "open",
- "state_reason": "",
+ "summary": "",
+ "state": "closed",
+ "state_reason": "completed",
"url": "https://github.com/OWASP/www-policy/issues/106",
"number": 106,
"sequence_id": 1320832729,
"is_locked": false,
"lock_reason": "",
"comments_count": 0,
- "closed_at": null,
+ "closed_at": "2024-09-13T12:28:52Z",
"created_at": "2022-07-28T12:05:39Z",
- "updated_at": "2022-07-28T12:05:40Z",
+ "updated_at": "2024-09-13T12:28:52Z",
"author": 198,
"repository": 597,
"assignees": [
@@ -13667,21 +14126,22 @@
"pk": 476,
"fields": {
"nest_created_at": "2024-09-11T20:18:07.717Z",
- "nest_updated_at": "2024-09-11T20:18:07.717Z",
+ "nest_updated_at": "2024-09-13T15:21:35.518Z",
"node_id": "I_kwDODQOii85OulA3",
"title": "Bylaw Policy Reconcilliation - Markdown Lint",
"body": "",
- "state": "open",
- "state_reason": "",
+ "summary": "",
+ "state": "closed",
+ "state_reason": "completed",
"url": "https://github.com/OWASP/www-policy/issues/107",
"number": 107,
"sequence_id": 1320833079,
"is_locked": false,
"lock_reason": "",
"comments_count": 0,
- "closed_at": null,
+ "closed_at": "2024-09-13T12:28:53Z",
"created_at": "2022-07-28T12:05:57Z",
- "updated_at": "2022-07-28T12:05:57Z",
+ "updated_at": "2024-09-13T12:28:53Z",
"author": 198,
"repository": 597,
"assignees": [
@@ -13697,21 +14157,22 @@
"pk": 477,
"fields": {
"nest_created_at": "2024-09-11T20:18:09.456Z",
- "nest_updated_at": "2024-09-11T20:18:09.456Z",
+ "nest_updated_at": "2024-09-13T15:21:36.975Z",
"node_id": "I_kwDODQOii85OulI7",
"title": "Bylaw Policy Reconcilliation - Change address to Edgewater Place",
"body": "",
- "state": "open",
- "state_reason": "",
+ "summary": "",
+ "state": "closed",
+ "state_reason": "completed",
"url": "https://github.com/OWASP/www-policy/issues/108",
"number": 108,
"sequence_id": 1320833595,
"is_locked": false,
"lock_reason": "",
"comments_count": 0,
- "closed_at": null,
+ "closed_at": "2024-09-13T12:28:53Z",
"created_at": "2022-07-28T12:06:24Z",
- "updated_at": "2022-07-28T12:06:24Z",
+ "updated_at": "2024-09-13T12:28:53Z",
"author": 198,
"repository": 597,
"assignees": [
@@ -13727,21 +14188,22 @@
"pk": 478,
"fields": {
"nest_created_at": "2024-09-11T20:18:11.145Z",
- "nest_updated_at": "2024-09-11T20:18:11.145Z",
+ "nest_updated_at": "2024-09-13T15:21:38.402Z",
"node_id": "I_kwDODQOii85OunTE",
"title": "Bylaws policy reconcilliation - remove references to the wiki",
"body": "Search for wiki & change to website\r\nSearch for index.php and find new URL",
- "state": "open",
- "state_reason": "",
+ "summary": "",
+ "state": "closed",
+ "state_reason": "completed",
"url": "https://github.com/OWASP/www-policy/issues/109",
"number": 109,
"sequence_id": 1320842436,
"is_locked": false,
"lock_reason": "",
"comments_count": 0,
- "closed_at": null,
+ "closed_at": "2024-09-13T12:28:53Z",
"created_at": "2022-07-28T12:14:05Z",
- "updated_at": "2022-07-28T12:14:05Z",
+ "updated_at": "2024-09-13T12:28:53Z",
"author": 198,
"repository": 597,
"assignees": [
@@ -13757,21 +14219,22 @@
"pk": 479,
"fields": {
"nest_created_at": "2024-09-11T20:18:12.823Z",
- "nest_updated_at": "2024-09-11T20:18:12.823Z",
+ "nest_updated_at": "2024-09-13T15:21:39.745Z",
"node_id": "I_kwDODQOii85Ounle",
"title": "Bylaws policy reconcilliation - remove outdated chapter handbook / advice",
"body": "Point to the new Chapter Handbook",
- "state": "open",
- "state_reason": "",
+ "summary": "",
+ "state": "closed",
+ "state_reason": "completed",
"url": "https://github.com/OWASP/www-policy/issues/110",
"number": 110,
"sequence_id": 1320843614,
"is_locked": false,
"lock_reason": "",
"comments_count": 0,
- "closed_at": null,
+ "closed_at": "2024-09-13T12:28:54Z",
"created_at": "2022-07-28T12:15:11Z",
- "updated_at": "2022-07-28T12:15:11Z",
+ "updated_at": "2024-09-13T12:28:54Z",
"author": 198,
"repository": 597,
"assignees": [
@@ -13787,21 +14250,22 @@
"pk": 480,
"fields": {
"nest_created_at": "2024-09-11T20:18:14.479Z",
- "nest_updated_at": "2024-09-11T20:18:14.479Z",
+ "nest_updated_at": "2024-09-13T15:21:41.086Z",
"node_id": "I_kwDODQOii85OvQlZ",
"title": "Bylaw policy reconcilliation - election policy update",
"body": "Add detail about non-voting members\r\nAdd detail around bylaw amendment, CoI and replacement",
- "state": "open",
- "state_reason": "",
+ "summary": "",
+ "state": "closed",
+ "state_reason": "completed",
"url": "https://github.com/OWASP/www-policy/issues/112",
"number": 112,
"sequence_id": 1321011545,
"is_locked": false,
"lock_reason": "",
"comments_count": 0,
- "closed_at": null,
+ "closed_at": "2024-09-13T12:28:54Z",
"created_at": "2022-07-28T14:22:10Z",
- "updated_at": "2022-07-28T14:22:11Z",
+ "updated_at": "2024-09-13T12:28:54Z",
"author": 198,
"repository": 597,
"assignees": [
@@ -13817,21 +14281,22 @@
"pk": 481,
"fields": {
"nest_created_at": "2024-09-11T20:18:16.126Z",
- "nest_updated_at": "2024-09-11T20:18:16.126Z",
+ "nest_updated_at": "2024-09-13T15:21:42.450Z",
"node_id": "I_kwDODQOii85OvRRI",
"title": "Update COVID policy - July 2022",
"body": "Change to endemic coverage",
- "state": "open",
- "state_reason": "",
+ "summary": "",
+ "state": "closed",
+ "state_reason": "completed",
"url": "https://github.com/OWASP/www-policy/issues/113",
"number": 113,
"sequence_id": 1321014344,
"is_locked": false,
"lock_reason": "",
"comments_count": 0,
- "closed_at": null,
+ "closed_at": "2024-09-13T12:28:54Z",
"created_at": "2022-07-28T14:24:10Z",
- "updated_at": "2022-07-28T14:24:10Z",
+ "updated_at": "2024-09-13T12:28:54Z",
"author": 198,
"repository": 597,
"assignees": [
@@ -13847,21 +14312,22 @@
"pk": 482,
"fields": {
"nest_created_at": "2024-09-11T20:18:17.767Z",
- "nest_updated_at": "2024-09-11T20:18:17.767Z",
+ "nest_updated_at": "2024-09-13T15:21:43.822Z",
"node_id": "I_kwDODQOii85OwThK",
"title": "Update signing authority to include staff",
"body": "Staff have a BAU requirement to approve expenses. We need a limit in the signing authority policy. \r\n",
- "state": "open",
- "state_reason": "",
+ "summary": "",
+ "state": "closed",
+ "state_reason": "completed",
"url": "https://github.com/OWASP/www-policy/issues/114",
"number": 114,
"sequence_id": 1321285706,
"is_locked": false,
"lock_reason": "",
"comments_count": 0,
- "closed_at": null,
+ "closed_at": "2024-09-13T12:28:55Z",
"created_at": "2022-07-28T17:54:15Z",
- "updated_at": "2022-07-28T17:54:15Z",
+ "updated_at": "2024-09-13T12:28:55Z",
"author": 198,
"repository": 597,
"assignees": [
@@ -13877,21 +14343,22 @@
"pk": 483,
"fields": {
"nest_created_at": "2024-09-11T20:18:19.413Z",
- "nest_updated_at": "2024-09-11T20:18:19.413Z",
+ "nest_updated_at": "2024-09-13T15:21:45.186Z",
"node_id": "I_kwDODQOii85UugpF",
"title": "Chapter Policy",
"body": "I'm afraid I have to disagree with referencing a 1997 memo from IETF to write an operational business policy. Polices need to be enforceable, with consequences for noncompliance. It also needs to be simple and understandable, even for non-technical individuals. \r\nLast, it needs to be manageable as just a part of a single team member's job responsibilities.\r\n\r\n \r\nWords such as **SHALL\", \"SHALL NOT,\" \"SHOULD,\" \"SHOULD NOT,\" \"RECOMMENDED,\" \"MAY,\" and \"OPTIONAL\"** \r\nImmediately, are not policy and if removed will shorten the document. {The RFC 2119 - Keywords for use in [RFCs ](https://www.ietf.org/rfc/rfc2119.txt)to Indicate Requirement Levels in March 1997.}\r\n\r\n**Definitions:**\r\n- Polices are requirements that are enforceable with consequences.\r\n- Guidelines are recommendations that are not enforceable with NO consequences.\r\n- Procedures establish the defined practices or steps to accomplish the objective.\r\n\r\n### Characteristics of Business Policies\r\n**Effective policies have the following characteristics:**\r\n\r\n1. It must be clear, specific, and easily understood\r\n2. Specify company rules\r\n3. Explain their purpose\r\n4. State when policy should be applied\r\n5. Who it covers\r\n6. Method of enforcement\r\n7. Describe the consequences of non-compliance\r\n\r\n**Policy Writing Guidance**\r\n1. Keep it simple. Policies should be written in plain language – not legalese. ...\r\n2. Keep it general. Policies cannot contemplate all possible situations. ...\r\n3. Make it relevant. ...\r\n4. Check for accuracy and compliance. ...\r\n5. Ensure the policy can be enforced. ...\r\n6. Clearly state who does what. ...\r\n7. Less is more.\r\n\r\n**Benefits of Policies**\r\nWell-written policies give employees a way to handle problems and issues without constantly involving management whenever they need to make a decision. Policies define the limits of decision-making and outline the alternatives. Employees understand the constraints of their jobs. Reactivation can be justified on a case-by-case basis. \r\n\r\n\r\n",
- "state": "open",
- "state_reason": "",
+ "summary": "",
+ "state": "closed",
+ "state_reason": "completed",
"url": "https://github.com/OWASP/www-policy/issues/119",
"number": 119,
"sequence_id": 1421478469,
"is_locked": false,
"lock_reason": "",
"comments_count": 1,
- "closed_at": null,
+ "closed_at": "2024-09-13T12:28:55Z",
"created_at": "2022-10-24T21:31:10Z",
- "updated_at": "2022-10-25T19:48:22Z",
+ "updated_at": "2024-09-13T12:28:55Z",
"author": 200,
"repository": 597,
"assignees": [],
@@ -13903,21 +14370,22 @@
"pk": 484,
"fields": {
"nest_created_at": "2024-09-11T20:18:20.282Z",
- "nest_updated_at": "2024-09-11T20:18:20.282Z",
+ "nest_updated_at": "2024-09-13T15:21:45.899Z",
"node_id": "I_kwDODQOii85U0eCq",
"title": "Leaders",
"body": "- Leaders are not required to be paid OWASP members yet we give them a FREE OWASP account that we don't require them to use. \r\n- I want the statement added to the policy that all OWASP Foundation communications, requests, and expense reimbursement approvals. will be sent to the leaders' OWASP email addresses. \r\n- Allowing 60 days for a leader to respond is not reasonable. The IRS requires expenses to be submitted within 60 days. Members will wait at most a week (7 days) for a leader to respond to an email sent.\r\n\r\n\r\n",
- "state": "open",
- "state_reason": "",
+ "summary": "",
+ "state": "closed",
+ "state_reason": "completed",
"url": "https://github.com/OWASP/www-policy/issues/120",
"number": 120,
"sequence_id": 1423040682,
"is_locked": false,
"lock_reason": "",
"comments_count": 0,
- "closed_at": null,
+ "closed_at": "2024-09-13T12:28:55Z",
"created_at": "2022-10-25T20:32:56Z",
- "updated_at": "2022-10-25T20:32:56Z",
+ "updated_at": "2024-09-13T12:28:55Z",
"author": 200,
"repository": 597,
"assignees": [],
@@ -13929,21 +14397,22 @@
"pk": 485,
"fields": {
"nest_created_at": "2024-09-11T20:18:21.102Z",
- "nest_updated_at": "2024-09-11T20:18:21.102Z",
+ "nest_updated_at": "2024-09-13T15:21:46.584Z",
"node_id": "I_kwDODQOii85U5g2F",
"title": "Expense Policy ",
"body": "For the items listed above and expenses under USD $250, there are no pre-set limits beyond the “fair and reasonable” test. Expenses not listed and exceeding USD $250 require approval from at least two leaders (or a leader + relevant committee if there is only one leader), and [pre-approval](https://owasporg.atlassian.net/servicedesk/customer/portal/4/group/14) by the Executive Director or their designate. When in doubt, individuals requesting expense reimbursement should apply for pre-approval.\r\n\r\n[](https://owasporg.atlassian.net/servicedesk/customer/portal/4/group/14) > This link goes to Funding Grant Requests.\r\n\r\nAndrew had me create a JIRA ticket for [Pre-Approval of Chapter Expenses](https://owasporg.atlassian.net/servicedesk/customer/portal/8/group/20/create/107). If I need to remove the ticket and chapter leaders need to use the Funding Grant Request ticket, please let me know if it is causing confusion. ",
- "state": "open",
- "state_reason": "",
+ "summary": "",
+ "state": "closed",
+ "state_reason": "completed",
"url": "https://github.com/OWASP/www-policy/issues/121",
"number": 121,
"sequence_id": 1424362885,
"is_locked": false,
"lock_reason": "",
"comments_count": 0,
- "closed_at": null,
+ "closed_at": "2024-09-13T12:28:56Z",
"created_at": "2022-10-26T16:44:35Z",
- "updated_at": "2022-10-26T16:44:35Z",
+ "updated_at": "2024-09-13T12:28:56Z",
"author": 200,
"repository": 597,
"assignees": [],
@@ -13959,6 +14428,7 @@
"node_id": "I_kwDODQOii85Vw3zI",
"title": "Supporters and Bartering Arrangements",
"body": "This section is very confusing to leaders. \r\nMaybe seperate the Bartering arrangements for the Contractual supporter agreements.\r\nThe (https://contact.owasp.org/) goes to General is there a specific topic the leader should submit to?\r\n\r\n\r\nSupporters and Bartering Arrangements\r\nChapter leaders are encouraged to obtain local chapter supporters via bartering arrangements (i.e., services, event spaces, or food and beverages paid for by a chapter supporter) and donations via the OWASP website. Chapters can define the levels and benefits of local Chapter Supporters, including logos on the introduction slides and the Chapter home page. Any contractual agreement, bartering arrangement, or financial transaction must be registered and processed by the OWASP Foundation through our [service desk](https://contact.owasp.org/)",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-policy/issues/122",
@@ -13985,6 +14455,7 @@
"node_id": "I_kwDODQOii85V4F0Q",
"title": "Chapter Funding Pre-Approval URL needs to be updated",
"body": "Shared Services\r\nOWASP Foundation will provide chapters with the following shared services at no cost. Chapters are encouraged to make use of these. These services can be accessed by submitting a ticket via [Contact Us](https://contact.owasp.org/). If, after 9 business days have passed, and reasonable efforts have been made to utilize shared services, no response is received, the chapter may, with due care, seek a reasonable alternative, filling out a [Chapter Funding Pre-Approval]**(https://owasporg.atlassian.net/servicedesk/customer/portal/8/group/20/create/107*) ticket.\r\n\r\nUpdate the Chapter Funding Pre-Approval URL to https://owasporg.atlassian.net/servicedesk/customer/portal/4\r\n",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-policy/issues/123",
@@ -14011,6 +14482,7 @@
"node_id": "I_kwDODQOii85bcEhZ",
"title": "دانشجو",
"body": "",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-policy/issues/126",
@@ -14037,6 +14509,7 @@
"node_id": "I_kwDODQOii857DbIm",
"title": "Events Policy \"Events in a box\" link dead",
"body": "https://owasp.org/www-policy/operational/events\r\n\r\nAT the bottom the link to \"Events in a box\" is dead.",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-policy/issues/136",
@@ -14063,6 +14536,7 @@
"node_id": "I_kwDODQOii8584LR8",
"title": "Google's feedback ",
"body": "\n\n",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-policy/issues/137",
@@ -14085,10 +14559,11 @@
"pk": 491,
"fields": {
"nest_created_at": "2024-09-11T20:18:26.302Z",
- "nest_updated_at": "2024-09-11T20:18:26.302Z",
+ "nest_updated_at": "2024-09-13T15:21:47.278Z",
"node_id": "I_kwDODQOii85_Zp9K",
"title": "Website shows ancient WIP draft Chapters policy",
"body": "When browsing on the OWASP website (owasp.org) and you browse to chapter policies\r\n\r\nAbout->Policies\r\n\r\nThen select [Chapters Policy](https://owasp.org/www-policy/operational/chapters)\r\n\r\nYou will notice on the top right a \"next\" selection entitled \"Chapters Policy - Draft (WIP)\"\r\n\r\nWhen selecting this entry you will be sent to a page that is out of date and states:\r\n\r\n\"Members are invited to provide feedback on this draft policy until January 22, 1970\"\r\n\r\nThis page needs to be removed or at least updated.",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-policy/issues/138",
@@ -14111,10 +14586,11 @@
"pk": 492,
"fields": {
"nest_created_at": "2024-09-11T20:18:30.994Z",
- "nest_updated_at": "2024-09-11T20:18:30.994Z",
+ "nest_updated_at": "2024-09-13T15:21:50.896Z",
"node_id": "I_kwDODP8dT85BDtP1",
"title": "Incorrect Mail To links",
"body": "For the linked emails each email has // before the email address so the mail to address will not function correctly. \r\nExample: mailto://test@owasp.org instead of mailto:test@owasp.org\r\nThis is also present on the \"OWASP WIA, Diversity and Inclusion Committee\" page.",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-compliance/issues/1",
@@ -14137,10 +14613,11 @@
"pk": 493,
"fields": {
"nest_created_at": "2024-09-11T20:18:35.207Z",
- "nest_updated_at": "2024-09-11T20:18:35.207Z",
+ "nest_updated_at": "2024-09-13T15:21:54.350Z",
"node_id": "I_kwDODP8ZEc5AJp1h",
"title": "Consider renaming default branch to \"main\"",
"body": "Please consider renaming the default branch from \"master\" to \"main\"\r\n\r\nThe process, and detailed explanation/justification can be found at this link:\r\nhttps://github.com/github/renaming\r\n\r\nIf possible, could this be done on every other OWASP open source project? Some companies are getting stricter about inclusive language, and this can help them ensure that their own code that references OWASP projects stays in compliance with design standards.",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-committee-wia/issues/6",
@@ -14167,6 +14644,7 @@
"node_id": "I_kwDODOEwJ86Hksim",
"title": "OWASP Mobile Top 10 refers to MASVS ",
"body": "Hi,\r\nCan we do (and help me to) mapping OWASP Mobile Top 10 to MASVS ?\r\n\r\nFor example:\r\nM1 (2024) - Improper Credential Usage:\r\n - MASVS-STORAGE\r\n - MASVS-...\r\nM2 (2024) - Inadequate Supply Chain Security:\r\n - MASVS-...\r\n - MASVS-...\r\n...\r\n...\r\nM10 (2024) - Insufficient Cryptography:\r\n - MASVS-...\r\n - MASVS-...\r\n\r\nThanks.",
+ "summary": "The issue discusses the need for mapping the OWASP Mobile Top 10 vulnerabilities to the Mobile Application Security Verification Standard (MASVS). The request includes examples for specific vulnerabilities, like M1 (Improper Credential Usage) and M2 (Inadequate Supply Chain Security), and seeks to establish corresponding MASVS categories for each. To address this, a detailed mapping of all M1 to M10 vulnerabilities to their relevant MASVS counterparts needs to be created.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-mobile-top-10/issues/63",
@@ -14189,10 +14667,11 @@
"pk": 495,
"fields": {
"nest_created_at": "2024-09-11T20:19:00.154Z",
- "nest_updated_at": "2024-09-11T20:19:00.154Z",
+ "nest_updated_at": "2024-09-13T15:22:13.415Z",
"node_id": "I_kwDODOEwJ86OePLH",
"title": "How to mapping OWASP Mobile Top 10 to CWE",
"body": "Dear Crew Staff\r\nI'm a researcher of mobile secuity. OWASP Mobile top 10 is the critical issue to solve and discuss so according to my research, I wonder if there is a mapping table that can help me to map owasp mobile top 10 and CWE or owasp top 10. I survey lots of document and nothing can solve my problem. Could anyone help me if the mapping table exist? Or the inner document can release privately. Thanks a lot.",
+ "summary": "The issue discusses the need for a mapping table that connects the OWASP Mobile Top 10 vulnerabilities to the Common Weakness Enumeration (CWE) or the OWASP Top 10. The researcher has not found any existing documentation that addresses this need and is seeking assistance in locating such a mapping or obtaining related documents privately. It may be helpful to explore existing resources or databases that specialize in vulnerability mappings or to consider creating a new mapping table if one does not exist.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-mobile-top-10/issues/64",
@@ -14215,10 +14694,11 @@
"pk": 496,
"fields": {
"nest_created_at": "2024-09-11T20:19:11.900Z",
- "nest_updated_at": "2024-09-11T20:19:11.900Z",
+ "nest_updated_at": "2024-09-13T15:22:22.506Z",
"node_id": "I_kwDODMGC3c5qYHjA",
"title": "run a daily workflow action to check all links ",
"body": "It would be good to automate checking the links for the SecurityRAT OWASP project pages, in particular :\r\n* https://securityrat.org/\r\n* https://securityrat.github.io/",
+ "summary": "Implement a daily workflow action to automate link checking for SecurityRAT OWASP project pages. Focus on the following URLs:\n\n- https://securityrat.org/\n- https://securityrat.github.io/\n\n1. Utilize a CI/CD tool like GitHub Actions to schedule the daily workflow.\n2. Set up a link-checking tool, such as `html-proofer` or `linkchecker`, within the workflow.\n3. Create a script that runs the link checker against the specified URLs.\n4. Configure the workflow to trigger on a daily schedule using cron syntax.\n5. Ensure notifications are sent (e.g., via email or Slack) if broken links are detected.\n6. Test the workflow to confirm it runs correctly and handles errors gracefully.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-securityrat/issues/6",
@@ -14245,6 +14725,7 @@
"node_id": "MDU6SXNzdWU1OTQzODU5Mzc=",
"title": "v1.0 release versions seems not to be working",
"body": "I downloaded the Raspberry Pi 2 image, used balenaEtcher to write it to the SD card. Then I had tried booting it in Raspberry Pi 4 , but all I got is just a black screen, I also tried to boot it in Raspberry Pi 2, but it just hangs with the rainbow screen. Both versions were tried (sysupgrade and the other one). Later on, I have tried using another software for writing to SD – Win32DiskImager. But that didn‘t helped. To make sure that this is not related to my machine, I used other computers to write to the card, but the effect is the same. \r\n\r\nSome additional information\r\n•\tOS that I use: Windows 10\r\n•\tSize of the SD cards I‘ve tried to write to: 64 GB and 16 GB\r\n\r\nI‘ve also tried VDI image with VirtualBox (version 6.1.4 r136177). It gets to the GRUB loader, but after this, it just crashes. From what I can see from logs, It looks like that it results in critical error when trying to boot the kernel. \r\n\r\nI’ve also downloaded VMware to check if this would work, but unfortunately this seems to be not working also. After the grub loader screen, it starts booting, but at some point (crng init done), it just hangs. I’ve waited for about 40 minutes, but nothing progressed.\r\n",
+ "summary": "There are issues with the v1.0 release versions not booting correctly on Raspberry Pi devices. The user attempted to write the Raspberry Pi 2 image to an SD card using multiple tools (balenaEtcher and Win32DiskImager) and tested it on both Raspberry Pi 4 and 2, but encountered a black screen and a hanging rainbow screen, respectively. Additionally, attempts to run the image in VirtualBox and VMware resulted in crashes or hanging after the GRUB loader. The user is using Windows 10 and has tried both 64 GB and 16 GB SD cards. \n\nTo address this issue, it may require investigating the integrity of the release images or exploring compatibility with the Raspberry Pi models.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/IoTGoat/issues/2",
@@ -14271,6 +14752,7 @@
"node_id": "MDU6SXNzdWU2NzUxNjQ2NTU=",
"title": "IoTGoat as a container",
"body": "Run IoTGoat within a docker container https://github.com/openwrt/docker",
+ "summary": "The issue suggests running IoTGoat within a Docker container, referencing the OpenWrt Docker repository. To address this, the next steps involve creating a suitable Dockerfile or configuration that can encapsulate IoTGoat, ensuring its dependencies and environment are properly set up for containerization.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/IoTGoat/issues/4",
@@ -14293,10 +14775,11 @@
"pk": 499,
"fields": {
"nest_created_at": "2024-09-11T20:19:18.133Z",
- "nest_updated_at": "2024-09-12T00:15:15.904Z",
+ "nest_updated_at": "2024-09-13T17:00:02.383Z",
"node_id": "I_kwDODKzRYM5uc4an",
"title": "Not able to build from source",
"body": "Hello. I am facing an error while building from the source. The error is regarding absence of gcc and g++ but in my kali linux virtual machine I have gcc and g++ v13.1.0. \r\n\r\n",
+ "summary": "The issue reported involves an error during the build process from source, specifically indicating that gcc and g++ are absent despite having version 13.1.0 installed on a Kali Linux virtual machine. To resolve this, it may be beneficial to check the system paths for gcc and g++, ensure that they are correctly set, or verify that the build environment is configured to recognize these compilers.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/IoTGoat/issues/9",
@@ -14323,6 +14806,7 @@
"node_id": "I_kwDODKzRYM5zJY1p",
"title": "port 5000 closed by default?",
"body": "upnp port 5000 seems to be closed. tired restarting the machine (VM in oracle virtual box). do I need to activate upnp service myself?\r\n\r\n",
+ "summary": "The issue reports that port 5000 appears to be closed by default, even after restarting the virtual machine in Oracle VirtualBox. The user is questioning whether they need to manually activate the UPnP service to resolve this. To address the problem, it may be necessary to check the configuration settings related to UPnP and ensure that the service is enabled and properly configured.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/IoTGoat/issues/10",
@@ -14345,10 +14829,11 @@
"pk": 501,
"fields": {
"nest_created_at": "2024-09-11T20:21:59.383Z",
- "nest_updated_at": "2024-09-11T20:21:59.383Z",
+ "nest_updated_at": "2024-09-13T15:24:28.713Z",
"node_id": "I_kwDODGgRDc5nCnH-",
"title": "Non-Responsive Website",
"body": "## Issue\r\nThe website does not appear to be responsive and does not adapt to different screen sizes or resolutions. As a result, the content gets distorted, and the layout becomes unorganized, making it challenging to read and access the information.\r\n\r\n## Impact\r\nThe lack of responsiveness negatively affects user experience and hinders accessibility. With the increasing number of users accessing websites from mobile devices, it is crucial to ensure a responsive design to provide a seamless browsing experience.\r\n\r\nSteps to Reproduce:\r\n\r\n- Open the website on a Chrome or Firefox browser.\r\n- Resize the browser window to simulate different screen sizes or resolutions.\r\n- Observe the layout and content display.\r\n- Expected Behavior:\r\nThe website should adapt to different screen sizes, maintaining a consistent layout, readability, and usability across devices.\r\n\r\n## Actual Behavior\r\nThe website does not adjust its layout when viewed on smaller devices, resulting in content overlapping, text being cut off, and elements becoming difficult to interact with.\r\n\r\n## Attached Screenshots\r\n\r\n\r\nPlease let me know if you require any further information to address this issue effectively. I believe that resolving this problem will greatly enhance the user experience of the OWASP Netherlands Chapter website.\r\n\r\nThank you for your attention to this matter.\r\n\r\n",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-chapter-netherlands/issues/1",
@@ -14371,10 +14856,11 @@
"pk": 502,
"fields": {
"nest_created_at": "2024-09-11T20:22:27.862Z",
- "nest_updated_at": "2024-09-11T20:22:27.862Z",
+ "nest_updated_at": "2024-09-13T15:24:50.928Z",
"node_id": "MDU6SXNzdWU4MjAxNDY4ODQ=",
"title": "Blank link and broken image",
"body": "On the index page edgescan is a blank link and the EY image is broken - assuming this should be Mmk?",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-chapter-dublin/issues/2",
@@ -14397,10 +14883,11 @@
"pk": 503,
"fields": {
"nest_created_at": "2024-09-11T20:25:44.120Z",
- "nest_updated_at": "2024-09-11T20:25:44.120Z",
+ "nest_updated_at": "2024-09-13T15:55:13.001Z",
"node_id": "I_kwDODGgJzM5mQudb",
"title": "Social Media Links no longer work",
"body": "While trying to get ahold of the OWASP chapter in omaha, I found the following links no longer work:\r\n- https://www.facebook.com/blu3gl0w13\r\n- https://www.instagram.com/blu3gl0w13/",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-chapter-omaha/issues/27",
@@ -14423,10 +14910,11 @@
"pk": 504,
"fields": {
"nest_created_at": "2024-09-11T20:30:51.744Z",
- "nest_updated_at": "2024-09-11T20:30:51.744Z",
+ "nest_updated_at": "2024-09-13T15:59:21.954Z",
"node_id": "MDU6SXNzdWU5MjA2Nzg1OTU=",
"title": "Please re-activate using activation policy",
"body": "Ricardo and Hector,\r\n\r\nThe Paraguay chapter has been suspended due to inactivity. The last meeting activity on the OWASP chapter page was March of 2019. The chapter has not submitted the ticket to request shared services like the Meetup Pro account and zoom to host virtual meetings. \r\n\r\nMany chapters failed to complete the chapter re-activation process and have been deactivated. This doesn't mean gone forever; it means you need to take some additional steps, very similar to what you needed to do before the deadlines. Under the Chapter Policy, you can contact the Chapter Committee and ask for new leadership or elections, or you can follow this re-activation process:\r\n\r\nThe complete re-activation process:\r\nFind at least 50% new leadership. There is a two leader minimum requirement for all new and re-activated chapters; ensure that the chapter requirements do not fall to one person. If life gets busy for one leader, members can still have chapter meetings in their area.Enter a ticket to re-activate your chapter, and use this ticket to add the new leaders to your chapter.https://owasporg.atlassian.net/servicedesk/customer/portal/7/group/18/create/73 ALL requested information for all the leaders is to be included in the ticket to process.Once the GitHub and Meetup are active, a minimum of two leaders has access to GitHub and Meetup. Preferably all leaders should have access to spread the load.Follow the migration instructions to customize your chapter home page:Simple to follow instructions https://owasp.org/migration/Simple to follow video https://youtu.be/tEm-YCeQno0 If you are getting build errors, install a local Jekyll instance following the instructions in the Migration Guide and work out what's wrong. If you still can't fix it, please log a non-funding request at https://contact.owasp.org straight away.Schedule a meeting or activity that the public can attend to occur within 90 days, and make sure it's on your chapter's home page. If you use Meetup Pro, add this to your index.md file to automatically be included:{% include chapter_events.html group=page.meetup-group %}This only works if the OWASP Foundation is the organizer of your Meetup, you are co-organizers and the metadata on the index.md includes your meetup groups name:\r\nmeetup-group: OWASP-Colorado-Springs-Meetup\r\nLastly, it would be best if you kept in contact with your members, speakers, the Chapter Committee, or the OWASP Foundation by monitoring and responding to your owasp.org email address. It's okay to forward this email address to another you use, but we strongly prefer leaders using the owasp.org email address for official chapter business. The policy requires you to respond within 7 to 30 days depending on the request, so please make sure you're regularly checking mails, particularly in the lead-up to your meetings.\r\n\r\nYou can find the Monthly Slides and many helpful resources, such as the speaker's bureau at the Chapter Committee's Resources page:\r\nhttps://owasp.org/www-committee-chapter/#div-resources_for_chapters\r\nYou can find the Chapter Policy, to which this process complies here: \r\nhttps://owasp.org/www-policy/operational/chapters\r\nThe Chapter policy requires that a meeting is held within 90 days of re-activation, or leadership will again be cleared and the chapter deactivated. \r\n\r\nWe look forward to seeing many more meetings from your chapter! \r\n\r\nThanks,\r\n\r\nAndrew van der Stock, Executive Director\r\nLisa Jones, Community Manager",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-chapter-asuncion/issues/4",
@@ -14449,10 +14937,11 @@
"pk": 505,
"fields": {
"nest_created_at": "2024-09-11T20:31:05.853Z",
- "nest_updated_at": "2024-09-11T20:31:05.853Z",
+ "nest_updated_at": "2024-09-13T15:59:33.409Z",
"node_id": "I_kwDODGgAjc5fOqRT",
"title": "Como fazer parte?",
"body": "Olá! Já sou membro no OWASP, gostaria de fazer parte do chapter SP.\r\n\r\nObrigado.",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-chapter-sao-paulo/issues/1",
@@ -14475,10 +14964,11 @@
"pk": 506,
"fields": {
"nest_created_at": "2024-09-11T20:31:39.399Z",
- "nest_updated_at": "2024-09-11T20:31:39.399Z",
+ "nest_updated_at": "2024-09-13T16:00:00.501Z",
"node_id": "MDU6SXNzdWU1NjM3MjExODk=",
"title": "error with _layouts/col-sidebar.htmlI ",
"body": "Hi!\r\n\r\nIm in troubles...During the push I got an error message that I can't do anything with:\r\n\r\n> The page build failed for the `master` branch with the following error:\r\n> \r\n> A file was included in `/_layouts/col-sidebar.html` that is a symlink or does not exist in your `_includes` directory. For more information, see https://help.github.com/en/github/working-with-github-pages/troubleshooting-jekyll-build-errors-for-github-pages-sites#file-is-a-symlink.\r\n\r\nBUT... the file is not in my repo ??\r\n /_layouts/col-sidebar.htmlI don't find it in the repo. I\r\n\r\nI think this is an error yet informed by other capters (I saw it in germany chapter)\r\n\r\nTIA!!",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-chapter-chile/issues/2",
@@ -14505,6 +14995,7 @@
"node_id": "I_kwDODGf8oM551hKp",
"title": "Make flyer to invite to discord server",
"body": "",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-chapter-albuquerque/issues/3",
@@ -14531,6 +15022,7 @@
"node_id": "I_kwDODGf8oM551hO0",
"title": "Print more business cards",
"body": "",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-chapter-albuquerque/issues/4",
@@ -14553,10 +15045,11 @@
"pk": 509,
"fields": {
"nest_created_at": "2024-09-11T20:33:06.843Z",
- "nest_updated_at": "2024-09-11T20:33:06.843Z",
+ "nest_updated_at": "2024-09-13T16:01:09.074Z",
"node_id": "I_kwDODGf8oM551hT3",
"title": "Find contacts for other colleges in area",
"body": "",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-chapter-albuquerque/issues/5",
@@ -14579,10 +15072,11 @@
"pk": 510,
"fields": {
"nest_created_at": "2024-09-11T20:33:45.402Z",
- "nest_updated_at": "2024-09-11T20:33:45.403Z",
+ "nest_updated_at": "2024-09-13T16:01:39.087Z",
"node_id": "MDU6SXNzdWU3MDQ0OTQyNjA=",
"title": "Info.md file with wrong linkage",
"body": "Please update your links in info.md to:\r\n1.) Remove Become a Member (it is at the top of the page)\r\n2.) Remove www and index.php references that are holdovers from the wiki (use the owasp.org and current links)",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-chapter-cairo/issues/1",
@@ -14605,10 +15099,11 @@
"pk": 511,
"fields": {
"nest_created_at": "2024-09-11T20:33:52.920Z",
- "nest_updated_at": "2024-09-11T20:33:52.920Z",
+ "nest_updated_at": "2024-09-13T16:01:45.022Z",
"node_id": "I_kwDODGf7Oc6Euih_",
"title": "Social links broken",
"body": "I am interested in a local meet up! I noticed that the social links are broken. Are there updated links?",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-chapter-halifax/issues/1",
@@ -14631,10 +15126,11 @@
"pk": 512,
"fields": {
"nest_created_at": "2024-09-11T20:36:38.848Z",
- "nest_updated_at": "2024-09-11T20:36:38.848Z",
+ "nest_updated_at": "2024-09-13T16:03:56.523Z",
"node_id": "I_kwDODGf2JM6BytHM",
"title": "Please add a description to this project",
"body": "",
+ "summary": "The issue requests the addition of a project description to provide clarity and context for users. It is important to create a concise and informative summary of the project's purpose, features, and usage guidelines to enhance understanding and engagement. Consider drafting a description that highlights the key aspects of the project.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-internet-of-things-top-10/issues/3",
@@ -14657,10 +15153,11 @@
"pk": 513,
"fields": {
"nest_created_at": "2024-09-11T20:37:00.746Z",
- "nest_updated_at": "2024-09-11T20:37:00.746Z",
+ "nest_updated_at": "2024-09-13T04:12:43.304Z",
"node_id": "I_kwDODGf1dM5zL19X",
"title": "Update utilities section for Dragon-GPT",
"body": "It would be good to add [Dragon-GPT ](https://github.com/LuizBoina/dragon-gpt)into the documentation, and update the section on utilities",
+ "summary": "Update the utilities section in the documentation to include Dragon-GPT. Ensure that you provide a comprehensive overview of Dragon-GPT's features, installation instructions, and usage examples. \n\nFirst, clone the Dragon-GPT repository to your local machine. Review the current documentation structure and identify where the utilities section is located. Draft content that highlights the advantages of using Dragon-GPT, emphasizing key functionalities and potential use cases. \n\nConsider adding code snippets to demonstrate how to integrate Dragon-GPT into existing workflows. Finally, submit a pull request with your updates for review.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-threat-dragon/issues/66",
@@ -14690,6 +15187,7 @@
"node_id": "MDU6SXNzdWU1NTI5ODU5NzY=",
"title": "Add synonyms/related terms?",
"body": "\"Password spraying\" - testing a list of credentials for a known account - is a subset of credential cracking but the term is getting increased global coverage ([ACSC](https://www.cyber.gov.au/threats/advisory-2019-130), [Mitre](https://attack.mitre.org/techniques/T1110/), [ArsTechnica](https://arstechnica.com/information-technology/2020/01/iranian-hackers-have-been-password-spraying-the-us-grid/)). [Google trends](https://trends.google.com/trends/explore?date=today%205-y&geo=US&q=%22password%20spraying%22,%22credential%20cracking%22) shows that password spraying captures far more searches than credential cracking.\r\n\r\n\"Password replay\", \"credential replay\", and \"password reuse attacks\" have all been used to refer to credential stuffing ([NCSC](https://www.ncsc.gov.uk/news/alert-vpn-vulnerabilities), [Krebs](https://krebsonsecurity.com/tag/credential-replay-attacks/), [1password](https://blog.1password.com/how-to-protect-yourself-from-password-reuse-attacks/)). Credential stuffing is the more popular term but the others keep popping up.\r\n\r\nThe differences, if there are any, across these terms is confusing. There isn't a central location that captures the relationships and readers are left googling terms that net poor results.\r\n\r\nIncluding terms that become common in popular usage will also help drive OWASP awareness.",
+ "summary": "The issue highlights the need for clarity surrounding the terminology related to credential attacks, specifically \"password spraying,\" \"password replay,\" \"credential replay,\" and \"credential stuffing.\" It points out that while certain terms gain popularity and usage, there is a lack of a centralized resource to explain their relationships and differences. The suggestion is to add synonyms and related terms to improve understanding and awareness within the OWASP community. Action may involve compiling a comprehensive list of these terms and their definitions for better accessibility.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-automated-threats-to-web-applications/issues/1",
@@ -14712,10 +15210,11 @@
"pk": 515,
"fields": {
"nest_created_at": "2024-09-11T20:37:21.085Z",
- "nest_updated_at": "2024-09-11T20:37:21.085Z",
+ "nest_updated_at": "2024-09-13T16:04:29.614Z",
"node_id": "I_kwDODGf08s56hh6D",
"title": "Will there be any update plan?",
"body": "The last update was 4 years ago, will there be any update plan?",
+ "summary": "The issue raises a concern about the lack of updates for the project, highlighting that the last update was four years ago. It inquires about the possibility of a future update plan. To address this, it may be beneficial to provide a roadmap or outline any upcoming developments to reassure users.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-automated-threats-to-web-applications/issues/3",
@@ -14738,10 +15237,11 @@
"pk": 516,
"fields": {
"nest_created_at": "2024-09-11T20:37:28.792Z",
- "nest_updated_at": "2024-09-11T20:37:28.792Z",
+ "nest_updated_at": "2024-09-13T16:04:35.675Z",
"node_id": "MDU6SXNzdWU4NTkxMzI5NDY=",
"title": "Can we update the benchmark scorecards?",
"body": "I was looking at the _\"tool support / results\"_ tab:\r\n\r\n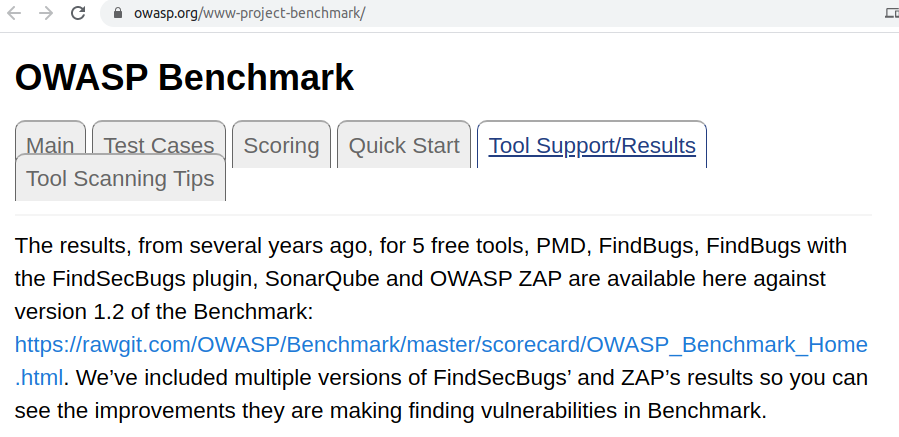\r\n\r\nAnd found that we have very nice results in this link:\r\nhttps://rawgit.com/OWASP/Benchmark/master/scorecard/OWASP_Benchmark_Home.html\r\n\r\nHowever:\r\n- [ ] It was generated in 2016, can we run results today and update the image?\r\n- [ ] We ([Fluid Attacks](https://fluidattacks.com)) would like to include our security vulnerability detection tool in which we've recently evaluated results against the benchmark:\r\n - https://fluidattacks.com/blog/owasp-benchmark-fluid-attacks/ \r\n - https://docs.fluidattacks.com/machine/scanner/reproducibility (this link is experimental)\r\n \r\n Could you please give us some orientation on how to appear in the results? here: https://owasp.org/www-project-benchmark/ \r\n- [ ] rawgit.com \"RawGit has reached the end of its useful life\", can we host it in other place?\r\n\r\nI volunteer myself for any task needed, just let me know how could we push this forward\r\n\r\nThanks!",
+ "summary": "Update the benchmark scorecards to reflect current results. Follow these steps:\n\n1. **Run Updated Benchmarks**: Re-evaluate the benchmark results as the existing data is from 2016. Ensure to use the latest tools and methodologies available.\n\n2. **Include Fluid Attacks Tool**: Integrate the security vulnerability detection tool from Fluid Attacks into the benchmark. Review their evaluation results documented in:\n - https://fluidattacks.com/blog/owasp-benchmark-fluid-attacks/\n - https://docs.fluidattacks.com/machine/scanner/reproducibility (note: this link is experimental)\n\n3. **Host Scorecard Elsewhere**: Since RawGit is no longer viable, find an alternative hosting solution for the scorecard, such as GitHub Pages or another static site hosting service.\n\n4. **Coordinate Contributions**: Reach out to contributors for assistance and collaboration on these tasks. Utilize the volunteers, including the one who offered help, to manage the update process efficiently.\n\n5. **Document the Process**: Ensure to keep thorough documentation of changes made and the rationale behind them to maintain transparency.\n\nProceed with these steps to enhance the benchmark scorecards and integrate new tools effectively.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-benchmark/issues/7",
@@ -14764,10 +15264,11 @@
"pk": 517,
"fields": {
"nest_created_at": "2024-09-11T20:37:32.842Z",
- "nest_updated_at": "2024-09-11T20:37:32.842Z",
+ "nest_updated_at": "2024-09-13T16:04:39.575Z",
"node_id": "I_kwDODGf0rc5C0F52",
"title": "Sort list entries by GH stars and/or age (instead of alphabetically)",
"body": "**As an** author of a popular and up-to-date vulnerable application **I want** that app to show up at the top of the relevant VWAD lists **so that** the lists in general represent more of a ranking than an index.\r\n\r\nWith the current implementation of grabbing the GitHub star badges only (i.e. not having the actual number of stars available during rendering) this is probably not possible. The actual number of stars needs to be retrieved in some way. This could for example be a nightly job creating a simple JSON file in the repo that could then be processed during rendering.",
+ "summary": "The issue highlights the need to sort list entries by GitHub stars or age instead of the current alphabetical order. The goal is to ensure that popular and up-to-date applications appear at the top of the VWAD lists, creating a ranking system rather than a simple index. The current setup only retrieves GitHub star badges, lacking the actual star count during rendering. A proposed solution involves implementing a nightly job to collect and store the star counts in a JSON file, which can then be used during the rendering process.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-vulnerable-web-applications-directory/issues/72",
@@ -14793,10 +15294,11 @@
"pk": 518,
"fields": {
"nest_created_at": "2024-09-11T20:37:54.397Z",
- "nest_updated_at": "2024-09-11T20:37:54.397Z",
+ "nest_updated_at": "2024-09-13T16:04:56.268Z",
"node_id": "MDU6SXNzdWU1NTE4OTkzNjQ=",
"title": "Wiki Deck pages",
"body": "The previous wiki deck have not been recreated on the new GitHub site. The archived media wiki pages are at:\r\n\r\nhttps://wiki.owasp.org/index.php/Cornucopia_-_Ecommerce_Website_Edition_-_Wiki_Deck\r\n\r\nWondering if there is a way to script the autogeneration of multiple language versions of card pages from say an xml data file. Can anyone suggest ideas\"",
+ "summary": "The issue highlights that the previous wiki deck has not been recreated on the new GitHub site, and provides a link to the archived media wiki pages. It suggests exploring the possibility of scripting the autogeneration of multiple language versions of card pages from an XML data file. Suggestions for implementation ideas are requested. It may be beneficial to research automation tools or libraries that can facilitate this process.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-cornucopia/issues/1",
@@ -14823,10 +15325,11 @@
"pk": 519,
"fields": {
"nest_created_at": "2024-09-11T20:38:02.504Z",
- "nest_updated_at": "2024-09-11T20:38:02.504Z",
+ "nest_updated_at": "2024-09-13T16:05:02.796Z",
"node_id": "I_kwDODGfz8s6BytEW",
"title": "Please add a description to this project",
"body": "",
+ "summary": "The issue requests the addition of a project description to provide clarity and context. To address this, a concise and informative description should be drafted and incorporated into the project documentation.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-hacking-lab/issues/2",
@@ -14849,10 +15352,11 @@
"pk": 520,
"fields": {
"nest_created_at": "2024-09-11T20:38:44.786Z",
- "nest_updated_at": "2024-09-11T20:38:44.786Z",
+ "nest_updated_at": "2024-09-13T16:05:35.916Z",
"node_id": "I_kwDODGfyyM5CvJfI",
"title": "Repetition of Security Checks in Projects ASVS L1 - Authentication Verification Requirements",
"body": "I was navigating through the application and found that there is a repetition of each check in Projects -> ASVS L1 -> Authentication Verification Requirements\r\n\r\nNot sure if this is by design or an error. Though both entries consist of same details! \r\n\r\nhttps://demo.securityknowledgeframework.org/projects/summary/\r\n\r\n\r\n\r\nThank You! \r\n",
+ "summary": "Investigate the issue of repeated security checks in Projects -> ASVS L1 -> Authentication Verification Requirements. Confirm whether this duplication is intentional or a result of an error. \n\n1. Review the code responsible for generating the ASVS L1 section to identify where checks are being added.\n2. Compare the entries to ensure they contain identical details and note any discrepancies.\n3. Check the database or data source to see if the duplication exists at the data level.\n4. If confirmed as an error, implement a fix by removing the duplicates.\n5. Test the application to ensure the changes do not affect other functionalities.\n6. Document the findings and the resolution process for future reference. \n\nEnsure to communicate with the team regarding the findings and possible implications on user experience or security.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-security-knowledge-framework/issues/12",
@@ -14879,6 +15383,7 @@
"node_id": "MDU6SXNzdWU5NzQ5MzM5MDU=",
"title": "Stable IDs for (sub-)Headlines of the Proactive Controles",
"body": "Hi \r\nI wonder if you could generate stable IDs for main headlines or even more detailed subtitles as far as you like, please. This could help anybody to get a stable link to the proactive controls (e.g. references from the OWASP Top 10).\r\nThe IDs should be strctured ID numbers (like in a table of contents). If possible these IDs should be usable as http-anchors to access them from other projects and documents. These IDs should stay stable within a version of the cheat sheets.\r\n\r\nPlease let me know if I can help you.\r\n\r\nThanks and Cheers\r\nTorsten",
+ "summary": "The issue discusses the need for stable IDs for main headlines and subtitles in the Proactive Controls documentation. The proposed IDs would facilitate stable linking and referencing, such as from the OWASP Top 10. The IDs should be structured like those in a table of contents and function as HTTP anchors for use in other projects and documents. It is suggested that these IDs remain stable within a version of the cheat sheets. Assistance is offered for this task. \n\nTo address this issue, consider implementing a structured ID system for the headlines and subtitles in the documentation.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-proactive-controls/issues/8",
@@ -14905,6 +15410,7 @@
"node_id": "I_kwDODGfyac6FDdEt",
"title": "Missing concept #2: Trust boundary ",
"body": "The second concept I'll advocate is trust boundaries. They are also a tremendously important part of \"most important areas of concern that software developers must be aware of. \"\r\n\r\nSuggested core text: \"Know what code is trusted to make security decisions, and why that code is resistant to attack by an attacker with a debugger or a proxy.\"",
+ "summary": "The issue discusses the need to include the concept of \"trust boundaries\" as a critical area of concern for software developers. It emphasizes the importance of understanding which code is trusted to make security decisions and the reasons behind its resilience against potential attacks, such as those using a debugger or proxy. It suggests incorporating this concept into the relevant documentation for better awareness and security practices. To address this, the documentation should be updated to include a clear explanation of trust boundaries and their significance in software security.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-proactive-controls/issues/33",
@@ -14929,10 +15435,11 @@
"pk": 523,
"fields": {
"nest_created_at": "2024-09-11T20:39:00.443Z",
- "nest_updated_at": "2024-09-11T20:39:00.443Z",
+ "nest_updated_at": "2024-09-13T04:14:20.834Z",
"node_id": "I_kwDODGfyac6FOTSq",
"title": "Missing concept: supply chain security",
"body": "Modern web development is most commonly done by composing an application from open-source dependencies. \r\n\r\nI suppose most of the supply chain mitigations are not proactive and that's why they're not here yet, but a proactive approach to supply-chain security is possible and emerging.\r\n\r\nSome aspects of Content-Security-Policy are relevant to this. Similarly, Node.js and Deno offer means to limit access to powerful APIs on the process level in case malicious code gets pulled into an app from dependencies.\r\n\r\nUsing tools that inject themselves early in the process and often don't rely on known vulnerability databases (eg. socket.dev) \r\n\r\nEven more proactively, runtime protections can be introduced - see LavaMoat\r\nIntroduction to the concepts:\r\nhttps://www.w3.org/2023/03/secure-the-web-forward/talks/hardened-supply-chain.html\r\n\r\nI'd be interested to contribute to this site if some guidance is provided.",
+ "summary": "The issue discusses the lack of attention to supply chain security in modern web development, where applications are often built using open-source dependencies. It highlights the need for a proactive approach to supply chain security, mentioning relevant tools and concepts such as Content-Security-Policy, Node.js, Deno, and runtime protections like LavaMoat. The author expresses interest in contributing to the project and seeks guidance on how to proceed. \n\nTo address this issue, it may be beneficial to outline specific steps or areas where contributions are needed related to supply chain security practices and tools.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-proactive-controls/issues/36",
@@ -14963,6 +15470,7 @@
"node_id": "I_kwDODGfyac6U_Y6l",
"title": "check v3 markdown files, move information to cheat sheet series and, remove them from the project",
"body": "we still have the doc/pdfs from the 2018/`v3` series stored. The markdown files in `v3/en` are not used by anything.\r\n\r\nCheck if some of the content from the v3 version could be moved to the owasp cheat sheet series. If so, move it there and remove the markdown files from this project.",
+ "summary": "The issue suggests reviewing the markdown files from the v3 series stored in `v3/en`, as they are not currently in use. It proposes transferring relevant content to the OWASP cheat sheet series and subsequently deleting the markdown files from the project. The next steps would involve checking the content for relevance and making the necessary updates.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-proactive-controls/issues/59",
@@ -14987,10 +15495,11 @@
"pk": 525,
"fields": {
"nest_created_at": "2024-09-11T20:39:09.782Z",
- "nest_updated_at": "2024-09-11T20:39:09.782Z",
+ "nest_updated_at": "2024-09-13T16:05:52.597Z",
"node_id": "I_kwDODGfyKs59hvQk",
"title": "SAMM ToolBox point to incorrect link",
"body": "In the file info.md, the \"SAMM Toolbox v2\" point to a broken Excel spread sheet : https://github.com/owaspsamm/core/releases/download/v2.0.3/SAMM_spreadsheet.xlsx\r\nThe correct ressource is located here : https://github.com/owaspsamm/core/releases/download/v2.0.8/SAMM_spreadsheet.xlsx\r\n",
+ "summary": "The issue reports that the link to the \"SAMM Toolbox v2\" in the info.md file directs to a broken Excel spreadsheet. The correct link to the spreadsheet is provided, which points to version 2.0.8 instead of the broken version 2.0.3. The necessary action involves updating the link in the info.md file to the correct resource.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-samm/issues/4",
@@ -15017,6 +15526,7 @@
"node_id": "I_kwDODGfx7M47bKJz",
"title": "Improve the test coverage",
"body": "Unit and integration tests should be written to make sure that the functionalities still work as expected. It would limit the possible regression issues caused by new development/refactorings, especially since there are several possible configuration permutations.\r\n\r\nDetailed manual testing scenarios, executions flows would be very helpful as well because it could also act as a documentation/functional requirements.",
+ "summary": "Improve test coverage by writing comprehensive unit and integration tests to ensure that all functionalities perform as expected. Focus on limiting regression issues that may arise from new developments or refactorings, especially given the various configuration permutations.\n\nStart by identifying critical functionalities and creating a list of test cases that cover different scenarios. Implement automated tests for both unit and integration levels, prioritizing areas with the highest risk of regression. \n\nAdditionally, document detailed manual testing scenarios and execution flows to serve as both functional requirements and a reference for future testing efforts. \n\nBegin by:\n1. Conducting a code review to identify untested areas.\n2. Creating a test plan that outlines key functionalities and configurations to be tested.\n3. Writing unit tests for individual components.\n4. Developing integration tests that simulate real-world usage with different configurations.\n5. Compiling manual testing documentation for comprehensive coverage.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-csrfguard/issues/24",
@@ -15032,9 +15542,9 @@
"repository": 958,
"assignees": [],
"labels": [
+ 128,
126,
- 127,
- 128
+ 127
]
}
},
@@ -15047,6 +15557,7 @@
"node_id": "I_kwDODGfx7M47bK1c",
"title": "Lots of copied code from the Grouper repository",
"body": "The following classes: \r\n* `org.owasp.csrfguard.config.overlay.ConfigPropertiesCascadeBase` ([original source code](https://github.com/Internet2/grouper/blob/master/grouper-misc/grouperActivemq/dist/bin/edu/internet2/middleware/grouperActivemq/config/ConfigPropertiesCascadeBase.java))\r\n* `org.owasp.csrfguard.config.overlay.ConfigPropertiesCascadeCommonUtils`\r\n* `org.owasp.csrfguard.config.overlay.ConfigPropertiesCascadeUtils`\r\n\r\nwere copied from the [Grouper](https://github.com/Internet2/grouper) repository. \r\n\r\n\r\nIt seems that only a few changes has been made:\r\n* Logging: although the code is commented out, so it's not relevant (`org.owasp.csrfguard.config.overlay.ConfigPropertiesCascadeBase#iLogger`)\r\n* Skipping the Expression Language (EL) related processing in `org.owasp.csrfguard.config.overlay.ConfigPropertiesCascadeBase#propertiesHelper`: again this is only relevant if there are keys with \".elConfig\" suffix\r\n* The following lines of code: \r\n \r\n ```java\r\n //InputStream inputStream = configFile.getConfigFileType().inputStream(configFile.getConfigFileTypeConfig(), this);\r\n try {\r\n //get the string and store it first (to see if it changes later)\r\n String configFileContents = configFile.retrieveContents(this);\r\n configFile.setContents(configFileContents);\r\n result.properties.load(new StringReader(configFileContents));\r\n ```\r\n in `org.owasp.csrfguard.config.overlay.ConfigPropertiesCascadeBase#retrieveFromConfigFiles` which seem to do the same as the original code.\r\n\r\nThe question is, **are these modifications really needed**? If not, the original code could be used as a maven dependency: \r\n```xml\r\n\r\n edu.internet2.middleware.grouper\r\n grouper-activemq\r\n 2.5.29\r\n\r\n```\r\n\r\n**Side note**: the Grouper project is outdated/bulky/poorly written with a lot of duplicated code from the `org.apache.commons:commons-lang3` and other common libraries. It would be nice to replace with a better alternative\r\n",
+ "summary": "Identify and remove duplicated code from the OWASP CSRFGuard repository that originates from the Grouper repository. Specifically, focus on the following classes:\n\n- `org.owasp.csrfguard.config.overlay.ConfigPropertiesCascadeBase`\n- `org.owasp.csrfguard.config.overlay.ConfigPropertiesCascadeCommonUtils`\n- `org.owasp.csrfguard.config.overlay.ConfigPropertiesCascadeUtils`\n\nEvaluate the modifications made to these classes:\n\n1. Review the logging implementation in `ConfigPropertiesCascadeBase#iLogger` to determine if it's necessary.\n2. Assess the impact of skipping Expression Language (EL) processing in `ConfigPropertiesCascadeBase#propertiesHelper`.\n3. Analyze the modifications in `ConfigPropertiesCascadeBase#retrieveFromConfigFiles`, particularly the code that retrieves and loads configuration file contents.\n\nDetermine if the changes are essential. If they are not, consider using the original Grouper code as a Maven dependency:\n\n```xml\n\n edu.internet2.middleware.grouper\n grouper-activemq\n 2.5.29\n\n```\n\nAs a side note, explore the possibility of replacing the outdated and bulky Grouper codebase with more efficient alternatives, particularly avoiding duplicated code from libraries like `org.apache.commons:commons-lang3`. \n\n**First Steps:**\n- Clone the OWASP CSRFGuard repository and set up a development environment.\n- Create a comparison of the copied code against the original code in the Grouper repository using a diff tool.\n- Document the findings regarding the necessity of the modifications.\n- Propose a plan to refactor the code or to replace it with the original Grouper dependency if appropriate.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-csrfguard/issues/25",
@@ -15075,6 +15586,7 @@
"node_id": "I_kwDODGfx7M48hgVP",
"title": "Document the configurations options that enables overwriting values through the web.xml",
"body": "Fix the TODO in: `org.owasp.csrfguard.config.properties.javascript.JavaScriptConfigParameters`",
+ "summary": "Document configuration options to enable overwriting values via `web.xml`. Focus on detailing how to utilize parameters effectively for various scenarios. \n\nAddress the TODO in `org.owasp.csrfguard.config.properties.javascript.JavaScriptConfigParameters`. Investigate the existing code and identify the intended functionality that needs implementation. \n\nFirst steps:\n1. Review the `web.xml` documentation to understand configuration structure.\n2. Explore the `JavaScriptConfigParameters` class to identify the specific TODO and the context around it.\n3. Develop a plan to implement the missing functionality, ensuring compatibility with existing configurations.\n4. Write unit tests to confirm that overwriting values through `web.xml` works as intended after implementation.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-csrfguard/issues/29",
@@ -15090,9 +15602,9 @@
"repository": 958,
"assignees": [],
"labels": [
- 127,
128,
- 129
+ 129,
+ 127
]
}
},
@@ -15105,6 +15617,7 @@
"node_id": "I_kwDODGfx7M48hyEV",
"title": "Add automation to minify and transpile the JS code to ES5",
"body": "- [ ] Releases should work with minified JS code to improve performance.\r\n- [ ] Logging messages should be removed.\r\n- [ ] Transpile to ES5 to support older browsers",
+ "summary": "Implement automation for minifying and transpiling JavaScript code to ES5. \n\n1. Set up a build tool like Webpack, Gulp, or Grunt to handle the minification and transpilation processes.\n2. Configure the chosen tool to minify the JS code, ensuring it runs during the build process for releases. Use UglifyJS or Terser for efficient minification.\n3. Integrate Babel into the build process to transpile JavaScript code to ES5, supporting older browsers. Create a `.babelrc` file with the necessary presets, such as `@babel/preset-env`.\n4. Add a script to the package.json to automate the build process, running both the minification and transpilation commands sequentially.\n5. Remove all logging messages from the production build to enhance performance. Implement a setting to toggle logging during development versus production environments.\n\nBegin by installing the required dependencies and setting up the initial configuration for the build tool of choice.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-csrfguard/issues/31",
@@ -15134,6 +15647,7 @@
"node_id": "I_kwDODGfx7M5h6mfG",
"title": "Create integration tests",
"body": "Currently the integration of the solution is manually validated through the test application.\r\n\r\nIt would be much nicer to automate the scenarios and make it part of the CI build.\r\n\r\nPart of: https://github.com/OWASP/www-project-csrfguard/issues/24",
+ "summary": "Implement integration tests to automate the validation of the solution currently done manually using the test application. Integrate these tests into the CI build process for efficiency and reliability.\n\n**Technical Details:**\n1. Identify key scenarios that require testing within the integration workflow.\n2. Utilize a testing framework compatible with the existing application stack (e.g., JUnit, TestNG).\n3. Write automated test cases that cover all identified scenarios, ensuring they mimic the manual validation process.\n4. Ensure tests can be executed within the CI pipeline using tools like Jenkins, GitHub Actions, or Travis CI.\n5. Verify that test results are logged and reported appropriately after each CI build.\n\n**Possible First Steps:**\n- Review existing manual test cases and document them in a structured format.\n- Set up the chosen testing framework within the project environment.\n- Begin writing automated tests for the most critical scenarios.\n- Configure the CI build to include the execution of these tests.\n- Run initial tests and refine based on feedback and results.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-csrfguard/issues/192",
@@ -15165,6 +15679,7 @@
"node_id": "I_kwDODGfx7M6FarBk",
"title": "Master csrf token incorrectly returned as page token",
"body": "### Discussed in https://github.com/OWASP/www-project-csrfguard/discussions/257\r\n\r\n
\r\n\r\nOriginally posted by **musaka872** March 27, 2024\r\nHi,\r\nI'm trying to integrate csrfguard 4.3.0 in our project. \r\nI've configured it to use per-session tokens and not per-page tokens. But when I receive the token in the response header it is returned as page token in this form `{pageTokens:{\"/page/uri\":\"csrf-token\"}}` and then when I send this token in a subsequent request csrfguard compares {pageTokens:{\"/page/uri\":\"csrf-token\"}} to \"csrf-token\" and it fails.\r\nI debugged CsrfGuardFilter and in handleSession method we have:\r\n```\r\nprivate void handleSession(final HttpServletRequest httpServletRequest, final InterceptRedirectResponse interceptRedirectResponse, final FilterChain filterChain,\r\n final LogicalSession logicalSession, final CsrfGuard csrfGuard) throws IOException, ServletException {\r\n\r\n final String logicalSessionKey = logicalSession.getKey();\r\n\r\n if (new CsrfValidator().isValid(httpServletRequest, interceptRedirectResponse)) {\r\n filterChain.doFilter(httpServletRequest, interceptRedirectResponse);\r\n } else {\r\n logInvalidRequest(httpServletRequest);\r\n }\r\n\r\n final String requestURI = httpServletRequest.getRequestURI();\r\n final String generatedToken = csrfGuard.getTokenService().generateTokensIfAbsent(logicalSessionKey, httpServletRequest.getMethod(), requestURI);\r\n\r\n CsrfGuardUtils.addResponseTokenHeader(csrfGuard, httpServletRequest, interceptRedirectResponse, new TokenTO(Collections.singletonMap(requestURI, generatedToken)));\r\n }\r\n```\r\nIn generateTokenIfAbsent it checks whether the per-page or master token should be generated and generates the correct master token. But then as you can see when TokenTO is created the master token is passed as per-page token and it is send as such in the response header.\r\n\r\nIs this a bug or I'm missing something?\r\n\r\nI don't want to parse the response header to retrieve the \"csrf-token\" that csrfguard returns.\r\n\r\nBest regards,\r\nMartin
",
+ "summary": "Investigate the issue of CSRF token being incorrectly returned as a page token in csrfguard 4.3.0. Confirm that the configuration is set to use per-session tokens, and verify the response header format, which currently appears as `{pageTokens:{\"/page/uri\":\"csrf-token\"}}`. \n\nDebug the `CsrfGuardFilter` class, specifically the `handleSession` method. Observe that the method generates a token using `generateTokensIfAbsent`, which correctly identifies whether to create a master or page token. However, the `TokenTO` constructor incorrectly passes the master token as a per-page token, leading to a mismatch during validation.\n\nAddress the following steps to resolve the issue:\n\n1. Review the implementation of `generateTokensIfAbsent` to ensure it handles master tokens properly.\n2. Modify the `TokenTO` instantiation to correctly identify and pass the generated master token instead of treating it as a page token.\n3. Test the changes to confirm that the response header now reflects the correct token type and that subsequent requests validate successfully. \n\nIf necessary, consult additional documentation on csrfguard or seek input from the community discussion linked in the original issue for further clarification on intended behavior.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-csrfguard/issues/262",
@@ -15180,8 +15695,8 @@
"repository": 958,
"assignees": [],
"labels": [
- 127,
- 130
+ 130,
+ 127
]
}
},
@@ -15190,10 +15705,11 @@
"pk": 532,
"fields": {
"nest_created_at": "2024-09-11T20:39:29.358Z",
- "nest_updated_at": "2024-09-11T20:39:29.358Z",
+ "nest_updated_at": "2024-09-13T16:05:58.377Z",
"node_id": "I_kwDODGfx7M6V-OuV",
"title": "The isValidUrl method in csrfguard.js uses an insecure string-matching technique",
"body": "We had run a scan after upgrading csrfguard library to version 4.3.0 and found below vulnerability with severity 5.4 .\r\nIt also reported that there is no non-vulnerable version of this component.\r\n\r\n**Explanation**\r\nThe csrfguard package is vulnerable to Cross-Site Request Forgery (CSRF). The isValidUrl method in csrfguard.js uses an insecure string-matching technique. Consequently, an attacker could exploit this vulnerability to cause tokens to leak in links to external (attacker-controlled) domains.\r\n\r\n**Version Affected**\r\n[3.1.0,4.4.0]\r\n\r\n**CVSS Details**\r\nSonatype CVSS 3 : 5.4\r\nCVSS Vector : CVSS:3.0/AV:N/AC:L/PR:N/UI:R/S:U/C:L/I:L/A:N",
+ "summary": "Identify and address the vulnerability in the `isValidUrl` method in `csrfguard.js`. This method utilizes an insecure string-matching technique leading to a Cross-Site Request Forgery (CSRF) vulnerability. The vulnerability allows attackers to exploit the method, potentially leaking tokens via links to external, attacker-controlled domains.\n\n**Affected Versions**: 3.1.0 to 4.4.0.\n**Severity**: CVSS score of 5.4.\n\n**First Steps**:\n1. Review the implementation of the `isValidUrl` method for potential flaws in string matching.\n2. Replace the current string-matching logic with a more secure method, such as using regular expressions or a URL parsing library to validate URLs against a whitelist.\n3. Conduct thorough testing to ensure the new implementation effectively mitigates CSRF attacks without introducing new vulnerabilities.\n4. Run security scans post-implementation to confirm the vulnerability is resolved before deploying the updated library.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-csrfguard/issues/299",
@@ -15216,10 +15732,11 @@
"pk": 533,
"fields": {
"nest_created_at": "2024-09-11T20:39:33.539Z",
- "nest_updated_at": "2024-09-11T20:39:33.539Z",
+ "nest_updated_at": "2024-09-13T04:14:37.188Z",
"node_id": "MDU6SXNzdWU1Nzc0OTI2NjQ=",
"title": "Link to the Official CS Website",
"body": "In order to maintain a live updated website, the official website will be the following: https://cheatsheetseries.owasp.org/\r\nCheat sheets will be added in here as ToCs in order to allow for people to link to them if need be.\r\n\r\nThis could be amended once an easy way is created to properly push the live changes from the main repository to the `www` repository of the cheatsheets.",
+ "summary": "The issue discusses the need to link to the official CS website at https://cheatsheetseries.owasp.org/ for maintaining a live updated resource. It suggests that cheat sheets should be added as Table of Contents (ToCs) to facilitate easy linking. Additionally, it mentions that this approach may be revised once a more efficient method for pushing live changes from the main repository to the `www` repository is established. To address this, exploring automation or a streamlined process for updates could be beneficial.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-cheat-sheets/issues/16",
@@ -15246,6 +15763,7 @@
"node_id": "I_kwDODGfxq85wCTBV",
"title": "Typo on repo description",
"body": "Hi there, \r\n\r\nI found a typo on repo description. It's written as `Respository` while it should be `Repository`.\r\n\r\n",
+ "summary": "Correct the typo in the repository description. Change `Respository` to `Repository`. \n\n**Technical Steps:**\n1. Navigate to the repository settings on GitHub.\n2. Locate the section for editing the repository description.\n3. Update the description text to fix the typo.\n4. Save the changes to update the repository description. \n\nVerify that the change is reflected in the repository overview after saving.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-webgoat/issues/8",
@@ -15268,10 +15786,11 @@
"pk": 535,
"fields": {
"nest_created_at": "2024-09-11T20:39:38.591Z",
- "nest_updated_at": "2024-09-11T20:39:38.591Z",
+ "nest_updated_at": "2024-09-13T16:06:05.179Z",
"node_id": "I_kwDODGfxq854eFXk",
"title": "There is no space on the left side.",
"body": "\r\n\r\n\r\nOn the left side there should be some space or padding , it will look good.\r\n",
+ "summary": "Add padding or margin to the left side of the layout. Ensure that the design appears balanced and visually appealing by introducing appropriate spacing. \n\n1. Identify the CSS class or element responsible for the left side layout.\n2. Modify the CSS properties by adding a `padding-left` or `margin-left` value to create the desired space.\n3. Test the changes across various screen sizes to maintain responsiveness.\n4. Review the design in different browsers to ensure consistency. \n\nImplement these steps to enhance the user interface.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-webgoat/issues/9",
@@ -15298,6 +15817,7 @@
"node_id": "MDU6SXNzdWU2ODg1OTAwNjY=",
"title": "Broken links in reviewing-code-for-cross-site-request-forgery-issues.md",
"body": "All of the links in www-project-code-review-guide/pages/reviewing-code-for-cross-site-request-forgery-issues.md are broken. I will submit a pull request to fix the ones that I can find/correct.",
+ "summary": "The issue reports that all links in the document \"reviewing-code-for-cross-site-request-forgery-issues.md\" are broken. A pull request is planned to address and correct the faulty links. To resolve this, identifying and fixing the broken links in the document is necessary.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-code-review-guide/issues/1",
@@ -15324,6 +15844,7 @@
"node_id": "I_kwDODGfxjM48fkhM",
"title": "Investigate unknown/unclear sources for information presented in previous Guide 2017",
"body": "In the previous guide (2017), which can be found here https://owasp.org/www-project-code-review-guide/assets/OWASP_Code_Review_Guide_v2.pdf, are several graphs and very interesting statements and facts that do not have proper attribution of source. \r\n\r\nFor example, in Figure 1, a survey result that is only very briefly mentioned is depict. The quality of this source, and its legitimacy needs to be investigated. Based on what the investigation finds, we either will keep, remove or update the information for the upcoming version of the guide.\r\n\r\n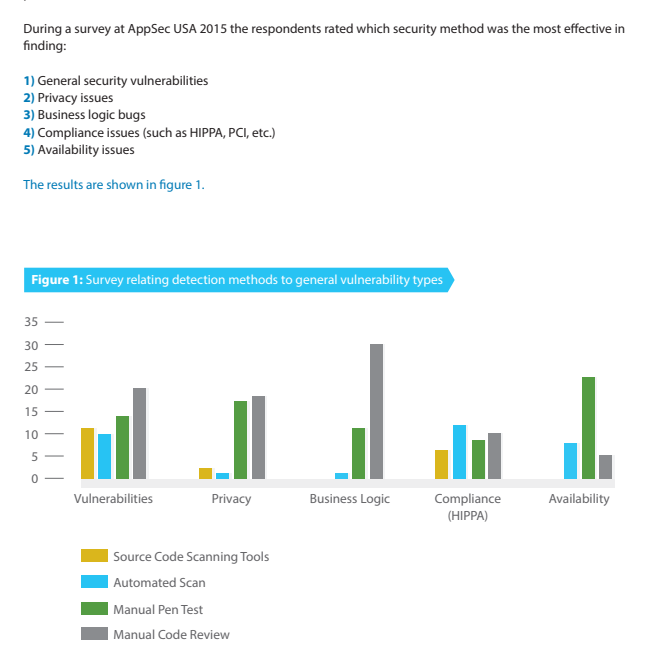\r\n\r\n\r\nInformation and statements that are hard to judge given the information in the guide can be found throughout. Each one of those has to be investigated and evaluated for future-fit in the updated/new guide.\r\n\r\nEach piece of information that needs to be investigated and evaluated should probably become its own issue. This issue can serve as a \"parent\" issue. ",
+ "summary": "The issue highlights the need to investigate and verify the sources of information presented in the 2017 Code Review Guide, particularly regarding graphs and statements that lack proper attribution. The legitimacy of these sources must be examined to determine whether the information should be retained, removed, or updated in the upcoming version of the guide. It suggests that each specific piece of information requiring investigation should be documented as a separate issue, with this issue serving as a parent for those discussions. A thorough review of the guide's content is necessary for its future update.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-code-review-guide/issues/4",
@@ -15350,6 +15871,7 @@
"node_id": "I_kwDODGfxjM49hm0C",
"title": "Add readme file for potential contributors",
"body": "Add a readme that explains \r\n\r\n- the purpose and goal of the guide\r\n- the current state it is in\r\n- how to contribute to the project.\r\n\r\nWe also have to make sure the readme is not part of the deployment process.",
+ "summary": "The issue requests the addition of a README file aimed at potential contributors. The README should outline the purpose and goals of the guide, describe the current state of the project, and provide instructions on how to contribute. Additionally, it is important to ensure that the README is excluded from the deployment process.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-code-review-guide/issues/5",
@@ -15372,10 +15894,11 @@
"pk": 539,
"fields": {
"nest_created_at": "2024-09-11T20:39:45.457Z",
- "nest_updated_at": "2024-09-11T20:39:45.457Z",
+ "nest_updated_at": "2024-09-13T04:14:44.616Z",
"node_id": "I_kwDODGfxjM49nWdQ",
"title": "Create discussable outline from code review guide 2.0",
"body": "Create a markdown document that covers the outline of the secure code review guide 2.0, and also has a discussion and decision section for each chapter/section/part. \r\n\r\nThis should guide our discussion on what to keep, adjust and remove from the 2.0 to the 3.0 version. ",
+ "summary": "The issue requests the creation of a markdown document that outlines the secure code review guide 2.0. It should include a discussion and decision section for each chapter, section, or part, facilitating a comprehensive review of what elements to retain, modify, or discard as the guide transitions from version 2.0 to version 3.0. The next step involves developing the markdown document based on these specifications.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-code-review-guide/issues/7",
@@ -15404,6 +15927,7 @@
"node_id": "MDU6SXNzdWU2MDcyMTE3NTU=",
"title": "Mobile should be a high priority",
"body": "The previous layout was much better in mobile.",
+ "summary": "The issue highlights that the current mobile layout is not satisfactory and suggests that the previous version was more effective. It indicates that improving the mobile layout should be a high priority. A review and potential redesign of the mobile interface may be necessary to enhance user experience.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-top-ten/issues/15",
@@ -15432,6 +15956,7 @@
"node_id": "MDU6SXNzdWU2MjY4ODA4NzY=",
"title": "Add back content for 2013 and 2010 Top 10 Risks",
"body": "Apologies if this has already been discussed during your meetings, but we would find it super valuable to have pages for the older 2010 and 2013 Top 10 vulnerabilities. Our particular use case is that we link to the OWASP pages in our vulnerability reports, and we still find linking to _OWASP TOP 10 2013: Cross-site Request Forgery - CSRF_ useful for our readers. There is also an interest in preserving those pages from a historical perspective, as a lot of good content existed prior to the migration to Jekyll.\r\n\r\nWe totally recognize that OWASP isn't interested in maintaining these older pages (Especially with the 2020 version on the horizon) but I think that could easily be mitigated by making it clear that they've been superseded by the latest version on the pages themselves.\r\n\r\nWe also recognize that the PDF archives still exist (Although not easily found or accessible via the website at this time), but the PDFs are fairly large and heavyweight to serve as a quick reference.\r\n\r\nIf the team is not philosophically opposed to the 2013/2010 content, we might be able to spend the time in creating a PR to get it back on the site.",
+ "summary": "The issue highlights the need for restoring the content related to the 2010 and 2013 Top 10 vulnerabilities on the site. The requester emphasizes the value of these pages for linking in vulnerability reports and preserving historical content, despite the OWASP's focus on newer versions. They acknowledge the existence of PDF archives but note their inaccessibility and size as limitations for quick reference. The suggestion is made that if the team is amenable to this idea, they could potentially create a pull request to reinstate the content. It would be beneficial to clarify that the older versions have been superseded by newer ones if implemented.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-top-ten/issues/21",
@@ -15462,6 +15987,7 @@
"node_id": "MDU6SXNzdWU3ODg3MDI4ODc=",
"title": "Statistical data for OWASP 2021",
"body": "https://lab.wallarm.com/owasp-top-10-2021-proposal-based-on-a-statistical-data/\r\n\r\nPlease consider reusing this data:\r\n#OWASP | Top-10 2021 | Vulners search query | Avg. CVSS | # of bulletins | Overall score\r\n-- | -- | -- | -- | -- | --\r\nA1 | Injections | injection OR traversal OR lfi OR “os command” OR SSTI OR RCE OR “remote code” | 4.83 | 34061 | 164514.63\r\nA2 | Broken Authentication | authentication | 4.08 | 13735 | 56038.8\r\nA3 | Cross-Site Scripting (XSS) | xss | 0.1 | 433353 | 43335.3\r\nA4 | Sensitive Data Exposure | sensitive AND data | 3.55 | 5990 | 21264.5\r\nA5 | Insecure Deserialization | XXE OR deserialize OR deserialization OR “external entities” | 5.33 | 2985 | 15910.05\r\nA6 | Broken Access Control | access control | 0.72 | 16967 | 12216.24\r\nA7 | Insufficient Logging & Monitoring | logging | 3.35 | 2309 | 7735.15\r\nA8 | Server Side Request Forgery (SSRF) | SSRF OR “server side request forgery” | 3.8 | 1139 | 4328.2\r\nA9 | Known Vulnerabilities | type:cve and (http OR web OR html) | 5.38 | 376 | 2022.88\r\nA10 | Security Misconfiguration | misconfiguration OR misconfigure OR misconfig | 2.27 | 480 | 1089.6\r\n\r\n",
+ "summary": "The issue discusses the potential reuse of statistical data related to the OWASP Top 10 vulnerabilities for 2021. A summary table is provided, which includes details such as average CVSS scores, the number of bulletins for each category, and overall scores for various vulnerabilities, including Injections, Broken Authentication, and Cross-Site Scripting. \n\nTo proceed, it is suggested to consider how this data can be integrated or leveraged in the current project or documentation to enhance understanding or improve security measures.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-top-ten/issues/31",
@@ -15488,6 +16014,7 @@
"node_id": "MDU6SXNzdWU3OTU1NTM2ODg=",
"title": "Updates",
"body": "It is important to emphasize the updates to new methods, new API's, new versions or fetures. \r\n",
+ "summary": "The issue highlights the need to emphasize updates related to new methods, APIs, versions, or features within the project. To address this, it may be necessary to create a clear documentation or communication strategy that outlines these updates effectively.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-top-ten/issues/32",
@@ -15514,6 +16041,7 @@
"node_id": "MDU6SXNzdWU5MzY0ODUzNTg=",
"title": "Minor erros and missing pages on \"A10:2017-Insufficient Logging & Monitoring\" page",
"body": "As it uses the & character, the name of the file for the page is `A10_2017-Insufficient_Logging%26Monitoring.md`, but when you are ON the page, the `view on github` link seems to be broken (404).\r\n\r\nThe correct URL (Found on the project main page) is `https://owasp.org/www-project-top-ten/2017/A10_2017-Insufficient_Logging%2526Monitoring`.\r\n\r\nThe second issue is the `https://www-01.ibm.com/common/ssi/cgi-bin/ssialias?htmlfid=SEL03130WWEN&` link on `average of 191 days` on the same page. The link leads us to a \"Deleted or moved\" page. As the information about the amount itself is not wrong, it's from 2016, therefore, I believe it's not up to date with the Ponemon institute.\r\n\r\n(Sorry if I posted this in the worng place)",
+ "summary": "The issue reports minor errors and missing pages on the \"A10:2017-Insufficient Logging & Monitoring\" page. The file name for the page is incorrectly formatted, leading to a broken \"view on GitHub\" link (404 error). The correct URL is noted. Additionally, a link to an IBM resource regarding the \"average of 191 days\" is broken, as it leads to a deleted or moved page, and the information is outdated. To resolve these issues, the file name and links need to be corrected and updated.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-top-ten/issues/38",
@@ -15540,6 +16068,7 @@
"node_id": "I_kwDODGfxcc4-Z73Z",
"title": "Comment about A4 on the T10 hompage",
"body": "Page: https://owasp.org/Top10/\r\n\r\nThis sentence under A4 does not make total sense to me, can you help me understand it?\r\n\r\n_An insecure design cannot be fixed by a perfect implementation as by definition, needed security controls were never created to defend against specific attacks._\r\n\r\nI think a little cleanup here would be prudent.\r\n\r\nRespectfully, Jim",
+ "summary": "The issue highlights a sentence under A4 on the OWASP Top 10 homepage that is unclear and potentially confusing. The commenter suggests that the phrasing could benefit from clarification to enhance understanding. A revision of the wording may be necessary to improve its clarity and effectiveness.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-top-ten/issues/46",
@@ -15566,6 +16095,7 @@
"node_id": "I_kwDODGfxcc5K3jv-",
"title": "Portuguese language change produces missing image",
"body": "From a JIRA ticket: \r\n\r\nIf you go to https://owasp.org/Top10/A00_2021_Introduction/ then change the language setting to Português (Brasil) then you can see the image is not showing. There is error 404 for the image. Please fix as soon as possible.",
+ "summary": "The issue reports that changing the language setting to Português (Brasil) on the specified OWASP page results in a missing image, leading to a 404 error. To resolve this, the missing image needs to be addressed to ensure it displays correctly in the Portuguese version.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-top-ten/issues/48",
@@ -15590,10 +16120,11 @@
"pk": 547,
"fields": {
"nest_created_at": "2024-09-11T20:39:57.653Z",
- "nest_updated_at": "2024-09-11T20:39:57.653Z",
+ "nest_updated_at": "2024-09-13T04:14:50.707Z",
"node_id": "I_kwDODGfxcc5RqCR6",
"title": "Informe sobre sistemas\nBy tkmx_nc_tkmx ",
"body": "Un pequeño informe sobre las vulnerabilidades ,ataques y puertas traceras sobre un sistema ",
+ "summary": "The issue discusses the need for a brief report on vulnerabilities, attacks, and backdoors in a system. It suggests a focus on identifying and outlining these security concerns. To address this, it may be beneficial to research and compile relevant information on various types of vulnerabilities and potential threats to system integrity.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-top-ten/issues/50",
@@ -15620,6 +16151,7 @@
"node_id": "I_kwDODGfvrc5khoNz",
"title": "fix: merge review from @robvanderveer",
"body": "the following is an initial review taken from Slack logs: https://owasp.slack.com/archives/C04PESBUWRZ/p1677192099712519\r\n\r\nby @robvanderveer\r\n\r\n\r\n---\r\nDear all,\r\nI did a first scan through the list to mainly look at taxonomy. Here are my remarks.\r\n1.\r\nML01\r\nIn 'literature' the term ‘adversarial’ is often used for input manipulation attacks, but also for data poisoning, model extraction etc. Therefore in order to avoid confusion it is probably better to rename the ML01 adversarial attack entry to input manipulation?\r\n2.\r\nIt is worth considering to add ‘model evasion’ aka black box input manipulation to your top 10? Or do you prefer to have one entry for input manipulation all together?\r\n3.\r\nML03\r\nIt is not clear to me how scenarios 1 and 2 work. I must be missing something. Usually model inversion is explained by manipulating synthesized faces until the algorithm behaves like it recognizes the face.\r\n4\r\nML04\r\nIt is not clear to me how scenario 1 works.\r\nStandard methods against overtraining are missing form the ‘how to prevent’ part. Instead the advice is to reduce the training set size - which typically increases the overfitting problem.\r\n5\r\nML05\r\nModel stealing describes a scenario where an attacker steals model parameters, but generally this attack takes place by ways of black box: gathering input-output pairs and training a new model on it.\r\n6\r\nML07\r\nI don’t understand exactly how the presented scenario should work. I do know about the scenario where a pre-trained model was obtained that has been altered by an attacker. This matches the description.\r\n7\r\nML08\r\nIsn’t model skewing the same as data poisoning? If there’s a difference, to me they are not apparent from the scenario and description.\r\n8\r\nML10 is called Neural net reprogramming but I guess the attack of changing parameters will work on any type of algorithm - not just neural networks. The description also mentions changing the training data, but perhaps that is better left out to avoid confusion with data poisoning?",
+ "summary": "The issue addresses a review of the taxonomy related to machine learning attack types. Key points for consideration include:\n\n1. Renaming the \"adversarial attack\" entry to \"input manipulation\" to reduce confusion.\n2. The potential addition of \"model evasion\" to the top 10 list.\n3. Clarification needed on specific scenarios related to model inversion and overfitting.\n4. The distinction between model stealing and black box attacks should be elaborated.\n5. Confusion over the definitions of model skewing and data poisoning.\n6. Reassessing the terminology used in ML10 to ensure it applies broadly across algorithms.\n\nTo resolve these points, a thorough review and clarification of the taxonomy entries, scenarios, and descriptions are necessary.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/2",
@@ -15652,6 +16184,7 @@
"node_id": "I_kwDODGfvrc5sUuDt",
"title": "feat(docs): create page on calculating severity",
"body": "Each of the Top 10 items are scored according to [OWASP's Risk Rating Methodology](https://owasp.org/www-community/OWASP_Risk_Rating_Methodology). There should be a page defining how to use the ratings to provide a severity score. This will assist practitioners in knowing 'what to fix' and 'when'.",
+ "summary": "The issue proposes creating a documentation page that explains how to calculate severity scores based on OWASP's Risk Rating Methodology for the Top 10 items. This resource aims to guide practitioners on prioritizing what needs to be fixed and when. To address this, a detailed page that outlines the rating process and its application should be developed.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/15",
@@ -15669,9 +16202,9 @@
238
],
"labels": [
+ 136,
134,
- 135,
- 136
+ 135
]
}
},
@@ -15684,6 +16217,7 @@
"node_id": "I_kwDODGfvrc5sc_TG",
"title": "fix: merge existing body of work from EthicalML https://ethical.institute",
"body": "### Type\n\nDocumentation Issue Report\n\n### What would you like to report?\n\nThere is a comprehensive existing body of work at: https://ethical.institute\r\n\r\nThe intent would be to review the [current Top 10 list in this project](https://owasp.org/www-project-machine-learning-security-top-10/) and:\r\n- merge content where appropriate\r\n- create new content missing \r\n- suggest improvements for areas/scope not curently covered\n\n### Code of Conduct\n\n- [X] I agree to follow this project's Code of Conduct",
+ "summary": "The issue reports a need to merge existing documentation from EthicalML at https://ethical.institute with the current Top 10 list in the project linked. The tasks suggested include merging relevant content, creating new content where it is lacking, and proposing improvements for areas that are currently not covered.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/30",
@@ -15698,12 +16232,12 @@
"author": 238,
"repository": 981,
"assignees": [
- 238,
- 240
+ 240,
+ 238
],
"labels": [
- 132,
- 137
+ 137,
+ 132
]
}
},
@@ -15716,6 +16250,7 @@
"node_id": "I_kwDODGfvrc5ucL4j",
"title": "[Fortnightly] Working Group Meeting - 2023-Aug-17",
"body": "* Date: [Thursday, August 17 at 0500 UTC](https://dateful.com/convert/utc?t=5am&d=2023-08-02)\r\n* Previous agenda: #42 \r\n\r\n## Current agenda\r\n\r\n1. General project status - [v0.2 milestone complete](https://github.com/OWASP/www-project-machine-learning-security-top-10/releases/tag/v0.2)\r\n2. Contributions and [current help wanted](https://github.com/OWASP/www-project-machine-learning-security-top-10/issues?q=is%3Aissue+is%3Aopen+label%3A%22help+wanted%22)\r\n3. Introductions (for new contributors)\r\n\r\n## Discussions\r\n\r\n* [Join the OWASP Slack group](https://owasp.org/slack/invite) and the [#project-mlsec-top-10 channel](https://owasp.slack.com/archives/C04PESBUWRZ)\r\n* [Github Discussions](https://github.com/OWASP/www-project-machine-learning-security-top-10/discussions)\r\n\r\n## Calendar Event\r\n[Download calendar event (ICS)](https://calendar.google.com/calendar/ical/c_f818ec1e3dea1d4c80cb0f872566eccb82c5df9cc1161f3077f93eafc47889dc%40group.calendar.google.com/public/basic.ics)",
+ "summary": "A working group meeting is scheduled for August 17 at 0500 UTC. The agenda includes updates on the project's general status, confirming the completion of the v0.2 milestone, discussing current contributions and help needed, and introducing new contributors. Participants are encouraged to join the OWASP Slack group and the relevant channel, as well as engage in GitHub Discussions. To stay organized, a calendar event is available for download. Further action includes reviewing the contributions and help wanted sections to identify areas for involvement.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/63",
@@ -15746,6 +16281,7 @@
"node_id": "I_kwDODGfvrc5vN6es",
"title": "feat(rendering): make PDF output from Markdown files more presentable",
"body": "The Top 10 list is being rendered using Markdown at https://mltop10.info\r\n\r\nThe site is being rendered using [Quarto](https://quarto.org) and the files from https://github.com/OWASP/www-project-machine-learning-security-top-10/tree/master/docs are mirrored to https://github.com/mltop10-info/mltop10.info\r\n\r\nCurrently a manual process is run for the https://github.com/mltop10-info/mltop10.info locally to render the HTML and PDF outputs which are stored in https://github.com/mltop10-info/mltop10.info/tree/main/docs and used by Github Pages.\r\n\r\nThe rendering for PDF is currently using the default method of LaTeX - example at: https://github.com/mltop10-info/mltop10.info/blob/main/docs/OWASP-Machine-Learning-Security-Top-10.pdf\r\n\r\nQuarto has a lot of formatting options for generating PDF and this needs to be explored to make the PDF and ePUB formats look more presentable.",
+ "summary": "The issue highlights the need to enhance the presentation of PDF outputs generated from Markdown files for a website that lists the Top 10 machine learning security concerns. The current PDF rendering relies on LaTeX's default settings, which are not optimal. It is suggested to explore Quarto's various formatting options to improve the appearance of the PDF and ePUB formats. This involves reviewing and possibly updating the rendering process to utilize these formatting capabilities more effectively.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/99",
@@ -15763,9 +16299,9 @@
238
],
"labels": [
+ 136,
134,
- 135,
- 136
+ 135
]
}
},
@@ -15778,6 +16314,7 @@
"node_id": "I_kwDODGfvrc5vvtvV",
"title": "[Fortnightly] Working Group Meeting - 2023-Aug-31",
"body": "## Current agenda\r\n\r\n1. General project status [v0.3 - in progress](https://github.com/OWASP/www-project-machine-learning-security-top-10/milestone/3)\r\n2. Contributions and [current help wanted](https://github.com/OWASP/www-project-machine-learning-security-top-10/issues?q=is%3Aissue+is%3Aopen+label%3A%22help+wanted%22)\r\n3. Introductions (for new contributors)\r\n\r\n## Discussions\r\n\r\n* [Join the OWASP Slack group](https://owasp.org/slack/invite) and the [#project-mlsec-top-10 channel](https://owasp.slack.com/archives/C04PESBUWRZ)\r\n* [Github Discussions](https://github.com/OWASP/www-project-machine-learning-security-top-10/discussions)\r\n\r\n## Calendar Event\r\n[Download calendar event (ICS)](https://calendar.google.com/calendar/ical/c_f818ec1e3dea1d4c80cb0f872566eccb82c5df9cc1161f3077f93eafc47889dc%40group.calendar.google.com/public/basic.ics)",
+ "summary": "The GitHub issue outlines the agenda for the upcoming working group meeting scheduled for August 31, 2023. Key points include the current status of the project (version 0.3), ongoing contributions, and a welcome for new contributors. Additionally, it encourages joining the OWASP Slack group and engaging in GitHub Discussions. To prepare for the meeting, contributors should check for open issues labeled \"help wanted\" and consider participating in the discussions to enhance collaboration.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/102",
@@ -15808,6 +16345,7 @@
"node_id": "I_kwDODGfvrc5wjpZK",
"title": "Model stealing through interaction is not mentioned",
"body": "The current model stealing only describes the model being stolen through parameters, but the model can also be stolen by presenting inputs, capturing the output and using those combinations to train your own model. See AI guide",
+ "summary": "The issue highlights a gap in the documentation regarding model stealing techniques. It points out that the existing description focuses solely on parameter theft, neglecting the method of stealing a model by interacting with it—specifically by submitting inputs and capturing outputs to train a new model. To address this, the documentation should be updated to include information on this interaction-based model stealing approach.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/113",
@@ -15838,6 +16376,7 @@
"node_id": "I_kwDODGfvrc5w8-kb",
"title": "[Fortnightly] Working Group Meeting - 2023-Sep-14",
"body": "## Current agenda\r\n\r\n1. General project status [v0.3 - in progress](https://github.com/OWASP/www-project-machine-learning-security-top-10/milestone/3)\r\n2. Notable PRs completed since last meeting:\r\n - #104 \r\n - #110 \r\n3. Notable discussions:\r\n - #107\r\n - #108\r\n - #109\r\n 4. Meetings:\r\n - WG meeting will change forward a few hours to accomodate EU morning time zones\r\n5. Contributions and [current help wanted](https://github.com/OWASP/www-project-machine-learning-security-top-10/issues?q=is%3Aissue+is%3Aopen+label%3A%22help+wanted%22)\r\n6. Introductions (for new contributors)\r\n\r\n## Discussions\r\n\r\n* [Join the OWASP Slack group](https://owasp.org/slack/invite) and the [#project-mlsec-top-10 channel](https://owasp.slack.com/archives/C04PESBUWRZ)\r\n* [Github Discussions](https://github.com/OWASP/www-project-machine-learning-security-top-10/discussions)\r\n\r\n## Calendar Event\r\n[Download calendar event (ICS)](https://calendar.google.com/calendar/ical/c_f818ec1e3dea1d4c80cb0f872566eccb82c5df9cc1161f3077f93eafc47889dc%40group.calendar.google.com/public/basic.ics)",
+ "summary": "The issue discusses the agenda for a working group meeting, highlighting the current project status (v0.3 in progress), notable pull requests completed since the last meeting, and key discussions. It mentions the scheduling of future meetings to accommodate EU time zones and encourages contributions, with a link to current help wanted issues. New contributors are welcomed, and it promotes joining the OWASP Slack group and participating in GitHub Discussions. \n\nTo move forward, participants should review the project status and notable discussions, and consider contributing to the identified open issues.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/114",
@@ -15869,6 +16408,7 @@
"node_id": "I_kwDODGfvrc51QqDw",
"title": "feat(docs): create guide for how to use Top 10 list as a ML Engineer",
"body": "Reference https://github.com/OWASP/www-project-machine-learning-security-top-10/blob/master/GUIDELINES.md#ml-engineeranalyst \r\n\r\n- [ ] Create a detailed guidelines document for how to use the information in the Top 10 list for use day to day",
+ "summary": "The issue suggests creating a comprehensive guidelines document for Machine Learning Engineers on how to effectively utilize the Top 10 list in their daily work. This document should draw references from existing materials and provide clear instructions or best practices. The task involves outlining practical applications of the Top 10 list in the context of machine learning security.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/130",
@@ -15884,9 +16424,9 @@
"repository": 981,
"assignees": [],
"labels": [
- 135,
136,
- 139
+ 139,
+ 135
]
}
},
@@ -15899,6 +16439,7 @@
"node_id": "I_kwDODGfvrc51QrtJ",
"title": "feat(docs): create guide for how to use Top 10 list as a AppSec Engineer",
"body": "Reference https://github.com/OWASP/www-project-machine-learning-security-top-10/blob/master/GUIDELINES.md#pentestersecurity-engineer\r\n\r\n- [ ] Create a detailed guidelines document for how to use the information in the Top 10 list for use day to day",
+ "summary": "The issue requests the creation of a detailed guidelines document aimed at assisting AppSec Engineers in utilizing the Top 10 list effectively in their daily work. It references existing documentation for context. The task involves outlining practical applications and recommendations based on the Top 10 list.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/131",
@@ -15914,9 +16455,9 @@
"repository": 981,
"assignees": [],
"labels": [
- 135,
136,
- 139
+ 139,
+ 135
]
}
},
@@ -15929,6 +16470,7 @@
"node_id": "I_kwDODGfvrc51QsL5",
"title": "feat(docs): create guide for how to use Top 10 list as a CISO",
"body": "Reference https://github.com/OWASP/www-project-machine-learning-security-top-10/blob/master/GUIDELINES.md#ciso\r\n\r\n- [ ] Create a detailed guidelines document for how to use the information in the Top 10 list for use day to day",
+ "summary": "The issue proposes the creation of a detailed guidelines document aimed at helping users understand how to effectively utilize the Top 10 list in their daily activities as a Chief Information Security Officer (CISO). It references existing guidelines and emphasizes the need for a comprehensive resource. To address this, the task involves developing a structured document that outlines practical applications of the Top 10 list in the CISO's workflow.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/132",
@@ -15944,9 +16486,9 @@
"repository": 981,
"assignees": [],
"labels": [
- 135,
136,
- 139
+ 139,
+ 135
]
}
},
@@ -15959,6 +16501,7 @@
"node_id": "I_kwDODGfvrc51QsbP",
"title": "feat(docs): create guide for how to use Top 10 list as a Developer",
"body": "Reference https://github.com/OWASP/www-project-machine-learning-security-top-10/blob/master/GUIDELINES.md#developers\r\n\r\n- [ ] Create a detailed guidelines document for how to use the information in the Top 10 list for use day to day",
+ "summary": "The issue proposes the creation of a detailed guidelines document to help developers effectively utilize the Top 10 list. It references existing guidelines and emphasizes the need for practical, daily use instructions. The task involves drafting comprehensive documentation that outlines this usage.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/133",
@@ -15974,9 +16517,9 @@
"repository": 981,
"assignees": [],
"labels": [
- 135,
136,
- 139
+ 139,
+ 135
]
}
},
@@ -15989,6 +16532,7 @@
"node_id": "I_kwDODGfvrc51Qswv",
"title": "feat(docs): create guide for how to use Top 10 list as an MLOps Engineer",
"body": "Reference https://github.com/OWASP/www-project-machine-learning-security-top-10/blob/master/GUIDELINES.md#mlops\r\n\r\n- [ ] Create a detailed guidelines document for how to use the information in the Top 10 list for use day to day",
+ "summary": "The issue requests the creation of a comprehensive guidelines document aimed at MLOps Engineers, detailing how to effectively utilize the Top 10 list in their daily operations. It references existing documentation for context. To address this, a structured and informative guide needs to be developed.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/134",
@@ -16004,9 +16548,9 @@
"repository": 981,
"assignees": [],
"labels": [
- 135,
136,
- 139
+ 139,
+ 135
]
}
},
@@ -16019,6 +16563,7 @@
"node_id": "I_kwDODGfvrc51QtFx",
"title": "feat(docs): create guide for how to use Top 10 list as a Data Engineer",
"body": "Reference https://github.com/OWASP/www-project-machine-learning-security-top-10/blob/master/GUIDELINES.md#data-engineer\r\n\r\n- [ ] Create a detailed guidelines document for how to use the information in the Top 10 list for use day to day",
+ "summary": "The issue requests the creation of a comprehensive guidelines document aimed at Data Engineers, detailing how to effectively utilize the information provided in the Top 10 list. The guidelines should enhance daily operations and integrate the insights into routine practices. To address this, a structured document outlining practical applications and best practices is needed.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/135",
@@ -16034,9 +16579,9 @@
"repository": 981,
"assignees": [],
"labels": [
- 135,
136,
- 139
+ 139,
+ 135
]
}
},
@@ -16049,6 +16594,7 @@
"node_id": "I_kwDODGfvrc51QyzM",
"title": "feat(docs): create a recorded demo of ML01 Input Manipulation Attack",
"body": "- [ ] Create a recorded video demo (no audio)\r\n\r\nVideo will be uploaded to [OWASP Youtube Channel](https://www.youtube.com/@owasp-mltop10)\r\n",
+ "summary": "A request has been made to create a recorded video demonstration of the ML01 Input Manipulation Attack, which should be without audio. The completed video will be uploaded to the OWASP YouTube Channel. To proceed, the video needs to be recorded and prepared for upload.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/136",
@@ -16066,9 +16612,9 @@
239
],
"labels": [
- 135,
136,
- 140
+ 140,
+ 135
]
}
},
@@ -16081,6 +16627,7 @@
"node_id": "I_kwDODGfvrc51Qz2Z",
"title": "feat(docs): create a recorded demo of ML02 Data Poisoning Attack",
"body": "- [ ] Create a recorded video demo (no audio)\n\nVideo will be uploaded to [OWASP Youtube Channel](https://www.youtube.com/@owasp-mltop10)",
+ "summary": "A request has been made to create a recorded video demo of the ML02 Data Poisoning Attack, which should not include audio. The finished video will be uploaded to the OWASP YouTube Channel. To move forward, the demo needs to be recorded and prepared for upload.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/137",
@@ -16096,9 +16643,9 @@
"repository": 981,
"assignees": [],
"labels": [
- 135,
136,
- 140
+ 140,
+ 135
]
}
},
@@ -16111,6 +16658,7 @@
"node_id": "I_kwDODGfvrc51Q0B7",
"title": "feat(docs): create a recorded demo of ML03 Model Inversion Attack",
"body": "- [ ] Create a recorded video demo (no audio)\n\nVideo will be uploaded to [OWASP Youtube Channel](https://www.youtube.com/@owasp-mltop10)",
+ "summary": "The issue requests the creation of a recorded video demo showcasing the ML03 Model Inversion Attack, with the stipulation that the video should not include audio. The completed video will be uploaded to the OWASP YouTube Channel. To address this issue, the demo needs to be recorded and edited accordingly.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/138",
@@ -16126,9 +16674,9 @@
"repository": 981,
"assignees": [],
"labels": [
- 135,
136,
- 140
+ 140,
+ 135
]
}
},
@@ -16141,6 +16689,7 @@
"node_id": "I_kwDODGfvrc51Q0I_",
"title": "feat(docs): create a recorded demo of ML04 Membership Inference Attack",
"body": "- [ ] Create a recorded video demo (no audio)\n\nVideo will be uploaded to [OWASP Youtube Channel](https://www.youtube.com/@owasp-mltop10)",
+ "summary": "The issue requests the creation of a recorded video demo showcasing the ML04 Membership Inference Attack, specifically noting that the video should not include audio. The completed video will be uploaded to the OWASP YouTube Channel. To address this, a video demonstration needs to be produced and recorded.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/139",
@@ -16156,9 +16705,9 @@
"repository": 981,
"assignees": [],
"labels": [
- 135,
136,
- 140
+ 140,
+ 135
]
}
},
@@ -16171,6 +16720,7 @@
"node_id": "I_kwDODGfvrc51Q0N7",
"title": "feat(docs): create a recorded demo of ML05 Model Theft",
"body": "- [ ] Create a recorded video demo (no audio)\n\nVideo will be uploaded to [OWASP Youtube Channel](https://www.youtube.com/@owasp-mltop10)",
+ "summary": "The issue requests the creation of a recorded video demo showcasing the ML05 Model Theft feature. The video should be without audio and will be uploaded to the OWASP YouTube Channel. To address this issue, a video demonstrating the feature needs to be produced.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/140",
@@ -16186,9 +16736,9 @@
"repository": 981,
"assignees": [],
"labels": [
- 135,
136,
- 140
+ 140,
+ 135
]
}
},
@@ -16201,6 +16751,7 @@
"node_id": "I_kwDODGfvrc51Q0Sr",
"title": "feat(docs): create a recorded demo of ML06 AI Supply Chain Attacks",
"body": "- [ ] Create a recorded video demo (no audio)\n\nVideo will be uploaded to [OWASP Youtube Channel](https://www.youtube.com/@owasp-mltop10)",
+ "summary": "The issue requests the creation of a recorded video demo showcasing ML06 AI Supply Chain Attacks, with the stipulation that no audio is required. The completed video will be uploaded to the OWASP YouTube Channel. To address this, a video recording should be planned and executed accordingly.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/141",
@@ -16218,9 +16769,9 @@
241
],
"labels": [
- 135,
136,
- 140
+ 140,
+ 135
]
}
},
@@ -16233,6 +16784,7 @@
"node_id": "I_kwDODGfvrc51Q0X6",
"title": "feat(docs): create a recorded demo of ML07 Transfer Learning Attack",
"body": "- [ ] Create a recorded video demo (no audio)\n\nVideo will be uploaded to [OWASP Youtube Channel](https://www.youtube.com/@owasp-mltop10)",
+ "summary": "The issue requests the creation of a recorded video demo showcasing the ML07 Transfer Learning Attack. The video should not include audio and is intended to be uploaded to the OWASP YouTube Channel. To address this, a video demonstrating the attack needs to be produced and prepared for upload.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/142",
@@ -16250,9 +16802,9 @@
242
],
"labels": [
- 135,
136,
- 140
+ 140,
+ 135
]
}
},
@@ -16265,6 +16817,7 @@
"node_id": "I_kwDODGfvrc51Q0gj",
"title": "feat(docs): create a recorded demo of ML08 Model Skewing",
"body": "- [ ] Create a recorded video demo (no audio)\n\nVideo will be uploaded to [OWASP Youtube Channel](https://www.youtube.com/@owasp-mltop10)",
+ "summary": "The issue suggests creating a recorded video demo showcasing the ML08 Model Skewing topic, with the requirement that the video should not include audio. Once completed, the video is intended to be uploaded to the OWASP YouTube Channel. Action needs to be taken to produce and finalize the demo.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/143",
@@ -16282,9 +16835,9 @@
239
],
"labels": [
- 135,
136,
- 140
+ 140,
+ 135
]
}
},
@@ -16297,6 +16850,7 @@
"node_id": "I_kwDODGfvrc51Q0l5",
"title": "feat(docs): create a recorded demo of ML09 Output Integrity Attack",
"body": "- [ ] Create a recorded video demo (no audio)\n\nVideo will be uploaded to [OWASP Youtube Channel](https://www.youtube.com/@owasp-mltop10)",
+ "summary": "The issue requests the creation of a recorded video demo showcasing the ML09 Output Integrity Attack, specifically noting that the video should have no audio. Once completed, the video is intended to be uploaded to the OWASP YouTube Channel. To proceed, a video demonstration needs to be recorded and prepared for upload.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/144",
@@ -16312,9 +16866,9 @@
"repository": 981,
"assignees": [],
"labels": [
- 135,
136,
- 140
+ 140,
+ 135
]
}
},
@@ -16327,6 +16881,7 @@
"node_id": "I_kwDODGfvrc51Q0p-",
"title": "feat(docs): create a recorded demo of ML10 Model Poisoning",
"body": "- [ ] Create a recorded video demo (no audio)\n\nVideo will be uploaded to [OWASP Youtube Channel](https://www.youtube.com/@owasp-mltop10)",
+ "summary": "The issue requests the creation of a recorded video demo showcasing the ML10 Model Poisoning topic, specifying that the video should not include audio. Once completed, the video will be uploaded to the OWASP YouTube Channel. To address this issue, a video recording demonstrating the concept should be produced.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/145",
@@ -16342,9 +16897,9 @@
"repository": 981,
"assignees": [],
"labels": [
- 135,
136,
- 140
+ 140,
+ 135
]
}
},
@@ -16357,6 +16912,7 @@
"node_id": "I_kwDODGfvrc51Q5WF",
"title": "feat(docs): create a cheatsheet for ML01 Input Manipulation Attacks ",
"body": "- [ ] Is there existing cheatsheets at [OWASP Cheatsheets](https://cheatsheetseries.owasp.org/Glossary.html)\r\n- [ ] If there is an existing cheatsheet, does it need updating at the source to cater for machine learning use cases?\r\n- [ ] Is there a need for a new cheatsheet topic?\r\n- [ ] Add existing or new cheatsheet as a reference to the Top 10 risk document\r\n\r\nExample Cheatsheet: [Input Validation Cheatsheet](https://github.com/OWASP/CheatSheetSeries/blob/master/cheatsheets/Input_Validation_Cheat_Sheet.md)\r\n\r\nExample of Top 10 risk referencing cheatsheets: [ML01 Input Manipulation Attacks - Cheatsheets](https://github.com/OWASP/www-project-machine-learning-security-top-10/blob/master/docs/cheatsheets/ML01_2023-Input_Manipulation_Attack-Cheatsheet.md)\r\n\r\n",
+ "summary": "The issue discusses the need for a cheatsheet focused on ML01 Input Manipulation Attacks. It outlines several tasks: checking for existing cheatsheets on the OWASP site, determining if they require updates for machine learning applications, assessing the necessity for a new cheatsheet topic, and potentially adding the new or updated cheatsheet as a reference in the Top 10 risk document. To move forward, it would be essential to review the current resources and identify any gaps related to input manipulation in the context of machine learning.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/147",
@@ -16371,14 +16927,14 @@
"author": 238,
"repository": 981,
"assignees": [
+ 243,
238,
- 239,
- 243
+ 239
],
"labels": [
- 135,
136,
- 141
+ 141,
+ 135
]
}
},
@@ -16391,6 +16947,7 @@
"node_id": "I_kwDODGfvrc51RcoQ",
"title": "feat(docs): create a cheatsheet for ML02 Data Poisoning Attack ",
"body": "- [ ] Is there existing cheatsheets at [OWASP Cheatsheets](https://cheatsheetseries.owasp.org/Glossary.html)\n- [ ] If there is an existing cheatsheet, does it need updating at the source to cater for machine learning use cases?\n- [ ] Is there a need for a new cheatsheet topic?\n- [ ] Add existing or new cheatsheet as a reference to the Top 10 risk document\n\nExample Cheatsheet: [Input Validation Cheatsheet](https://github.com/OWASP/CheatSheetSeries/blob/master/cheatsheets/Input_Validation_Cheat_Sheet.md)\n\nExample of Top 10 risk referencing cheatsheets: [ML01 Input Manipulation Attacks - Cheatsheets](https://github.com/OWASP/www-project-machine-learning-security-top-10/blob/master/docs/cheatsheets/ML01_2023-Input_Manipulation_Attack-Cheatsheet.md)",
+ "summary": "The issue discusses the creation of a cheatsheet specifically for the ML02 Data Poisoning Attack. It outlines several tasks: checking for existing cheatsheets on the OWASP platform, assessing whether any need updates to include machine learning use cases, determining if a new cheatsheet topic is necessary, and referencing the existing or new cheatsheet in the Top 10 risk document. To proceed, review the current OWASP cheatsheets and identify any gaps related to data poisoning attacks.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/150",
@@ -16409,9 +16966,9 @@
239
],
"labels": [
- 135,
136,
- 141
+ 141,
+ 135
]
}
},
@@ -16424,6 +16981,7 @@
"node_id": "I_kwDODGfvrc51Rc0V",
"title": "feat(docs): create a cheatsheet for ML03 Model Inversion Attack",
"body": "- [ ] Is there existing cheatsheets at [OWASP Cheatsheets](https://cheatsheetseries.owasp.org/Glossary.html)\n- [ ] If there is an existing cheatsheet, does it need updating at the source to cater for machine learning use cases?\n- [ ] Is there a need for a new cheatsheet topic?\n- [ ] Add existing or new cheatsheet as a reference to the Top 10 risk document\n\nExample Cheatsheet: [Input Validation Cheatsheet](https://github.com/OWASP/CheatSheetSeries/blob/master/cheatsheets/Input_Validation_Cheat_Sheet.md)\n\nExample of Top 10 risk referencing cheatsheets: [ML01 Input Manipulation Attacks - Cheatsheets](https://github.com/OWASP/www-project-machine-learning-security-top-10/blob/master/docs/cheatsheets/ML01_2023-Input_Manipulation_Attack-Cheatsheet.md)",
+ "summary": "The issue discusses the need to create a cheatsheet for the ML03 Model Inversion Attack. Several tasks have been outlined, including checking for existing cheatsheets on the OWASP website, determining if any existing cheatsheets require updates for machine learning contexts, assessing the necessity for a new cheatsheet topic, and adding relevant cheatsheets to the Top 10 risk document. \n\nTo proceed, one should first review existing resources and identify gaps that the new cheatsheet could fill.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/151",
@@ -16439,9 +16997,9 @@
"repository": 981,
"assignees": [],
"labels": [
- 135,
136,
- 141
+ 141,
+ 135
]
}
},
@@ -16454,6 +17012,7 @@
"node_id": "I_kwDODGfvrc51Rc9t",
"title": "feat(docs): create a cheatsheet for ML04 Membership Inference Attack",
"body": "- [ ] Is there existing cheatsheets at [OWASP Cheatsheets](https://cheatsheetseries.owasp.org/Glossary.html)\n- [ ] If there is an existing cheatsheet, does it need updating at the source to cater for machine learning use cases?\n- [ ] Is there a need for a new cheatsheet topic?\n- [ ] Add existing or new cheatsheet as a reference to the Top 10 risk document\n\nExample Cheatsheet: [Input Validation Cheatsheet](https://github.com/OWASP/CheatSheetSeries/blob/master/cheatsheets/Input_Validation_Cheat_Sheet.md)\n\nExample of Top 10 risk referencing cheatsheets: [ML01 Input Manipulation Attacks - Cheatsheets](https://github.com/OWASP/www-project-machine-learning-security-top-10/blob/master/docs/cheatsheets/ML01_2023-Input_Manipulation_Attack-Cheatsheet.md)",
+ "summary": "The issue discusses the creation of a cheatsheet specifically for the ML04 Membership Inference Attack. Key tasks include checking for existing cheatsheets on the OWASP site, determining if any need updates to address machine learning scenarios, assessing the necessity for a new cheatsheet topic, and referencing the relevant cheatsheet in the Top 10 risk document. To move forward, one should begin by reviewing existing resources and identifying gaps related to membership inference attacks.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/152",
@@ -16469,9 +17028,9 @@
"repository": 981,
"assignees": [],
"labels": [
- 135,
136,
- 141
+ 141,
+ 135
]
}
},
@@ -16484,6 +17043,7 @@
"node_id": "I_kwDODGfvrc51RdB5",
"title": "feat(docs): create a cheatsheet for ML05 Model Theft",
"body": "- [ ] Is there existing cheatsheets at [OWASP Cheatsheets](https://cheatsheetseries.owasp.org/Glossary.html)\n- [ ] If there is an existing cheatsheet, does it need updating at the source to cater for machine learning use cases?\n- [ ] Is there a need for a new cheatsheet topic?\n- [ ] Add existing or new cheatsheet as a reference to the Top 10 risk document\n\nExample Cheatsheet: [Input Validation Cheatsheet](https://github.com/OWASP/CheatSheetSeries/blob/master/cheatsheets/Input_Validation_Cheat_Sheet.md)\n\nExample of Top 10 risk referencing cheatsheets: [ML01 Input Manipulation Attacks - Cheatsheets](https://github.com/OWASP/www-project-machine-learning-security-top-10/blob/master/docs/cheatsheets/ML01_2023-Input_Manipulation_Attack-Cheatsheet.md)",
+ "summary": "The issue suggests creating a cheatsheet specifically for ML05 Model Theft. It involves checking if there are existing cheatsheets on the OWASP Cheatsheets site and determining whether they require updates for machine learning contexts. Additionally, it explores the necessity of a new cheatsheet topic and proposes adding either the existing or new cheatsheet as a reference to the Top 10 risk document. To proceed, it is essential to evaluate current resources and identify any gaps related to model theft in machine learning.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/153",
@@ -16499,9 +17059,9 @@
"repository": 981,
"assignees": [],
"labels": [
- 135,
136,
- 141
+ 141,
+ 135
]
}
},
@@ -16514,6 +17074,7 @@
"node_id": "I_kwDODGfvrc51RdHk",
"title": "feat(docs): create a cheatsheet for ML06 AI Supply Chain Attacks",
"body": "- [ ] Is there existing cheatsheets at [OWASP Cheatsheets](https://cheatsheetseries.owasp.org/Glossary.html)\n- [ ] If there is an existing cheatsheet, does it need updating at the source to cater for machine learning use cases?\n- [ ] Is there a need for a new cheatsheet topic?\n- [ ] Add existing or new cheatsheet as a reference to the Top 10 risk document\n\nExample Cheatsheet: [Input Validation Cheatsheet](https://github.com/OWASP/CheatSheetSeries/blob/master/cheatsheets/Input_Validation_Cheat_Sheet.md)\n\nExample of Top 10 risk referencing cheatsheets: [ML01 Input Manipulation Attacks - Cheatsheets](https://github.com/OWASP/www-project-machine-learning-security-top-10/blob/master/docs/cheatsheets/ML01_2023-Input_Manipulation_Attack-Cheatsheet.md)",
+ "summary": "The issue proposes the creation of a cheatsheet specifically for ML06 AI Supply Chain Attacks. It includes several tasks: checking for existing cheatsheets on the OWASP Cheatsheets site, determining if any need updates for machine learning contexts, assessing the necessity for a new cheatsheet topic, and linking the relevant cheatsheet to the Top 10 risk document. To proceed, one should start by reviewing the current resources and identifying gaps that need to be addressed.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/154",
@@ -16528,14 +17089,14 @@
"author": 238,
"repository": 981,
"assignees": [
+ 241,
238,
- 239,
- 241
+ 239
],
"labels": [
- 135,
136,
- 141
+ 141,
+ 135
]
}
},
@@ -16548,6 +17109,7 @@
"node_id": "I_kwDODGfvrc51RdMG",
"title": "feat(docs): create a cheatsheet for ML07 Transfer Learning Attack",
"body": "- [ ] Is there existing cheatsheets at [OWASP Cheatsheets](https://cheatsheetseries.owasp.org/Glossary.html)\n- [ ] If there is an existing cheatsheet, does it need updating at the source to cater for machine learning use cases?\n- [ ] Is there a need for a new cheatsheet topic?\n- [ ] Add existing or new cheatsheet as a reference to the Top 10 risk document\n\nExample Cheatsheet: [Input Validation Cheatsheet](https://github.com/OWASP/CheatSheetSeries/blob/master/cheatsheets/Input_Validation_Cheat_Sheet.md)\n\nExample of Top 10 risk referencing cheatsheets: [ML01 Input Manipulation Attacks - Cheatsheets](https://github.com/OWASP/www-project-machine-learning-security-top-10/blob/master/docs/cheatsheets/ML01_2023-Input_Manipulation_Attack-Cheatsheet.md)",
+ "summary": "The issue discusses the need to create a cheatsheet for the ML07 Transfer Learning Attack. It raises several points for consideration: checking for existing cheatsheets on the OWASP site, determining if any existing cheatsheets require updates for machine learning applications, assessing the necessity for a new cheatsheet topic, and including the new or updated cheatsheet as a reference in the Top 10 risk document. \n\nTo move forward, it would be helpful to review the current cheatsheets and identify any gaps related to transfer learning attacks.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/155",
@@ -16562,14 +17124,14 @@
"author": 238,
"repository": 981,
"assignees": [
- 238,
- 239,
242,
- 244
+ 244,
+ 238,
+ 239
],
"labels": [
- 135,
- 141
+ 141,
+ 135
]
}
},
@@ -16582,6 +17144,7 @@
"node_id": "I_kwDODGfvrc51RdSW",
"title": "feat(docs): create a cheatsheet for ML08 Model Skewing",
"body": "- [ ] Is there existing cheatsheets at [OWASP Cheatsheets](https://cheatsheetseries.owasp.org/Glossary.html)\n- [ ] If there is an existing cheatsheet, does it need updating at the source to cater for machine learning use cases?\n- [ ] Is there a need for a new cheatsheet topic?\n- [ ] Add existing or new cheatsheet as a reference to the Top 10 risk document\n\nExample Cheatsheet: [Input Validation Cheatsheet](https://github.com/OWASP/CheatSheetSeries/blob/master/cheatsheets/Input_Validation_Cheat_Sheet.md)\n\nExample of Top 10 risk referencing cheatsheets: [ML01 Input Manipulation Attacks - Cheatsheets](https://github.com/OWASP/www-project-machine-learning-security-top-10/blob/master/docs/cheatsheets/ML01_2023-Input_Manipulation_Attack-Cheatsheet.md)",
+ "summary": "The issue proposes the creation of a cheatsheet specifically for ML08 Model Skewing. It outlines several tasks, including checking for existing cheatsheets on the OWASP Cheatsheets site, determining if any need updates to address machine learning scenarios, and assessing the necessity of a new cheatsheet topic. Additionally, it suggests adding either the existing or new cheatsheet to the Top 10 risk document. To proceed, a review of current resources and potential updates or additions is needed.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/156",
@@ -16597,9 +17160,9 @@
"repository": 981,
"assignees": [],
"labels": [
- 135,
136,
- 141
+ 141,
+ 135
]
}
},
@@ -16612,6 +17175,7 @@
"node_id": "I_kwDODGfvrc51RdYf",
"title": "feat(docs): create a cheatsheet for ML09 Output Integrity Attack",
"body": "- [ ] Is there existing cheatsheets at [OWASP Cheatsheets](https://cheatsheetseries.owasp.org/Glossary.html)\n- [ ] If there is an existing cheatsheet, does it need updating at the source to cater for machine learning use cases?\n- [ ] Is there a need for a new cheatsheet topic?\n- [ ] Add existing or new cheatsheet as a reference to the Top 10 risk document\n\nExample Cheatsheet: [Input Validation Cheatsheet](https://github.com/OWASP/CheatSheetSeries/blob/master/cheatsheets/Input_Validation_Cheat_Sheet.md)\n\nExample of Top 10 risk referencing cheatsheets: [ML01 Input Manipulation Attacks - Cheatsheets](https://github.com/OWASP/www-project-machine-learning-security-top-10/blob/master/docs/cheatsheets/ML01_2023-Input_Manipulation_Attack-Cheatsheet.md)",
+ "summary": "The issue discusses the creation of a cheatsheet for the ML09 Output Integrity Attack. It outlines several tasks, including checking for existing cheatsheets on the OWASP site, determining if they require updates for machine learning contexts, assessing the need for a new cheatsheet, and adding any relevant cheatsheet to the Top 10 risk document. It references examples of existing cheatsheets and how they are linked to the Top 10 risks. The next steps involve conducting the checks and updates as outlined.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/157",
@@ -16627,9 +17191,9 @@
"repository": 981,
"assignees": [],
"labels": [
- 135,
136,
- 141
+ 141,
+ 135
]
}
},
@@ -16642,6 +17206,7 @@
"node_id": "I_kwDODGfvrc51RddC",
"title": "feat(docs): create a cheatsheet for ML10 Model Poisoning",
"body": "- [ ] Is there existing cheatsheets at [OWASP Cheatsheets](https://cheatsheetseries.owasp.org/Glossary.html)\n- [ ] If there is an existing cheatsheet, does it need updating at the source to cater for machine learning use cases?\n- [ ] Is there a need for a new cheatsheet topic?\n- [ ] Add existing or new cheatsheet as a reference to the Top 10 risk document\n\nExample Cheatsheet: [Input Validation Cheatsheet](https://github.com/OWASP/CheatSheetSeries/blob/master/cheatsheets/Input_Validation_Cheat_Sheet.md)\n\nExample of Top 10 risk referencing cheatsheets: [ML01 Input Manipulation Attacks - Cheatsheets](https://github.com/OWASP/www-project-machine-learning-security-top-10/blob/master/docs/cheatsheets/ML01_2023-Input_Manipulation_Attack-Cheatsheet.md)",
+ "summary": "The issue discusses the creation of a cheatsheet for ML10 Model Poisoning. Key tasks include checking for existing cheatsheets on the OWASP website, determining if any need updates to address machine learning use cases, assessing the necessity for a new cheatsheet topic, and incorporating either an existing or a new cheatsheet as a reference in the Top 10 risk document. It may be beneficial to explore related examples for guidance.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/158",
@@ -16657,9 +17222,9 @@
"repository": 981,
"assignees": [],
"labels": [
- 135,
136,
- 141
+ 141,
+ 135
]
}
},
@@ -16672,6 +17237,7 @@
"node_id": "I_kwDODGfvrc51Rx2I",
"title": "chore(admin): assign owner(s) for ML01 Input Validation Attack",
"body": "- [x] Assigned Lead Contributor for ML01\n- [x] Update CODEOWNERS with contributor details\n\nIdeally the Lead Contributor for ML01 will also be assigned to the cheatsheet - ref: #147 ",
+ "summary": "The issue involves assigning an owner for the ML01 Input Validation Attack and updating the CODEOWNERS file with the relevant contributor details. It has been noted that the Lead Contributor for ML01 should also be linked to the associated cheatsheet, as referenced in a previous issue. To complete the task, ensure that the Lead Contributor is assigned appropriately and that the CODEOWNERS file reflects these changes.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/163",
@@ -16703,6 +17269,7 @@
"node_id": "I_kwDODGfvrc51RzAU",
"title": "chore(admin): assign owner(s) for ML03 Model Inversion Attack",
"body": "- [ ] Assigned Lead Contributor for ML03\n- [ ] Update CODEOWNERS with contributor details\n\nIdeally the Lead Contributor for ML03 will also be assigned to the cheatsheet - ref: #151 ",
+ "summary": "The issue involves assigning a lead contributor for the ML03 Model Inversion Attack and updating the CODEOWNERS file with the contributor's details. Additionally, it is suggested that the lead contributor for ML03 should also be assigned to the associated cheatsheet referenced in another issue. The tasks to be completed include identifying and assigning the lead contributor and making the necessary updates to the CODEOWNERS file.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/165",
@@ -16732,6 +17299,7 @@
"node_id": "I_kwDODGfvrc51RzRE",
"title": "chore(admin): assign owner(s) for ML04 Membership Inference Attack",
"body": "- [ ] Assigned Lead Contributor for ML04\n- [ ] Update CODEOWNERS with contributor details\n\nIdeally the Lead Contributor for ML04 will also be assigned to the cheatsheet - ref: #152 ",
+ "summary": "The issue involves assigning a Lead Contributor for the ML04 Membership Inference Attack and updating the CODEOWNERS file with the contributor's details. It is suggested that the Lead Contributor for ML04 should also be designated for the associated cheatsheet. Action items include identifying and assigning the Lead Contributor and making the necessary updates to the CODEOWNERS file.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/166",
@@ -16761,6 +17329,7 @@
"node_id": "I_kwDODGfvrc51Rzdy",
"title": "chore(admin): assign owner(s) for ML05 Model Theft",
"body": "- [ ] Assigned Lead Contributor for ML05\n- [ ] Update CODEOWNERS with contributor details\n\nIdeally the Lead Contributor for ML05 will also be assigned to the cheatsheet - ref: #153 ",
+ "summary": "The issue involves assigning a Lead Contributor for the ML05 Model Theft project and updating the CODEOWNERS file to include the contributor's details. Additionally, it suggests that the Lead Contributor should also be assigned to the related cheatsheet. To resolve this, the necessary assignments and updates should be made.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/167",
@@ -16790,6 +17359,7 @@
"node_id": "I_kwDODGfvrc51R0Mh",
"title": "chore(admin): assign owner(s) for ML08 Model Skewing",
"body": "- [ ] Assigned Lead Contributor for ML08\n- [ ] Update CODEOWNERS with contributor details\n\nIdeally the Lead Contributor for ML08 will also be assigned to the cheatsheet - ref: #156 ",
+ "summary": "The issue involves assigning a Lead Contributor for the ML08 Model Skewing and updating the CODEOWNERS file with the contributor's details. Additionally, it suggests that the Lead Contributor should also be involved with the cheatsheet referenced in another issue. To address this, the assigned contributor needs to be determined and the necessary updates made to the CODEOWNERS file.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/170",
@@ -16819,6 +17389,7 @@
"node_id": "I_kwDODGfvrc51R0Zy",
"title": "chore(admin): assign owner(s) for ML09 Output Integrity Attack",
"body": "- [ ] Assigned Lead Contributor for ML09\n- [ ] Update CODEOWNERS with contributor details\n\nIdeally the Lead Contributor for ML09 will also be assigned to the cheatsheet - ref: #157 ",
+ "summary": "The issue highlights the need to assign a Lead Contributor for the ML09 Output Integrity Attack and to update the CODEOWNERS file with the contributor's details. Additionally, it suggests that the Lead Contributor should also be linked to the cheatsheet referenced in another issue. Action items include assigning a contributor and updating relevant documentation.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/171",
@@ -16848,6 +17419,7 @@
"node_id": "I_kwDODGfvrc51R0sl",
"title": "chore(admin): assign owner(s) for ML10 Model Poisoning",
"body": "- [ ] Assigned Lead Contributor for ML10\n- [ ] Update CODEOWNERS with contributor details\n\nIdeally the Lead Contributor for ML10 will also be assigned to the cheatsheet - ref: #158 ",
+ "summary": "The issue discusses the need to assign a Lead Contributor for the ML10 Model Poisoning project and to update the CODEOWNERS file with the contributor's details. Additionally, it suggests that the Lead Contributor should also be assigned to the related cheatsheet as referenced in another issue. To resolve this, the necessary assignments should be made and the CODEOWNERS file updated accordingly.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/172",
@@ -16877,6 +17449,7 @@
"node_id": "I_kwDODGfvrc51eUcp",
"title": "feat(docs): create a GLOSSARY page of commonly used terms",
"body": "Machine Learning has a lot of terminology that may be new to people. Need to create a glossary page of commonly used terms.",
+ "summary": "A request has been made to create a glossary page that defines commonly used terms in Machine Learning, as the existing terminology may be unfamiliar to many users. To address this, a glossary with clear definitions of key terms should be developed.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/178",
@@ -16894,8 +17467,8 @@
244
],
"labels": [
- 134,
- 136
+ 136,
+ 134
]
}
},
@@ -16908,6 +17481,7 @@
"node_id": "I_kwDODGfvrc51e28g",
"title": "fix: merge review from @harrietf",
"body": "[Harriet Farlow](https://www.linkedin.com/in/harriet-farlow-654963b7/) sent through her feedback via mail.\r\n\r\nUploading Word doc and also outputting to Markdown in this issue to track.\r\n\r\nFeedback in Word doc: [OWASP Top Ten Feedback.docx](https://github.com/OWASP/www-project-machine-learning-security-top-10/files/13219930/OWASP.Top.Ten.Feedback.docx)\r\n\r\n\r\n\r\nOutput in Markdown from Word doc below:\r\n---\r\n**General feedback - the list and the home page**\r\n\r\nI think this is great! Some feedback below:\r\n\r\n- I would also add something about these kinds of mitigations being\r\n risk-based, and that each organisation should make a risk-based\r\n assessment of which specific attacks are more likely to be employed\r\n against their models, how impactful the ramifications would be (ie.\r\n would they lose massive amounts of PII or would someone just get a\r\n bad customer experience) and then decide on their ML security\r\n posture from there.\r\n\r\n- I would also highlight some of the reasons why ML systems are\r\n different to traditional cyber systems (ie. ML systems are\r\n probabilistic while cyber systems are rules based, ML systems learn\r\n and evolve while cyber systems are more static). From an\r\n organisational perspective this means that it is unfair to expect\r\n cyber security professionals to automatically know how best to\r\n secure ML systems, and that there should be investment in training\r\n and/or the creation of new roles\r\n\r\n- I would also add a comment about the terminology here being ML\r\n security vs AI security. Clarify the difference between ML and AI\r\n (ie. AI is a broader set of technologies while ML refers\r\n specifically to the AI sub-field of ML models). For example, I\r\n usually refer to my work as AI Security to be more encompassing of\r\n an audience who is used to hearing about AI, but most of what I talk\r\n about is actually ML security. Adding something here about these\r\n terms would be useful for non-ML folk.\r\n\r\n**Some questions before I give feedback on these things**\r\n\r\n- Is the intention of the top 10 list that it is also ordered so that\r\n #1 is most threatening, or is it unordered?\r\n\r\n- What is the methodology/reference for the risk factor values under\r\n each threat? (I know it comes from the specific scenarios, but is it\r\n meant to also be representative of 'common' ways of implementing the\r\n attack? Because it could be very different and might be interpreted\r\n as generic)\r\n\r\n**ML01:2023 Input Manipulation Attack**\r\n\r\n- Where Adversarial Attacks are referenced in the intro, I usually see\r\n this referred to as an Adversarial Example instead and this language\r\n would be more consistent. I'm also not sure what it means by saying\r\n it's an umbrella term, what are the other attacks underneath this\r\n umbrella?\r\n\r\n- The list Adversarial Training, Robust Models, Input Validation are\r\n all good to include but could be rephrased to be mutually exclusive\r\n (ie. the Robust Models section references both Adversarial Training\r\n and Input Validation as the way to make models robust). You could\r\n start with Robust Models first and still mention that those other\r\n two techniques are some ways to make model robust, but is also\r\n dependent on good data quality (clean data, good feature\r\n engineering, complete data), model selection (having to choose an\r\n appropriate balance between accuracy and interpretability, which are\r\n usually trade-offs), considering ensemble methods instead of a\r\n single model (ie. you get results from multiple models as a way of\r\n checking and validation output). You can then into system level\r\n security measures (input validation) and process-based security\r\n measures (incorporating adversarial training).\r\n\r\n- Love the scenarios, that really helps to put it in perspective.\r\n\r\n**ML02:2023 Data Poisoning Attack**\r\n\r\n- Would be worth including more here in the 'about' section around how\r\n this normally happens (an organisation's data repository could have\r\n new data added, or existing data altered), why it works (an ML\r\n model's heavy reliance on training data and the fact that it needs\r\n LOTS of it, which means it's also very hard to spot if it has been\r\n tampered with), who is likely to do this (someone either needs\r\n access to the data repository if the model is trained on internal\r\n data only in which case an insider threat scenario is more likely,\r\n or if the model learns from 'new' data fed from the outside like a\r\n chatbot that learns off inputs without validation (ie. Tay) then\r\n this is a much easier attack) etc. Also clarify how this is\r\n different to the cyber security challenge of just keeping data\r\n secure (ie. it's more about the statistical distribution of the data\r\n than the likelihood of data breach).\r\n\r\n- The 'how to prevent this section' is great, and adding more info\r\n beforehand might help make it clearer as to why these measures are\r\n important, and why the measure to secure data is for different\r\n reasons than traditional cyber security threat models.\r\n\r\n- Again, great examples. You could add something like a chatbot or\r\n social media model here to underscore how publicly injected data is\r\n also a risk here.\r\n\r\n**ML03:2023 Model Inversion Attack & ML04:2023 Membership Inference\r\nAttack**\r\n\r\n- These two attacks could be clarified as to exactly how they're\r\n different, and depending on how you expect someone might use the Top\r\n 10, you could consider combining them. For example, they both leak\r\n information about data used to train the model but model inversion\r\n is about leaking any data, and membership inference is more about\r\n seeing if a specific piece of data was used. Or you could clarify\r\n that they are separate based on the different controls used to\r\n prevent it (ie. is the focus on differentiation here the goal of\r\n attack, or the means of securing it)\r\n\r\n- Model inversion - you could explain what it means to\r\n 'reverse-engineer' in this context. Ie. it is more about targeted\r\n prompting than reverse engineering in a cyber context. This would\r\n also explain why the suggested controls (ie. input verification)\r\n work.\r\n\r\n**ML05:2023 Model Stealing**\r\n\r\n- This is good! Again, I think a longer description would be helpful.\r\n\r\n**ML06:2023 AI Supply Chain Attacks**\r\n\r\n- Yes, love that this is included!\r\n\r\n- Why are we switching from ML to AI here?\r\n\r\n**ML07:2023 Transfer Learning Attack**\r\n\r\n- This is interesting.. In my mind this is more an AI misuse issue\r\n than an AI security issue, but it really depends on the scenario I\r\n think. Could be worth clarifying here.\r\n\r\n**ML08:2023 Model Skewing**\r\n\r\n- This also seems the same as the data poisoning attack.. It's not\r\n clear how they're different. Usually when I saw model skewing the\r\n attack is based on being able to change the model outcome without\r\n actually touching the training data.. Would be worth clarifying\r\n here.\r\n\r\n**ML09:2023 Output Integrity Attack**\r\n\r\n- Again, this attack overlaps a lot with other attacks already listed\r\n that aim to impact the output, it's almost more of an umbrella term\r\n in my mind. Or it could be different but it depends on how an\r\n organisation creates its threat model. The controls mentioned here\r\n are good though, so it's worth clarifying why this is listed as a\r\n different attack and if it's based on the controls suggested (which\r\n would also apply to the other attacks listed).\r\n\r\n**ML10:2023 Model Poisoning**\r\n\r\n- Yes, this is good, and worth adding a bit more about how this can\r\n happen (ie. direct alteration of the model through injection, the\r\n interaction with hardware here and how this can be done in memory\r\n etc)\r\n\r\n",
+ "summary": "The issue discusses feedback received on a project related to ML security threats. The feedback covers various aspects, including the need for a risk-based approach to mitigations, differentiation between ML and AI security, and clarity on specific attacks. Detailed comments are provided on each of the top ten threats, suggesting improvements in terminology, descriptions, and explanations of concepts. \n\nKey points include:\n\n- Incorporating risk assessments for organizations regarding potential attacks.\n- Highlighting the differences between ML and traditional cyber systems.\n- Clarifying terminology used for ML and AI security.\n- Providing further details on specific attacks and their prevention methods.\n\nTo address the feedback, it may be necessary to revise the documentation with clearer definitions, additional context for the attacks, and an explanation of the methodology behind the rankings and risk factors.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/182",
@@ -16940,6 +17514,7 @@
"node_id": "I_kwDODGfvrc53FVcs",
"title": "[FEEDBACK]: Sync attack names between LLMT10 and MLT10 where appropriate",
"body": "### Type\n\nSuggestions for Improvement\n\n### What would you like to report?\n\nI would like to make the suggestion that we consolidate the terms used in the LLM and ML top 10 documents.\r\n\r\nMany of the top 10 items in each are closely related or even the same.\r\nWhere possible, the same term should be used (i.e. Model Theft vs Model Stealing, Data Poisoning Attack vs Training data Poisoning).\r\n\r\nThanks!\n\n### Code of Conduct\n\n- [X] I agree to follow this project's Code of Conduct",
+ "summary": "The issue suggests consolidating the terminology used in the LLM and ML top 10 documents, as many items are closely related or identical. It recommends using consistent terms, such as \"Model Theft\" instead of \"Model Stealing,\" and \"Data Poisoning Attack\" instead of \"Training Data Poisoning.\" The next steps would involve reviewing both documents to identify and align the related terms.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/187",
@@ -16971,6 +17546,7 @@
"node_id": "I_kwDODGfvrc53Iuq0",
"title": "[FEEDBACK]: Include MLOps vulnerabilties somewhere in the Supply Chain Security category ",
"body": "### Type\r\n\r\nSuggestions for Improvement\r\n\r\n### What would you like to report?\r\n\r\n**Context**\r\nOne of the parts of the supply chain in modern ML systems is MLOps software - like i.e. MLFlow, Prefect etc. Those systems are vulnerable to classic web based attacks and they seem to be \"misconfured by default\". I've described it here: https://hackstery.com/2023/10/13/no-one-is-prefect-is-your-mlops-infrastructure-leaking-secrets/ or here: https://github.com/logspace-ai/langflow/issues/1145 \r\n\r\n**Suggestion for improvement**\r\nI'd suggest including MLOps-related vulnerabilities in the ML06 (or maybe in some other categories as well? I am open for suggestions). \r\n\r\n### Code of Conduct\r\n\r\n- [X] I agree to follow this project's Code of Conduct",
+ "summary": "The issue raises a suggestion to include MLOps-related vulnerabilities within the Supply Chain Security category, highlighting that MLOps software, such as MLFlow and Prefect, is susceptible to common web-based attacks and often comes misconfigured by default. The author points to existing resources that elaborate on these vulnerabilities. The suggestion is to categorize these vulnerabilities appropriately, potentially under ML06 or other suitable categories. To address this, it may be necessary to assess current categories and determine where MLOps vulnerabilities can be integrated.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/188",
@@ -16985,8 +17561,8 @@
"author": 241,
"repository": 981,
"assignees": [
- 238,
- 241
+ 241,
+ 238
],
"labels": [
132,
@@ -17003,6 +17579,7 @@
"node_id": "I_kwDODGfvrc53sTiJ",
"title": "[FEEDBACK]: Include a page with a brief descriptions of each of the vulnerabilities",
"body": "### Type\n\nSuggestions for Improvement\n\n### What would you like to report?\n\nFor example in Top10 for LLM there's this page with a summary of each of the vulnerabilities, which I think would be pretty useful to have in Top10 for ML as well. \r\n\r\nSometimes when you e.g. work on some slides for a presentation, you just want to get a short summary of each of the vulnerabilities. In my opinion including such a page in Top10 for ML would be an improvement: \r\n\r\n\n\n### Code of Conduct\n\n- [X] I agree to follow this project's Code of Conduct",
+ "summary": "The issue suggests adding a page that provides brief descriptions of each vulnerability in the Top10 for Machine Learning (ML) project. This addition is seen as beneficial for users who may need quick summaries for presentations or other purposes, similar to a feature available in the Top10 for LLM. Implementing this suggestion could enhance the usability of the resource.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/189",
@@ -17034,6 +17611,7 @@
"node_id": "I_kwDODGfvrc6B14iW",
"title": "OWASP Top 10 ML Summaries",
"body": "Ref: https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/189\r\n\r\nAs of now, do we need a markdown file for this or a powerpoint page which contains all the summaries for the project? \r\n\r\ncc: @shsingh @mik0w ",
+ "summary": "The issue discusses whether a markdown file or a PowerPoint slide should be created to compile all the summaries related to the OWASP Top 10 Machine Learning Security project. It is suggested that a decision needs to be made on the format for presenting this information. Consideration should be given to the best way to organize and share the summaries effectively.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/205",
@@ -17058,10 +17636,11 @@
"pk": 595,
"fields": {
"nest_created_at": "2024-09-11T20:42:41.693Z",
- "nest_updated_at": "2024-09-11T20:42:41.693Z",
+ "nest_updated_at": "2024-09-13T16:07:00.058Z",
"node_id": "I_kwDODGfvrc6LD2Xp",
"title": "[FEEDBACK]: Description of ML04 Membership Inference Attack",
"body": "### Type\n\nGeneral Feedback\n\n### What would you like to report?\n\nhttps://github.com/OWASP/www-project-machine-learning-security-top-10/blob/f1cf662ca9ce5cfcd4c72ab8d4bff91ea64f46d7/docs/ML04_2023-Membership_Inference_Attack.md?plain=1#L27\r\n\r\nHere, the documentation states that `an attacker manipulates the model’s training data`, but from my understanding the objective of a membership inference attack is to [\"\\[...\\] predict whether or not a particular example was contained in the model’s training dataset.\"](https://ieeexplore.ieee.org/document/9833649), so the attacker shouldn't have access to the training data.\r\n\r\nI can create a pull request to update the documentation. Let me know if you'd like me to proceed.\n\n### Code of Conduct\n\n- [X] I agree to follow this project's Code of Conduct",
+ "summary": "The issue raises a concern regarding the description of a membership inference attack in the documentation. It points out that the current wording suggests the attacker manipulates the model's training data, which contradicts the fundamental nature of such attacks, where the attacker typically does not have access to the training data. The user is willing to create a pull request to clarify this point in the documentation if it is deemed necessary. \n\nTo address this, a review of the documentation's language is suggested to ensure it accurately reflects the mechanics of membership inference attacks.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-machine-learning-security-top-10/issues/210",
@@ -17079,8 +17658,8 @@
238
],
"labels": [
- 132,
- 143
+ 143,
+ 132
]
}
},
@@ -17089,10 +17668,11 @@
"pk": 596,
"fields": {
"nest_created_at": "2024-09-11T20:43:41.238Z",
- "nest_updated_at": "2024-09-11T20:43:41.238Z",
+ "nest_updated_at": "2024-09-13T16:07:47.175Z",
"node_id": "I_kwDODGftyc5feB1C",
"title": "small grammar error",
"body": "In the index.md, and in CNAS-1: Insecure cloud, container or orchestration configuration\r\nshould 'Imrpoper permissions' change to 'Improper permissions' ?",
+ "summary": "There is a small grammar error in the index.md file, specifically in the section titled \"CNAS-1: Insecure cloud, container or orchestration configuration.\" The term \"Imrpoper permissions\" should be corrected to \"Improper permissions.\" A fix is needed to update the text accordingly.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-cloud-native-application-security-top-10/issues/8",
@@ -17115,10 +17695,11 @@
"pk": 597,
"fields": {
"nest_created_at": "2024-09-11T20:43:57.448Z",
- "nest_updated_at": "2024-09-11T20:43:57.448Z",
+ "nest_updated_at": "2024-09-13T16:08:00.528Z",
"node_id": "I_kwDODGftZc5fWt9s",
"title": "SwSec 5D Survey",
"body": "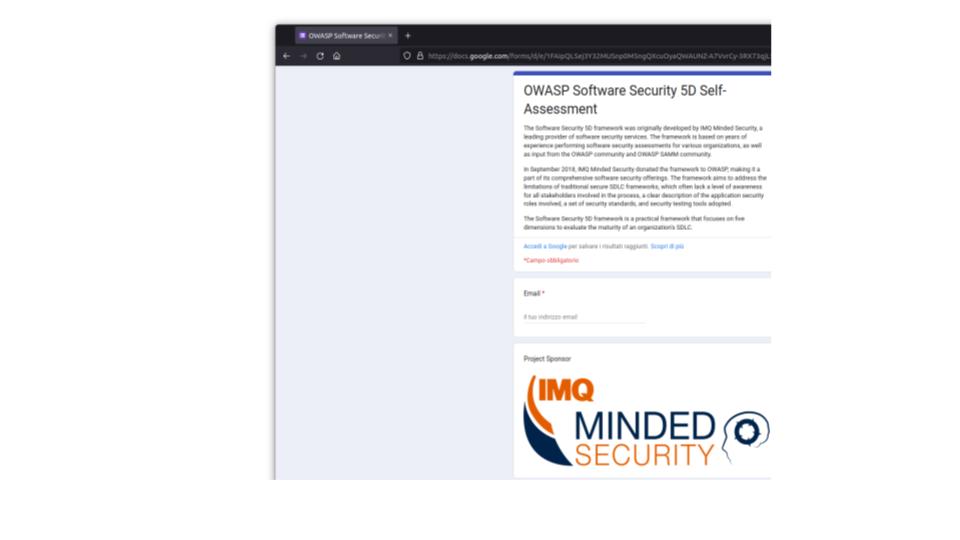\r\n",
+ "summary": "The issue discusses the SwSec 5D Survey and includes an image related to it. The main focus appears to be on gathering feedback or information regarding the survey. It may be beneficial to clarify the survey's objectives or instructions for participants to ensure effective responses.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-software-security-5d-framework/issues/1",
@@ -17141,10 +17722,11 @@
"pk": 598,
"fields": {
"nest_created_at": "2024-09-11T20:44:07.768Z",
- "nest_updated_at": "2024-09-11T20:44:07.768Z",
+ "nest_updated_at": "2024-09-13T16:08:09.039Z",
"node_id": "MDU6SXNzdWU5NTIzODAyOTU=",
"title": "Add a Matrix ",
"body": "Add a matrix (likely an .xlsx file) that:\r\n\r\n* Can be used as a checklist and note-taking / task-tracking\r\n* Includes links from each task’s inputs and outputs to their corresponding tasks, as described in the OWASP-Vuln-MGM-Guide-Jul23-2020.pdf file",
+ "summary": "The issue suggests adding a matrix, probably in the form of an .xlsx file, that serves as a checklist and allows for note-taking or task tracking. It should also include links connecting each task's inputs and outputs to related tasks as outlined in the specified OWASP guide. To address this, one would need to create the matrix and ensure it aligns with the guidelines from the referenced document.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-vulnerability-management-guide/issues/1",
@@ -17171,10 +17753,11 @@
"pk": 599,
"fields": {
"nest_created_at": "2024-09-11T20:44:19.189Z",
- "nest_updated_at": "2024-09-11T20:44:19.189Z",
+ "nest_updated_at": "2024-09-13T16:08:18.147Z",
"node_id": "I_kwDODGfszM5G7xDs",
"title": "Attack Surface Detector plugin will allways start spider",
"body": "After executing Attack Surface Detector plugin in Owasp Zap Proxy, it will try to spider the application even if the checkbox **Automatically start spider after importing endpoints** is unchecked. \r\n\r\nHere is a print showing the problem:\r\n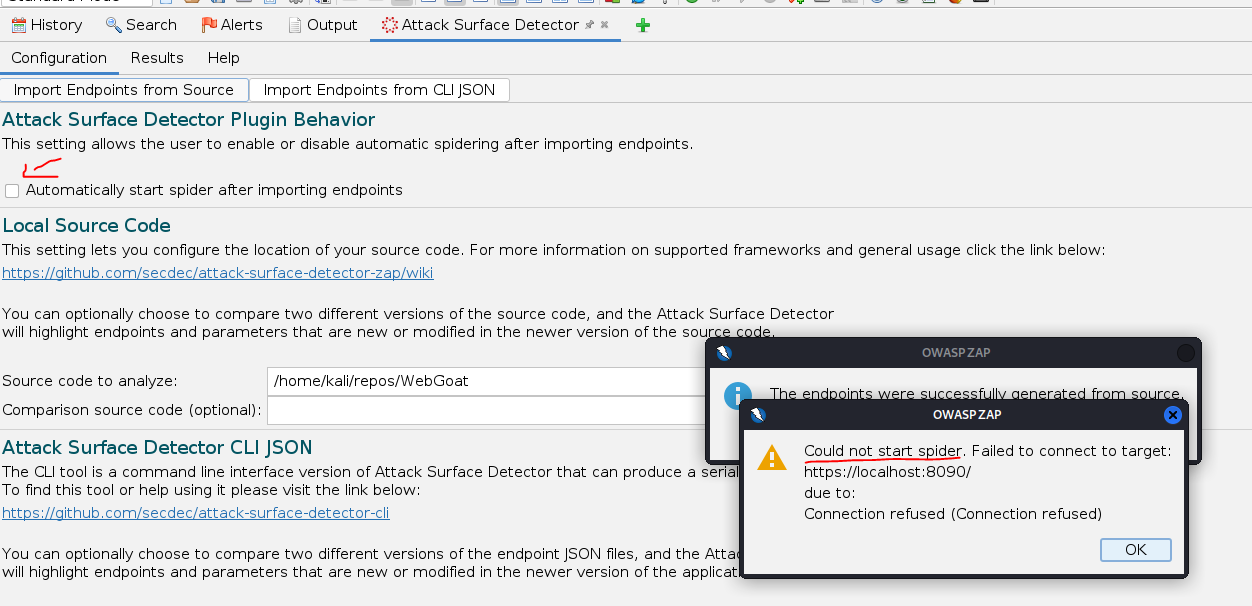\r\n",
+ "summary": "Investigate the Attack Surface Detector plugin behavior in OWASP ZAP Proxy. Confirm that the plugin starts the spider process regardless of the **Automatically start spider after importing endpoints** option being unchecked. \n\n1. Review the code responsible for the Attack Surface Detector plugin's configuration and execution flow.\n2. Identify where the spider initiation logic is implemented and check for any conditional checks tied to the checkbox state.\n3. Implement logging to capture the state of the checkbox when the plugin runs and the spider starts.\n4. Test the plugin with various configurations to reproduce the issue consistently.\n5. Propose a solution to ensure the spider only starts when the checkbox is checked. \n\nDocument findings and submit a pull request with the necessary changes once the root cause is identified.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-project-attack-surface-detector/issues/1",
@@ -17201,6 +17784,7 @@
"node_id": "MDU6SXNzdWU2MDE3MzM5MDI=",
"title": "Request for a bit more explanation",
"body": "Congrats on the new release! Looks really useful!\r\nI do wonder about a few requirements (See below): can you help me understand what they mean? It would be great if the document can provide a bit more explanation on them!\r\n\r\n`2.8: SBOM is analyzed for risk` ; what type of risk? is that development risks ? (e.g. continuity due to often changing signature of the functions it provides?) or only security risk? Or project continuity risk (e.g. license removed, etc.)\r\n\r\n`2.11: SBOM contains metadata about the asset or software the SBOM describes `: what type of metadata are we talking about :) ?\r\n\r\n`3.9; Application build pipeline prohibits alteration of certificate trust stores` ; if you do infrastructure as code and use a 4-eyed/hardened/etc. gitlab configuring pipeline which triggers a certificate manager from the underlying infrastructure to update trust-stores... then that is a lot safer than doing it my hand ... Ofcourse the requirement says application build pipeline, but i wonder: is there a split between configuration pipeline/application pipeline/infra pipeline?\r\n\r\n` 4.6: Package repository supports security incident reporting` ; where should it report to?\r\n\r\n`4.18: Package manager does not execute code` : do you mean of the packages that it stores, because a lot of the functionality described is only available when you \"upgrade\"/\"install plugin X for manager Y\", etc.?\r\n\r\nI love the standard already; it helps explaining what people should expect from the component management side of things! Will you incorporate something like a verification guide and/or a compliancy list like at the MSTG does for the MASVS? Then it could be easily incorporated into existing standardization processes!",
+ "summary": "There is a request for clarification on several requirements related to a recent software release. The user seeks additional details on the following points:\n\n1. Requirement 2.8 regarding the types of risks analyzed in the SBOM (Software Bill of Materials), questioning whether it includes development, security, or project continuity risks.\n2. Requirement 2.11 asks for specifics on the type of metadata included in the SBOM.\n3. Requirement 3.9 raises concerns about the distinction between different types of pipelines (configuration, application, infrastructure) in relation to certificate trust stores.\n4. Requirement 4.6 inquires about the destination for security incident reporting.\n5. Requirement 4.18 seeks clarification on whether the restriction on executing code pertains to the packages stored in the manager.\n\nAdditionally, there is a suggestion to create a verification guide or compliance list similar to existing standards to enhance integration into standardization processes. A response addressing these points would be beneficial.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/Software-Component-Verification-Standard/issues/9",
@@ -17229,6 +17813,7 @@
"node_id": "MDU6SXNzdWU2MDMzNDE5MjM=",
"title": "David A. Wheeler's comments",
"body": "Comments on OWASP “Software Component Verification Standard” by David A. Wheeler\r\n\r\nHere are my comments on the “Software Component Verification Standard” Version 1.0.0-RC.1 (Public Preview), 16 April 2020, https://owasp-scvs.gitbook.io/scvs/ My apologies that’s it’s one long document; I wrote my comments & then discovered that comments were wanted via GitHub. Had I known that I would have split this up. But hopefully they’ll be useful anyway.\r\n\r\nThis document’s frontispiece says it’s a “grouping of controls, separated by domain, which can be used by architects, developers, security, legal, and compliance to define, build, and verify the integrity of their software supply chain.” So I’m commenting on the document based on that understanding. My high-level comments:\r\n\r\n1. Many requirements are far too vague. It’s impossible to properly review this document without knowing what is necessary to meet its requirements. For every requirement it should be clear how it could and could not be met. See my detailed comments before for many examples of this vagueness. In many cases a key issue is “how far down (transitively) does this go?” Similarly, how much provenance & pedigree information is enough? It appears that the world wide web plus Google would count as a package manager; I presume that was not intended. I recommend trying to rewrite the requirements assuming that a lazy or malicious contractor will re-interpret them in the lowest-cost or most evil way. You can’t really make requirements impossible to re-interpret that way, but that exercise will help turn these vague requirements into more precise requirements that can be reviewed and applied.\r\n2. It’s not clear some of these requirements are practical (again, primarily because they’re too vague to know what they mean). In some cases, depending on their interpretation, the only way they’ll be widely practical is if their implementations are embedded into OSS package managers as OSS. If OWASP intends to help implement some of these in OSS package managers, that could be great, but OWASP needs to avoid mandating impractical or excessively expensive requirements.\r\n3. Does this really apply to entire organizations? The preface suggests it, but in many cases this is probably impractical. The document should instead discuss it being applied to specific projects within an organization, or a specific project, not necessarily a whole organization. That way, organizations can grow into these requirements if they desire.\r\n4. Is this intended to apply to open source software (OSS) projects themselves? OSS projects themselves have supply chains, and their supply chains become the supply chains of their downstream users. I wanted to see if these requirements could be practically met by OSS projects. However, the vagueness of the requirements makes it impossible to determine if an OSS project could implement them. I think the requirements need to be revised to specifically ensure that OSS projects could meet the requirements. Imagine at least one way that an OSS project could implement them. If these requirements can’t be met by OSS projects, then this document is probably impractical, because those dependencies will become transitive requirements on all their users including practically all serious projects today. So again: please review these requirements with that specifically in mind (once the requirements are reworded enough so they can be analyzed).\r\n5. There is no identified threat model, nor any mapping to show that these controls are both adequate and the minimum necessary to counter those threats. What attacks are you trying to counter? Are there controls missing? Are some unnecessary?\r\n6. In some cases it’s not even obvious why anyone would want to meet the requirement as stated. Why would that help? In some cases that would help the vagueness, e.g., “Do XYZ so that will be detected/countered”, because that would help people determine if a particular implementation would meet the need, and it would also help people understand the risk of not meeting some requirement. It would especially help people decide when waivers are fine.\r\n7. The document’s title is extremely misleading & needs to be changed. This document is not about Software Component Verification, so don’t say that it is. Change the title to accurately reflect its comments, in particular that it focuses on supply chain, as I discuss in my specific comments.\r\n\r\nBelow are specific comments.\r\n\r\n=======================\r\n\r\n\r\nSpecific comments:\r\n\r\nTitle: The title is wrong or at least dreadfully misleading. The title needs to be changed to something that accurately reflects its contents. Currently the title says it’s the “Software Component Verification Standard” - yet this specification doesn’t cover software component verification. Per ISO/IEC 15288 and ISO/IEC 12207 the purpose of verification is to ensure that the system meets its requirements (including regulations and such). Yet nothing in this specification verifies that the component meets its requirements, so by definition this document doesn’t support (general) verification. It doesn’t even verify that a component meets its security requirements (never mind ALL its requirements). In addition, the “component analysis” section is inadequate for a serious security analysis; it doesn’t include many important measures to analyze the security of a component. The frontispiece also makes it clear that the title is wrong; the frontispiece says that this specification focuses on the “integrity of their software supply chain.” Since this document is actually focused on software security *supply chain* issues, and not software component verification, the title should be changed to reflect its actual purpose instead of its current misleading name. Please change the title to reflect the document’s actual purpose. An example would be “Software Component Supply Chain Integrity Verification Standard”; I’m sure there are many other possible names. Please ensure that the title clearly and accurately reflects its contents to readers who have not yet read it.\r\n\r\nIn chapter ”Assessment and Certification”:\r\n\r\nIt says, “The recommended way of verifying compliance of a software supply chain with SCVS is by performing an \"open book\" review, meaning that the auditors are granted access to key resources such as legal, procurement, build engineers, developers, repositories, documentation, and build environments with source code.” This text - specifically the term “auditors” - seems to presume that the specification will only be used for third-party audits. Yet I expect a major use of this document (if used) will be for organizations to determine, for themselves, if they meet the requirements. The frontispiece says that the document’s users include “architects, developers, security, legal,...” - but there isn’t any discussion on how THESE groups would use this document The use of this by organizations on themselves needs to be discussed somewhere. I recommend that somewhere before this section there be a discussion on how organizations can use this themselves in various roles, and THEN discuss certification, to make it clear that uses other than certification are supported.\r\n\r\nIn chapter “Using SCVS”:\r\n\r\nNowhere does this document explain V1 through V6, we just get dumped into this list in those sections. What are you calling them (domains? families?)? There should be a hint of that early in the document. I suggest as the first section of “Using SCVS” there be a subsection called “Control families” with text like this: “This specification identifies a set of 6 control families. Each family has an identifier (V1 through V6) and contains a number of specific controls (numbered V1.1, V1.2, and so on). These six families are inventory (V1), software bill of materials (V2), build environment (V3), package management (V4), component analysis (V5), and pedigree and provenance (V6).”\r\n\r\nIt’s claimed that it’s to “Develop a common taxonomy of activities, controls, and best-practices that can reduce risk in a software supply chain”. There’s no real taxonomy here, at least not in the meaning many would accept. State what you’re *actually* doing instead, don’t call it a taxonomy.\r\n\r\nIn chapter “V1 Inventory”\r\nChapters V1 and V2 make a big deal out of distinguishing “inventory” from “software bill of material” (SBOM) yet there seems to be no real difference and it certainly isn’t clearly explained. The term “inventory” is not defined in the glossary. From the text it appears that an SBOM is simply the inventory list. if so, why use two different words for basically the same thing? I’m guessing a difference was intended but the document doesn’t clearly state it. This concern affects everything that uses the term “inventory” and “SBOM”. As a result, I don’t know exactly what many of these requirements actually mean, making it hard to review & hard to apply.\r\nAdd underlined, as that’s another common way to identify something: “Component identification varies based on the ecosystem the component is part of. Therefore, for all inventory purposes, the use of identifiers such as Package URL or (name of common package manager repository + name of package within it) may be used to standardize and normalize naming conventions for managed dependencies.\r\nIn the text, “Having organizational inventory of all first-party, third-party, and open source components…” - that doesn’t make sense, OSS components are also first-party or third-party, Perhaps rewrite to “all first-party and third-party components, whether they are proprietary or open source software, …”\r\n\r\nRequirement V1.1: “All components and their versions are known at completion of a build” - does this include transitive dependencies? Does it include the underlying operating system & database (which may be tracked by a different system)? How about compile/build tools like compilers and test frameworks? It’s so brief that I have no idea what this requirement means.\r\n\r\nV1.2: Says, “Package managers are used to manage all third-party binary components” - what is meant by a package manager? If I have a project-local shell script that re-downloads field from URLs listed in a file, does that count? Shouldn’t there be a separate requirement for third-party source code (not binary) components at higher levels? E.g., JavaScript & Python? I strongly encourage people to use package managers, but it’s not clear that demanding them for all binary components is practical, especially for embedded systems like IoT systems.\r\n\r\nV1.3 & V1.4: V1.3 says “Inventory”, V1.4 says “bill of materials”. As noted earlier, I see no serious difference, say “bill of materials” in both places or explain why they’re different somewhere. These need clear definitions.\r\n\r\nV1.3: “An accurate inventory of all third-party components is available in a machine-readable format” - again, is this transitive? Most proprietary libraries will NOT allow their recipients to find out what’s transitively included. I think it’s a great goal, but I think a minority of current projects could manage this today; I have concerns about its practicality unless you’re only applying this to green field development.\r\n\r\nV1.5: “Software bill-of-materials are required for new procurements” - does this mean GENERATING an SBOM is required, or that software for use must come with an SBOM? If it’s the latter, today that’s often impractical, especially for any proprietary software. Also, once again, this is the “inventory” section but it’s asking about SBOMs… which again leads me to believe there’s no real difference.\r\n\r\n\r\nV1,10 “Point of origin is known for all components” - what does this mean? I record the https URL? I have the passport numbers for all the authors? I have no idea how to verify this, or really even what it means.\r\n\r\n\r\nIn chapter V2:\r\n\r\nV2.1: “A structured, machine readable software bill-of-materials (SBOM) format is present” - this is yet another requirement that needs to be reworded to be clear. You mean that I have to find a random SBOM off the street & put it in my directory? Is this the SBOM for the software I’m developing? Are these SBOMs for the software I’m ingesting? How deep do they need to go - can it be just direct dependencies?\r\nV2.3: “Each SBOM has a unique identifier” - why? I presume what’s meant is that there be a way in the SBOM to indicate exactly what version(s) of software it applies to. Otherwise, every time I regenerate an SBOM I would have to create a unique ID, which would make reproducible builds impossible (and that would be terrible).\r\nV2.4: “SBOM has been signed by publisher, supplier, or certifying authority.” - Absolutely not. An SBOM should be signed this way when its corresponding software is RELEASED, but the requirement doesn’t clearly say that. The same problem happens with many of the rest of the requirements, there’s a failure to indicate WHEN things need to happen with the SBOM.\r\n2.9 “SBOM contains a complete and accurate inventory of all components the SBOM describes” - proprietary vendors will not permit that; at best they’ll let you refer to direct dependencies, not indirect ones. This whole document needs to clarify direct vs. transitive dependencies, when are which required?\r\n\r\nIn chapter V3:\r\n\r\nV3.1: “Application uses a repeatable build” - I presume this simply means that if you repeat a build with unchanged inputs, you get the same (bit-for-bit) result, and that makes sense for level 1. That needs to be clarified, because that’s not the same as a reproducible build. However, at higher levels (say level 3) I would expect another additional criterion that specifically require reproducible builds (not just repeatable builds): “Application uses an independently-verified reproducible build” - that is, someone *else* can take the inputs and produce the same (bit-for-bit) result.\r\n\r\n3.1 - does this include ensuring that machine learning results are repeatable given the same data sets? Often training results are considered “data” - yet that data affects execution. It probably should include it.\r\n\r\n3.2 - “Documentation exists on how the application is built and instructions for repeating the build”. I understand you want to make automation optional, but this goes too far. If you have to follow instructions, instead of initiating a build command, you are basically guaranteeing disaster over time. People almost never read instructions, at best they copy & paste a command. Change this to something like: “Documentation exists on how the application is built and there are instructions on how to re-execute the automated system for (re)building the system”.\r\n\r\n3.4 “Application build pipeline prohibits alteration of build outside of the job performing the build” - this is very unclear. What is meant by this? This needs clarification. Same for most of the rest of this section.\r\n\r\n3.10 Application build pipeline enforces authentication and defaults to deny & 3.11 Application build pipeline enforces authorization and defaults to deny. - What is meant here? By what?\r\nMost of the requirements in 3 presume that the application build pipeline survives a build. Yet in many cases they’re in containers or temporary VMs, so these questions make no sense.\r\n\r\n3.15 “Application build pipeline has required maintenance cadence where the entire stack is updated, patched, and re-certified for use” - what is a “required maintenance cadence”? Why do I want one? How would I know when I have it? This is way too vague.\r\n\r\n3.17 “All build-time manipulations to source or binaries are known and well defined”. Clarify that this should include compiler optimizations from past executions (e.g., branch-probabilities and JIT warm-ups).\r\n\r\n3.18 “Checksums of all first-party and third-party components are documented for every build” - this should be recorded - not documented (nobody looks at the documentation) & automatically checked later. If this checking is not automated it will generally not happen. It says “checksums”; I think that should be “cryptographic hashes” at least, since a “checksum” need not be a cryptographic hash.\r\n\r\n3.19 - again, “checksum” should be “cryptographic hash”.\r\n\r\n3.21 - “Unused direct and transitive components have been removed from the application”. Agree for direct components, but for transitive components this needs more nuance. Often it’s hard to tell if something is “unused.” In addition, there are risks to removing components if it’s indirect that can cause other failures.\r\n\r\nIn chapter V4:\r\n\r\nThe term “package manager” is unclear. The glossary definition (“Package manager - A distribution mechanism that makes software artifacts discoverable by requesters.”) doesn’t really help. As defined, the world wide web and Google are a package manager.\r\n\r\n4.1 “Binary components are retrieved from a package repository” - I applaud the goal, but this is probably unrealistic unless “World Wide Web” counts as a package repository. If a proprietary vendor sells a binary component, they often won’t use traditional package manager interfaces; what is expected here? Not all OSS is in a package manager’s repo. Are you expecting developers to stop using these components? That seems impractical today; what are the options when that is not possible? What is the actual problem this is trying to solve, so that we can identify appropriate workarounds?\r\n\r\nV4.2: “Package repository contents are congruent to an authoritative point of origin for open source components” - what does “congruent” mean? Reword or define, this is unclear. I have no idea if this is good or not.\r\nV4.3: “Package repository requires strong authentication” - for what? To read? Probably not, most such package repositories allow anyone to read. I presume what’s meant is to modify (create, update, delete), but that’s not stated. Also: Many package repos won’t require this, and OWASP can’t make them. Instead, you could require that the packages YOU DOWNLOAD have strong authentication for modification; that’s far more practical.\r\n4.11 - “Package repository provides auditability when components are updated” - it sounds good, but what does “auditability” mean in this context?\r\n4.13/4.14 - what can projects do if their package manager doesn’t support these capabilities? I’m guessing that that they could augment their package manager with these functions and call the combination their “package manager” - yes? I don’t think all package managers support these functions, so you need to discuss how to handle these cases.\r\n4.16 “Package manager validates TLS certificate chain to repository and fails securely when validation fails” - many organizations use HTTPS (TLS) intercepting proxies (and re-sign with their own certs). I am not a fan of this approach, but it’s widespread, and if you forbid it this is a no-go. This requirement needs to be rewritten or clarified so that it can work in such settings, or acknowledge that a large number of organizations will not be able to use this specification. E.g., change “to repository” into “to repository or that organization’s authorized proxy”.\r\nV4.18 “Package manager does not execute code” - I presume what’s meant is that it doesn’t execute code merely when it downloads the code (it should say that instead). I applaud the sentiment, but I think that’s probably a non-starter today. I believe many package managers still can execute code when packages are downloaded. At the language repo level, at least JavaScript/NPM, Python, and Ruby support execution (Source: “Typosquatting programming language package managers” by Nikolai Tschacher, 2016, https://incolumitas.com/2016/06/08/typosquatting-package-managers/ ). The RPM package system (used by Red Hat Enterprise Linux, CentOS, Fedora, and SuSE) includes %pre and %post sections that execute scripts. OWASP won’t be able to change whether or not packages execute programs on installation for a long time, if ever, because of backwards compatibility issues. People will just ignore impractical advice like “don’t use the normal package manager for your situation” - and ignore OWASP if it tries to enforce this. Bedies, other requirements in this document require a package manager. OWASP could recommend a flag or something, or more pragmatically, require builds in a safe sandbox (such as a container or VM) that restricts the potential impact of executing code.\r\n\r\nV4: Should add “Anti-typosquatting measures are established when using public package repos by the project or repo” for level 3. Typosquatting is a big problem. Not all public package repos counter typosquatting; projects should have measures in place where the public repo does not.\r\n\r\nChapter V5:\r\n\r\nV5.1 “Component can be analyzed with linters and/or static analysis tools” - that’s pointless, anything CAN be. Do you mean that the source code is available, so that *source* code analysis can be used? Do you mean that the source code is not obfuscated?\r\n\r\n5.2 “Component is analyzed using linters and/or static analysis tools prior to use” and 5.3\r\n“Linting and/or static analysis is performed with every upgrade of a component” - why bother? I can run some tools & throw away the results. Unless you require someone to do something (e.g., analyze those results to determine if there’s an unusual/unacceptable level of risk), this is a complete waste of time.\r\n\r\n5.4: “An automated process of identifying all publicly disclosed vulnerabilities in third-party and open source components is used” - this just be “components” - first-party gets no free ride. This also seems to be a one-time thing; continuous monitoring is important too, as reports can happen later.\r\n\r\nI don’t see any requirement to *do* anything when a publicly disclosed vulnerability is found; without that, why bother? There should be a triaging so that less-important and unexploitable vulnerabilities are deferred (ideally you fix everything instantly, but that’s impractical & rushing everything may increase the likelihood of making even worse mistakes). However, important exploitable vulnerabilities caused by a component DO need to addressed rapidly.\r\n\r\nThis section doesn’t begin to seriously require security analysis. Fuzzing, assurance cases, red teams, secure requirements / design / implementation, training of developers, and so on. That’s fine if the real focus is supply chain analysis, as noted earlier.\r\n\r\nChapter V6:\r\n\r\nThe terms “pedigree” and “provenance” are not defined adequately enough so that anyone would agree on what counts and what doesn’t count.\r\n\r\n6.1 “Provenance of modified components is known and documented” - how far? E.g., I download a JavaScript package named X via NPM. Is that enough? After all, “it came from NPM”. If not, what IS enough?\r\n\r\n6.2 - “Pedigree of component modification is documented and verifiable”. If I record “Fred modified it” & sign it, is that enough? It appears that the answer is “yes”; if that wasn’t intended, then what was intended needs to be defined. The glossary definition of Pedigree is “Data which describes the lineage and/or process for which software has been created or altered.” - and that’s too vague to be useful.\r\n\r\nChapter “Guidance: Open Source Policy”\r\n\r\nAgain, this isn’t a taxonomy.\r\n\r\nSince this isn’t a requirement, should this be in the document at all? This should probably be in a separate document.\r\n\r\n“All organizations that use open source software should have an open source policy” - this is a joke, right? Who uses software & doesn’t use OSS? Even proprietary software practically always has OSS embedded within it.\r\n\r\nA policy that tries to declare “How many major or minor revisions old are acceptable” is probably impractical. There are too many variations in applications and components for that to make much sense.\r\n\r\n\r\nChapter Appendix A: Glossary\r\n\r\nThese definitions are too high-level. E.g., “Package manager” is “A distribution mechanism that makes software artifacts discoverable by requesters.” - so I guess the world wide web + Google is a package manager.\r\n\r\nChapter Appendix B: References:\r\nI’m surprised that NIST SP 800-161 (“Supply Chain Risk Management Practices for Federal Information Systems and Organizations”) wasn’t referenced. Have you examined it?\r\n\r\nI didn’t see the “Open Trusted Technology Provider (O-TTPS)” standard referenced, “Open Trusted Technology Provider™ Standard – Mitigating Maliciously Tainted and Counterfeit Products (O-TTPS)”. It was developed by the Open Group, and is now ISO/IEC 20243:2015. That focuses more on “best practices for global supply chain security and the integrity of commercial off-the-shelf (COTS) information and communication technology (ICT) products” - but it should probably be mentioned & reviewed.\r\n\r\nI suspect this should refer to OpenChain, and again, review it. https://wiki.linuxfoundation.org/_media/openchain/openchainspec-2.0.pdf\r\n\r\nConsider adding the CII Best Practices badge in the references, and reviewing it. See: https://github.com/coreinfrastructure/best-practices-badge/blob/master/doc/criteria.md and https://github.com/coreinfrastructure/best-practices-badge/blob/master/doc/other.md\r\n\r\nThese comments are my own, but I hope they help. Good luck!",
+ "summary": "The issue raises extensive concerns regarding the OWASP \"Software Component Verification Standard\" Version 1.0.0-RC.1. Key points include:\n\n1. **Vagueness of Requirements**: Many requirements are deemed too vague, making it difficult to determine how to meet them. A recommendation is made to rewrite the requirements to ensure clarity and prevent misinterpretation by parties with potentially malicious intentions.\n\n2. **Practicality**: There are doubts about the practicality of some requirements, especially for open source software projects. There is a suggestion to consider if these requirements can realistically be implemented.\n\n3. **Scope of Application**: The document may need to clarify whether it applies to entire organizations or specific projects, suggesting that focusing on individual projects may be more practical.\n\n4. **Threat Model and Justification**: The lack of a defined threat model raises concerns about whether the controls are adequate or necessary. Clear connections between requirements and potential threats should be established.\n\n5. **Misleading Title**: The title of the document is considered misleading, with a suggestion to rename it to accurately reflect its focus on software supply chain integrity rather than verification.\n\n6. **Specific Comments**: Numerous sections of the document are critiqued for lack of clarity, inadequate definitions, and impractical requirements, particularly regarding inventory, software bill of materials (SBOM), and package management. \n\nTo address these issues, a thorough review and revision of the requirements for clarity, practicality, and applicability are necessary. Additionally, the document's scope, purpose, and terminology should be clearly defined to ensure users can effectively implement its guidelines.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/Software-Component-Verification-Standard/issues/21",
@@ -17257,6 +17842,7 @@
"node_id": "MDU6SXNzdWU2NDU2MTg1MjY=",
"title": "Control Mapping",
"body": "Possible improvements to the spec would be to map SCVC controls to existing control documents including:\r\n* NIST 800-53\r\n* NIST 800-171\r\n* CMMC\r\n* OWASP ASVS\r\n* OWASP SAMM\r\n* BSIMM",
+ "summary": "The issue suggests that there are potential enhancements to the control mapping specifications by aligning SCVC controls with established control frameworks such as NIST 800-53, NIST 800-171, CMMC, OWASP ASVS, OWASP SAMM, and BSIMM. To address this, a review and mapping of SCVC controls to these existing documents should be conducted.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/Software-Component-Verification-Standard/issues/28",
@@ -17285,6 +17871,7 @@
"node_id": "MDU6SXNzdWU5Nzg5MjExNzM=",
"title": "lvl 2 and lvl 3 is impossible due to requiring both reproducability and non-reproducability of SBOMs",
"body": "`2.2 SBOM creation is automated and reproducible` means the SBOM must be reproducible, a good requirement for lvl2 and lvl3.\r\n`2.7 SBOM is timestamped` requires a timestamp for every level. \r\n\r\nTimestamps are the bane of reproducibility.\r\n\r\n> Timestamps make the biggest source of reproducibility issues. Many build tools record the current date and time. The filesystem does, and most archive formats will happily record modification times on top of their own timestamps. It is also customary to record the date of the build in the software itself…\r\n> \r\n> **Timestamps are best avoided**\r\n\r\nhttps://reproducible-builds.org/docs/timestamps/\r\n\r\nI can understand the desire for a timestamp but if it's included there needs to be details around the idea of `SOURCE_DATE_EPOCH` (a timestamp based on the last modification to any of the source or some other fixed timestamp). It needs to be clearly explained that this is allowed for the timestamp and is in fact required once you require reproducibility for lvl2+.\r\n\r\nhttps://reproducible-builds.org/docs/source-date-epoch/\r\n\r\nTangentially related to #9 request for more explanation",
+ "summary": "The issue discusses the conflicting requirements for achieving levels 2 and 3 in SBOM (Software Bill of Materials) creation, specifically addressing the challenges posed by the need for both reproducibility and non-reproducibility due to timestamp requirements. It highlights that while automated and reproducible SBOM creation is essential, timestamps can undermine reproducibility by introducing variability in builds. The discussion emphasizes the need for an alternative approach, such as using `SOURCE_DATE_EPOCH`, to maintain reproducibility while still incorporating timestamps. Additional clarity around this alternative is suggested, as well as a connection to another related issue. \n\nTo address this, a proposal could be made to clarify the use of `SOURCE_DATE_EPOCH` in the guidelines for SBOM timestamping.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/Software-Component-Verification-Standard/issues/31",
@@ -17311,6 +17898,7 @@
"node_id": "I_kwDODDeA9s5N5KKD",
"title": "Translate to Japanese",
"body": "I want to translate this standards to Japanese. \r\nHow can I contribute ?\r\n\r\nI just translate markdowns in \"en/\" directories?\r\n\r\nhttps://github.com/OWASP/Software-Component-Verification-Standard/tree/master/en",
+ "summary": "The issue discusses the desire to translate standards into Japanese and seeks guidance on how to contribute. It questions whether the translation should focus solely on markdown files located in the \"en/\" directories. To assist, it may be helpful to clarify the contribution process and confirm the specific files that need translation.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/Software-Component-Verification-Standard/issues/33",
@@ -17337,6 +17925,7 @@
"node_id": "I_kwDODDeA9s5OTspK",
"title": "Create BOM Maturity Model Taxonomy",
"body": "The mindmap of the taxonomy currently in development is located:\r\nhttps://drive.google.com/file/d/1Uot5Ntm0NB3kJgHAc7fDtZTleJIhZS2P/view?usp=sharing\r\n\r\nUse [XMind](https://www.xmind.net/) to view.\r\n\r\nA preview of the taxonomy is here (may not always be update to date):\r\nhttps://drive.google.com/file/d/1_GIylG4K3mT_TPeGJlIUj4HtouNRgtPQ/view?usp=sharing\r\n\r\n\r\n",
+ "summary": "The issue discusses the creation of a BOM (Bill of Materials) Maturity Model Taxonomy. A mind map for the taxonomy is currently under development and can be accessed via a Google Drive link. Users are advised to use XMind to view the mind map. Additionally, there is a preview link provided that may not always reflect the latest updates. It seems necessary to further develop or refine the taxonomy based on the existing resources.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/Software-Component-Verification-Standard/issues/34",
@@ -17367,6 +17956,7 @@
"node_id": "I_kwDODDeA9s5OTs4-",
"title": "Develop BOM Maturity Model - maturity levels and assignments",
"body": "Depends on: #34",
+ "summary": "The issue discusses the need to develop a Bill of Materials (BOM) Maturity Model, which includes defining various maturity levels and their corresponding assignments. It is dependent on another issue, identified as #34. To address this, one should first review the details of issue #34 and then proceed to outline the maturity levels for the BOM model.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/Software-Component-Verification-Standard/issues/35",
@@ -17397,6 +17987,7 @@
"node_id": "I_kwDODDeA9s5OTtAa",
"title": "Create BOM Maturity Model in JSON",
"body": "Depends on: #34 and #35",
+ "summary": "The issue requests the creation of a Bill of Materials (BOM) Maturity Model in JSON format. It also indicates that this task is dependent on the completion of issues #34 and #35. Therefore, it would be necessary to address those dependencies before proceeding with the BOM Maturity Model.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/Software-Component-Verification-Standard/issues/36",
@@ -17427,6 +18018,7 @@
"node_id": "I_kwDODDeA9s5W7auq",
"title": "PDF links",
"body": "Re: https://github.com/OWASP/Software-Component-Verification-Standard/releases\r\n\r\nIn the PDF, all the links in Appendix B: References do not appear to be hyperlinks.\r\n",
+ "summary": "The issue reports that the links in Appendix B: References of the PDF are not functioning as hyperlinks. To resolve this, the links should be made clickable in the PDF document.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/Software-Component-Verification-Standard/issues/37",
@@ -17451,10 +18043,11 @@
"pk": 609,
"fields": {
"nest_created_at": "2024-09-11T20:45:03.351Z",
- "nest_updated_at": "2024-09-12T02:41:10.855Z",
+ "nest_updated_at": "2024-09-13T16:25:21.266Z",
"node_id": "I_kwDODDeA9s5nCeMu",
"title": "Translate standard documentation into Vietnamese language",
"body": "Software Supply Chain Security become a critical approach for many security programs. Vietnam is also a country that adopts new standards, and processes to enhance their chance in Software Supply Chain. Having a Vietnamese version of the standard may help easier to understand to onboard the standard for those users who are not security professional",
+ "summary": "The issue discusses the need for translating standard documentation related to Software Supply Chain Security into Vietnamese. This translation is essential for enhancing understanding among users in Vietnam who may not be security professionals. To address this, the translation work should be initiated to make the standards more accessible.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/Software-Component-Verification-Standard/issues/38",
@@ -17481,6 +18074,7 @@
"node_id": "MDU6SXNzdWU0NzE0MDIyMDM=",
"title": "Mobile Operating System Threats",
"body": "Compile a list of all Mobile Operating System Threats",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/Mobile-Threatmodel/issues/1",
@@ -17510,6 +18104,7 @@
"node_id": "MDU6SXNzdWU0NzE0MDk0ODU=",
"title": "Threat Template",
"body": "Template to be used for writing up threats ",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/Mobile-Threatmodel/issues/2",
@@ -17534,10 +18129,11 @@
"pk": 612,
"fields": {
"nest_created_at": "2024-09-11T20:45:49.447Z",
- "nest_updated_at": "2024-09-11T20:45:49.447Z",
+ "nest_updated_at": "2024-09-13T16:09:17.627Z",
"node_id": "MDU6SXNzdWU0NzM2MjAzMzE=",
"title": "Complete GitHub Checklist ",
"body": "",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/Mobile-Threatmodel/issues/4",
@@ -17566,6 +18162,7 @@
"node_id": "MDU6SXNzdWU1OTAzOTg5Mzg=",
"title": "Copper: Webhook from Stripe to update Membership info on People",
"body": "Utilize copper api for updating People with current membership information",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-staff/issues/9",
@@ -17594,6 +18191,7 @@
"node_id": "MDU6SXNzdWU1OTQ0Njg2MjM=",
"title": "Discussion on Topic x",
"body": "asfasfdj;sdlfkads fasd;fljsd;fjasj",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-staff/issues/10",
@@ -17620,6 +18218,7 @@
"node_id": "MDU6SXNzdWU3MDc1MDY1OTc=",
"title": "Regional Event in a box",
"body": "Created by Andrew van der Stock via monday.com integration. 🎉",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-staff/issues/11",
@@ -17646,6 +18245,7 @@
"node_id": "MDU6SXNzdWU3MDc1MDY3MDQ=",
"title": "Trainer splits",
"body": "Created by Andrew van der Stock via monday.com integration. 🎉",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-staff/issues/12",
@@ -17672,6 +18272,7 @@
"node_id": "MDU6SXNzdWU3MDc1MDY3OTE=",
"title": "Hack-a-thon",
"body": "Created by Andrew van der Stock via monday.com integration. 🎉",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-staff/issues/13",
@@ -17694,10 +18295,11 @@
"pk": 618,
"fields": {
"nest_created_at": "2024-09-11T20:45:59.179Z",
- "nest_updated_at": "2024-09-11T20:45:59.179Z",
+ "nest_updated_at": "2024-09-13T16:09:21.728Z",
"node_id": "I_kwDOC7ie5M5ae9Ca",
"title": "Missing README.md",
"body": "hello there this branch is missing Readme.md file.",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-staff/issues/15",
@@ -17724,6 +18326,7 @@
"node_id": "I_kwDOC7ie5M5a7HXp",
"title": "Marking of pages",
"body": "Please mark (or tag, or make it in another way visible) staff pages as only applicable to staff. \r\n\r\nE.g. the \"Getting a Current Member List\" page, refers to Copper CRM. Logging on as an \"ordinary\" member does not bring me to the OWASP CRM, but one that I create by joining.",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www-staff/issues/16",
@@ -17750,6 +18353,7 @@
"node_id": "MDU6SXNzdWU1MjU5MDk3MzY=",
"title": "An adequate Replacement for the Central Mediawiki File Management is needed",
"body": "In my opinion we do need a good replacement for the mediawiki file management.\r\nThere is a need to provide OWASP-wide File management to avoid locally managed duplicates and a mass of unknown versions from the same document.\r\nLinks to internal OWASP-documents should look dirrerent from external links. \r\nPlease deploy a solution and give us guidelines how to use it.\r\n\r\nI am sorry to say, but in my opinion this is a critical factor for any migration.\r\nCheers\r\nTorsten",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/owasp.github.io/issues/21",
@@ -17780,6 +18384,7 @@
"node_id": "I_kwDOC5rsi85eRwcl",
"title": "Other languages",
"body": "The page is only in English, but, shouldn't be a version in Spanish or other languages?\n\nThis could make the project more visible to the world and be able to reach more people.",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/owasp.github.io/issues/213",
@@ -17806,6 +18411,7 @@
"node_id": "I_kwDOC5rsi85kf_Pm",
"title": "There are some issues on starting the owasp virtual machine",
"body": "# There are some issues on starting the owasp virtual machine with the lastest version of virtualbox, and I didn`t get the solution of it!\r\n# version of virtualbox: 7.0.6\r\n# version of owasp: 1.2\r\n# Could you please give me some of the solutions on starting up the virtual machine? Thanks a lot! ",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/owasp.github.io/issues/235",
@@ -17832,6 +18438,7 @@
"node_id": "I_kwDOC5rsi853m96u",
"title": "ddf",
"body": "vpn",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/owasp.github.io/issues/288",
@@ -17858,6 +18465,7 @@
"node_id": "I_kwDOC5rsi858uHNU",
"title": "OWASP",
"body": "",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/owasp.github.io/issues/296",
@@ -17884,6 +18492,7 @@
"node_id": "I_kwDOC5rsi858uzh1",
"title": "verification of employment agreement ",
"body": "HTTP/1.1 200 OK\n\nAccept-Ranges: bytes\n\nCache-Control: max-age=0, private, must-revalidate\n\nContent-Security-Policy: default-src 'none'; base-uri 'self'; child-src github.com/assets-cdn/worker/ gist.github.com/assets-cdn/worker/; connect-src 'self' uploads.github.com www.githubstatus.com collector.github.com raw.githubusercontent.com api.github.com github-cloud.s3.amazonaws.com github-production-repository-file-5c1aeb.s3.amazonaws.com github-production-upload-manifest-file-7fdce7.s3.amazonaws.com github-production-user-asset-6210df.s3.amazonaws.com cdn.optimizely.com logx.optimizely.com/v1/events api.githubcopilot.com objects-origin.githubusercontent.com *.actions.githubusercontent.com wss://*.actions.githubusercontent.com productionresultssa0.blob.core.windows.net/ productionresultssa1.blob.core.windows.net/ productionresultssa2.blob.core.windows.net/ productionresultssa3.blob.core.windows.net/ productionresultssa4.blob.core.windows.net/ productionresultssa5.blob.core.windows.net/ productionresultssa6.blob.core.windows.net/ productionresultssa7.blob.core.windows.net/ productionresultssa8.blob.core.windows.net/ productionresultssa9.blob.core.windows.net/ github-production-repository-image-32fea6.s3.amazonaws.com github-production-release-asset-2e65be.s3.amazonaws.com insights.github.com wss://alive.github.com; font-src github.githubassets.com; form-action 'self' github.com gist.github.com objects-origin.githubusercontent.com; frame-ancestors 'none'; frame-src viewscreen.githubusercontent.com notebooks.githubusercontent.com octocaptcha.com; img-src 'self' data: github.githubassets.com media.githubusercontent.com camo.githubusercontent.com identicons.github.com avatars.githubusercontent.com github-cloud.s3.amazonaws.com objects.githubusercontent.com secured-user-images.githubusercontent.com/ user-images.githubusercontent.com/ private-user-images.githubusercontent.com opengraph.githubassets.com github-production-user-asset-6210df.s3.amazonaws.com customer-stories-feed.github.com spotlights-feed.github.com objects-origin.githubusercontent.com *.githubusercontent.com; manifest-src 'self'; media-src github.com user-images.githubusercontent.com/ secured-user-images.githubusercontent.com/ private-user-images.githubusercontent.com github-production-user-asset-6210df.s3.amazonaws.com gist.github.com; script-src github.githubassets.com; style-src 'unsafe-inline' github.githubassets.com; upgrade-insecure-requests; worker-src github.com/assets-cdn/worker/ gist.github.com/assets-cdn/worker/\n\nContent-Type: text/html; charset=utf-8\n\nDate: Sun, 21 Jan 2024 15:52:06 GMT\n\nETag: W/\"f8454f92b2a4aa386c6c70ba970e7239\"\n\nReferrer-Policy: origin-when-cross-origin, strict-origin-when-cross-origin\n\nServer: GitHub.com\n\nSet-Cookie: _gh_sess=e7U%2FTQ5KxEerNJgmTJq8Fx9TKw6uyZM4Wwzgj9tJ%2FTwmwZGU7cM3oG4Xldq5C6WsVfmo6Odyl%2FrvYLrSGtUKInxbEh87UnXTLFLeOcpQtOGU5AARG3RVAbSEHSsyQU3aeB3C8jP3oUNKr03XaKhJ%2Bl8btrzDq3ZykrWKCRAipsgZZYQB3DVEoXFCVql2FBV5Qo9JRxKB%2FFcrOSsmGtXxa0JfP%2FIDfyM9di0ah3kvLaxfjgACyc1zZbpM0z3C%2FLk98u3EgkIzYLmToA%2B6DU%2BB%2Fg%3D%3D--Px7IQLSsZR2icfgh--IG6YyYrXh9jRUkBgWWKcqQ%3D%3D; Path=/; HttpOnly; Secure; SameSite=Lax\n\nSet-Cookie: _octo=GH1.1.1517904614.1705852326; Path=/; Domain=github.com; Expires=Tue, 21 Jan 2025 15:52:06 GMT; Secure; SameSite=Lax\n\nSet-Cookie: logged_in=no; Path=/; Domain=github.com; Expires=Tue, 21 Jan 2025 15:52:06 GMT; HttpOnly; Secure; SameSite=Lax\n\nStrict-Transport-Security: max-age=31536000; includeSubdomains; preload\n\nTransfer-Encoding: chunked\n\nVary: X-PJAX, X-PJAX-Container, Turbo-Visit, Turbo-Frame, Accept-Encoding, Accept, X-Requested-With\n\nX-Content-Type-Options: nosniff\n\nX-Frame-Options: deny\n\nX-GitHub-Request-Id: 7F29:7B30:1652281:1E8980E:65AD3DA6\n\nX-XSS-Protection: 0\n\n\n \n\n\n\n\n\n\n\n\n\n\n\n\n \n \n \n \n \n \n \n \n\n \n\n \n \n \n \n \n \n\n \n\n \n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n\n \n\n Forgot your password? · GitHub\n\n\n\n \n\n \n \n\n\n \n\n\n\n\n \n \n\n \n \n\n \n \n \n \n \n\n\n\n \n\n \n\n\n\n\n \n\n \n\n \n\n \n \n \n \n \n \n \n \n \n \n \n \n \n \n \n \n \n \n \n \n \n \n \n\n \n \n \n \n \n \n \n \n \n \n\n\n\n \n\n\n\n \n\n\n \n \n \n \n\n \n\n \n\n\n\n \n\n\n \n\n \n\n \n\n \n \n \n\n\n\n\n\n \n\n \n\n \n
\n \n \n You signed in with another tab or window. Reload to refresh your session.\n You signed out in another tab or window. Reload to refresh your session.\n You switched accounts on another tab or window. Reload to refresh your session.\n\n Dismiss alert\n\n\n \n
\n
\n\n \n\n\n\n\n\n\n\n\n\n \n \n\n\n\n\n\n\n
\n \n \n
\n
\n\n \n
\n\n \n\n\n \n\n\n
\n \n \n You can’t perform that action at this time.\n
\n\n \n \n \n \n \n \n \n \n\n\n
\n
\n
\n
\n\n \n
\n \n \n \n \n
\n\n\n
\n \n \n \n \n
\n\n\n\n\n\n
\n\n \n \n \n",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/owasp.github.io/issues/297",
@@ -17910,6 +18519,7 @@
"node_id": "I_kwDOC5rsi858vun1",
"title": "error message not found ",
"body": "https://github.com/microsoft/vscode/issues/202966",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/owasp.github.io/issues/298",
@@ -17936,6 +18546,7 @@
"node_id": "I_kwDOC5rsi86LHHRP",
"title": "The number of URLs is increasing for 'CSP: script-src unsafe-inline' and 'CSP: style-src unsafe-inline' after fixing 'CSP: Wildcard Directive'",
"body": "Hello.\r\n1) I has the next report:\r\n\r\nThe value of CSP was \r\n**\"default-src 'self'; script-src 'self' cdn.jsdelivr.net 'unsafe-inline'; img-src 'self' validator.swagger.io bootswatch.com getbootstrap.com data:; style-src 'self' cdn.jsdelivr.net 'unsafe-inline'; font-src 'self' cdn.jsdelivr.net data:; connect-src 'self' bootswatch.com;\"**\r\n2) I fixed CSP: Wildcard Directive by adding **form-action 'self'; frame-ancestors 'self'** and received the next report:\r\n\r\n3) My question is why the number of URLs in **CSP: script-src unsafe-inline** and **CSP: style-src unsafe-inline** was increased? ",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/owasp.github.io/issues/313",
@@ -17958,10 +18569,11 @@
"pk": 628,
"fields": {
"nest_created_at": "2024-09-11T20:46:12.507Z",
- "nest_updated_at": "2024-09-11T20:46:12.507Z",
+ "nest_updated_at": "2024-09-13T04:18:07.591Z",
"node_id": "I_kwDOC5rsi86LdgsJ",
"title": "@yaseenaljamal",
"body": "https://www.apache.org/licenses/LICENSE-2.0.txt",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/owasp.github.io/issues/317",
@@ -17988,6 +18600,7 @@
"node_id": "MDU6SXNzdWU1MzgwNTk3MzA=",
"title": "Subdirs",
"body": "\r\n.. are not working or am I missing something?\r\n\r\nFor our German Chapter we have links like https://www.owasp.org/index.php/OWASP_German_Chapter_Stammtisch_Initiative/Hamburg and https://www.owasp.org/index.php/Germany/Chapter_Meetings (cc @bkimminich).\r\n\r\nThx, Dirk\r\n\r\n\r\n\r\n\r\n",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www--site-theme/issues/32",
@@ -18014,6 +18627,7 @@
"node_id": "MDU6SXNzdWU1NzAwMjE0NTE=",
"title": "Twitter cards social preview content needed",
"body": "It looks like the site inserts OpenGraph metatags (for Facebook etc), however it doesn't insert the tags necessary for Twitter which is used quite extensively as a sharing platform in the industry. It would be nice if the necessary tags for that were populated as well.\r\n\r\nRefs:\r\n- https://developer.twitter.com/en/docs/tweets/optimize-with-cards/overview/abouts-cards\r\n- https://brianbunke.com/blog/2017/09/06/twitter-cards-on-jekyll/\r\n\r\nEdit: It seems twitter will fall back to OpenGraph.",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www--site-theme/issues/52",
@@ -18040,6 +18654,7 @@
"node_id": "MDU6SXNzdWU2NTI5OTczNDU=",
"title": "Cookie banner not compliant with EU law",
"body": "I'm not a lawyer, but I think we might be making fools of ourselves with this cookie banner (see screenshot) that doesn't even meet current EU legislation demanding an \"opt in\" to all tracking and non-essential cookies and not accepting plain \"Accept\"-banners any longer...\n\n https://edpb.europa.eu/sites/edpb/files/files/file1/edpb_guidelines_202005_consent_en.pdf\n\n\n\nI ran an automated conformity test, and the `_ga` and `_gid` cookies (Google Analytics) need to be locked until explicitly accepted by the user in an opt-in fashion. The website I used marked the other cookies from CloudFlare and Stripe as essential and therefore compliant.\n\nReport can be found in the corresponding Slack discussion: https://owasp.slack.com/files/U1S23SNE7/F016556FB61/report-owasporg-4183554.pdf\n\n_Sent from my Pixel 3 XL using [FastHub](https://play.google.com/store/apps/details?id=com.fastaccess.github)_",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www--site-theme/issues/65",
@@ -18066,6 +18681,7 @@
"node_id": "MDU6SXNzdWU4NDIxNjY5NTk=",
"title": "OWASP/www-chapter-colombo: Page build failure",
"body": "After updating Colombo chapter page repo [1], I noticed there was a page build failure. \r\nI highly appreciate if you can help me to fix this.\r\n\r\n`The page build failed for the `master` branch with the following error:\r\n\r\nA file was included in `/_layouts/col-sidebar.html` that is a symlink or does not exist in your `_includes` directory. For more information, see https://docs.github.com/github/working-with-github-pages/troubleshooting-jekyll-build-errors-for-github-pages-sites#file-is-a-symlink.\r\n\r\nFor information on troubleshooting Jekyll see:\r\n\r\n https://docs.github.com/articles/troubleshooting-jekyll-builds\r\n\r\nIf you have any questions you can submit a request at https://support.github.com/contact?repo_id=221790172&page_build_id=242477598\r\n\r\n`\r\n[1] https://github.com/OWASP/www-chapter-colombo\r\n",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www--site-theme/issues/79",
@@ -18088,10 +18704,11 @@
"pk": 633,
"fields": {
"nest_created_at": "2024-09-11T20:46:20.590Z",
- "nest_updated_at": "2024-09-11T20:46:20.590Z",
+ "nest_updated_at": "2024-09-13T16:09:29.696Z",
"node_id": "I_kwDOC5rfps6FvH03",
"title": "Improve the OWASP UI",
"body": "The overall UI for the entire OWASP site looks and feels inconsistent, which can cause new visitors to quickly lose their trust in OWASP itself, which doesn't give a good impression, especially for an organisation that focuses on cybersecurity.\r\n\r\nI'm aware that OWASP use a customised theme for the site, but I believe that they can benefit from having their website redesigned from the ground up, so that it looks more consistent, trustworthy, and professional, not just for newcomers, but also for returning visitors and paying members.\r\n\r\nFurther, isn't one of OWASP's Top 10 items Insecure Design?\r\n\r\nNot only does that relate to how cybersecurity solutions themselves are designed (so that they can be secure), but also how those solutions (specifically user interfaces on an app, websites, etc) look visually, since in this case, you pretty much have to judge a book by its cover to determine whether it is secure and trustworthy by design (both in terms of security and aesthetics), since poorly designed websites and badly formatted emails can be viewed as a cybersecurity threat, and in most cases, that's how they're often perceived.\r\n\r\nSource: I am currently a UI Designer for a cybersecurity startup, so improving the UI for OWASP is something that I am fully able to do.",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/www--site-theme/issues/113",
@@ -18114,10 +18731,11 @@
"pk": 634,
"fields": {
"nest_created_at": "2024-09-11T21:07:22.730Z",
- "nest_updated_at": "2024-09-11T21:07:22.730Z",
+ "nest_updated_at": "2024-09-13T16:09:36.725Z",
"node_id": "I_kwDOC5InlM5bC3YM",
"title": "Head and Body tag is missing in 'index.html' file.",
"body": "Head and Body tag is missing in 'index.html' file. This may cause problem in live reloading as well in SEO.",
+ "summary": "",
"state": "open",
"state_reason": "reopened",
"url": "https://github.com/OWASP/video-portal/issues/7",
@@ -18144,6 +18762,7 @@
"node_id": "MDU6SXNzdWU0OTQ0OTQ3MDg=",
"title": "The Output of the script is showing jumbled characters",
"body": "Hello team, \r\n\r\n Nice work with this static tool. I love it. \r\n\r\nThis issue might not be of code and more of my linux (ubuntu) setup. Please find attached image showing this. \r\n\r\nRequest your inputs on how to make it look like a neat table like in your screenshot..\r\n\r\n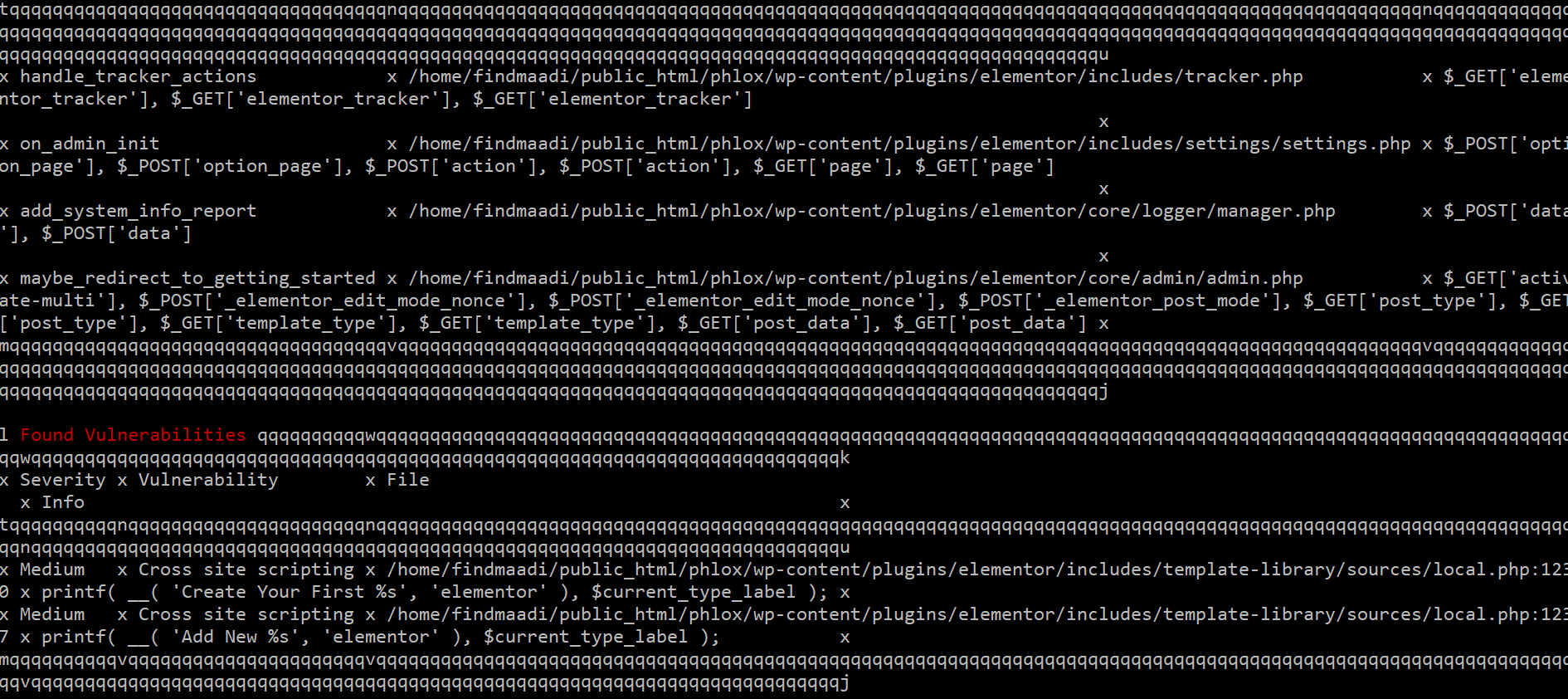\r\n",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/wpBullet/issues/1",
@@ -18166,10 +18785,11 @@
"pk": 636,
"fields": {
"nest_created_at": "2024-09-11T21:07:32.198Z",
- "nest_updated_at": "2024-09-11T21:07:32.198Z",
+ "nest_updated_at": "2024-09-13T16:09:43.429Z",
"node_id": "MDU6SXNzdWU0OTQ0OTY4NzE=",
"title": "Installation of PIP doesn't work",
"body": "Hi team, \r\n\r\n This might be obvious to few people, but i wanted to put it out here anyway for future reference. \r\n\r\nI tried installing pip-python, but it installs an older version by default and doesn't install the requirements as well. \r\n\r\nWhat worked is when i installed pip3/python3 as follows in UBUNTU:\r\n\r\n apt install python3\r\n apt install python3-pip\r\n pip3 install -r requirements.txt\r\n python3 wpbullet.py -path=\"\"",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/wpBullet/issues/2",
@@ -18192,10 +18812,11 @@
"pk": 637,
"fields": {
"nest_created_at": "2024-09-11T21:08:24.538Z",
- "nest_updated_at": "2024-09-11T21:08:24.538Z",
+ "nest_updated_at": "2024-09-13T04:19:07.338Z",
"node_id": "MDU6SXNzdWU0NTIxNjQ3MTA=",
"title": "CheatSheetSeries.Java.pdf not clear about the fact it's java",
"body": "The list of cheatsheets at the bottom, are not presented as Java related. All we have is a Java logo on the top left, and that's it. We should say how to do it.\r\n",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/community-docs/issues/1",
@@ -18224,6 +18845,7 @@
"node_id": "MDU6SXNzdWU0MzE2NDAwNjg=",
"title": "Better define MFA support",
"body": "Take NPM as an example - there is an option to configure MFA, and you can enforce it per package (see the [docs](https://docs.npmjs.com/about-two-factor-authentication)). But once MFA is enabled, you cannot use a CI to publish your packages - publish is done automatically. I think this should be reflected somehow, WDYT?",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/packman/issues/2",
@@ -18250,6 +18872,7 @@
"node_id": "MDU6SXNzdWU0MzY0MTE1NTg=",
"title": "Add WAPM",
"body": "Web Assembly Package Manager was announced today, seems like a valid modern package manager to add to the list.\r\n\r\nhttps://medium.com/wasmer/announcing-wapm-the-webassembly-package-manager-18d52fae0eea",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/packman/issues/12",
@@ -18276,6 +18899,7 @@
"node_id": "MDU6SXNzdWU0MzkwMzkwNjg=",
"title": "Review proposed Golang ecosystem changes",
"body": "https://go.googlesource.com/proposal/+/master/design/25530-sumdb.md",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/packman/issues/13",
@@ -18304,6 +18928,7 @@
"node_id": "MDU6SXNzdWU1NjQ2NzY3OTk=",
"title": "Clarification of support levels for items in Tiers",
"body": "Can we add a description of values and their definitions for security criteria items so that it is better understood what each mean. For example, I found the following for [npm.md](./npm.md) unclear:\r\n* Strong authentication: Partial - what does it mean?\r\n* Update notifications - Partials - means what exactly? Is it just the single maintainer who published but not all others who are listed as maintainers or the team that manages it?\r\n* Package Manager Does Not Run Code - Optional - If it is optional, how does this score? is it a +1 for flagging as passing the criteria or not?\r\n\r\n",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/packman/issues/18",
@@ -18330,6 +18955,7 @@
"node_id": "MDU6SXNzdWU1NjQ3MjExNjc=",
"title": "Suggestion: add new criteria for Package Manager FS operations",
"body": "I would like to add a new criteria for package managers which revolves around other operations that they do. We've nailed down running code with `Package Manager Does Not Run Code` and doing call-home stuff with `Package Manager Does Not Collect Info` but package managers also do filesystem operations, such as linking and so I want to add a new item `Package Managers Does FS Linking` (or can think of a better name if you have suggestions).\r\n\r\nThis is based on the recent security vulnerabilities that impacted all three popular JS package managers (npm, yarn and pnpm) due to their filesystem operations when packages with executables defined are installed. Full story for reference: https://snyk.io/blog/understanding-filesystem-takeover-vulnerabilities-in-npm-javascript-package-manager/",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/packman/issues/19",
@@ -18356,6 +18982,7 @@
"node_id": "MDU6SXNzdWU1NjQ3MjM0ODA=",
"title": "Suggestion: adding lockfile and security related to it",
"body": "Due to recent research I published with regards to [lockfile security](https://snyk.io/blog/why-npm-lockfiles-can-be-a-security-blindspot-for-injecting-malicious-modules/) - I'd be happy if we also cover lockfiles as a general use-case, but also the traits of lockfiles, such as whether they are tracked for direct or all dependencies, do they include signatures or checksums and so on.",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/packman/issues/20",
@@ -18382,6 +19009,7 @@
"node_id": "MDU6SXNzdWU1NzAyNTE5OTk=",
"title": "Operating System package Managers",
"body": "I think OS packager managers are a major blind spot and I have done extensive research on many of them and their various ranking in this system.\r\n\r\nWould there be interest in me contributing my research on the security of brew, pacman, apt, yum, etc?",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/packman/issues/21",
@@ -18408,6 +19036,7 @@
"node_id": "MDU6SXNzdWU2MDE5ODg0MTY=",
"title": "Update Python for recent and upcoming PyPI changes",
"body": "Is this effort still active? I can't see much mention of it or activity around it apart from this repository and the original blog post announcement.\r\n\r\n[PyPI Now Supports Two-Factor Login via WebAuthn](http://pyfound.blogspot.com/2019/06/pypi-now-supports-two-factor-login-via.html) and [API tokens for uploads](http://pyfound.blogspot.com/2020/01/start-using-2fa-and-api-tokens-on-pypi.html), I'd be happy to write up a Packman page for Python/PyPI.\r\n",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/packman/issues/22",
@@ -18434,6 +19063,7 @@
"node_id": "MDU6SXNzdWU4MTkxMzcxODE=",
"title": "Corrections / Clarifications about Swift Package Manager",
"body": "Thank you for putting together this resource. For the past year, I've been working on a proposal to add a [registry to Swift Package Manager](https://github.com/apple/swift-evolution/blob/main/proposals/0292-package-registry-service.md), and I found the information in this project helpful as I considered how that should work.\r\n\r\nI'm happy to report that the new registry interface promises to substantially improve the security and reliability story for Swift Package Manager (both immediately, and in follow-up proposals building on top of the original). \r\n\r\nIn the meantime, I wanted to contribute to this project by offering corrections or clarifications to some details in the current report:\r\n\r\n* * *\r\n\r\n> ### Security Contacts and Process\r\n>\r\n> To satisfy this requirement, the package manager must have a way to receive security information from the community and a process for handling such feedback. A published email such as security@, together with a mechanism to ensure that the feedback is captured and responded to would satisfy this requirement.\r\n\r\nThe majority of Swift packages (>99.9%) are hosted on GitHub. Some packages define a security policy on GitHub (for example, see [@Flight-School/Money](https://github.com/Flight-School/Money/security/policy)). \r\n\r\nThis is currently listed as **No**. If we follow the same criteria as we do for MFA, should this be updated to **Optional** with a comment? \r\n\r\n* * *\r\n\r\n> ### Code Signing\r\n>\r\n> It should be possible for developers to sign their code. When they do, the package manager should verify the signatures and provide a way for those to be distributed to consumers of the package.\r\n\r\n\r\nSwift Package Manager has built-in support for [code-signed binary artifacts](https://github.com/apple/swift-evolution/blob/master/proposals/0272-swiftpm-binary-dependencies.md#binary-signatures). \r\n\r\nThis is currently listed as **No**. Should this be updated to **Partial** with a comment?\r\n\r\n* * *\r\n\r\n> ### Package Manager Does Not Run Code\r\n>\r\n> The package manager should not run code on package install.\r\n\r\nIn fact, Swift Package Manager _does_ evaluate package manifests. On macOS, it's run through a sandboxed process (`sandbox-exec`) to mitigate the effects of arbitrary code execution (see https://github.com/apple/swift-package-manager/blob/4bef41f12f082d55a4cb979a489379bfcf69f0c7/Sources/PackageLoading/ManifestLoader.swift#L776). \r\n\r\nThis is currently listed as **Yes** (that is, it doesn't run code). Should this entry be updated to **No** or something else?\r\n\r\n* * *\r\n\r\n> ### Integrity verification\r\n> \r\n> Package manager provides a method for verifying the integrity of the downloaded package.\r\n>\r\n> None - no integrity verification is done Partial - integrity verification is done using a weak method* Yes - Verification is done using a sufficiently secure method\r\n\r\nIntegrity verification is offered for [binary artifacts](https://github.com/apple/swift-evolution/blob/master/proposals/0272-swiftpm-binary-dependencies.md#checksum-computation), using SHA256. Conventional, source-based dependencies don't currently offer any integrity verification functionality. \r\n\r\nThis isn't currently listed for Swift Package Manager. Should this entry be set to **No**, **Partial**, or something else?",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/packman/issues/23",
@@ -18456,10 +19086,11 @@
"pk": 647,
"fields": {
"nest_created_at": "2024-09-11T21:08:37.437Z",
- "nest_updated_at": "2024-09-11T21:08:37.437Z",
+ "nest_updated_at": "2024-09-13T16:10:29.385Z",
"node_id": "I_kwDOCsQxbc5VqGws",
"title": "Track if a published version can be deleted",
"body": "Very useful project. Thank you.\r\n\r\nThere was a twitter thread a few months ago, around the time of [this incident](https://twitter.com/balloob/status/1545510244381446144).\r\n\r\n\"which package ecosystems protect downstream libs/apps from a published version of an upstream lib being deleted\"\r\nhttps://twitter.com/mrinal/status/1546250871784108033\r\n\r\nThe thread captured, state of this for:\r\n\r\n- Rust https://twitter.com/mrinal/status/1546250872711024641\r\n- JS https://twitter.com/mrinal/status/1546250873851875329\r\n- Go https://twitter.com/mrinal/status/1546250874908921858\r\n- Erlang/Elixir https://twitter.com/mrinal/status/1546250875961675776\r\n- Java / Scala/ other JVM https://twitter.com/mrinal/status/1546250876997685249\r\n- Ruby https://twitter.com/mrinal/status/1546250878008537089\r\n- Swift https://twitter.com/mrinal/status/1546250878977380353\r\n- Python https://twitter.com/mrinal/status/1546250879853928448\r\n- Github Packages https://twitter.com/mrinal/status/1546265142580547584\r\n- Docker Hub https://twitter.com/mrinal/status/1546266861989351424\r\n\r\nI [wished at the time](https://twitter.com/mrinal/status/1546270715334180866) for something like packman and today someone pointed me packman :)\r\n\r\nIf there is interest in tracking this aspect, I'd be happy to send pull request.\r\n",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/packman/issues/24",
@@ -18482,10 +19113,11 @@
"pk": 648,
"fields": {
"nest_created_at": "2024-09-11T21:08:53.173Z",
- "nest_updated_at": "2024-09-11T21:08:53.173Z",
+ "nest_updated_at": "2024-09-13T04:19:24.722Z",
"node_id": "MDU6SXNzdWU1NzQyMjQ5MTI=",
"title": "Make hook feature for report",
"body": "Dot env for send data:\r\n`hook=\"URL$text\"`",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/D4N155/issues/11",
@@ -18514,6 +19146,7 @@
"node_id": "MDU6SXNzdWU2OTE2MTQ1MzM=",
"title": "Não está salvando a lista de textos estaticos apartir da forma interativa",
"body": "Bct q ódio, resolver esse B.O né",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/D4N155/issues/17",
@@ -18536,10 +19169,11 @@
"pk": 650,
"fields": {
"nest_created_at": "2024-09-11T21:09:12.077Z",
- "nest_updated_at": "2024-09-11T21:09:12.077Z",
+ "nest_updated_at": "2024-09-13T16:10:49.058Z",
"node_id": "MDU6SXNzdWU2Njg2ODQ3OTY=",
"title": "Link to OWASP project page gives 404",
"body": "It's shown in github's main page: https://www.owasp.org/index.php/OWASP_Vulnerable_Web_Application\r\nThanks",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/Vulnerable-Web-Application/issues/4",
@@ -18566,6 +19200,7 @@
"node_id": "MDU6SXNzdWU4NTUzNDEzMTE=",
"title": "mysqli_error()",
"body": "https://github.com/OWASP/Vulnerable-Web-Application/blob/c0f2689f4adc3dab4e310a4c709bbee9386c6b02/SQL/sql3.php#L43\r\nshould be mysqli_error()",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/Vulnerable-Web-Application/issues/8",
@@ -18592,6 +19227,7 @@
"node_id": "MDU6SXNzdWU2MDYxODk1MTY=",
"title": "Maintain and develop new test cases for Cloud Testing guide",
"body": "Hi everyone,\r\n\r\nI have seen this repo not update anymore from Nov 29, 2019. I have some question below:\r\n\r\n- How can I contribute for this repo?\r\n- Where I will find a template for test cases?\r\n- I think *Cloud Security Testing Guide* is better than this name now\r\n\r\nThank for your response.",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/owasp-cstg/issues/3",
@@ -18614,10 +19250,11 @@
"pk": 653,
"fields": {
"nest_created_at": "2024-09-11T21:09:26.043Z",
- "nest_updated_at": "2024-09-11T21:09:26.043Z",
+ "nest_updated_at": "2024-09-13T16:10:58.503Z",
"node_id": "I_kwDOClJnec5DVrCk",
"title": "Project Development",
"body": "**What would you like added?**\r\nThis is 2 years from the last Project update. Seem like this project is not developed anymore. So please assign me to join as a leader and contribute for this one. \r\n\r\nWould you like to be assigned to this issue?\r\n- [x] Assign me, please!\r\n",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/owasp-cstg/issues/6",
@@ -18642,10 +19279,11 @@
"pk": 654,
"fields": {
"nest_created_at": "2024-09-11T21:09:35.034Z",
- "nest_updated_at": "2024-09-11T21:09:35.034Z",
+ "nest_updated_at": "2024-09-13T16:11:05.605Z",
"node_id": "MDU6SXNzdWU2MTkzMTkwMDM=",
"title": "Update Contribution Guidelines",
"body": "Enhance the 'CONTRIBUTING.md' doc with more details",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/Intelligent-Intrusion-Detection-System/issues/7",
@@ -18677,6 +19315,7 @@
"node_id": "MDU6SXNzdWU2MTkzMjA2NjQ=",
"title": "Add coding guidelines doc",
"body": "Add a document describing the coding guidelines and standards that the developers of this project should follow",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/Intelligent-Intrusion-Detection-System/issues/8",
@@ -18708,6 +19347,7 @@
"node_id": "MDU6SXNzdWU2NTgzNzE3OTE=",
"title": "Add a Feature Extractor for input Pcap files",
"body": "A Feature Extractor, which would extract all the necessary features from the Input Pcap file and save it in a csv format.\r\nThe list of features could be found [here ](https://kdd.ics.uci.edu/databases/kddcup99/task.html)\r\n\r\nFor reference, Check this [Repo](https://github.com/nrajasin/Network-intrusion-dataset-creator)",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/Intelligent-Intrusion-Detection-System/issues/14",
@@ -18734,6 +19374,7 @@
"node_id": "MDU6SXNzdWU4MjA5NDc3MDY=",
"title": "Wiki page have some bugs",
"body": "**Describe the bug**\r\nThe view of the wiki page is broken. The HTML tags are visible rather than the sentence also the image is also giving a 404 error. Also, the resources which are provided are repeating also all the links are broken and pointing to just the home of the Github repo.\r\n\r\n**To Reproduce**\r\nSteps to reproduce the behavior:\r\n1. We just have to simply modify the wiki page on GitHub.\r\n\r\n**Expected behavior**\r\nThe page should not contain HTML tags and the image should be visible.\r\n\r\n**Screenshots**\r\n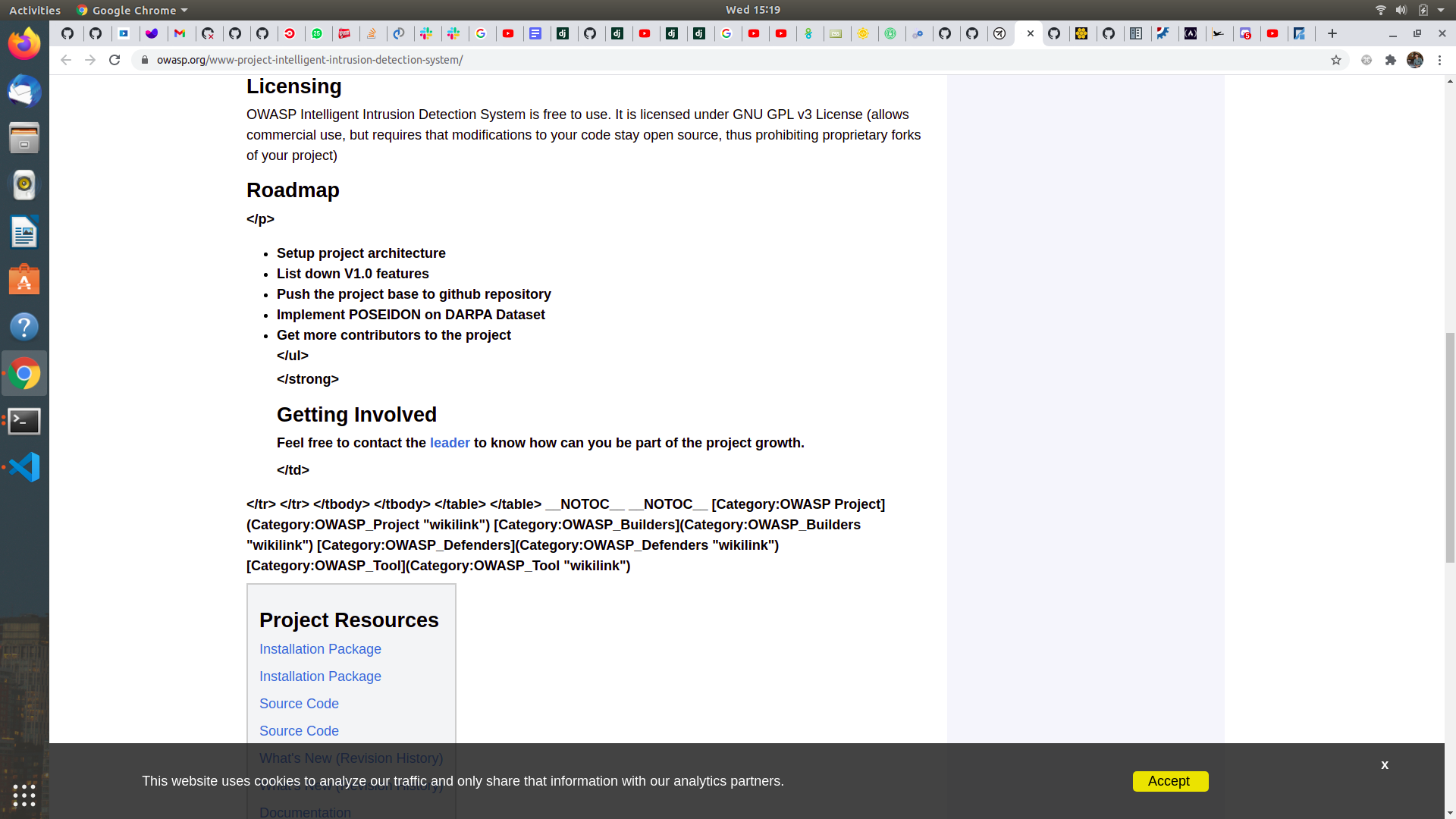\r\n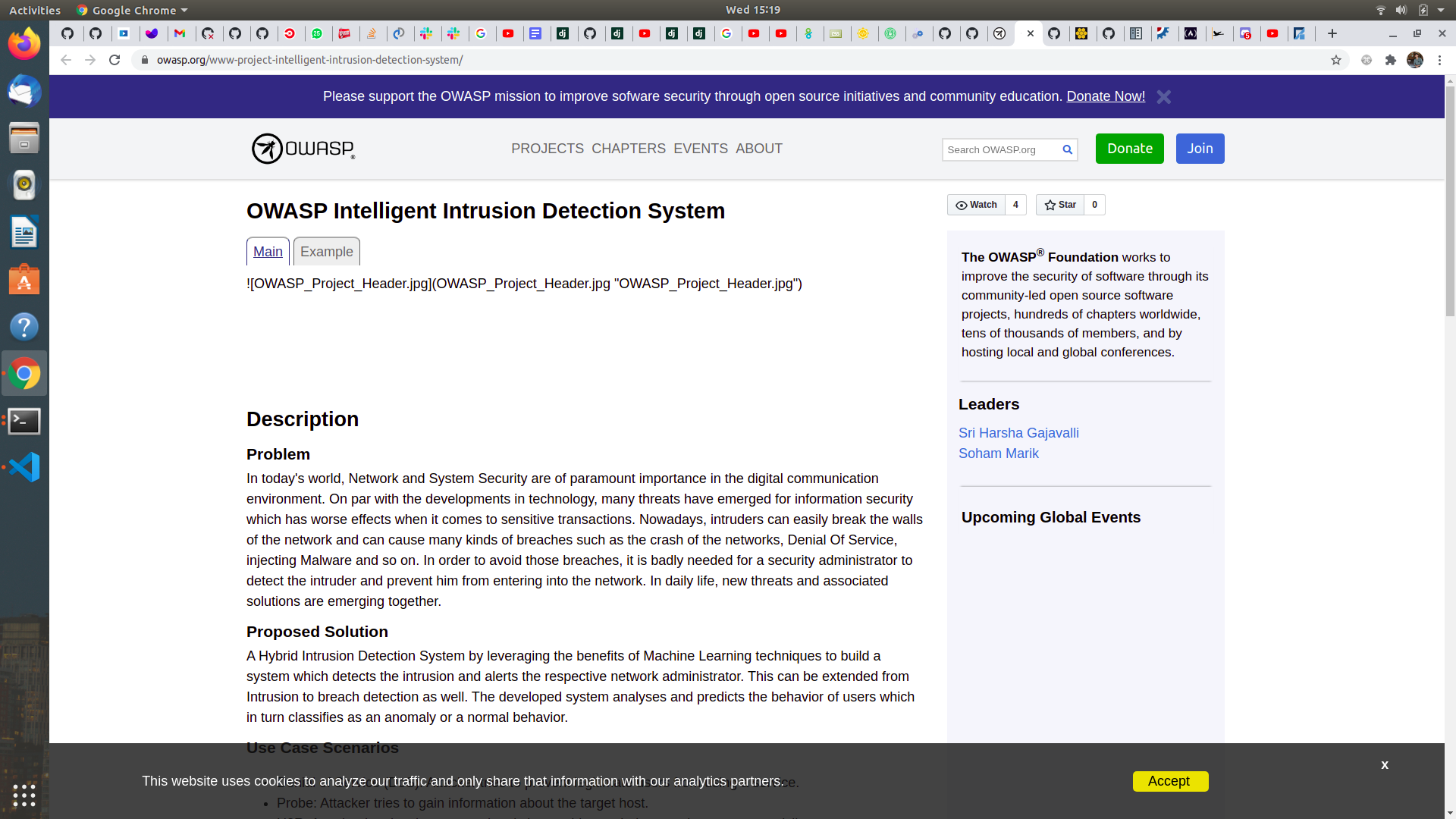\r\n\r\n\r\n**Desktop (please complete the following information):**\r\n - OS: Ubuntu 18.04\r\n - Browser: Chrome\r\n\r\n**Additional context**\r\n@hardlyhuman I would like to fix it, also will you please give some details on the image that has to be there.\r\nThanks\r\n",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/Intelligent-Intrusion-Detection-System/issues/24",
@@ -18760,6 +19401,7 @@
"node_id": "MDU6SXNzdWU4MzA4NzQyNTE=",
"title": "requirements.txt Not Present",
"body": "Django CI/build is failing as there is no **requirements.txt** file. ",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/Intelligent-Intrusion-Detection-System/issues/26",
@@ -18782,10 +19424,11 @@
"pk": 659,
"fields": {
"nest_created_at": "2024-09-11T21:09:45.435Z",
- "nest_updated_at": "2024-09-11T21:09:45.436Z",
+ "nest_updated_at": "2024-09-13T16:11:10.336Z",
"node_id": "MDU6SXNzdWU0MTM5MzE3NjQ=",
"title": "Add Secure-Header-Checker tool written in python",
"body": "Hi, Can I make PR that contain Secure-Header-Checker tool written in python for this project?",
+ "summary": "",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/Secure-Headers/issues/1",
@@ -18812,6 +19455,7 @@
"node_id": "MDU6SXNzdWU0NjQ3Mzk0NTI=",
"title": "Information about tools and payloads",
"body": "Hi, team! Thanks for the great project. I think it would be useful to add more information about different tools that can be used to test an application and detect security issues. For example, Arachni, ZAP, Burp Suite, .etc. One more thing that would be useful for application developers, QA engineers, security experts - links or examples of possible payloads that can be used to test an application API. There is a cool repository, called PayloadAllTheThings that contains a lot of payload examples to use during a security testing process. I can provide more information about tools and useful sources if it needed.\r\n\r\nBest regards,\r\nIhor",
+ "summary": "The issue suggests enhancing the project by including more information on various tools for application testing and security issue detection, such as Arachni, ZAP, and Burp Suite. Additionally, it proposes adding links or examples of potential payloads for API testing, referencing the \"PayloadAllTheThings\" repository as a valuable resource. The author is open to providing further information about tools and sources if required. \n\nTo address this issue, consider compiling a list of recommended tools and payloads along with relevant documentation or examples.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/API-Security/issues/17",
@@ -18842,6 +19486,7 @@
"node_id": "MDU6SXNzdWU0OTY2ODQ0OTc=",
"title": "Translation es ",
"body": "Hi, I found this resources super helpful and I would like non-english speakers to be able to benefit from this as well. \r\n\r\nDo you have translation projects or do you accept translation PRs? \r\nI see the sources are in `2019/en`, so I guess I could create a `2019/es` for a Spanish translation to work in. \r\n\r\nWould that be something you'd want/like? I'm willing to make the Spanish translation, but let me know if there is an established way to contribute on this matter (maybe there is a separated repo for translations or you already have a Spanish version that I couldn't find. ",
+ "summary": "A user has expressed interest in translating resources into Spanish to make them accessible for non-English speakers. They are inquiring whether there are existing translation projects or if translation pull requests (PRs) are accepted. The user proposes creating a `2019/es` folder for the Spanish translation and is willing to contribute. Clarification is sought on how to proceed with this contribution, including any established protocols or existing resources. \n\nTo address this, it would be beneficial to provide information on the translation process, any existing repositories for translations, or confirmation if a Spanish version is already available.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/API-Security/issues/22",
@@ -18874,6 +19519,7 @@
"node_id": "MDU6SXNzdWU2Nzg3MDkzODk=",
"title": "Translation German",
"body": "Hey!\r\n\r\nI really appreciate your efforts and think this projects should be translated into as many languages as possible.\r\nI'd like to provide a translation into german.\r\n\r\nCheers,\r\nch4rl353y",
+ "summary": "A user has expressed appreciation for the project's efforts and is interested in contributing by providing a German translation. It may be beneficial to outline the process for submitting translations and any specific guidelines that should be followed.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/API-Security/issues/36",
@@ -18905,6 +19551,7 @@
"node_id": "MDU6SXNzdWU5MzQ4NjU0NDE=",
"title": "Translation Dutch",
"body": "Hi everybody,\r\n\r\nWe want to translate API-Security to the Dutch language, any contributors are welcome!",
+ "summary": "The issue discusses the need for translating the API-Security documentation into Dutch and invites contributors to assist with this task. If interested, potential contributors should offer their help in the translation process.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/API-Security/issues/44",
@@ -18936,6 +19583,7 @@
"node_id": "I_kwDOCdd2e85AjLIG",
"title": "Translation Chinese",
"body": "Hi Team, \r\n\r\nGlad to see this project, I am an engineer in this area, I could contribute to the Chinese version.\r\n\r\n\r\n\r\n",
+ "summary": "A user is expressing interest in contributing to the project by providing a Chinese translation. It would be beneficial to coordinate with this individual to facilitate the translation process and integrate their contributions effectively.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/API-Security/issues/46",
@@ -18967,6 +19615,7 @@
"node_id": "I_kwDOCdd2e85PJnlO",
"title": "Turkish Translation",
"body": "Hey!\r\n\r\nFirst of all thanks for this outstanding repository about API security.\r\nI would be happy to contribute to the translation of this repository into **Turkish**.\r\n\r\n\r\n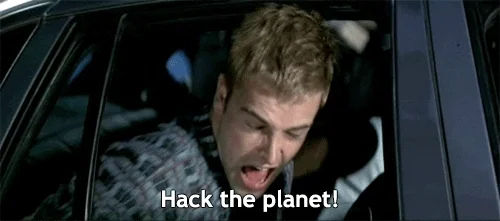",
+ "summary": "A user has expressed interest in contributing to the translation of the repository into Turkish. To move forward, it would be beneficial to establish a process for managing translations and possibly provide guidelines for contributors.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/API-Security/issues/58",
@@ -18998,6 +19647,7 @@
"node_id": "I_kwDOCdd2e85aRUqH",
"title": "Hindi Translation",
"body": "Hey there,\r\nMore than happy to do translation in hindi. I'm developer from India",
+ "summary": "A user has expressed their willingness to contribute by providing Hindi translations for the project. It may be beneficial to coordinate with them to outline specific areas or content that require translation.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/API-Security/issues/59",
@@ -19029,6 +19679,7 @@
"node_id": "I_kwDOCdd2e85glIOu",
"title": "Odata with EF and .Net core Security risks with Front End queries through web components",
"body": "A demo would be good to show Odata with EF and .Net core Security risks while we query using expression trees that hold any query.'\r\nWould the security be compromised at some point in operation?",
+ "summary": "The issue discusses potential security risks associated with using OData with Entity Framework in a .NET Core application, particularly when handling front-end queries through web components. The author suggests that a demonstration would be beneficial to illustrate these risks, especially in the context of expression trees that can represent any query. The main concern is whether the security of the application could be compromised during operations involving these queries. It may be necessary to explore and implement security measures to mitigate these risks.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/API-Security/issues/99",
@@ -19055,6 +19706,7 @@
"node_id": "I_kwDOCdd2e85x6X4h",
"title": "Contradictory risk classification for \"Unsafe Consumption of APIs\"",
"body": "The *Exploitability* of [API10:2023][1] is graded with the highest rating of `easy`.\r\nAt the same time, the corresponding textual explanation actually tells the opposite, that exploitation of this should be rather hard:\r\n\r\n```\r\nExploiting this issue requires attackers to identify and potentially compromise other APIs/services the target API integrated with. Usually, this information is not publicly available or the integrated API/service is not easily exploitable.\r\n```\r\n\r\n[1]: https://owasp.org/API-Security/editions/2023/en/0xaa-unsafe-consumption-of-apis/",
+ "summary": "There is a contradiction in the risk classification for \"Unsafe Consumption of APIs\" where the exploitability is rated as `easy`, while the explanation suggests that exploitation is actually difficult due to the need for attackers to identify and compromise other integrated APIs/services, which are typically not publicly accessible or easily exploitable. \n\nTo resolve this issue, a review of the exploitability rating and the accompanying explanation is needed to ensure they align accurately.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/API-Security/issues/123",
@@ -19070,8 +19722,8 @@
"repository": 1067,
"assignees": [],
"labels": [
- 159,
- 160
+ 160,
+ 159
]
}
},
@@ -19084,6 +19736,7 @@
"node_id": "I_kwDOCdd2e85yAnUc",
"title": "Persian Translation for 2023",
"body": "Hi Guys\r\nI could contribute to translate the API TOP10 2023RC to Farsi/Persian & would want to know your opinion on this matter. We did the Persian translation for 2019 version as well.\r\n@PauloASilva \r\n",
+ "summary": "A request has been made to contribute a Persian translation for the API TOP10 2023RC, building on a previous translation effort for the 2019 version. Feedback and opinions on this contribution are being sought. It would be beneficial to assess the feasibility and scope of this translation project.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/API-Security/issues/124",
@@ -19101,8 +19754,8 @@
298
],
"labels": [
- 156,
- 160
+ 160,
+ 156
]
}
},
@@ -19115,6 +19768,7 @@
"node_id": "I_kwDOCdd2e856DNXN",
"title": "Translation to Portuguese (pt-PT) for 2023 version",
"body": "Hello,\r\nI want to help with the translation to Portuguese (pt-PT) for 2023 version.\r\nI've followed the instructions detailed here: [https://github.com/OWASP/API-Security/issues/124#issuecomment-1737308376](https://github.com/OWASP/API-Security/issues/124#issuecomment-1737308376)\r\nAnd I'm working on the translation here: [https://github.com/RiuSalvi/API-Security/tree/translation/pt-pt](https://github.com/RiuSalvi/API-Security/tree/translation/pt-pt)\r\n\r\nBest regards,\r\nRui Silva",
+ "summary": "The issue discusses the intention to assist with the translation of the 2023 version into Portuguese (pt-PT). The contributor has followed the provided instructions and is currently working on the translation in a designated repository. To progress, further collaboration or review of the translation work may be needed.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/API-Security/issues/127",
@@ -19132,8 +19786,8 @@
299
],
"labels": [
- 156,
- 160
+ 160,
+ 156
]
}
},
@@ -19146,6 +19800,7 @@
"node_id": "I_kwDOCdd2e86Eehb0",
"title": "Translation to brasilian portuguese (pt-BR)",
"body": "Hi Guys\r\nI could contribute to translate the API TOP10 2023 to Brasilian Portuguese (pt-BR). \r\n\r\nRegards,\r\nLuca Regne",
+ "summary": "A user has expressed willingness to contribute by translating the API TOP10 2023 to Brazilian Portuguese (pt-BR). It may be useful to review the translation process and guidelines to facilitate this contribution.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/API-Security/issues/131",
@@ -19161,8 +19816,8 @@
"repository": 1067,
"assignees": [],
"labels": [
- 156,
- 160
+ 160,
+ 156
]
}
},
@@ -19171,10 +19826,11 @@
"pk": 672,
"fields": {
"nest_created_at": "2024-09-11T21:10:16.989Z",
- "nest_updated_at": "2024-09-12T02:32:00.271Z",
+ "nest_updated_at": "2024-09-13T16:26:39.350Z",
"node_id": "I_kwDOCdd2e86UP58v",
"title": "Reference to OWASP Risk Rating Methodology",
"body": "https://github.com/OWASP/API-Security/blob/ef8e6b306de85950cd6df8a30671566e5bd134e3/editions/2023/en/0xd0-about-data.md?plain=1#L68C1-L68C67\r\n\r\nOWASP Risk Rating Methodology (https://owasp.org/www-community/OWASP_Risk_Rating_Methodology) references to OWASP Risk Assessment Framework (https://owasp.org/www-project-risk-assessment-framework/).\r\n\r\nProbably due to the changes on OWASP project pages.",
+ "summary": "The issue highlights the need for updated references to the OWASP Risk Rating Methodology and the OWASP Risk Assessment Framework within the provided documentation link. It suggests that changes to OWASP project pages may have affected the existing links. To resolve this, the documentation should be reviewed and corrected to ensure accurate and current references are provided.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/API-Security/issues/133",
@@ -19201,6 +19857,7 @@
"node_id": "MDU6SXNzdWU4MTI2NTEzNDc=",
"title": "New CS proposal: React Security CheatSheet",
"body": "## What is the proposed Cheat Sheet about?\r\nBuilding secure React applications by avoiding common vulnerabilities.\r\n\r\n## What security issues are commonly encountered related to this area?\r\nCWE-79: Improper Neutralization of Input During Web Page Generation ('Cross-site Scripting')\r\nCWE-602: Client-Side Enforcement of Server-Side Security\r\nCWE-603: Use of Client-Side Authentication\r\nCWE-22: Improper Limitation of a Pathname to a Restricted Directory ('Path Traversal')\r\nCWE-89: Improper Neutralization of Special Elements used in an SQL Command\r\nCWE-94: Improper Control of Generation of Code ('Code Injection')\r\n\r\n## What is the objective of the Cheat Sheet?\r\nExamples of vulnerable code and how to fix it. \r\n\r\n## What other resources exist in this area?\r\nI've written about this topic, and made videos related to it in the past. I want to make some new content that goes deeper and broader here.\r\n\r\nhttps://www.youtube.com/watch?v=VtNotePFuJY\r\nhttps://snyk.io/blog/10-react-security-best-practices/\r\nhttps://medium.com/javascript-security/avoiding-xss-in-react-is-still-hard-d2b5c7ad9412\r\nhttps://medium.com/javascript-security/avoiding-xss-via-markdown-in-react-91665479900\r\nhttps://www.synopsys.com/software-integrity/training/software-security-courses/react-js-security.html",
+ "summary": "A new proposal has been made for a React Security Cheat Sheet aimed at helping developers build secure React applications by addressing common vulnerabilities. Key issues identified include cross-site scripting (CWE-79), client-side security enforcement (CWE-602), client-side authentication (CWE-603), path traversal (CWE-22), SQL command vulnerabilities (CWE-89), and code injection (CWE-94). The objective is to provide examples of vulnerable code alongside solutions for fixing these issues. The proposal also references existing resources and content that delve into these security topics. To move forward, it would be beneficial to compile and organize these examples and solutions into a comprehensive guide.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/CheatSheetSeries/issues/543",
@@ -19232,6 +19889,7 @@
"node_id": "MDU6SXNzdWU4NjY5Mjk3Nzk=",
"title": "Update: Vulnerable Dependency Management Cheat Sheet with Dependency Confusion",
"body": "## What is missing or needs to be updated?\r\n\r\nI have found this [post](https://medium.com/@alex.birsan/dependency-confusion-4a5d60fec610) about *Dependency Confusion* attack and I think that it can be interesting to add a section about protection against this attack in the [Vulnerable Dependency Management Cheat Sheet](https://github.com/OWASP/CheatSheetSeries/blob/master/cheatsheets/Vulnerable_Dependency_Management_Cheat_Sheet.md).\r\n\r\n## How should this be resolved?\r\n\r\nI propose to add a small section showing some protection that can applied.\r\n\r\nThanks a lot in advance and also thanks a lot for your amazing work on this project ❤️ \r\n",
+ "summary": "The issue suggests adding a section on \"Dependency Confusion\" attacks to the Vulnerable Dependency Management Cheat Sheet. It references a blog post that provides insights on this topic and proposes including protective measures against such attacks. An update should be made to the cheat sheet to incorporate this information.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/CheatSheetSeries/issues/641",
@@ -19263,6 +19921,7 @@
"node_id": "MDU6SXNzdWU5MjY0MTcwMTM=",
"title": "Update: [Multifactor Authentication Cheat Sheet]: Further info about TOTP secret-key storage",
"body": "## What is missing or needs to be updated?\r\nThere is no info about the proper way to store users' secrets for TOTP MFA. Should these secrets be stored in plaintext or be 2-way encrypted? If encrypted should it use a dedicated key on the server or use something like the user's password or email address or username?\r\n\r\n## How should this be resolved?\r\nA brief subsection or bullet point, or perhaps a link to a separate cheat-sheet, describing the industry-standard (if such exists) for how to store the users' secret-keys for TOTP authentication. Or at least a line saying whether these secrets need to be encrypted or not.\r\n\r\nI don't know the answer to the above question, so I won't propose any text.",
+ "summary": "The issue highlights the absence of guidance on the proper storage methods for users' secrets related to TOTP MFA. Specifically, it questions whether these secrets should be stored in plaintext or encrypted, and if encrypted, what key should be used for encryption. \n\nTo resolve this, it is suggested to add a subsection or bullet point to the cheat sheet that outlines the industry standards for storing TOTP secret keys, including recommendations on encryption practices. If no definitive answer exists, at minimum, a statement regarding the necessity of encryption should be included.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/CheatSheetSeries/issues/678",
@@ -19293,6 +19952,7 @@
"node_id": "I_kwDOCbL1IM5C5qWm",
"title": "Update: Secrets_Management_CheatSheet.",
"body": "## What is missing or needs to be updated?\r\n\r\nThe following tasks require to be executed post-mvp of the cheatsheet as agreed with various team-members:\r\n\r\n- [ ] Further extend and re-evaluate the concepts of https://github.com/OWASP/CheatSheetSeries/pull/842\r\n- [ ] show more how you can create an architecture that allows for rotation in examples (so not just tell, but show examples)\r\n- [ ] show how passwordless (openID connect) can help, but tokens need security as well (might be a cheatsheet for to refer to?)\r\n- [ ] The ease of onboarding needs to be further expanded as explained by @dominikdesmit https://owasp.slack.com/archives/C02LSHXKVU5/p1643453874955679?thread_ts=1643442691.915449&cid=C02LSHXKVU5\r\n\r\n\r\n\r\nPlease note that I only file this issue for tracking as requested by multiple team-members for now, but will not have the time to pick this up timely myself.",
+ "summary": "The issue outlines several tasks that need to be addressed for the Secrets Management CheatSheet after the MVP phase. The tasks include:\n\n- Extending and re-evaluating concepts from a specific pull request.\n- Providing examples of architecture that facilitates rotation.\n- Demonstrating how passwordless authentication (like OpenID Connect) can enhance security, while also addressing token security.\n- Expanding on the ease of onboarding, as discussed by a team member.\n\nThis issue serves as a tracking mechanism for these updates, indicating that further contributions are needed from the team to progress on these items.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/CheatSheetSeries/issues/845",
@@ -19323,6 +19983,7 @@
"node_id": "I_kwDOCbL1IM5FfdWK",
"title": "New Security with CORS CS ",
"body": "## What is missing or needs to be updated?\r\nThe [HTML5 security cheat sheet](https://cheatsheetseries.owasp.org/cheatsheets/HTML5_Security_Cheat_Sheet.html) currently says\r\n\r\n> Keep in mind that CORS does not prevent the requested data from going to an unauthorized location. It's still important for the server to perform usual [CSRF](https://cheatsheetseries.owasp.org/cheatsheets/Cross-Site_Request_Forgery_Prevention_Cheat_Sheet.html) prevention.\r\n\r\nIt is absolutely true that CSRF protection is required for CORS, but it's a little imprecise. Specifically, the [Cross-Site Request Forgery Prevention Cheat Sheet](https://cheatsheetseries.owasp.org/cheatsheets/Cross-Site_Request_Forgery_Prevention_Cheat_Sheet.html#cross-site-request-forgery-prevention-cheat-sheet) lists the double-submit cookie as the first line of defense, but that pattern is not possible in a CORS environment. (Unless the Origin and request domains happen to be subdomains of a shared parent on which the cookie could be set.)\r\n\r\n## How should this be resolved?\r\n\r\nI suggest clarifying -- in either or both of the cheat sheets -- which CSRF protection mechanisms are best suited to CORS environments.\r\n\r\nMixMax's [Using CORS policies to implement CSRF protection](https://www.mixmax.com/engineering/modern-csrf) has some additional information on using Origin verification to protect against CSRF attacks on CORS requests.",
+ "summary": "The issue highlights a gap in the current HTML5 security cheat sheet regarding the relationship between CORS and CSRF protection. It points out that while CSRF protection is necessary in CORS scenarios, the existing guidance may not adequately address the limitations of the double-submit cookie technique in such environments. The author suggests that the cheat sheets should clarify which CSRF protection mechanisms are most effective for CORS and references additional resources for further information on this topic. \n\nTo resolve this, it would be beneficial to update the cheat sheets to include guidance on appropriate CSRF protection strategies specifically tailored for CORS contexts.",
"state": "open",
"state_reason": "",
"url": "https://github.com/OWASP/CheatSheetSeries/issues/875",
@@ -19353,6 +20014,7 @@
"node_id": "I_kwDOCbL1IM5Ww4_8",
"title": "Update: Cross-Site Request Forgery Prevention Cheat Sheet",
"body": "## What is missing or needs to be updated?\r\n\r\nCross-Site Request Forgery attacks occur because untrusted browser code can cause requests to be sent to a vulnerable server that are \"special\" in a way that the attacker could not send from their own machines. Typically this \"special\"-ness is that they contain cookies from a targeted user's browser. Less commonly, this could mean they contain basic auth credentials or that the server makes authorization assumptions based on the client's network (eg, explicitly looking at the client's IP address to make decisions, or by putting the server on a private network and assuming that any client talking to this server is authorized).\r\n\r\nThe mitigations discussed in the cheat sheet all assume that these \"special\" request qualities must exist in your system. However, an entirely different approach to solving CSRF is to avoid giving special treatment based on cookies, basic auth, and network properties. This approach may be appropriate for web servers that are primarily serving \"API-style\" traffic and which websites only communicate with via AJAX-style calls.\r\n\r\nFor example, a server that makes authentication decisions only based on a custom HTTP header (perhaps set from a value stored in browser `localStorage`) or a value in the body of a POST and not on cookies or network should be considered successfully defended against CSRF. Developers who have built servers with this property should not feel like they need to also set up token-based mitigation or (if #1010 is accepted) header-based mitigation.\r\n\r\n\r\n## How should this be resolved?\r\n\r\nA new top-level section should be added describing \"don't care about cookies/etc\" as an appropriate mitigation technique, with the drawback that it is not helpful if you need your endpoint to be accessible via HTML `